レーザーガンの実装
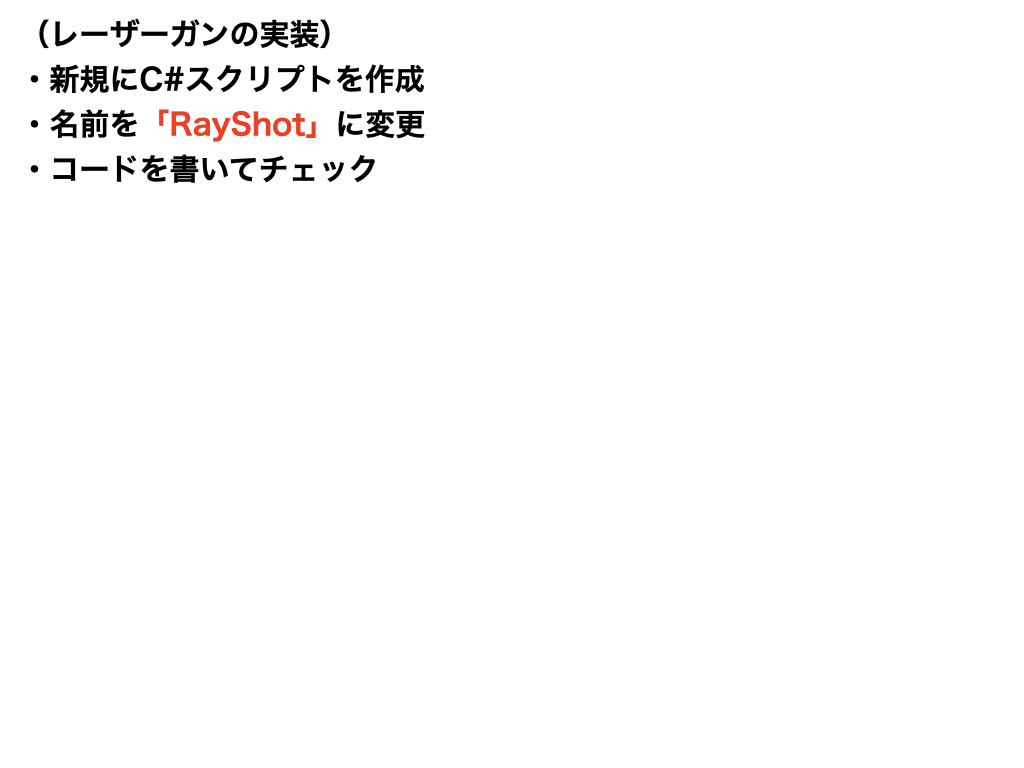
レーザーガンの実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[RequireComponent(typeof(LineRenderer))]
[RequireComponent(typeof(AudioSource))]
public class RayShot : MonoBehaviour
{
public Transform gunPoint; // CreateEmptyで銃口を作成してそこをレーザーの始点にする。
private float fireRate = 0.25f;
private float weaponRange = 50f;
private float hitForce = 300f;
private Camera FPSCamera;
private WaitForSeconds shotDuration = new WaitForSeconds(0.07f); // 待機時間の設定
private AudioSource gunAudio;
private LineRenderer laserLine;
private float nextFire;
void Start()
{
laserLine = GetComponent<LineRenderer>();
gunAudio = GetComponent<AudioSource>();
FPSCamera = GetComponent<Camera>();
laserLine.enabled = false;
}
void Update()
{
Debug.DrawRay(transform.position, transform.forward * 60, Color.red);
// マウス左クリックで発射
if (Input.GetMouseButtonDown(0) && Time.time > nextFire)
{
// fireRate分の時間は発射できない。
nextFire = Time.time + fireRate;
// コルーチンの実行
StartCoroutine(ShotEffect());
// ビューポートの座標をワールド座標に変換する
Vector3 rayOrigin = FPSCamera.ViewportToWorldPoint(new Vector3(0.5f, 0.5f, 0));
RaycastHit hit;
// レーザーの始点
// ignore raycastの設定を忘れないこと(重要ポイント)
laserLine.SetPosition(0, gunPoint.position);
if (Physics.Raycast(rayOrigin, FPSCamera.transform.forward, out hit, weaponRange))
{
// レーザーの終点
laserLine.SetPosition(1, hit.point);
if(hit.rigidbody != null)
{
// 「hit.normal」の使い方をマスターする。
// 法線ベクトル(ある面に垂直なベクトル)
hit.rigidbody.AddForce(-hit.normal * hitForce);
}
}
else
{
laserLine.SetPosition(1, rayOrigin + (FPSCamera.transform.forward * weaponRange));
}
}
}
private IEnumerator ShotEffect()
{
// Play On Awakeのチェックを外すこと(ポイント)
gunAudio.Play();
laserLine.enabled = true;
yield return shotDuration;
// 0.07秒後にレーザーラインが消える。(0.07秒間待機して後、レーザーラインが消える。)
laserLine.enabled = false;
}
}
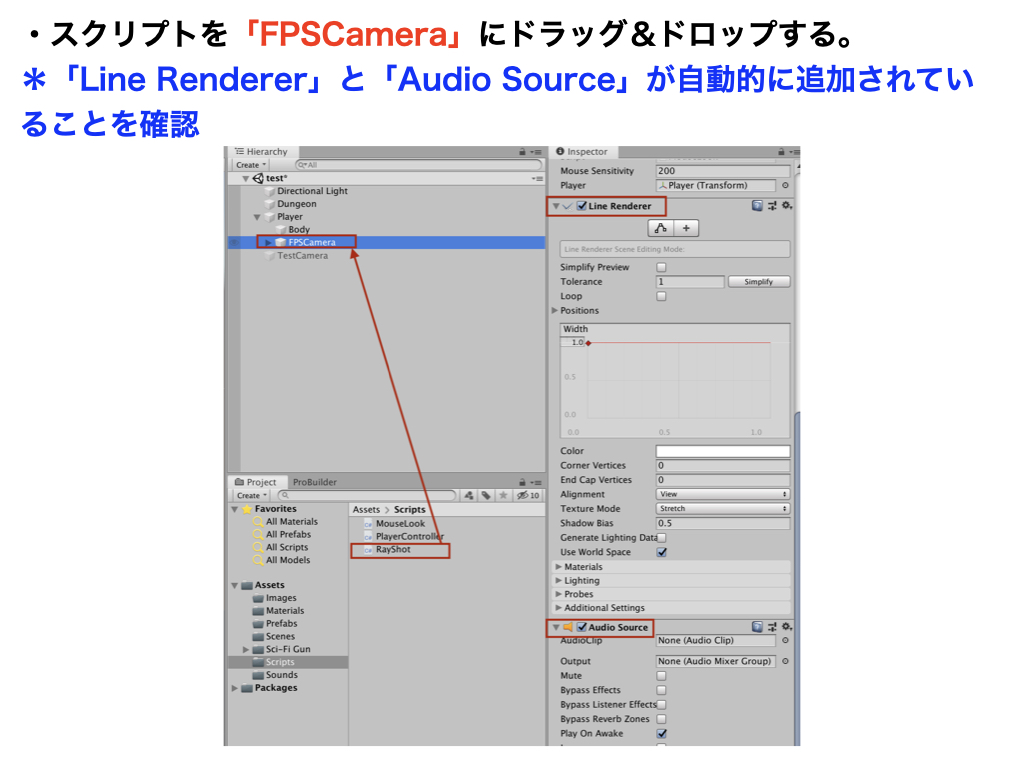
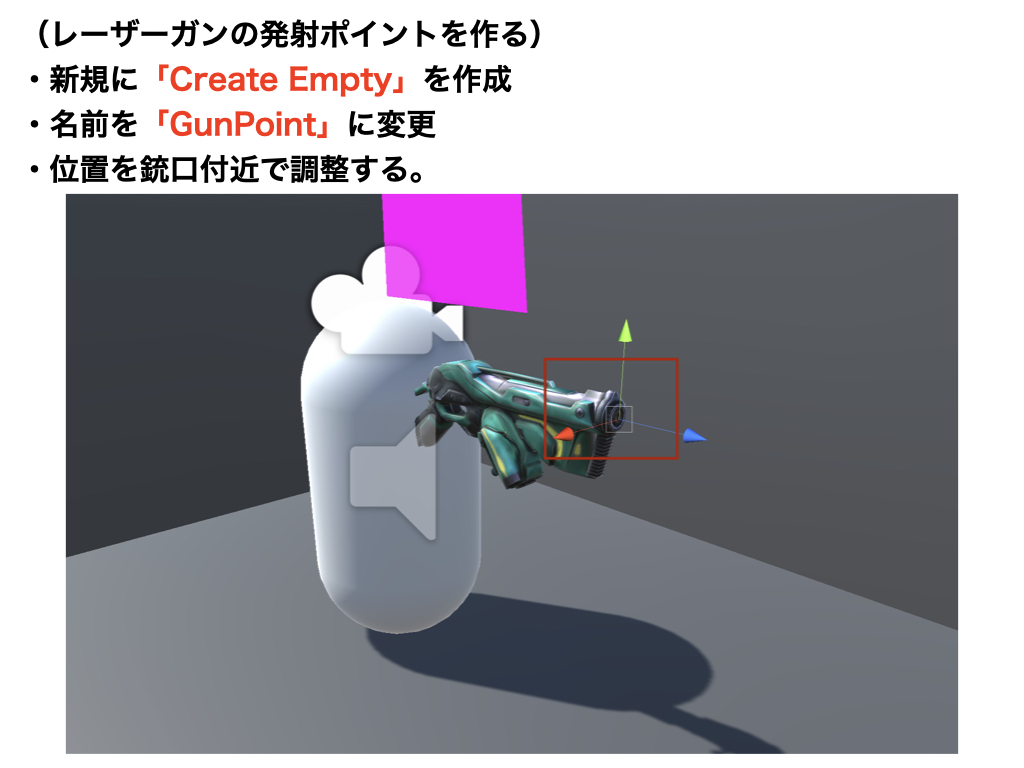
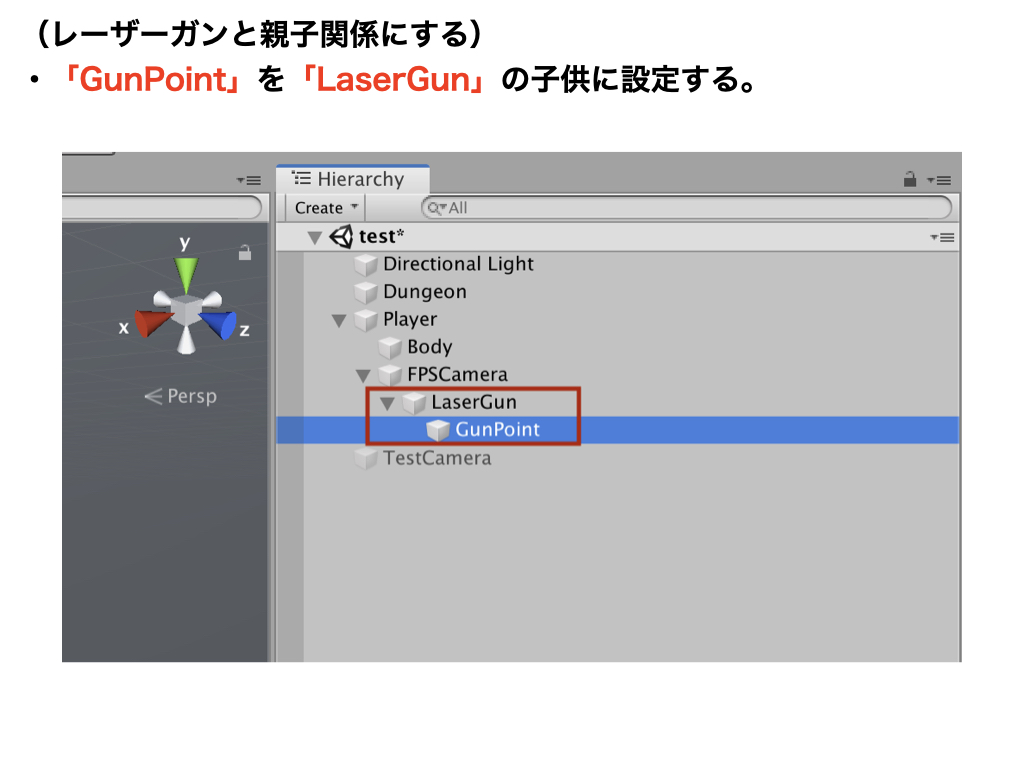
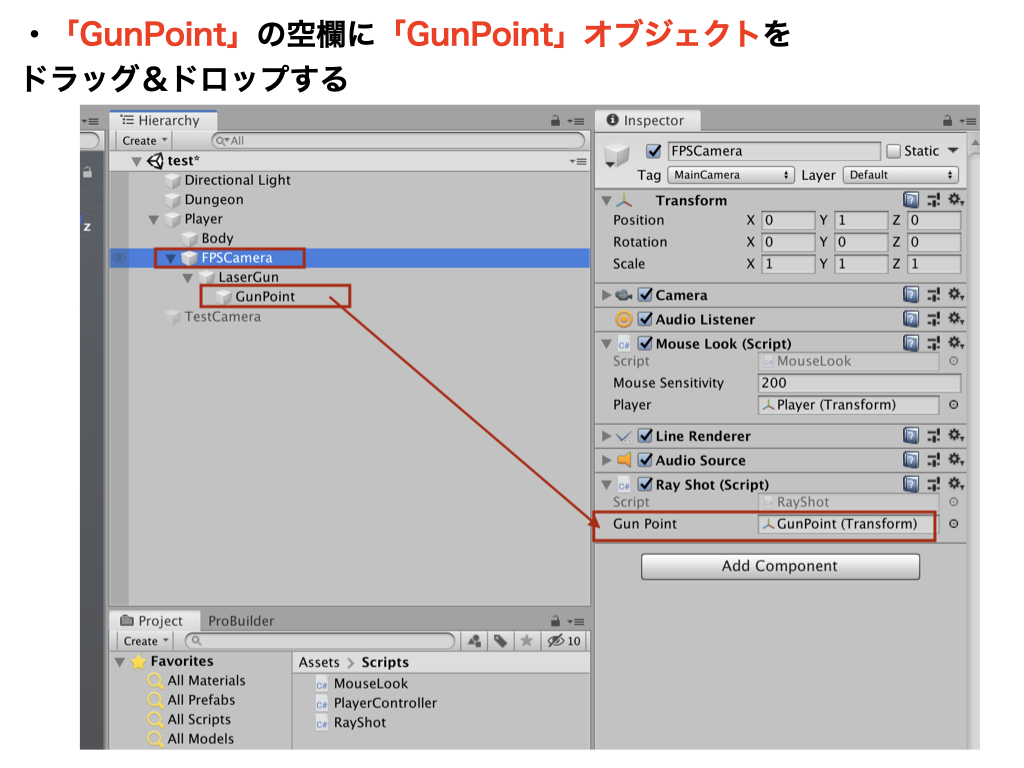
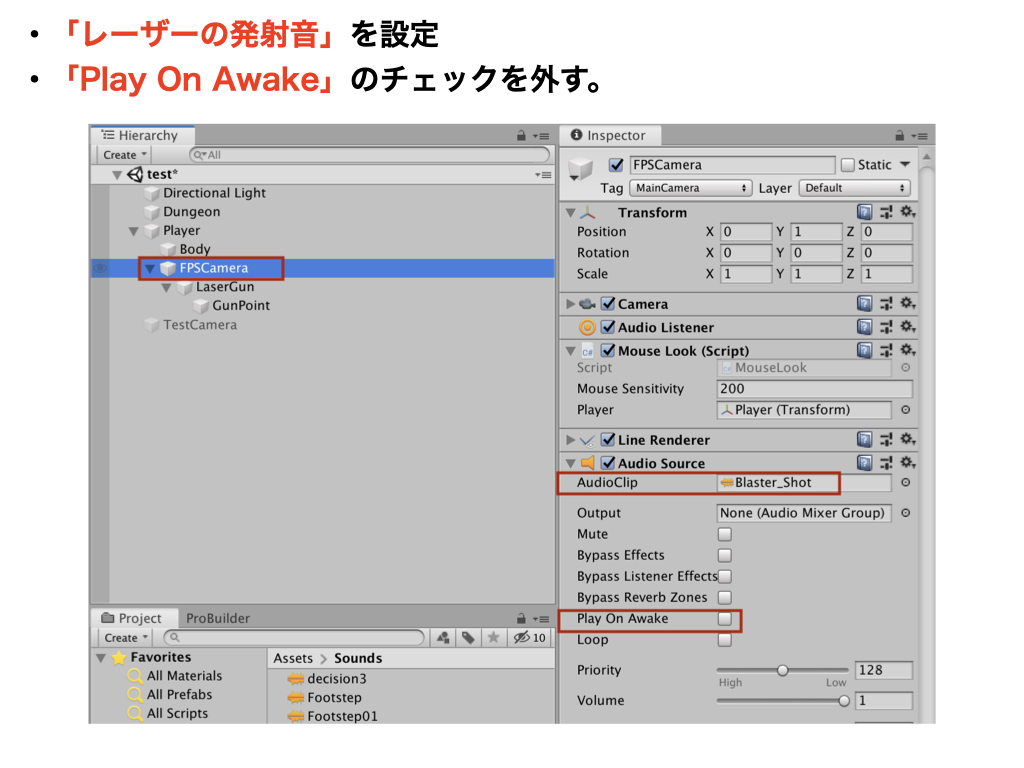
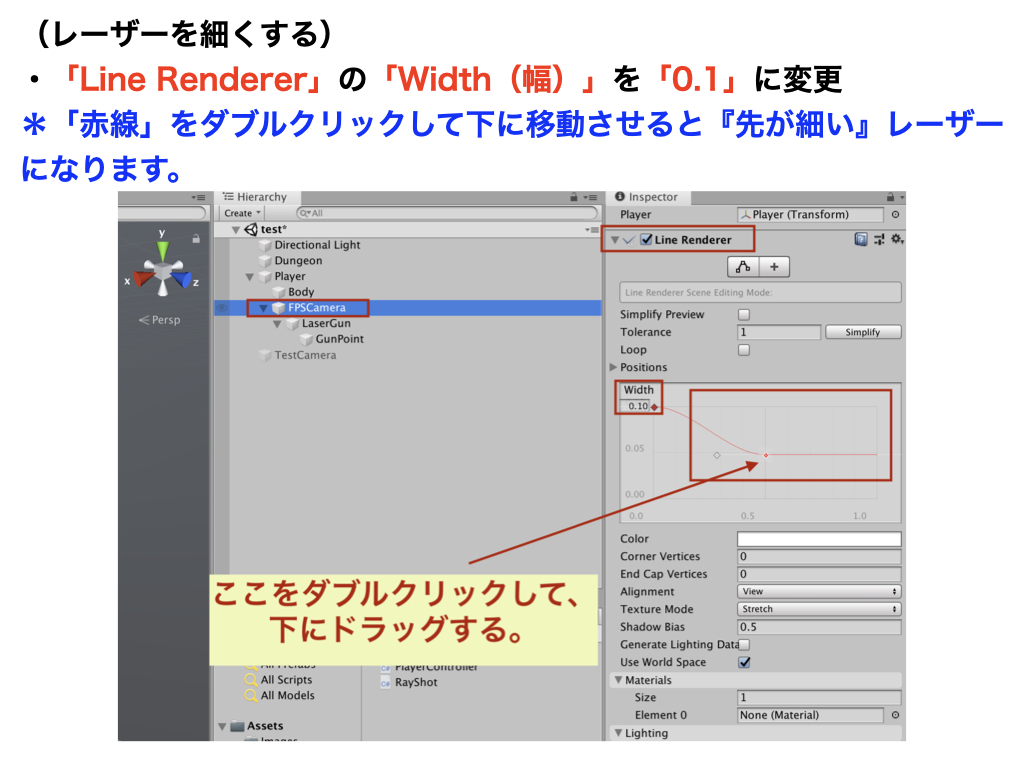
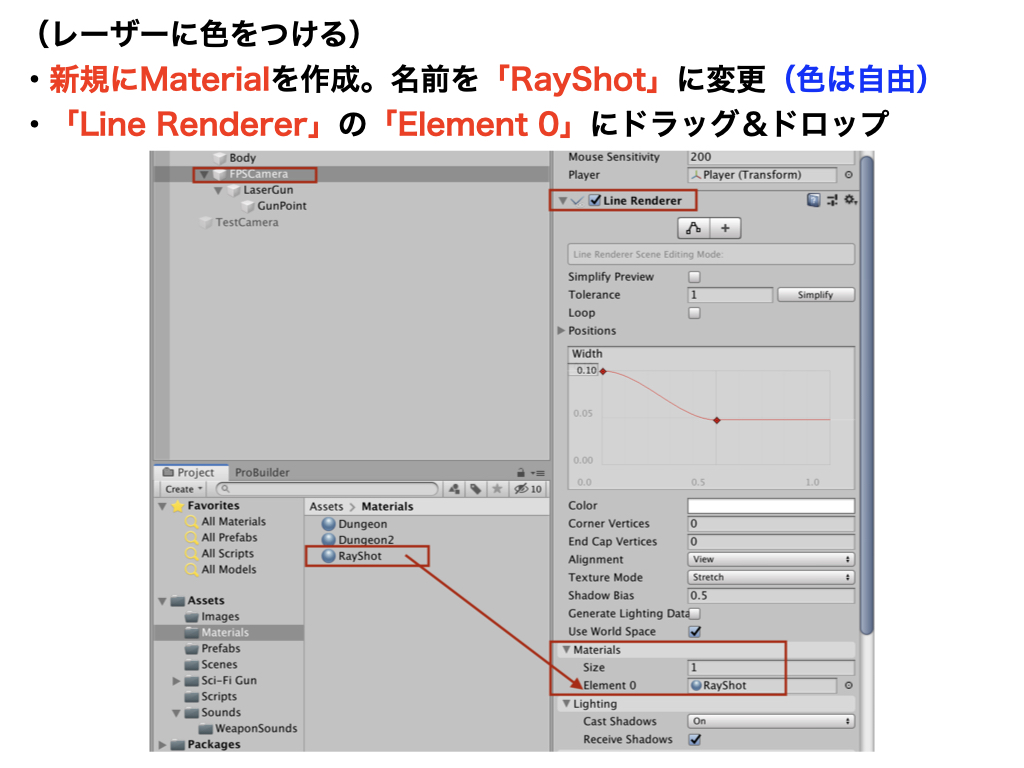
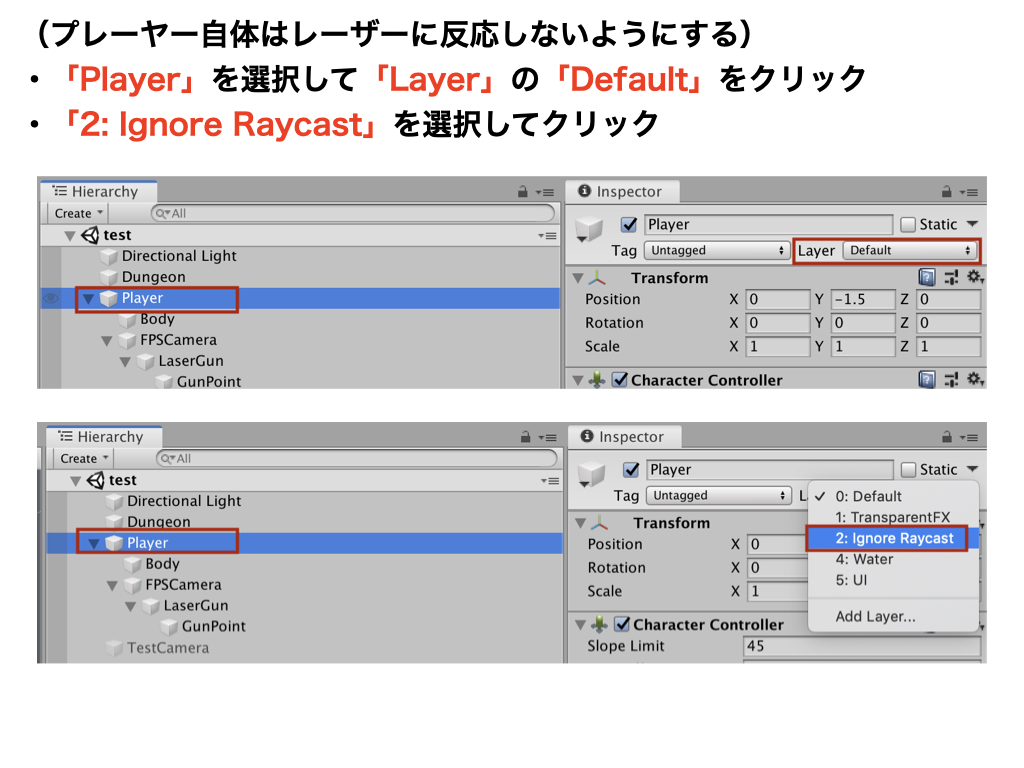
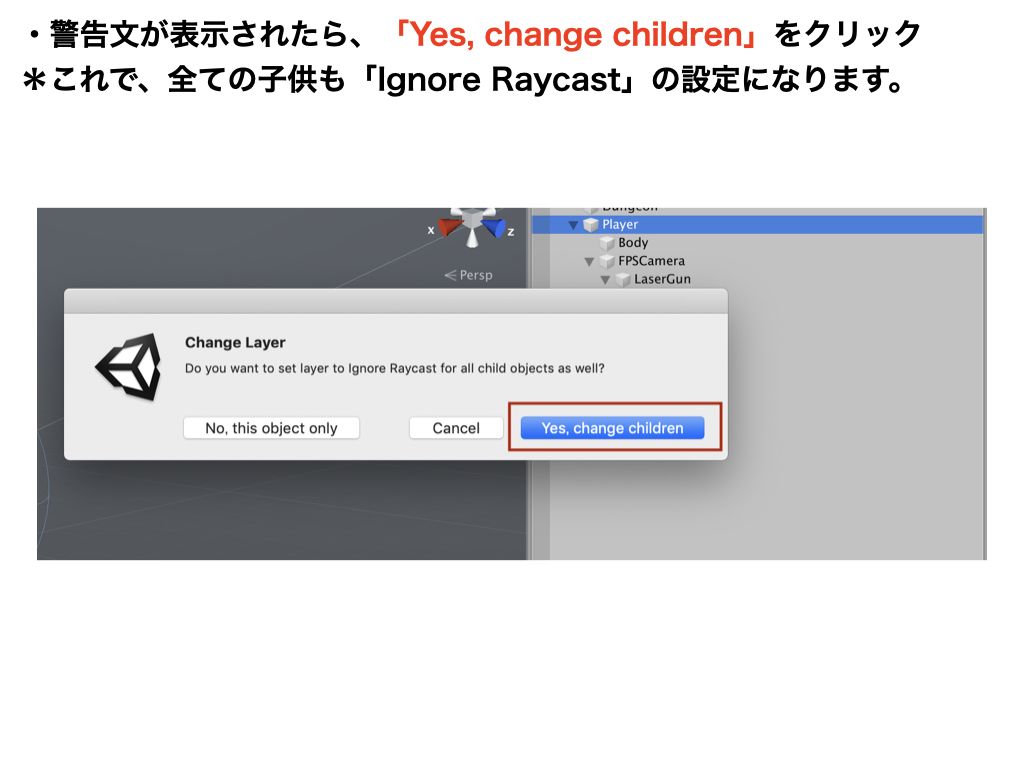
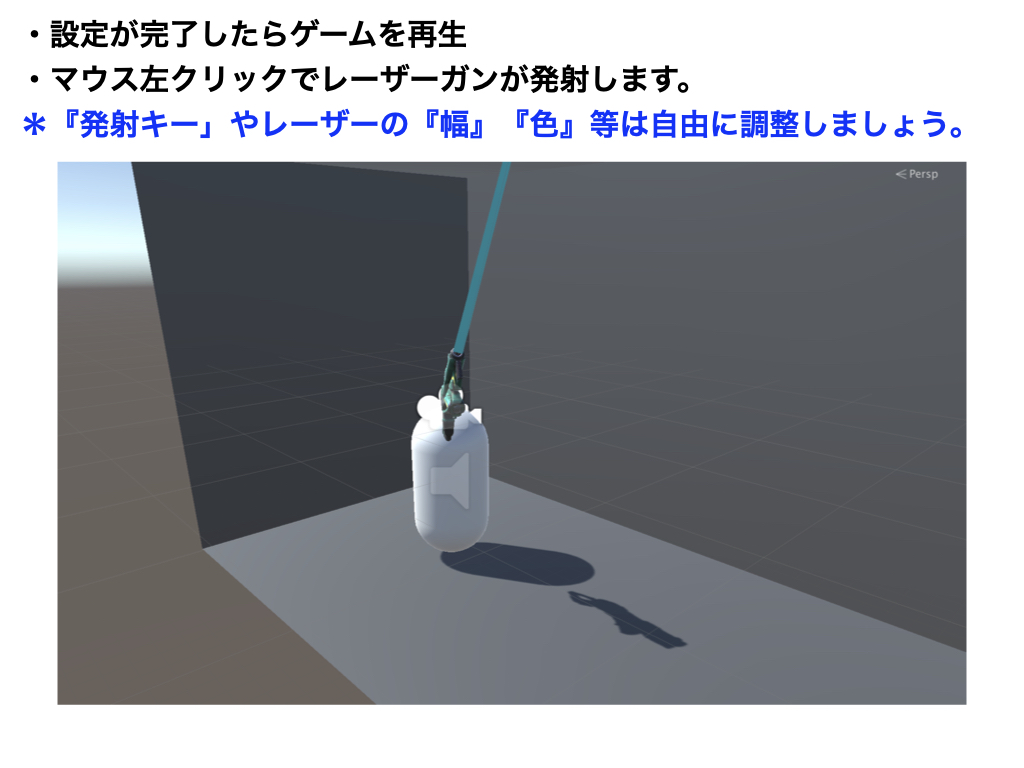
【2019版】X_Mission(基礎/全51回)
他のコースを見る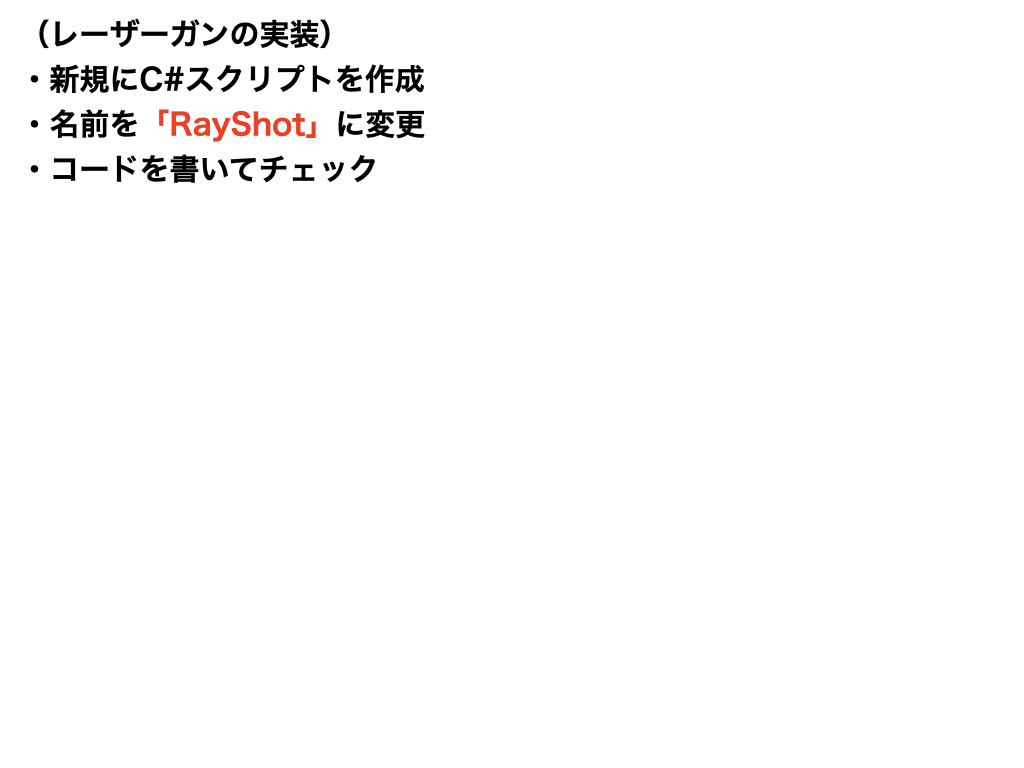
レーザーガンの実装
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[RequireComponent(typeof(LineRenderer))]
[RequireComponent(typeof(AudioSource))]
public class RayShot : MonoBehaviour
{
public Transform gunPoint; // CreateEmptyで銃口を作成してそこをレーザーの始点にする。
private float fireRate = 0.25f;
private float weaponRange = 50f;
private float hitForce = 300f;
private Camera FPSCamera;
private WaitForSeconds shotDuration = new WaitForSeconds(0.07f); // 待機時間の設定
private AudioSource gunAudio;
private LineRenderer laserLine;
private float nextFire;
void Start()
{
laserLine = GetComponent<LineRenderer>();
gunAudio = GetComponent<AudioSource>();
FPSCamera = GetComponent<Camera>();
laserLine.enabled = false;
}
void Update()
{
Debug.DrawRay(transform.position, transform.forward * 60, Color.red);
// マウス左クリックで発射
if (Input.GetMouseButtonDown(0) && Time.time > nextFire)
{
// fireRate分の時間は発射できない。
nextFire = Time.time + fireRate;
// コルーチンの実行
StartCoroutine(ShotEffect());
// ビューポートの座標をワールド座標に変換する
Vector3 rayOrigin = FPSCamera.ViewportToWorldPoint(new Vector3(0.5f, 0.5f, 0));
RaycastHit hit;
// レーザーの始点
// ignore raycastの設定を忘れないこと(重要ポイント)
laserLine.SetPosition(0, gunPoint.position);
if (Physics.Raycast(rayOrigin, FPSCamera.transform.forward, out hit, weaponRange))
{
// レーザーの終点
laserLine.SetPosition(1, hit.point);
if(hit.rigidbody != null)
{
// 「hit.normal」の使い方をマスターする。
// 法線ベクトル(ある面に垂直なベクトル)
hit.rigidbody.AddForce(-hit.normal * hitForce);
}
}
else
{
laserLine.SetPosition(1, rayOrigin + (FPSCamera.transform.forward * weaponRange));
}
}
}
private IEnumerator ShotEffect()
{
// Play On Awakeのチェックを外すこと(ポイント)
gunAudio.Play();
laserLine.enabled = true;
yield return shotDuration;
// 0.07秒後にレーザーラインが消える。(0.07秒間待機して後、レーザーラインが消える。)
laserLine.enabled = false;
}
}
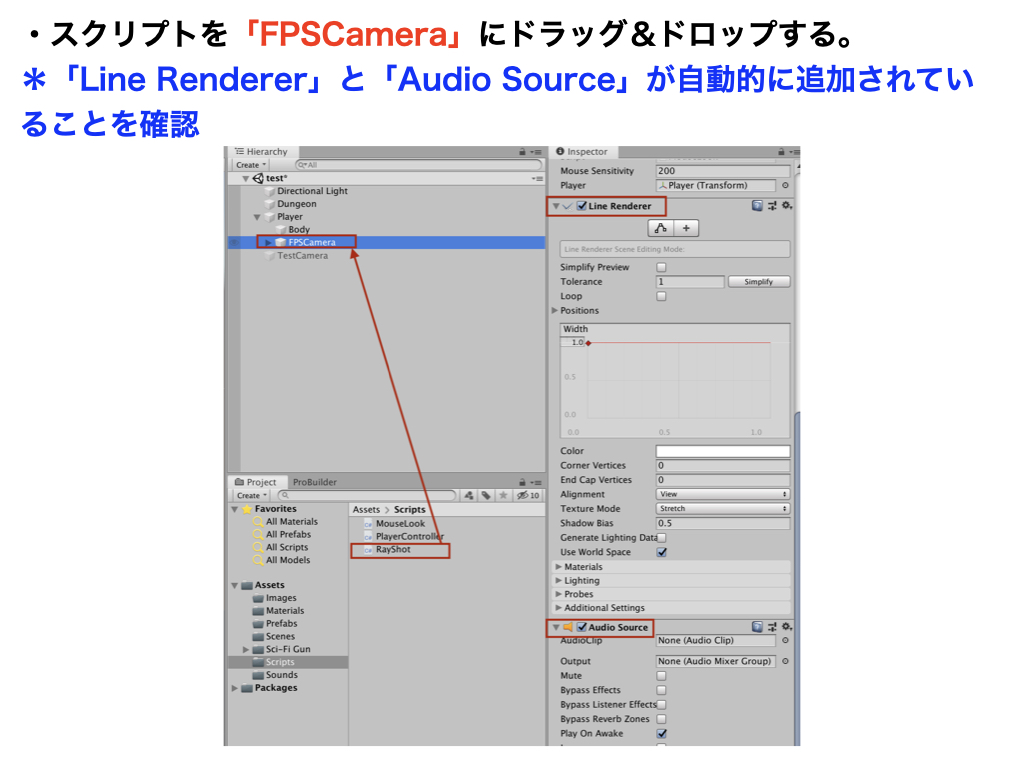
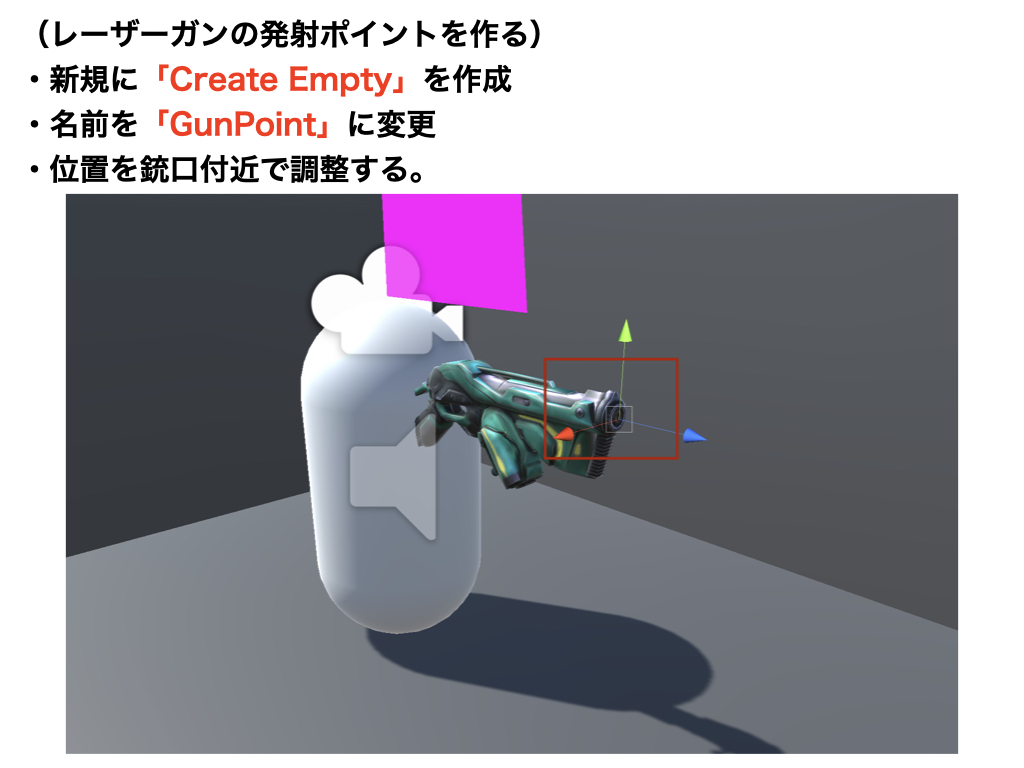
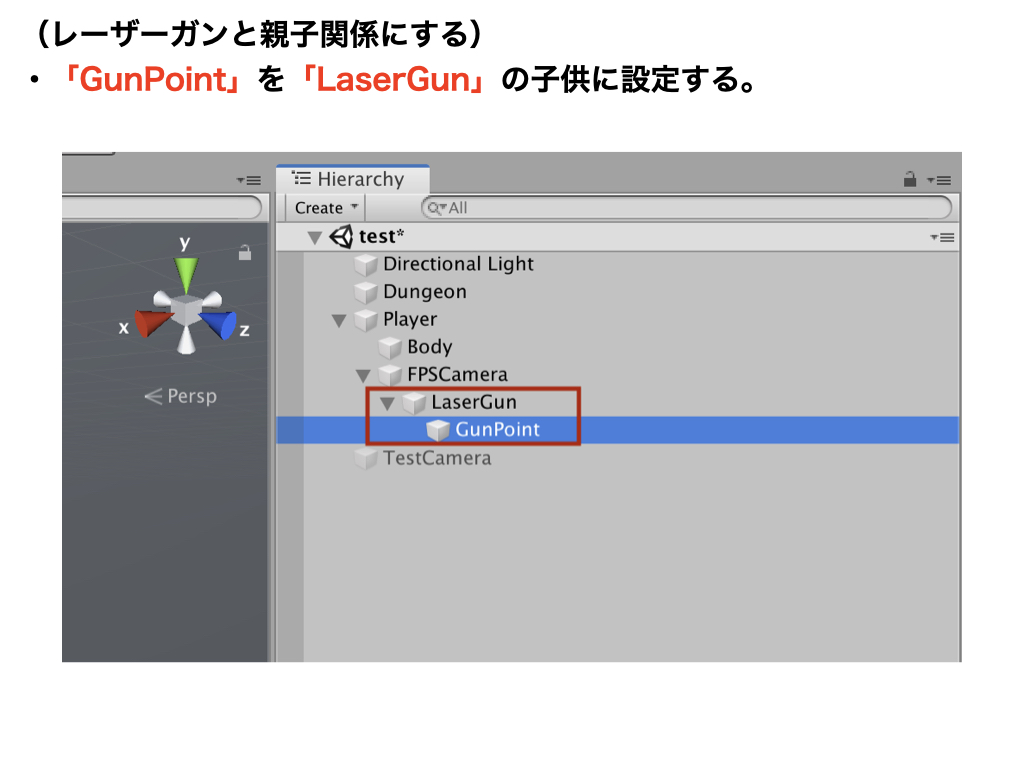
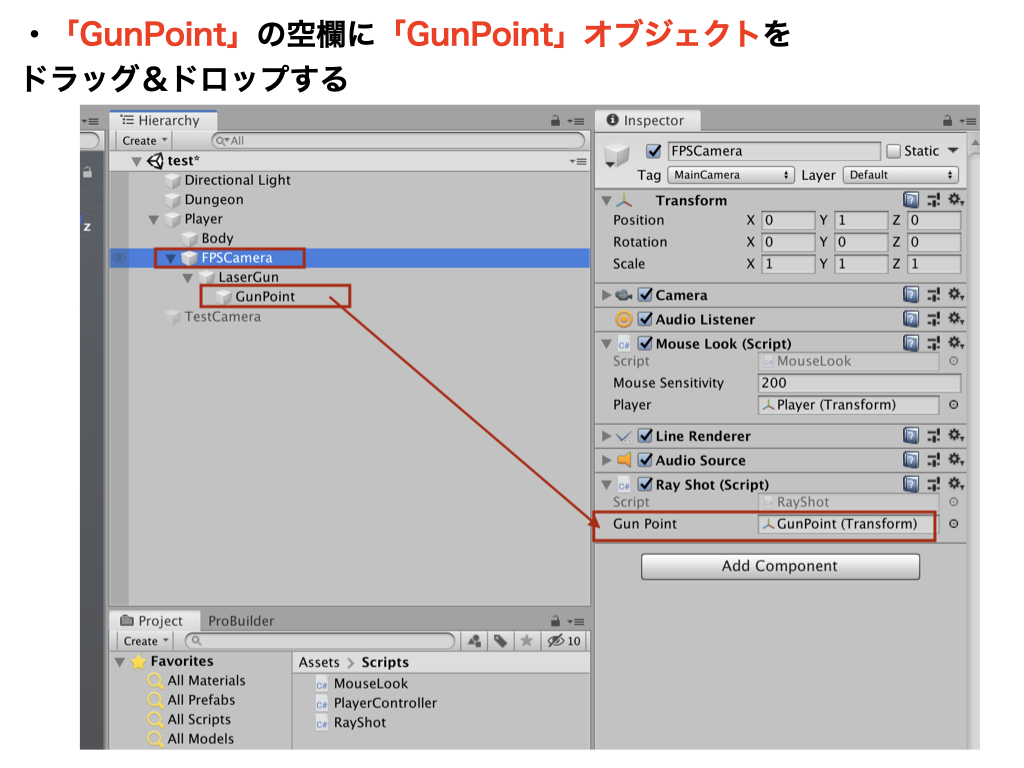
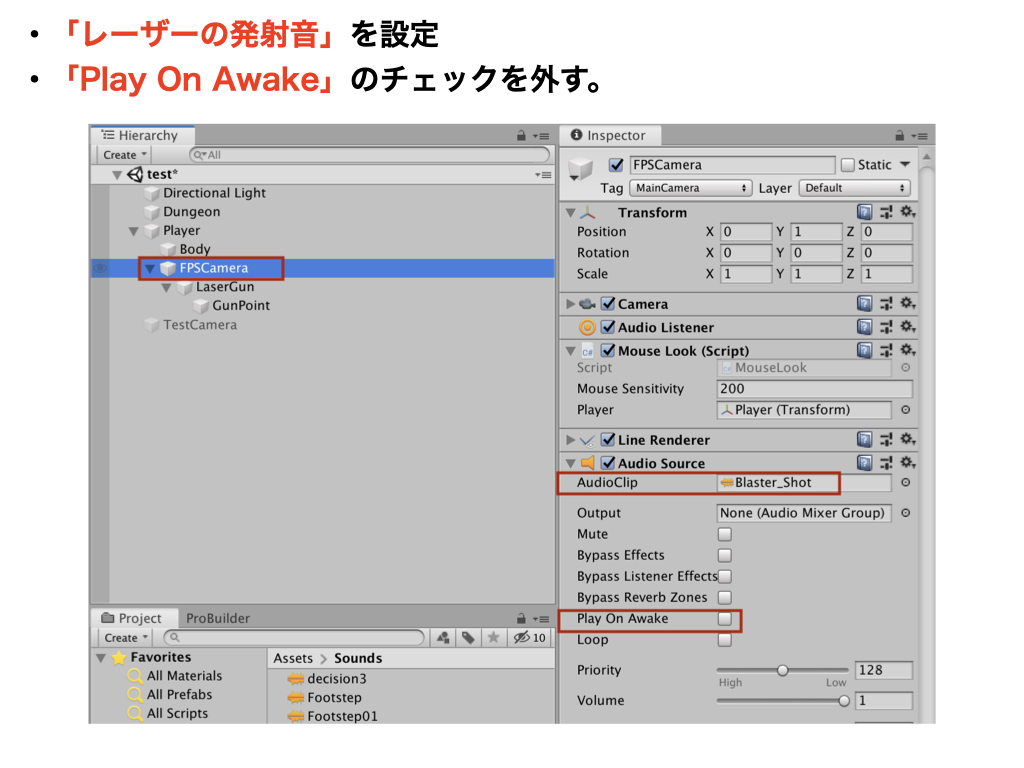
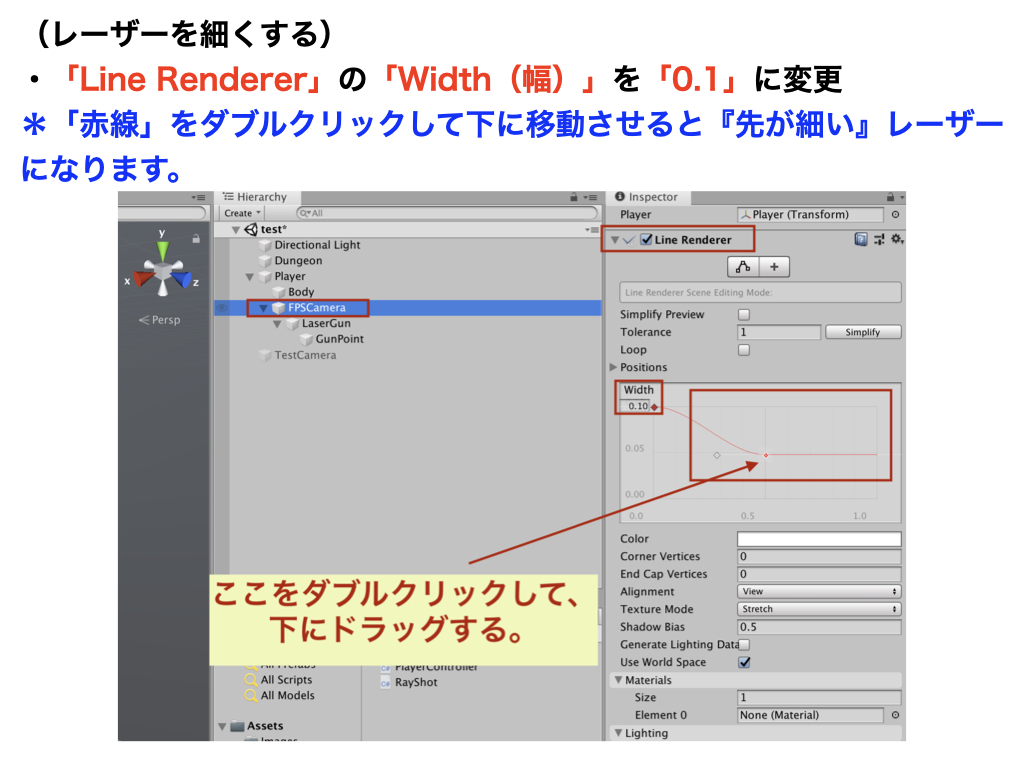
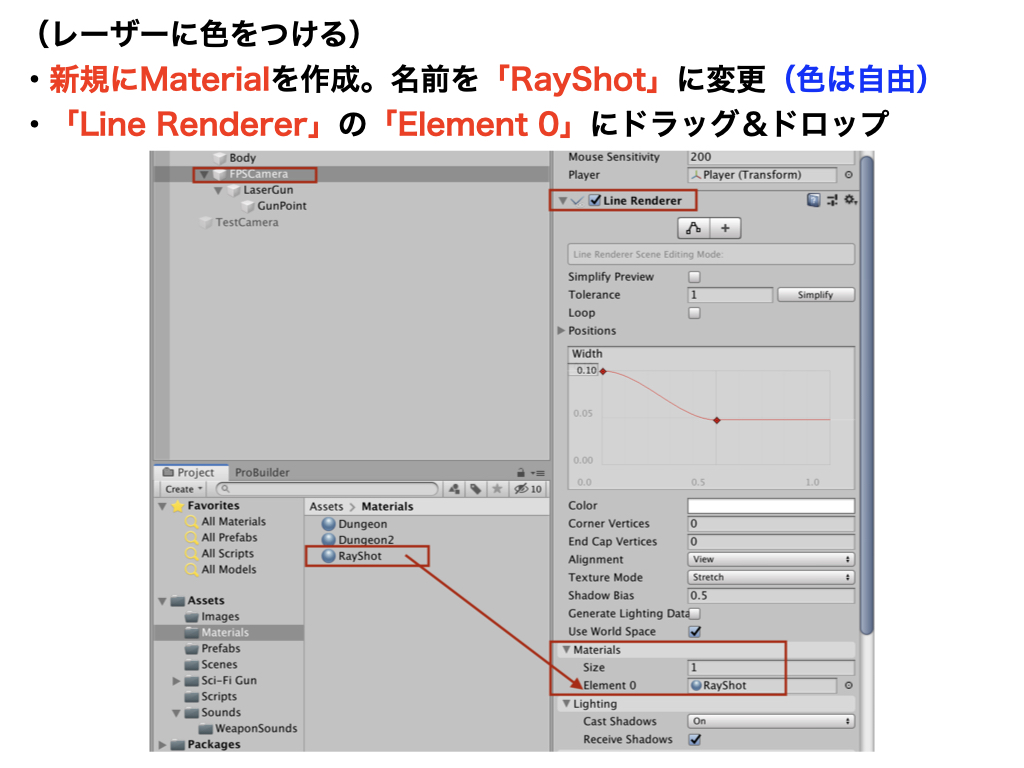
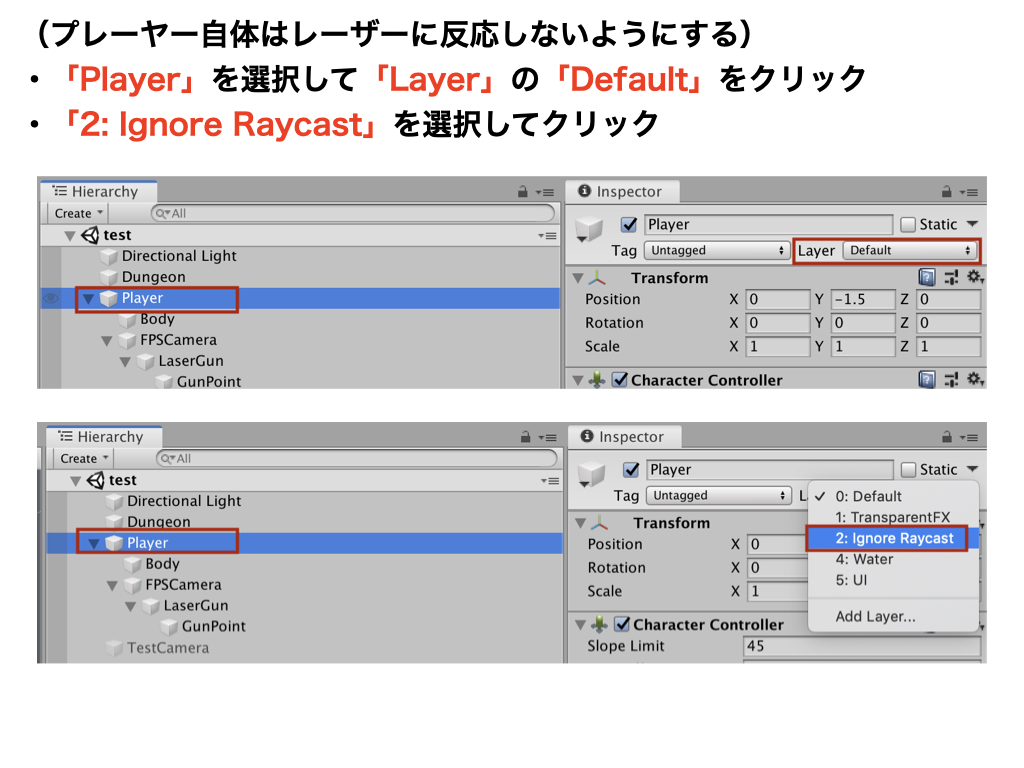
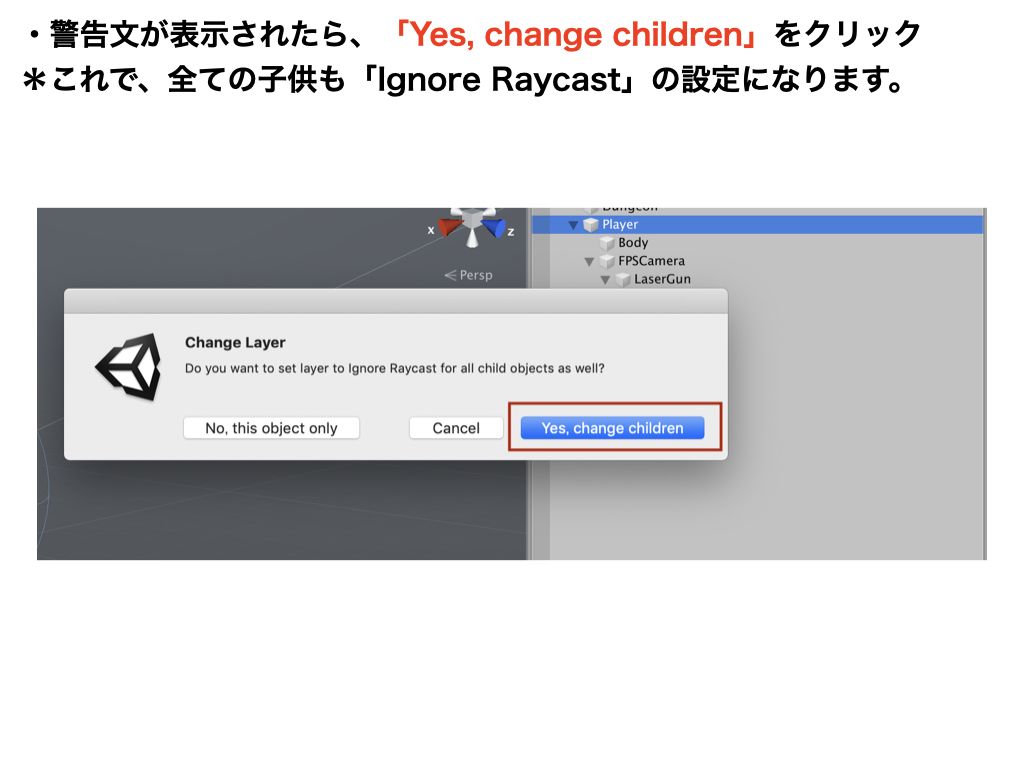
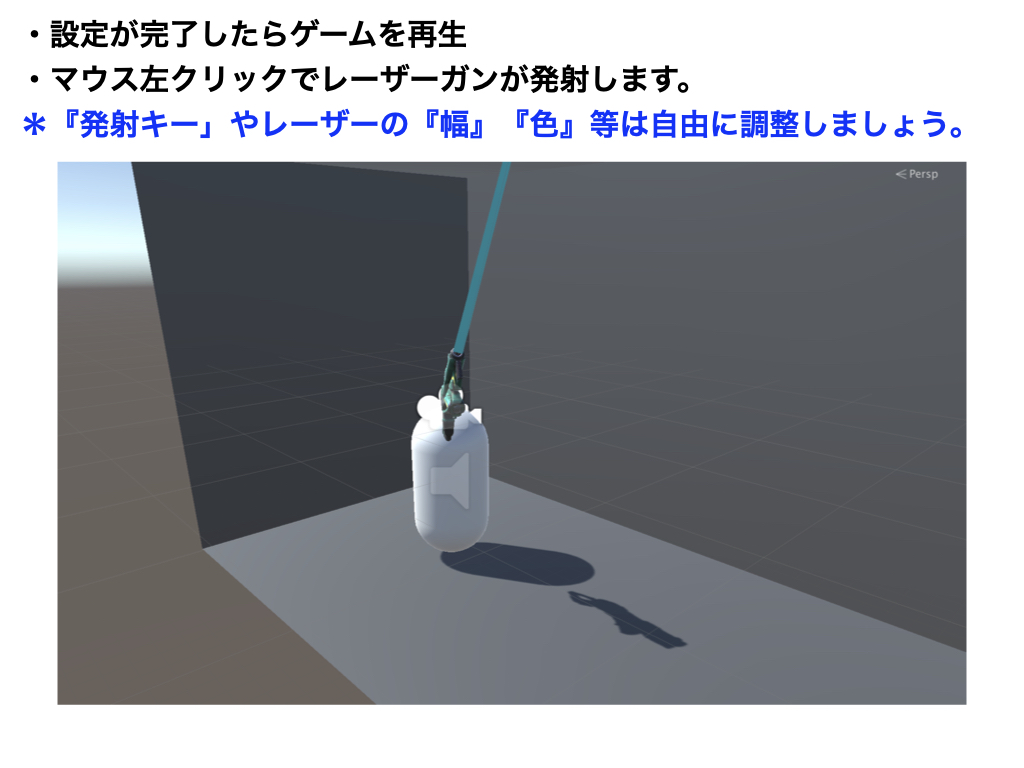
レーザーガンの実装