ソニックビームを発射する
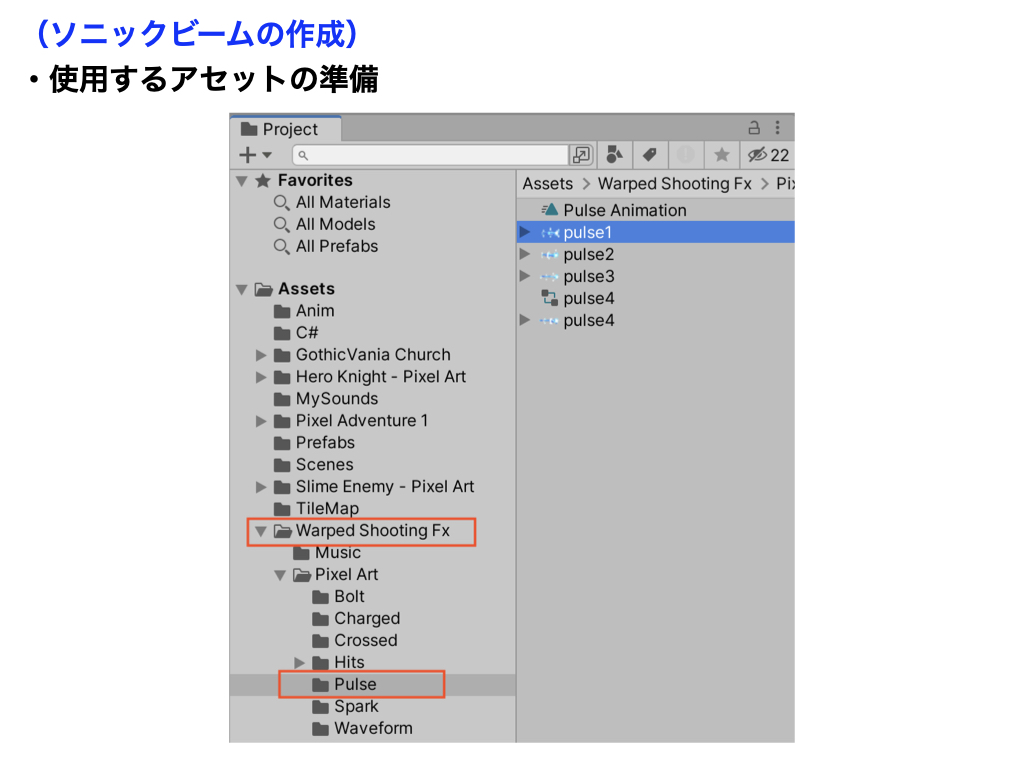
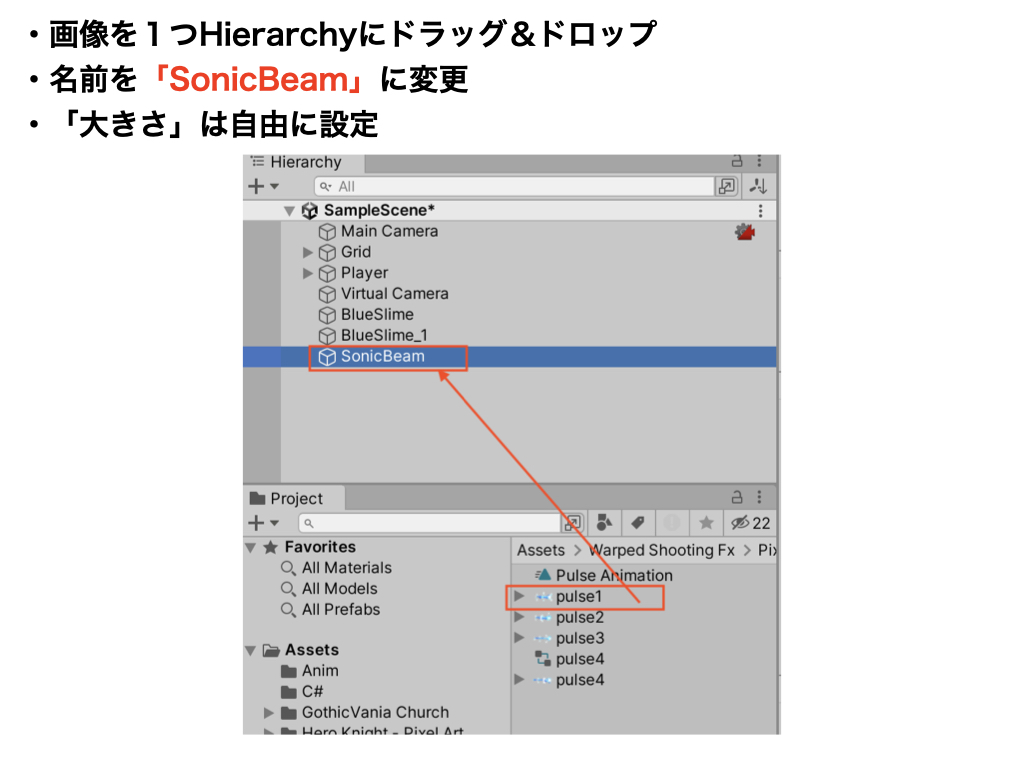
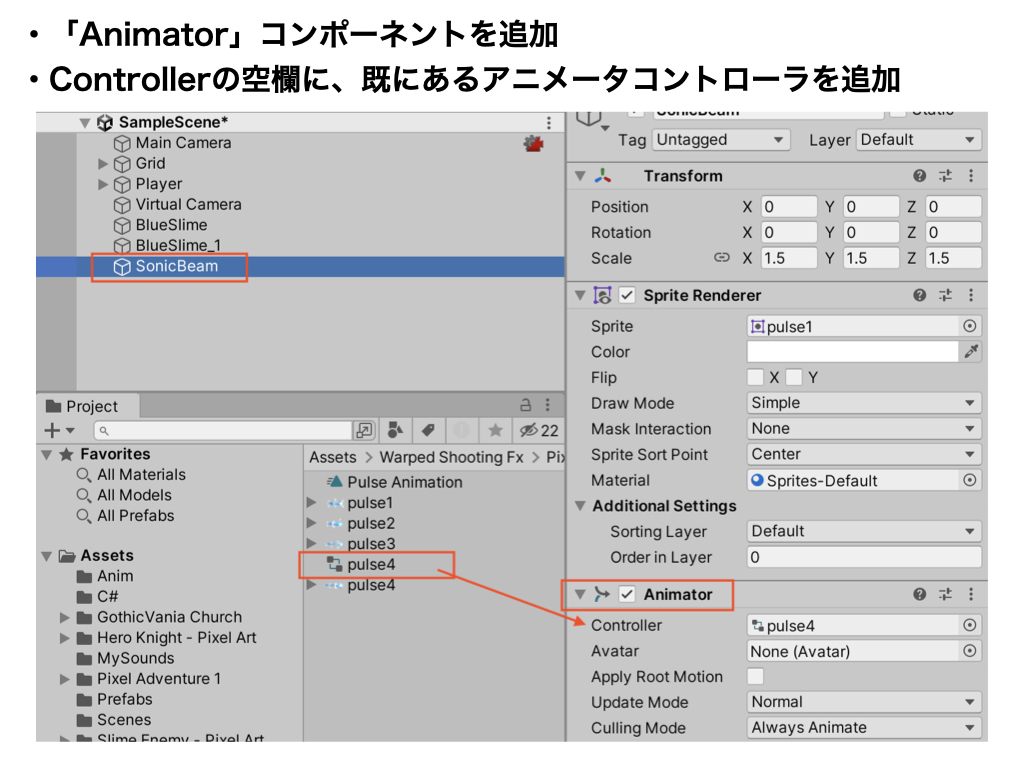
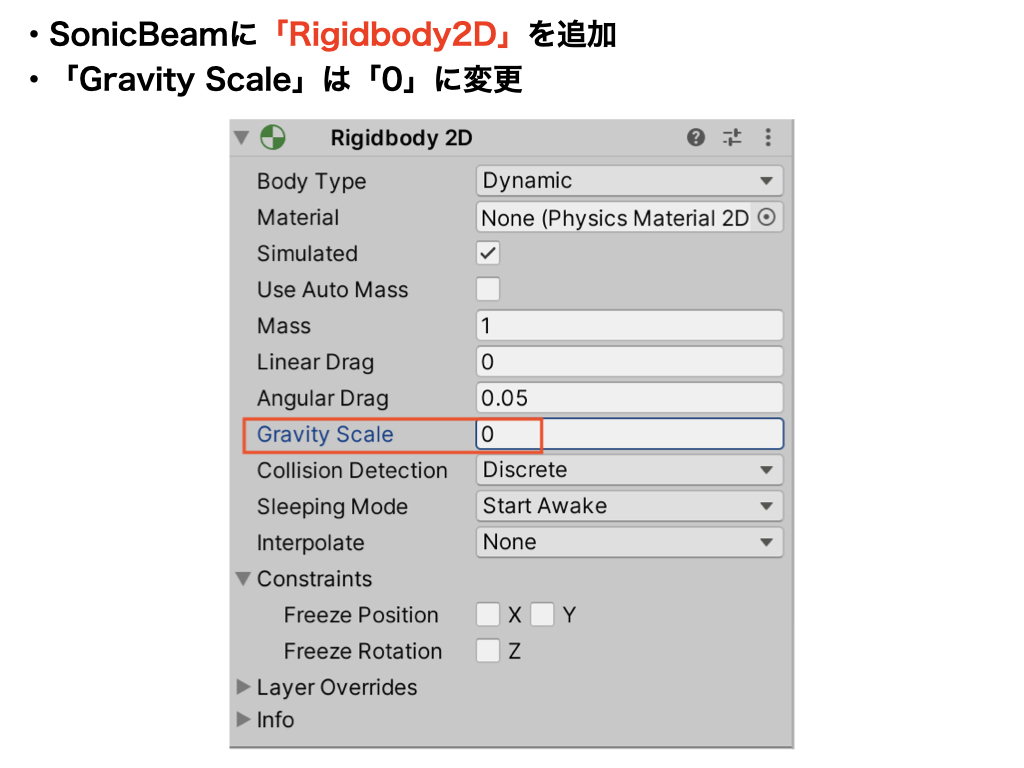
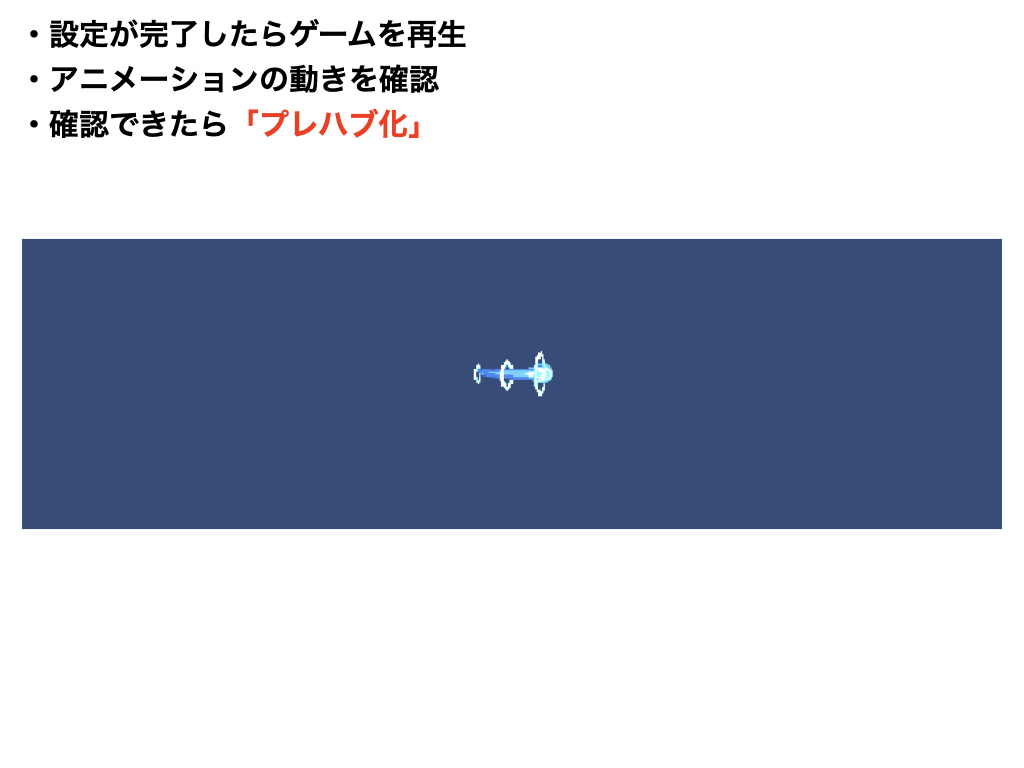
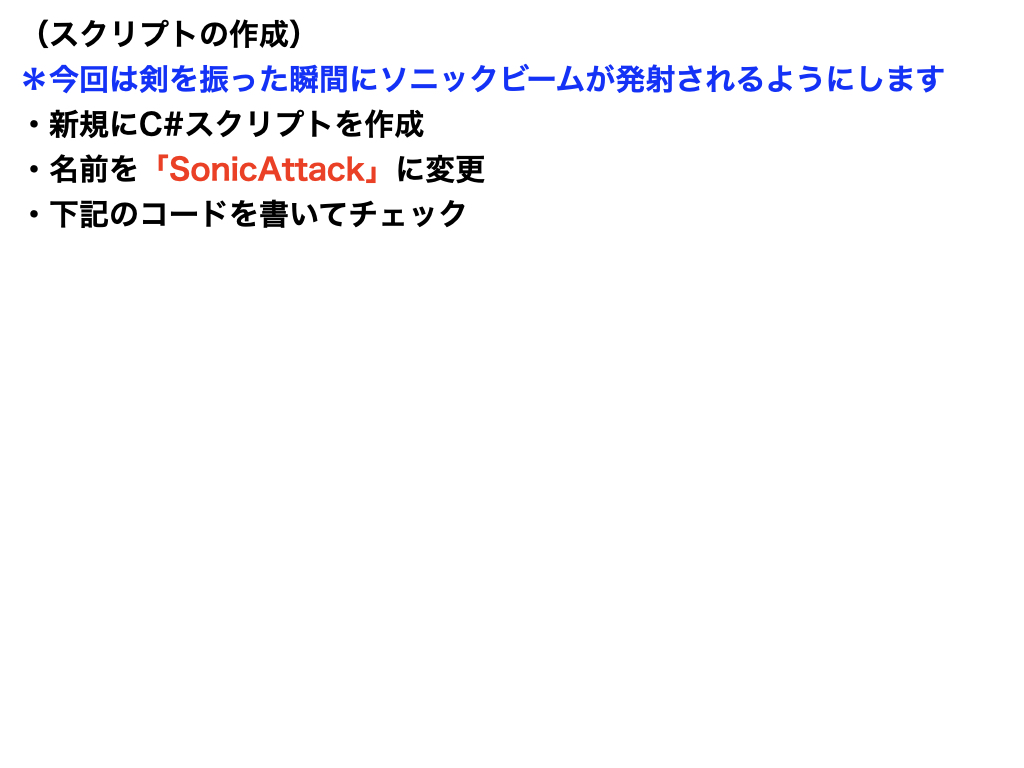
ソニックを発射する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SonicAttack : MonoBehaviour
{
public GameObject sonicPrefab;
public GameObject attackPoint;
public GameObject attack_front;
public GameObject attack_back;
private PlayerMovement pm;
private float num;
private int sonicRotation;
private int sonicNum = 1;
void Start()
{
pm = GetComponent<PlayerMovement>();
attackPoint.transform.position = attack_front.transform.position;
}
void Update()
{
// Playerの向きと「ソニックの画像の向き」「ソニックを飛ばす向き」を合致させる(ポイント)
num = pm.moveH;
if (num == 1)
{
attackPoint.transform.position = attack_front.transform.position;
sonicRotation = 0; // ソニックの画像を右向きにする
sonicNum = 1; // ソニックを右方向に飛ばす
}
else if (num == -1)
{
attackPoint.transform.position = attack_back.transform.position;
sonicRotation = 180; // ソニックの画像を左向きにする
sonicNum = -1; // ソニックを左方向に飛ばす
}
}
// ソニックの発射はアニメションから実行
public void S_Attack()
{
// sonicRotationでソニックビームの画像の向きを調整
GameObject sonic = Instantiate(sonicPrefab, attackPoint.transform.position, Quaternion.Euler(0, sonicRotation, 0));
Rigidbody2D sonicRb2d = sonic.GetComponent<Rigidbody2D>();
// numを使って、ソニックの飛ぶ方向を調整
sonicRb2d.AddForce(transform.right * 1000 * sonicNum);
Destroy(sonic, 5.0f);
}
}
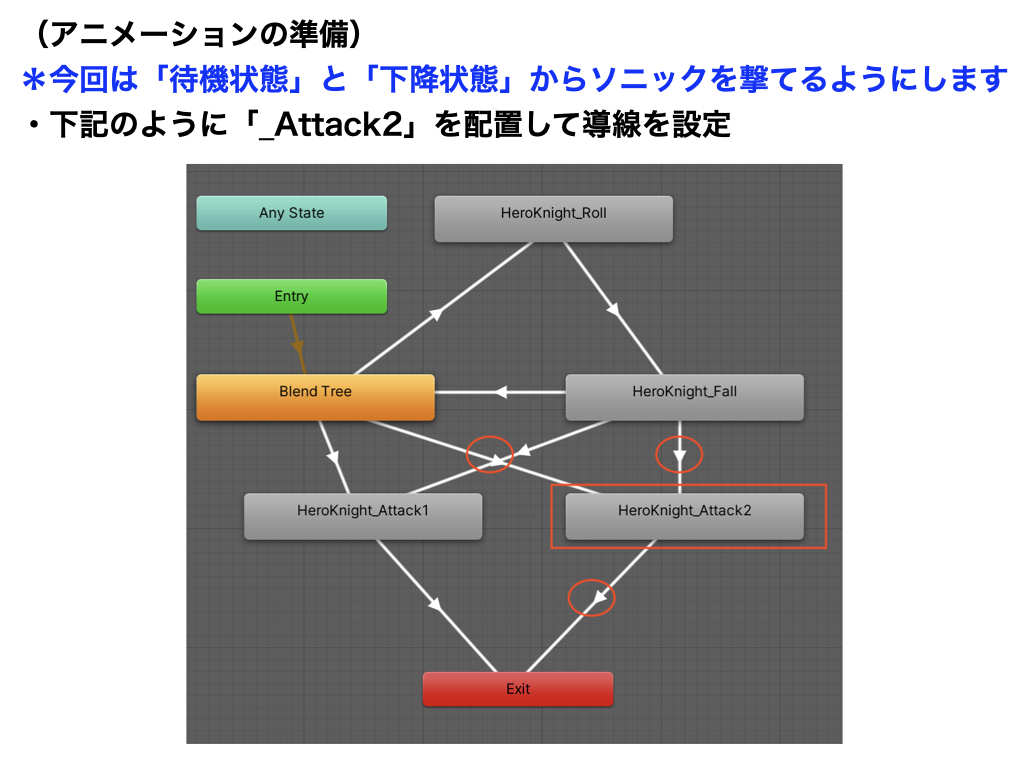
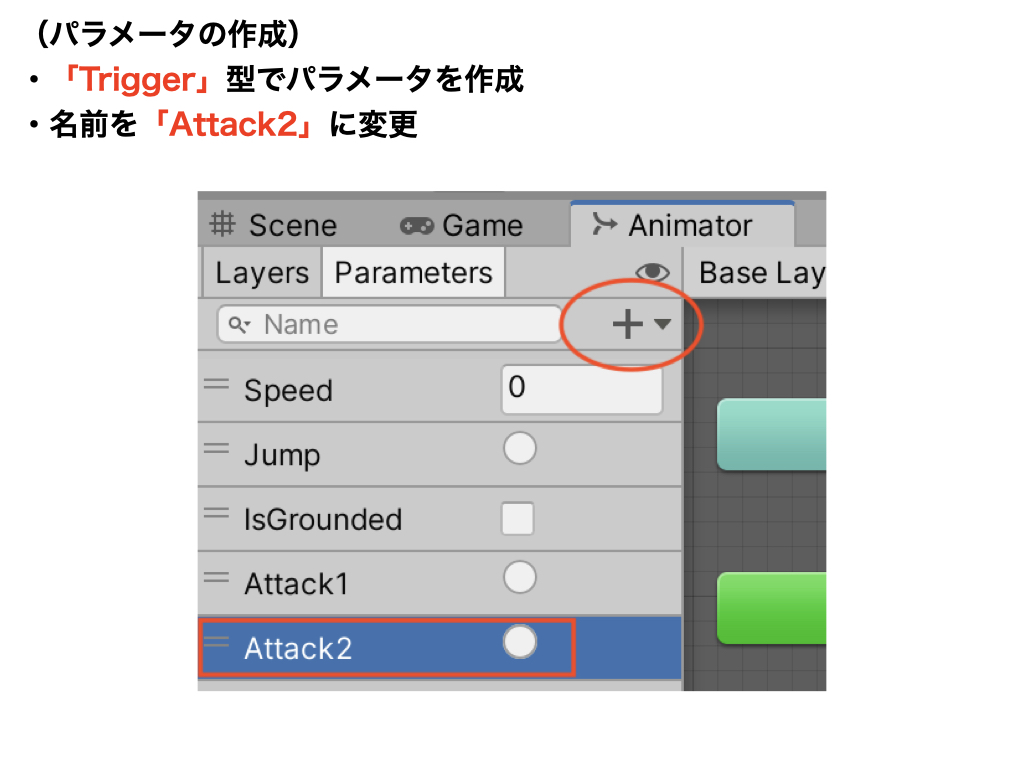
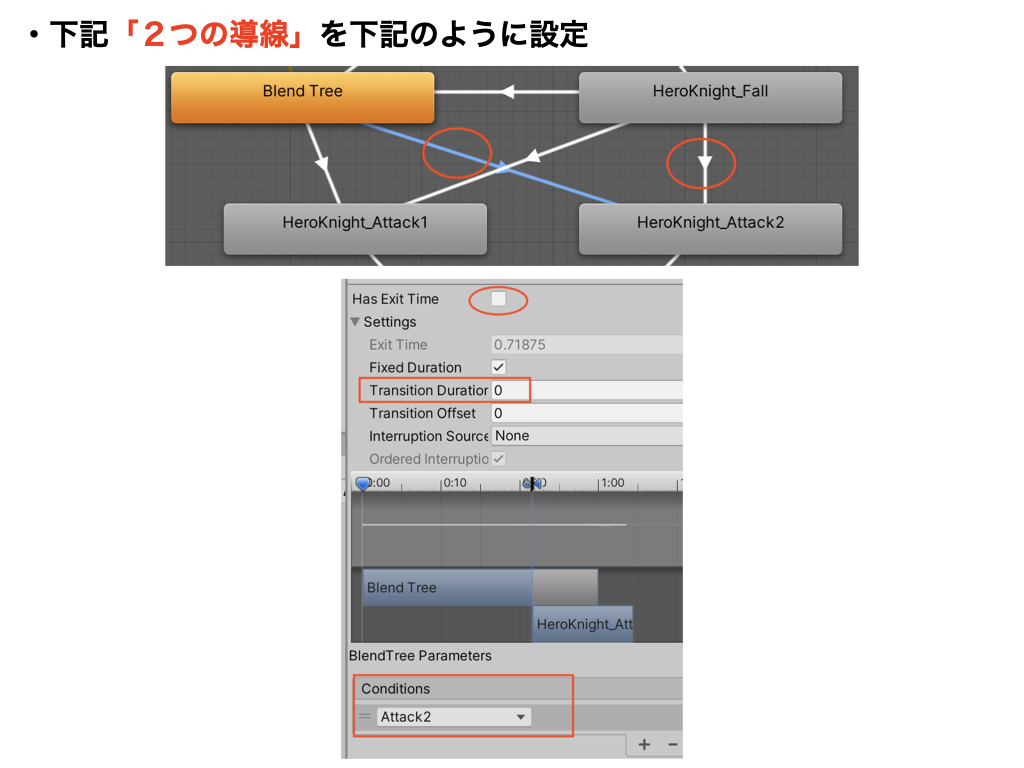
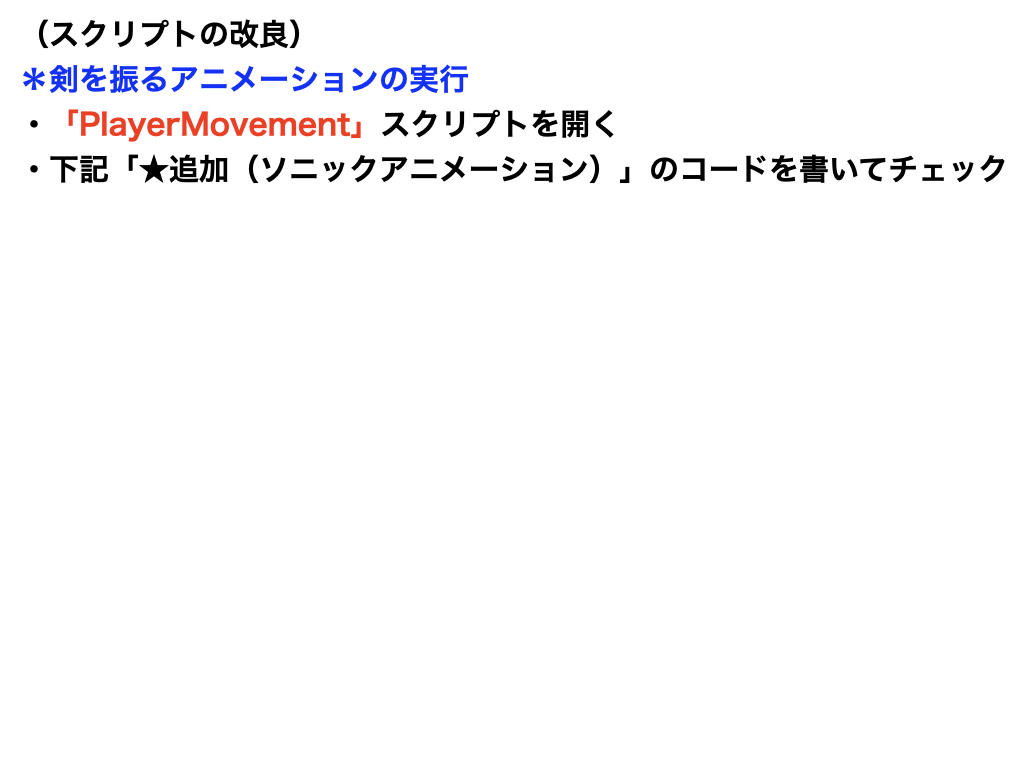
剣を振るアニメーション
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed;
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
// ★追加(ソニックアニメーション)
public AudioClip sonicSound;
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
}
void Update()
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
// ★追加(ソニックアニメーション)
else if (Input.GetKeyDown(KeyCode.X)) // キーは自由に変更可能
{
// 移動中は不可
if (moveH != 0)
{
return;
}
AudioSource.PlayClipAtPoint(sonicSound, transform.position);
animator.SetTrigger("Attack2");
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
}
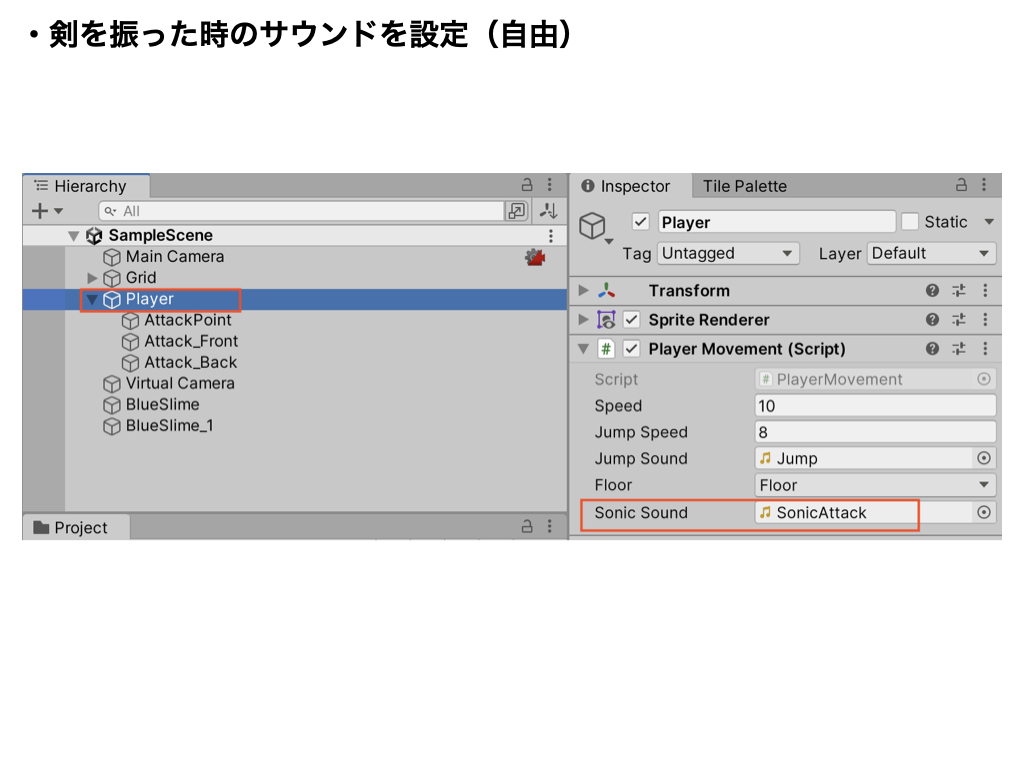
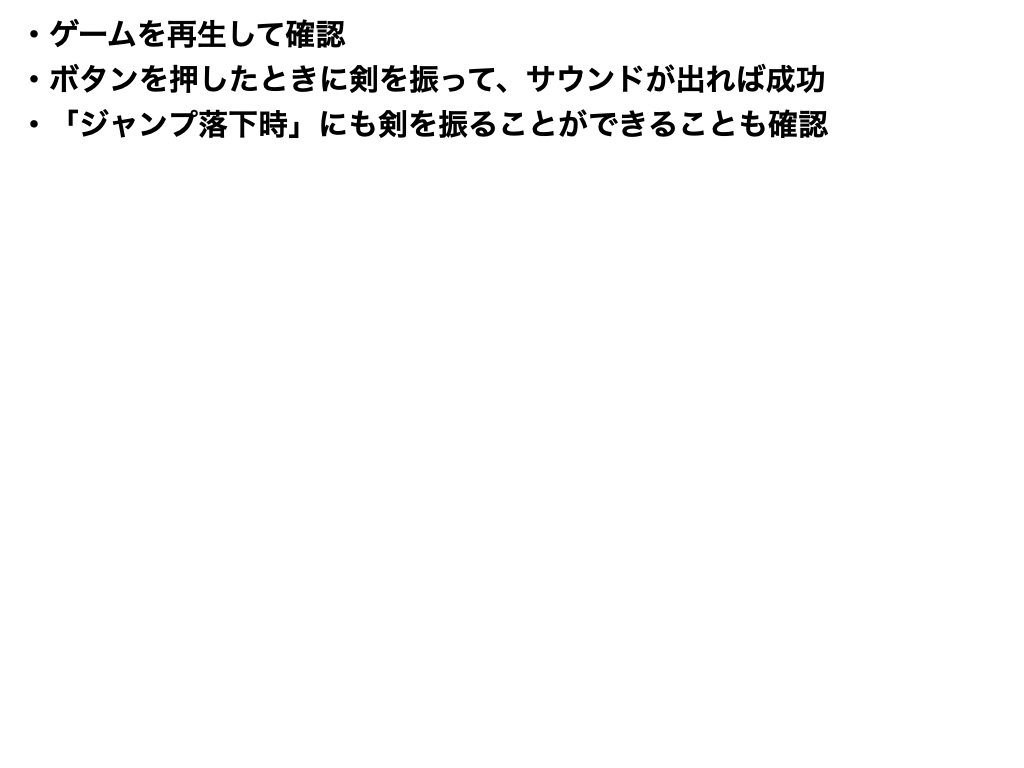
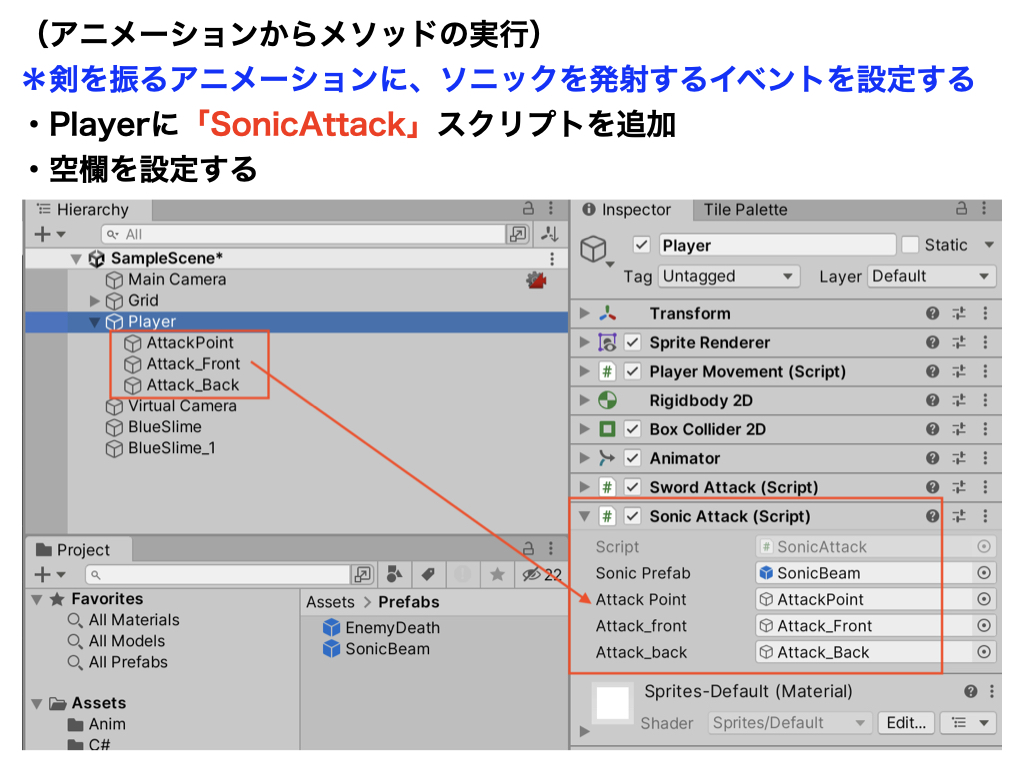
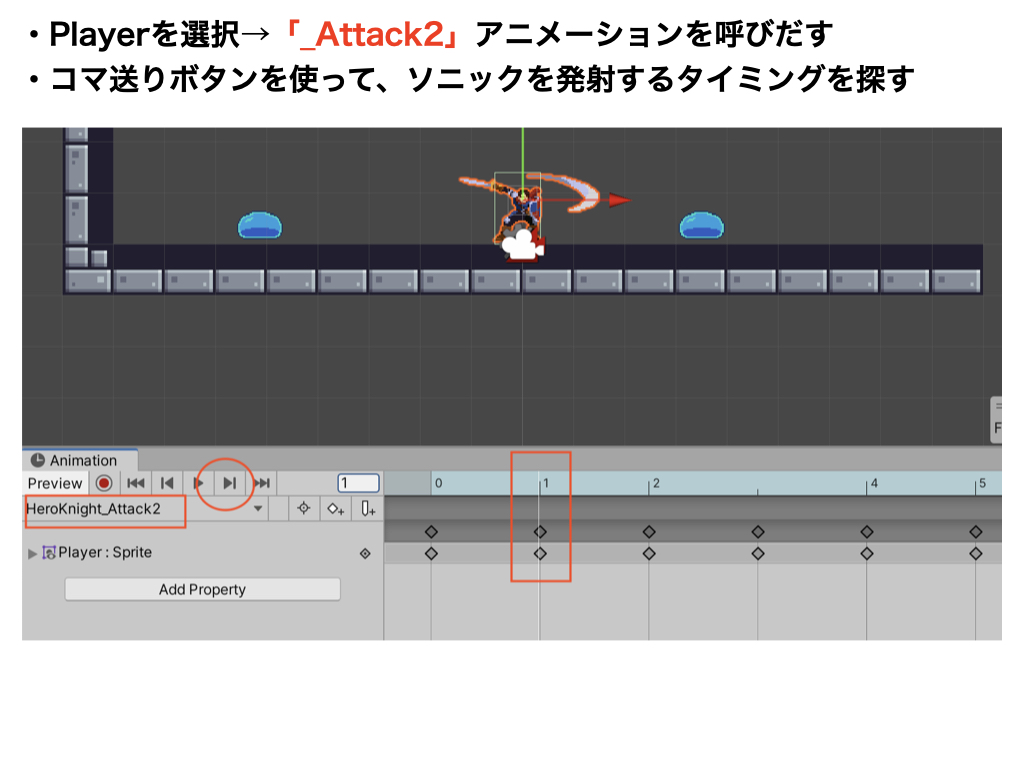
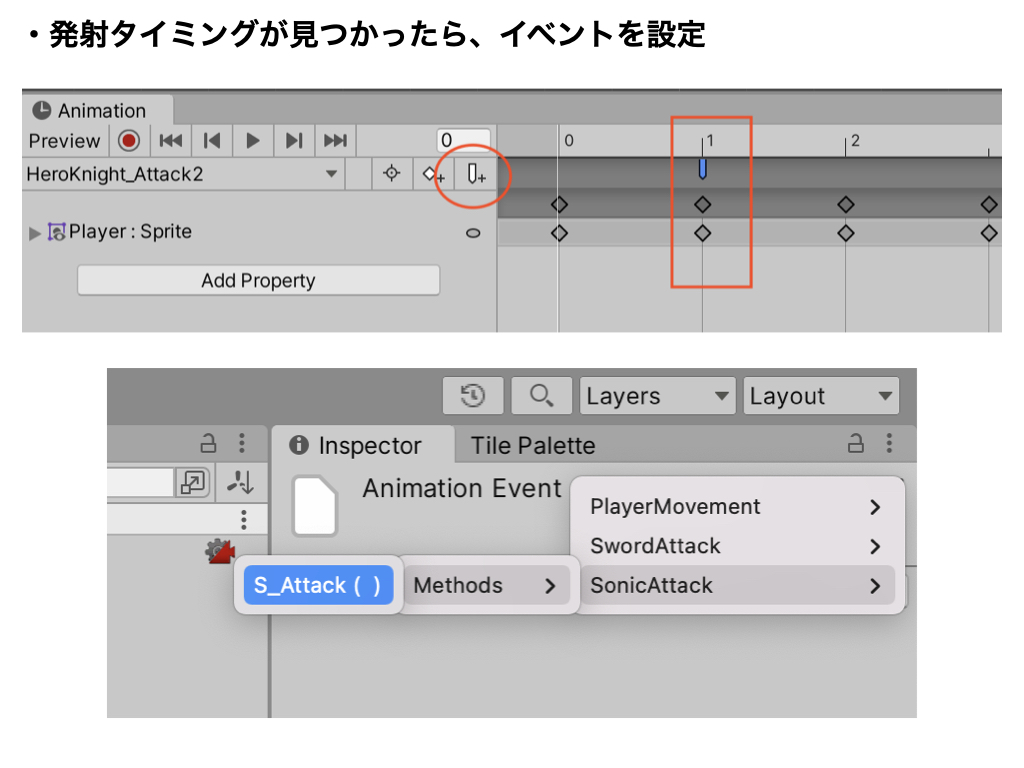
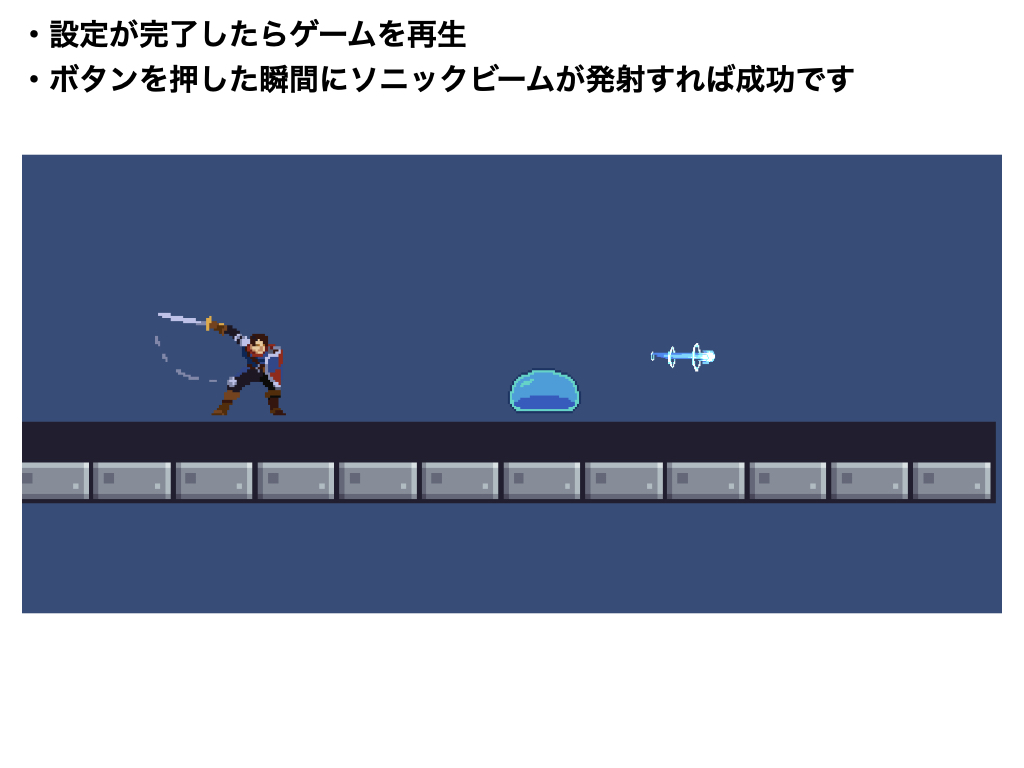
【2022版】DarkCastle(全39回)
他のコースを見る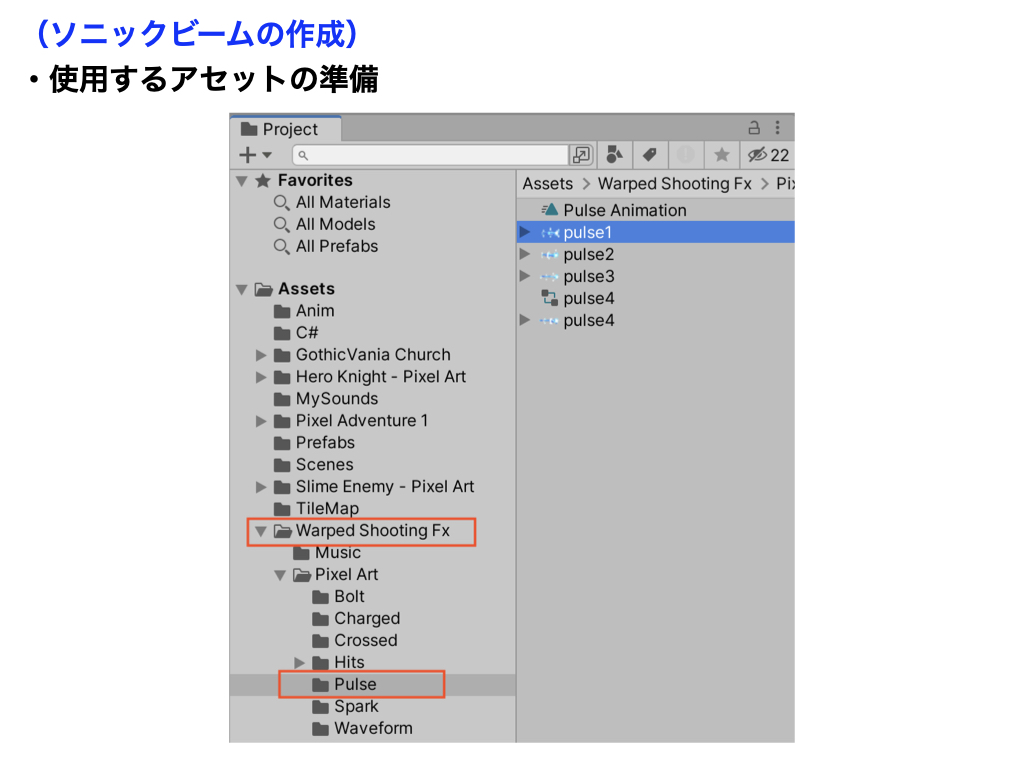
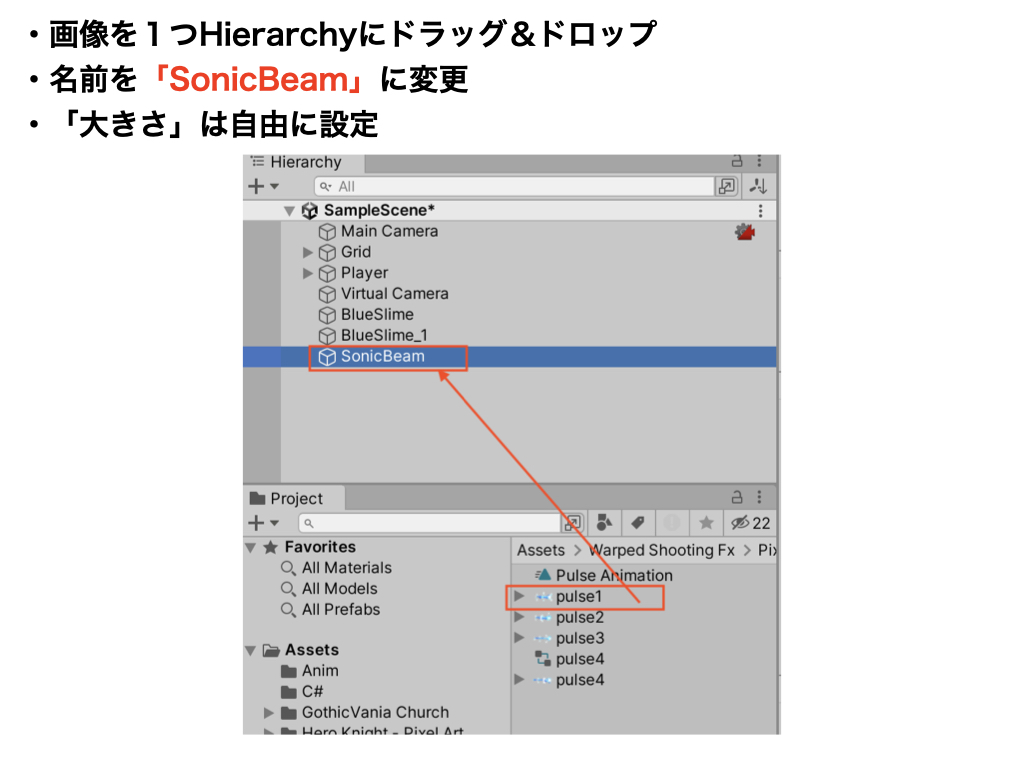
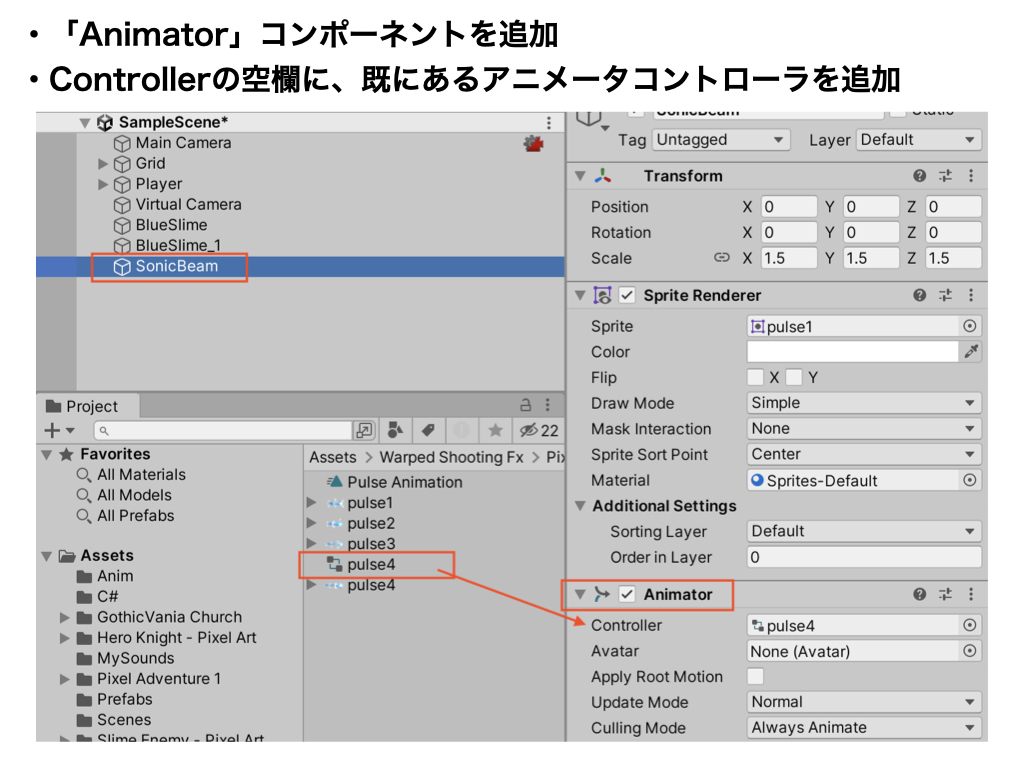
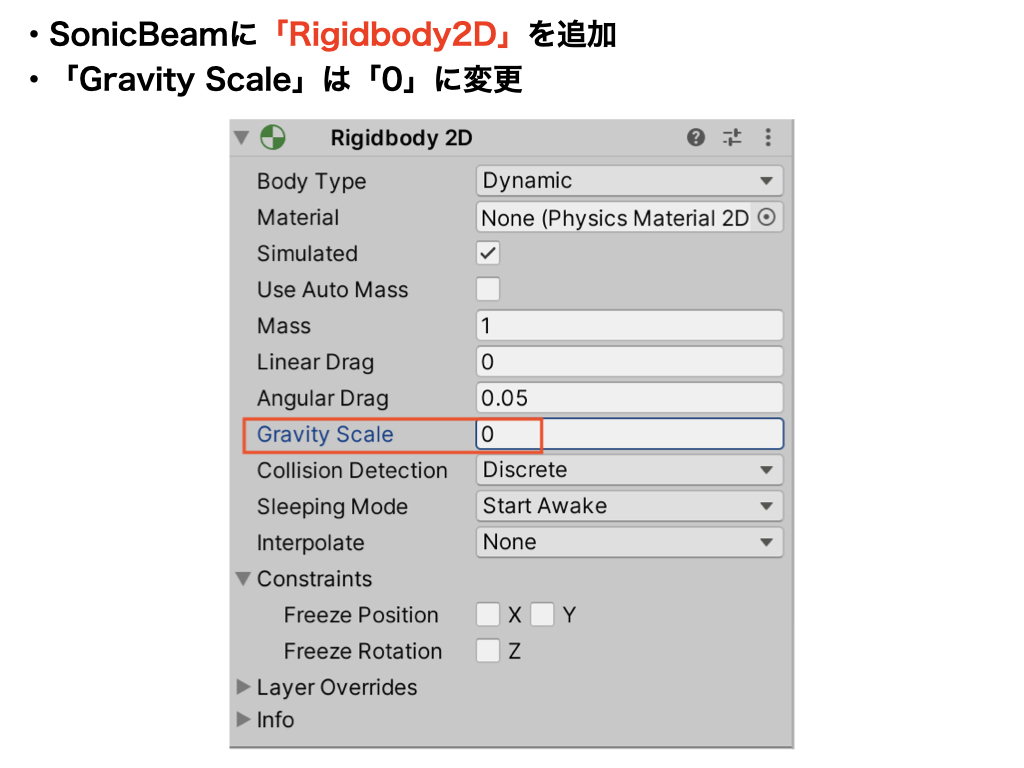
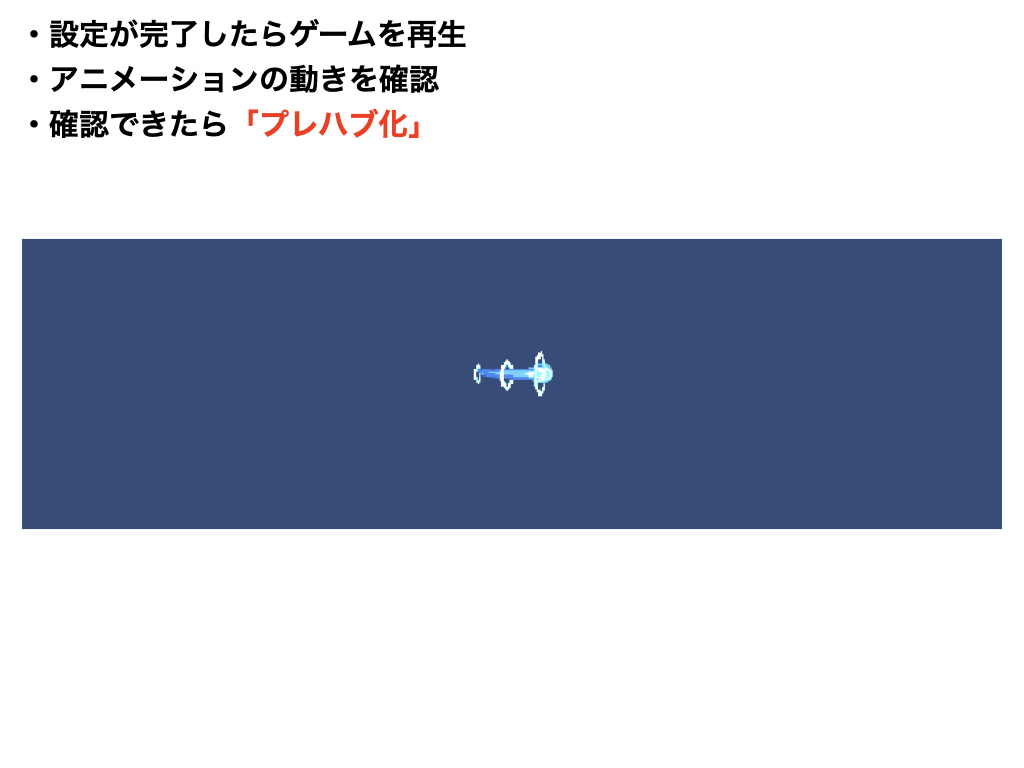
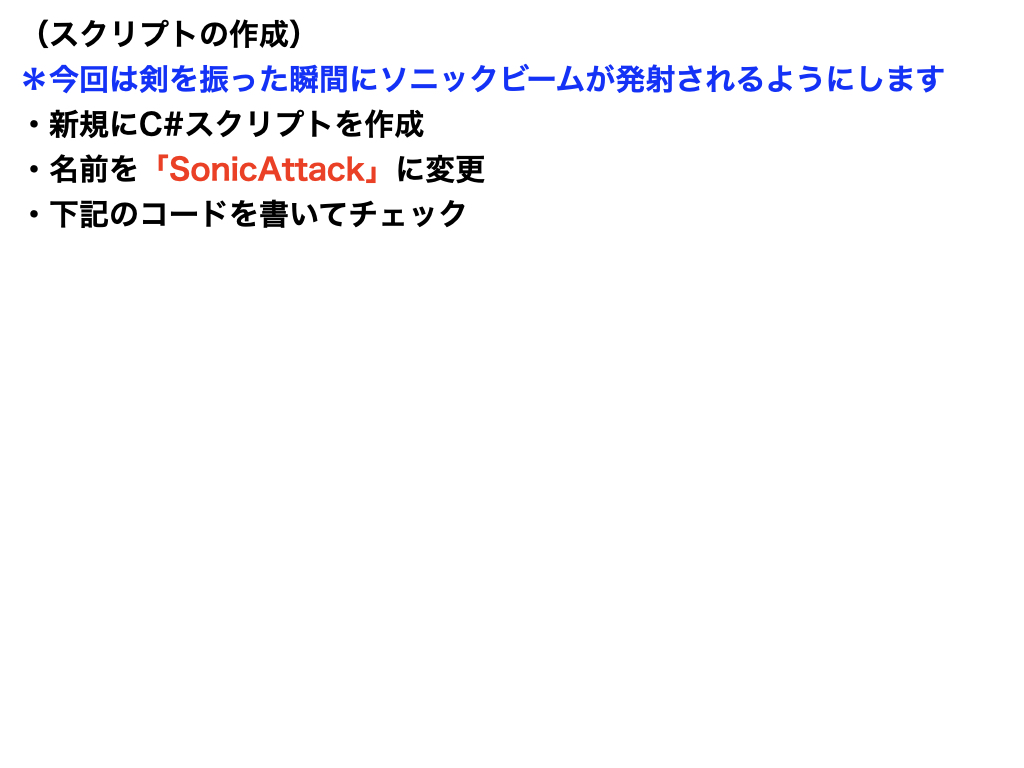
ソニックを発射する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SonicAttack : MonoBehaviour
{
public GameObject sonicPrefab;
public GameObject attackPoint;
public GameObject attack_front;
public GameObject attack_back;
private PlayerMovement pm;
private float num;
private int sonicRotation;
private int sonicNum = 1;
void Start()
{
pm = GetComponent<PlayerMovement>();
attackPoint.transform.position = attack_front.transform.position;
}
void Update()
{
// Playerの向きと「ソニックの画像の向き」「ソニックを飛ばす向き」を合致させる(ポイント)
num = pm.moveH;
if (num == 1)
{
attackPoint.transform.position = attack_front.transform.position;
sonicRotation = 0; // ソニックの画像を右向きにする
sonicNum = 1; // ソニックを右方向に飛ばす
}
else if (num == -1)
{
attackPoint.transform.position = attack_back.transform.position;
sonicRotation = 180; // ソニックの画像を左向きにする
sonicNum = -1; // ソニックを左方向に飛ばす
}
}
// ソニックの発射はアニメションから実行
public void S_Attack()
{
// sonicRotationでソニックビームの画像の向きを調整
GameObject sonic = Instantiate(sonicPrefab, attackPoint.transform.position, Quaternion.Euler(0, sonicRotation, 0));
Rigidbody2D sonicRb2d = sonic.GetComponent<Rigidbody2D>();
// numを使って、ソニックの飛ぶ方向を調整
sonicRb2d.AddForce(transform.right * 1000 * sonicNum);
Destroy(sonic, 5.0f);
}
}
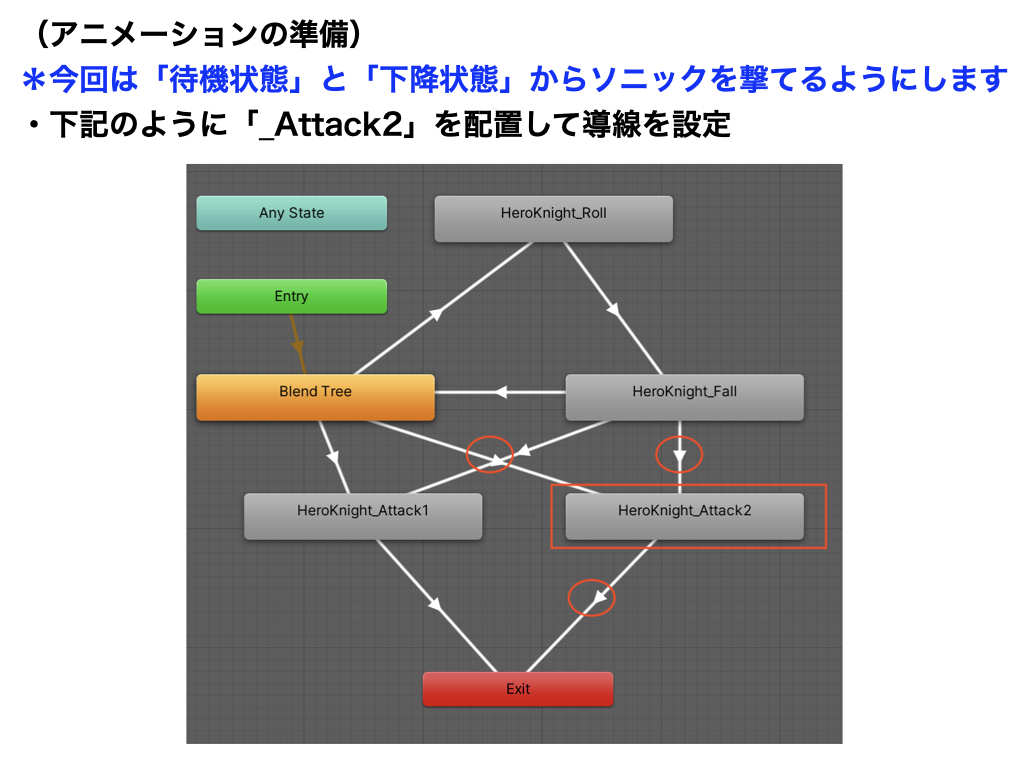
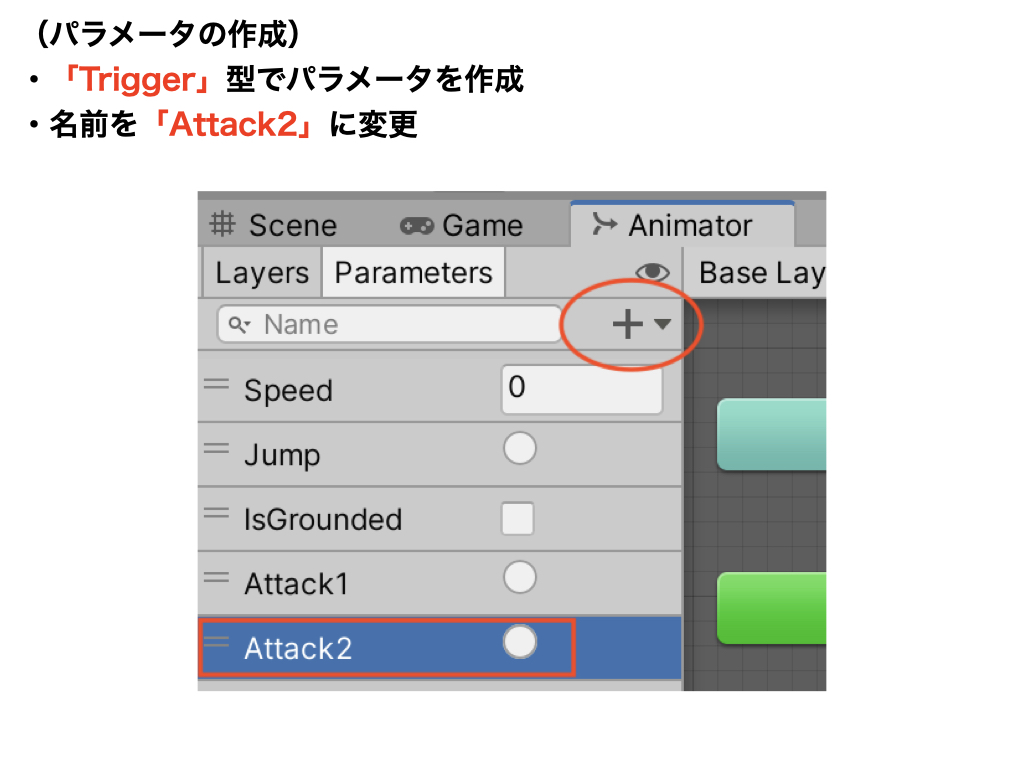
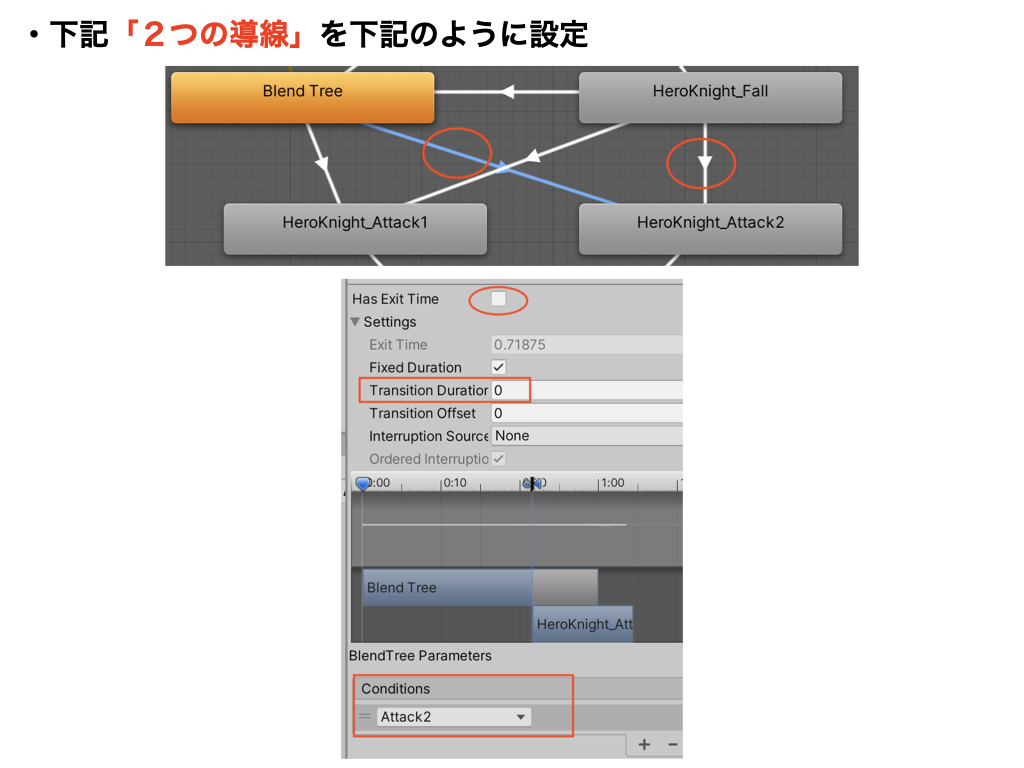
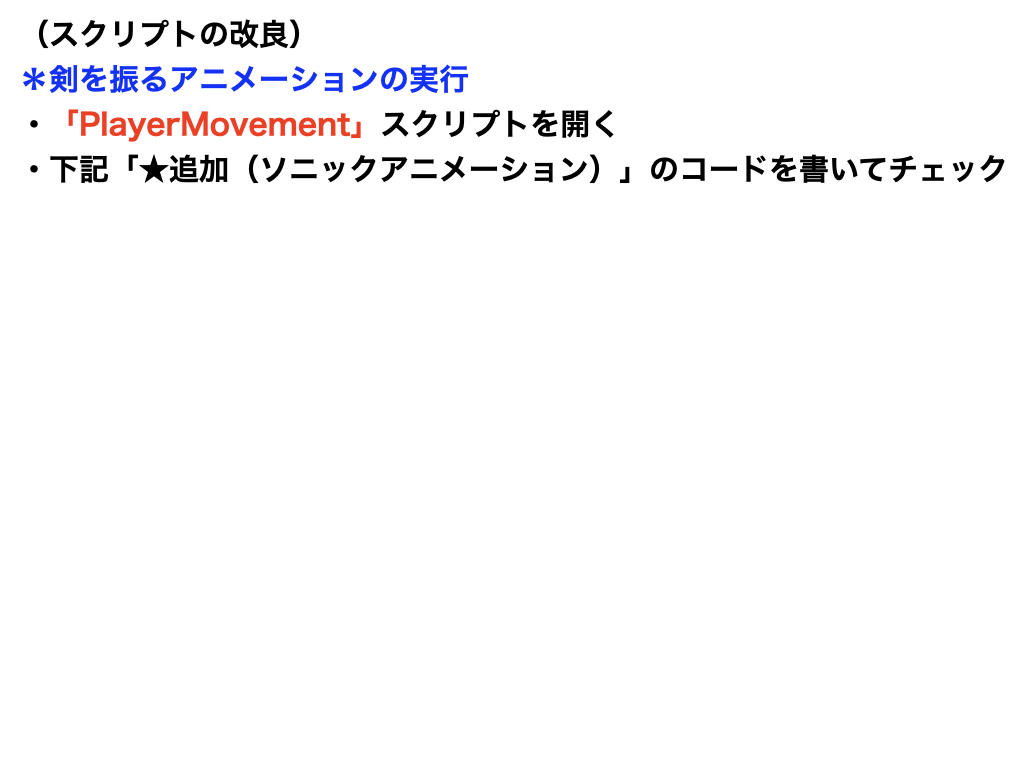
剣を振るアニメーション
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed;
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
// ★追加(ソニックアニメーション)
public AudioClip sonicSound;
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
}
void Update()
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
// ★追加(ソニックアニメーション)
else if (Input.GetKeyDown(KeyCode.X)) // キーは自由に変更可能
{
// 移動中は不可
if (moveH != 0)
{
return;
}
AudioSource.PlayClipAtPoint(sonicSound, transform.position);
animator.SetTrigger("Attack2");
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
}
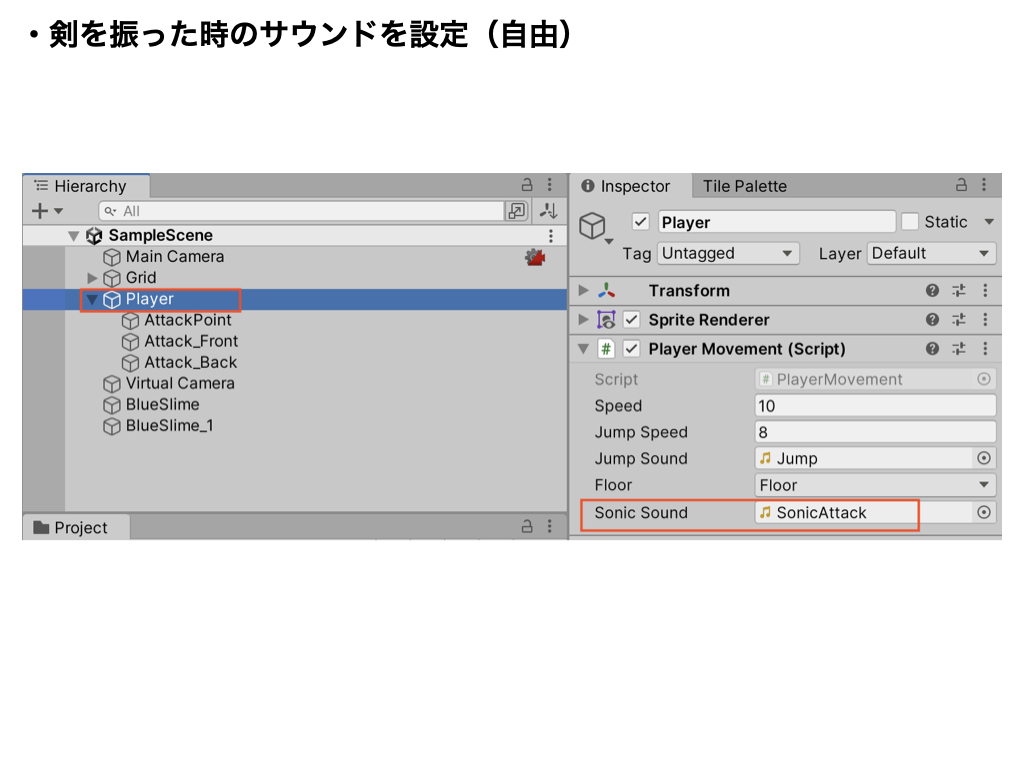
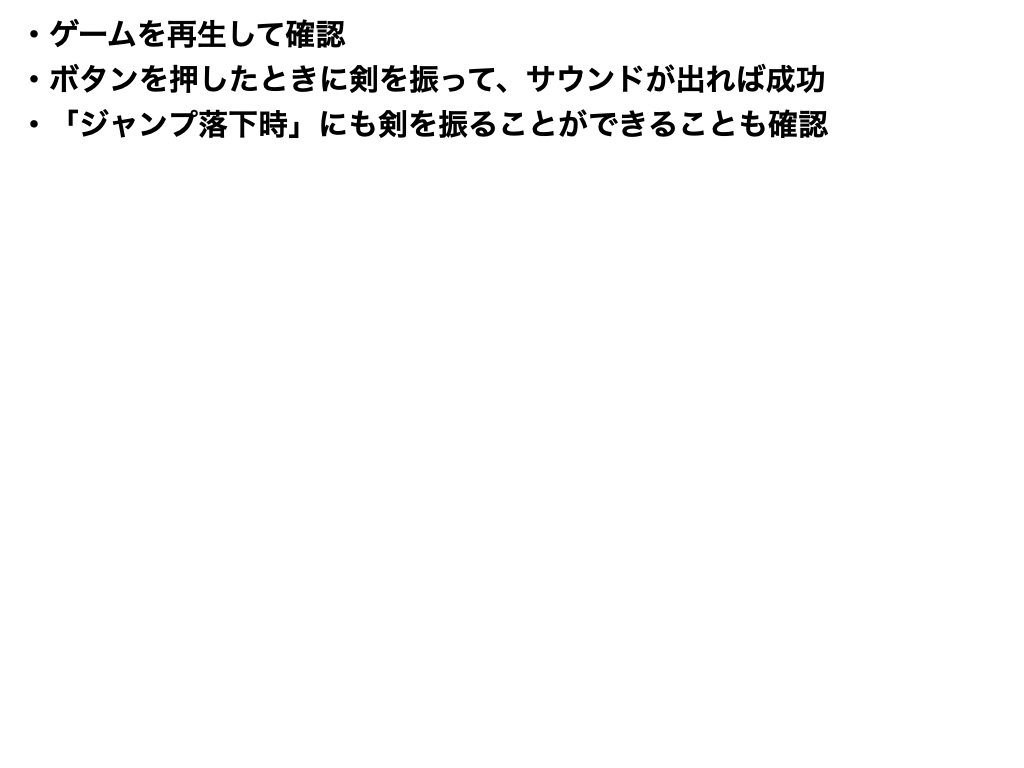
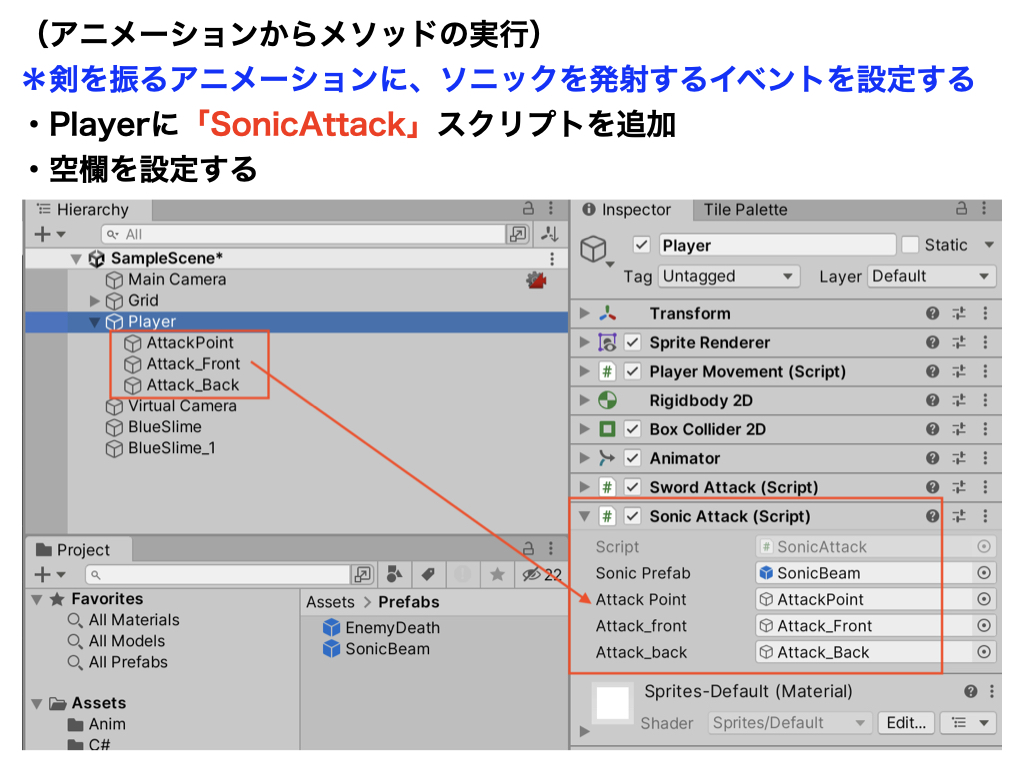
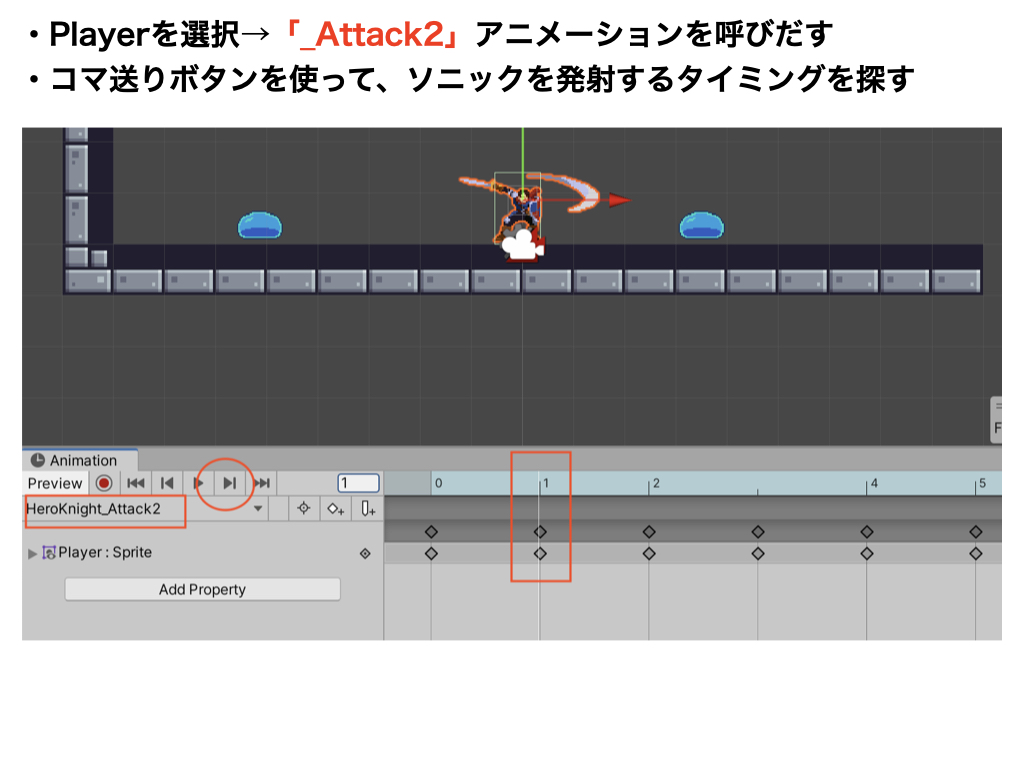
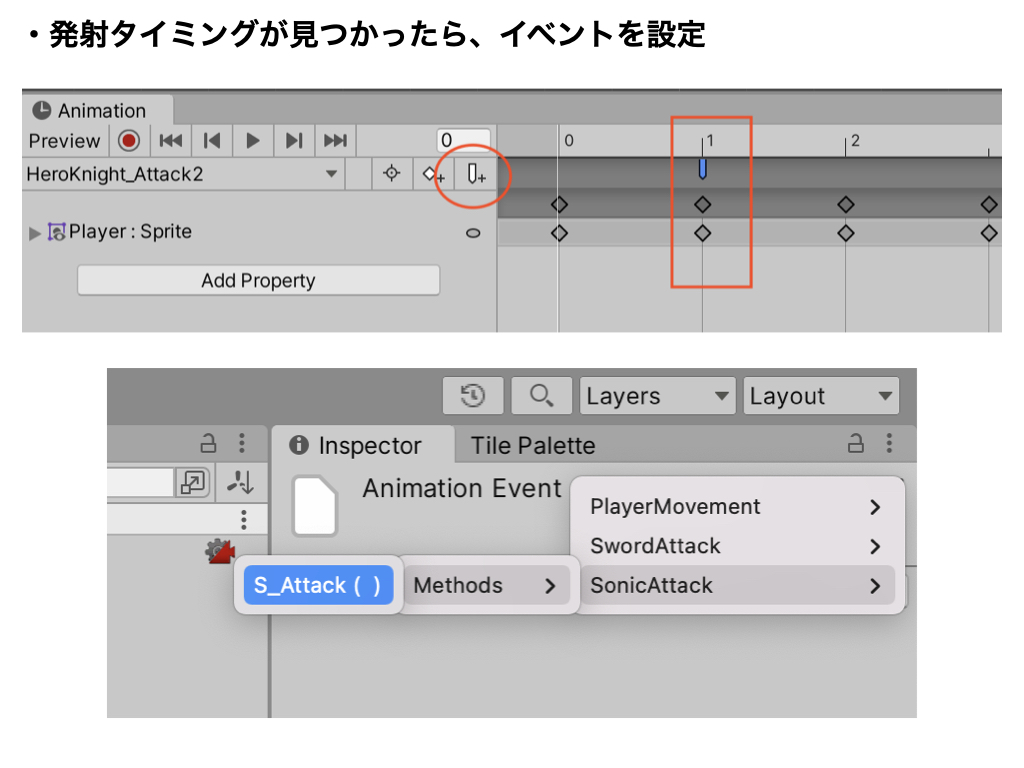
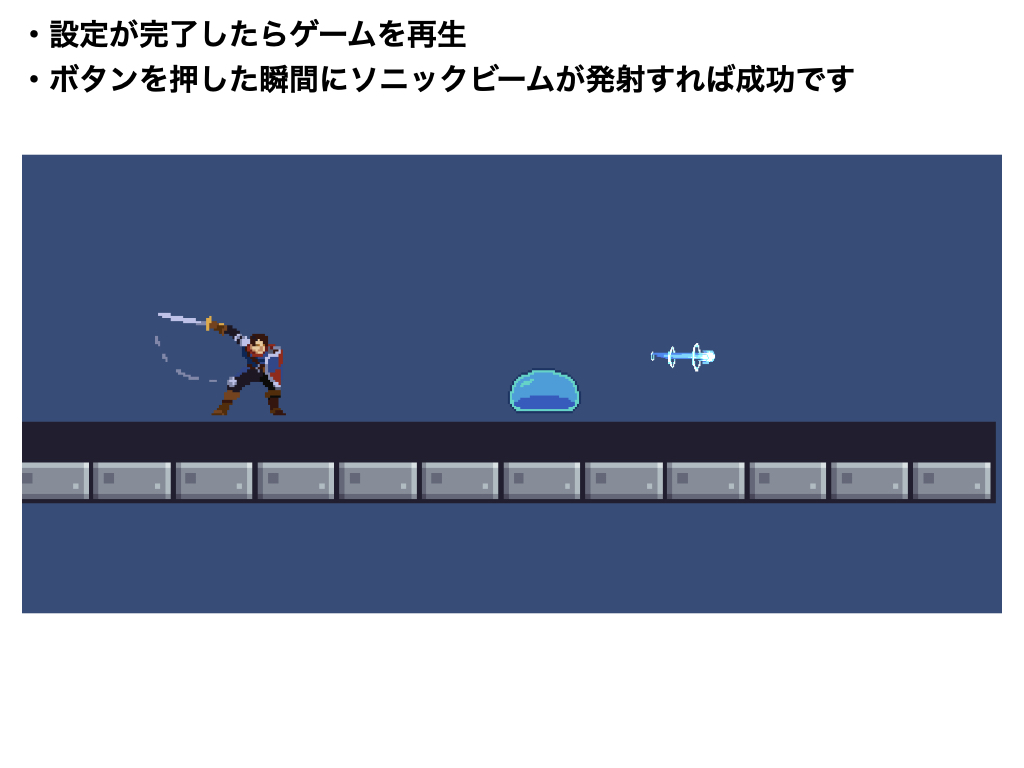
ソニックビームを発射する