ステージの拡張1(ドアのオープン&クローズ)
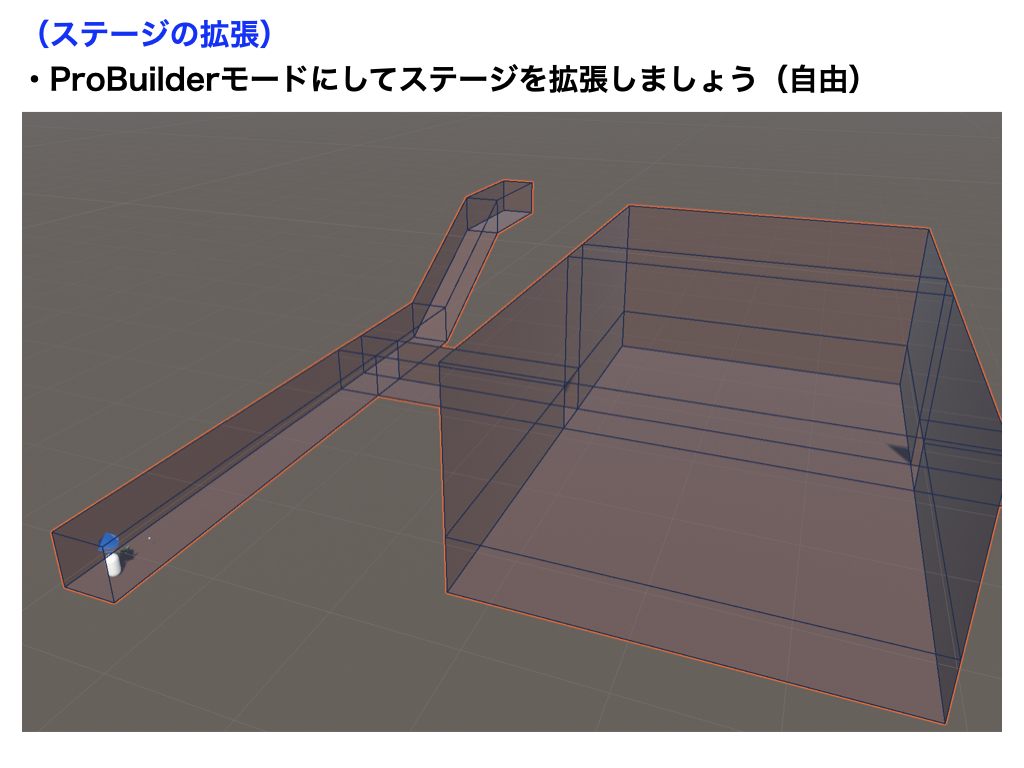
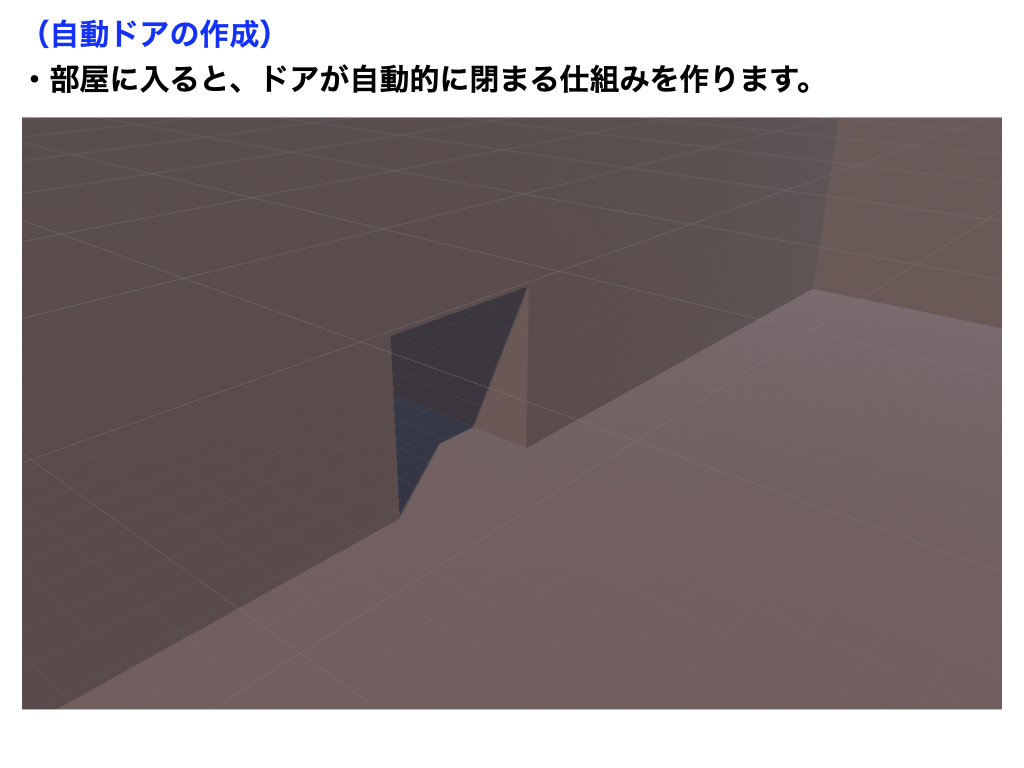
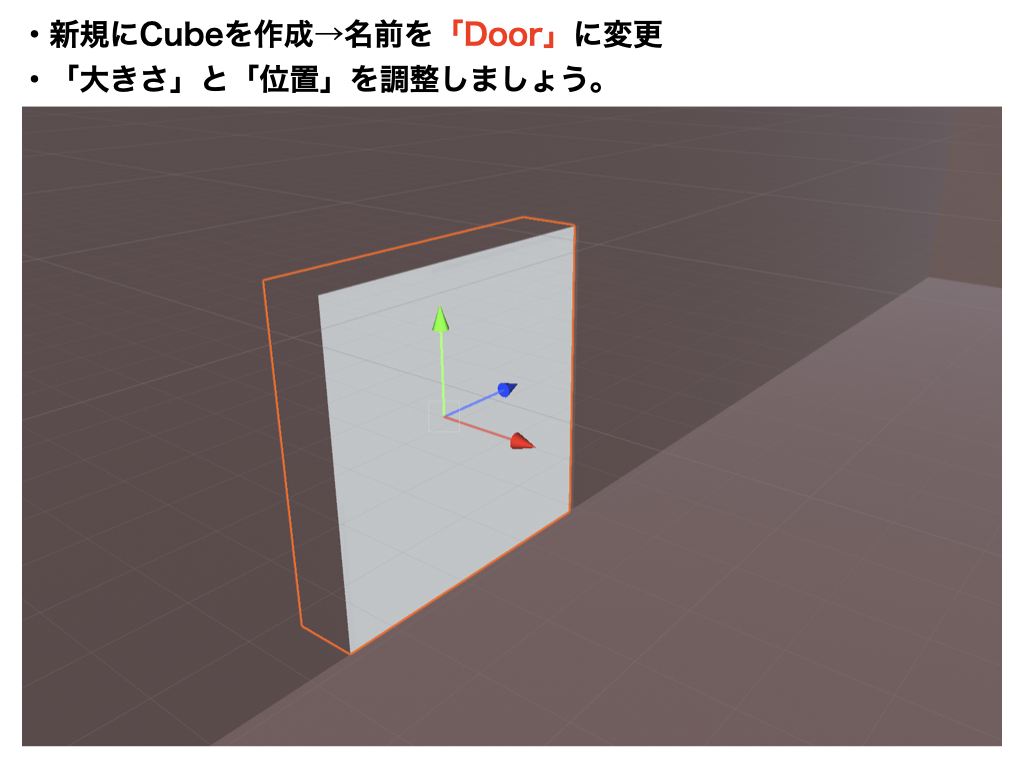
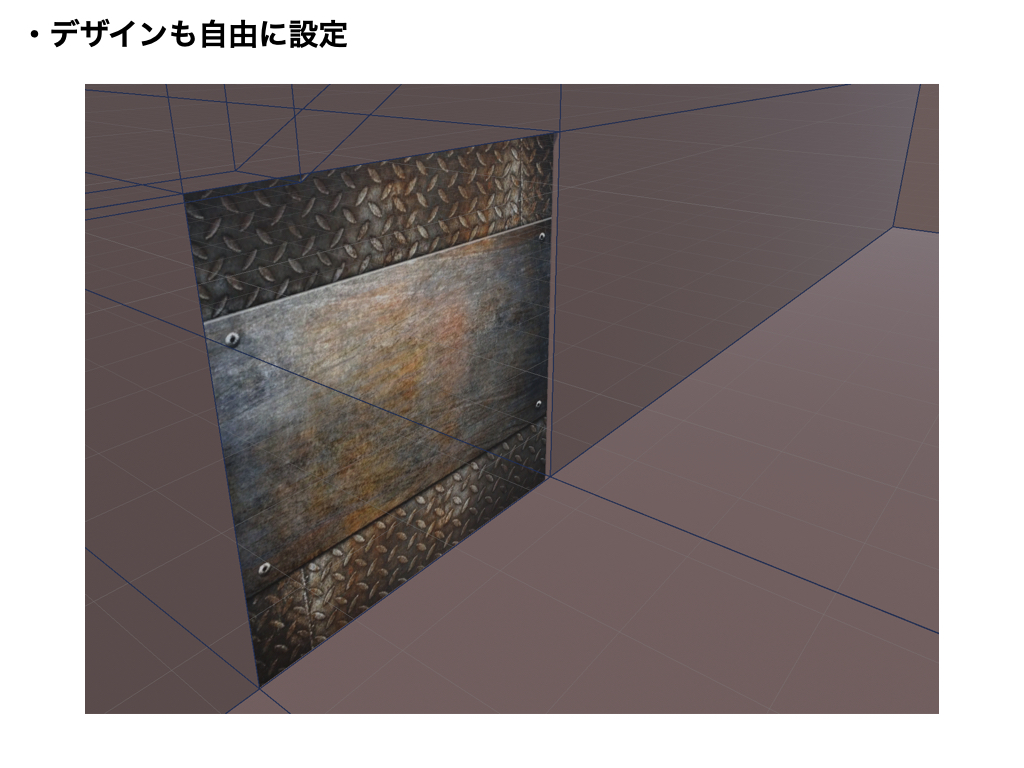
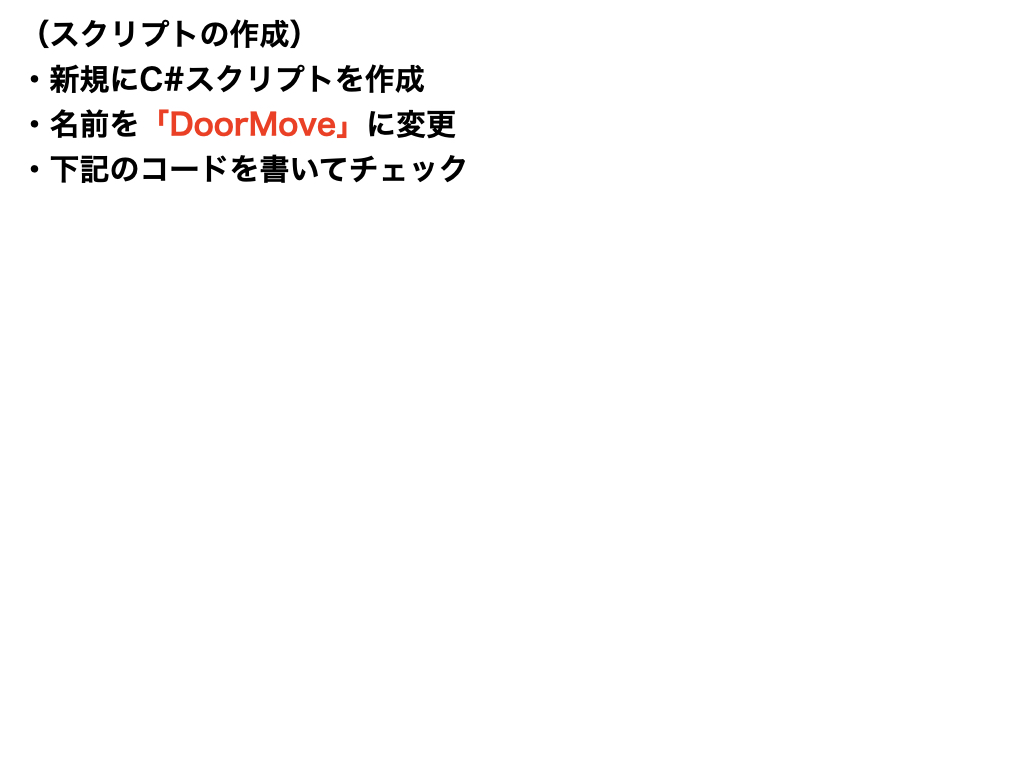
ドアを動かす
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class DoorMove : MonoBehaviour
{
private Vector3 initialPos;
public float goalPos;
void Start()
{
initialPos = transform.position;
}
void Update()
{
// 今回はY方向に動くドアを想定
transform.position = Vector3.MoveTowards(transform.position, new Vector3(initialPos.x, goalPos, initialPos.z), Time.deltaTime);
}
}
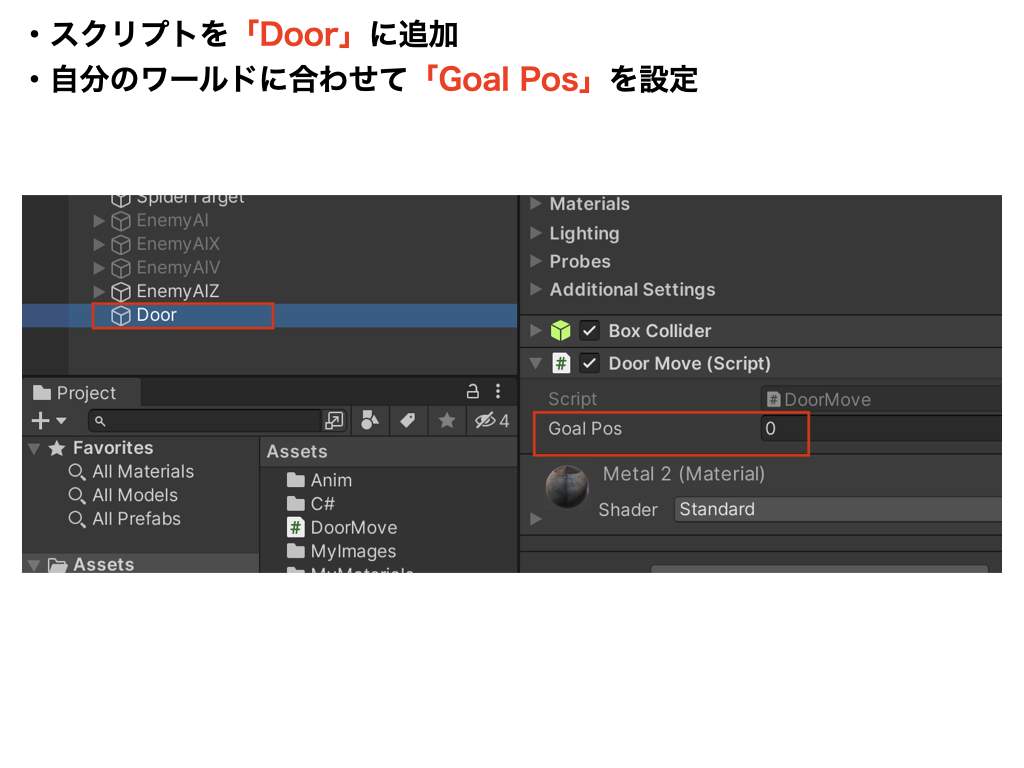
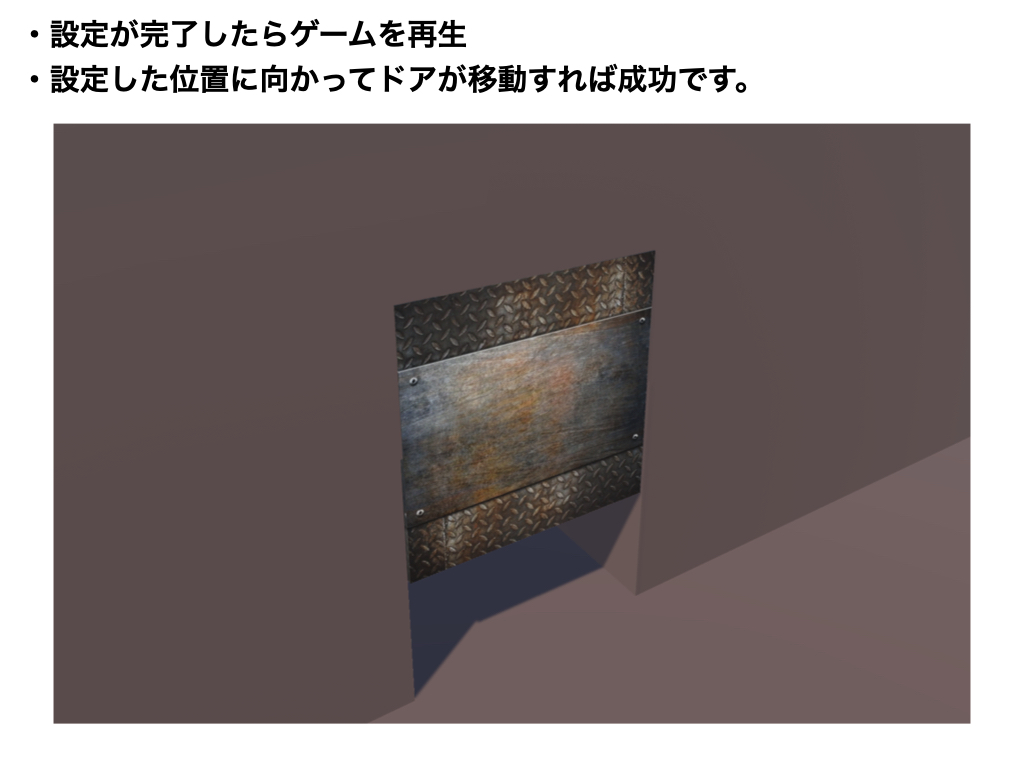
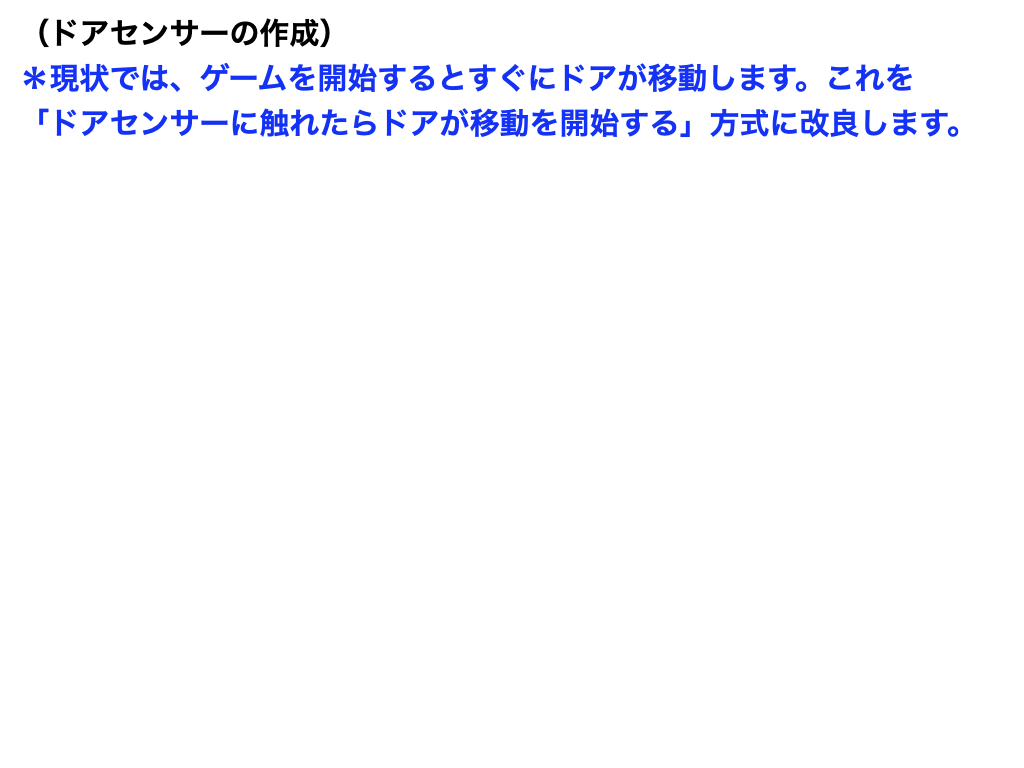
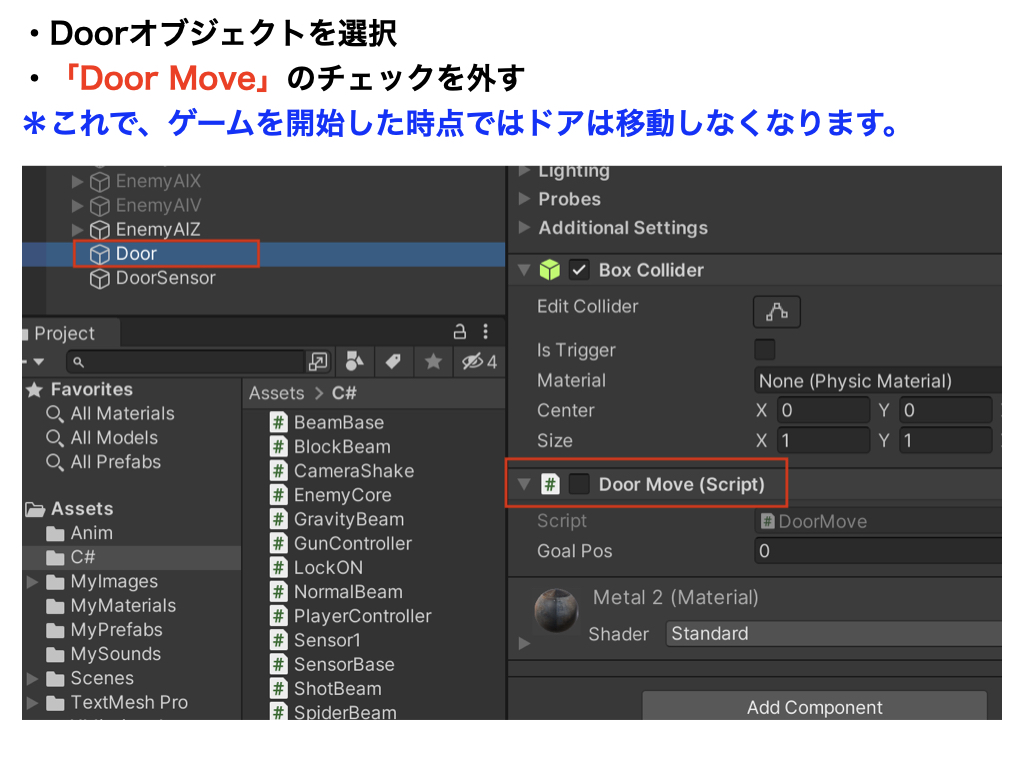
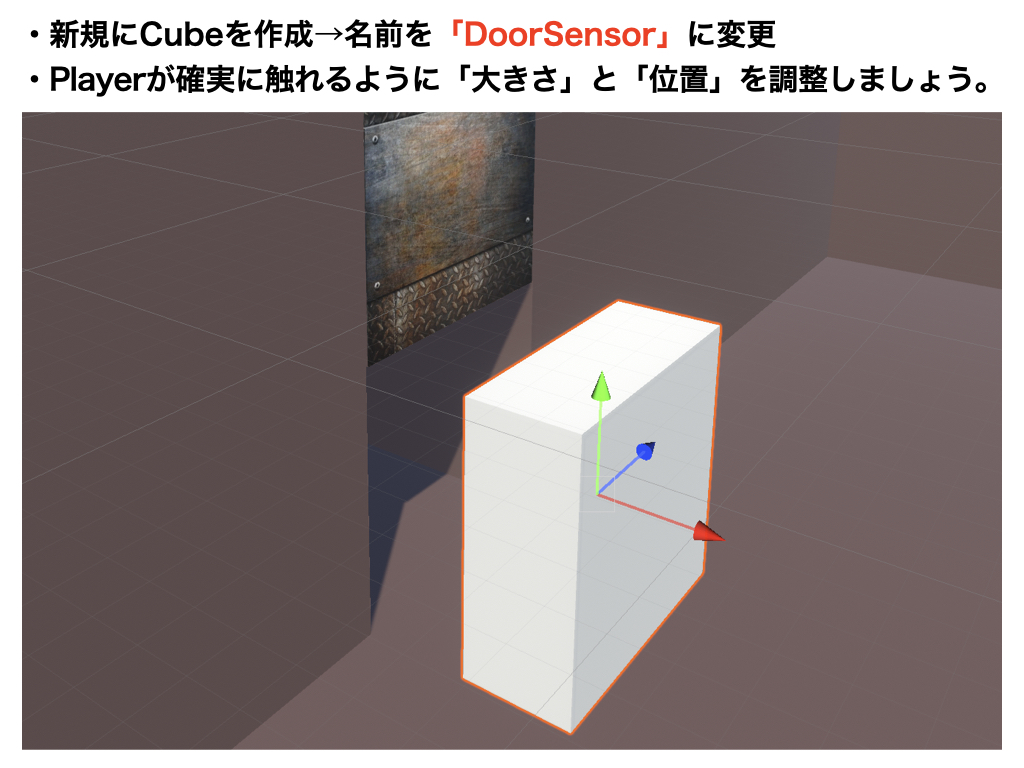
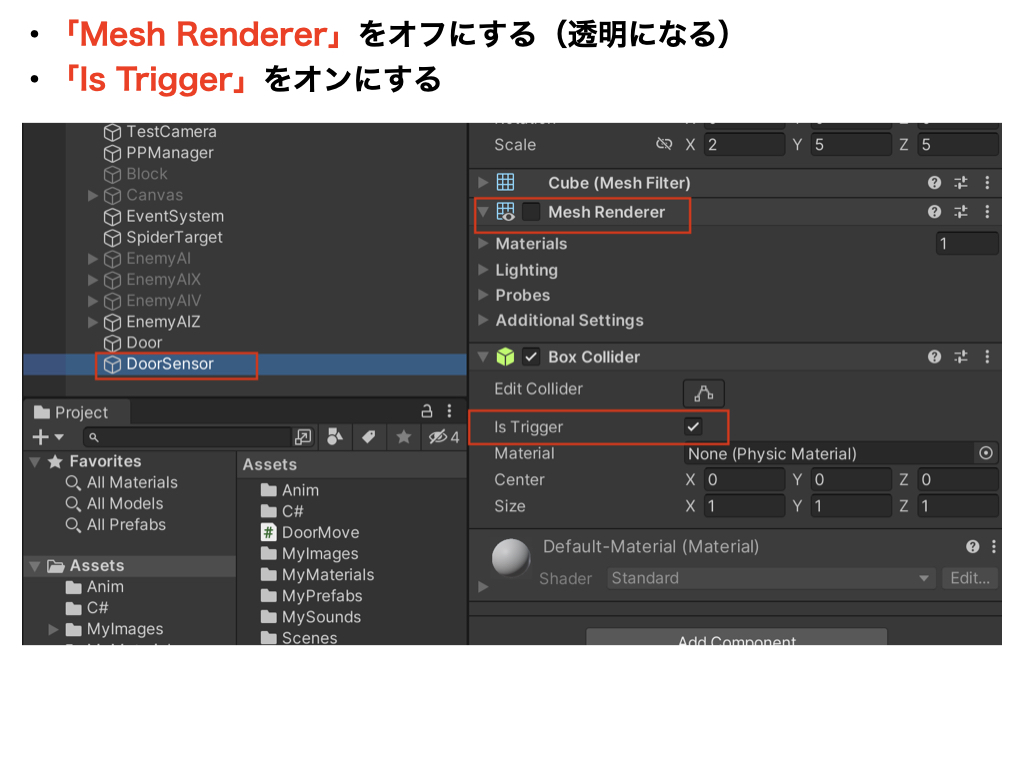
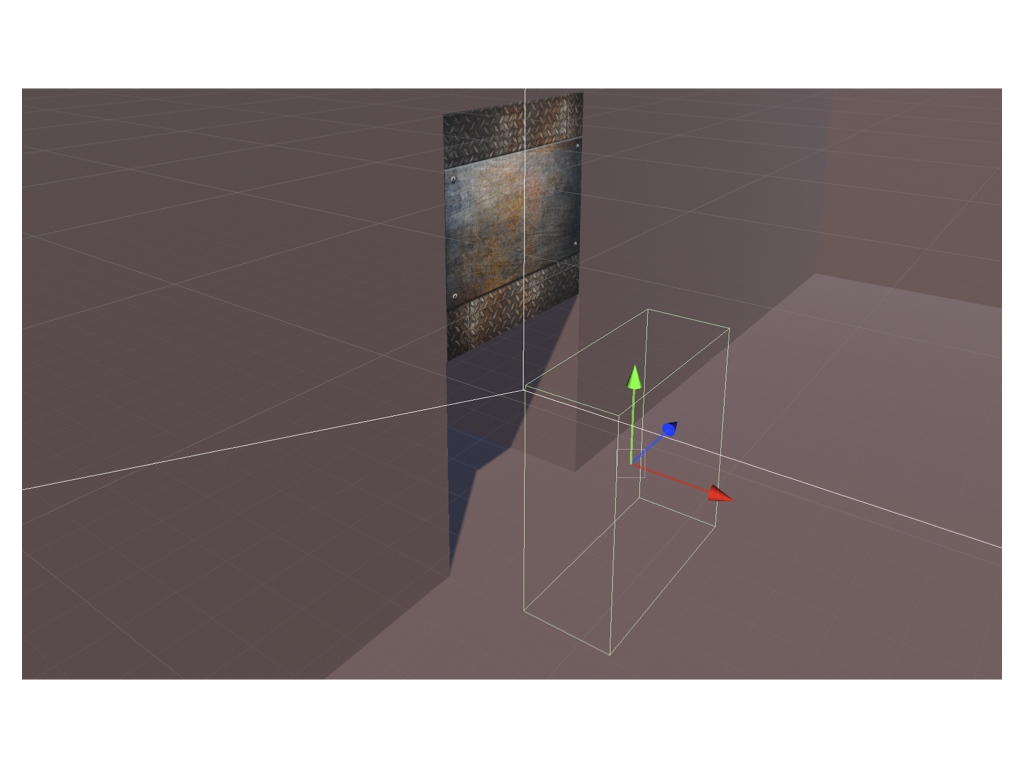
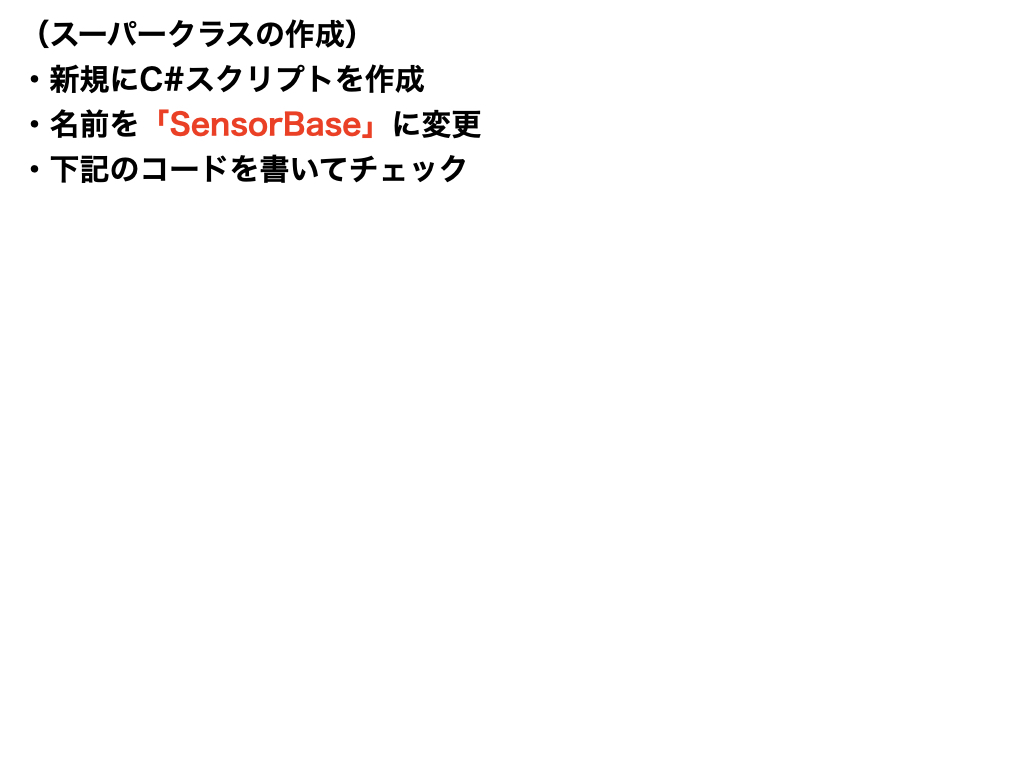
センサーのスーパークラス
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// 「abstract」キーワードの追加
public abstract class SensorBase : MonoBehaviour
{
public GameObject target;
public AudioClip sound;
public virtual void OnTriggerEnter(Collider other)
{
this.gameObject.SetActive(false); // センサーの使用は1回のみ
}
}
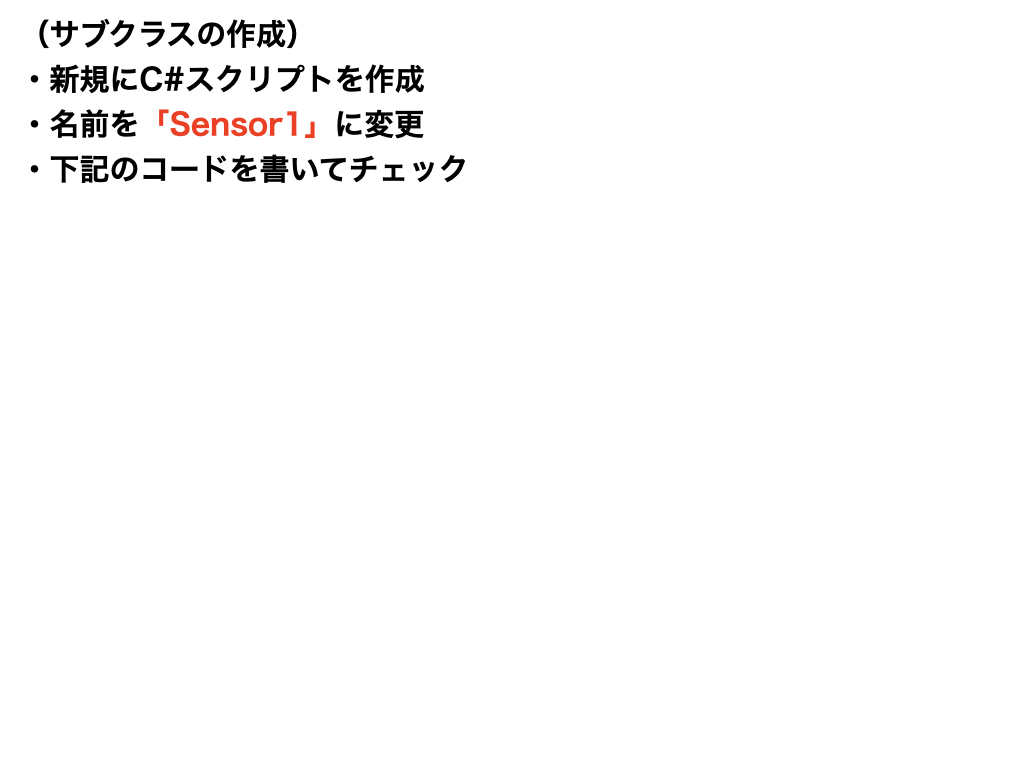
センサーのサブクラス
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Sensor1 : SensorBase // 変更
{
public override void OnTriggerEnter(Collider other)
{
base.OnTriggerEnter(other);
if (other.CompareTag("Player"))
{
AudioSource.PlayClipAtPoint(sound, Camera.main.transform.position);
target.GetComponent<DoorMove>().enabled = true;
}
}
}
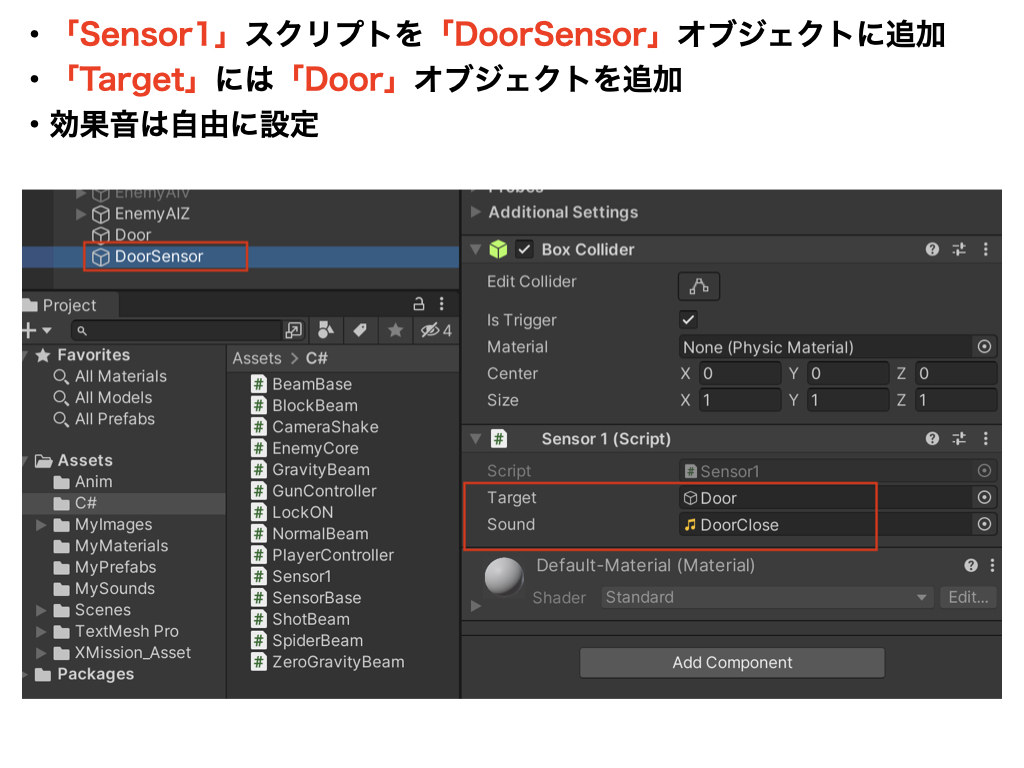
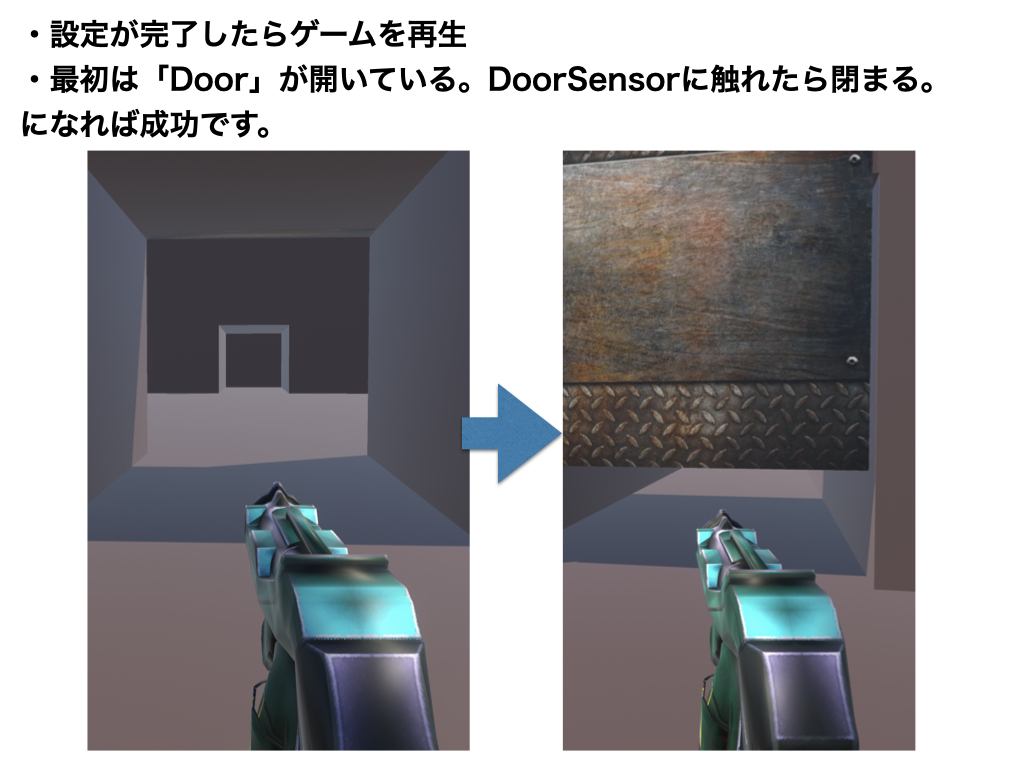
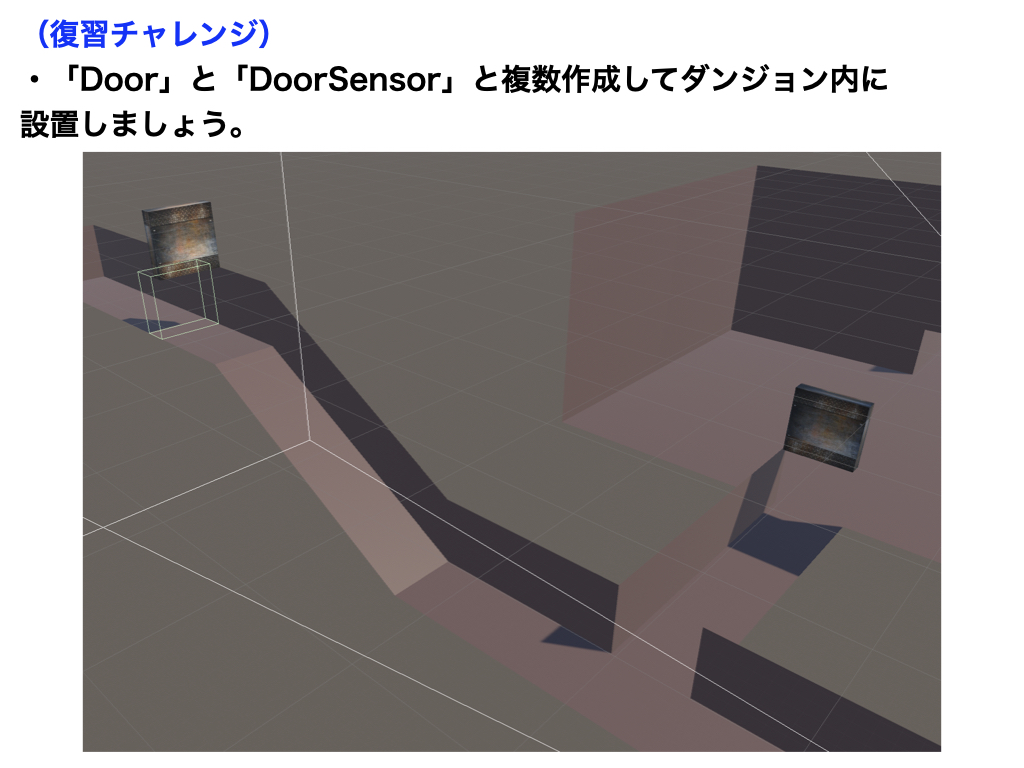
【2021版】X_Mission(全34回)
他のコースを見る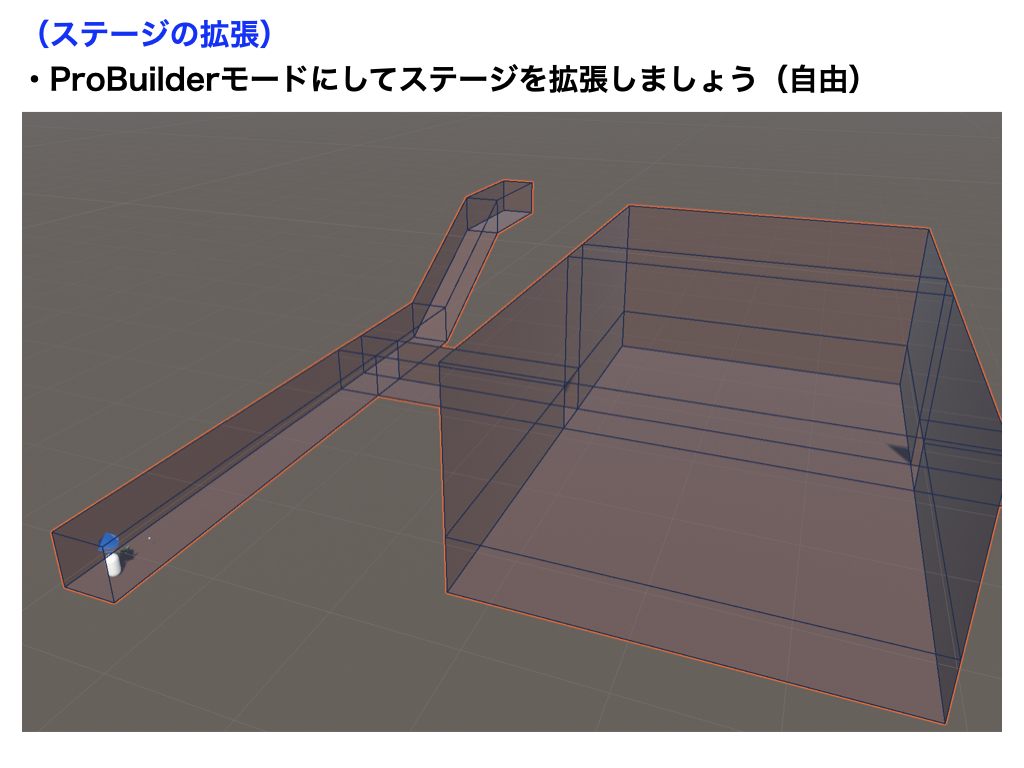
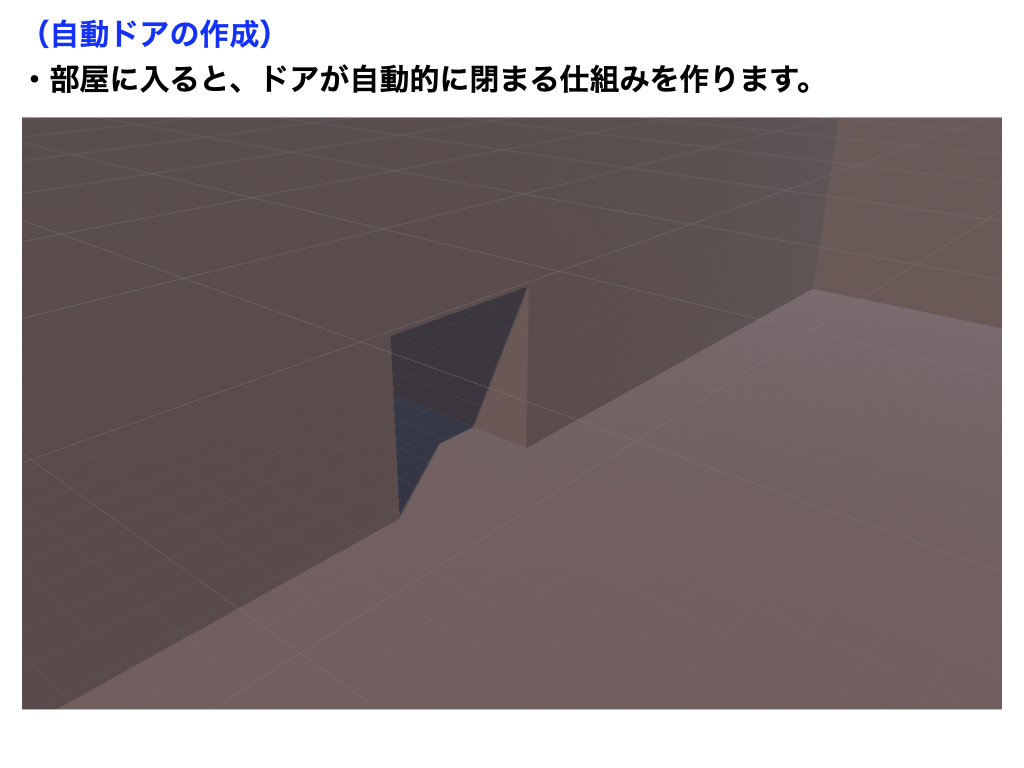
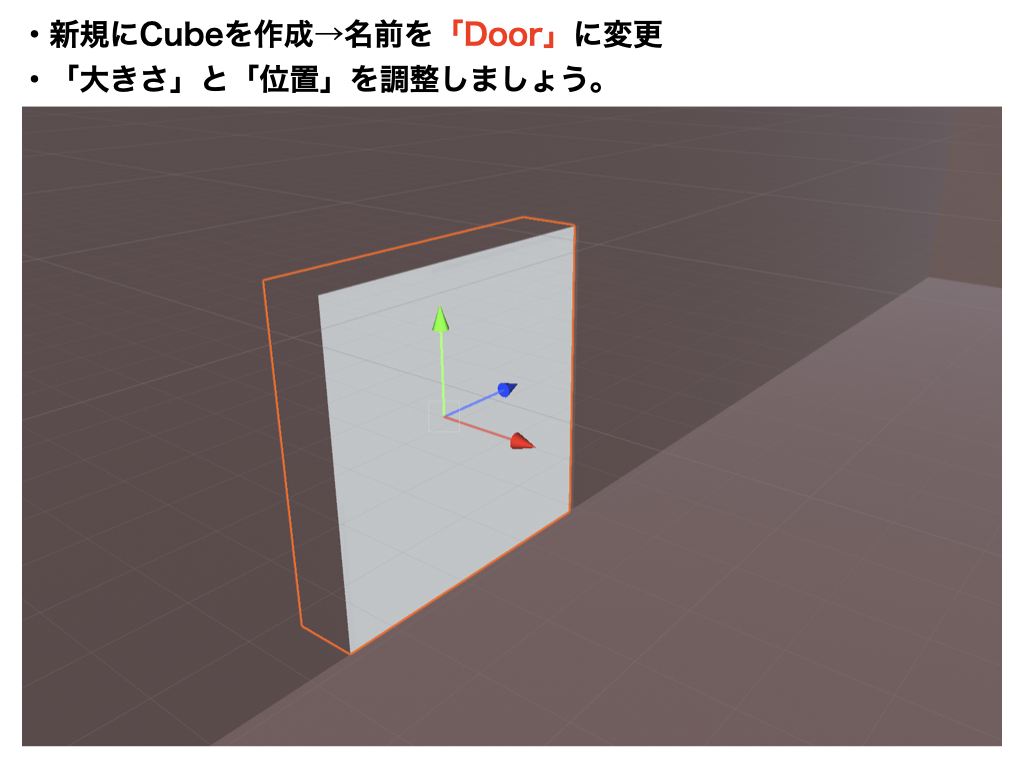
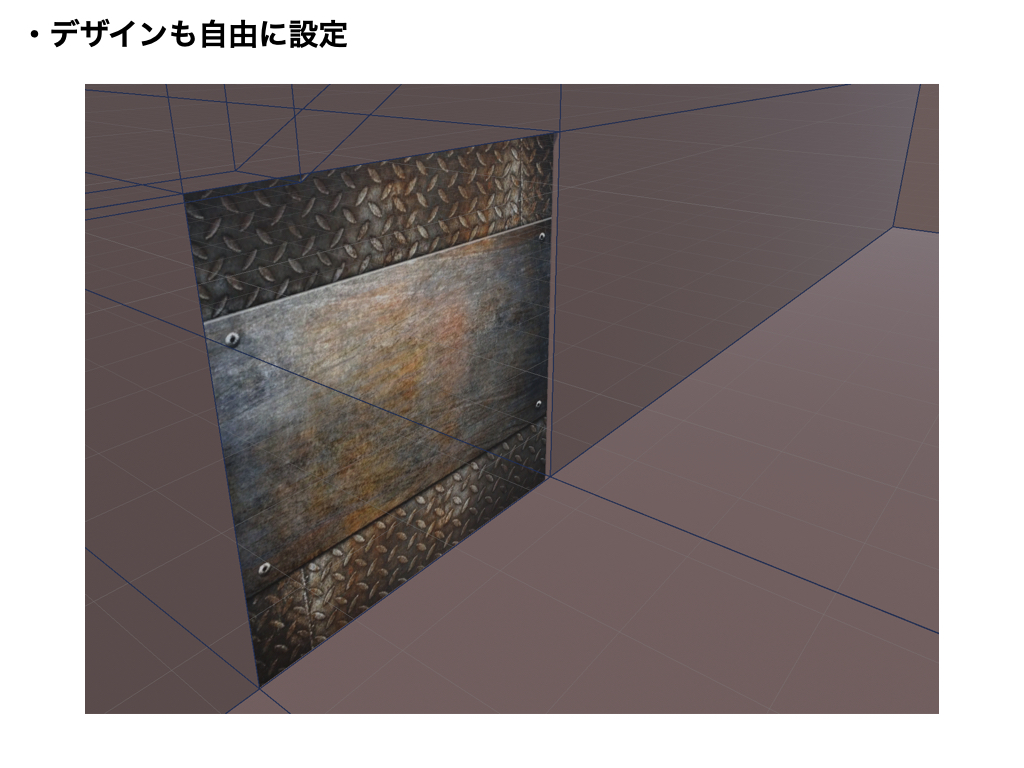
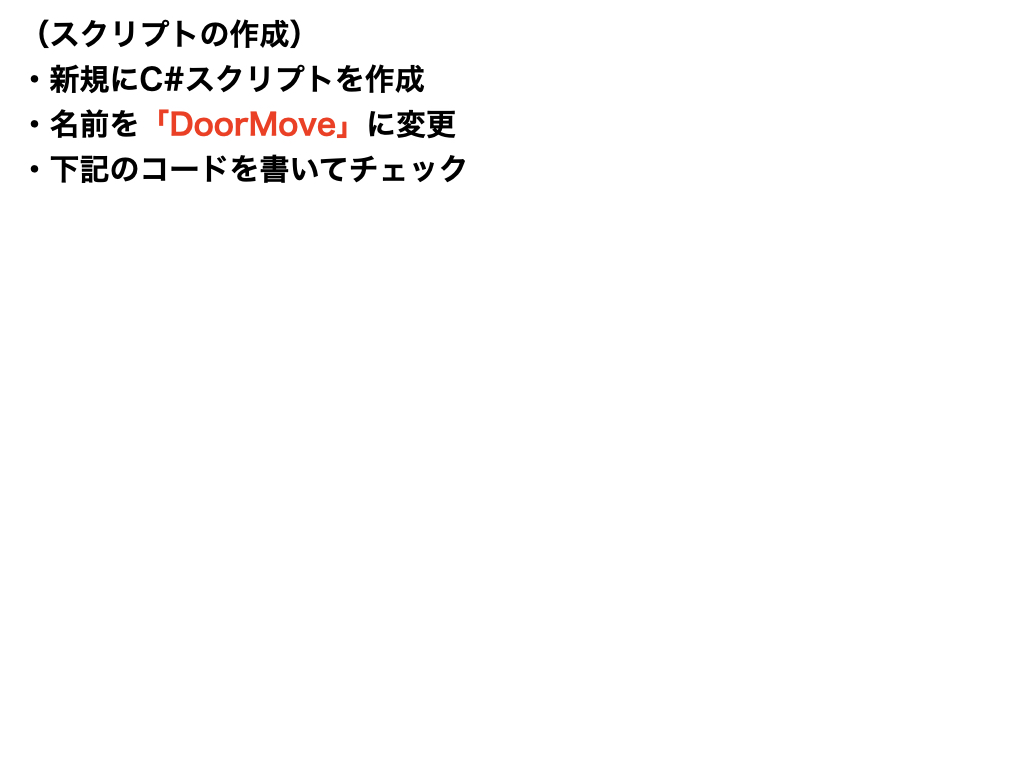
ドアを動かす
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class DoorMove : MonoBehaviour
{
private Vector3 initialPos;
public float goalPos;
void Start()
{
initialPos = transform.position;
}
void Update()
{
// 今回はY方向に動くドアを想定
transform.position = Vector3.MoveTowards(transform.position, new Vector3(initialPos.x, goalPos, initialPos.z), Time.deltaTime);
}
}
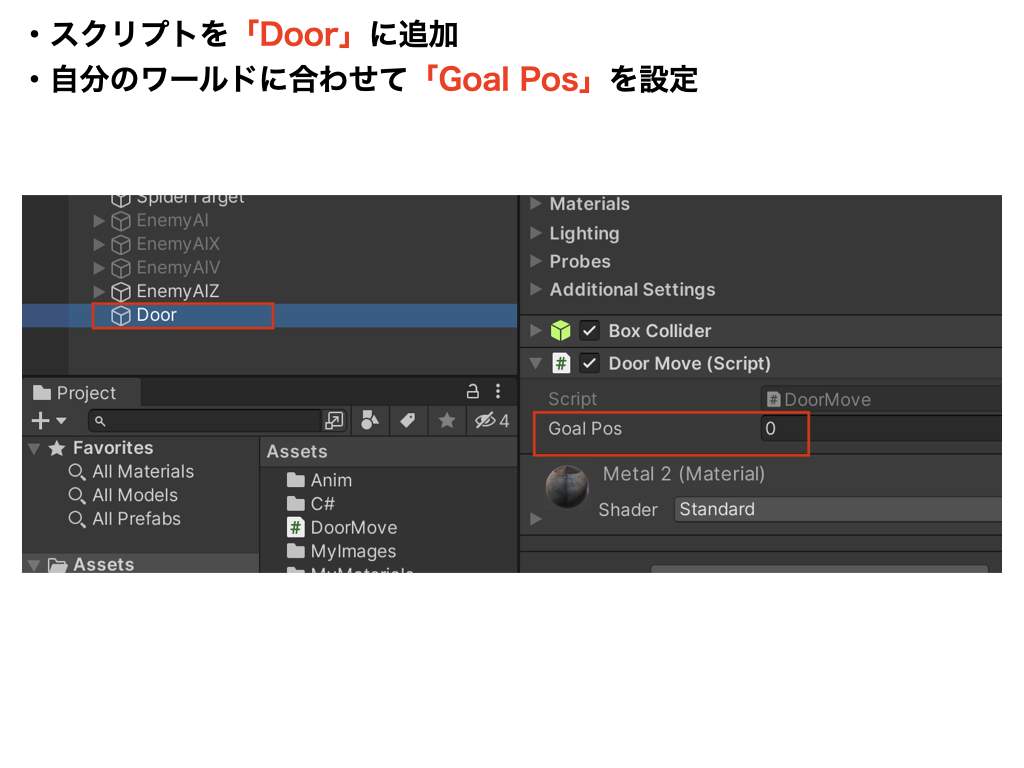
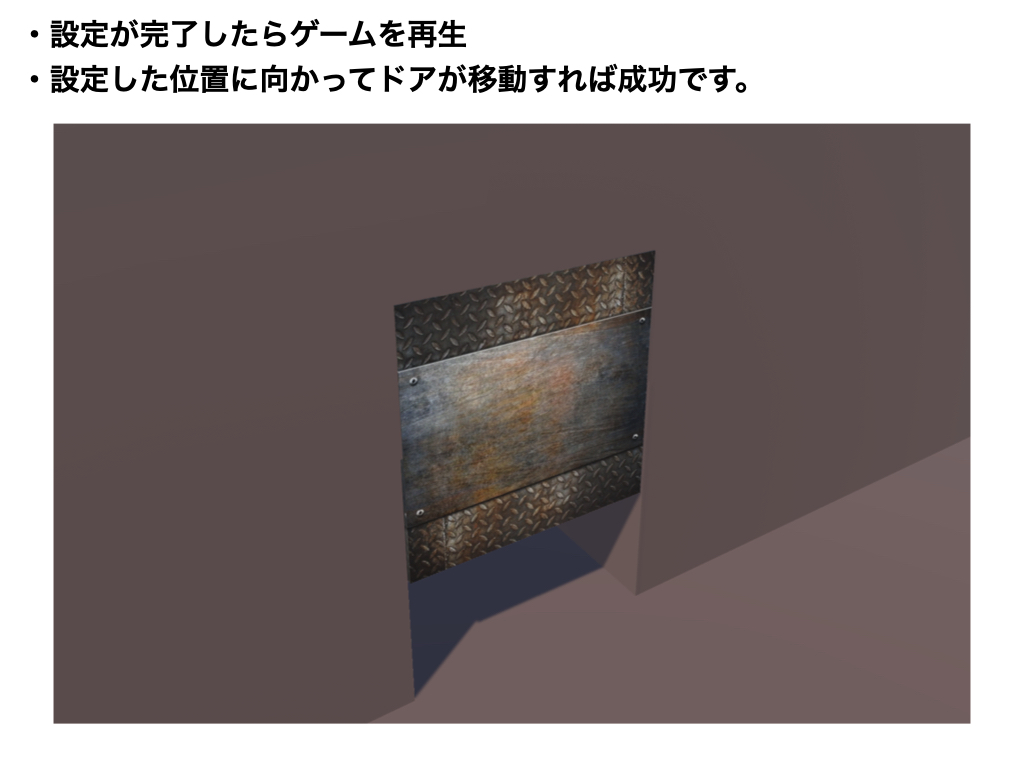
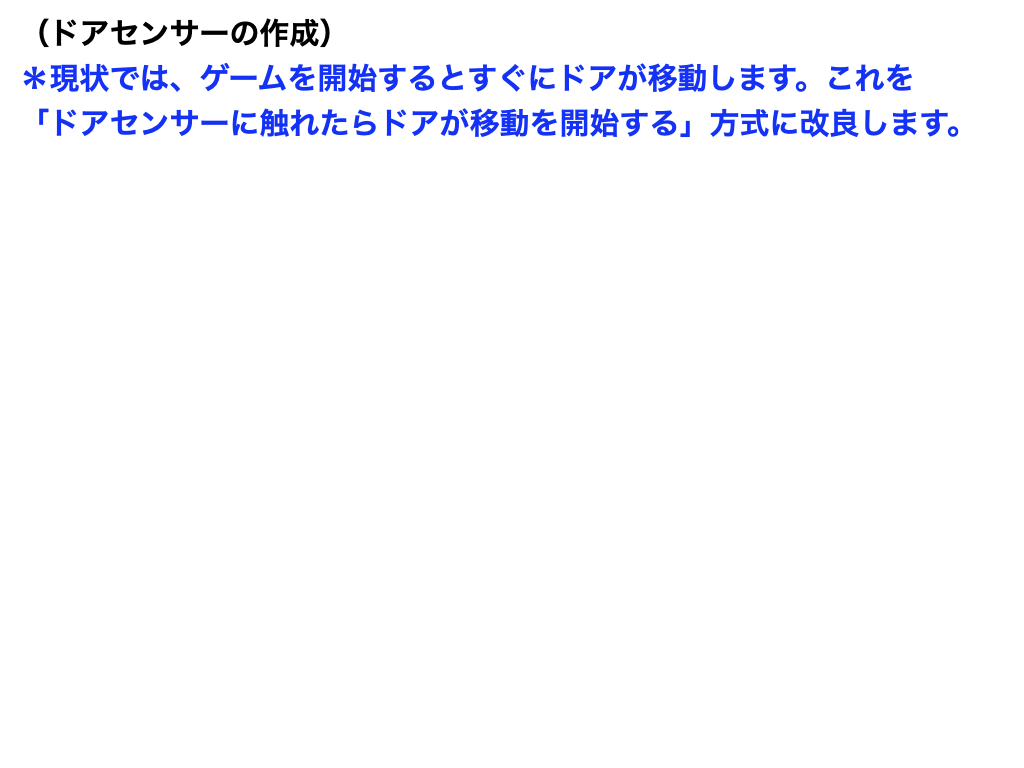
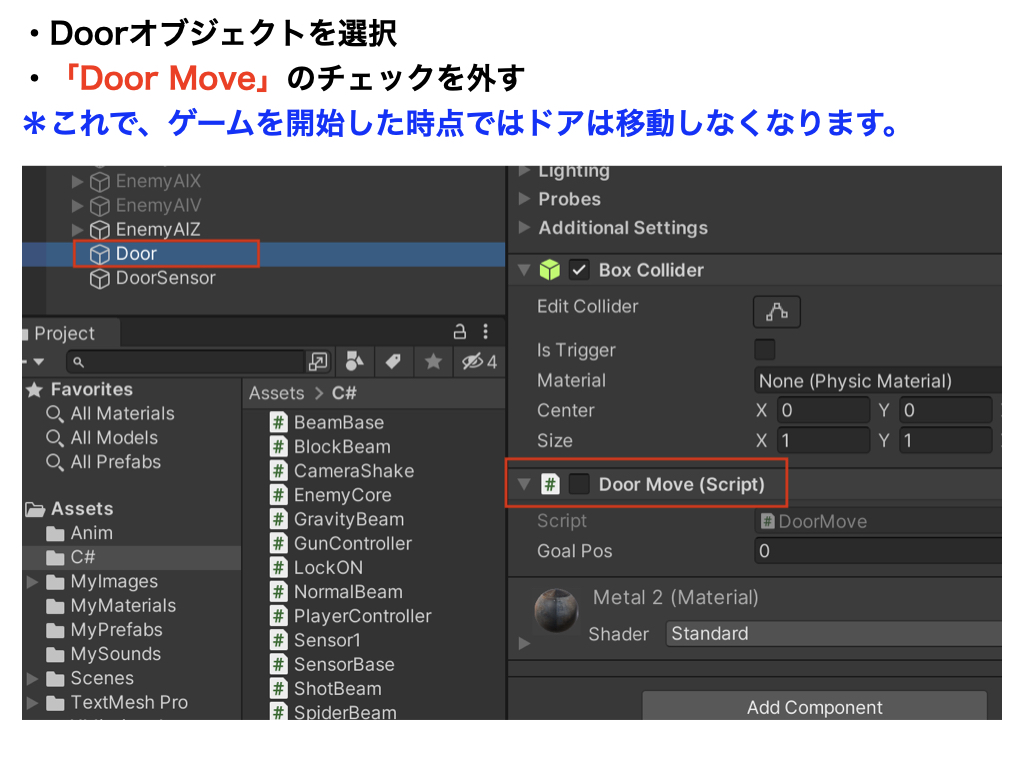
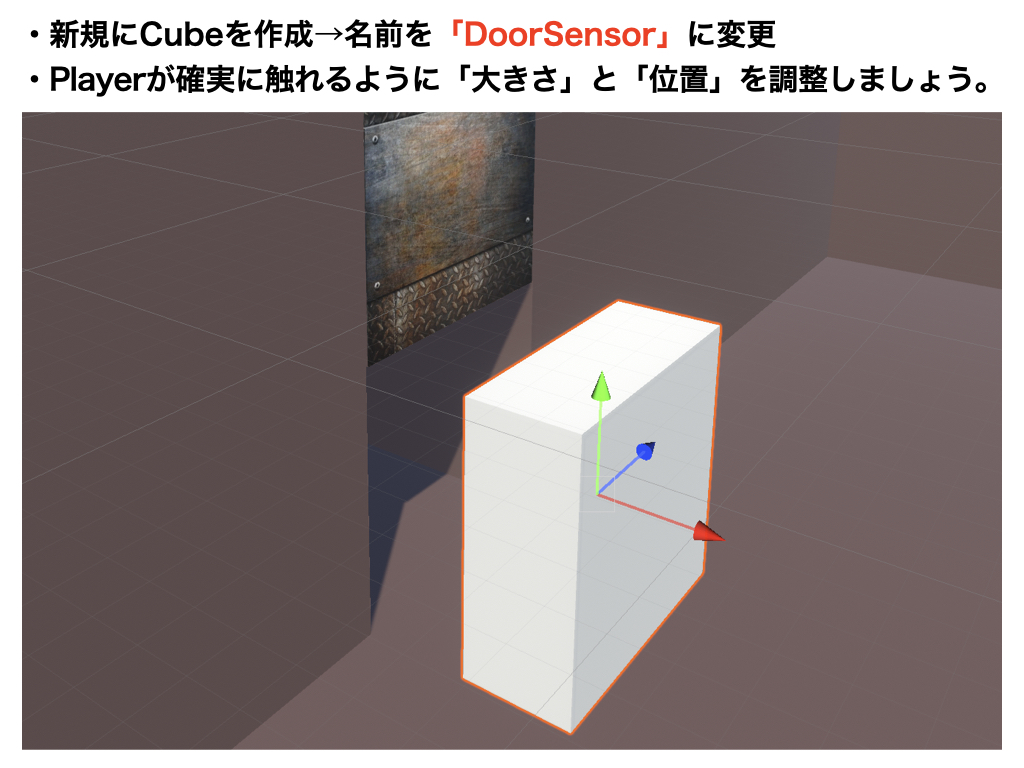
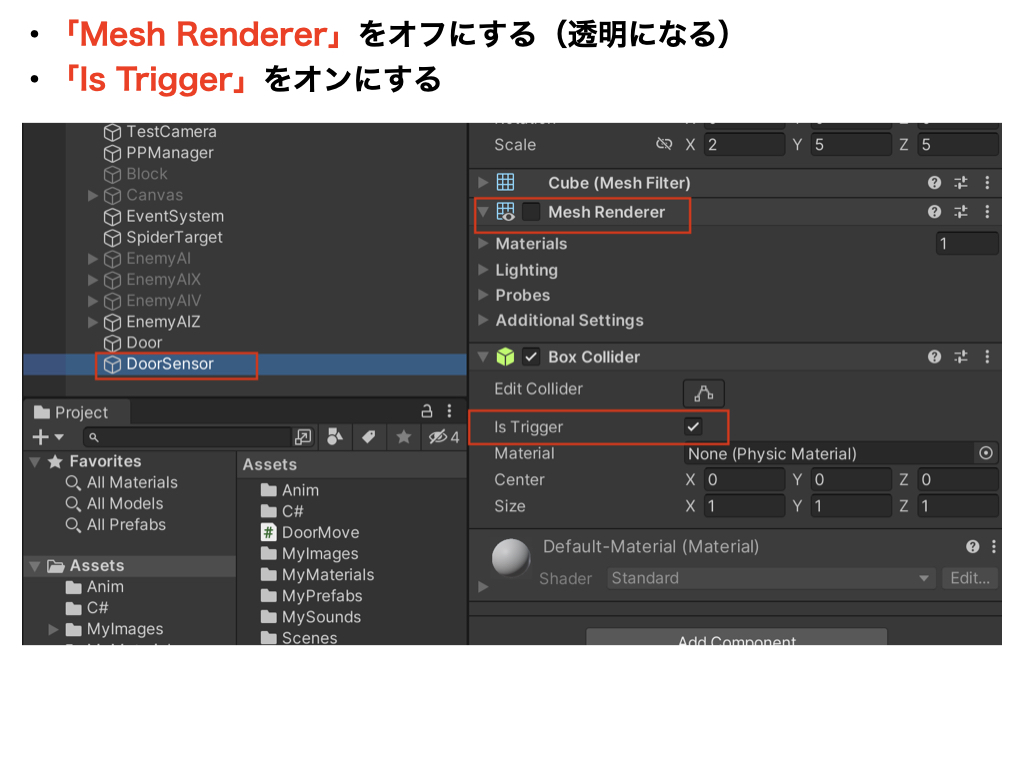
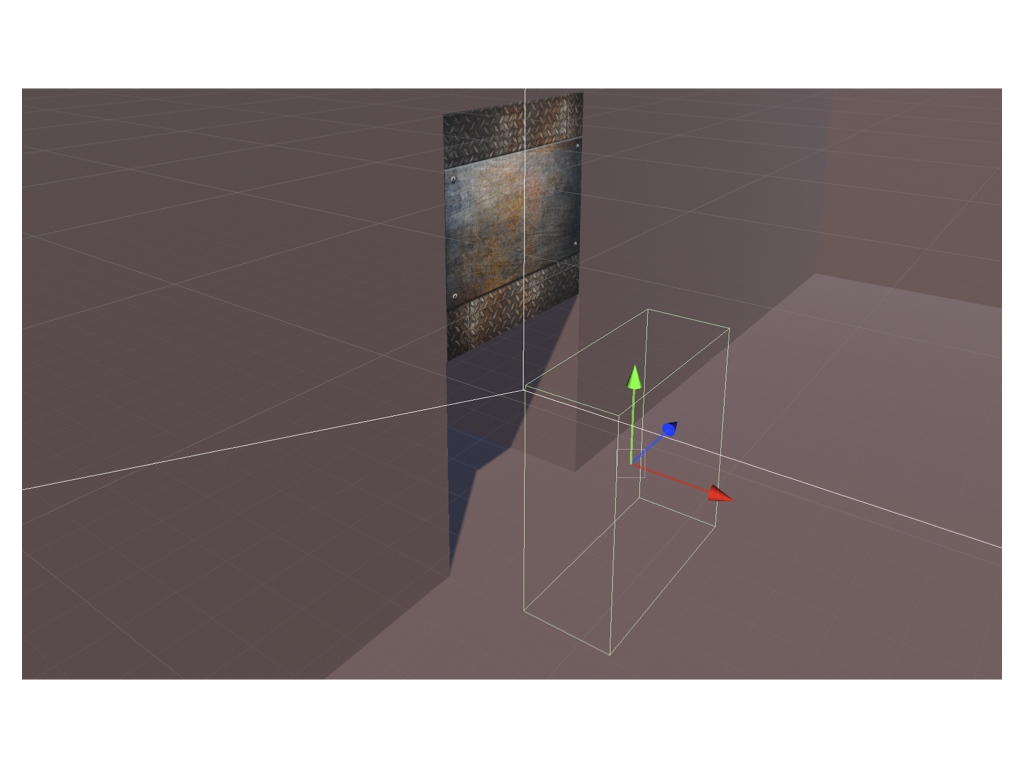
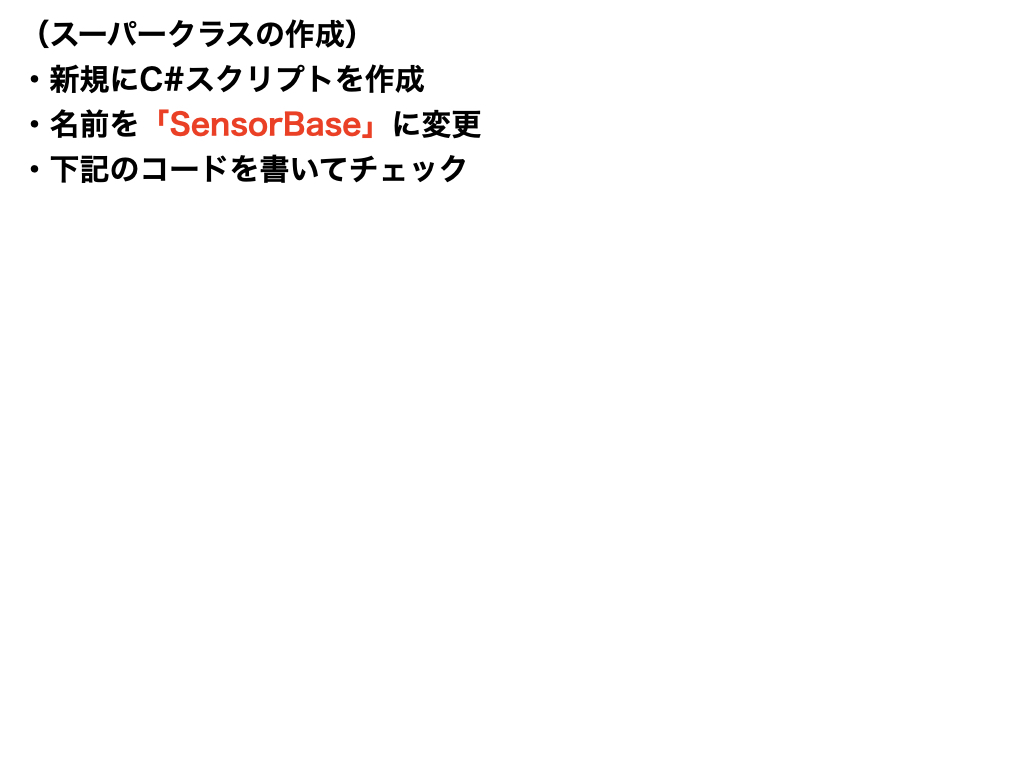
センサーのスーパークラス
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// 「abstract」キーワードの追加
public abstract class SensorBase : MonoBehaviour
{
public GameObject target;
public AudioClip sound;
public virtual void OnTriggerEnter(Collider other)
{
this.gameObject.SetActive(false); // センサーの使用は1回のみ
}
}
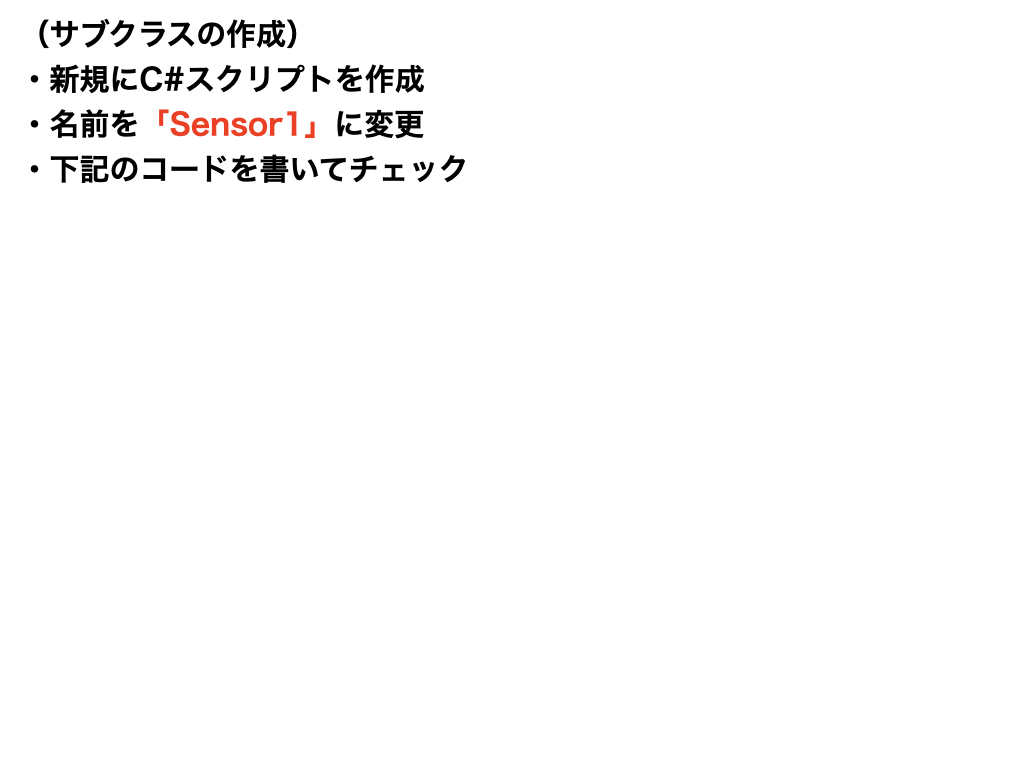
センサーのサブクラス
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Sensor1 : SensorBase // 変更
{
public override void OnTriggerEnter(Collider other)
{
base.OnTriggerEnter(other);
if (other.CompareTag("Player"))
{
AudioSource.PlayClipAtPoint(sound, Camera.main.transform.position);
target.GetComponent<DoorMove>().enabled = true;
}
}
}
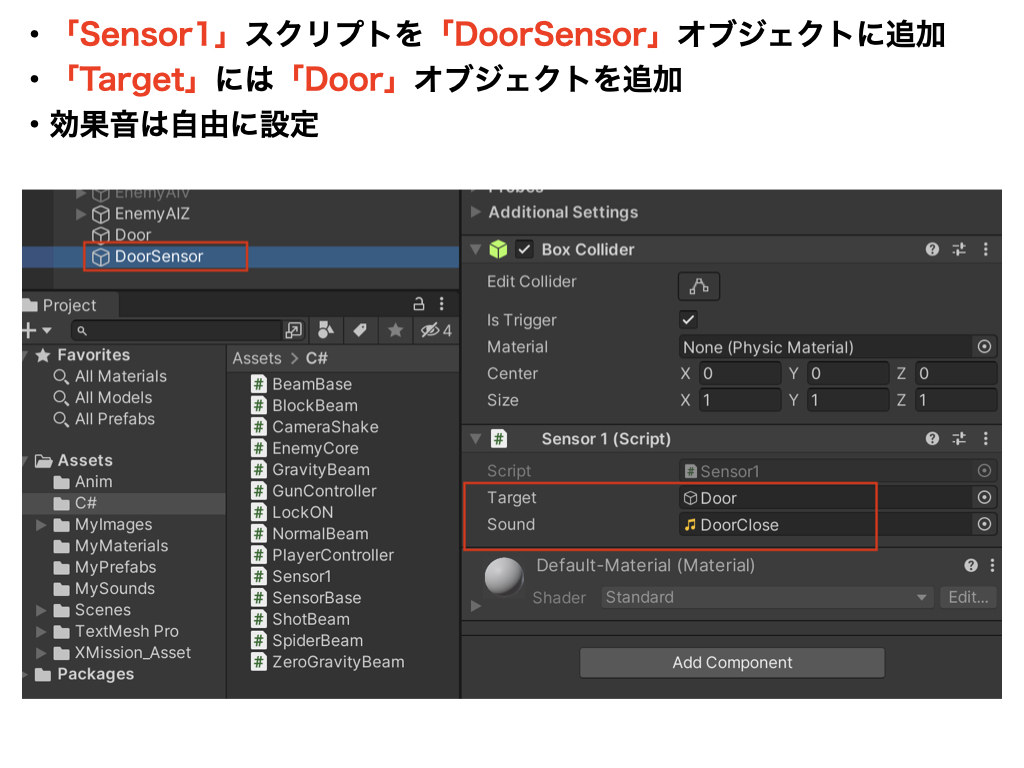
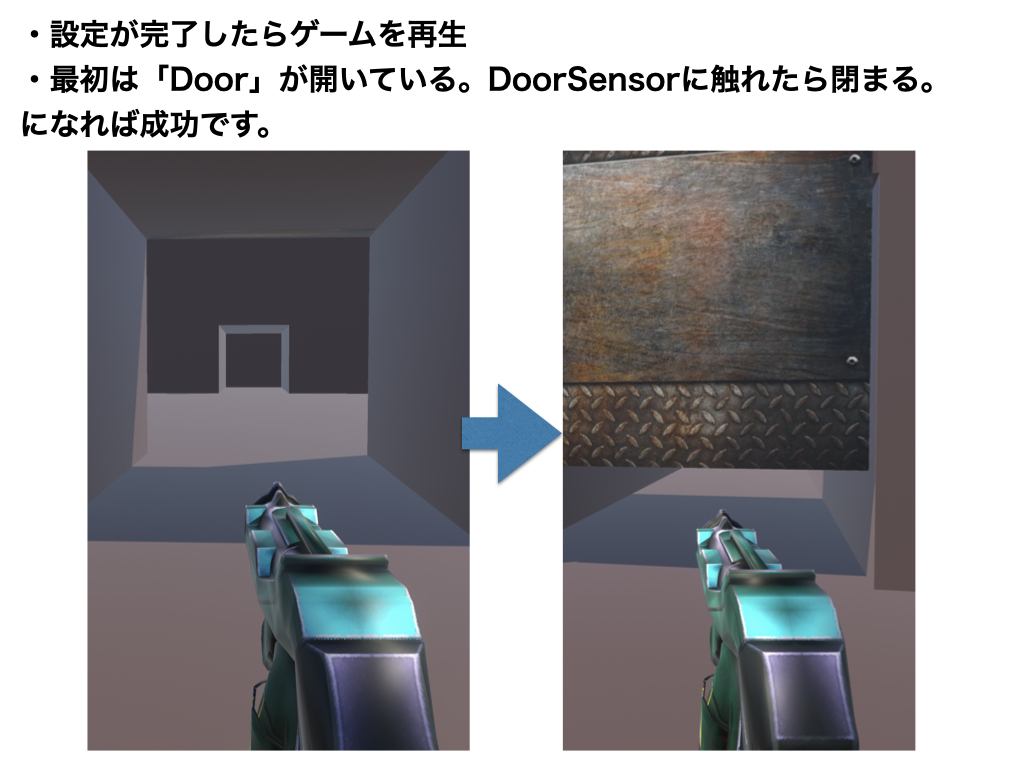
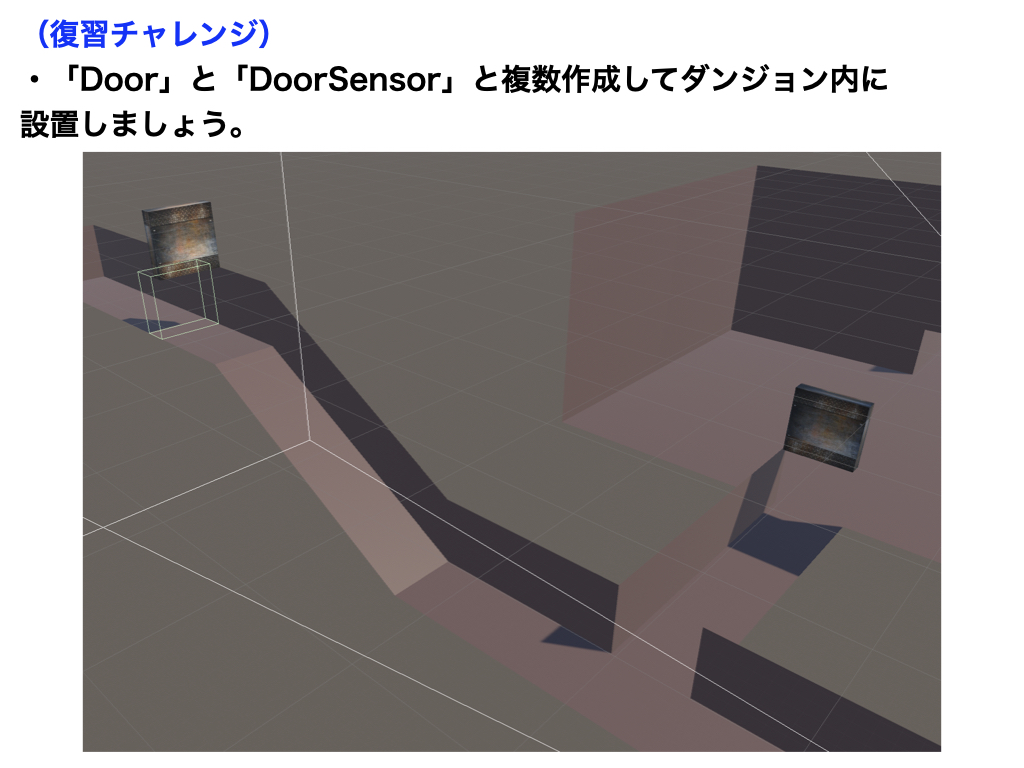
ステージの拡張1(ドアのオープン&クローズ)