衝突時に、周辺のオブジェクトを吹き飛ばす
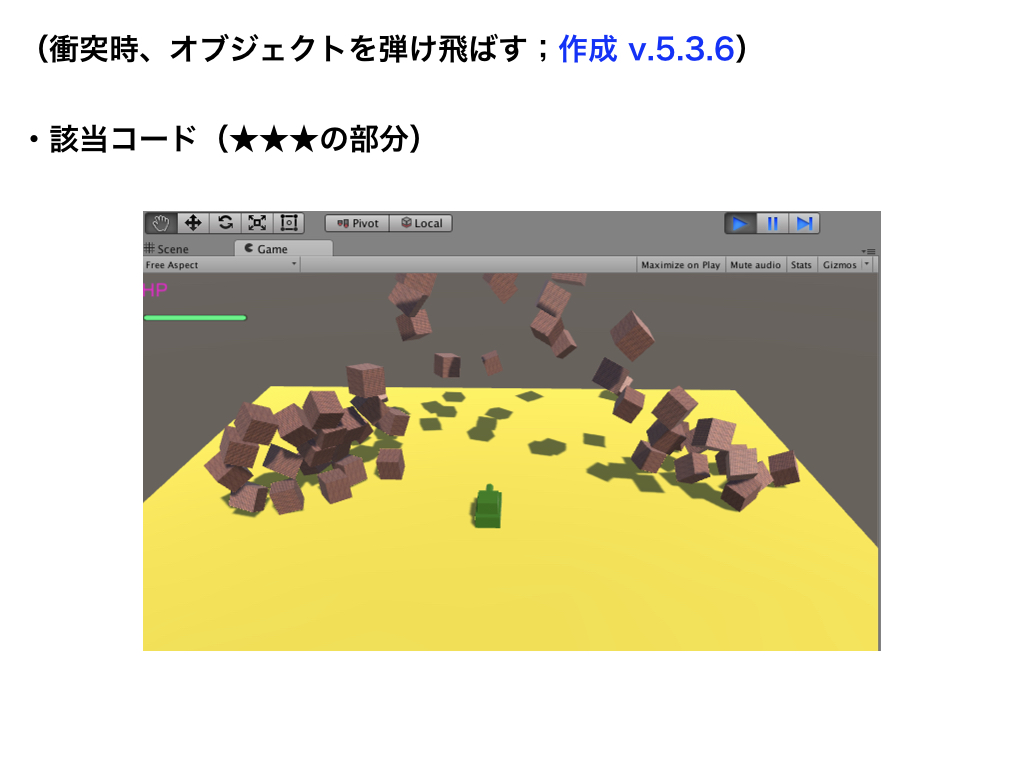
衝突の衝撃を表現する(オブジェクトに力を加えて吹き飛ばす)
using UnityEngine;
using System.Collections;
public class TankMovement : MonoBehaviour {
public float moveSpeed;
public float turnSpeed;
public float boostSpeed = 200.0f;
private Rigidbody rb;
private float movementInputValue;
private float turnInputValue;
// ★★★
public float radius = 10.0f;
public float power = 100.0f;
void Start () {
rb = GetComponent<Rigidbody>();
}
void Update () {
Move();
Turn();
if(Input.GetKeyDown(KeyCode.B)){
rb.velocity = transform.forward * boostSpeed;
}
}
void FixedUpdate(){
Debug.Log("只今の速度" + rb.velocity.magnitude);
}
void Move(){
movementInputValue = Input.GetAxis("Vertical");
Vector3 movement = transform.forward * movementInputValue * moveSpeed * Time.deltaTime;
rb.MovePosition(rb.position + movement);
}
void Turn(){
turnInputValue = Input.GetAxis("Horizontal");
float turn = turnInputValue * turnSpeed * Time.deltaTime;
Quaternion turnRotation = Quaternion.Euler(0, turn, 0);
rb.MoveRotation(rb.rotation * turnRotation);
}
// ★★★
void OnCollisionEnter(Collision other){
if(other.gameObject.tag == "Block"){
// 力を発生させる場所
Vector3 explosionPos = transform.position;
// 中心点から設定した半径の中にあるcolliderの配列を取得する。
Collider[] colliders = Physics.OverlapSphere(explosionPos, radius);
foreach(Collider hit in colliders){
// 力を及ぼしたいオブジェクトにRigidbodyが付いていることが必要(ポイント)
Rigidbody rb = hit.GetComponent<Rigidbody>();
if(rb != null){
// 取得したRigidbodyに力を加える
// 3つの引数(加える力の強さ、力の中心点、力を及ぼす半径)
rb.AddExplosionForce(power, explosionPos, 5.0f);
}
}
}
}
}
Unity Code Memo
他のコースを見る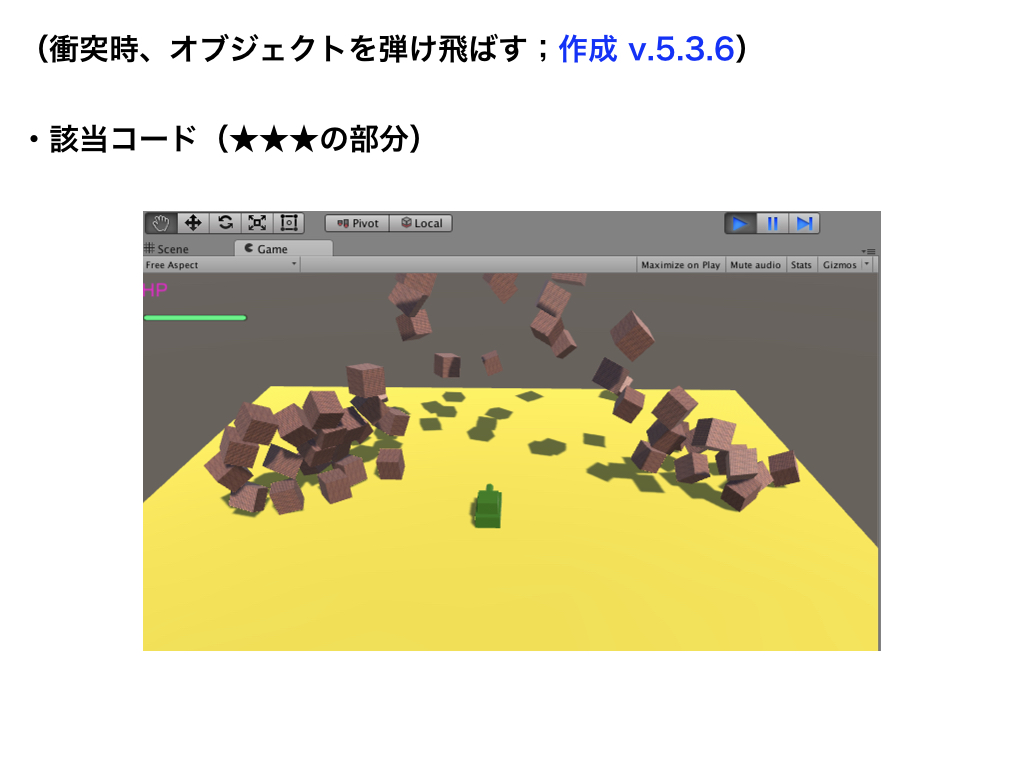
衝突の衝撃を表現する(オブジェクトに力を加えて吹き飛ばす)
using UnityEngine;
using System.Collections;
public class TankMovement : MonoBehaviour {
public float moveSpeed;
public float turnSpeed;
public float boostSpeed = 200.0f;
private Rigidbody rb;
private float movementInputValue;
private float turnInputValue;
// ★★★
public float radius = 10.0f;
public float power = 100.0f;
void Start () {
rb = GetComponent<Rigidbody>();
}
void Update () {
Move();
Turn();
if(Input.GetKeyDown(KeyCode.B)){
rb.velocity = transform.forward * boostSpeed;
}
}
void FixedUpdate(){
Debug.Log("只今の速度" + rb.velocity.magnitude);
}
void Move(){
movementInputValue = Input.GetAxis("Vertical");
Vector3 movement = transform.forward * movementInputValue * moveSpeed * Time.deltaTime;
rb.MovePosition(rb.position + movement);
}
void Turn(){
turnInputValue = Input.GetAxis("Horizontal");
float turn = turnInputValue * turnSpeed * Time.deltaTime;
Quaternion turnRotation = Quaternion.Euler(0, turn, 0);
rb.MoveRotation(rb.rotation * turnRotation);
}
// ★★★
void OnCollisionEnter(Collision other){
if(other.gameObject.tag == "Block"){
// 力を発生させる場所
Vector3 explosionPos = transform.position;
// 中心点から設定した半径の中にあるcolliderの配列を取得する。
Collider[] colliders = Physics.OverlapSphere(explosionPos, radius);
foreach(Collider hit in colliders){
// 力を及ぼしたいオブジェクトにRigidbodyが付いていることが必要(ポイント)
Rigidbody rb = hit.GetComponent<Rigidbody>();
if(rb != null){
// 取得したRigidbodyに力を加える
// 3つの引数(加える力の強さ、力の中心点、力を及ぼす半径)
rb.AddExplosionForce(power, explosionPos, 5.0f);
}
}
}
}
}
衝突時に、周辺のオブジェクトを吹き飛ばす