砲弾の「オブジェクトプール」化
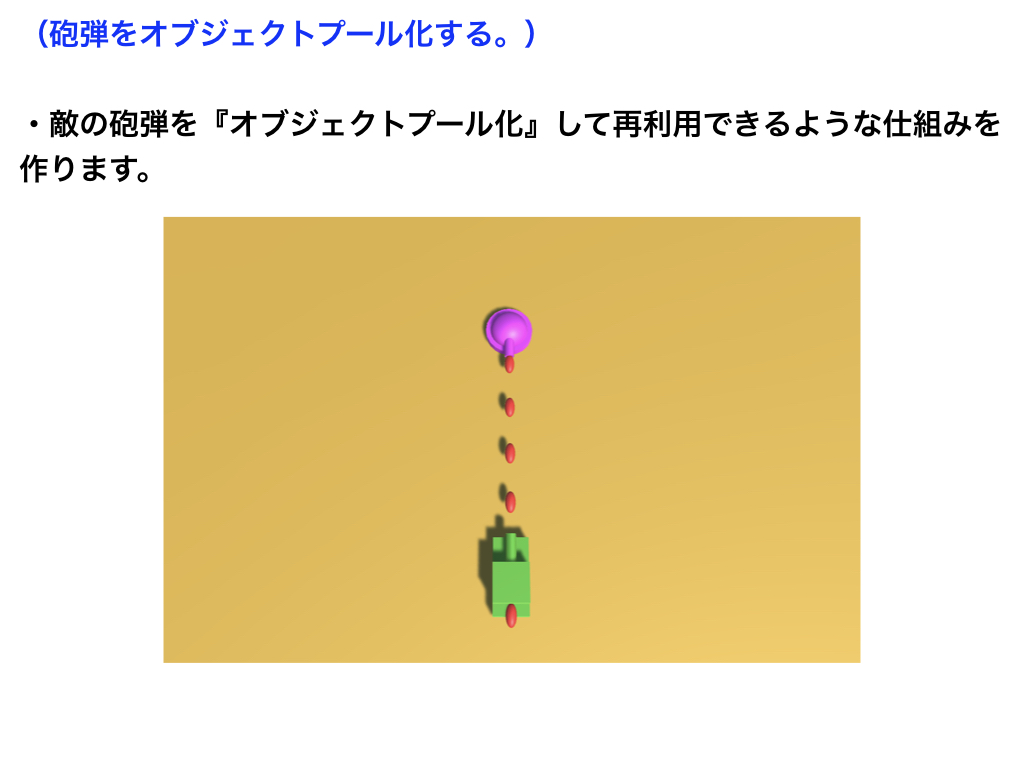
砲弾のオブジェクトプール化
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RadarShot : MonoBehaviour
{
private GameObject target;
public GameObject shellPrefab;
public AudioClip sound;
private int count;
// ★プール
private List<GameObject> shellList = new List<GameObject>();
void Start()
{
target = GameObject.FindGameObjectWithTag("Player");
// ★プール
// プール化する個数は自由に設定(今回は10個)
for(int i = 0; i < 10; i++)
{
GameObject shell = Instantiate(shellPrefab);
// 作成した砲弾は全てノナクティブにする。
shell.SetActive(false);
// 作成した砲弾は『リスト(List)』に追加して管理する。
shellList.Add(shell);
}
}
void Update()
{
if(Vector3.Distance(transform.position, target.transform.position) <= 10f)
{
transform.root.LookAt(target.transform.position);
count += 1;
if (count % 10 == 0)
{
// ★プール
for(int i = 0; i < shellList.Count; i++)
{
// 重要ポイント
// もしも○番目の砲弾がノンアクティブ状態ならば・・・(条件)
if (!shellList[i].activeSelf)
{
// アクティブ状態にする。
shellList[i].SetActive(true);
// 発射台と位置合わせ
shellList[i].transform.position = transform.position;
// 発射台と角度合わせ
shellList[i].transform.rotation = transform.rotation;
Rigidbody shellRb = shellList[i].GetComponent<Rigidbody>();
shellRb.AddForce(transform.forward * 500);
AudioSource.PlayClipAtPoint(sound, transform.position);
// 処理を抜ける(重要ポイント)
break;
}
}
}
}
}
}
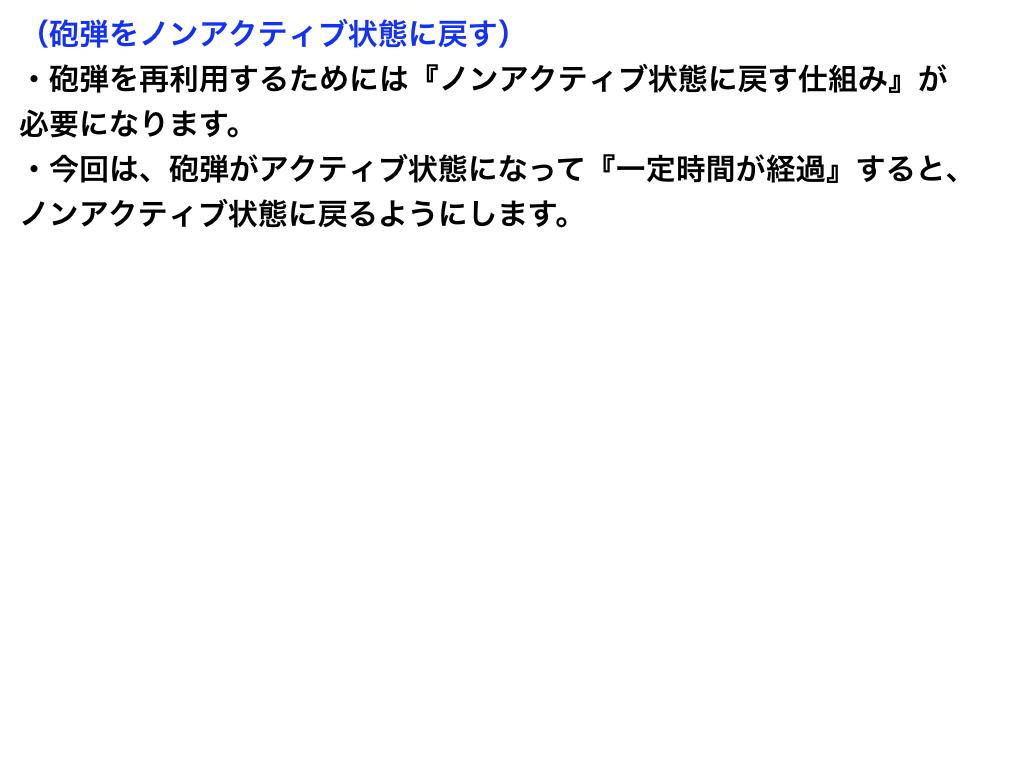
砲弾をノンアクティブに戻す
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShellLife : MonoBehaviour
{
// アクティブ状態になった場合に呼ばれる。
private void OnEnable()
{
// 砲弾の速度を0にリセットする(重要)
this.gameObject.GetComponent<Rigidbody>().velocity = Vector3.zero;
Invoke("NonActive", 3.0f);
}
void NonActive()
{
this.gameObject.SetActive(false);
}
}
Unity Code Memo
他のコースを見る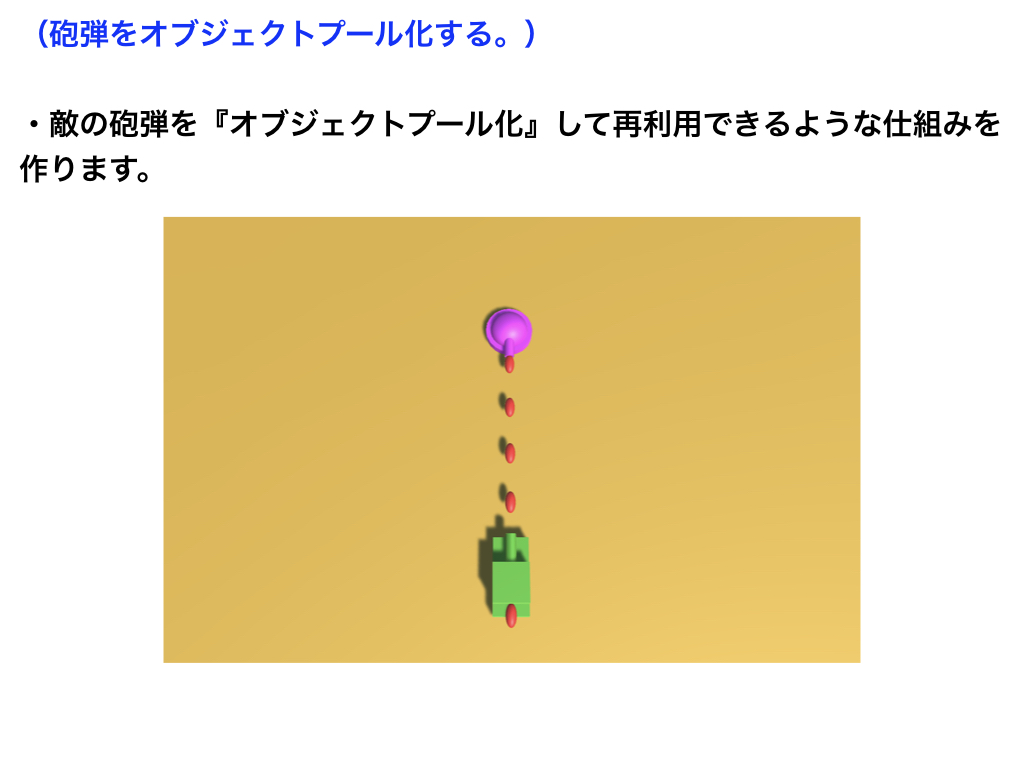
砲弾のオブジェクトプール化
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RadarShot : MonoBehaviour
{
private GameObject target;
public GameObject shellPrefab;
public AudioClip sound;
private int count;
// ★プール
private List<GameObject> shellList = new List<GameObject>();
void Start()
{
target = GameObject.FindGameObjectWithTag("Player");
// ★プール
// プール化する個数は自由に設定(今回は10個)
for(int i = 0; i < 10; i++)
{
GameObject shell = Instantiate(shellPrefab);
// 作成した砲弾は全てノナクティブにする。
shell.SetActive(false);
// 作成した砲弾は『リスト(List)』に追加して管理する。
shellList.Add(shell);
}
}
void Update()
{
if(Vector3.Distance(transform.position, target.transform.position) <= 10f)
{
transform.root.LookAt(target.transform.position);
count += 1;
if (count % 10 == 0)
{
// ★プール
for(int i = 0; i < shellList.Count; i++)
{
// 重要ポイント
// もしも○番目の砲弾がノンアクティブ状態ならば・・・(条件)
if (!shellList[i].activeSelf)
{
// アクティブ状態にする。
shellList[i].SetActive(true);
// 発射台と位置合わせ
shellList[i].transform.position = transform.position;
// 発射台と角度合わせ
shellList[i].transform.rotation = transform.rotation;
Rigidbody shellRb = shellList[i].GetComponent<Rigidbody>();
shellRb.AddForce(transform.forward * 500);
AudioSource.PlayClipAtPoint(sound, transform.position);
// 処理を抜ける(重要ポイント)
break;
}
}
}
}
}
}
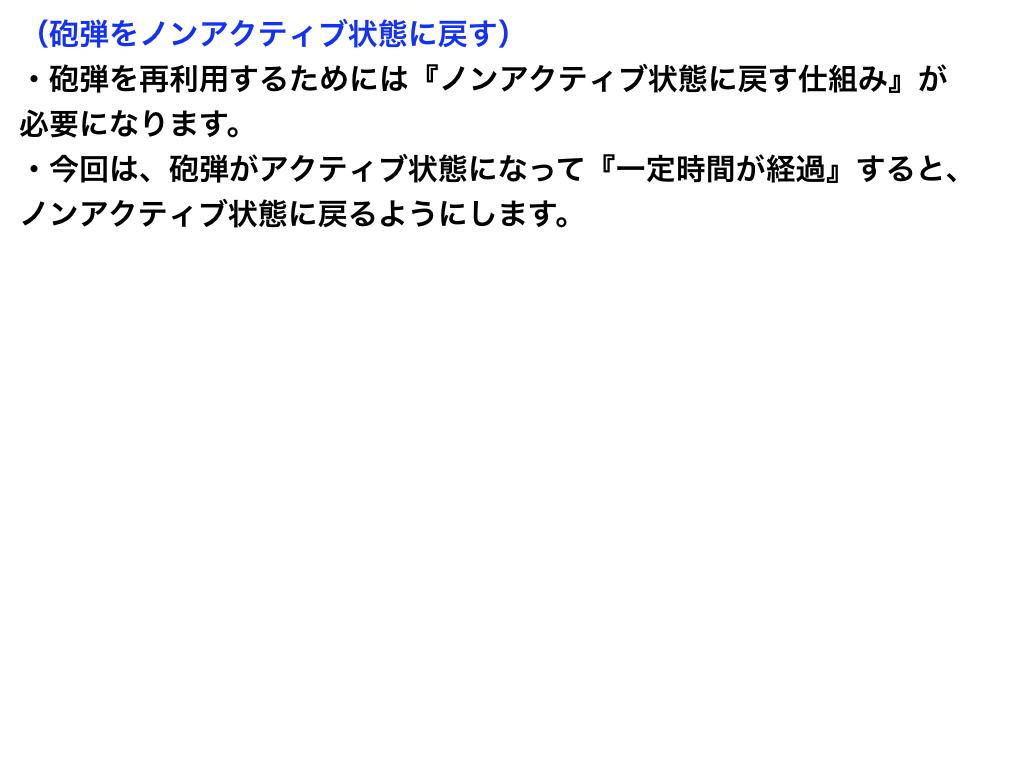
砲弾をノンアクティブに戻す
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShellLife : MonoBehaviour
{
// アクティブ状態になった場合に呼ばれる。
private void OnEnable()
{
// 砲弾の速度を0にリセットする(重要)
this.gameObject.GetComponent<Rigidbody>().velocity = Vector3.zero;
Invoke("NonActive", 3.0f);
}
void NonActive()
{
this.gameObject.SetActive(false);
}
}
砲弾の「オブジェクトプール」化