「動く床」に乗った時に一緒に移動させる方法
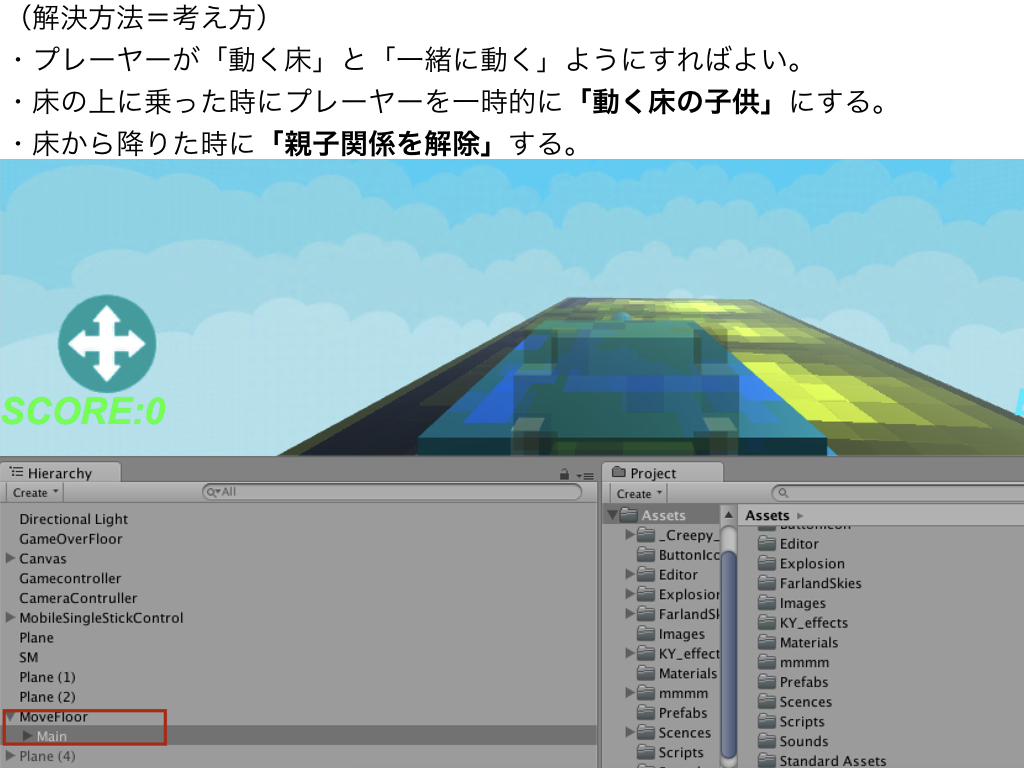
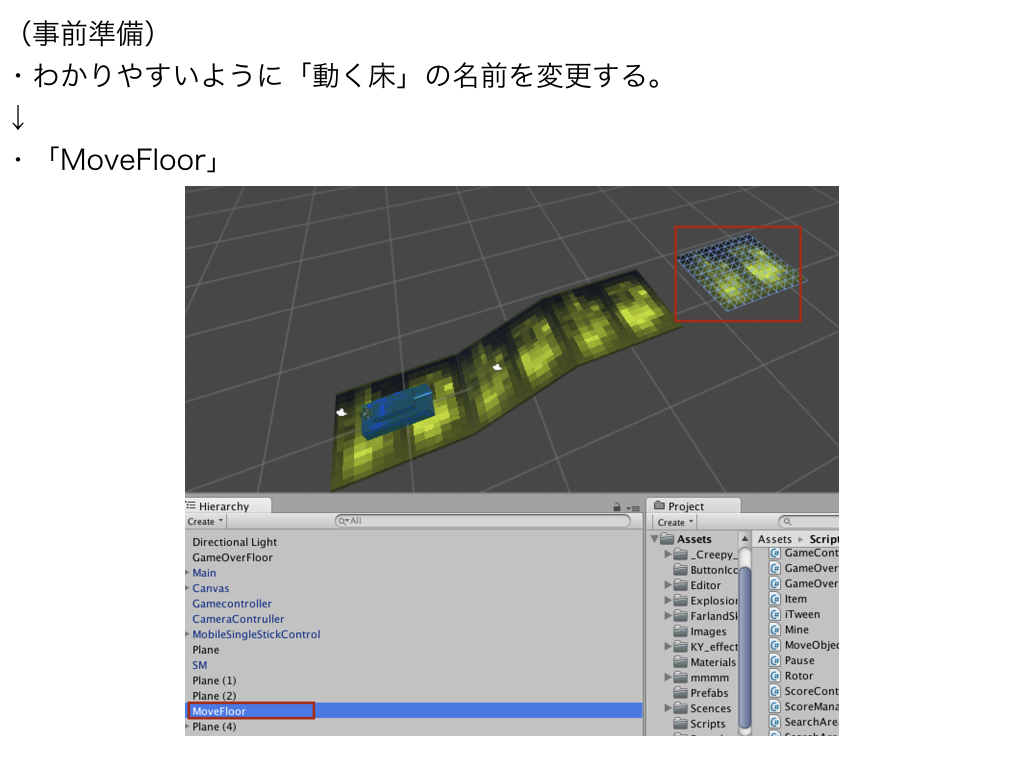
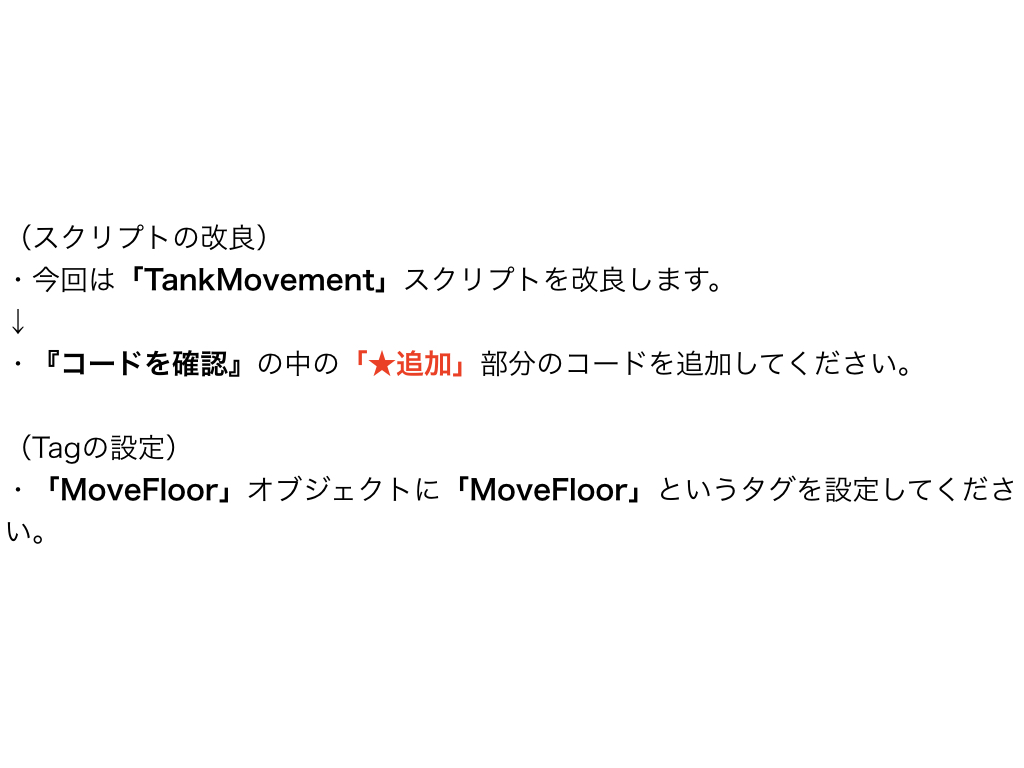
動く床と一緒に移動させる方法
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
using UnityStandardAssets.CrossPlatformInput;
using UnityEngine.SceneManagement;
public class TankMovement : MonoBehaviour {
public float moveSpeed;
public float turnSpeed;
public float boostSpeed;
private Rigidbody rb;
private float movementInputValue;
private float turnInputValue;
private int boostCount = 5;
private Text boostLabel;
void Awake(){
rb = GetComponent<Rigidbody> ();
}
void Start(){
boostLabel = GameObject.Find ("Boost").GetComponent<Text> ();
boostLabel.text = "Boost: " + boostCount;
}
/*void Update() {
//movementInputValue = Input.GetAxis("Vertical");
//turnInputValue = Input.GetAxis("Horizontal");
if(Input.GetButtonDown("Boost")){
if(boostCount <= 0) return;
rb.velocity = transform.forward * boostSpeed;
boostCount -= 1;
boostLabel.text = "Boost: " + boostCount;
}
}*/
public void OnBoostButtonClicked(){
if (boostCount < 1)
return;
rb.velocity = transform.forward * boostSpeed;
boostCount -= 1;
boostLabel.text = "Boost: " + boostCount;
}
void FixedUpdate(){
movementInputValue = CrossPlatformInputManager.GetAxisRaw("Vertical");
turnInputValue = CrossPlatformInputManager.GetAxisRaw ("Horizontal");
Move();
Turn();
}
void Move(){
Vector3 movement = transform.forward * movementInputValue * moveSpeed * Time.deltaTime;
rb.MovePosition (rb.position + movement);
}
void Turn(){
float turn = turnInputValue * turnSpeed * Time.deltaTime;
Quaternion turnRotation = Quaternion.Euler (0f, turn, 0f);
rb.MoveRotation (rb.rotation * turnRotation);
}
public void AddBoost(int amount){
boostCount += amount;
boostLabel.text = "Boost: " + boostCount;
}
// ★追加
void OnCollisionEnter(Collision other){
if(other.gameObject.CompareTag("MoveFloor")){
// ぶつかった相手を親にセットする。
transform.SetParent(other.transform);
}
}
// ★追加
void OnCollisionExit(Collision other){
if(other.gameObject.CompareTag("MoveFloor")){
// 親子関係を解除する。
// 「null」は「空っぽ(中身がない)」という意味
transform.SetParent(null);
}
}
}
Unity Code Memo
他のコースを見る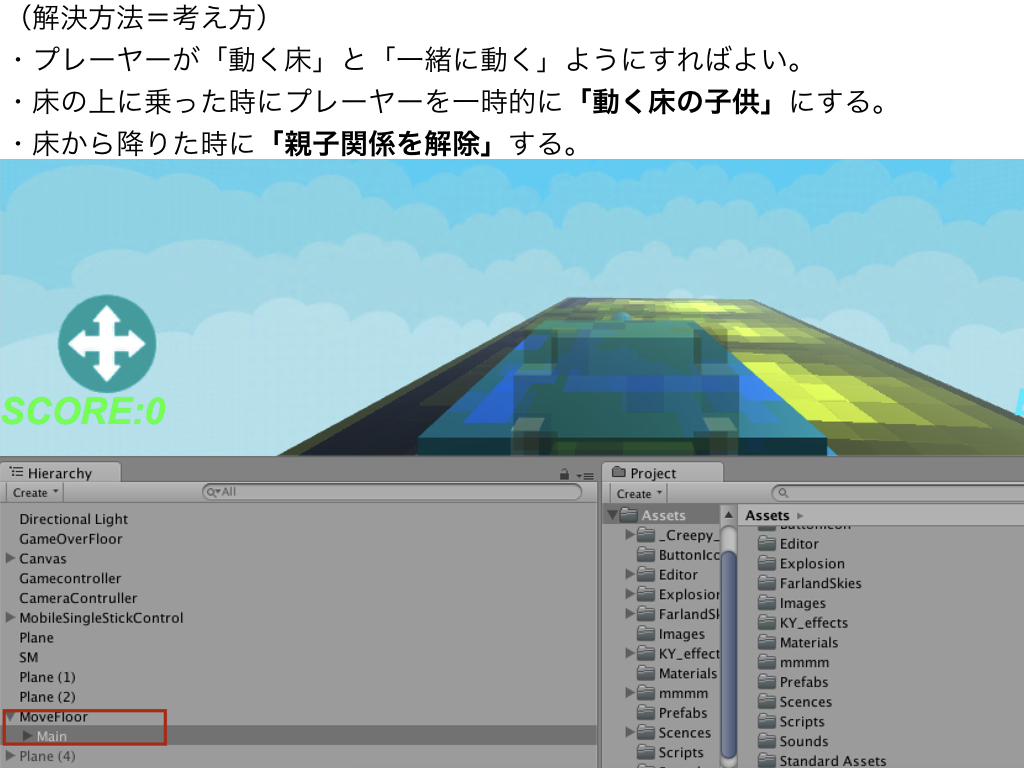
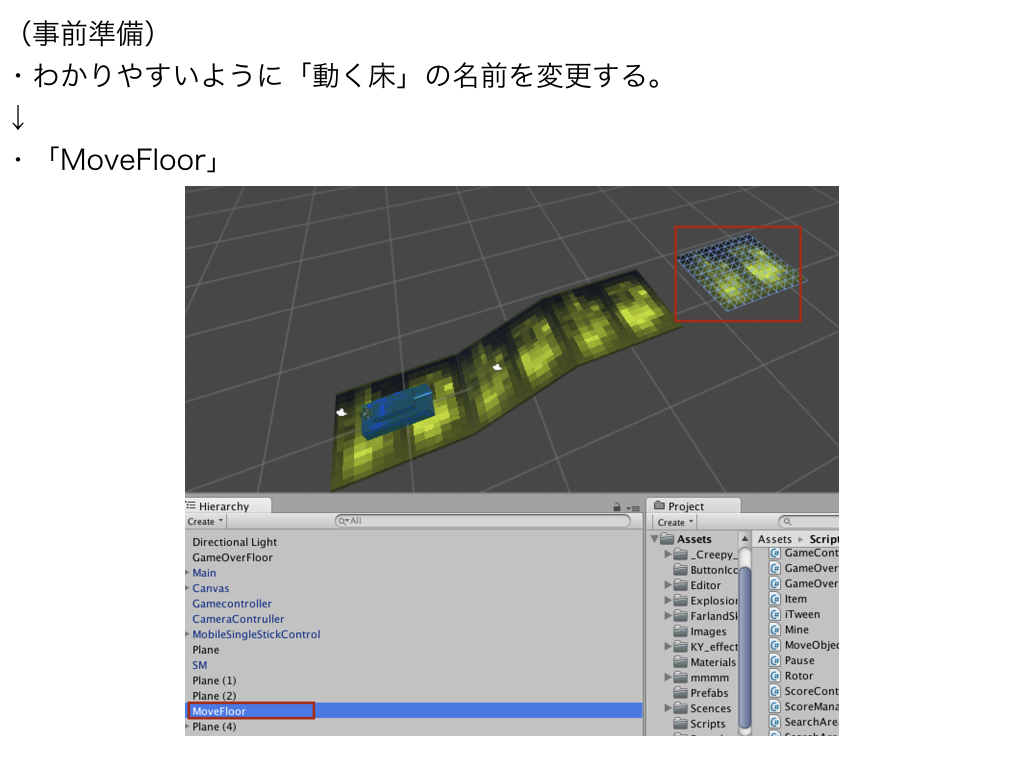
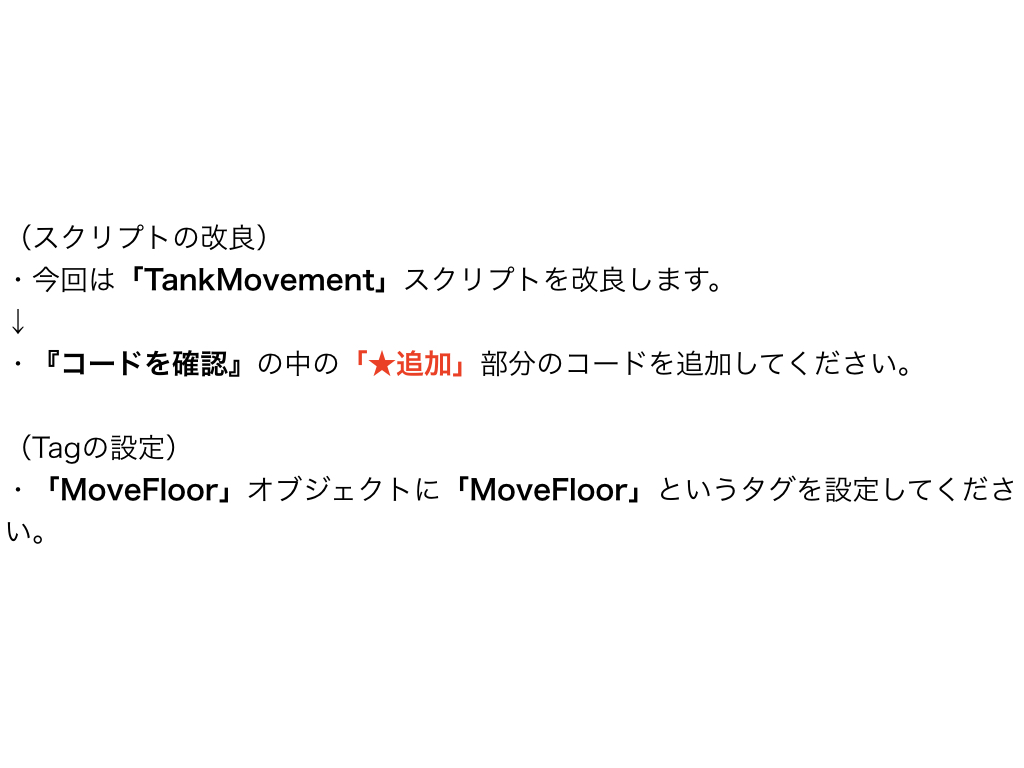
動く床と一緒に移動させる方法
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
using UnityStandardAssets.CrossPlatformInput;
using UnityEngine.SceneManagement;
public class TankMovement : MonoBehaviour {
public float moveSpeed;
public float turnSpeed;
public float boostSpeed;
private Rigidbody rb;
private float movementInputValue;
private float turnInputValue;
private int boostCount = 5;
private Text boostLabel;
void Awake(){
rb = GetComponent<Rigidbody> ();
}
void Start(){
boostLabel = GameObject.Find ("Boost").GetComponent<Text> ();
boostLabel.text = "Boost: " + boostCount;
}
/*void Update() {
//movementInputValue = Input.GetAxis("Vertical");
//turnInputValue = Input.GetAxis("Horizontal");
if(Input.GetButtonDown("Boost")){
if(boostCount <= 0) return;
rb.velocity = transform.forward * boostSpeed;
boostCount -= 1;
boostLabel.text = "Boost: " + boostCount;
}
}*/
public void OnBoostButtonClicked(){
if (boostCount < 1)
return;
rb.velocity = transform.forward * boostSpeed;
boostCount -= 1;
boostLabel.text = "Boost: " + boostCount;
}
void FixedUpdate(){
movementInputValue = CrossPlatformInputManager.GetAxisRaw("Vertical");
turnInputValue = CrossPlatformInputManager.GetAxisRaw ("Horizontal");
Move();
Turn();
}
void Move(){
Vector3 movement = transform.forward * movementInputValue * moveSpeed * Time.deltaTime;
rb.MovePosition (rb.position + movement);
}
void Turn(){
float turn = turnInputValue * turnSpeed * Time.deltaTime;
Quaternion turnRotation = Quaternion.Euler (0f, turn, 0f);
rb.MoveRotation (rb.rotation * turnRotation);
}
public void AddBoost(int amount){
boostCount += amount;
boostLabel.text = "Boost: " + boostCount;
}
// ★追加
void OnCollisionEnter(Collision other){
if(other.gameObject.CompareTag("MoveFloor")){
// ぶつかった相手を親にセットする。
transform.SetParent(other.transform);
}
}
// ★追加
void OnCollisionExit(Collision other){
if(other.gameObject.CompareTag("MoveFloor")){
// 親子関係を解除する。
// 「null」は「空っぽ(中身がない)」という意味
transform.SetParent(null);
}
}
}
「動く床」に乗った時に一緒に移動させる方法