敵に得点を付けて画面にスコア表示
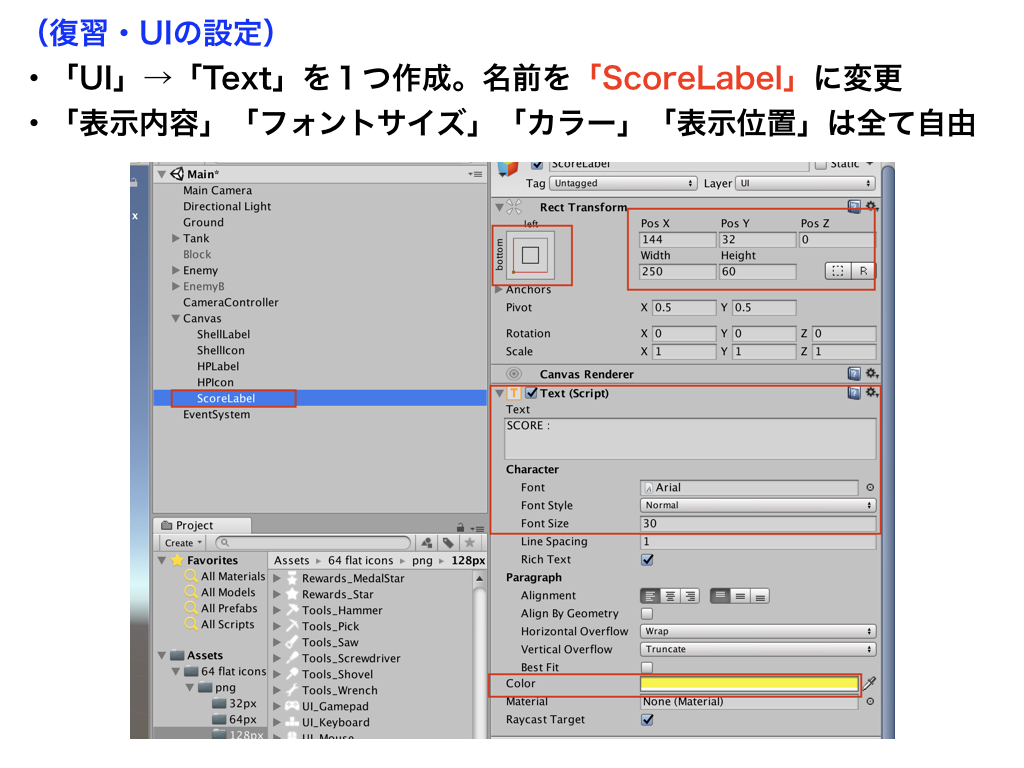
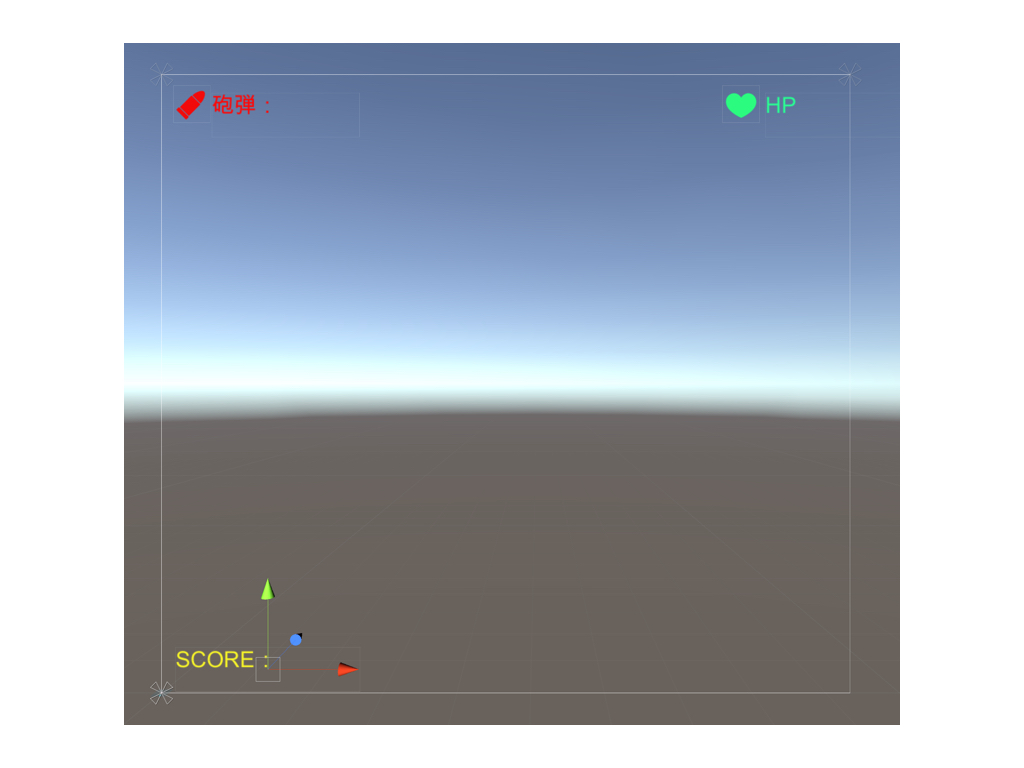
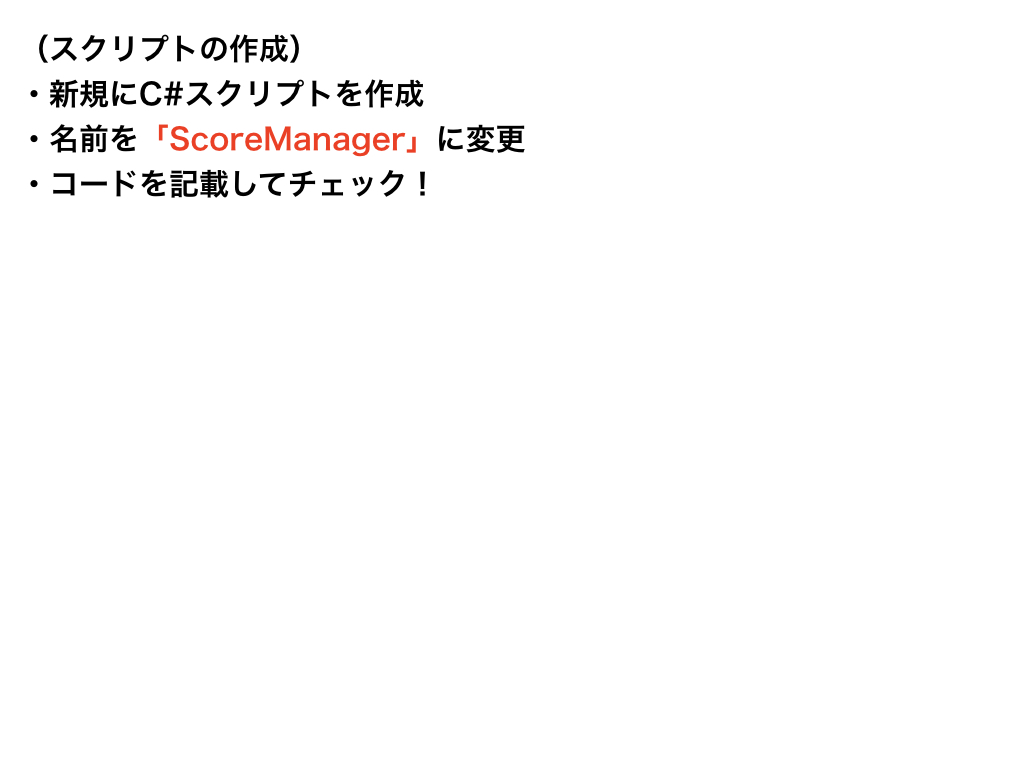
スコアを増加させるメソッド
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// UIを使う場合には忘れずに追加すること!
using UnityEngine.UI;
public class ScoreManager : MonoBehaviour
{
private int score = 0;
private Text scoreLabel;
void Start()
{
scoreLabel = GameObject.Find("ScoreLabel").GetComponent<Text>();
scoreLabel.text = "SCORE:" + score;
}
// スコアを増加させるメソッド
// 外部からアクセスするためpublicで定義する
public void AddScore(int amount)
{
score += amount;
scoreLabel.text = "SCORE:" + score;
}
}
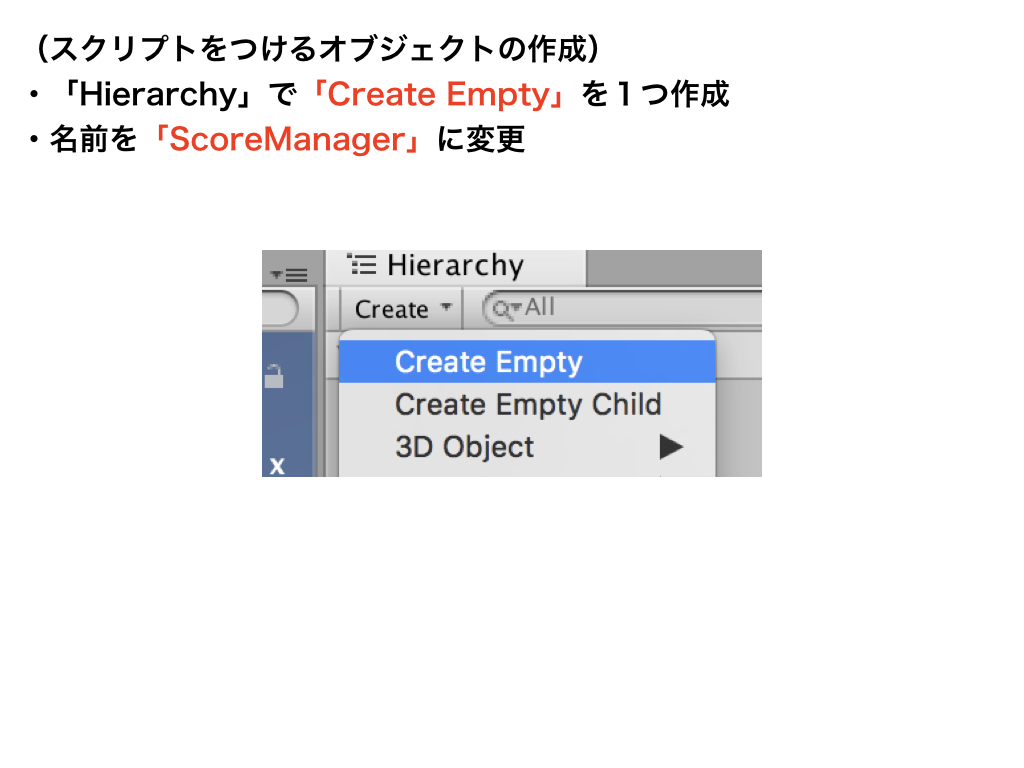
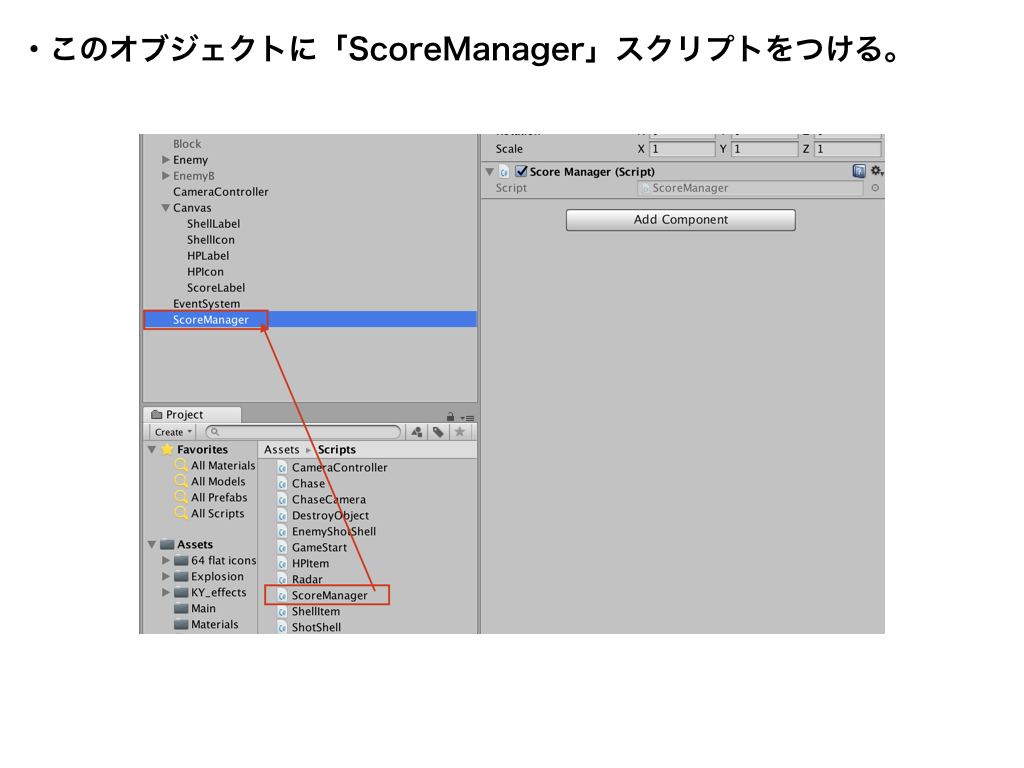
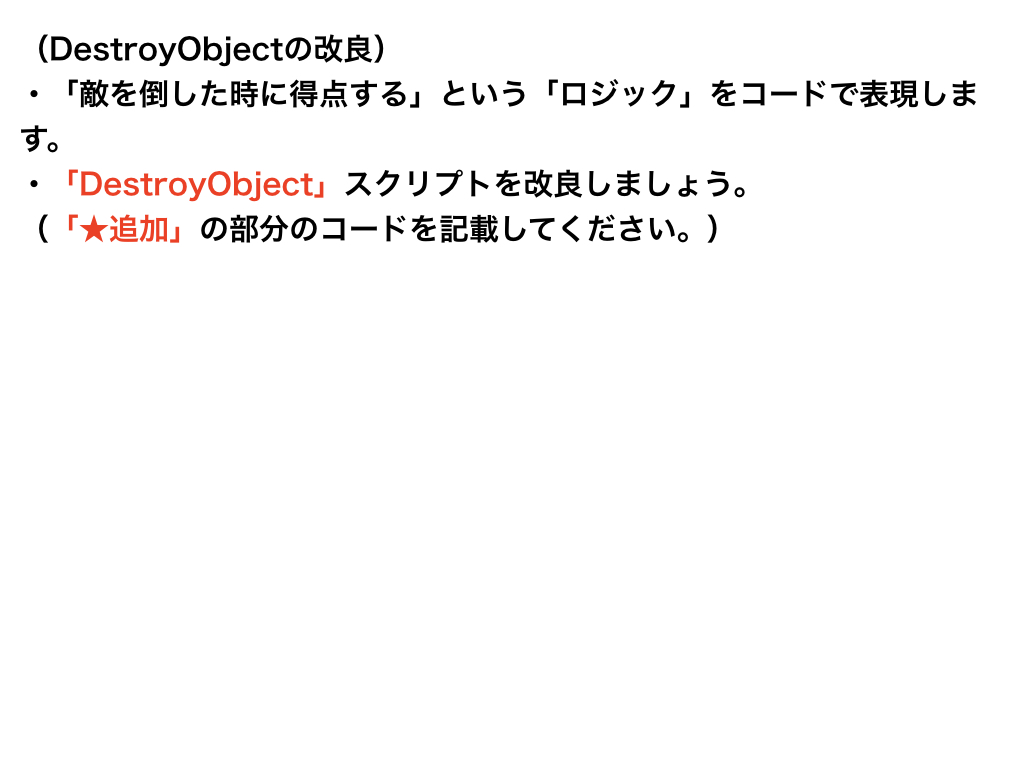
敵を倒すと点数が入る
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class DestroyObject : MonoBehaviour
{
public GameObject effectPrefab;
public GameObject effectPrefab2;
public int objectHP;
public GameObject[] itemPrefabs;
// ★追加
public int scoreValue; // これが敵を倒すと得られる点数になる
private ScoreManager sm;
// ★追加
void Start()
{
// 「ScoreManagerオブジェクト」に付いている「ScoreManagerスクリプト」の情報を取得して「sm」の箱に入れる。
sm = GameObject.Find("ScoreManager").GetComponent<ScoreManager>();
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Shell"))
{
objectHP -= 1;
if (objectHP > 0)
{
Destroy(other.gameObject);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 2.0f);
}
else
{
Destroy(other.gameObject);
GameObject effect2 = Instantiate(effectPrefab2, transform.position, Quaternion.identity);
Destroy(effect2, 2.0f);
Destroy(this.gameObject);
GameObject dropItem = itemPrefabs[Random.Range(0, itemPrefabs.Length)];
Vector3 pos = transform.position;
Instantiate(dropItem, new Vector3(pos.x, pos.y + 0.5f, pos.z), Quaternion.identity);
// ★追加
sm.AddScore(scoreValue);
}
}
}
}
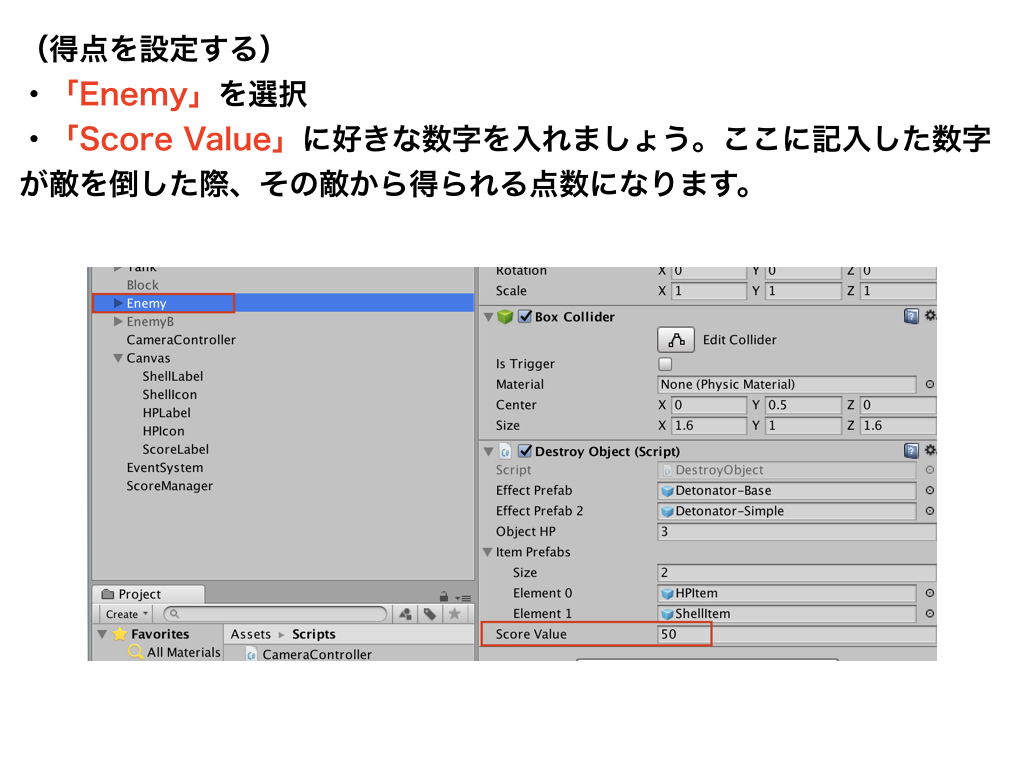
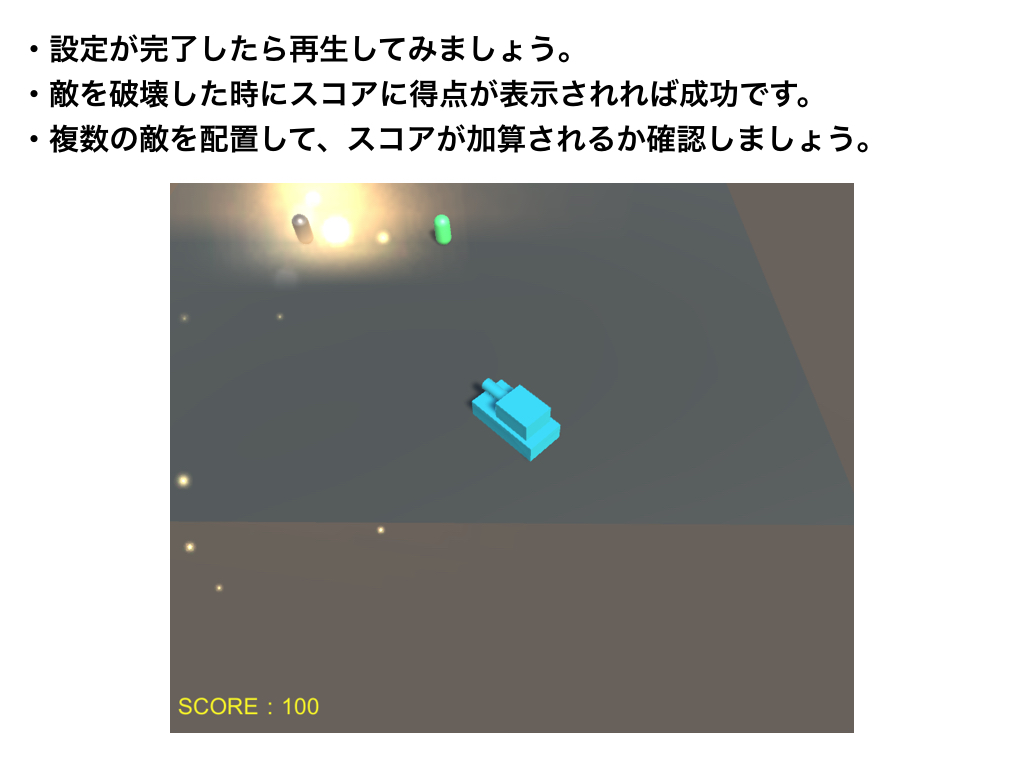
BattleTank(基礎/全31回)
他のコースを見る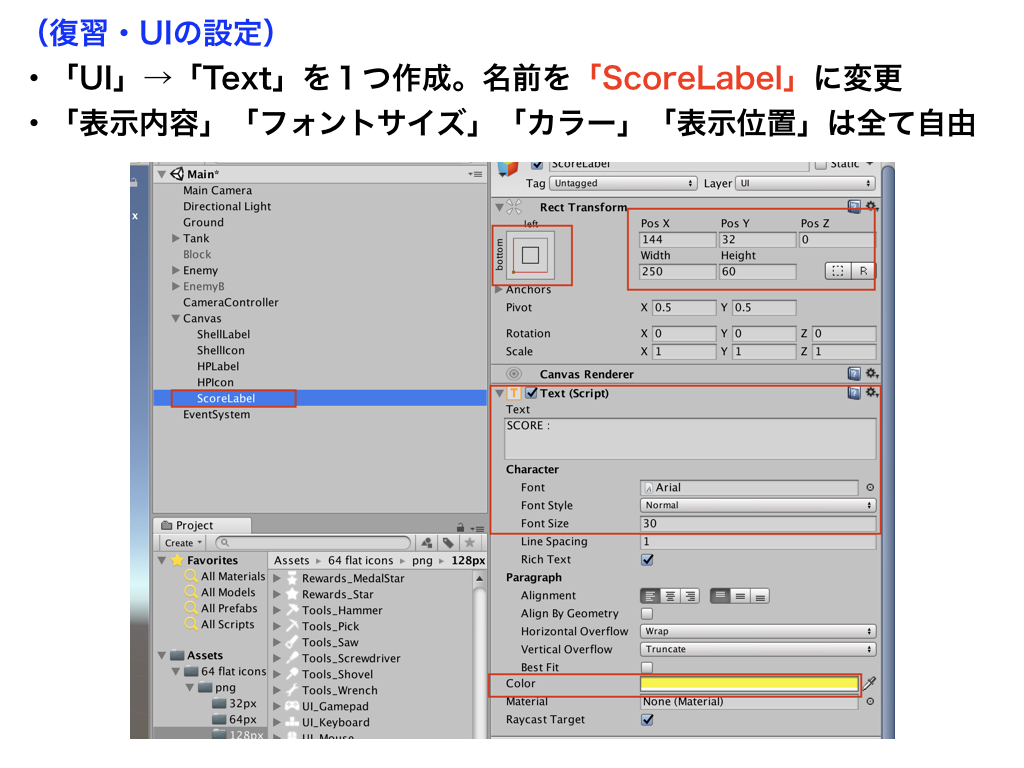
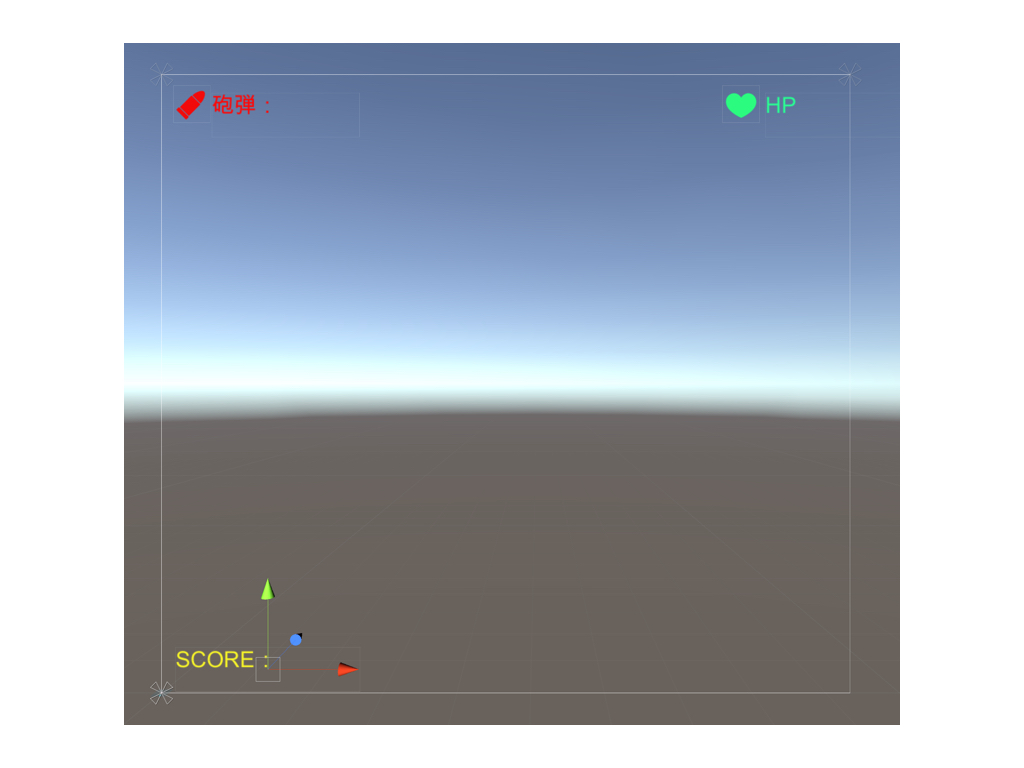
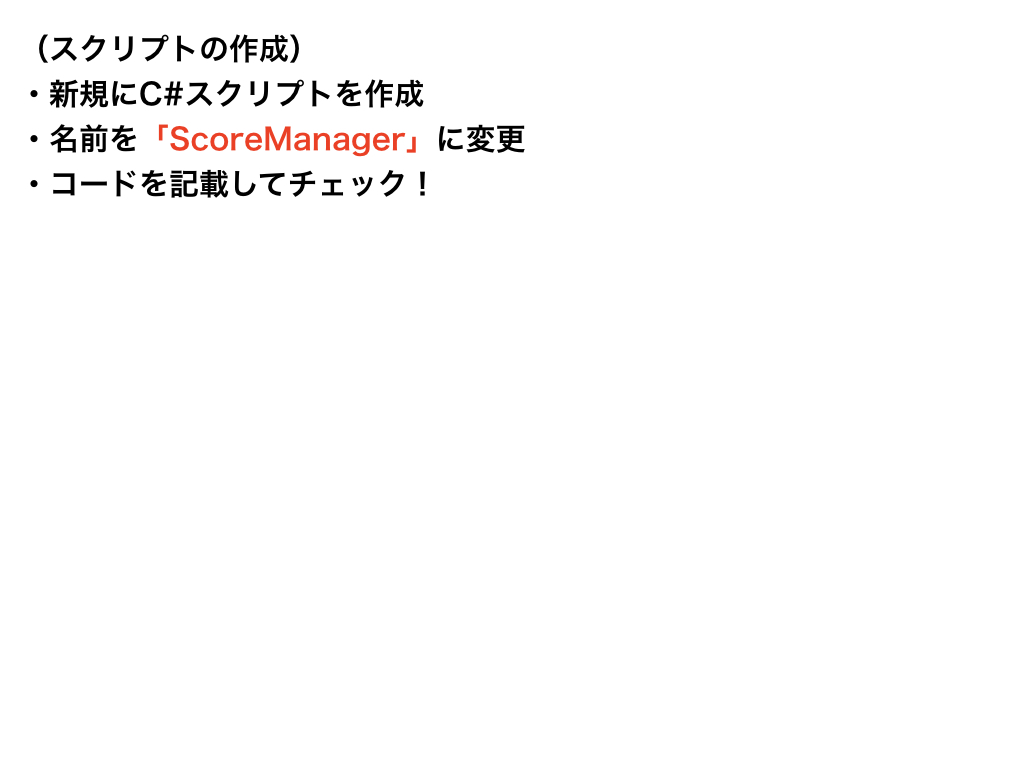
スコアを増加させるメソッド
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// UIを使う場合には忘れずに追加すること!
using UnityEngine.UI;
public class ScoreManager : MonoBehaviour
{
private int score = 0;
private Text scoreLabel;
void Start()
{
scoreLabel = GameObject.Find("ScoreLabel").GetComponent<Text>();
scoreLabel.text = "SCORE:" + score;
}
// スコアを増加させるメソッド
// 外部からアクセスするためpublicで定義する
public void AddScore(int amount)
{
score += amount;
scoreLabel.text = "SCORE:" + score;
}
}
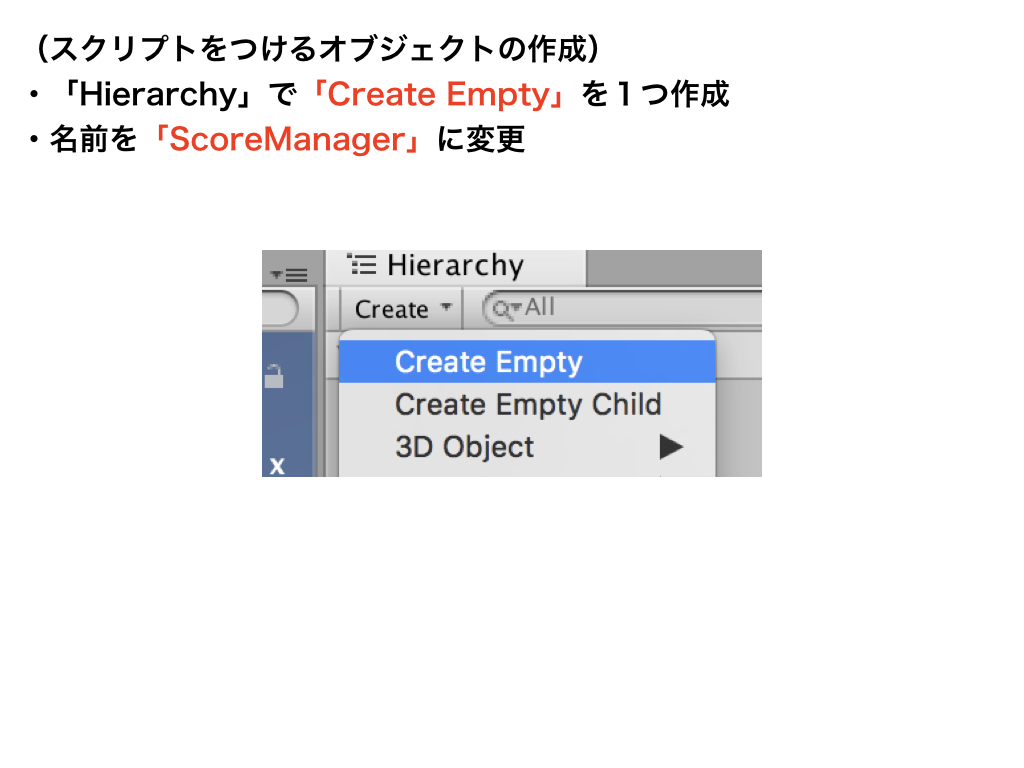
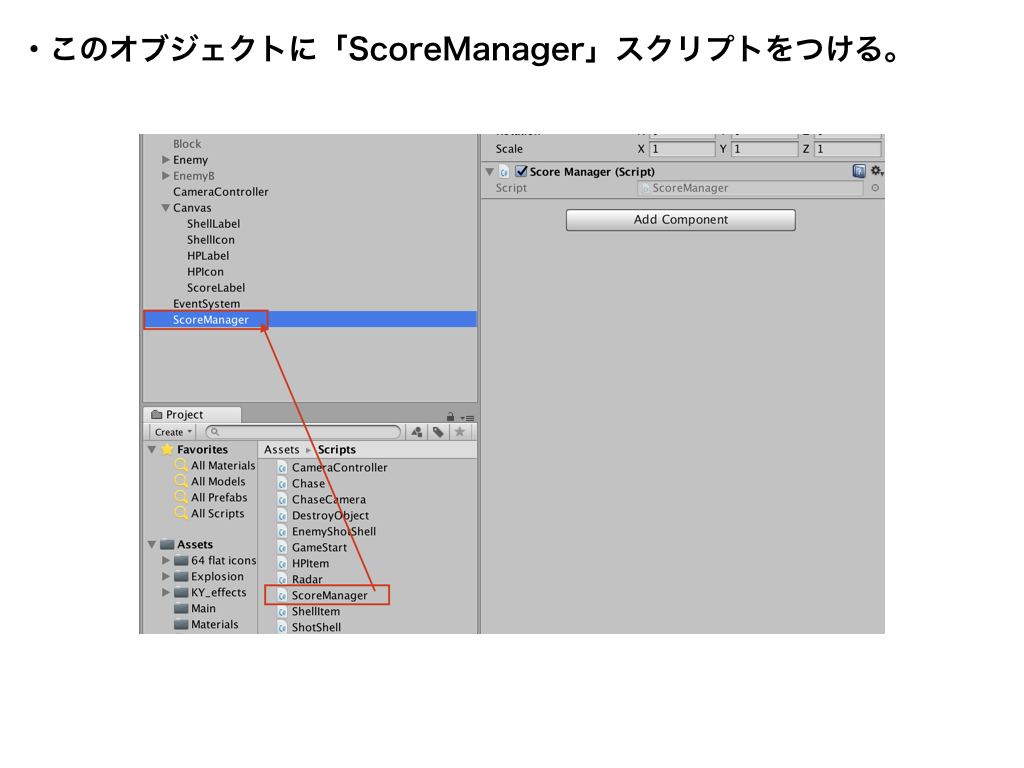
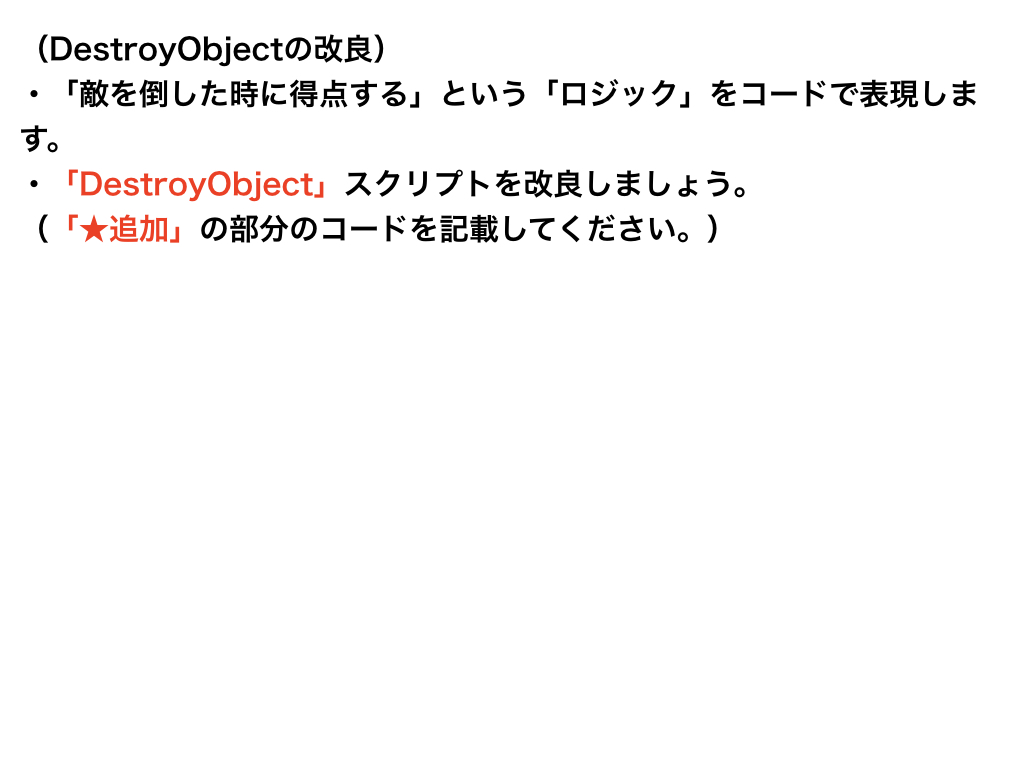
敵を倒すと点数が入る
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class DestroyObject : MonoBehaviour
{
public GameObject effectPrefab;
public GameObject effectPrefab2;
public int objectHP;
public GameObject[] itemPrefabs;
// ★追加
public int scoreValue; // これが敵を倒すと得られる点数になる
private ScoreManager sm;
// ★追加
void Start()
{
// 「ScoreManagerオブジェクト」に付いている「ScoreManagerスクリプト」の情報を取得して「sm」の箱に入れる。
sm = GameObject.Find("ScoreManager").GetComponent<ScoreManager>();
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Shell"))
{
objectHP -= 1;
if (objectHP > 0)
{
Destroy(other.gameObject);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 2.0f);
}
else
{
Destroy(other.gameObject);
GameObject effect2 = Instantiate(effectPrefab2, transform.position, Quaternion.identity);
Destroy(effect2, 2.0f);
Destroy(this.gameObject);
GameObject dropItem = itemPrefabs[Random.Range(0, itemPrefabs.Length)];
Vector3 pos = transform.position;
Instantiate(dropItem, new Vector3(pos.x, pos.y + 0.5f, pos.z), Quaternion.identity);
// ★追加
sm.AddScore(scoreValue);
}
}
}
}
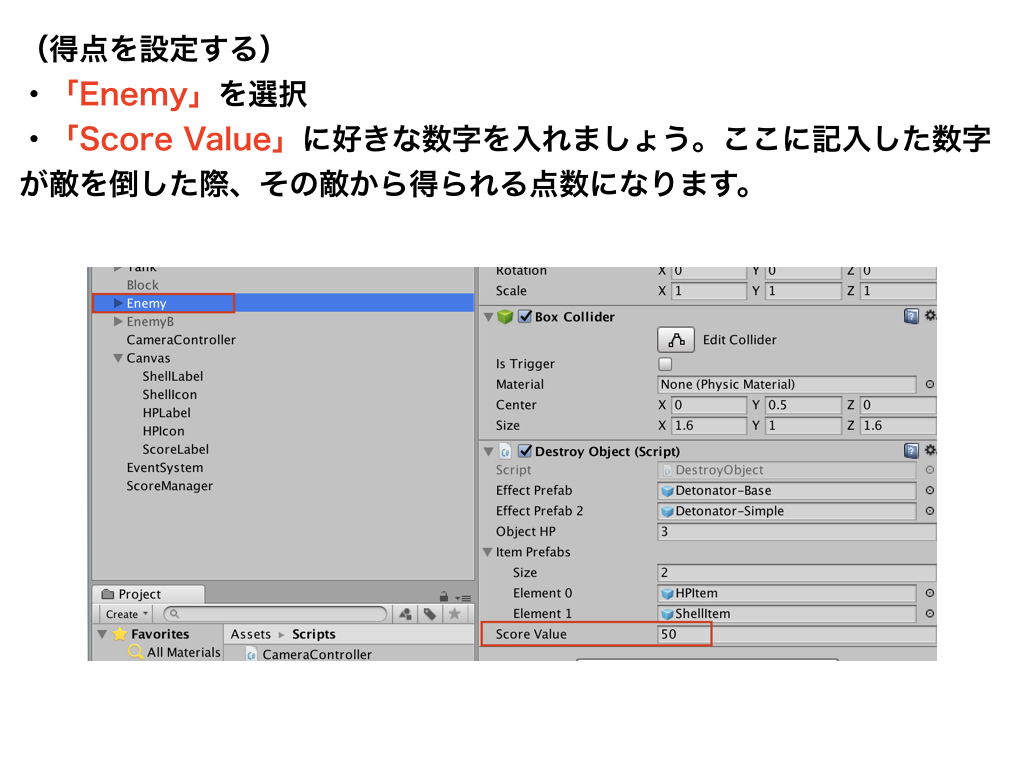
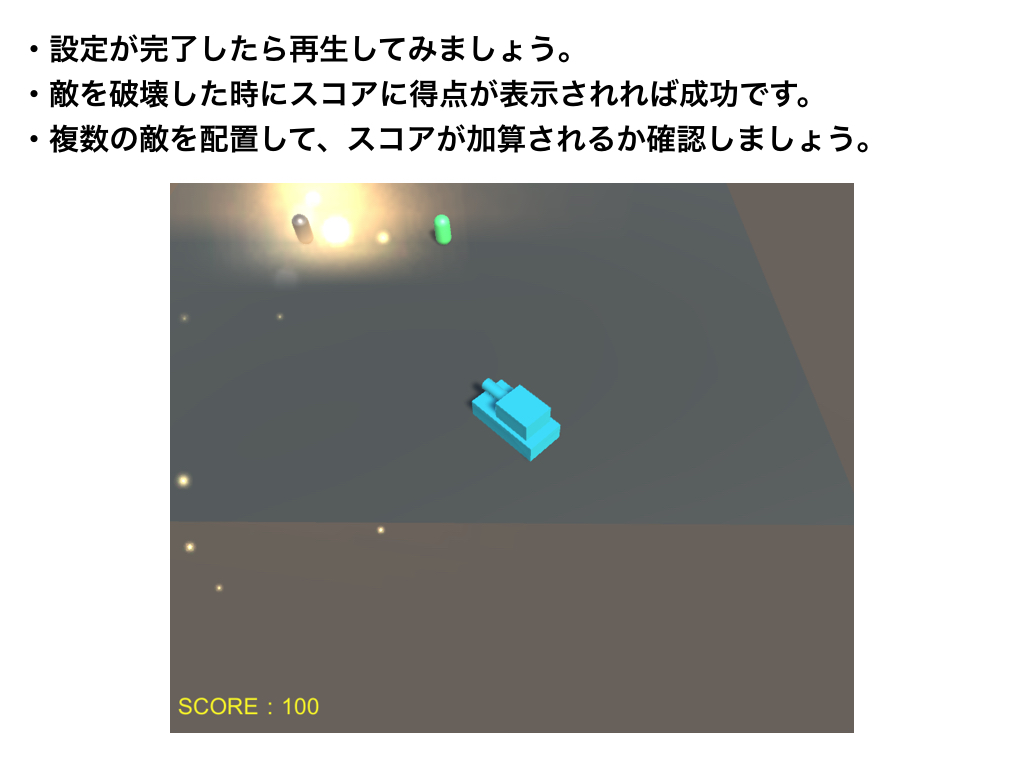
敵に得点を付けて画面にスコア表示