盾でFireBallをガードする
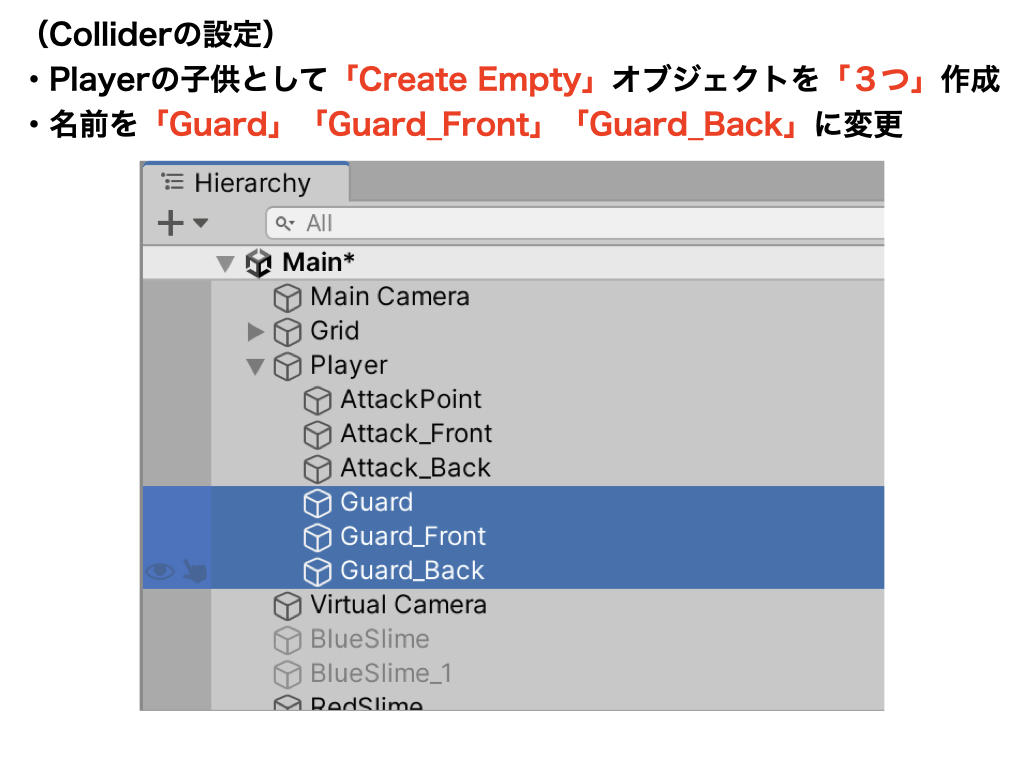
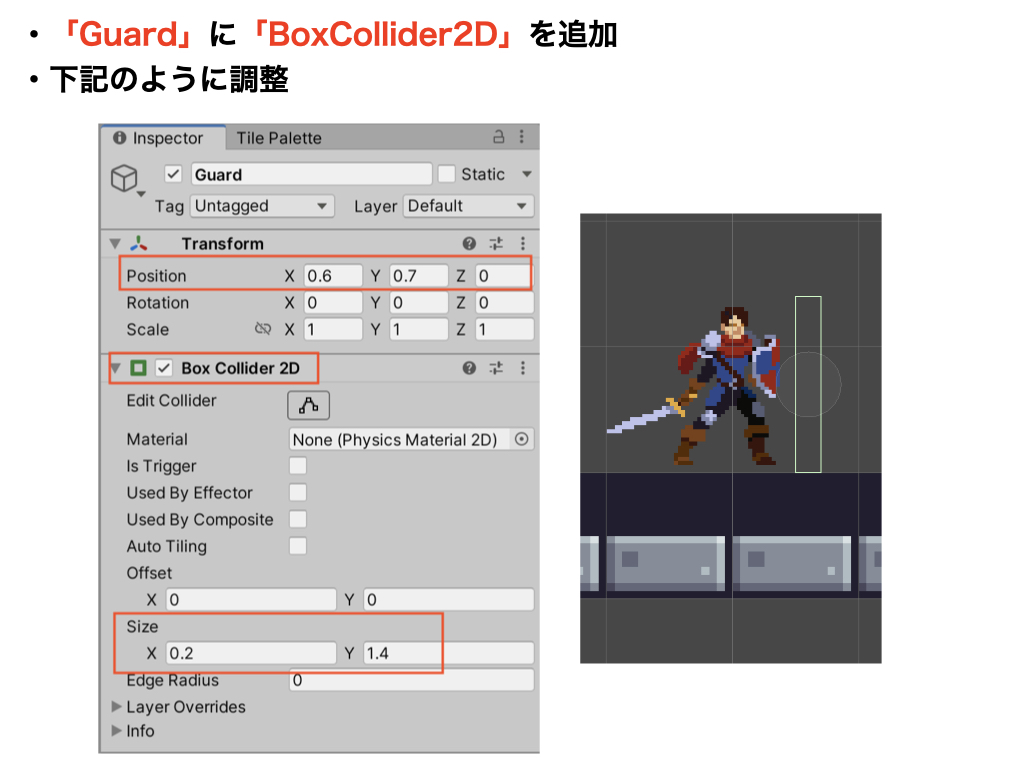
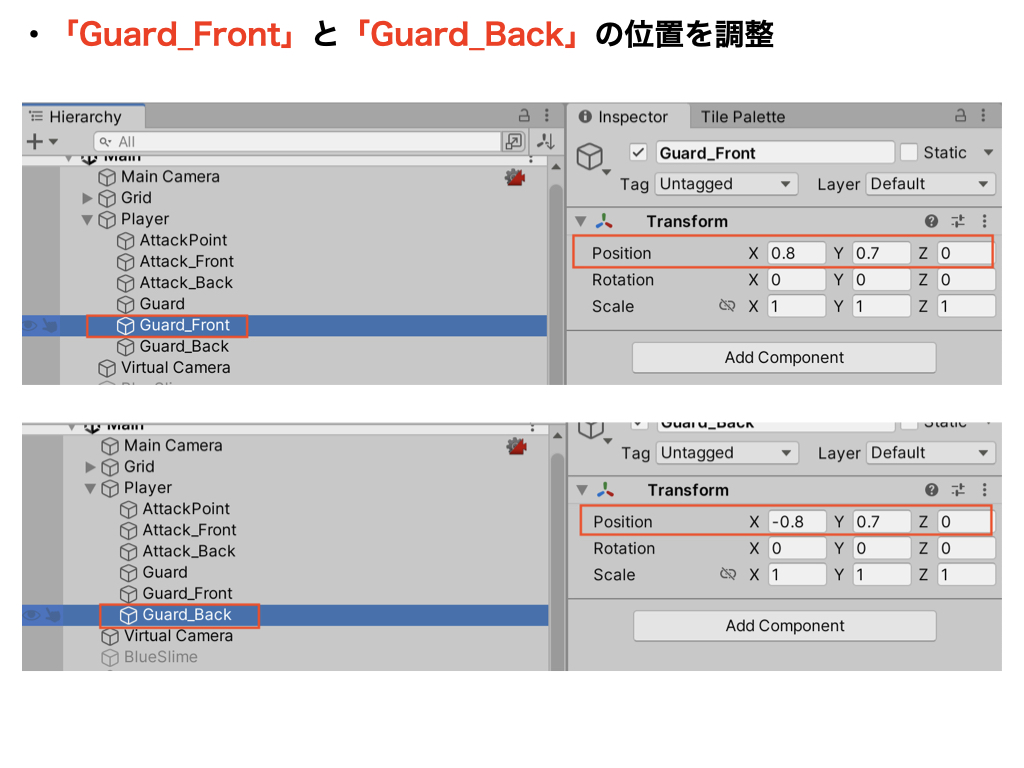
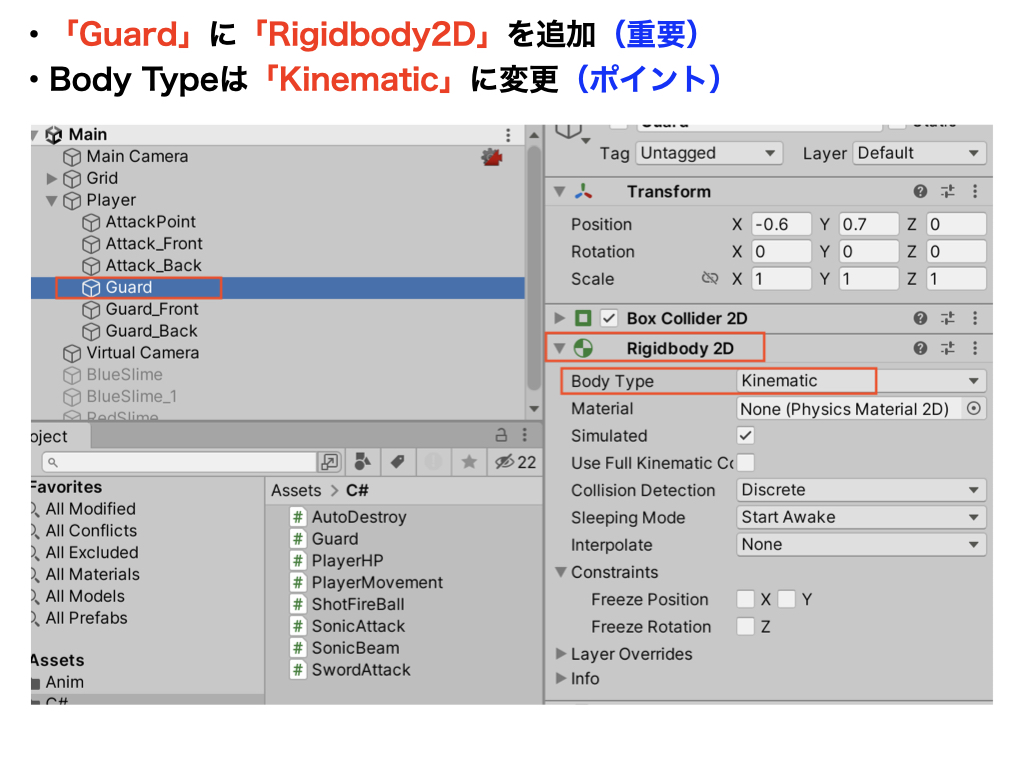
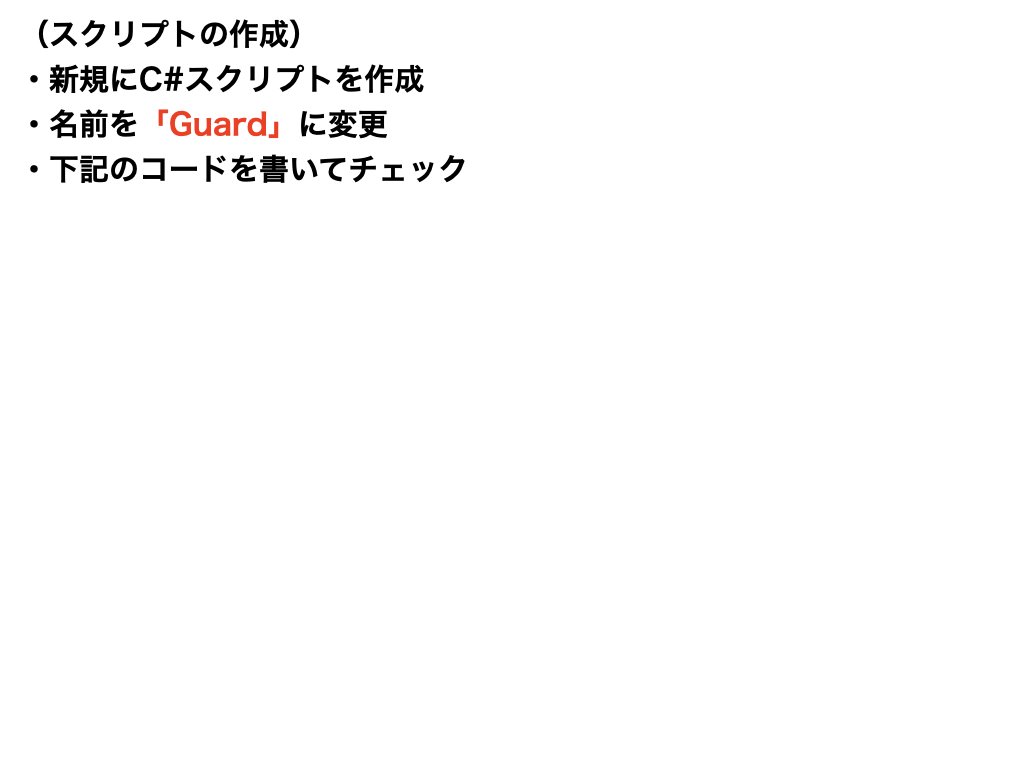
Guard機能の実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Guard : MonoBehaviour
{
public GameObject player;
public GameObject guard_front;
public GameObject guard_back;
public AudioClip sound;
private PlayerMovement pm;
void Start()
{
// ガードの初期位置
transform.position = guard_front.transform.position;
pm = player.GetComponent<PlayerMovement>();
}
private void Update()
{
// 「ガードの位置」を「Playerの向き」に合わせる。
if(pm.moveH == 1) // Playerが右を向いている場合
{
transform.position = guard_front.transform.position;
}
else if(pm.moveH == -1) // Playerが左を向いている場合
{
transform.position = guard_back.transform.position;
}
}
// 盾としての機能(FireBallを破壊する)
private void OnCollisionEnter2D(Collision2D collision)
{
if(collision.gameObject.CompareTag("FireBall"))
{
Destroy(collision.gameObject);
AudioSource.PlayClipAtPoint(sound, transform.position);
}
}
}
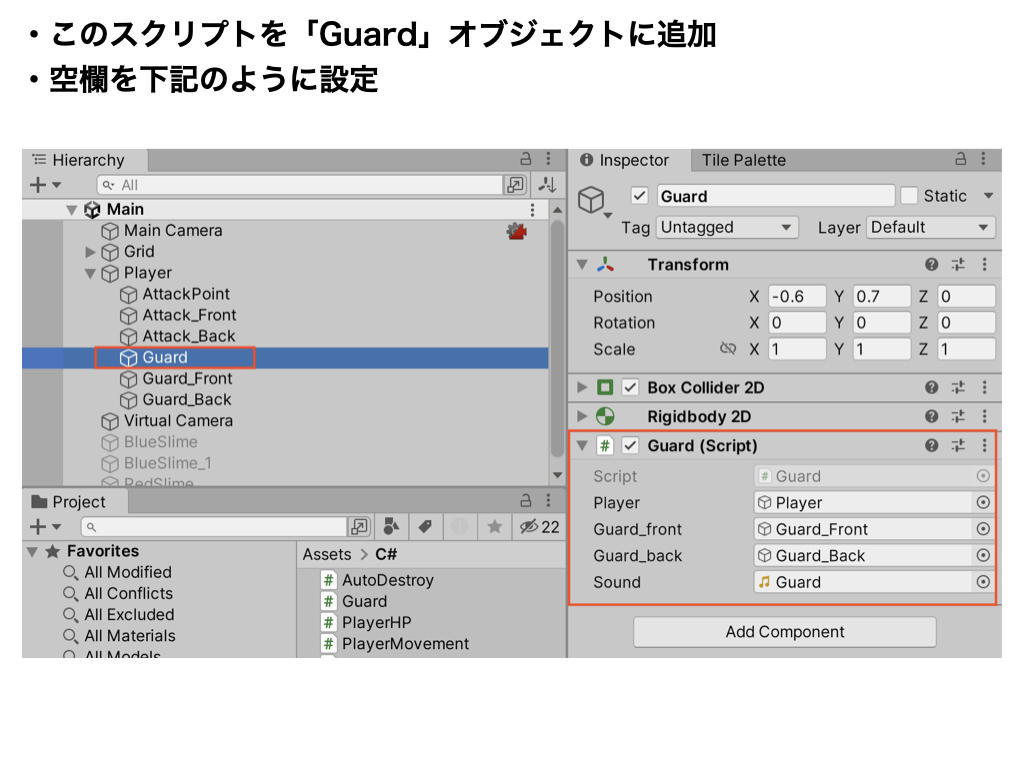
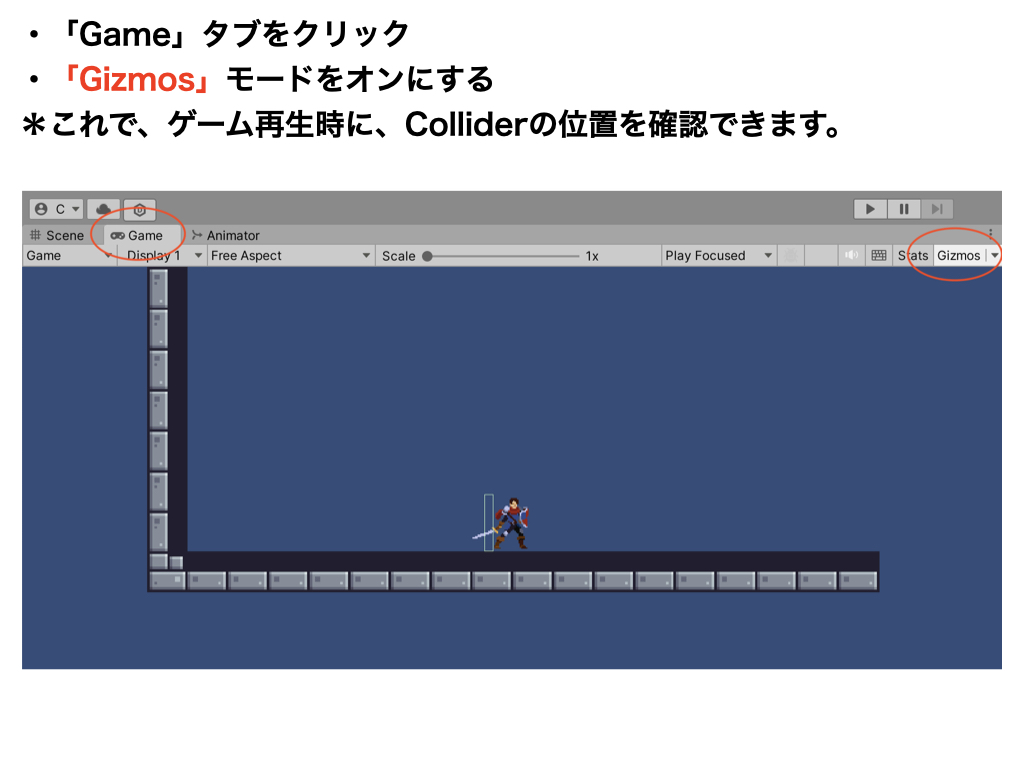
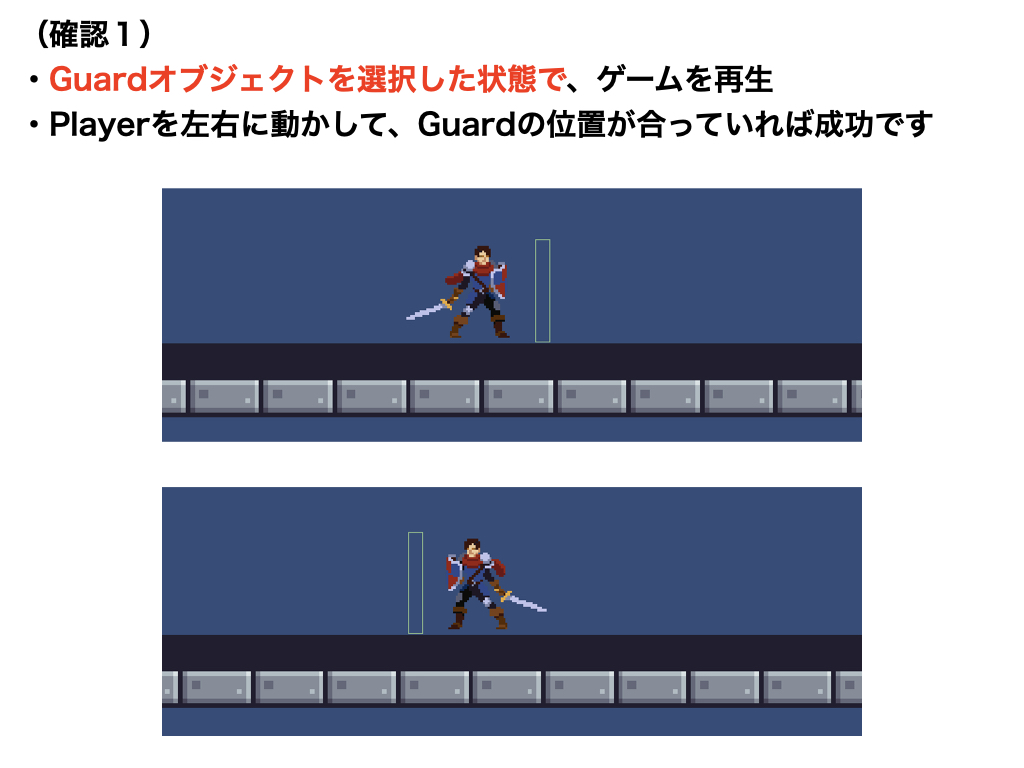
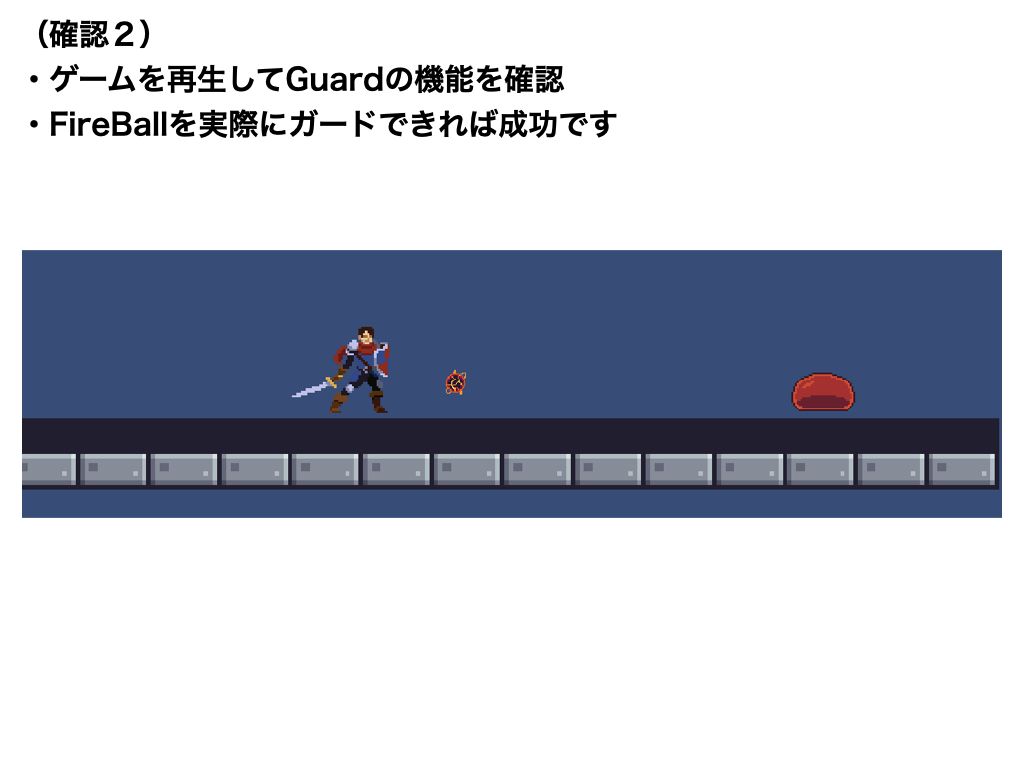
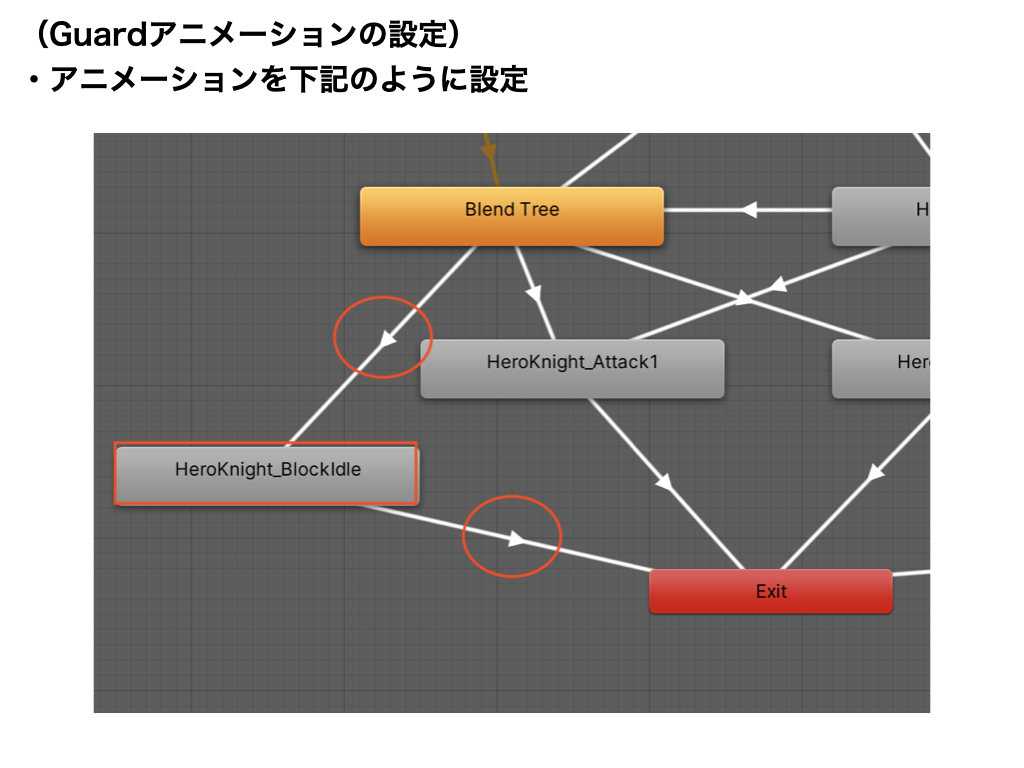
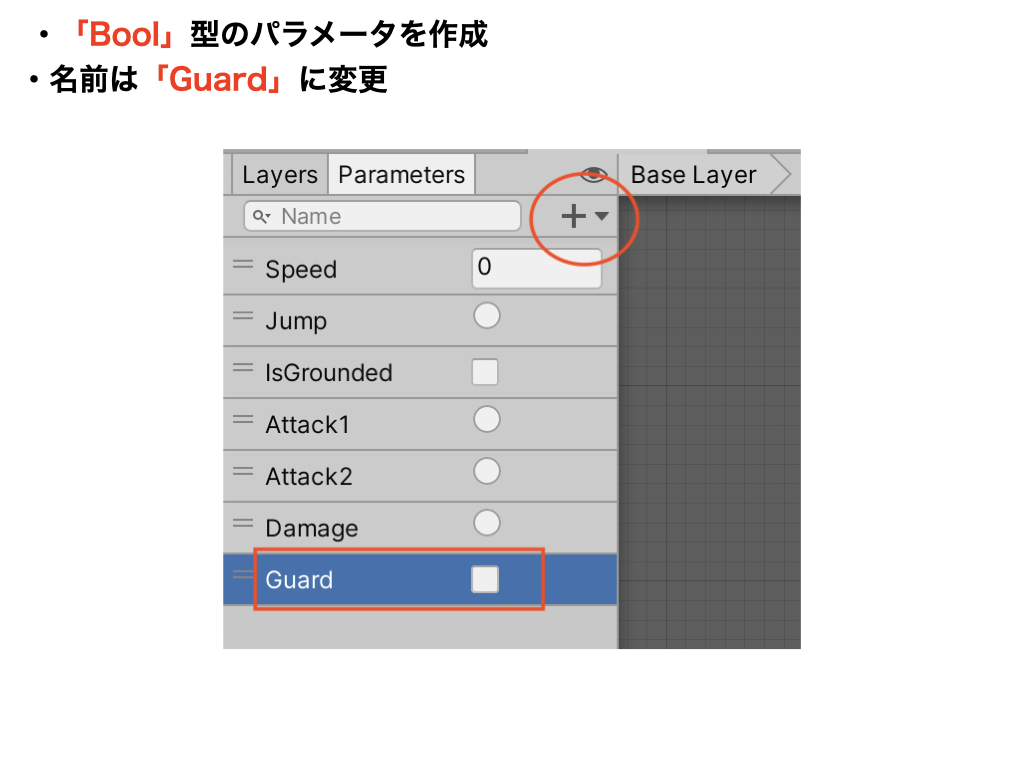
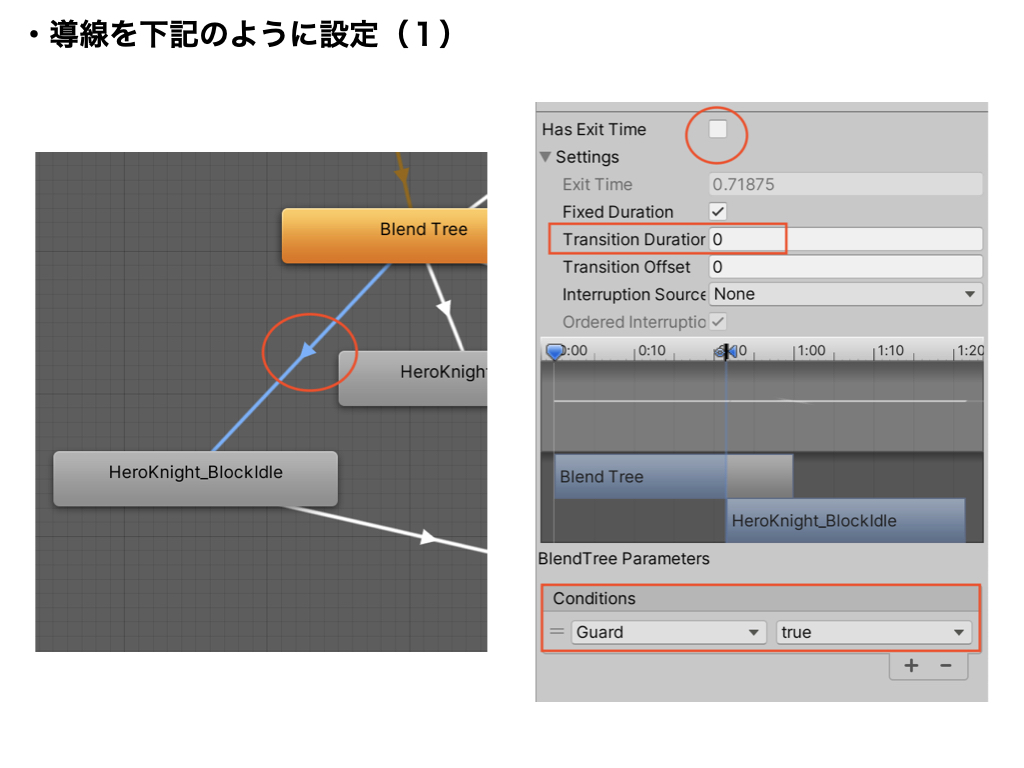
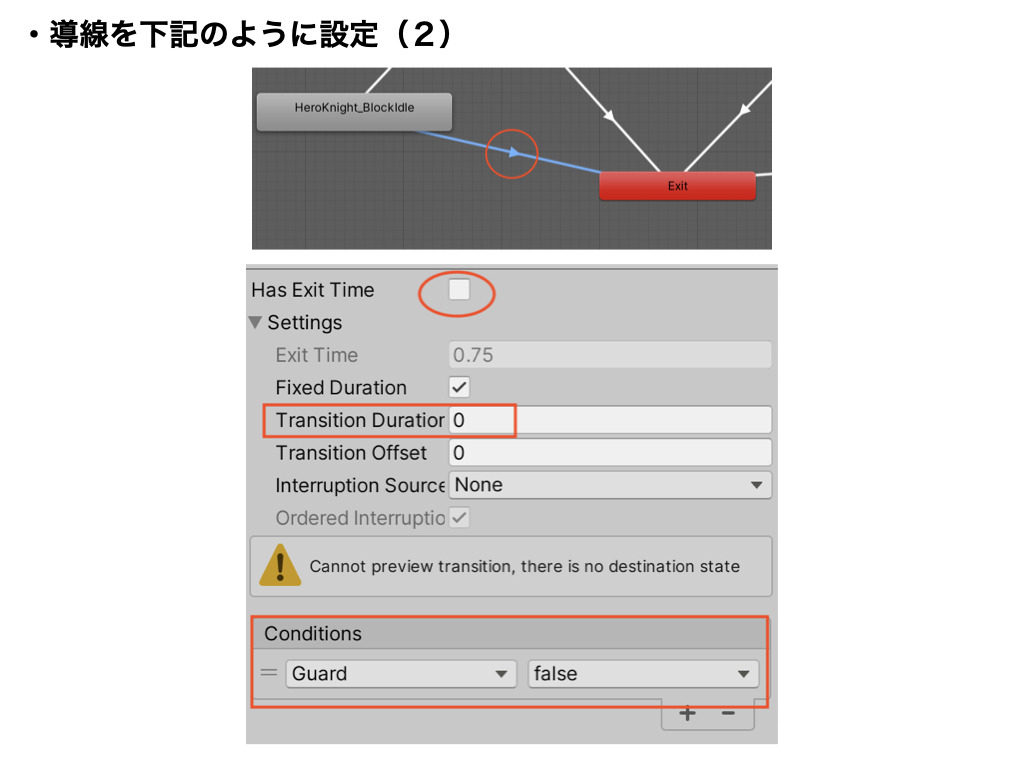
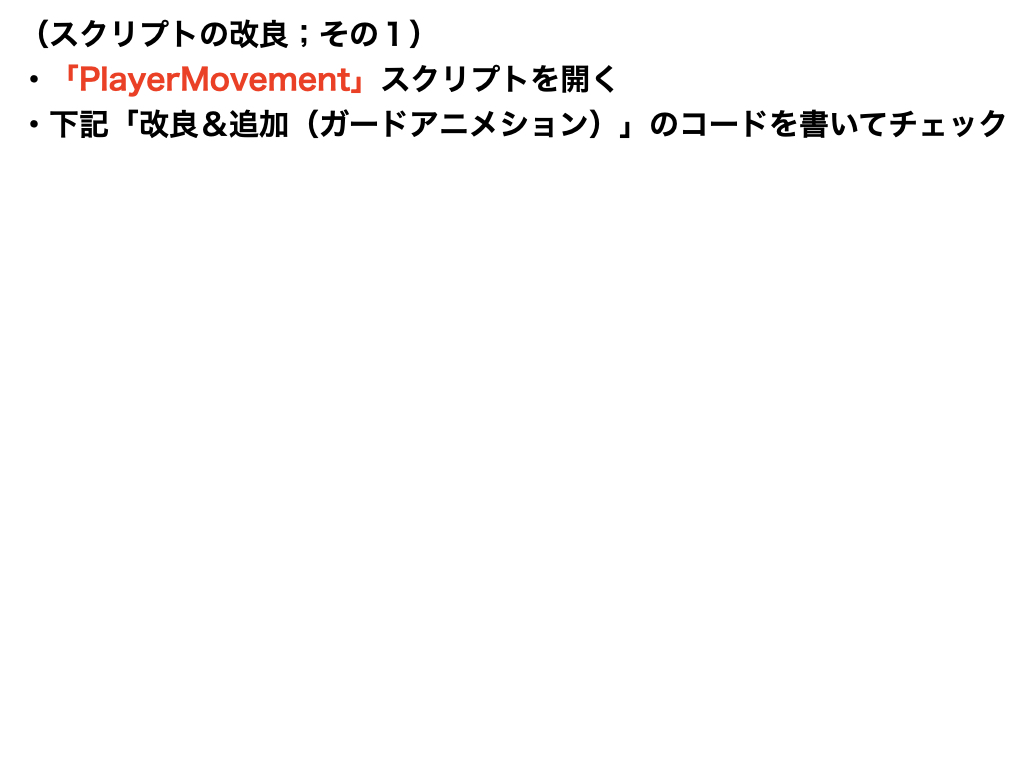
ガードアニメーションの実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed;
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
// ★改良(ガードアニメーション)
// ガード中はソニックの発射不可
// 下記のコードをコメントアウトする(もしくは削除)
//public AudioClip sonicSound;
// ★追加(ガードアニメーション)
private bool isGard = false;
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
}
void Update()
{
// ★追加(ガードアニメーション)
// ガード中は移動不可
if(isGard == false)
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
}
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
else if (Input.GetKeyDown(KeyCode.X))
{
if (moveH != 0)
{
return;
}
// ★改良(ガードアニメーション)
// ガード中はソニックの発射不可
// 下記のコードをコメントアウトする(もしくは削除)
//AudioSource.PlayClipAtPoint(sonicSound, transform.position);
animator.SetTrigger("Attack2");
}
// ★追加(ガードアニメーション)
if (Input.GetKeyDown(KeyCode.C)) // ガード発動のキーは自由に変更可能
{
// 移動中はガード不可
if (moveH != 0)
{
return;
}
animator.SetBool("Guard", true);
isGard = true;
}
else if (Input.GetKeyUp(KeyCode.C))
{
animator.SetBool("Guard", false);
isGard = false;
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
}
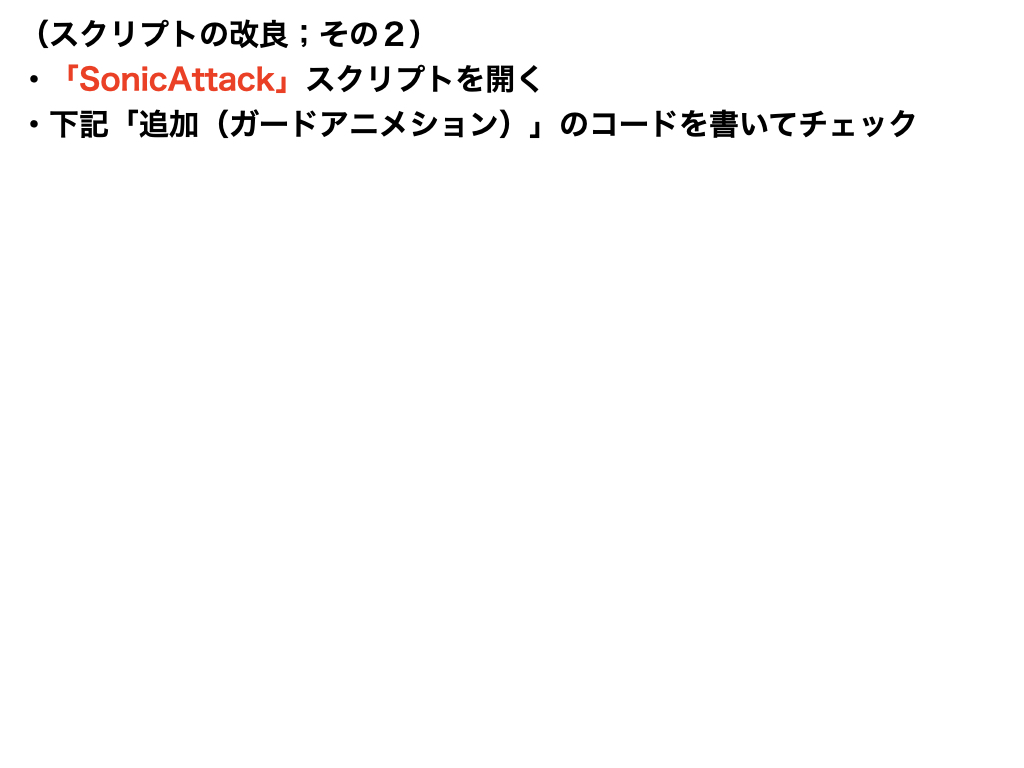
ソニックの発射音
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SonicAttack : MonoBehaviour
{
public GameObject sonicPrefab;
public GameObject attackPoint;
public GameObject attack_front;
public GameObject attack_back;
private PlayerMovement pm;
private float num;
private int sonicRotation;
private int sonicNum = 1;
// ★追加(ガードアニメーション)
public AudioClip sound;
void Start()
{
pm = GetComponent<PlayerMovement>();
attackPoint.transform.position = attack_front.transform.position;
}
void Update()
{
num = pm.moveH;
if (num == 1)
{
attackPoint.transform.position = attack_front.transform.position;
sonicRotation = 0;
sonicNum = 1;
}
else if (num == -1)
{
attackPoint.transform.position = attack_back.transform.position;
sonicRotation = 180;
sonicNum = -1;
}
}
public void S_Attack()
{
GameObject sonic = Instantiate(sonicPrefab, attackPoint.transform.position, Quaternion.Euler(0, sonicRotation, 0));
Rigidbody2D sonicRb2d = sonic.GetComponent<Rigidbody2D>();
sonicRb2d.AddForce(transform.right * 1000 * sonicNum);
// ★追加(ガードアニメーション)
// ソニックの発射音もアニメーションから実行する
AudioSource.PlayClipAtPoint(sound, Camera.main.transform.position);
Destroy(sonic, 5.0f);
}
}
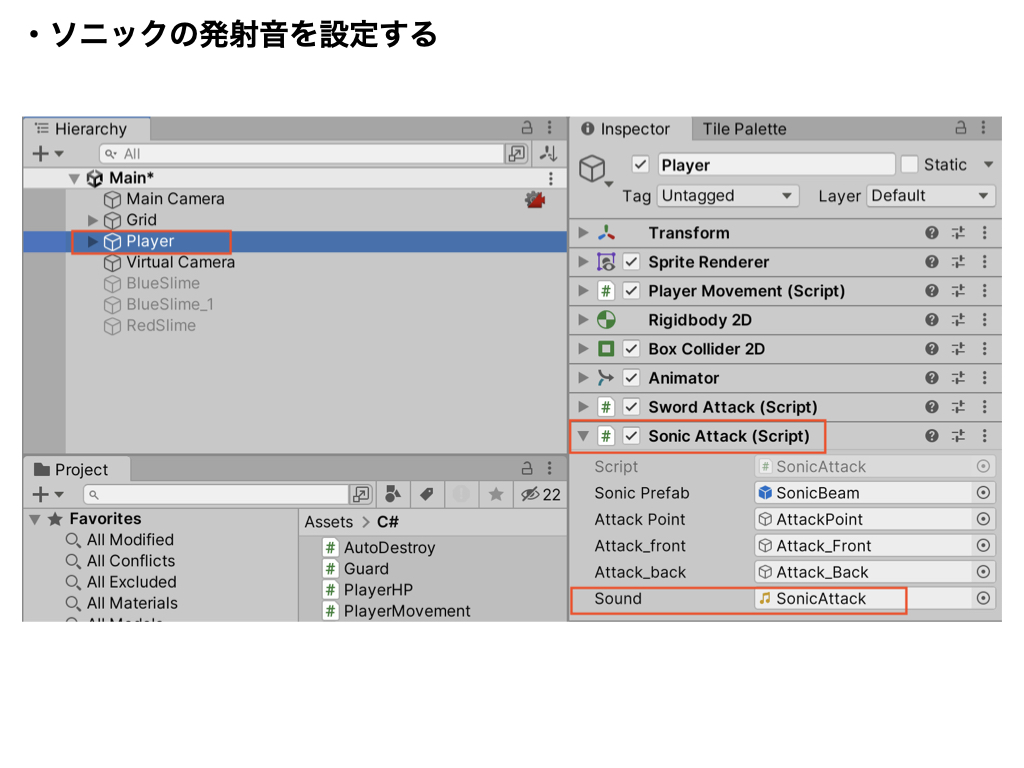
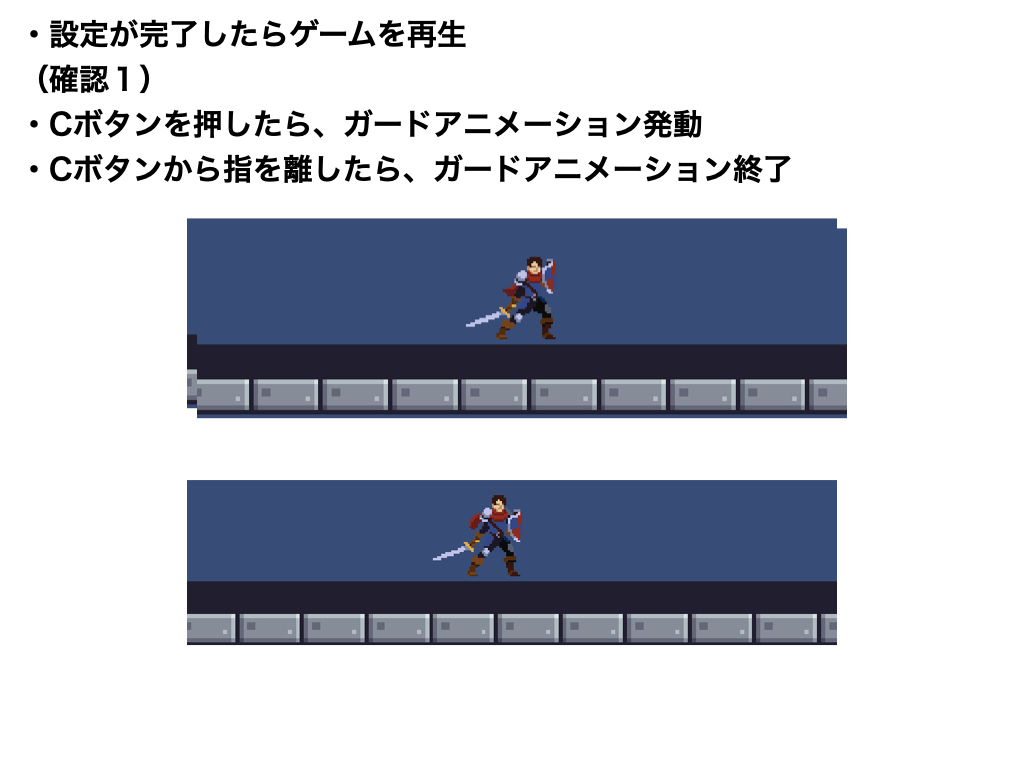
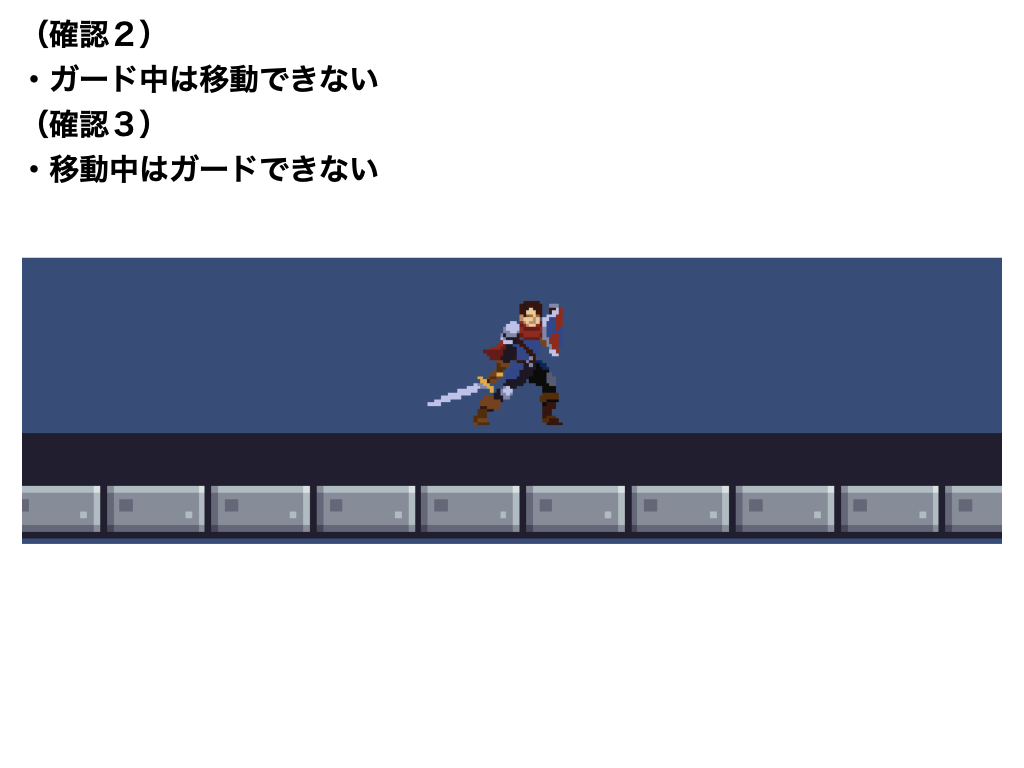
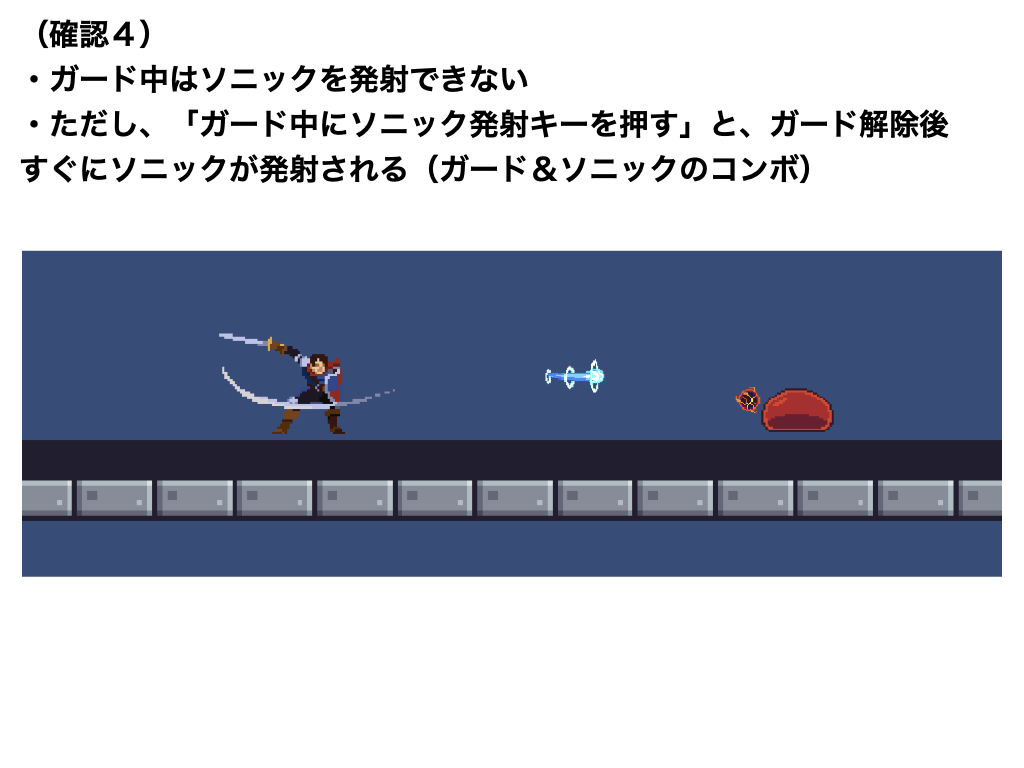
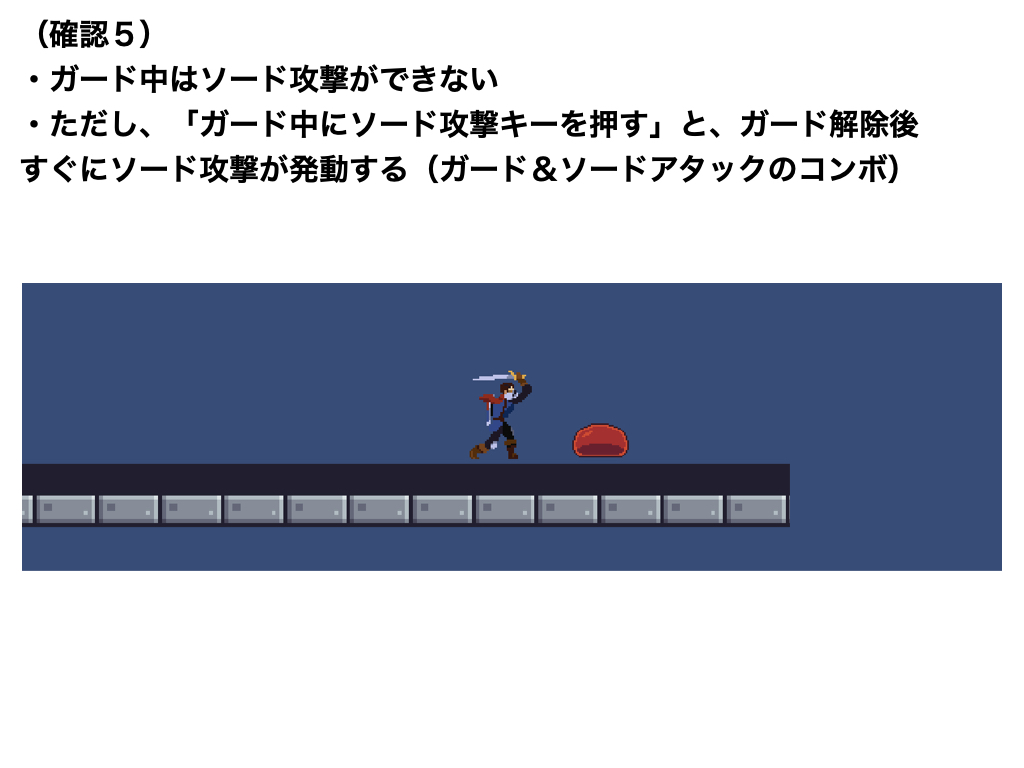
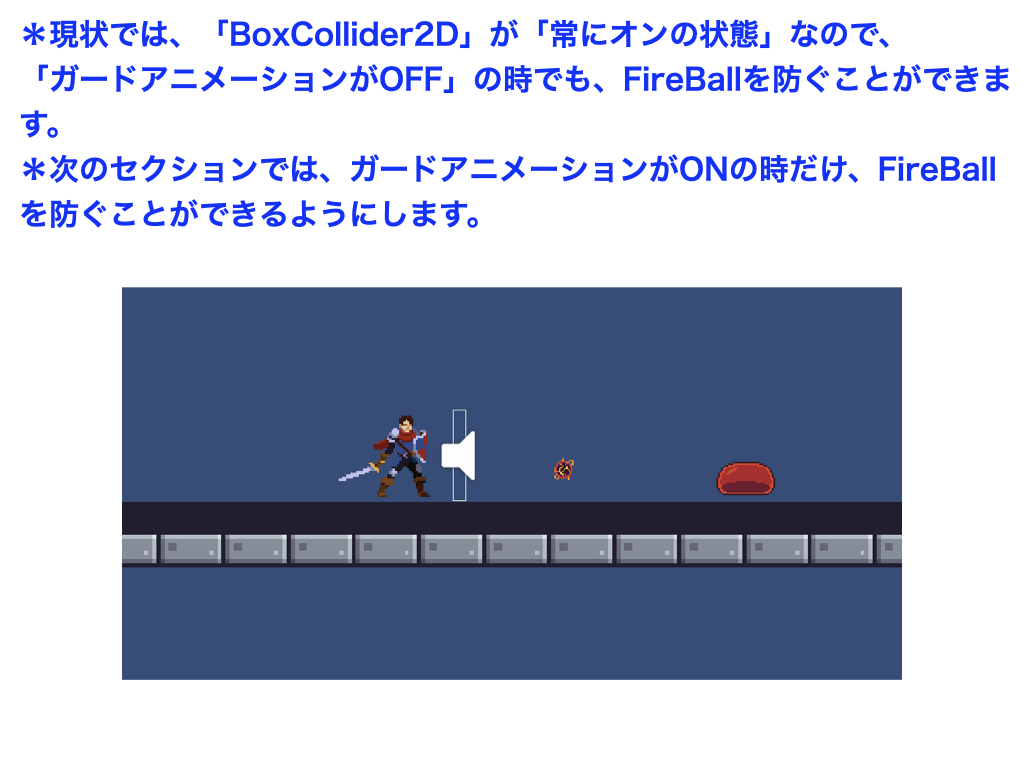
【2022版】DarkCastle(全39回)
他のコースを見る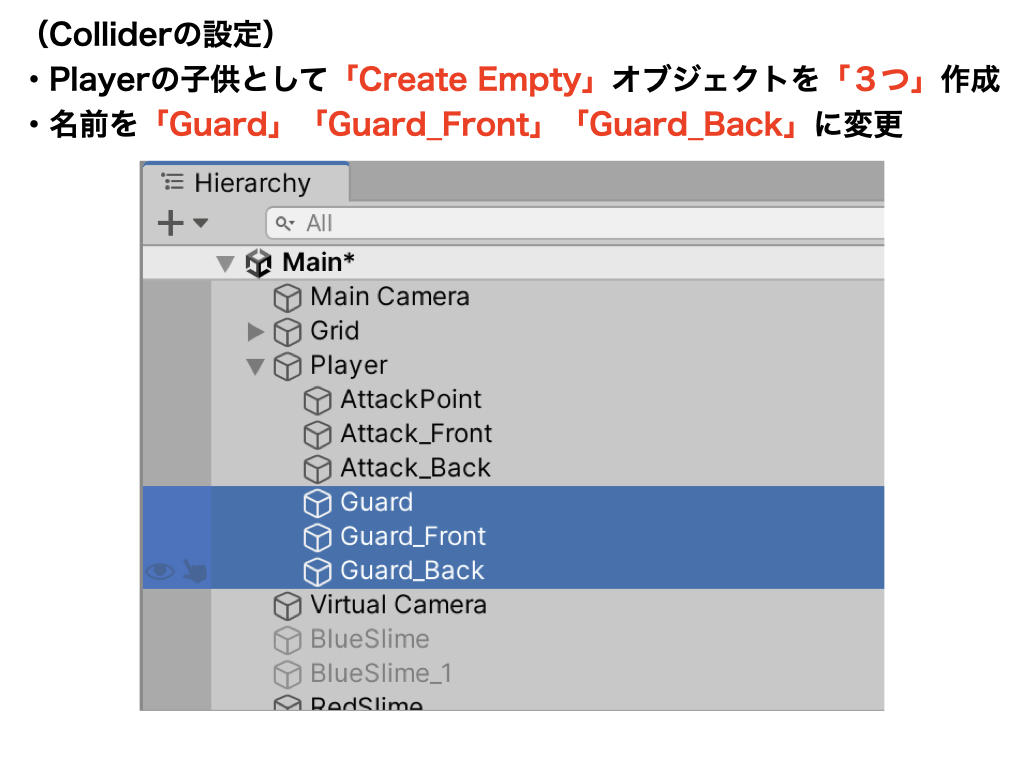
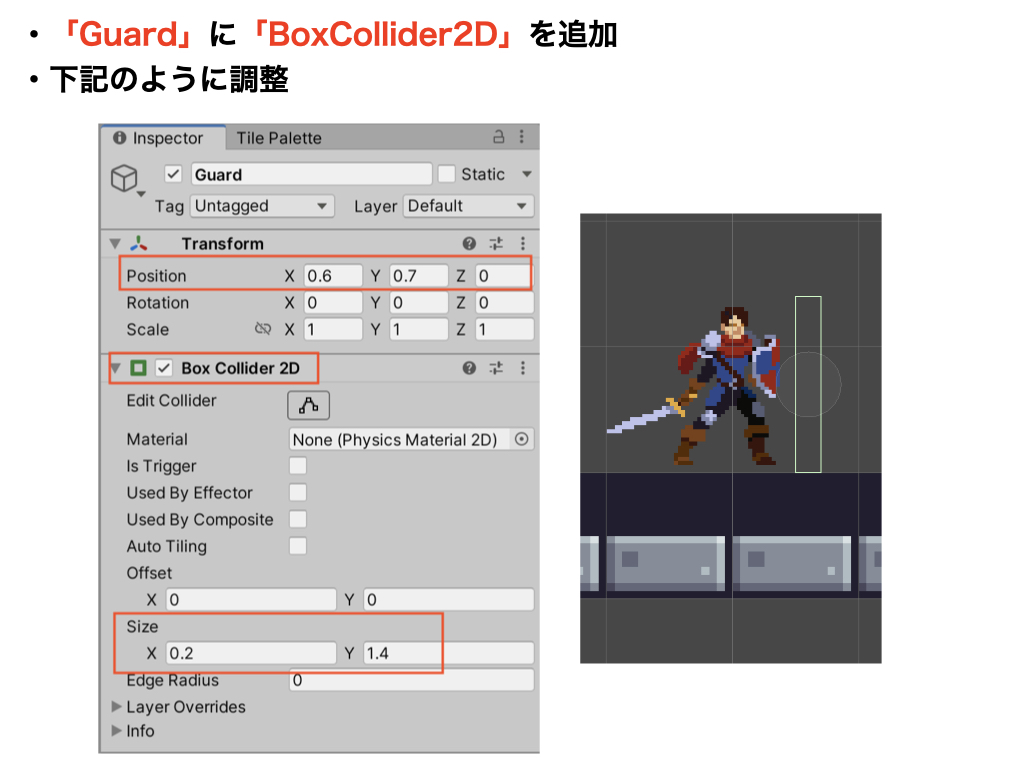
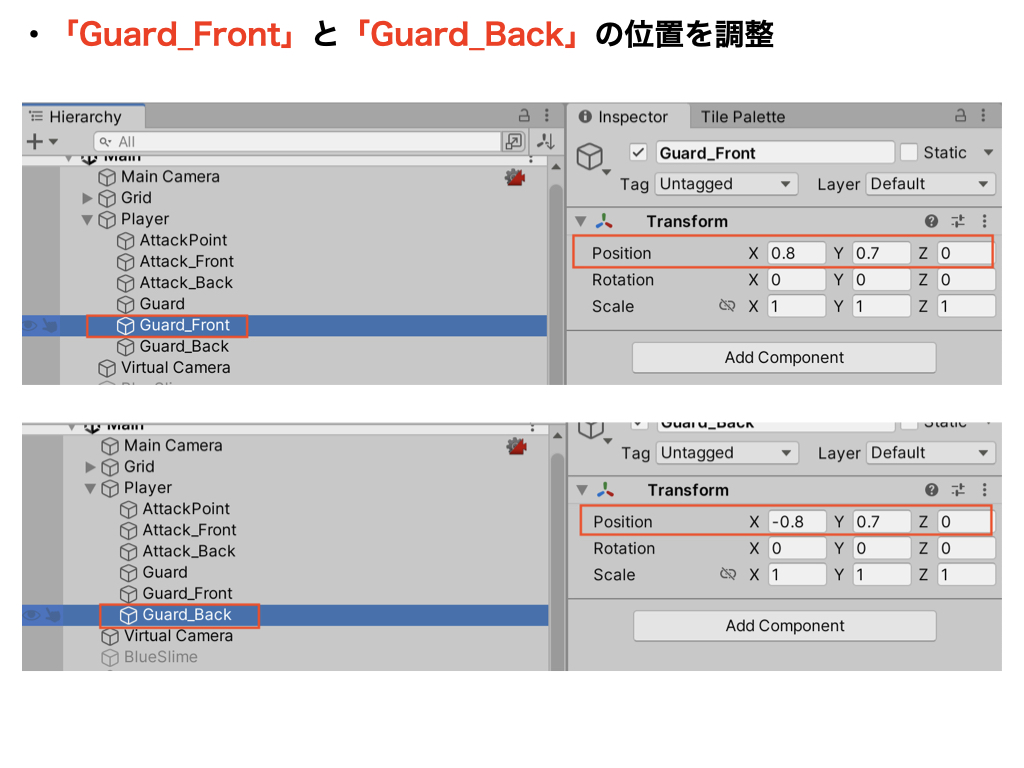
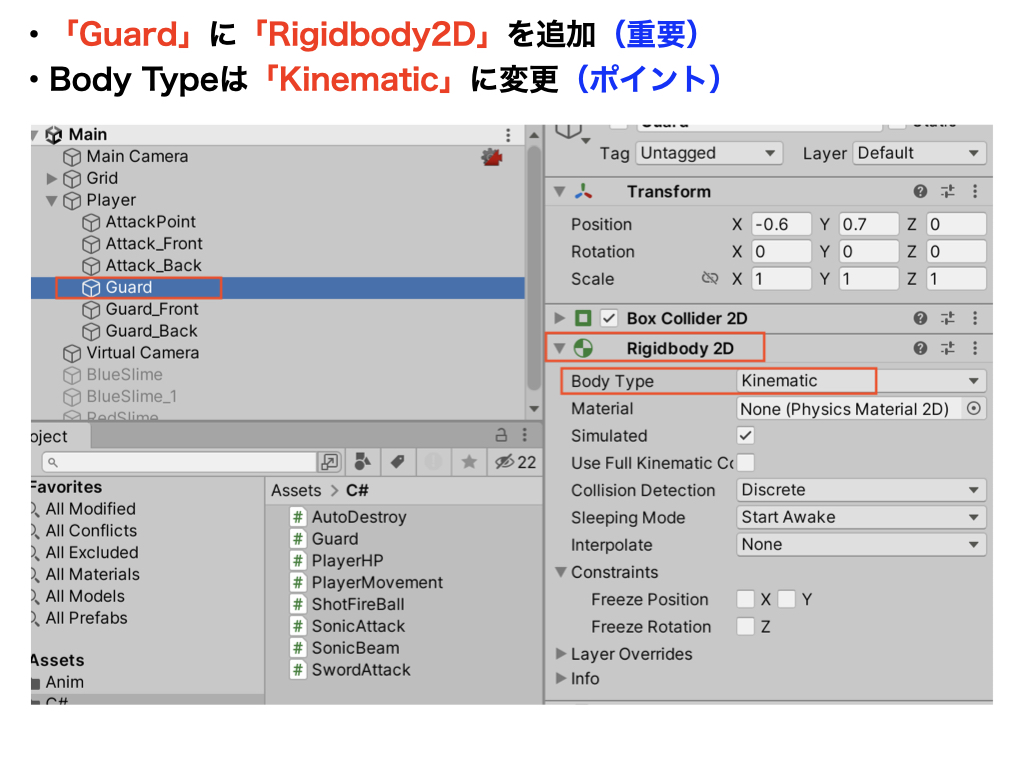
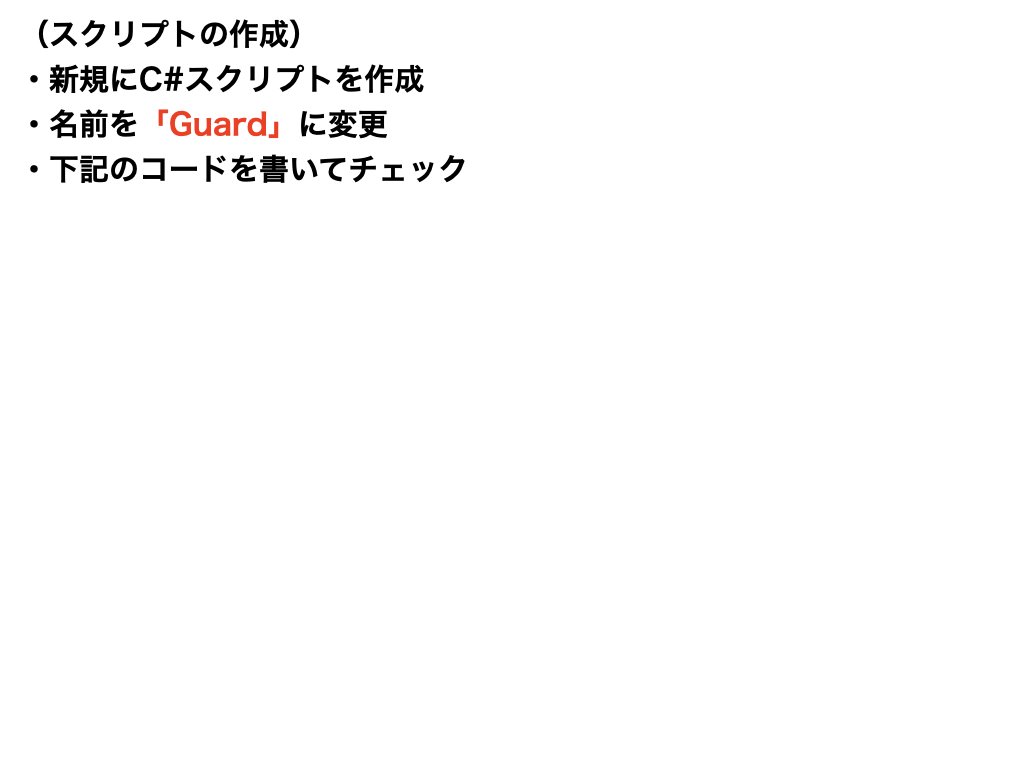
Guard機能の実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Guard : MonoBehaviour
{
public GameObject player;
public GameObject guard_front;
public GameObject guard_back;
public AudioClip sound;
private PlayerMovement pm;
void Start()
{
// ガードの初期位置
transform.position = guard_front.transform.position;
pm = player.GetComponent<PlayerMovement>();
}
private void Update()
{
// 「ガードの位置」を「Playerの向き」に合わせる。
if(pm.moveH == 1) // Playerが右を向いている場合
{
transform.position = guard_front.transform.position;
}
else if(pm.moveH == -1) // Playerが左を向いている場合
{
transform.position = guard_back.transform.position;
}
}
// 盾としての機能(FireBallを破壊する)
private void OnCollisionEnter2D(Collision2D collision)
{
if(collision.gameObject.CompareTag("FireBall"))
{
Destroy(collision.gameObject);
AudioSource.PlayClipAtPoint(sound, transform.position);
}
}
}
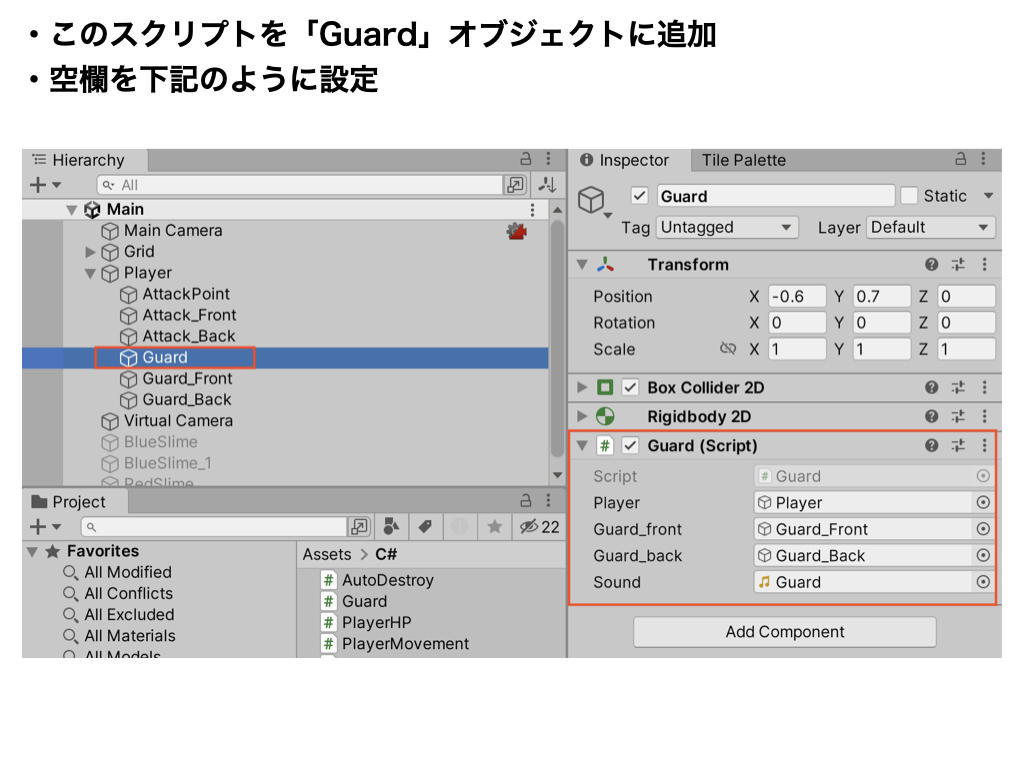
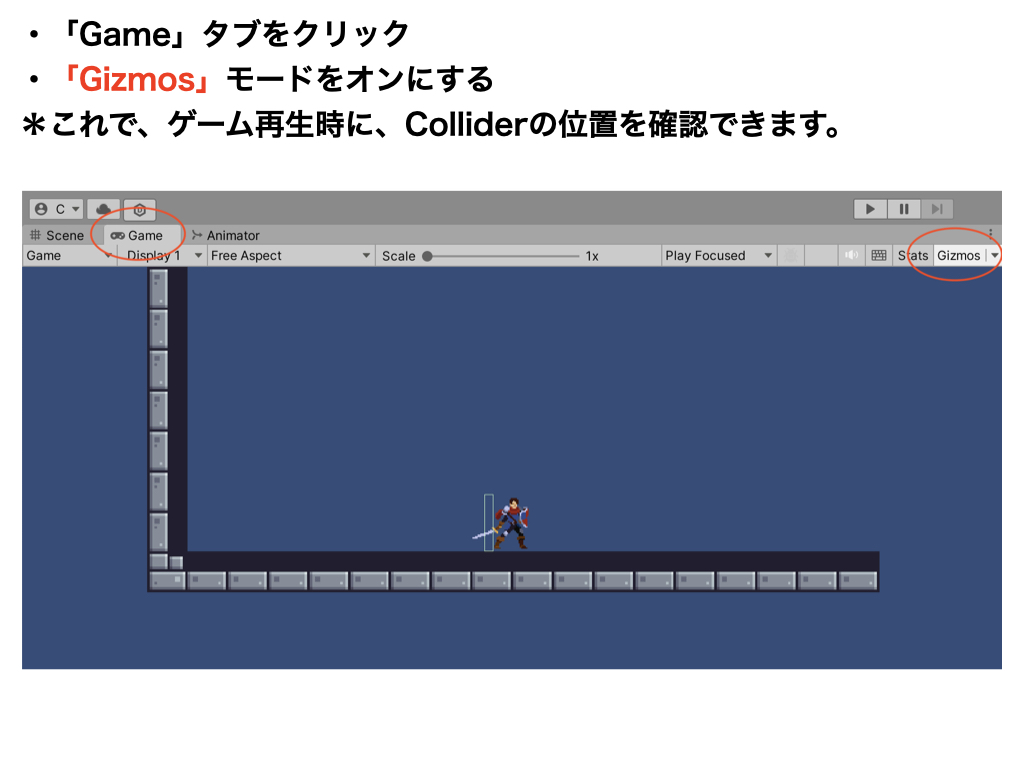
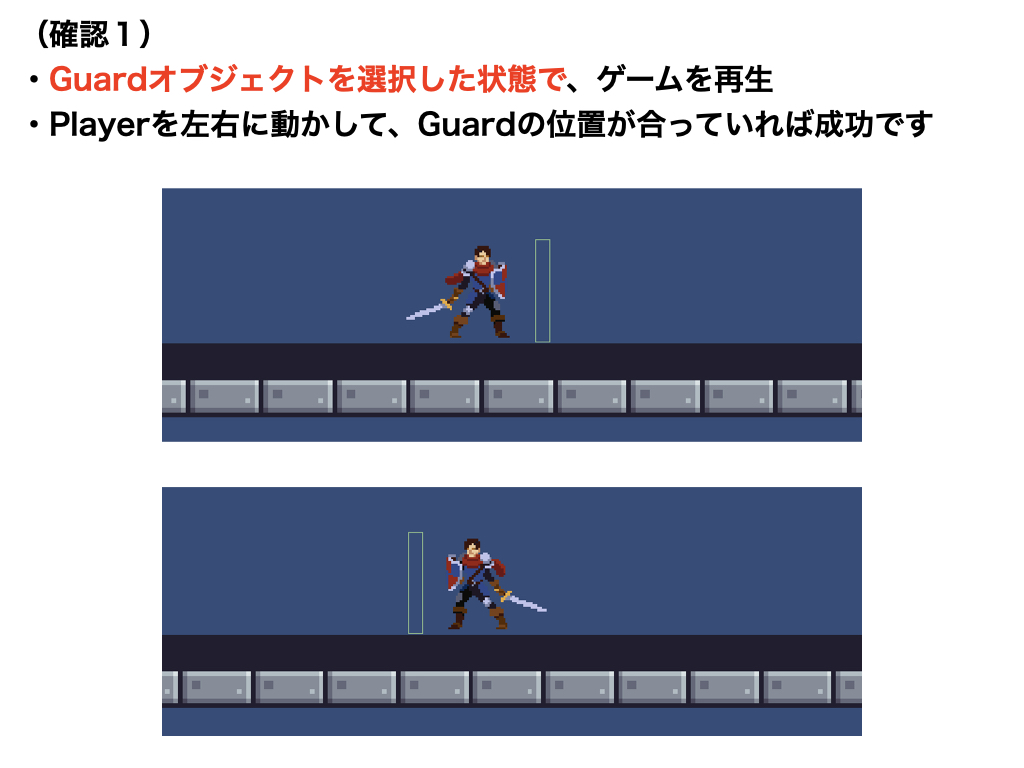
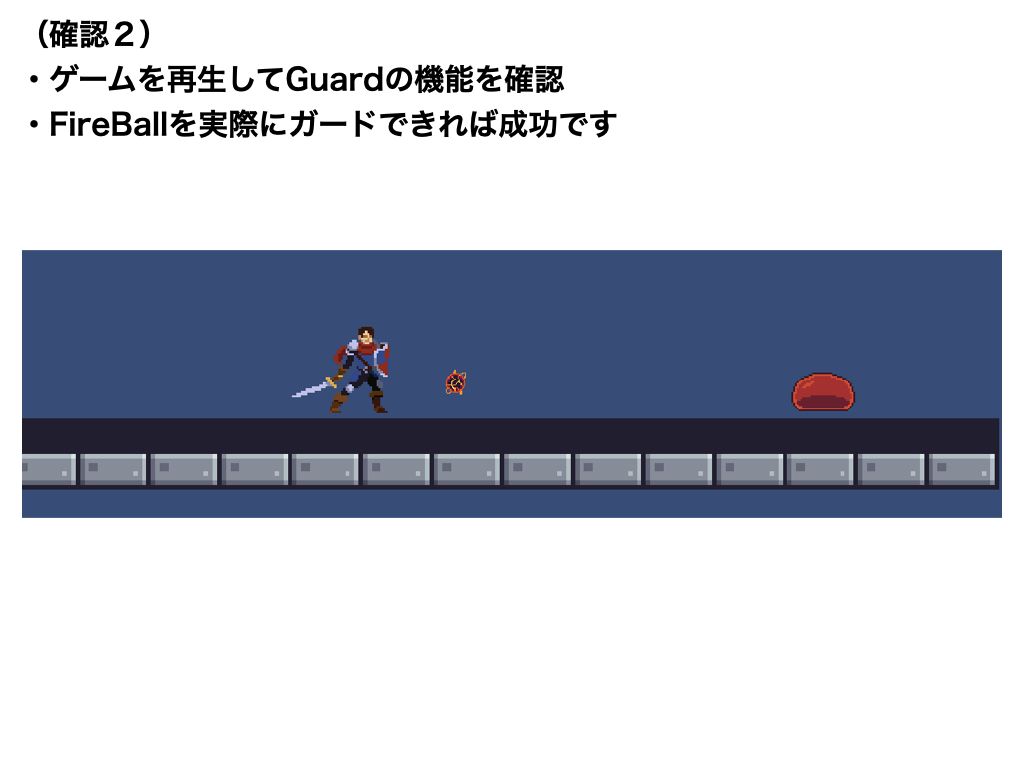
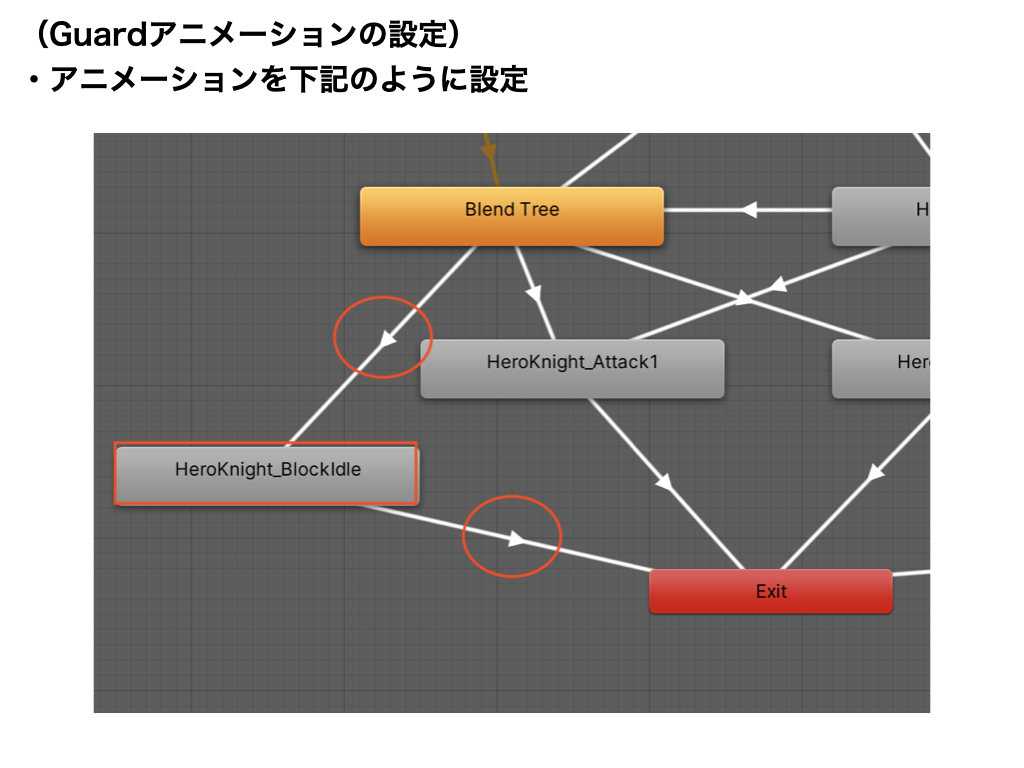
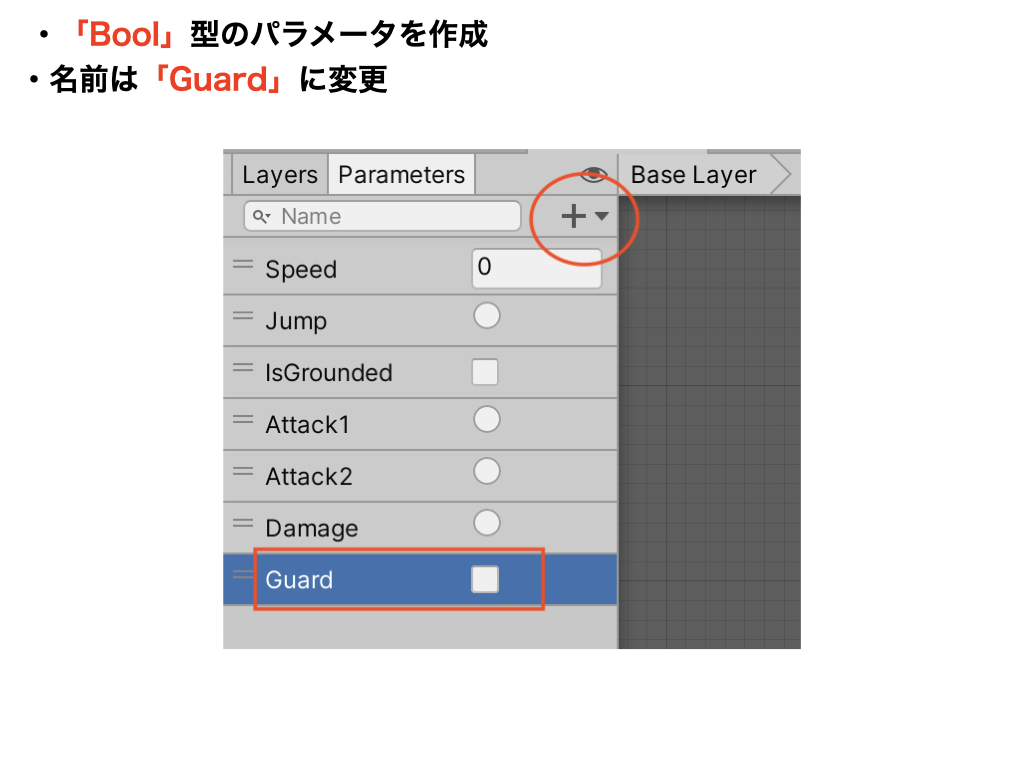
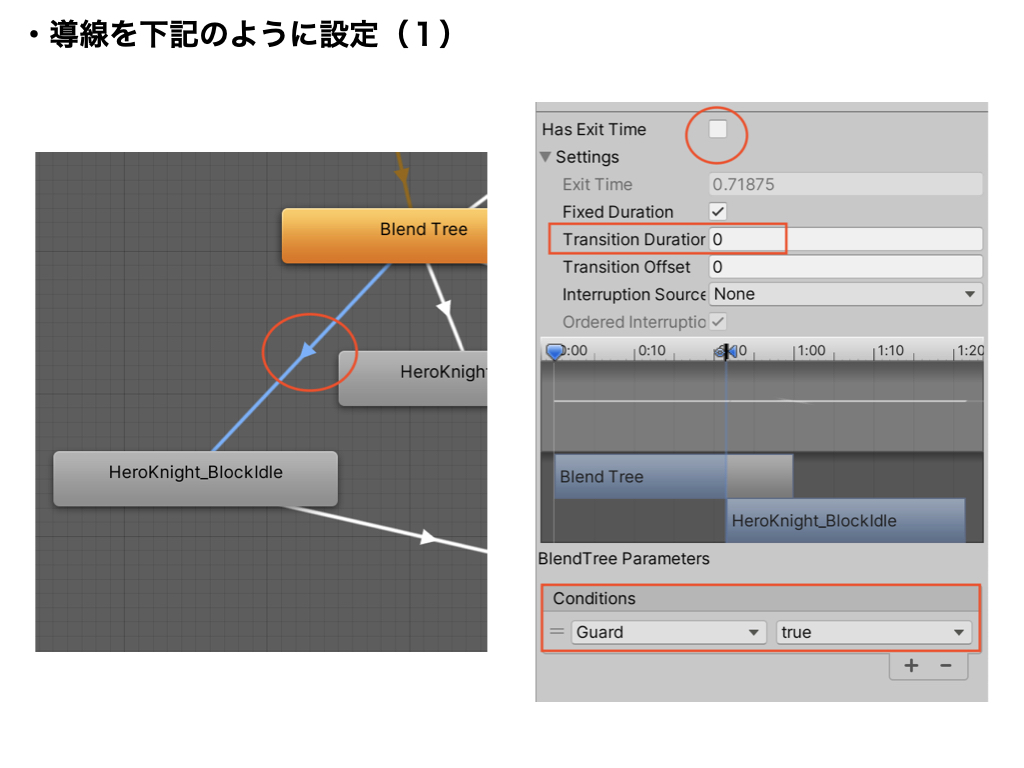
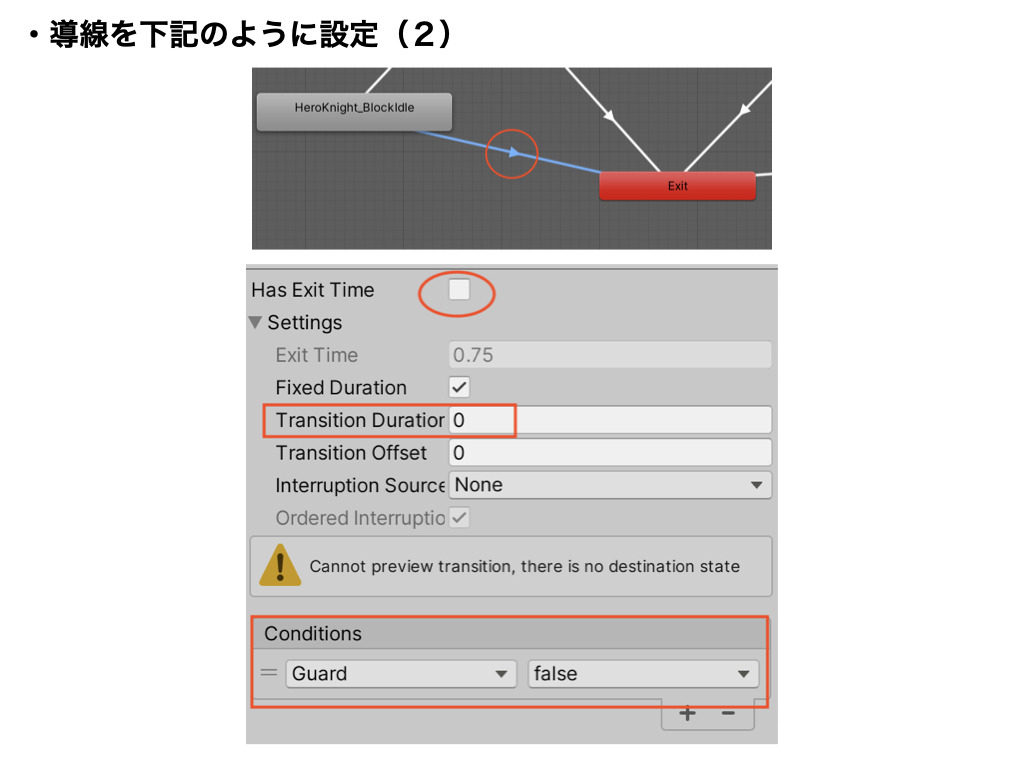
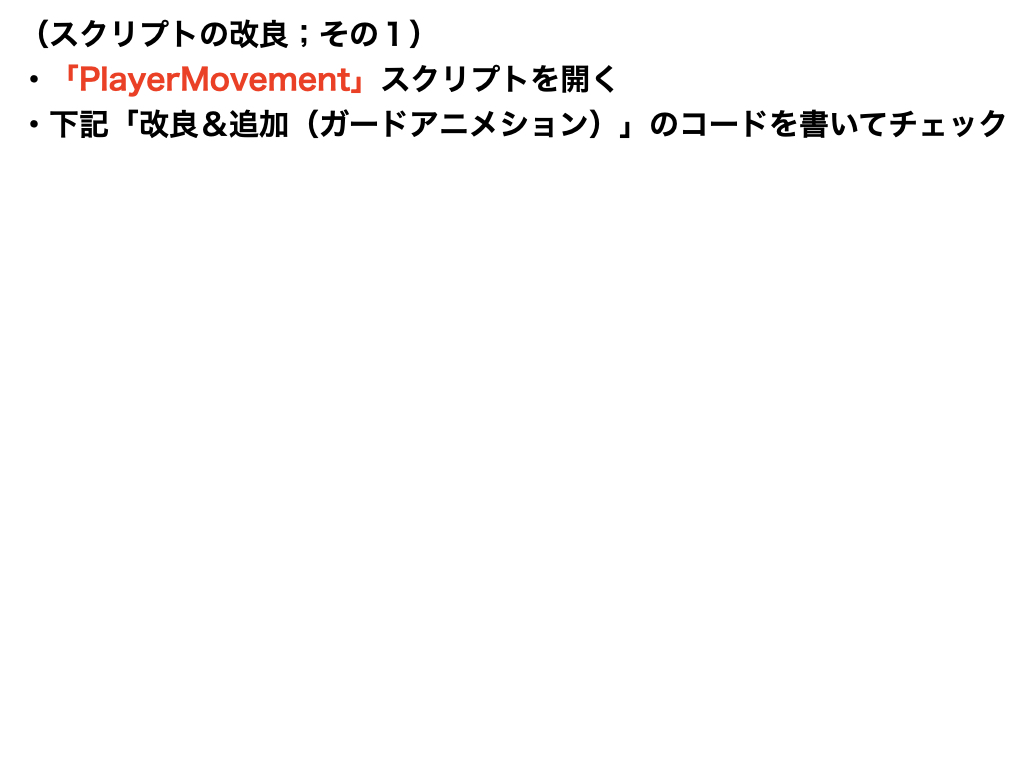
ガードアニメーションの実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed;
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
// ★改良(ガードアニメーション)
// ガード中はソニックの発射不可
// 下記のコードをコメントアウトする(もしくは削除)
//public AudioClip sonicSound;
// ★追加(ガードアニメーション)
private bool isGard = false;
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
}
void Update()
{
// ★追加(ガードアニメーション)
// ガード中は移動不可
if(isGard == false)
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
}
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
else if (Input.GetKeyDown(KeyCode.X))
{
if (moveH != 0)
{
return;
}
// ★改良(ガードアニメーション)
// ガード中はソニックの発射不可
// 下記のコードをコメントアウトする(もしくは削除)
//AudioSource.PlayClipAtPoint(sonicSound, transform.position);
animator.SetTrigger("Attack2");
}
// ★追加(ガードアニメーション)
if (Input.GetKeyDown(KeyCode.C)) // ガード発動のキーは自由に変更可能
{
// 移動中はガード不可
if (moveH != 0)
{
return;
}
animator.SetBool("Guard", true);
isGard = true;
}
else if (Input.GetKeyUp(KeyCode.C))
{
animator.SetBool("Guard", false);
isGard = false;
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
}
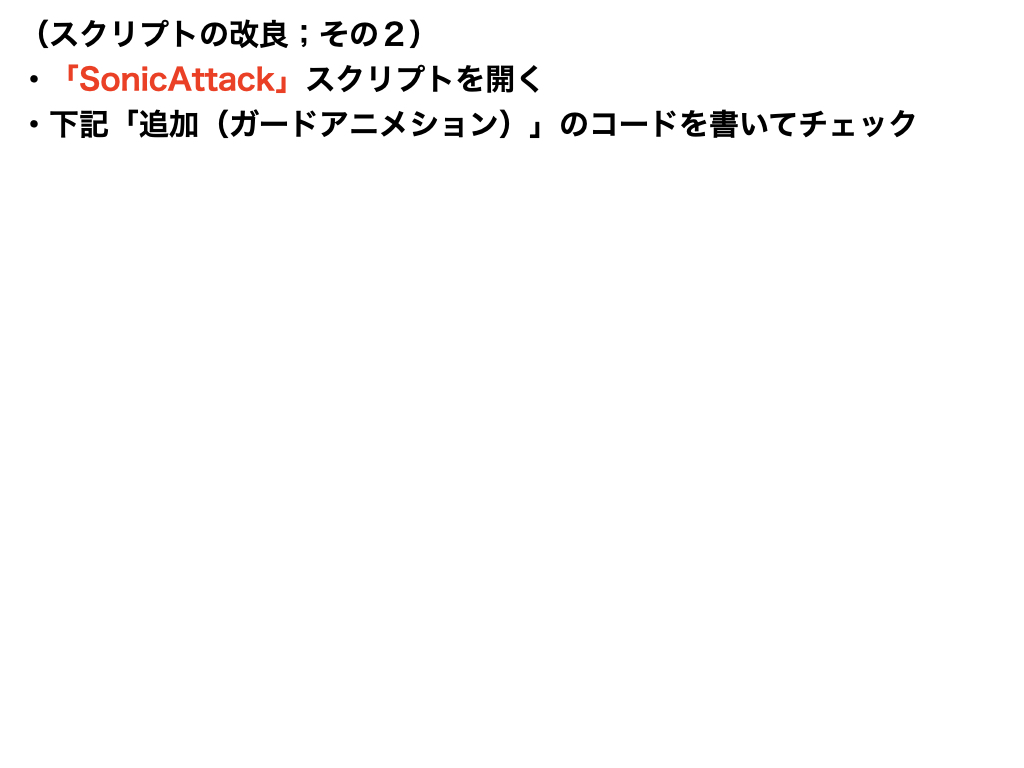
ソニックの発射音
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SonicAttack : MonoBehaviour
{
public GameObject sonicPrefab;
public GameObject attackPoint;
public GameObject attack_front;
public GameObject attack_back;
private PlayerMovement pm;
private float num;
private int sonicRotation;
private int sonicNum = 1;
// ★追加(ガードアニメーション)
public AudioClip sound;
void Start()
{
pm = GetComponent<PlayerMovement>();
attackPoint.transform.position = attack_front.transform.position;
}
void Update()
{
num = pm.moveH;
if (num == 1)
{
attackPoint.transform.position = attack_front.transform.position;
sonicRotation = 0;
sonicNum = 1;
}
else if (num == -1)
{
attackPoint.transform.position = attack_back.transform.position;
sonicRotation = 180;
sonicNum = -1;
}
}
public void S_Attack()
{
GameObject sonic = Instantiate(sonicPrefab, attackPoint.transform.position, Quaternion.Euler(0, sonicRotation, 0));
Rigidbody2D sonicRb2d = sonic.GetComponent<Rigidbody2D>();
sonicRb2d.AddForce(transform.right * 1000 * sonicNum);
// ★追加(ガードアニメーション)
// ソニックの発射音もアニメーションから実行する
AudioSource.PlayClipAtPoint(sound, Camera.main.transform.position);
Destroy(sonic, 5.0f);
}
}
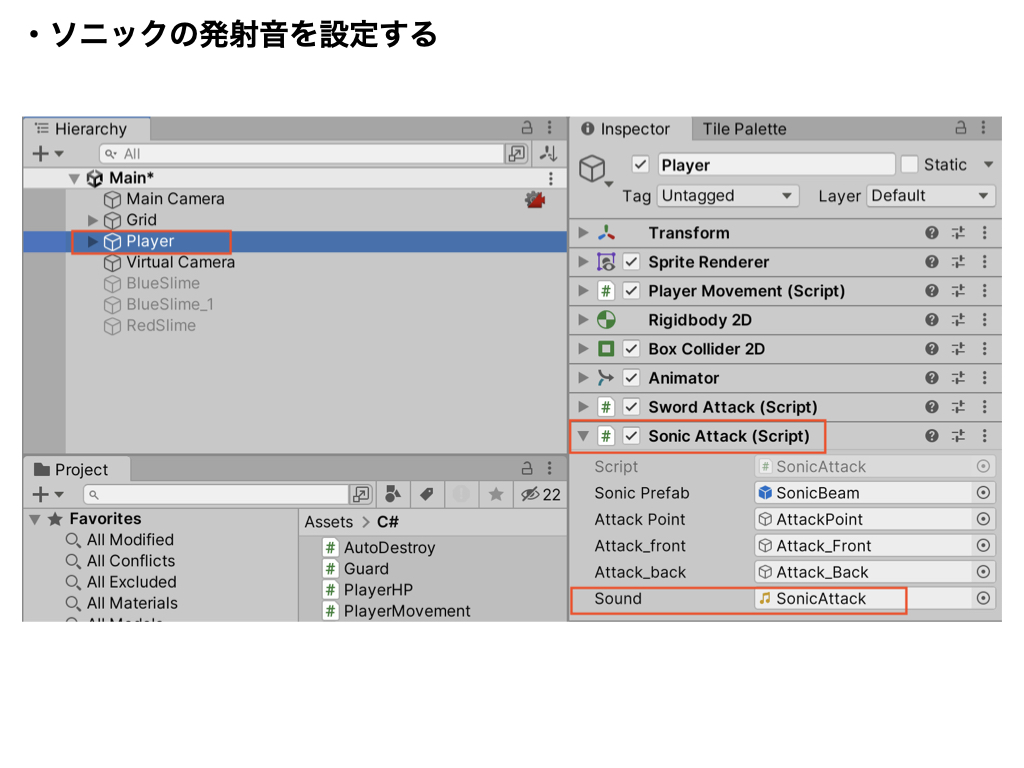
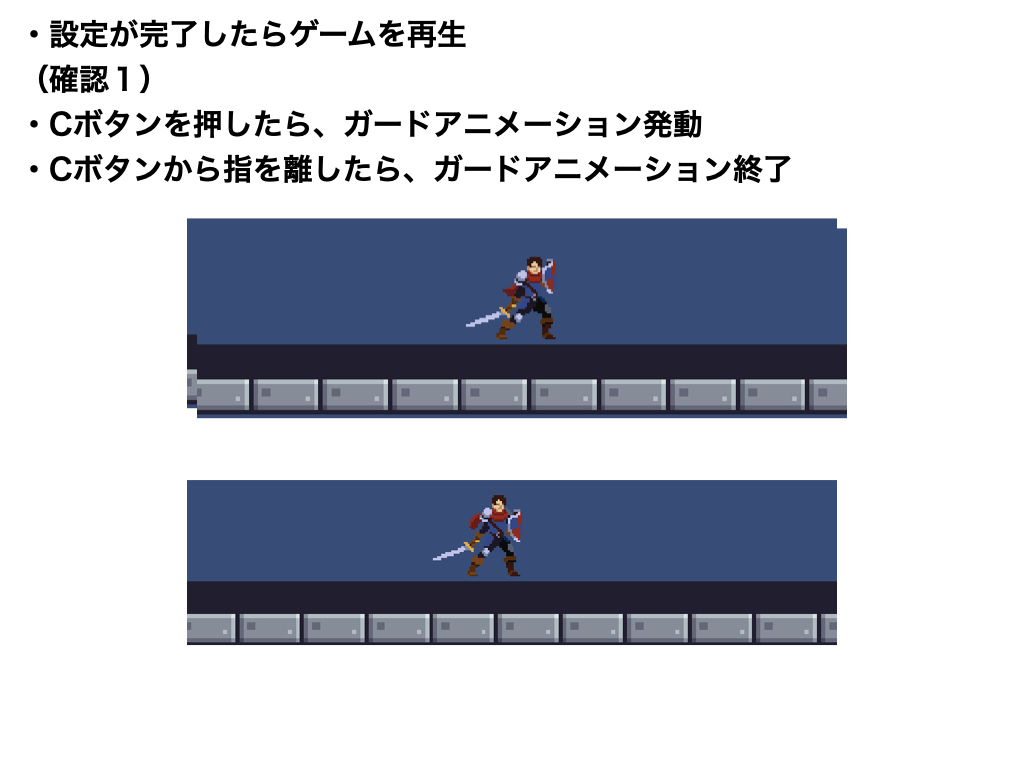
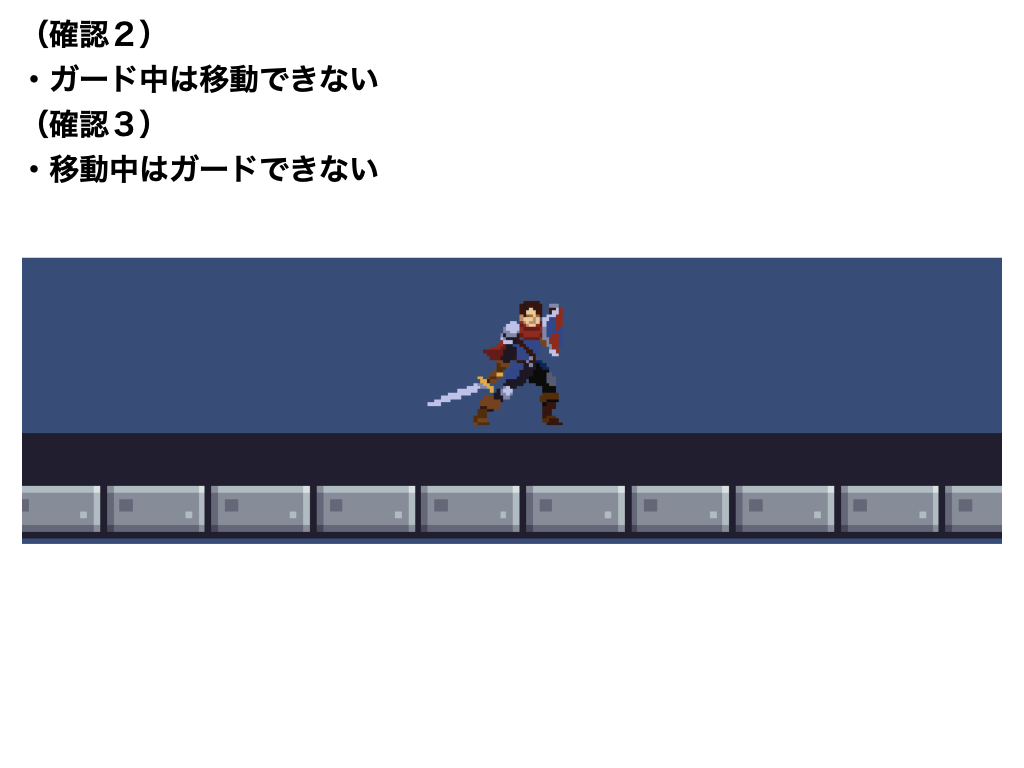
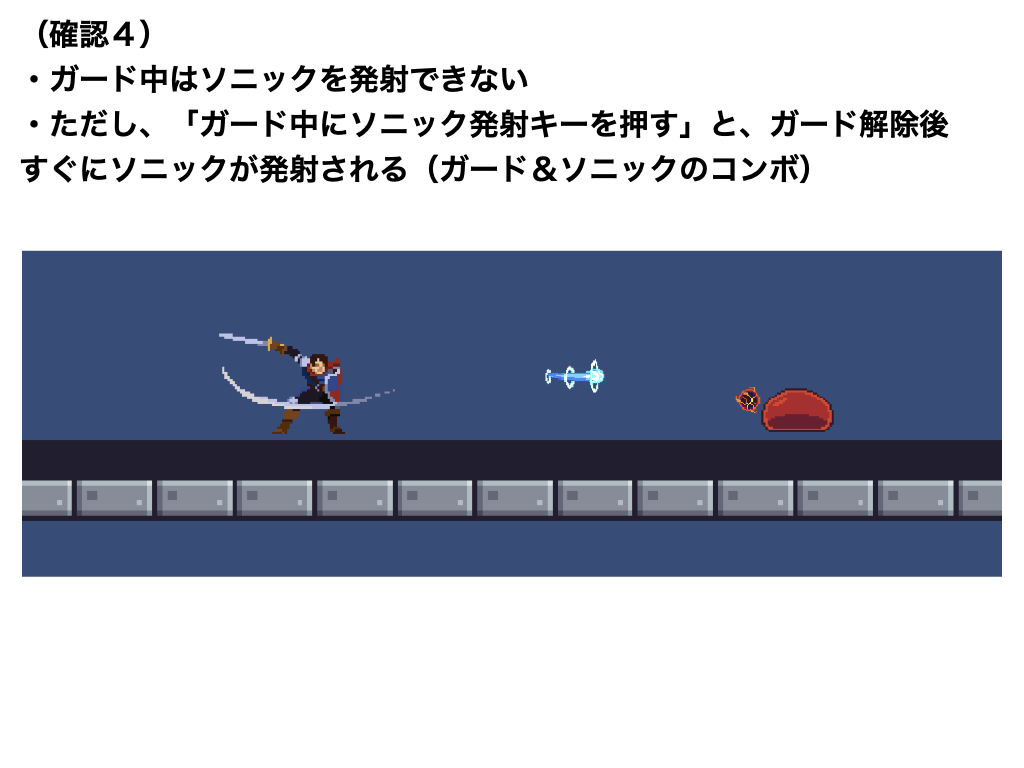
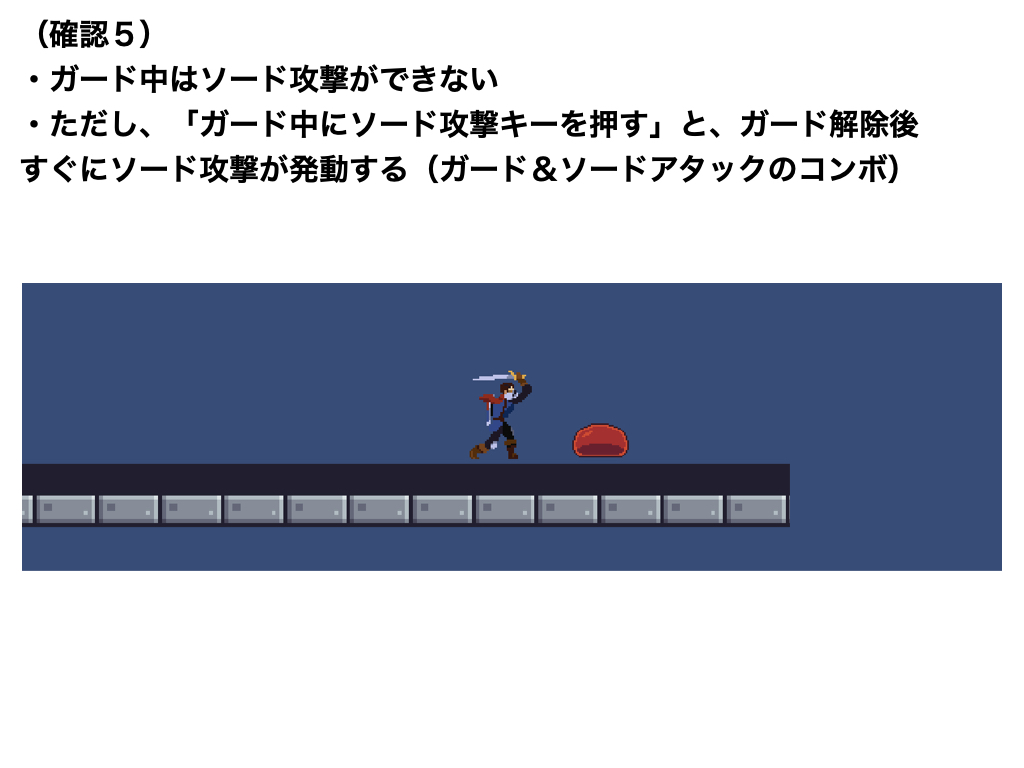
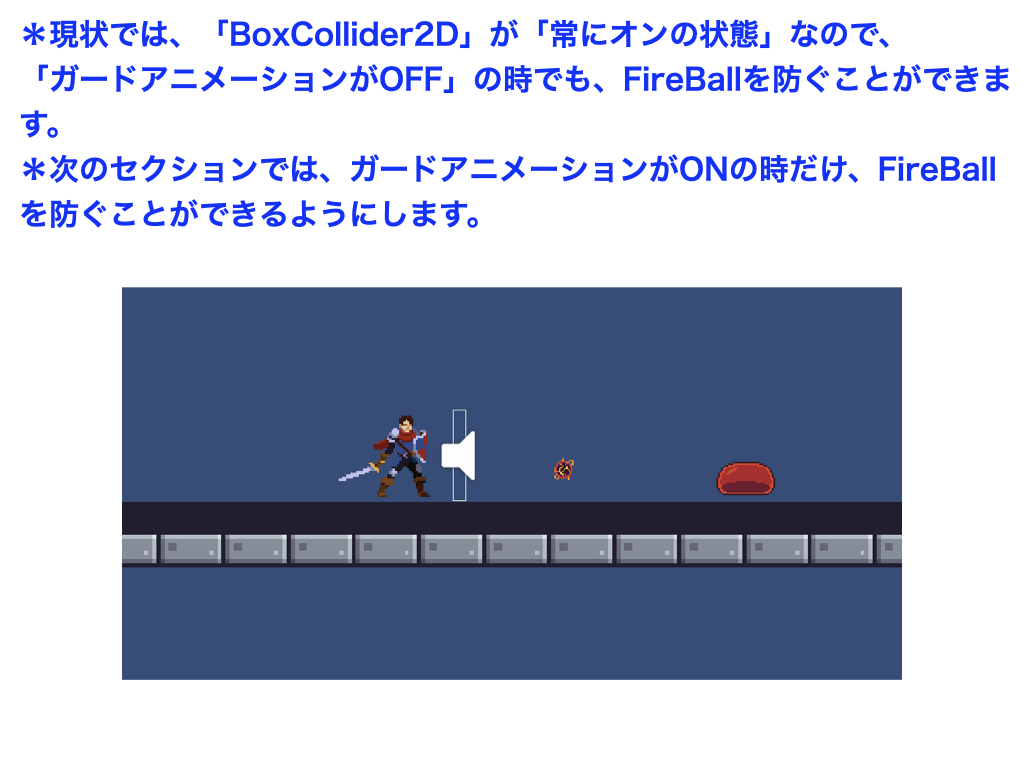
盾でFireBallをガードする