弾切れの発生④(ショットパワーを全回復させる)
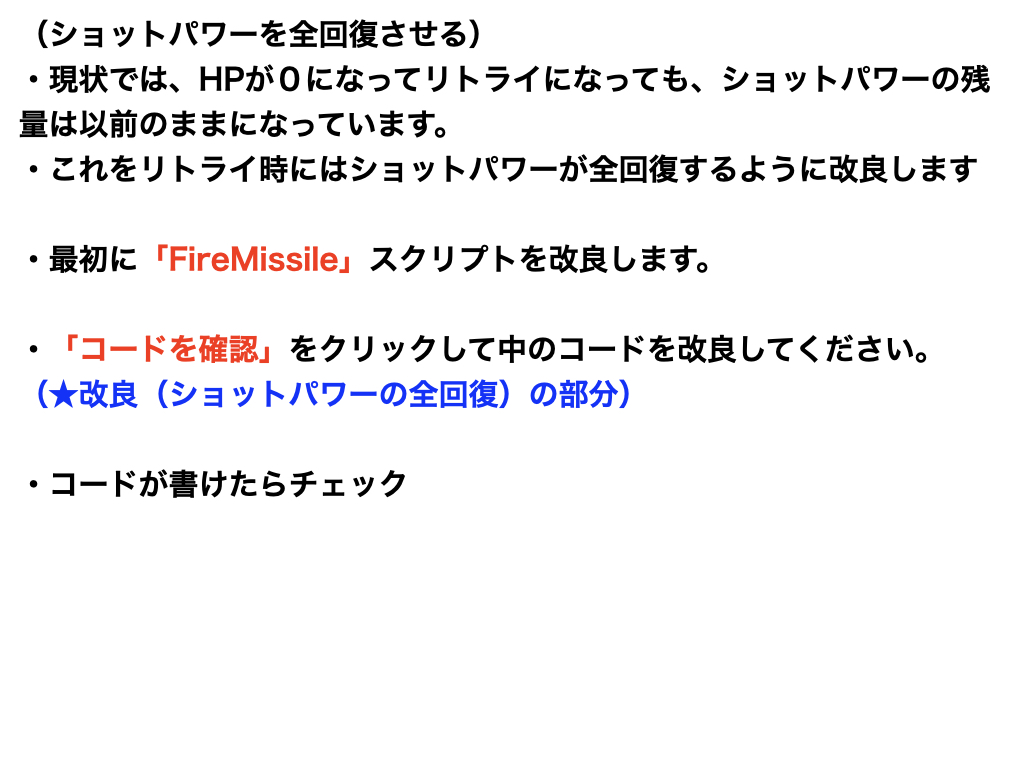
ショットパワーの全回復
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class FireMissile : MonoBehaviour
{
public GameObject missilePrefab;
public float missileSpeed;
public AudioClip fireSound;
private int timeCount;
// ★改良(ショットパワーの全回復)
// アクセス修飾子を「public」に変更する。
public int maxPower = 100;
public int shotPower;
public Slider powerSlider;
private void Start()
{
shotPower = maxPower;
powerSlider.maxValue = maxPower;
powerSlider.value = shotPower;
}
void Update()
{
timeCount += 1;
if (Input.GetButton("Jump"))
{
if(shotPower <= 0)
{
return;
}
shotPower -= 1;
if(shotPower < 0)
{
shotPower = 0;
}
powerSlider.value = shotPower;
if (timeCount % 5 == 0)
{
GameObject missile = Instantiate(missilePrefab, transform.position, Quaternion.identity);
Rigidbody missileRb = missile.GetComponent<Rigidbody>();
missileRb.AddForce(transform.forward * missileSpeed);
AudioSource.PlayClipAtPoint(fireSound, transform.position);
Destroy(missile, 2.0f);
}
}
else
{
shotPower += 1;
if(shotPower > maxPower)
{
shotPower = maxPower;
}
powerSlider.value = shotPower;
}
}
}
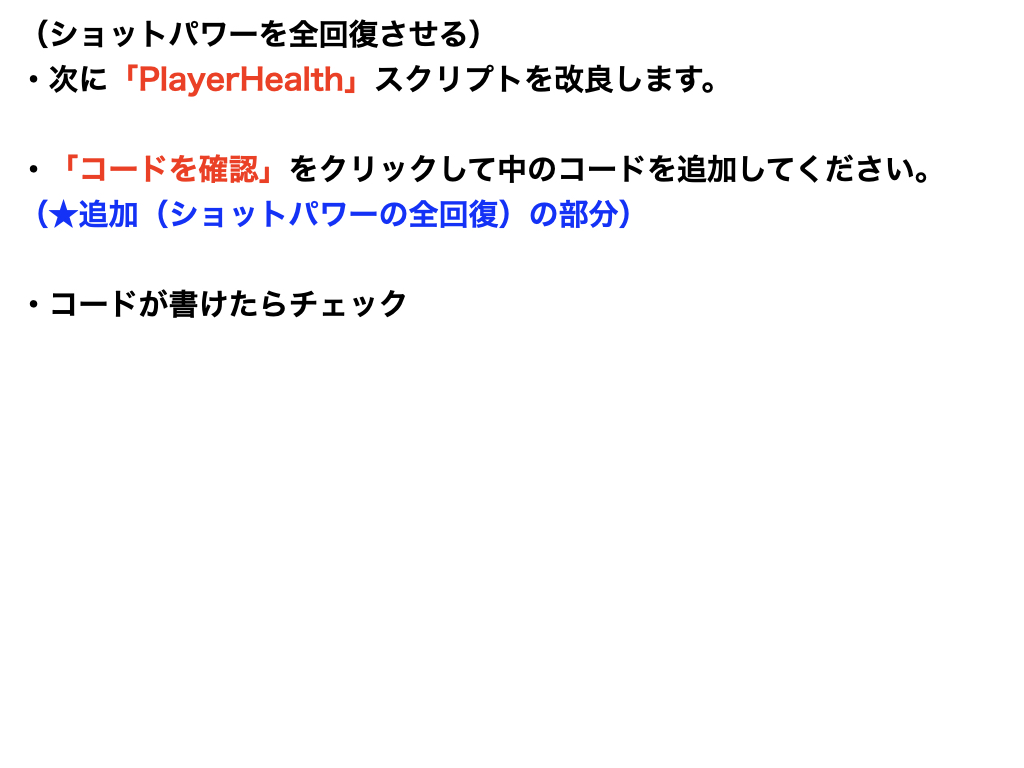
ショットパワーの全回復
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class PlayerHealth : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip damageSound;
public AudioClip destroySound;
private int playerHP;
private int maxHP = 5;
public Slider hpSlider;
public GameObject[] playerIcons;
public bool isMuteki = false;
public static int destroyCount = 0;
// ★追加(ショットパワーの全回復)
public FireMissile fireMissile;
private void Start()
{
UpdatePlayerIcons();
playerHP = maxHP;
hpSlider.maxValue = playerHP;
hpSlider.value = playerHP;
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("EnemyMissile") && isMuteki == false)
{
playerHP -= 1;
AudioSource.PlayClipAtPoint(damageSound, Camera.main.transform.position);
Destroy(other.gameObject);
hpSlider.value = playerHP;
if (playerHP == 0)
{
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 1.0f);
AudioSource.PlayClipAtPoint(destroySound, Camera.main.transform.position);
this.gameObject.SetActive(false);
destroyCount += 1;
UpdatePlayerIcons();
if(destroyCount < 5)
{
Invoke("Retry", 1.0f);
}
else
{
SceneManager.LoadScene("GameOver");
destroyCount = 0;
}
}
}
}
void UpdatePlayerIcons()
{
for (int i = 0; i < playerIcons.Length; i++)
{
if(destroyCount <= i)
{
playerIcons[i].SetActive(true);
}
else
{
playerIcons[i].SetActive(false);
}
}
}
void Retry()
{
this.gameObject.SetActive(true);
playerHP = maxHP;
hpSlider.value = playerHP;
isMuteki = true;
Invoke("MutekiOff", 2.0f);
// ★追加(ショットパワーの全回復)
fireMissile.shotPower = fireMissile.maxPower;
}
void MutekiOff()
{
isMuteki = false;
}
public void AddHP(int amount)
{
playerHP += amount;
if(playerHP > maxHP)
{
playerHP = maxHP;
}
hpSlider.value = playerHP;
}
public void Player1UP(int amount)
{
destroyCount -= amount;
if(destroyCount < 0)
{
destroyCount = 0;
}
UpdatePlayerIcons();
}
}
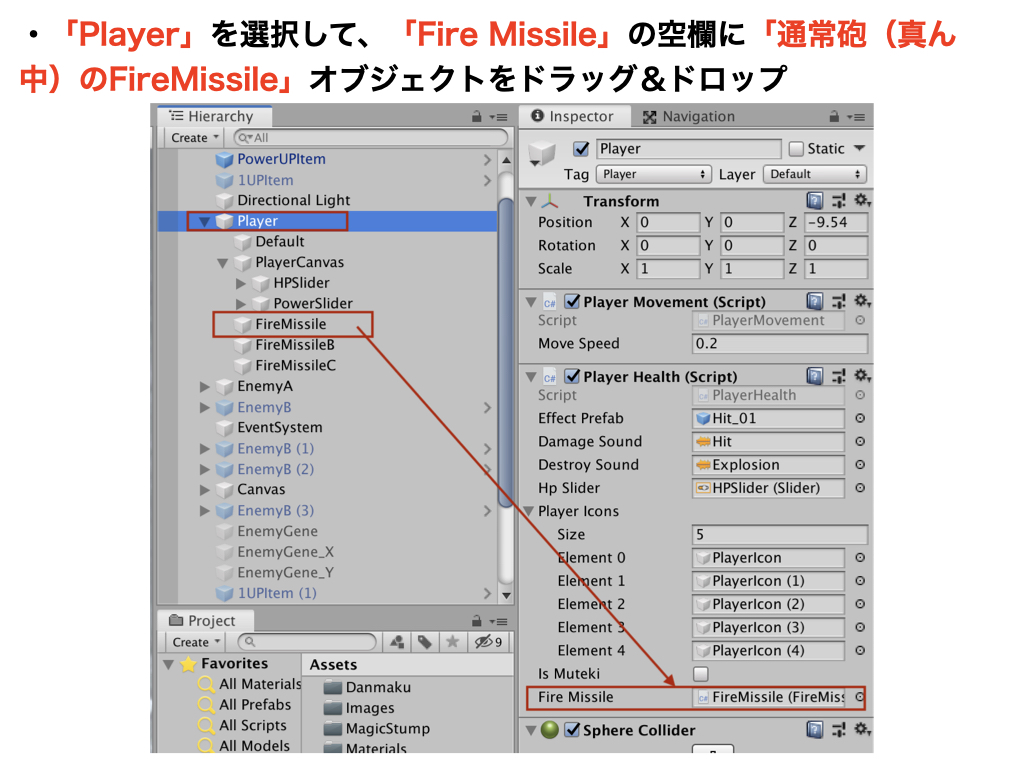
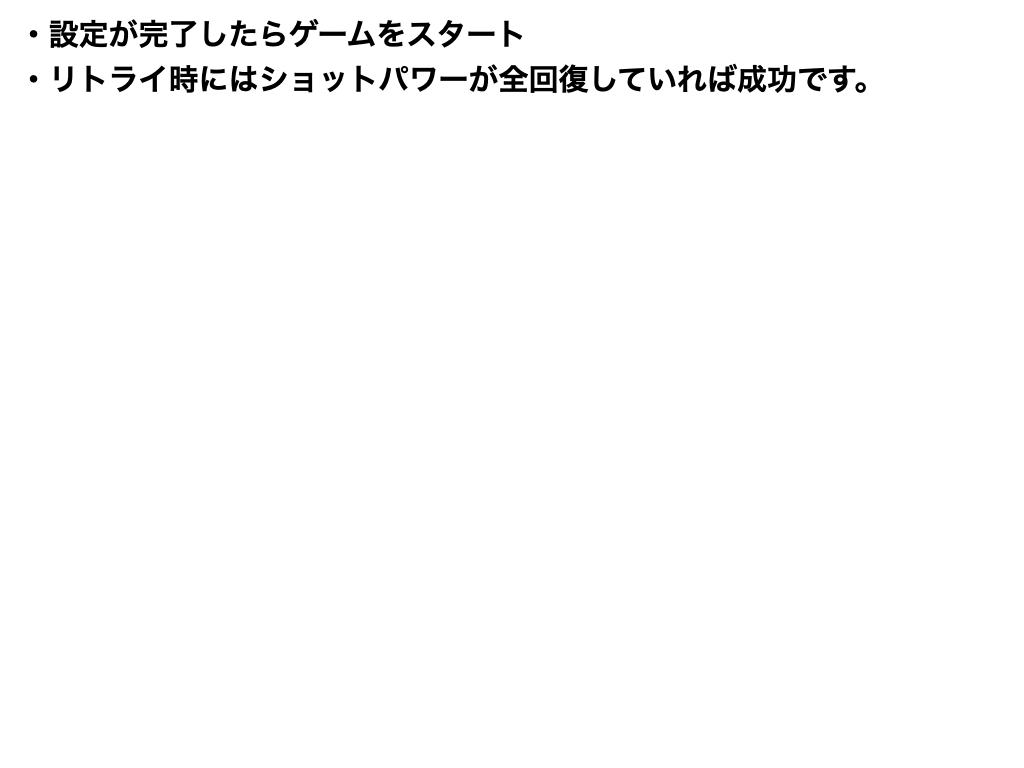
【2019版】Danmaku Ⅱ(基礎2/全38回)
他のコースを見る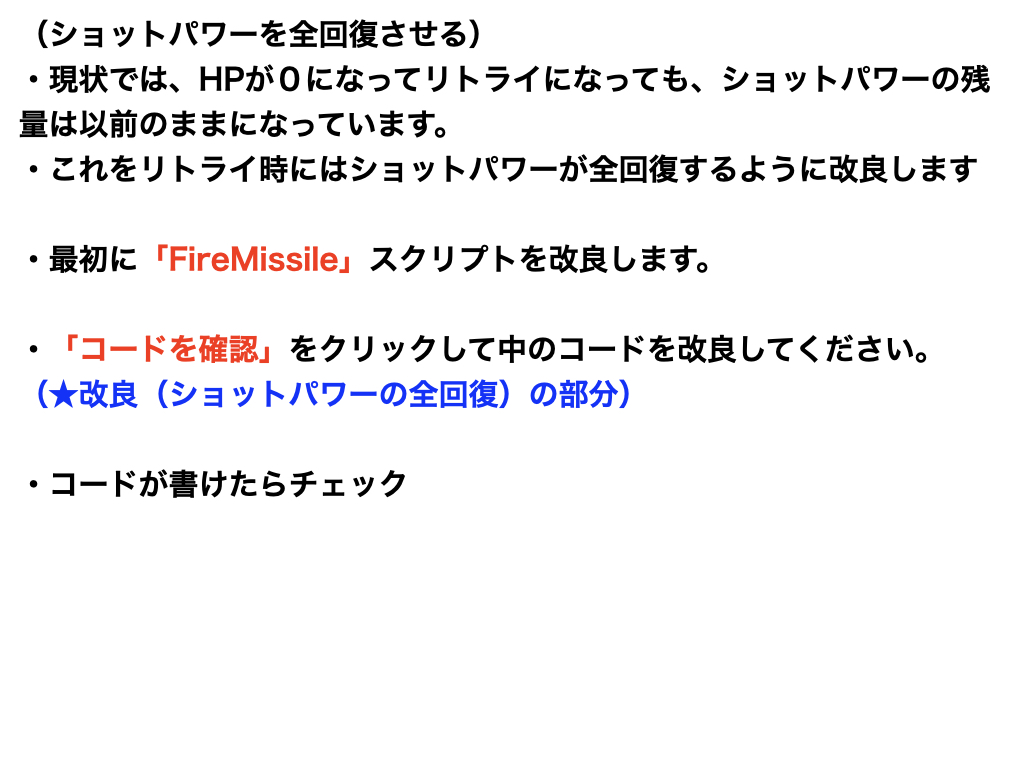
ショットパワーの全回復
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class FireMissile : MonoBehaviour
{
public GameObject missilePrefab;
public float missileSpeed;
public AudioClip fireSound;
private int timeCount;
// ★改良(ショットパワーの全回復)
// アクセス修飾子を「public」に変更する。
public int maxPower = 100;
public int shotPower;
public Slider powerSlider;
private void Start()
{
shotPower = maxPower;
powerSlider.maxValue = maxPower;
powerSlider.value = shotPower;
}
void Update()
{
timeCount += 1;
if (Input.GetButton("Jump"))
{
if(shotPower <= 0)
{
return;
}
shotPower -= 1;
if(shotPower < 0)
{
shotPower = 0;
}
powerSlider.value = shotPower;
if (timeCount % 5 == 0)
{
GameObject missile = Instantiate(missilePrefab, transform.position, Quaternion.identity);
Rigidbody missileRb = missile.GetComponent<Rigidbody>();
missileRb.AddForce(transform.forward * missileSpeed);
AudioSource.PlayClipAtPoint(fireSound, transform.position);
Destroy(missile, 2.0f);
}
}
else
{
shotPower += 1;
if(shotPower > maxPower)
{
shotPower = maxPower;
}
powerSlider.value = shotPower;
}
}
}
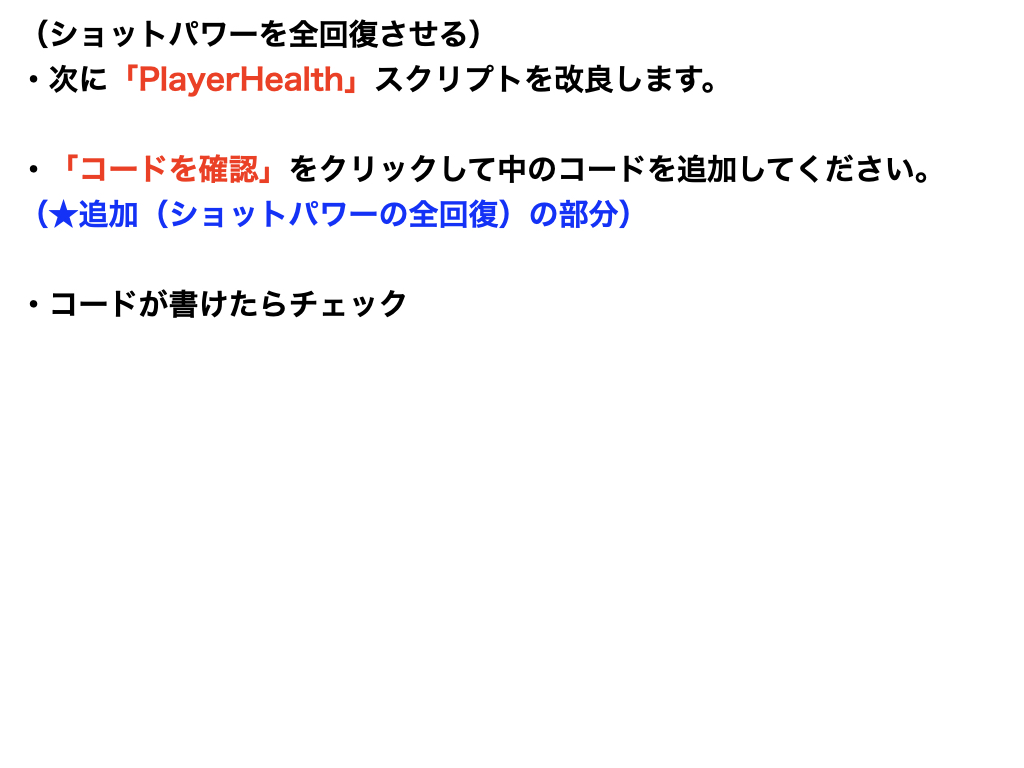
ショットパワーの全回復
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class PlayerHealth : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip damageSound;
public AudioClip destroySound;
private int playerHP;
private int maxHP = 5;
public Slider hpSlider;
public GameObject[] playerIcons;
public bool isMuteki = false;
public static int destroyCount = 0;
// ★追加(ショットパワーの全回復)
public FireMissile fireMissile;
private void Start()
{
UpdatePlayerIcons();
playerHP = maxHP;
hpSlider.maxValue = playerHP;
hpSlider.value = playerHP;
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("EnemyMissile") && isMuteki == false)
{
playerHP -= 1;
AudioSource.PlayClipAtPoint(damageSound, Camera.main.transform.position);
Destroy(other.gameObject);
hpSlider.value = playerHP;
if (playerHP == 0)
{
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 1.0f);
AudioSource.PlayClipAtPoint(destroySound, Camera.main.transform.position);
this.gameObject.SetActive(false);
destroyCount += 1;
UpdatePlayerIcons();
if(destroyCount < 5)
{
Invoke("Retry", 1.0f);
}
else
{
SceneManager.LoadScene("GameOver");
destroyCount = 0;
}
}
}
}
void UpdatePlayerIcons()
{
for (int i = 0; i < playerIcons.Length; i++)
{
if(destroyCount <= i)
{
playerIcons[i].SetActive(true);
}
else
{
playerIcons[i].SetActive(false);
}
}
}
void Retry()
{
this.gameObject.SetActive(true);
playerHP = maxHP;
hpSlider.value = playerHP;
isMuteki = true;
Invoke("MutekiOff", 2.0f);
// ★追加(ショットパワーの全回復)
fireMissile.shotPower = fireMissile.maxPower;
}
void MutekiOff()
{
isMuteki = false;
}
public void AddHP(int amount)
{
playerHP += amount;
if(playerHP > maxHP)
{
playerHP = maxHP;
}
hpSlider.value = playerHP;
}
public void Player1UP(int amount)
{
destroyCount -= amount;
if(destroyCount < 0)
{
destroyCount = 0;
}
UpdatePlayerIcons();
}
}
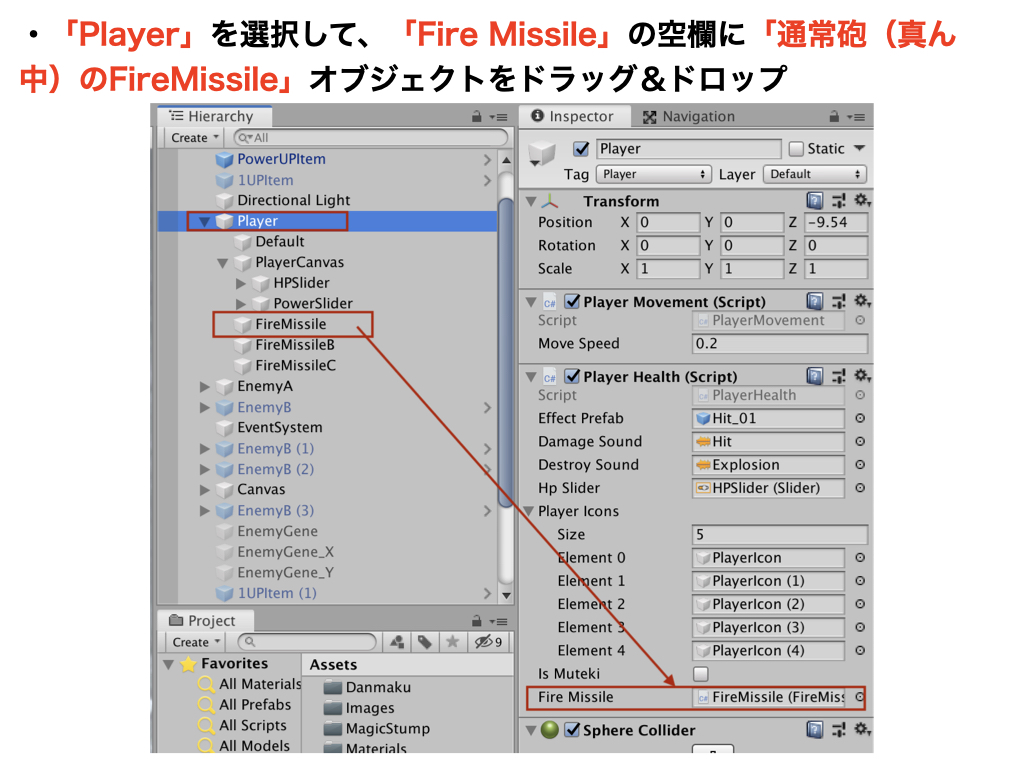
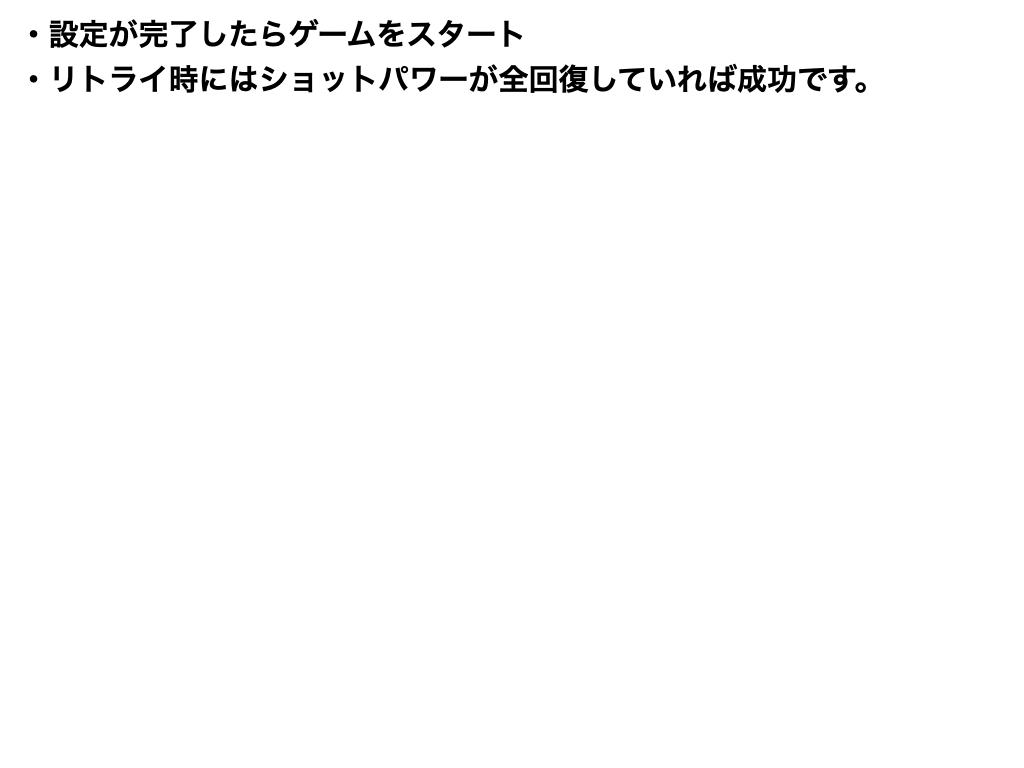
弾切れの発生④(ショットパワーを全回復させる)