コインの残り枚数を表示する/for文(繰り返し文)の使い方
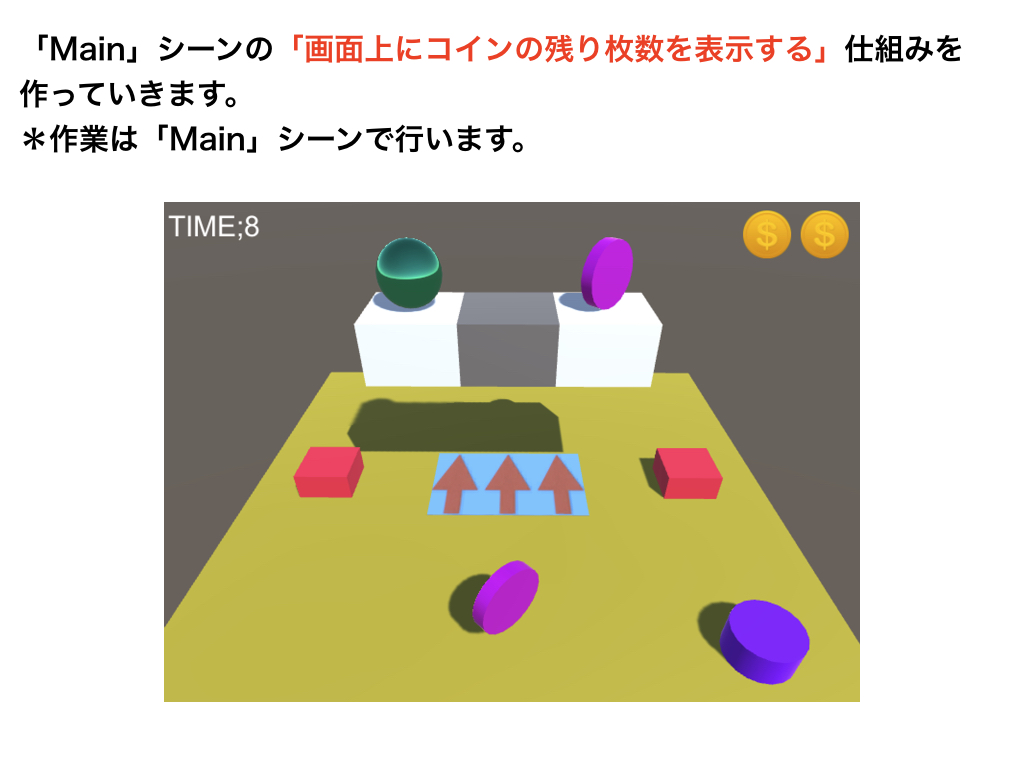
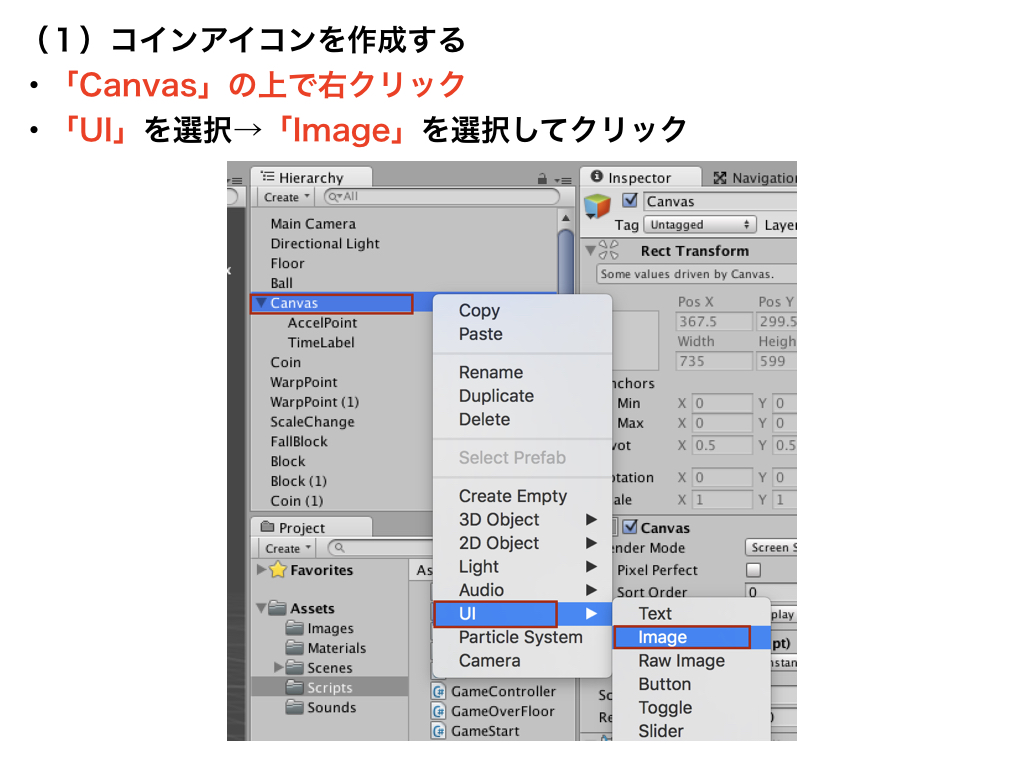
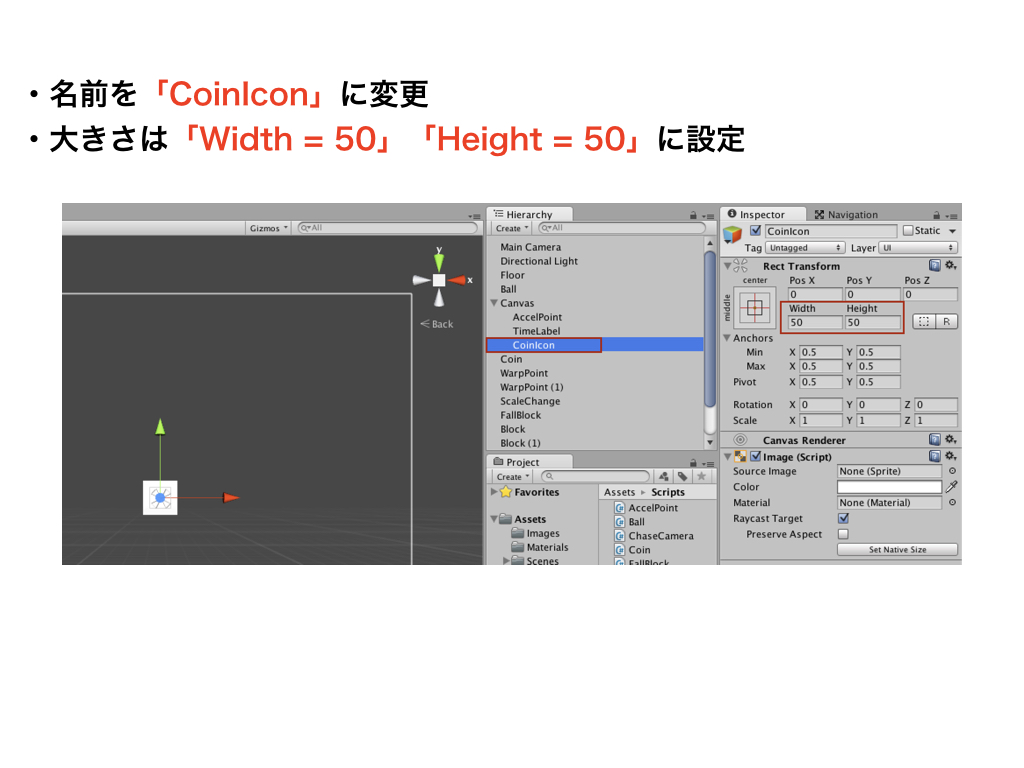
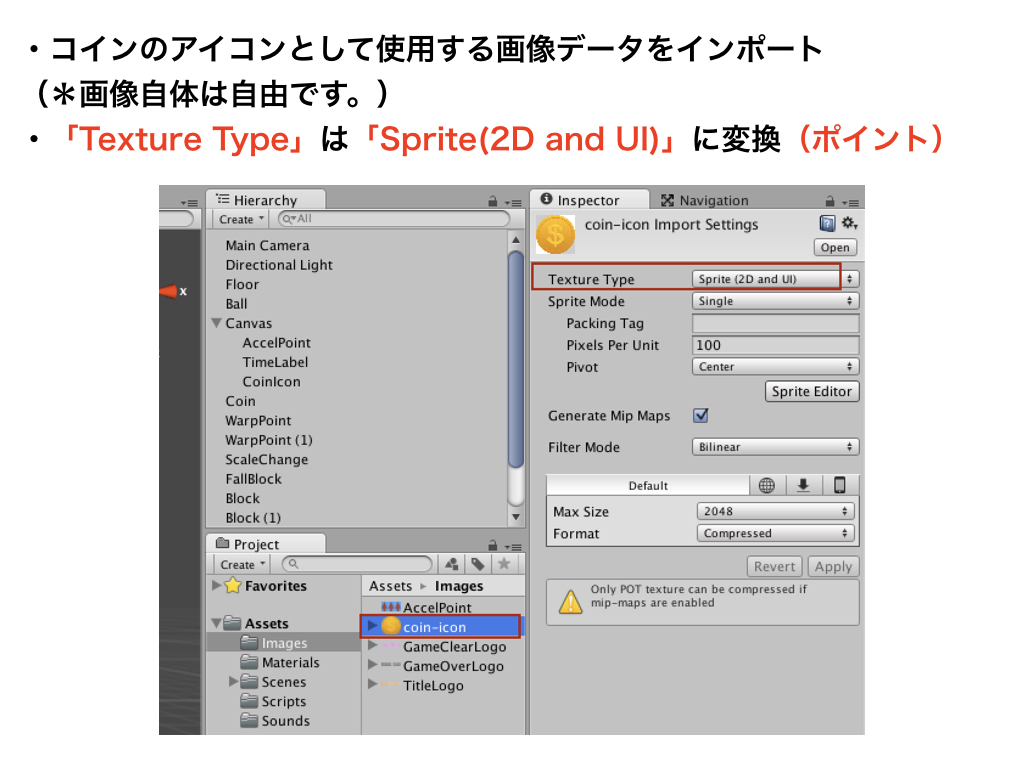
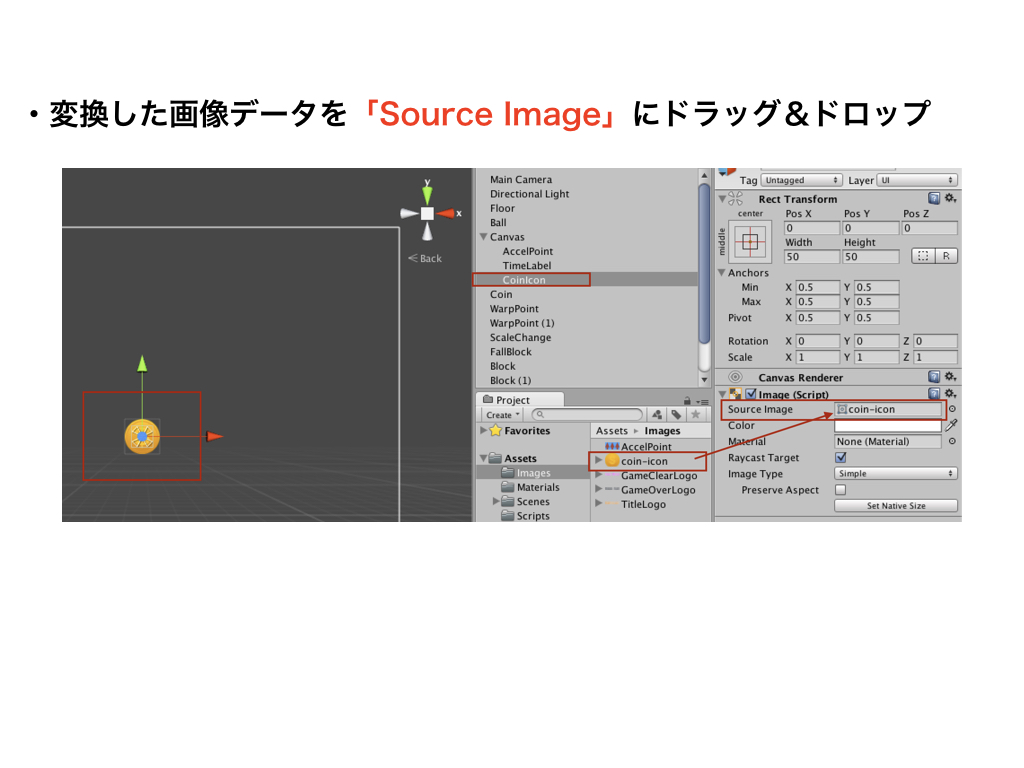
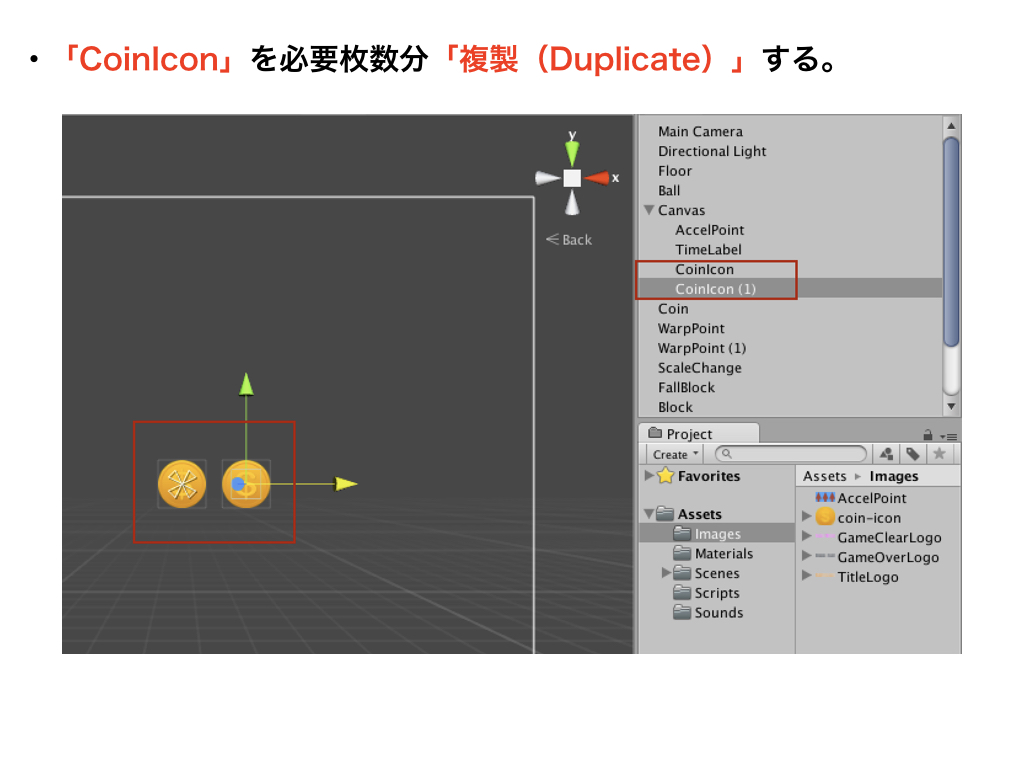
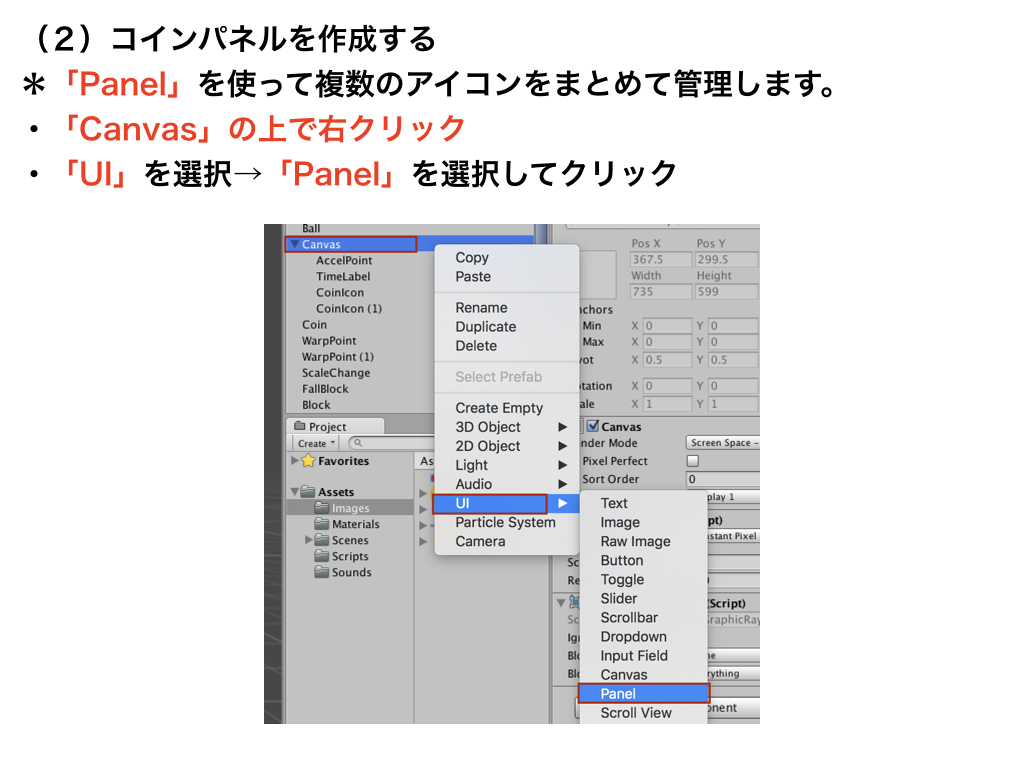
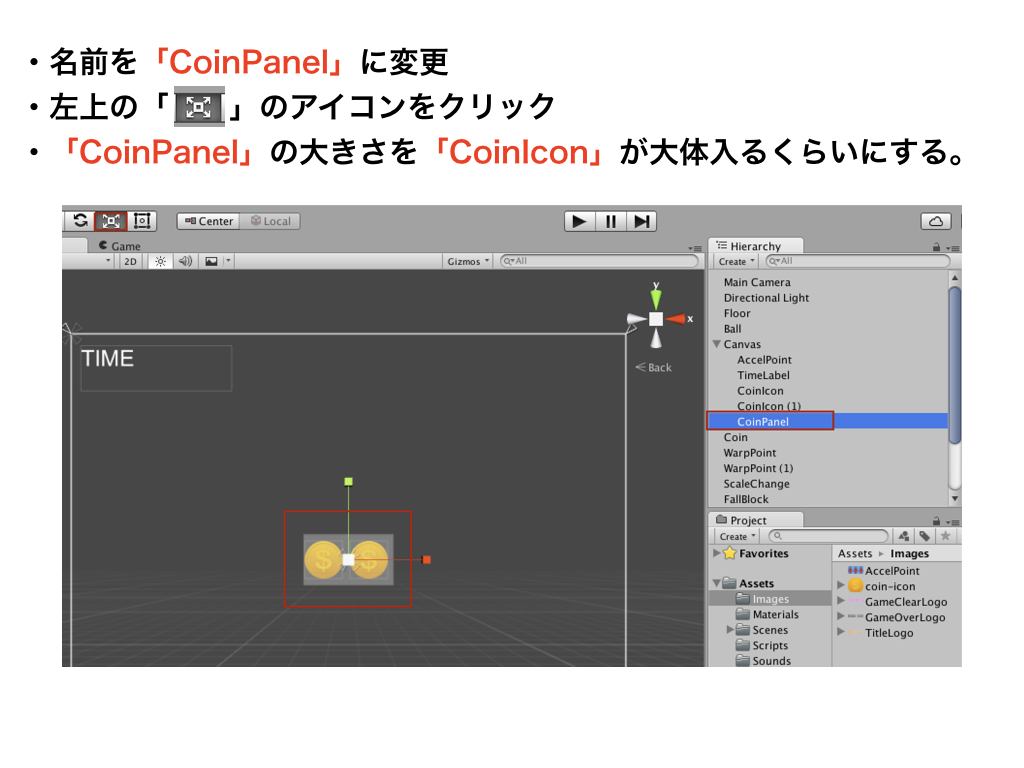
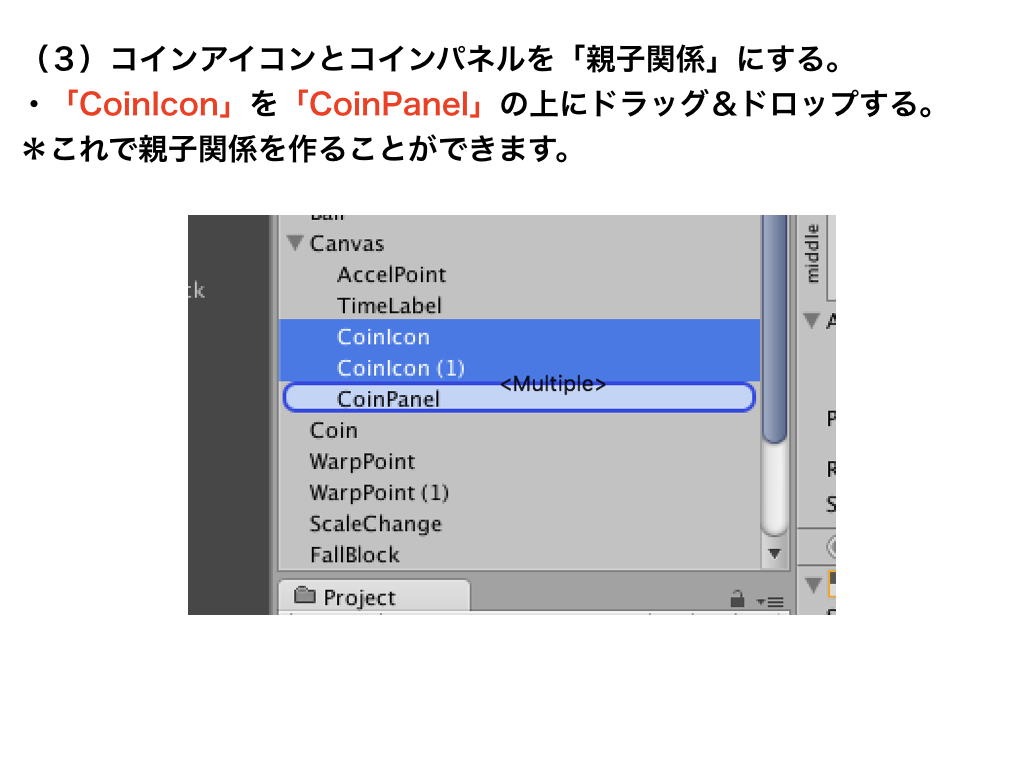
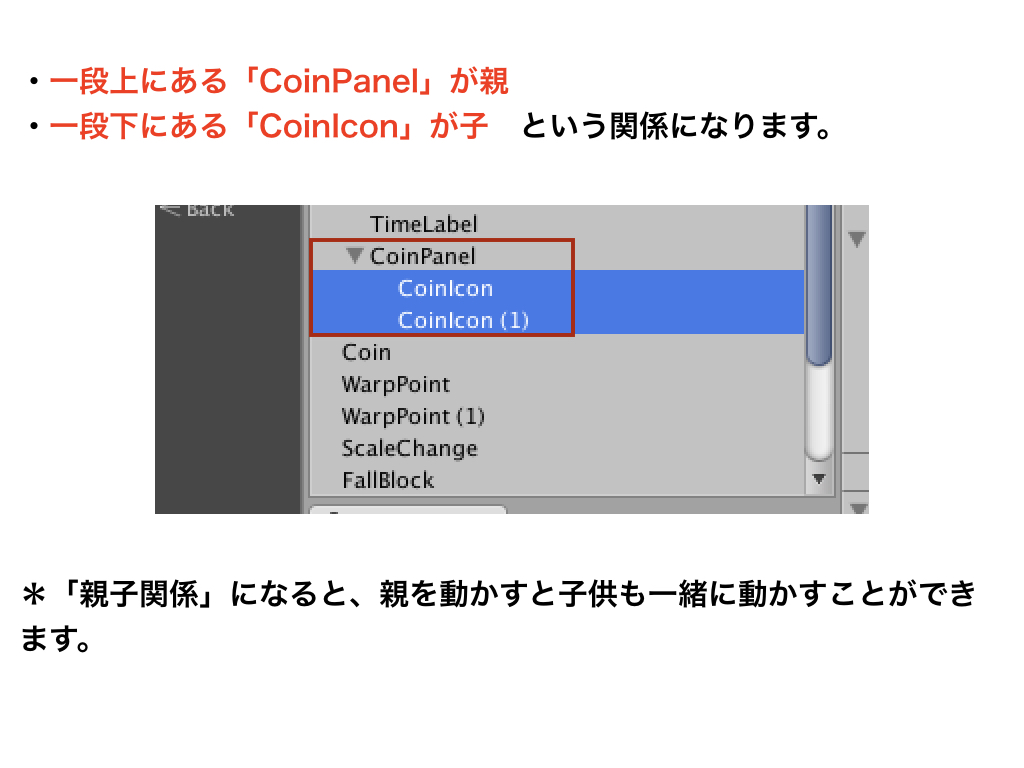
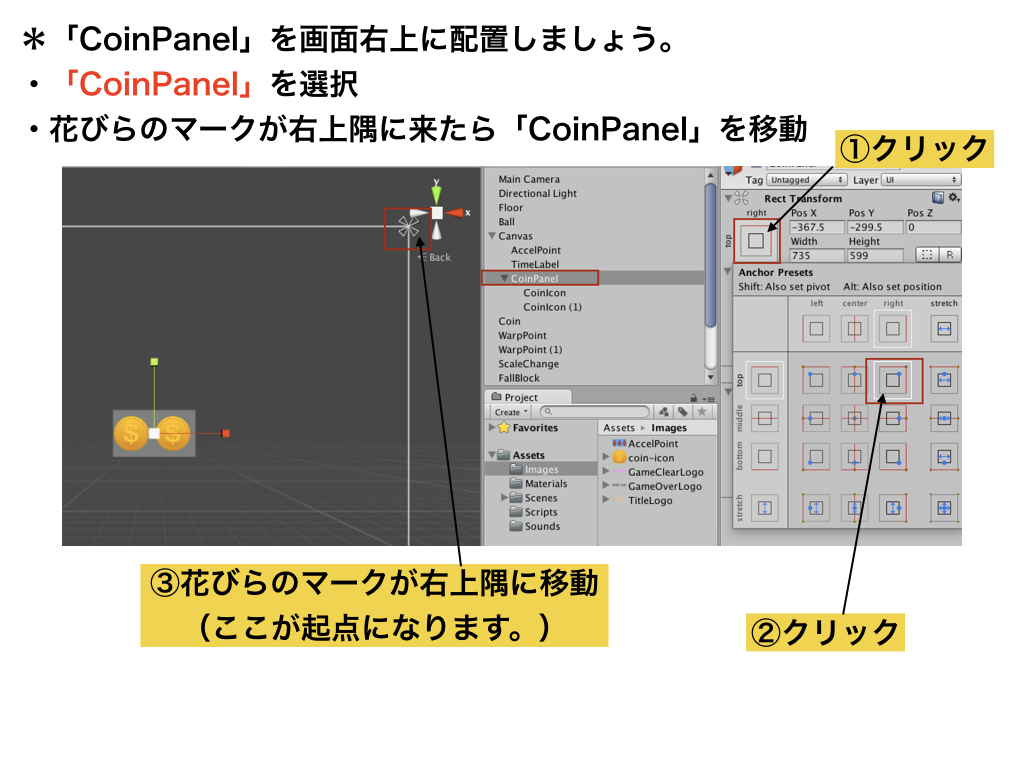
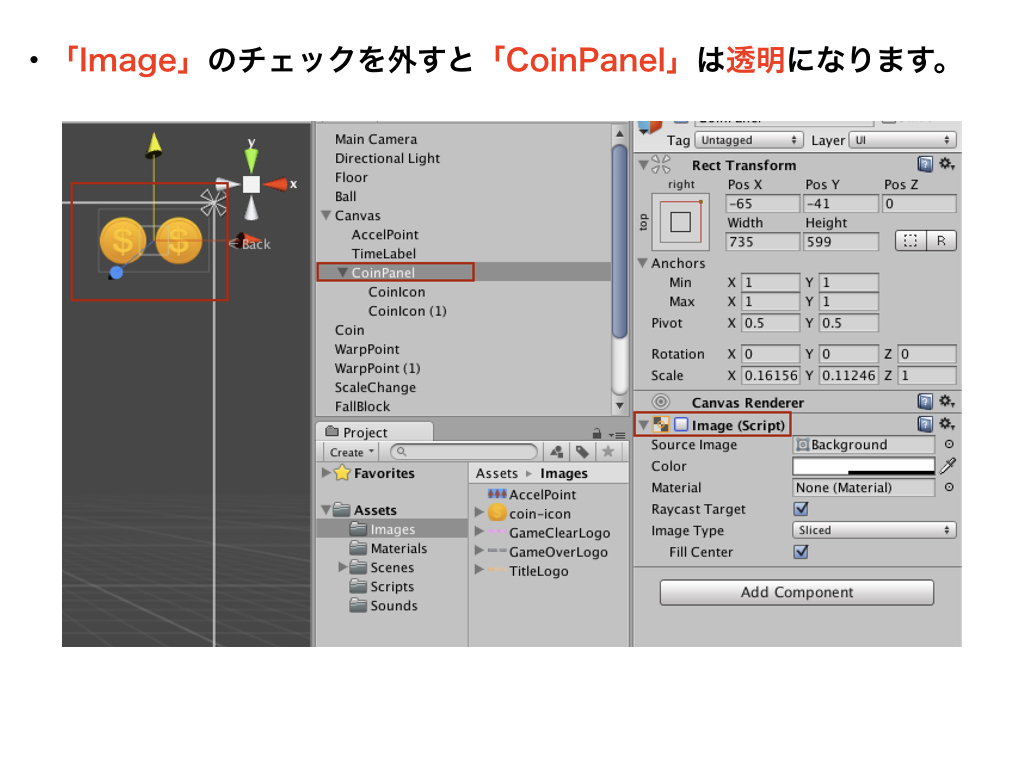
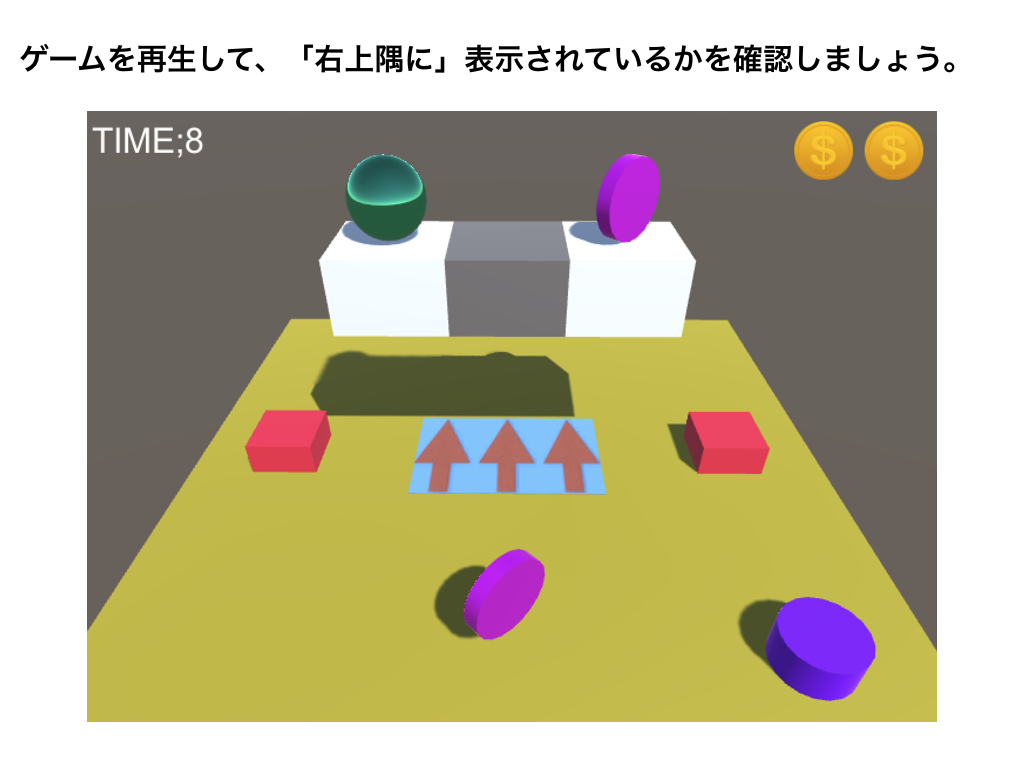
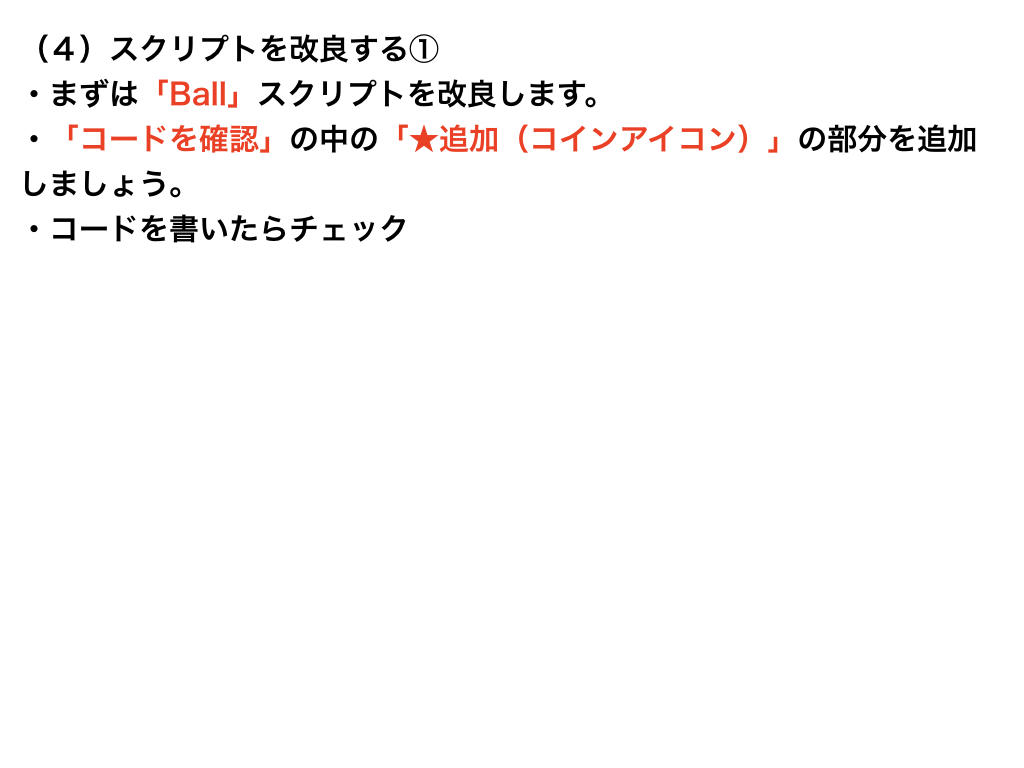
コインの残り枚数を表示する
using UnityEngine;
using System.Collections;
using UnityEngine.SceneManagement;
public class Ball : MonoBehaviour {
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
private int coinCount = 0;
void Start () {
rb = GetComponent<Rigidbody>();
}
void Update () {
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
if(Input.GetButtonDown("Jump") && isJumping == false){
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
void OnTriggerEnter(Collider other){
if(other.CompareTag("Coin")){
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet ,transform.position);
coinCount += 1;
if(coinCount == 2)
SceneManager.LoadScene("GameClear");
}
}
// ★追加(コインアイコン)
public int Coin(){
return coinCount;
}
void OnCollisionEnter(Collision other){
if(other.gameObject.CompareTag("Floor")){
isJumping = false;
}
}
}
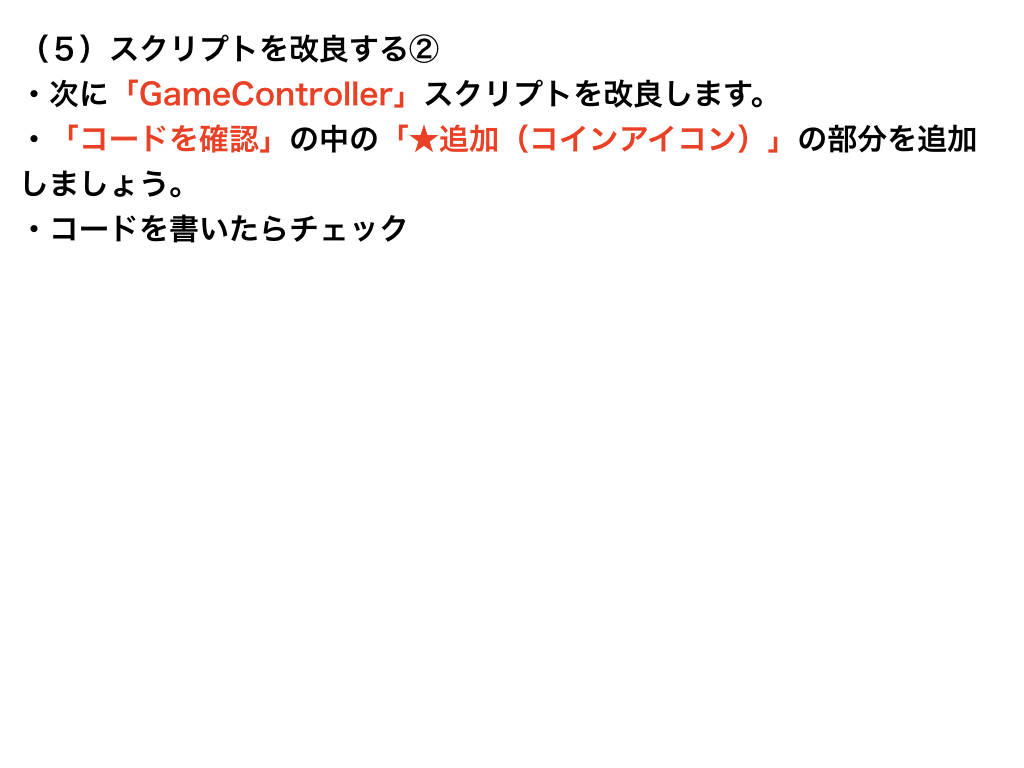
コインの残り枚数を表示する
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class GameController : MonoBehaviour {
public Text timeLabel;
public float timeCount;
// ★追加(コインアイコン)
// 配列(複数のデータを入れることのできる仕切りのある箱)
public GameObject[] icons;
private Ball ballScript;
void Start () {
timeLabel.text = "TIME;" + timeCount;
// ★追加(コインアイコン)
// 「Ball」オブジェクトに付いている「Ball」スクリプトにアクセスする。
ballScript = GameObject.Find("Ball").GetComponent<Ball>();
}
void Update () {
timeCount -= Time.deltaTime;
timeLabel.text = "TIME;" + timeCount.ToString("0");
if(timeCount < 0){
SceneManager.LoadScene("GameOver");
}
// ★追加(コインアイコン)
UpdateCoin(ballScript.Coin());
}
// ★追加(コインアイコン)
// コインアイコンを表示するメソッド
void UpdateCoin(int coin){
// for文(繰り返し文)
for(int i = 0; i < icons.Length; i++){
if(coin <= i){
// コインアイコンを表示状態にする。
icons[i].SetActive(true);
} else {
// コインアイコンを非表示状態にする。
icons[i].SetActive(false);
}
}
}
}
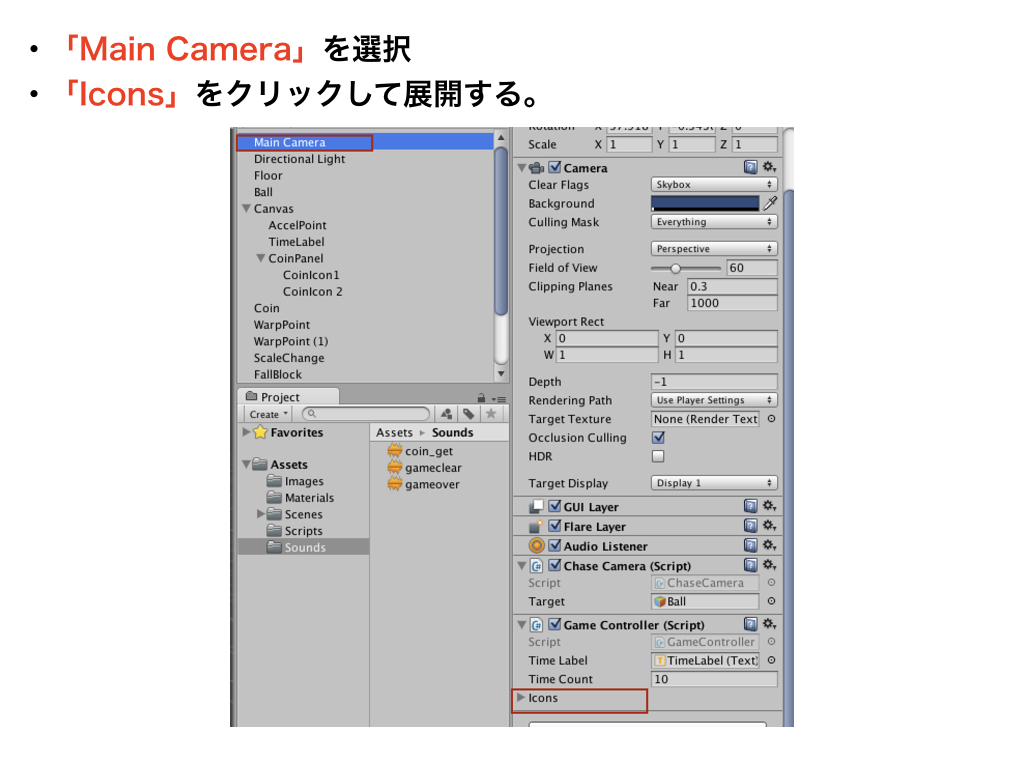
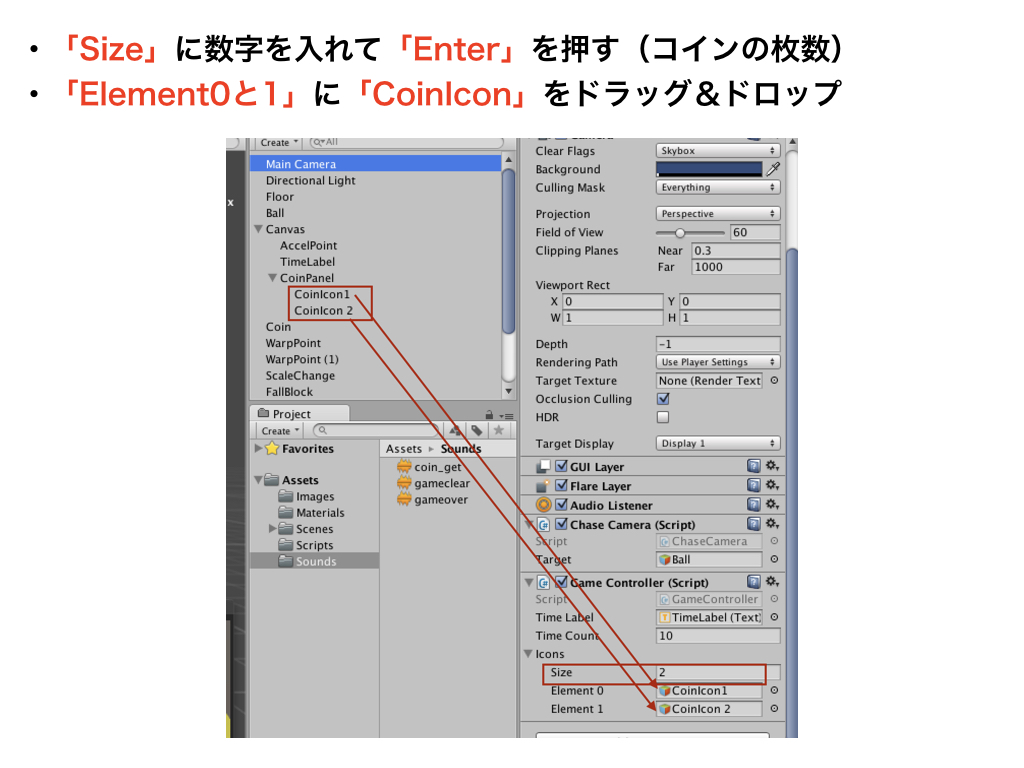
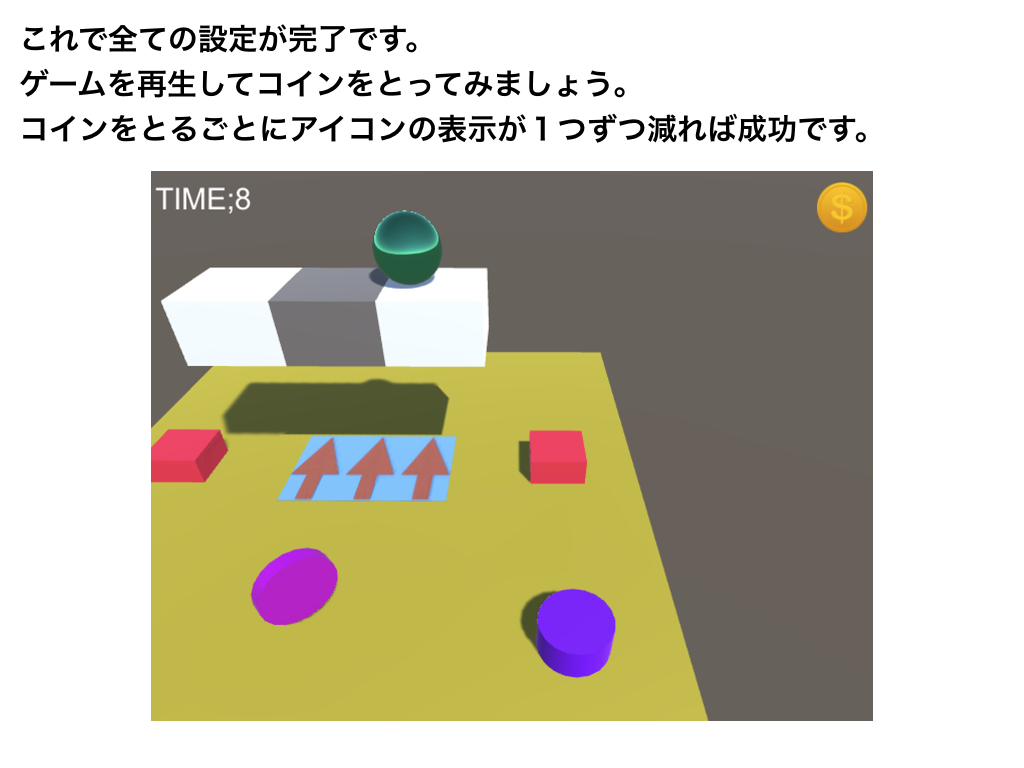
【旧版】BallGame(全25回)
他のコースを見る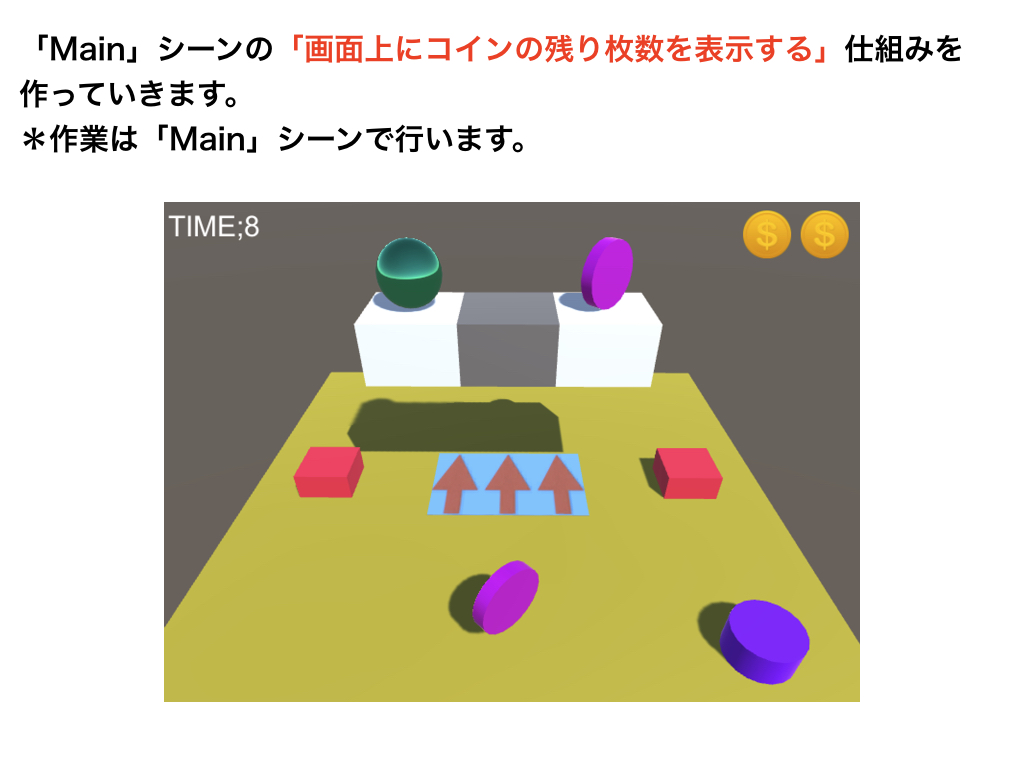
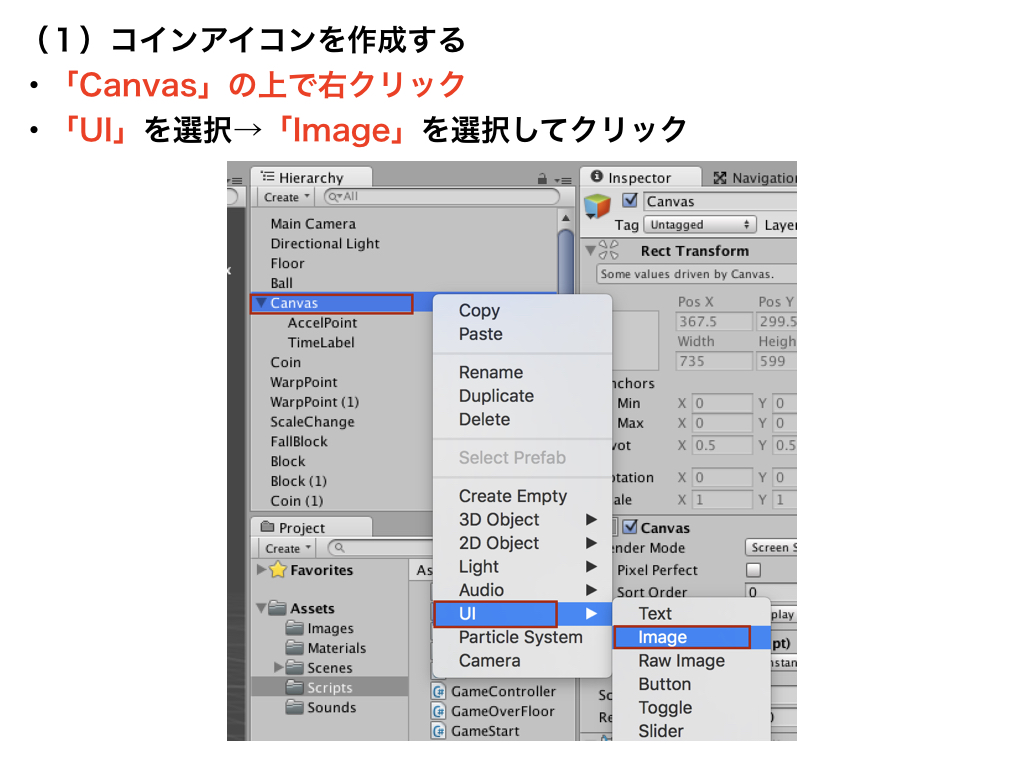
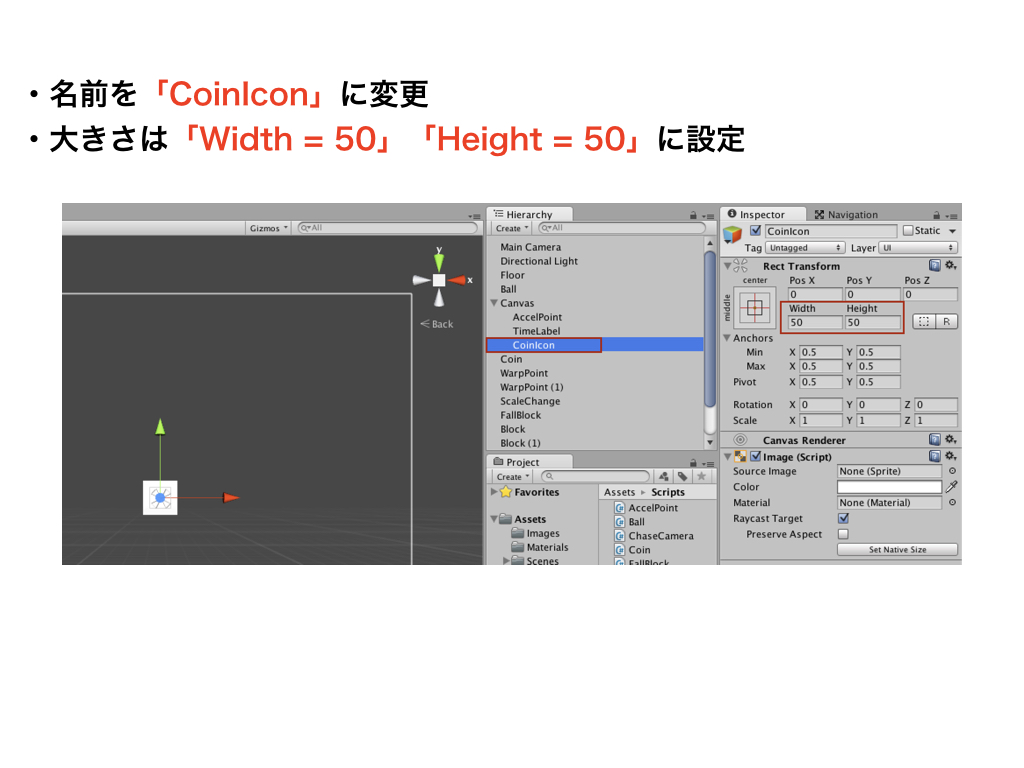
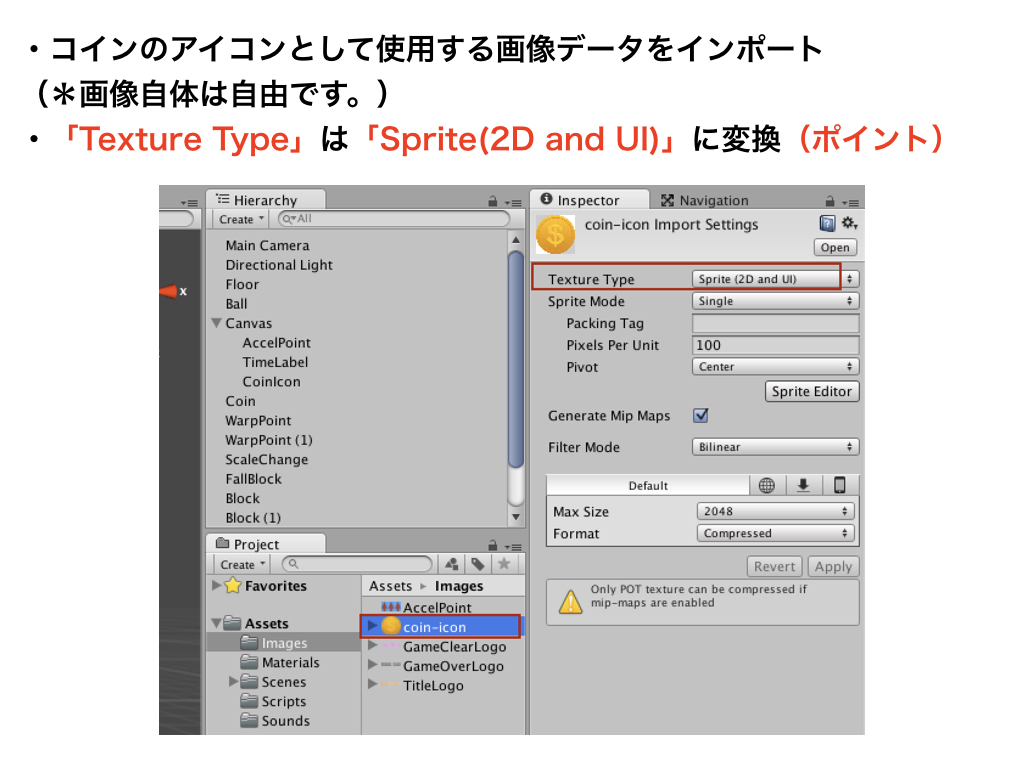
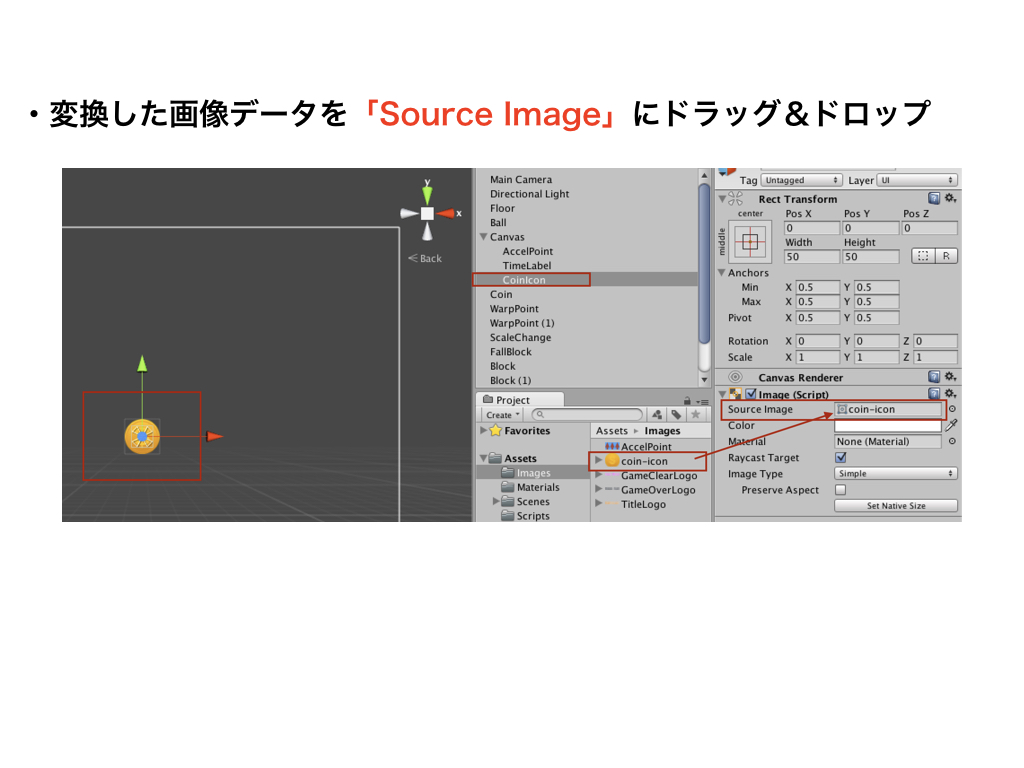
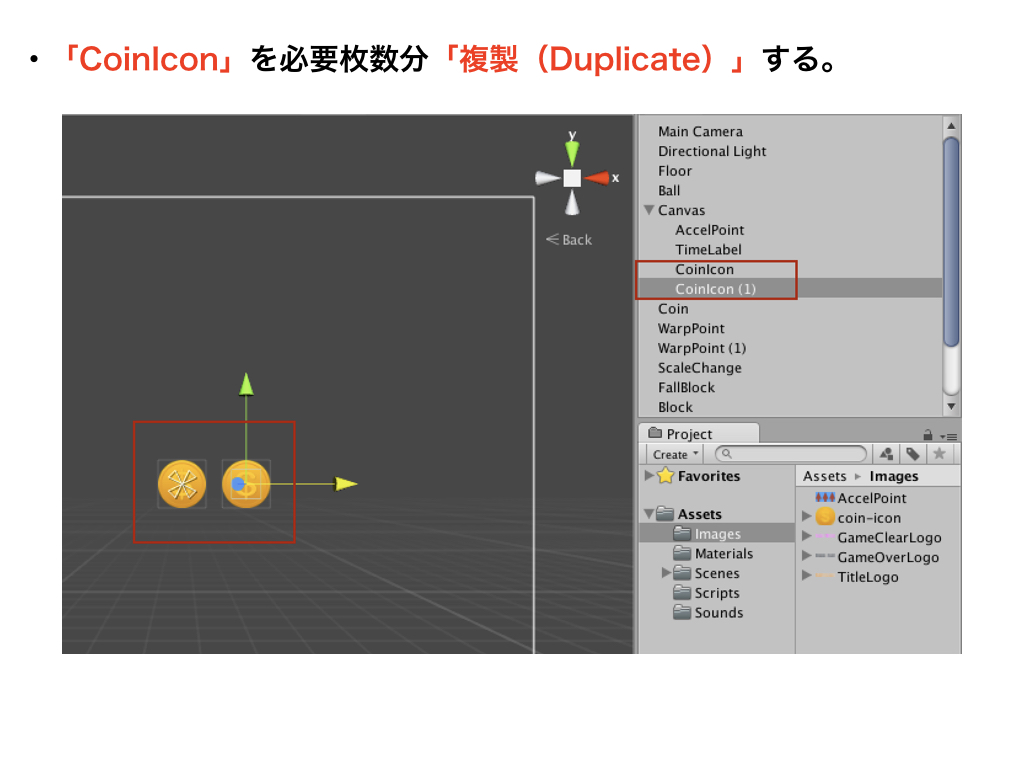
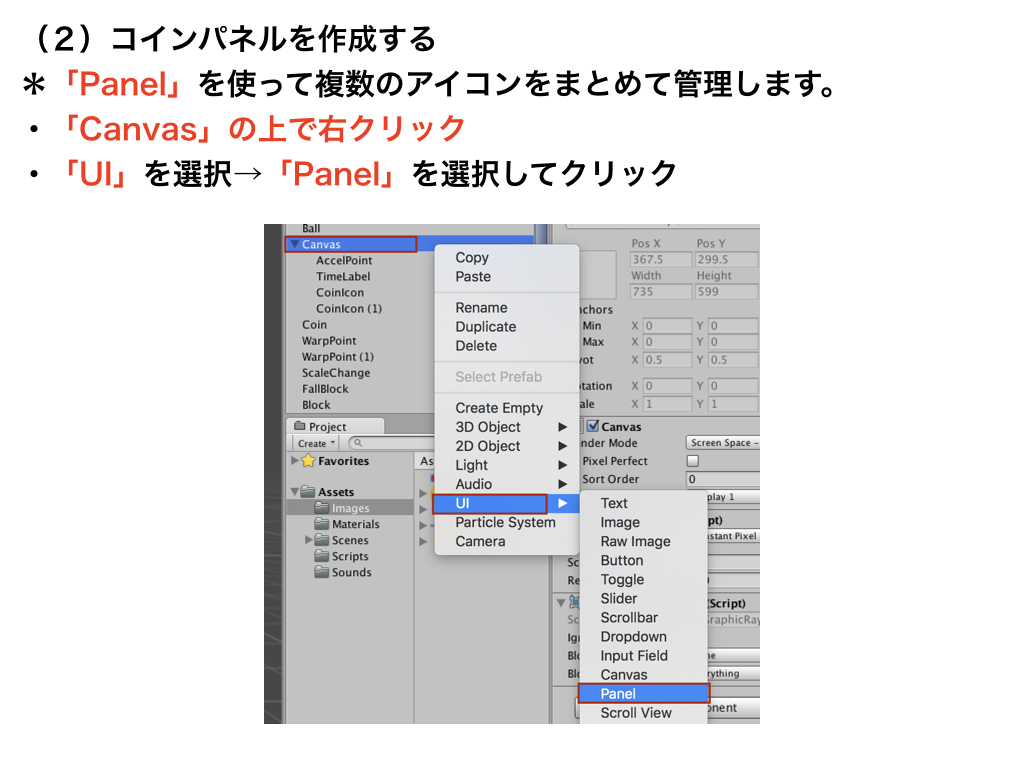
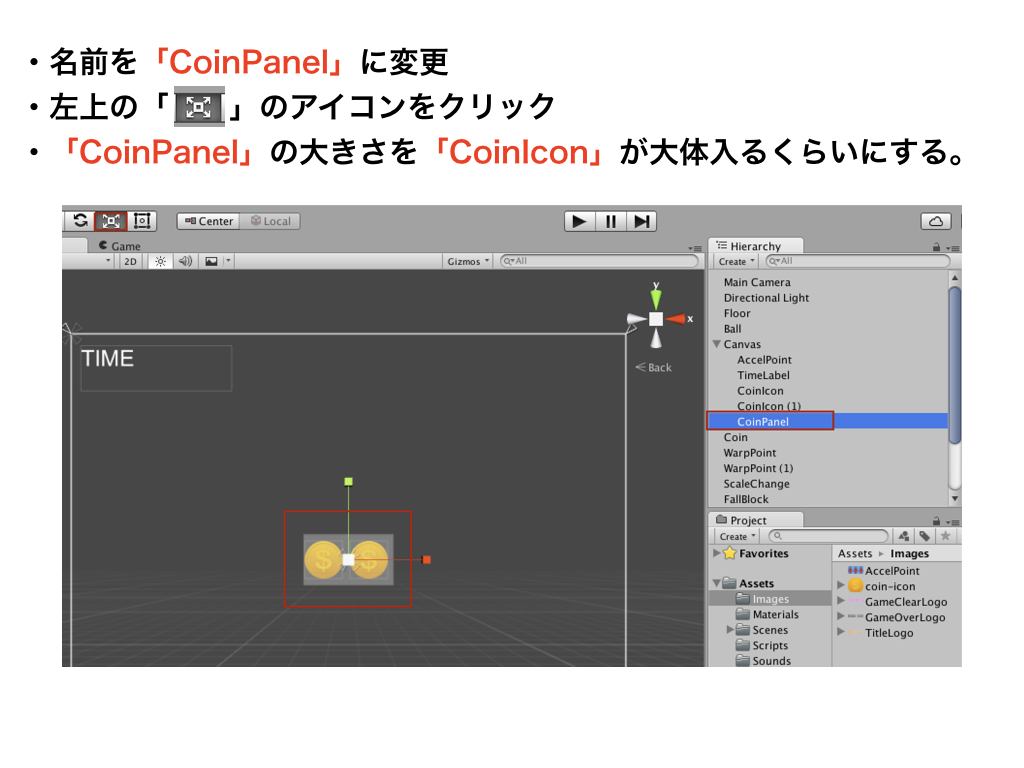
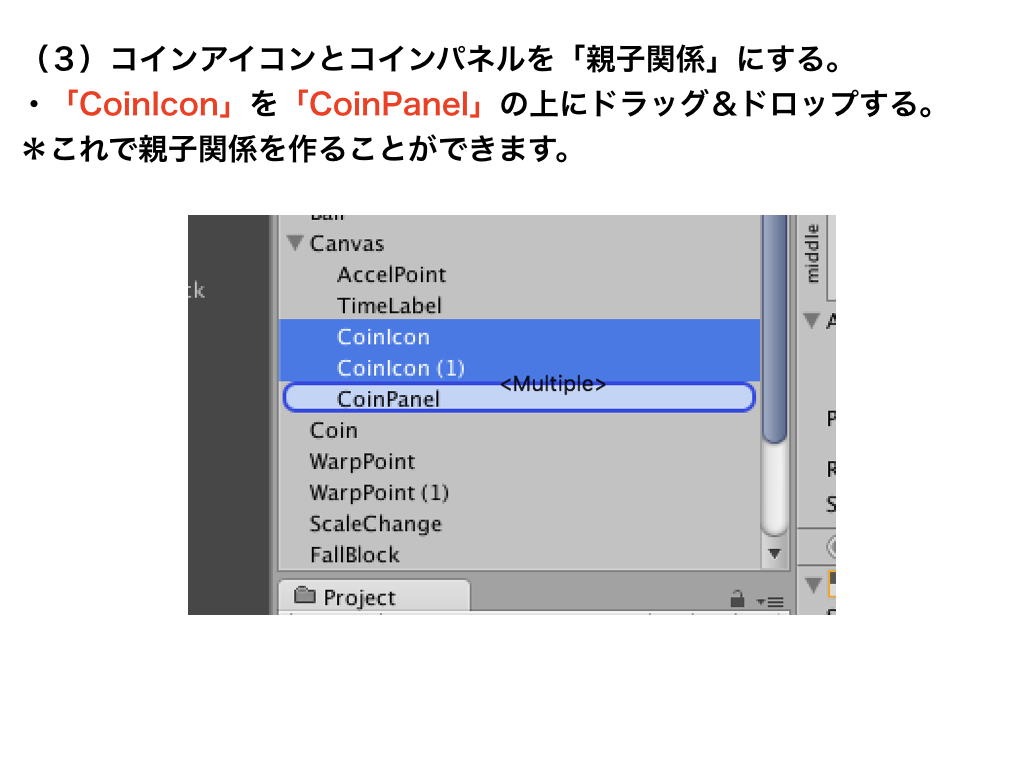
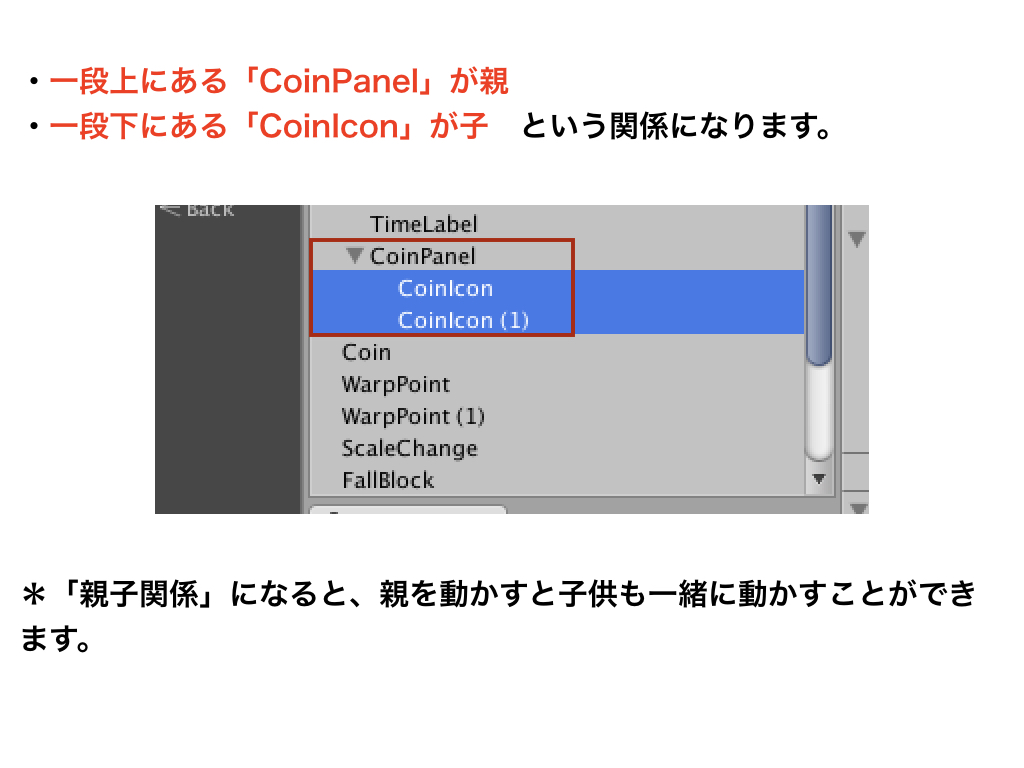
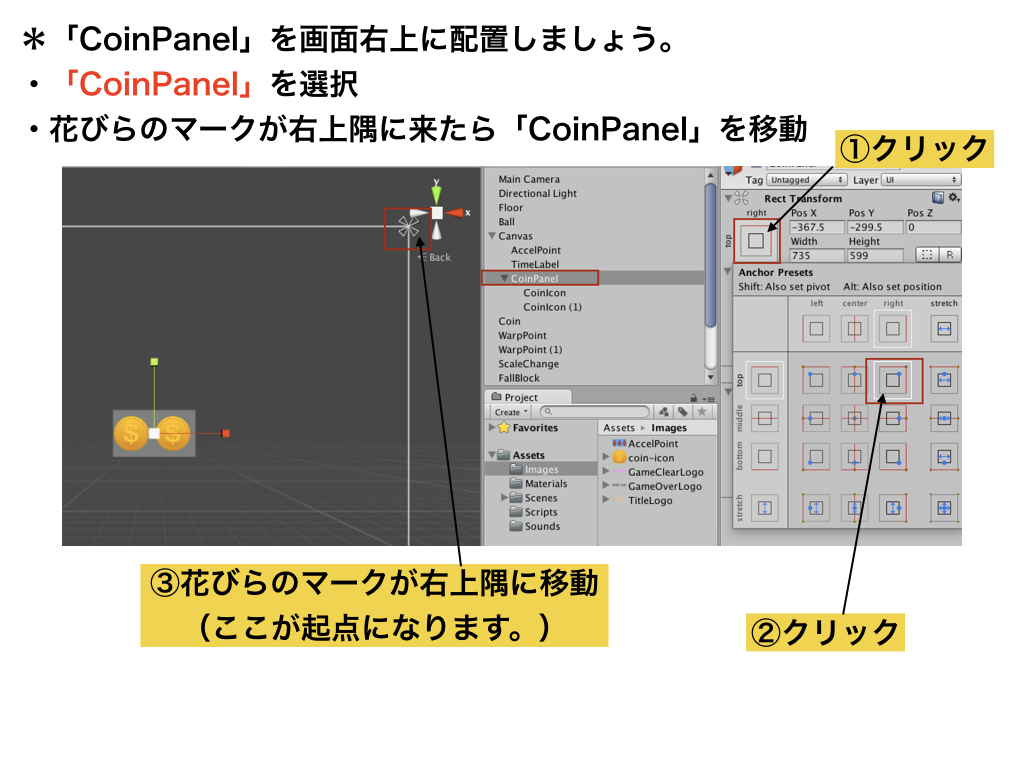
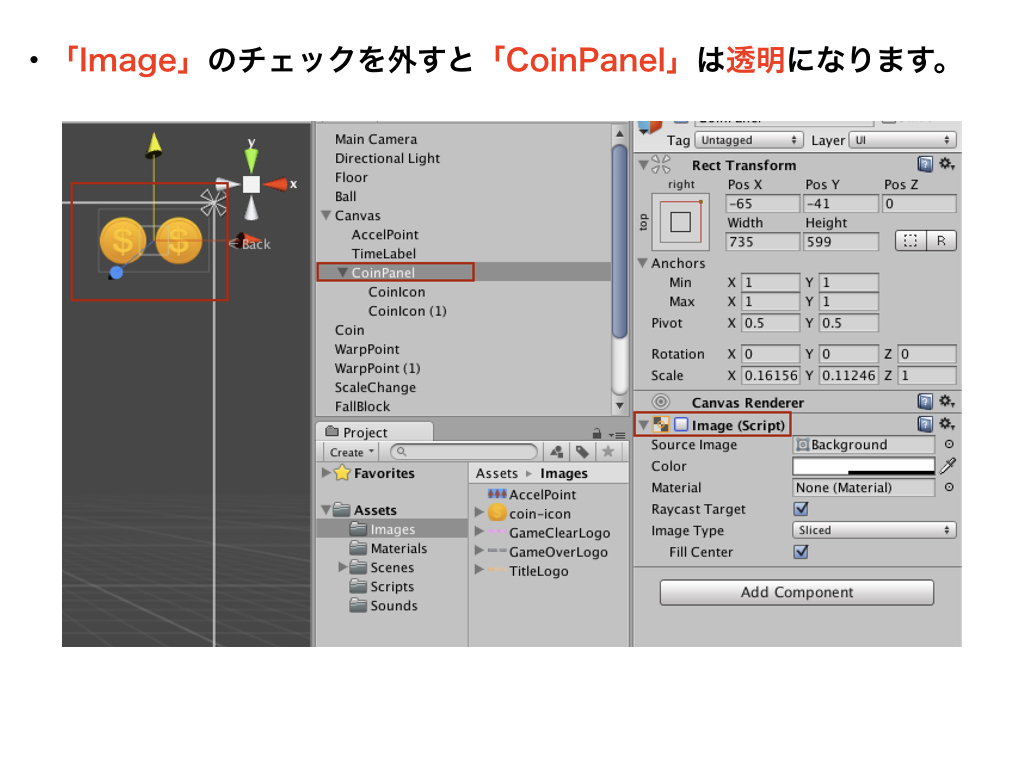
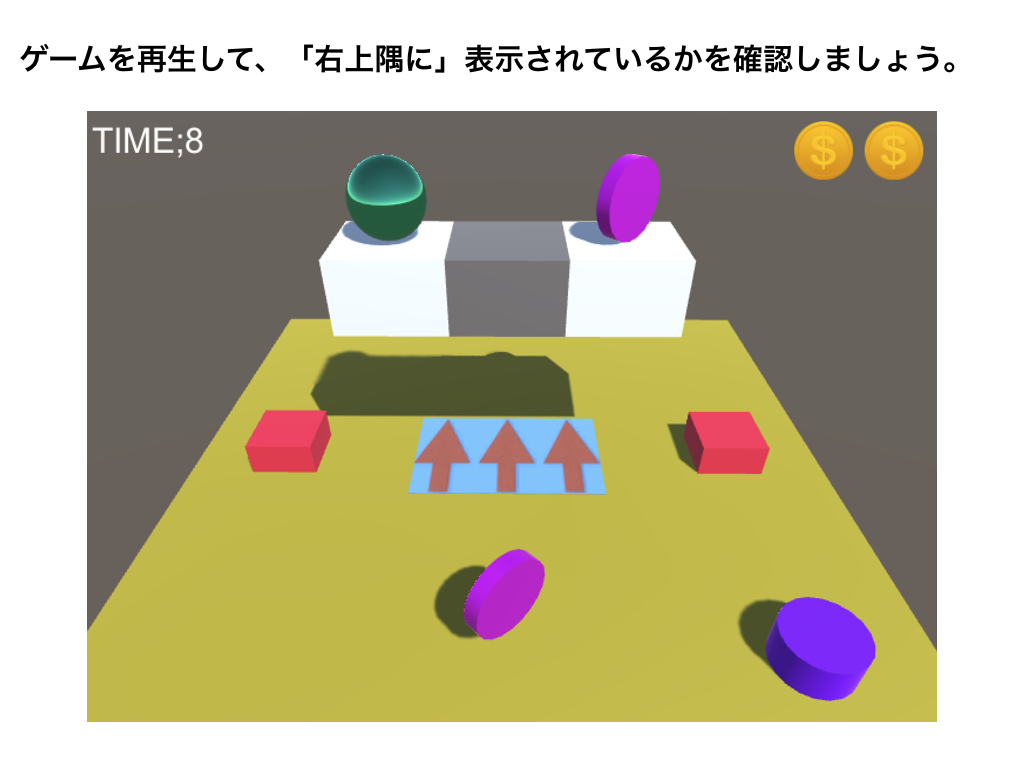
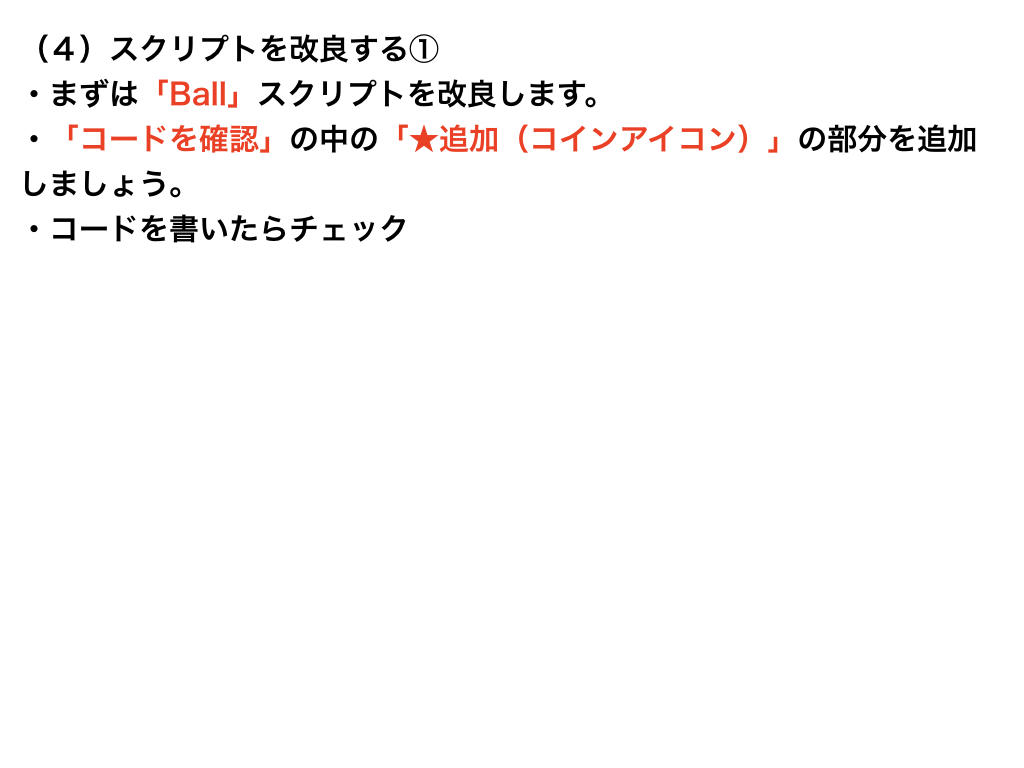
コインの残り枚数を表示する
using UnityEngine;
using System.Collections;
using UnityEngine.SceneManagement;
public class Ball : MonoBehaviour {
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
private int coinCount = 0;
void Start () {
rb = GetComponent<Rigidbody>();
}
void Update () {
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
if(Input.GetButtonDown("Jump") && isJumping == false){
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
void OnTriggerEnter(Collider other){
if(other.CompareTag("Coin")){
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet ,transform.position);
coinCount += 1;
if(coinCount == 2)
SceneManager.LoadScene("GameClear");
}
}
// ★追加(コインアイコン)
public int Coin(){
return coinCount;
}
void OnCollisionEnter(Collision other){
if(other.gameObject.CompareTag("Floor")){
isJumping = false;
}
}
}
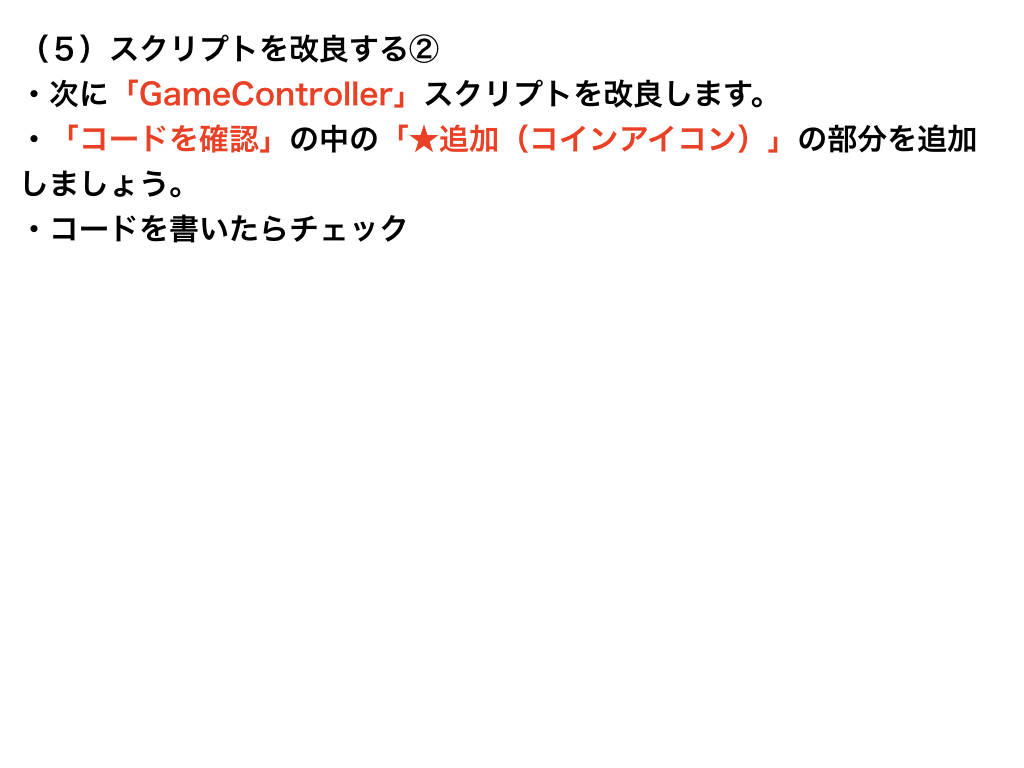
コインの残り枚数を表示する
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class GameController : MonoBehaviour {
public Text timeLabel;
public float timeCount;
// ★追加(コインアイコン)
// 配列(複数のデータを入れることのできる仕切りのある箱)
public GameObject[] icons;
private Ball ballScript;
void Start () {
timeLabel.text = "TIME;" + timeCount;
// ★追加(コインアイコン)
// 「Ball」オブジェクトに付いている「Ball」スクリプトにアクセスする。
ballScript = GameObject.Find("Ball").GetComponent<Ball>();
}
void Update () {
timeCount -= Time.deltaTime;
timeLabel.text = "TIME;" + timeCount.ToString("0");
if(timeCount < 0){
SceneManager.LoadScene("GameOver");
}
// ★追加(コインアイコン)
UpdateCoin(ballScript.Coin());
}
// ★追加(コインアイコン)
// コインアイコンを表示するメソッド
void UpdateCoin(int coin){
// for文(繰り返し文)
for(int i = 0; i < icons.Length; i++){
if(coin <= i){
// コインアイコンを表示状態にする。
icons[i].SetActive(true);
} else {
// コインアイコンを非表示状態にする。
icons[i].SetActive(false);
}
}
}
}
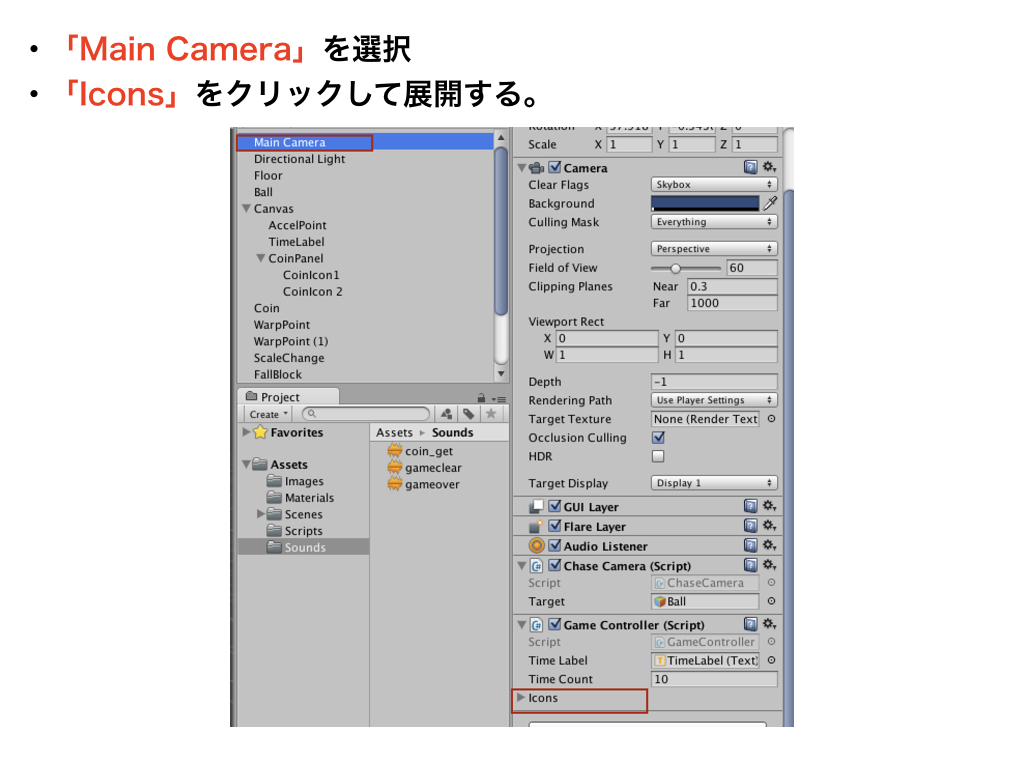
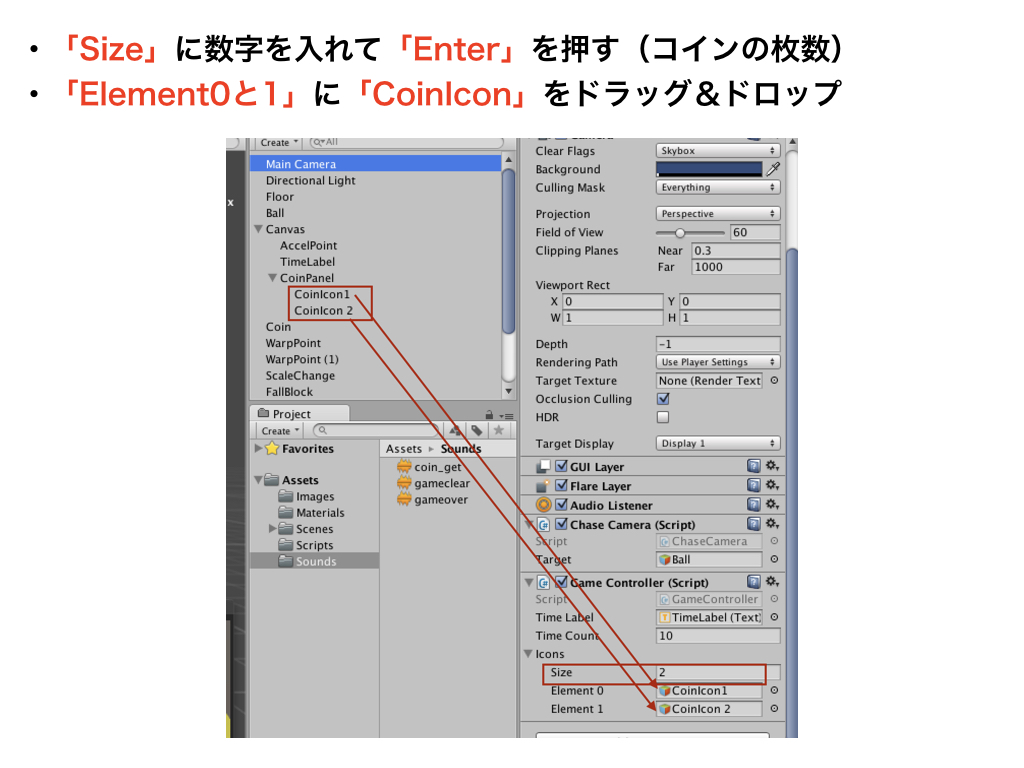
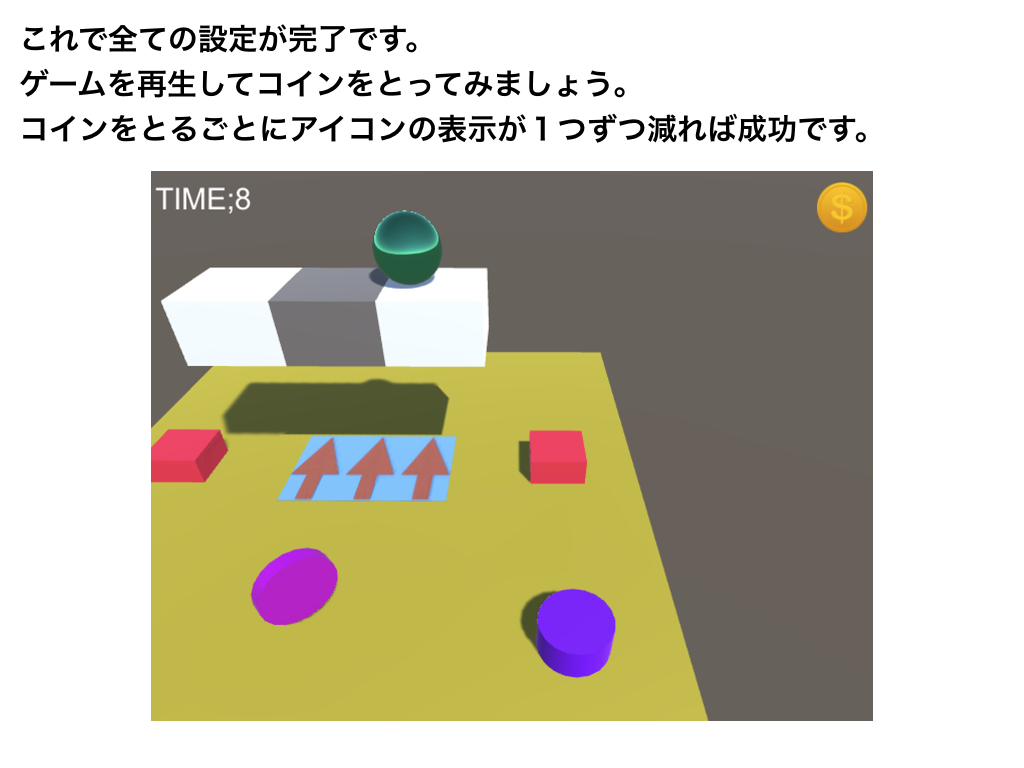
コインの残り枚数を表示する/for文(繰り返し文)の使い方