敵が前進と攻撃を繰り返すようにする(Listの活用法)
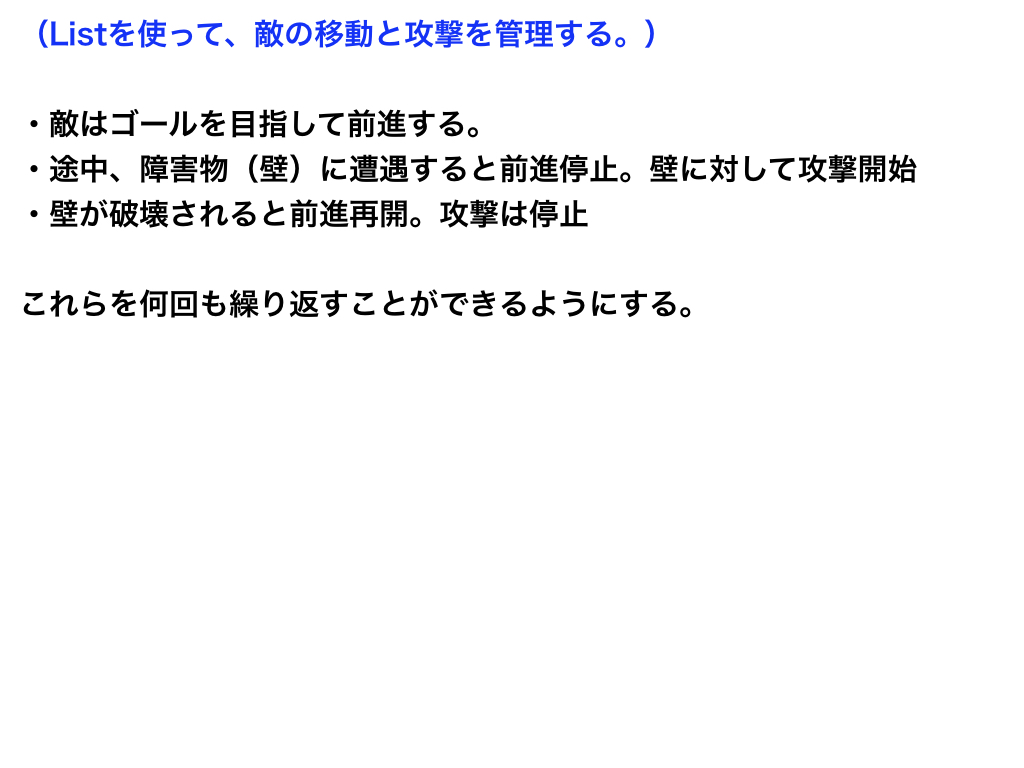
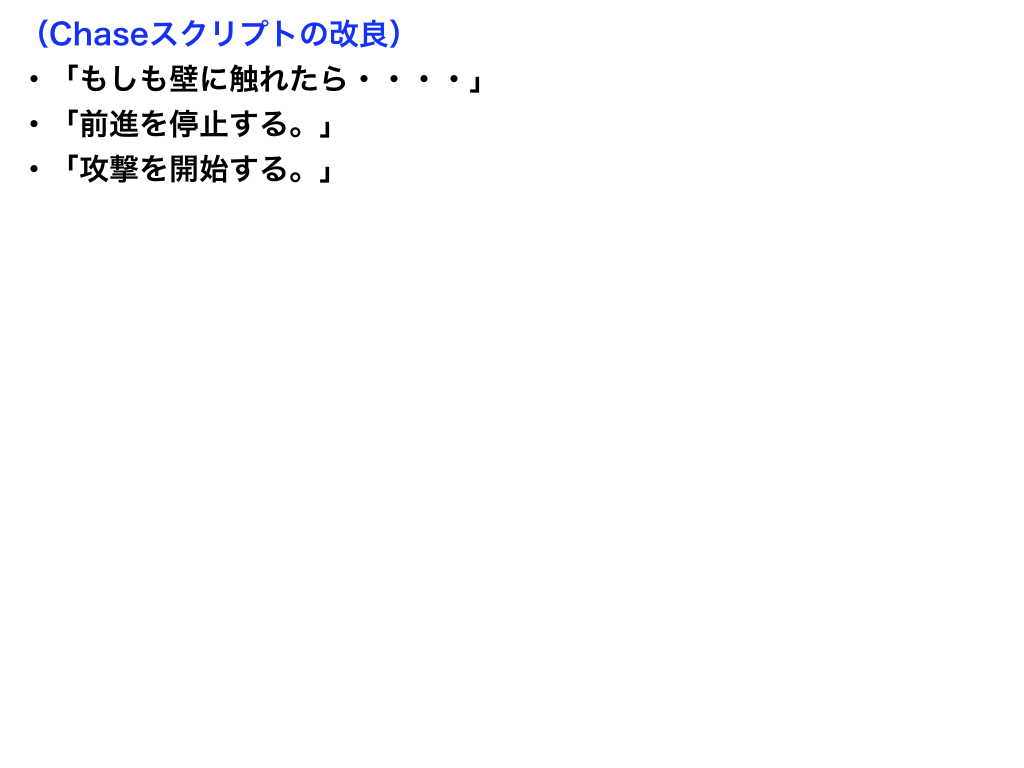
前進停止&攻撃開始
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.AI;
public class Chase : MonoBehaviour
{
private GameObject target;
private NavMeshAgent agent;
private EnemyShotShell2 shot2;
void Start()
{
target = GameObject.Find("Goal");
agent = GetComponent<NavMeshAgent>();
// ポイント
// 子供のコンポーネントを取得する。
shot2 = transform.GetComponentInChildren<EnemyShotShell2>();
}
void Update()
{
if (target != null)
{
agent.destination = target.transform.position;
}
}
// ★前進停止の仕組み
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.CompareTag("Wall"))
{
// 立ち止まる。
agent.isStopped = true;
// 攻撃を開始する。
shot2.enabled = true;
}
}
}
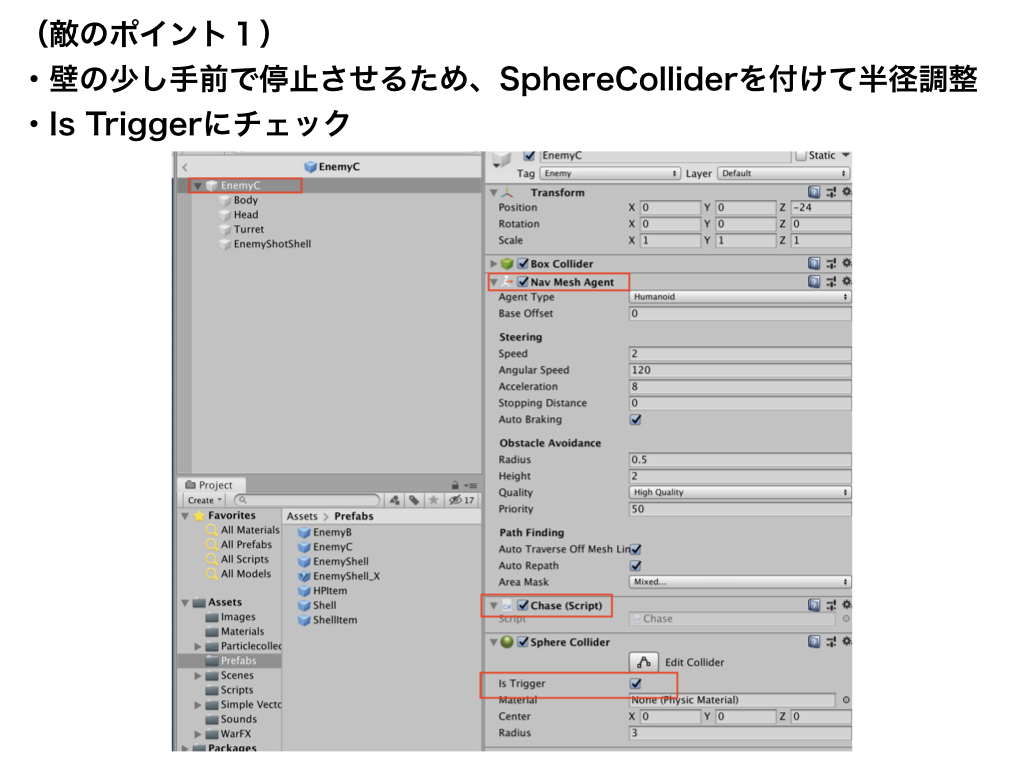
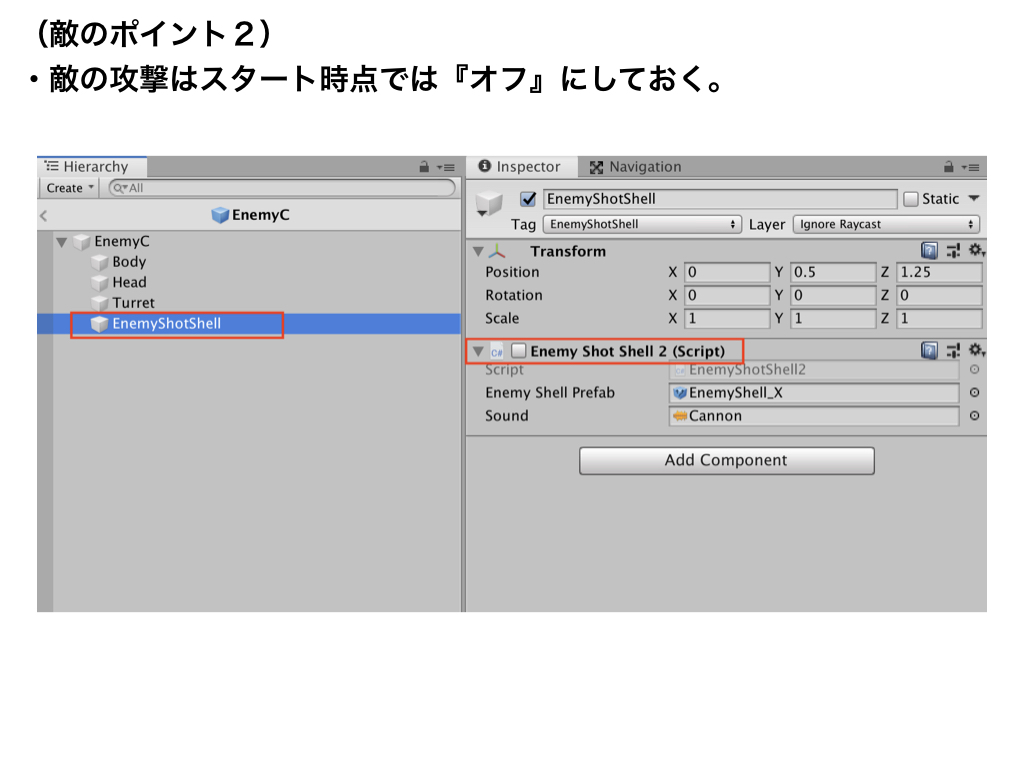
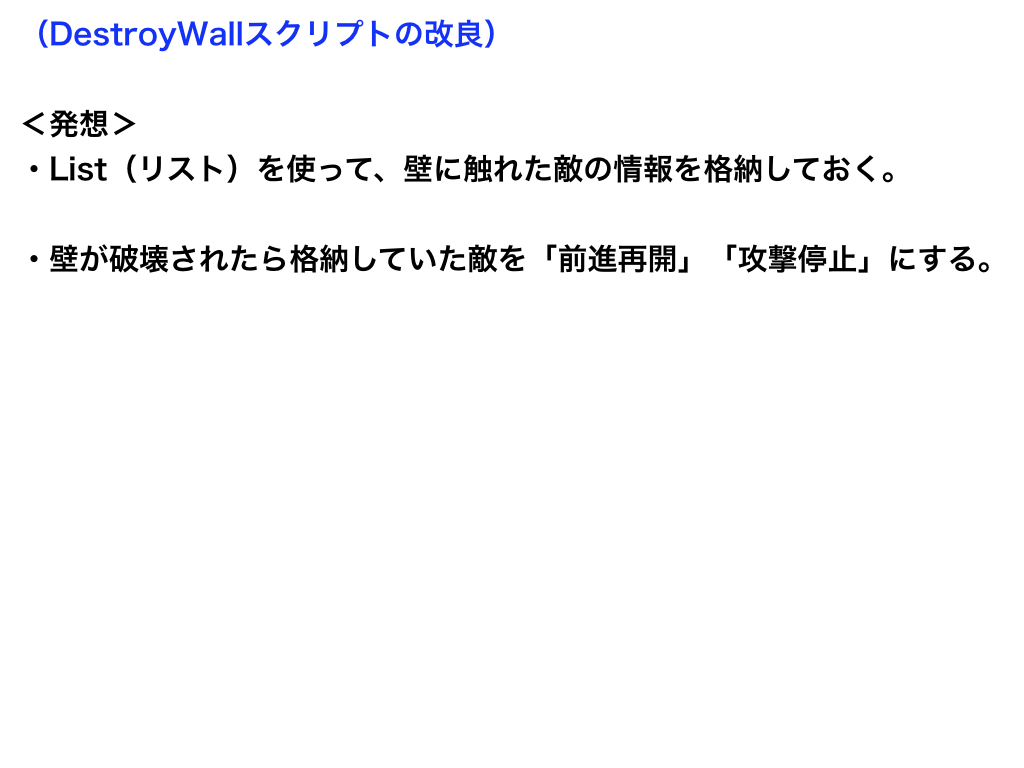
リストを使って複数の敵を管理する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.AI;
public class DestroyWall : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip sound;
private int HP = 20;
// ★リスト
private List<GameObject> enemyList = new List<GameObject>();
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Enemy")
{
// ★リスト
enemyList.Add(other.gameObject);
}
if (other.gameObject.CompareTag("EnemyShell"))
{
HP -= 1;
other.gameObject.SetActive(false);
AudioSource.PlayClipAtPoint(sound, transform.position);
GameObject effect = Instantiate(effectPrefab, other.transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
if(HP < 1)
{
this.gameObject.SetActive(false);
for (int i = 0; i < enemyList.Count; i++)
{
// ★リスト
// 前進を再開する。
enemyList[i].GetComponent<NavMeshAgent>().isStopped = false;
// 攻撃を停止する。
enemyList[i].GetComponentInChildren<EnemyShotShell2>().enabled = false;
}
}
}
}
}
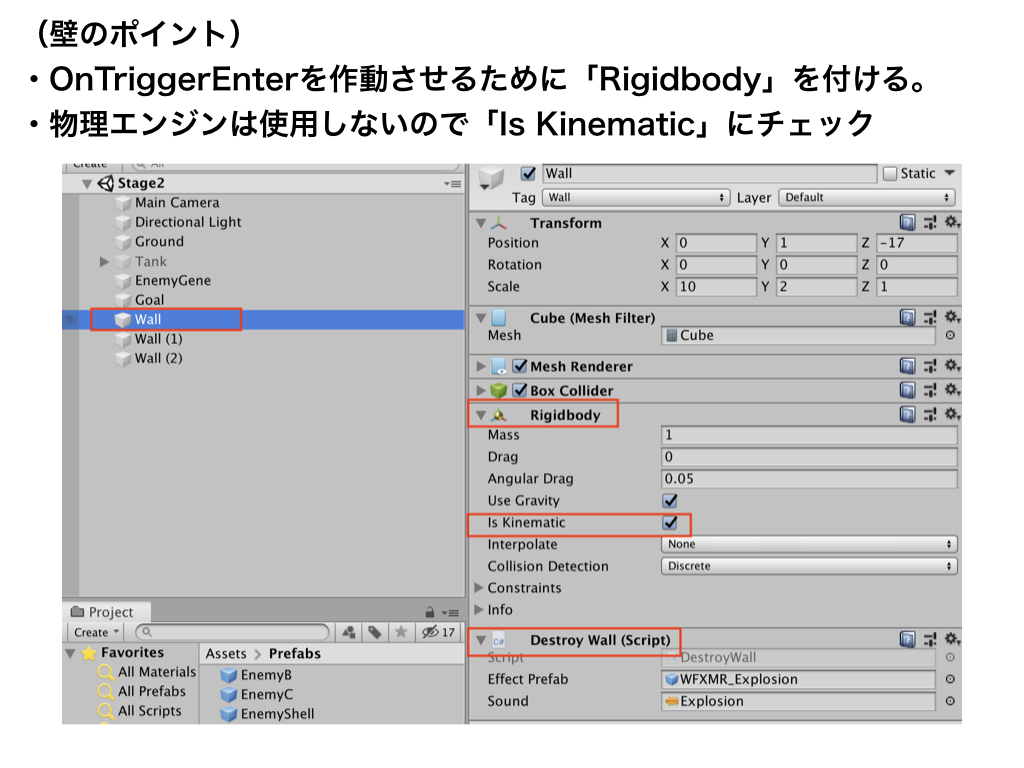
Unity Code Memo
他のコースを見る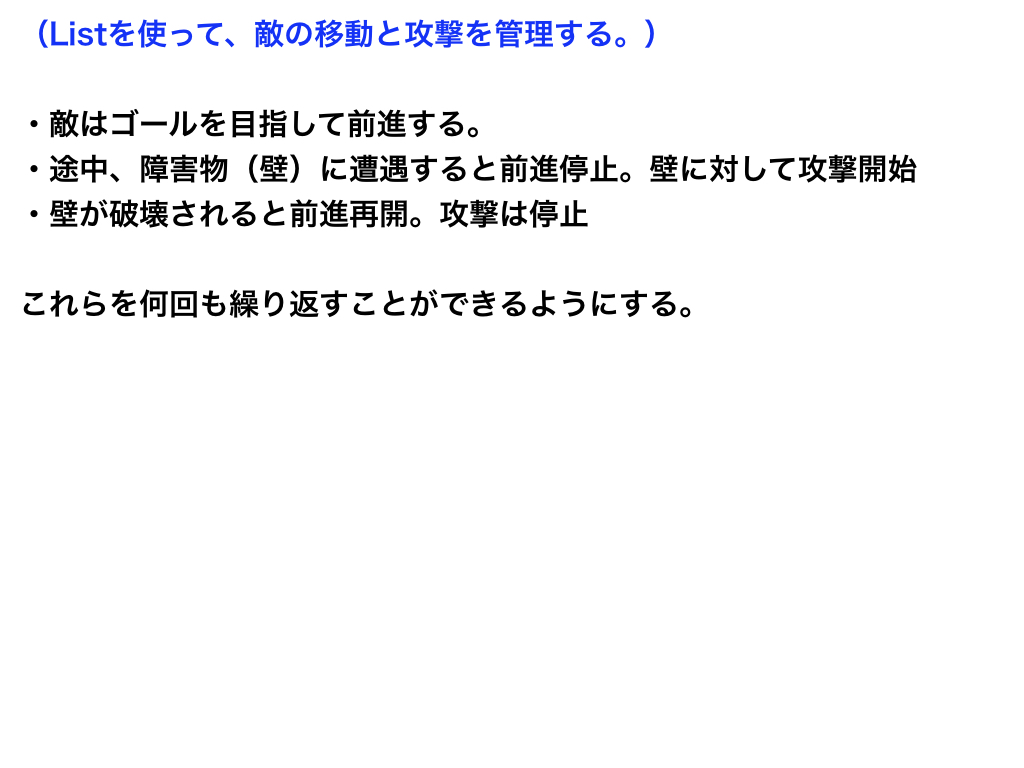
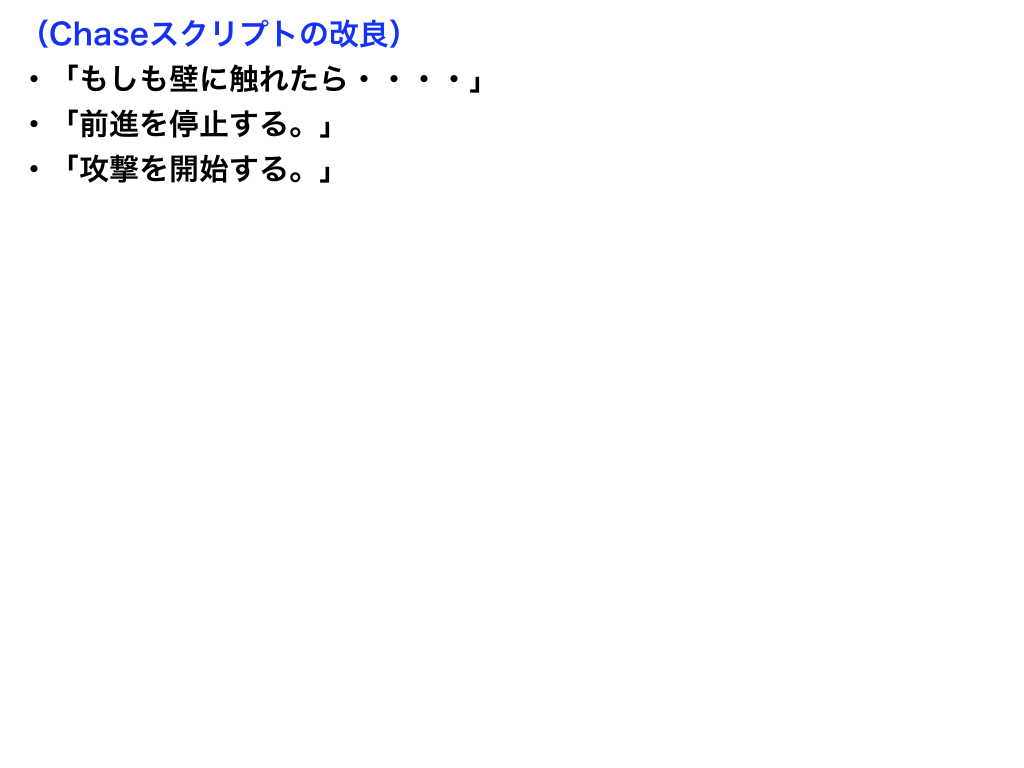
前進停止&攻撃開始
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.AI;
public class Chase : MonoBehaviour
{
private GameObject target;
private NavMeshAgent agent;
private EnemyShotShell2 shot2;
void Start()
{
target = GameObject.Find("Goal");
agent = GetComponent<NavMeshAgent>();
// ポイント
// 子供のコンポーネントを取得する。
shot2 = transform.GetComponentInChildren<EnemyShotShell2>();
}
void Update()
{
if (target != null)
{
agent.destination = target.transform.position;
}
}
// ★前進停止の仕組み
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.CompareTag("Wall"))
{
// 立ち止まる。
agent.isStopped = true;
// 攻撃を開始する。
shot2.enabled = true;
}
}
}
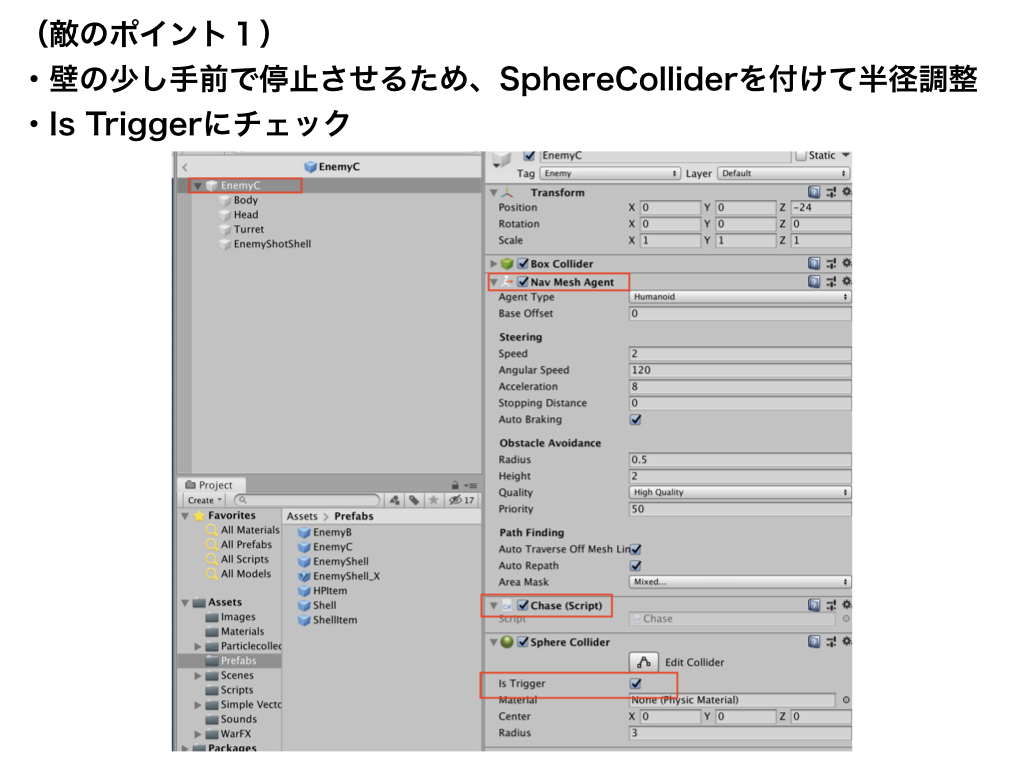
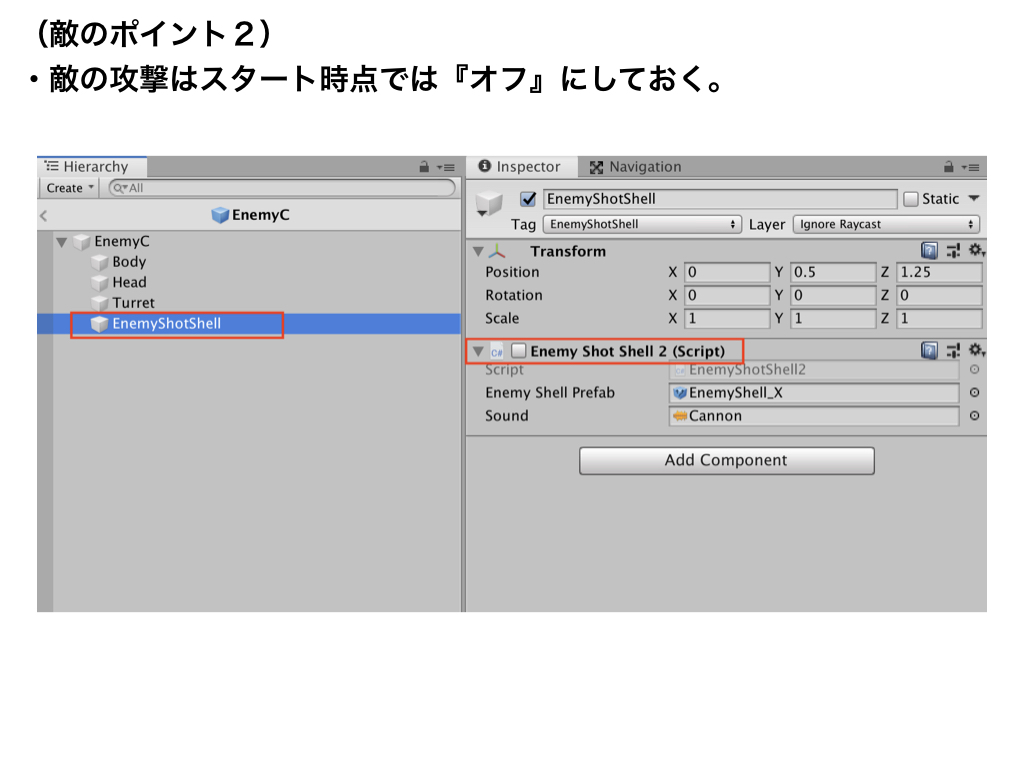
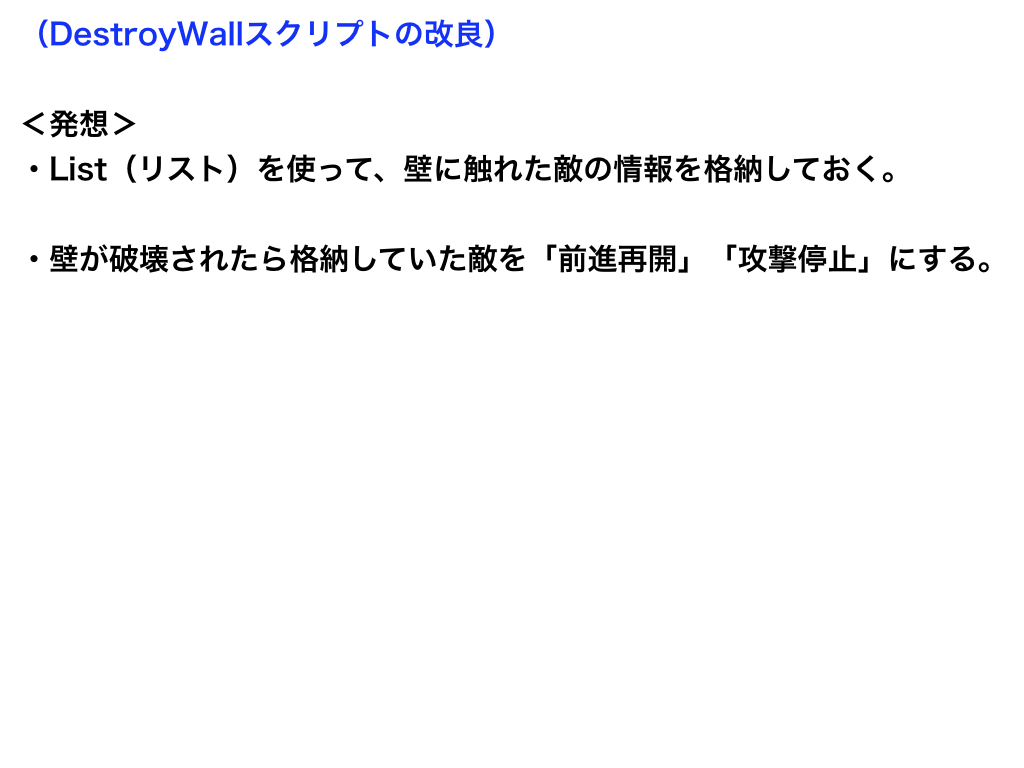
リストを使って複数の敵を管理する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.AI;
public class DestroyWall : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip sound;
private int HP = 20;
// ★リスト
private List<GameObject> enemyList = new List<GameObject>();
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Enemy")
{
// ★リスト
enemyList.Add(other.gameObject);
}
if (other.gameObject.CompareTag("EnemyShell"))
{
HP -= 1;
other.gameObject.SetActive(false);
AudioSource.PlayClipAtPoint(sound, transform.position);
GameObject effect = Instantiate(effectPrefab, other.transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
if(HP < 1)
{
this.gameObject.SetActive(false);
for (int i = 0; i < enemyList.Count; i++)
{
// ★リスト
// 前進を再開する。
enemyList[i].GetComponent<NavMeshAgent>().isStopped = false;
// 攻撃を停止する。
enemyList[i].GetComponentInChildren<EnemyShotShell2>().enabled = false;
}
}
}
}
}
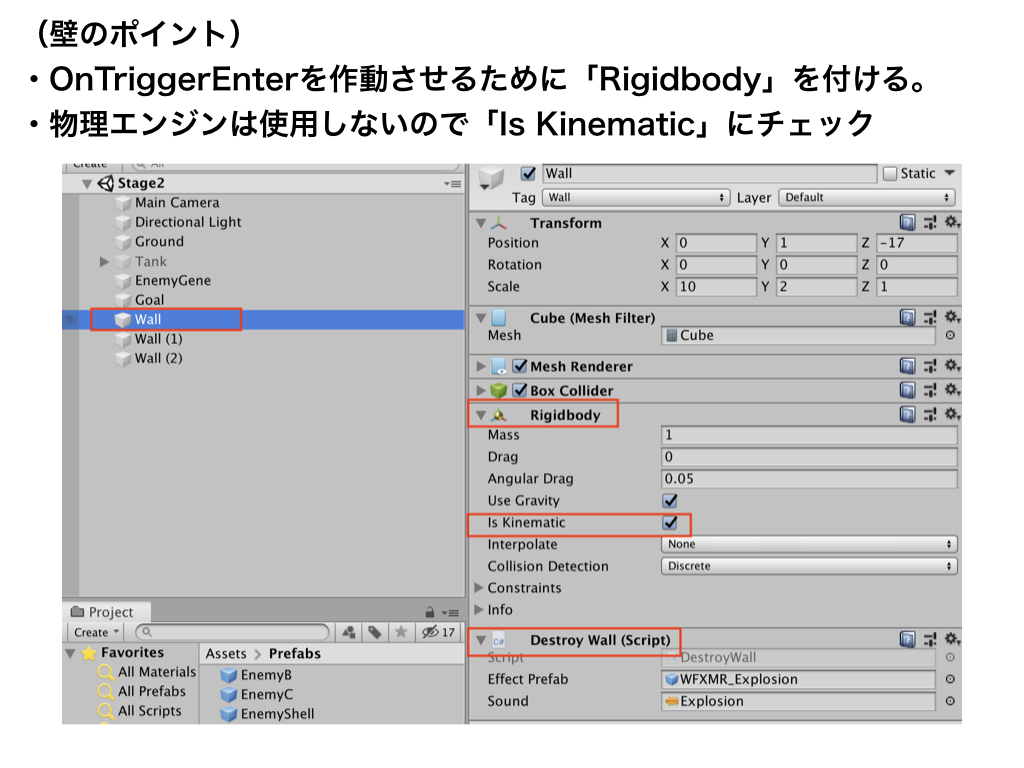
敵が前進と攻撃を繰り返すようにする(Listの活用法)