アイテムの作成⑤(防御シールド)
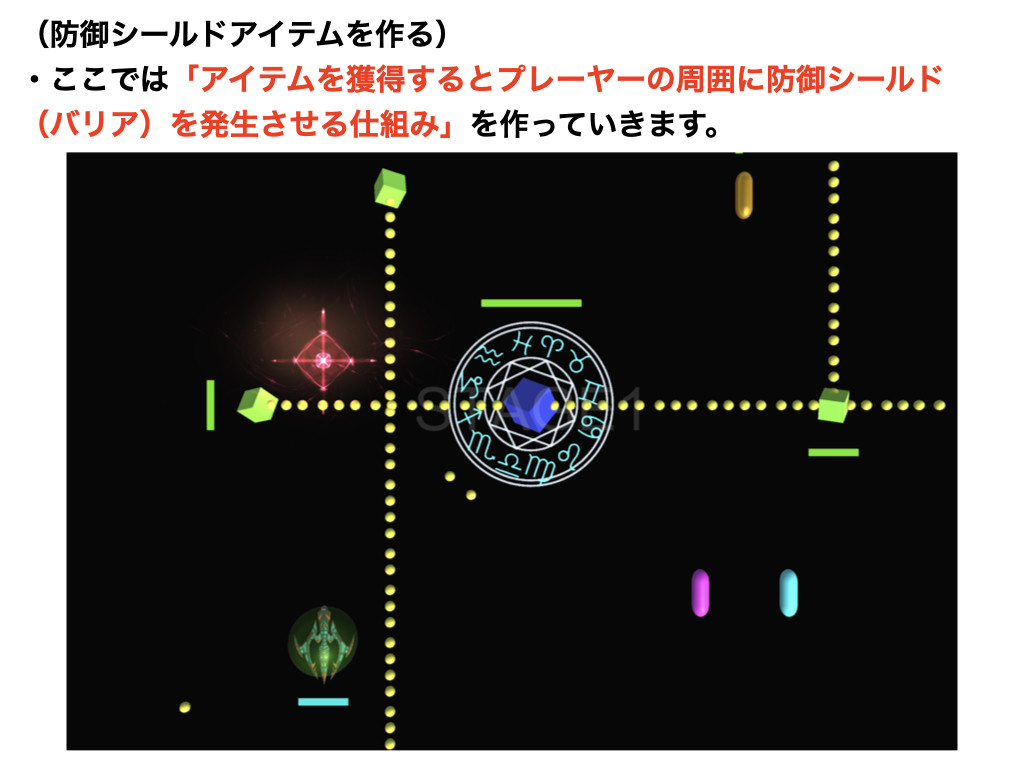
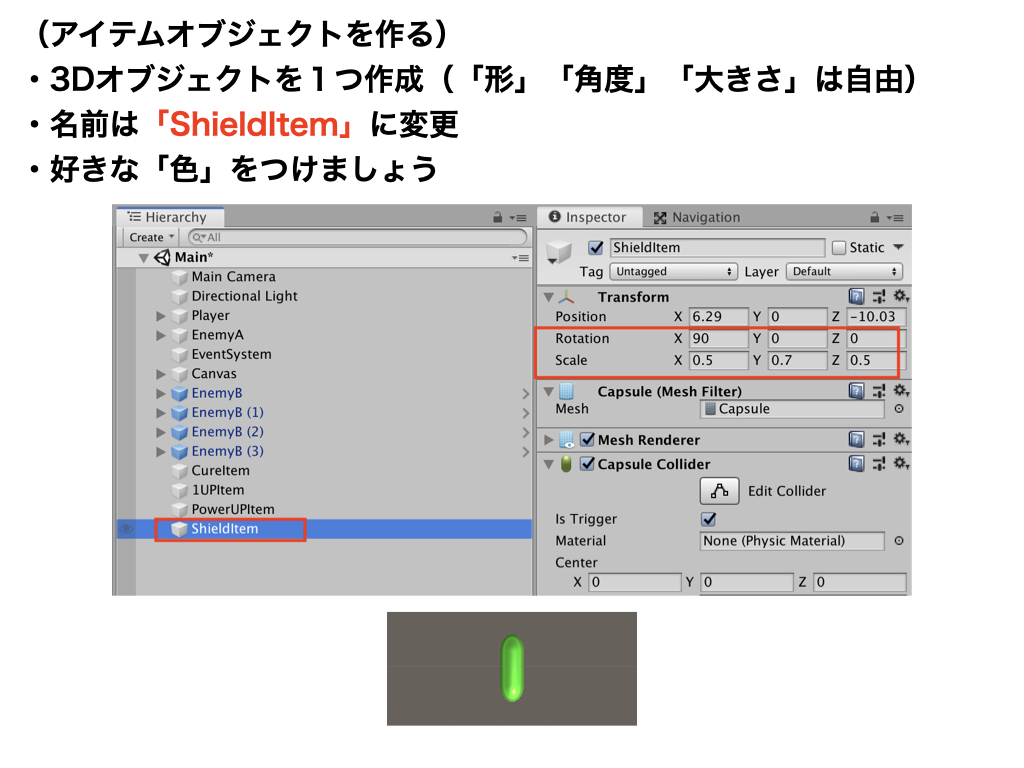
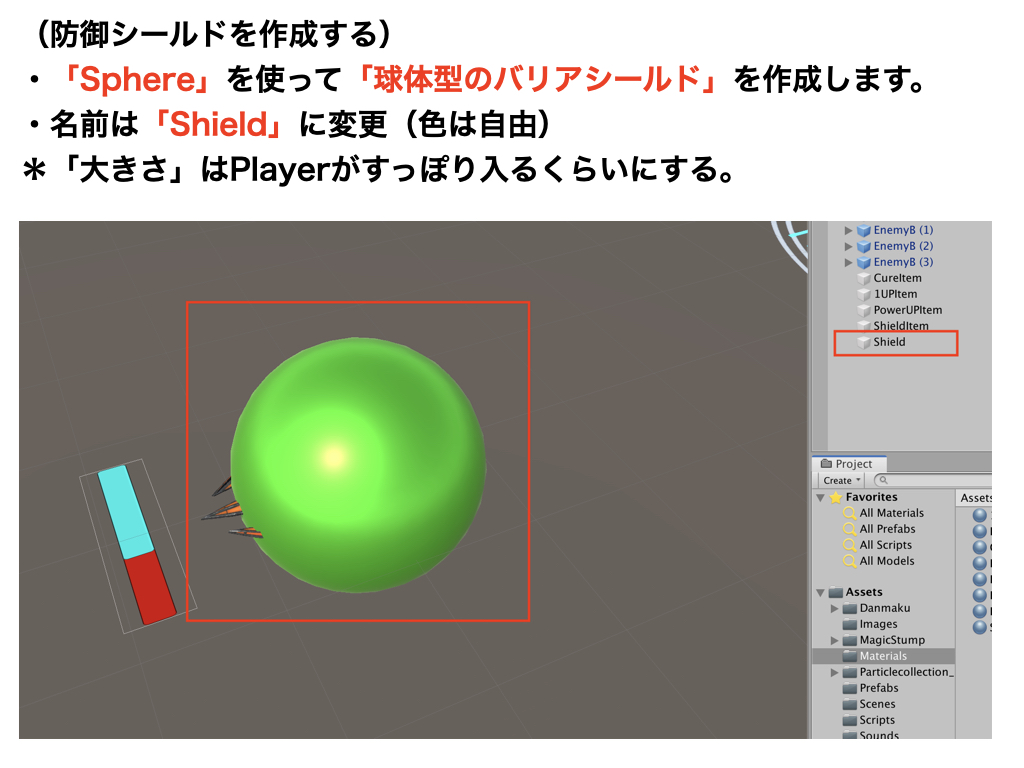
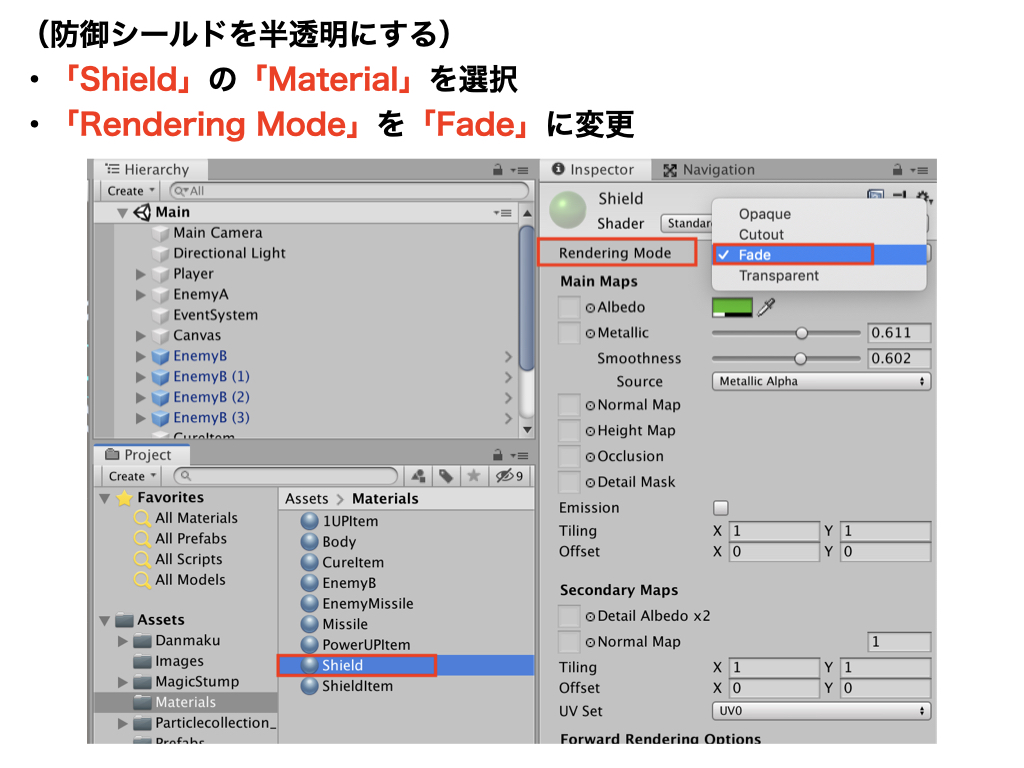
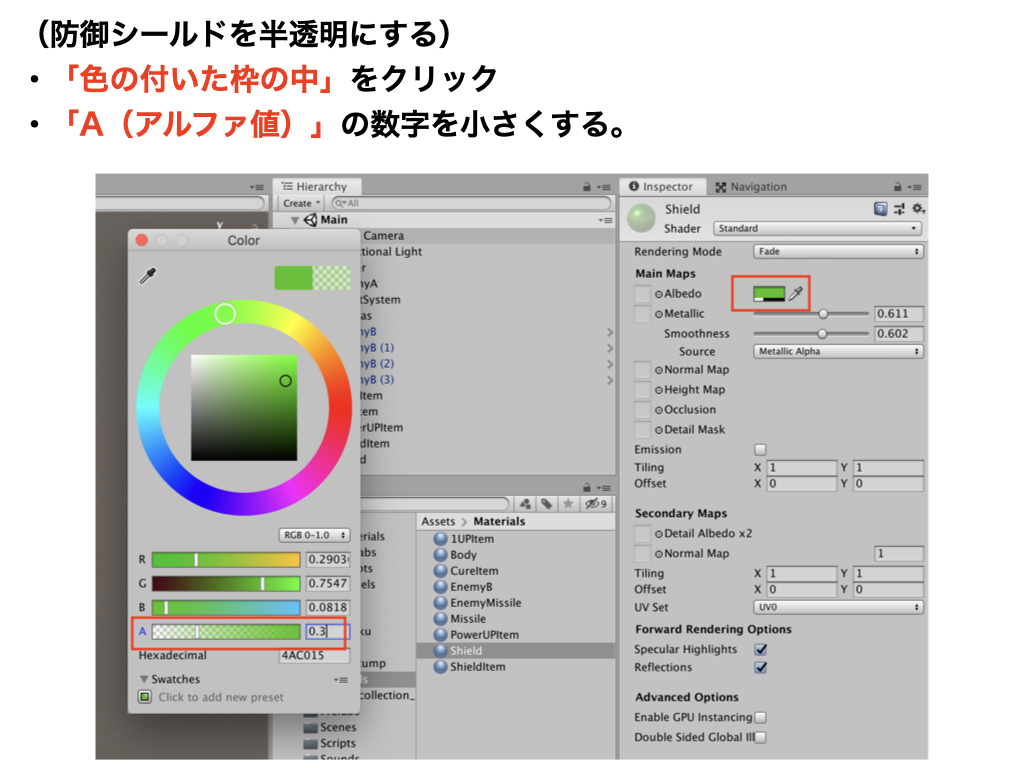
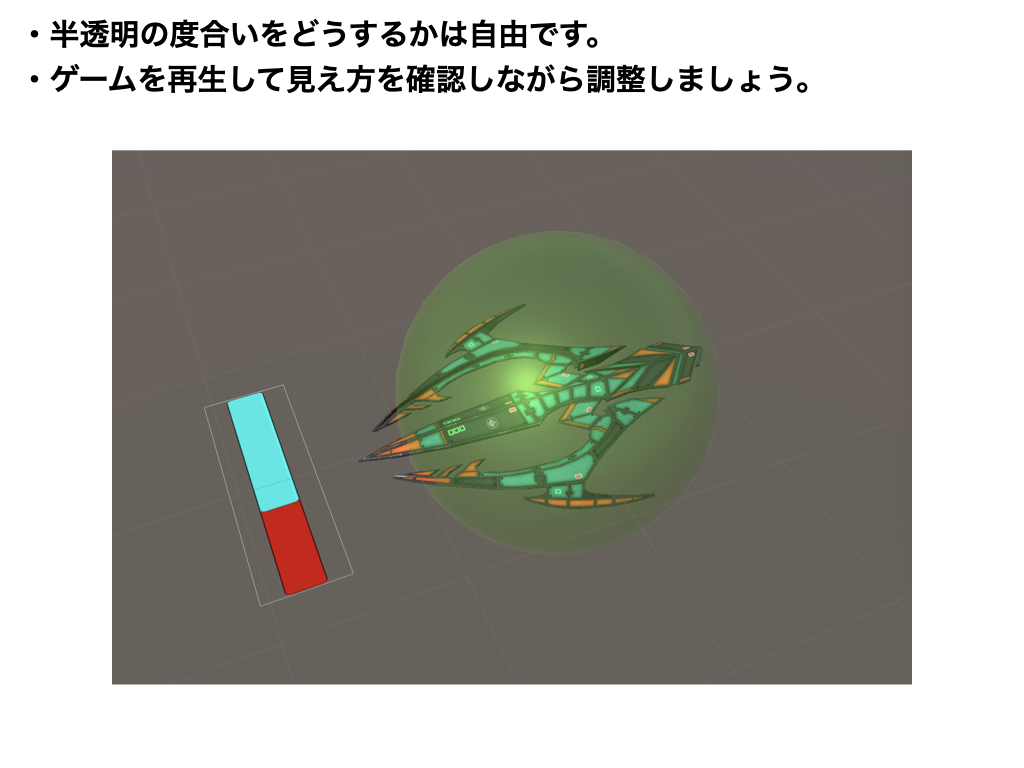
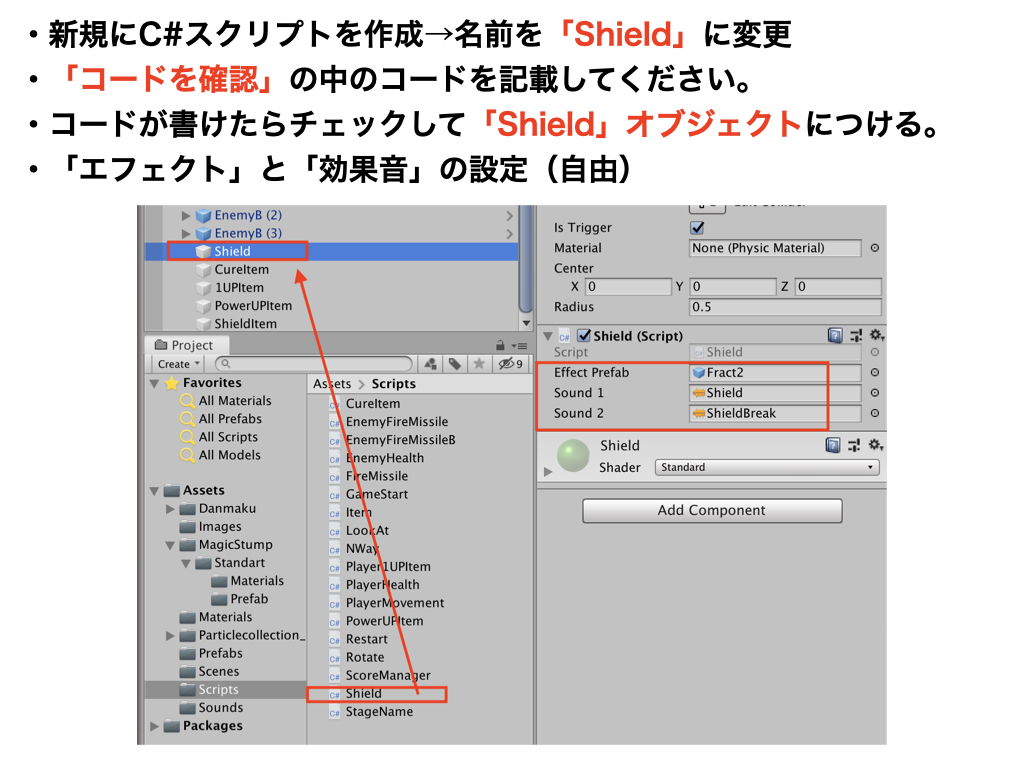
防御シールド
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Shield : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip sound1;
public AudioClip sound2;
void Start()
{
Invoke("DestroyShield", 5.0f);
}
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.tag == "EnemyMissile")
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(sound1, Camera.main.transform.position);
GameObject effect = Instantiate(effectPrefab, other.transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
}
}
void DestroyShield()
{
Destroy(gameObject);
AudioSource.PlayClipAtPoint(sound2, Camera.main.transform.position);
}
}
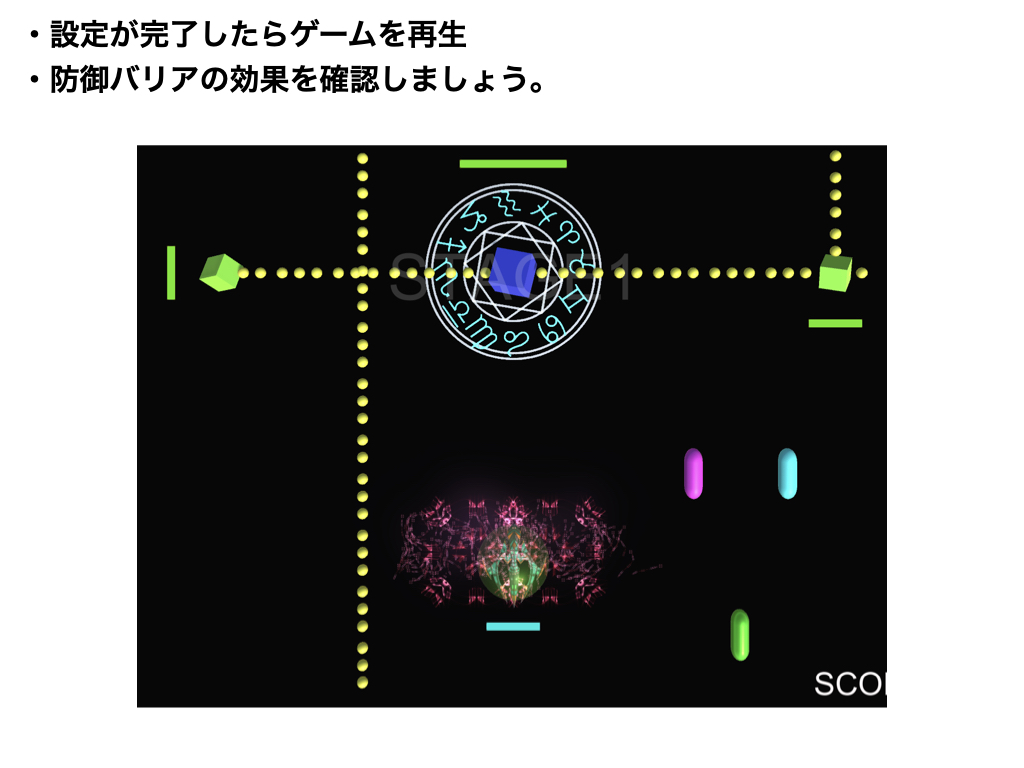
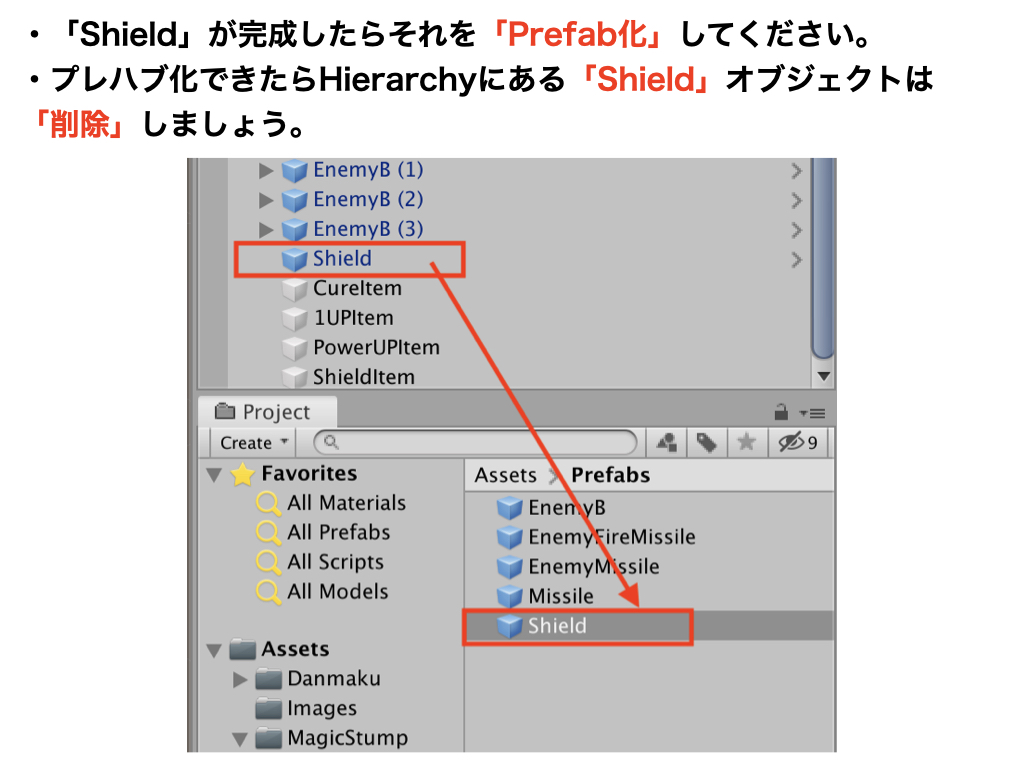
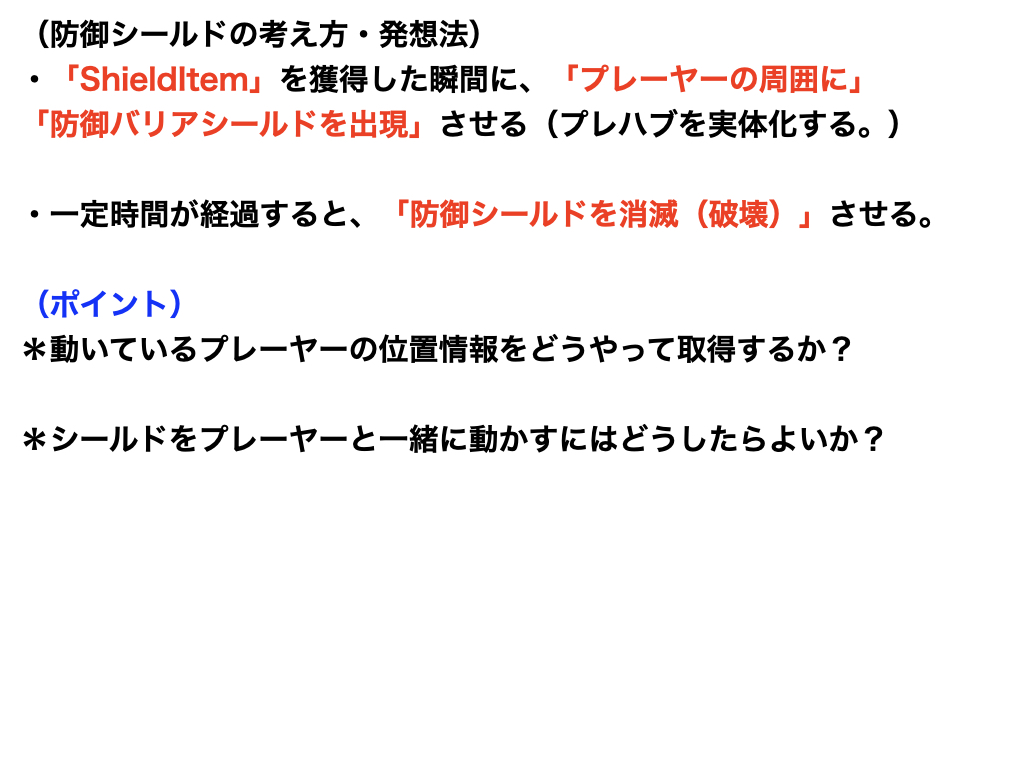
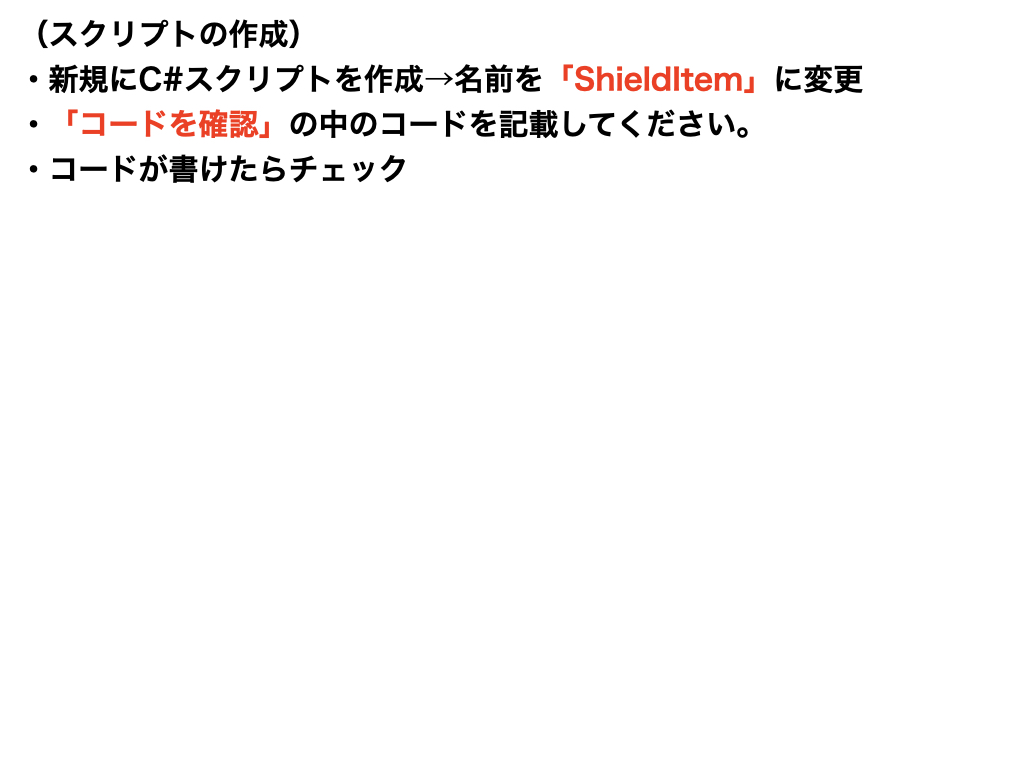
シールドアイテム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShieldItem : Item
{
public GameObject shieldPrefab;
private GameObject player;
private Vector3 playerPos;
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.tag == "Missile")
{
base.ItemBase(other.gameObject);
// プレーヤーの位置情報を取得する。
player = GameObject.Find("Player");
if(player)
{
playerPos = player.transform.position;
// プレーヤーの位置に防御シールドを発生させる。
GameObject shield = Instantiate(shieldPrefab, playerPos, Quaternion.identity);
// 発生させた防御シールドをプレーヤーの子供に設定する(親子関係)
// 親子関係にすることで一緒に動くようになる(ポイント)
shield.transform.SetParent(player.transform);
}
}
}
}
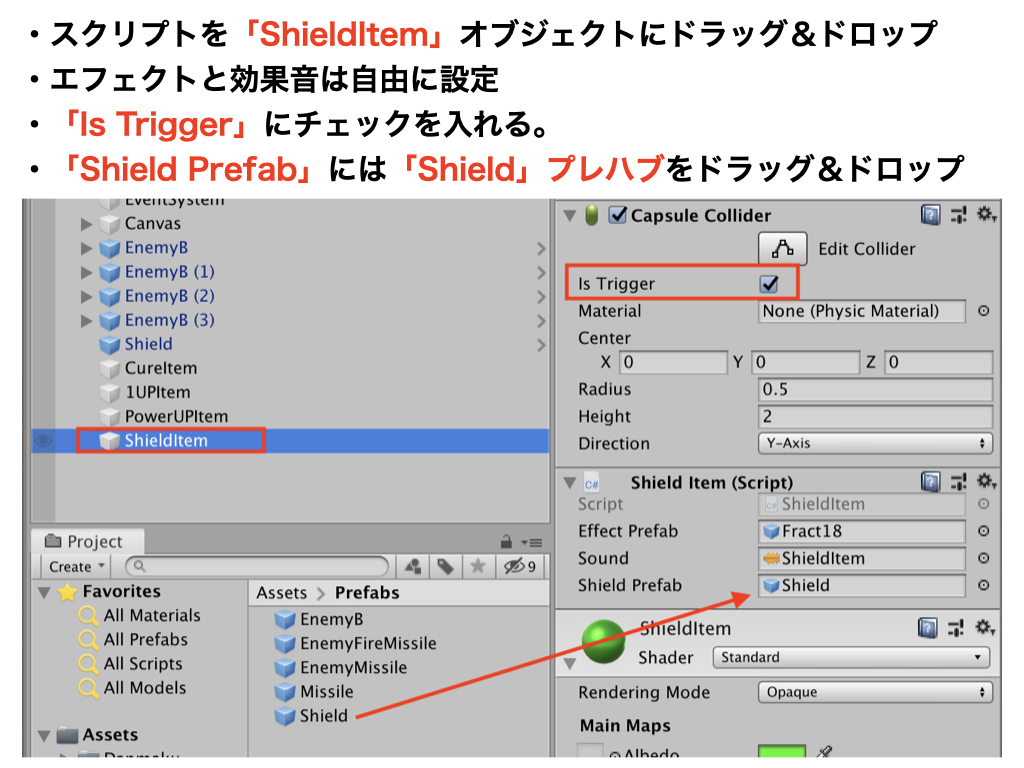
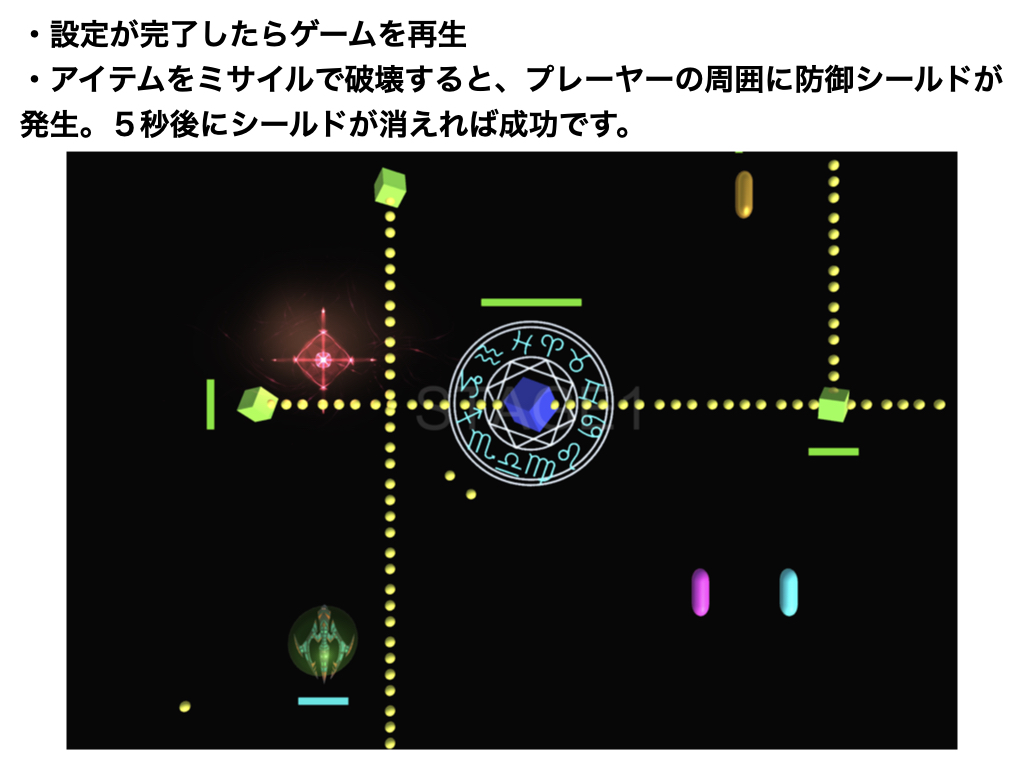
【2019版】Danmaku Ⅱ(基礎2/全38回)
他のコースを見る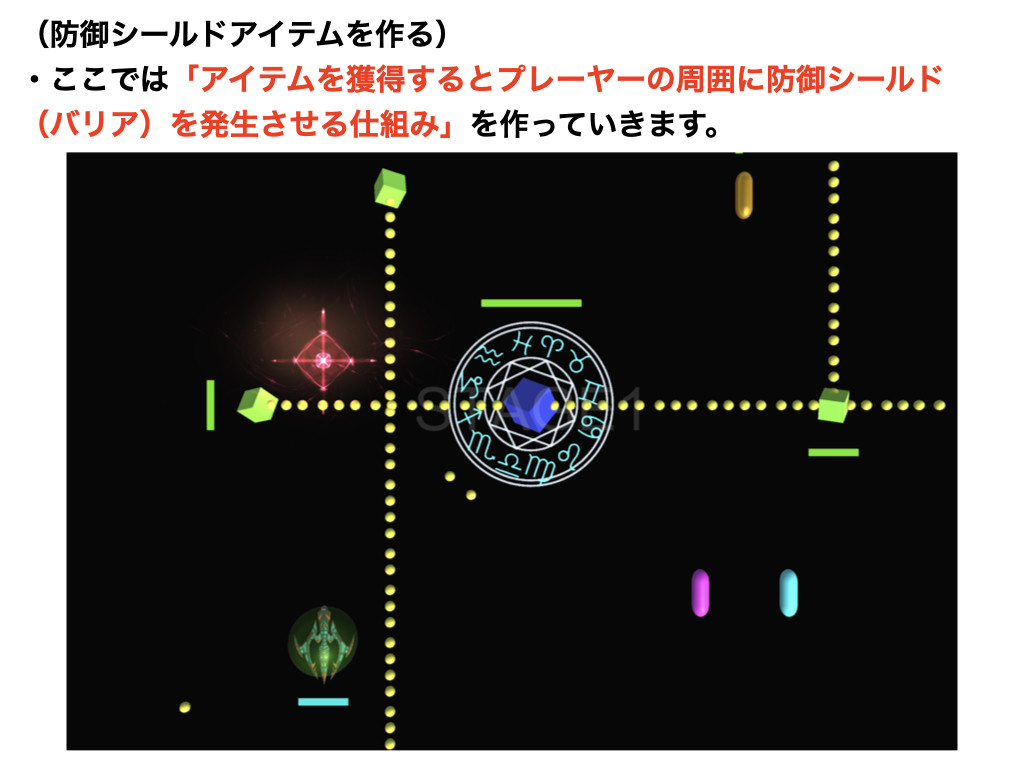
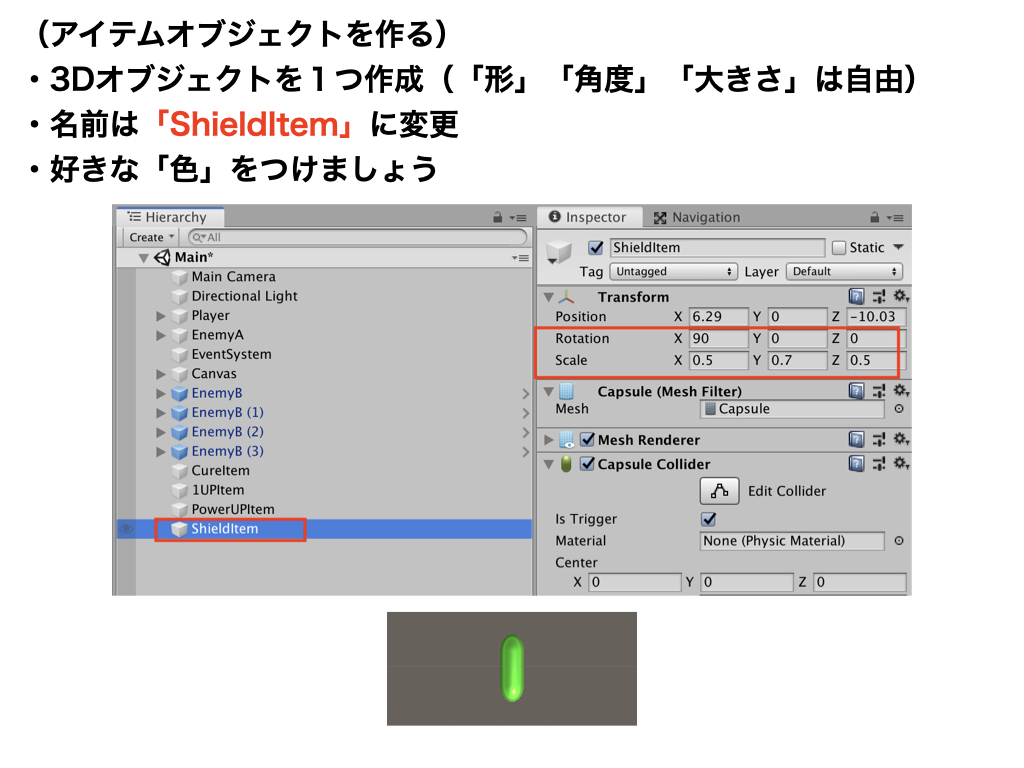
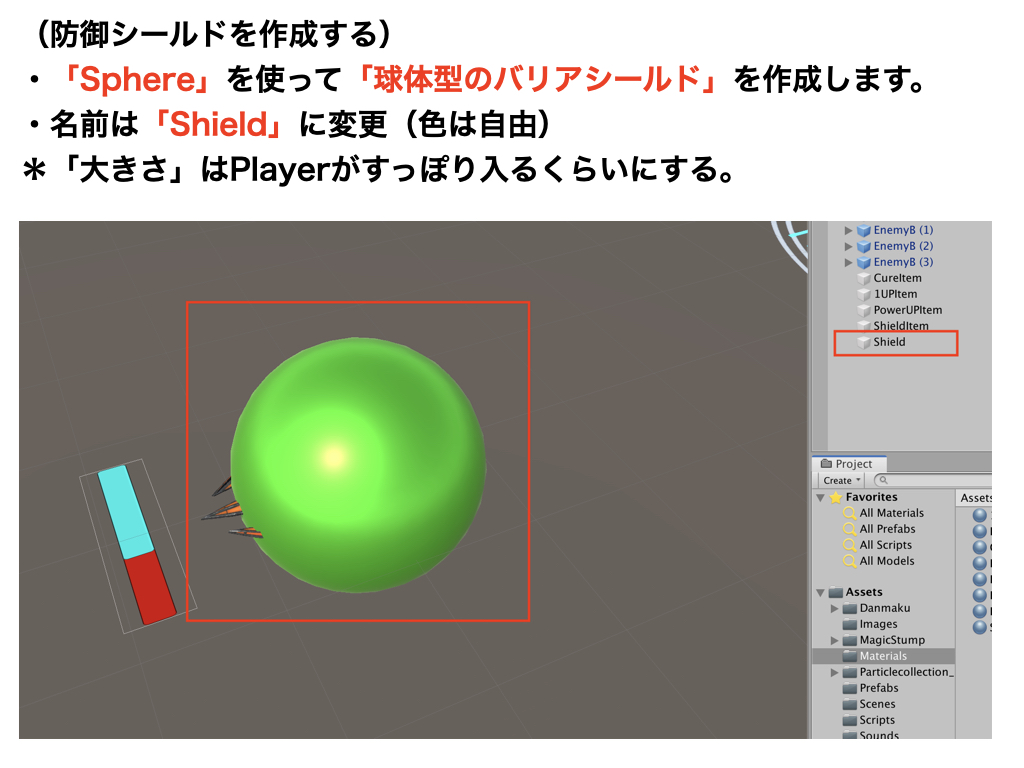
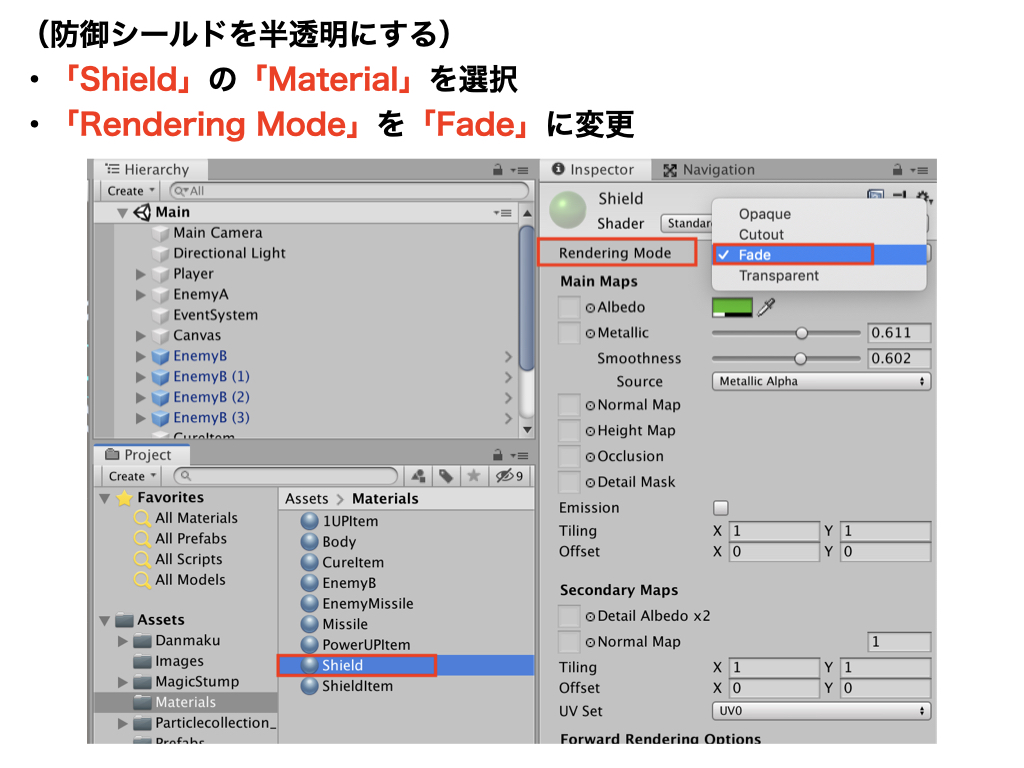
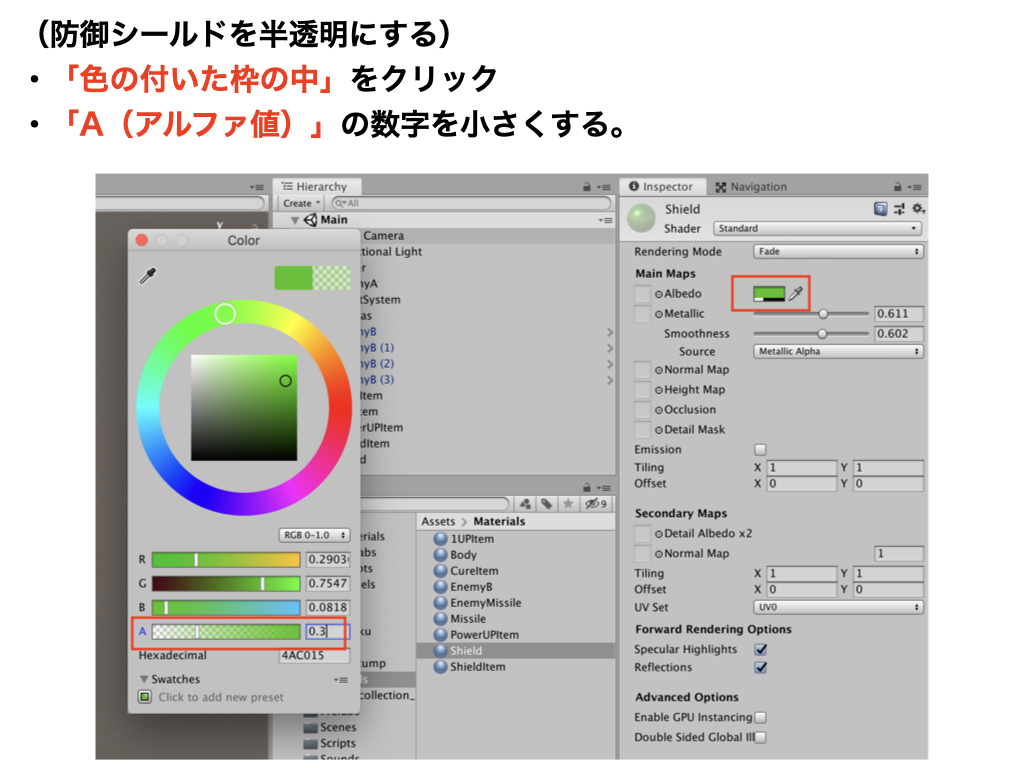
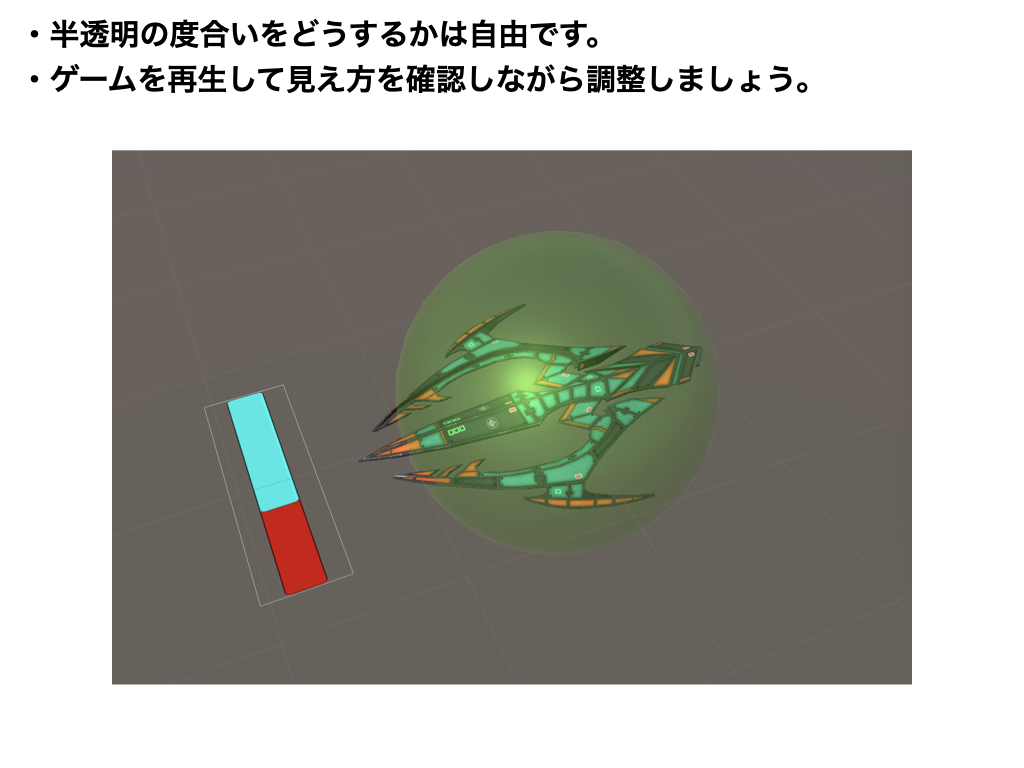
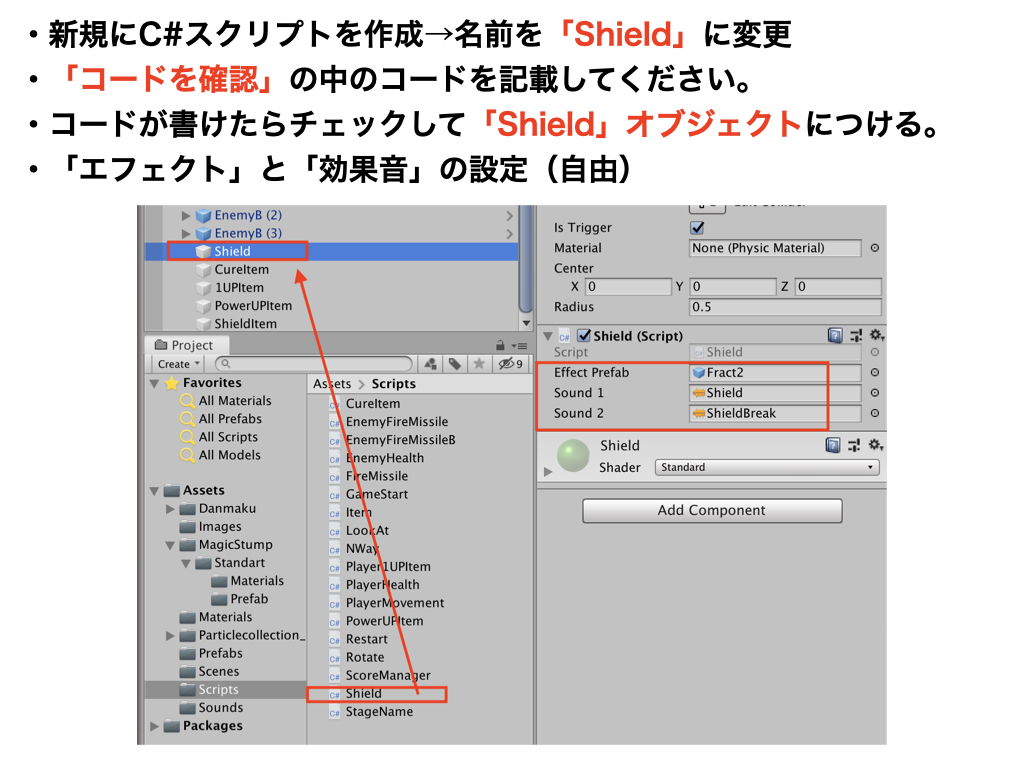
防御シールド
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Shield : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip sound1;
public AudioClip sound2;
void Start()
{
Invoke("DestroyShield", 5.0f);
}
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.tag == "EnemyMissile")
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(sound1, Camera.main.transform.position);
GameObject effect = Instantiate(effectPrefab, other.transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
}
}
void DestroyShield()
{
Destroy(gameObject);
AudioSource.PlayClipAtPoint(sound2, Camera.main.transform.position);
}
}
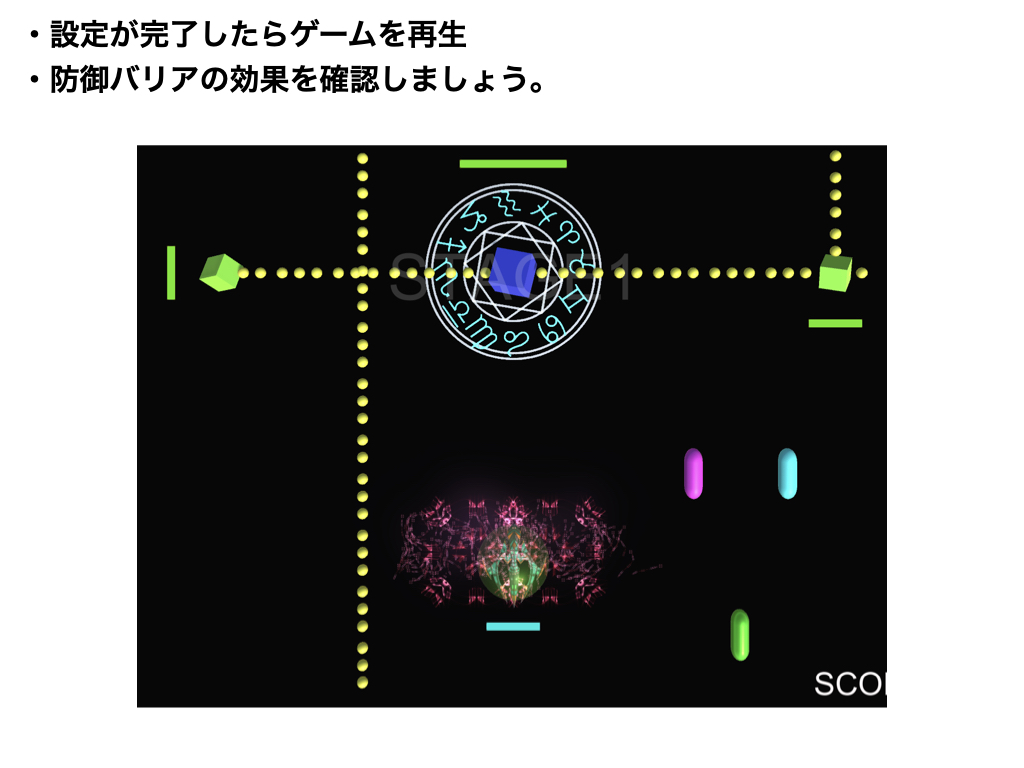
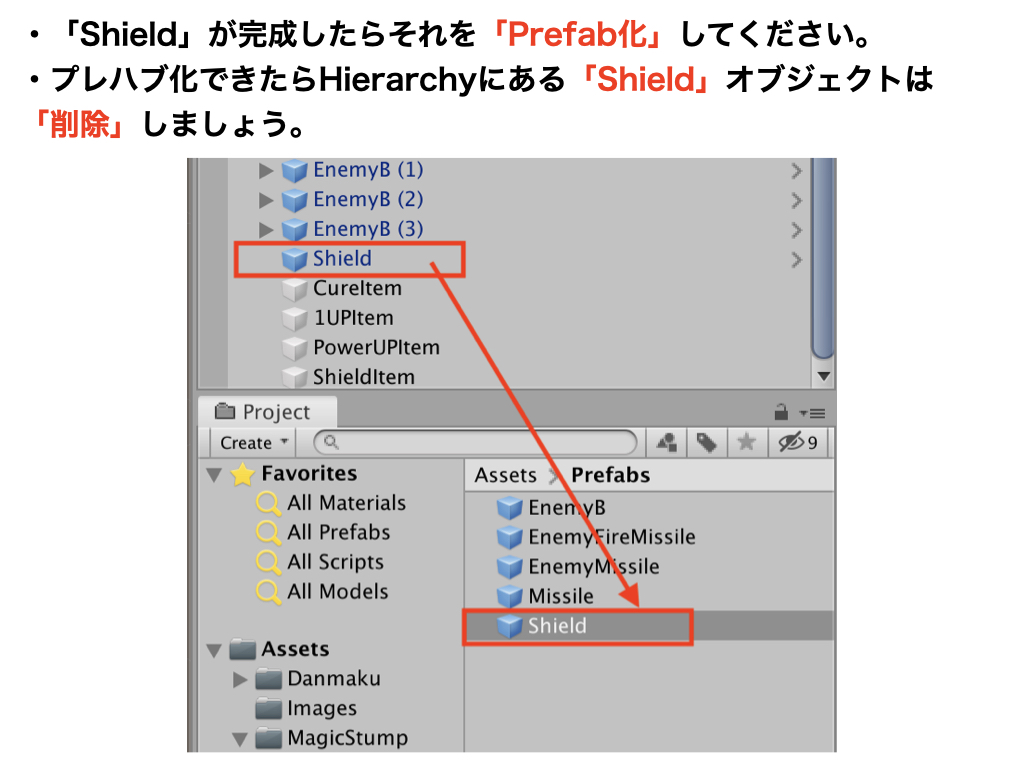
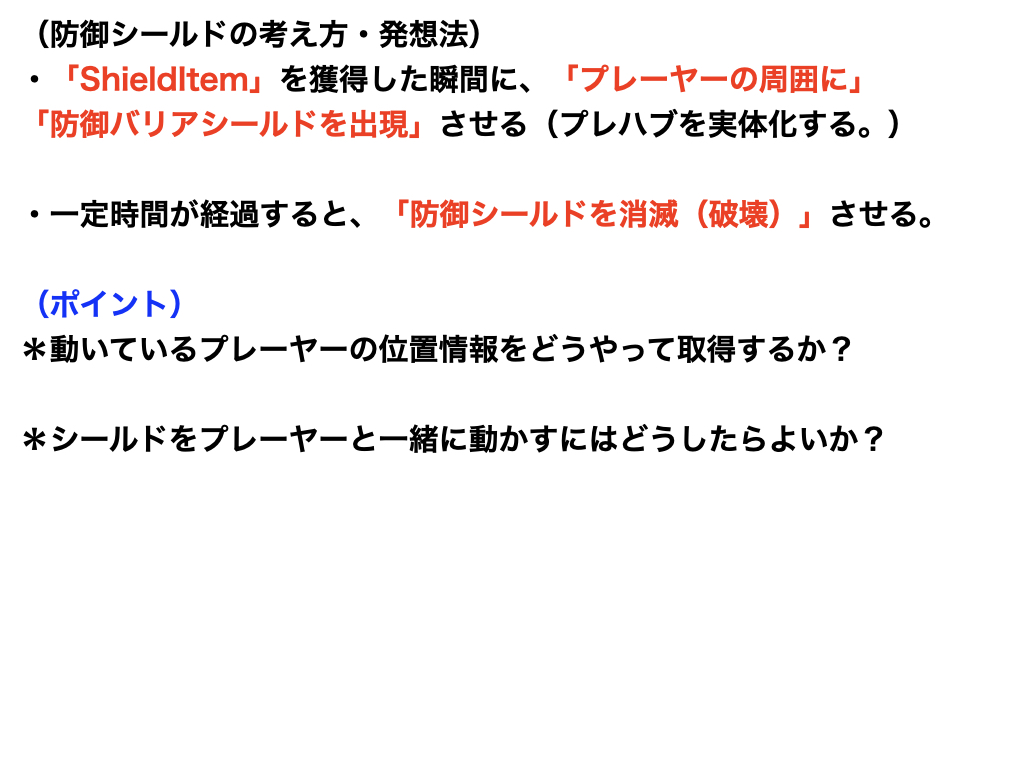
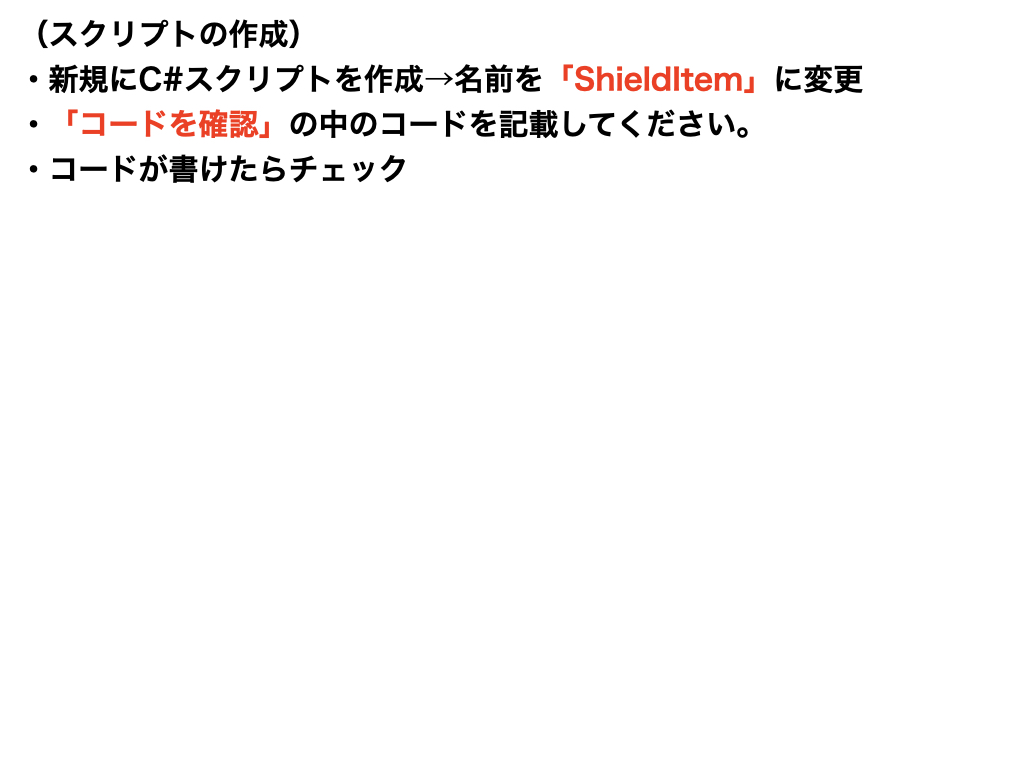
シールドアイテム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShieldItem : Item
{
public GameObject shieldPrefab;
private GameObject player;
private Vector3 playerPos;
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.tag == "Missile")
{
base.ItemBase(other.gameObject);
// プレーヤーの位置情報を取得する。
player = GameObject.Find("Player");
if(player)
{
playerPos = player.transform.position;
// プレーヤーの位置に防御シールドを発生させる。
GameObject shield = Instantiate(shieldPrefab, playerPos, Quaternion.identity);
// 発生させた防御シールドをプレーヤーの子供に設定する(親子関係)
// 親子関係にすることで一緒に動くようになる(ポイント)
shield.transform.SetParent(player.transform);
}
}
}
}
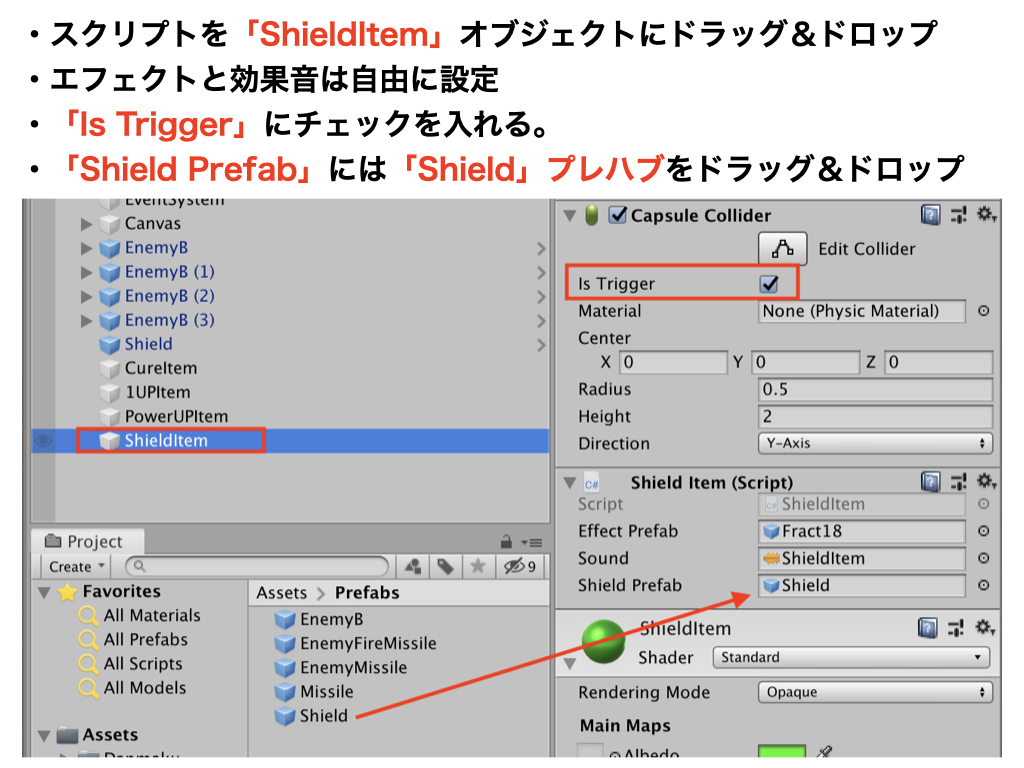
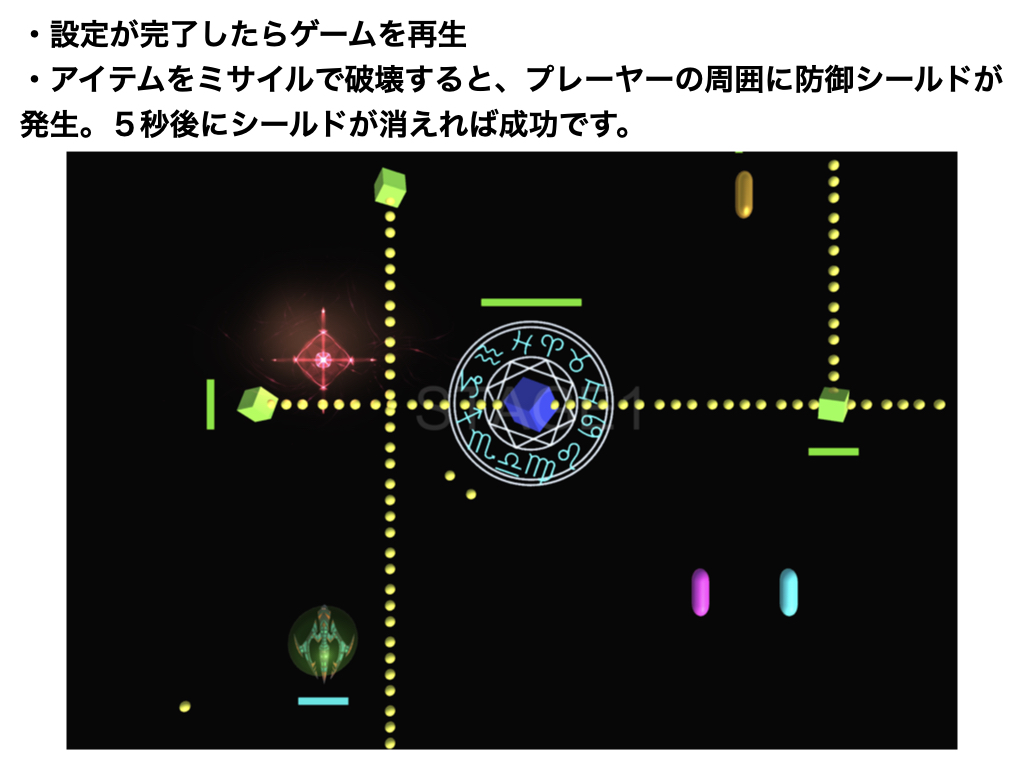
アイテムの作成⑤(防御シールド)