アイテムの作成⑤(連射能力のアップ)
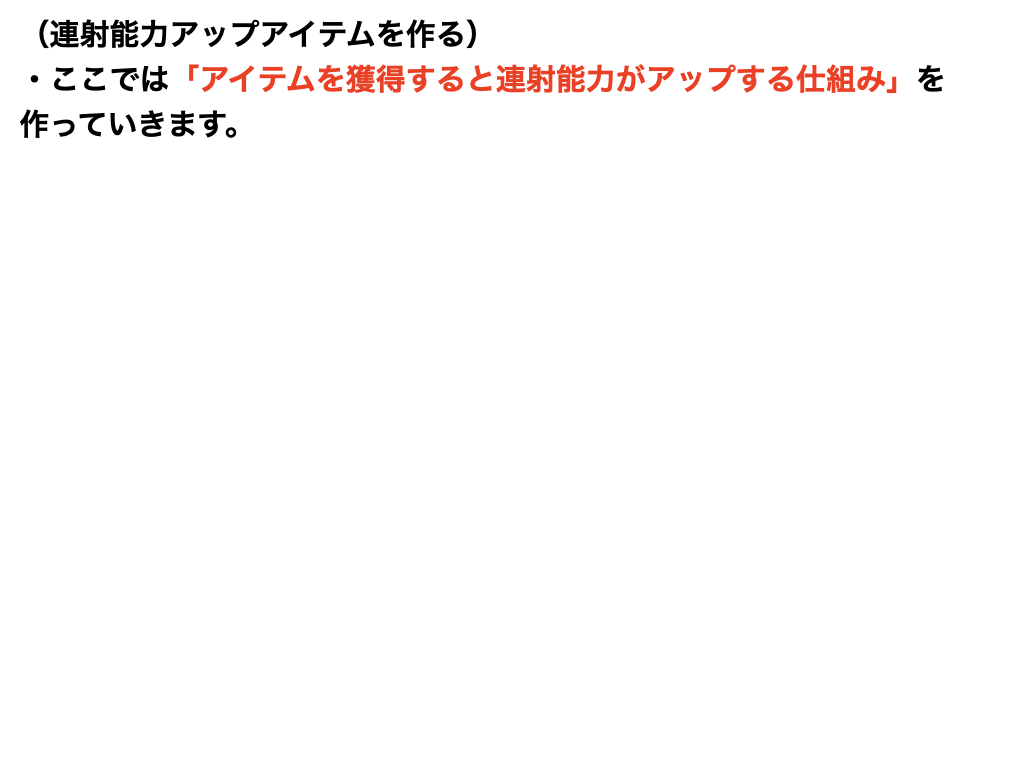
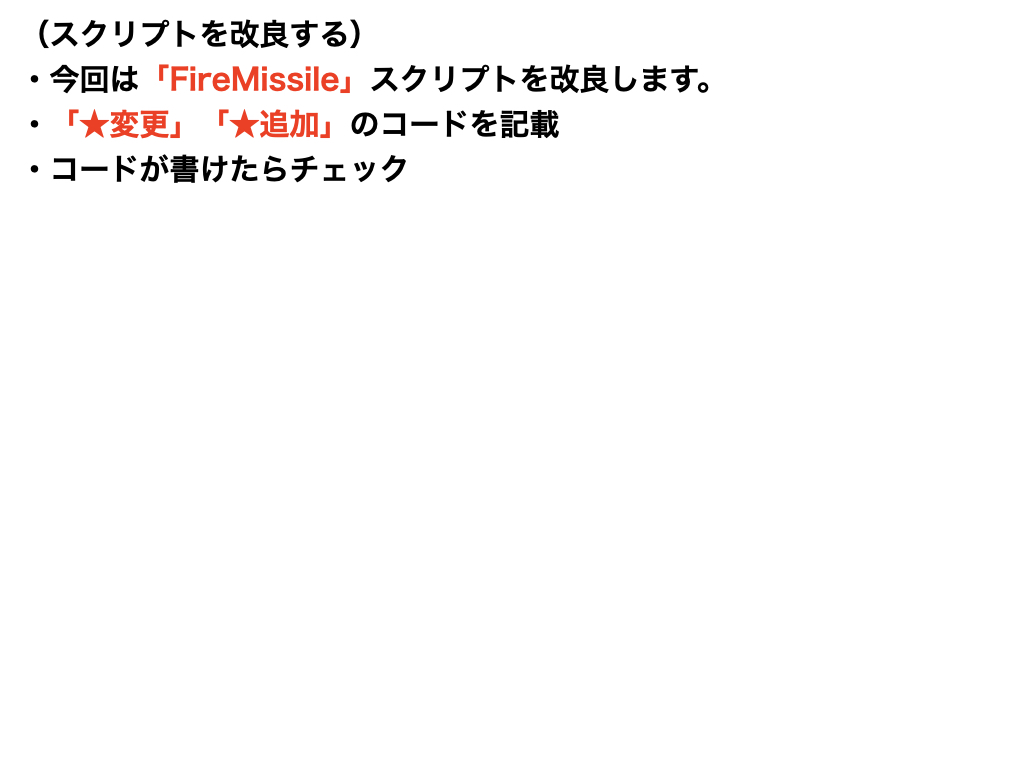
連射能力アップ
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FireMissile : MonoBehaviour
{
public GameObject missilePrefab;
private float speed = 300;
public AudioClip sound;
private int count;
// ★追加
// 初期のショット間隔は大きくすること
private int shotInterval = 30;
void FixedUpdate()
{
if (Input.GetKey(KeyCode.Space))
{
count += 1;
// ★変更
if (count % shotInterval == 0)
{
GameObject missile = Instantiate(missilePrefab, transform.position, Quaternion.identity);
Rigidbody missileRb = missile.GetComponent<Rigidbody>();
missileRb.AddForce(transform.forward * speed);
AudioSource.PlayClipAtPoint(sound, Camera.main.transform.position);
Destroy(missile, 2.0f);
}
}
}
public void AddShotSpeed(int amount)
{
speed += amount;
if (speed > 1000)
{
speed = 1000;
}
}
// ★追加
public void ReduceInterval(int amount)
{
shotInterval -= amount;
// 下限の設定
// ショット間隔をどこまで小さくするかは自由
if(shotInterval < 5)
{
shotInterval = 5;
}
}
}
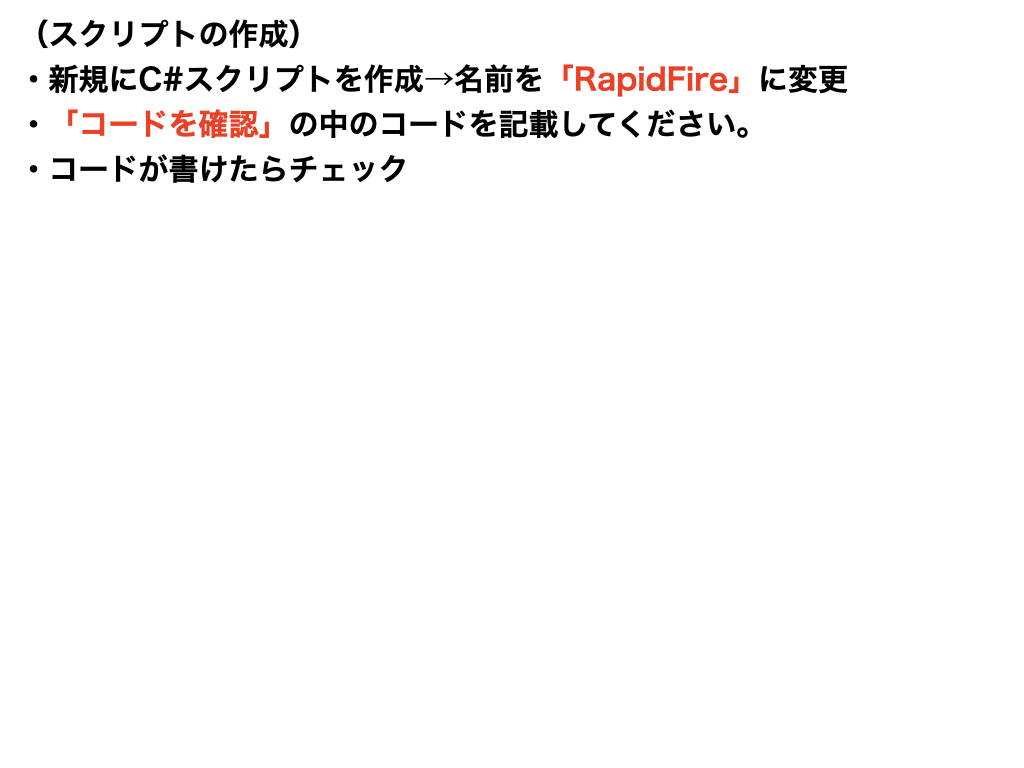
連射能力アップアイテム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RapidFire : ItemBase
{
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Player"))
{
ItemGet();
// Playerの子オブジェクトのコンポーネントを取得する(ポイント)
// 1個のアイテムゲットでどれだけショット間隔を下げるかは自由
other.GetComponentInChildren<FireMissile>().ReduceInterval(5);
}
}
}
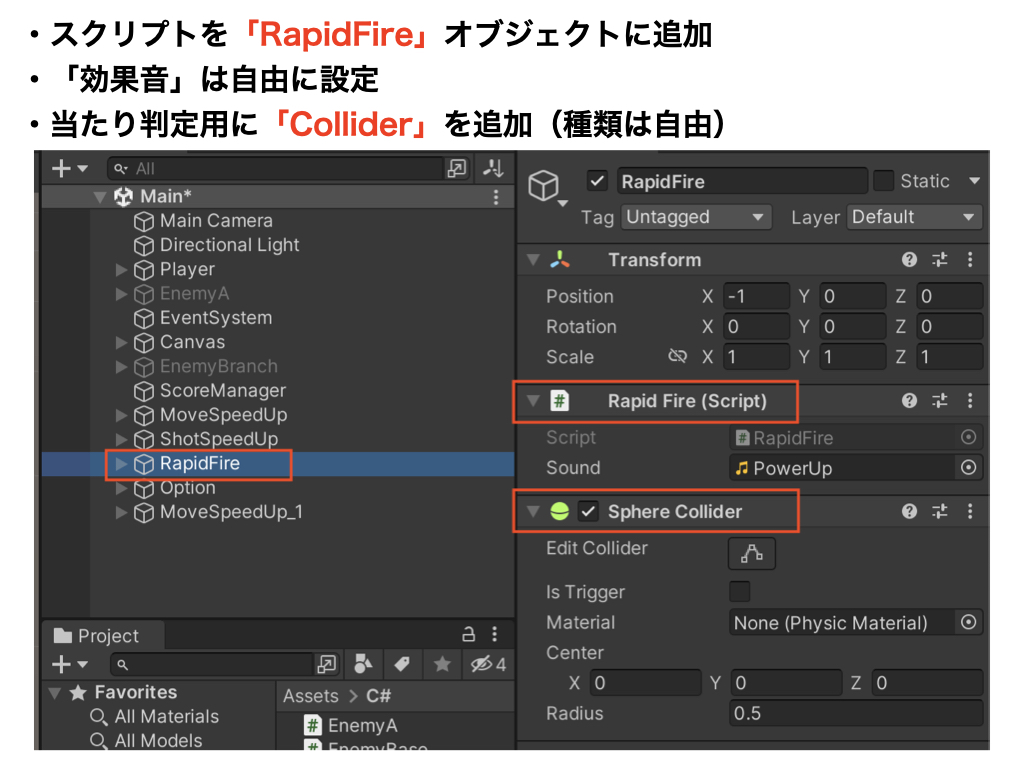
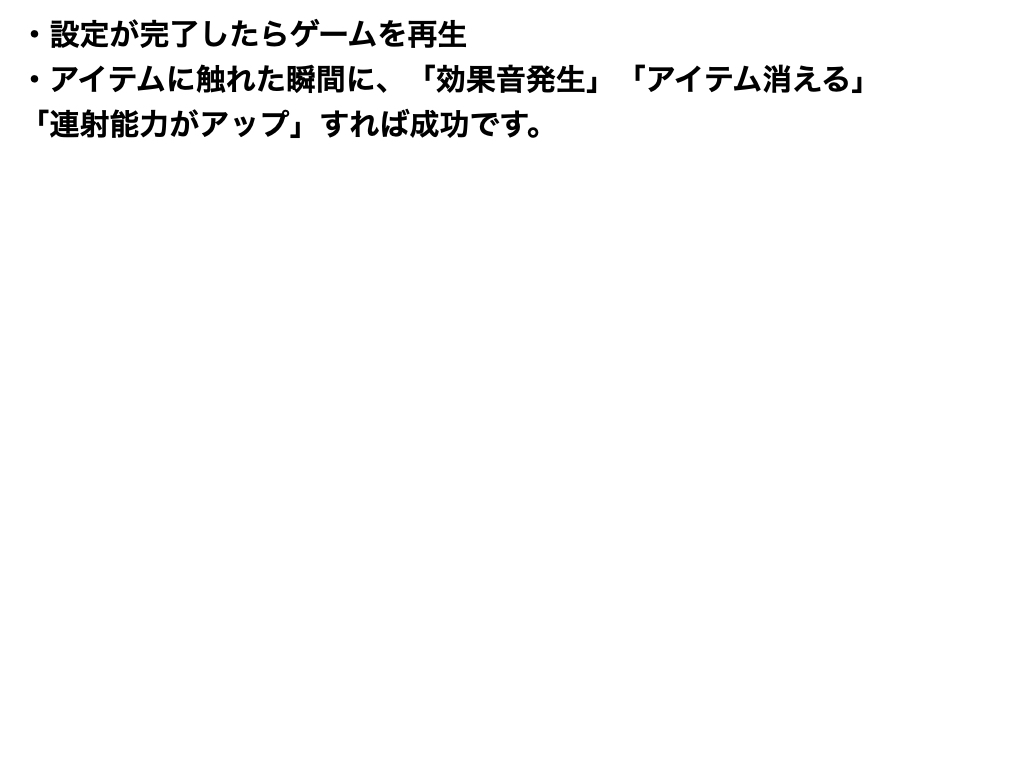
【2021版】Danmaku(基礎/全55回)
他のコースを見る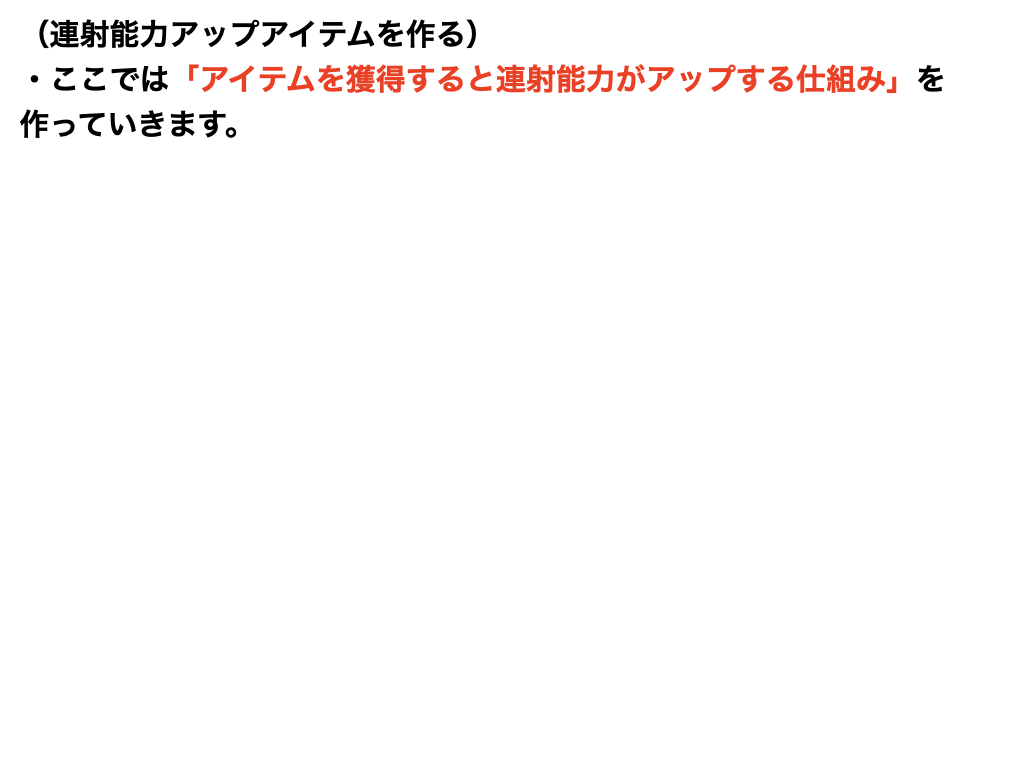
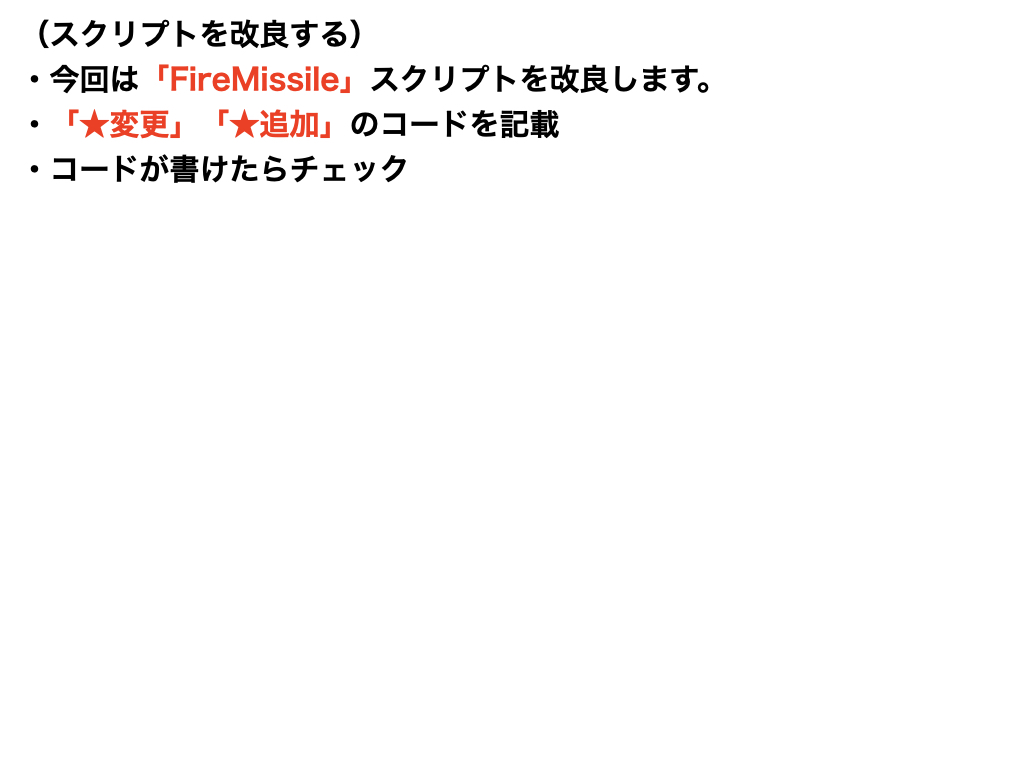
連射能力アップ
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FireMissile : MonoBehaviour
{
public GameObject missilePrefab;
private float speed = 300;
public AudioClip sound;
private int count;
// ★追加
// 初期のショット間隔は大きくすること
private int shotInterval = 30;
void FixedUpdate()
{
if (Input.GetKey(KeyCode.Space))
{
count += 1;
// ★変更
if (count % shotInterval == 0)
{
GameObject missile = Instantiate(missilePrefab, transform.position, Quaternion.identity);
Rigidbody missileRb = missile.GetComponent<Rigidbody>();
missileRb.AddForce(transform.forward * speed);
AudioSource.PlayClipAtPoint(sound, Camera.main.transform.position);
Destroy(missile, 2.0f);
}
}
}
public void AddShotSpeed(int amount)
{
speed += amount;
if (speed > 1000)
{
speed = 1000;
}
}
// ★追加
public void ReduceInterval(int amount)
{
shotInterval -= amount;
// 下限の設定
// ショット間隔をどこまで小さくするかは自由
if(shotInterval < 5)
{
shotInterval = 5;
}
}
}
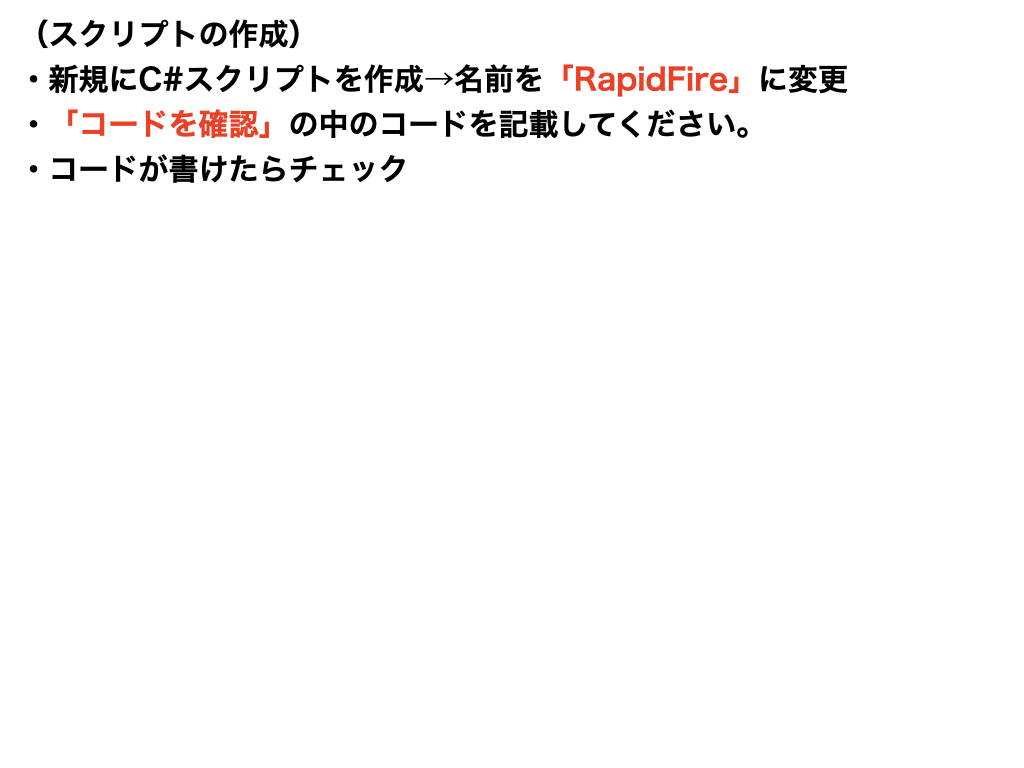
連射能力アップアイテム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RapidFire : ItemBase
{
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Player"))
{
ItemGet();
// Playerの子オブジェクトのコンポーネントを取得する(ポイント)
// 1個のアイテムゲットでどれだけショット間隔を下げるかは自由
other.GetComponentInChildren<FireMissile>().ReduceInterval(5);
}
}
}
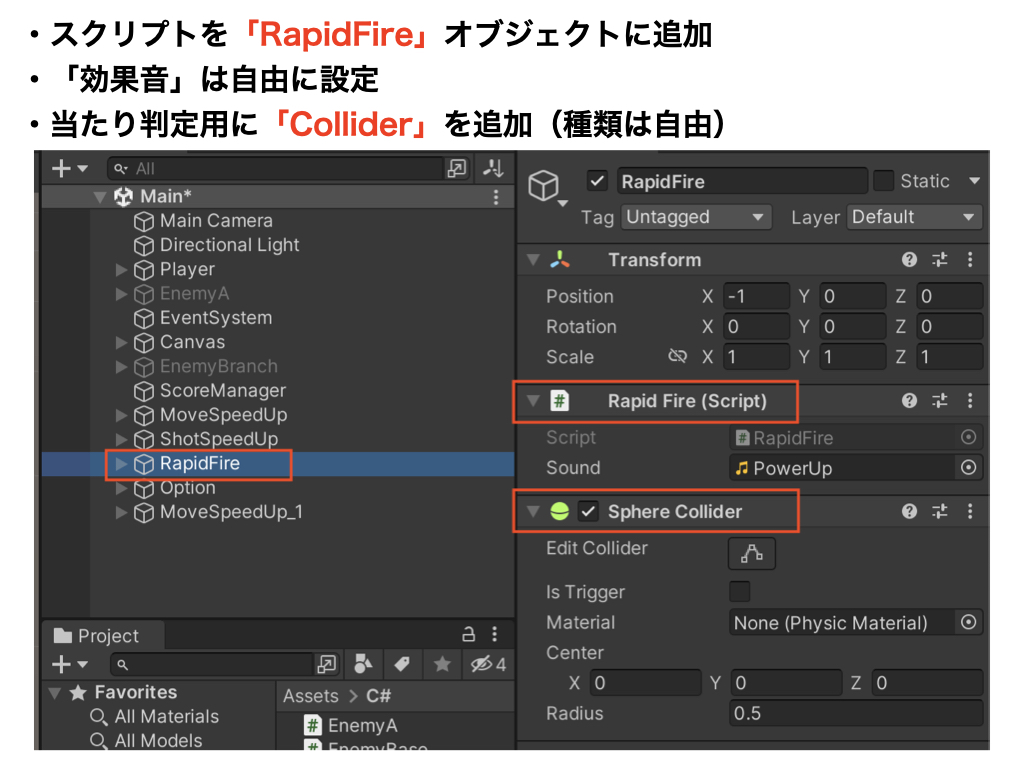
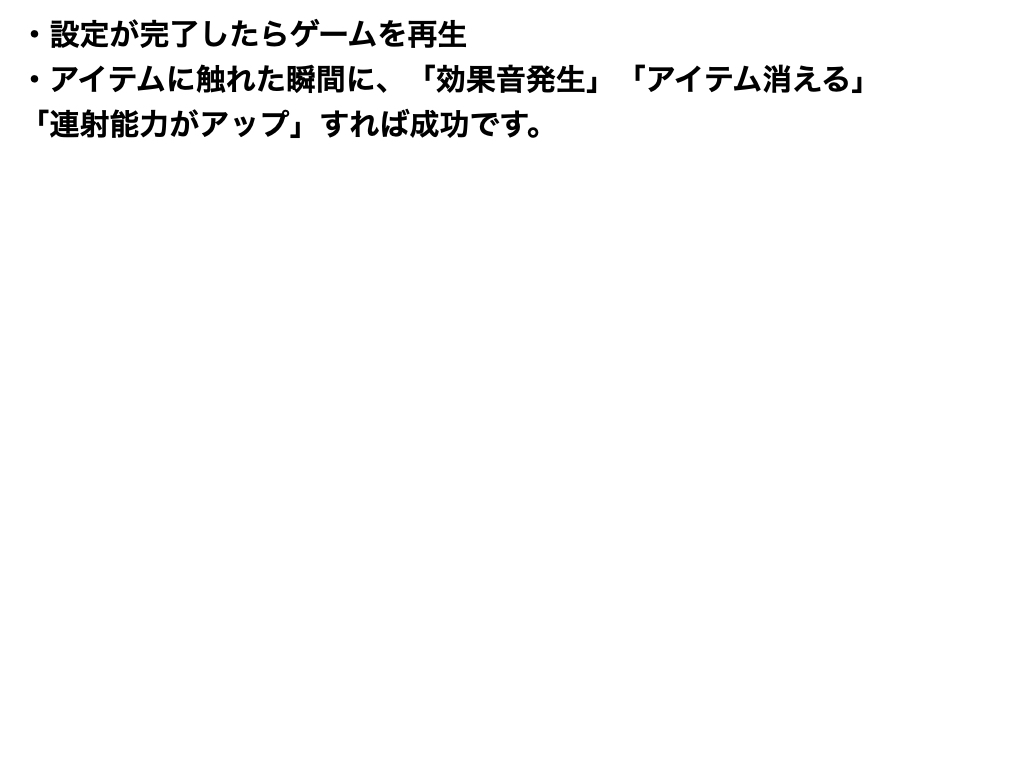
アイテムの作成⑤(連射能力のアップ)