敵の攻撃を停止させるアイテムの作成
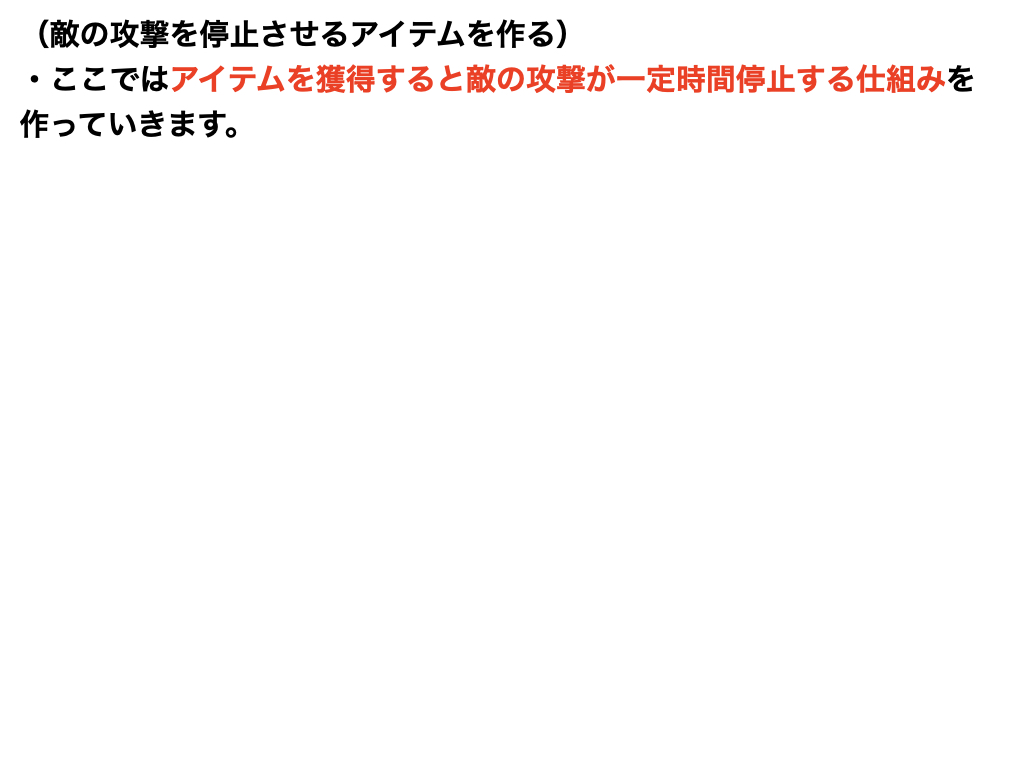
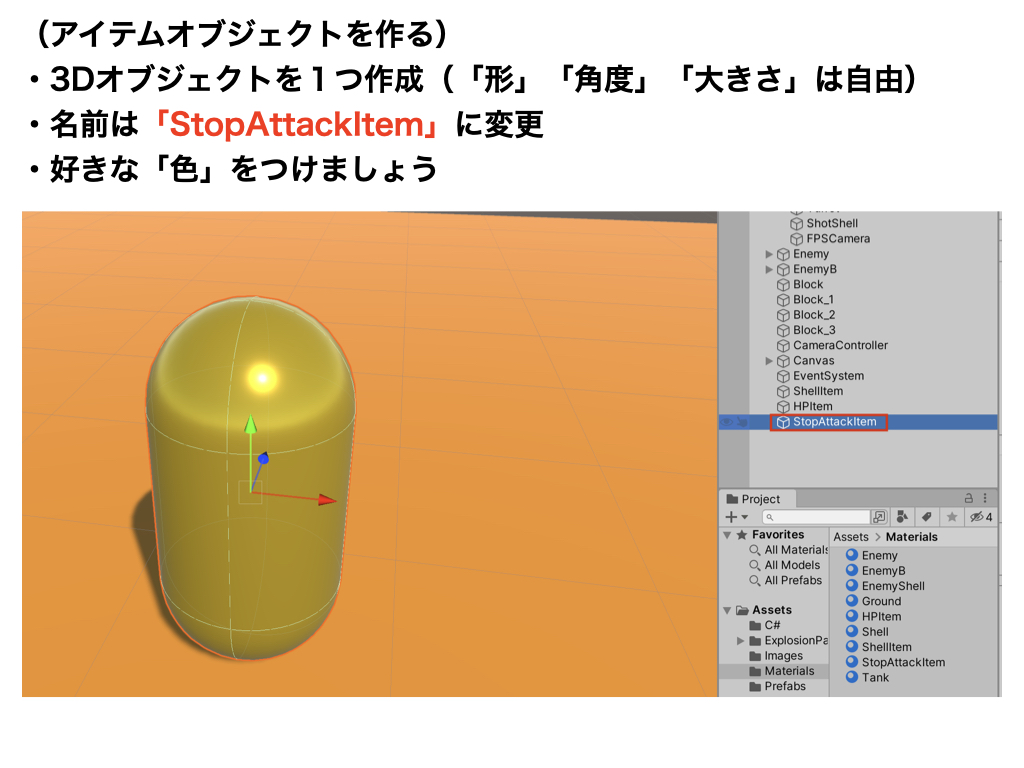
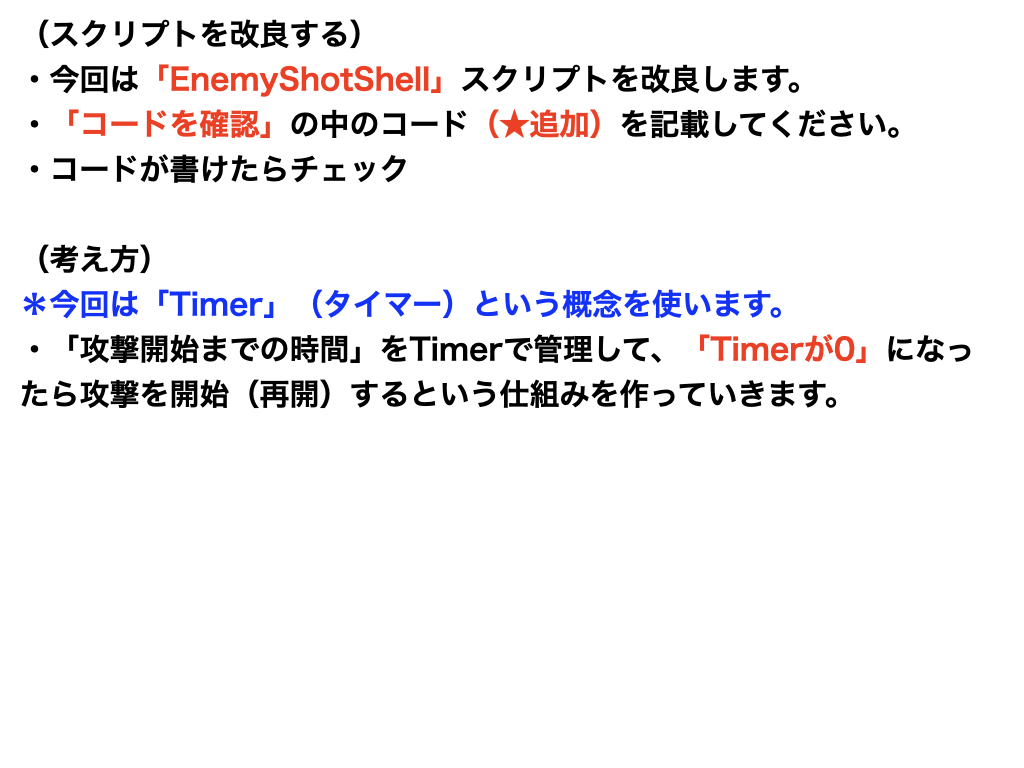
敵の攻撃の停止
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyShotShell : MonoBehaviour
{
public float shotSpeed;
public GameObject enemyShellPrefab;
public AudioClip shotSound;
private int count;
// ★追加
private float stopTimer = 5.0f; // 何秒間停止させるかは自由
private void Update()
{
count += 1;
// ★追加
stopTimer -= Time.deltaTime;
// ★追加
// ここの意味を考えてみよう。
if(stopTimer < 0)
{
stopTimer = 0;
}
// ★条件の追加
if(count % 100 == 0 && stopTimer <= 0)
{
GameObject enemyShell = Instantiate(enemyShellPrefab, transform.position, Quaternion.identity);
Rigidbody enemyShellRb = enemyShell.GetComponent<Rigidbody>();
enemyShellRb.AddForce(transform.forward * shotSpeed);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
Destroy(enemyShell, 3.0f);
}
}
// ★追加
// 敵の攻撃を停止させるメソッド
// (復習)このメソッドは外部からアクセスできるように「public」をつける(重要)
// (復習)このメソッドをStopAttackItemスクリプトから呼び出す。
public void AddStopTimer(float amount)
{
stopTimer += amount;
}
}
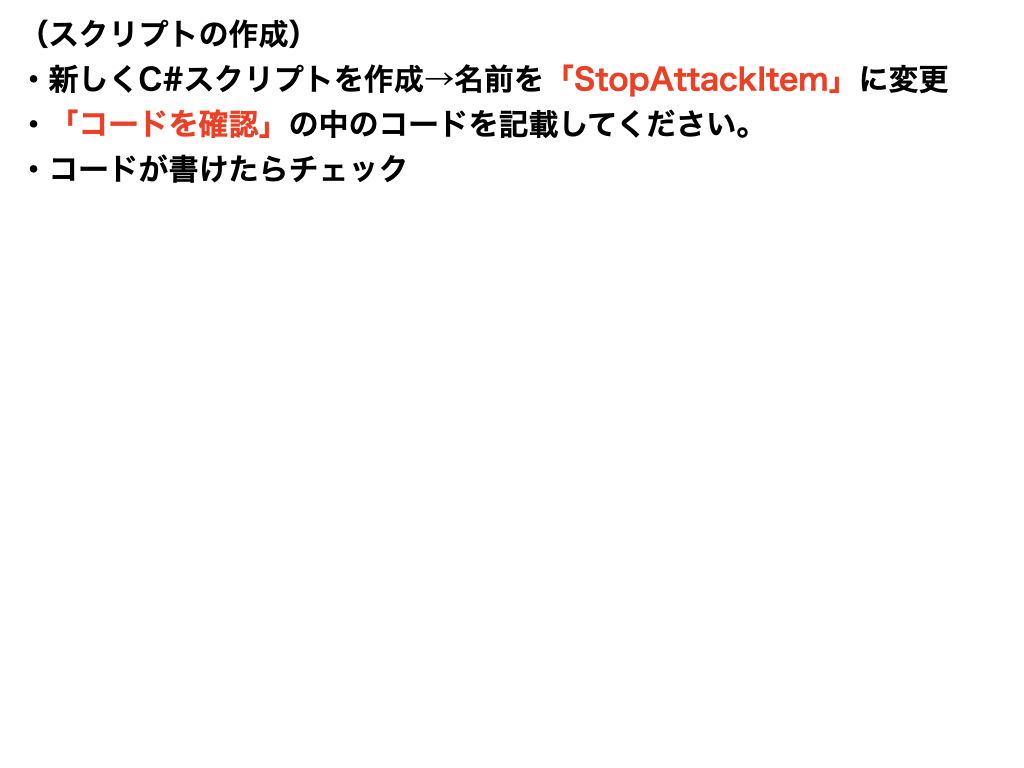
敵の攻撃の停止(アイテム)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class StopAttackItem : MonoBehaviour
{
// 「配列」(プログラミング・ポイント)
private GameObject[] targets;
public AudioClip getSound;
public GameObject effectPrefab;
private void OnTriggerEnter(Collider other)
{
if(other.CompareTag("Player"))
{
// 「EnemyShotShell」オブジェクトに「EnemyShotShell」タグを設定してください(ポイント)
targets = GameObject.FindGameObjectsWithTag("EnemyShotShell");
// 「繰り返し文」(プログラミング・ポイント)
// 「foreach」の使い方を学習しよう。
foreach(GameObject t in targets)
{
// 攻撃停止時間を「3秒」加算する(自由に変更可能)
t.GetComponent<EnemyShotShell>().AddStopTimer(3.0f);
}
Destroy(gameObject);
AudioSource.PlayClipAtPoint(getSound, transform.position);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
}
}
}
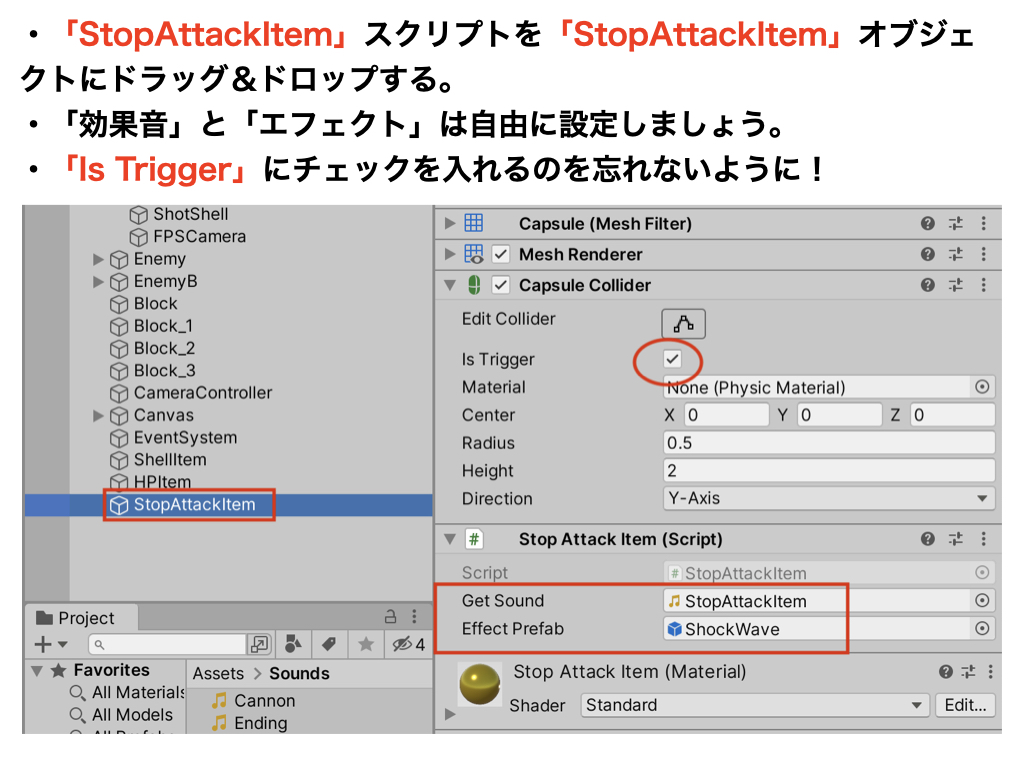
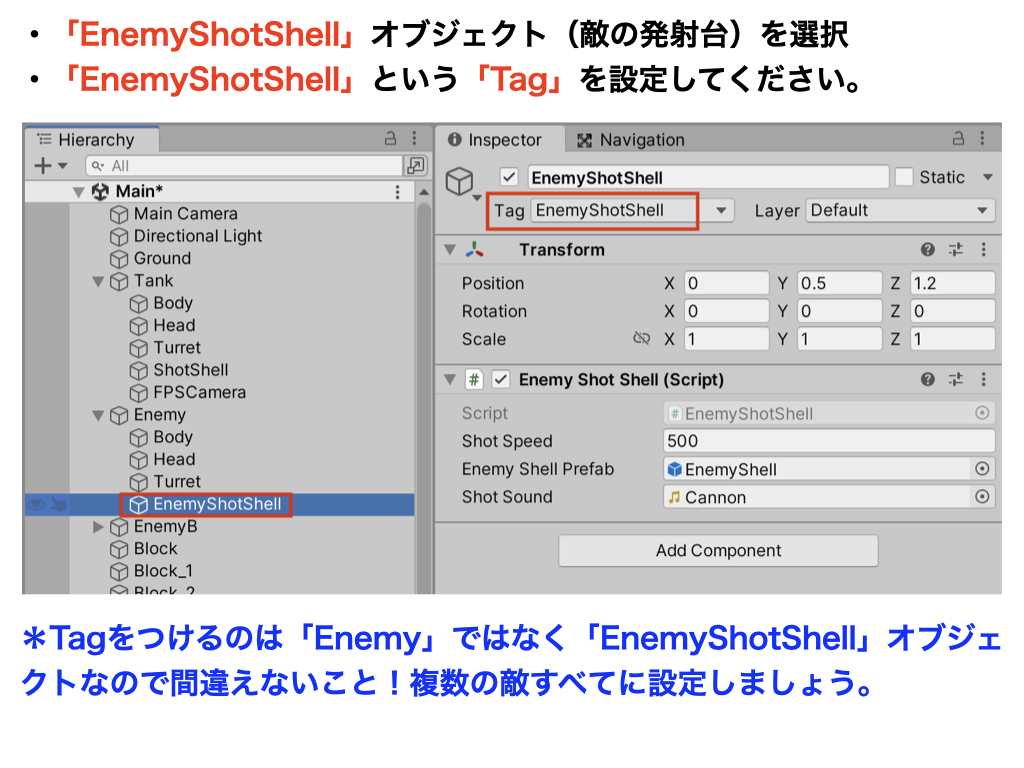
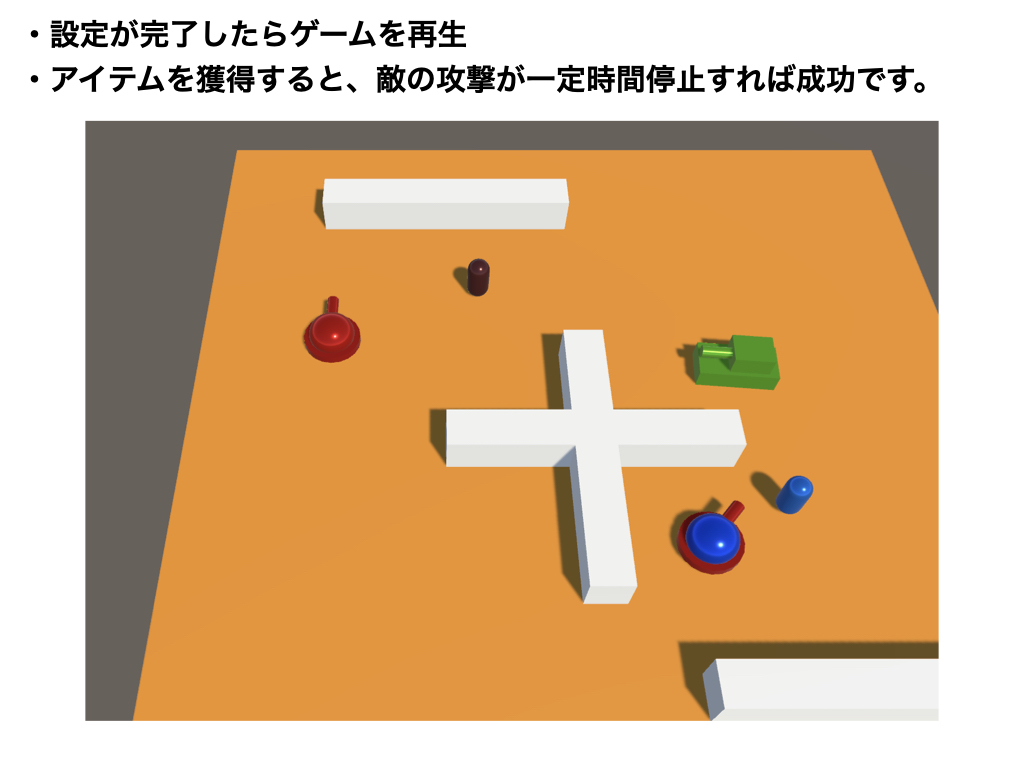
【2021版】BattleTank(基礎/全33回)
他のコースを見る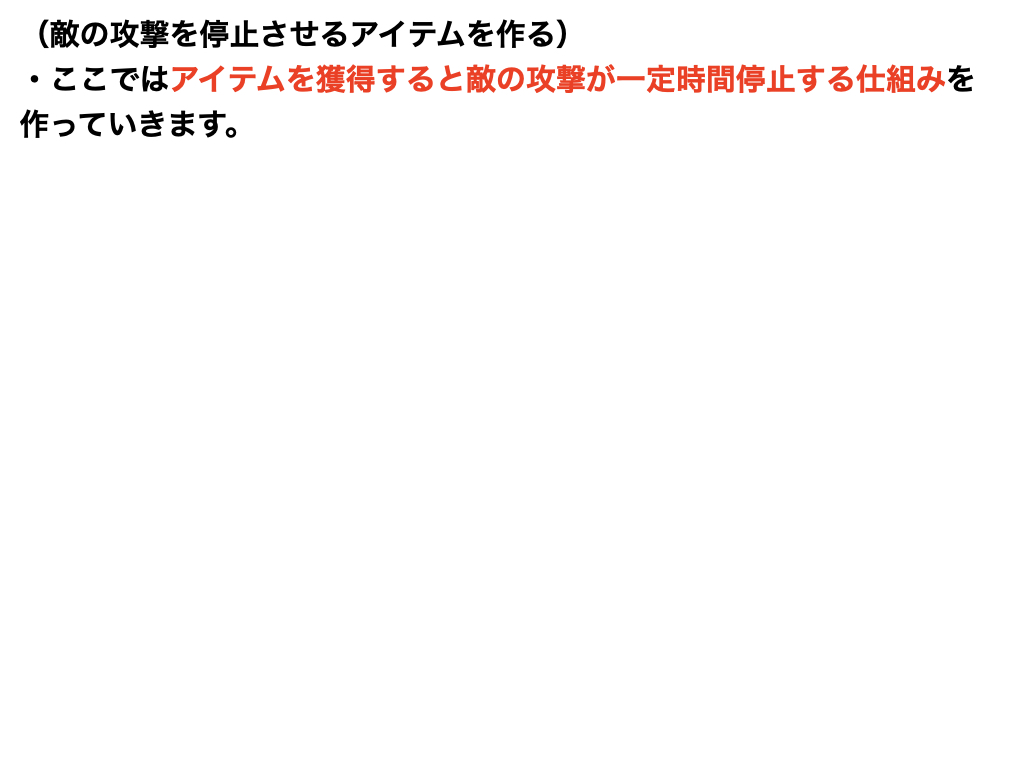
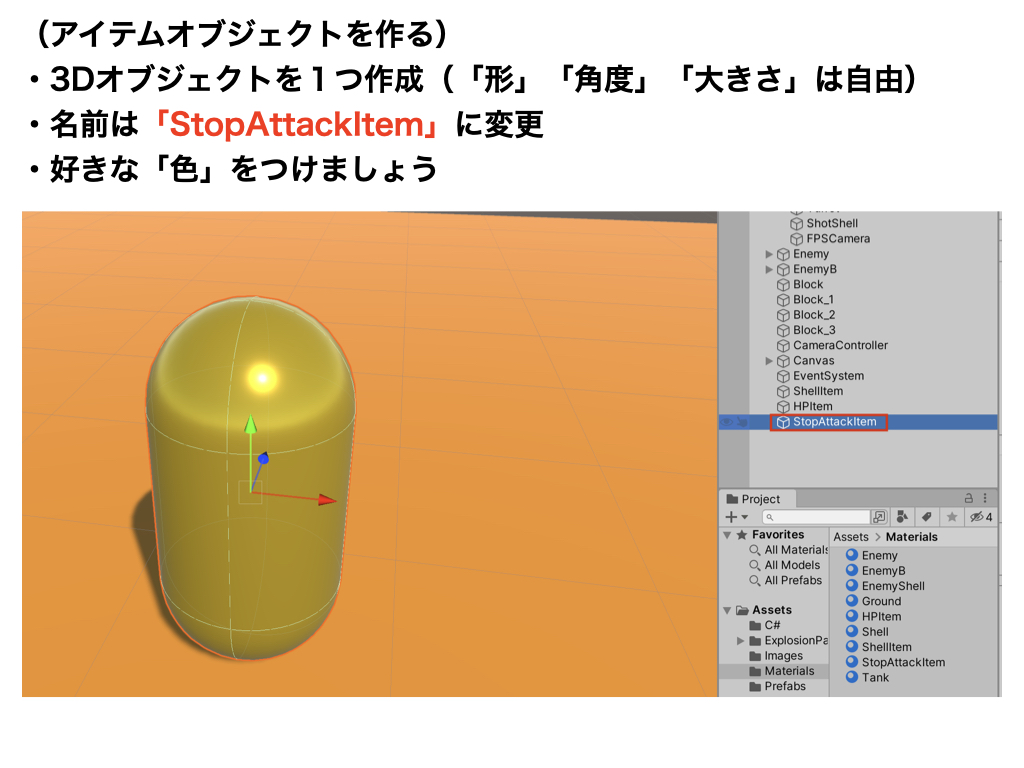
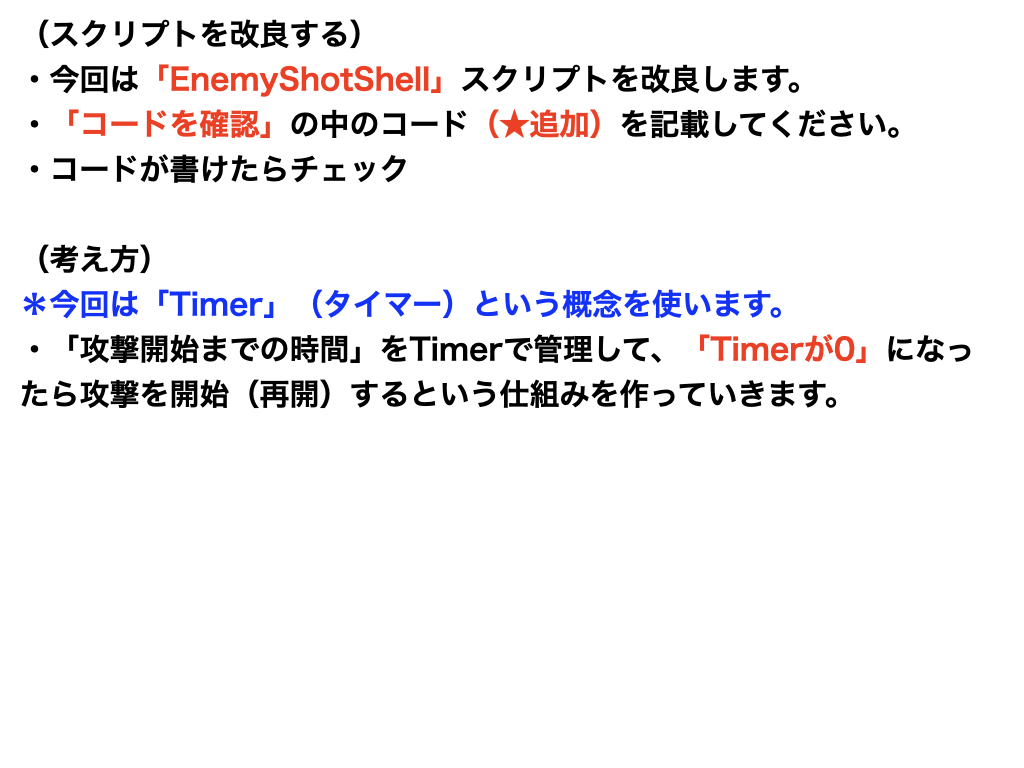
敵の攻撃の停止
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyShotShell : MonoBehaviour
{
public float shotSpeed;
public GameObject enemyShellPrefab;
public AudioClip shotSound;
private int count;
// ★追加
private float stopTimer = 5.0f; // 何秒間停止させるかは自由
private void Update()
{
count += 1;
// ★追加
stopTimer -= Time.deltaTime;
// ★追加
// ここの意味を考えてみよう。
if(stopTimer < 0)
{
stopTimer = 0;
}
// ★条件の追加
if(count % 100 == 0 && stopTimer <= 0)
{
GameObject enemyShell = Instantiate(enemyShellPrefab, transform.position, Quaternion.identity);
Rigidbody enemyShellRb = enemyShell.GetComponent<Rigidbody>();
enemyShellRb.AddForce(transform.forward * shotSpeed);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
Destroy(enemyShell, 3.0f);
}
}
// ★追加
// 敵の攻撃を停止させるメソッド
// (復習)このメソッドは外部からアクセスできるように「public」をつける(重要)
// (復習)このメソッドをStopAttackItemスクリプトから呼び出す。
public void AddStopTimer(float amount)
{
stopTimer += amount;
}
}
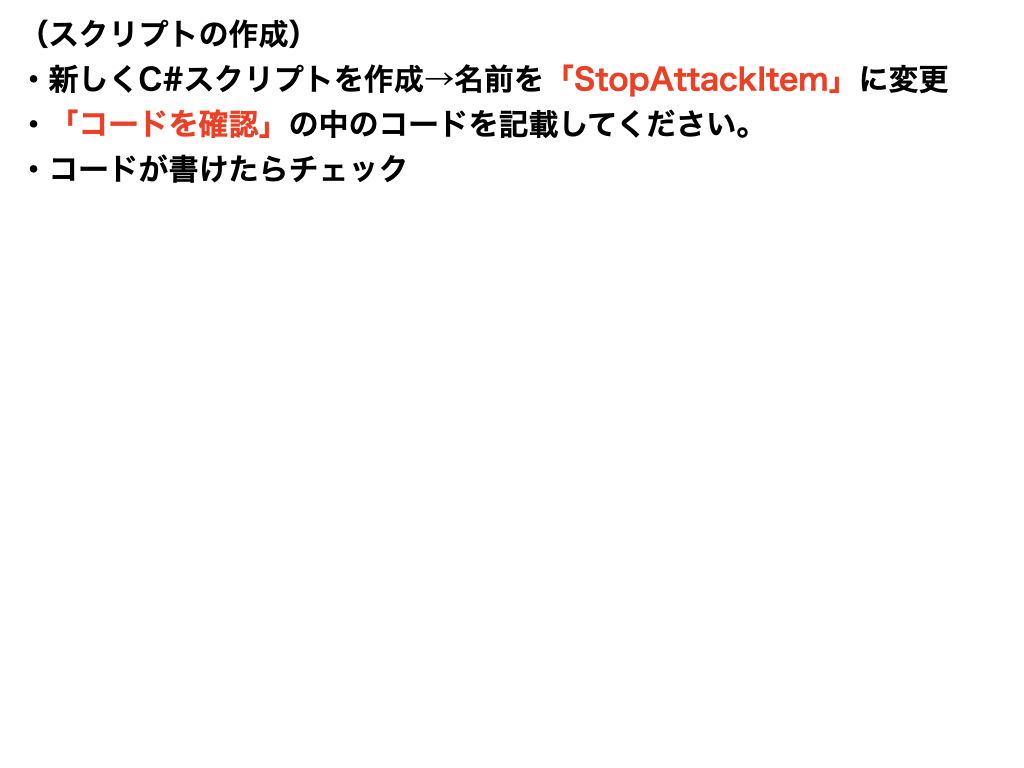
敵の攻撃の停止(アイテム)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class StopAttackItem : MonoBehaviour
{
// 「配列」(プログラミング・ポイント)
private GameObject[] targets;
public AudioClip getSound;
public GameObject effectPrefab;
private void OnTriggerEnter(Collider other)
{
if(other.CompareTag("Player"))
{
// 「EnemyShotShell」オブジェクトに「EnemyShotShell」タグを設定してください(ポイント)
targets = GameObject.FindGameObjectsWithTag("EnemyShotShell");
// 「繰り返し文」(プログラミング・ポイント)
// 「foreach」の使い方を学習しよう。
foreach(GameObject t in targets)
{
// 攻撃停止時間を「3秒」加算する(自由に変更可能)
t.GetComponent<EnemyShotShell>().AddStopTimer(3.0f);
}
Destroy(gameObject);
AudioSource.PlayClipAtPoint(getSound, transform.position);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
}
}
}
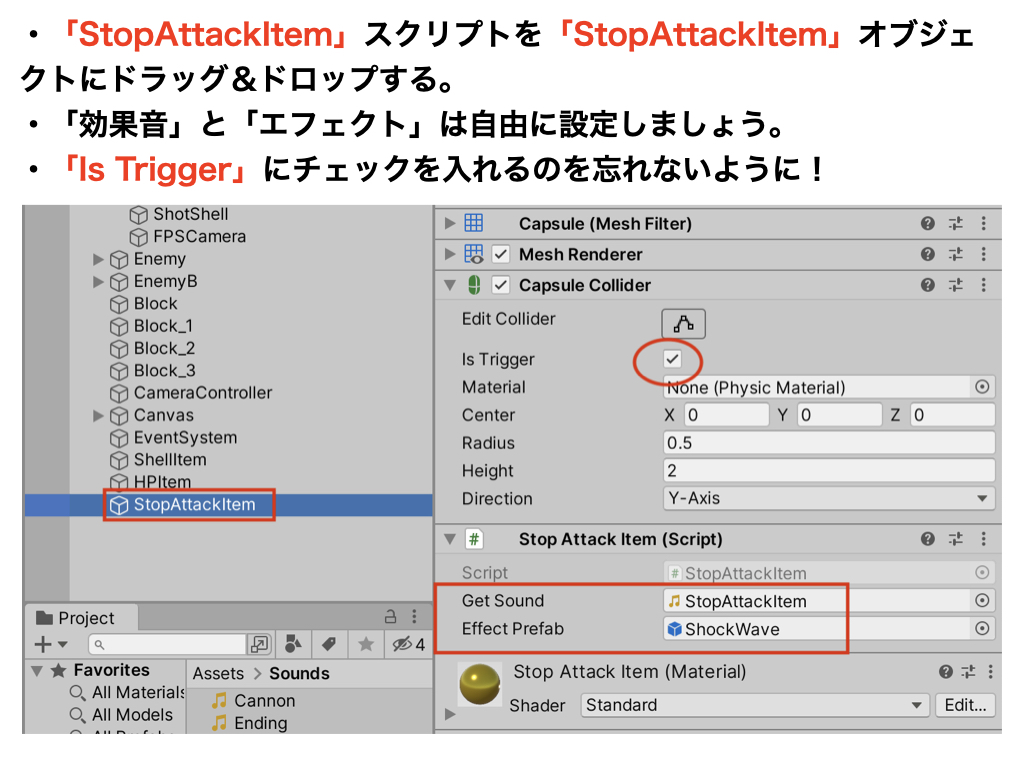
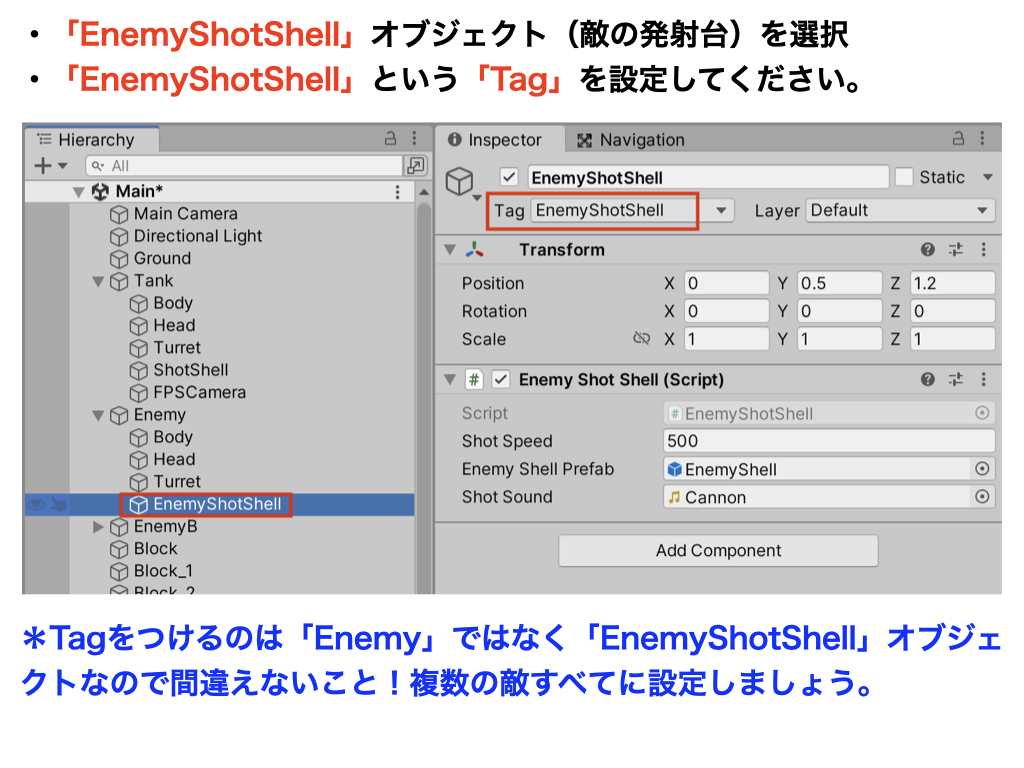
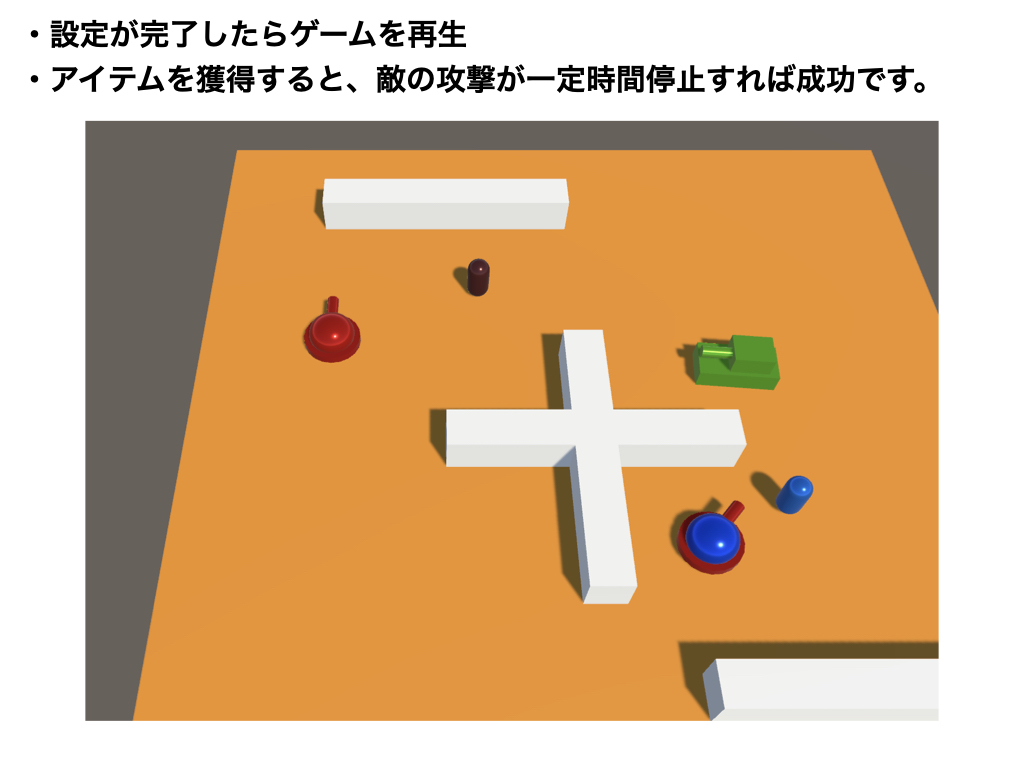
敵の攻撃を停止させるアイテムの作成