ソニックビームの発射に回数制限を加える
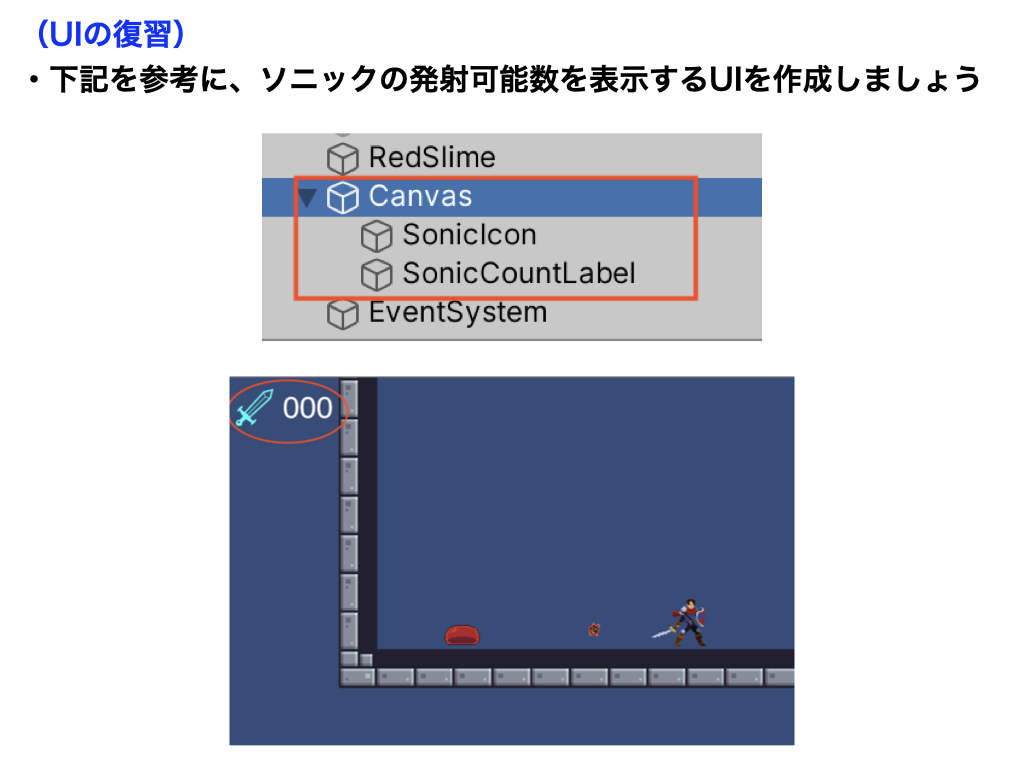
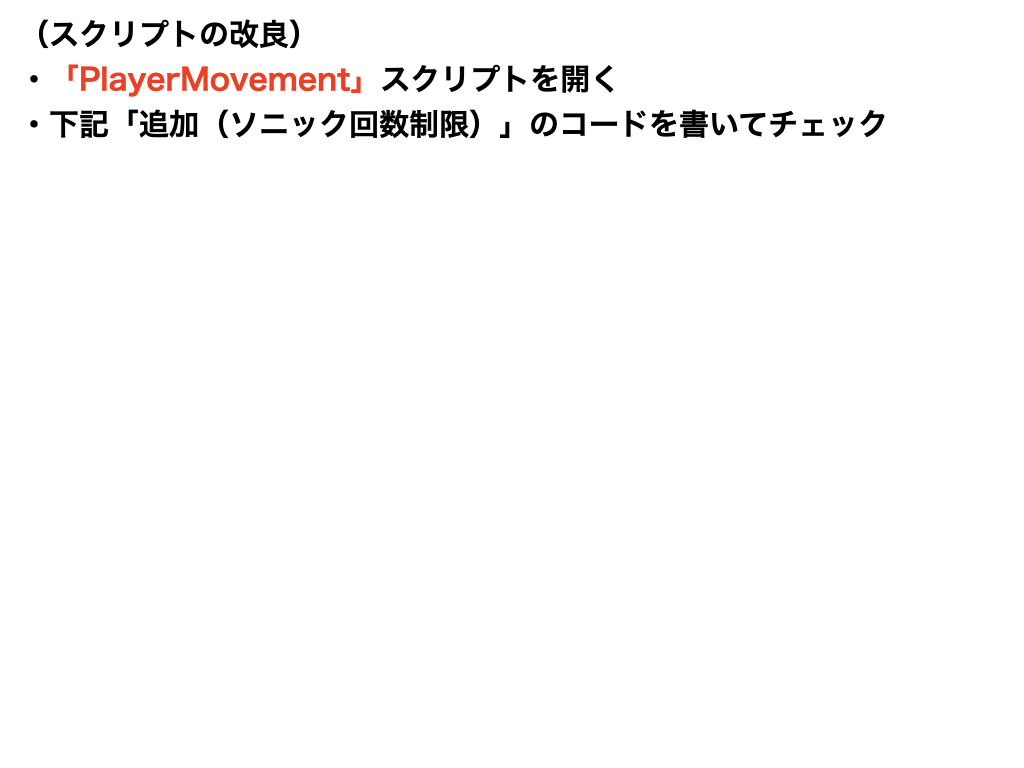
ソニック回数制限
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★追加(ソニック回数制限)
using TMPro;
public class PlayerMovement : MonoBehaviour
{
public float speed;
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
private bool isGard = false;
public BoxCollider2D guardCollider2D;
// ★追加(ソニック回数制限)
public TextMeshProUGUI sonicCountLabel;
private int sonicCount = 10; // 初期値は自由に変更可能
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
guardCollider2D.enabled = false;
// ★追加(ソニック回数制限)
sonicCountLabel.text = "" + sonicCount;
}
void Update()
{
if (isGard == false)
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
}
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
else if (Input.GetKeyDown(KeyCode.X) && sonicCount > 0) // ★追加(ソニック回数制限)・・・条件の追加
{
if (moveH != 0)
{
return;
}
// ★追加(ソニック回数制限)
sonicCount -= 1;
sonicCountLabel.text = "" + sonicCount;
animator.SetTrigger("Attack2");
}
if (Input.GetKeyDown(KeyCode.C))
{
if (moveH != 0)
{
return;
}
animator.SetBool("Guard", true);
isGard = true;
guardCollider2D.enabled = true;
}
else if (Input.GetKeyUp(KeyCode.C))
{
animator.SetBool("Guard", false);
isGard = false;
guardCollider2D.enabled = false;
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
}
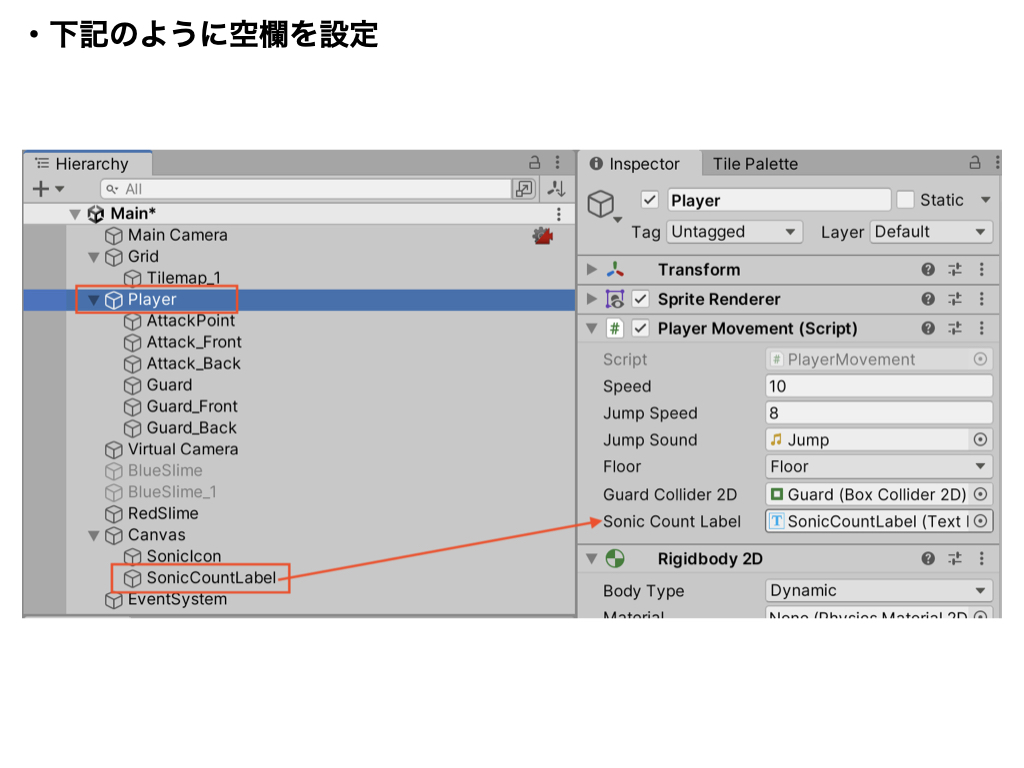
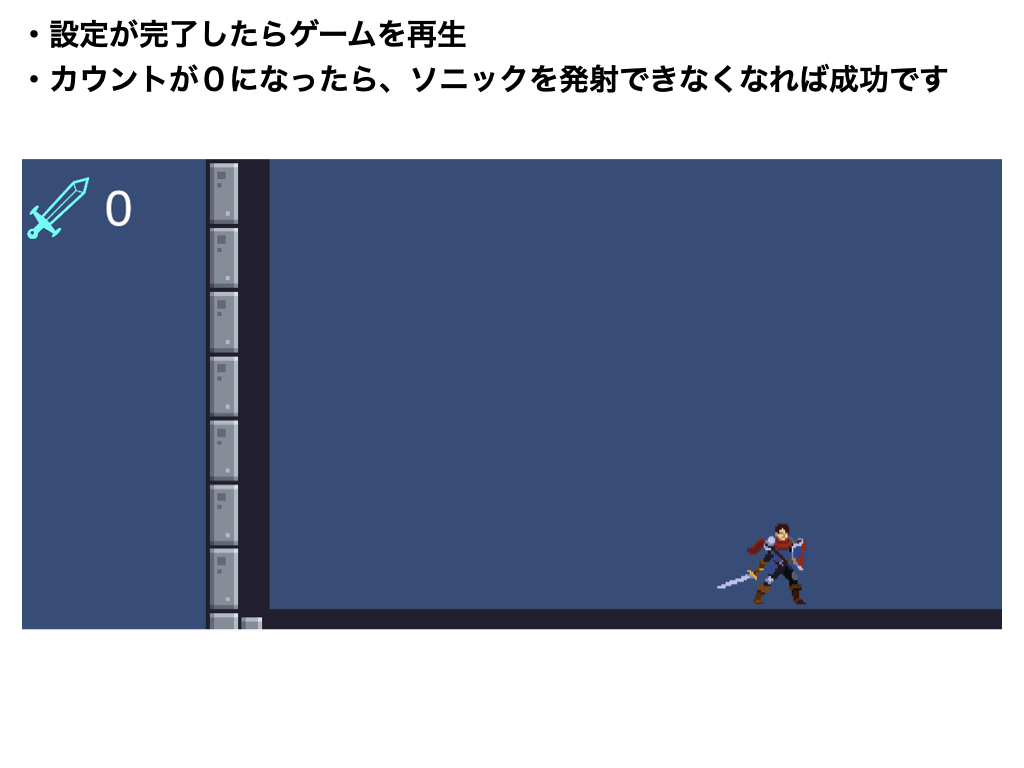
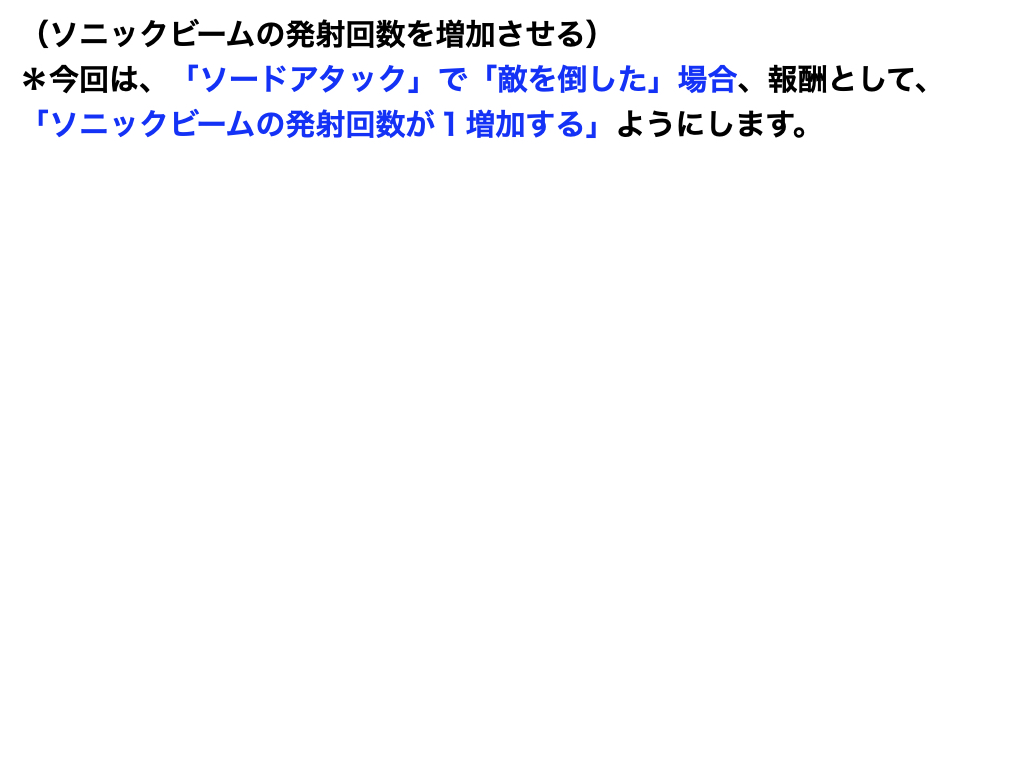
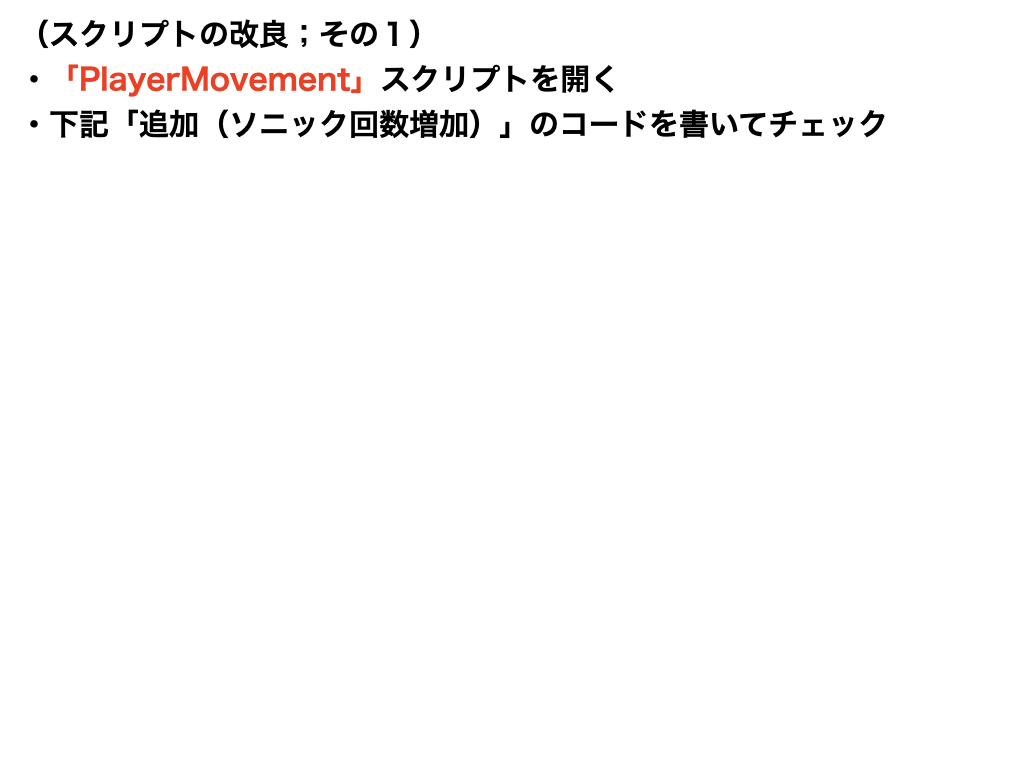
ソニックの回数増加
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using TMPro;
public class PlayerMovement : MonoBehaviour
{
public float speed;
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
private bool isGard = false;
public BoxCollider2D guardCollider2D;
public TextMeshProUGUI sonicCountLabel;
private int sonicCount = 10;
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
guardCollider2D.enabled = false;
sonicCountLabel.text = "" + sonicCount;
}
void Update()
{
if (isGard == false)
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
}
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
else if (Input.GetKeyDown(KeyCode.X) && sonicCount > 0)
{
if (moveH != 0)
{
return;
}
sonicCount -= 1;
sonicCountLabel.text = "" + sonicCount;
animator.SetTrigger("Attack2");
}
if (Input.GetKeyDown(KeyCode.C))
{
if (moveH != 0)
{
return;
}
animator.SetBool("Guard", true);
isGard = true;
guardCollider2D.enabled = true;
}
else if (Input.GetKeyUp(KeyCode.C))
{
animator.SetBool("Guard", false);
isGard = false;
guardCollider2D.enabled = false;
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
// 追加(ソニックの回数増加)
public void AddSonicCount(int amount)
{
sonicCount += amount;
sonicCountLabel.text = "" + sonicCount;
}
}
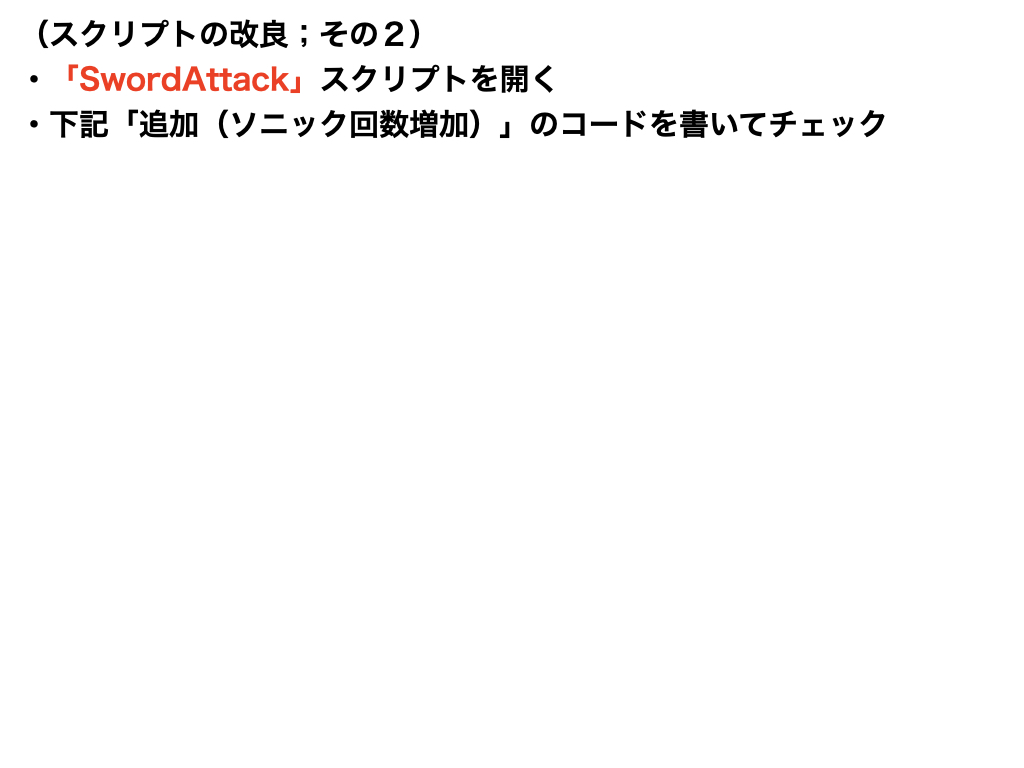
ソニックの回数増加
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SwordAttack : MonoBehaviour
{
public AudioClip sound;
public GameObject attackPoint;
public GameObject attack_front;
public GameObject attack_back;
private RaycastHit2D hit2d;
private PlayerMovement pm;
private float num;
public GameObject effectPrefab;
void Start()
{
pm = GetComponent<PlayerMovement>();
attackPoint.transform.position = attack_front.transform.position;
}
void Update()
{
num = pm.moveH;
if (num == 1)
{
attackPoint.transform.position = attack_front.transform.position;
}
else if (num == -1)
{
attackPoint.transform.position = attack_back.transform.position;
}
hit2d = Physics2D.Raycast(attackPoint.transform.position, Vector2.right * num, 0.3f);
Debug.DrawRay(attackPoint.transform.position, Vector2.right * num, Color.white, 0.3f);
}
public void Attack()
{
if (hit2d.collider != null)
{
if (hit2d.collider.CompareTag("Enemy"))
{
Destroy(hit2d.collider.gameObject);
AudioSource.PlayClipAtPoint(sound, transform.position);
Instantiate(effectPrefab, hit2d.collider.transform.position, Quaternion.identity);
// 追加(ソニックの回数増加)
pm.AddSonicCount(1); // 増加数は自由に変更可能
}
}
}
}
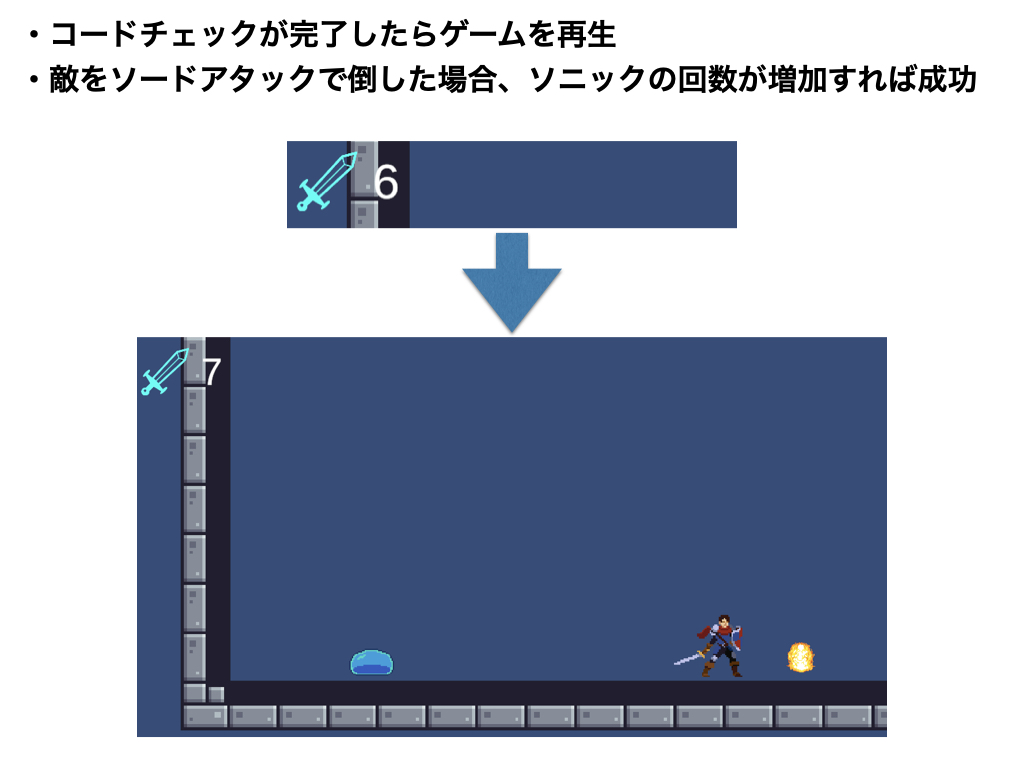
【2022版】DarkCastle(全39回)
他のコースを見る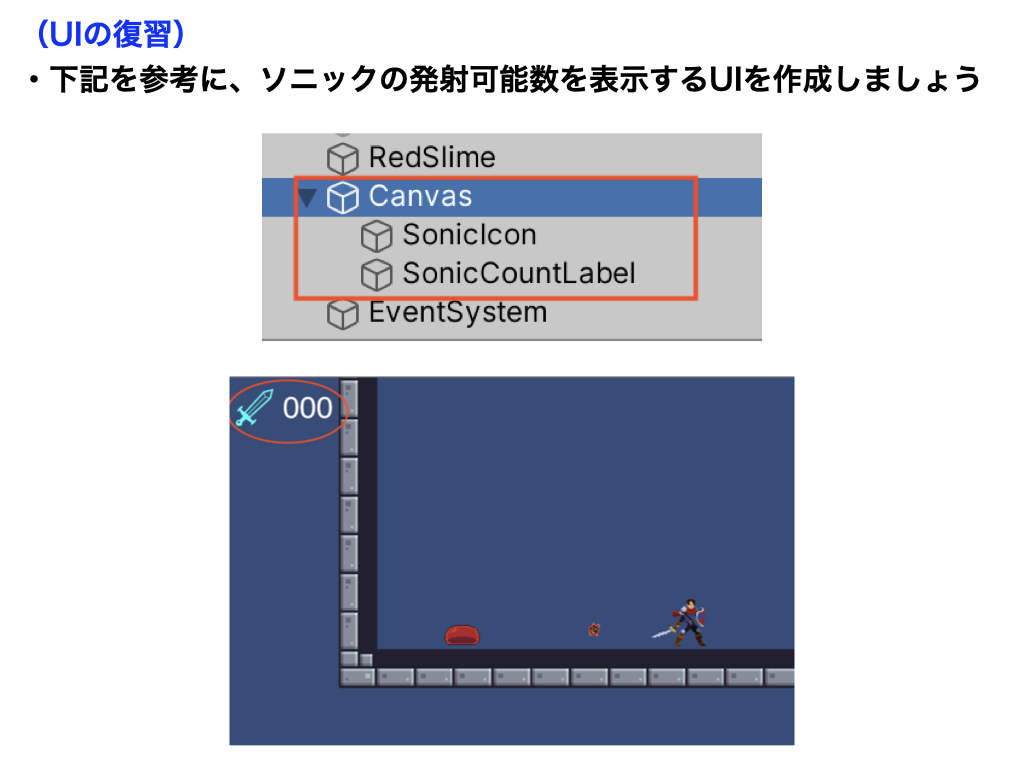
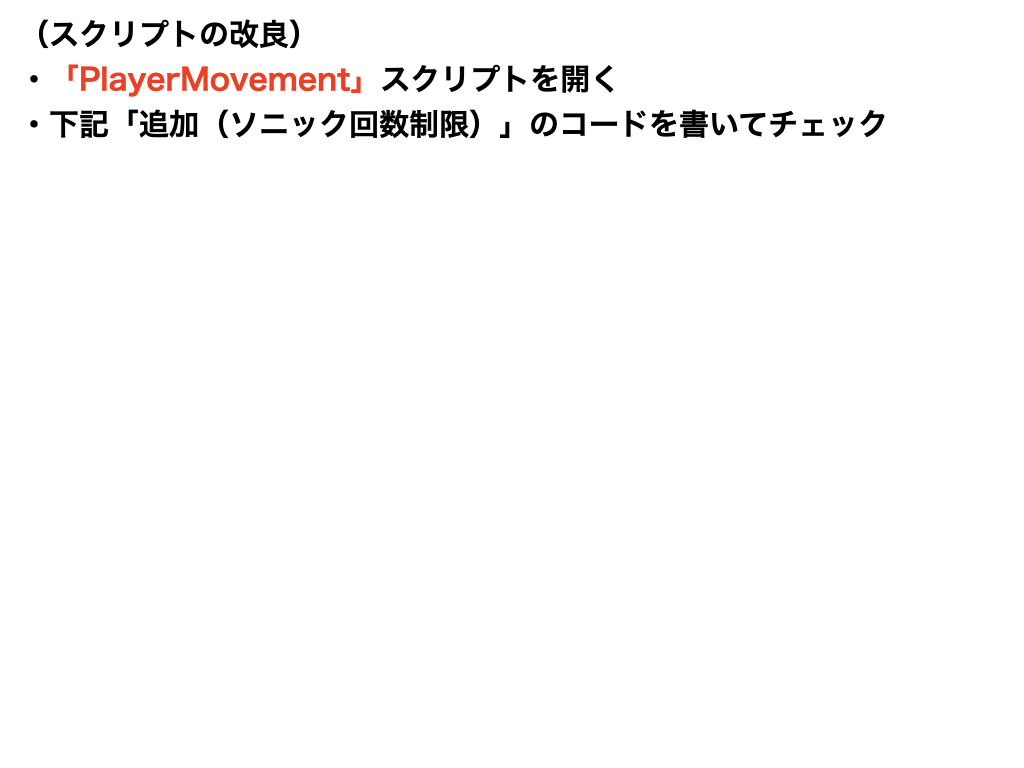
ソニック回数制限
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★追加(ソニック回数制限)
using TMPro;
public class PlayerMovement : MonoBehaviour
{
public float speed;
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
private bool isGard = false;
public BoxCollider2D guardCollider2D;
// ★追加(ソニック回数制限)
public TextMeshProUGUI sonicCountLabel;
private int sonicCount = 10; // 初期値は自由に変更可能
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
guardCollider2D.enabled = false;
// ★追加(ソニック回数制限)
sonicCountLabel.text = "" + sonicCount;
}
void Update()
{
if (isGard == false)
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
}
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
else if (Input.GetKeyDown(KeyCode.X) && sonicCount > 0) // ★追加(ソニック回数制限)・・・条件の追加
{
if (moveH != 0)
{
return;
}
// ★追加(ソニック回数制限)
sonicCount -= 1;
sonicCountLabel.text = "" + sonicCount;
animator.SetTrigger("Attack2");
}
if (Input.GetKeyDown(KeyCode.C))
{
if (moveH != 0)
{
return;
}
animator.SetBool("Guard", true);
isGard = true;
guardCollider2D.enabled = true;
}
else if (Input.GetKeyUp(KeyCode.C))
{
animator.SetBool("Guard", false);
isGard = false;
guardCollider2D.enabled = false;
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
}
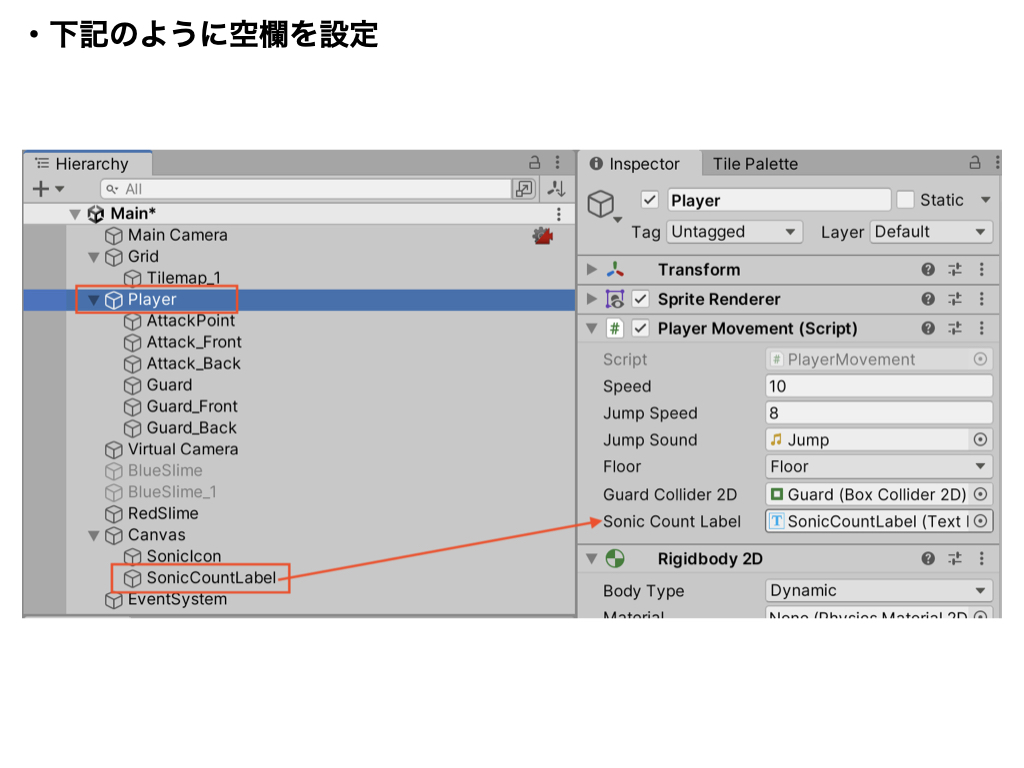
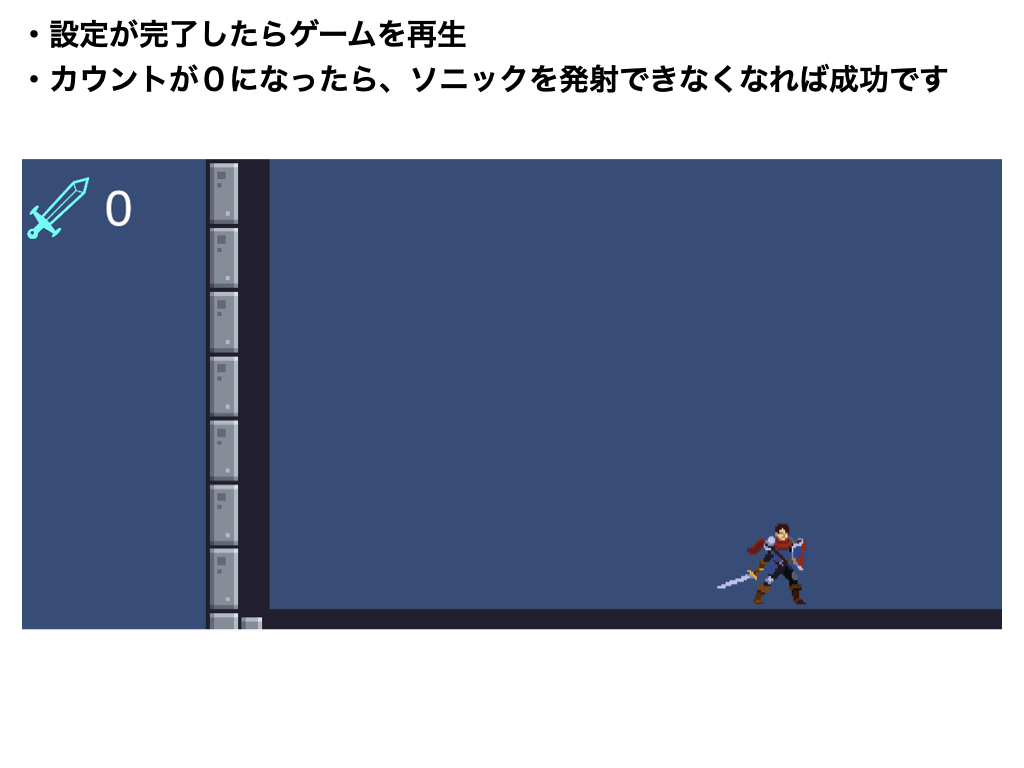
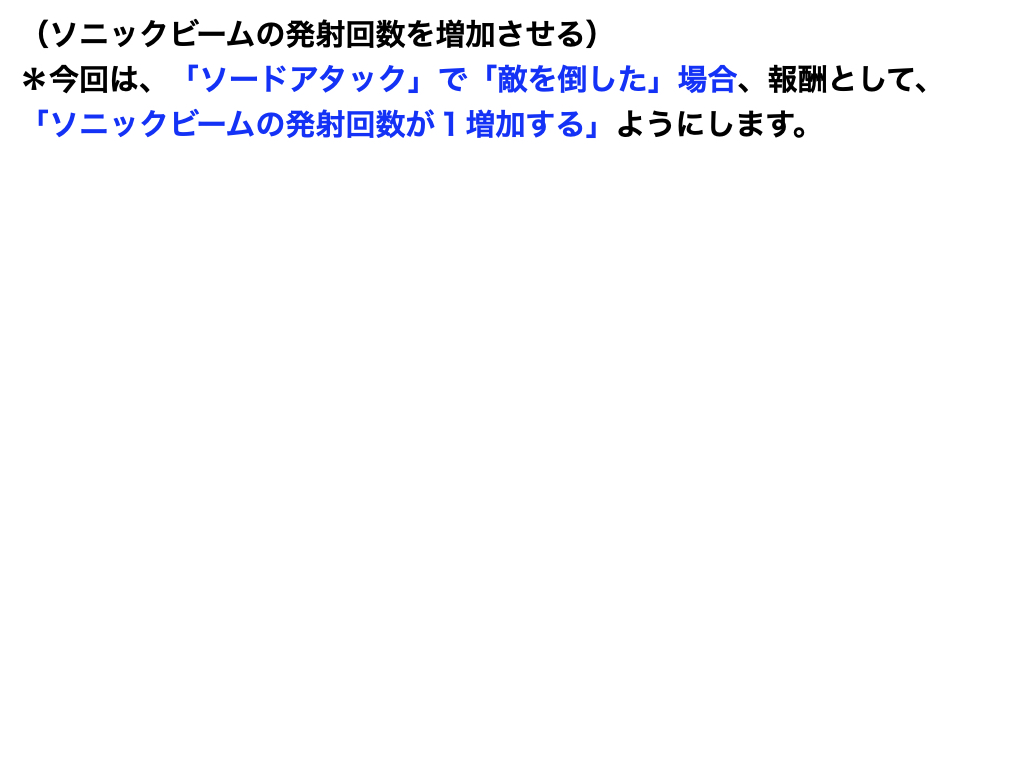
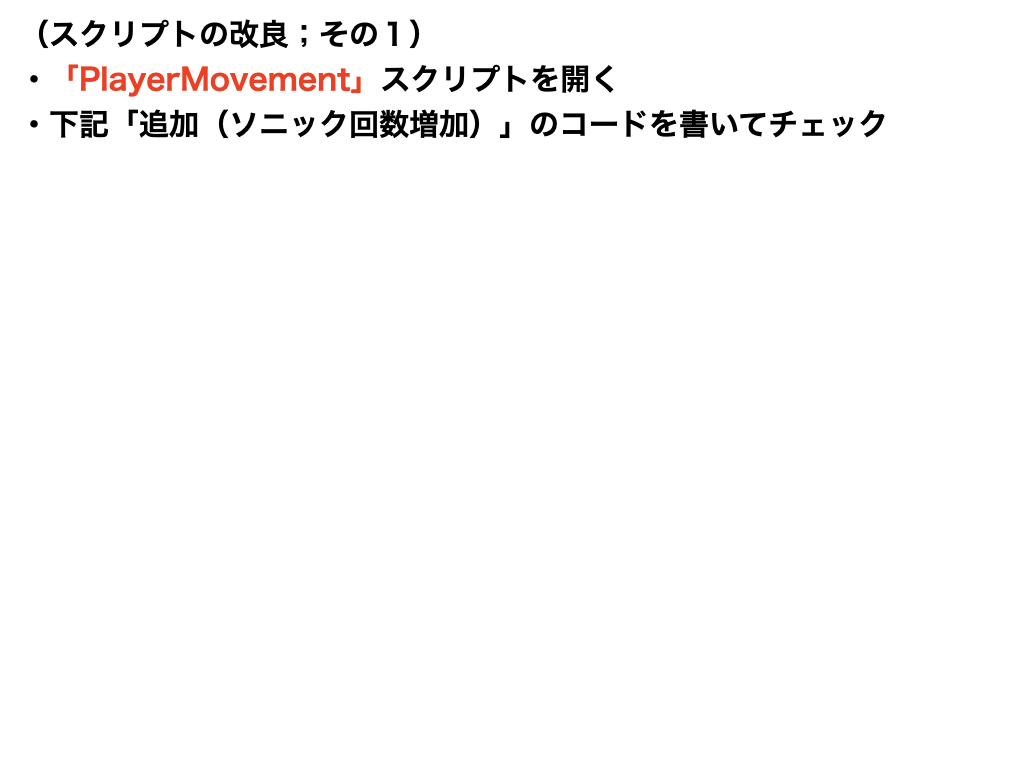
ソニックの回数増加
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using TMPro;
public class PlayerMovement : MonoBehaviour
{
public float speed;
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
private bool isGard = false;
public BoxCollider2D guardCollider2D;
public TextMeshProUGUI sonicCountLabel;
private int sonicCount = 10;
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
guardCollider2D.enabled = false;
sonicCountLabel.text = "" + sonicCount;
}
void Update()
{
if (isGard == false)
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
}
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
else if (Input.GetKeyDown(KeyCode.X) && sonicCount > 0)
{
if (moveH != 0)
{
return;
}
sonicCount -= 1;
sonicCountLabel.text = "" + sonicCount;
animator.SetTrigger("Attack2");
}
if (Input.GetKeyDown(KeyCode.C))
{
if (moveH != 0)
{
return;
}
animator.SetBool("Guard", true);
isGard = true;
guardCollider2D.enabled = true;
}
else if (Input.GetKeyUp(KeyCode.C))
{
animator.SetBool("Guard", false);
isGard = false;
guardCollider2D.enabled = false;
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
// 追加(ソニックの回数増加)
public void AddSonicCount(int amount)
{
sonicCount += amount;
sonicCountLabel.text = "" + sonicCount;
}
}
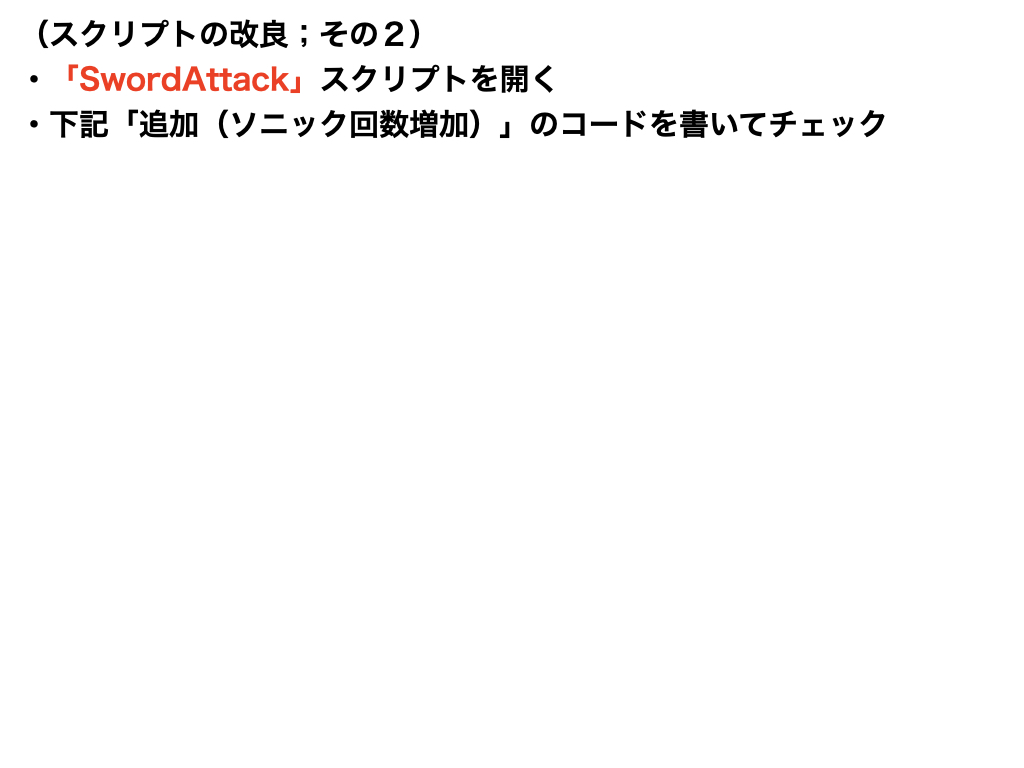
ソニックの回数増加
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SwordAttack : MonoBehaviour
{
public AudioClip sound;
public GameObject attackPoint;
public GameObject attack_front;
public GameObject attack_back;
private RaycastHit2D hit2d;
private PlayerMovement pm;
private float num;
public GameObject effectPrefab;
void Start()
{
pm = GetComponent<PlayerMovement>();
attackPoint.transform.position = attack_front.transform.position;
}
void Update()
{
num = pm.moveH;
if (num == 1)
{
attackPoint.transform.position = attack_front.transform.position;
}
else if (num == -1)
{
attackPoint.transform.position = attack_back.transform.position;
}
hit2d = Physics2D.Raycast(attackPoint.transform.position, Vector2.right * num, 0.3f);
Debug.DrawRay(attackPoint.transform.position, Vector2.right * num, Color.white, 0.3f);
}
public void Attack()
{
if (hit2d.collider != null)
{
if (hit2d.collider.CompareTag("Enemy"))
{
Destroy(hit2d.collider.gameObject);
AudioSource.PlayClipAtPoint(sound, transform.position);
Instantiate(effectPrefab, hit2d.collider.transform.position, Quaternion.identity);
// 追加(ソニックの回数増加)
pm.AddSonicCount(1); // 増加数は自由に変更可能
}
}
}
}
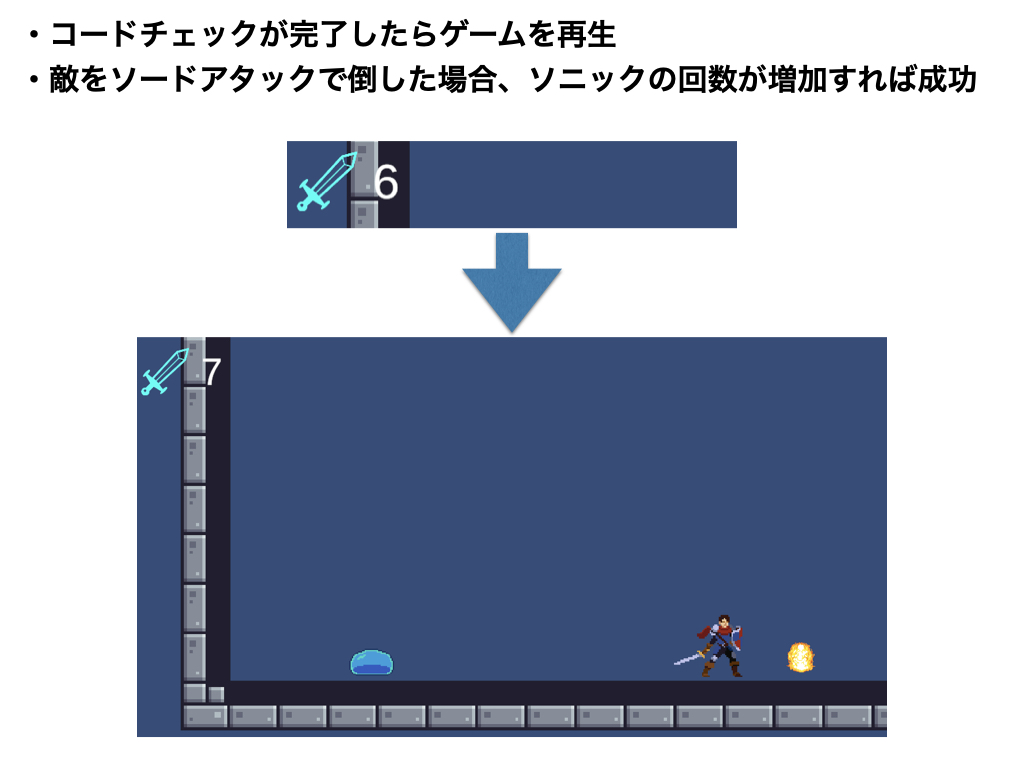
ソニックビームの発射に回数制限を加える