残弾数を回復させるアイテム
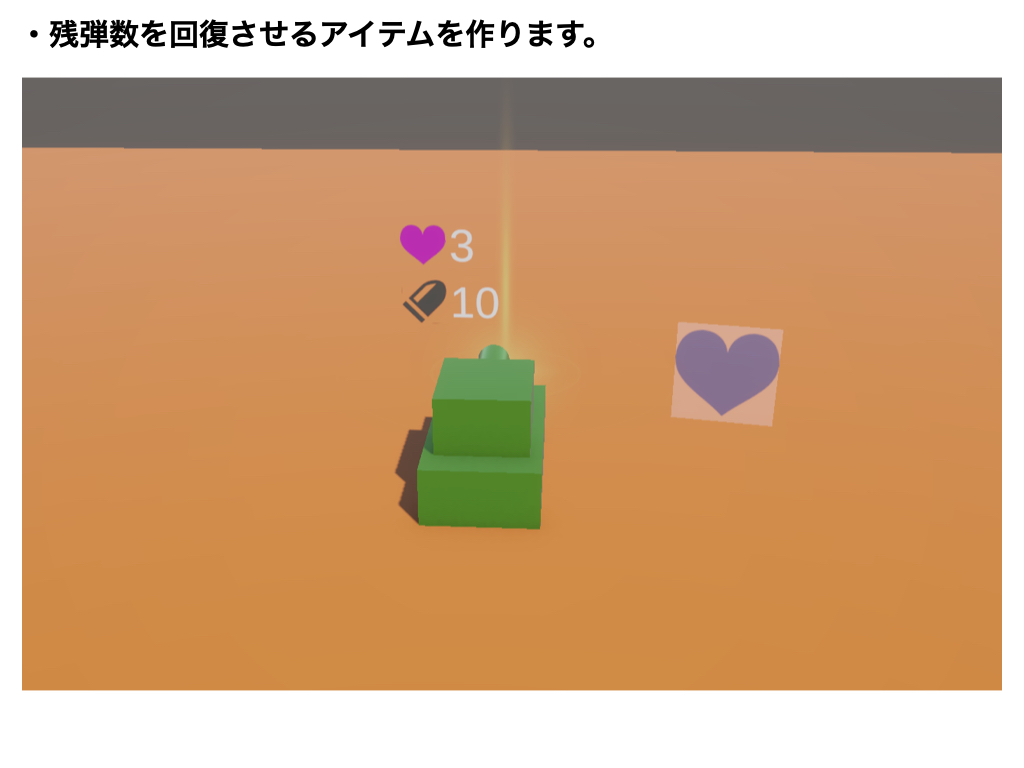
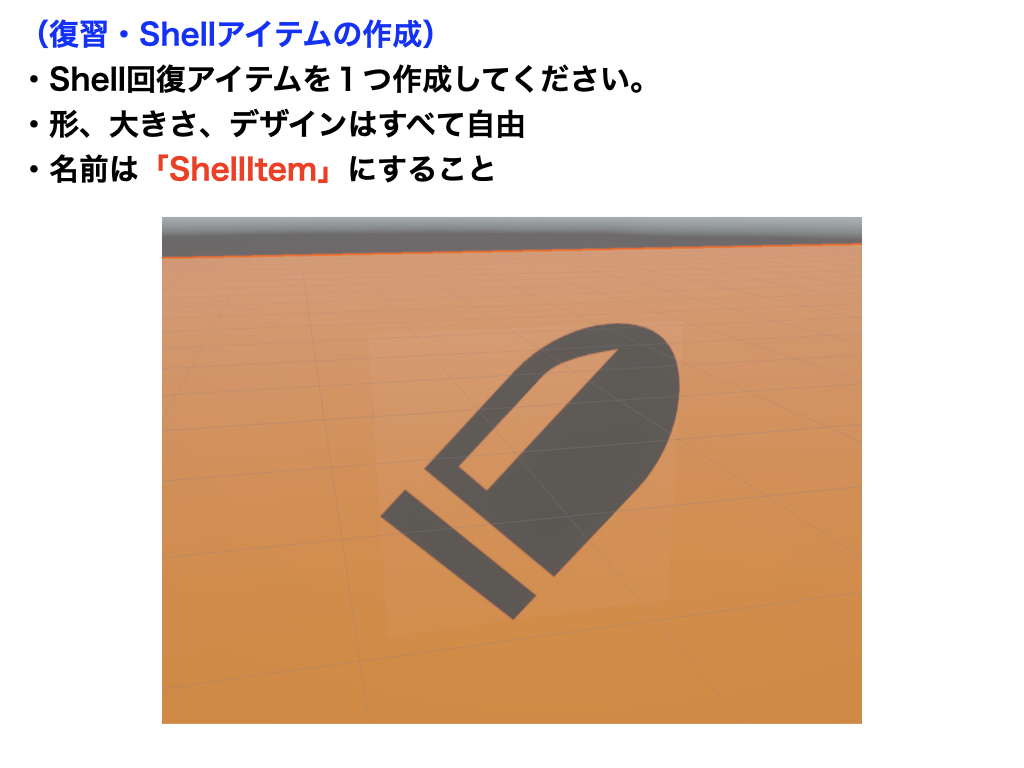
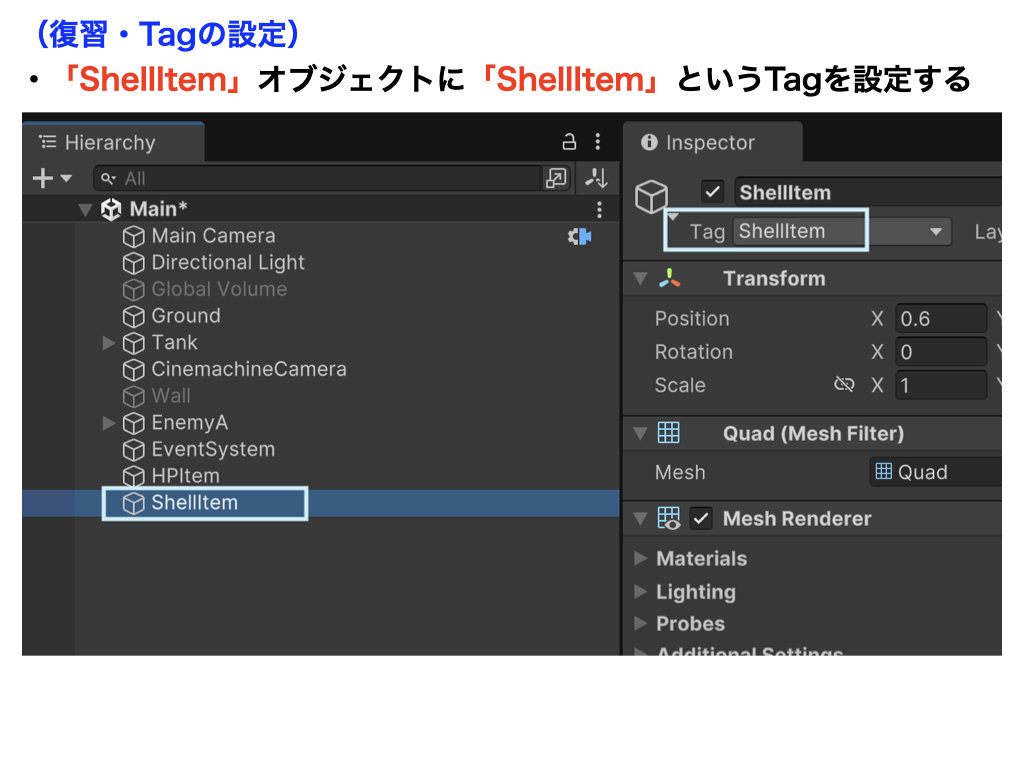
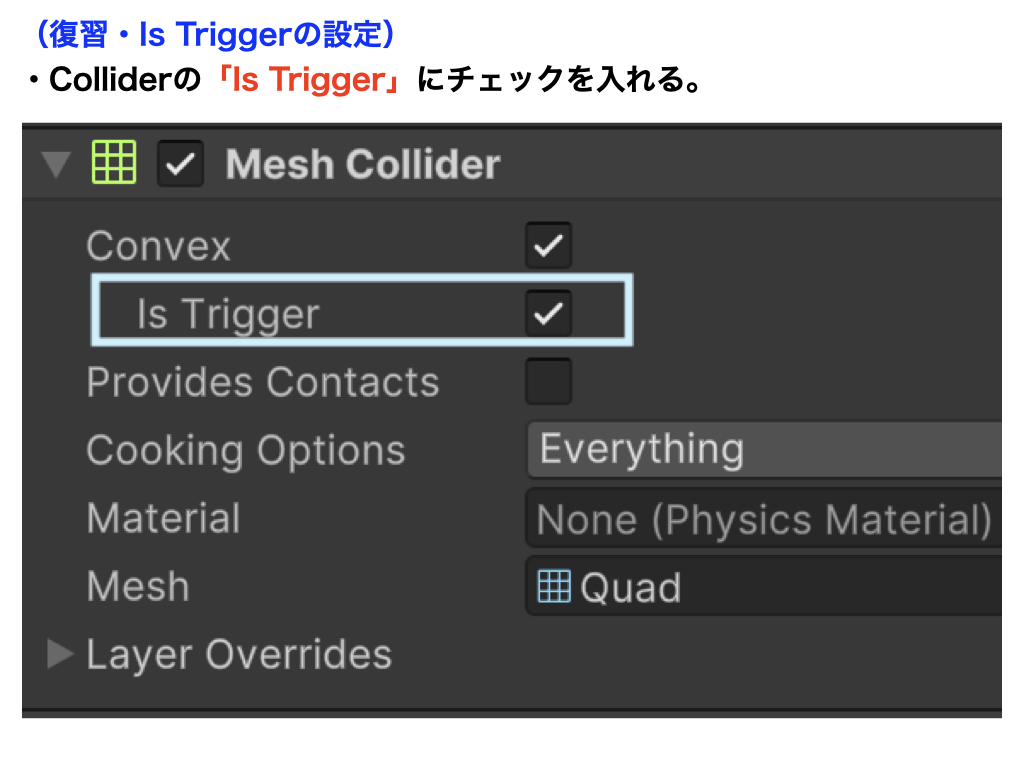
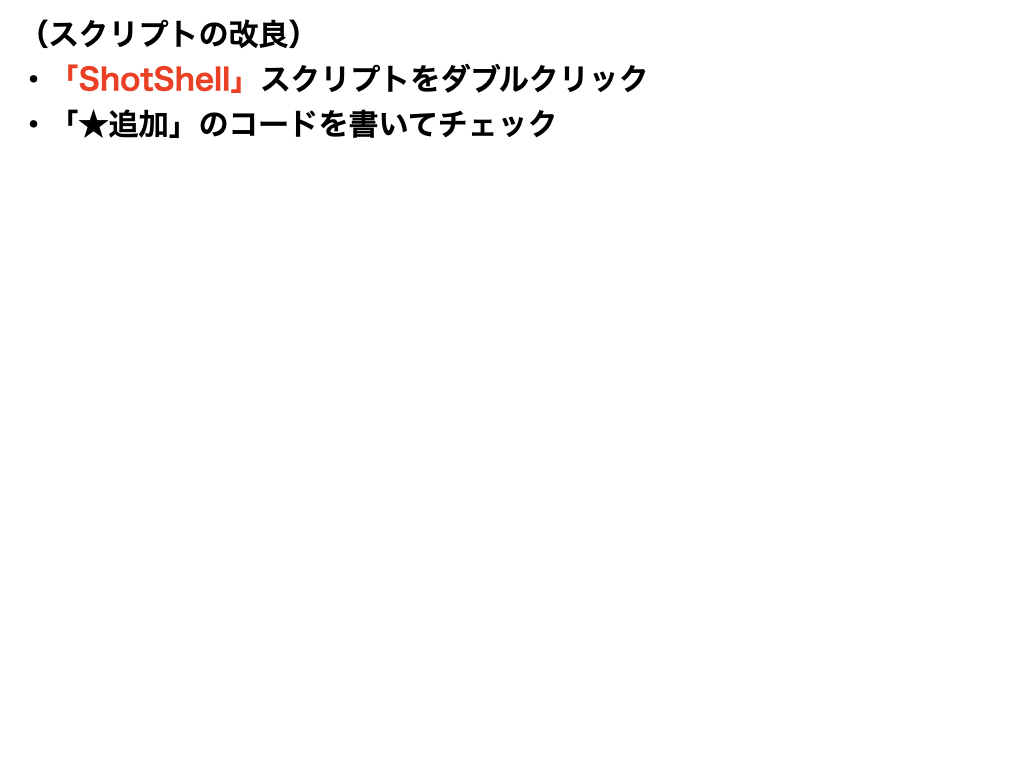
残弾数の回復アイテム
using UnityEngine;
using TMPro;
public class ShotShell : MonoBehaviour
{
private InputSystem_Actions isa;
public GameObject shellPrefab;
public AudioClip shotSound;
public float shotSpeed;
private float shotPower;
public GameObject shotPoint;
public int shellCount;
public TextMeshProUGUI shellLabel;
// ★追加
private int maxShell = 20; // 最大弾数
public AudioClip itemSound;
public GameObject effectPrefab;
void Start()
{
isa = new InputSystem_Actions();
isa.Enable();
shellLabel.text = "" + shellCount;
}
void Update()
{
if (shellCount < 1)
{
return;
}
if (isa.Player.Shot.triggered)
{
shellCount -= 1;
shellLabel.text = "" + shellCount;
GameObject shell = Instantiate(shellPrefab, shotPoint.transform.position, Quaternion.identity);
Rigidbody shellRb = shell.GetComponent<Rigidbody>();
shellRb.AddForce(transform.forward * shotSpeed);
Destroy(shell, 3.0f);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
}
if (isa.Player.Shot2.IsPressed())
{
shotPower += 15f;
if (shotPower > 900f)
{
shotPower = 900f;
}
}
if (isa.Player.Shot2.WasReleasedThisFrame())
{
shellCount -= 1;
shellLabel.text = "" + shellCount;
GameObject shell = Instantiate(shellPrefab, shotPoint.transform.position, Quaternion.identity);
Rigidbody shellRb = shell.GetComponent<Rigidbody>();
shellRb.useGravity = true;
shellRb.AddForce((transform.forward + new Vector3(0, 0.5f, 0)) * shotPower);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
Destroy(shell, 5.0f);
shotPower = 0;
}
}
// ★追加
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("ShellItem"))
{
// アイテムを消す
Destroy(other.gameObject);
// 効果音を出す
AudioSource.PlayClipAtPoint(itemSound, transform.position);
// エフェクトを出す
GameObject effect = Instantiate(effectPrefab, other.transform.position, Quaternion.identity);
// エフェクトを消す
Destroy(effect, 1.0f);
// Shellを「5」回復させる
shellCount += 5;
shellLabel.text = "" + shellCount;
// 弾数が最大値を超えないように制限する
if (shellCount > maxShell)
{
shellCount = maxShell;
shellLabel.text = "" + shellCount;
}
}
}
private void OnDisable()
{
isa.Disable();
}
}
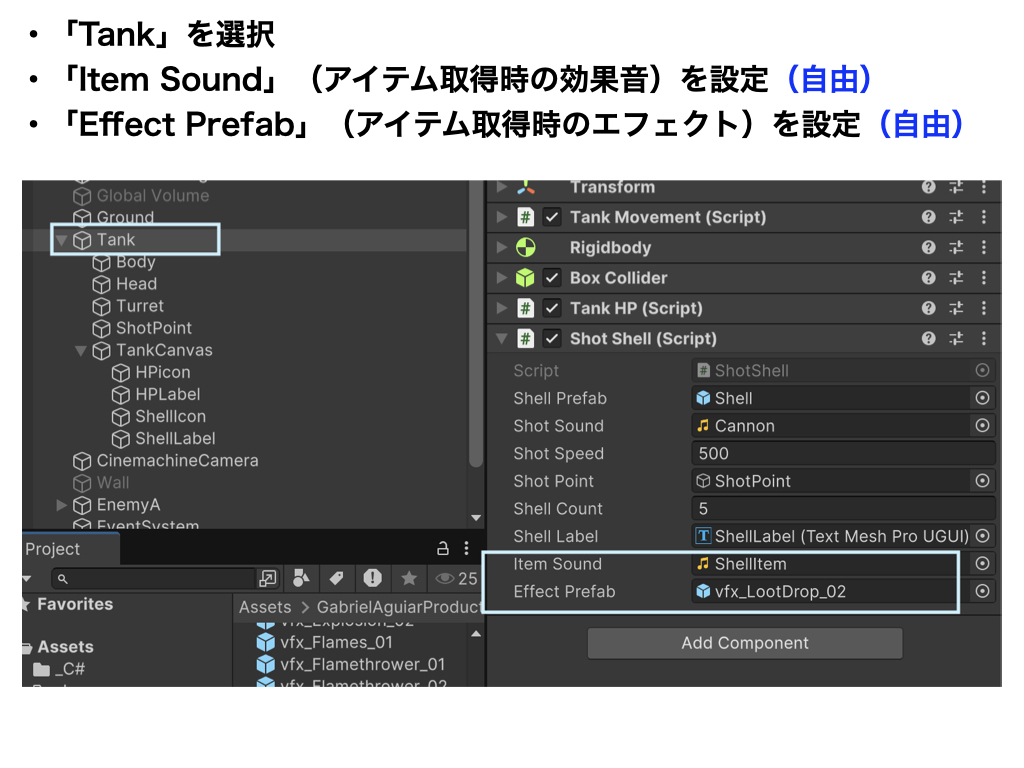
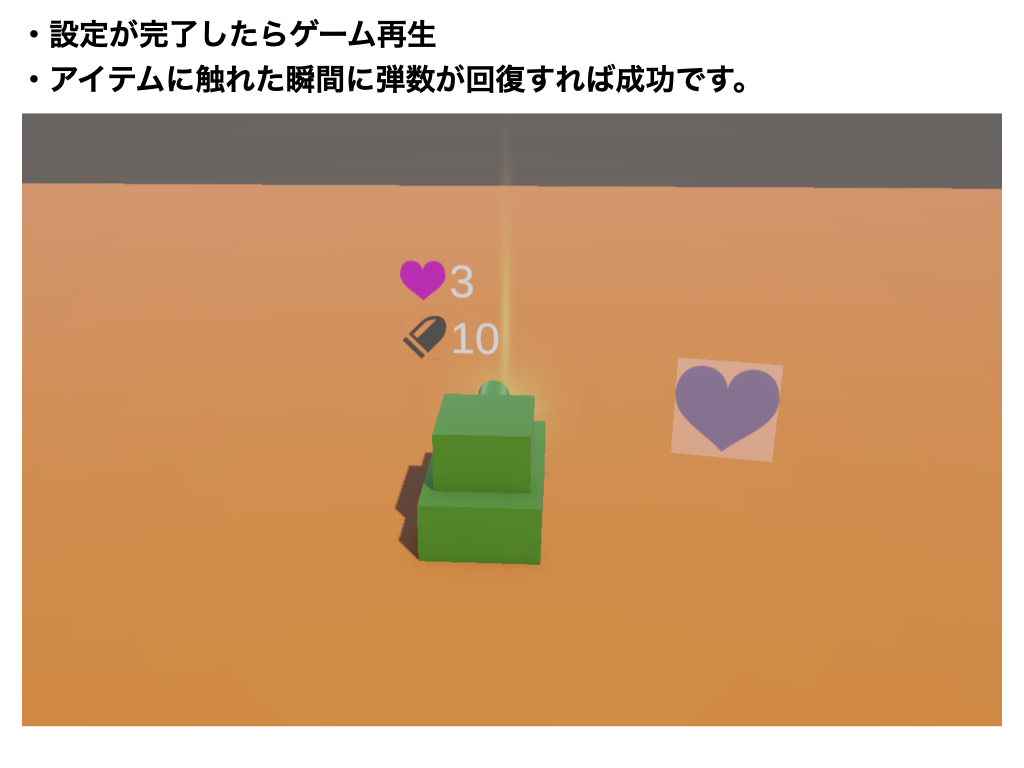
【Unity6版】BattleTank(全31回)
他のコースを見る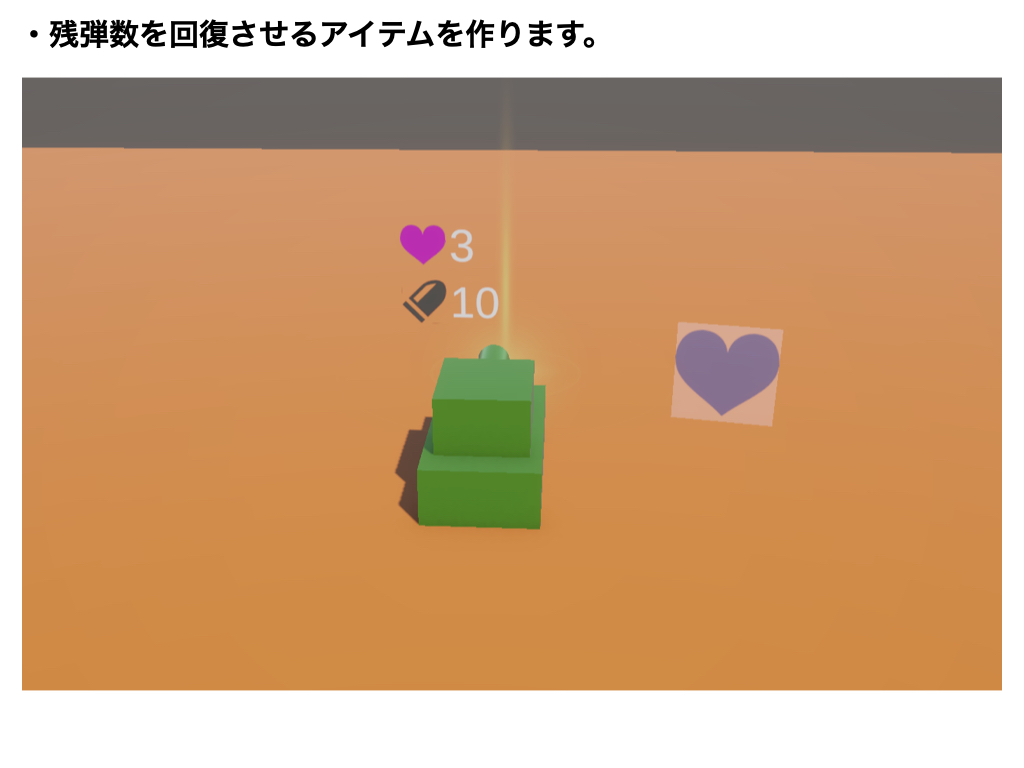
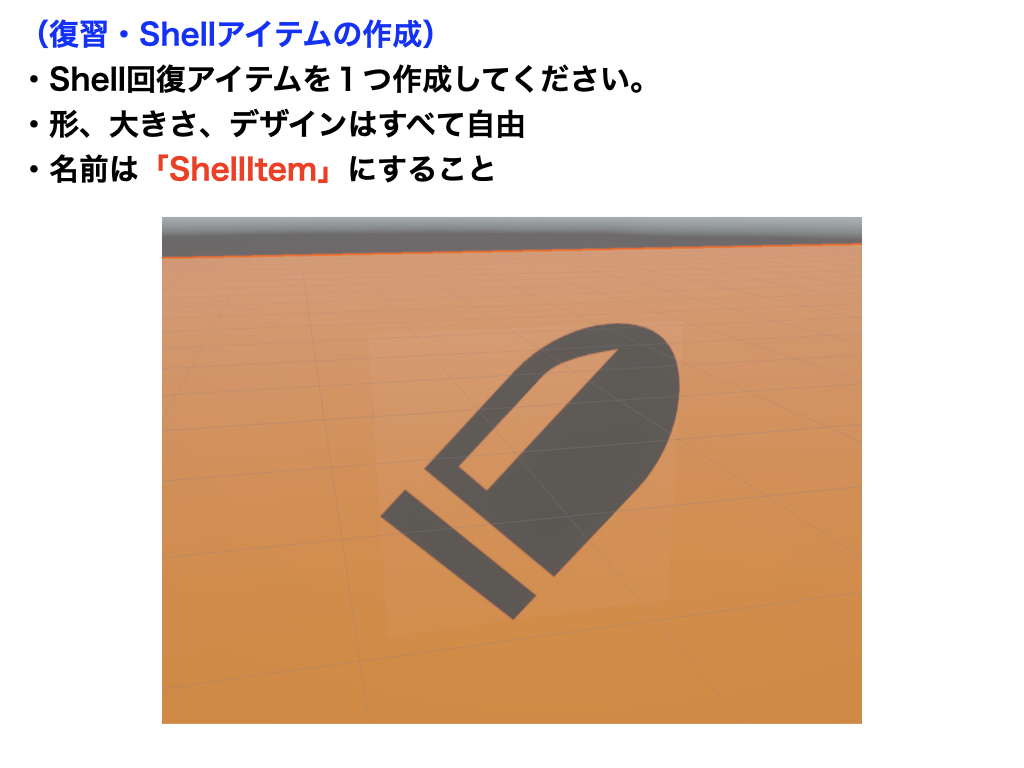
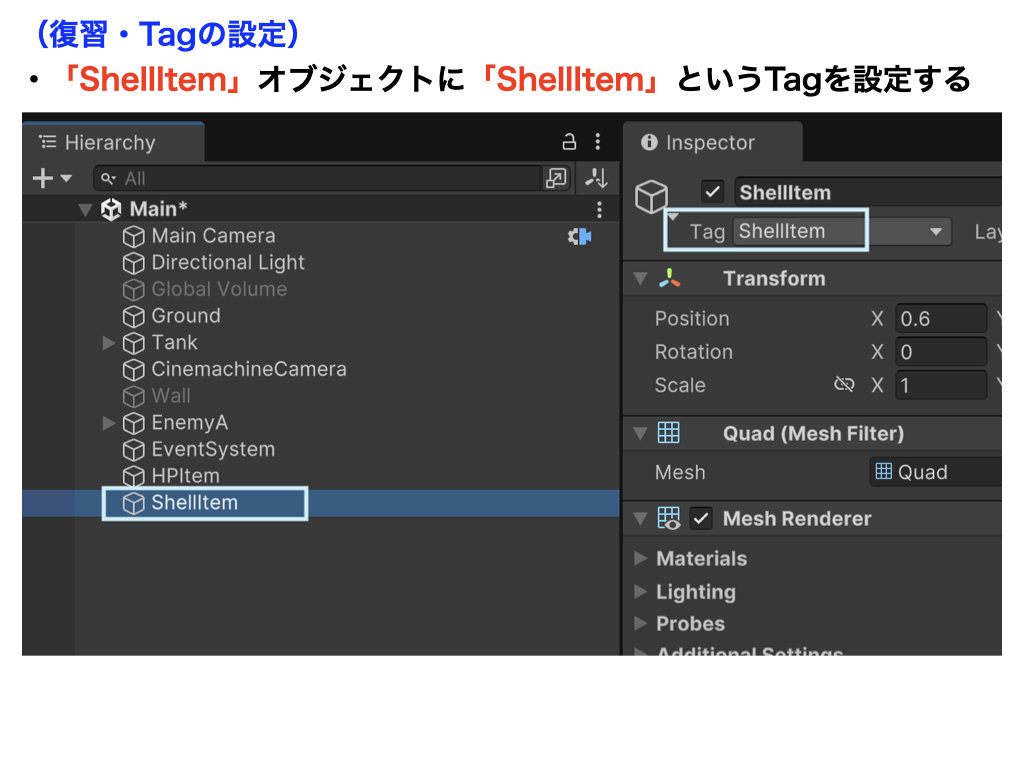
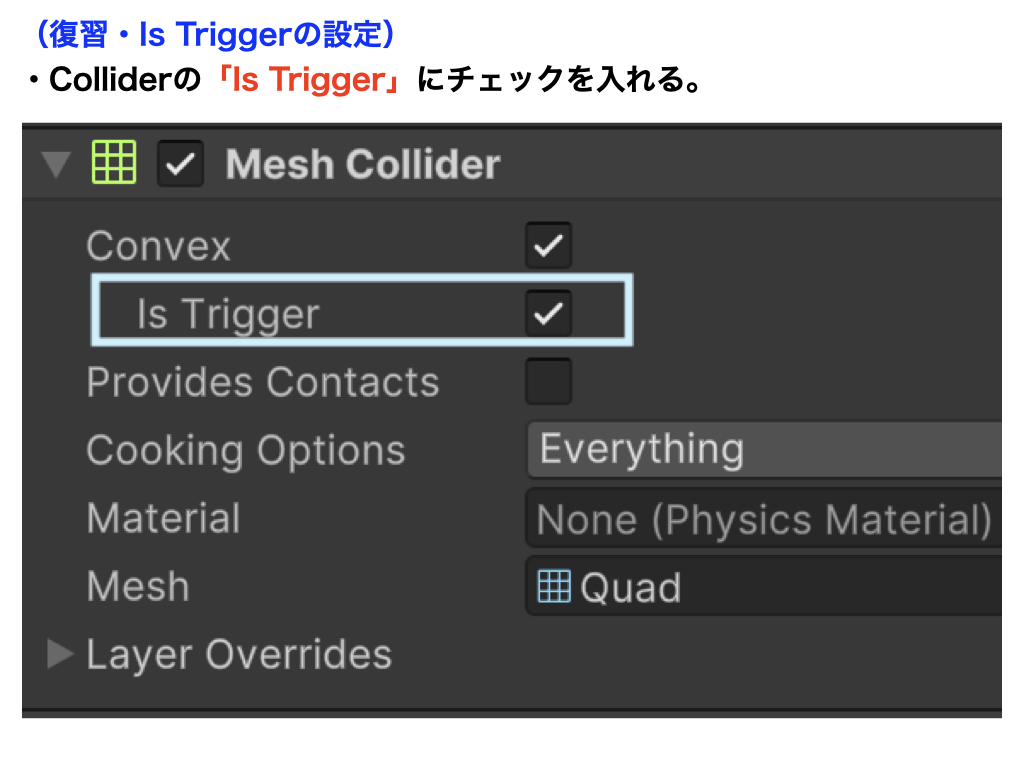
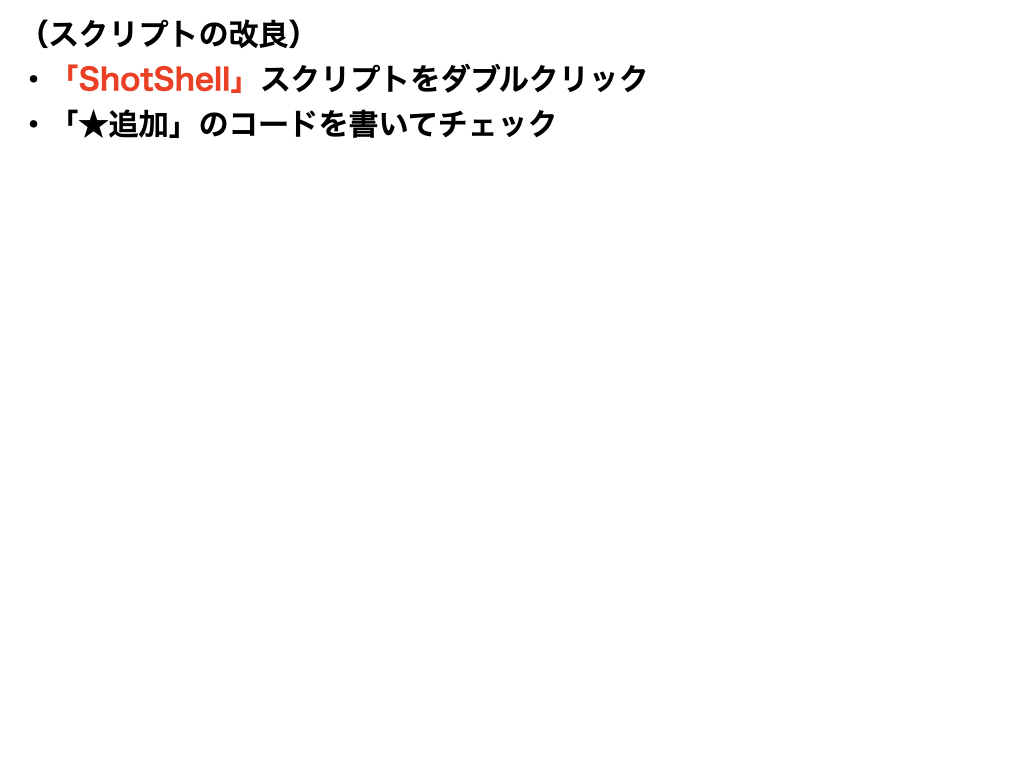
残弾数の回復アイテム
using UnityEngine;
using TMPro;
public class ShotShell : MonoBehaviour
{
private InputSystem_Actions isa;
public GameObject shellPrefab;
public AudioClip shotSound;
public float shotSpeed;
private float shotPower;
public GameObject shotPoint;
public int shellCount;
public TextMeshProUGUI shellLabel;
// ★追加
private int maxShell = 20; // 最大弾数
public AudioClip itemSound;
public GameObject effectPrefab;
void Start()
{
isa = new InputSystem_Actions();
isa.Enable();
shellLabel.text = "" + shellCount;
}
void Update()
{
if (shellCount < 1)
{
return;
}
if (isa.Player.Shot.triggered)
{
shellCount -= 1;
shellLabel.text = "" + shellCount;
GameObject shell = Instantiate(shellPrefab, shotPoint.transform.position, Quaternion.identity);
Rigidbody shellRb = shell.GetComponent<Rigidbody>();
shellRb.AddForce(transform.forward * shotSpeed);
Destroy(shell, 3.0f);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
}
if (isa.Player.Shot2.IsPressed())
{
shotPower += 15f;
if (shotPower > 900f)
{
shotPower = 900f;
}
}
if (isa.Player.Shot2.WasReleasedThisFrame())
{
shellCount -= 1;
shellLabel.text = "" + shellCount;
GameObject shell = Instantiate(shellPrefab, shotPoint.transform.position, Quaternion.identity);
Rigidbody shellRb = shell.GetComponent<Rigidbody>();
shellRb.useGravity = true;
shellRb.AddForce((transform.forward + new Vector3(0, 0.5f, 0)) * shotPower);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
Destroy(shell, 5.0f);
shotPower = 0;
}
}
// ★追加
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("ShellItem"))
{
// アイテムを消す
Destroy(other.gameObject);
// 効果音を出す
AudioSource.PlayClipAtPoint(itemSound, transform.position);
// エフェクトを出す
GameObject effect = Instantiate(effectPrefab, other.transform.position, Quaternion.identity);
// エフェクトを消す
Destroy(effect, 1.0f);
// Shellを「5」回復させる
shellCount += 5;
shellLabel.text = "" + shellCount;
// 弾数が最大値を超えないように制限する
if (shellCount > maxShell)
{
shellCount = maxShell;
shellLabel.text = "" + shellCount;
}
}
}
private void OnDisable()
{
isa.Disable();
}
}
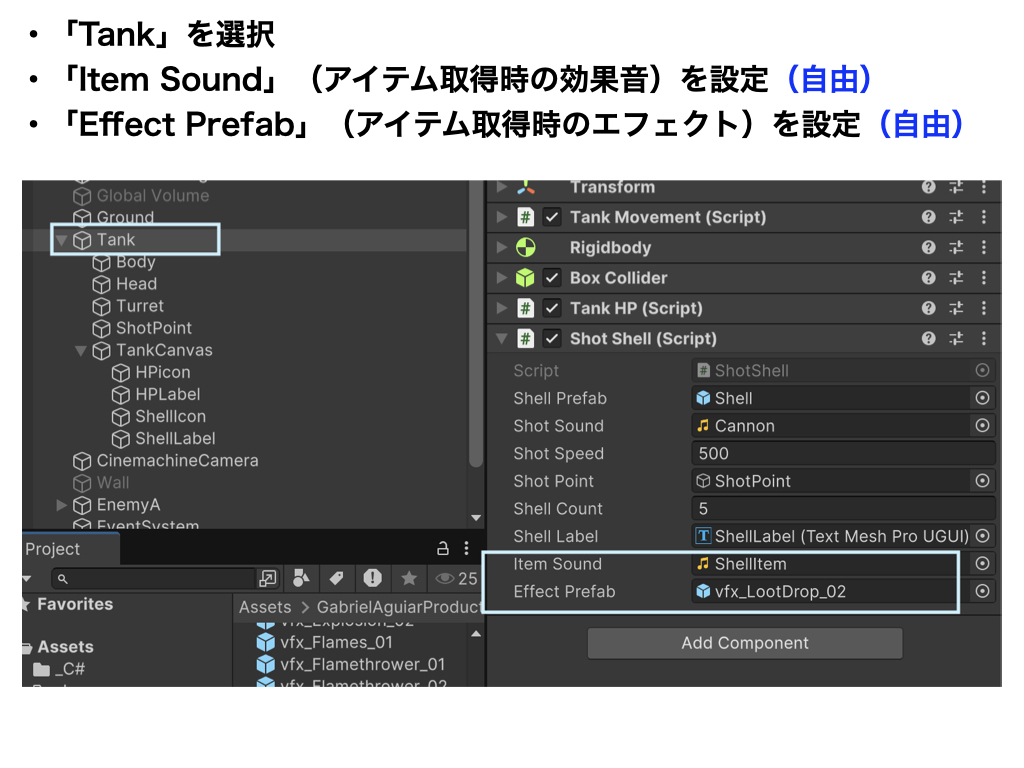
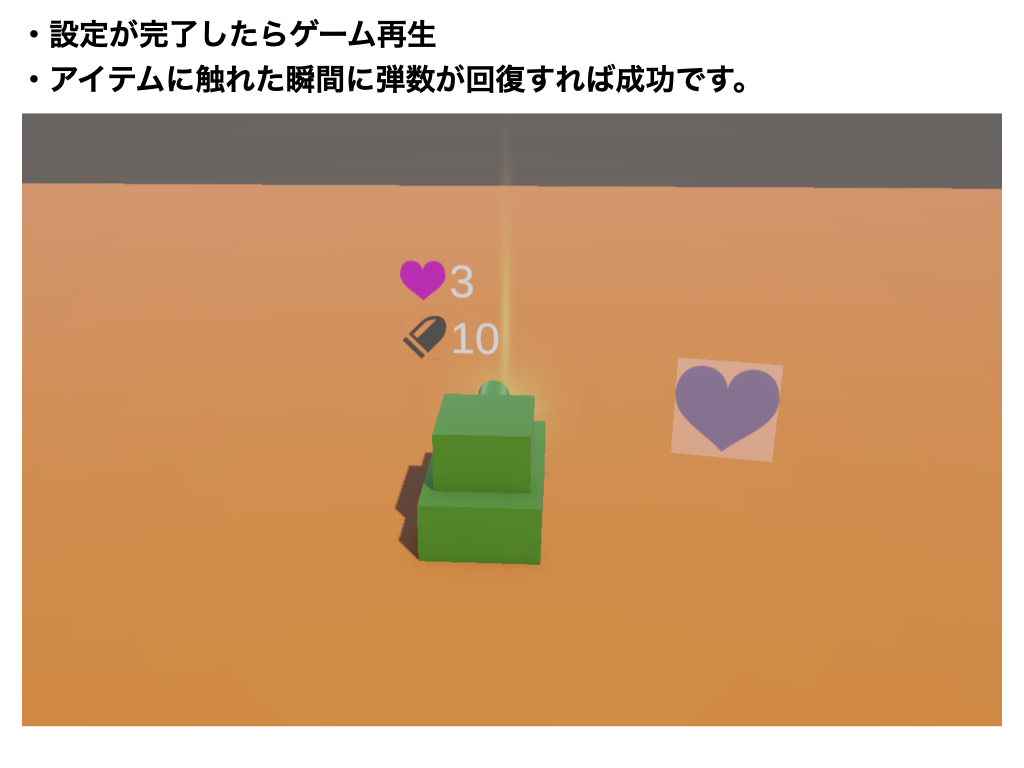
残弾数を回復させるアイテム