敵を倒す(通常攻撃)
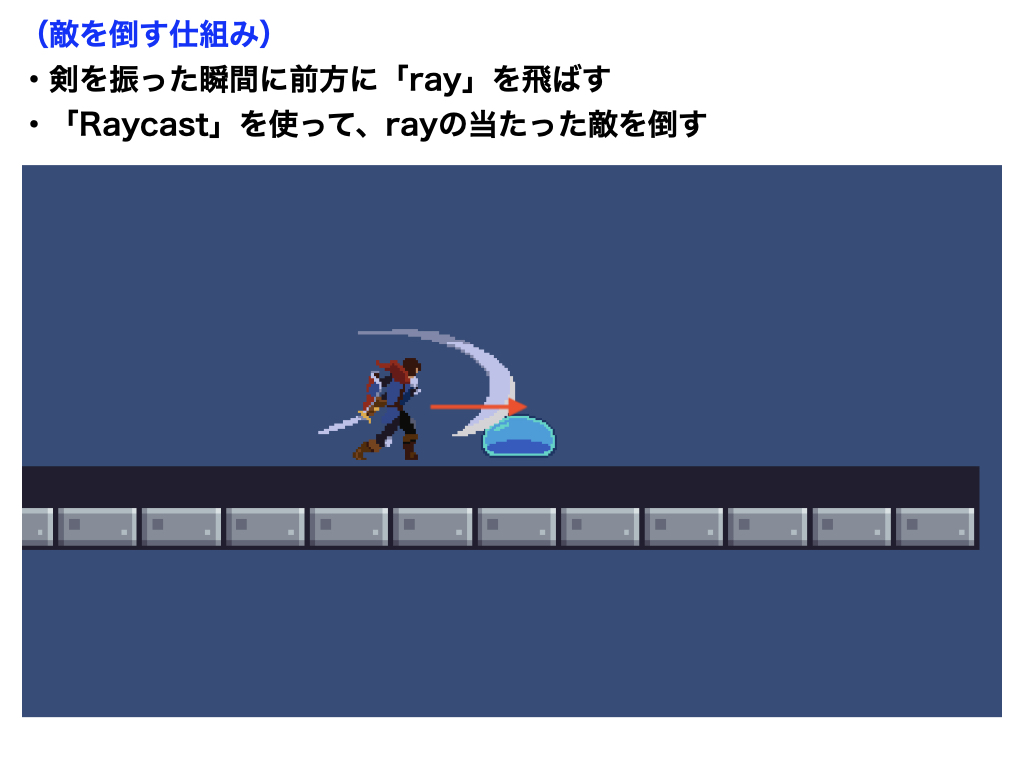
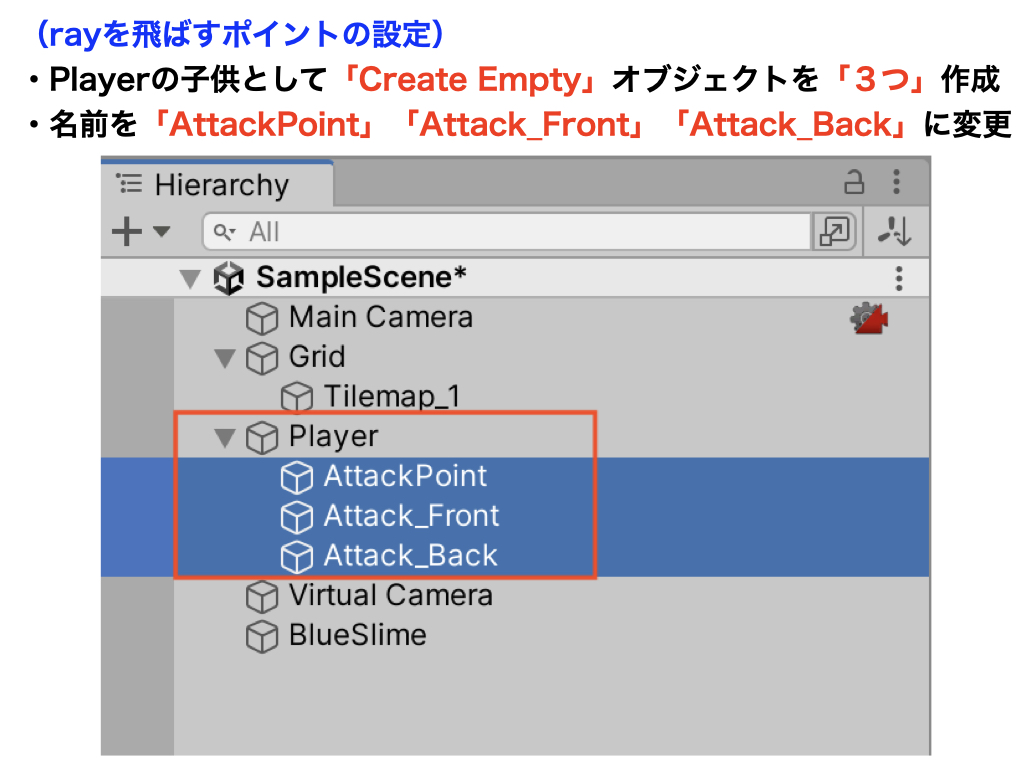
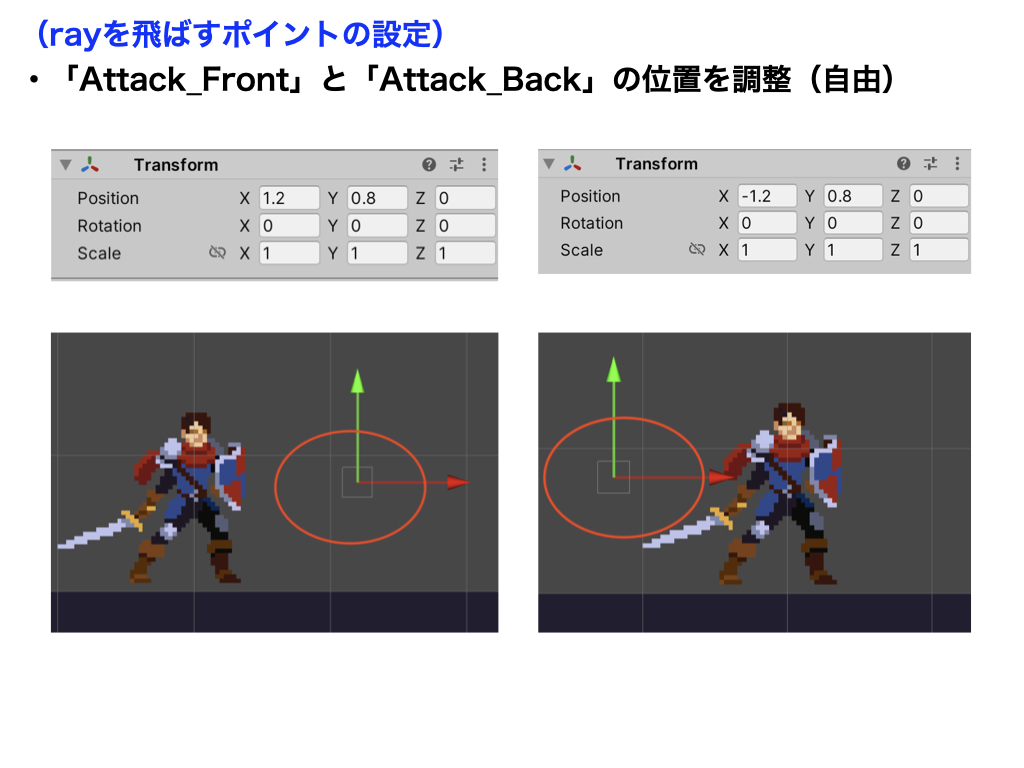
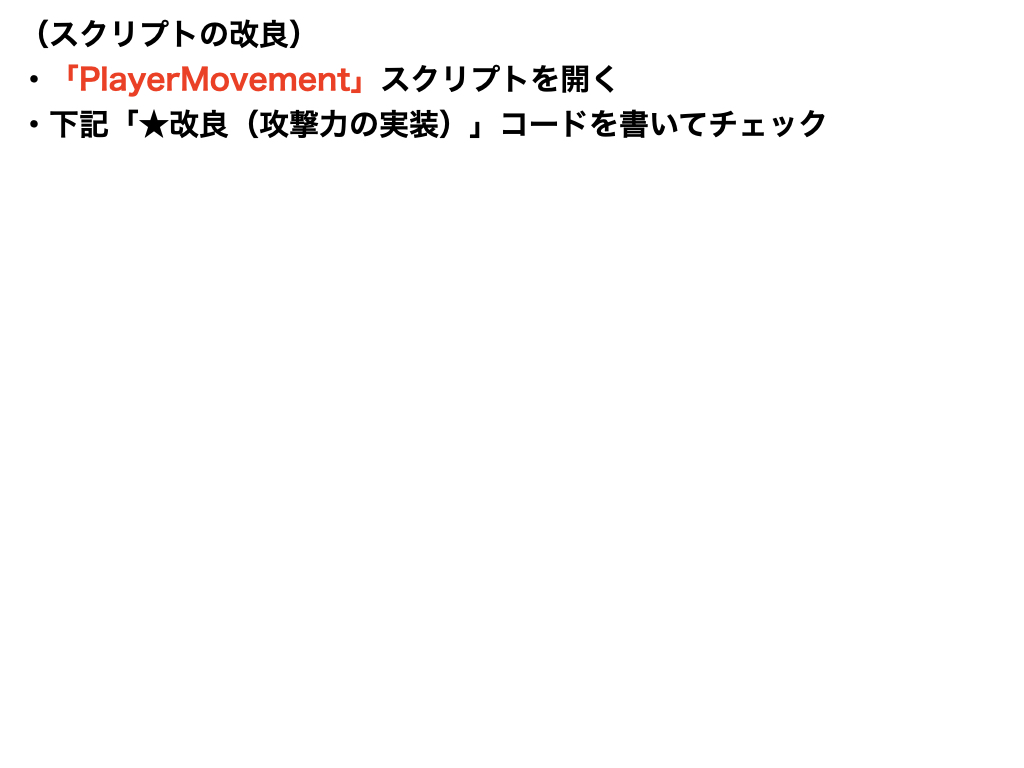
攻撃力の実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed;
// ★改良(攻撃力の実装)
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
}
void Update()
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
}
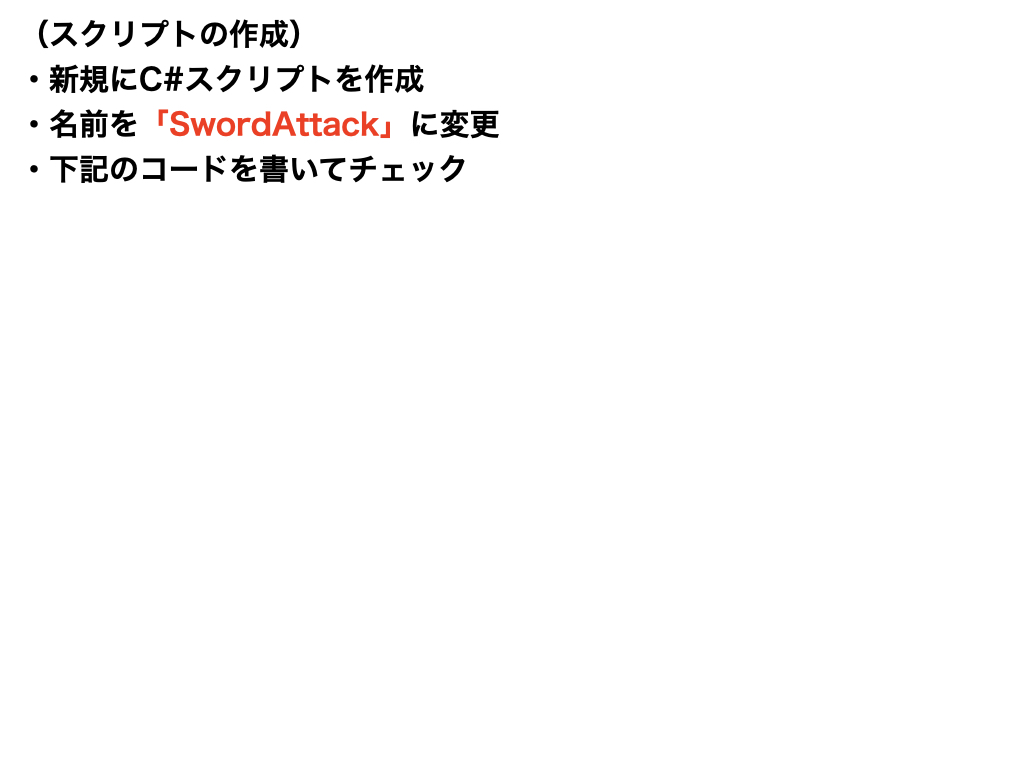
攻撃力の実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SwordAttack : MonoBehaviour
{
public AudioClip sound;
public GameObject attackPoint;
public GameObject attack_front;
public GameObject attack_back;
private RaycastHit2D hit2d;
private PlayerMovement pm;
private float num;
void Start()
{
pm = GetComponent<PlayerMovement>();
// アタックポイントの初期位置
attackPoint.transform.position = attack_front.transform.position;
}
void Update()
{
// numの値は「0」「1」「-1」のいずれかになる(テクニック)
num = pm.moveH;
// アタックポイントの位置
if (num == 1)
{
attackPoint.transform.position = attack_front.transform.position;
}
else if (num == -1)
{
attackPoint.transform.position = attack_back.transform.position;
}
// numが「1」の時は「右」に、numが「−1」の時は「左」にrayを飛ばす(ポイント)
hit2d = Physics2D.Raycast(attackPoint.transform.position, Vector2.right * num, 0.3f);
Debug.DrawRay(attackPoint.transform.position, Vector2.right * num, Color.white, 0.3f);
}
// <重要>必ず「public」にすること(アニメーションからメソッドを実行するので)
public void Attack()
{
if (hit2d.collider != null)
{
if (hit2d.collider.CompareTag("Enemy"))
{
Destroy(hit2d.collider.gameObject);
AudioSource.PlayClipAtPoint(sound, transform.position);
}
}
}
}
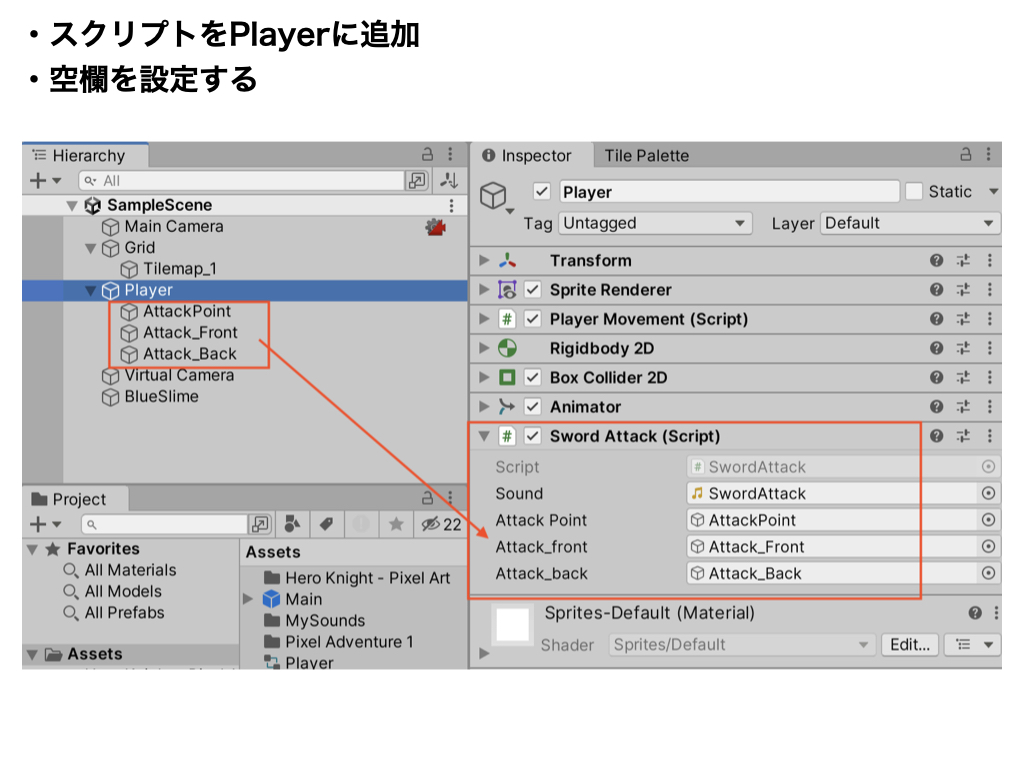
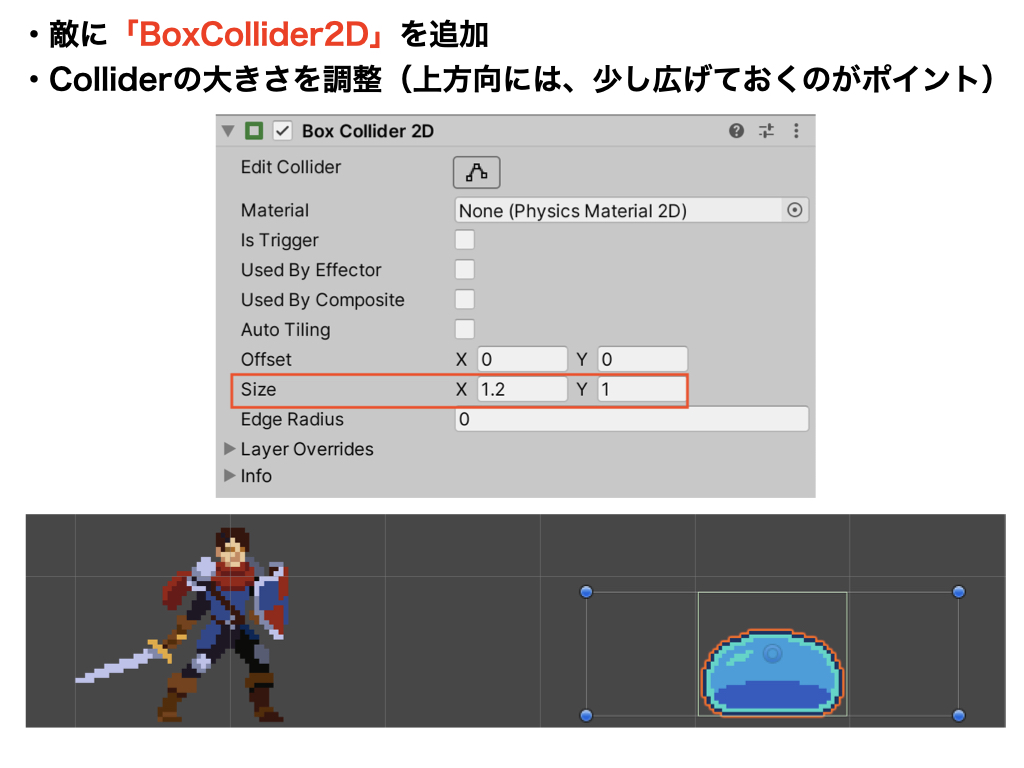
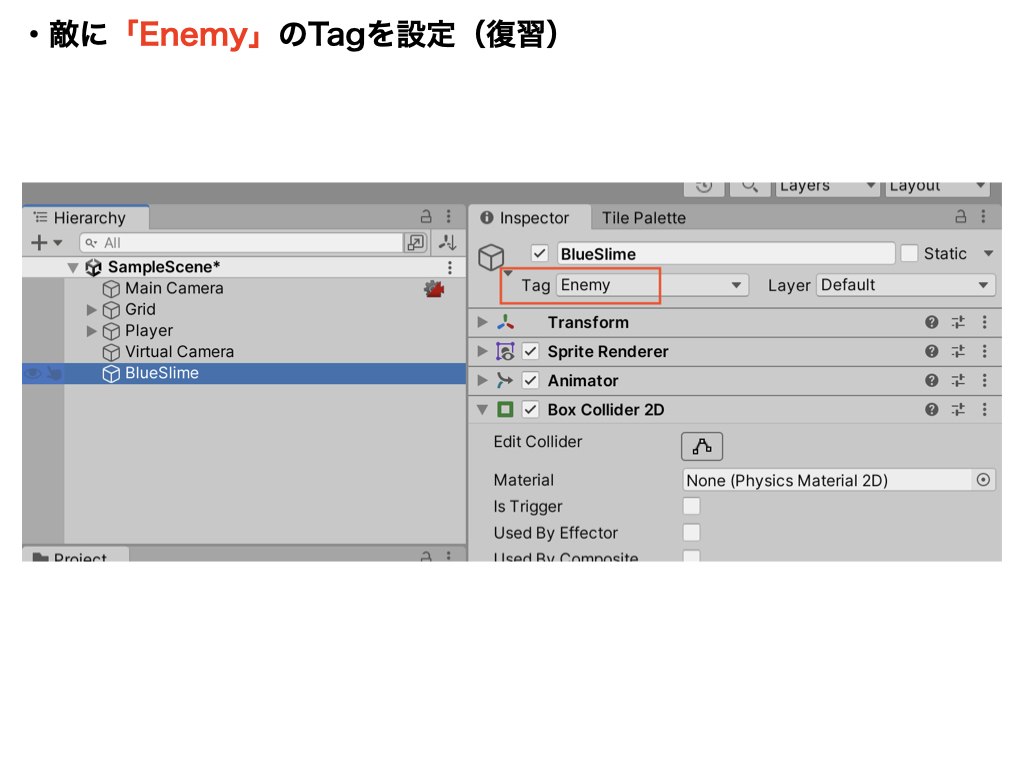
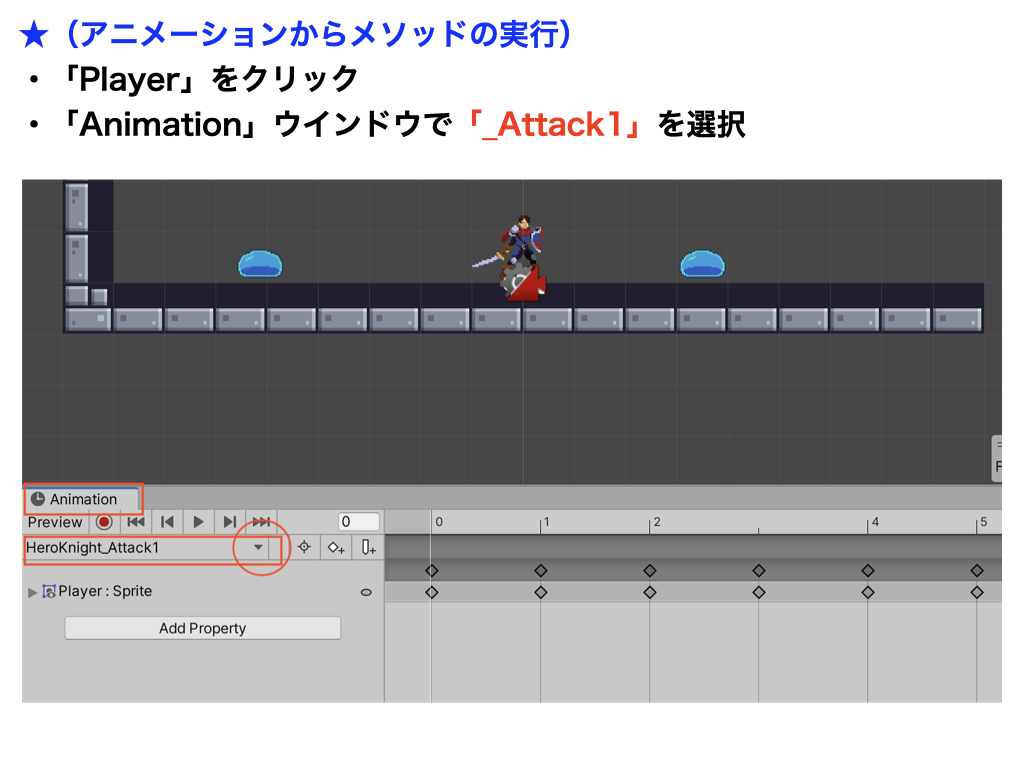
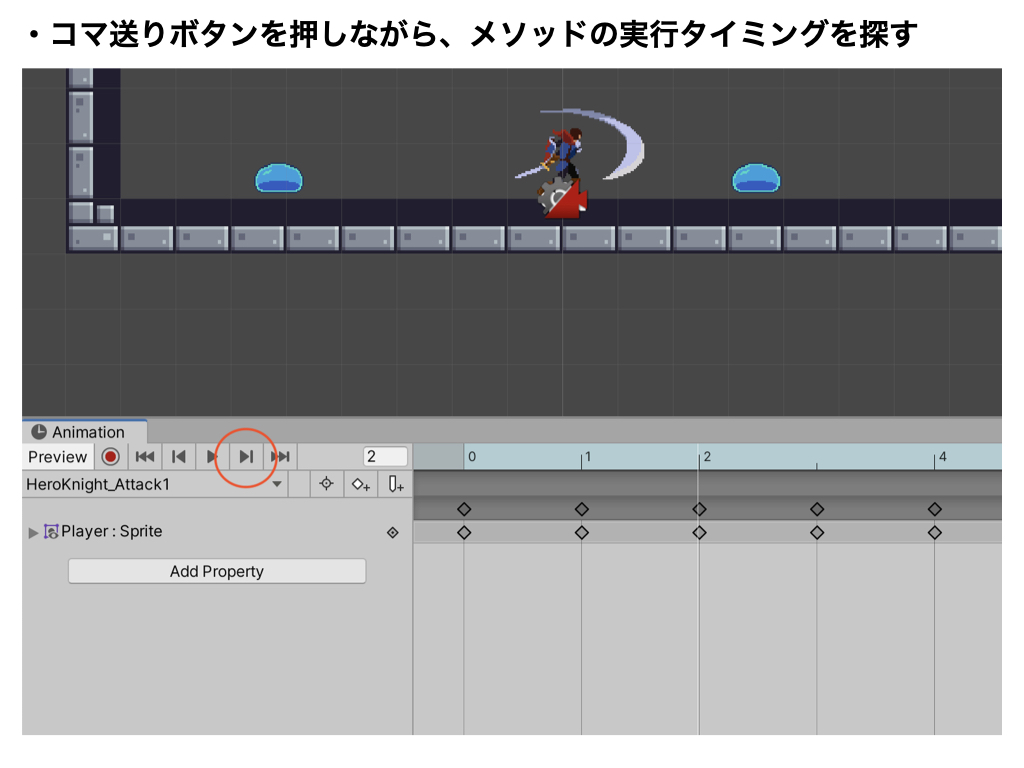
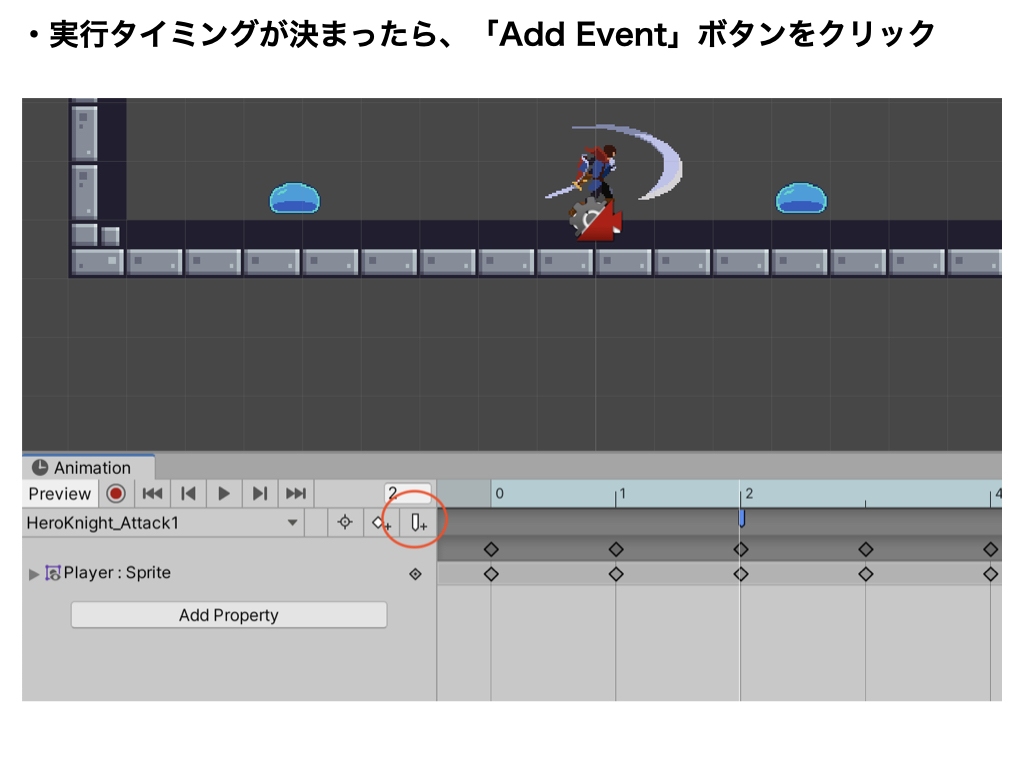
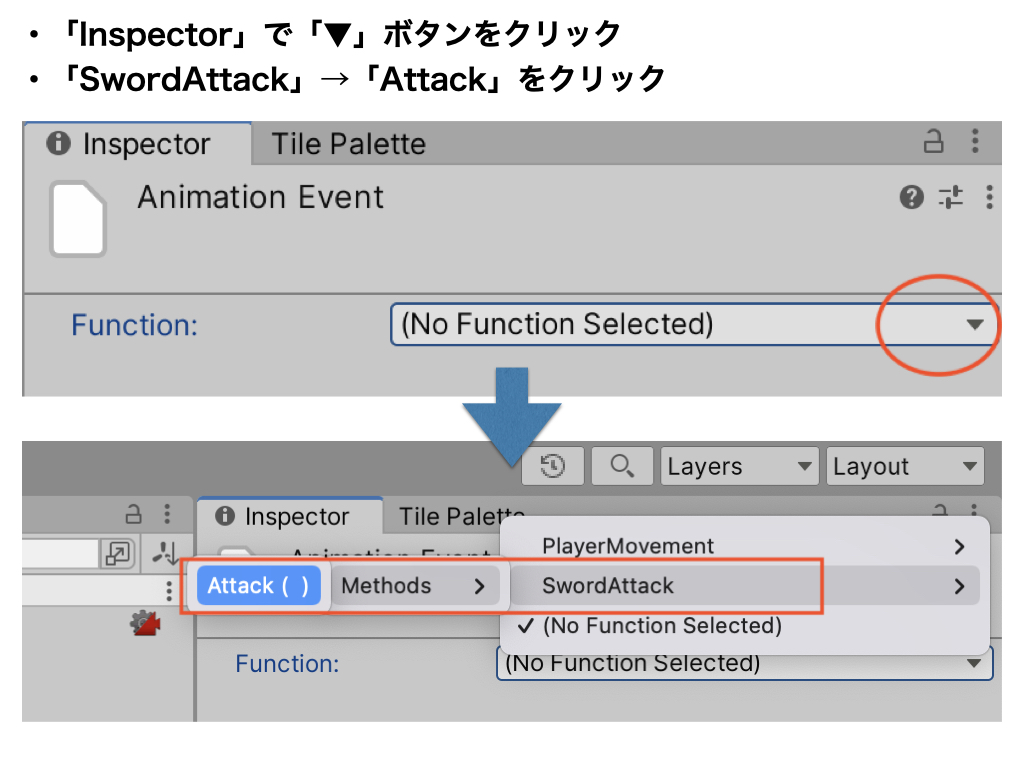
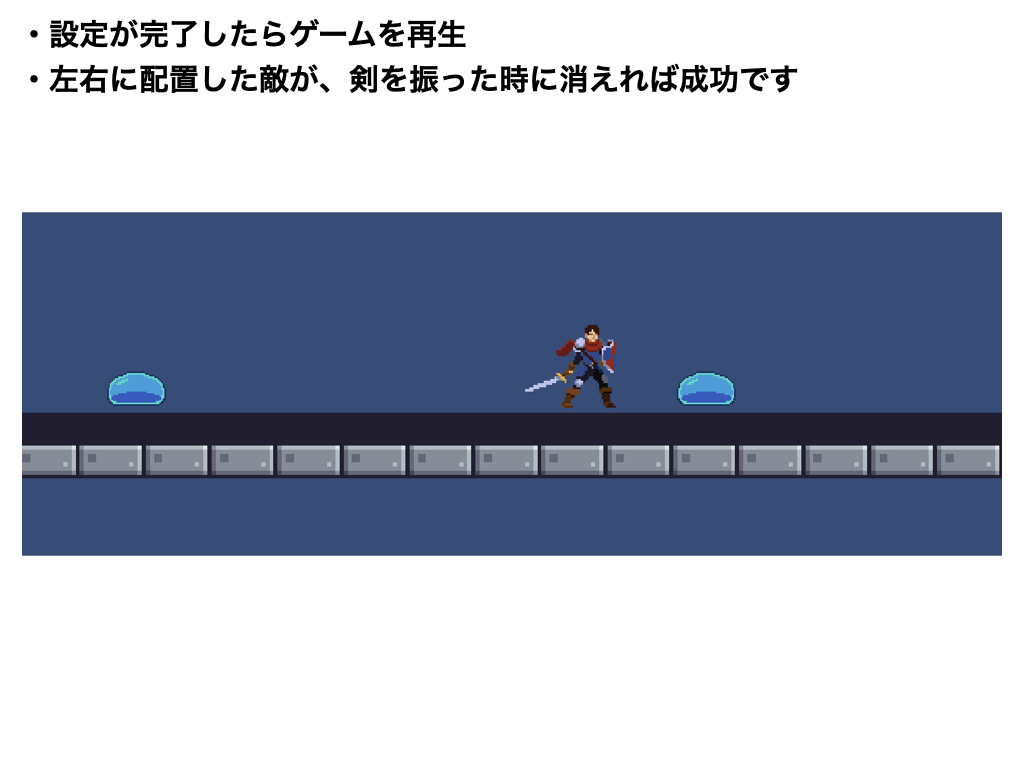
【2022版】DarkCastle(全39回)
他のコースを見る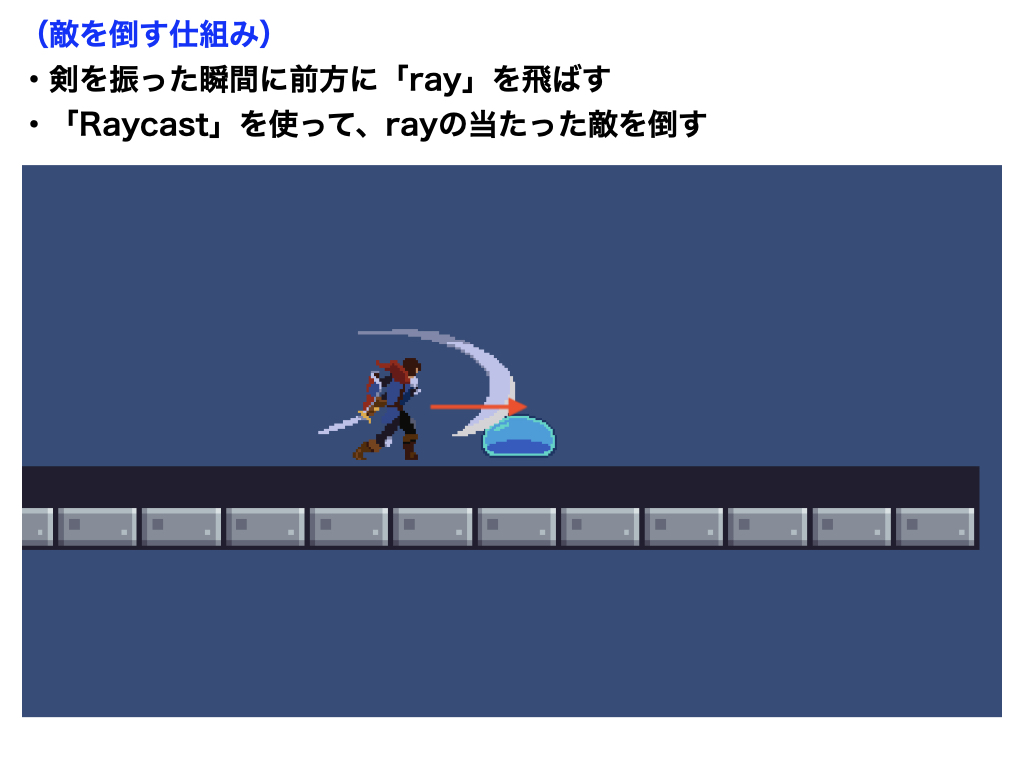
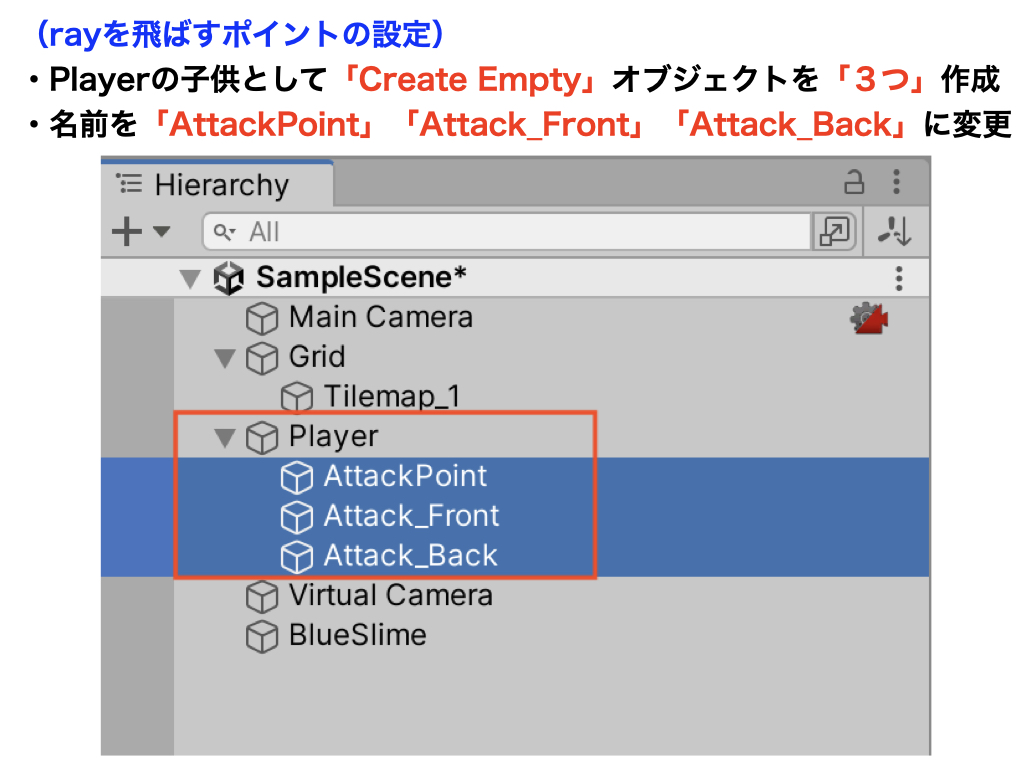
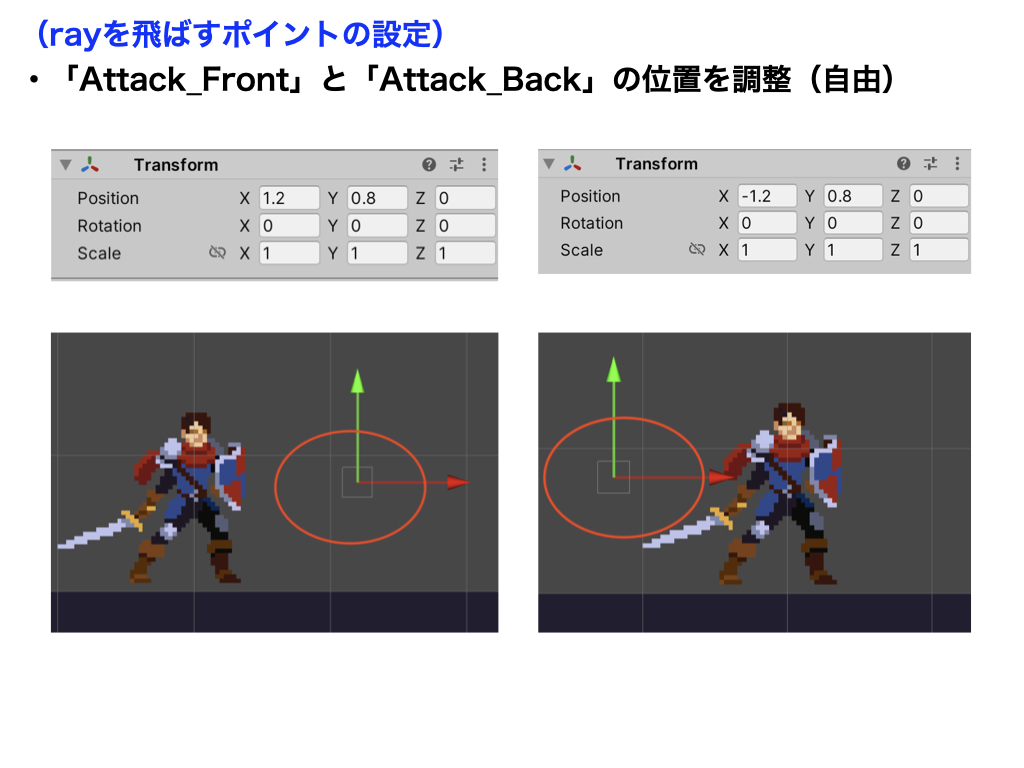
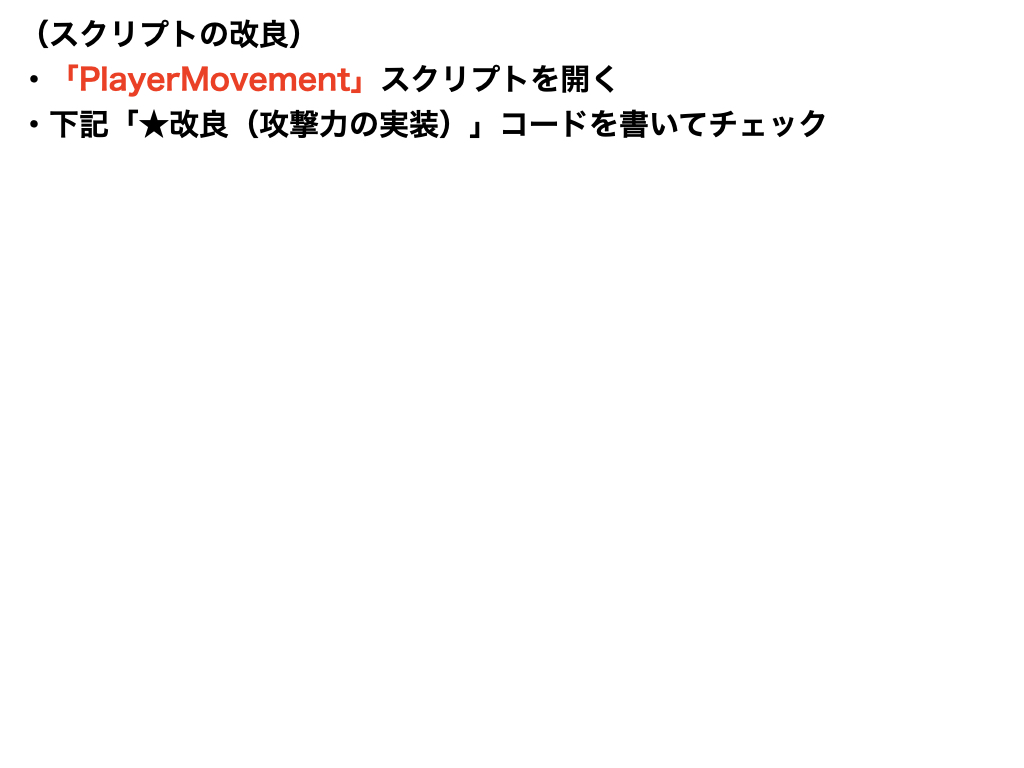
攻撃力の実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed;
// ★改良(攻撃力の実装)
public float moveH { get; set; }
private Rigidbody2D rb2d;
private Animator animator;
private SpriteRenderer spriteRenderer;
public float jumpSpeed;
public AudioClip jumpSound;
public LayerMask floor;
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
}
void Update()
{
moveH = Input.GetAxisRaw("Horizontal");
rb2d.velocity = new Vector2(moveH * speed, rb2d.velocity.y);
animator.SetFloat("Speed", moveH);
if (moveH > 0.5f)
{
spriteRenderer.flipX = false;
}
else if (moveH < -0.5f)
{
spriteRenderer.flipX = true;
}
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded())
{
rb2d.velocity = Vector2.up * jumpSpeed;
AudioSource.PlayClipAtPoint(jumpSound, Camera.main.transform.position);
animator.SetTrigger("Jump");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (moveH != 0)
{
return;
}
animator.SetTrigger("Attack1");
}
}
private bool IsGrounded()
{
RaycastHit2D hit2d = Physics2D.Raycast(transform.position, Vector2.down, 0.6f, floor);
return hit2d.collider != null;
}
}
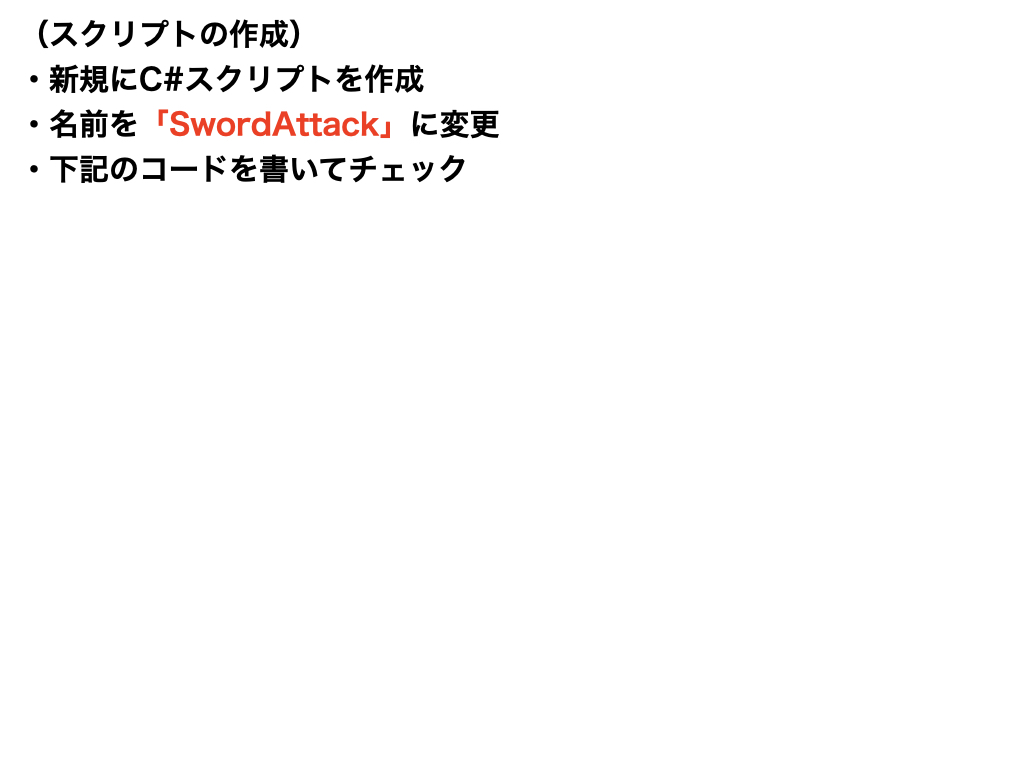
攻撃力の実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SwordAttack : MonoBehaviour
{
public AudioClip sound;
public GameObject attackPoint;
public GameObject attack_front;
public GameObject attack_back;
private RaycastHit2D hit2d;
private PlayerMovement pm;
private float num;
void Start()
{
pm = GetComponent<PlayerMovement>();
// アタックポイントの初期位置
attackPoint.transform.position = attack_front.transform.position;
}
void Update()
{
// numの値は「0」「1」「-1」のいずれかになる(テクニック)
num = pm.moveH;
// アタックポイントの位置
if (num == 1)
{
attackPoint.transform.position = attack_front.transform.position;
}
else if (num == -1)
{
attackPoint.transform.position = attack_back.transform.position;
}
// numが「1」の時は「右」に、numが「−1」の時は「左」にrayを飛ばす(ポイント)
hit2d = Physics2D.Raycast(attackPoint.transform.position, Vector2.right * num, 0.3f);
Debug.DrawRay(attackPoint.transform.position, Vector2.right * num, Color.white, 0.3f);
}
// <重要>必ず「public」にすること(アニメーションからメソッドを実行するので)
public void Attack()
{
if (hit2d.collider != null)
{
if (hit2d.collider.CompareTag("Enemy"))
{
Destroy(hit2d.collider.gameObject);
AudioSource.PlayClipAtPoint(sound, transform.position);
}
}
}
}
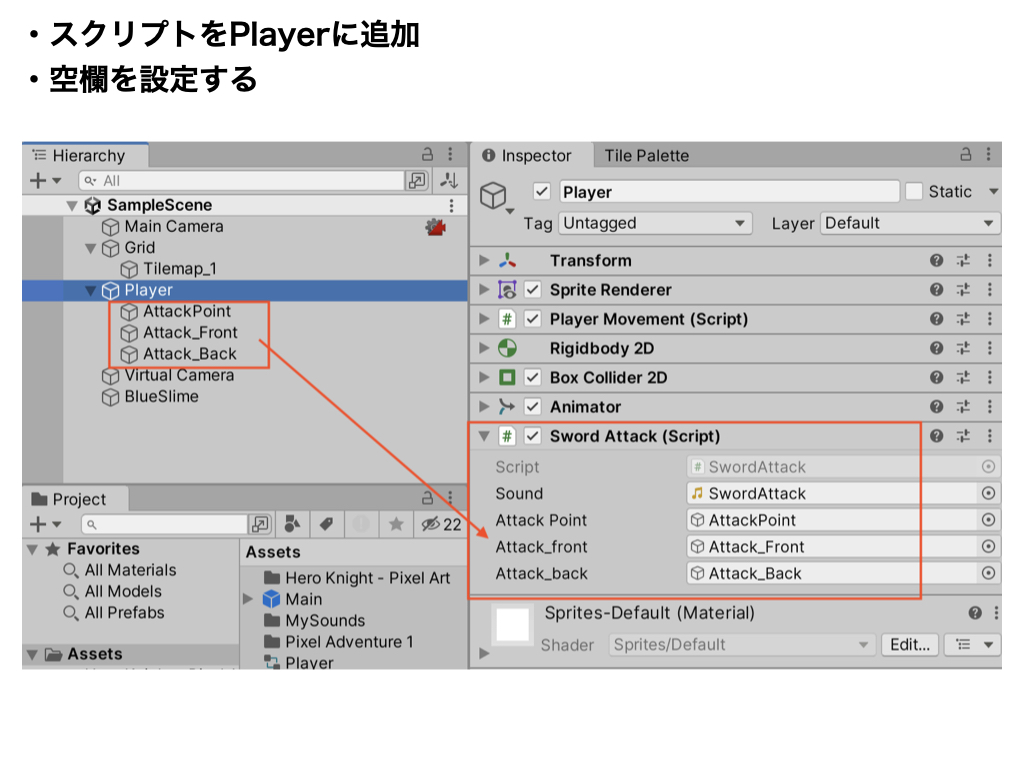
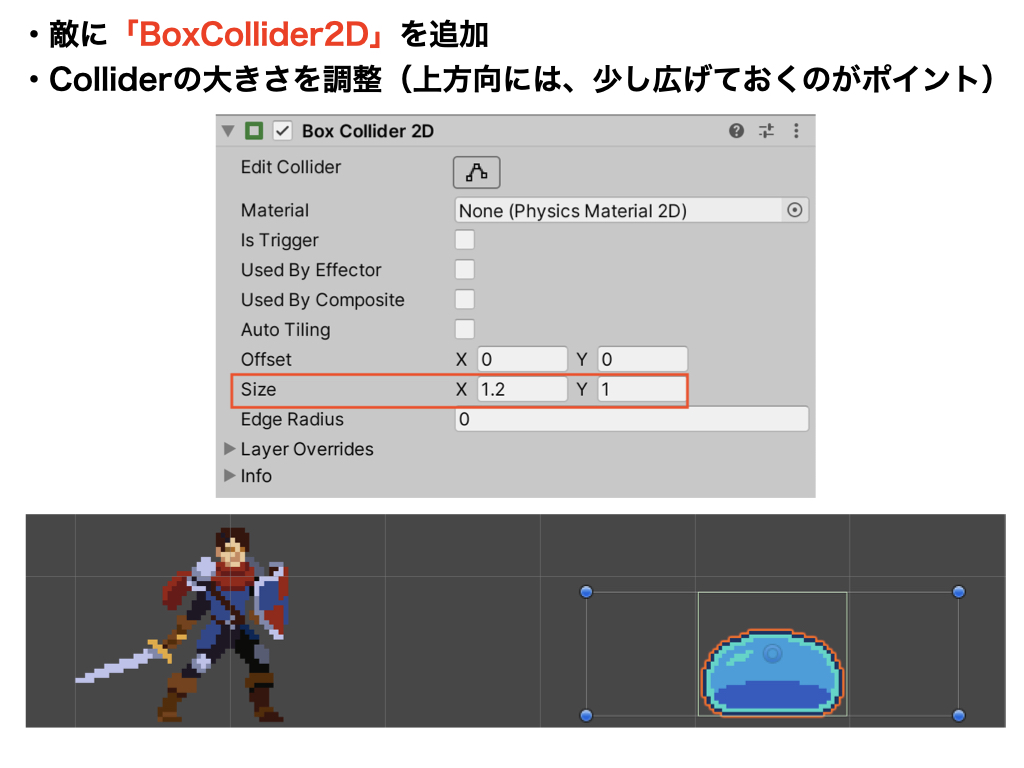
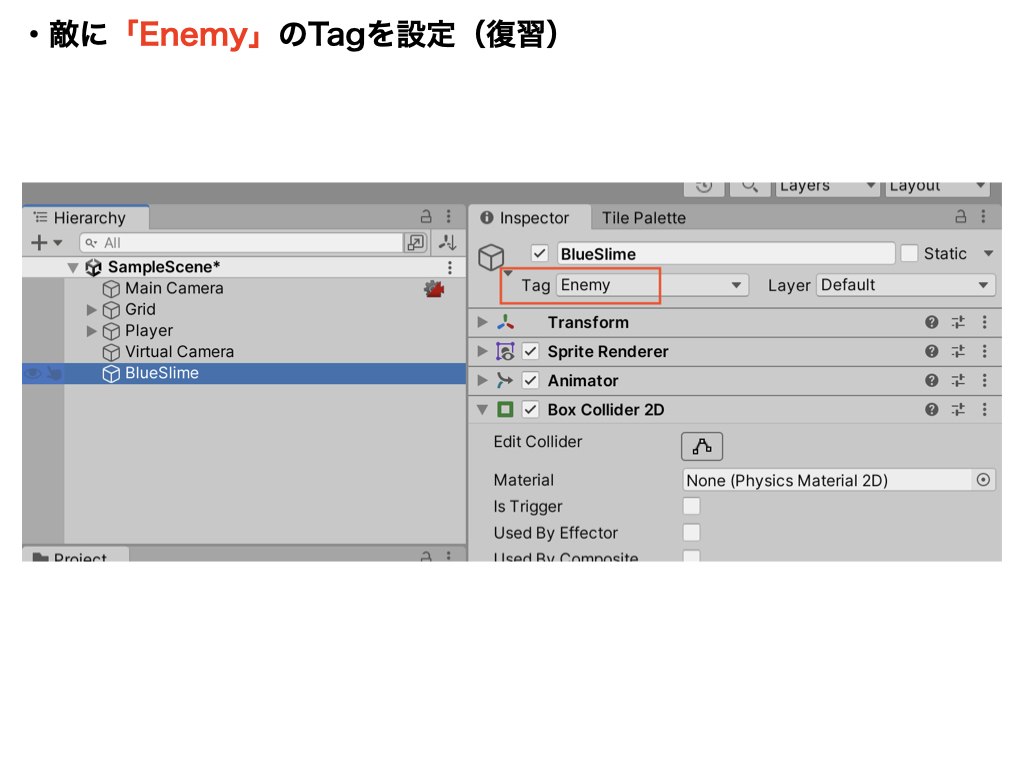
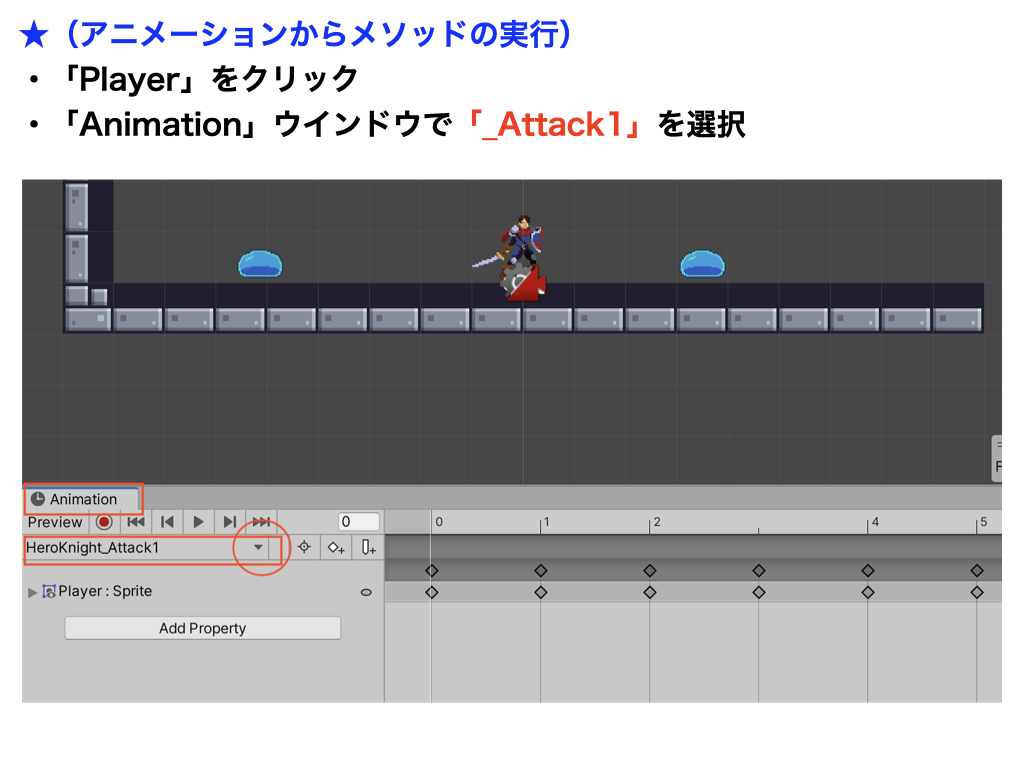
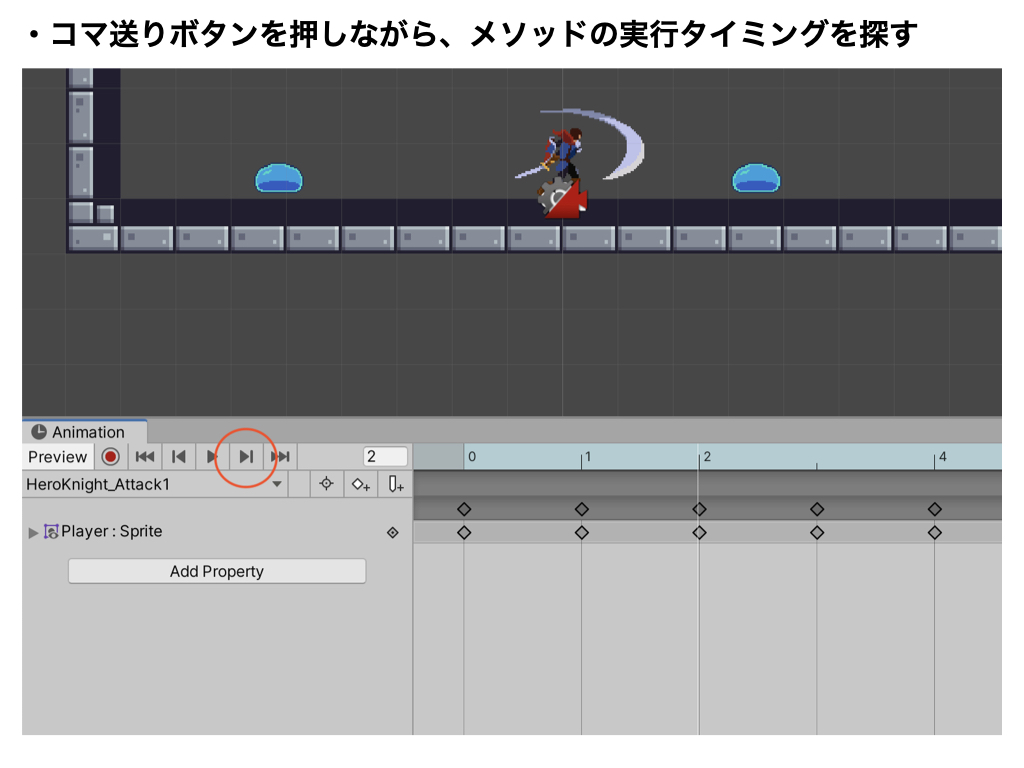
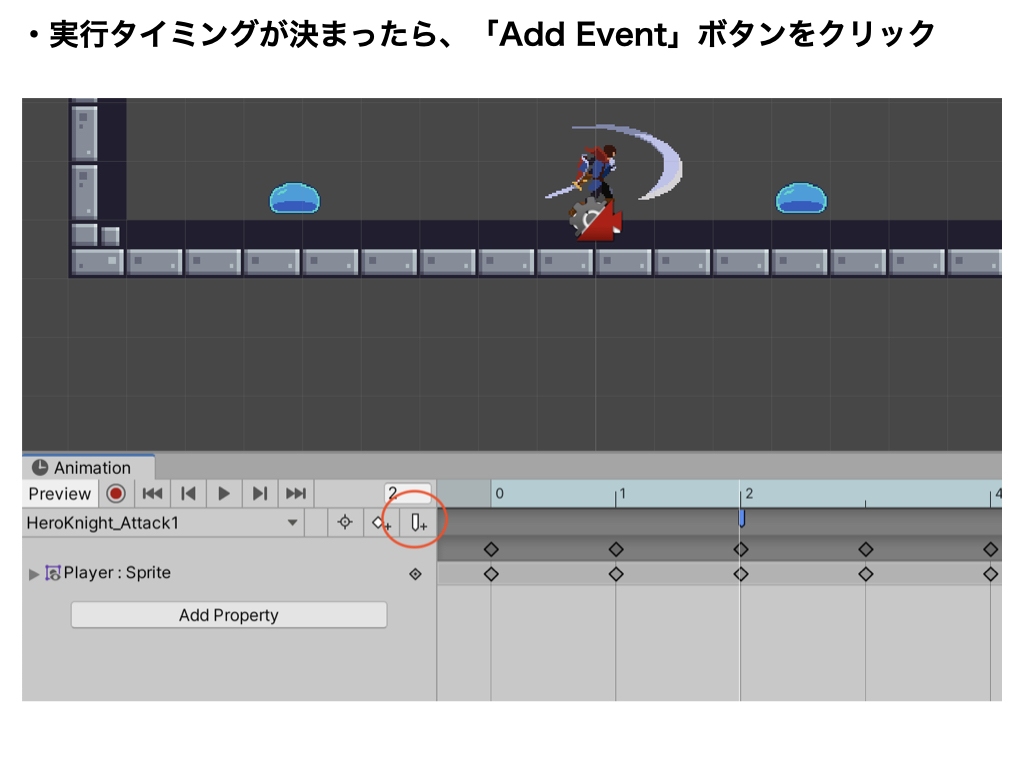
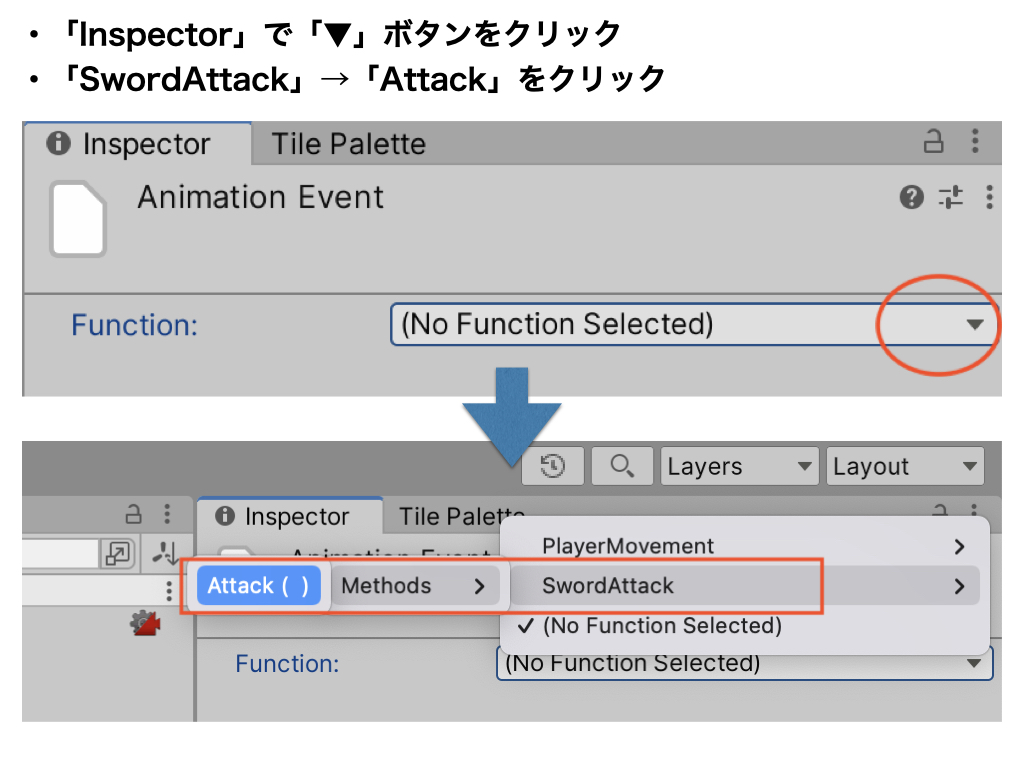
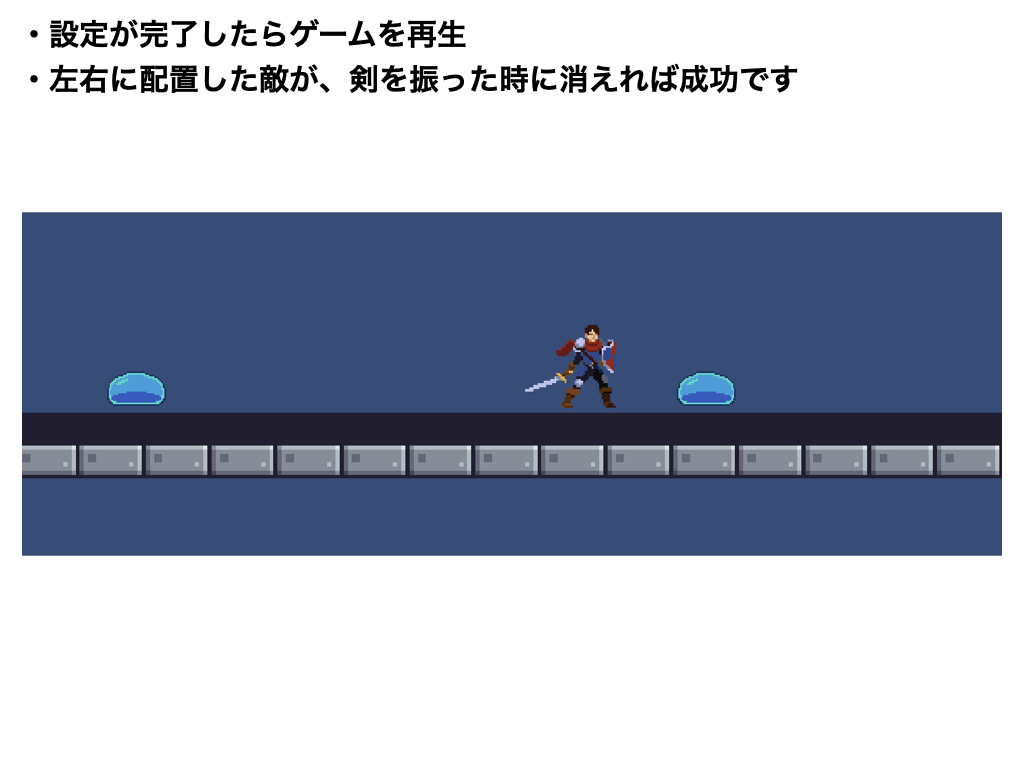
敵を倒す(通常攻撃)