プレーヤーをジャンプさせる
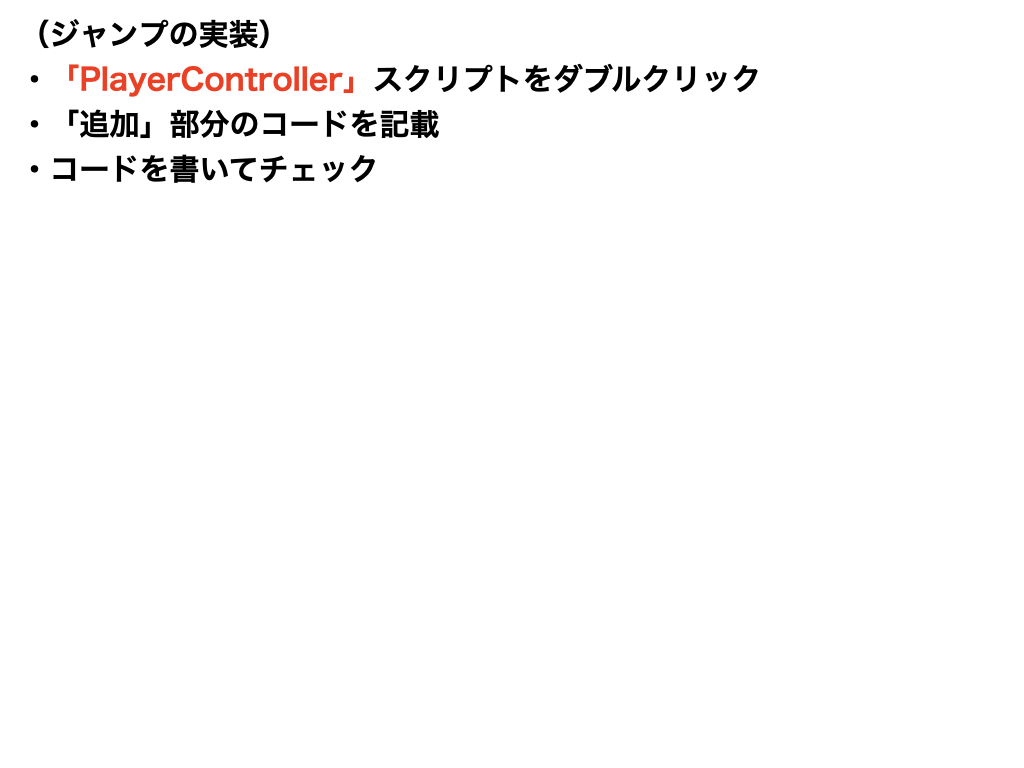
ジャンプ機能の実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[RequireComponent(typeof(CharacterController))]
[RequireComponent(typeof(AudioSource))]
public class PlayerController : MonoBehaviour
{
public float walkSpeed;
public float runSpeed;
private Vector3 movement;
private CharacterController characterController;
private AudioSource audioSource;
public GameObject FPSCamera;
private Vector3 moveDir = Vector3.zero;
// ★追加(ジャンプ)
private float gravity = 9.8f;
public float jumpPower;
public AudioClip jumpSound;
private void Start()
{
characterController = GetComponent<CharacterController>();
audioSource = GetComponent<AudioSource>();
}
private void Update()
{
PlayerMove();
}
void PlayerMove()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
movement = new Vector3(moveH, 0, moveV);
Vector3 desiredMove = FPSCamera.transform.forward * movement.z + FPSCamera.transform.right * movement.x;
moveDir.x = desiredMove.x * 5f;
moveDir.z = desiredMove.z * 5f;
if (Input.GetKey(KeyCode.LeftShift))
{
characterController.Move(moveDir * Time.deltaTime * runSpeed);
}
else
{
characterController.Move(moveDir * Time.deltaTime * walkSpeed);
}
// ★追加(ジャンプ)
if(characterController.isGrounded)
{
if(Input.GetKeyDown(KeyCode.Space))
{
moveDir.y = jumpPower;
audioSource.clip = jumpSound;
audioSource.Play();
}
}
else
{
// 擬似重力の実装
moveDir.y -= gravity * Time.deltaTime;
}
}
}
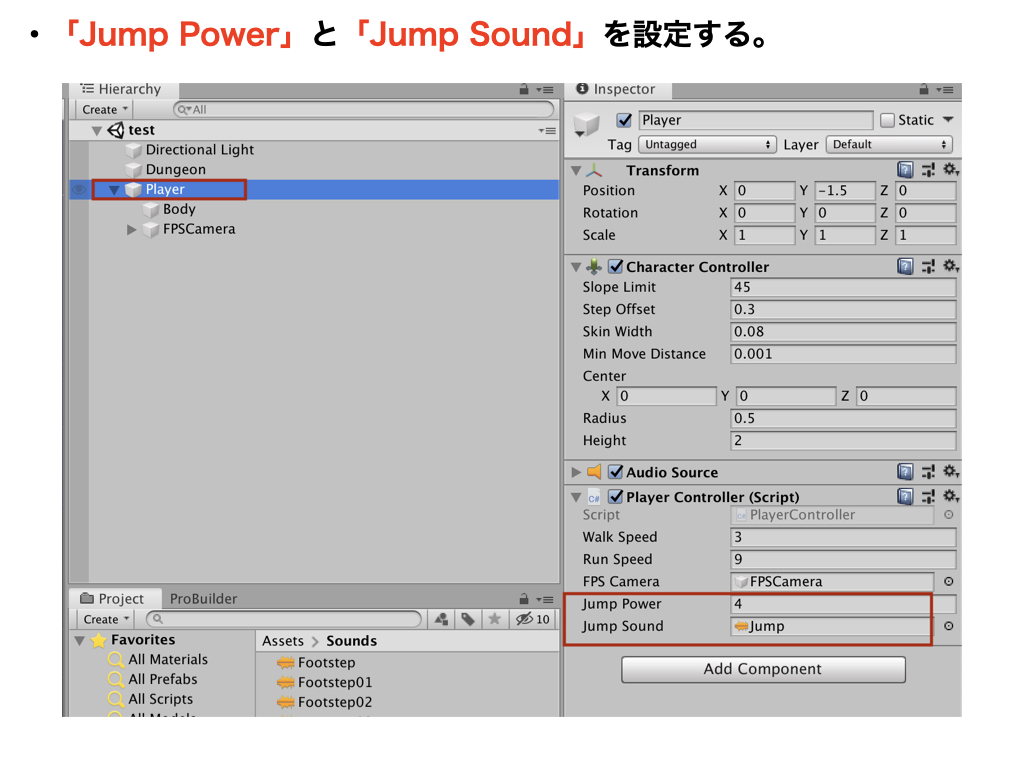
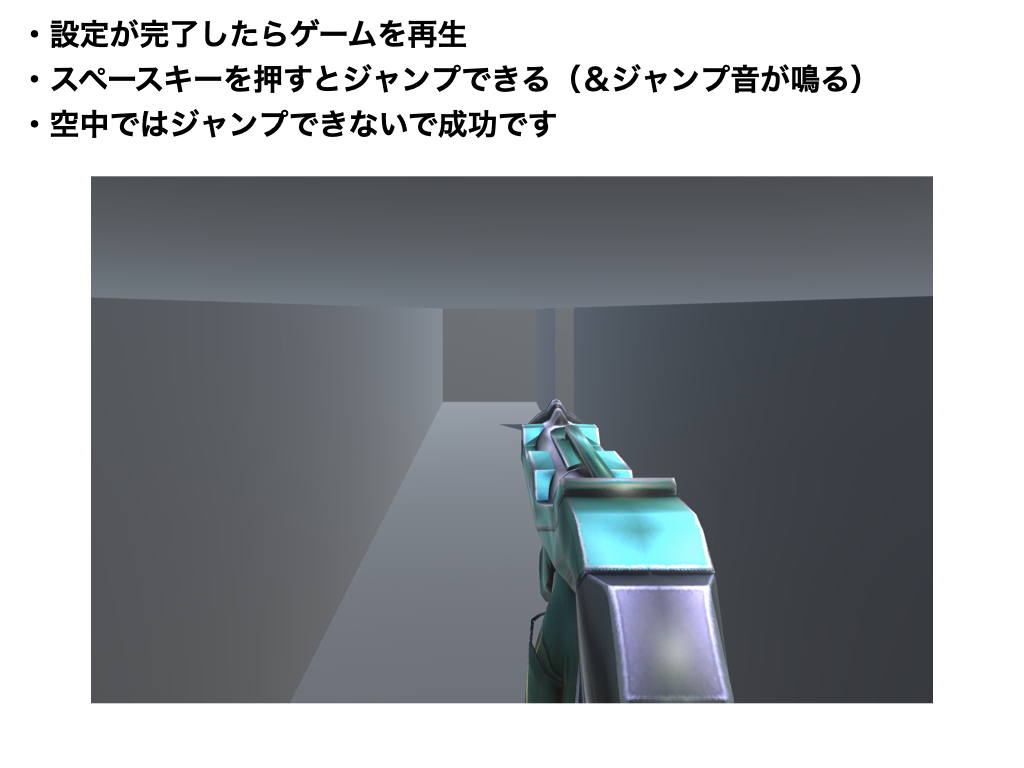
【2019版】X_Mission(基礎/全51回)
他のコースを見る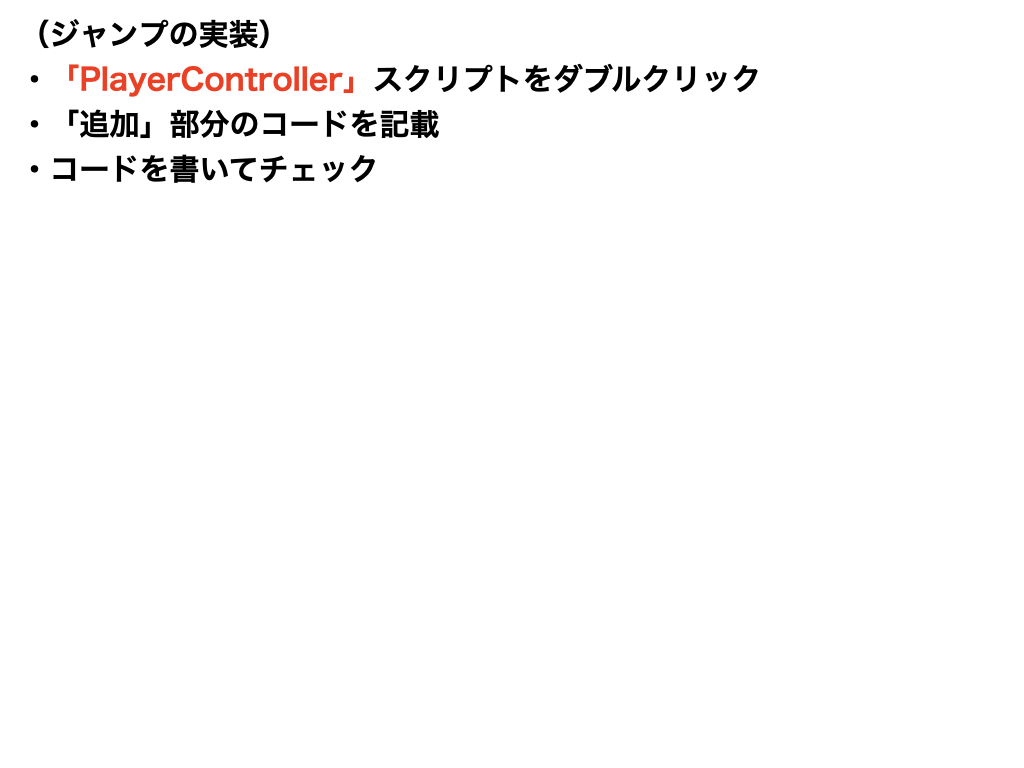
ジャンプ機能の実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[RequireComponent(typeof(CharacterController))]
[RequireComponent(typeof(AudioSource))]
public class PlayerController : MonoBehaviour
{
public float walkSpeed;
public float runSpeed;
private Vector3 movement;
private CharacterController characterController;
private AudioSource audioSource;
public GameObject FPSCamera;
private Vector3 moveDir = Vector3.zero;
// ★追加(ジャンプ)
private float gravity = 9.8f;
public float jumpPower;
public AudioClip jumpSound;
private void Start()
{
characterController = GetComponent<CharacterController>();
audioSource = GetComponent<AudioSource>();
}
private void Update()
{
PlayerMove();
}
void PlayerMove()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
movement = new Vector3(moveH, 0, moveV);
Vector3 desiredMove = FPSCamera.transform.forward * movement.z + FPSCamera.transform.right * movement.x;
moveDir.x = desiredMove.x * 5f;
moveDir.z = desiredMove.z * 5f;
if (Input.GetKey(KeyCode.LeftShift))
{
characterController.Move(moveDir * Time.deltaTime * runSpeed);
}
else
{
characterController.Move(moveDir * Time.deltaTime * walkSpeed);
}
// ★追加(ジャンプ)
if(characterController.isGrounded)
{
if(Input.GetKeyDown(KeyCode.Space))
{
moveDir.y = jumpPower;
audioSource.clip = jumpSound;
audioSource.Play();
}
}
else
{
// 擬似重力の実装
moveDir.y -= gravity * Time.deltaTime;
}
}
}
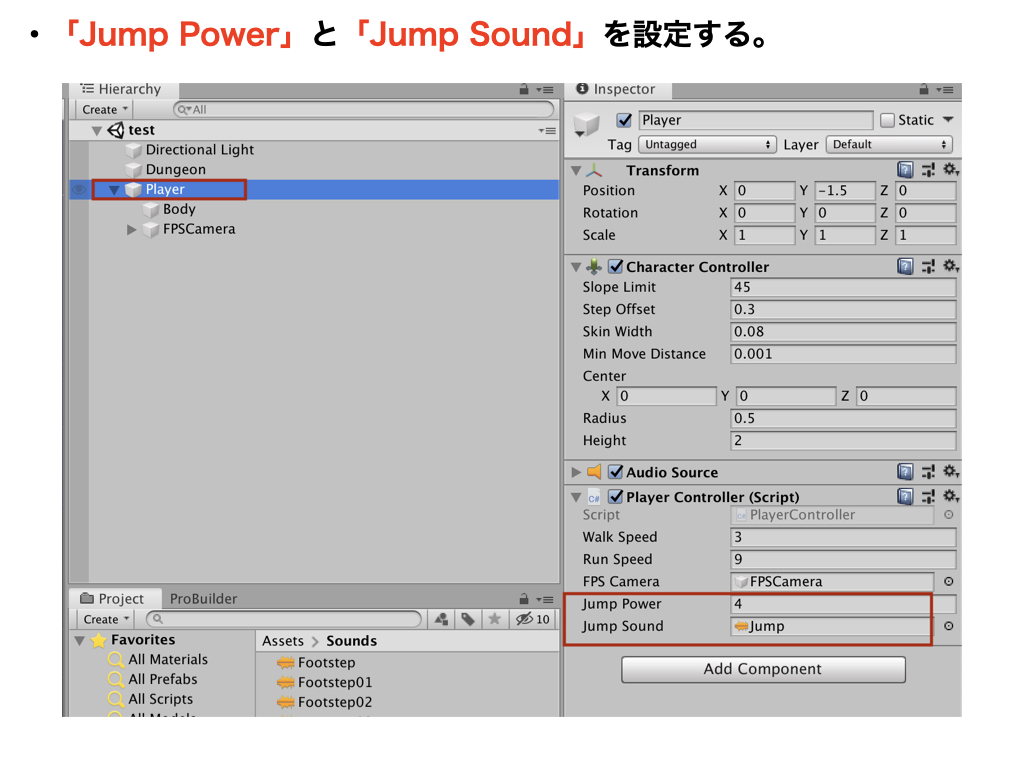
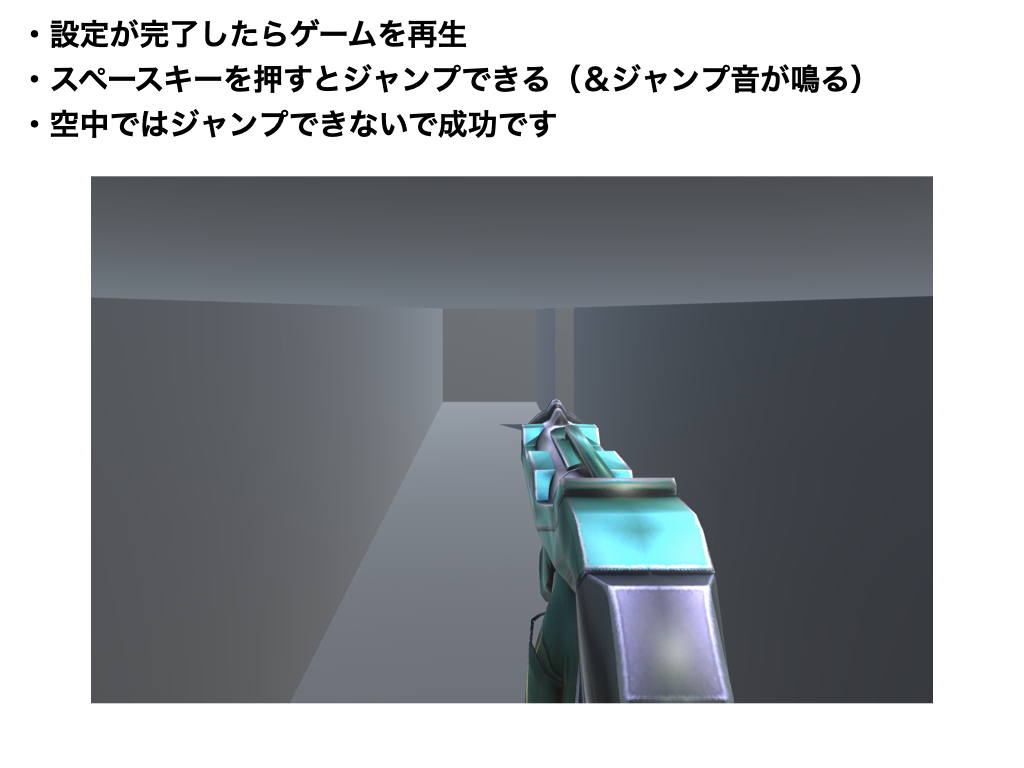
プレーヤーをジャンプさせる