動く敵の作成②(コルーチンで動きを作る)
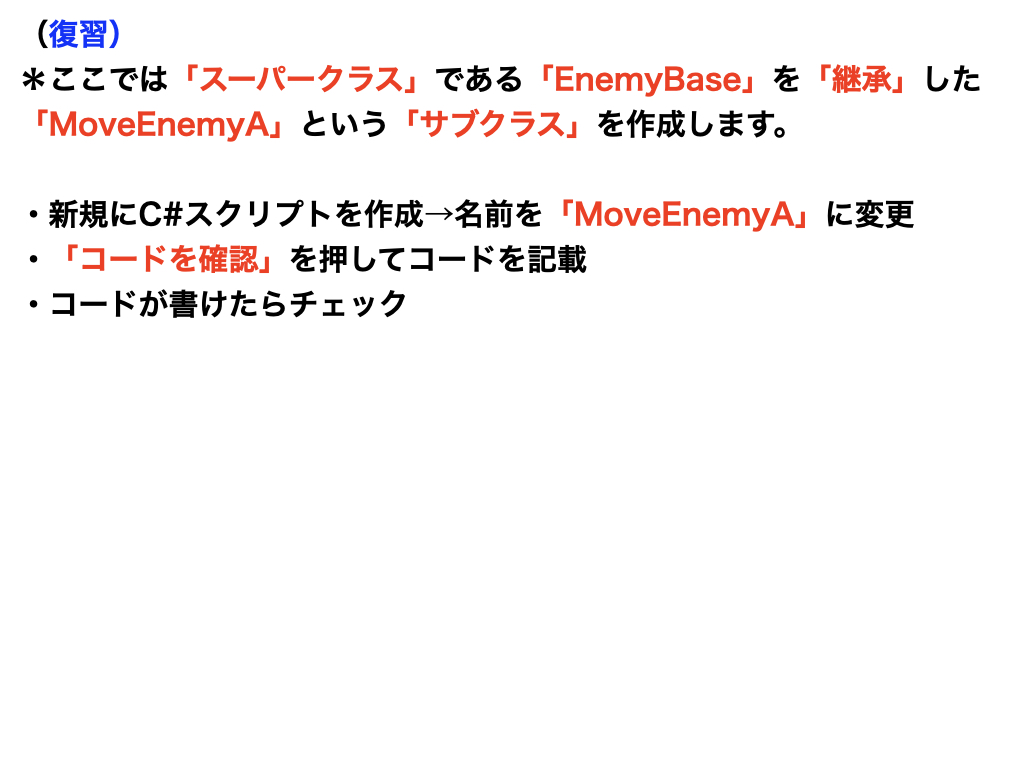
コルーチンで動く敵を作る
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MoveEnemyA : EnemyBase // 復習(クラスの継承)
{
private float x;
private float z;
private float speed;
void Start()
{
HP = 1;
// ★コルーチンの処理を開始する
StartCoroutine(MoveE());
}
void Update()
{
transform.Translate(new Vector3(x, 0, z) * speed * Time.deltaTime, Space.World);
}
// ★コルーチン(処理を途中で中断させることができる仕組み)
private IEnumerator MoveE()
{
// 初めに、speed「5」の速度で、下方向に移動する
x = 0;
z = -1;
speed = 5;
// 「3秒」経過したら、上記の処理を中断して・・・・、
yield return new WaitForSeconds(3f);
// 今度は下記の処理を行う
// speed「10」の速度で、右方向に移動する
x = 1;
z = 0;
speed = 10;
}
}
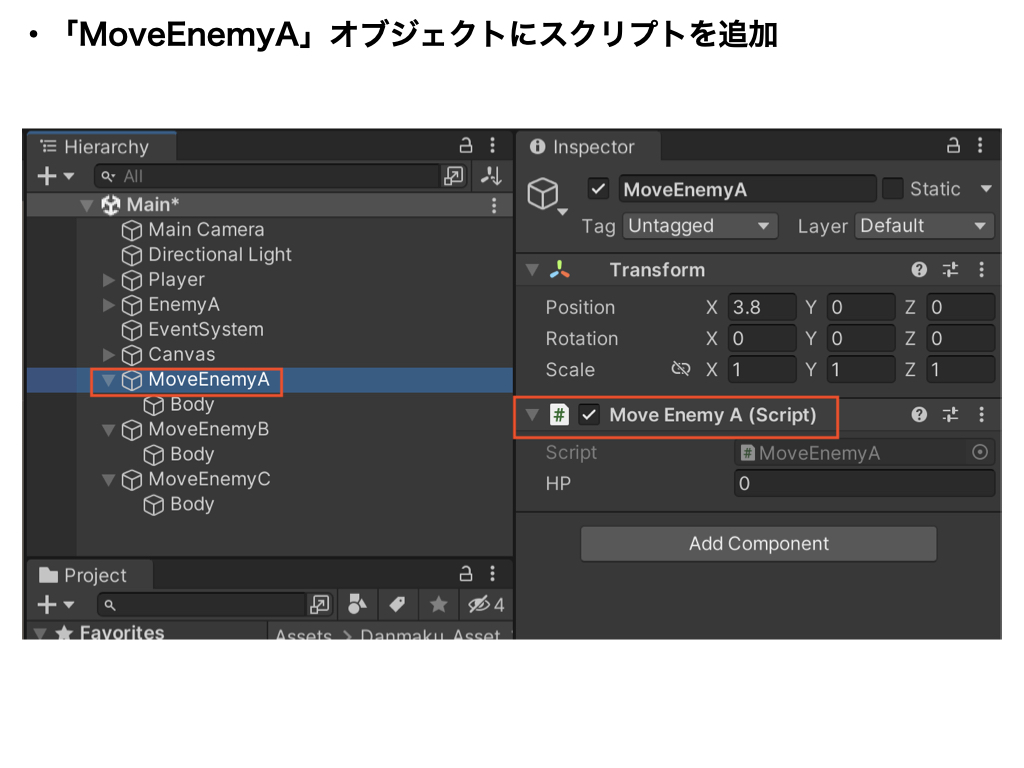
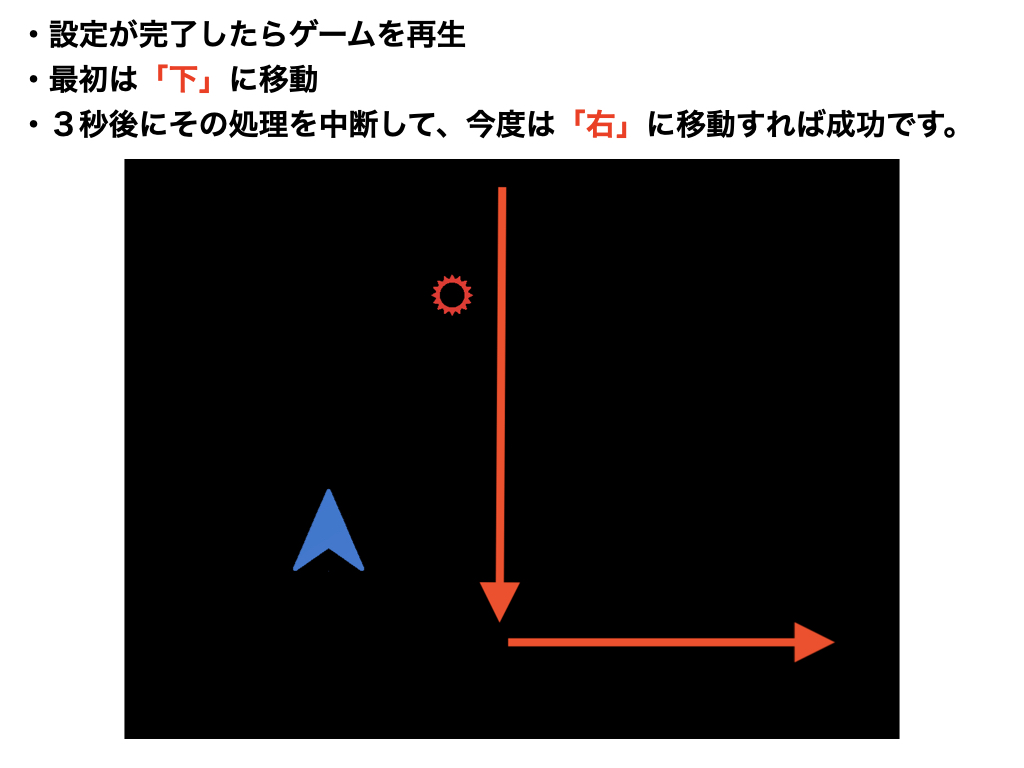
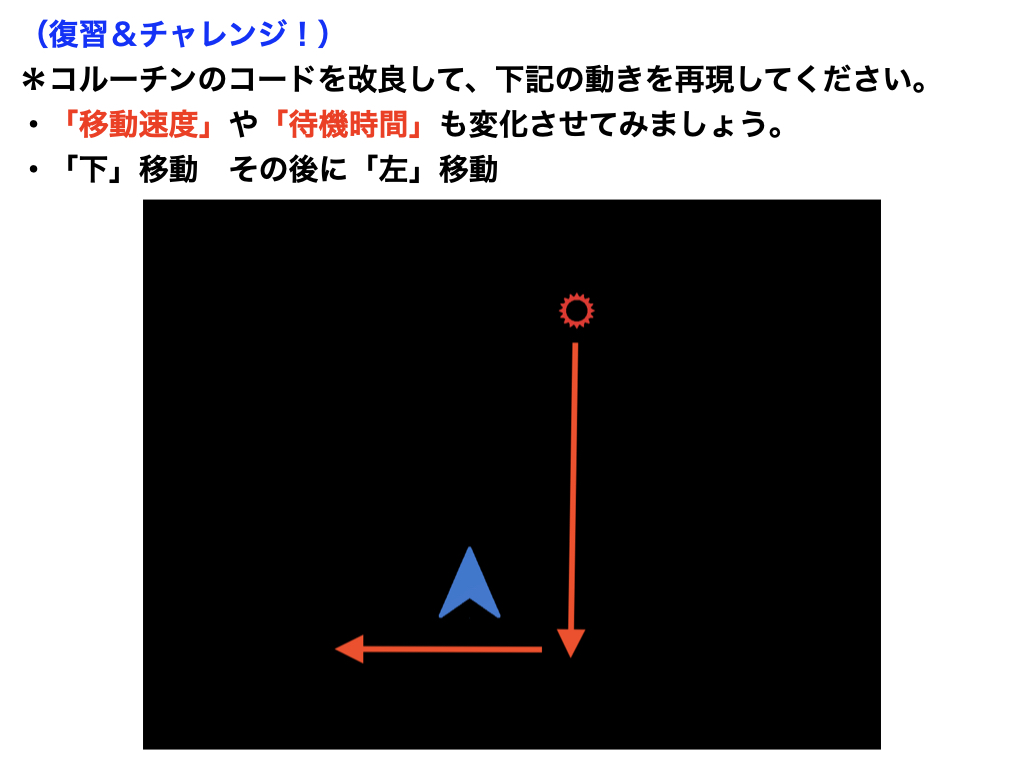
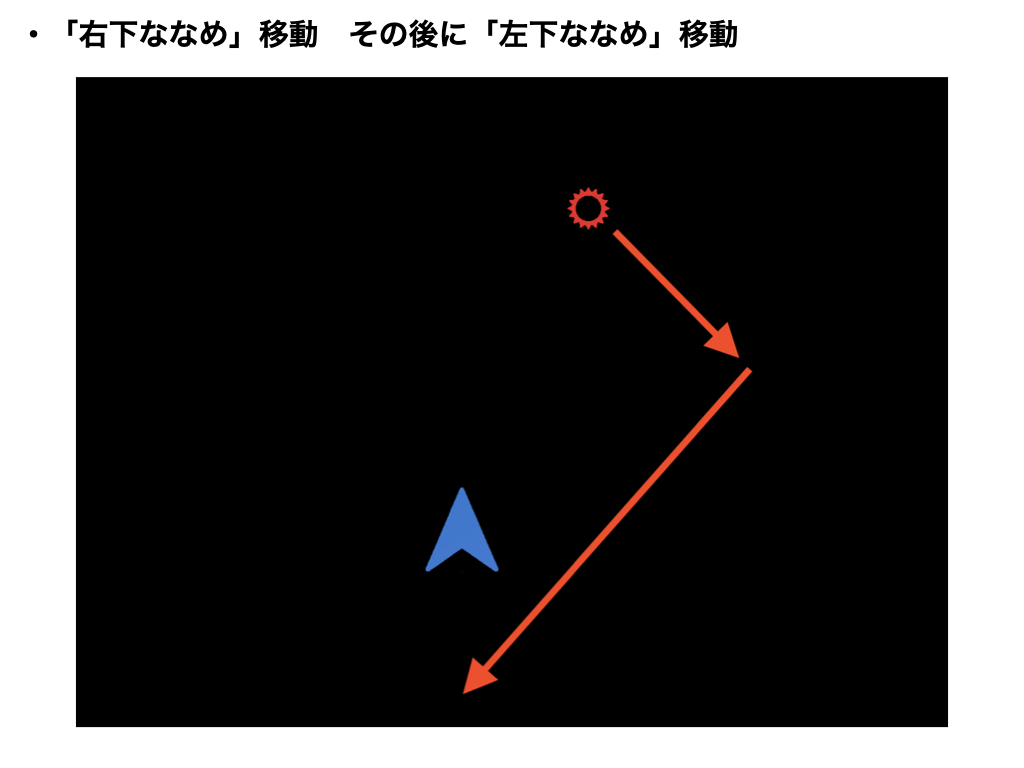
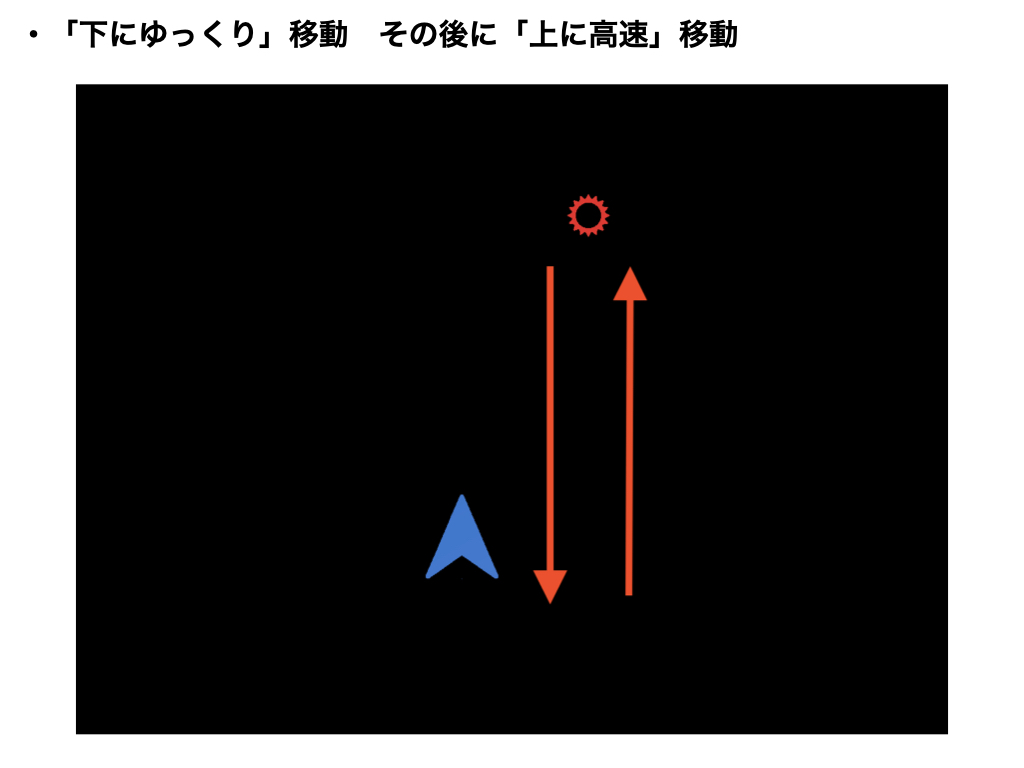
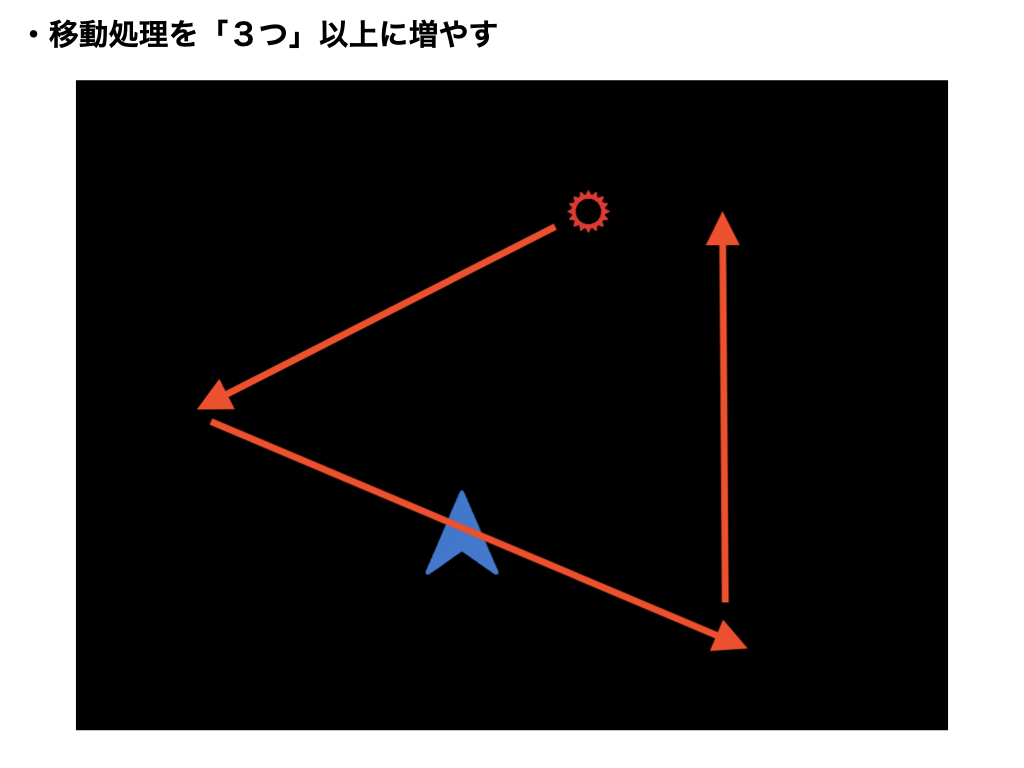
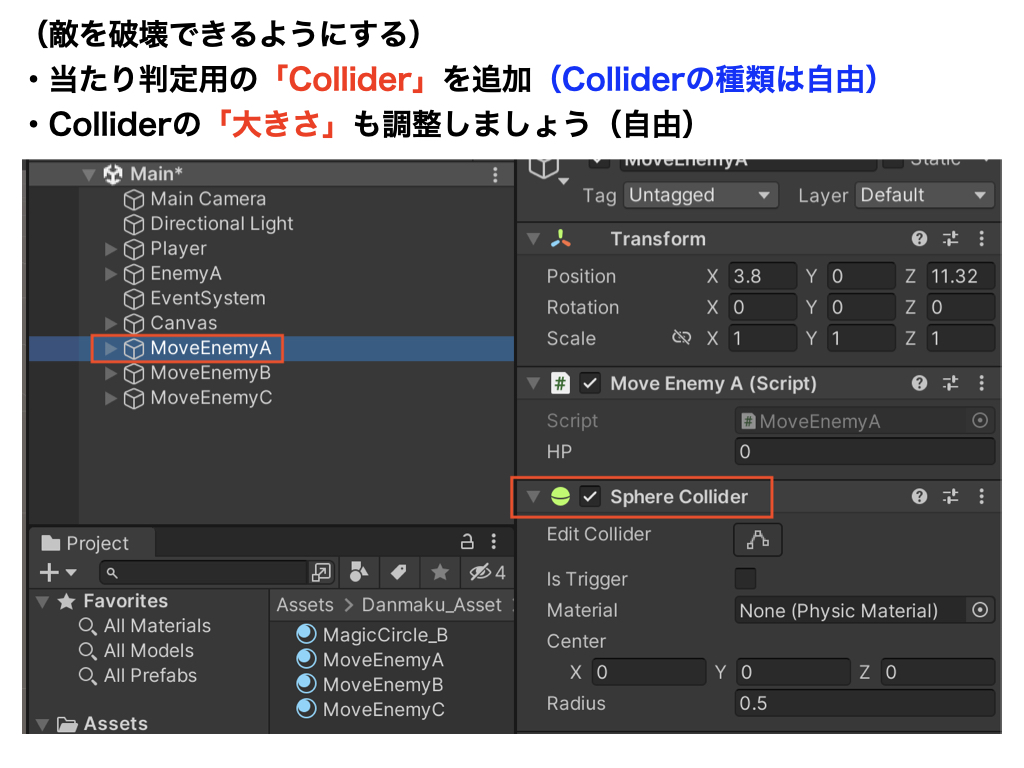
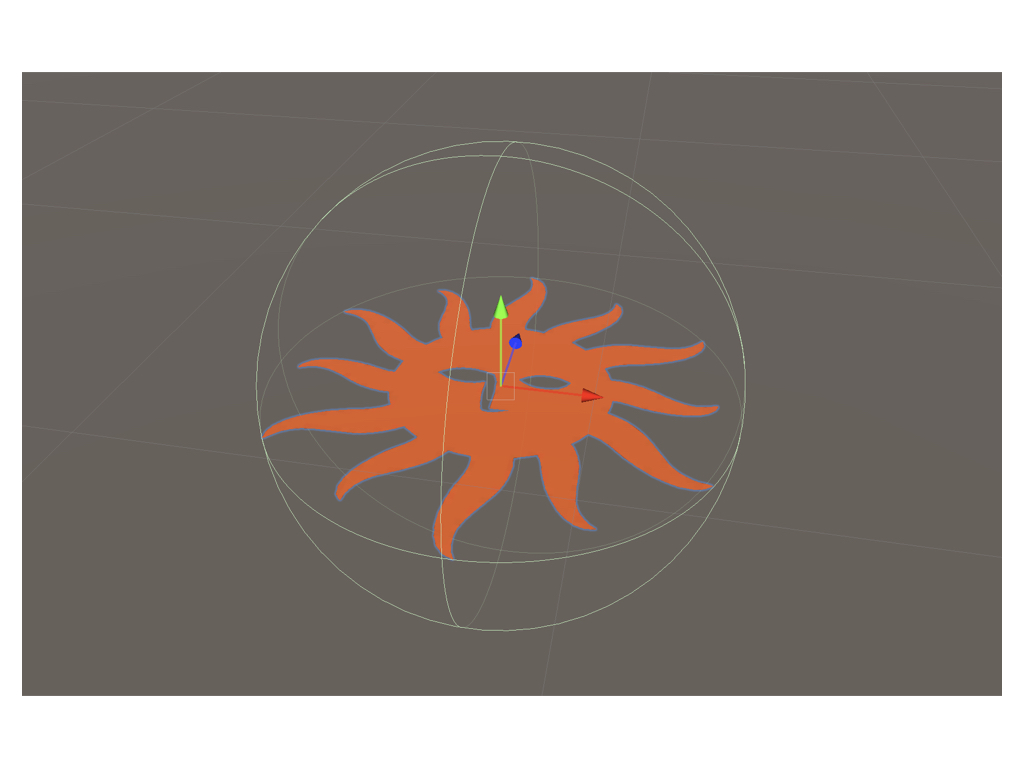
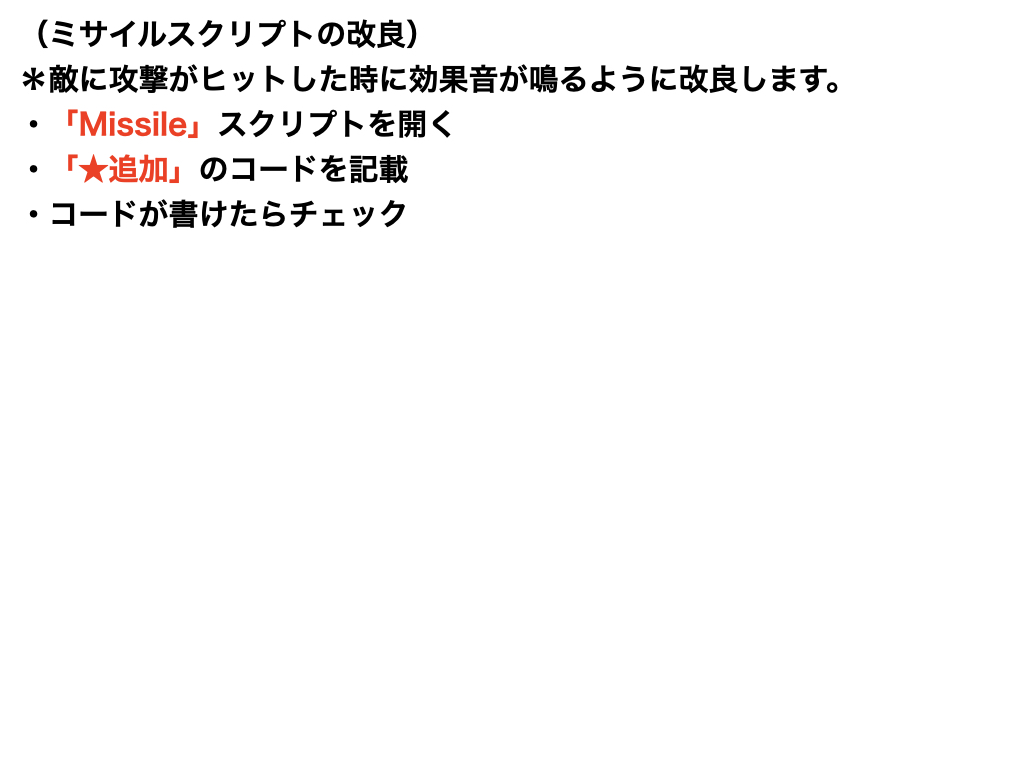
効果音の追加
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Missile : MonoBehaviour
{
public GameObject effectPrefab;
// ★追加
public AudioClip hitSound;
private void OnTriggerEnter(Collider other)
{
if (other.TryGetComponent(out EnemyBase enemy))
{
enemy.TakeDamage(1);
Destroy(gameObject);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 1.0f);
// ★追加
AudioSource.PlayClipAtPoint(hitSound, transform.position);
}
}
}
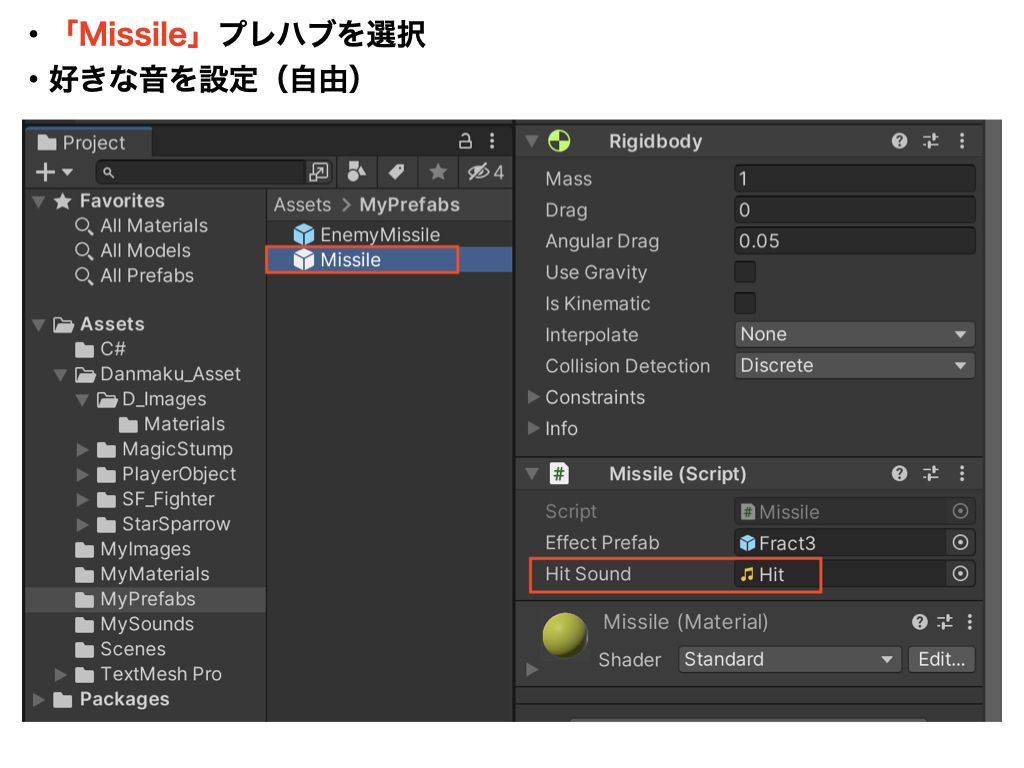
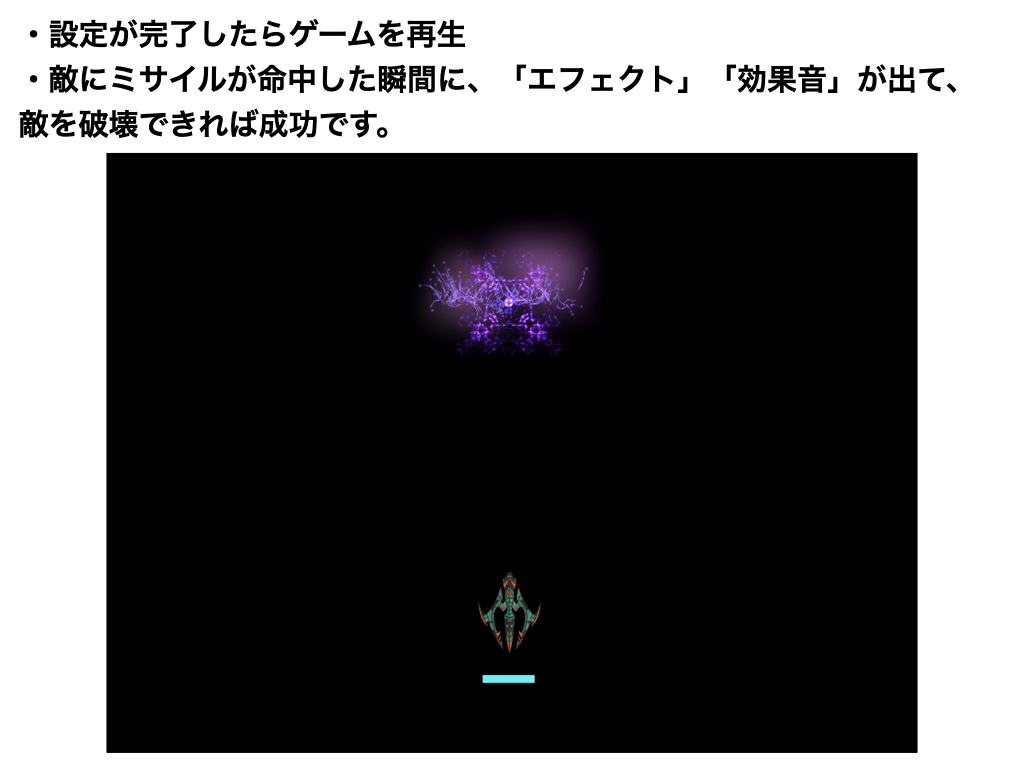
【2021版】Danmaku(基礎/全55回)
他のコースを見る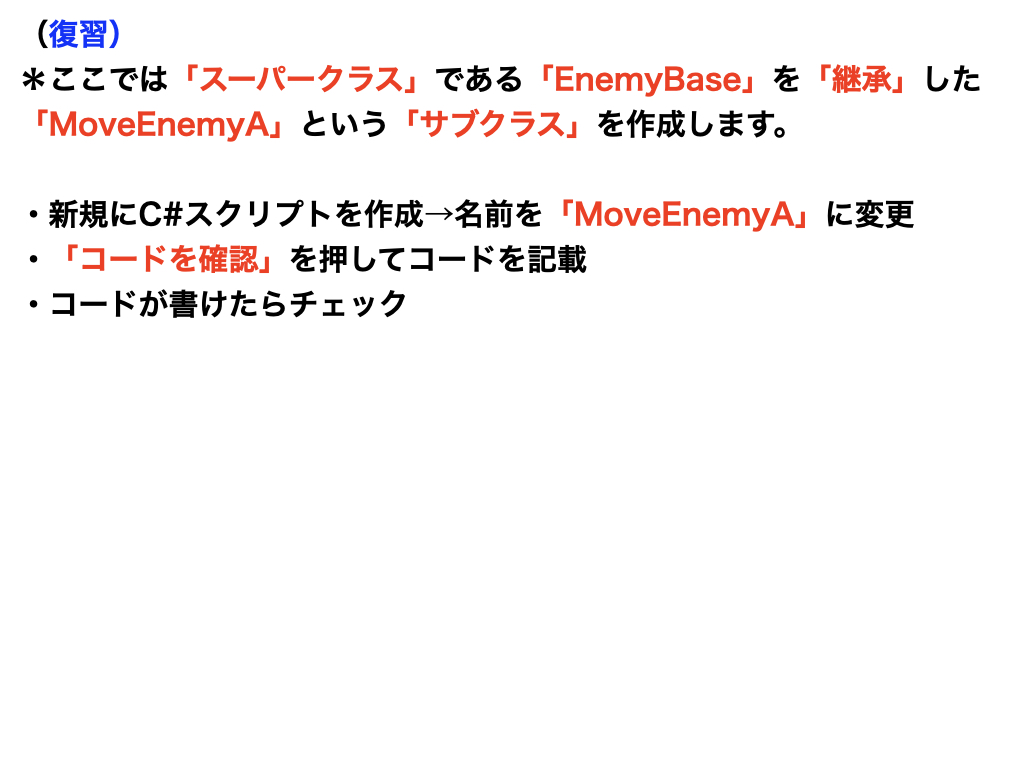
コルーチンで動く敵を作る
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MoveEnemyA : EnemyBase // 復習(クラスの継承)
{
private float x;
private float z;
private float speed;
void Start()
{
HP = 1;
// ★コルーチンの処理を開始する
StartCoroutine(MoveE());
}
void Update()
{
transform.Translate(new Vector3(x, 0, z) * speed * Time.deltaTime, Space.World);
}
// ★コルーチン(処理を途中で中断させることができる仕組み)
private IEnumerator MoveE()
{
// 初めに、speed「5」の速度で、下方向に移動する
x = 0;
z = -1;
speed = 5;
// 「3秒」経過したら、上記の処理を中断して・・・・、
yield return new WaitForSeconds(3f);
// 今度は下記の処理を行う
// speed「10」の速度で、右方向に移動する
x = 1;
z = 0;
speed = 10;
}
}
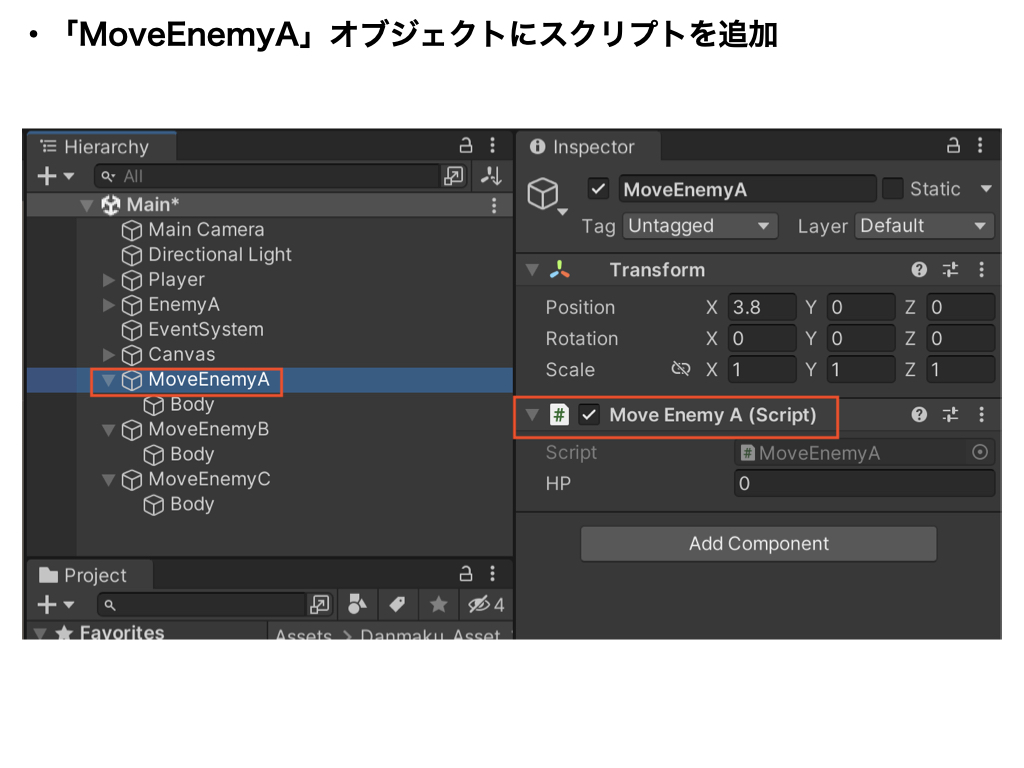
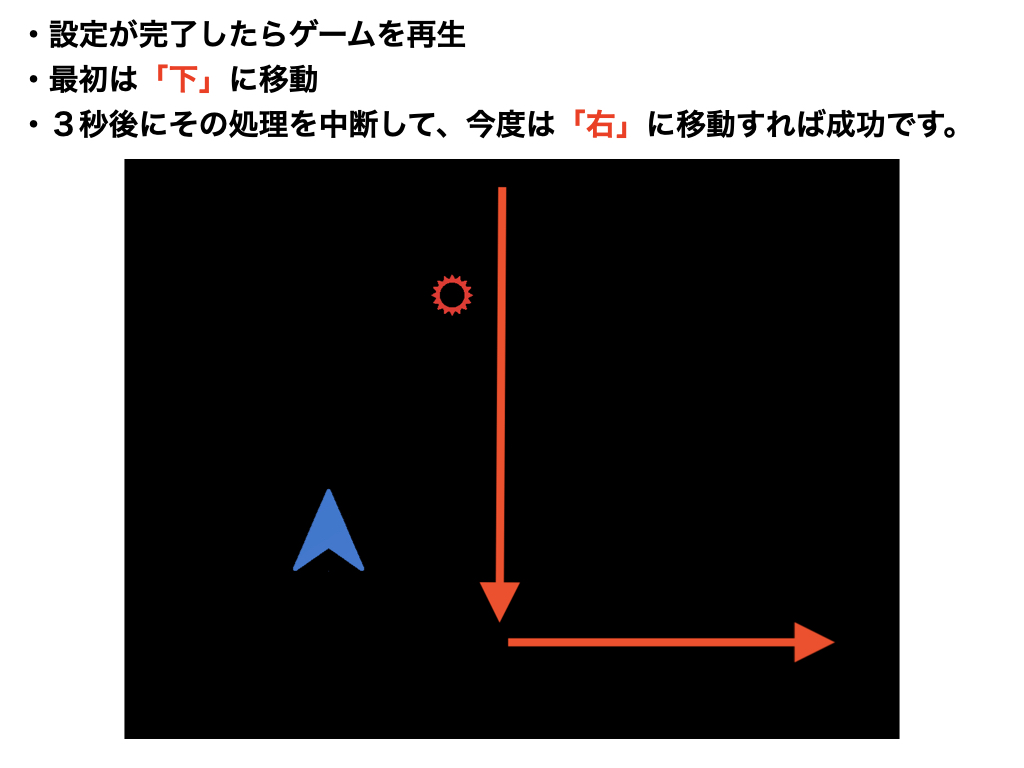
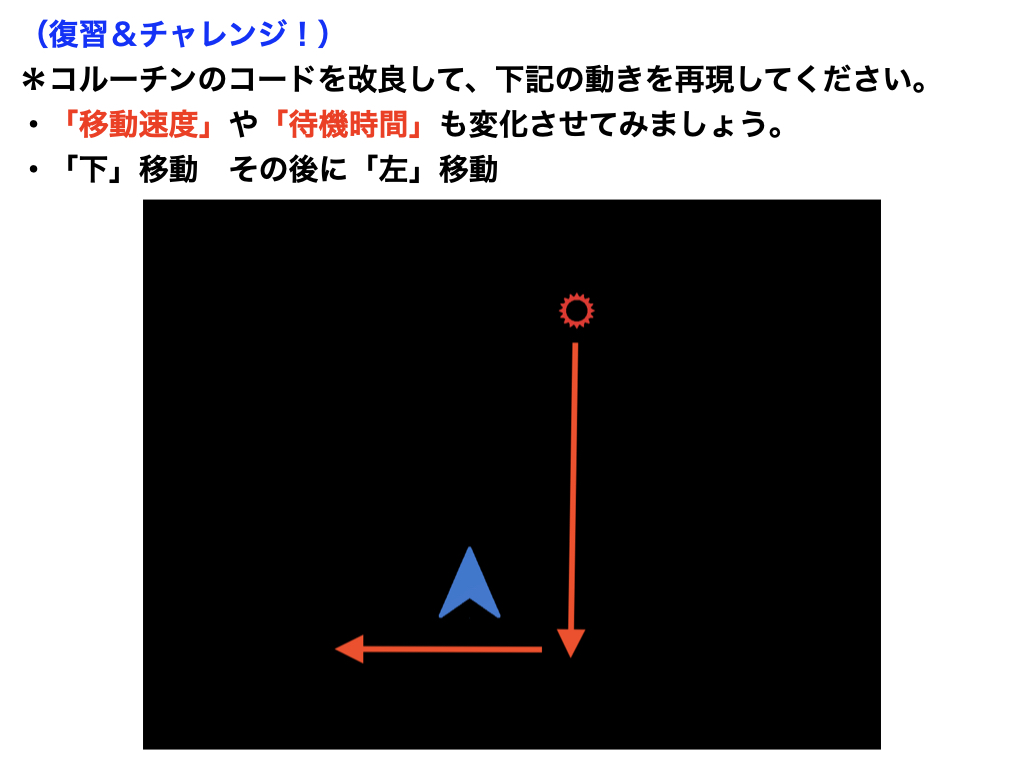
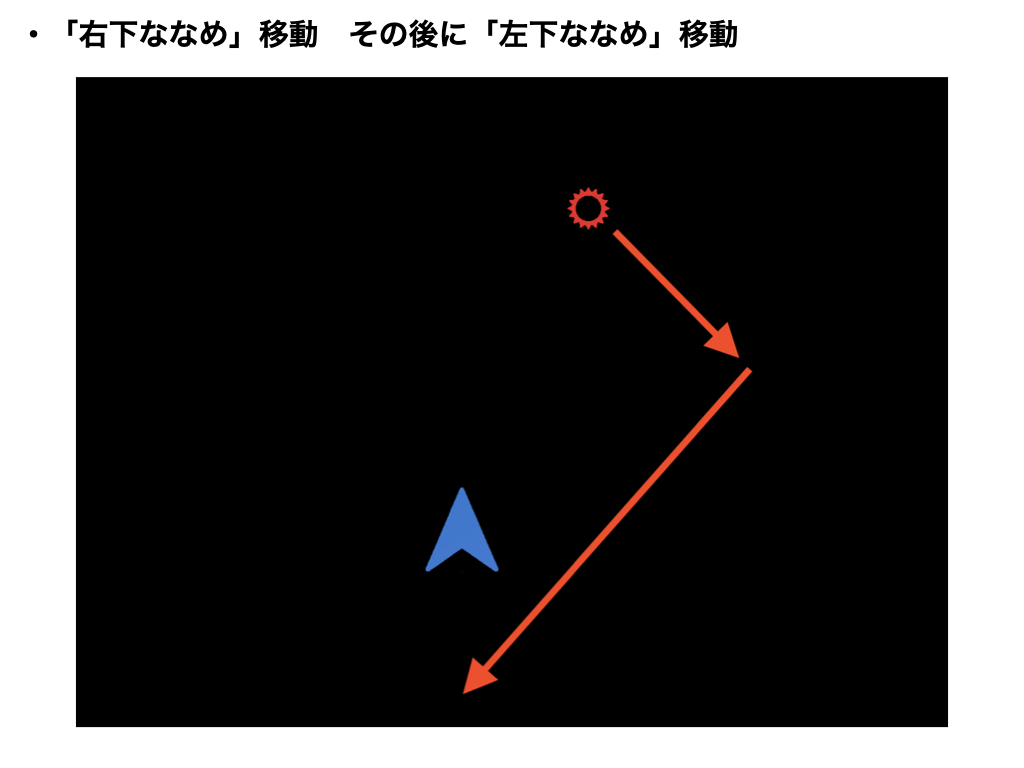
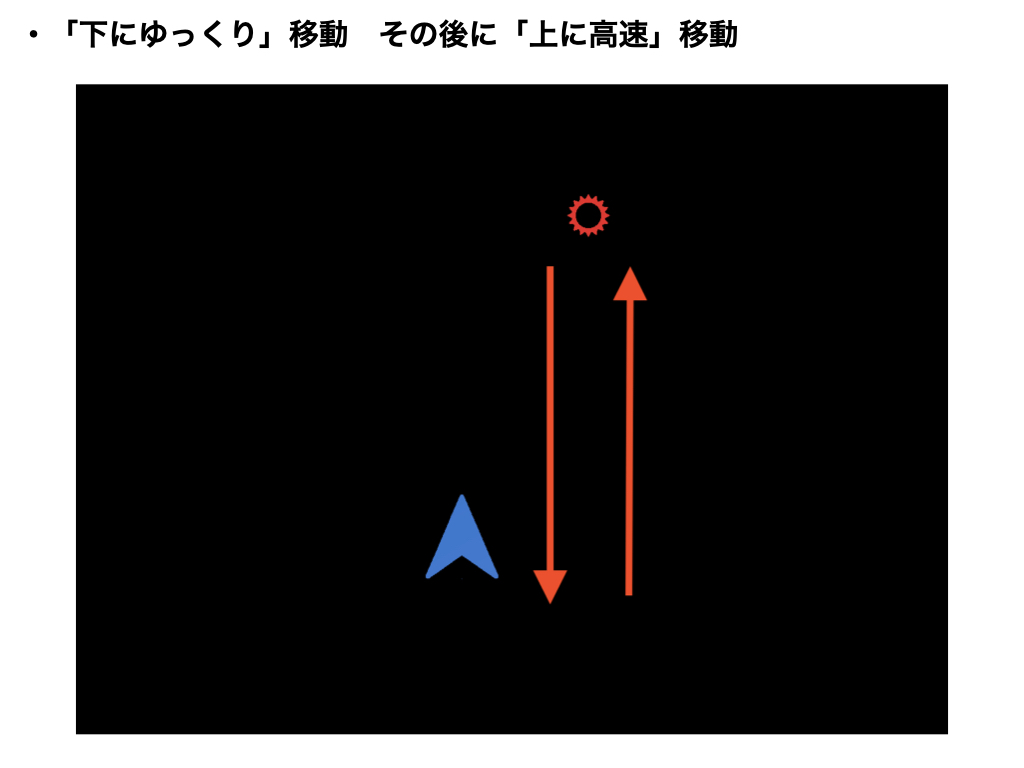
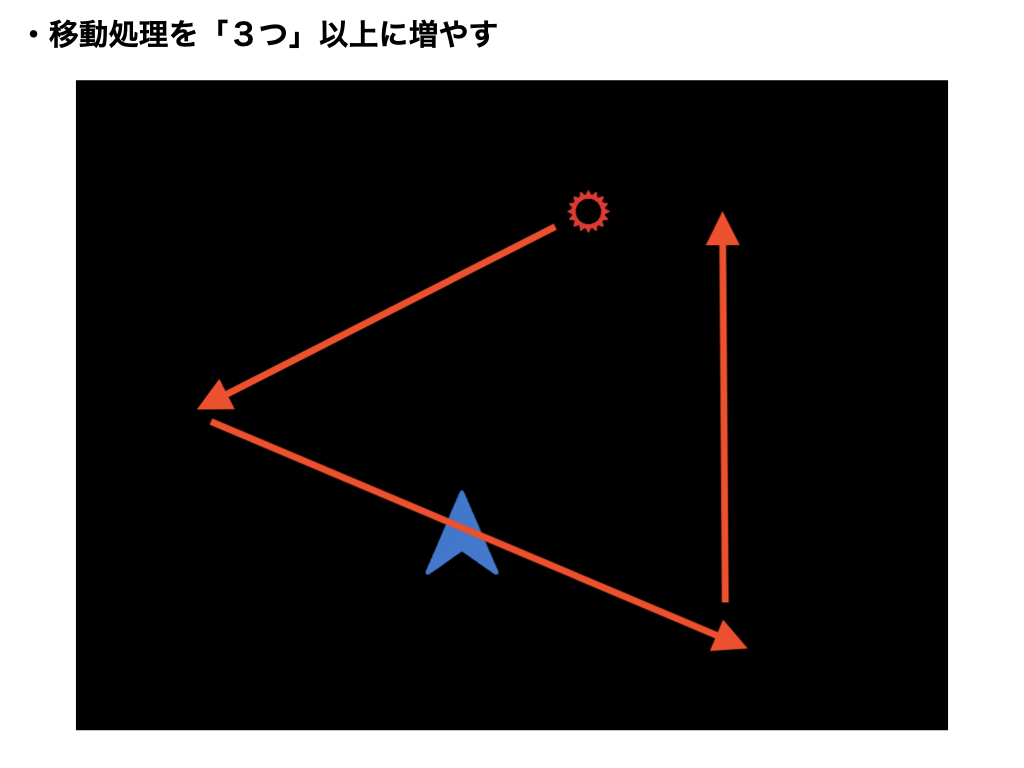
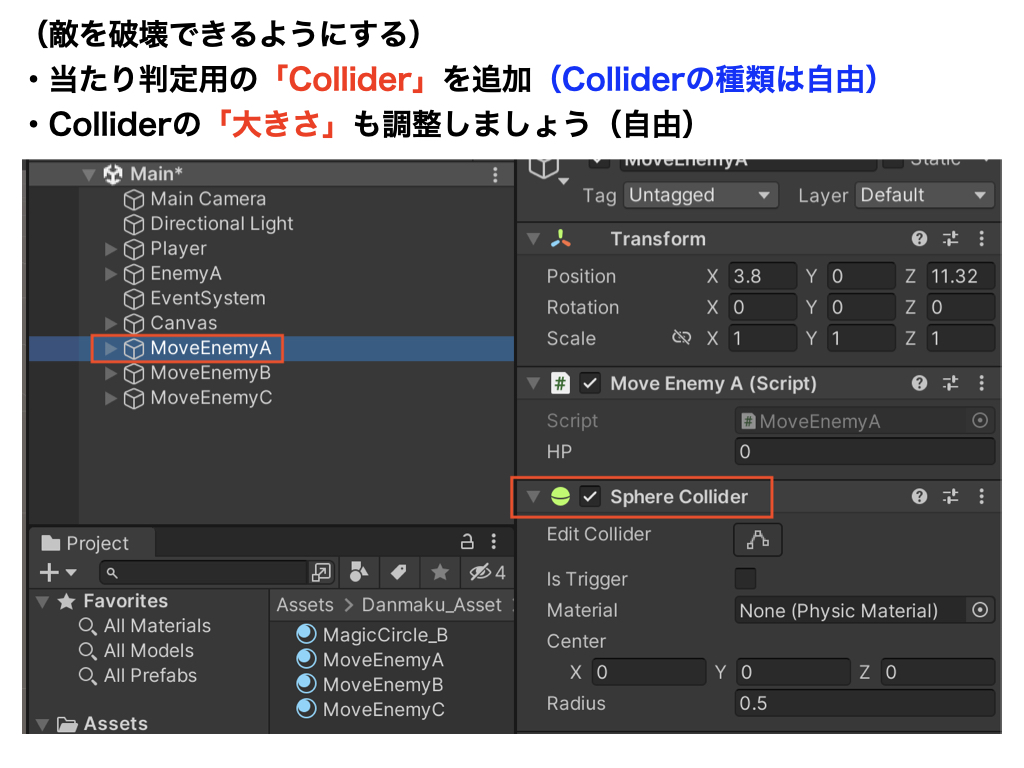
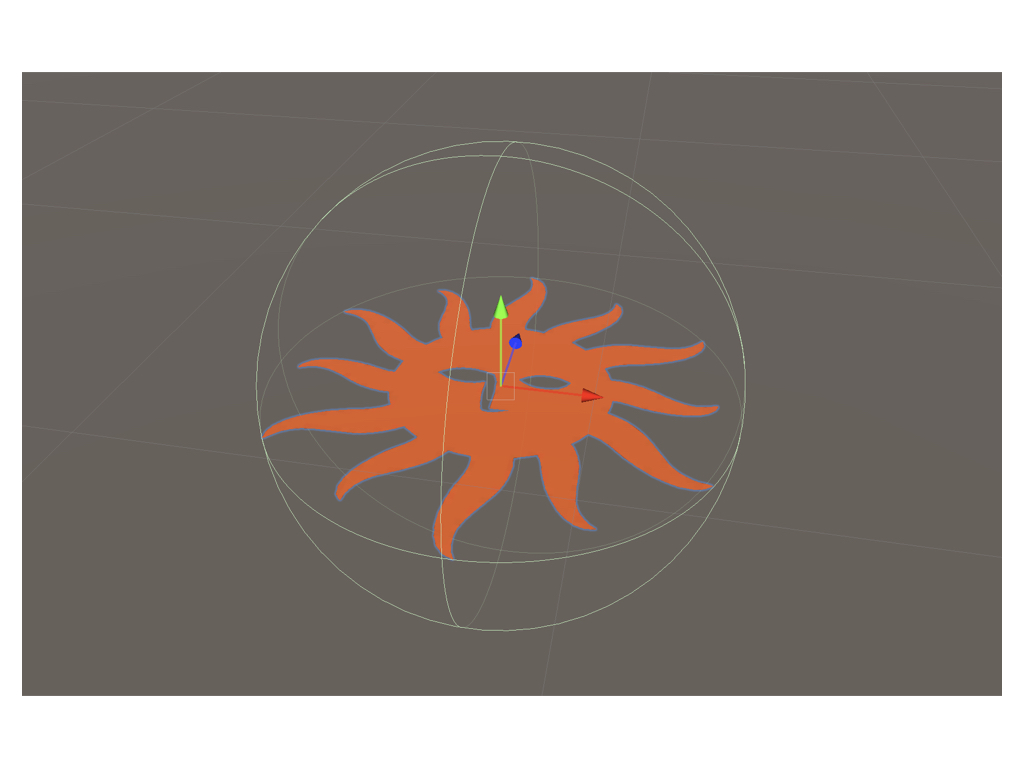
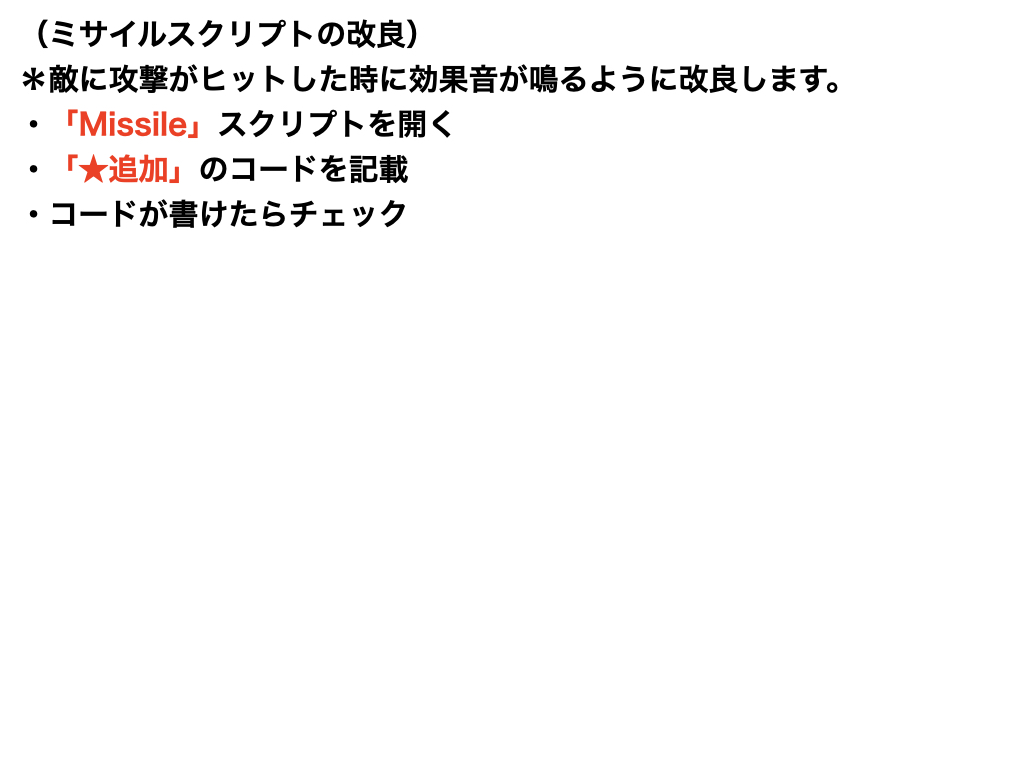
効果音の追加
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Missile : MonoBehaviour
{
public GameObject effectPrefab;
// ★追加
public AudioClip hitSound;
private void OnTriggerEnter(Collider other)
{
if (other.TryGetComponent(out EnemyBase enemy))
{
enemy.TakeDamage(1);
Destroy(gameObject);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 1.0f);
// ★追加
AudioSource.PlayClipAtPoint(hitSound, transform.position);
}
}
}
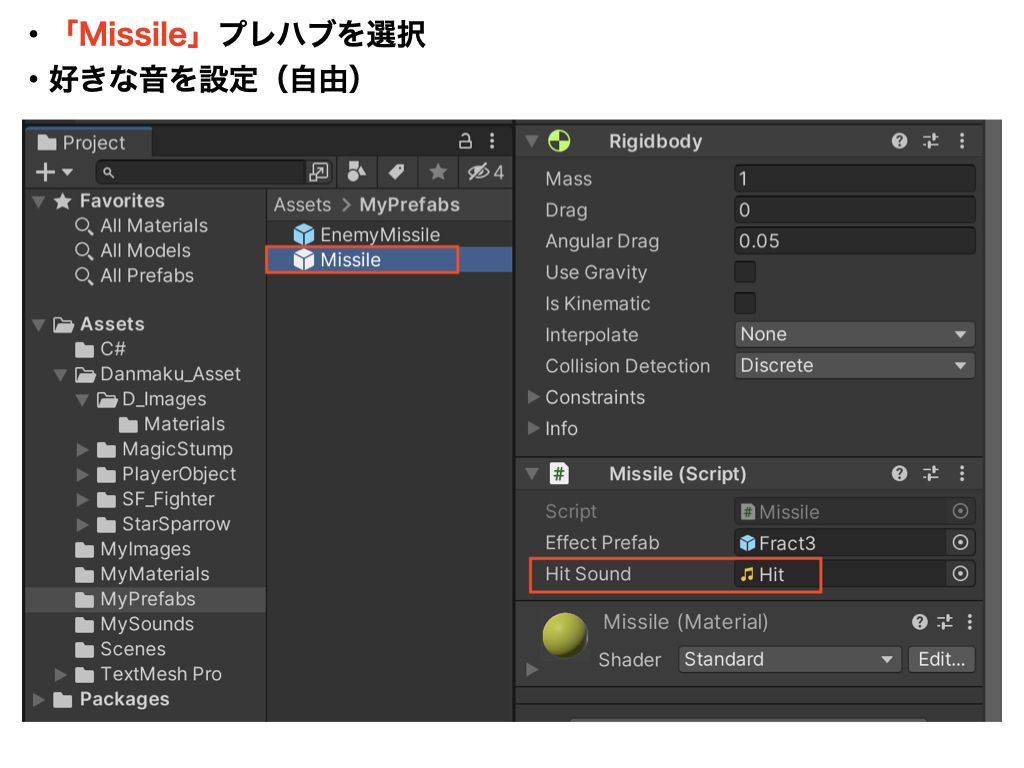
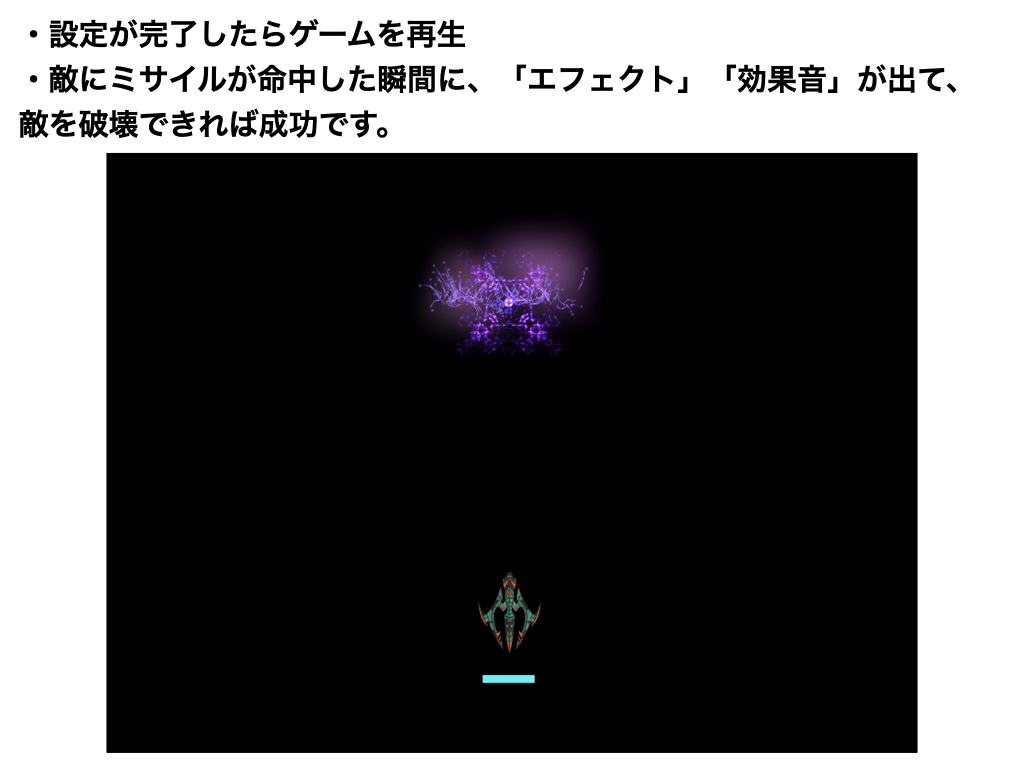
動く敵の作成②(コルーチンで動きを作る)