Guard機能のオンとオフをコントロールする
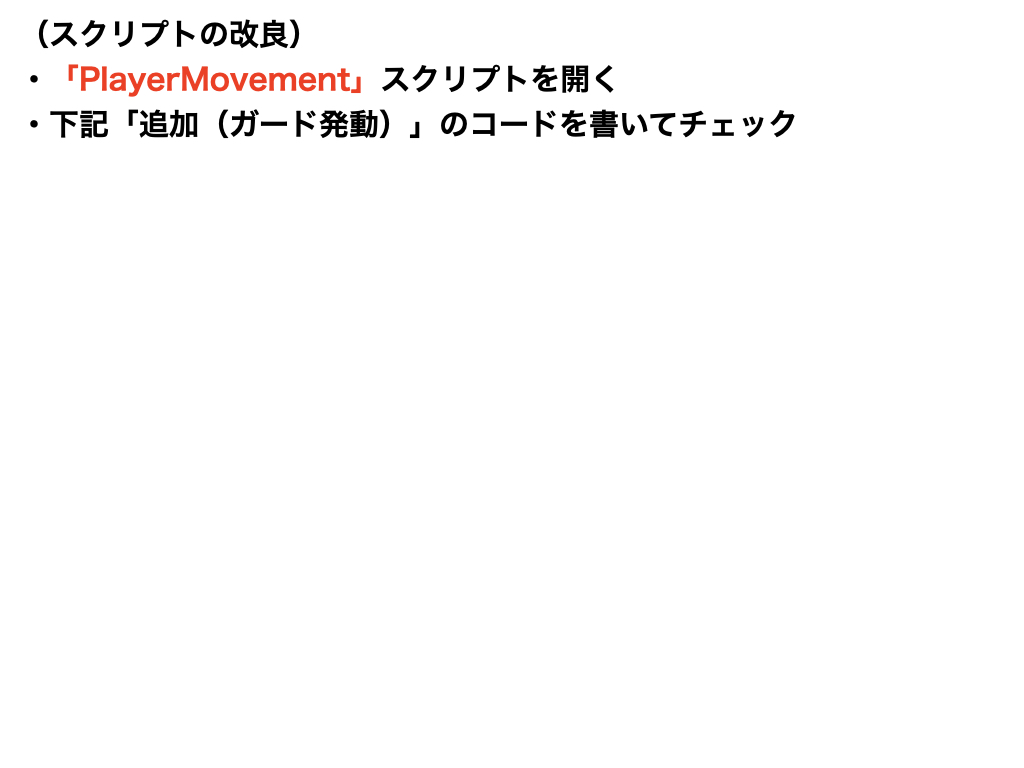
ガード発動
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed;
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
private bool isGard = false;
// ★追加(ガード発動)
public BoxCollider2D guardCollider2D;
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
// ★追加(ガード発動)
// ゲーム開始時点ではガードはOFF
guardCollider2D.enabled = false;
}
void Update()
{
if(isGard == false)
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
}
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
else if (Input.GetKeyDown(KeyCode.X))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack2");
}
if (Input.GetKeyDown(KeyCode.C))
{
if (moveH != 0)
{
return;
}
animator.SetBool("Guard", true);
isGard = true;
// ★追加(ガード発動)
guardCollider2D.enabled = true;
}
else if (Input.GetKeyUp(KeyCode.C))
{
animator.SetBool("Guard", false);
isGard = false;
// ★追加(ガード発動)
guardCollider2D.enabled = false;
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
}
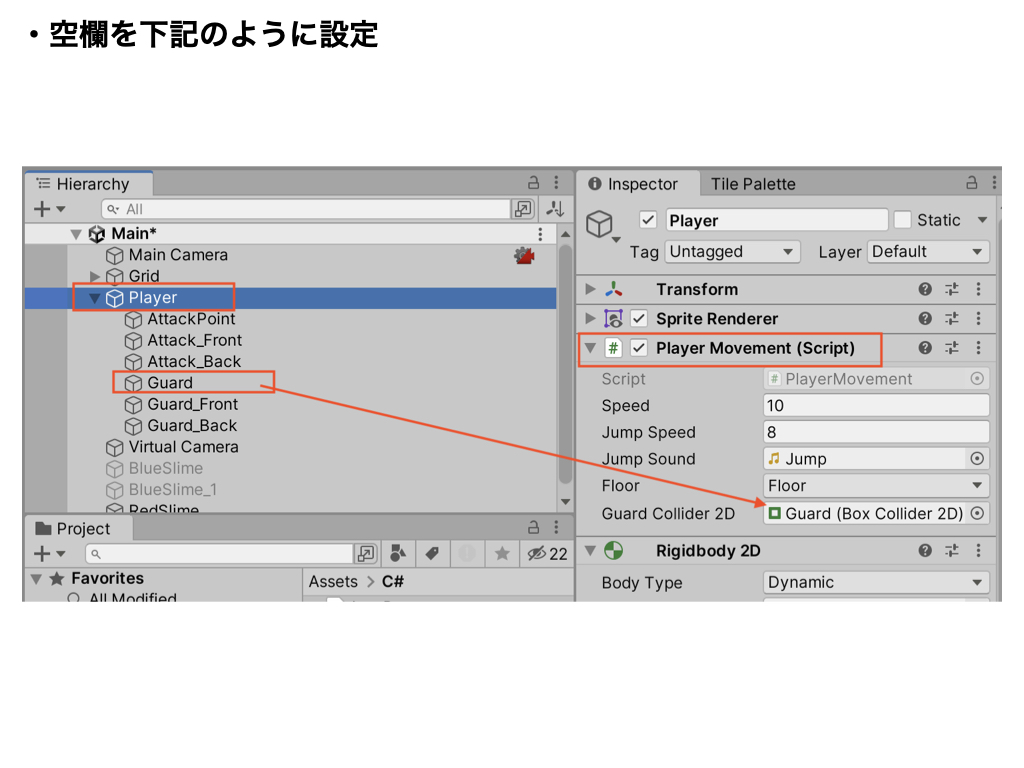
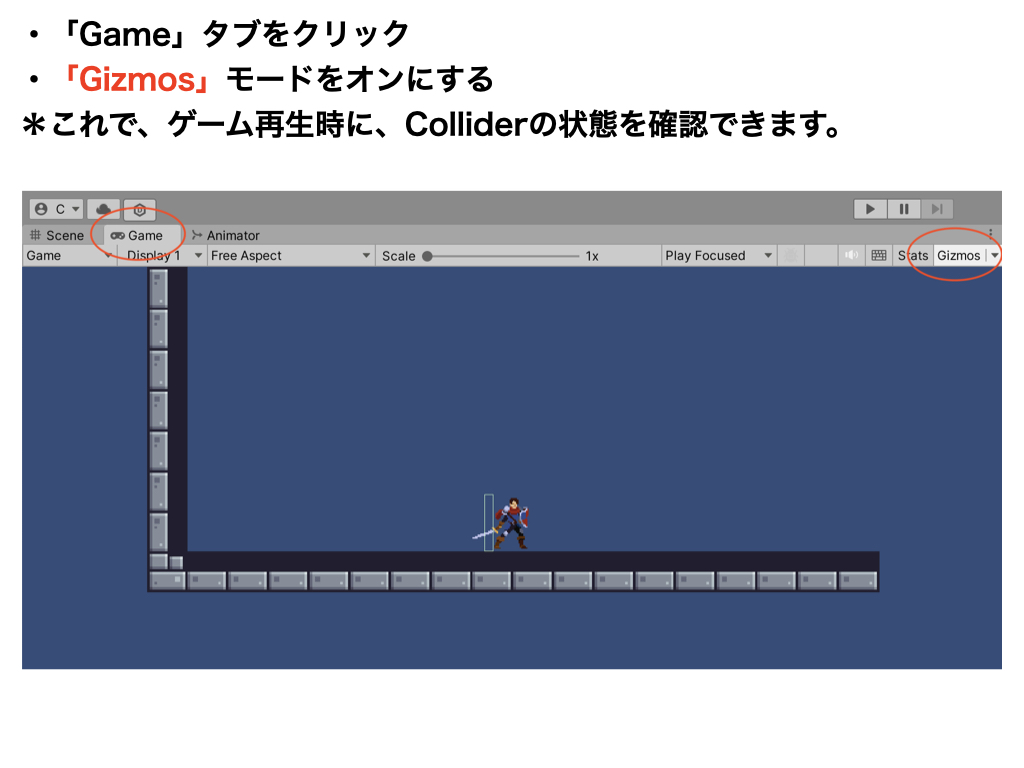
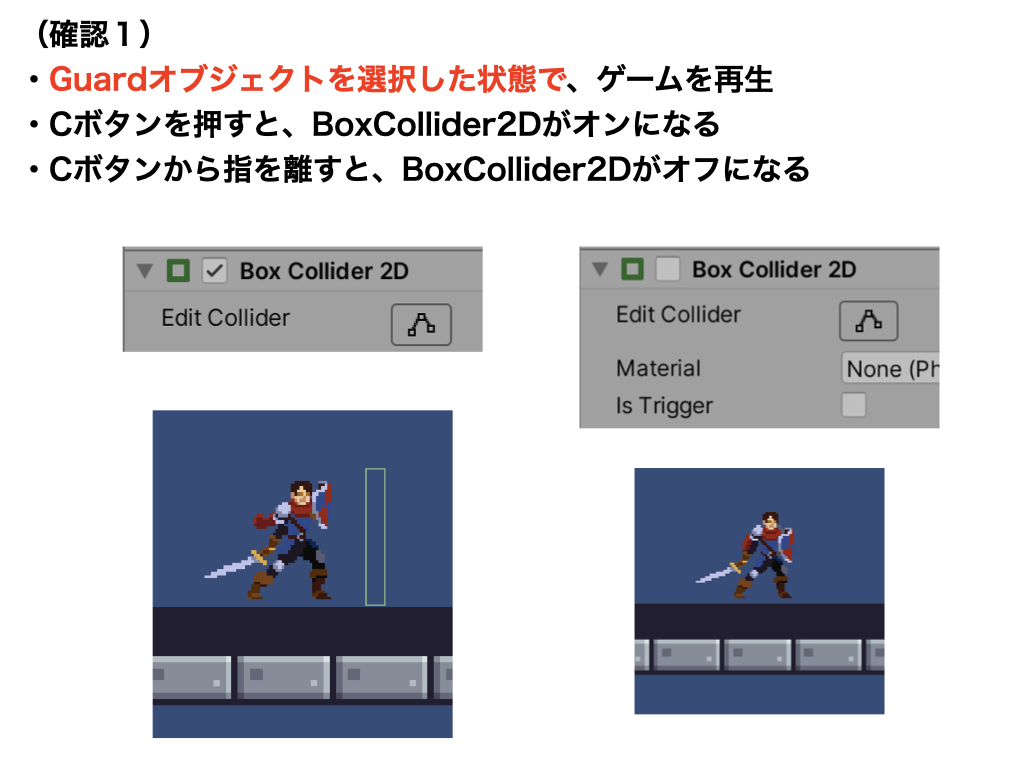
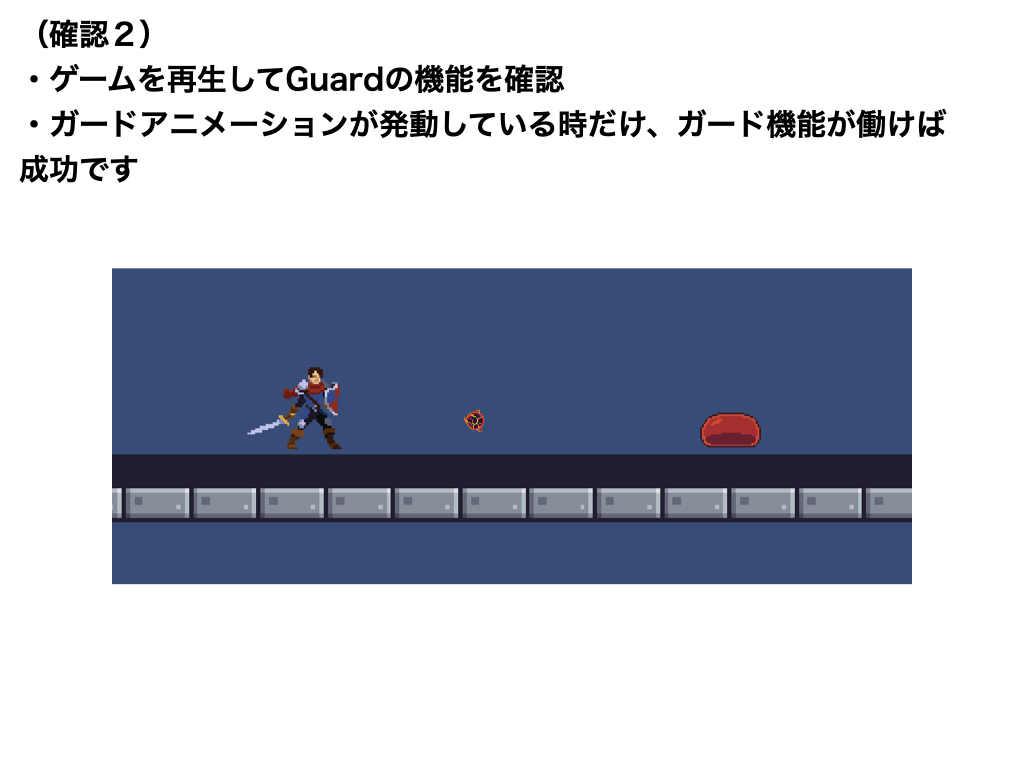
【2022版】DarkCastle(全39回)
他のコースを見る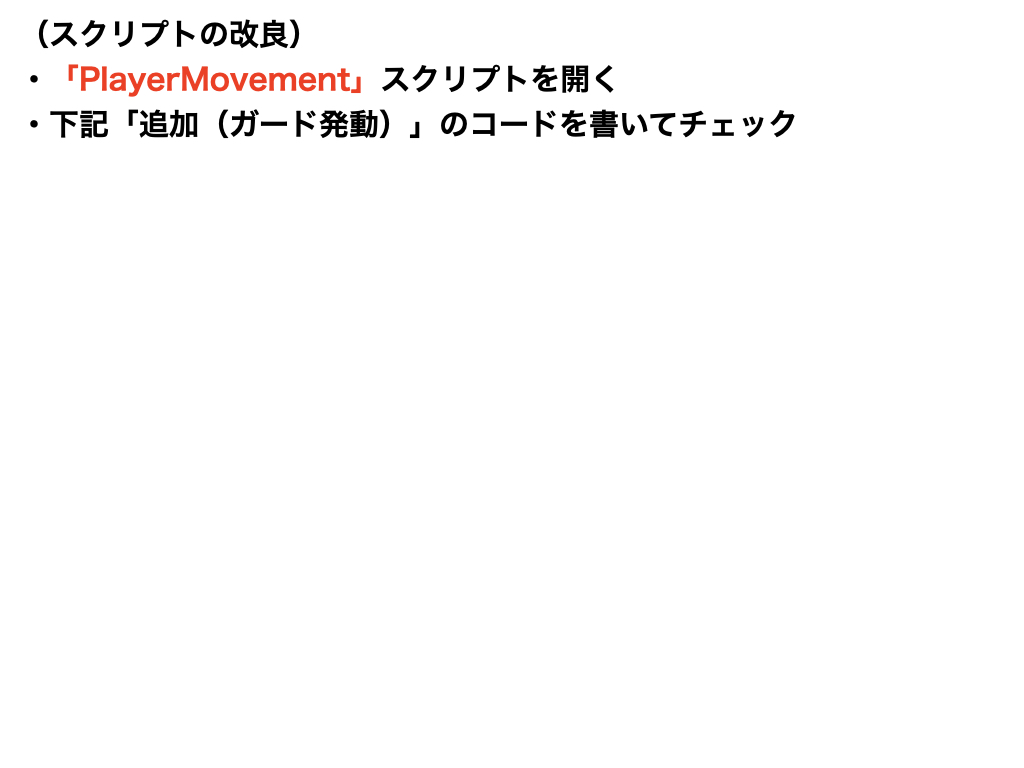
ガード発動
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed;
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
private bool isGard = false;
// ★追加(ガード発動)
public BoxCollider2D guardCollider2D;
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
// ★追加(ガード発動)
// ゲーム開始時点ではガードはOFF
guardCollider2D.enabled = false;
}
void Update()
{
if(isGard == false)
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
}
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
else if (Input.GetKeyDown(KeyCode.X))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack2");
}
if (Input.GetKeyDown(KeyCode.C))
{
if (moveH != 0)
{
return;
}
animator.SetBool("Guard", true);
isGard = true;
// ★追加(ガード発動)
guardCollider2D.enabled = true;
}
else if (Input.GetKeyUp(KeyCode.C))
{
animator.SetBool("Guard", false);
isGard = false;
// ★追加(ガード発動)
guardCollider2D.enabled = false;
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
}
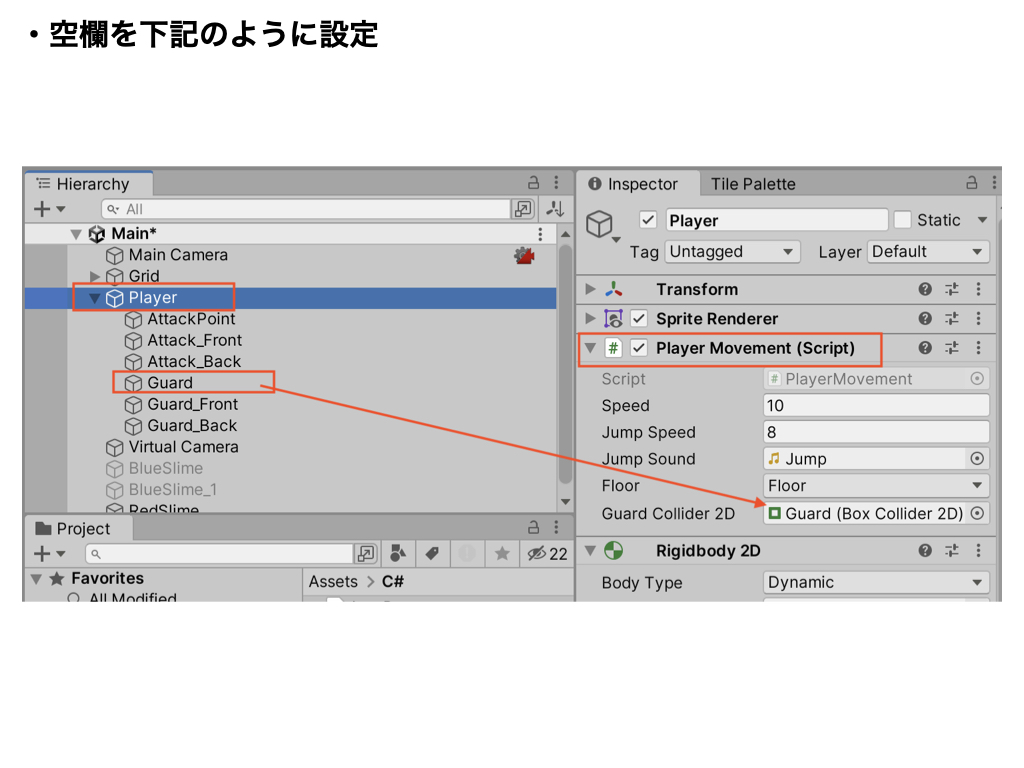
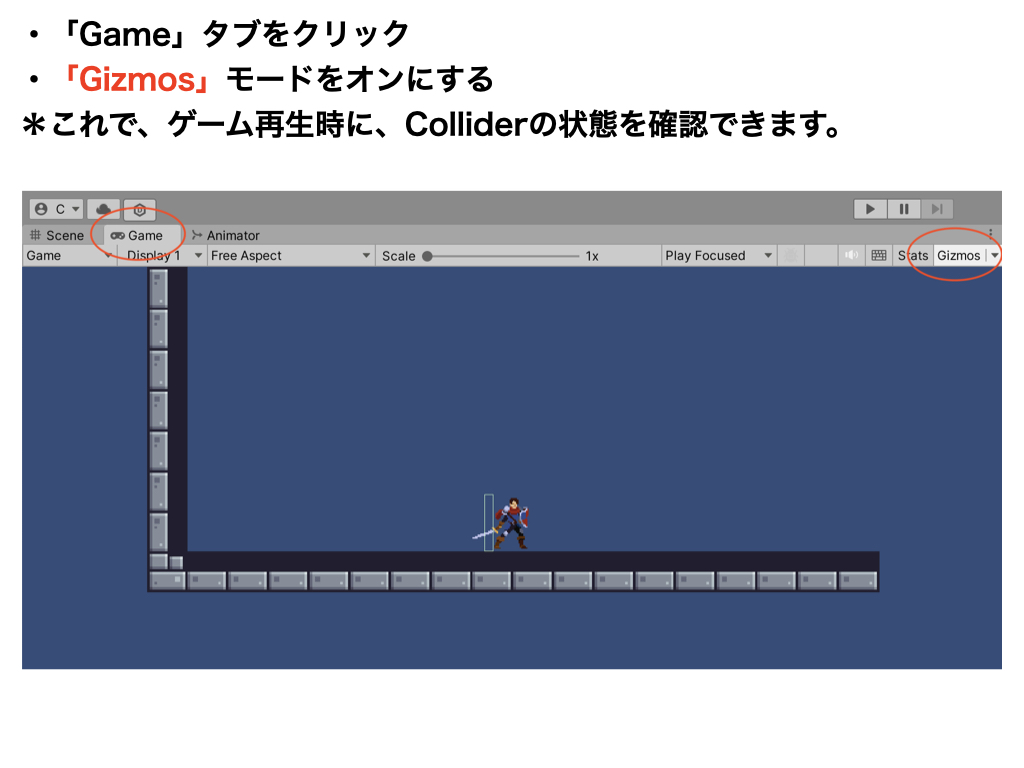
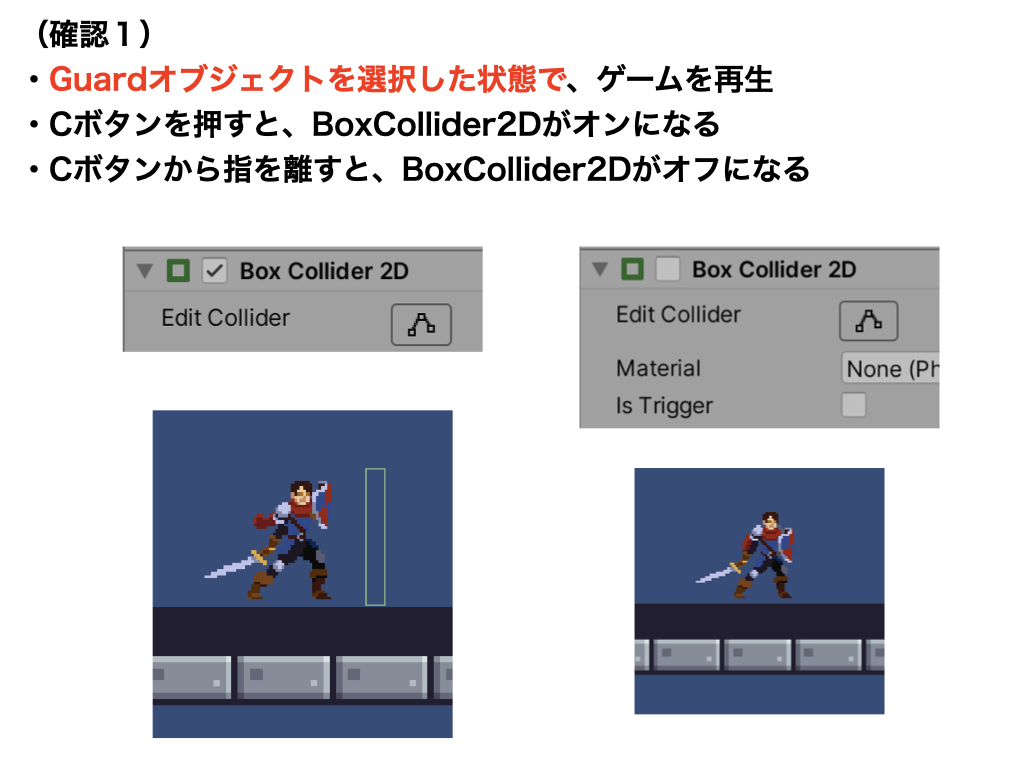
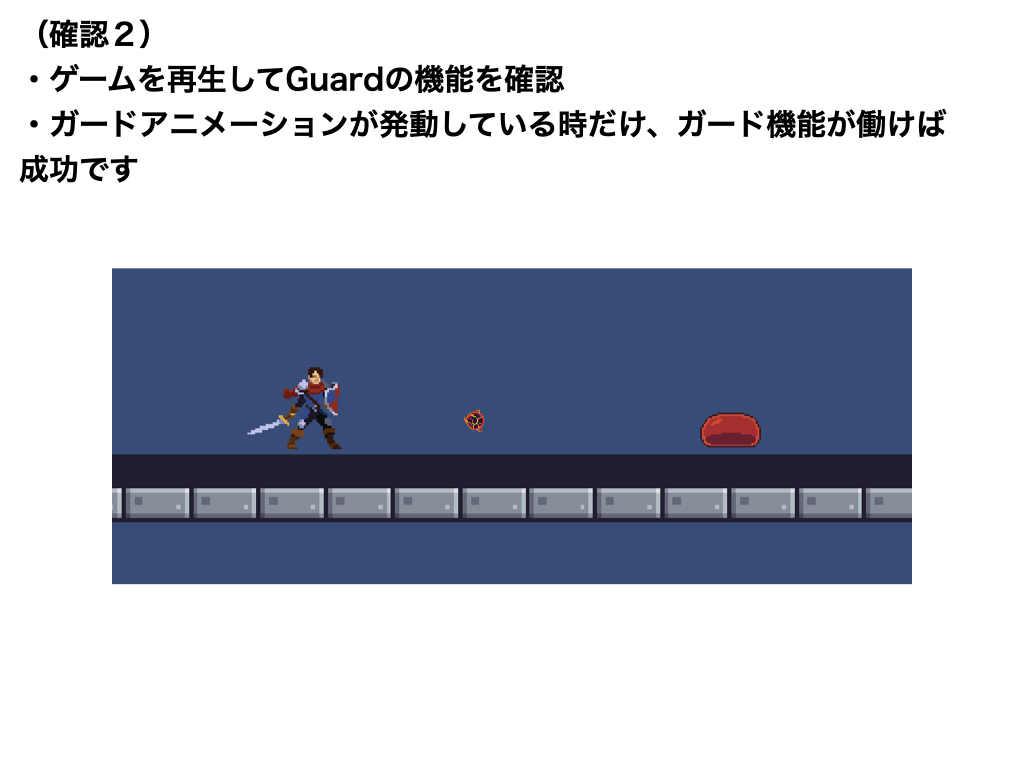
Guard機能のオンとオフをコントロールする