アイテムの作成③(自機1UP)
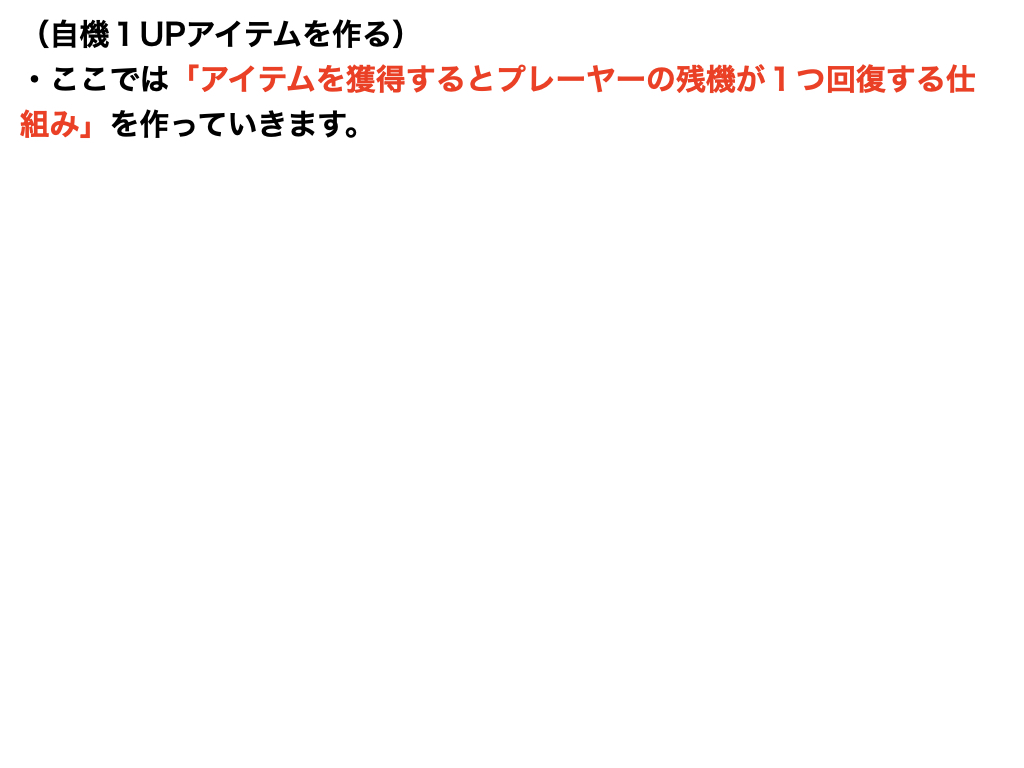
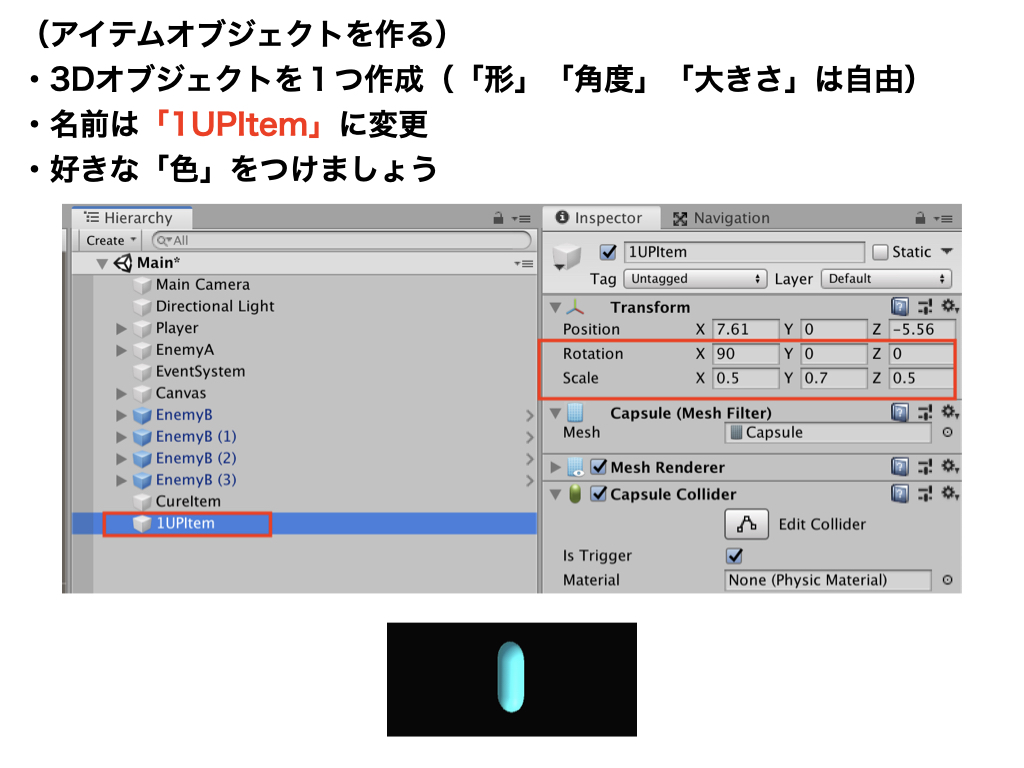
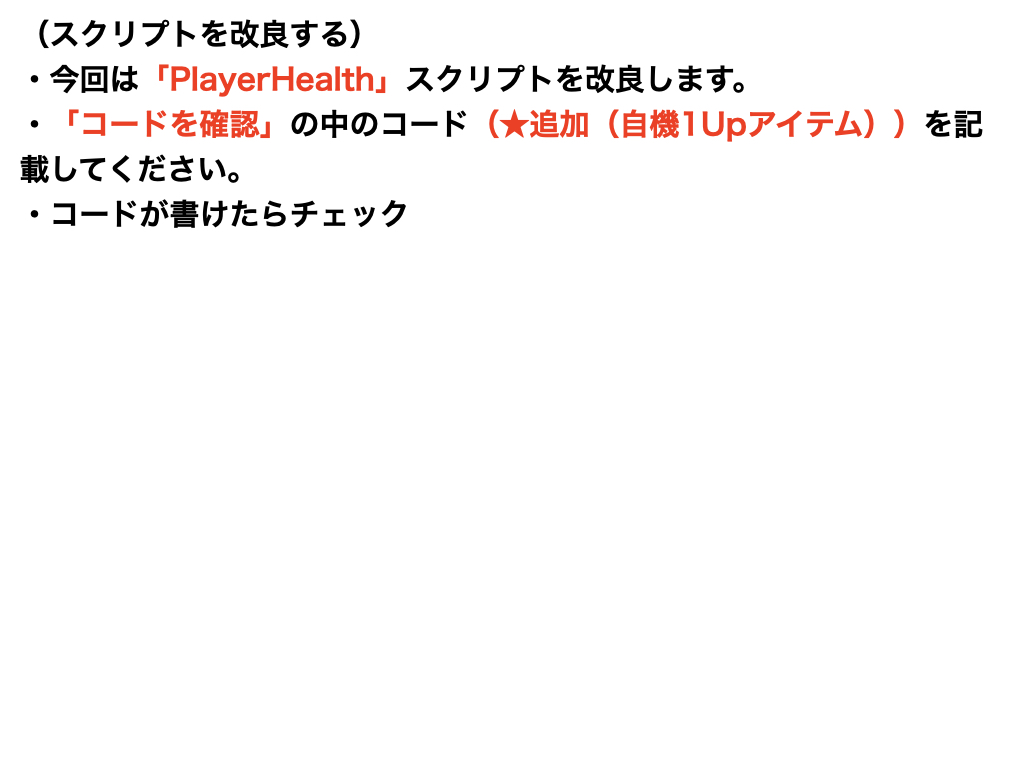
自機1UP
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class PlayerHealth : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip damageSound;
public AudioClip destroySound;
private int playerHP;
private int maxHP = 5;
public Slider hpSlider;
public GameObject[] playerIcons;
private int destroyCount = 0;
public bool isMuteki = false;
private void Start()
{
playerHP = maxHP;
hpSlider.maxValue = playerHP;
hpSlider.value = playerHP;
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("EnemyMissile") && isMuteki == false)
{
playerHP -= 1;
AudioSource.PlayClipAtPoint(damageSound, Camera.main.transform.position);
Destroy(other.gameObject);
hpSlider.value = playerHP;
if (playerHP == 0)
{
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 1.0f);
AudioSource.PlayClipAtPoint(destroySound, Camera.main.transform.position);
this.gameObject.SetActive(false);
destroyCount += 1;
UpdatePlayerIcons();
if(destroyCount < 5)
{
Invoke("Retry", 1.0f);
}
else
{
SceneManager.LoadScene("GameOver");
destroyCount = 0;
}
}
}
}
void UpdatePlayerIcons()
{
for (int i = 0; i < playerIcons.Length; i++)
{
if(destroyCount <= i)
{
playerIcons[i].SetActive(true);
}
else
{
playerIcons[i].SetActive(false);
}
}
}
void Retry()
{
this.gameObject.SetActive(true);
playerHP = maxHP;
hpSlider.value = playerHP;
isMuteki = true;
Invoke("MutekiOff", 2.0f);
}
void MutekiOff()
{
isMuteki = false;
}
public void AddHP(int amount)
{
playerHP += amount;
if(playerHP > maxHP)
{
playerHP = maxHP;
}
hpSlider.value = playerHP;
}
// ★追加(自機1UPアイテム)
// 「public」を付けること(ポイント)
public void Player1UP(int amount)
{
// amount分だけ自機の残機を回復させる。
// (考え方)破壊された回数(「destroyCount」)をamount分だけ減少させる。
destroyCount -= amount;
// 最大残機数を超えないようにする(破壊された回数が0未満にならないようにする)
if(destroyCount < 0)
{
destroyCount = 0;
}
// 残機数を表示するアイコン
UpdatePlayerIcons();
}
}
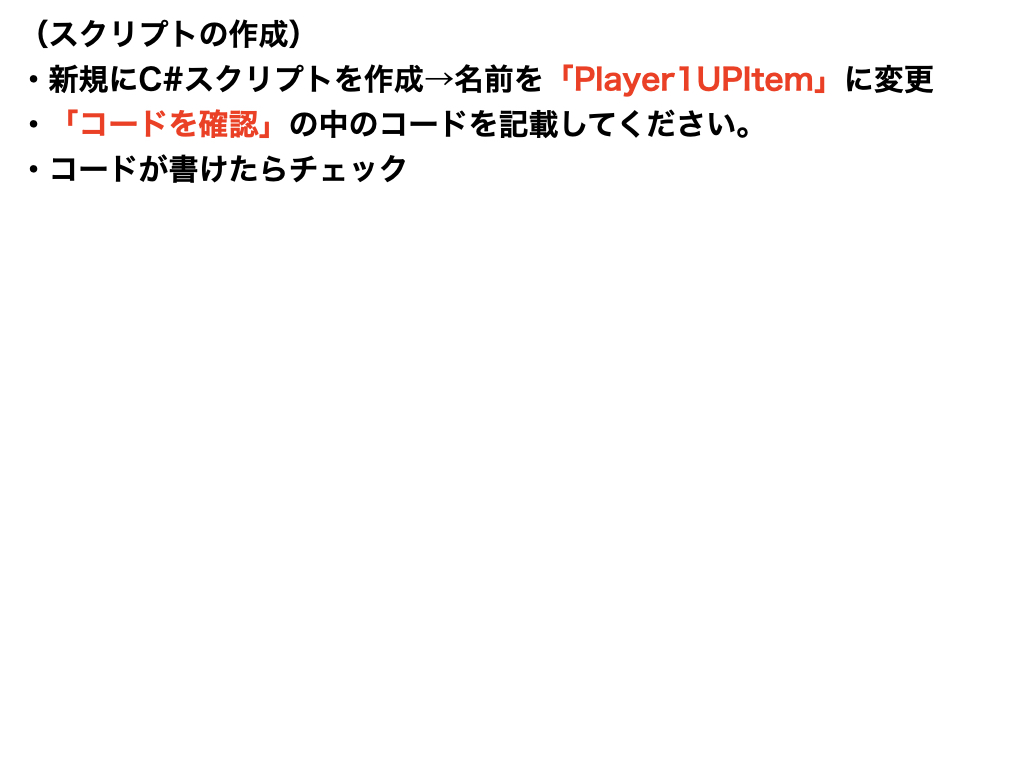
自機1UPアイテム(クラス承継)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player1UPItem : Item // 「MonoBehaviour」を「Item」に変更する(これで「Itemクラスを承継」することができます。)
{
private PlayerHealth ph;
private int reward = 1;
void Start()
{
ph = GameObject.Find("Player").GetComponent<PlayerHealth>();
}
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.tag == "Missile")
{
// (重要ポイント)ItemクラスのItemBaseメソッドを呼び出す。
base.ItemBase(other.gameObject);
if(ph != null)
{
// 自分で設定した分だけ自機が回復する。
ph.Player1UP(reward);
}
}
}
}
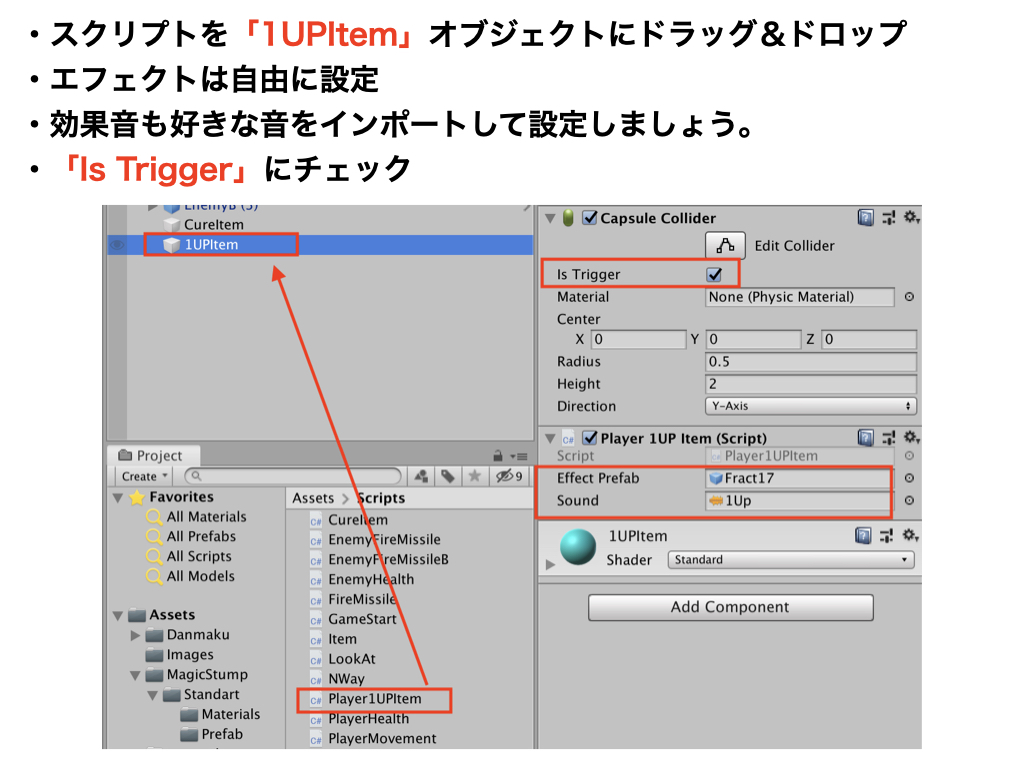
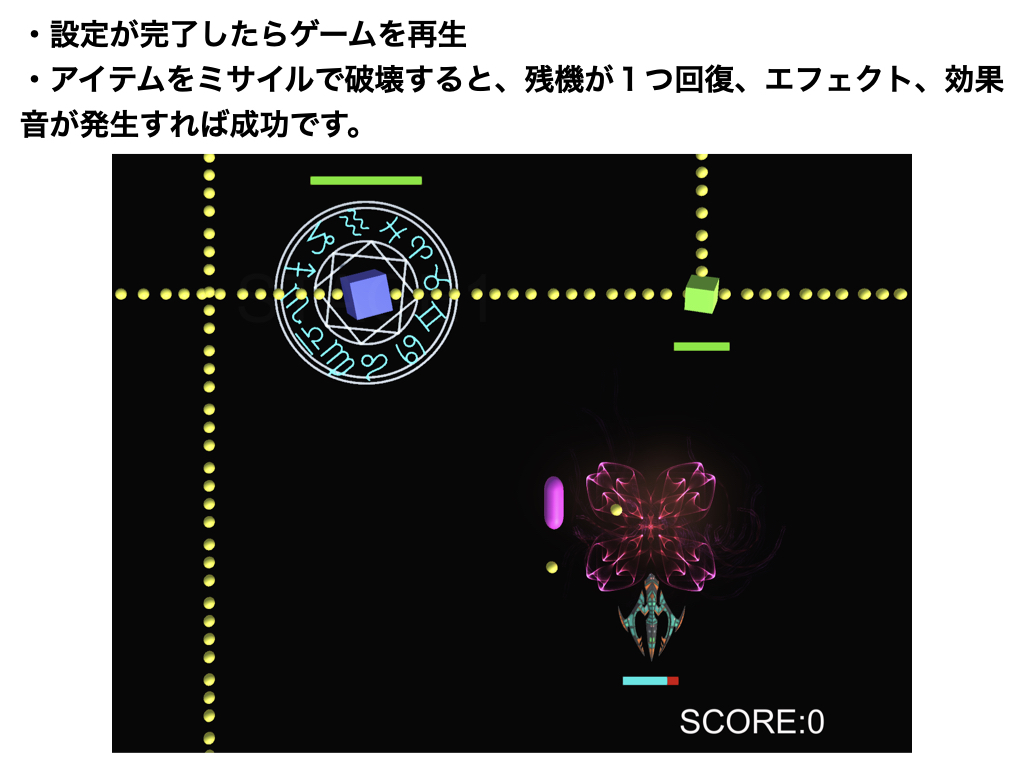
【2019版】Danmaku Ⅱ(基礎2/全38回)
他のコースを見る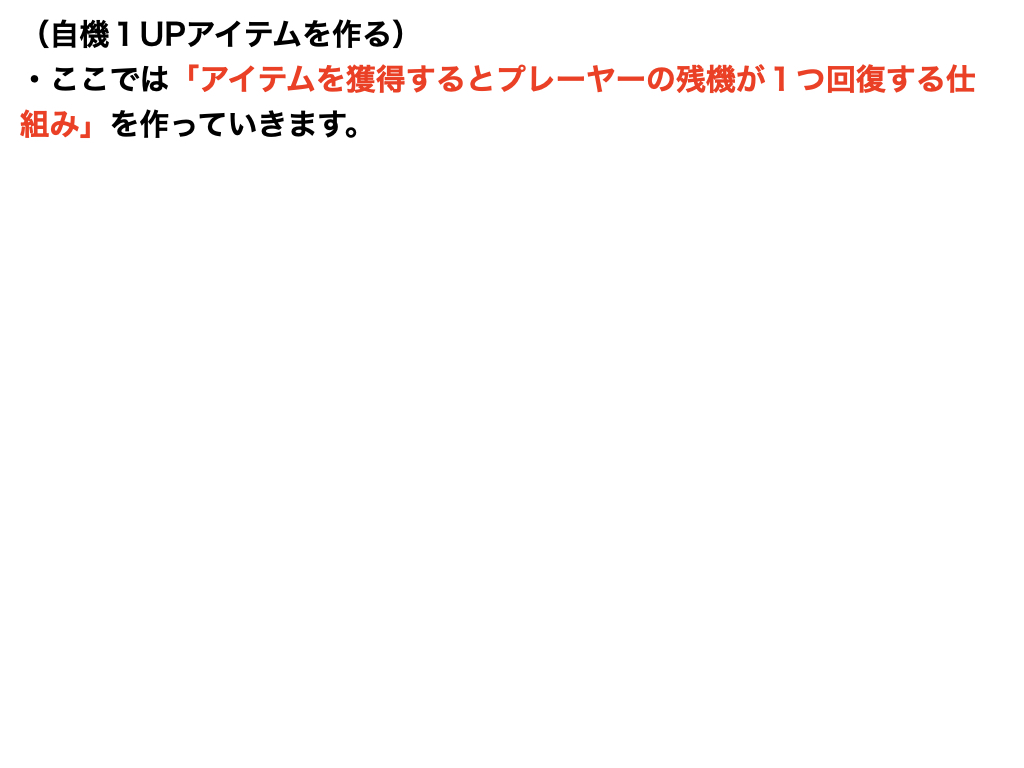
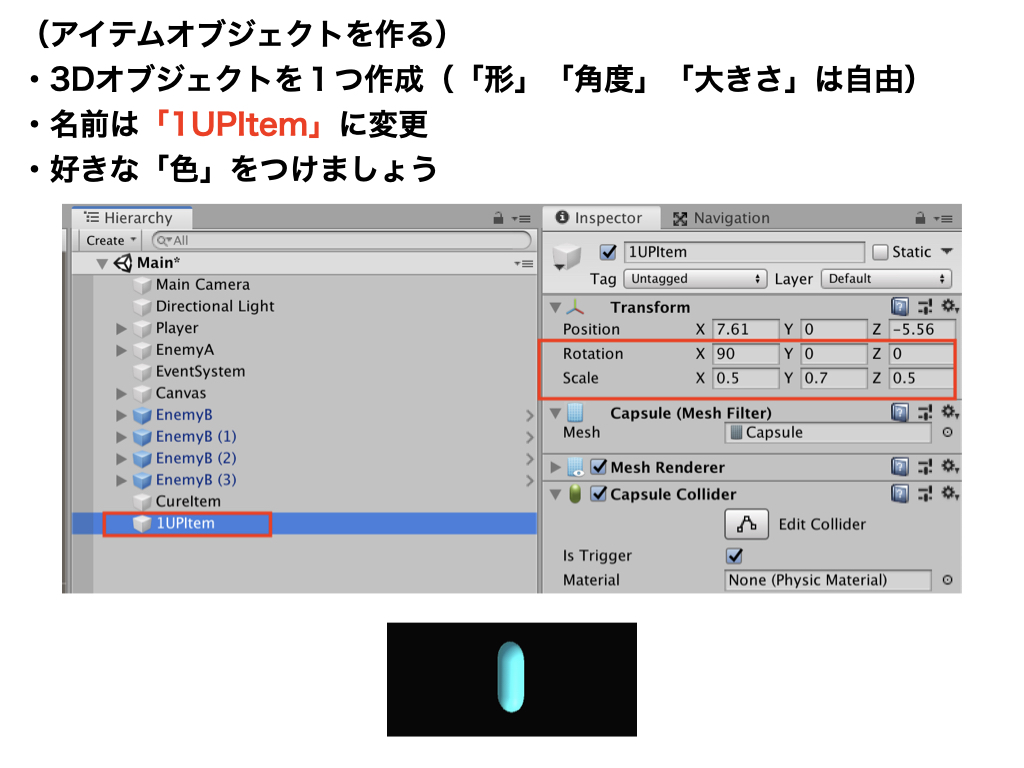
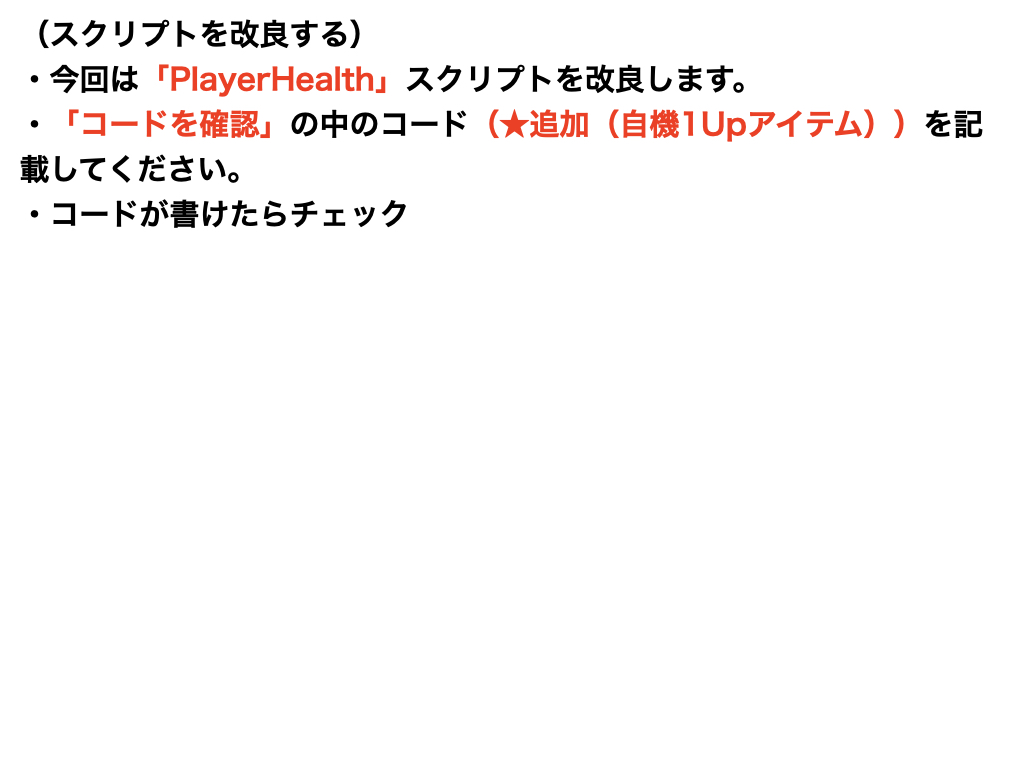
自機1UP
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class PlayerHealth : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip damageSound;
public AudioClip destroySound;
private int playerHP;
private int maxHP = 5;
public Slider hpSlider;
public GameObject[] playerIcons;
private int destroyCount = 0;
public bool isMuteki = false;
private void Start()
{
playerHP = maxHP;
hpSlider.maxValue = playerHP;
hpSlider.value = playerHP;
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("EnemyMissile") && isMuteki == false)
{
playerHP -= 1;
AudioSource.PlayClipAtPoint(damageSound, Camera.main.transform.position);
Destroy(other.gameObject);
hpSlider.value = playerHP;
if (playerHP == 0)
{
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 1.0f);
AudioSource.PlayClipAtPoint(destroySound, Camera.main.transform.position);
this.gameObject.SetActive(false);
destroyCount += 1;
UpdatePlayerIcons();
if(destroyCount < 5)
{
Invoke("Retry", 1.0f);
}
else
{
SceneManager.LoadScene("GameOver");
destroyCount = 0;
}
}
}
}
void UpdatePlayerIcons()
{
for (int i = 0; i < playerIcons.Length; i++)
{
if(destroyCount <= i)
{
playerIcons[i].SetActive(true);
}
else
{
playerIcons[i].SetActive(false);
}
}
}
void Retry()
{
this.gameObject.SetActive(true);
playerHP = maxHP;
hpSlider.value = playerHP;
isMuteki = true;
Invoke("MutekiOff", 2.0f);
}
void MutekiOff()
{
isMuteki = false;
}
public void AddHP(int amount)
{
playerHP += amount;
if(playerHP > maxHP)
{
playerHP = maxHP;
}
hpSlider.value = playerHP;
}
// ★追加(自機1UPアイテム)
// 「public」を付けること(ポイント)
public void Player1UP(int amount)
{
// amount分だけ自機の残機を回復させる。
// (考え方)破壊された回数(「destroyCount」)をamount分だけ減少させる。
destroyCount -= amount;
// 最大残機数を超えないようにする(破壊された回数が0未満にならないようにする)
if(destroyCount < 0)
{
destroyCount = 0;
}
// 残機数を表示するアイコン
UpdatePlayerIcons();
}
}
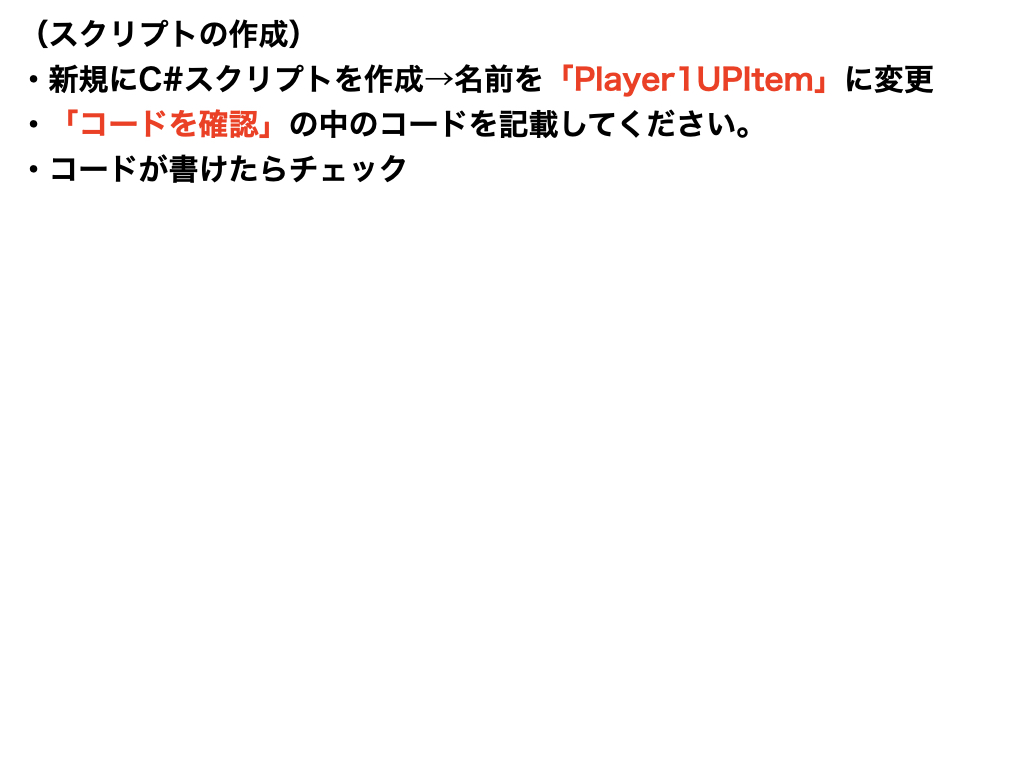
自機1UPアイテム(クラス承継)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player1UPItem : Item // 「MonoBehaviour」を「Item」に変更する(これで「Itemクラスを承継」することができます。)
{
private PlayerHealth ph;
private int reward = 1;
void Start()
{
ph = GameObject.Find("Player").GetComponent<PlayerHealth>();
}
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.tag == "Missile")
{
// (重要ポイント)ItemクラスのItemBaseメソッドを呼び出す。
base.ItemBase(other.gameObject);
if(ph != null)
{
// 自分で設定した分だけ自機が回復する。
ph.Player1UP(reward);
}
}
}
}
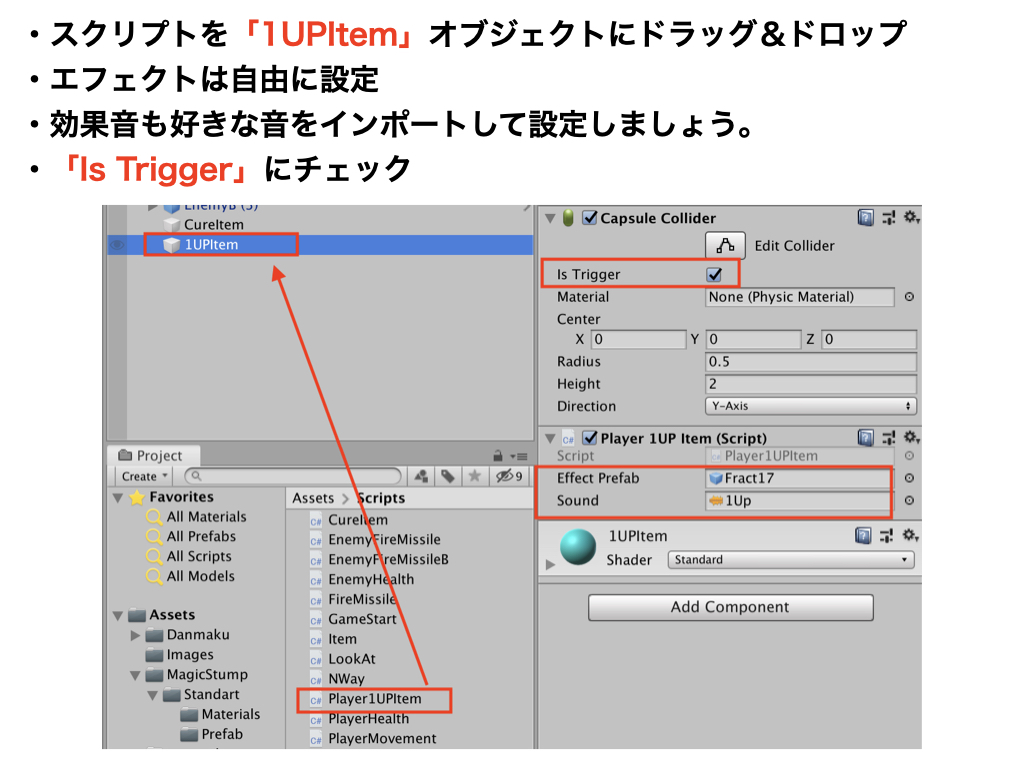
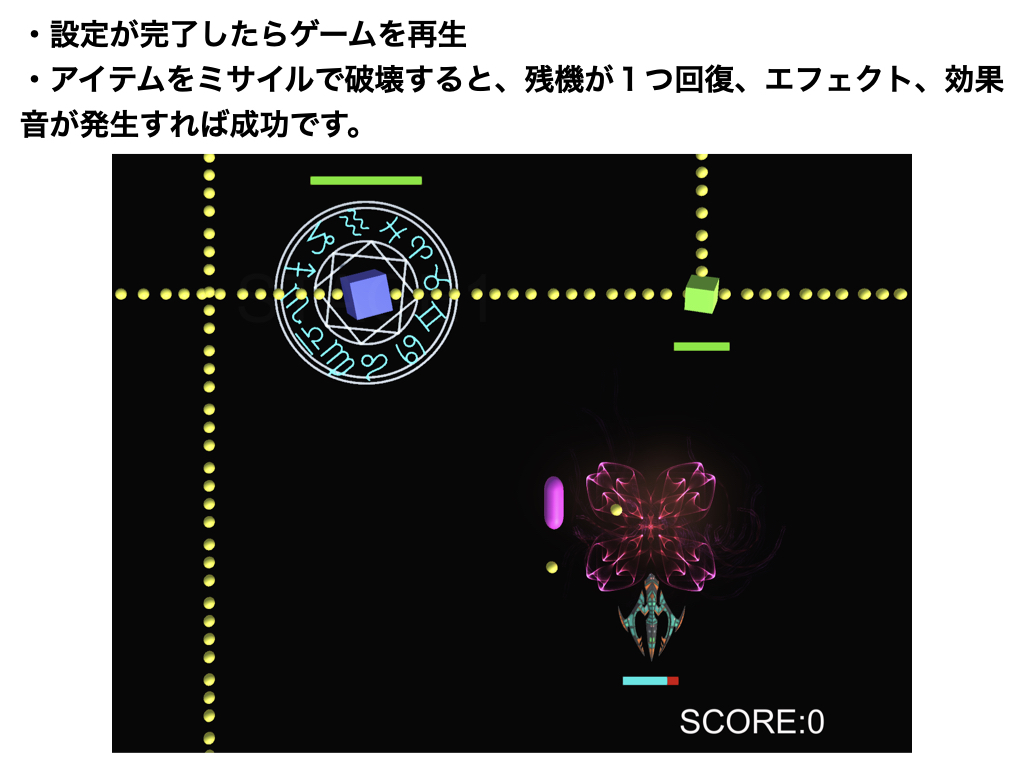
アイテムの作成③(自機1UP)