照準器を作る
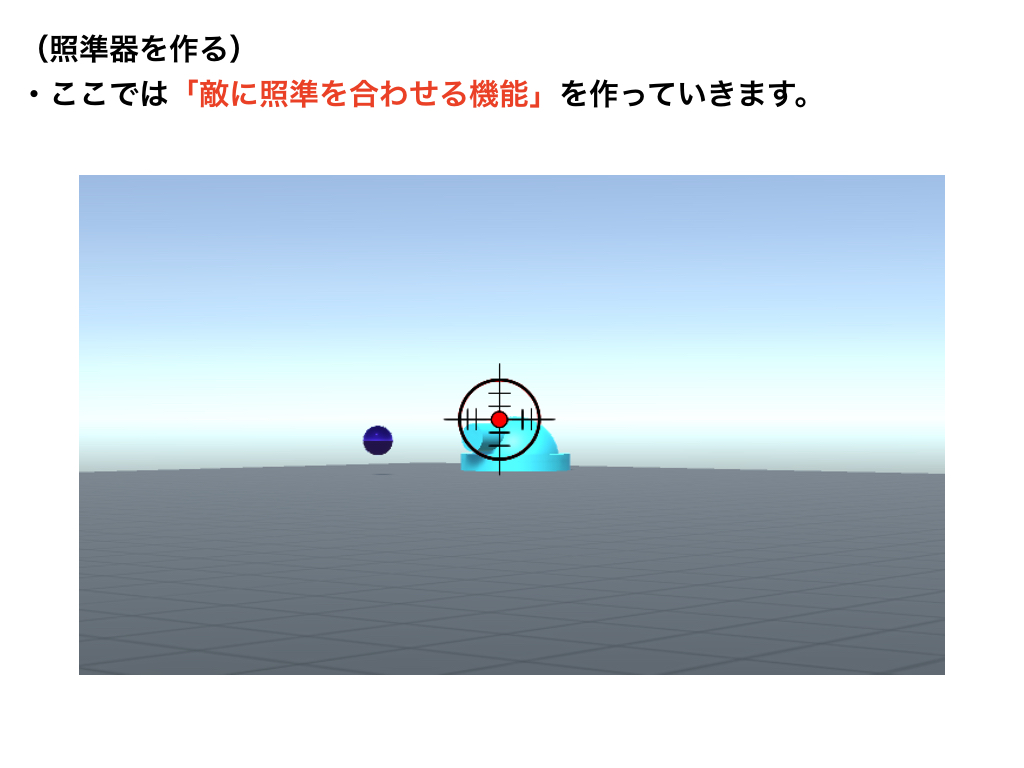
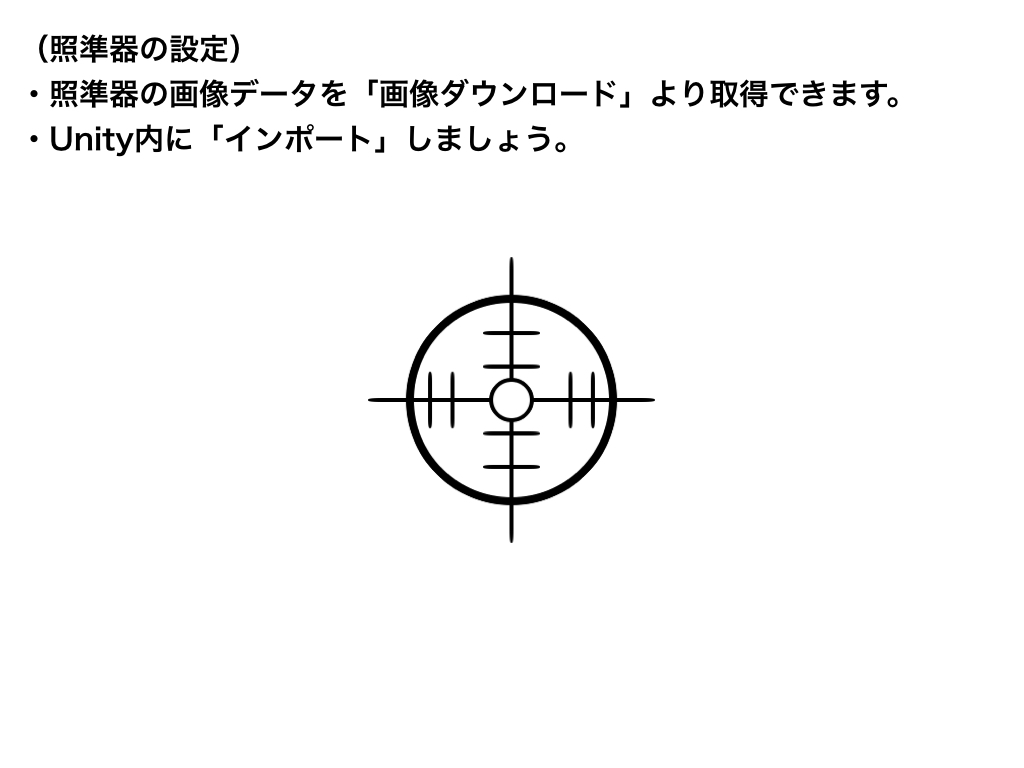
https://mono-pro.net/unity-codegenius-battletank-aim/aiming.zip画像ダウンロード
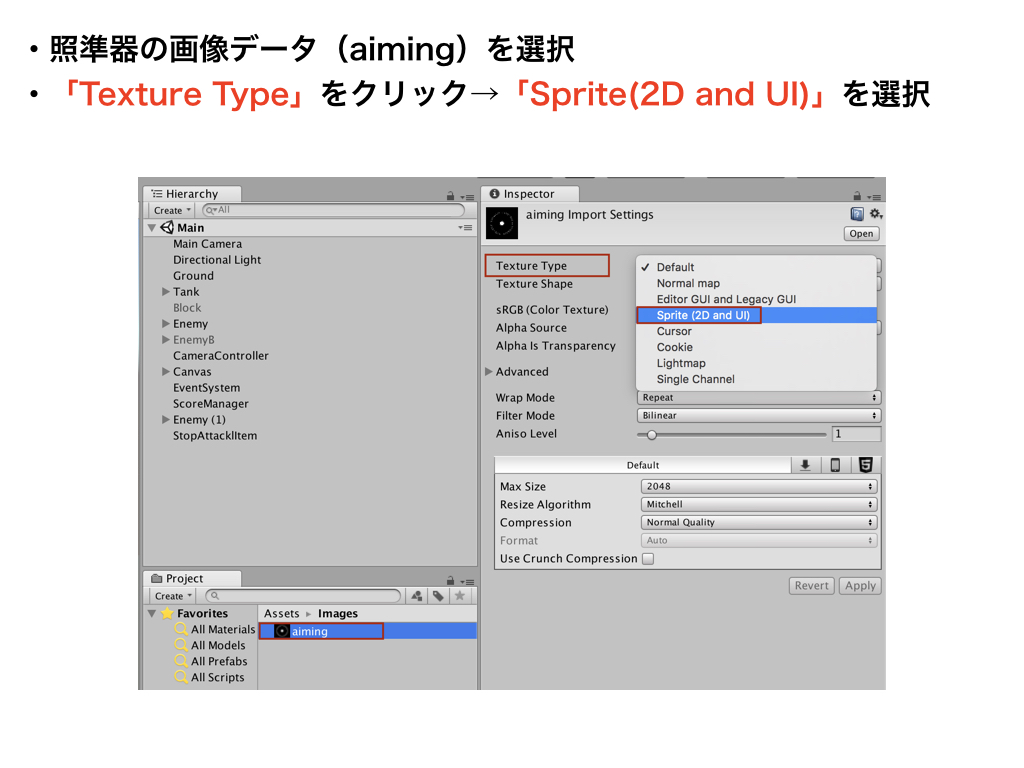
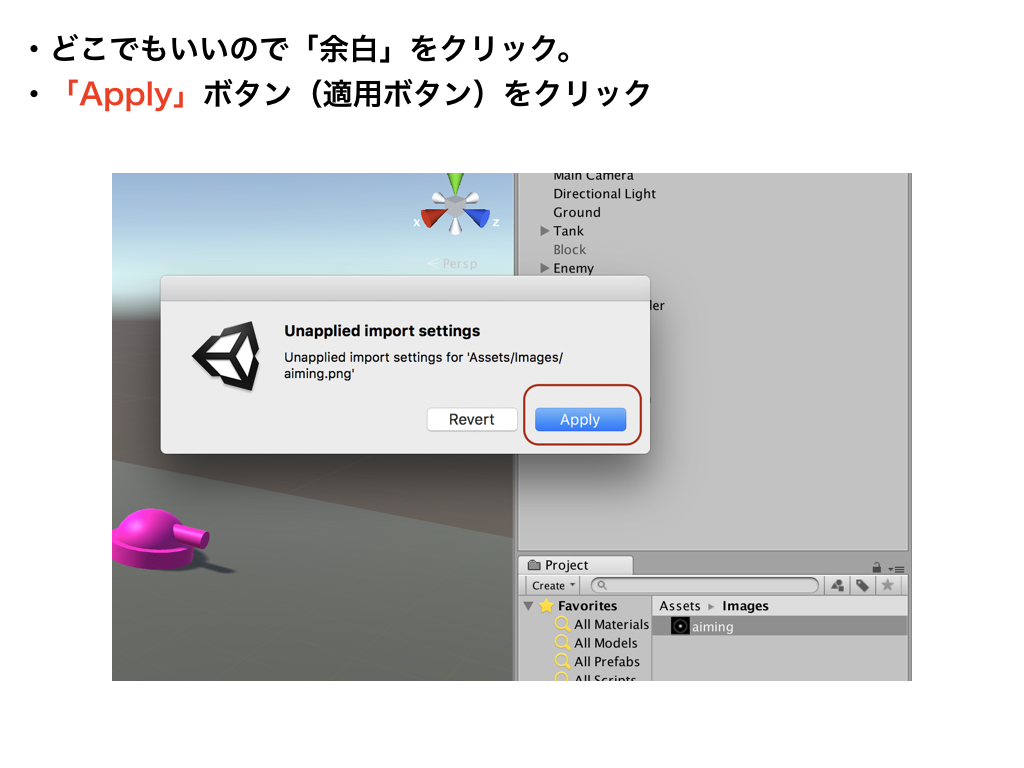
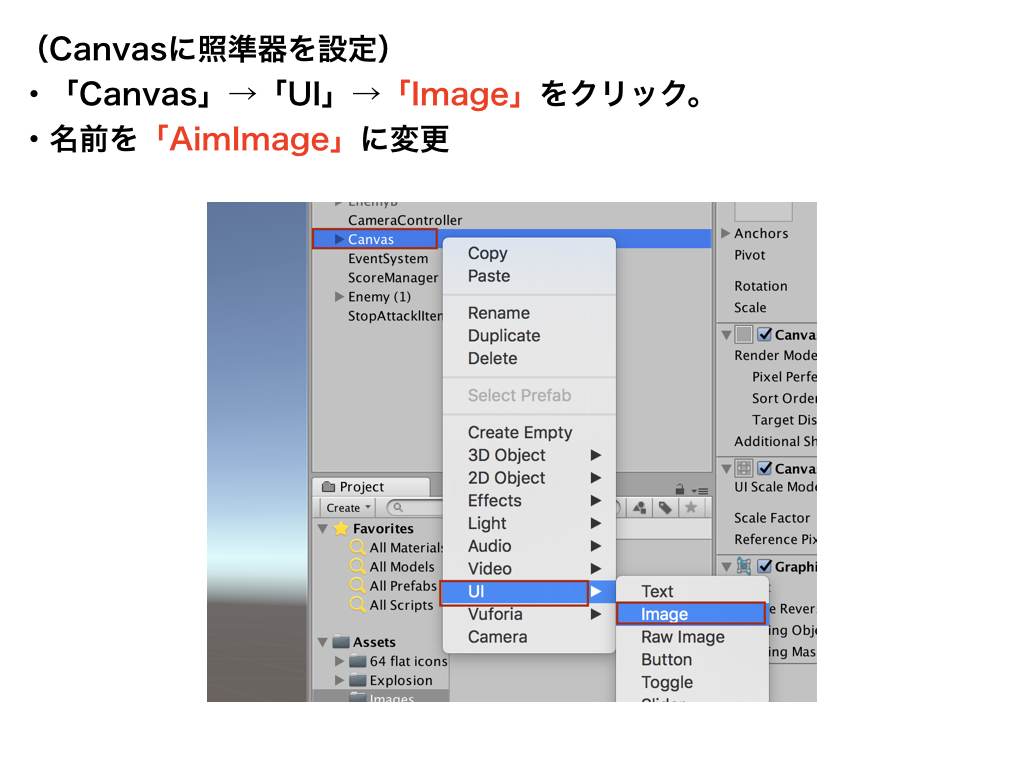
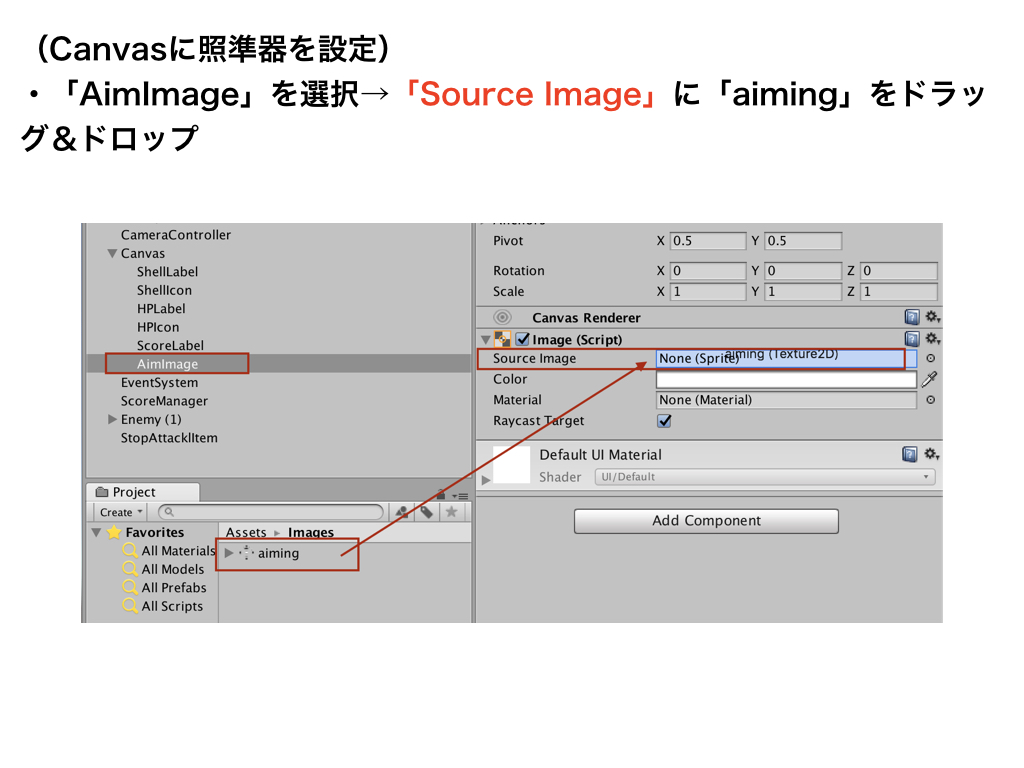
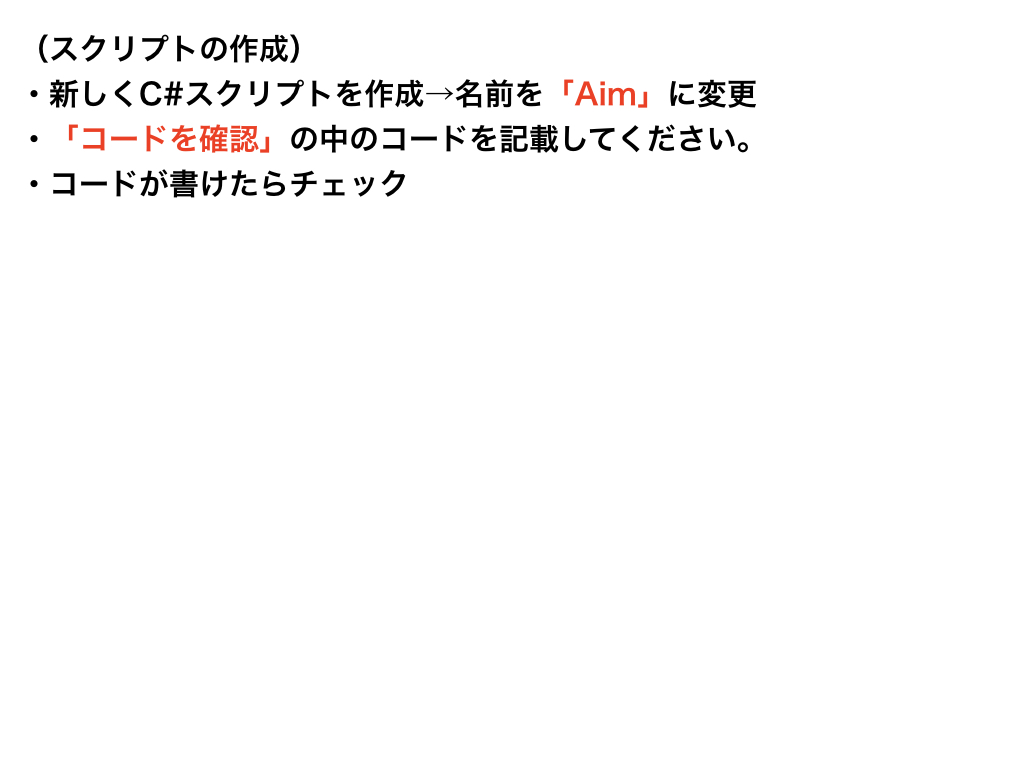
Rayで照準器を作る
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// 追加しましょう(ポイント)
using UnityEngine.UI;
public class Aim : MonoBehaviour
{
public Image aimImage;
void Update()
{
// レーザー(ray)を飛ばす「起点」と「方向」
Ray ray = new Ray(transform.position, transform.forward);
// rayのあたり判定の情報を入れる箱を作る。
RaycastHit hit;
if (Physics.Raycast(ray, out hit, 60))
{
string hitName = hit.transform.gameObject.tag;
if (hitName == "Enemy")
{
// 照準器の色を「赤」に変える(色は自由に変更してください。)
aimImage.color = new Color(1.0f, 0.0f, 0.0f, 1.0f);
}
else
{
// 照準器の色を「水色」(色は自由に変更してください。)
aimImage.color = new Color(0.0f, 1.0f, 1.0f, 1.0f);
}
}
else
{
// 照準器の色を「水色」(色は自由に変更してください。)
aimImage.color = new Color(0.0f, 1.0f, 1.0f, 1.0f);
}
}
}
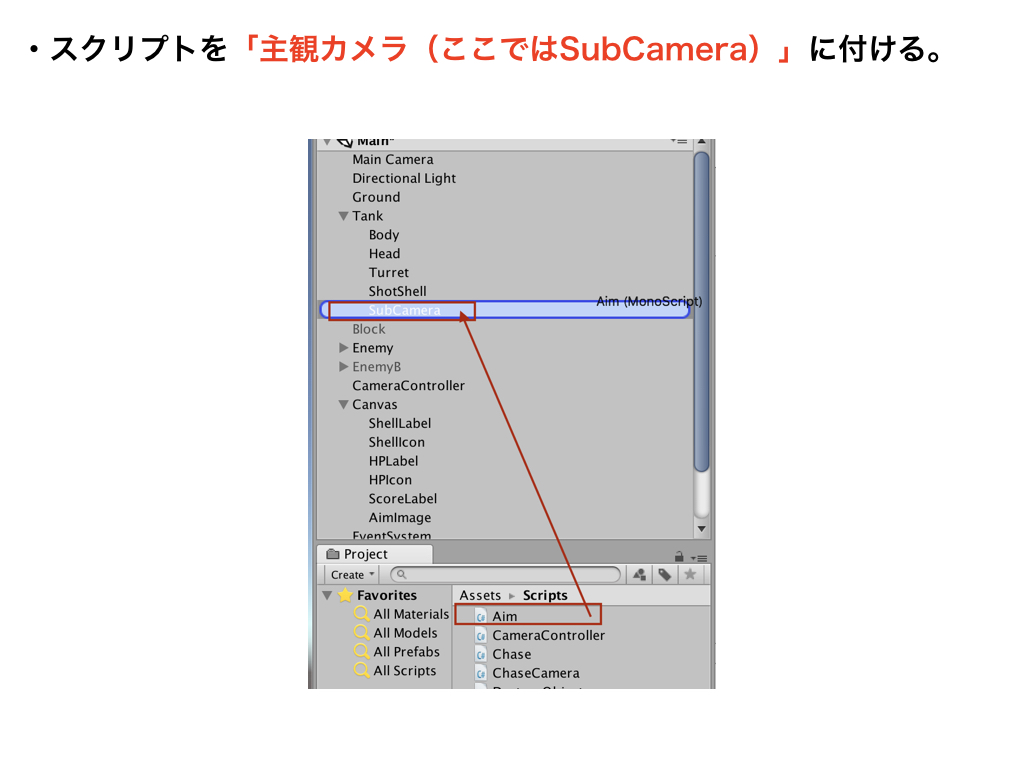
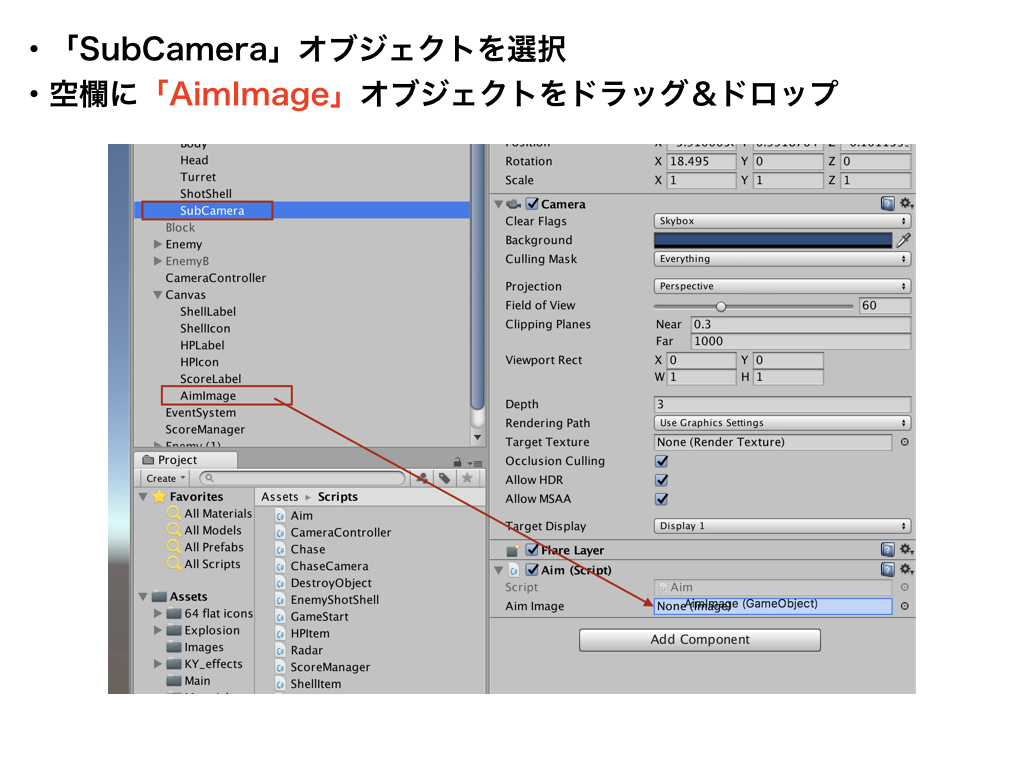
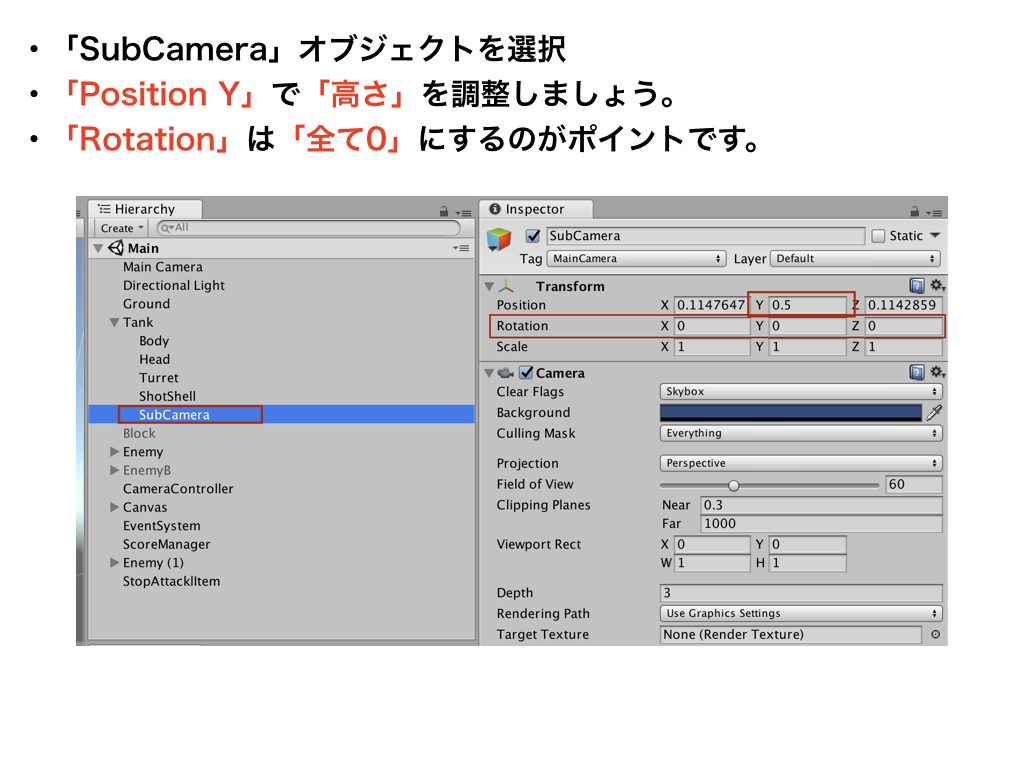
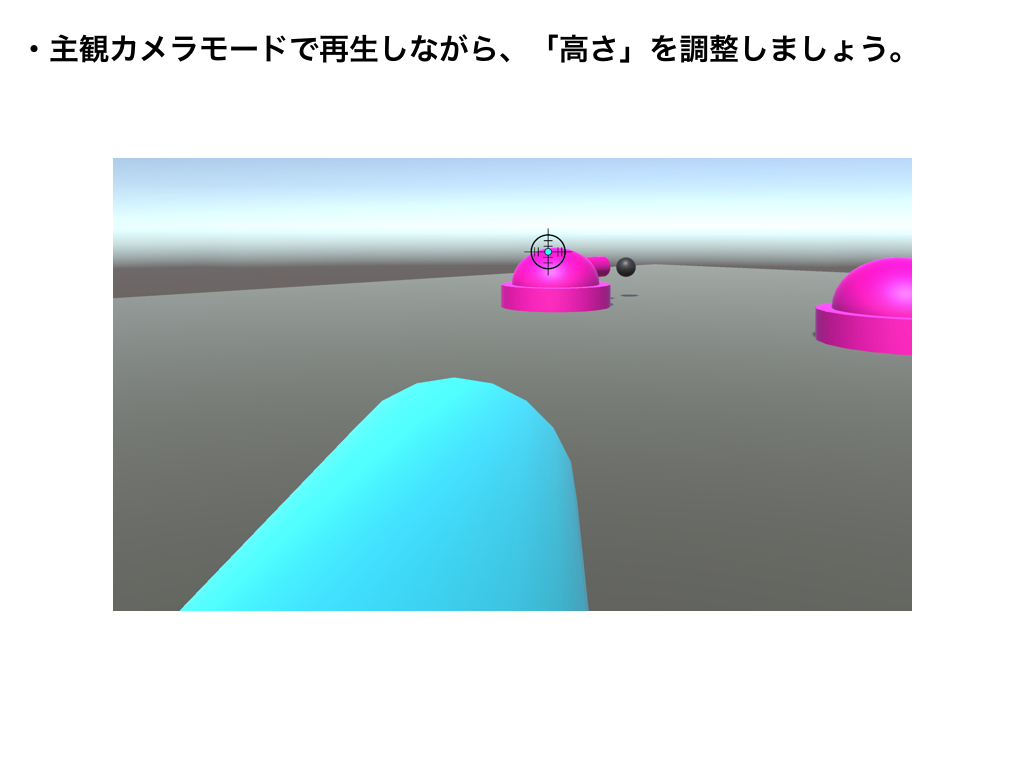
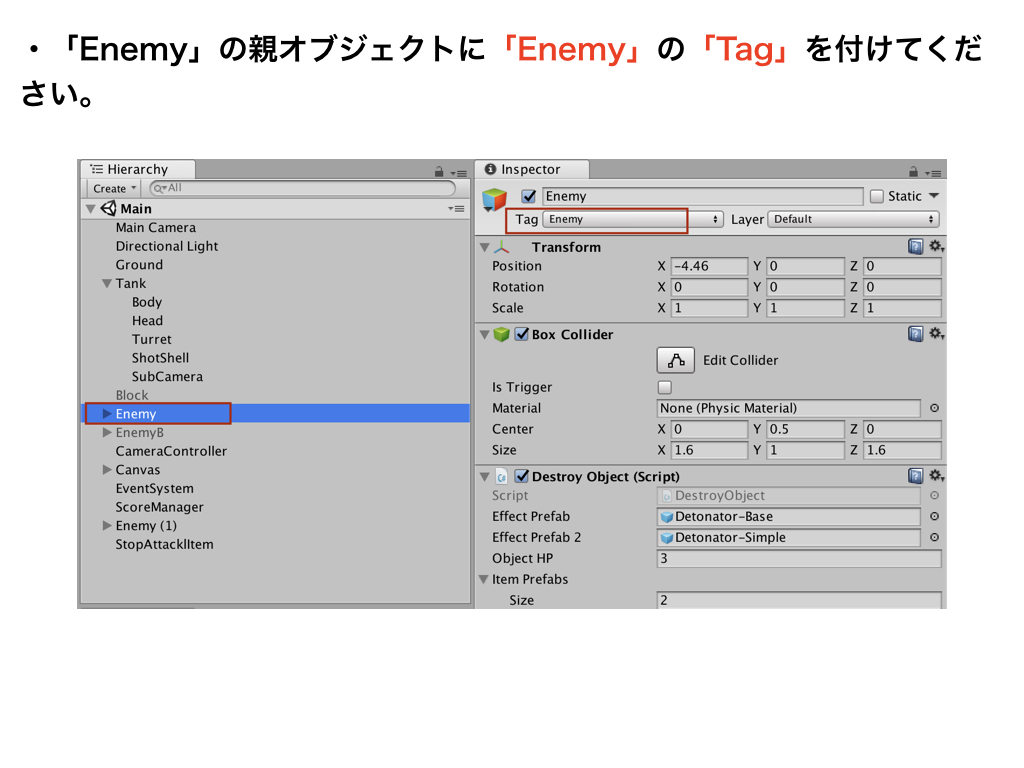
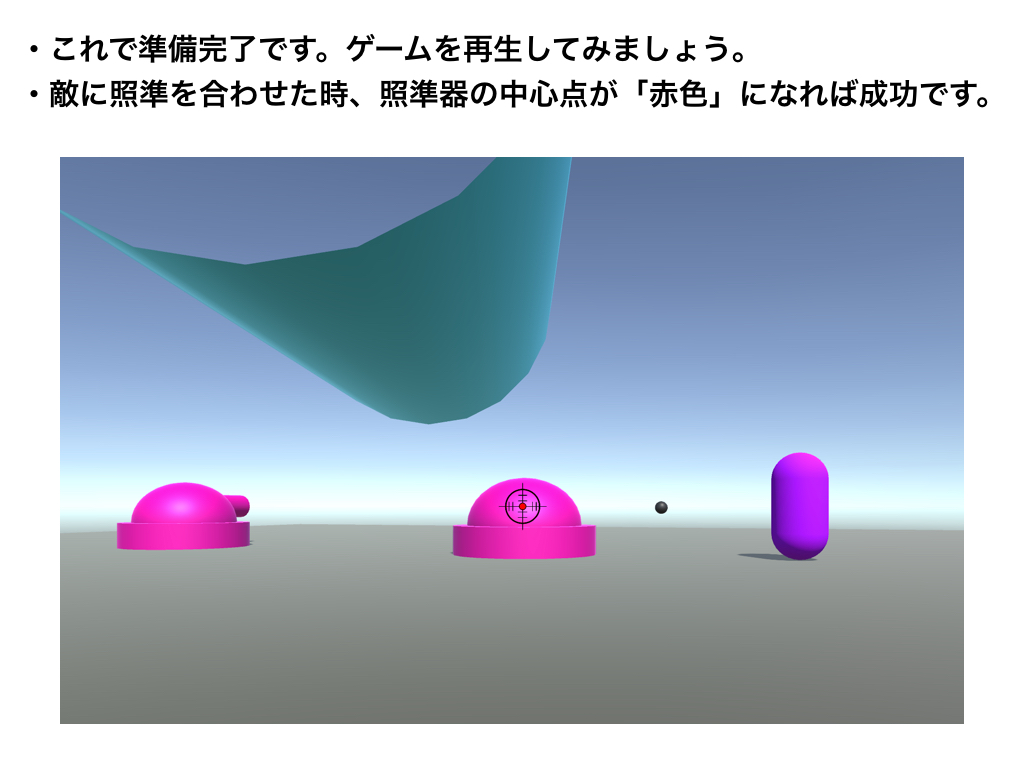
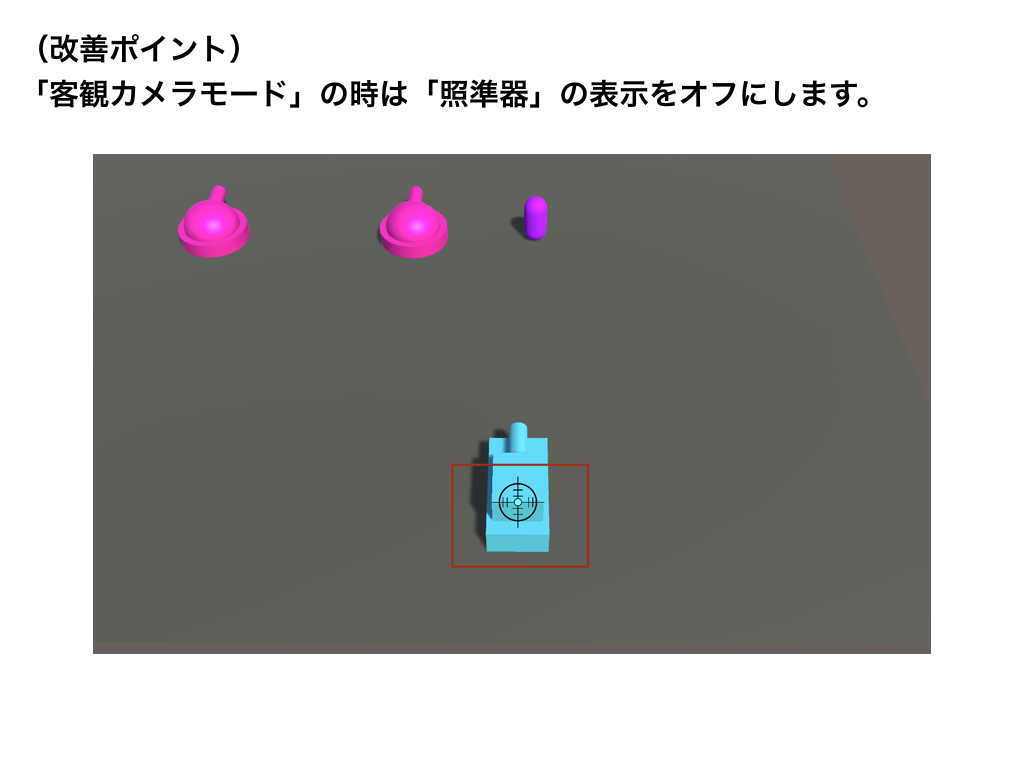
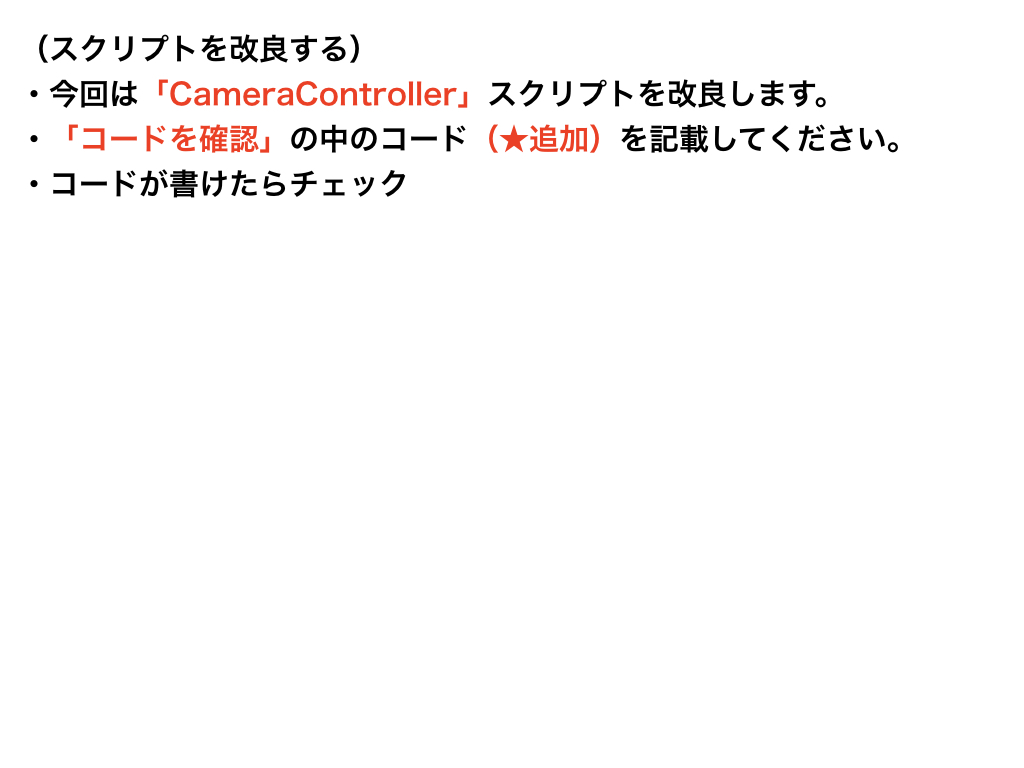
照準器のオンオフを切り替える
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CameraController : MonoBehaviour
{
public Camera mainCamera;
public Camera subCamera;
private bool mainCameraON = true;
// ★追加
public GameObject aimImage;
void Start()
{
mainCamera.enabled = true;
subCamera.enabled = false;
// ★追加
// 客観カメラの場合、照準器をオフにする。
aimImage.SetActive(false);
}
void Update()
{
if (Input.GetKeyDown(KeyCode.C) && mainCameraON == true)
{
mainCamera.enabled = false;
subCamera.enabled = true;
mainCameraON = false;
// ★追加
// 主観カメラの場合、照準器をオンにする。
aimImage.SetActive(true);
}
else if (Input.GetKeyDown(KeyCode.C) && mainCameraON == false)
{
mainCamera.enabled = true;
subCamera.enabled = false;
mainCameraON = true;
// ★追加
// 客観カメラの場合、照準器をオフにする。
aimImage.SetActive(false);
}
}
}
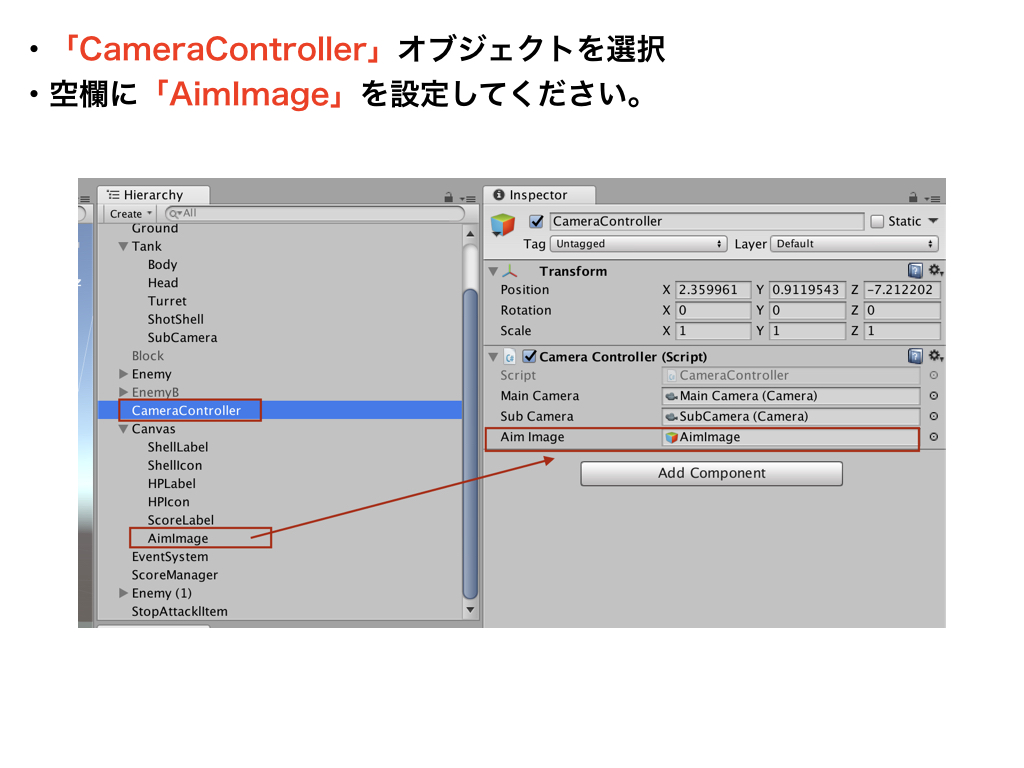
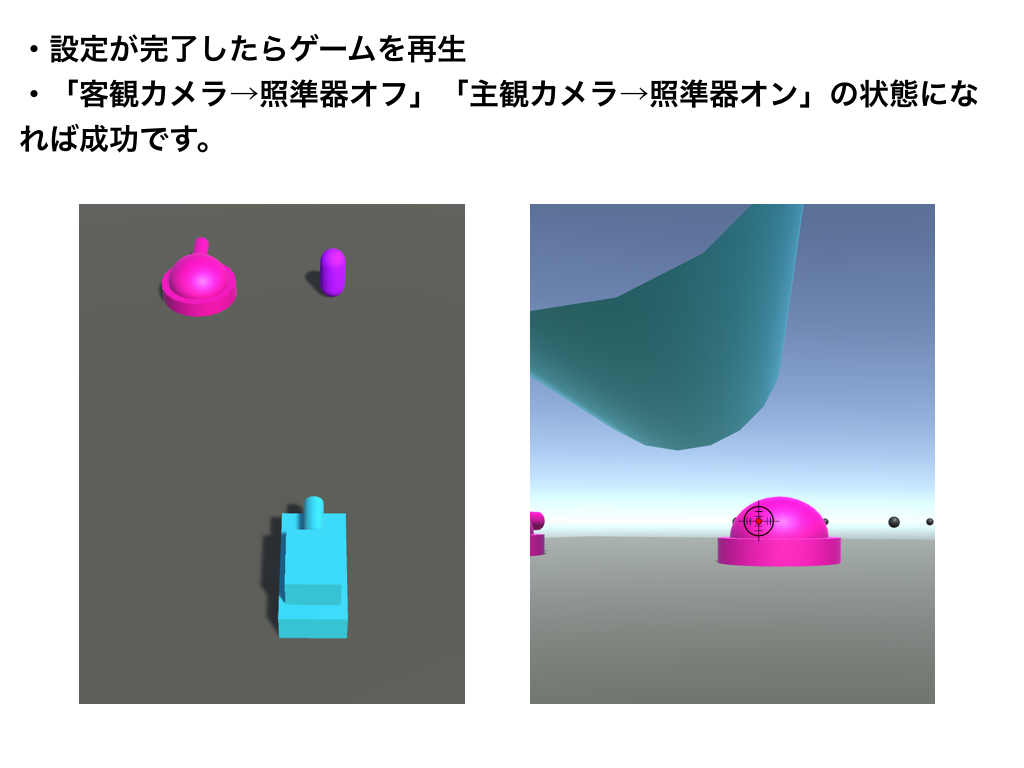
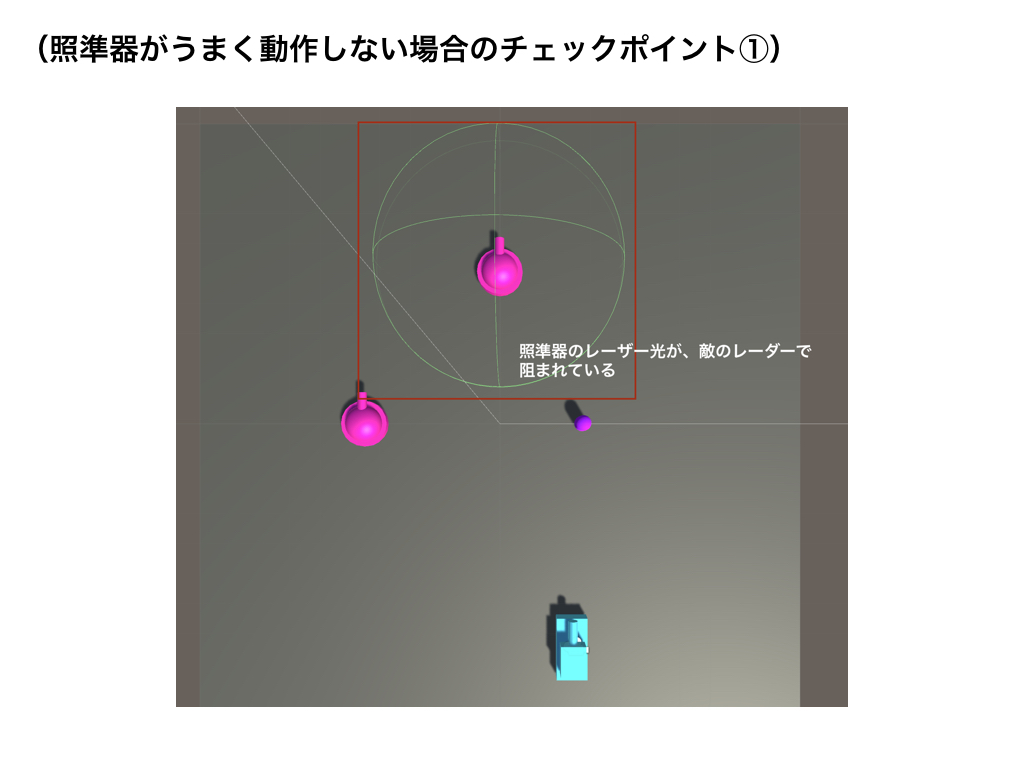
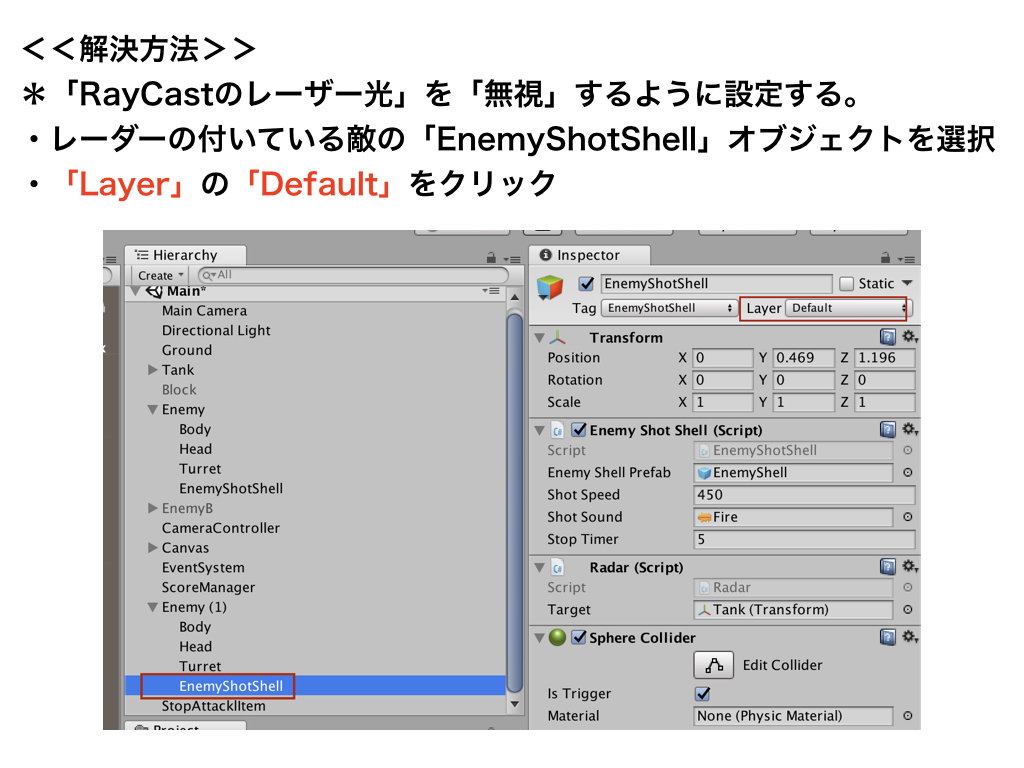
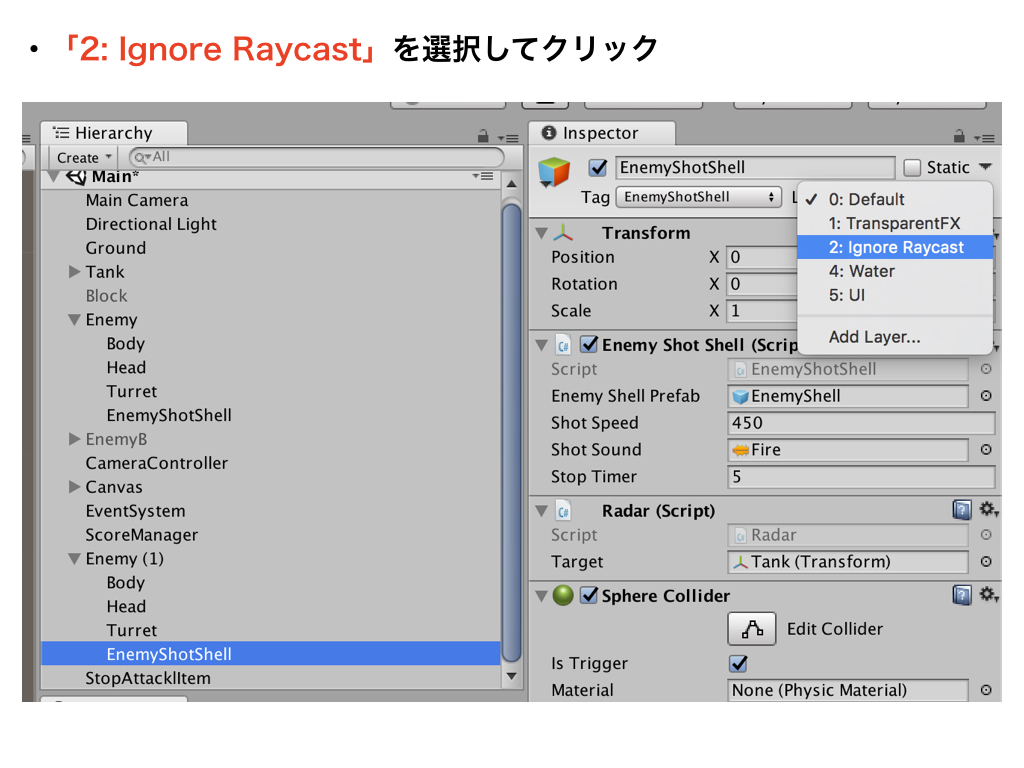
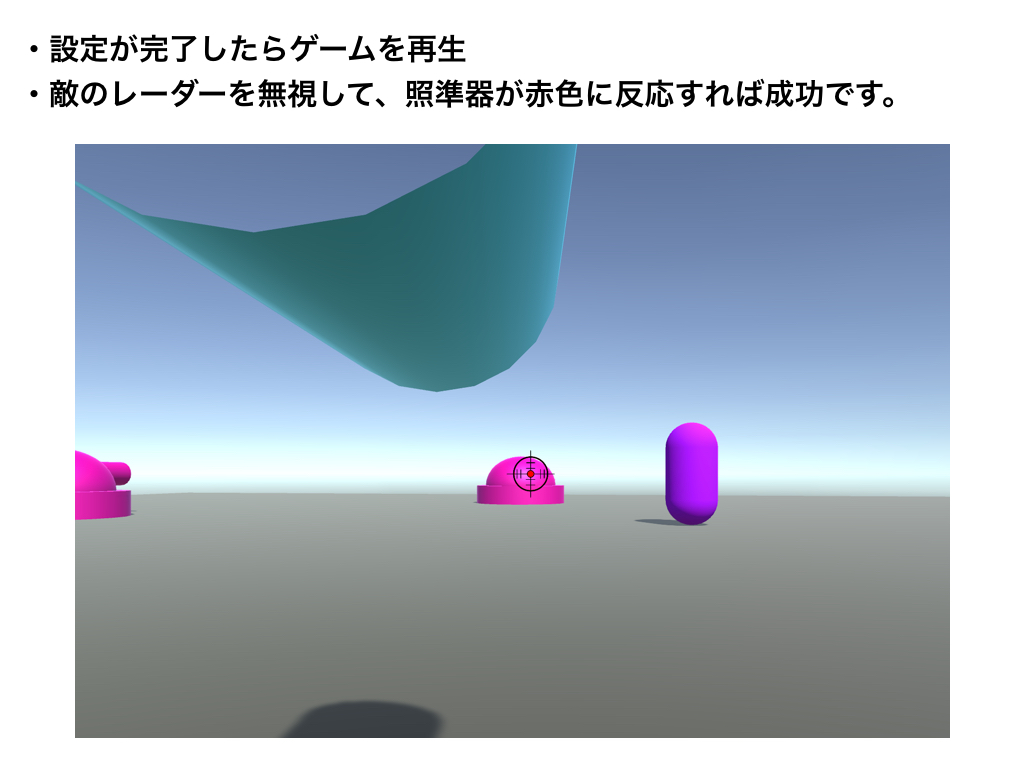
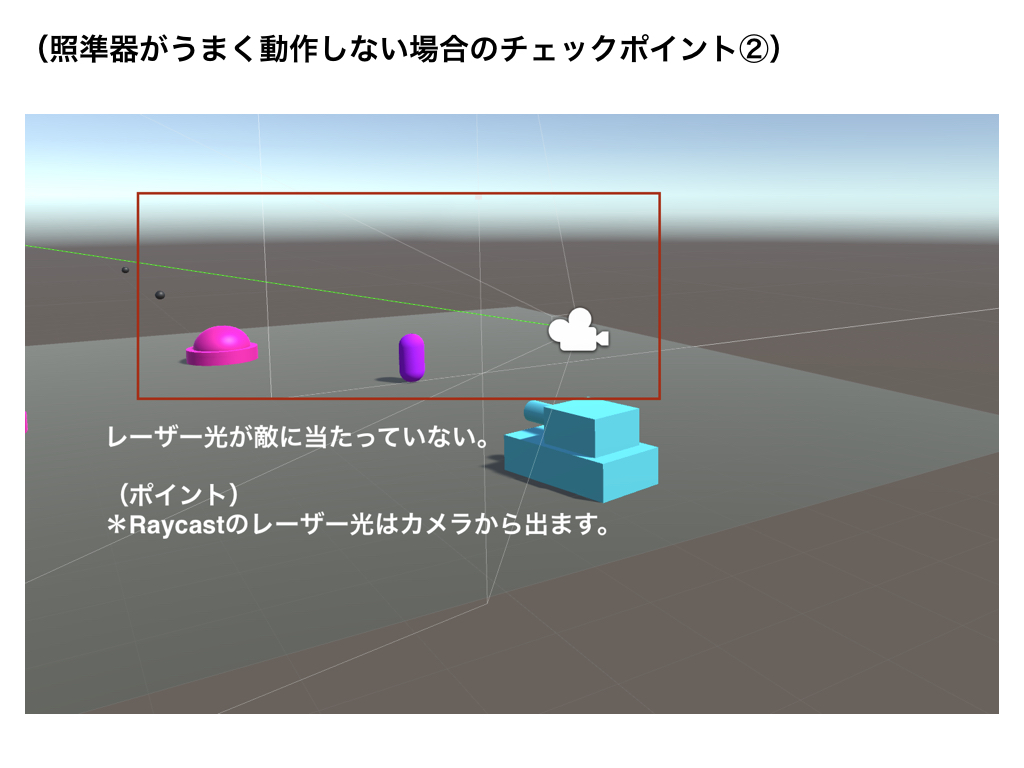
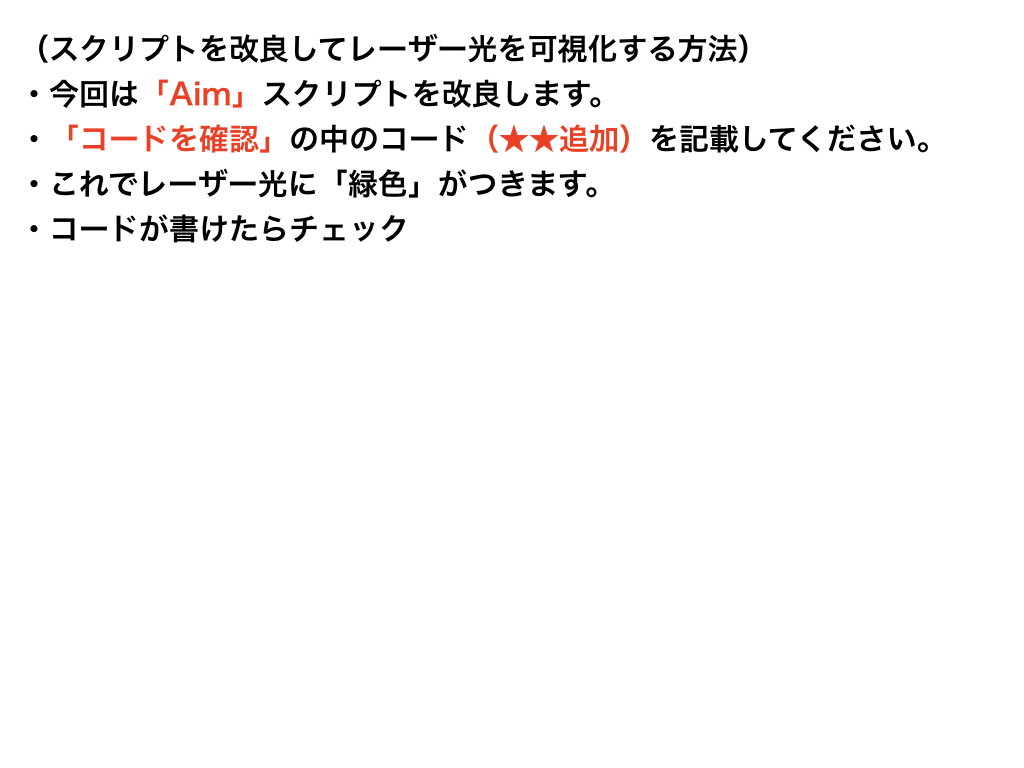
レーザー光を可視化する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class Aim : MonoBehaviour
{
public Image aimImage;
void Update()
{
Ray ray = new Ray(transform.position, transform.forward);
// ★★追加(レーザー光を可視化することができる)
Debug.DrawRay(transform.position, transform.forward * 30, Color.green);
RaycastHit hit;
if (Physics.Raycast(ray, out hit, 60))
{
string hitName = hit.transform.gameObject.tag;
if (hitName == "Enemy")
{
aimImage.color = new Color(1.0f, 0.0f, 0.0f, 1.0f);
}
else
{
aimImage.color = new Color(0.0f, 1.0f, 1.0f, 1.0f);
}
}
else
{
aimImage.color = new Color(0.0f, 1.0f, 1.0f, 1.0f);
}
}
}
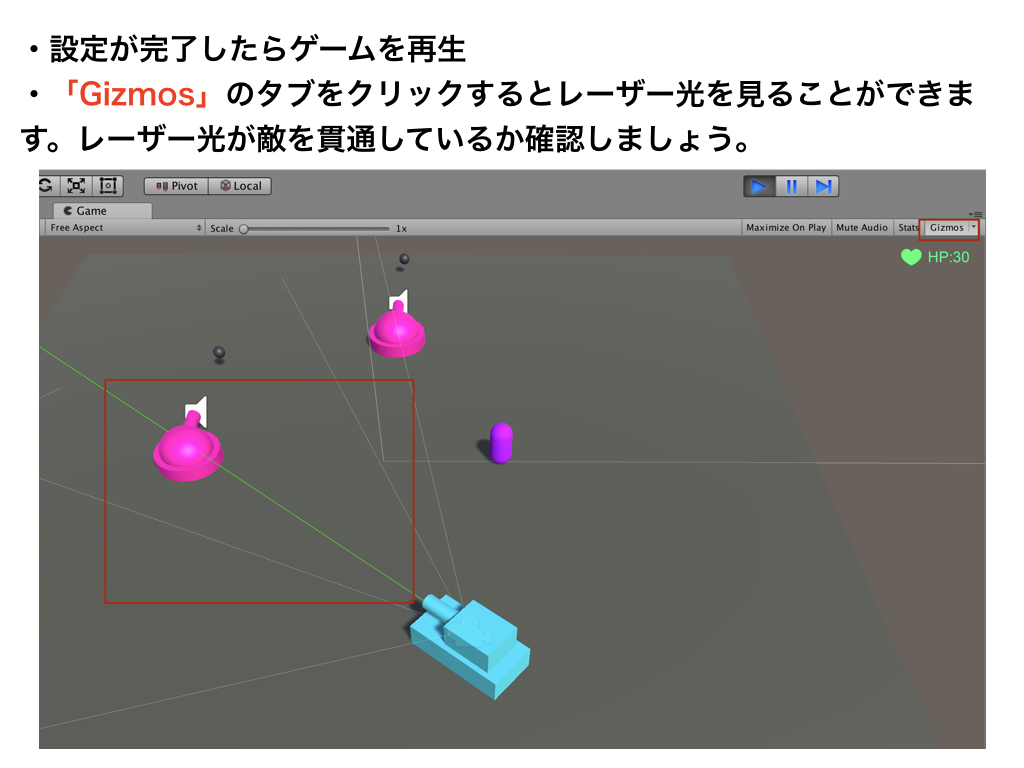
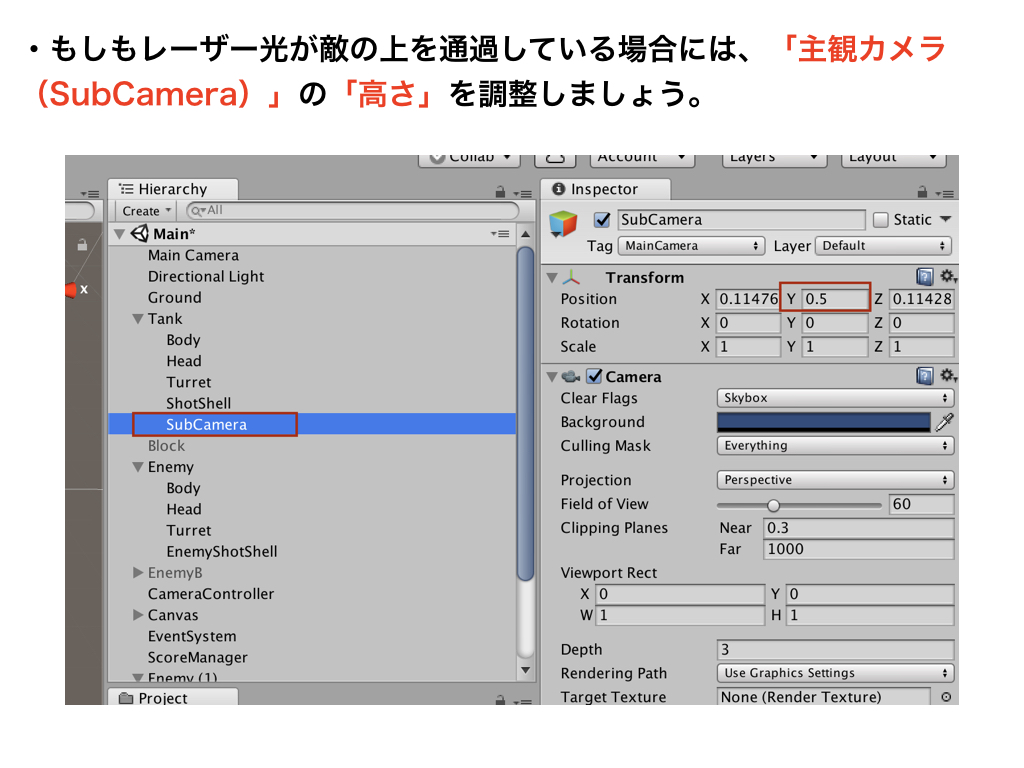
BattleTank(基礎/全31回)
他のコースを見る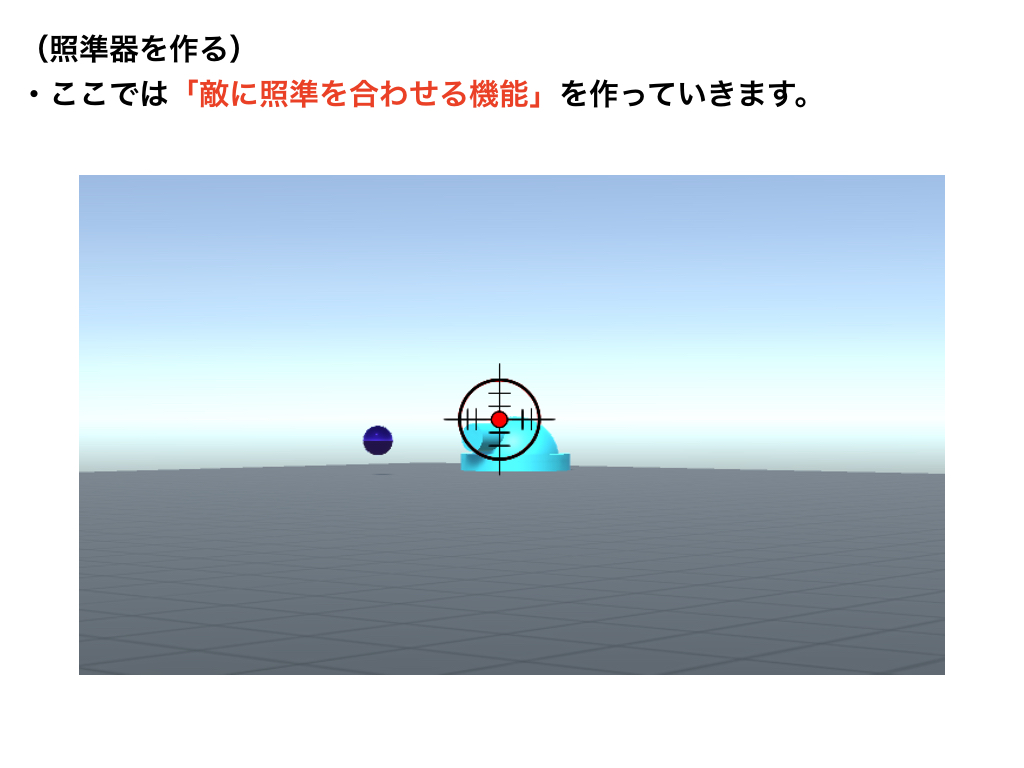
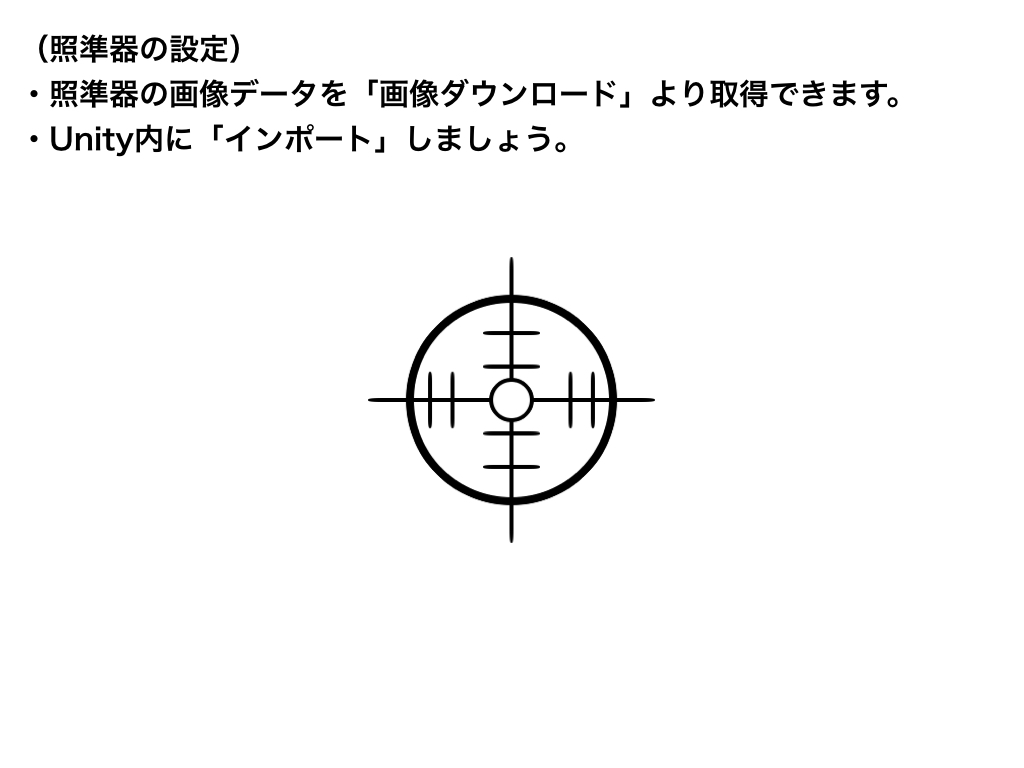
https://mono-pro.net/unity-codegenius-battletank-aim/aiming.zip画像ダウンロード
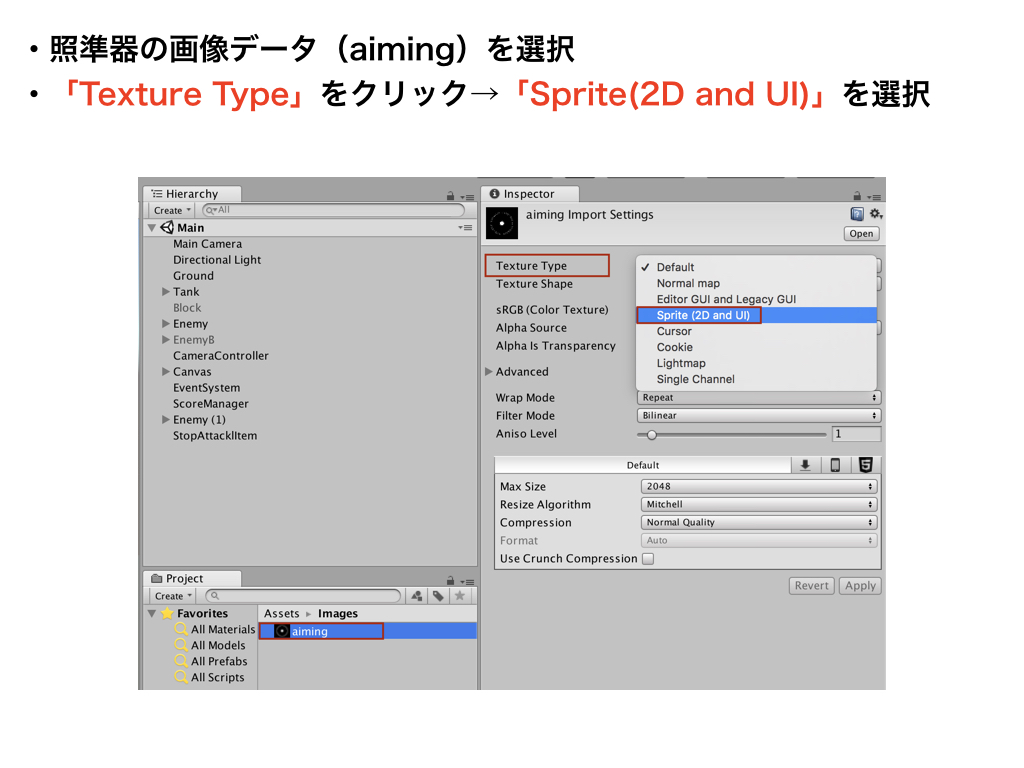
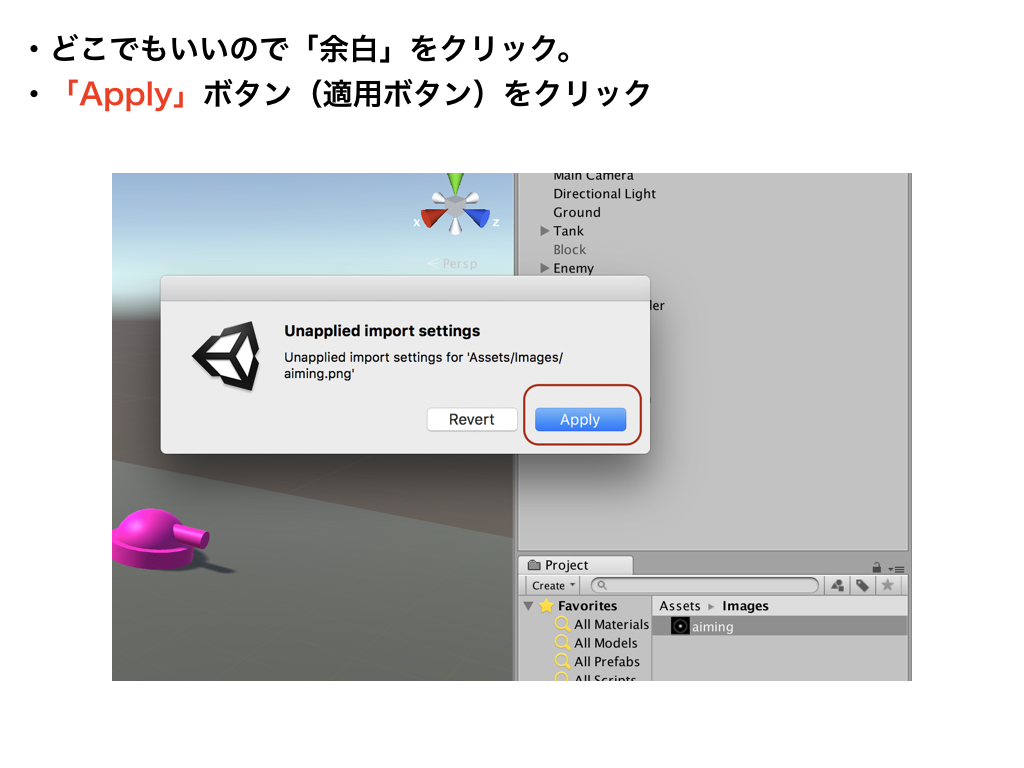
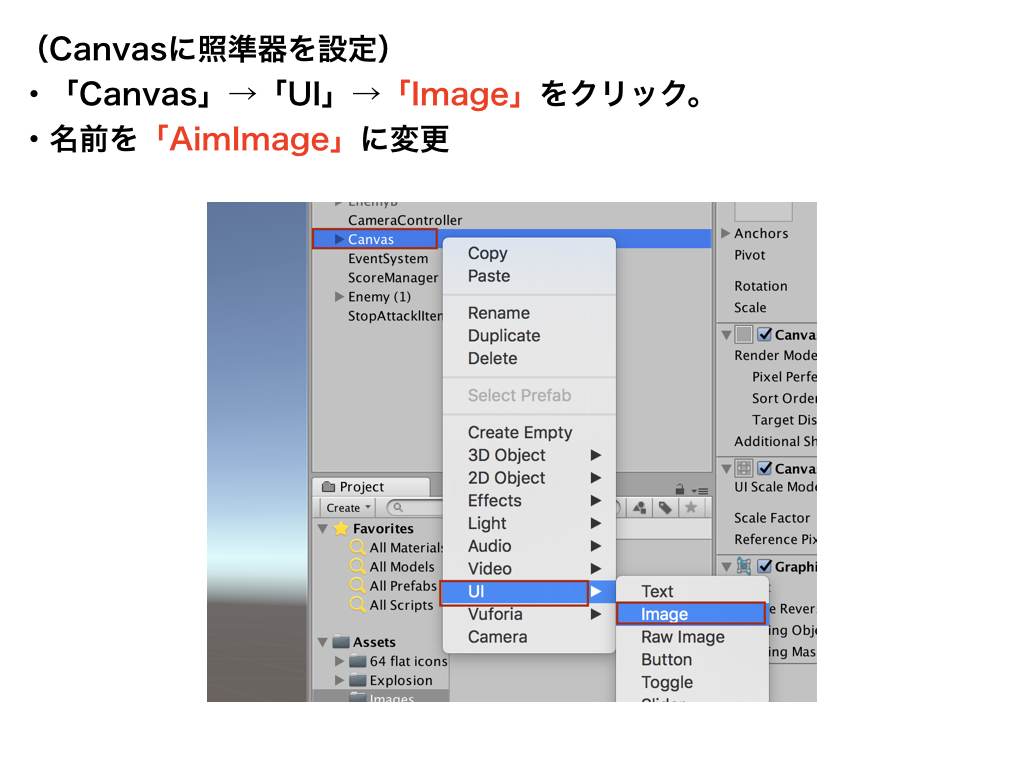
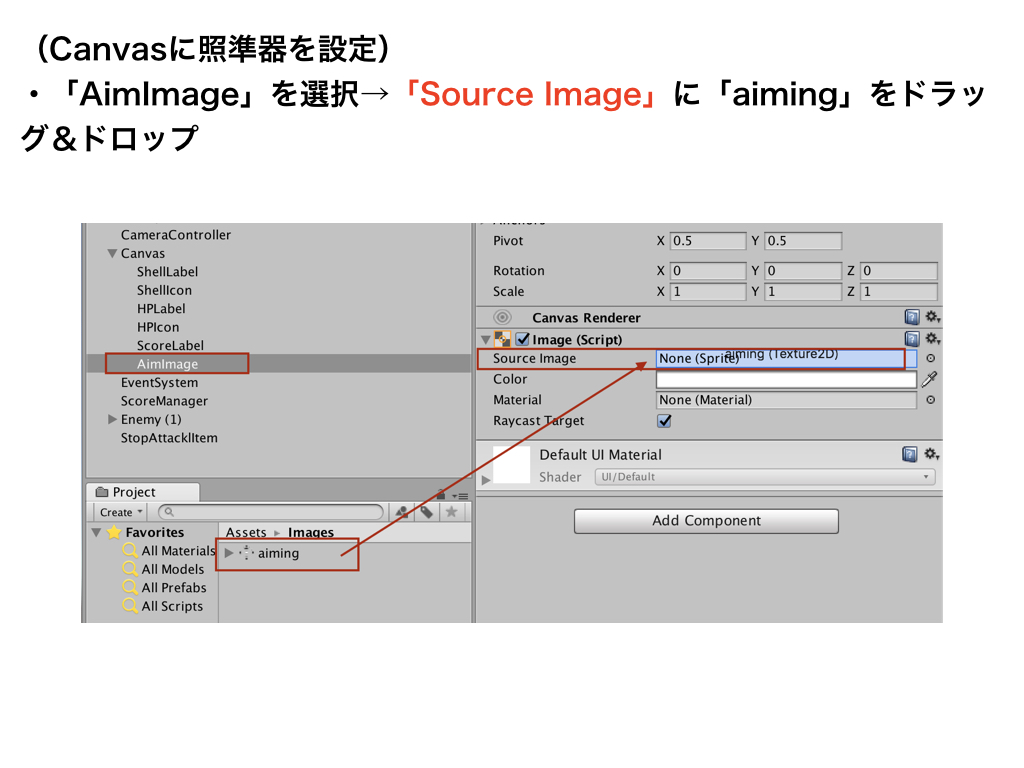
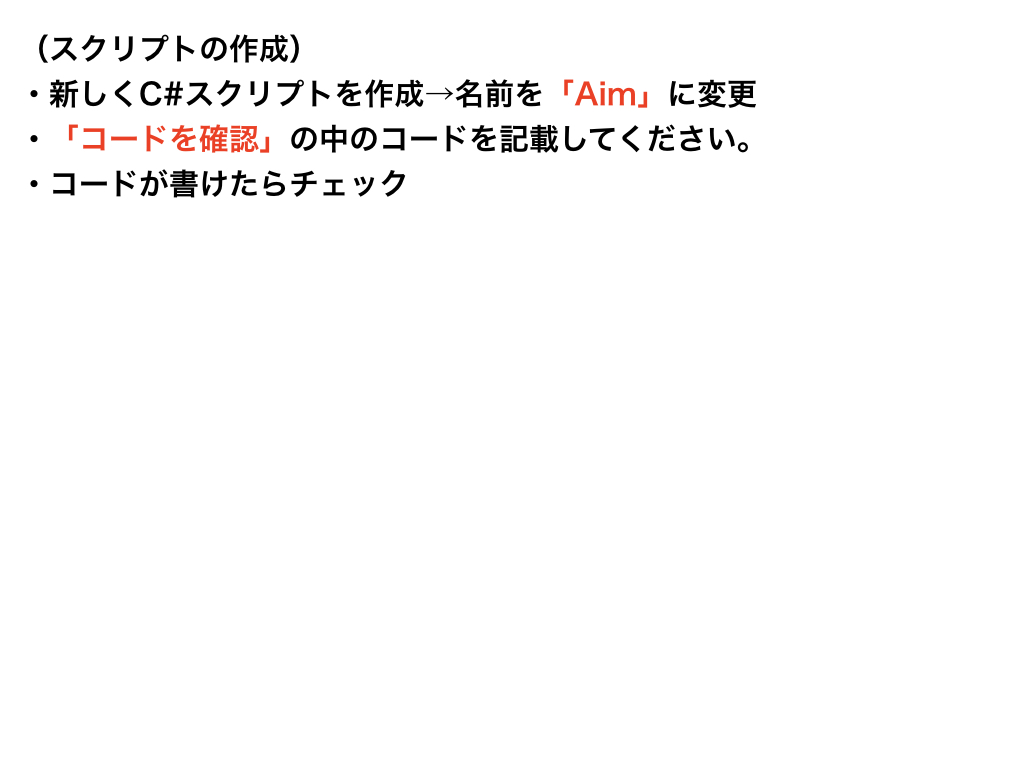
Rayで照準器を作る
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// 追加しましょう(ポイント)
using UnityEngine.UI;
public class Aim : MonoBehaviour
{
public Image aimImage;
void Update()
{
// レーザー(ray)を飛ばす「起点」と「方向」
Ray ray = new Ray(transform.position, transform.forward);
// rayのあたり判定の情報を入れる箱を作る。
RaycastHit hit;
if (Physics.Raycast(ray, out hit, 60))
{
string hitName = hit.transform.gameObject.tag;
if (hitName == "Enemy")
{
// 照準器の色を「赤」に変える(色は自由に変更してください。)
aimImage.color = new Color(1.0f, 0.0f, 0.0f, 1.0f);
}
else
{
// 照準器の色を「水色」(色は自由に変更してください。)
aimImage.color = new Color(0.0f, 1.0f, 1.0f, 1.0f);
}
}
else
{
// 照準器の色を「水色」(色は自由に変更してください。)
aimImage.color = new Color(0.0f, 1.0f, 1.0f, 1.0f);
}
}
}
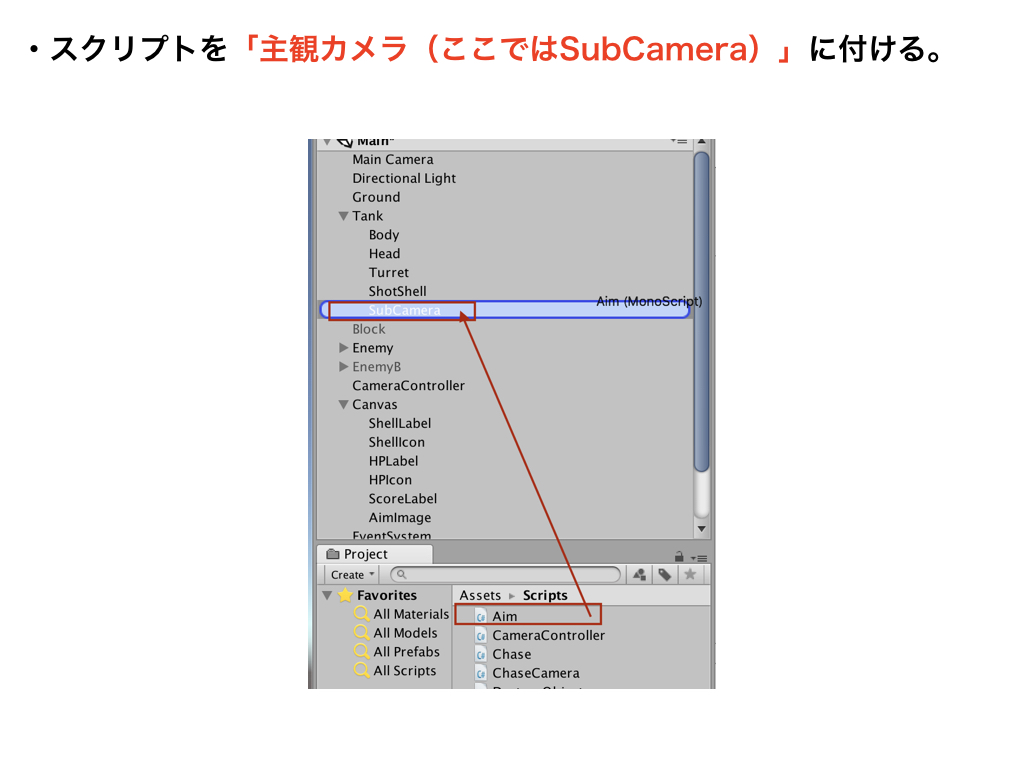
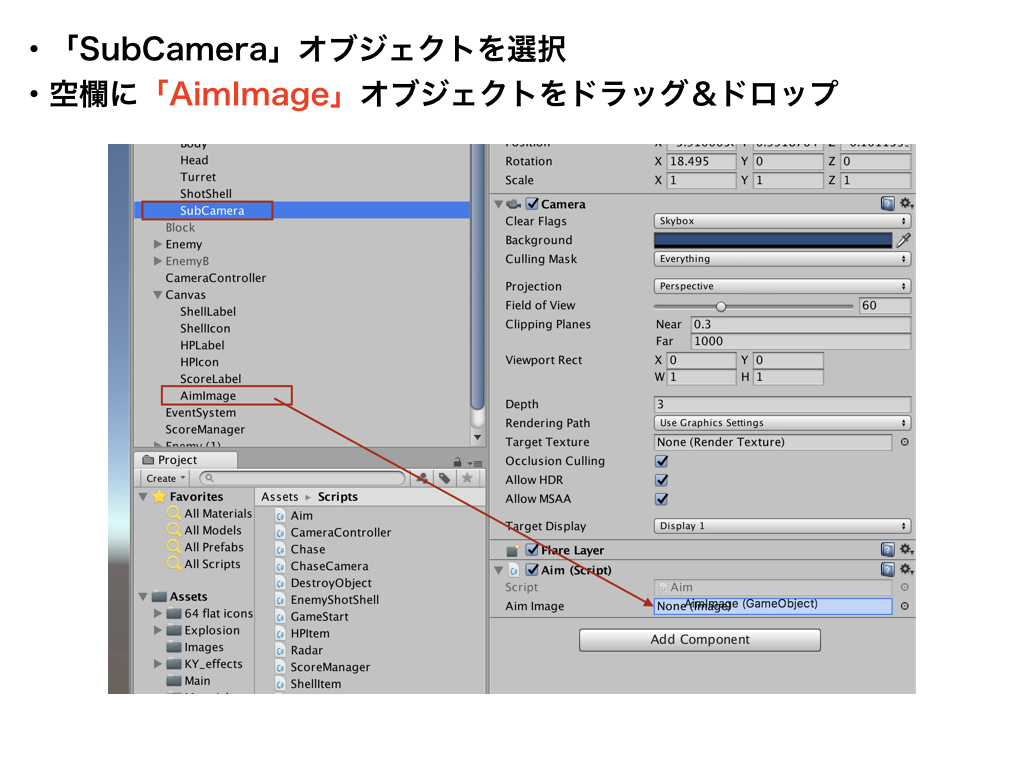
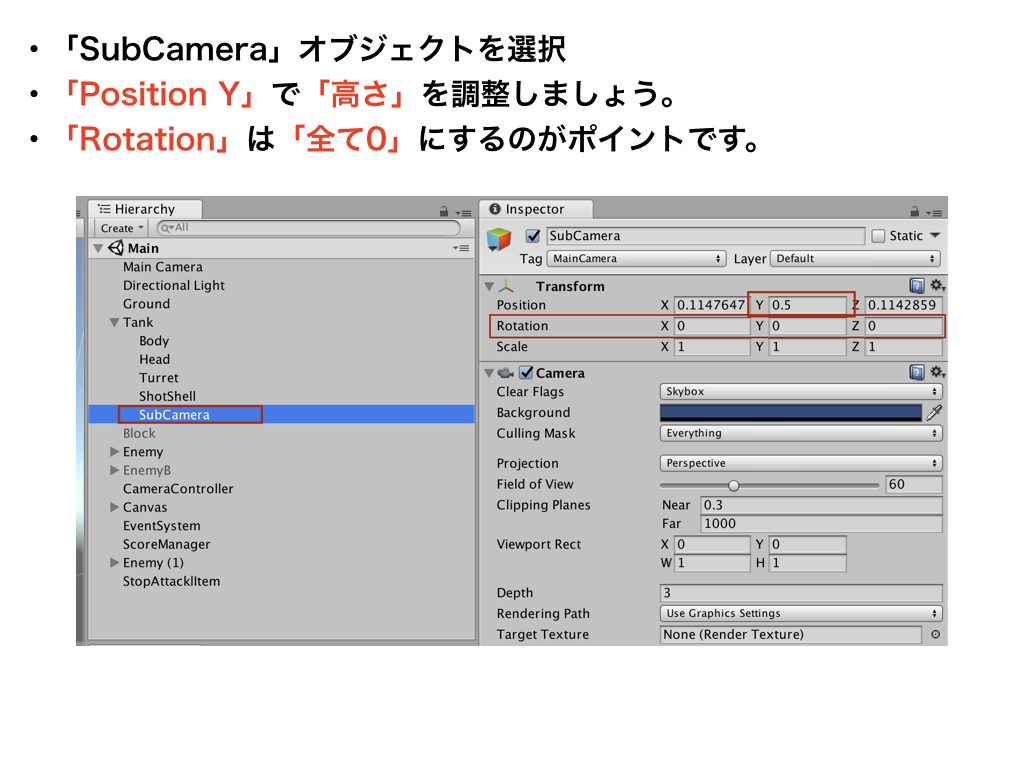
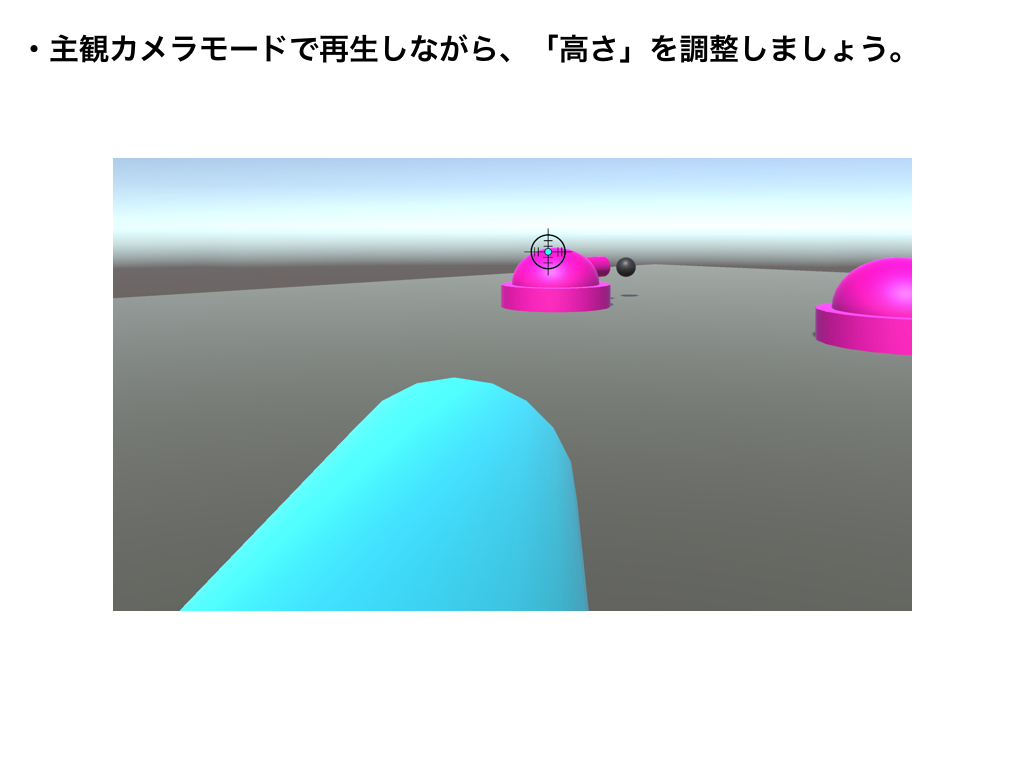
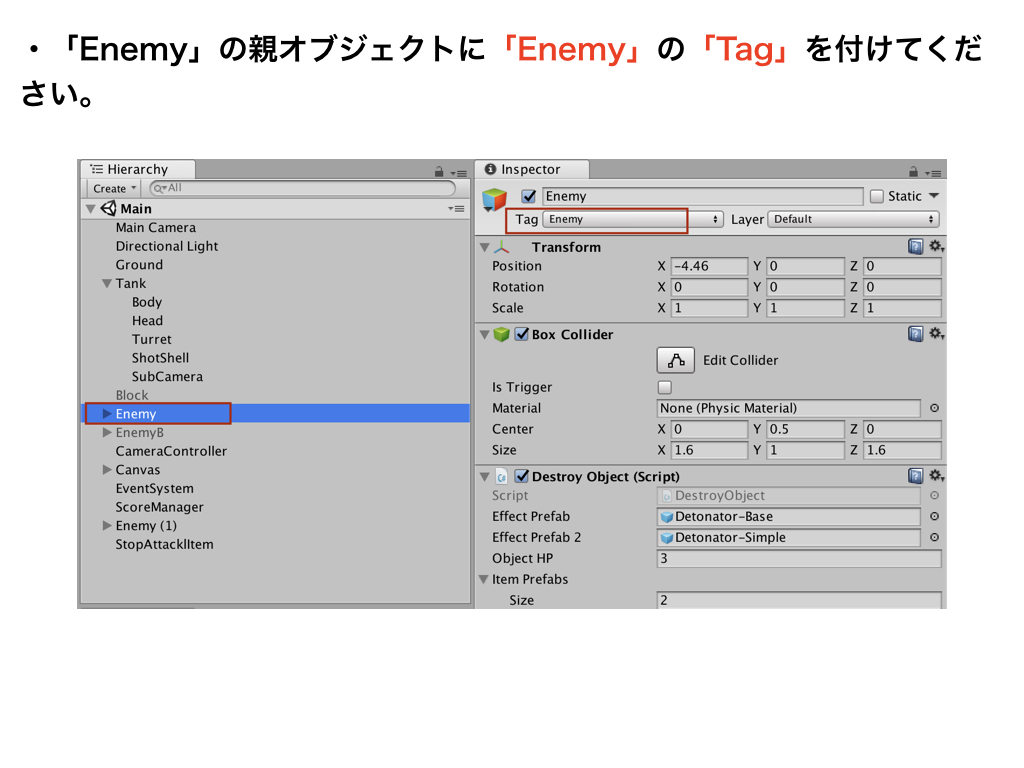
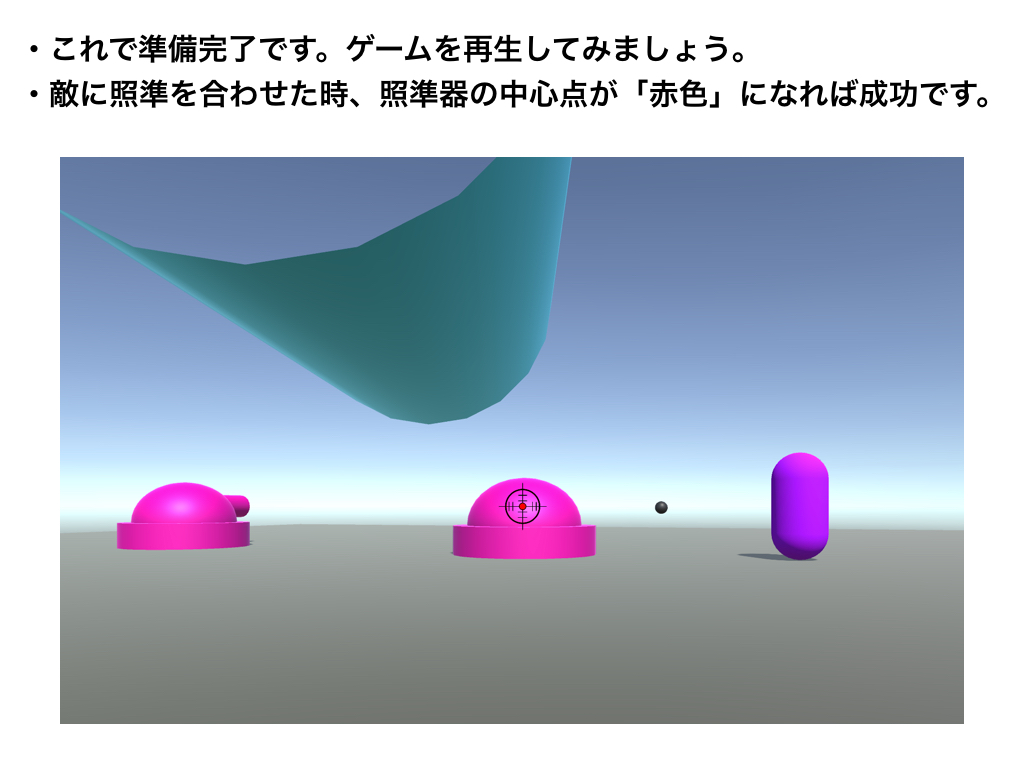
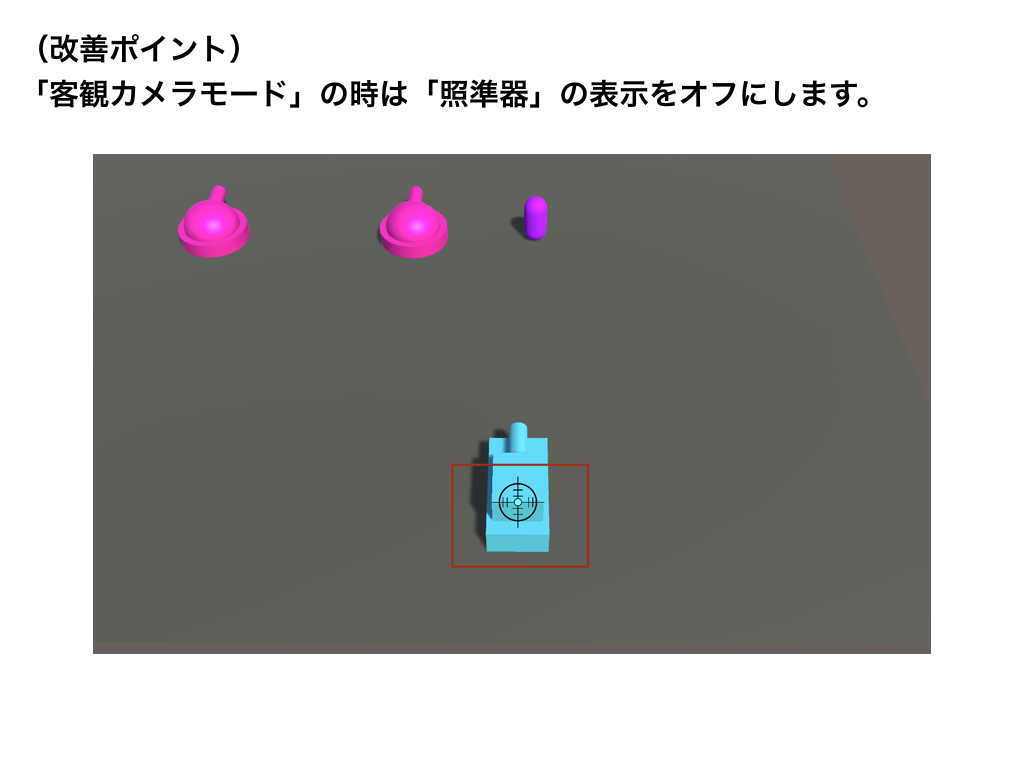
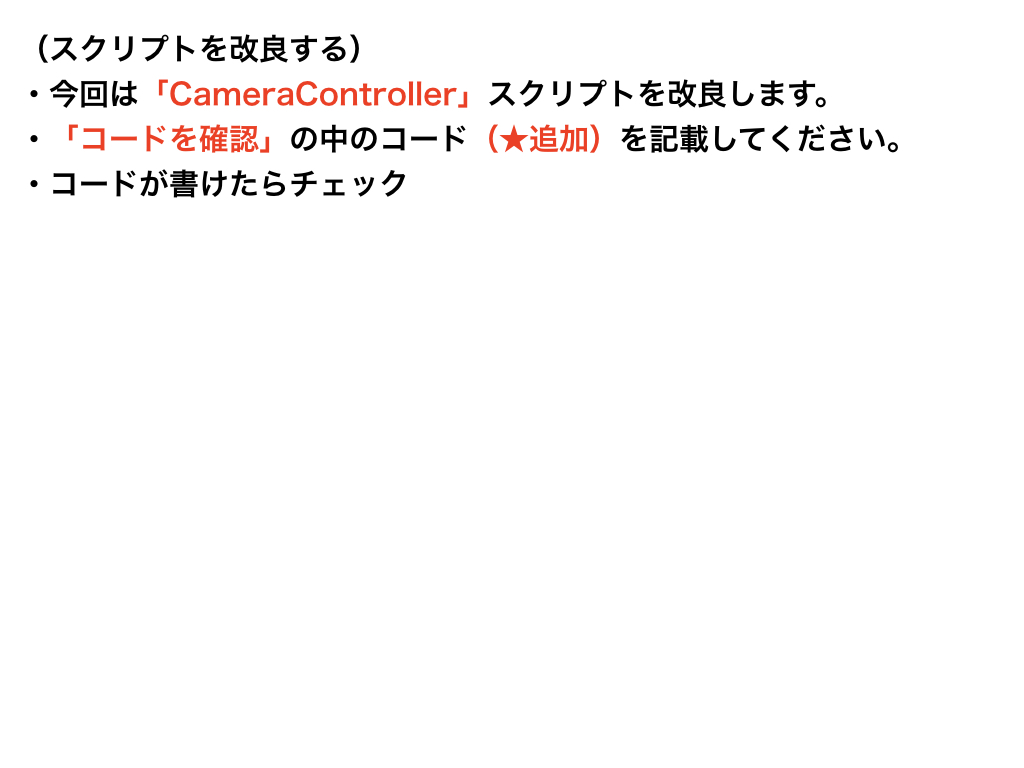
照準器のオンオフを切り替える
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CameraController : MonoBehaviour
{
public Camera mainCamera;
public Camera subCamera;
private bool mainCameraON = true;
// ★追加
public GameObject aimImage;
void Start()
{
mainCamera.enabled = true;
subCamera.enabled = false;
// ★追加
// 客観カメラの場合、照準器をオフにする。
aimImage.SetActive(false);
}
void Update()
{
if (Input.GetKeyDown(KeyCode.C) && mainCameraON == true)
{
mainCamera.enabled = false;
subCamera.enabled = true;
mainCameraON = false;
// ★追加
// 主観カメラの場合、照準器をオンにする。
aimImage.SetActive(true);
}
else if (Input.GetKeyDown(KeyCode.C) && mainCameraON == false)
{
mainCamera.enabled = true;
subCamera.enabled = false;
mainCameraON = true;
// ★追加
// 客観カメラの場合、照準器をオフにする。
aimImage.SetActive(false);
}
}
}
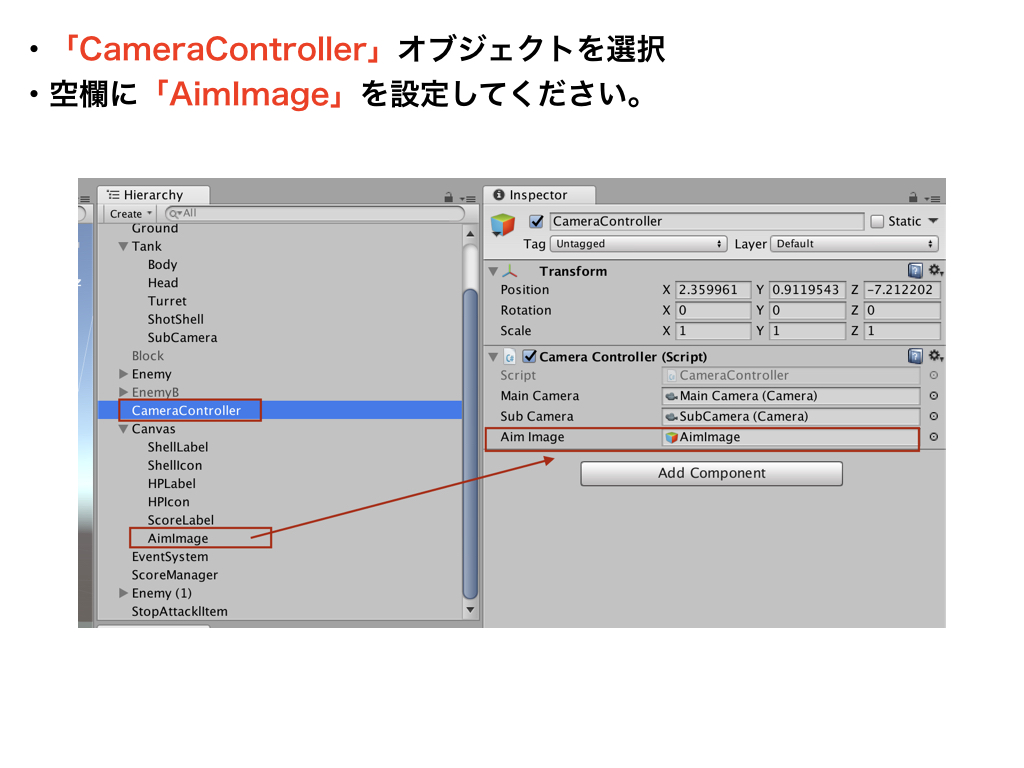
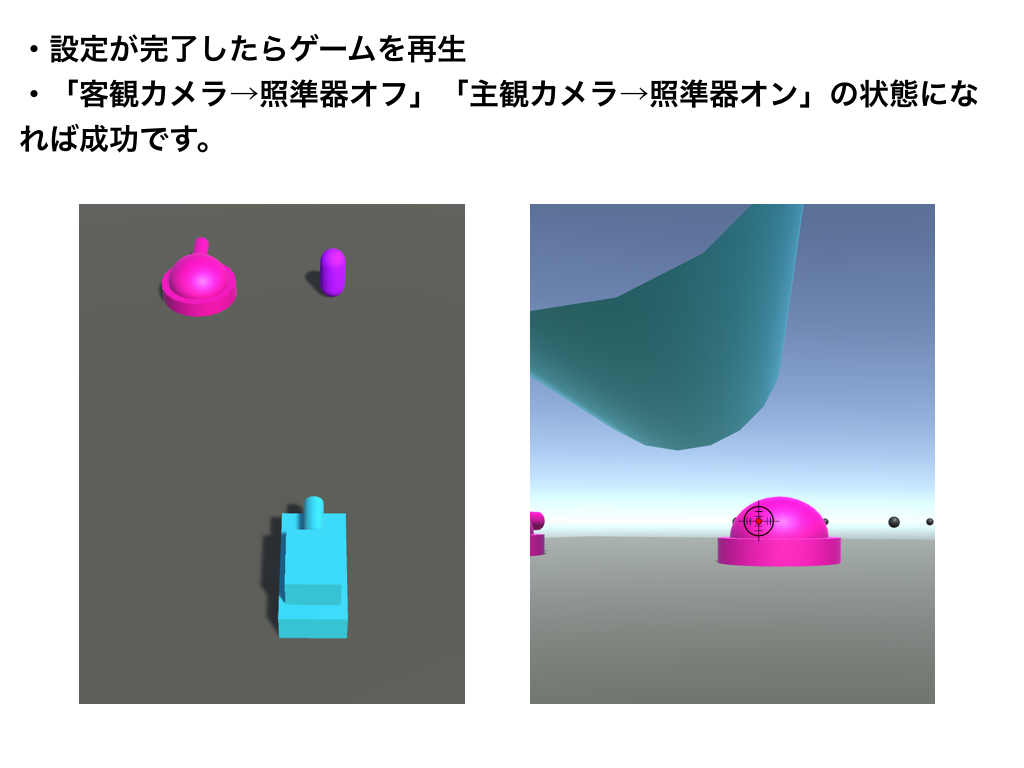
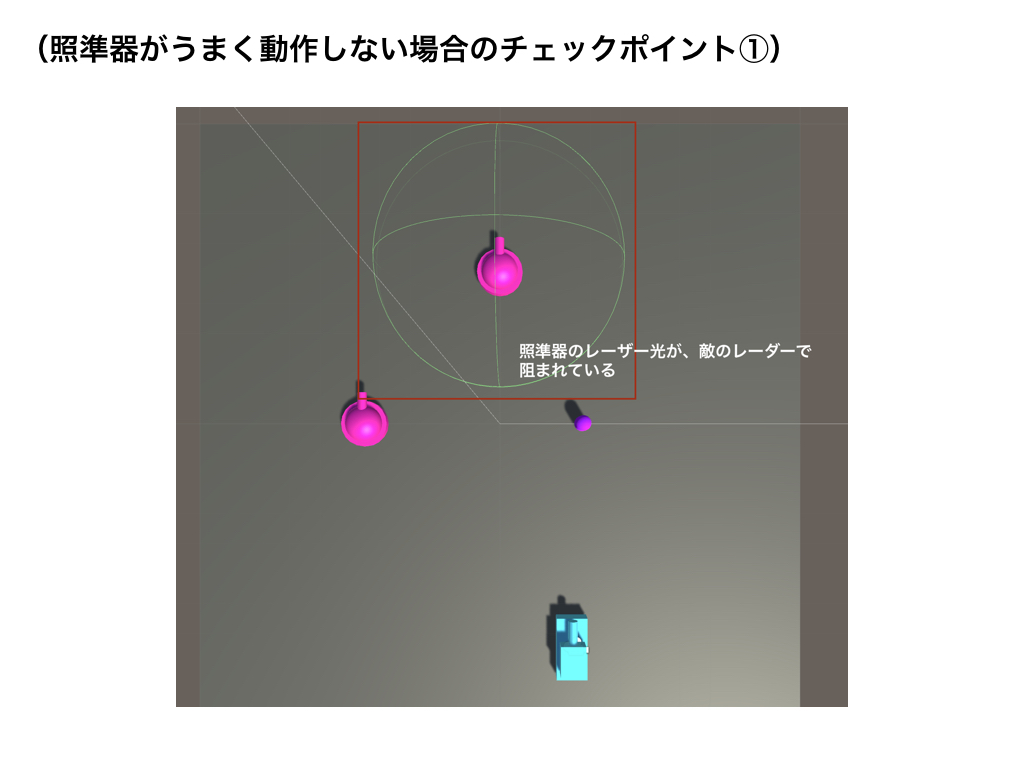
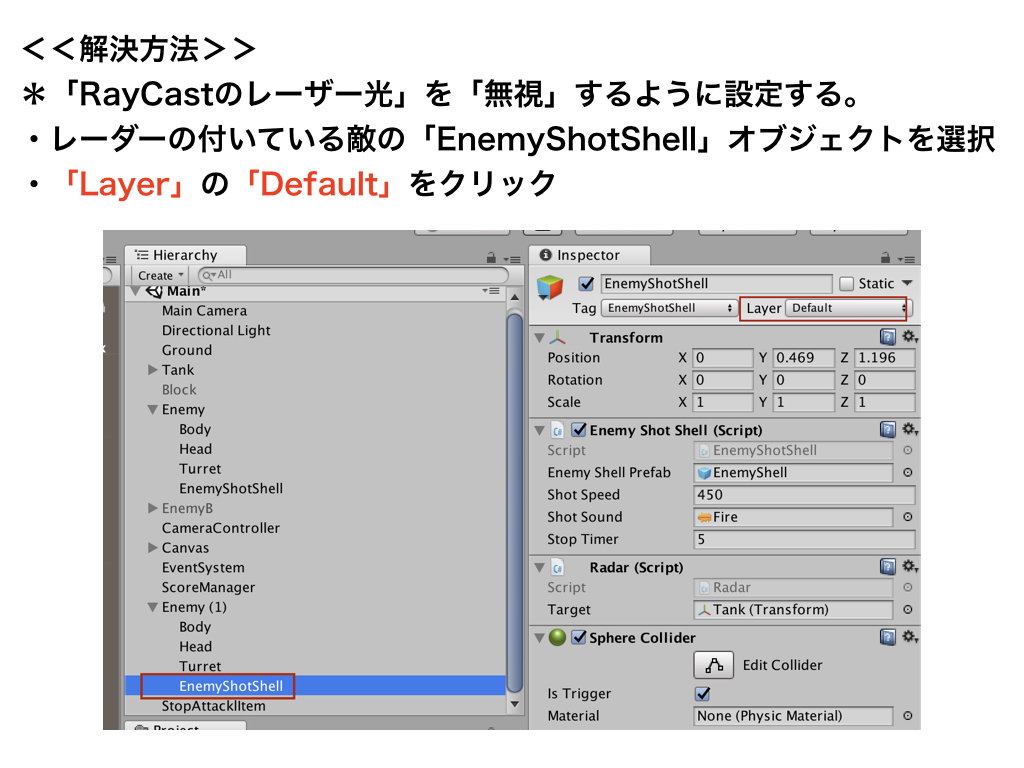
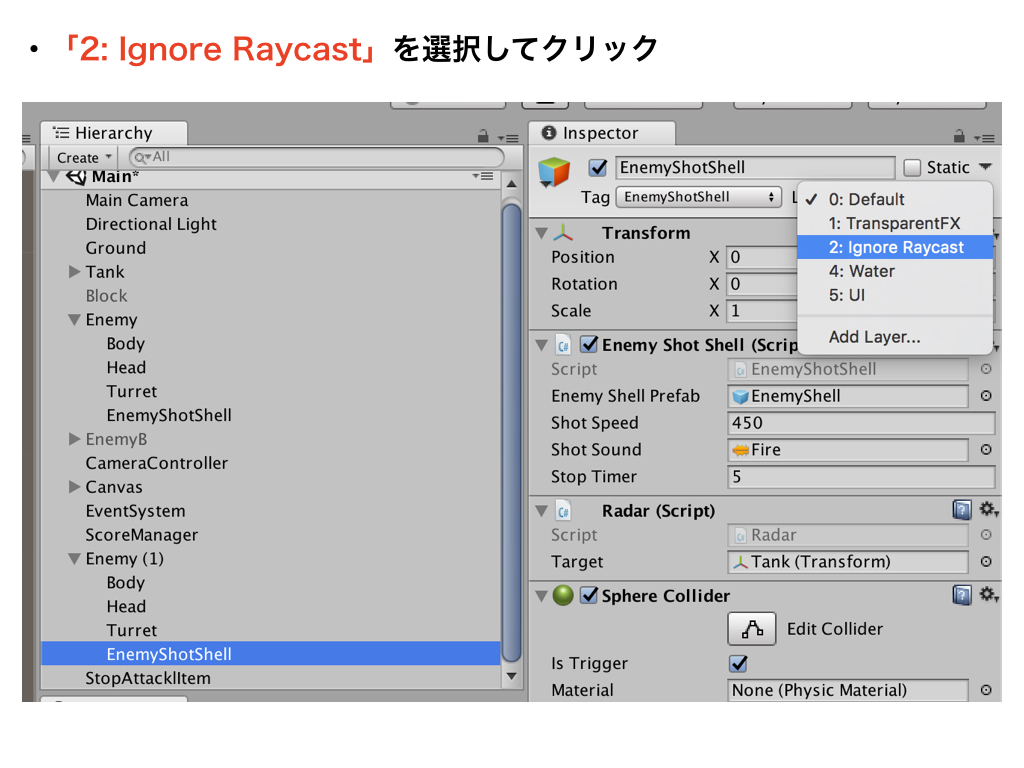
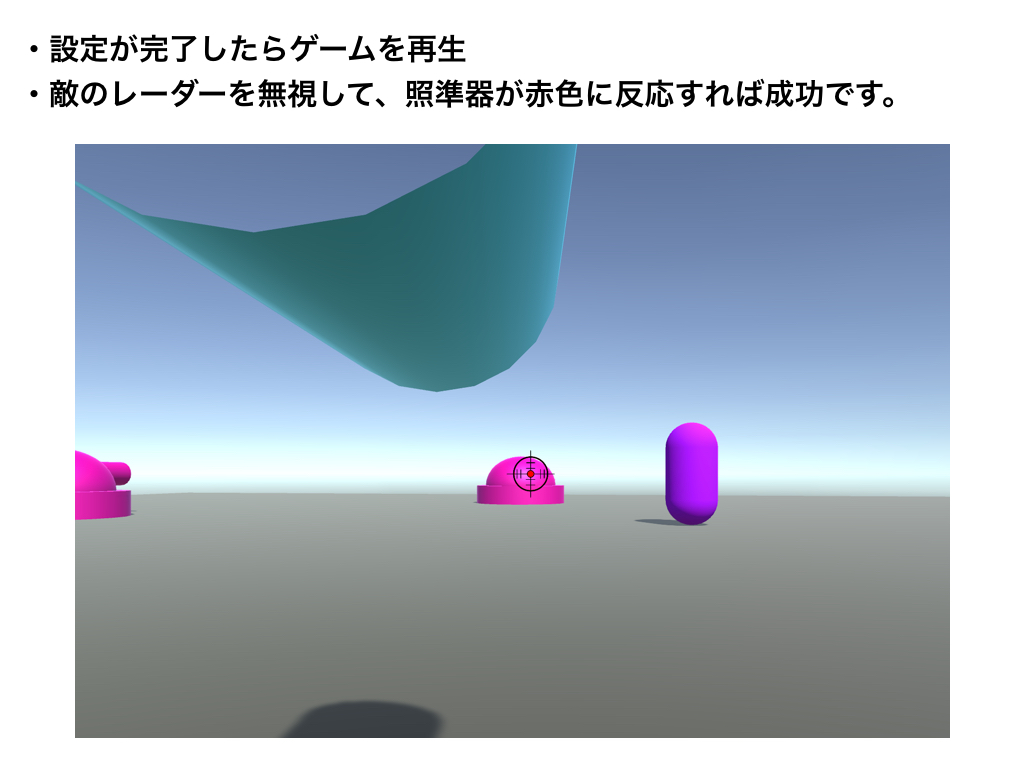
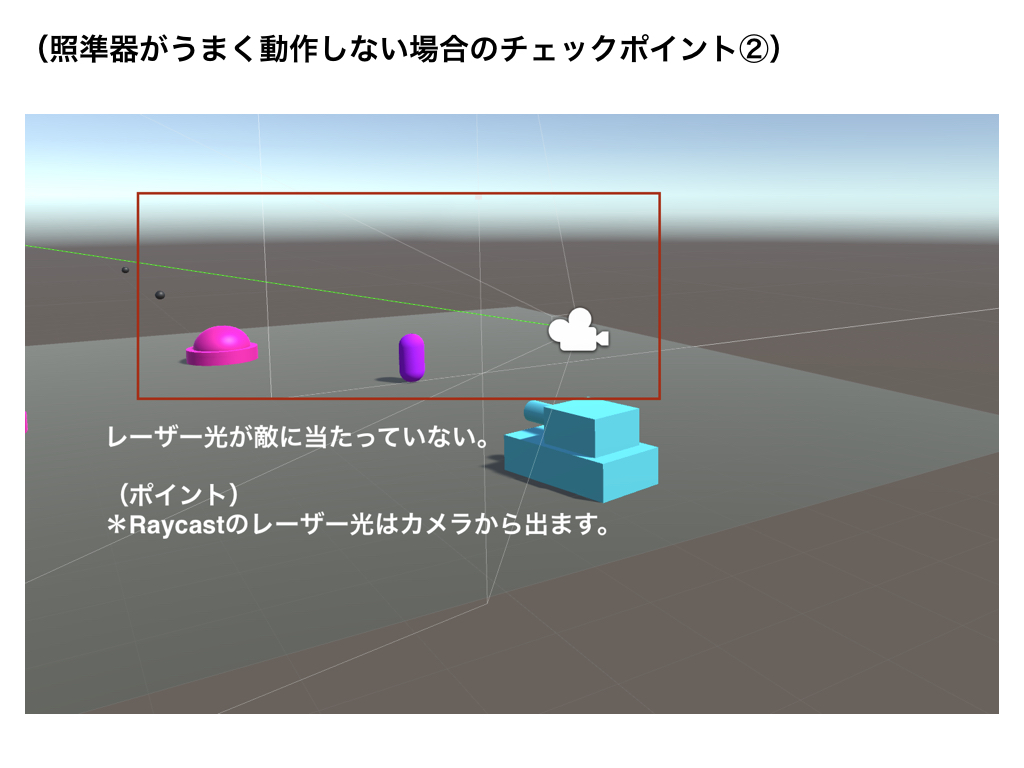
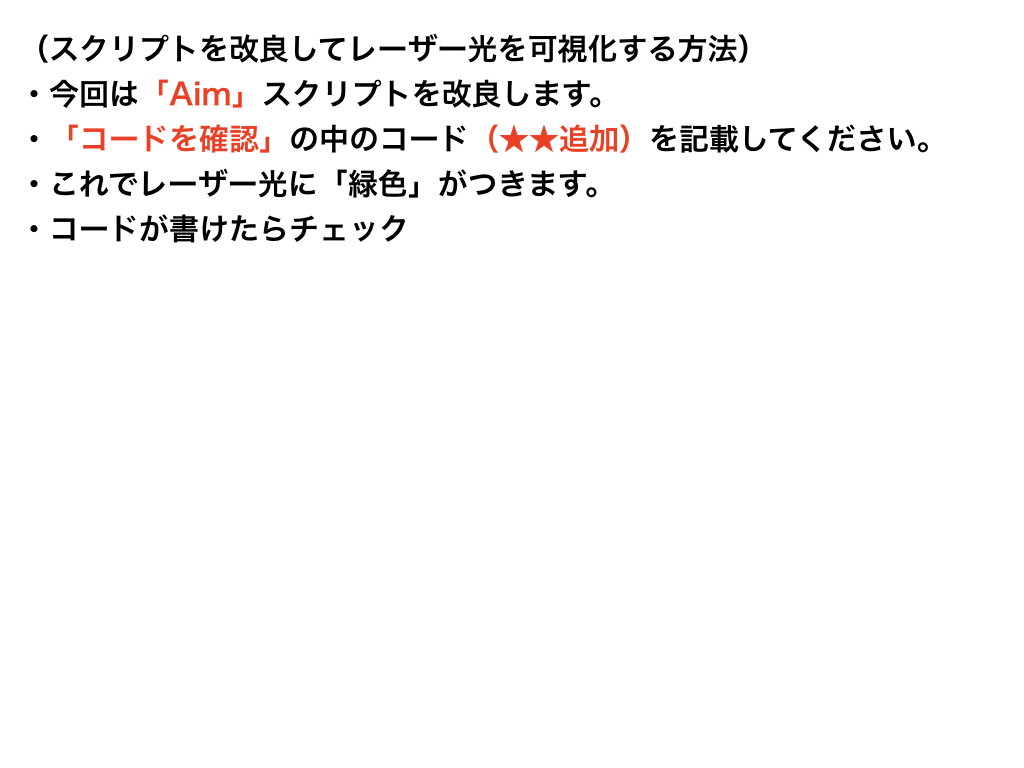
レーザー光を可視化する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class Aim : MonoBehaviour
{
public Image aimImage;
void Update()
{
Ray ray = new Ray(transform.position, transform.forward);
// ★★追加(レーザー光を可視化することができる)
Debug.DrawRay(transform.position, transform.forward * 30, Color.green);
RaycastHit hit;
if (Physics.Raycast(ray, out hit, 60))
{
string hitName = hit.transform.gameObject.tag;
if (hitName == "Enemy")
{
aimImage.color = new Color(1.0f, 0.0f, 0.0f, 1.0f);
}
else
{
aimImage.color = new Color(0.0f, 1.0f, 1.0f, 1.0f);
}
}
else
{
aimImage.color = new Color(0.0f, 1.0f, 1.0f, 1.0f);
}
}
}
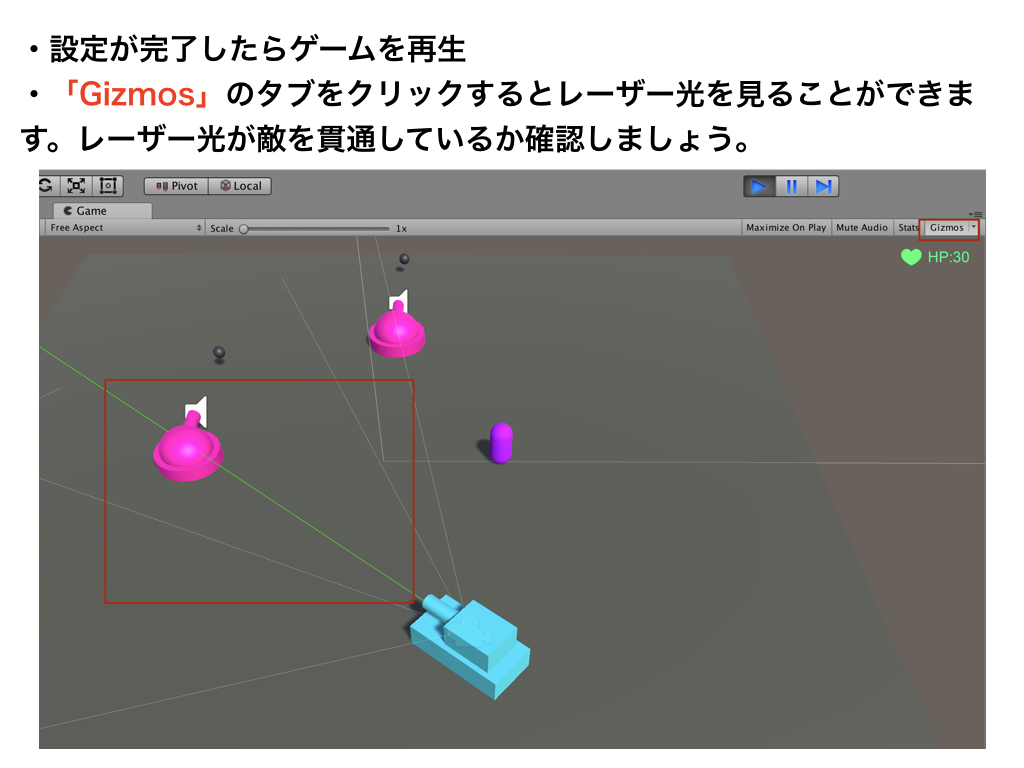
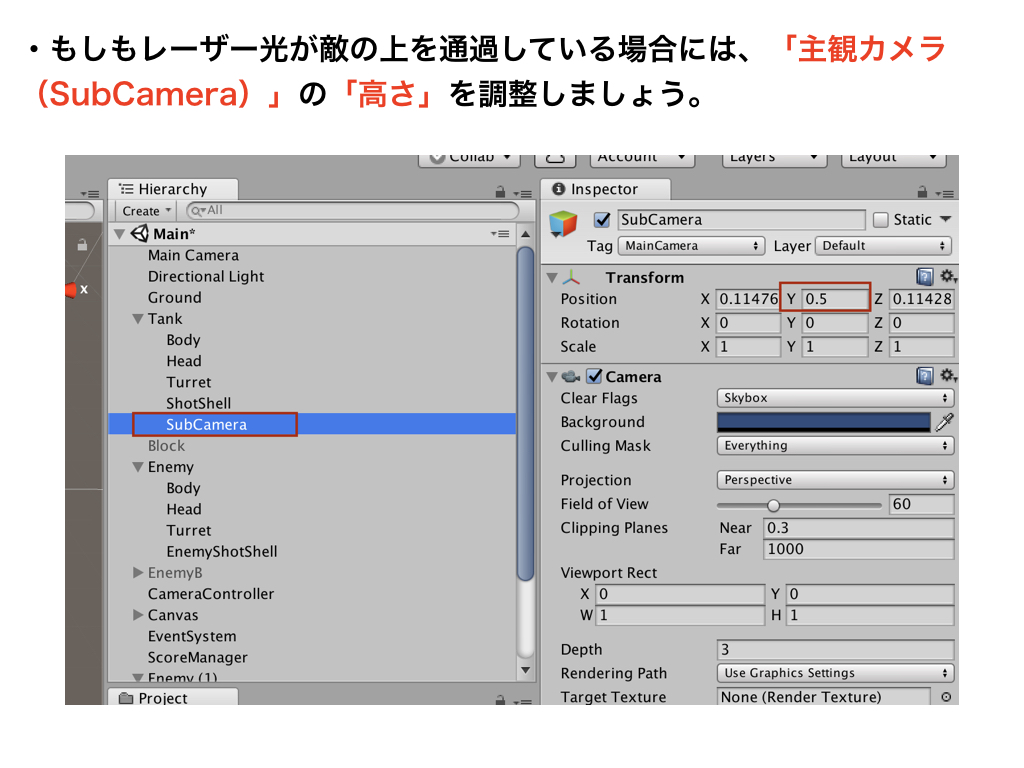
照準器を作る