移動時の頭振りの実装
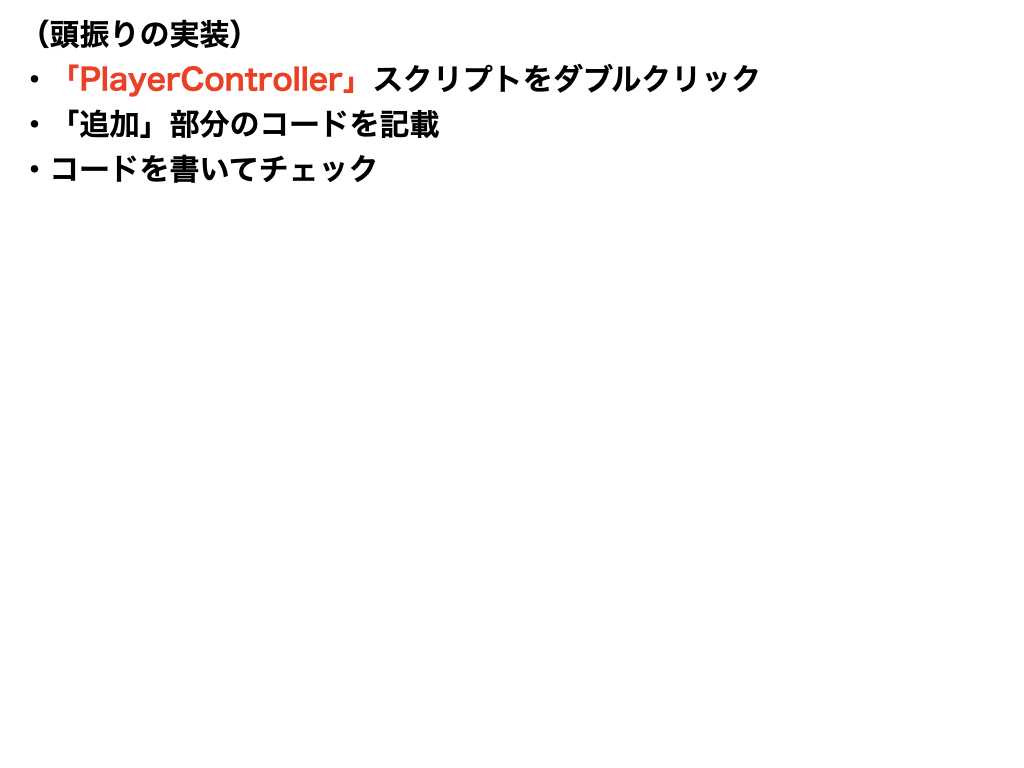
頭振りの実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[RequireComponent(typeof(CharacterController))]
[RequireComponent(typeof(AudioSource))]
public class PlayerController : MonoBehaviour
{
public float walkSpeed;
public float runSpeed;
private Vector3 movement;
private CharacterController characterController;
private AudioSource audioSource;
public GameObject FPSCamera;
private Vector3 moveDir = Vector3.zero;
private float gravity = 9.8f;
public float jumpPower;
public AudioClip jumpSound;
private float runStepLengthen = 0.7f;
private float stepInterval = 10f;
private float stepCycle;
private float nextStep;
private float speed;
private bool isWalking;
public AudioClip stepSound;
// ★追加(頭振り)
private Vector3 initialCameraPosition;
private float timer = 0;
private float bobbingSpeed = 10f;
private float walkBobbing = 0.04f;
private float runBobbing = 0.07f;
private void Start()
{
characterController = GetComponent<CharacterController>();
audioSource = GetComponent<AudioSource>();
// ★追加(頭振り)
initialCameraPosition = FPSCamera.transform.localPosition;
}
private void Update()
{
PlayerMove();
ProgressStepCycle(speed);
// ★追加(頭振り)
HeadBob();
}
void PlayerMove()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
movement = new Vector3(moveH, 0, moveV);
Vector3 desiredMove = FPSCamera.transform.forward * movement.z + FPSCamera.transform.right * movement.x;
moveDir.x = desiredMove.x * 5f;
moveDir.z = desiredMove.z * 5f;
if (Input.GetKey(KeyCode.LeftShift))
{
characterController.Move(moveDir * Time.deltaTime * runSpeed);
isWalking = false;
speed = runSpeed;
}
else
{
characterController.Move(moveDir * Time.deltaTime * walkSpeed);
isWalking = true;
speed = walkSpeed;
}
if (characterController.isGrounded)
{
if (Input.GetKeyDown(KeyCode.Space))
{
moveDir.y = jumpPower;
audioSource.clip = jumpSound;
audioSource.Play();
}
}
else
{
moveDir.y -= gravity * Time.deltaTime;
}
}
void PlayStepSound()
{
if(!characterController.isGrounded)
{
return;
}
audioSource.clip = stepSound;
audioSource.PlayOneShot(audioSource.clip);
}
void ProgressStepCycle(float speed)
{
if(characterController.velocity.sqrMagnitude > 0 && (movement.x != 0 || movement.z != 0))
{
stepCycle += (characterController.velocity.magnitude + (speed * (isWalking ? 1f : runStepLengthen))) * Time.deltaTime;
}
if(!(stepCycle > nextStep))
{
return;
}
nextStep = stepCycle + stepInterval;
PlayStepSound();
}
// ★追加(頭振り)
void HeadBob()
{
Vector3 newCameraPosition;
float bobbingAmount;
if(characterController.velocity.sqrMagnitude > 0 && (movement.x != 0 || movement.z != 0))
{
timer += (Time.deltaTime * bobbingSpeed);
// (テクニック)三項演算子
bobbingAmount = isWalking ? walkBobbing : runBobbing;
// walk時とrun時で頭振りの大きさを変化させる。
FPSCamera.transform.localPosition = new Vector3(0, initialCameraPosition.y + Mathf.Sin(timer) * bobbingAmount, 0);
newCameraPosition = FPSCamera.transform.localPosition;
}
else
{
timer = 0;
newCameraPosition = FPSCamera.transform.localPosition;
}
FPSCamera.transform.localPosition = newCameraPosition;
}
}
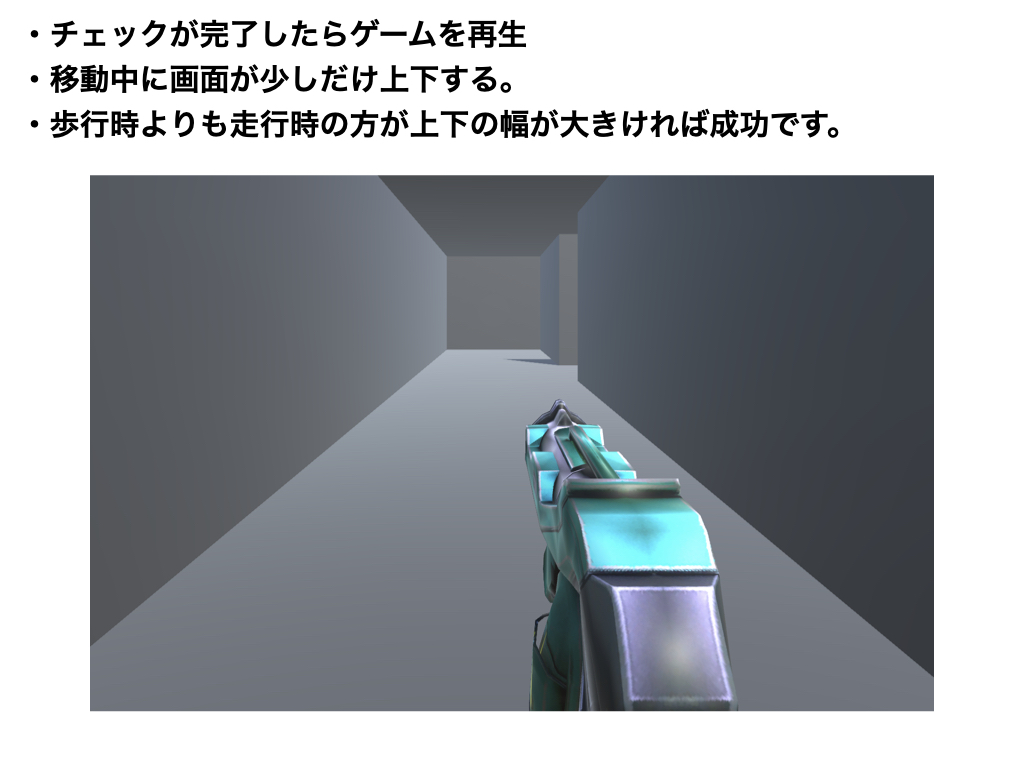
【2019版】X_Mission(基礎/全51回)
他のコースを見る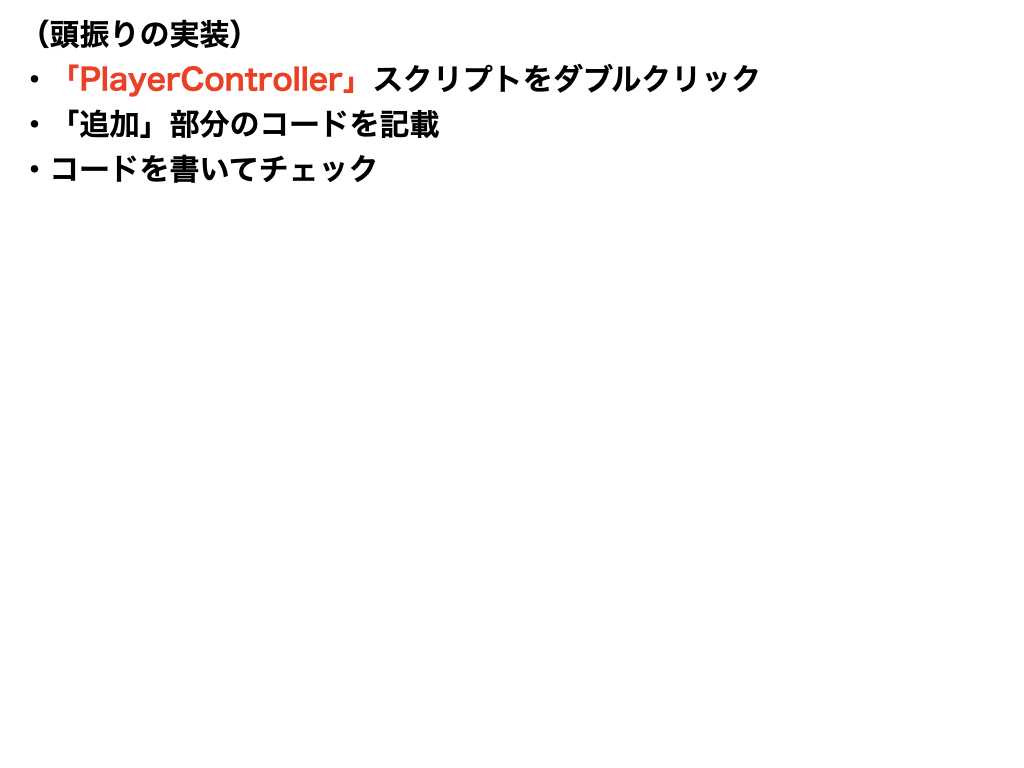
頭振りの実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[RequireComponent(typeof(CharacterController))]
[RequireComponent(typeof(AudioSource))]
public class PlayerController : MonoBehaviour
{
public float walkSpeed;
public float runSpeed;
private Vector3 movement;
private CharacterController characterController;
private AudioSource audioSource;
public GameObject FPSCamera;
private Vector3 moveDir = Vector3.zero;
private float gravity = 9.8f;
public float jumpPower;
public AudioClip jumpSound;
private float runStepLengthen = 0.7f;
private float stepInterval = 10f;
private float stepCycle;
private float nextStep;
private float speed;
private bool isWalking;
public AudioClip stepSound;
// ★追加(頭振り)
private Vector3 initialCameraPosition;
private float timer = 0;
private float bobbingSpeed = 10f;
private float walkBobbing = 0.04f;
private float runBobbing = 0.07f;
private void Start()
{
characterController = GetComponent<CharacterController>();
audioSource = GetComponent<AudioSource>();
// ★追加(頭振り)
initialCameraPosition = FPSCamera.transform.localPosition;
}
private void Update()
{
PlayerMove();
ProgressStepCycle(speed);
// ★追加(頭振り)
HeadBob();
}
void PlayerMove()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
movement = new Vector3(moveH, 0, moveV);
Vector3 desiredMove = FPSCamera.transform.forward * movement.z + FPSCamera.transform.right * movement.x;
moveDir.x = desiredMove.x * 5f;
moveDir.z = desiredMove.z * 5f;
if (Input.GetKey(KeyCode.LeftShift))
{
characterController.Move(moveDir * Time.deltaTime * runSpeed);
isWalking = false;
speed = runSpeed;
}
else
{
characterController.Move(moveDir * Time.deltaTime * walkSpeed);
isWalking = true;
speed = walkSpeed;
}
if (characterController.isGrounded)
{
if (Input.GetKeyDown(KeyCode.Space))
{
moveDir.y = jumpPower;
audioSource.clip = jumpSound;
audioSource.Play();
}
}
else
{
moveDir.y -= gravity * Time.deltaTime;
}
}
void PlayStepSound()
{
if(!characterController.isGrounded)
{
return;
}
audioSource.clip = stepSound;
audioSource.PlayOneShot(audioSource.clip);
}
void ProgressStepCycle(float speed)
{
if(characterController.velocity.sqrMagnitude > 0 && (movement.x != 0 || movement.z != 0))
{
stepCycle += (characterController.velocity.magnitude + (speed * (isWalking ? 1f : runStepLengthen))) * Time.deltaTime;
}
if(!(stepCycle > nextStep))
{
return;
}
nextStep = stepCycle + stepInterval;
PlayStepSound();
}
// ★追加(頭振り)
void HeadBob()
{
Vector3 newCameraPosition;
float bobbingAmount;
if(characterController.velocity.sqrMagnitude > 0 && (movement.x != 0 || movement.z != 0))
{
timer += (Time.deltaTime * bobbingSpeed);
// (テクニック)三項演算子
bobbingAmount = isWalking ? walkBobbing : runBobbing;
// walk時とrun時で頭振りの大きさを変化させる。
FPSCamera.transform.localPosition = new Vector3(0, initialCameraPosition.y + Mathf.Sin(timer) * bobbingAmount, 0);
newCameraPosition = FPSCamera.transform.localPosition;
}
else
{
timer = 0;
newCameraPosition = FPSCamera.transform.localPosition;
}
FPSCamera.transform.localPosition = newCameraPosition;
}
}
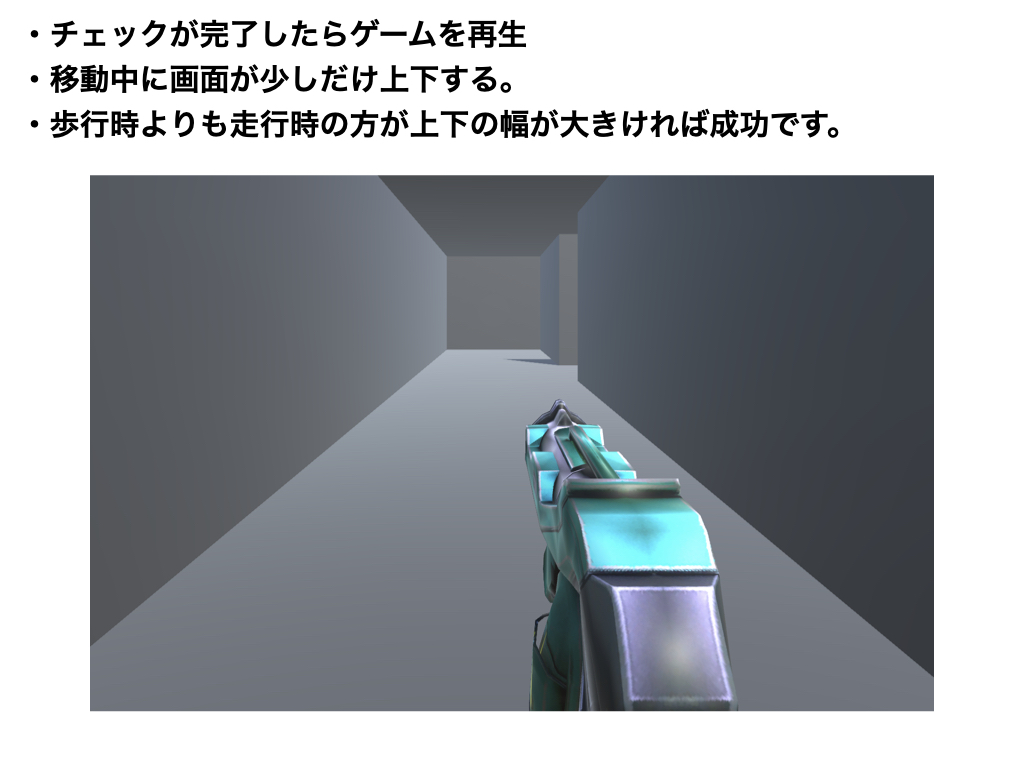
移動時の頭振りの実装