FireBallを投げる敵の作成
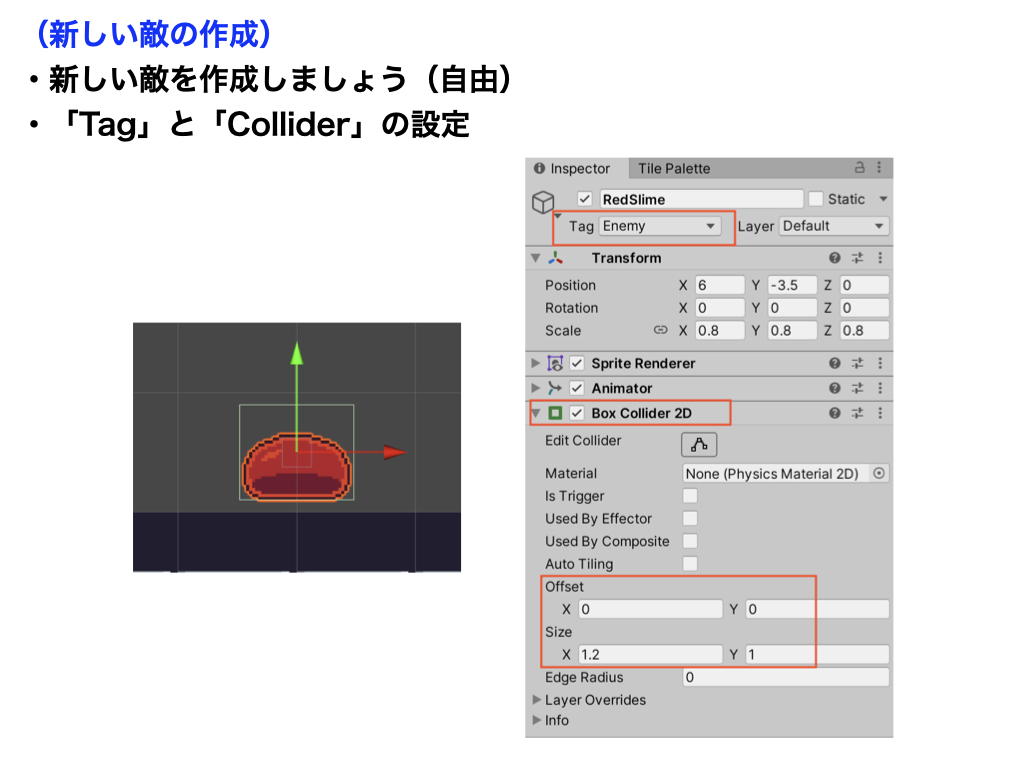
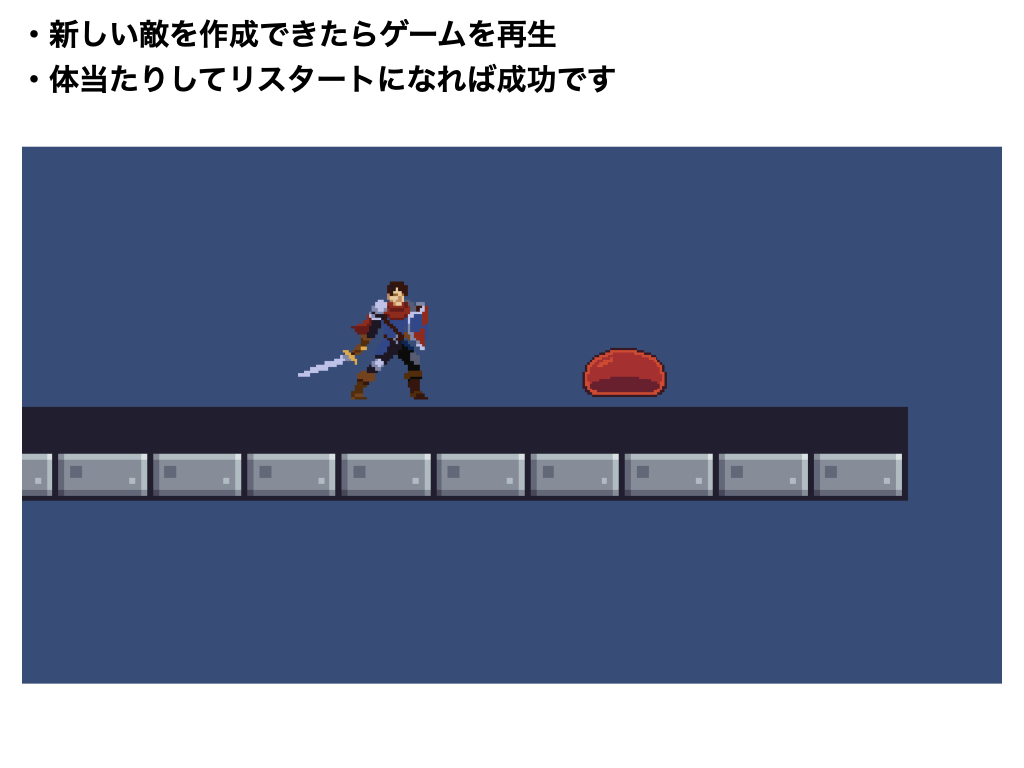
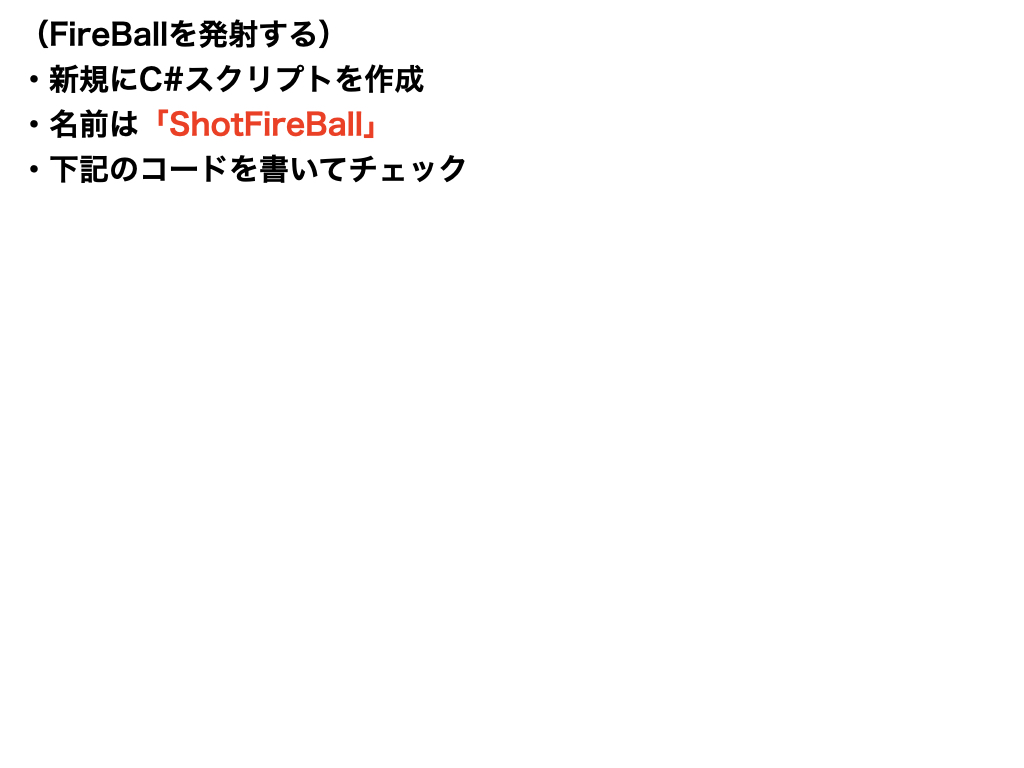
FireBallを発射する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShotFireBall : MonoBehaviour
{
public GameObject fireBallPrefab;
private GameObject target;
private Vector2 pos;
private Vector2 targetPos;
private void Start()
{
target = GameObject.Find("Player");
}
private void Update()
{
pos = transform.position;
targetPos = target.transform.position;
}
public void Shot()
{
GameObject fireBall = Instantiate(fireBallPrefab, transform.position, Quaternion.identity);
Rigidbody2D fireballRb2d = fireBall.GetComponent<Rigidbody2D>();
// Playerが自分よりも右の位置にいる場合には、右方向に発射する。
if(targetPos.x > pos.x)
{
fireballRb2d.AddForce(Vector2.right * 500);
}
// Playerが自分よりも左の位置にいる場合には、左方向に発射する。
else if(targetPos.x < pos.x)
{
fireballRb2d.AddForce(Vector2.left * 500);
}
}
}
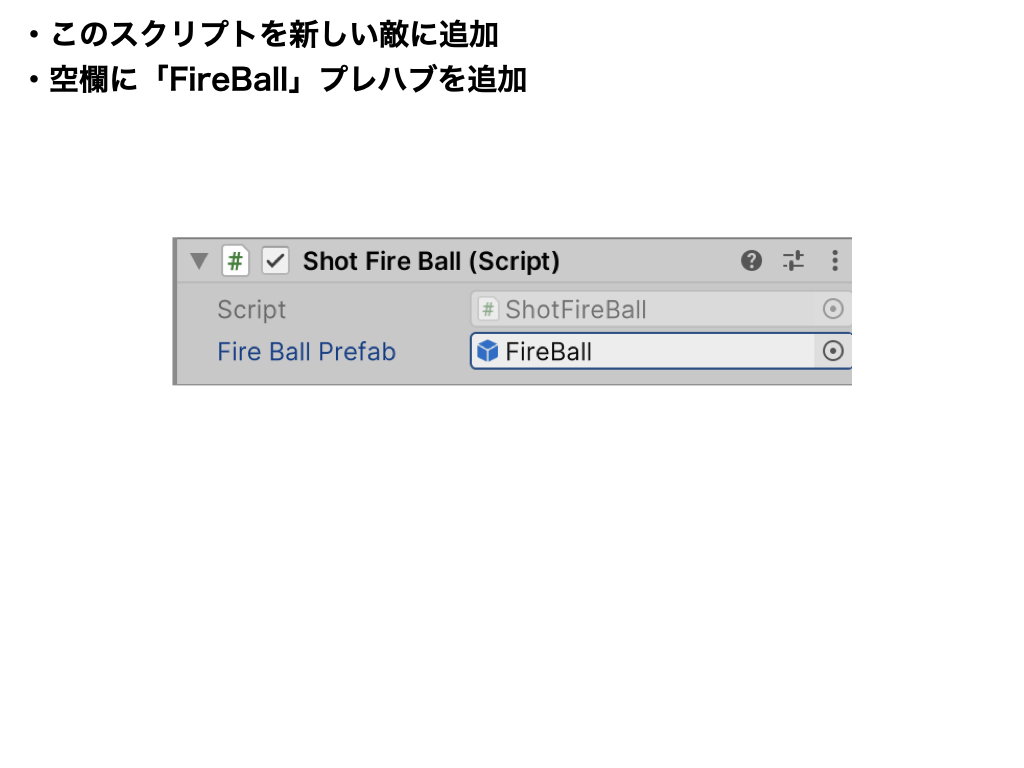
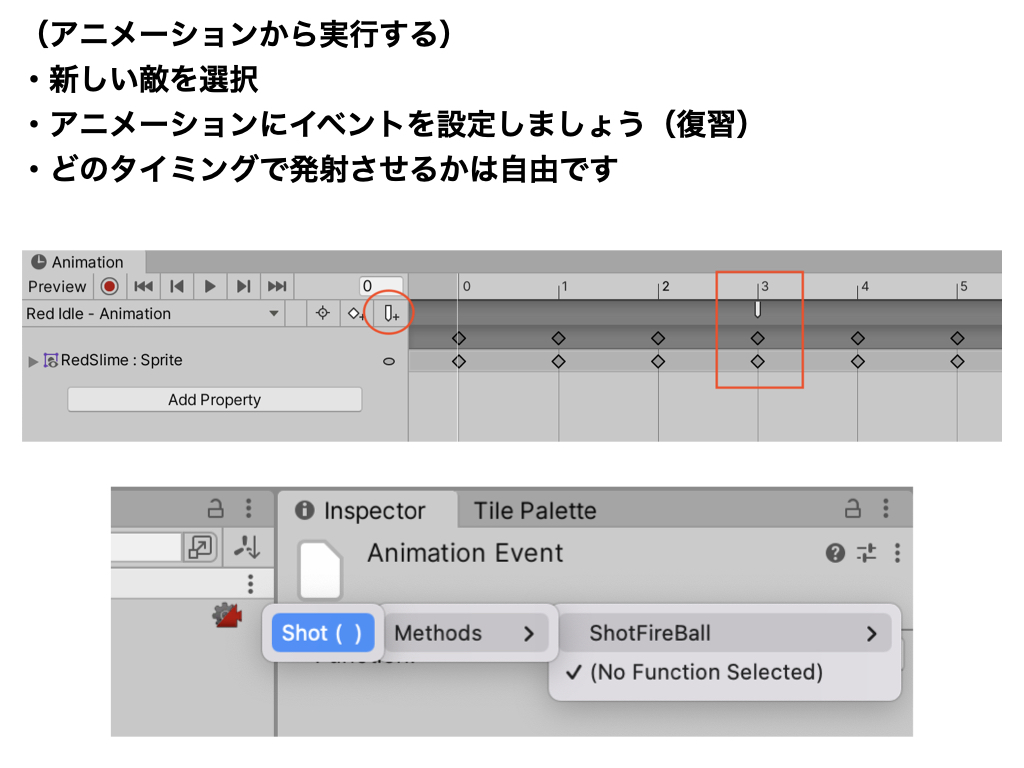
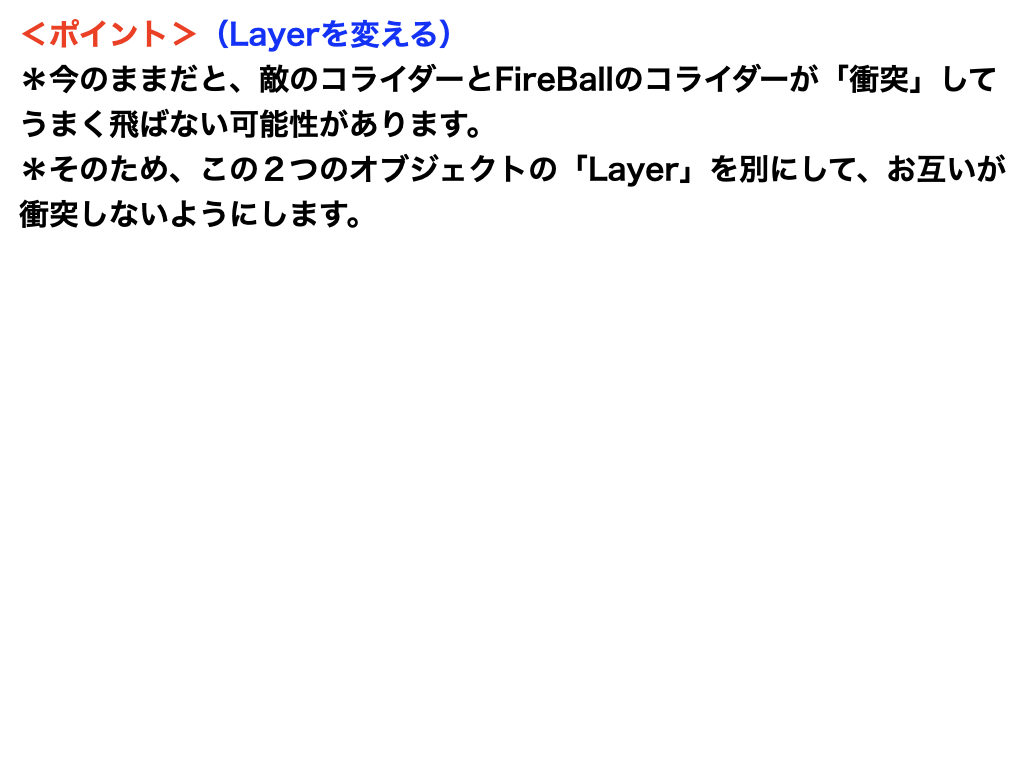
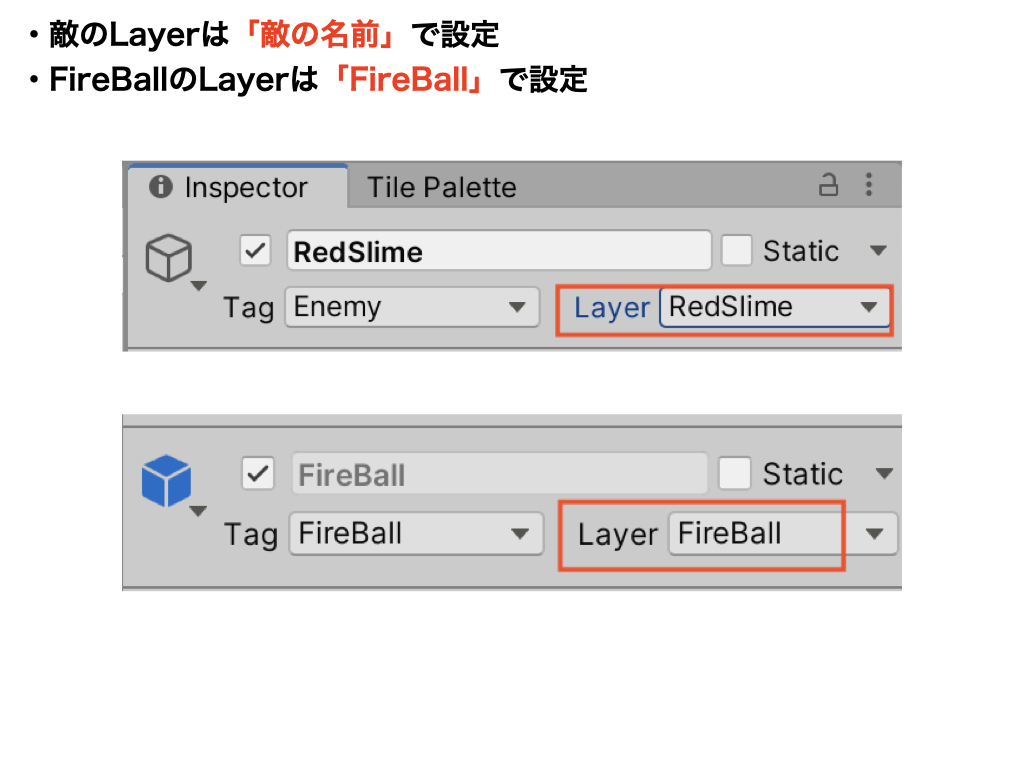
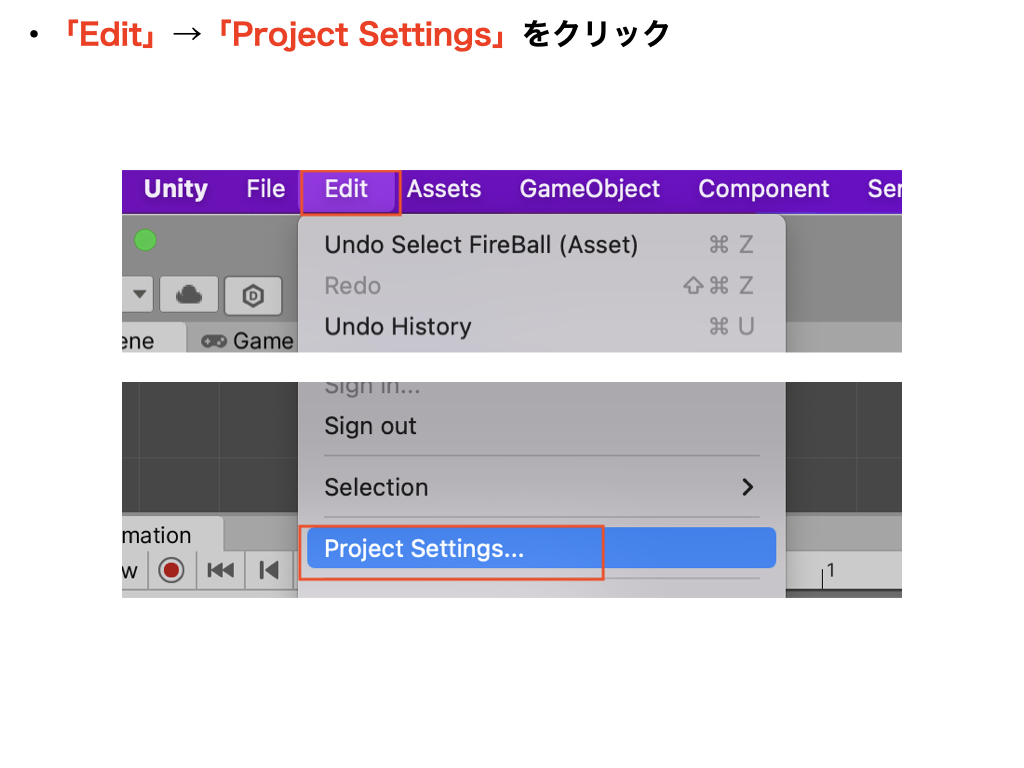
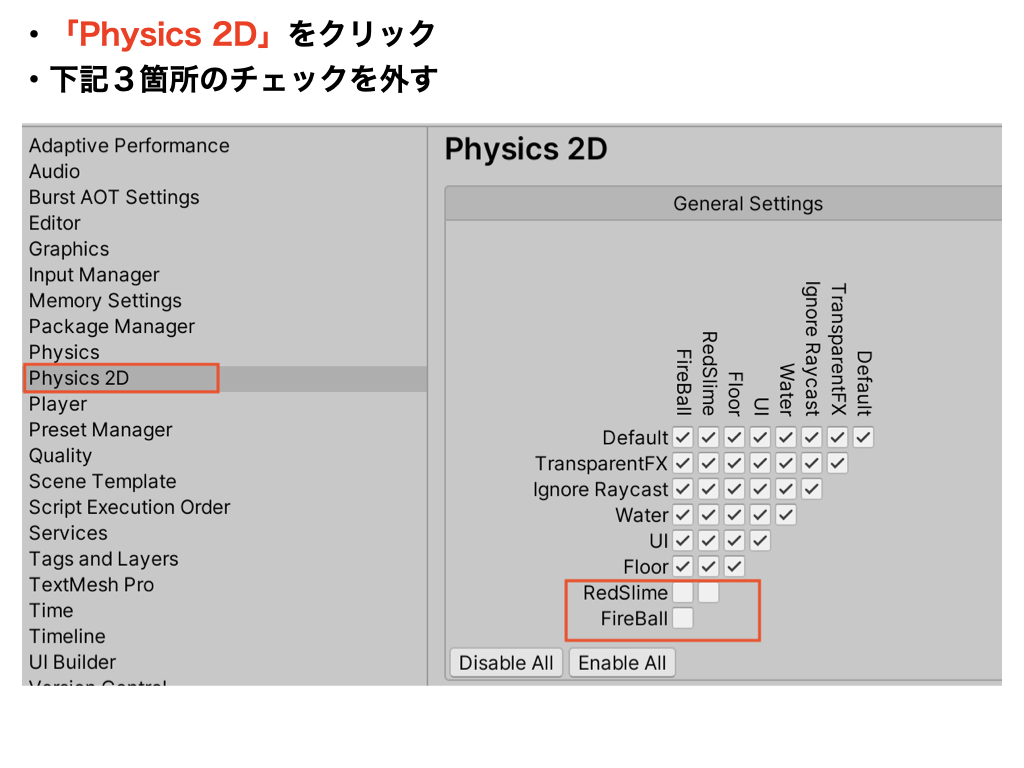
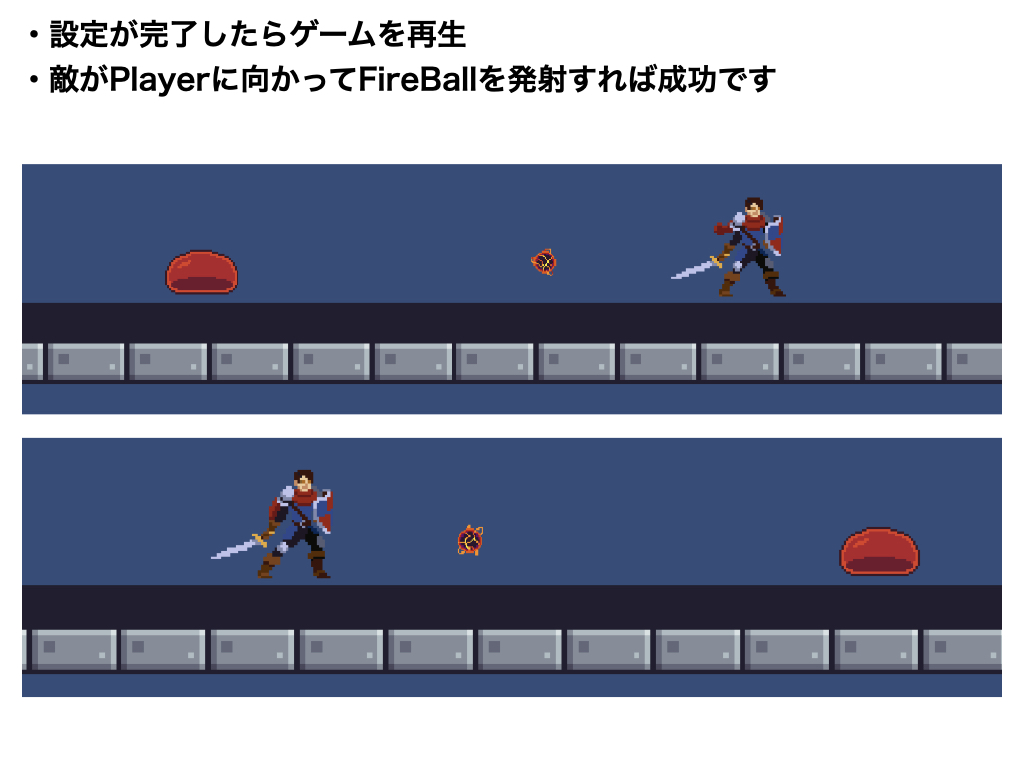
【2022版】DarkCastle(全39回)
他のコースを見る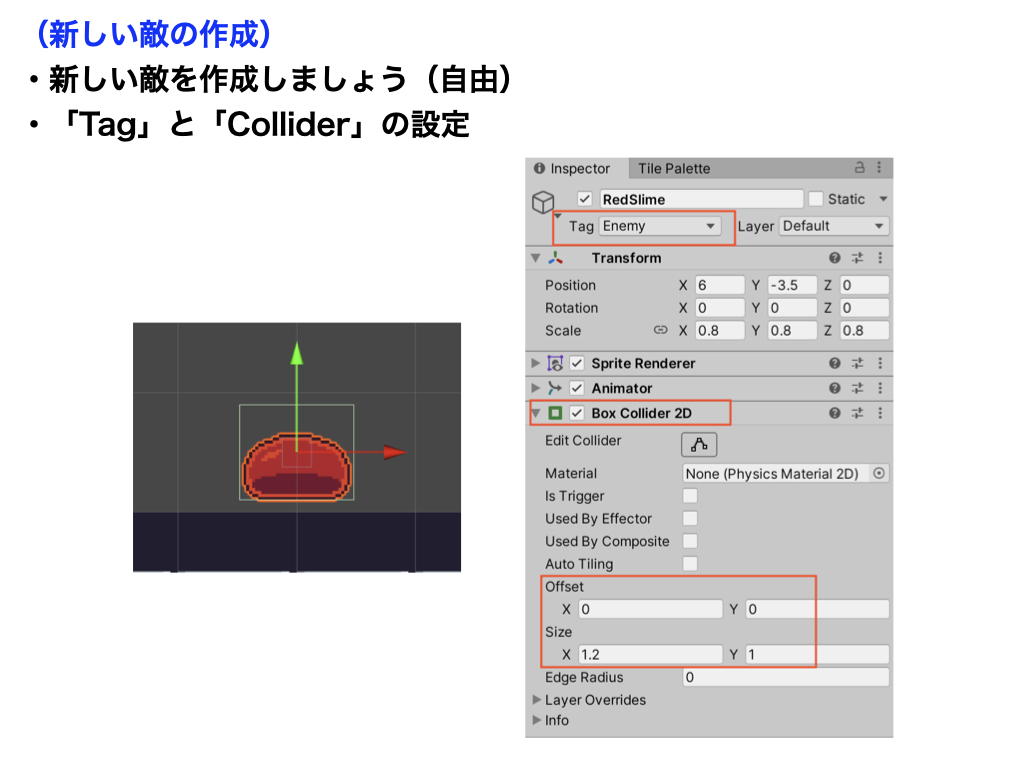
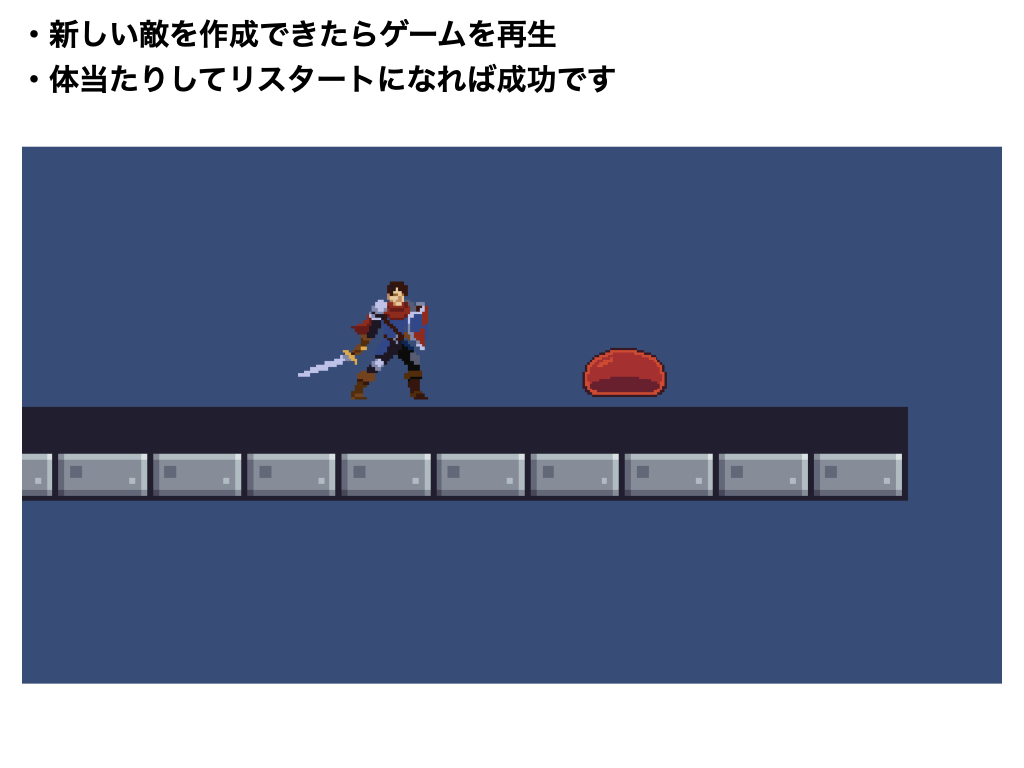
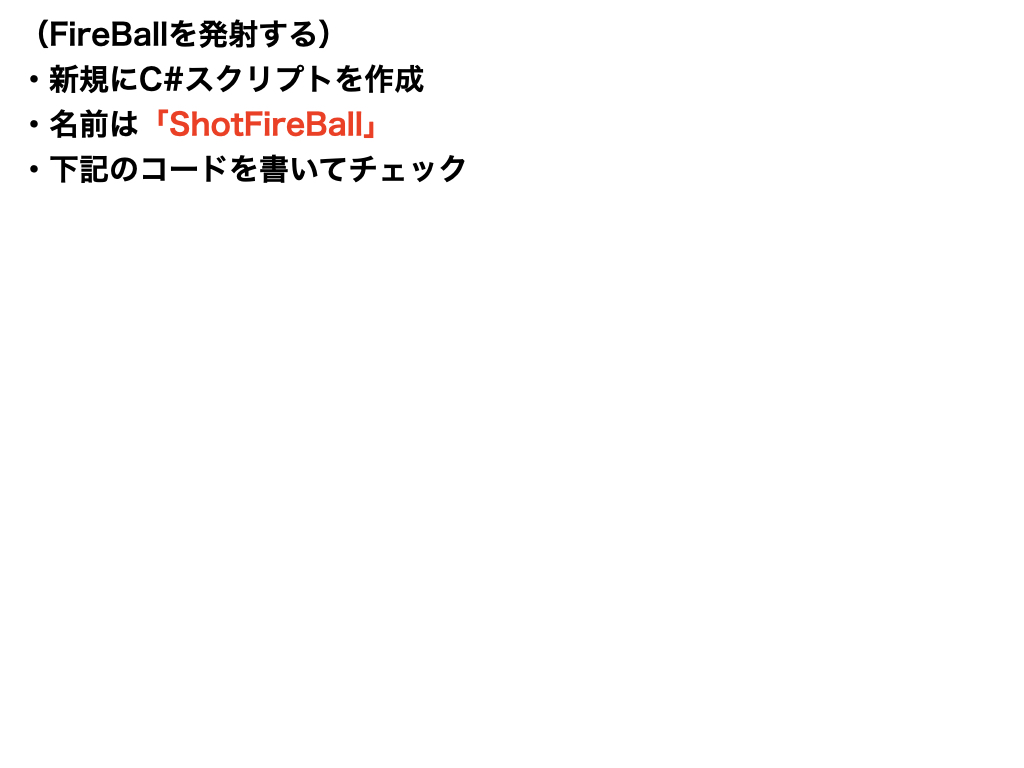
FireBallを発射する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShotFireBall : MonoBehaviour
{
public GameObject fireBallPrefab;
private GameObject target;
private Vector2 pos;
private Vector2 targetPos;
private void Start()
{
target = GameObject.Find("Player");
}
private void Update()
{
pos = transform.position;
targetPos = target.transform.position;
}
public void Shot()
{
GameObject fireBall = Instantiate(fireBallPrefab, transform.position, Quaternion.identity);
Rigidbody2D fireballRb2d = fireBall.GetComponent<Rigidbody2D>();
// Playerが自分よりも右の位置にいる場合には、右方向に発射する。
if(targetPos.x > pos.x)
{
fireballRb2d.AddForce(Vector2.right * 500);
}
// Playerが自分よりも左の位置にいる場合には、左方向に発射する。
else if(targetPos.x < pos.x)
{
fireballRb2d.AddForce(Vector2.left * 500);
}
}
}
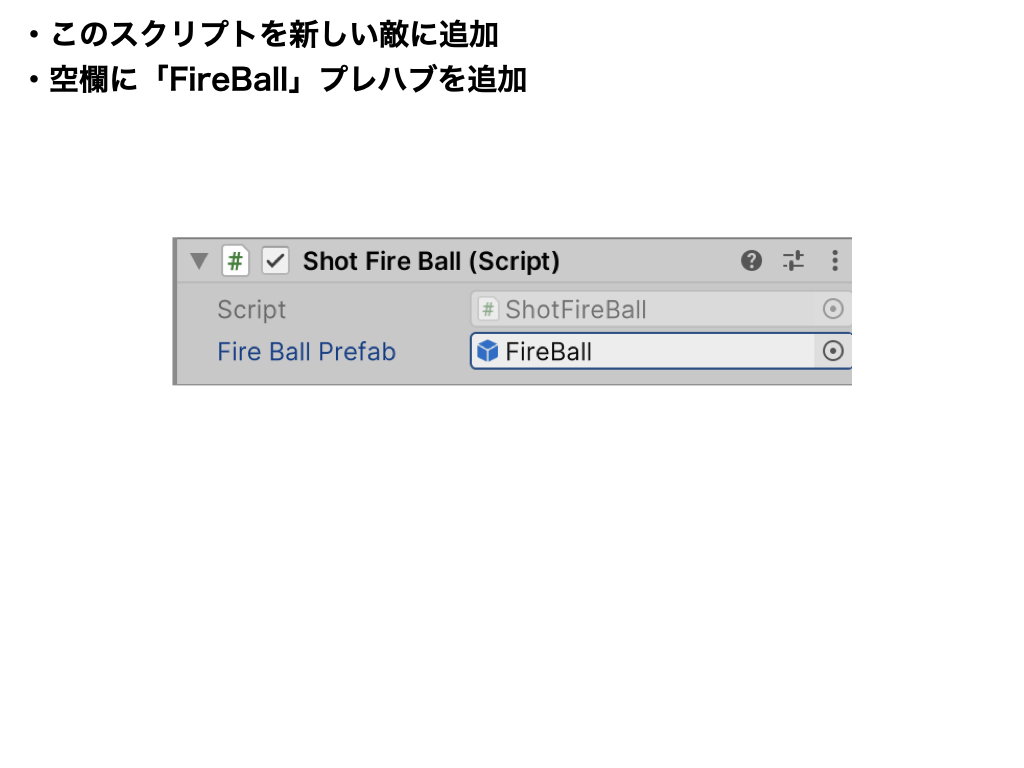
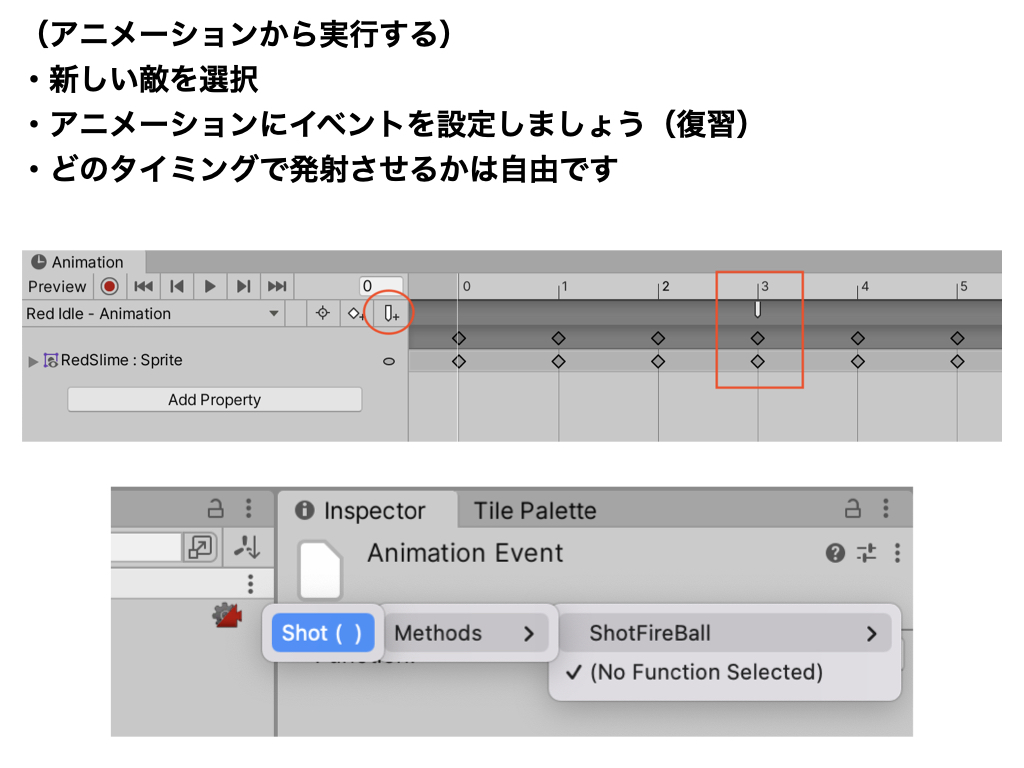
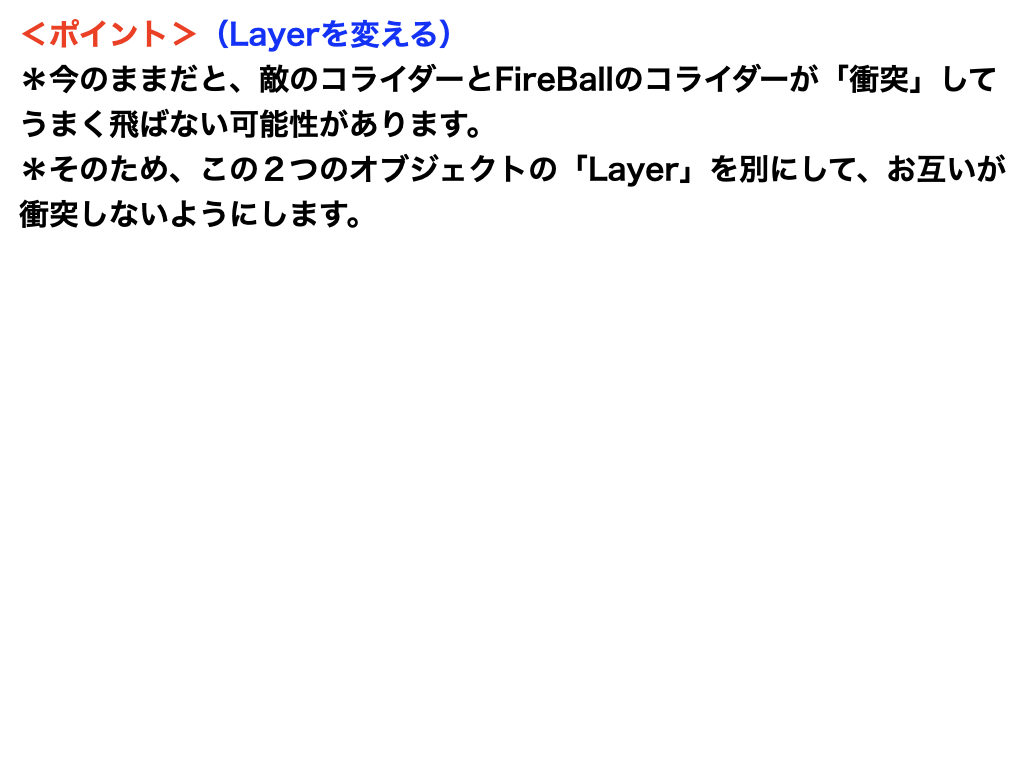
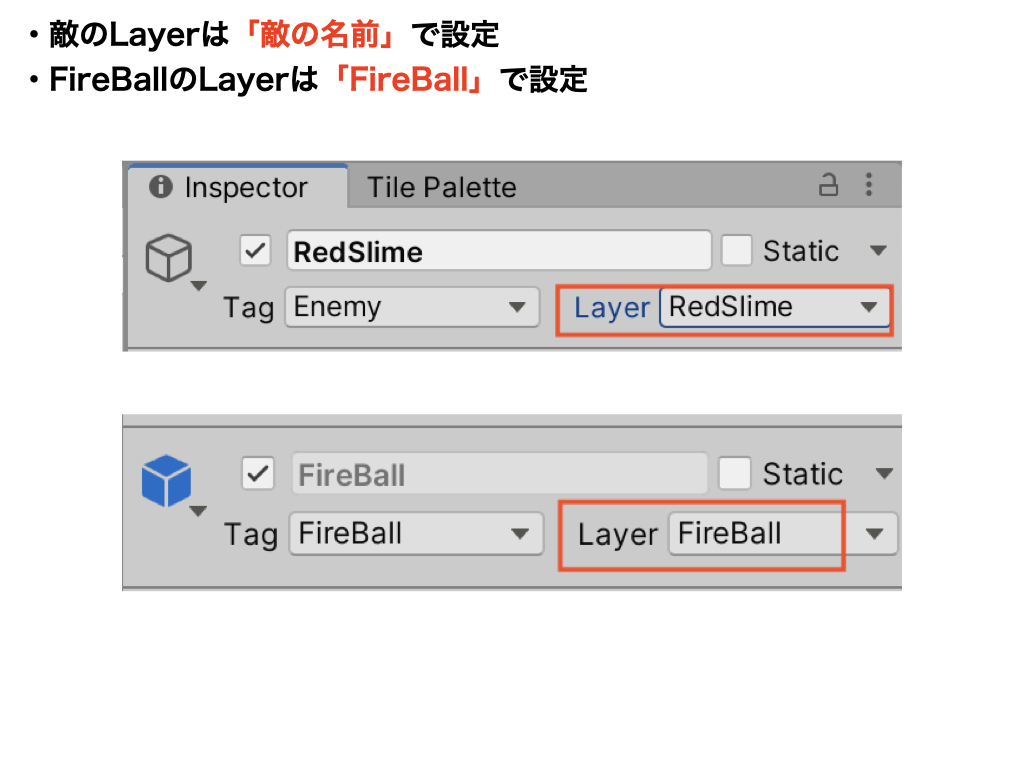
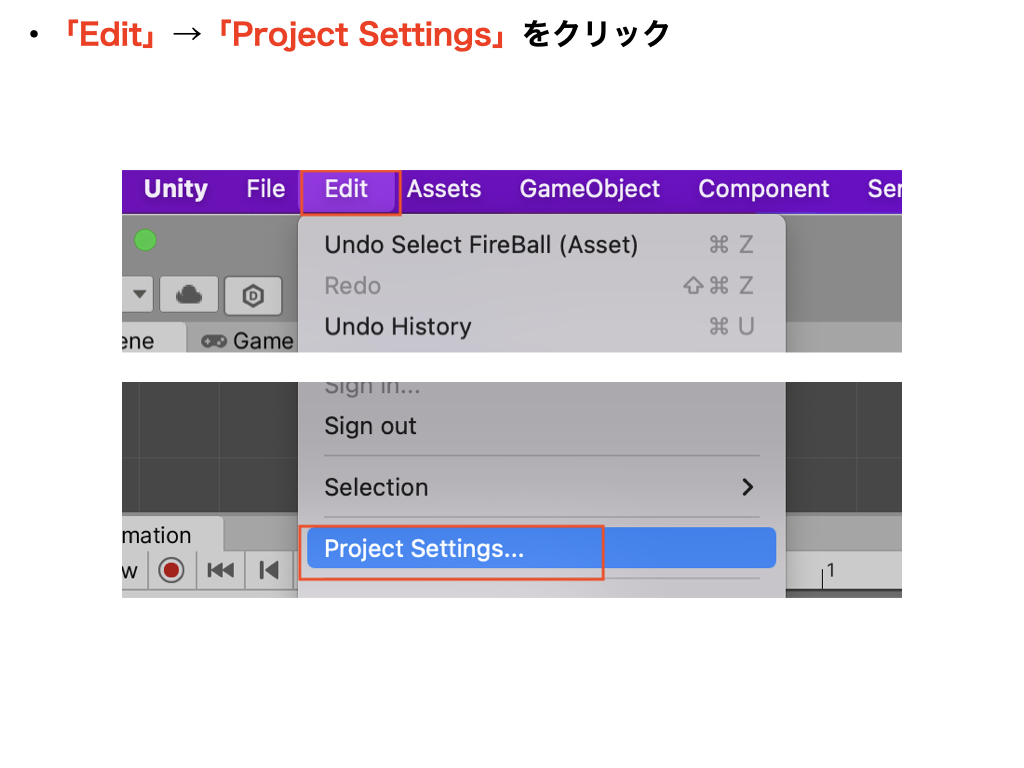
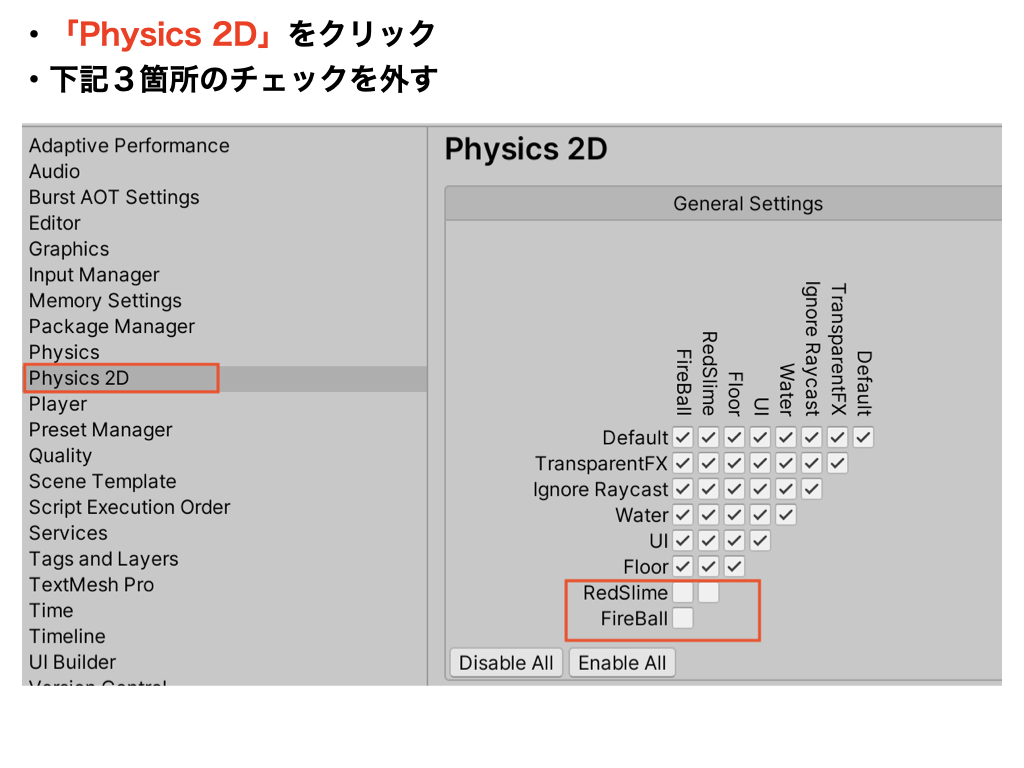
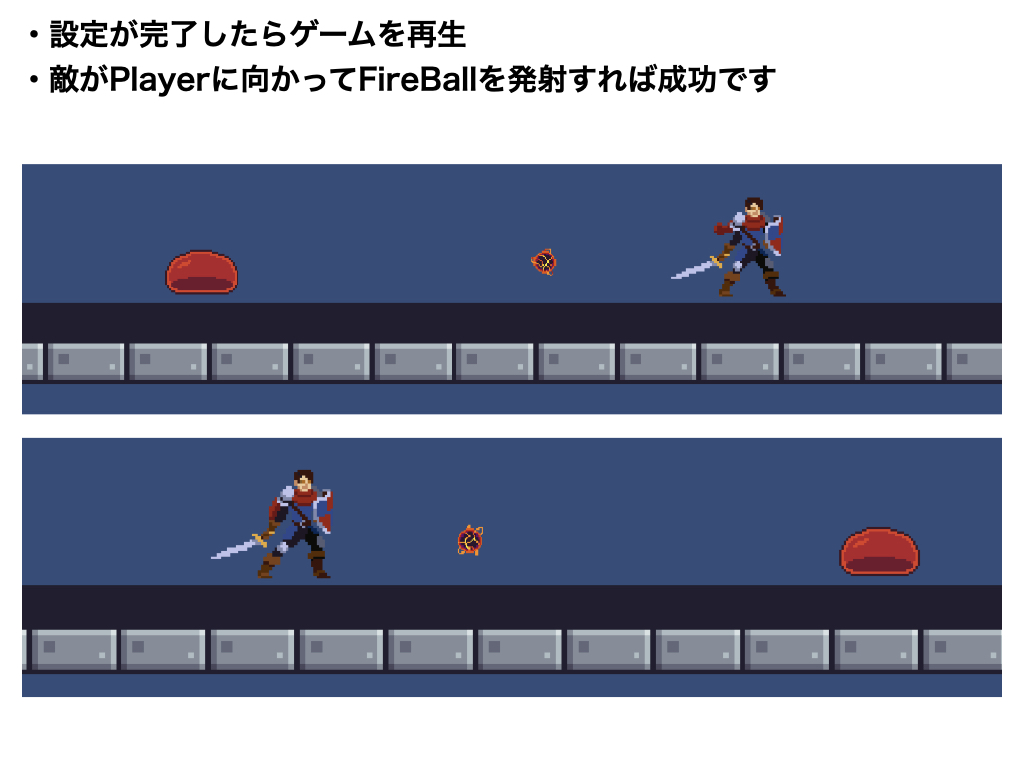
FireBallを投げる敵の作成