Playerの足音の実装
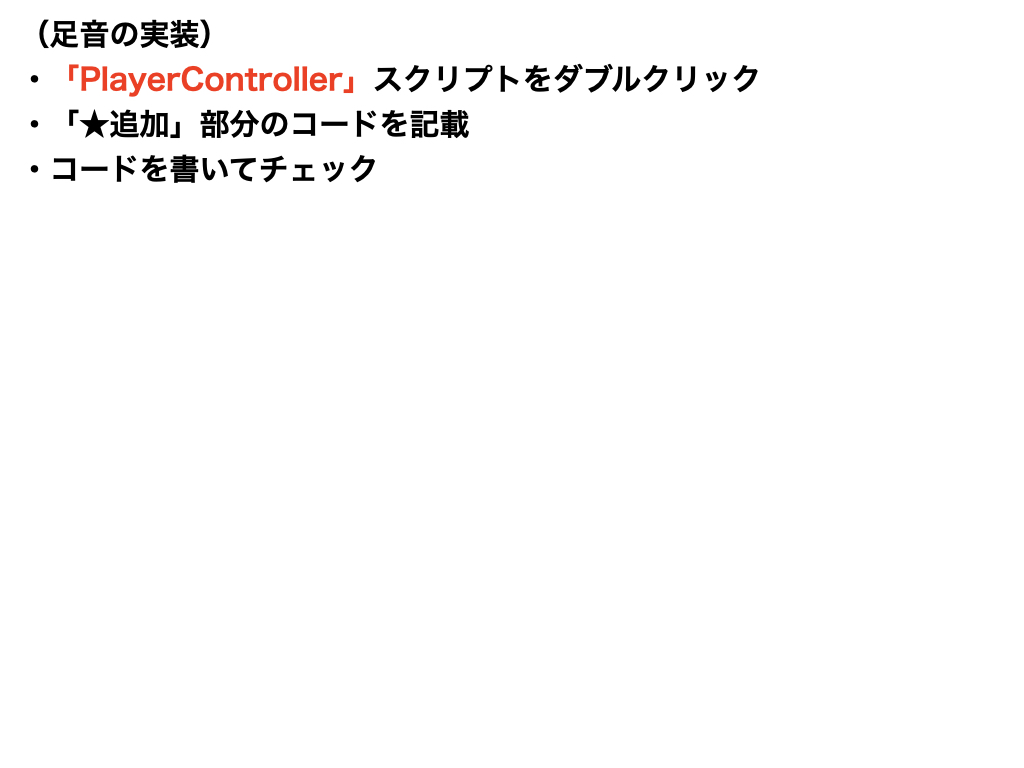
足音の実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float walkSpeed;
private float speed;
private Vector3 movement;
private CharacterController controller;
private int clickCount = 0;
public float runSpeed;
private Vector3 moveDir = Vector3.zero;
private float gravity = 3f;
public float jumpPower;
public AudioClip jumpSound;
private AudioSource audioS;
// ★追加(足音)
public AudioClip footSound;
private float runStep = 0.7f;
private float stepInterval = 10f;
private float stepCycle;
private float nextStep;
private bool isWalking = true; // スタート時は歩くモード
void Start()
{
audioS = GetComponent<AudioSource>();
controller = GetComponent<CharacterController>();
speed = walkSpeed;
}
void Update()
{
PlayerMove();
}
void PlayerMove()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
movement = new Vector3(moveH, 0, moveV);
Vector3 desiredMove = Camera.main.transform.forward * movement.z + Camera.main.transform.right * movement.x;
moveDir.x = desiredMove.x * 3f;
moveDir.z = desiredMove.z * 3f;
moveDir.y -= gravity * Time.deltaTime;
controller.Move(moveDir * Time.deltaTime * speed);
if (Input.GetKeyDown(KeyCode.W))
{
clickCount += 1;
Invoke("ResetCount", 0.3f);
}
if (Input.GetKeyUp(KeyCode.W))
{
speed = walkSpeed;
// ★追加(足音)
isWalking = true; // 歩くモードに戻す
}
if (controller.isGrounded)
{
moveDir.y = 0;
if (Input.GetKeyDown(KeyCode.Space))
{
moveDir.y = jumpPower;
audioS.clip = jumpSound;
audioS.Play();
}
}
// ★追加(足音)
StepSound();
}
void ResetCount()
{
if (clickCount != 2)
{
clickCount = 0;
return;
}
else
{
clickCount = 0;
speed = runSpeed;
// ★追加(足音)
isWalking = false; // 走るモードに変更
}
}
// ★追加(足音)
void StepSound()
{
// ジャンプ中は足音を発生させない。
if (!controller.isGrounded)
{
return;
}
if (controller.velocity.sqrMagnitude > 0 && (movement.x != 0 || movement.z != 0))
{
// 三項演算子(テクニック)
stepCycle += (controller.velocity.magnitude + (speed * (isWalking ? 1f : runStep))) * Time.deltaTime;
}
if (stepCycle < nextStep)
{
return;
}
// 足音の発生間隔・・・>歩く時は間隔が長い。走る時は短い。
nextStep = stepCycle + stepInterval;
audioS.clip = footSound;
audioS.PlayOneShot(audioS.clip);
}
}
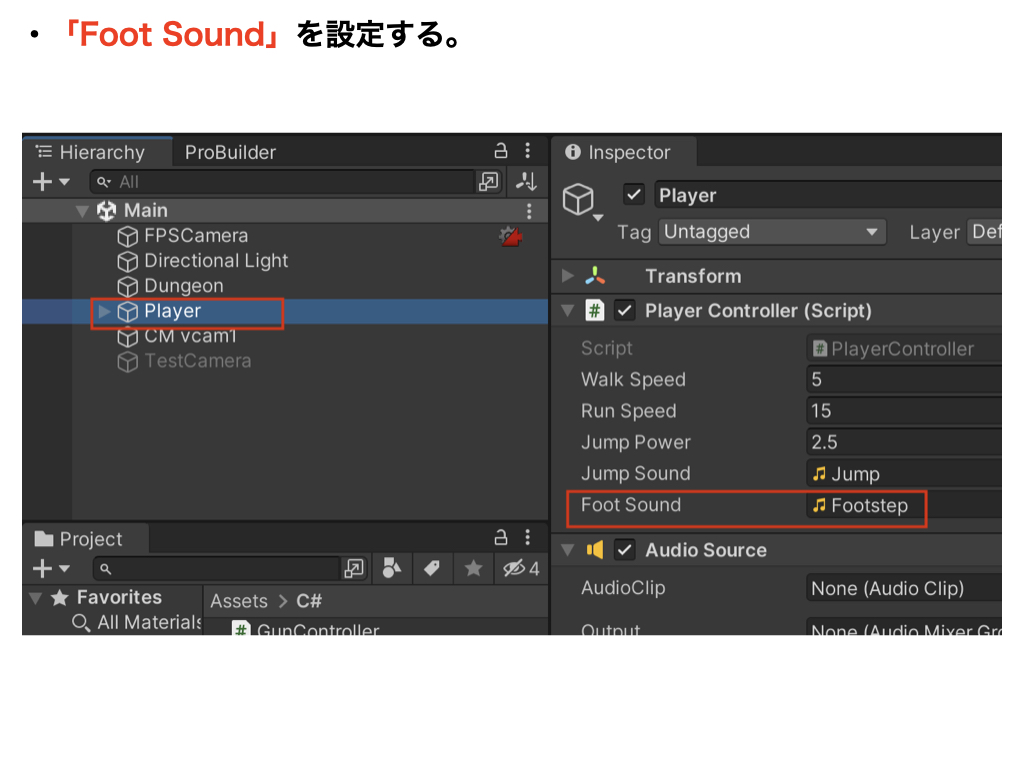
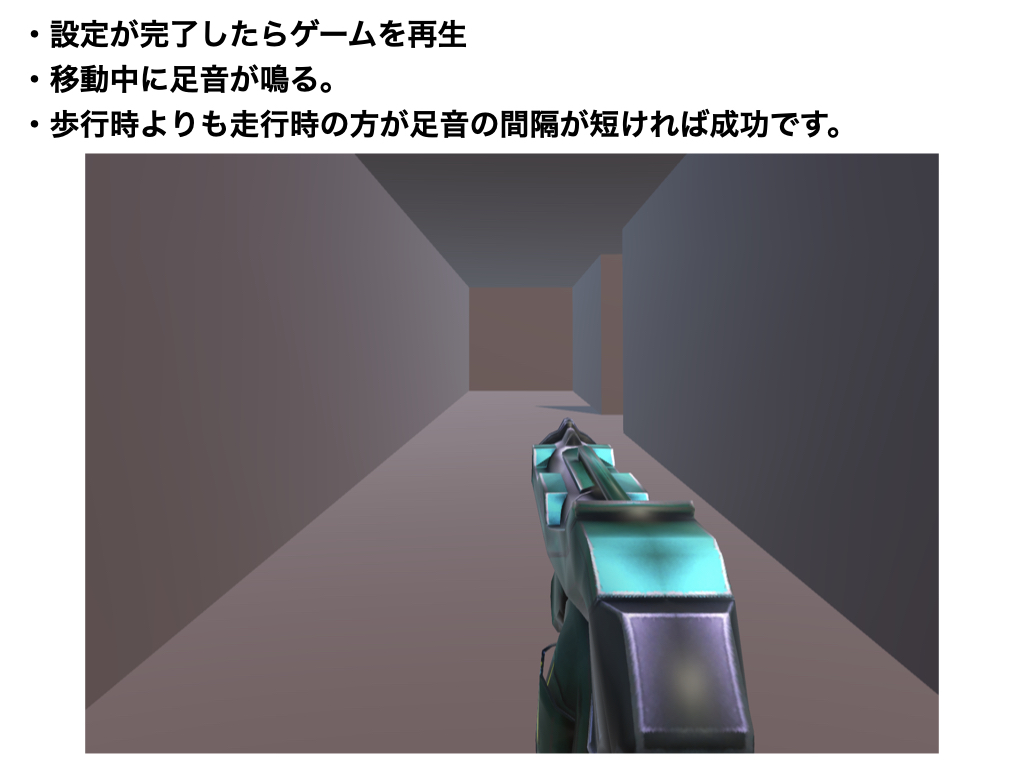
【2021版】X_Mission(全34回)
他のコースを見る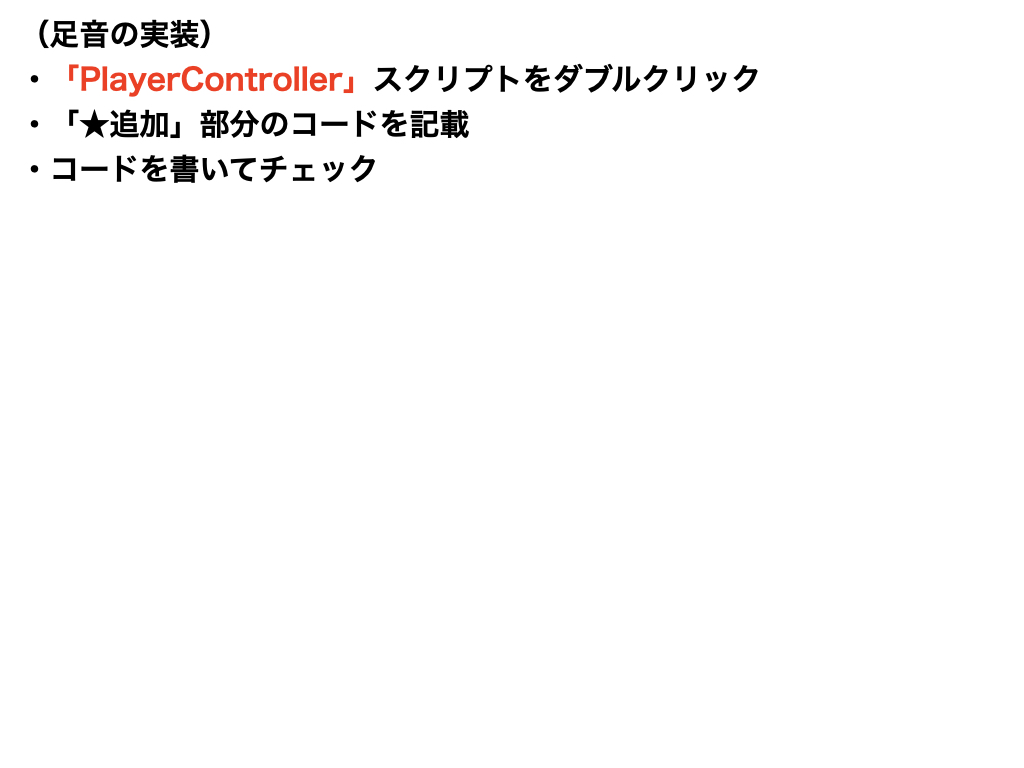
足音の実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float walkSpeed;
private float speed;
private Vector3 movement;
private CharacterController controller;
private int clickCount = 0;
public float runSpeed;
private Vector3 moveDir = Vector3.zero;
private float gravity = 3f;
public float jumpPower;
public AudioClip jumpSound;
private AudioSource audioS;
// ★追加(足音)
public AudioClip footSound;
private float runStep = 0.7f;
private float stepInterval = 10f;
private float stepCycle;
private float nextStep;
private bool isWalking = true; // スタート時は歩くモード
void Start()
{
audioS = GetComponent<AudioSource>();
controller = GetComponent<CharacterController>();
speed = walkSpeed;
}
void Update()
{
PlayerMove();
}
void PlayerMove()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
movement = new Vector3(moveH, 0, moveV);
Vector3 desiredMove = Camera.main.transform.forward * movement.z + Camera.main.transform.right * movement.x;
moveDir.x = desiredMove.x * 3f;
moveDir.z = desiredMove.z * 3f;
moveDir.y -= gravity * Time.deltaTime;
controller.Move(moveDir * Time.deltaTime * speed);
if (Input.GetKeyDown(KeyCode.W))
{
clickCount += 1;
Invoke("ResetCount", 0.3f);
}
if (Input.GetKeyUp(KeyCode.W))
{
speed = walkSpeed;
// ★追加(足音)
isWalking = true; // 歩くモードに戻す
}
if (controller.isGrounded)
{
moveDir.y = 0;
if (Input.GetKeyDown(KeyCode.Space))
{
moveDir.y = jumpPower;
audioS.clip = jumpSound;
audioS.Play();
}
}
// ★追加(足音)
StepSound();
}
void ResetCount()
{
if (clickCount != 2)
{
clickCount = 0;
return;
}
else
{
clickCount = 0;
speed = runSpeed;
// ★追加(足音)
isWalking = false; // 走るモードに変更
}
}
// ★追加(足音)
void StepSound()
{
// ジャンプ中は足音を発生させない。
if (!controller.isGrounded)
{
return;
}
if (controller.velocity.sqrMagnitude > 0 && (movement.x != 0 || movement.z != 0))
{
// 三項演算子(テクニック)
stepCycle += (controller.velocity.magnitude + (speed * (isWalking ? 1f : runStep))) * Time.deltaTime;
}
if (stepCycle < nextStep)
{
return;
}
// 足音の発生間隔・・・>歩く時は間隔が長い。走る時は短い。
nextStep = stepCycle + stepInterval;
audioS.clip = footSound;
audioS.PlayOneShot(audioS.clip);
}
}
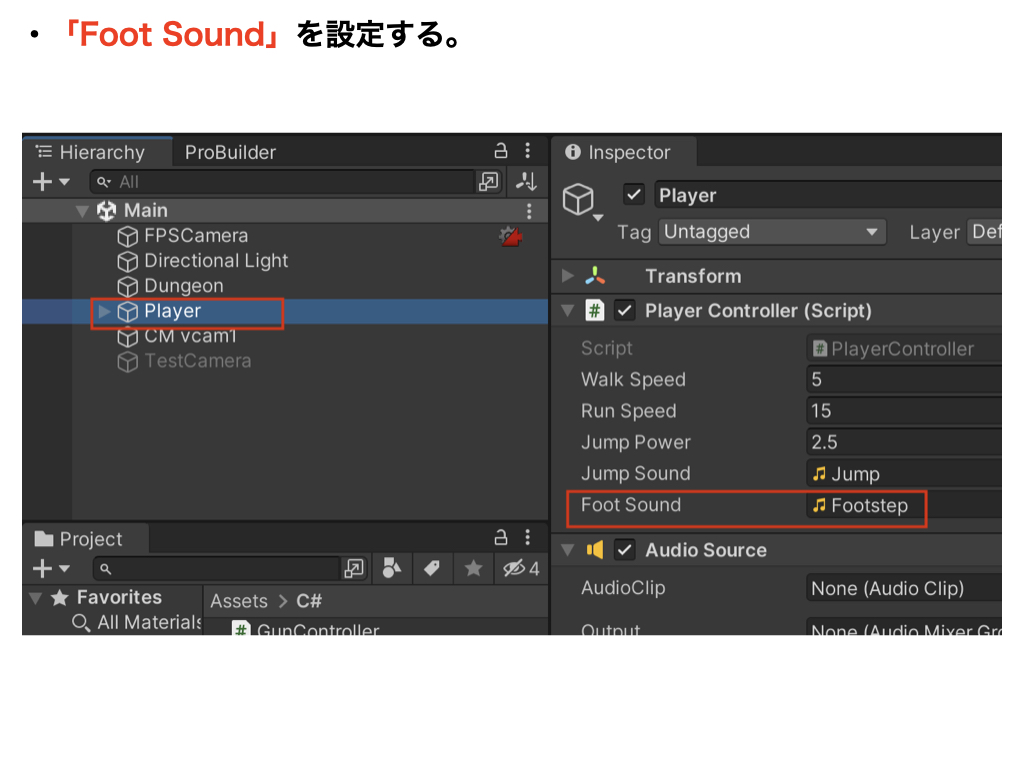
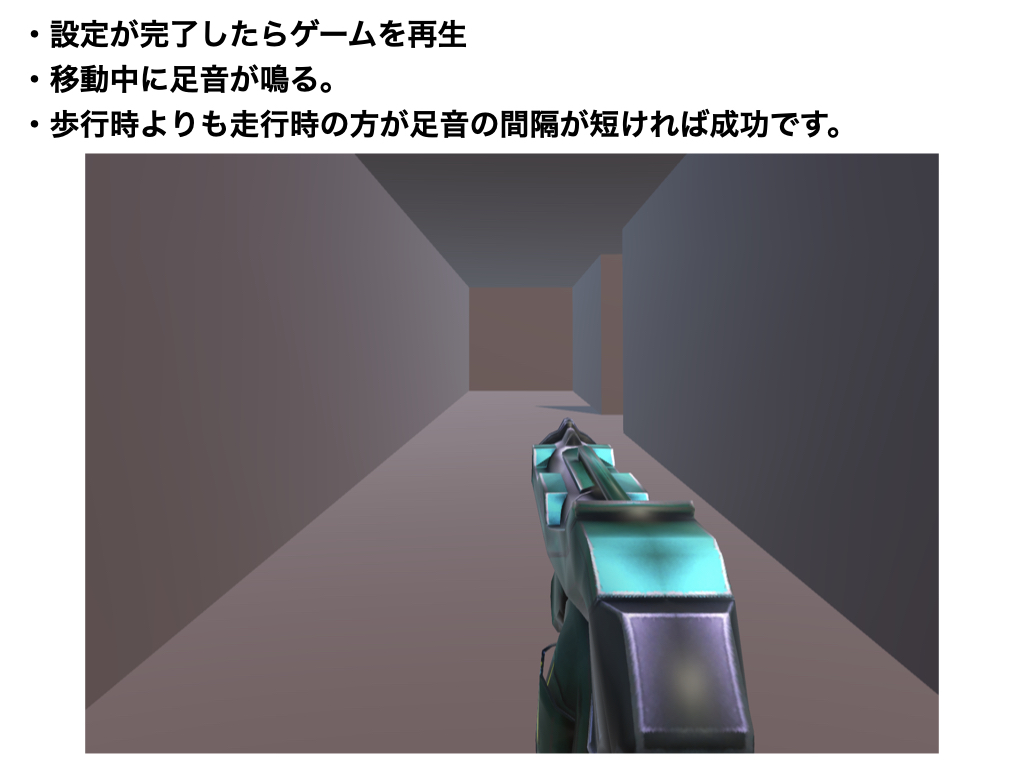
Playerの足音の実装