プレーヤーのHPを回復させるアイテムの作成
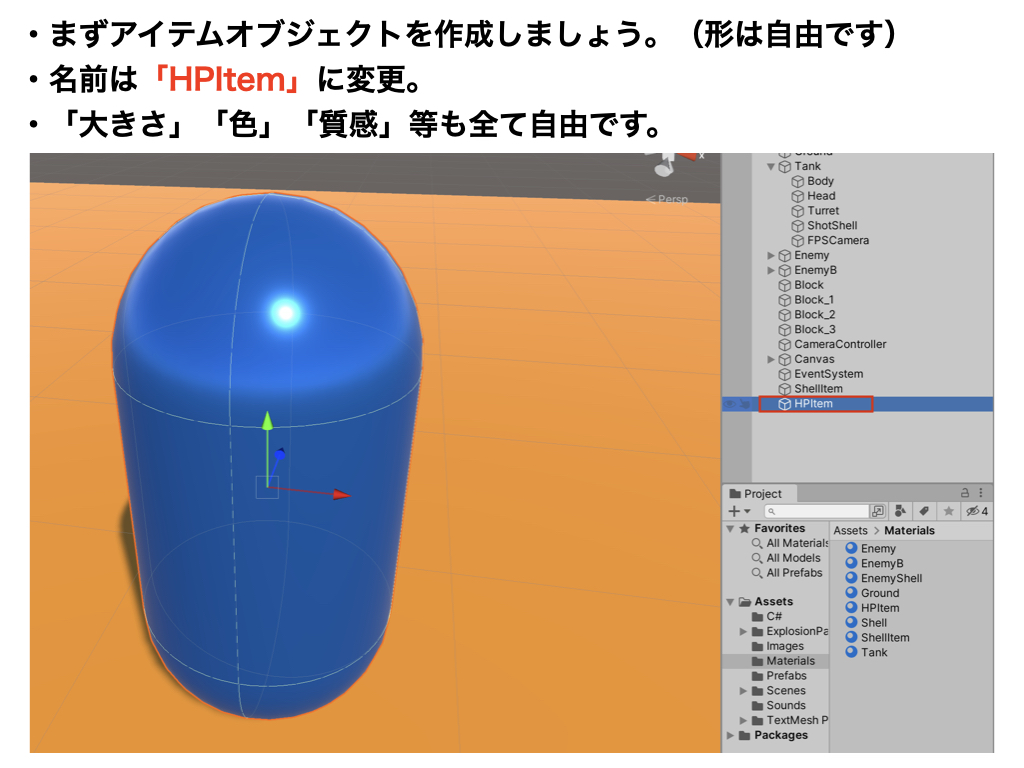
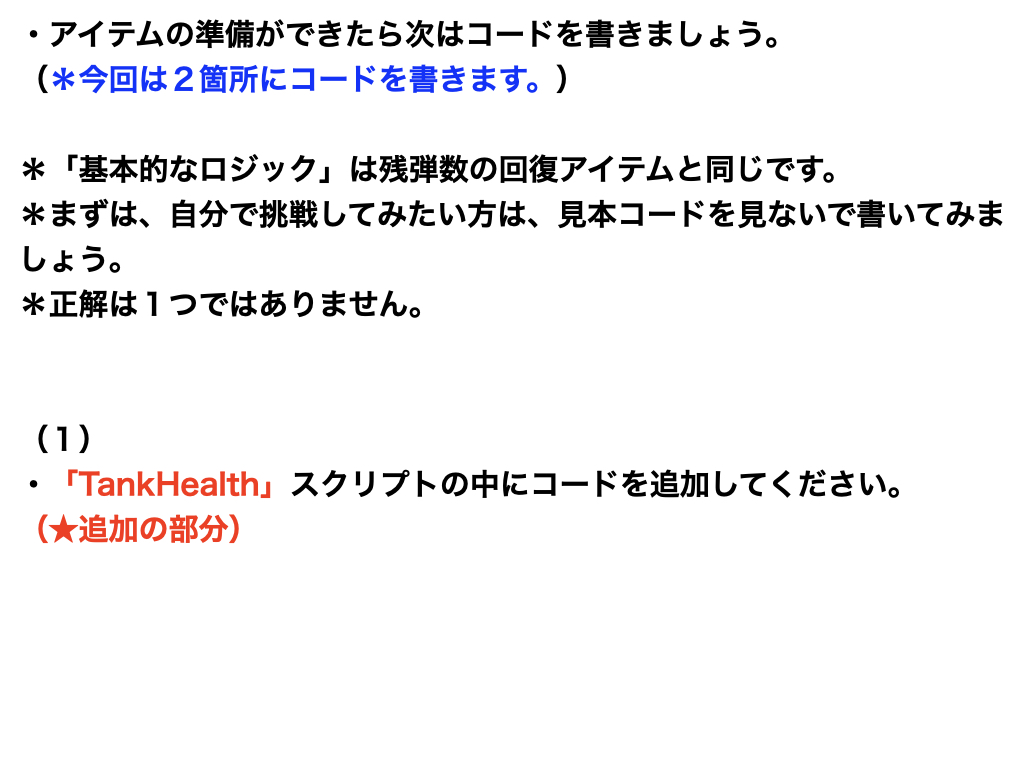
HPを回復させる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using TMPro;
public class TankHealth : MonoBehaviour
{
public GameObject effectPrefab1;
public GameObject effectPrefab2;
public int tankHP;
public TextMeshProUGUI hpLabel;
// ★追加
private int tankMaxHP = 10; // 最大値は自由
private void Start()
{
// ★追加
tankHP = tankMaxHP;
hpLabel.text = "" + tankHP;
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("EnemyShell"))
{
tankHP -= 1;
hpLabel.text = "" + tankHP;
Destroy(other.gameObject);
if (tankHP > 0)
{
GameObject effect1 = Instantiate(effectPrefab1, transform.position, Quaternion.identity);
Destroy(effect1, 1.0f);
}
else
{
GameObject effect2 = Instantiate(effectPrefab2, transform.position, Quaternion.identity);
Destroy(effect2, 1.0f);
this.gameObject.SetActive(false);
Invoke("GoToGameOver", 1.5f);
}
}
}
void GoToGameOver()
{
SceneManager.LoadScene("GameOver");
}
// ★追加
// publicをつけること(「public」の意味を復習しましょう!)
public void AddHP(int amount)
{
tankHP += amount;
// ここは何をコントロールしているでしょうか?
if(tankHP > tankMaxHP)
{
tankHP = tankMaxHP;
}
hpLabel.text = "" + tankHP;
}
}
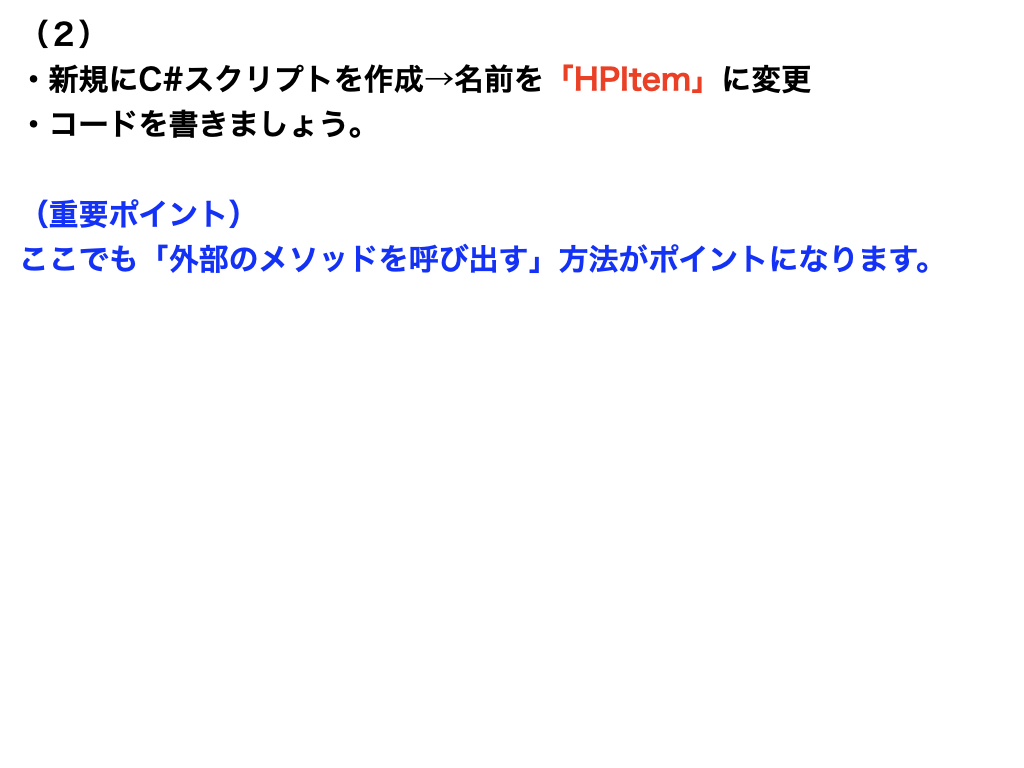
HPを回復させる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HPItem : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip getSound;
private TankHealth th;
private int reward = 3; // いくつ回復させるかは自由!
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Player"))
{
// Find()メソッドの使い方をマスターすること
th = GameObject.Find("Tank").GetComponent<TankHealth>();
// ここの意味をしっかり復習すること。
// AddHP()メソッドを呼び出して「引数」にrewardを与えている。
th.AddHP(reward);
Destroy(gameObject);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
AudioSource.PlayClipAtPoint(getSound, transform.position);
}
}
}
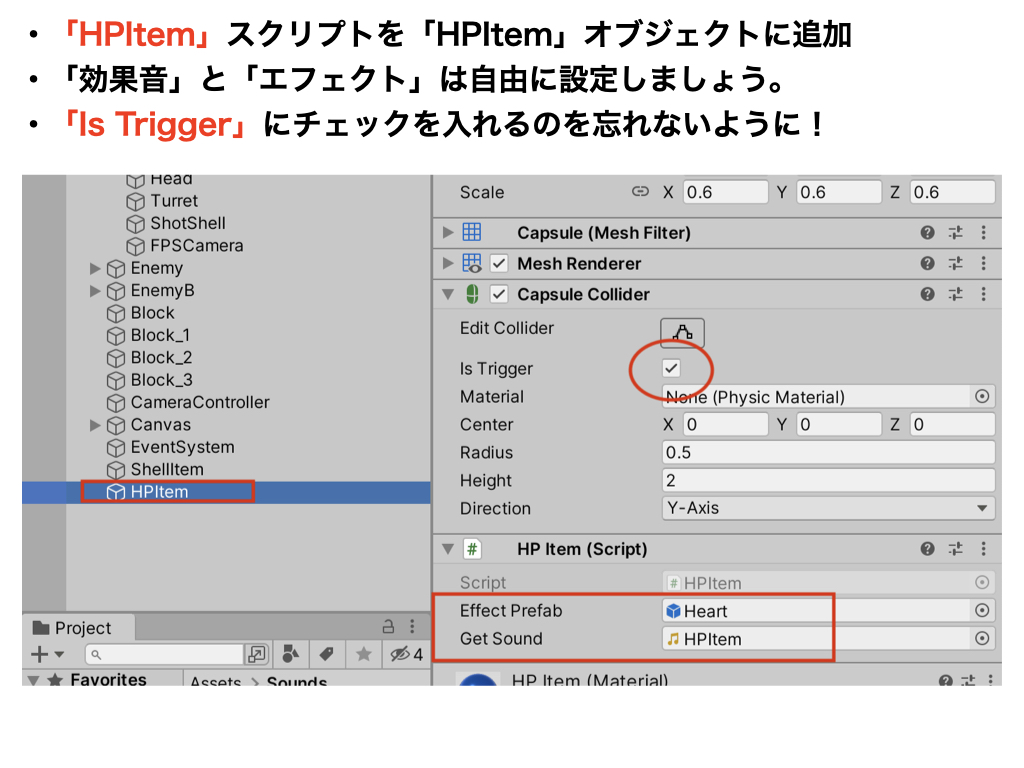
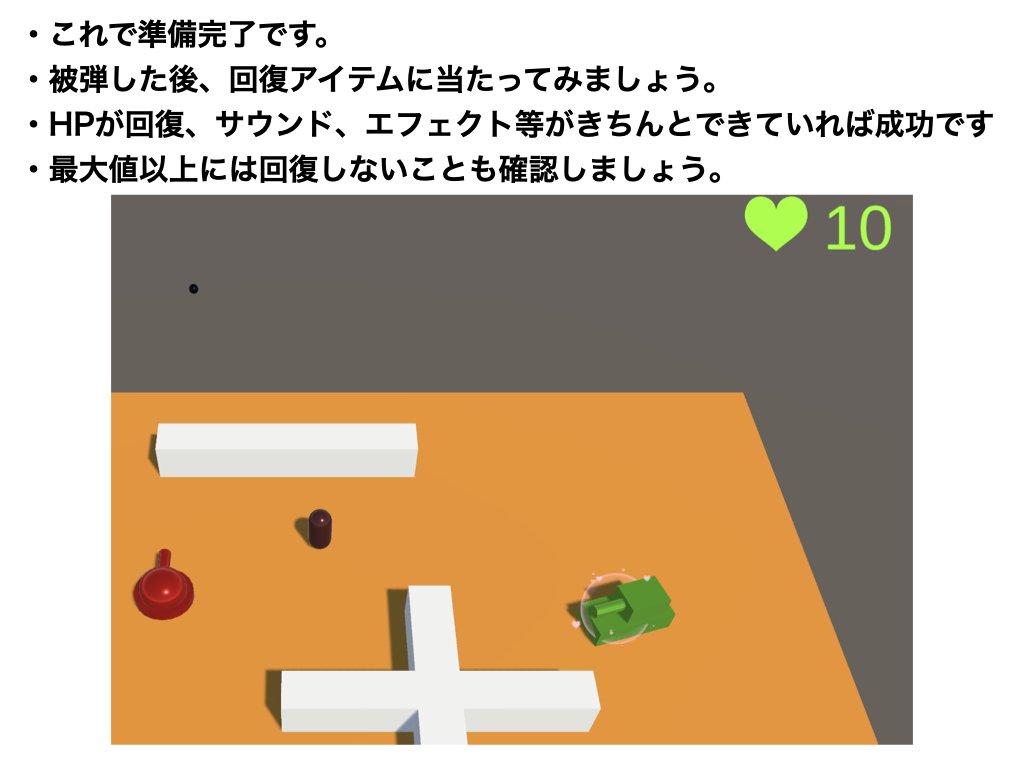
【2021版】BattleTank(基礎/全33回)
他のコースを見る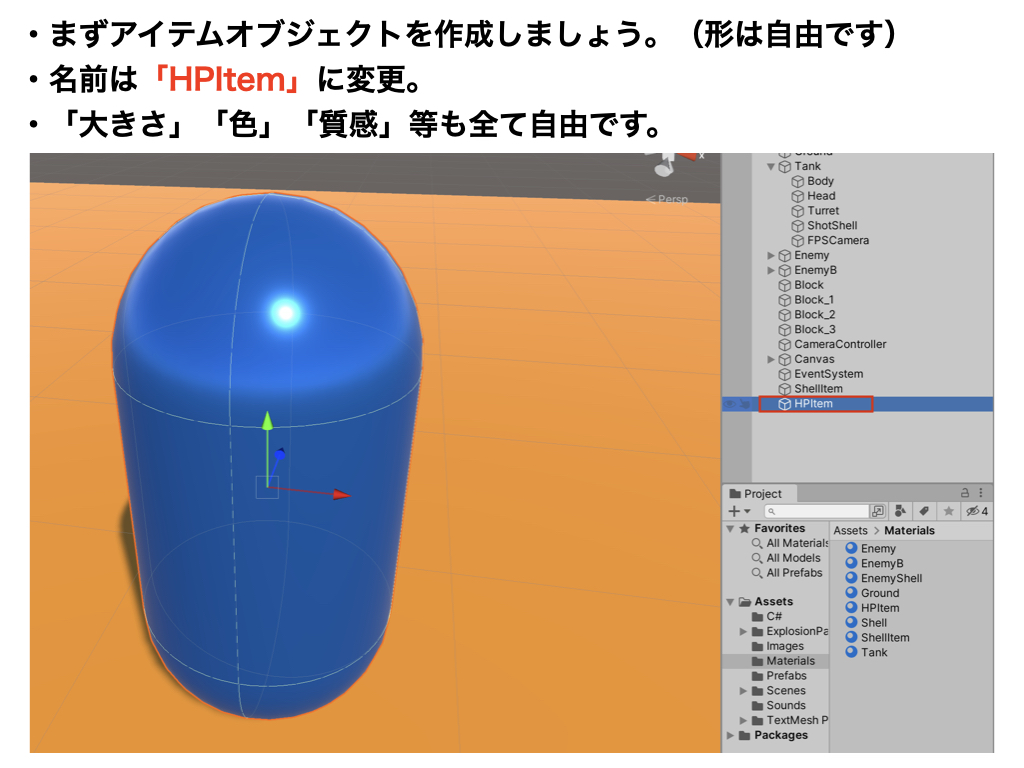
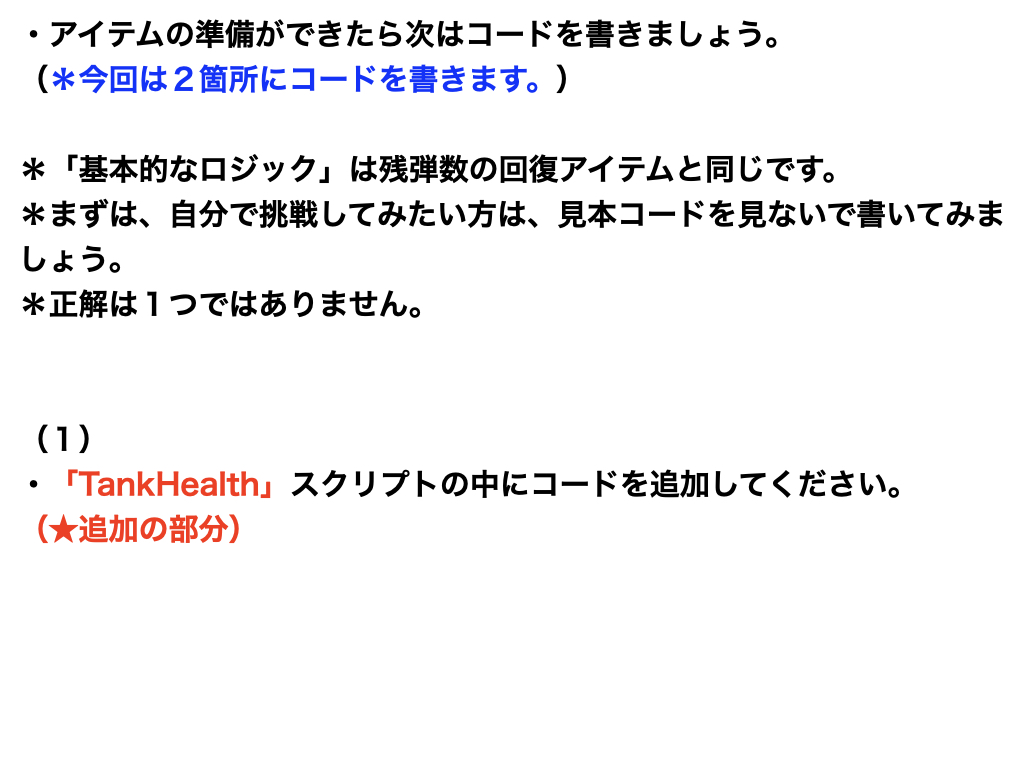
HPを回復させる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using TMPro;
public class TankHealth : MonoBehaviour
{
public GameObject effectPrefab1;
public GameObject effectPrefab2;
public int tankHP;
public TextMeshProUGUI hpLabel;
// ★追加
private int tankMaxHP = 10; // 最大値は自由
private void Start()
{
// ★追加
tankHP = tankMaxHP;
hpLabel.text = "" + tankHP;
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("EnemyShell"))
{
tankHP -= 1;
hpLabel.text = "" + tankHP;
Destroy(other.gameObject);
if (tankHP > 0)
{
GameObject effect1 = Instantiate(effectPrefab1, transform.position, Quaternion.identity);
Destroy(effect1, 1.0f);
}
else
{
GameObject effect2 = Instantiate(effectPrefab2, transform.position, Quaternion.identity);
Destroy(effect2, 1.0f);
this.gameObject.SetActive(false);
Invoke("GoToGameOver", 1.5f);
}
}
}
void GoToGameOver()
{
SceneManager.LoadScene("GameOver");
}
// ★追加
// publicをつけること(「public」の意味を復習しましょう!)
public void AddHP(int amount)
{
tankHP += amount;
// ここは何をコントロールしているでしょうか?
if(tankHP > tankMaxHP)
{
tankHP = tankMaxHP;
}
hpLabel.text = "" + tankHP;
}
}
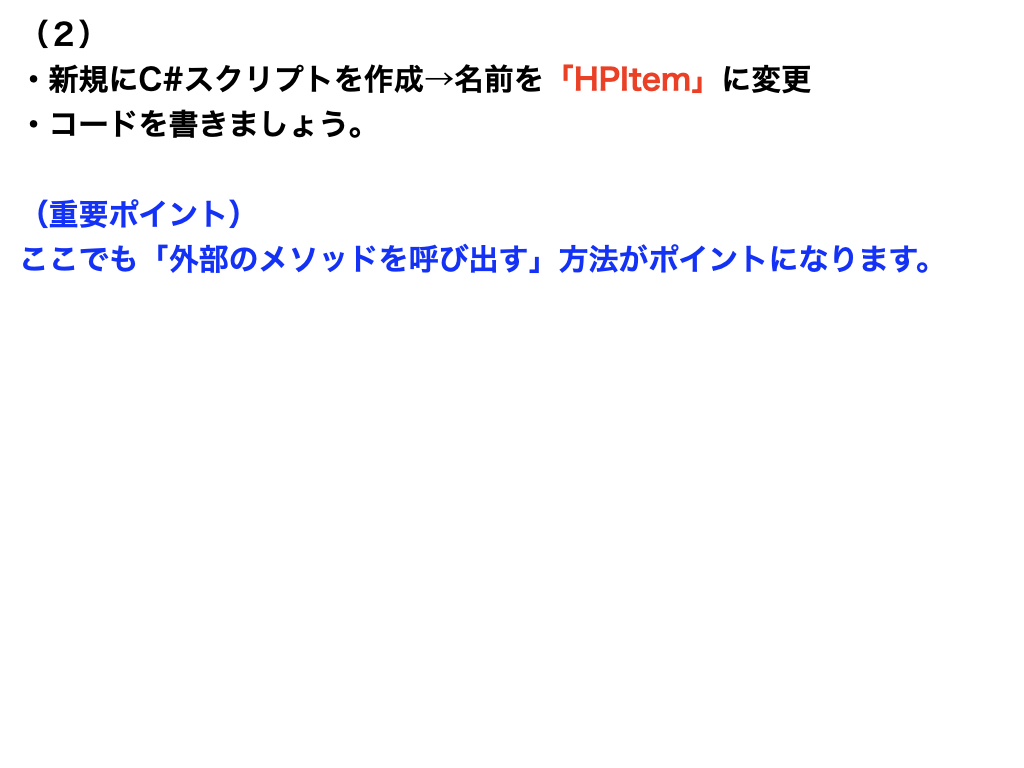
HPを回復させる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HPItem : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip getSound;
private TankHealth th;
private int reward = 3; // いくつ回復させるかは自由!
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Player"))
{
// Find()メソッドの使い方をマスターすること
th = GameObject.Find("Tank").GetComponent<TankHealth>();
// ここの意味をしっかり復習すること。
// AddHP()メソッドを呼び出して「引数」にrewardを与えている。
th.AddHP(reward);
Destroy(gameObject);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
AudioSource.PlayClipAtPoint(getSound, transform.position);
}
}
}
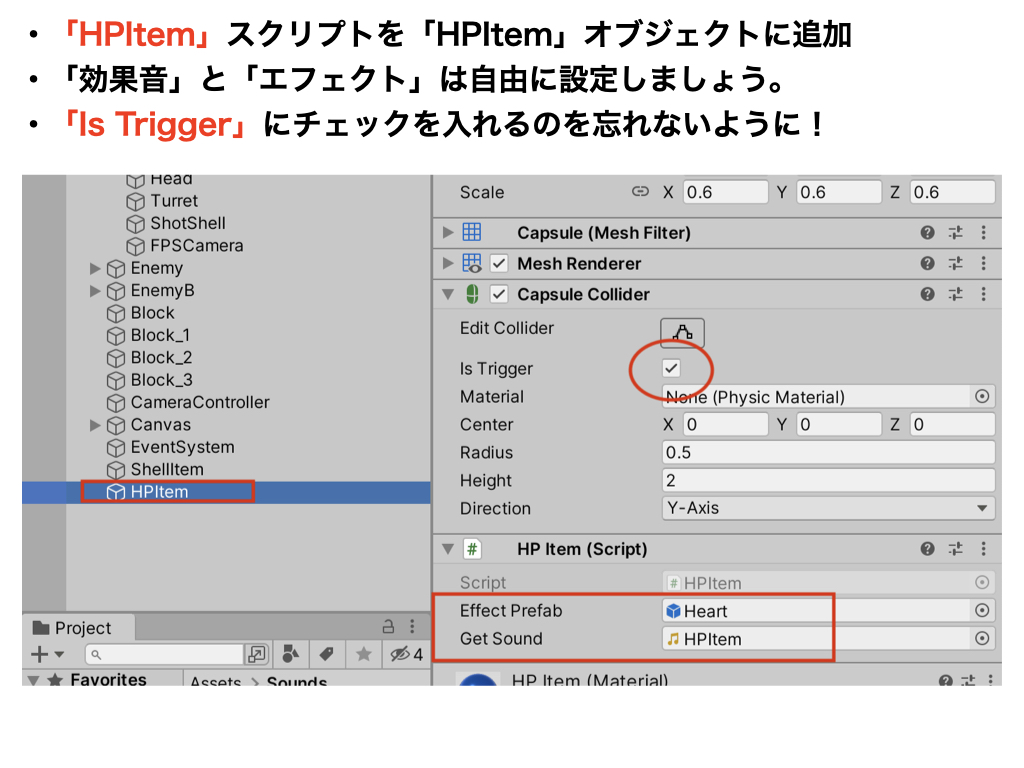
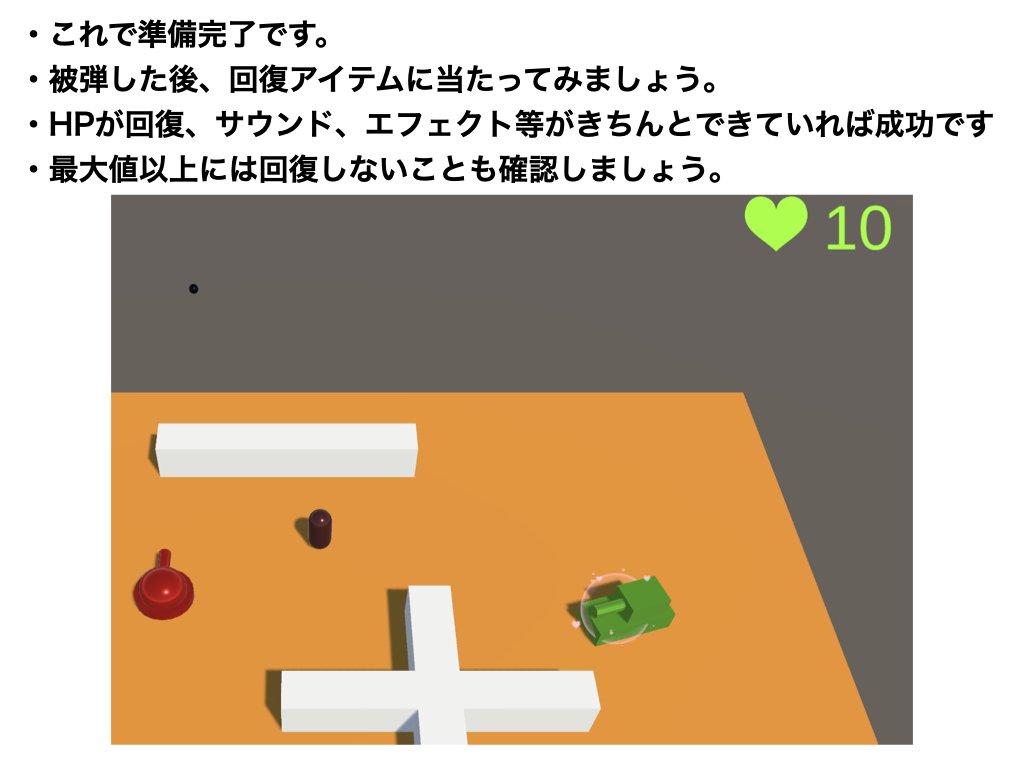
プレーヤーのHPを回復させるアイテムの作成