コインをゲットしよう
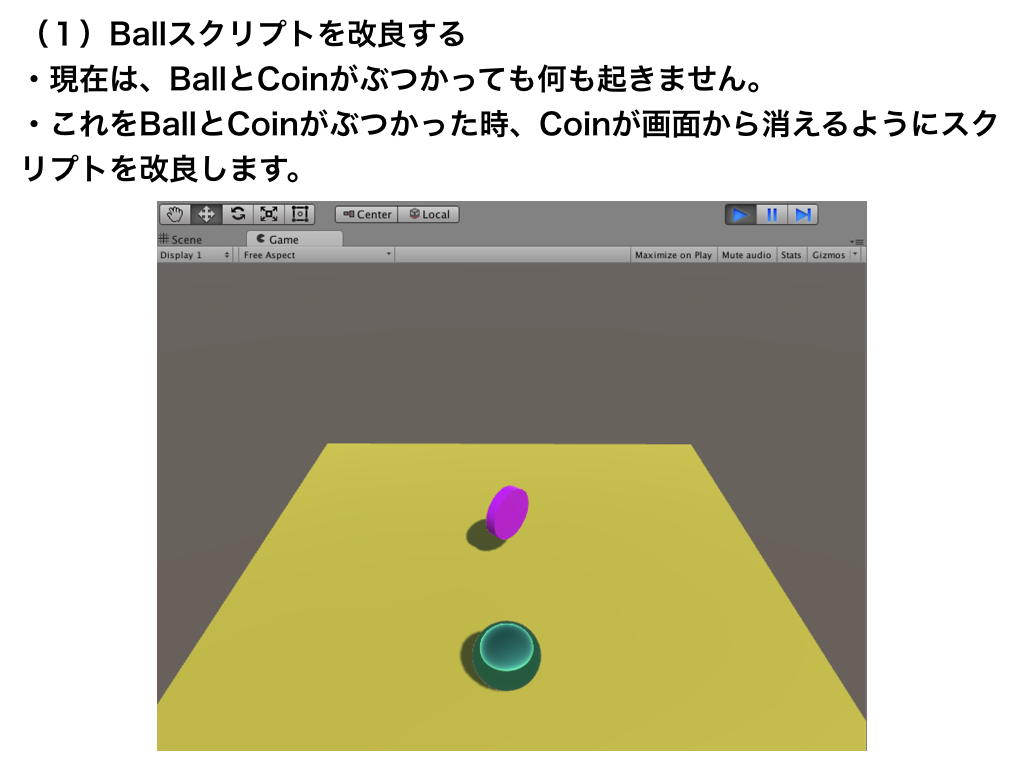
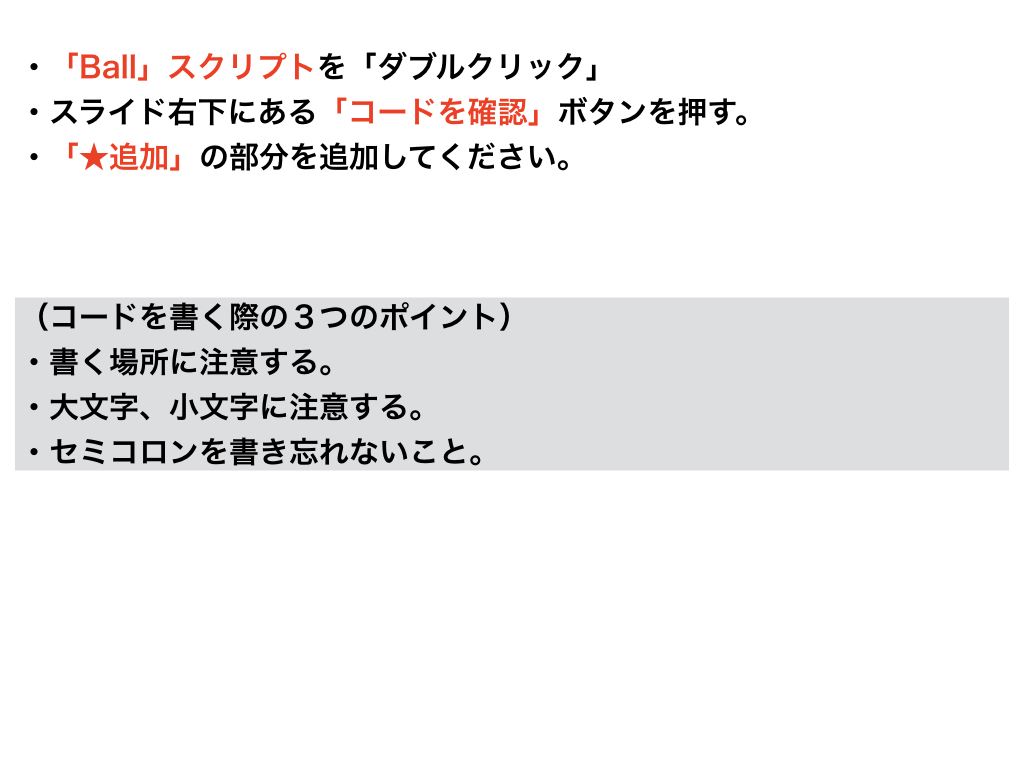
コインゲット
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
}
private void OnTriggerEnter(Collider other)
{
// もしもぶつかった相手に「Coin」という「Tag」が付いていたならば(条件)
if (other.CompareTag("Coin"))
{
// ぶつかった相手を破壊する(実行)
Destroy(other.gameObject);
}
}
}
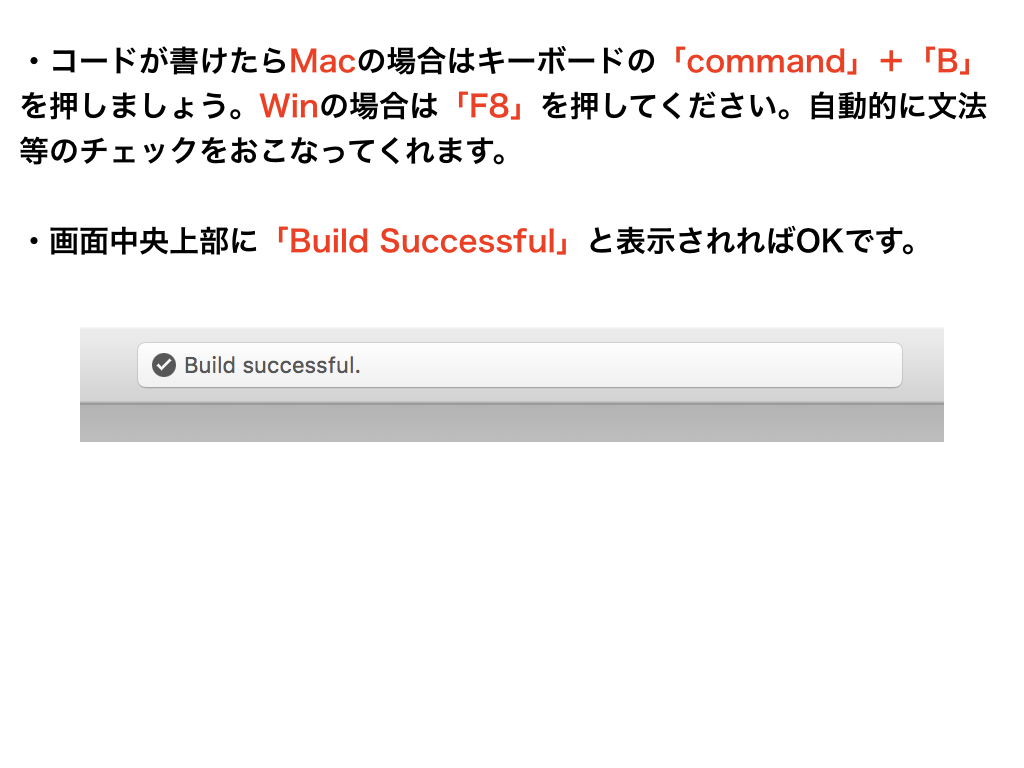
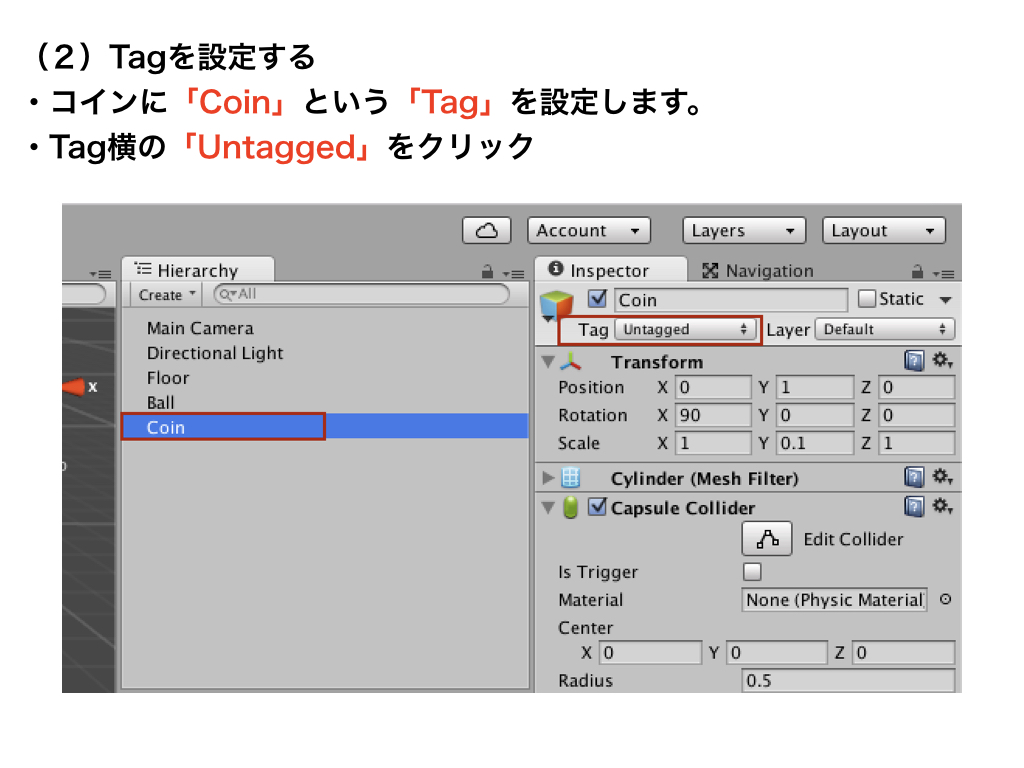
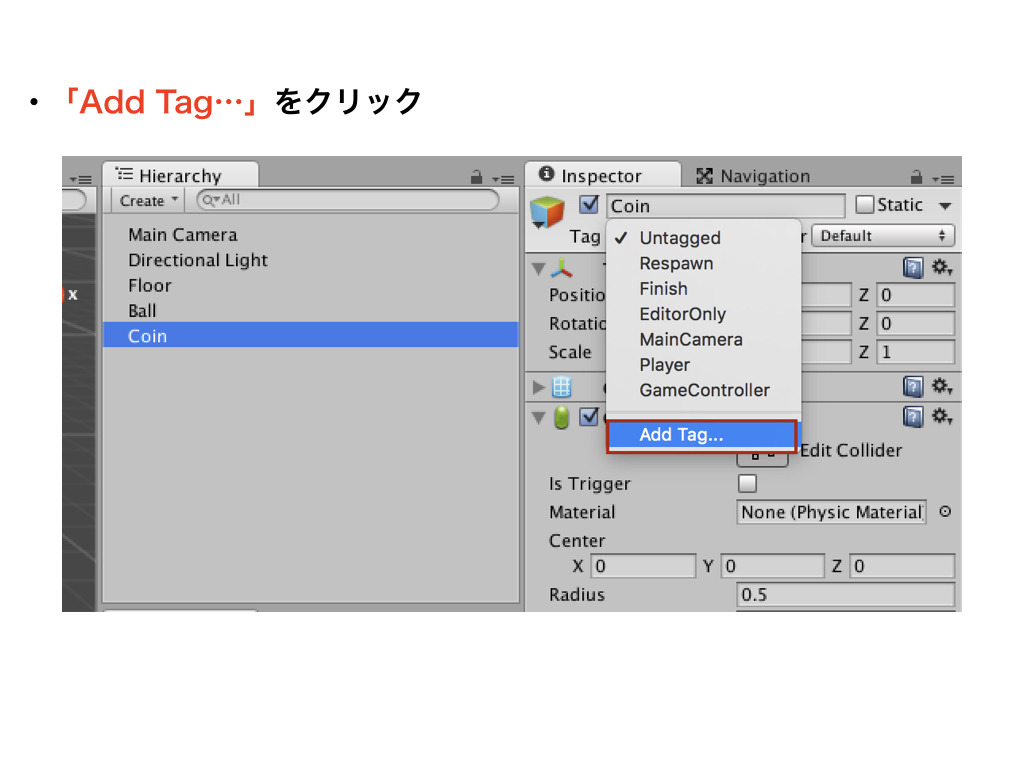
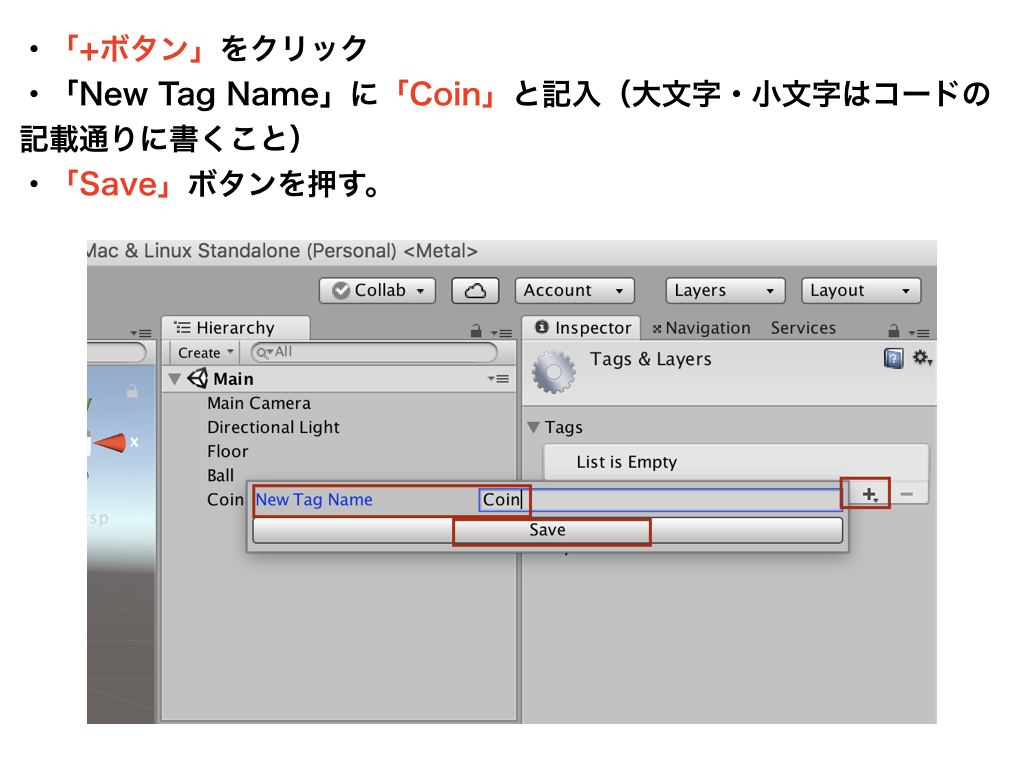
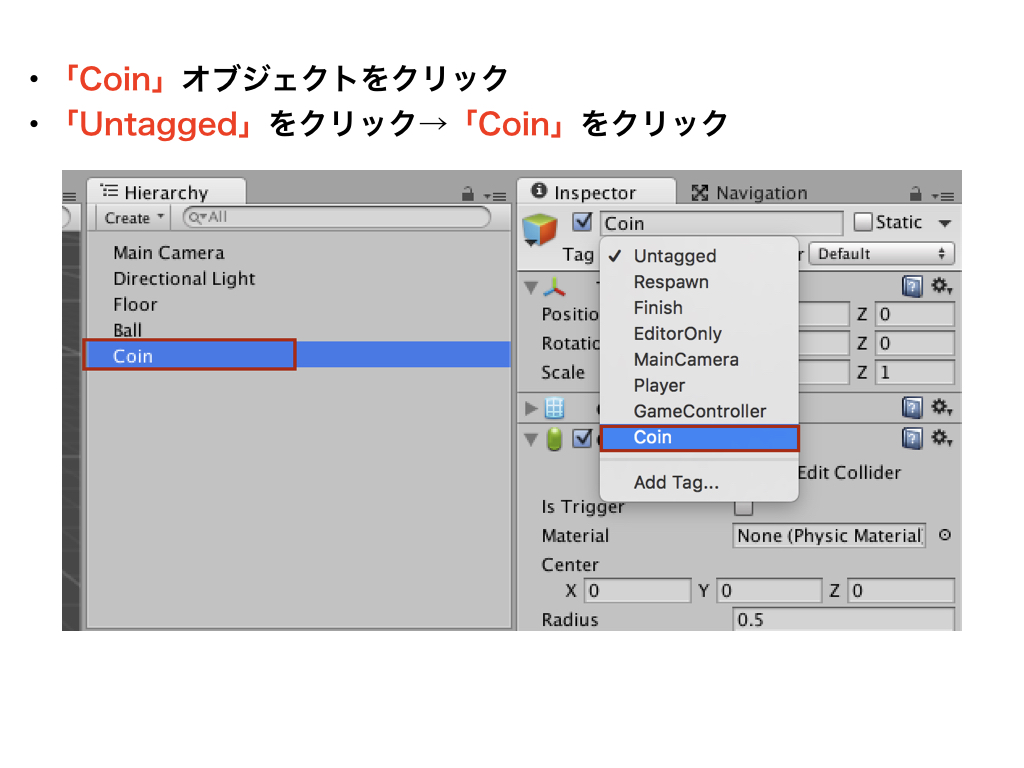
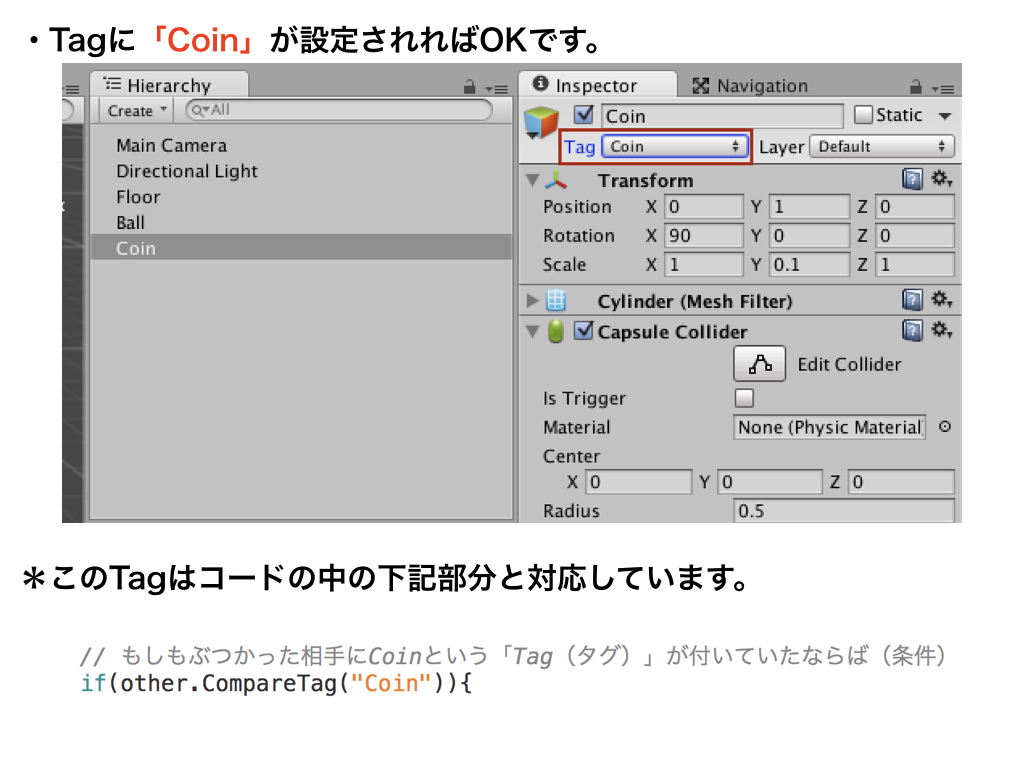
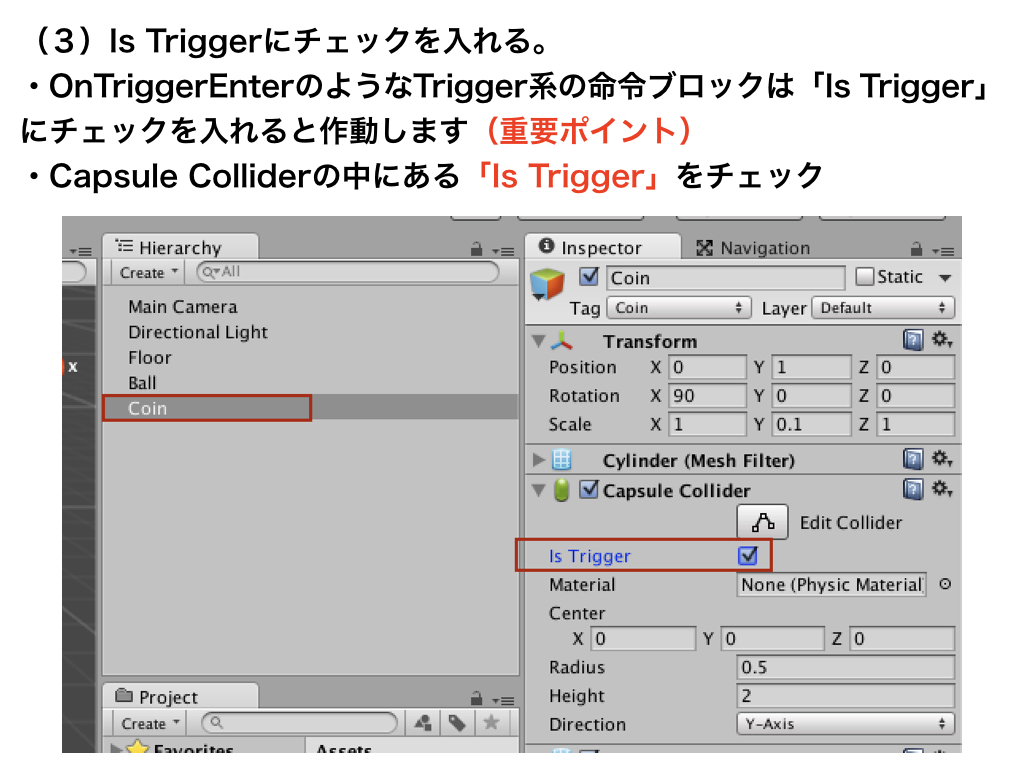
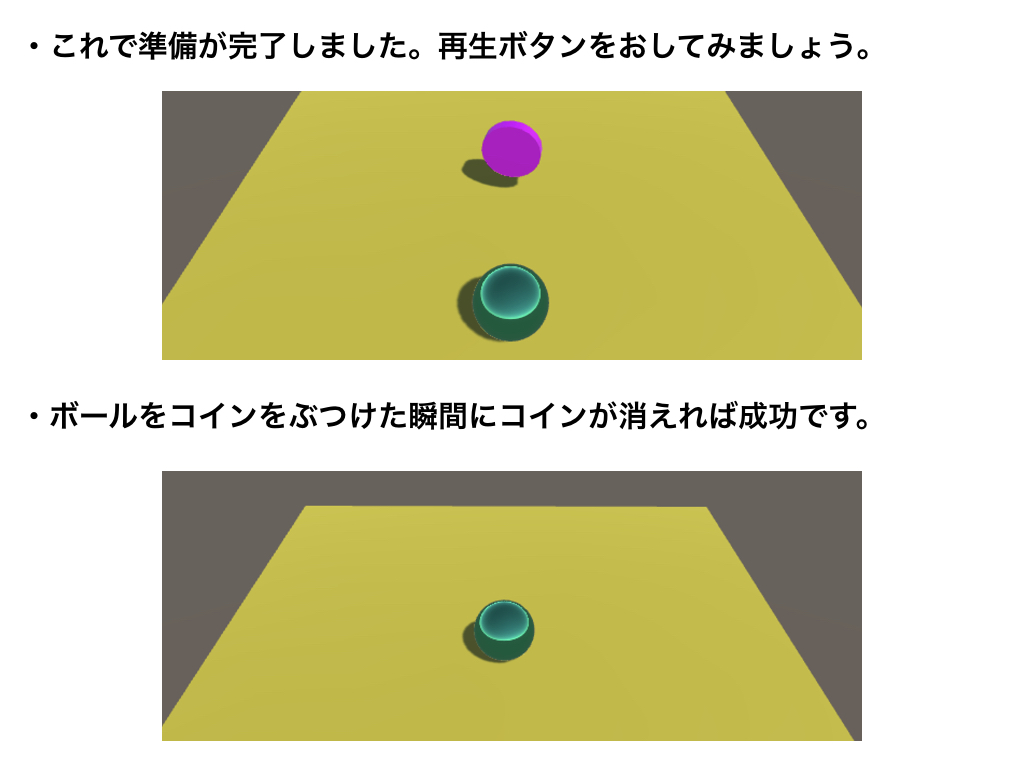
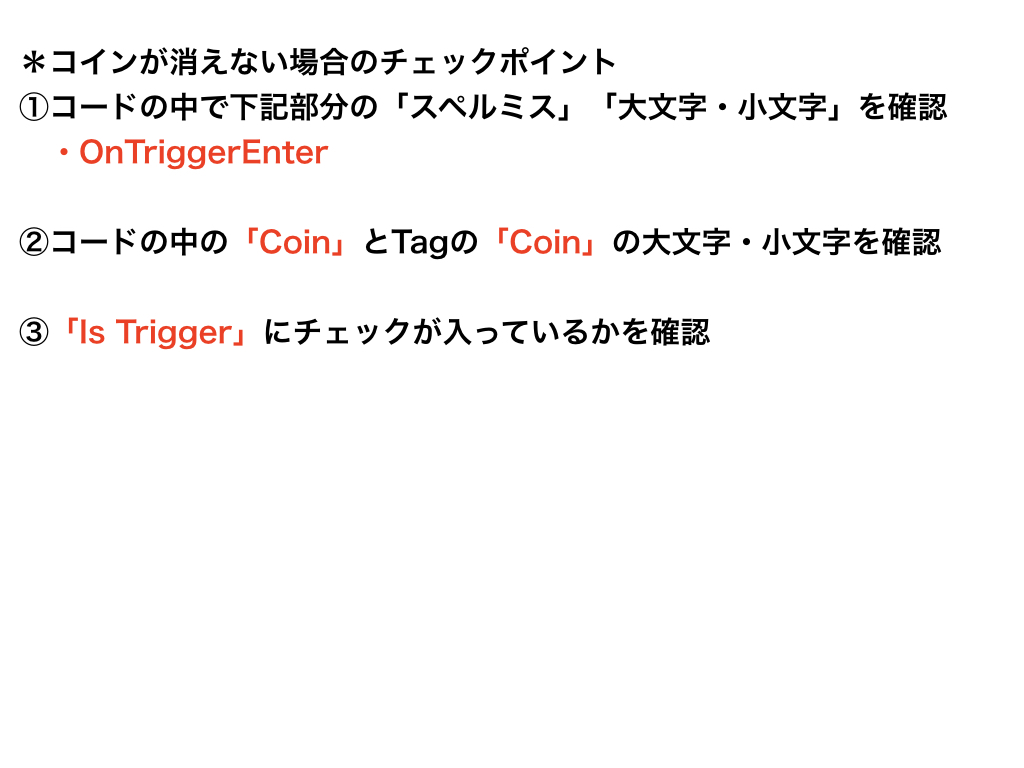
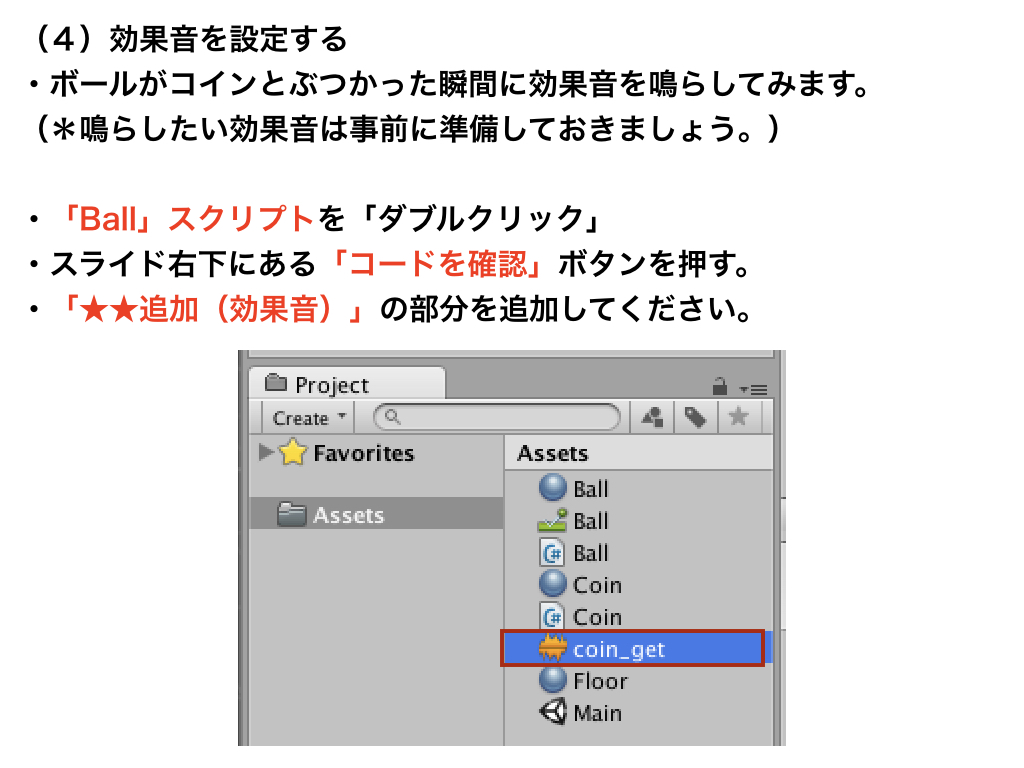
効果音の追加
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
// ★★追加(効果音)
public AudioClip coinGet;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
}
// ★追加
private void OnTriggerEnter(Collider other)
{
// もしもぶつかった相手に「Coin」という「Tag」が付いていたならば(条件)
if (other.CompareTag("Coin"))
{
// ぶつかった相手を破壊する(実行)
Destroy(other.gameObject);
// ★★追加(効果音)
AudioSource.PlayClipAtPoint(coinGet, transform.position);
}
}
}
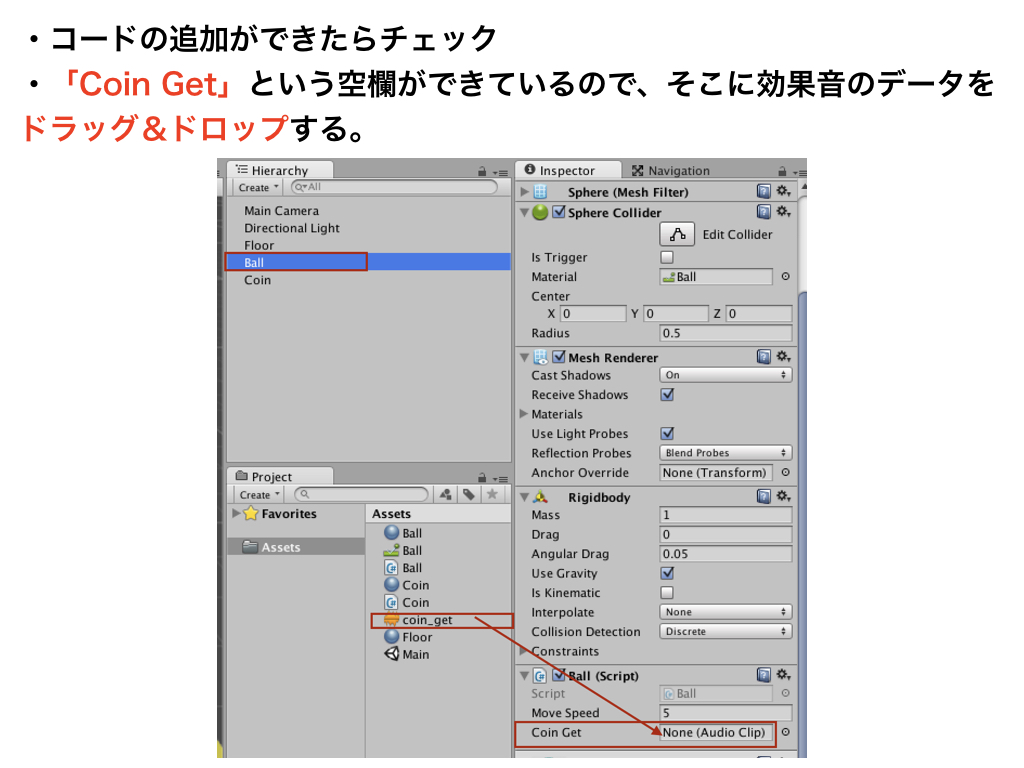
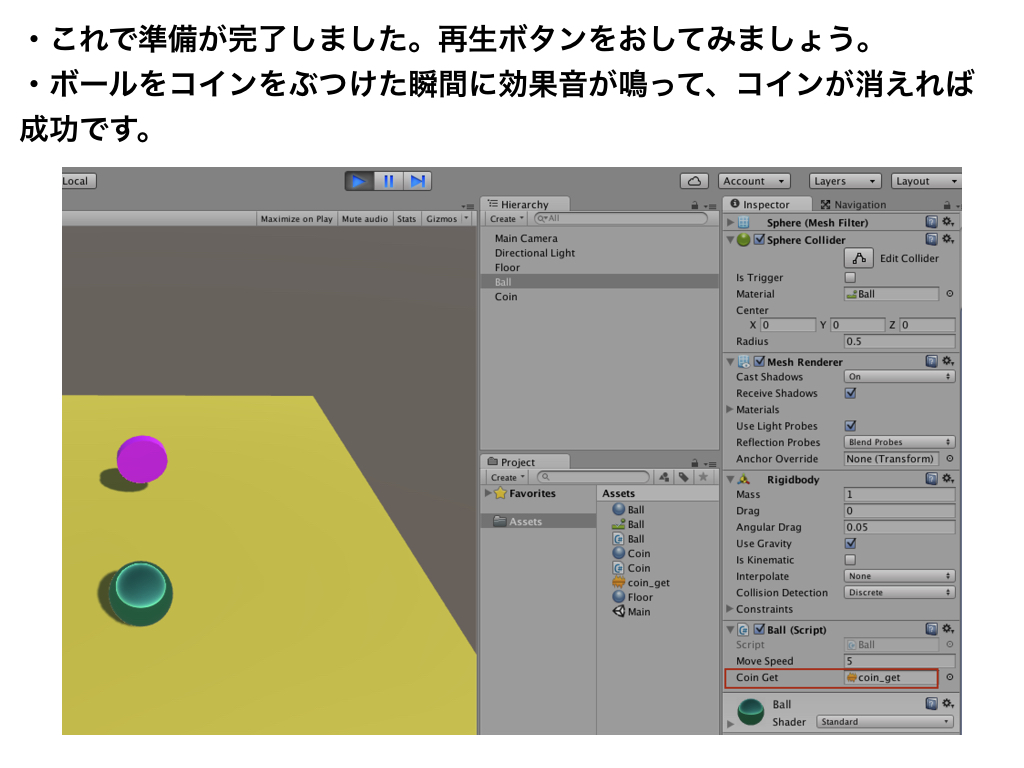
【2018版】BallGame(全25回)
他のコースを見る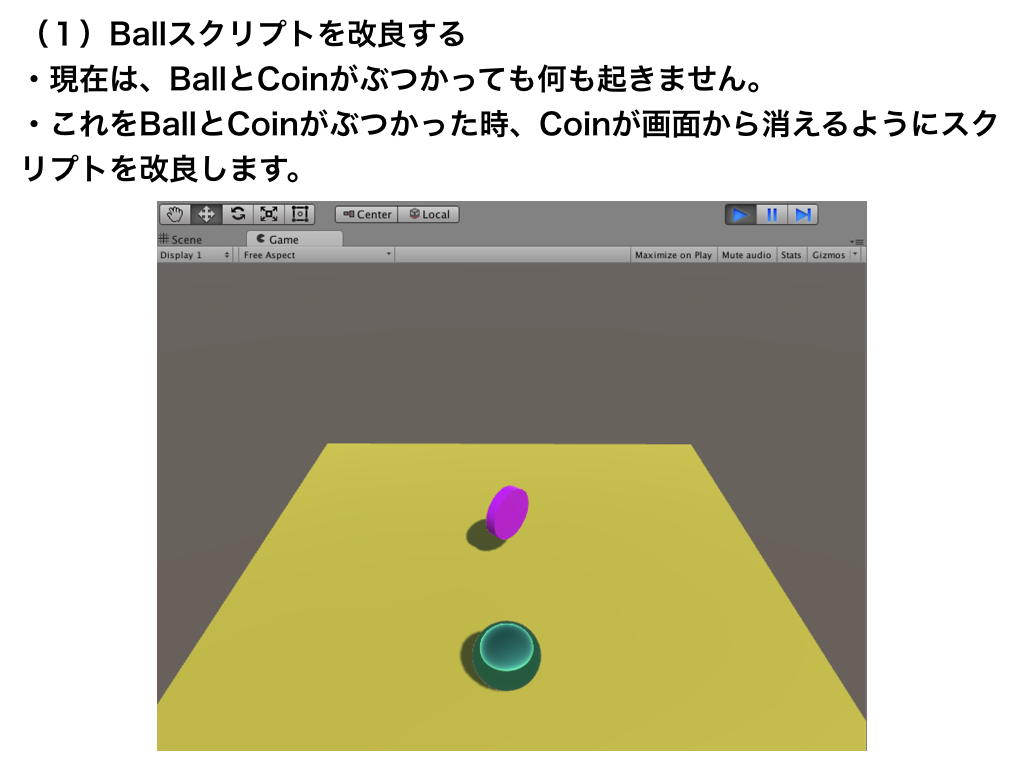
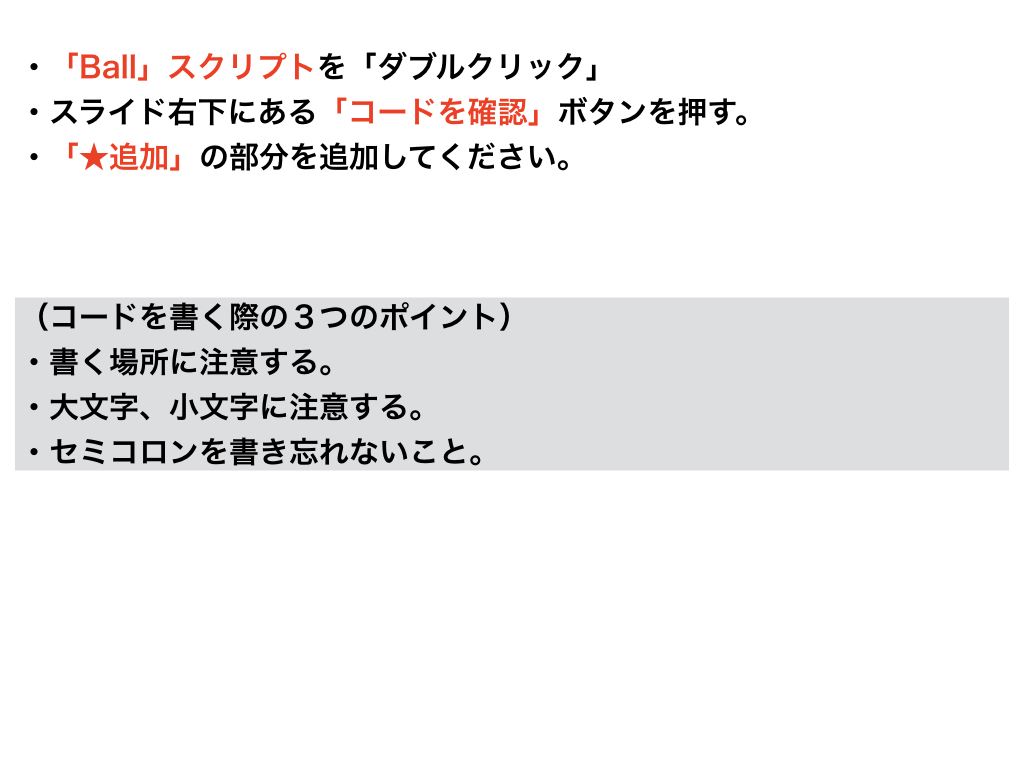
コインゲット
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
}
private void OnTriggerEnter(Collider other)
{
// もしもぶつかった相手に「Coin」という「Tag」が付いていたならば(条件)
if (other.CompareTag("Coin"))
{
// ぶつかった相手を破壊する(実行)
Destroy(other.gameObject);
}
}
}
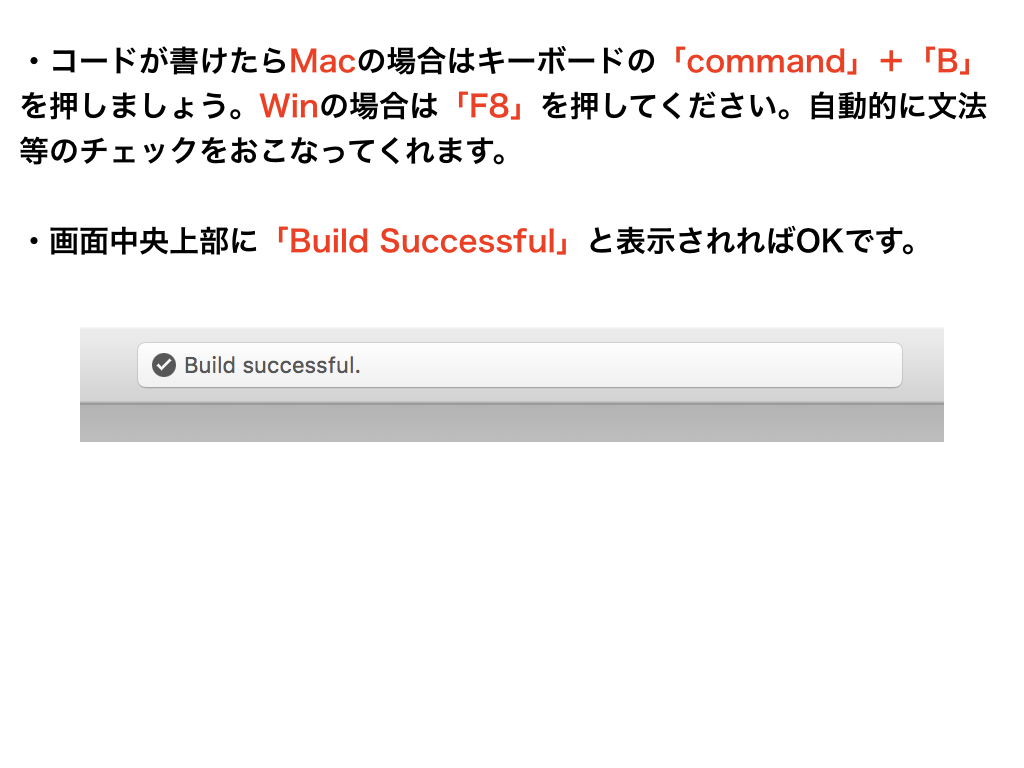
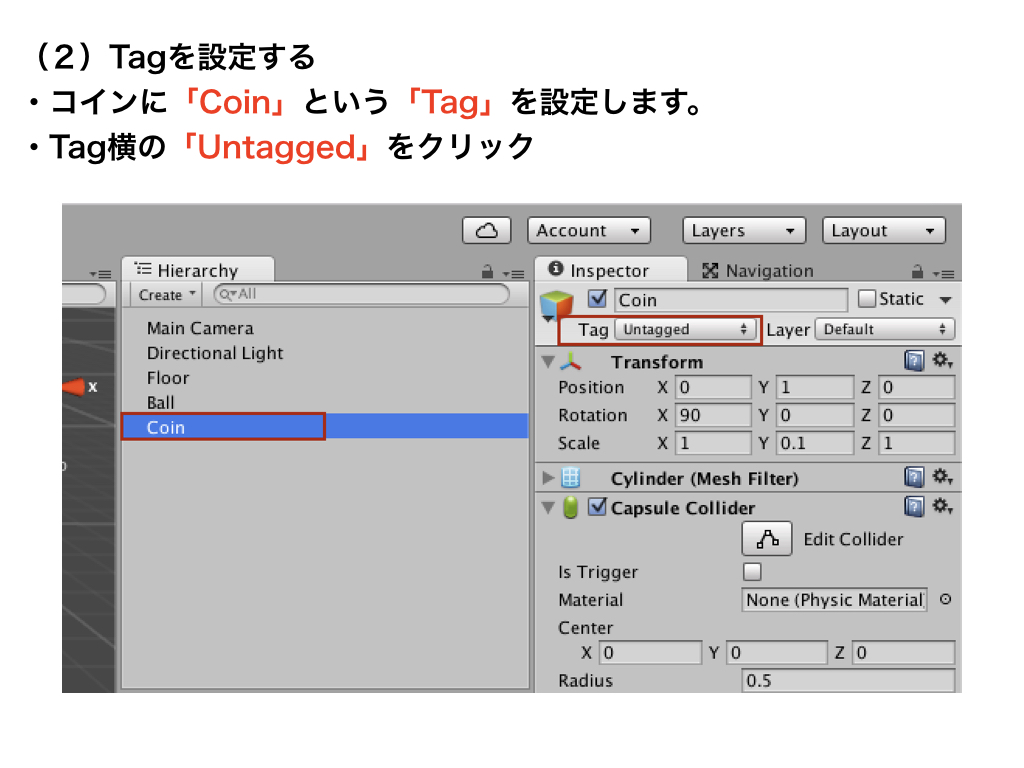
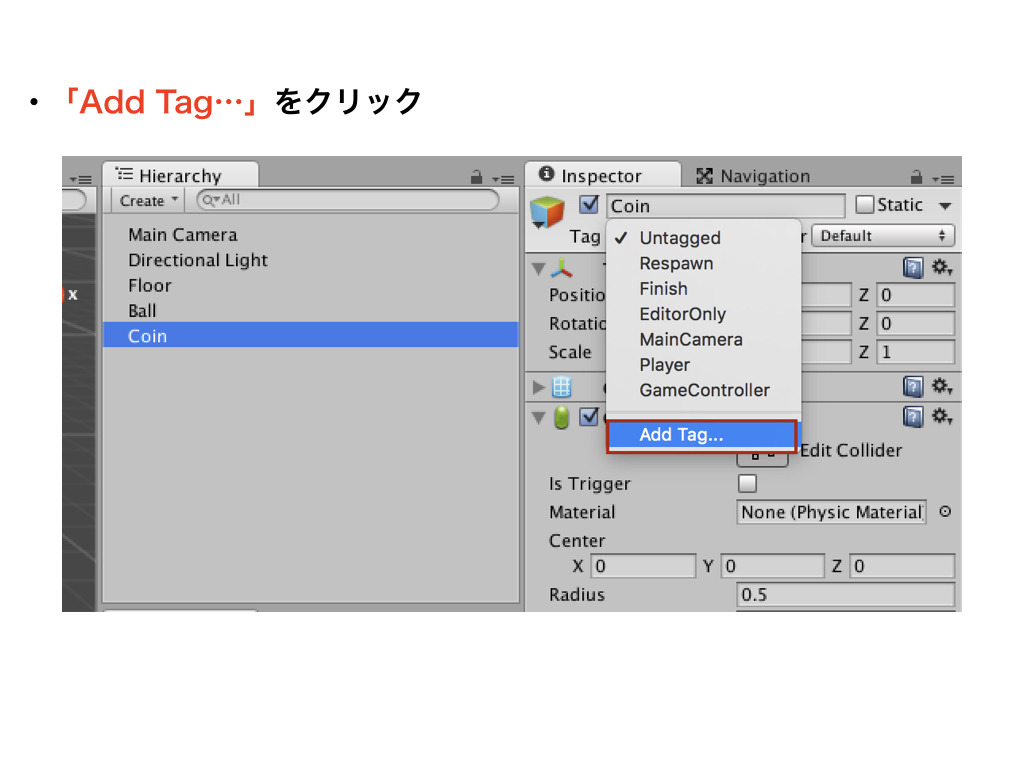
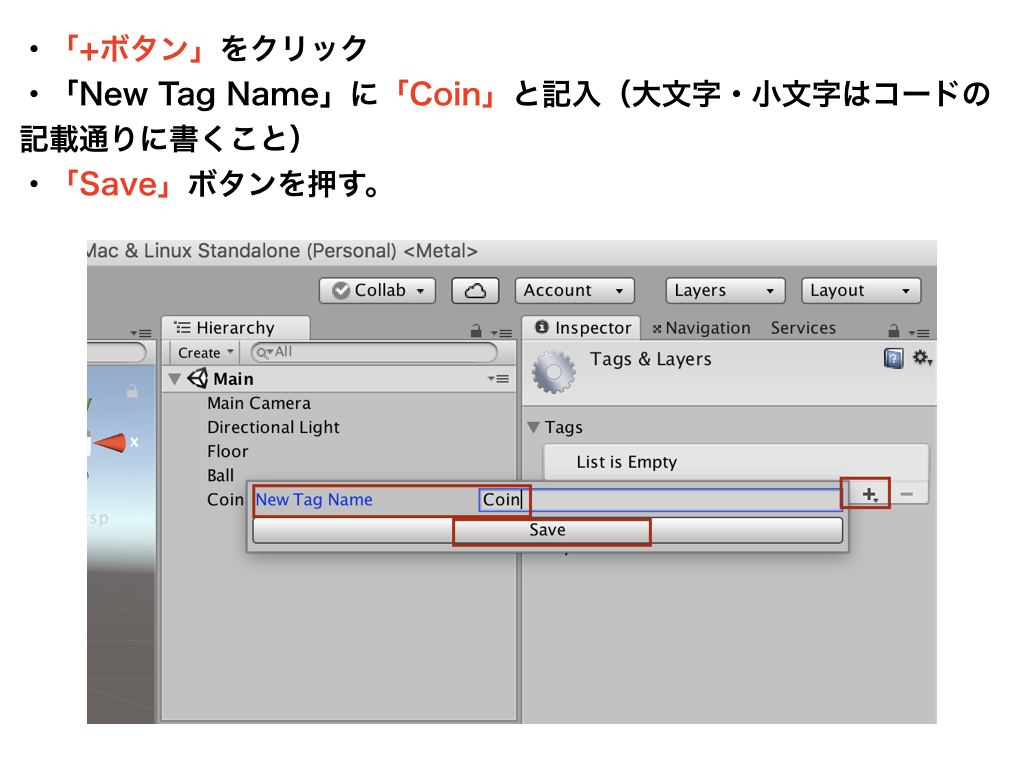
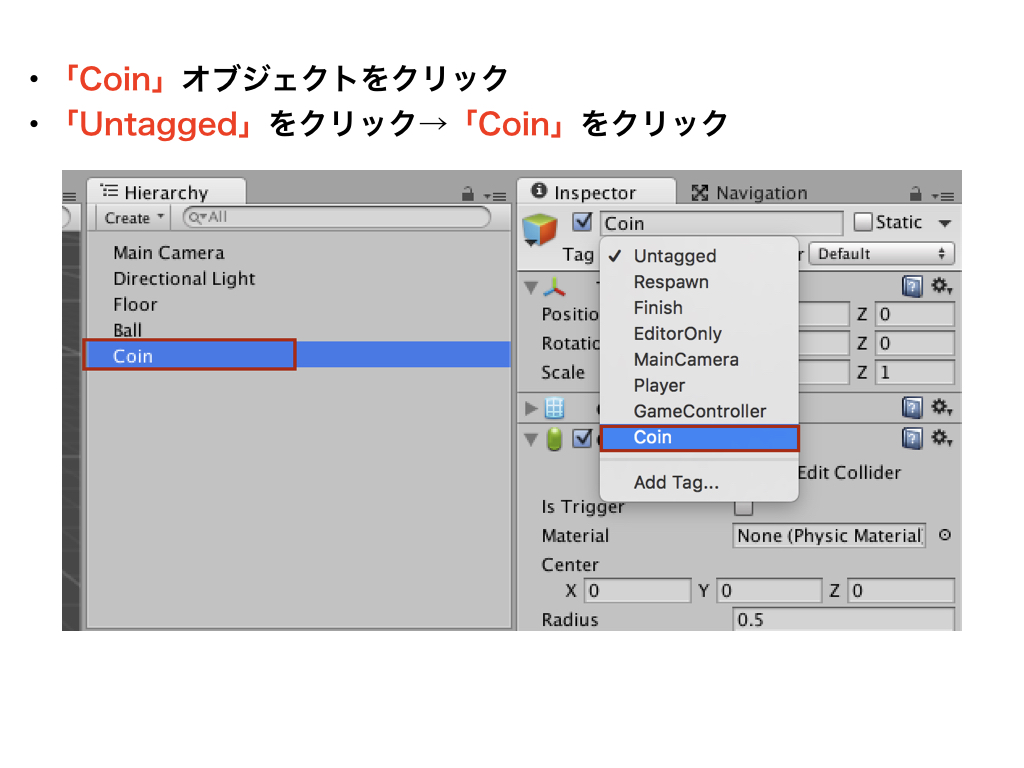
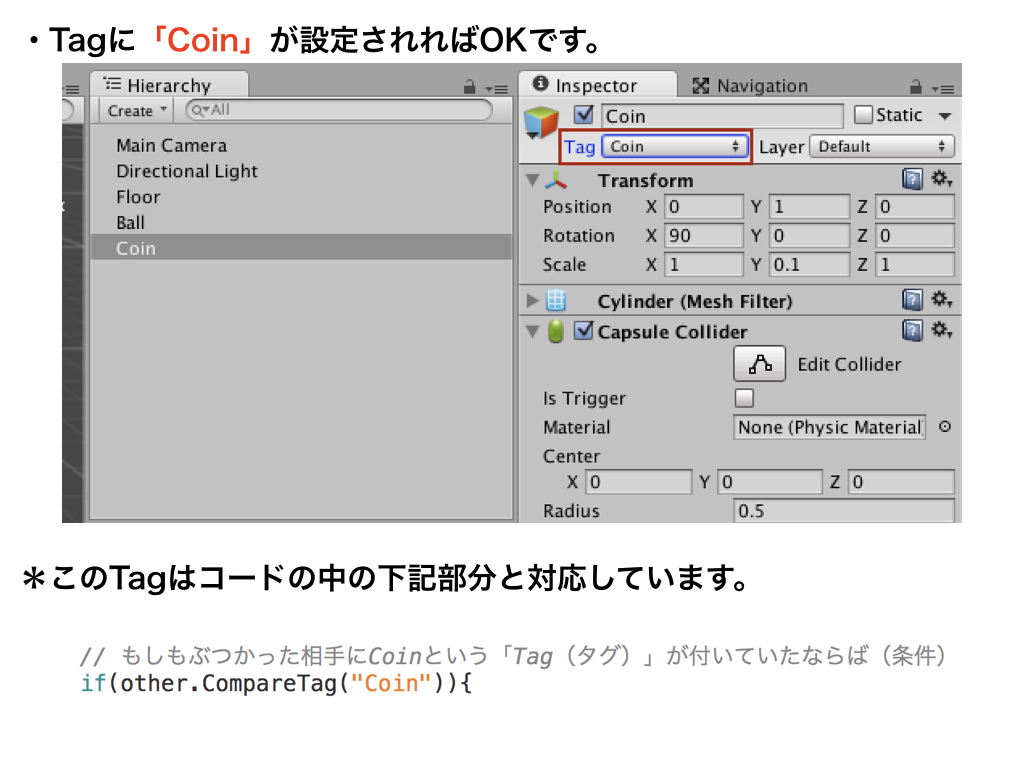
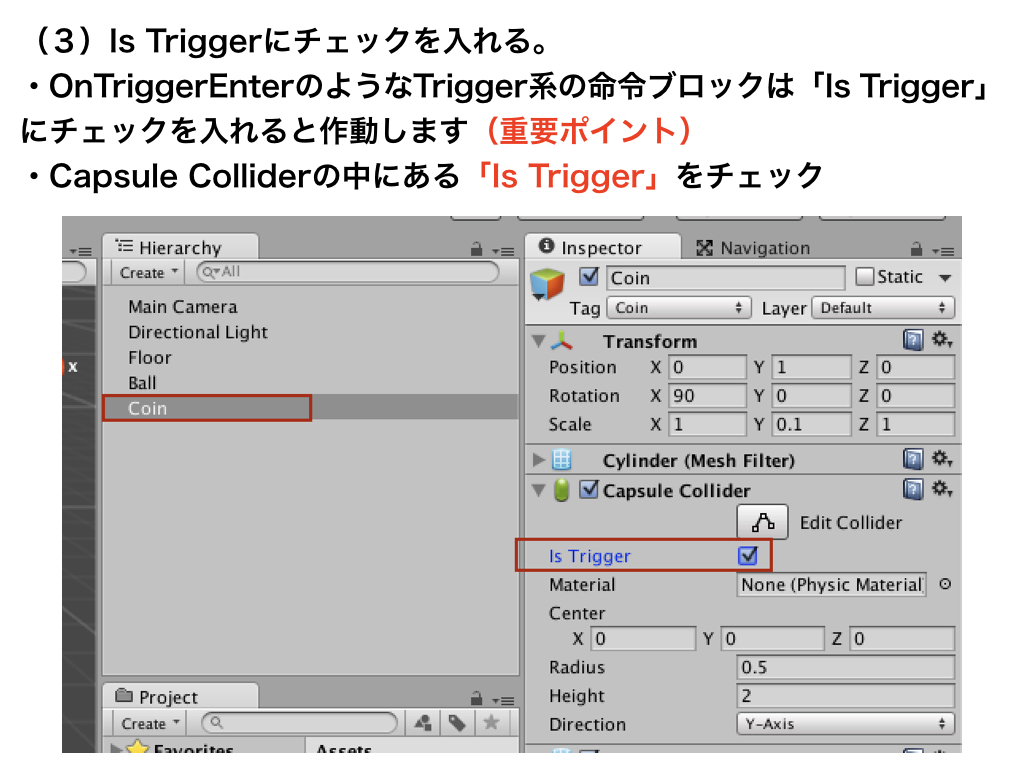
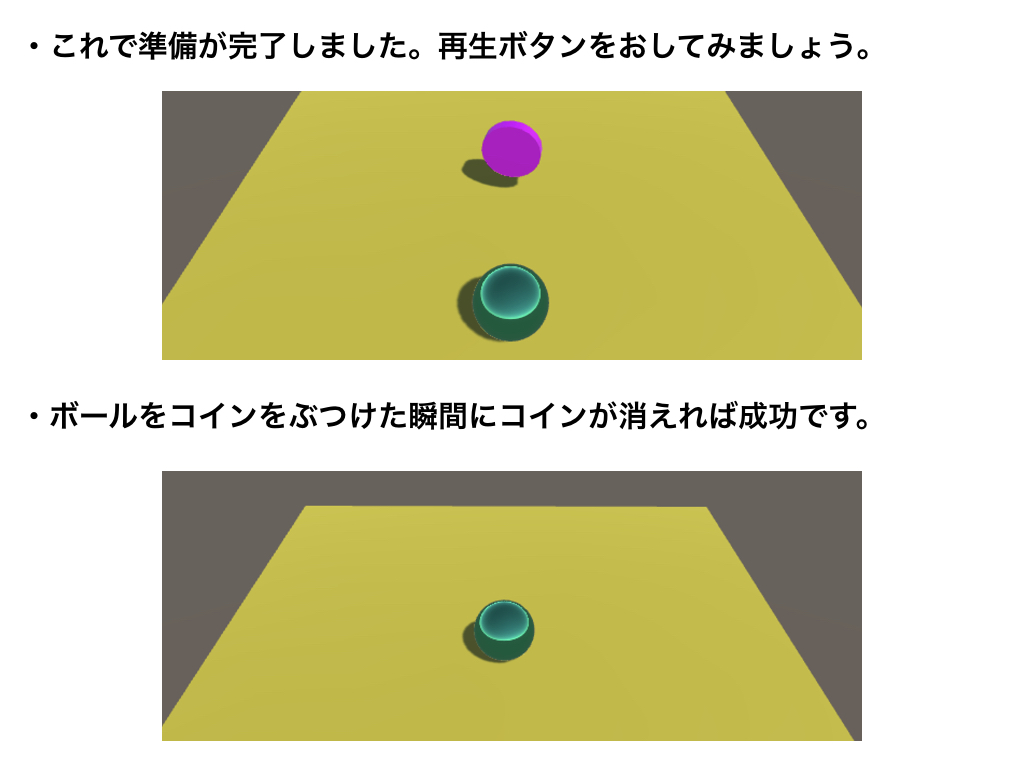
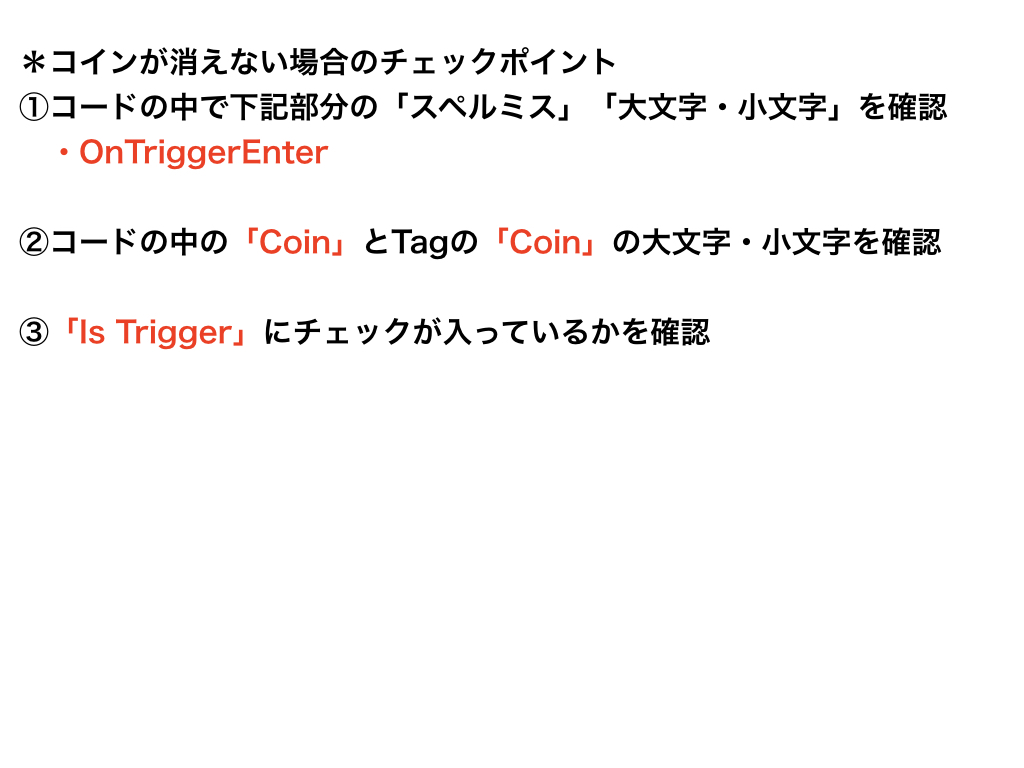
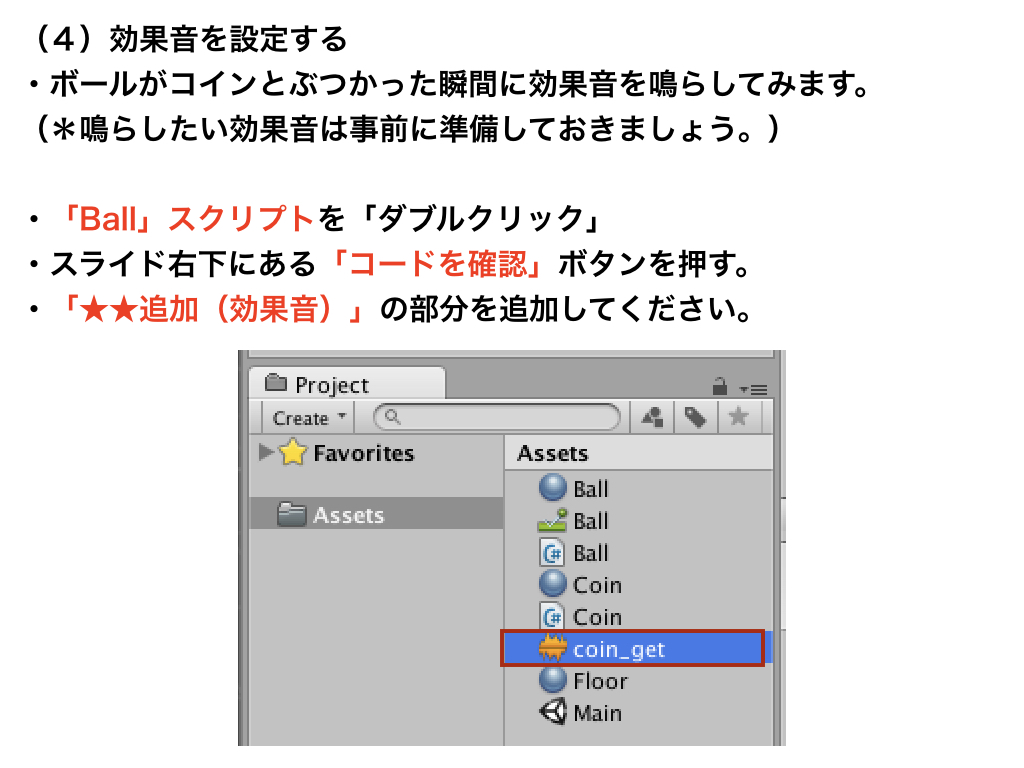
効果音の追加
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
// ★★追加(効果音)
public AudioClip coinGet;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
}
// ★追加
private void OnTriggerEnter(Collider other)
{
// もしもぶつかった相手に「Coin」という「Tag」が付いていたならば(条件)
if (other.CompareTag("Coin"))
{
// ぶつかった相手を破壊する(実行)
Destroy(other.gameObject);
// ★★追加(効果音)
AudioSource.PlayClipAtPoint(coinGet, transform.position);
}
}
}
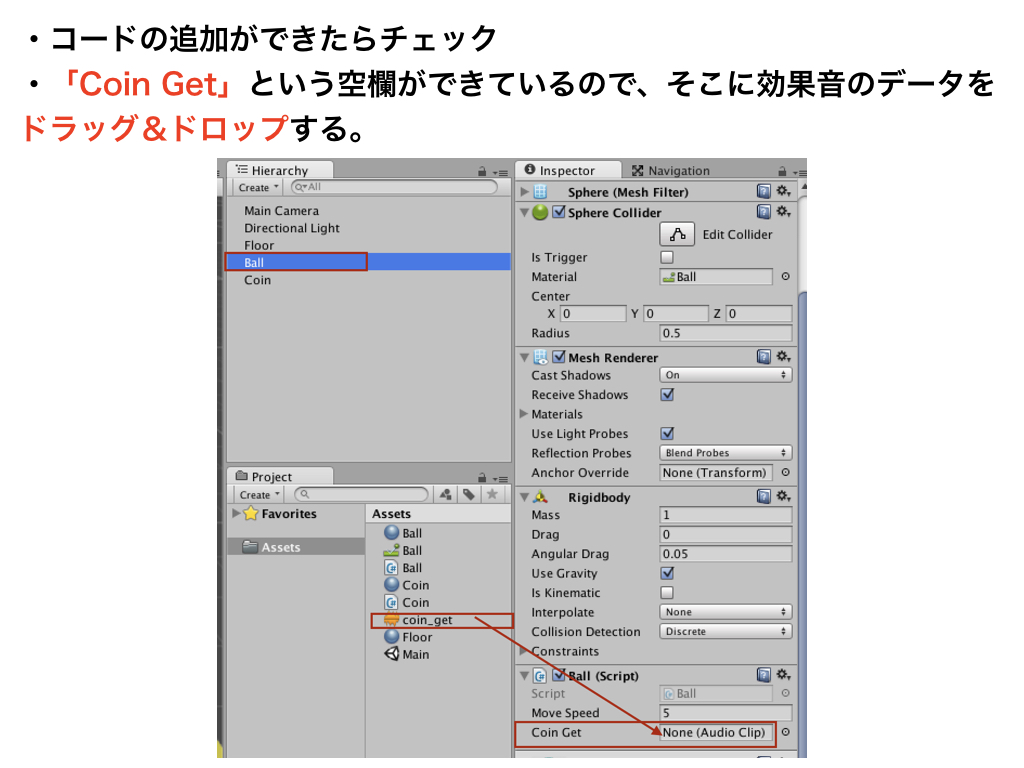
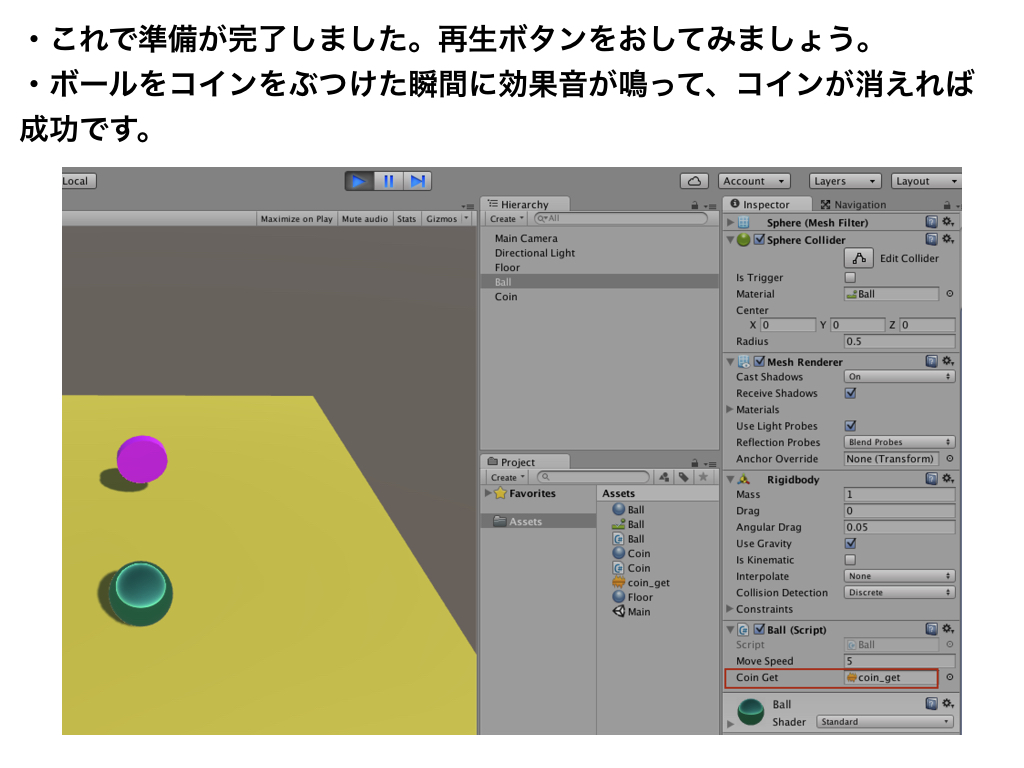
コインをゲットしよう