スコアの獲得と表示
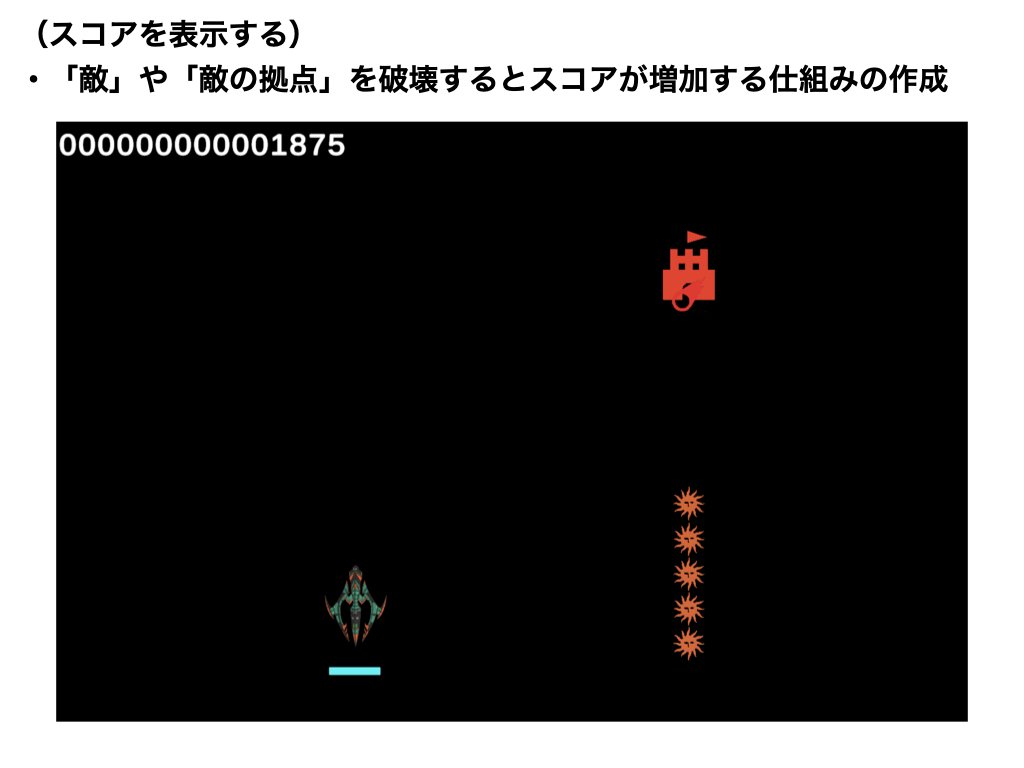
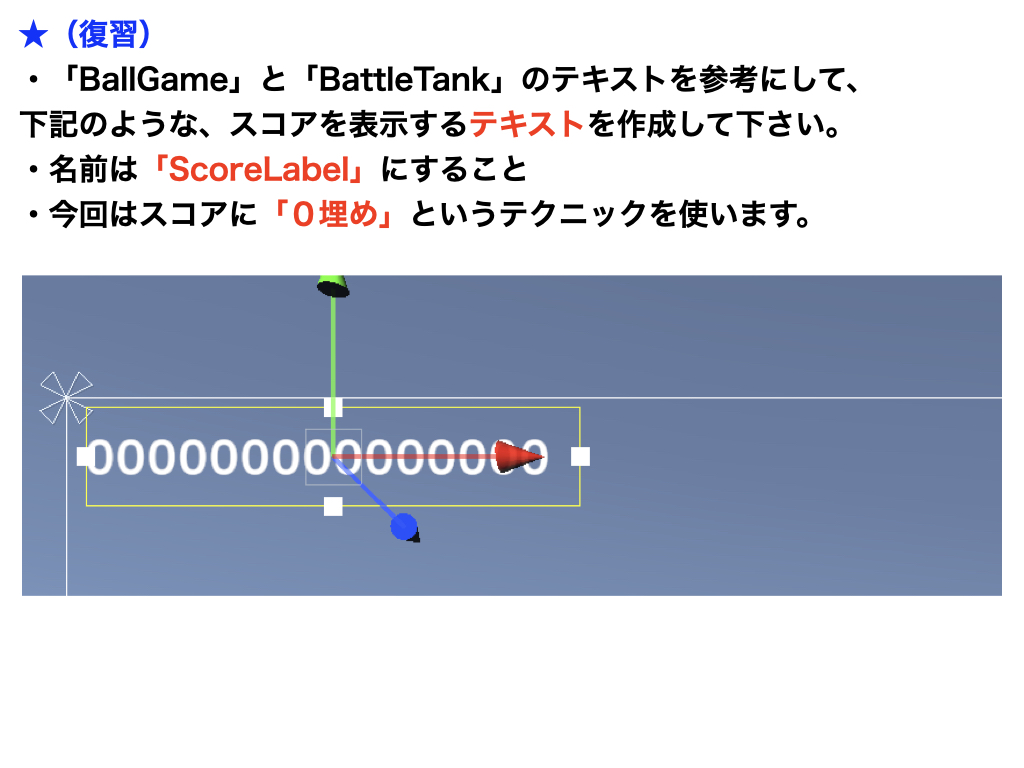
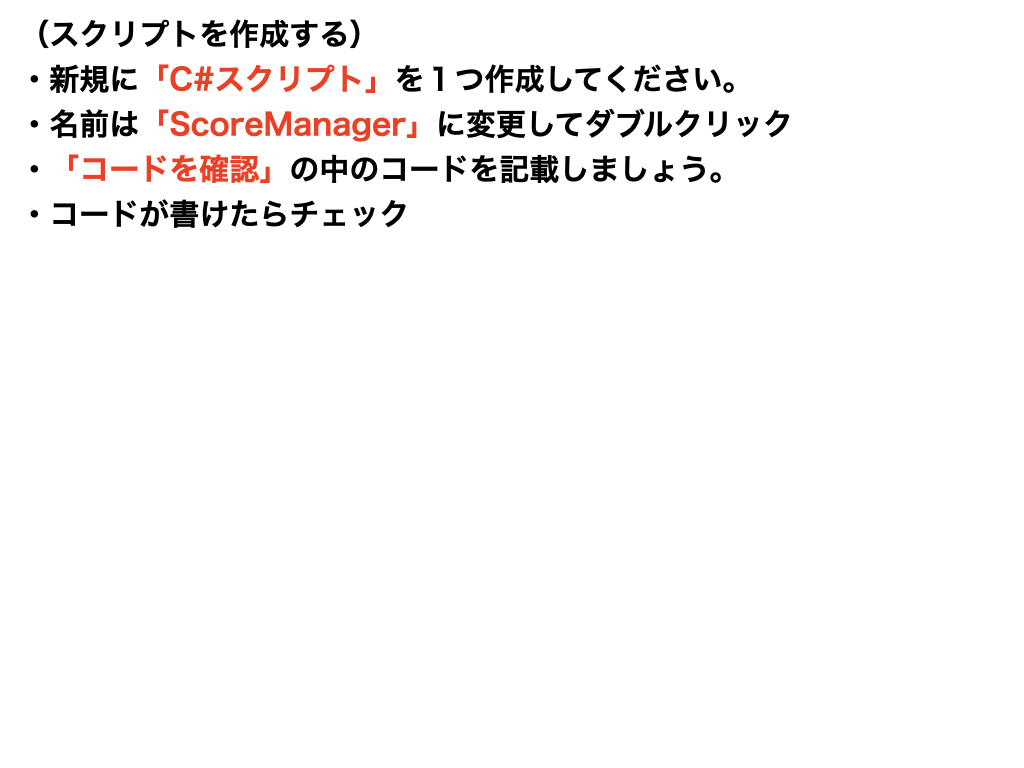
ScoreManager
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★追加
using TMPro;
public class ScoreManager : MonoBehaviour
{
public TextMeshProUGUI scoreLabel;
private int score;
void Start()
{
// 0埋めのテクニック(0を何個並べるかは自由。ここでは15個)
scoreLabel.text = "" + score.ToString("D15");
}
// メソッド経由でscoreの値を変更する。
public void AddScore(int amount)
{
score += amount;
scoreLabel.text = "" + score.ToString("D15");
}
}
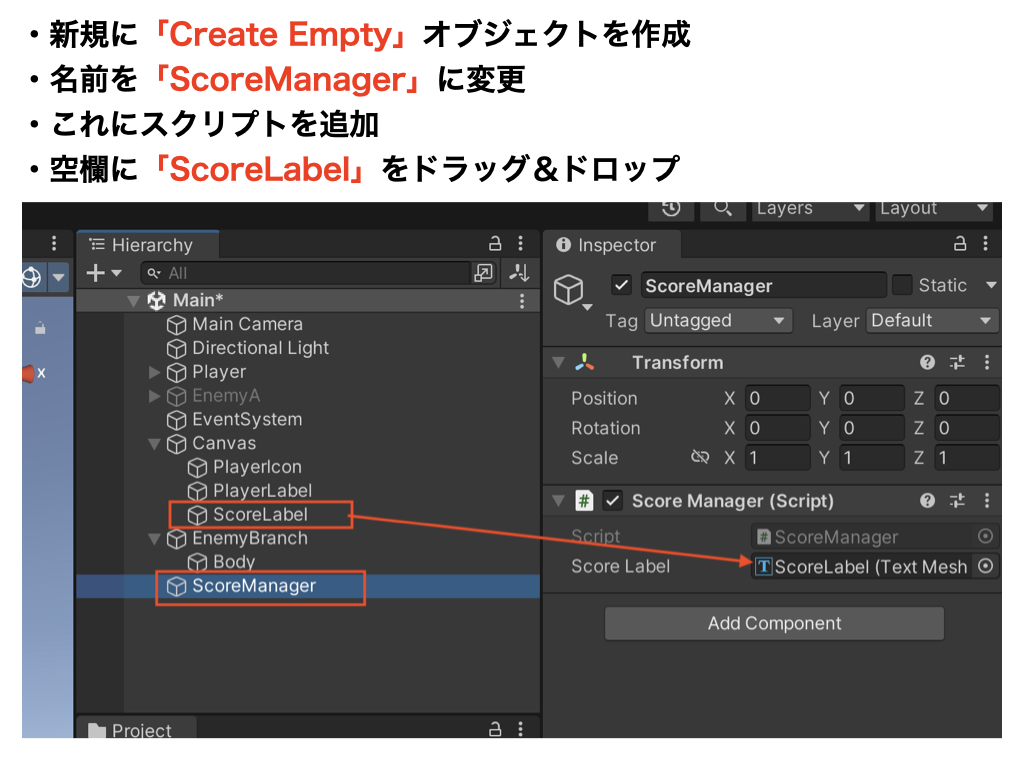
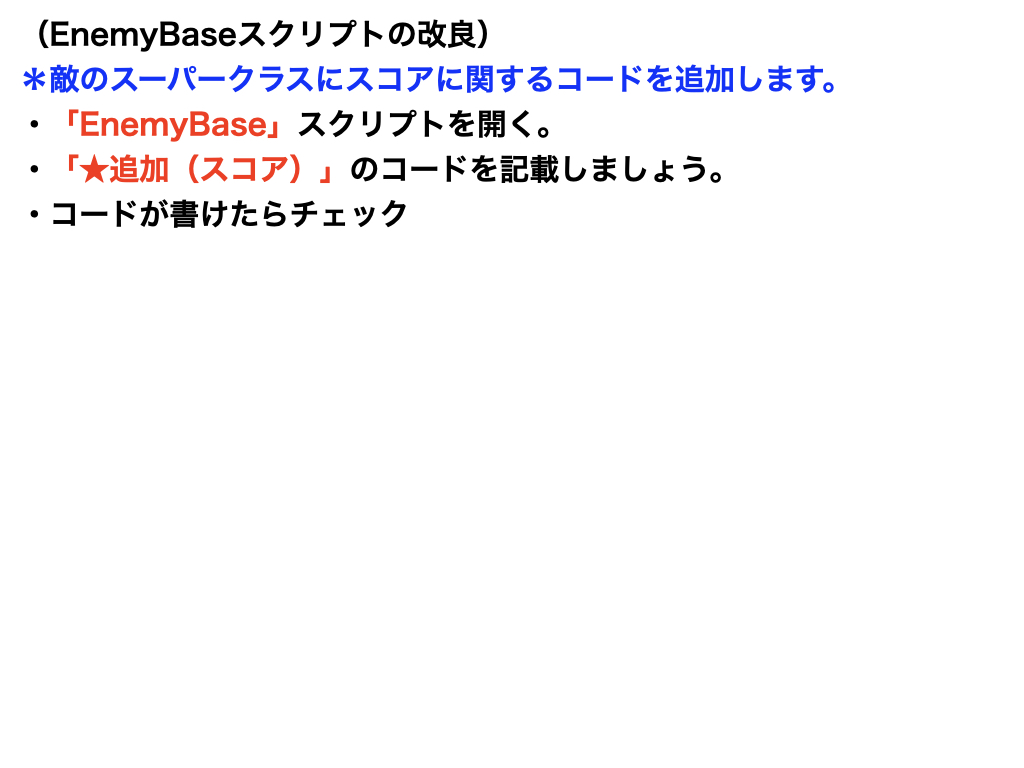
スーパークラスにスコア増加の機能を実装する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public abstract class EnemyBase : MonoBehaviour
{
public int HP;
// ★追加(スコア)
public int ScoreValue;
public virtual void TakeDamage(int missilePower)
{
HP -= missilePower;
if (HP < 1)
{
Destroy(gameObject);
// ★追加(スコア)
GameObject.Find("ScoreManager").GetComponent<ScoreManager>().AddScore(ScoreValue);
}
}
}
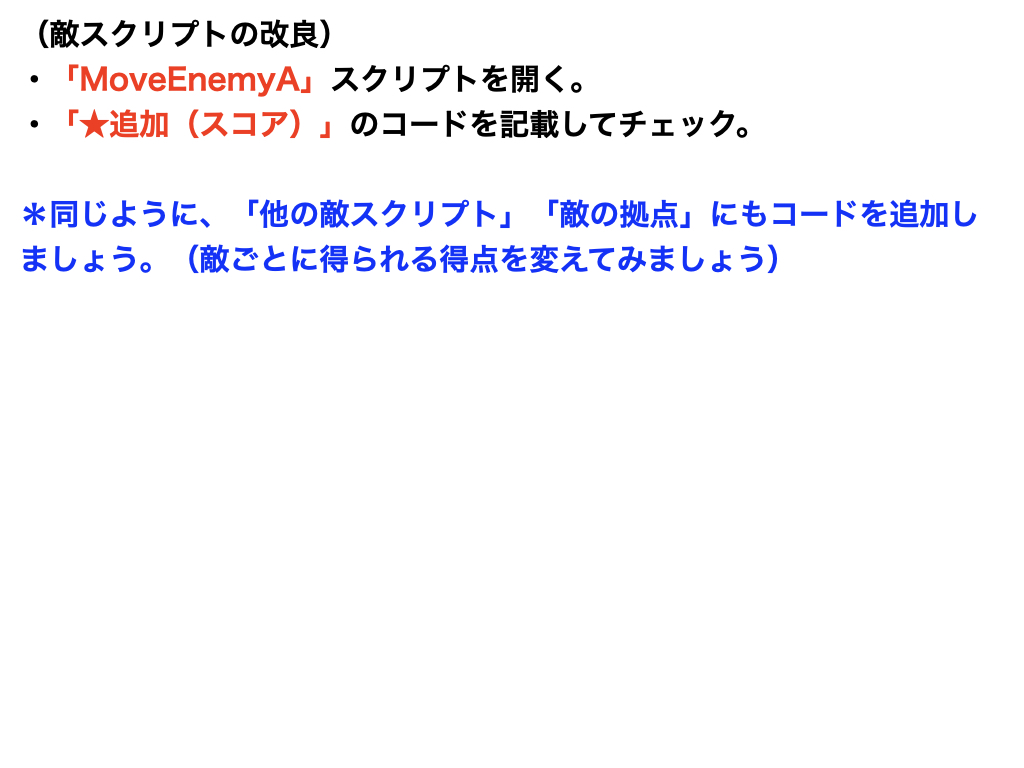
スコアの機能の追加
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MoveEnemyA : EnemyBase
{
private float x;
private float z;
private float speed;
void Start()
{
HP = 1;
StartCoroutine(MoveE());
// ★追加(スコア)
ScoreValue = 100;
}
void Update()
{
transform.Translate(new Vector3(x, 0, z) * speed * Time.deltaTime, Space.World);
}
private IEnumerator MoveE()
{
x = 0;
z = -1;
speed = 5;
yield return new WaitForSeconds(3f);
x = 1;
z = 0;
speed = 10;
}
}
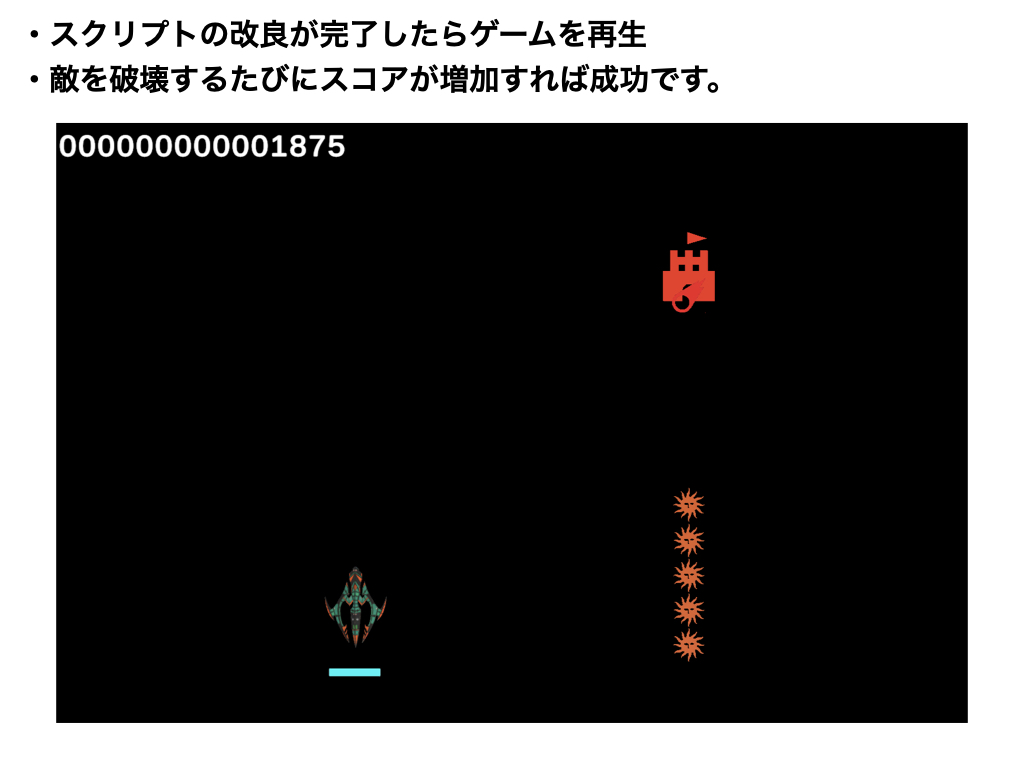
【2021版】Danmaku(基礎/全55回)
他のコースを見る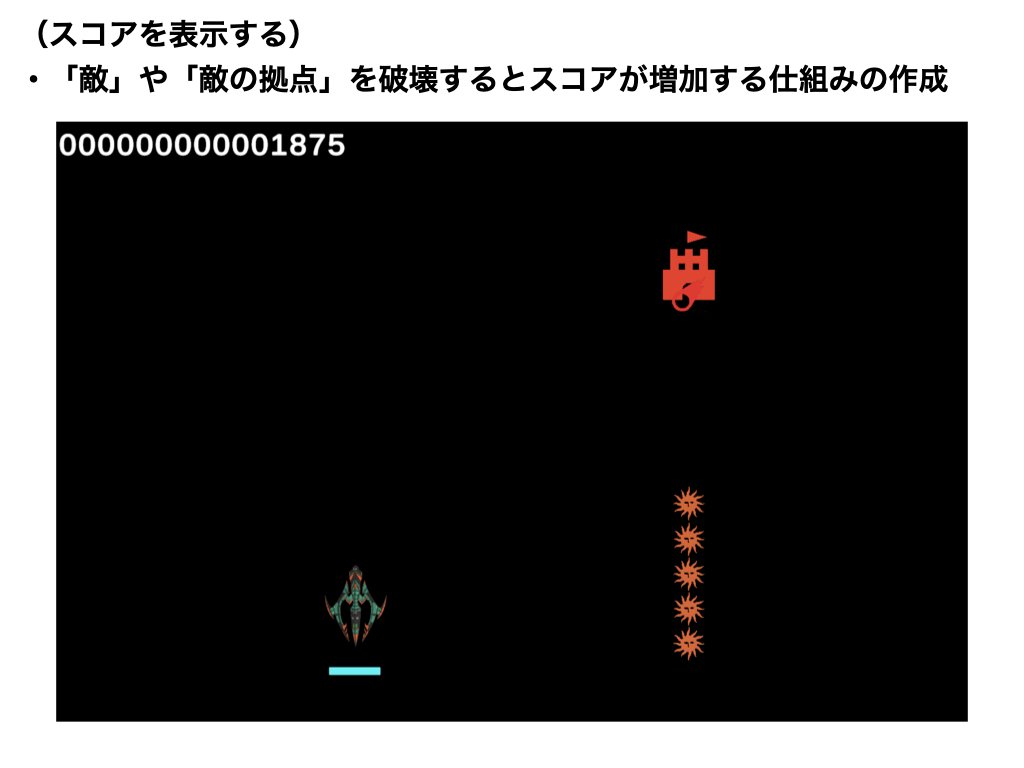
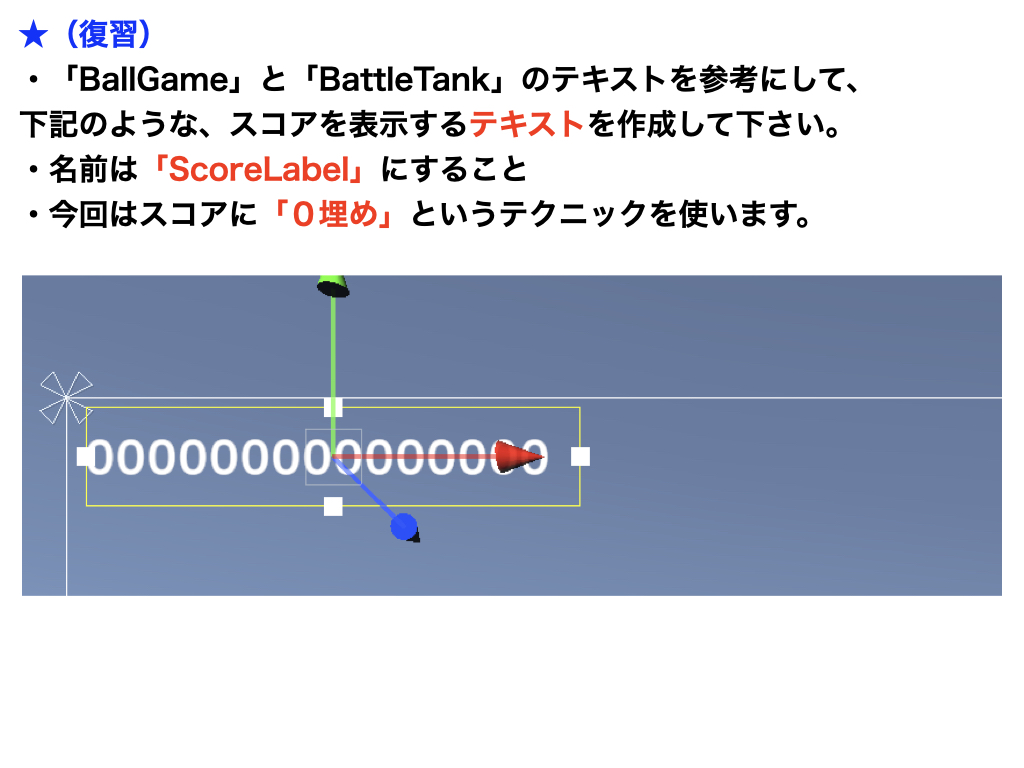
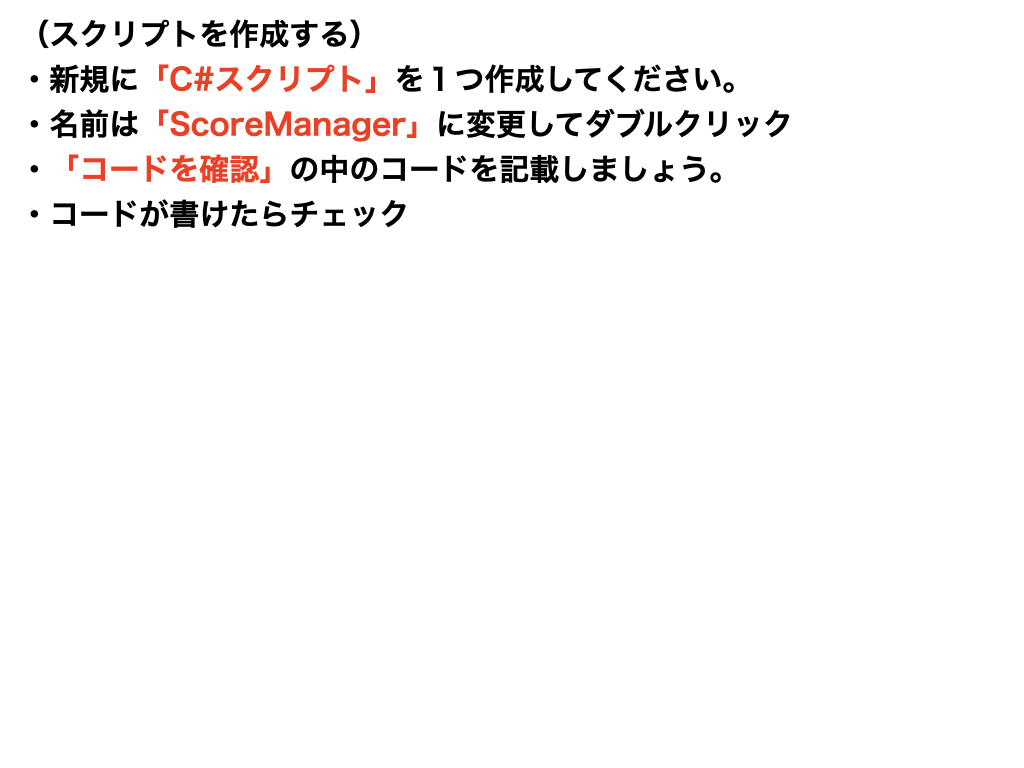
ScoreManager
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★追加
using TMPro;
public class ScoreManager : MonoBehaviour
{
public TextMeshProUGUI scoreLabel;
private int score;
void Start()
{
// 0埋めのテクニック(0を何個並べるかは自由。ここでは15個)
scoreLabel.text = "" + score.ToString("D15");
}
// メソッド経由でscoreの値を変更する。
public void AddScore(int amount)
{
score += amount;
scoreLabel.text = "" + score.ToString("D15");
}
}
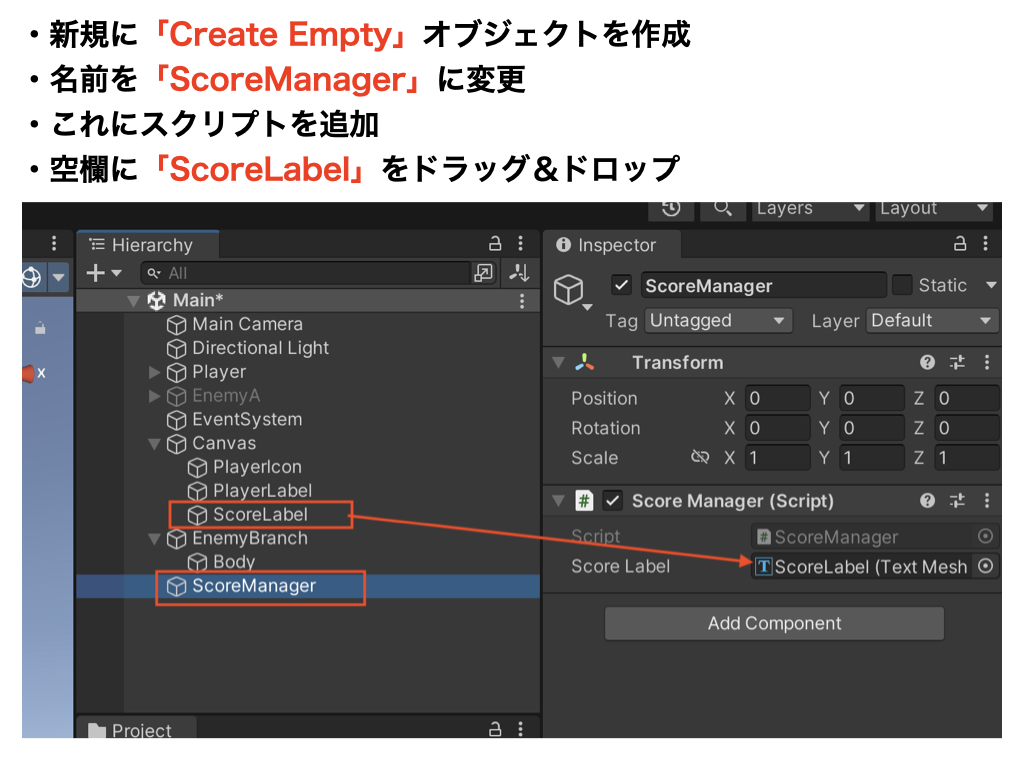
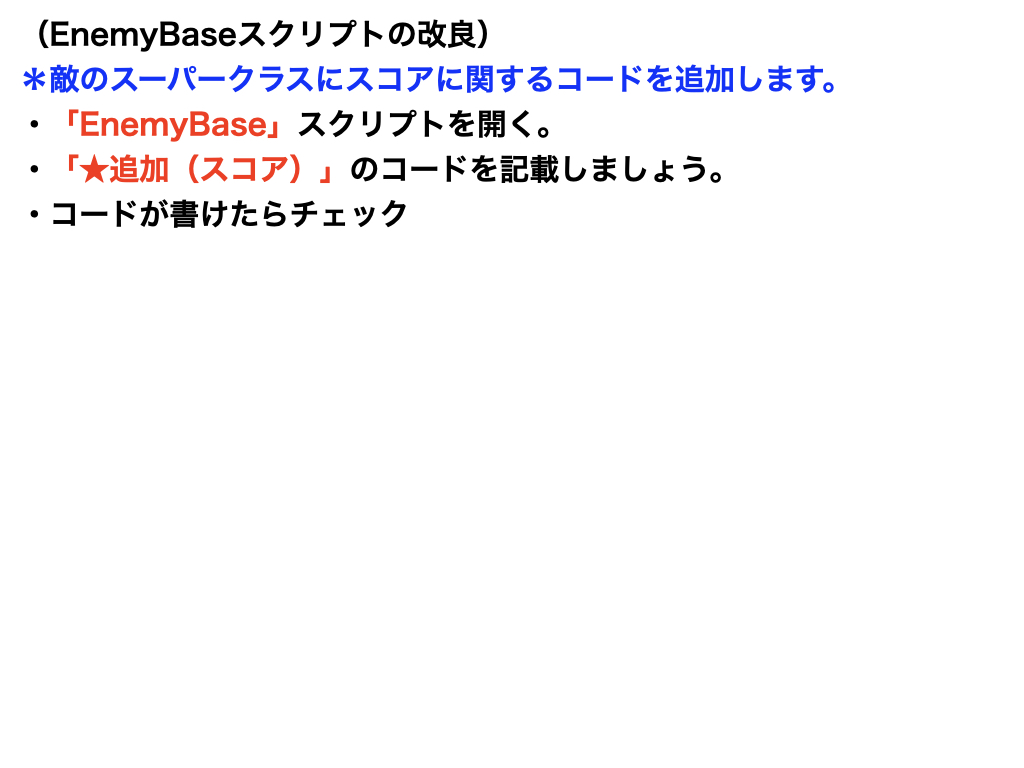
スーパークラスにスコア増加の機能を実装する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public abstract class EnemyBase : MonoBehaviour
{
public int HP;
// ★追加(スコア)
public int ScoreValue;
public virtual void TakeDamage(int missilePower)
{
HP -= missilePower;
if (HP < 1)
{
Destroy(gameObject);
// ★追加(スコア)
GameObject.Find("ScoreManager").GetComponent<ScoreManager>().AddScore(ScoreValue);
}
}
}
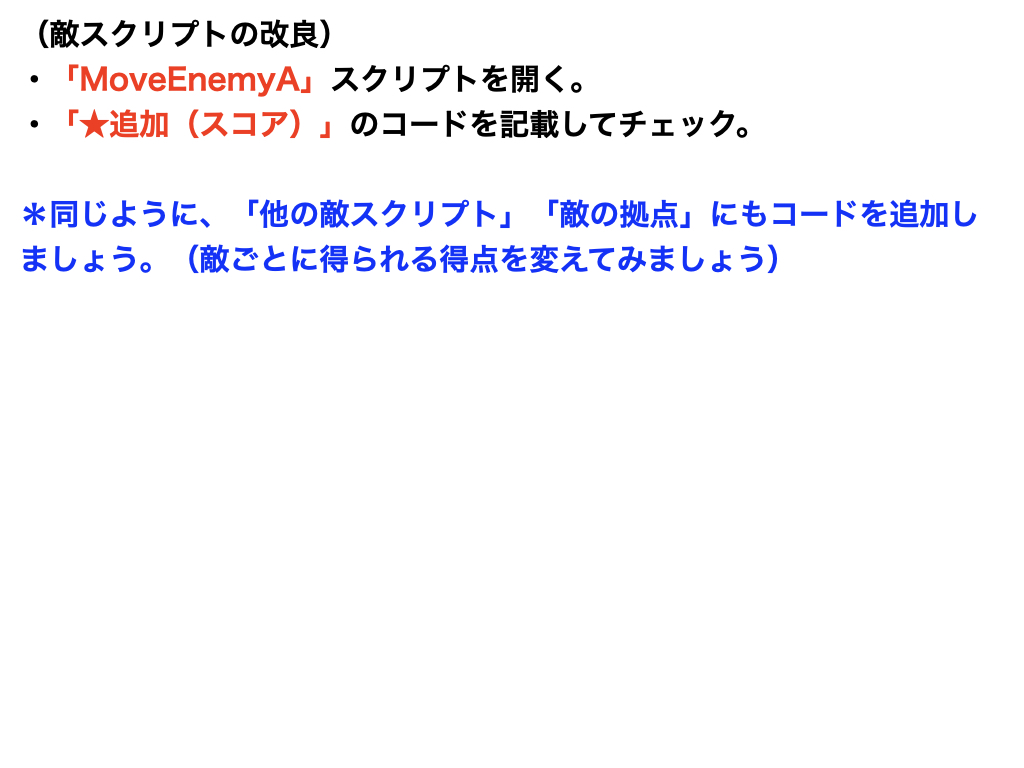
スコアの機能の追加
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MoveEnemyA : EnemyBase
{
private float x;
private float z;
private float speed;
void Start()
{
HP = 1;
StartCoroutine(MoveE());
// ★追加(スコア)
ScoreValue = 100;
}
void Update()
{
transform.Translate(new Vector3(x, 0, z) * speed * Time.deltaTime, Space.World);
}
private IEnumerator MoveE()
{
x = 0;
z = -1;
speed = 5;
yield return new WaitForSeconds(3f);
x = 1;
z = 0;
speed = 10;
}
}
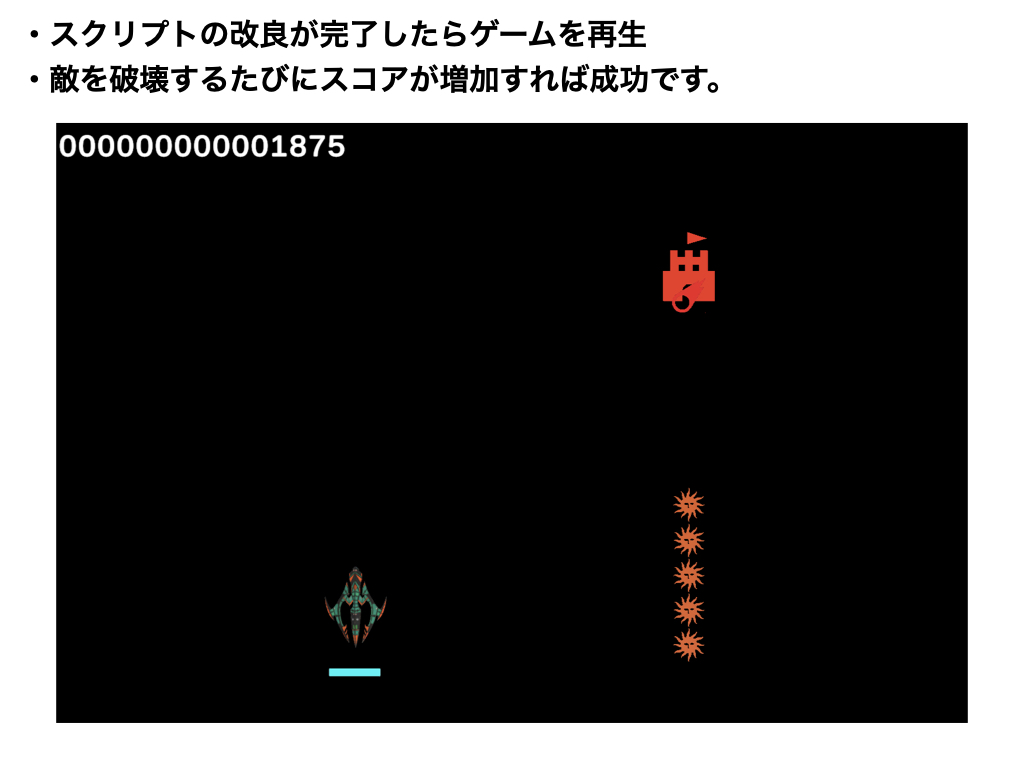
スコアの獲得と表示