扉のオープン&クローズ
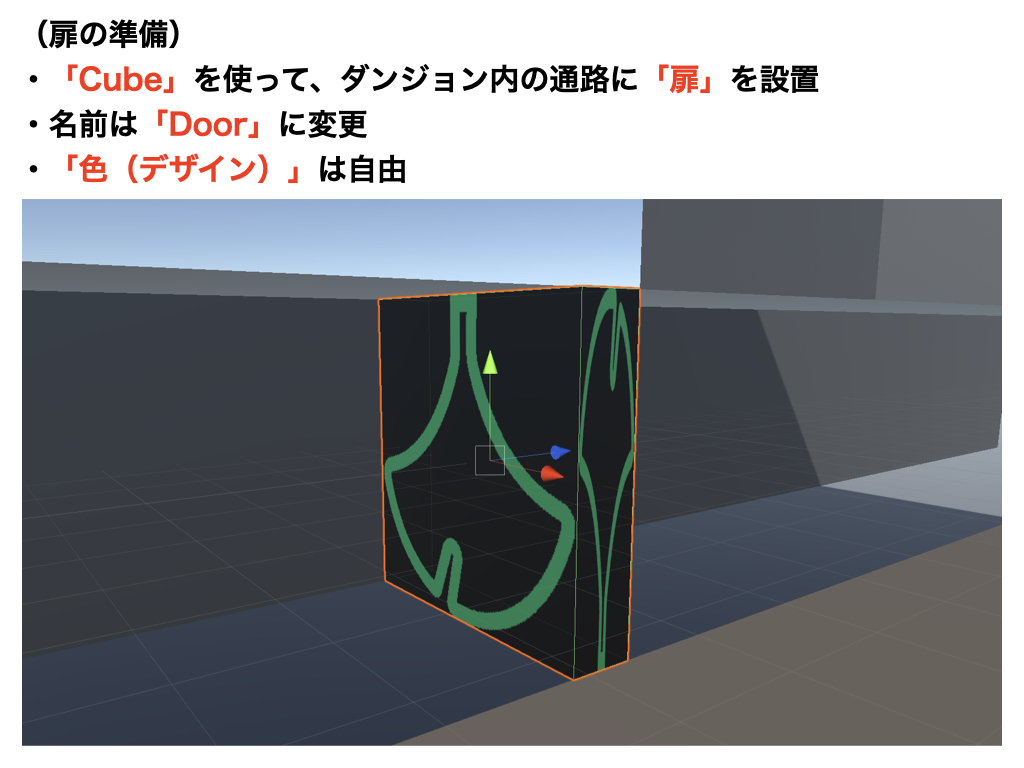
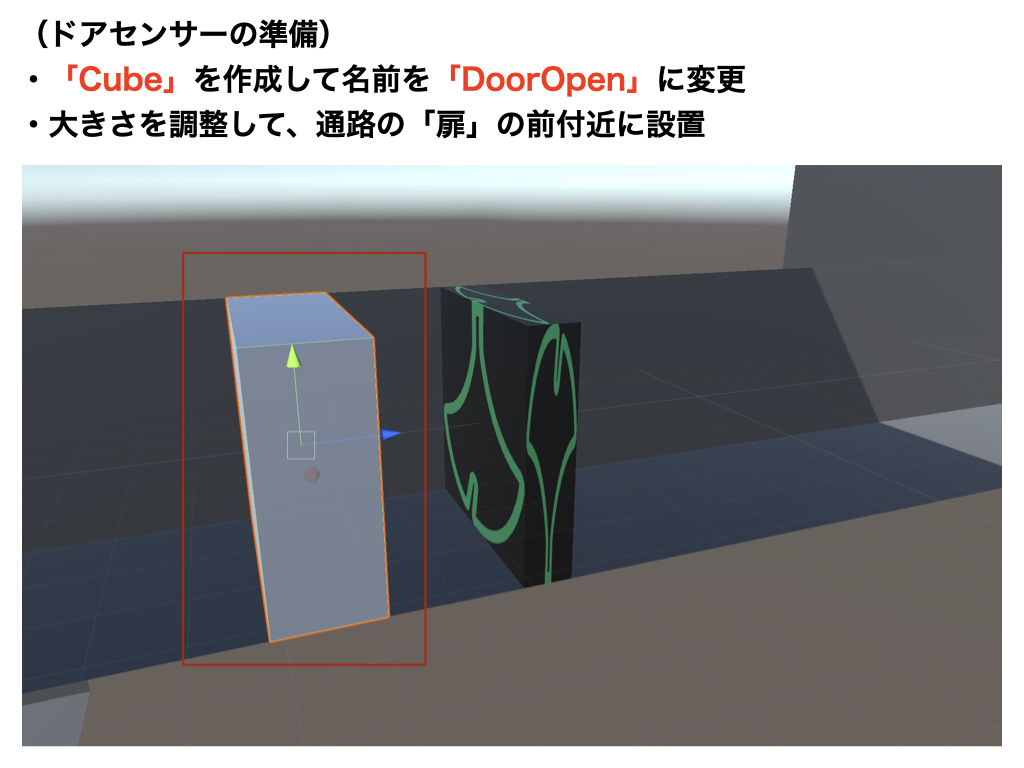
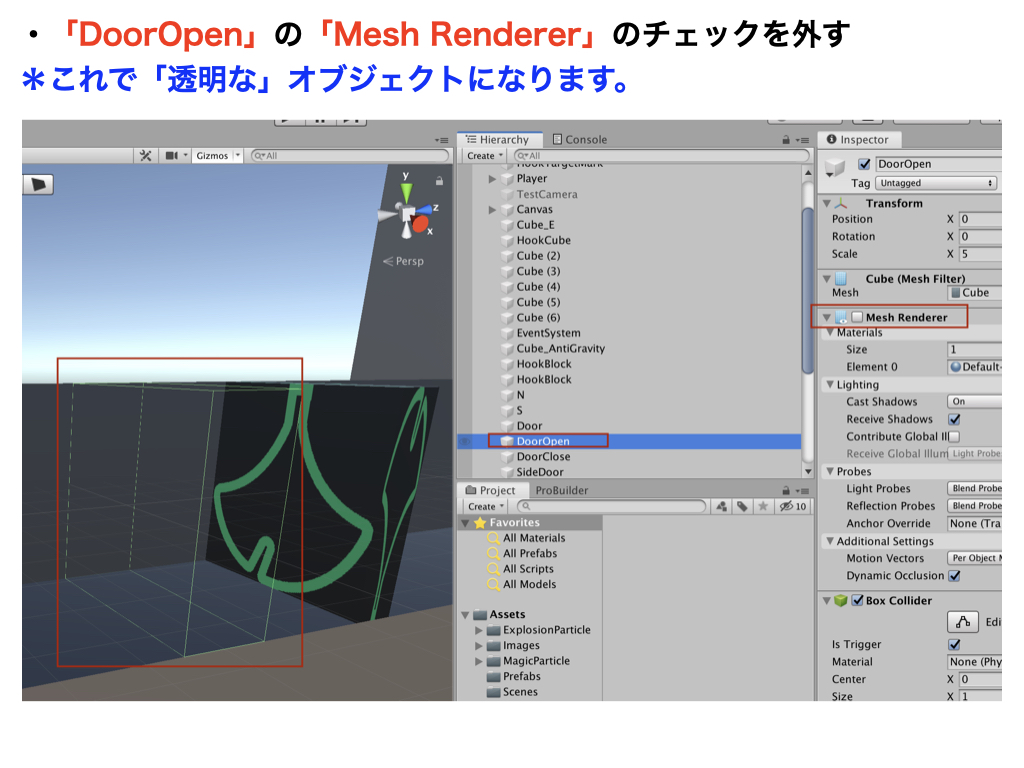
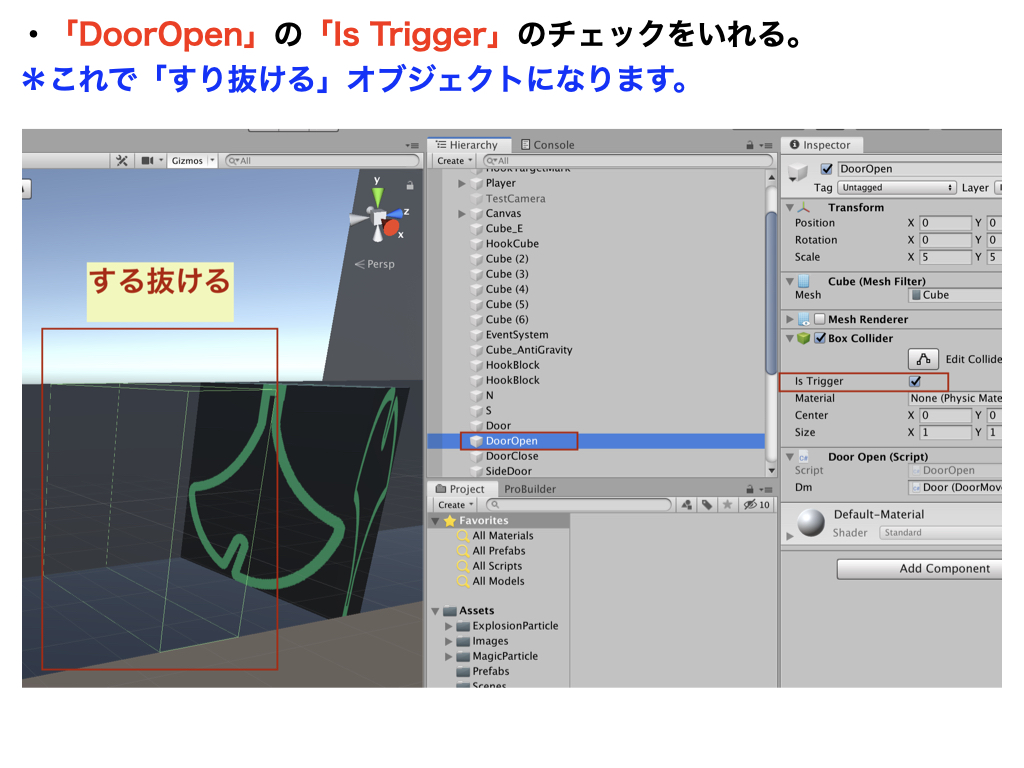
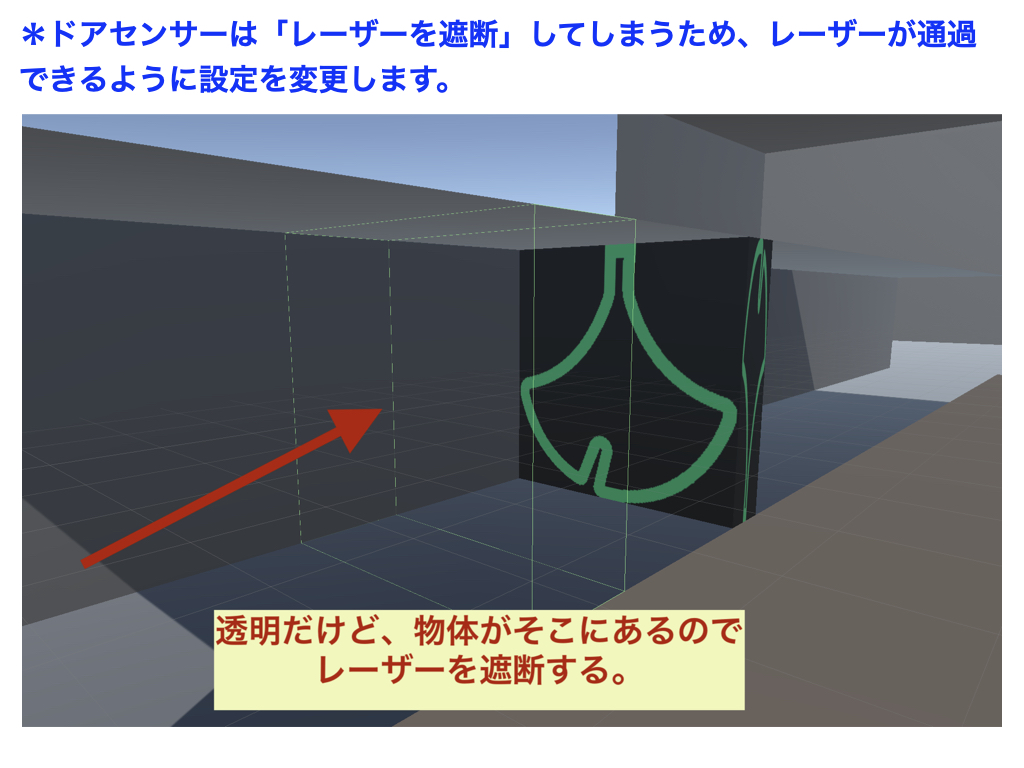
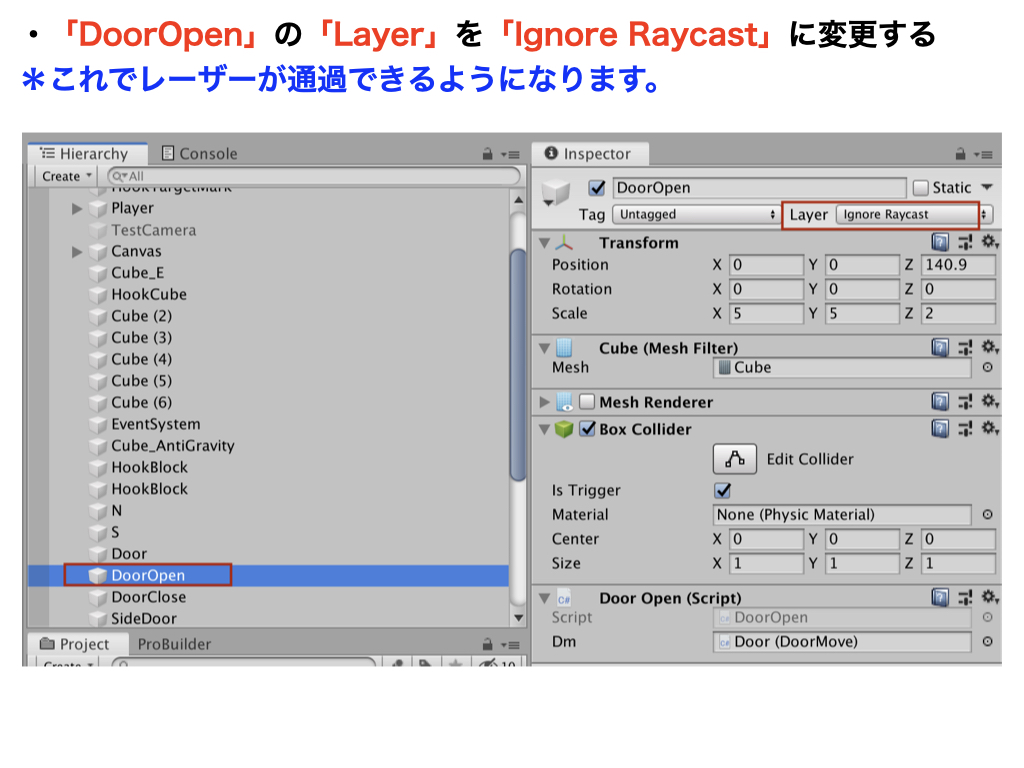
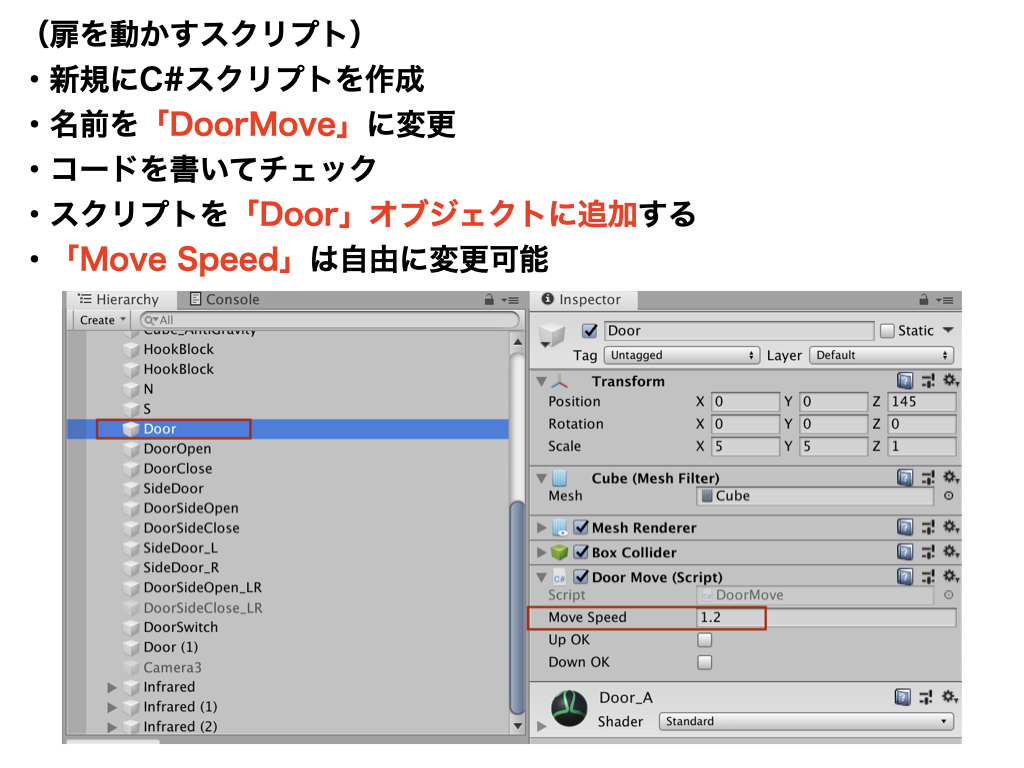
扉を上下に動かす
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// 扉を上下に動かす
public class DoorMove : MonoBehaviour
{
public float moveSpeed = 1.2f;
public bool upOK = false;
public bool downOK = false;
private void Update()
{
Vector3 pos = transform.position;
if (upOK)
{
// 「up」は「上」方向
transform.Translate(Vector3.up * Time.deltaTime * moveSpeed);
// 扉が移動できる範囲の設定(扉の高さがこの設定より大きくなったら止まる)
if (pos.y > 5.01f)
{
upOK = false;
}
}
if (downOK)
{
// 「down」は「下」方向
transform.Translate(Vector3.down * Time.deltaTime * moveSpeed);
// 扉が移動できる範囲の設定(扉の高さがこの設定より小さくなったら止まる)
if (pos.y < 0f)
{
downOK = false;
}
}
}
}
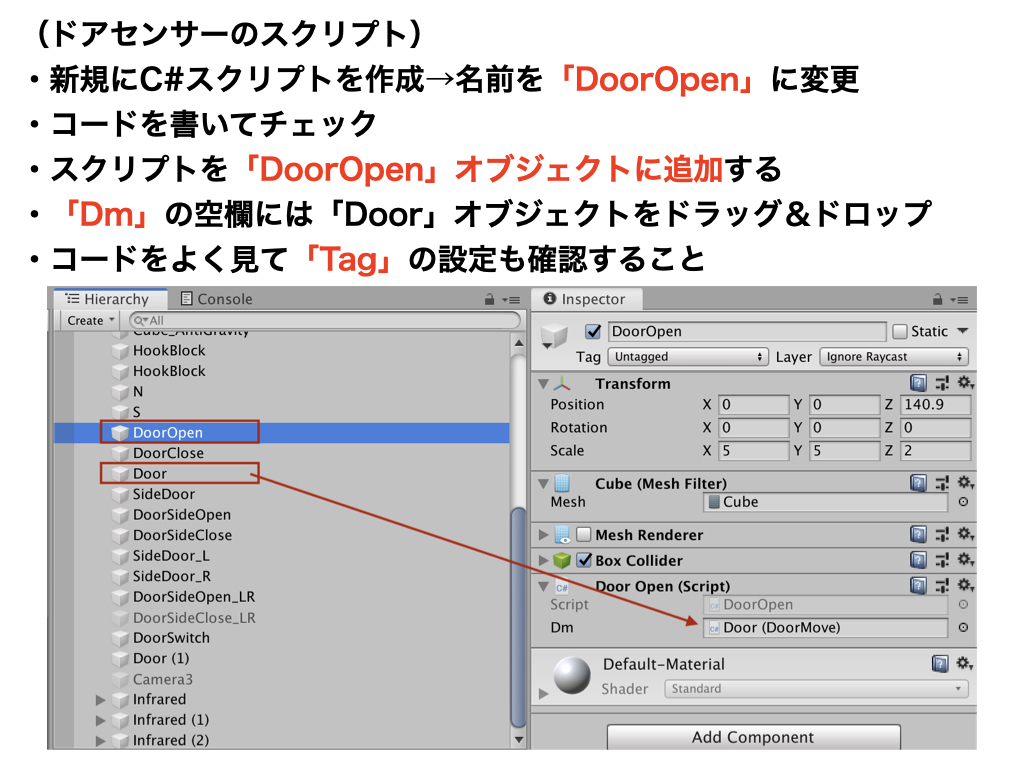
ドアセンサー(上に開く)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ドアセンサー(上に開く)
public class DoorOpen : MonoBehaviour
{
public DoorMove dm;
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Player"))
{
// ここの意味(仕組み)を考えてみよう!
dm.upOK = true;
dm.downOK = false;
}
}
}
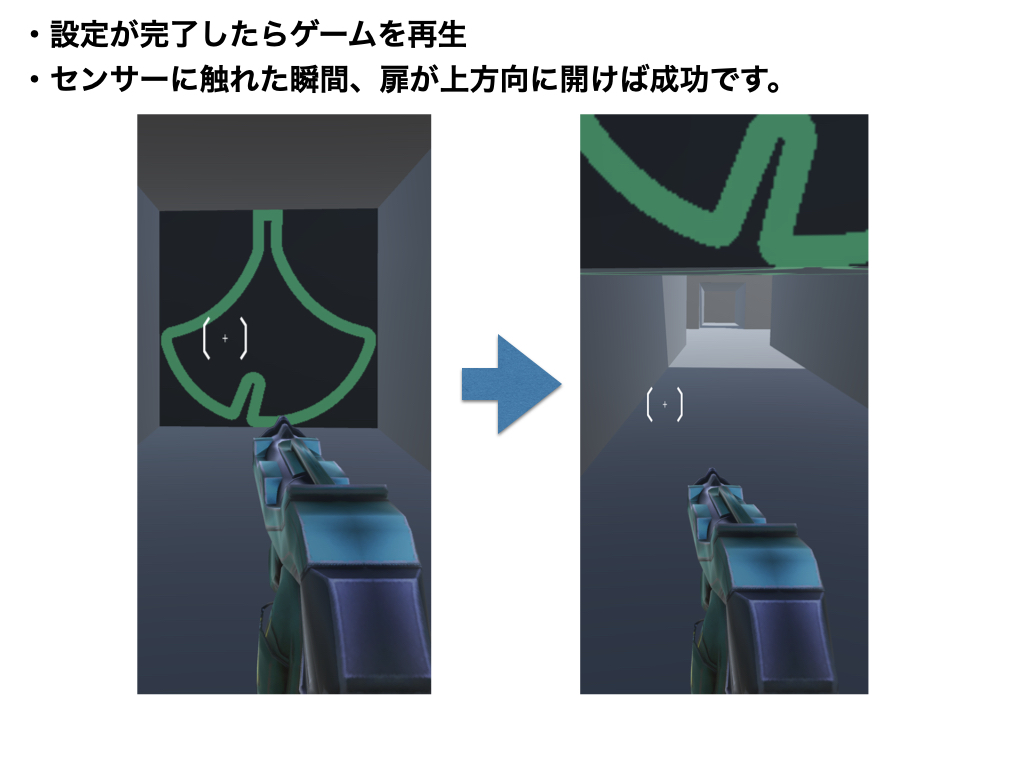
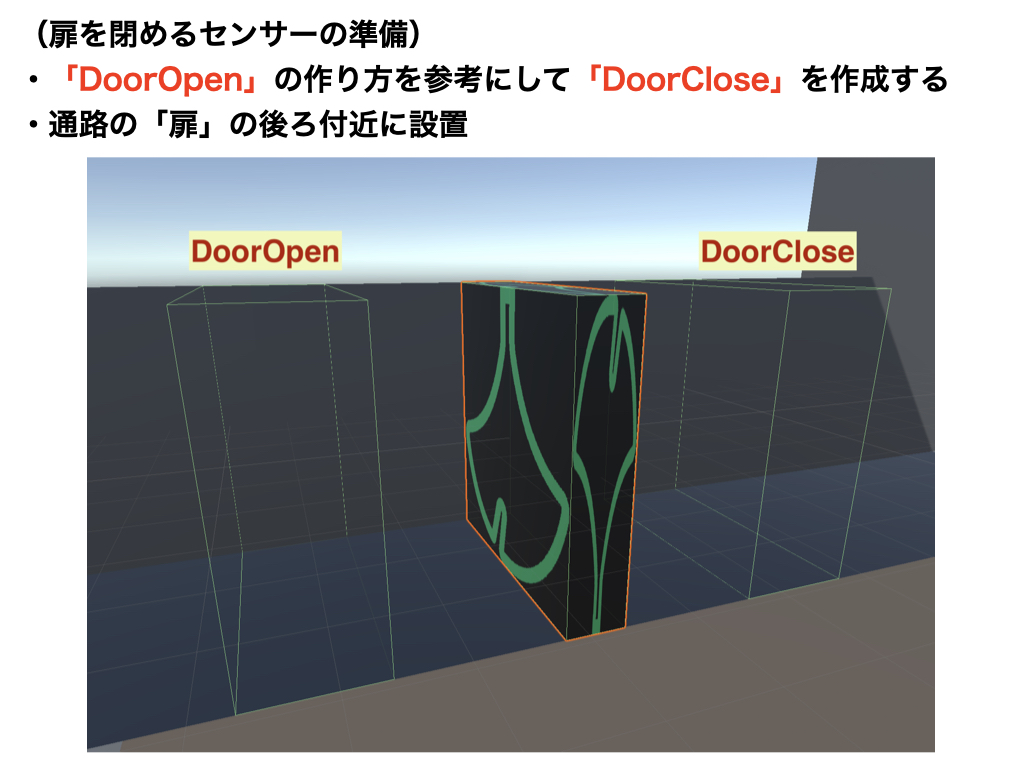
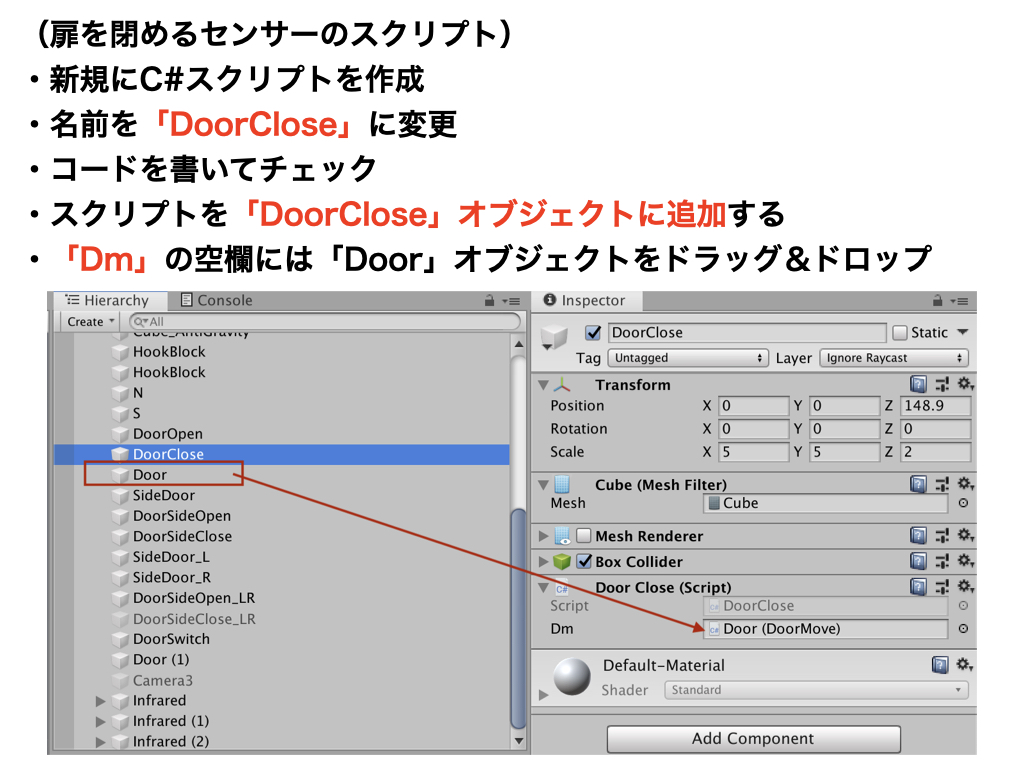
ドアセンサー(下に閉じる)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ドアセンサー(下に閉じる)
public class DoorClose : MonoBehaviour
{
public DoorMove dm;
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Player"))
{
// DoorOpenとどこが異なるかよく確認しよう!
dm.downOK = true;
dm.upOK = false;
}
}
}
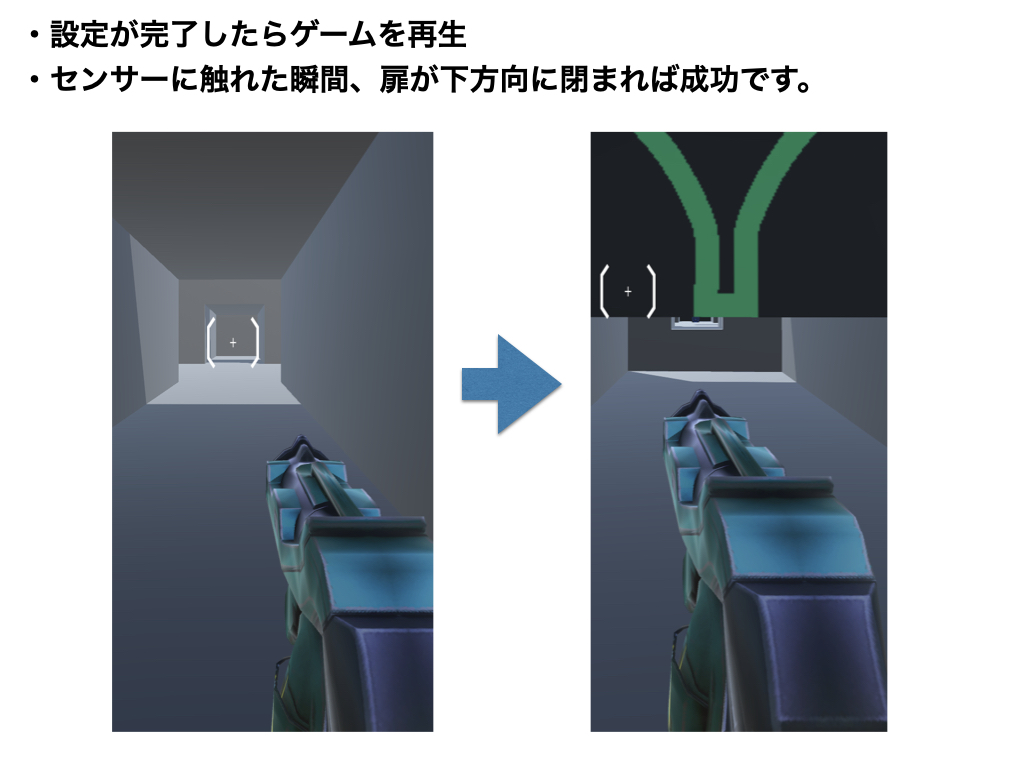
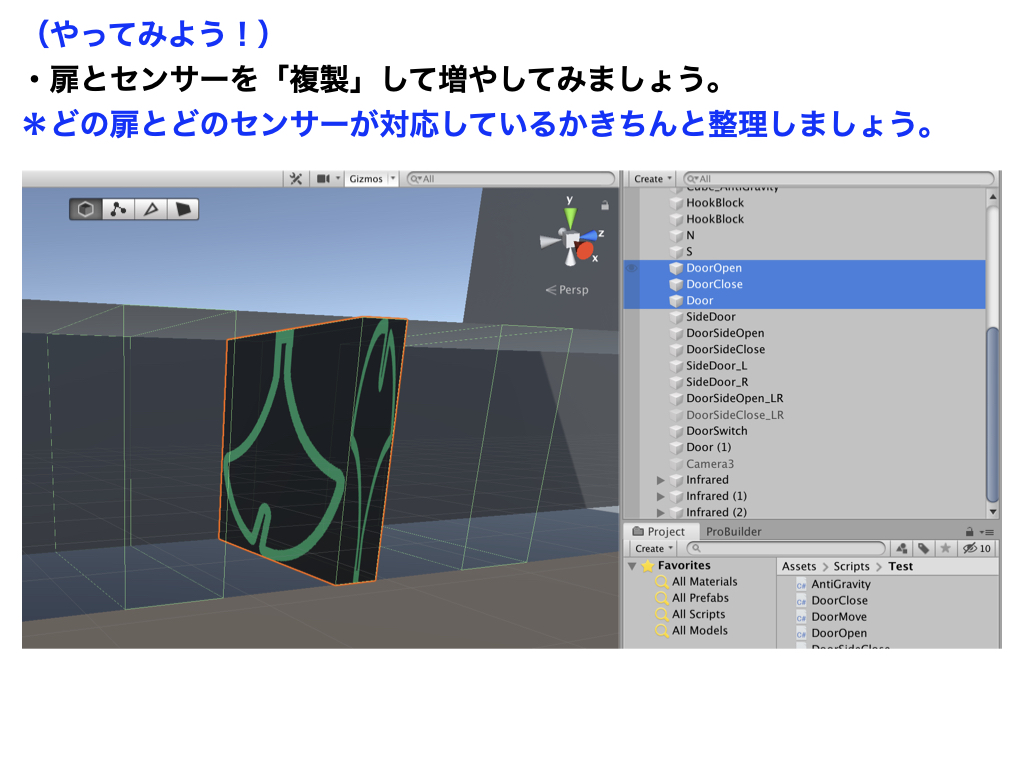
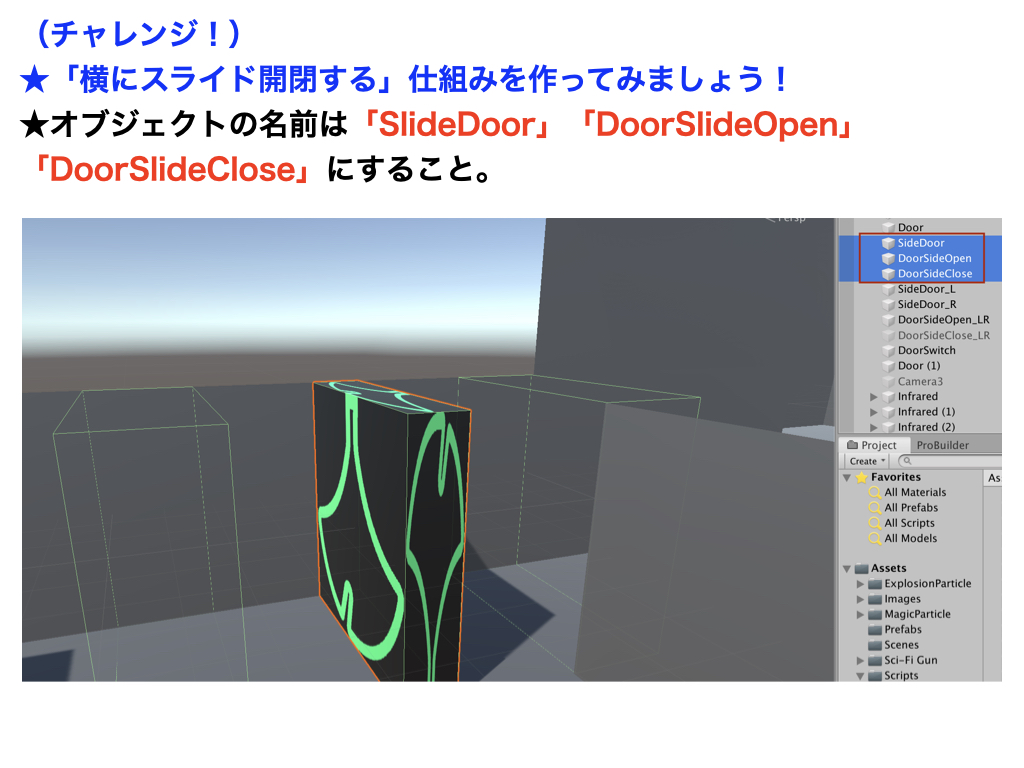
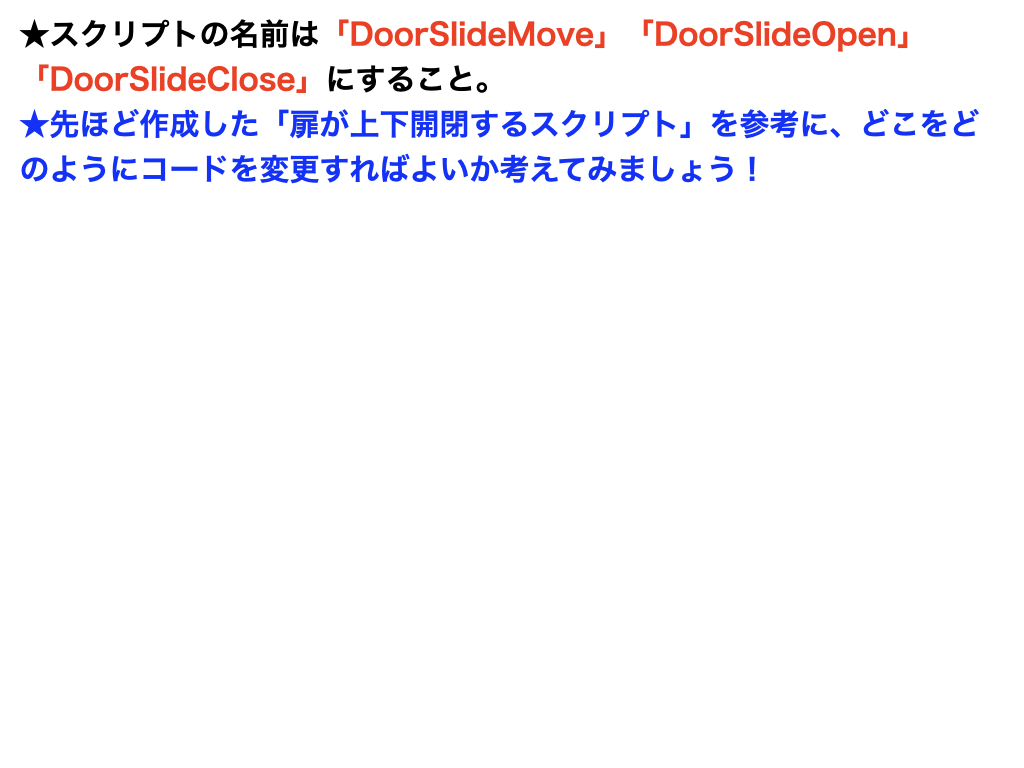
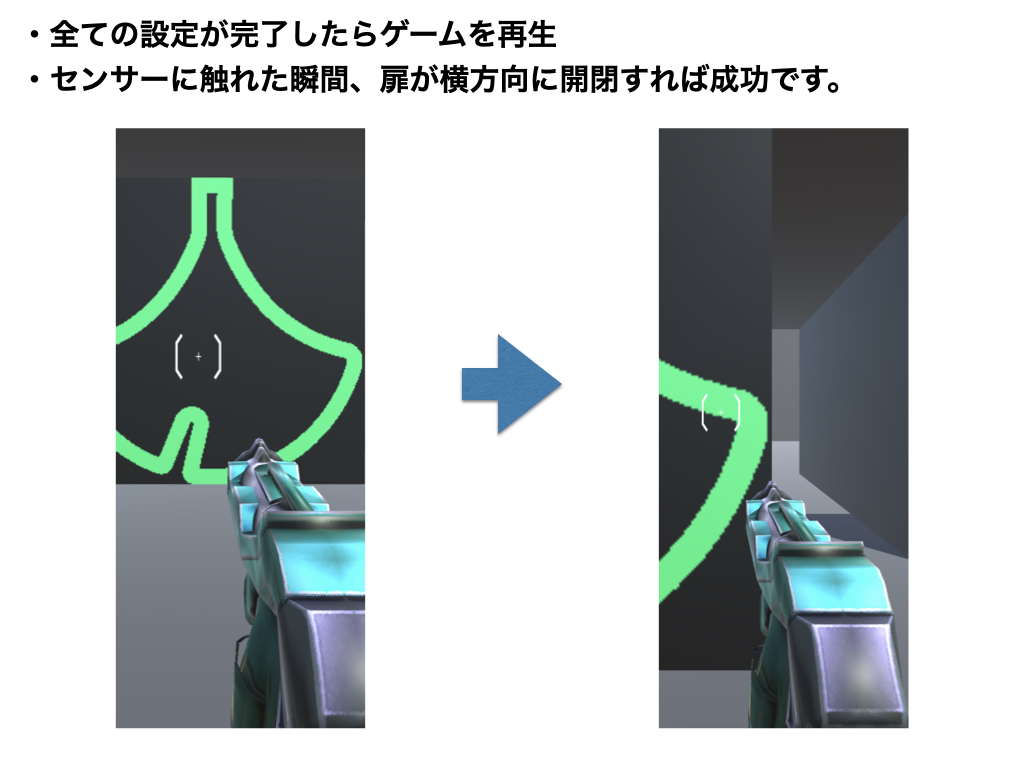
【2019版】X_Mission(基礎/全51回)
他のコースを見る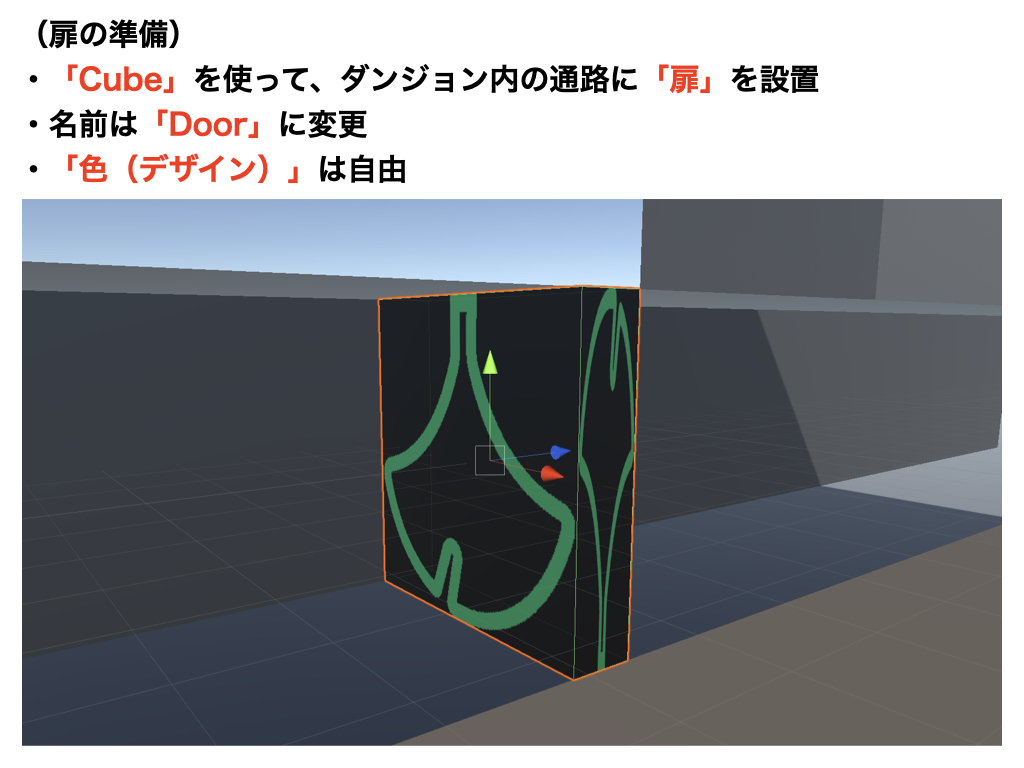
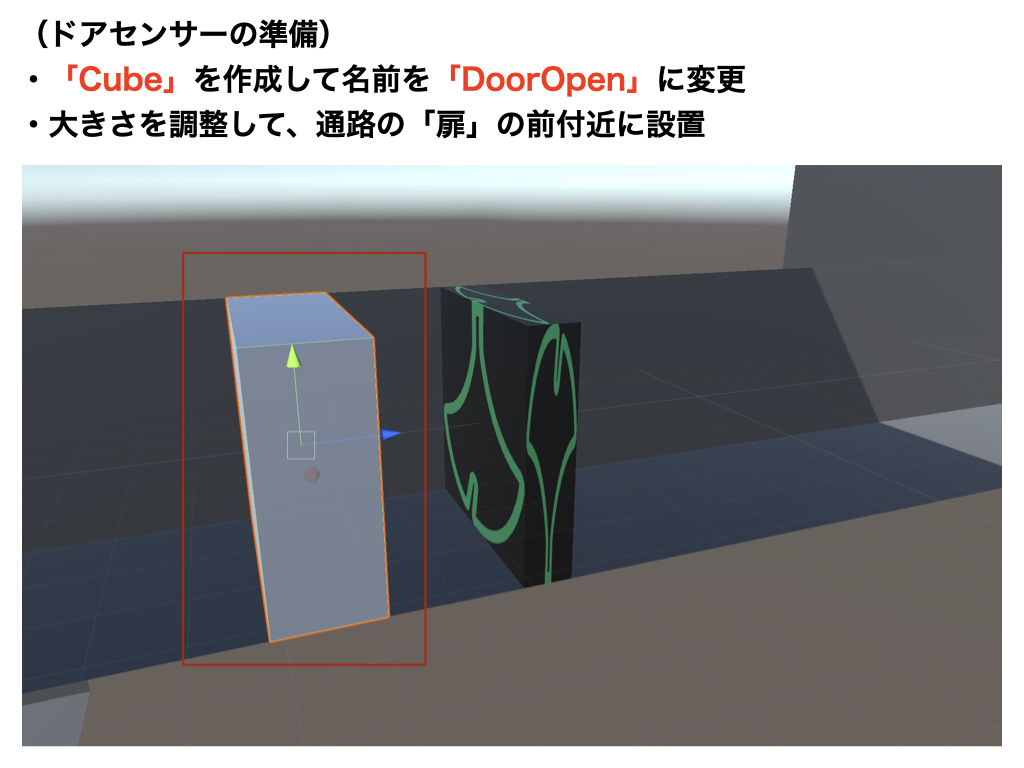
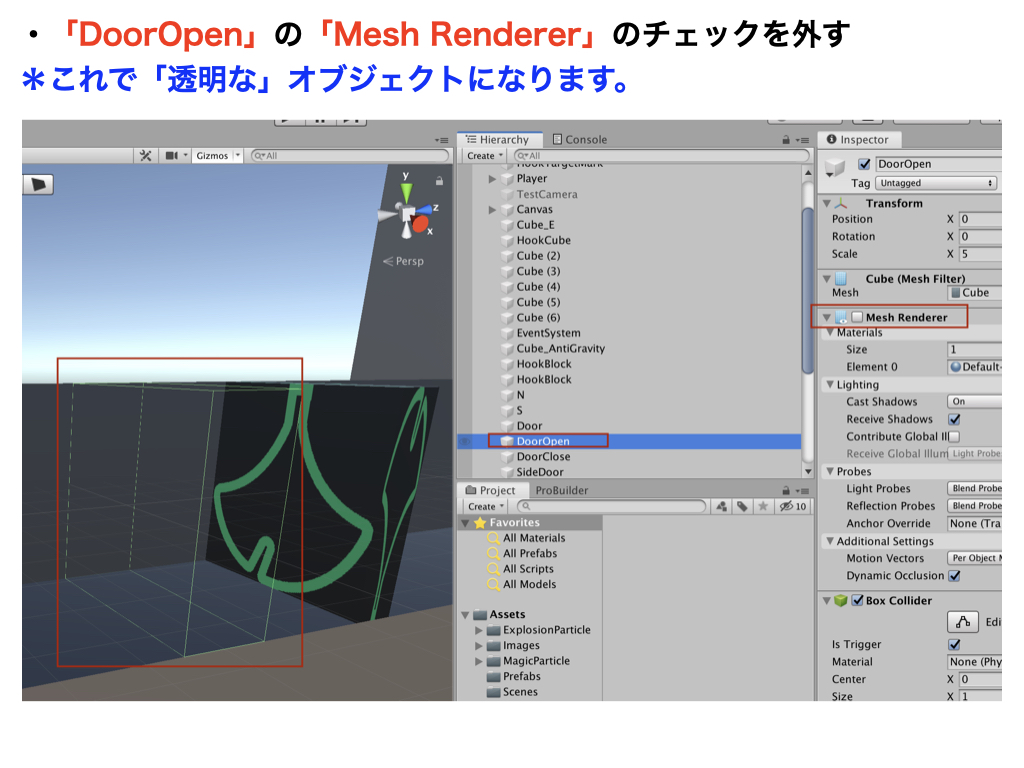
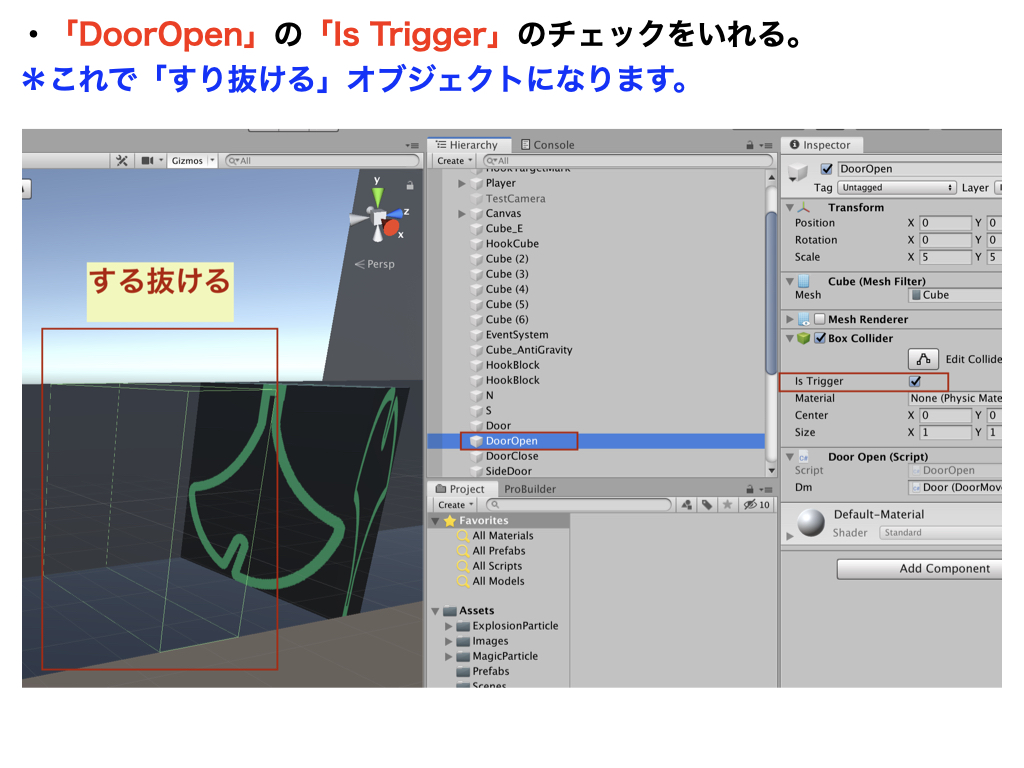
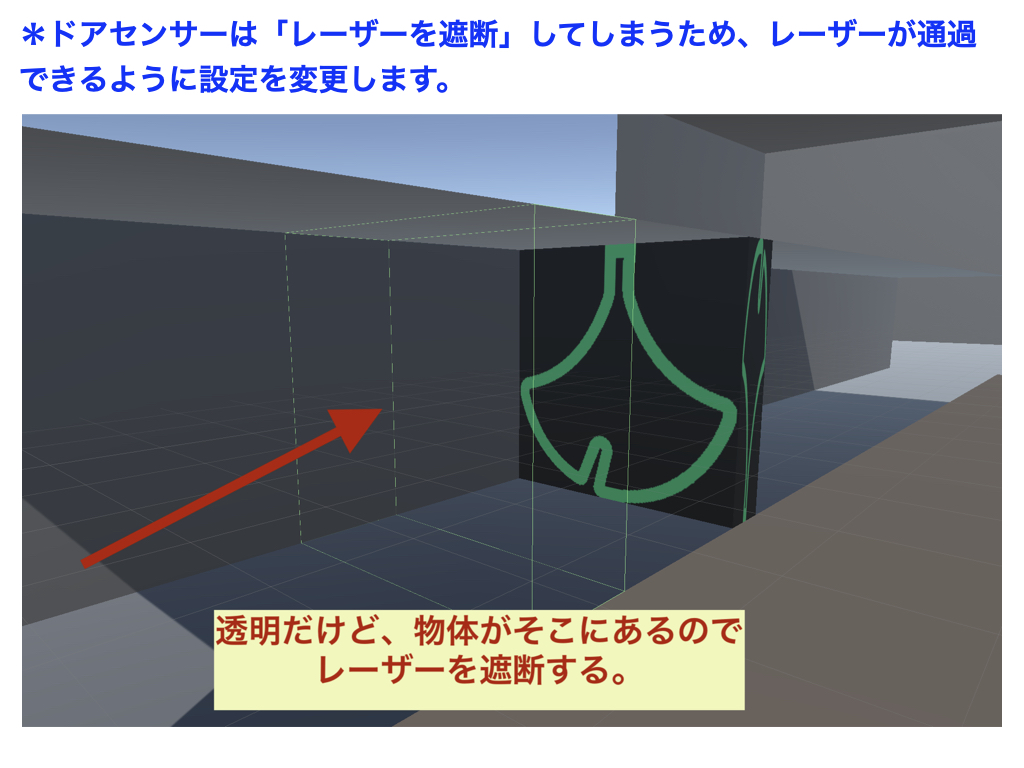
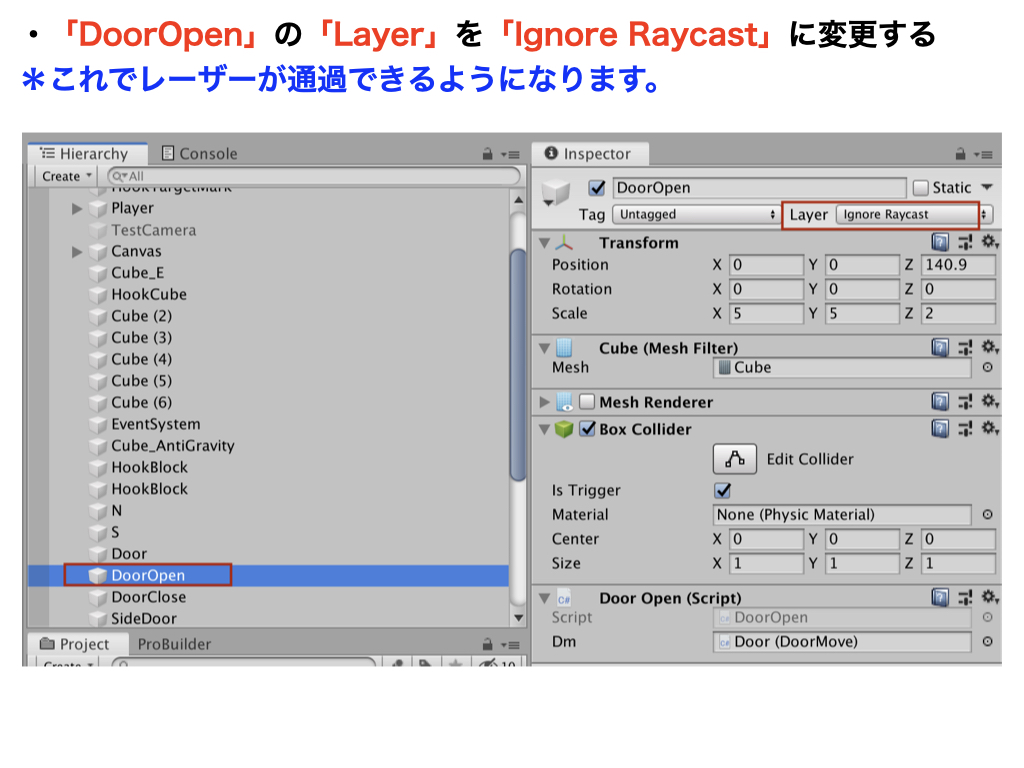
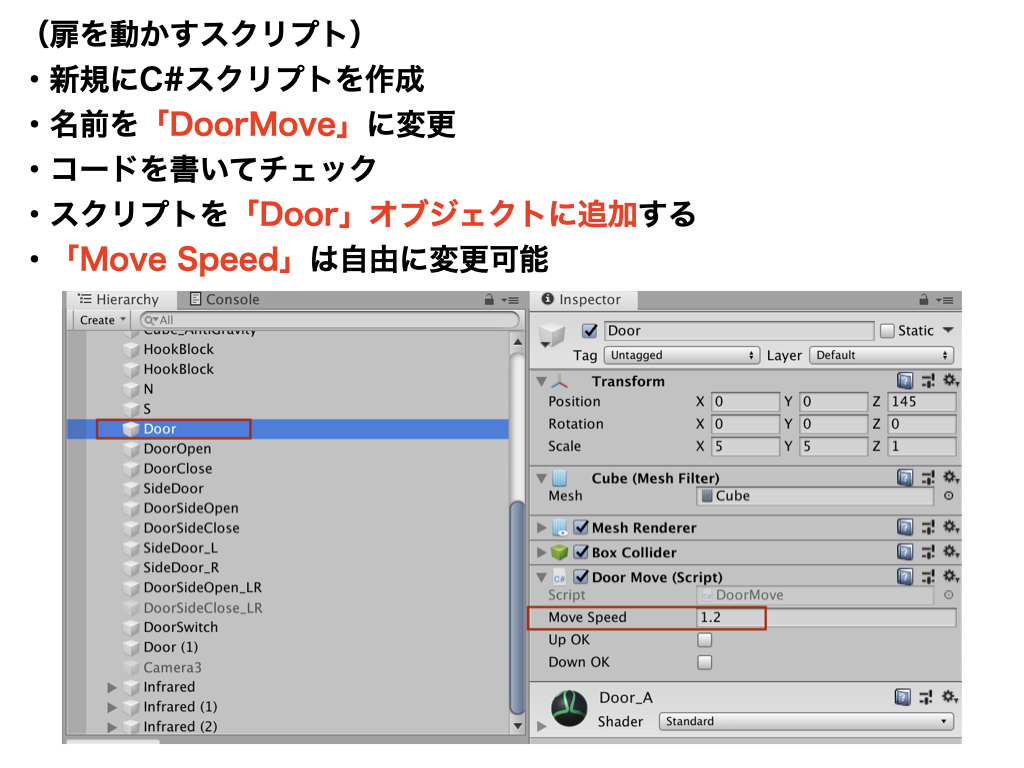
扉を上下に動かす
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// 扉を上下に動かす
public class DoorMove : MonoBehaviour
{
public float moveSpeed = 1.2f;
public bool upOK = false;
public bool downOK = false;
private void Update()
{
Vector3 pos = transform.position;
if (upOK)
{
// 「up」は「上」方向
transform.Translate(Vector3.up * Time.deltaTime * moveSpeed);
// 扉が移動できる範囲の設定(扉の高さがこの設定より大きくなったら止まる)
if (pos.y > 5.01f)
{
upOK = false;
}
}
if (downOK)
{
// 「down」は「下」方向
transform.Translate(Vector3.down * Time.deltaTime * moveSpeed);
// 扉が移動できる範囲の設定(扉の高さがこの設定より小さくなったら止まる)
if (pos.y < 0f)
{
downOK = false;
}
}
}
}
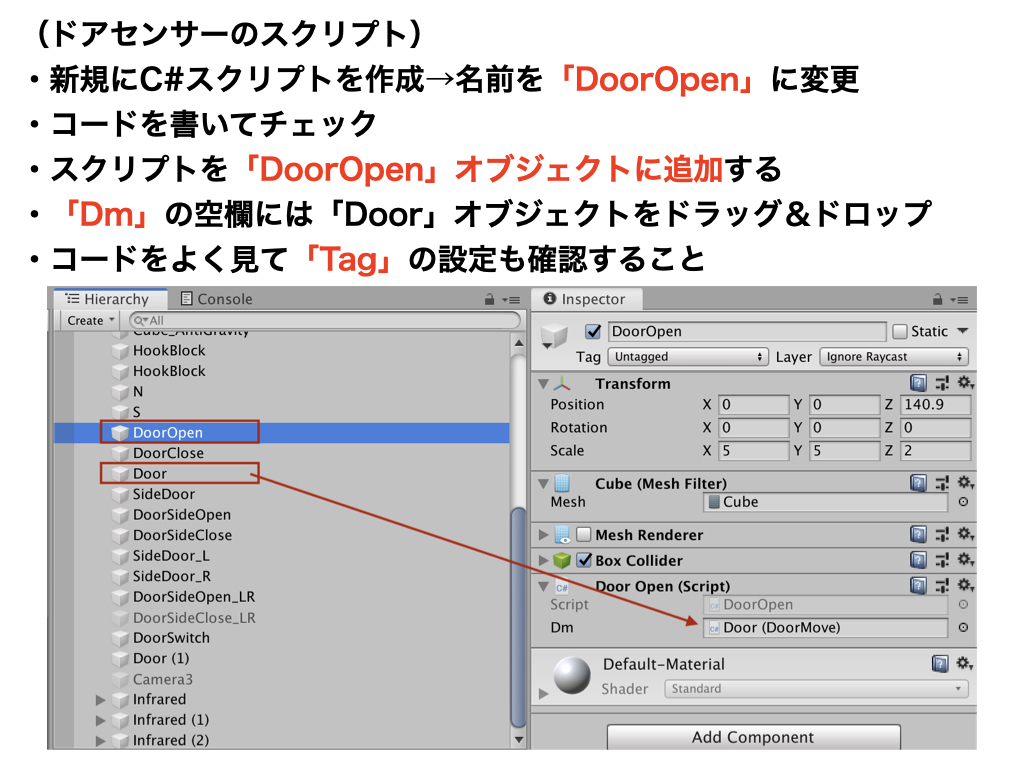
ドアセンサー(上に開く)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ドアセンサー(上に開く)
public class DoorOpen : MonoBehaviour
{
public DoorMove dm;
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Player"))
{
// ここの意味(仕組み)を考えてみよう!
dm.upOK = true;
dm.downOK = false;
}
}
}
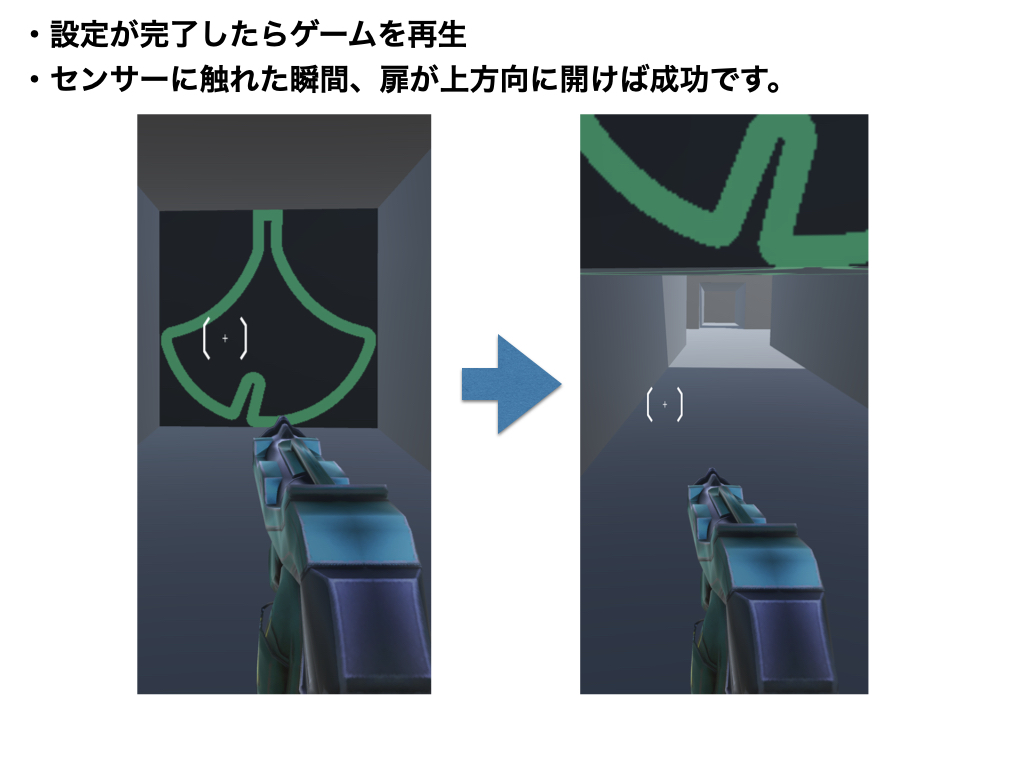
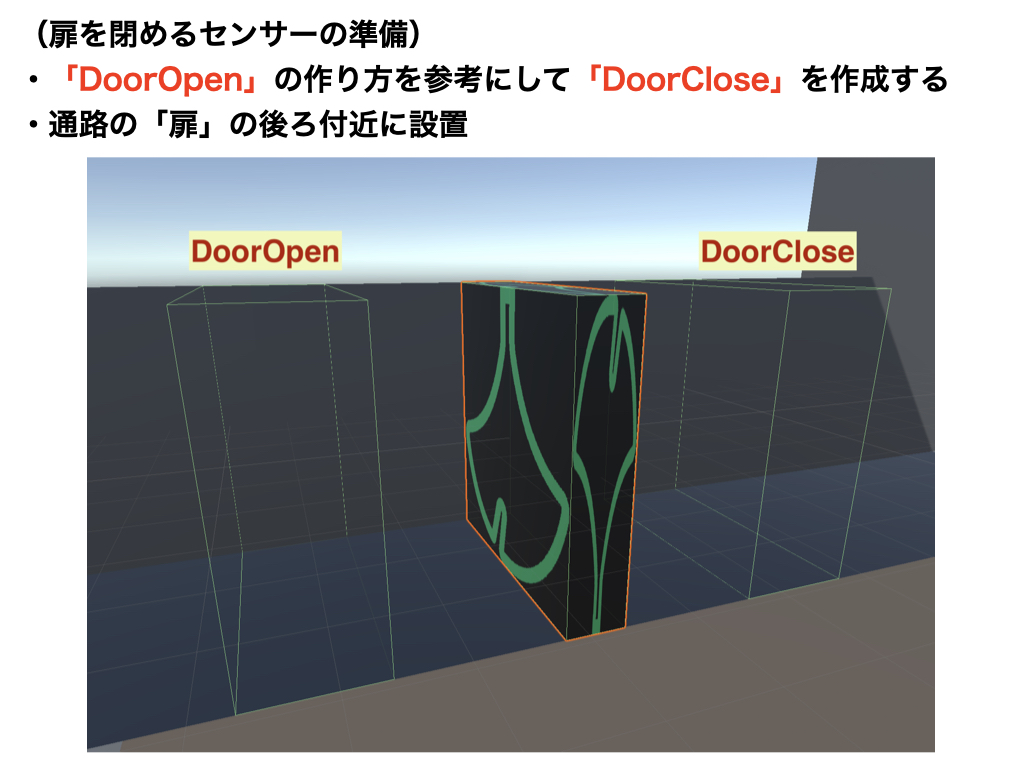
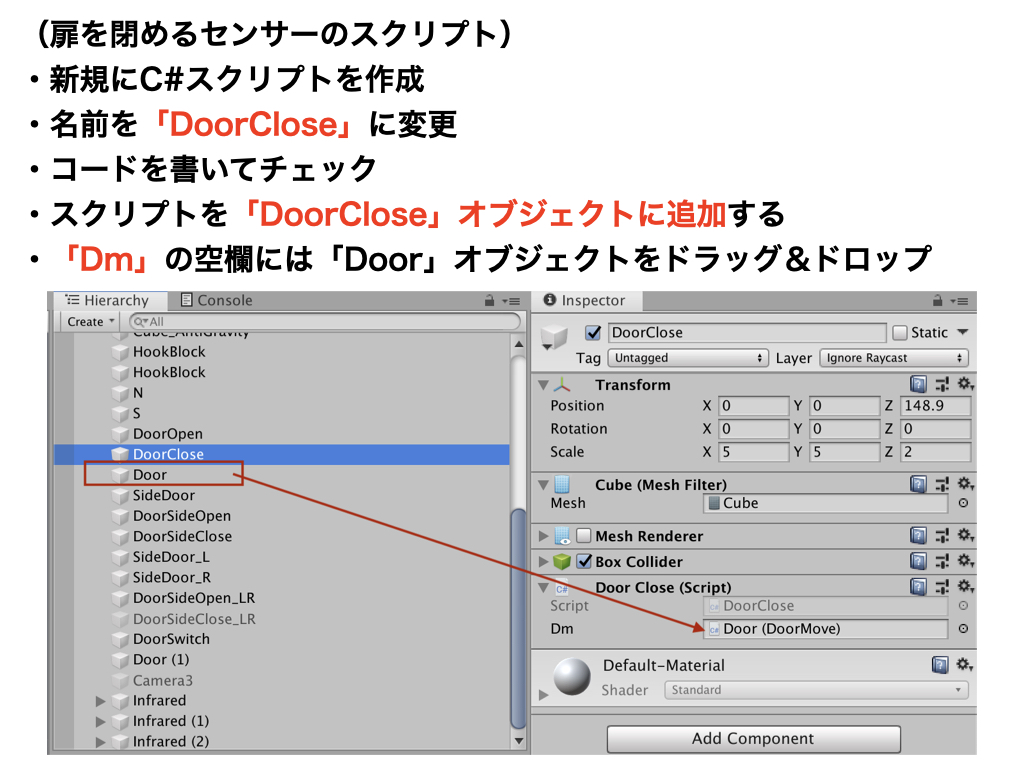
ドアセンサー(下に閉じる)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ドアセンサー(下に閉じる)
public class DoorClose : MonoBehaviour
{
public DoorMove dm;
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Player"))
{
// DoorOpenとどこが異なるかよく確認しよう!
dm.downOK = true;
dm.upOK = false;
}
}
}
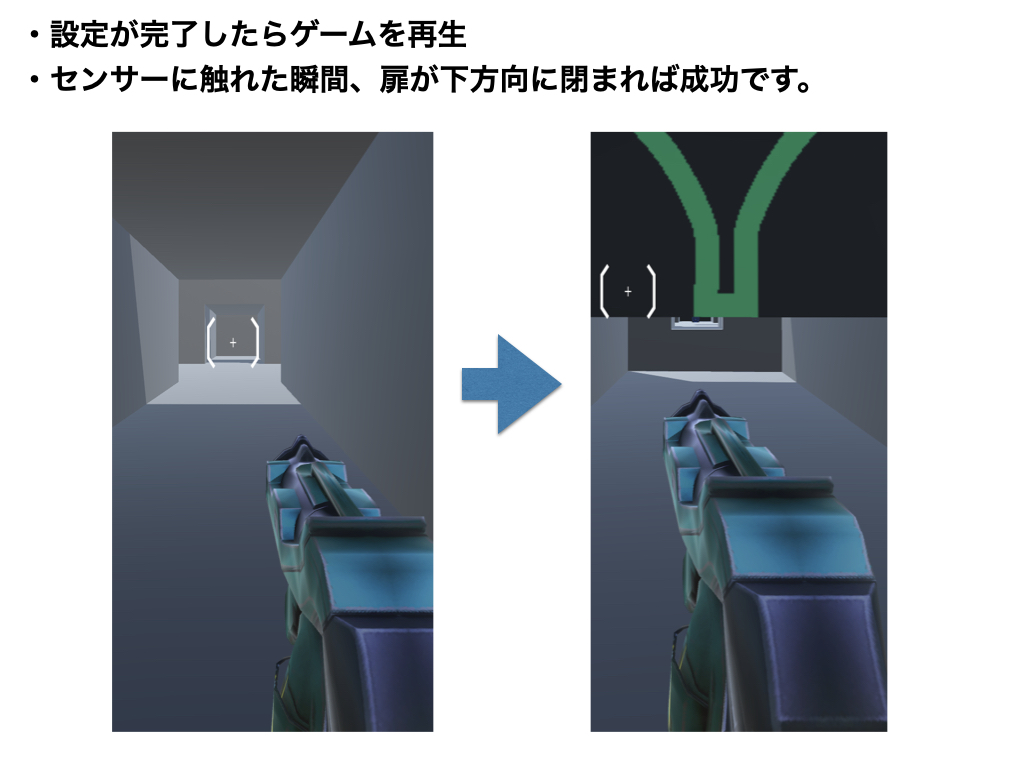
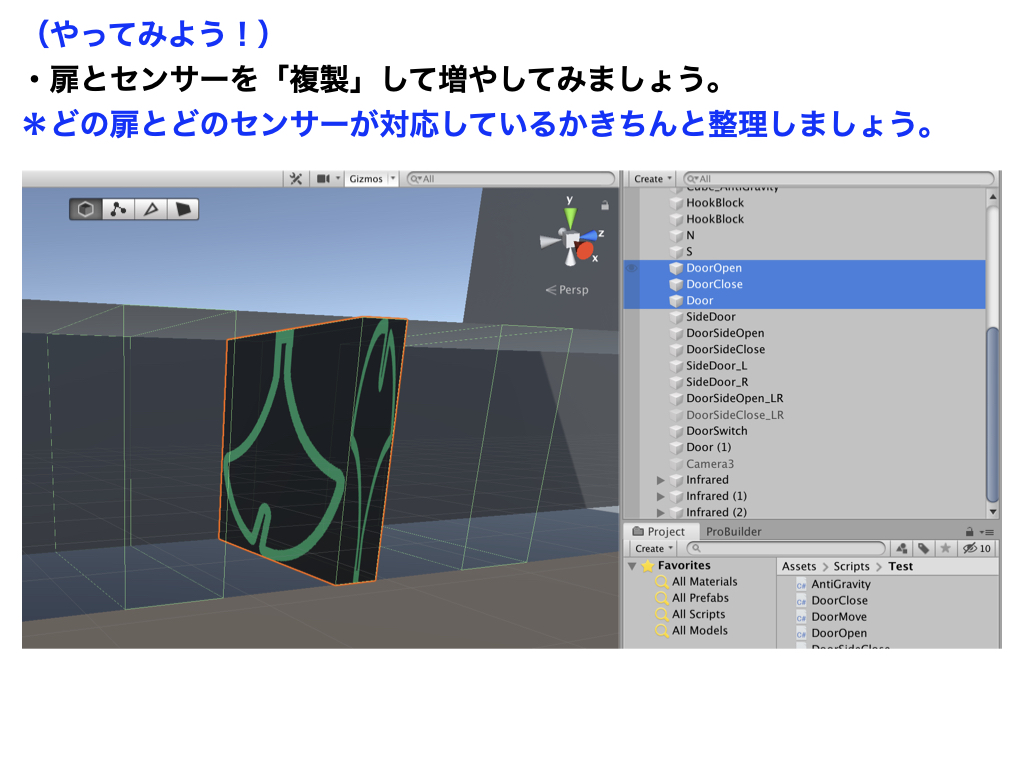
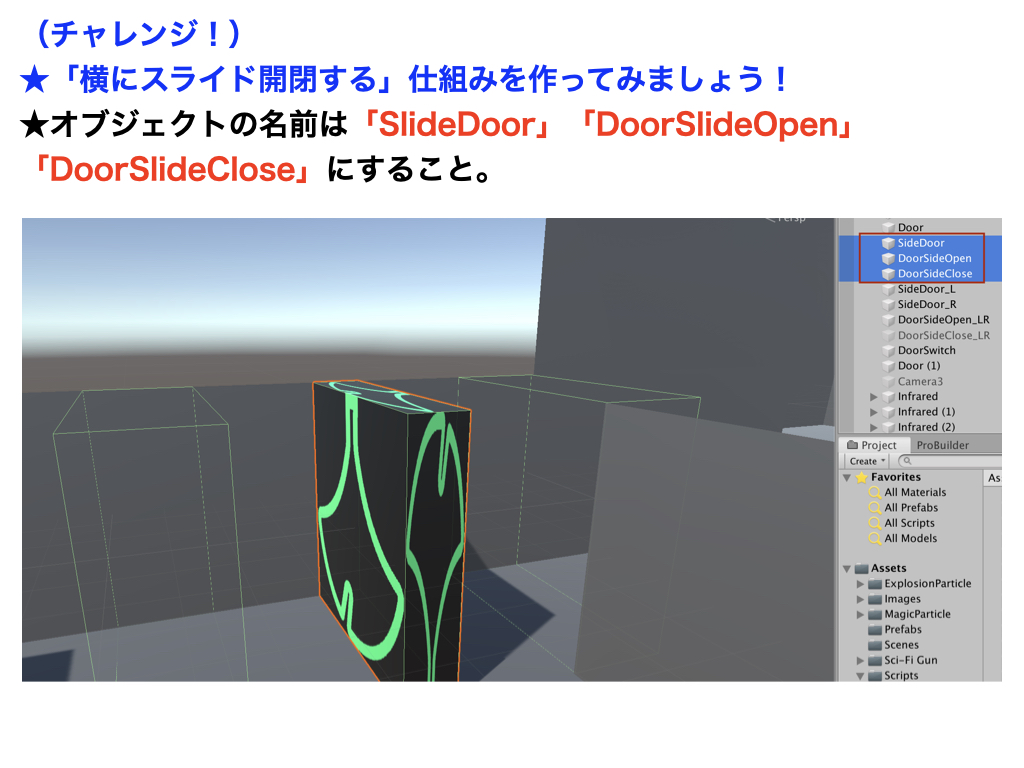
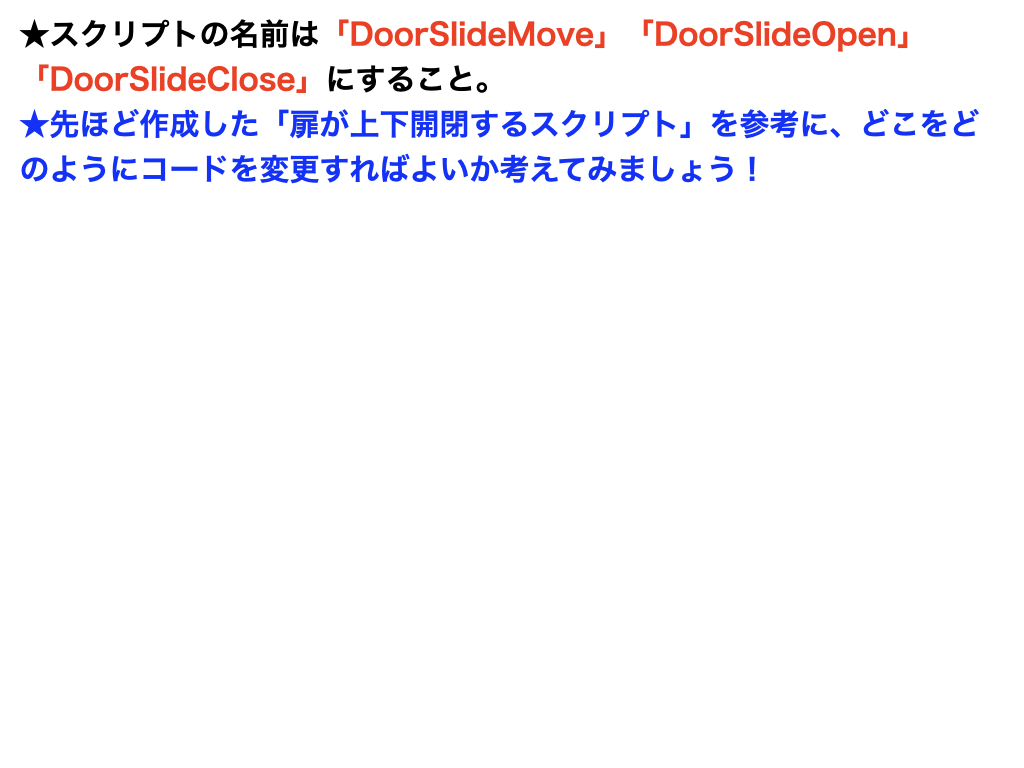
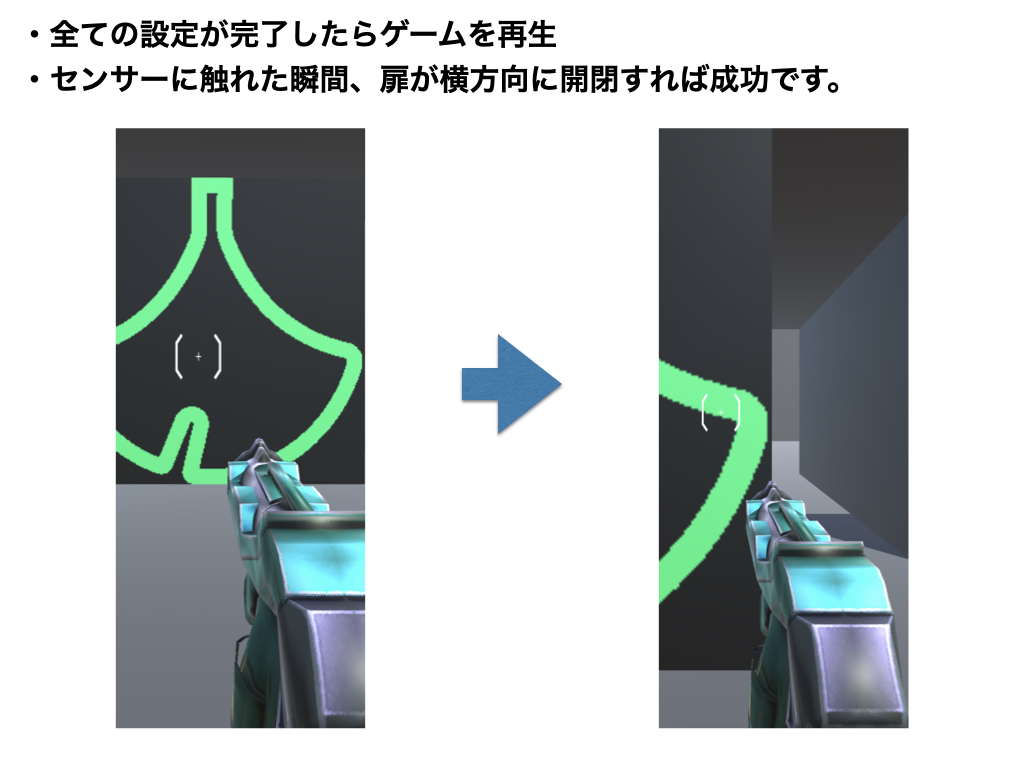
扉のオープン&クローズ