UI②(スコアを画面に表示する)
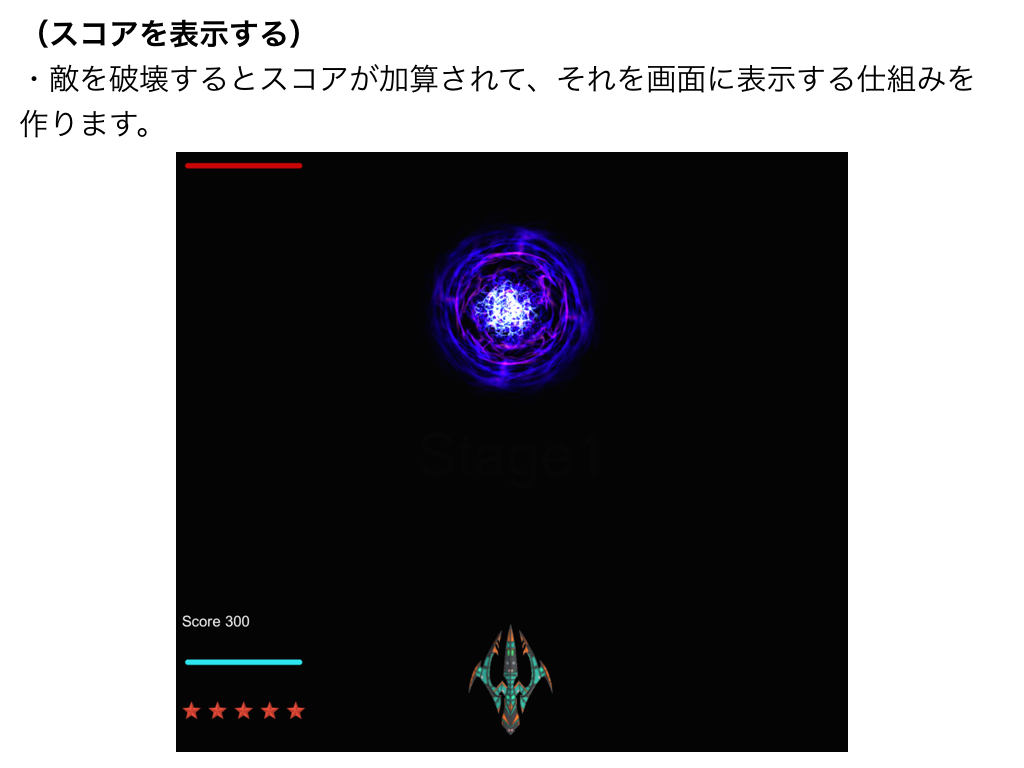
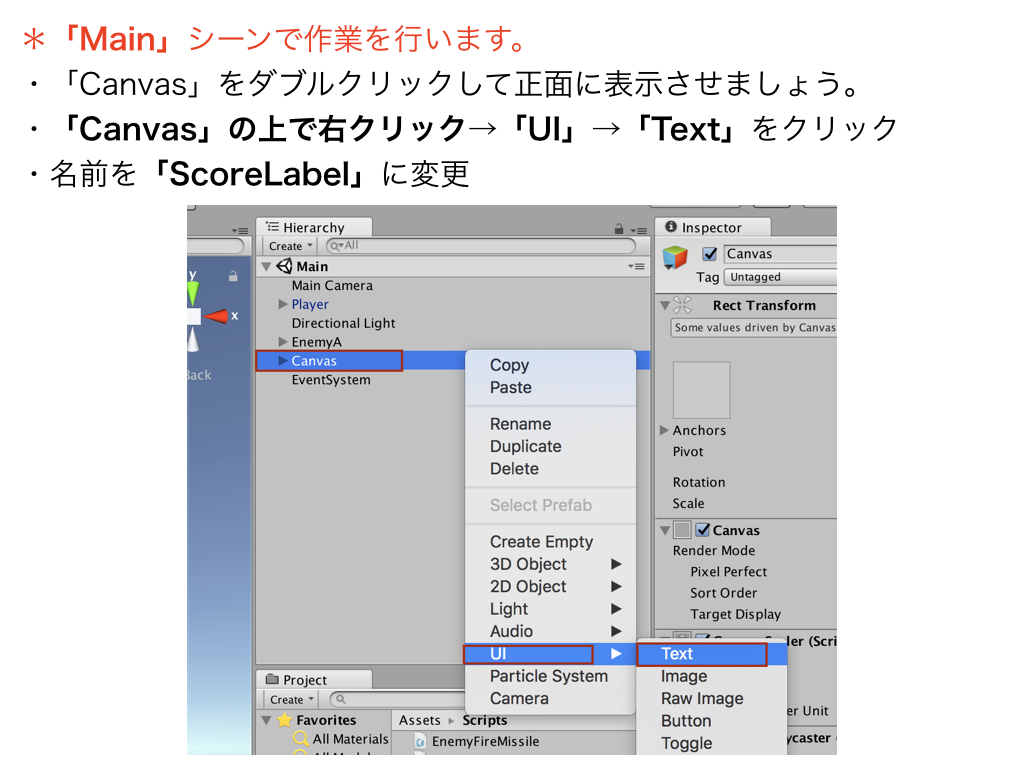
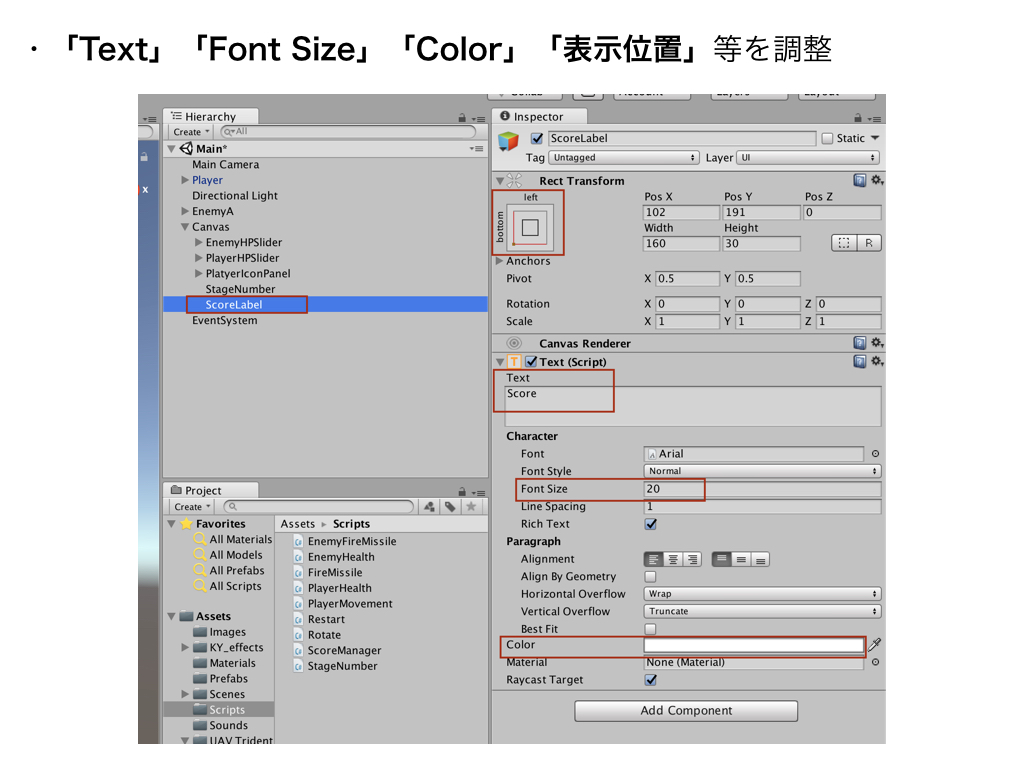
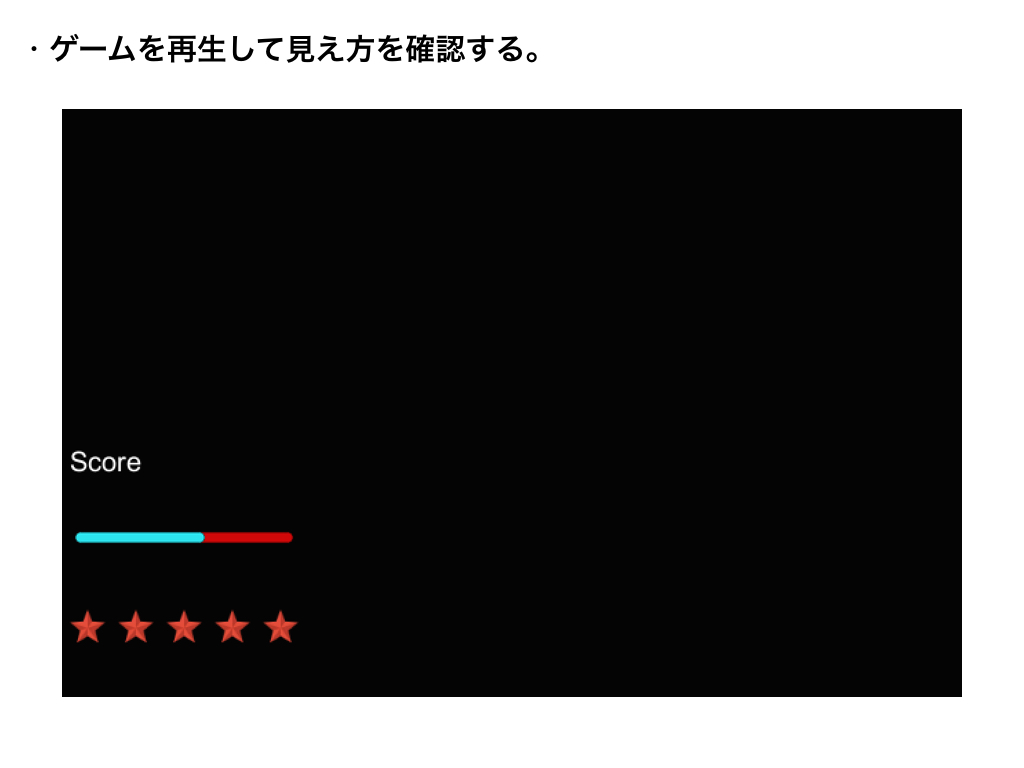
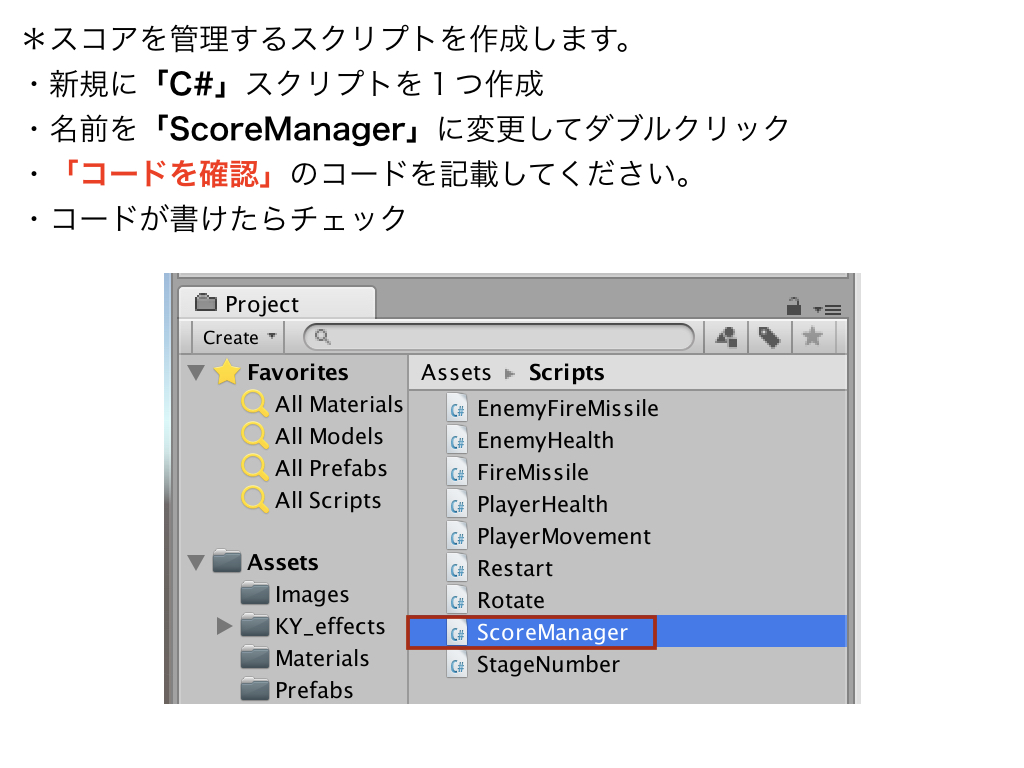
スコアを管理する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★追加
using UnityEngine.UI;
public class ScoreManager : MonoBehaviour
{
// ★追加
private int score = 0;
private Text scoreLabel;
void Start()
{
// ★追加
// 「Text」コンポーネントにアクセスして取得する(ポイント)
scoreLabel = this.gameObject.GetComponent<Text>();
scoreLabel.text = "Score " + score;
}
// ★追加
// スコアを加算するメソッド(命令ブロック)
// 「public」をつけて外部からこのメソッドにアクセスできるようにする(重要ポイント)
public void AddScore(int amount)
{
// 「amount」に入ってくる数値分を加算していく。
score += amount;
scoreLabel.text = "Score " + score;
}
}
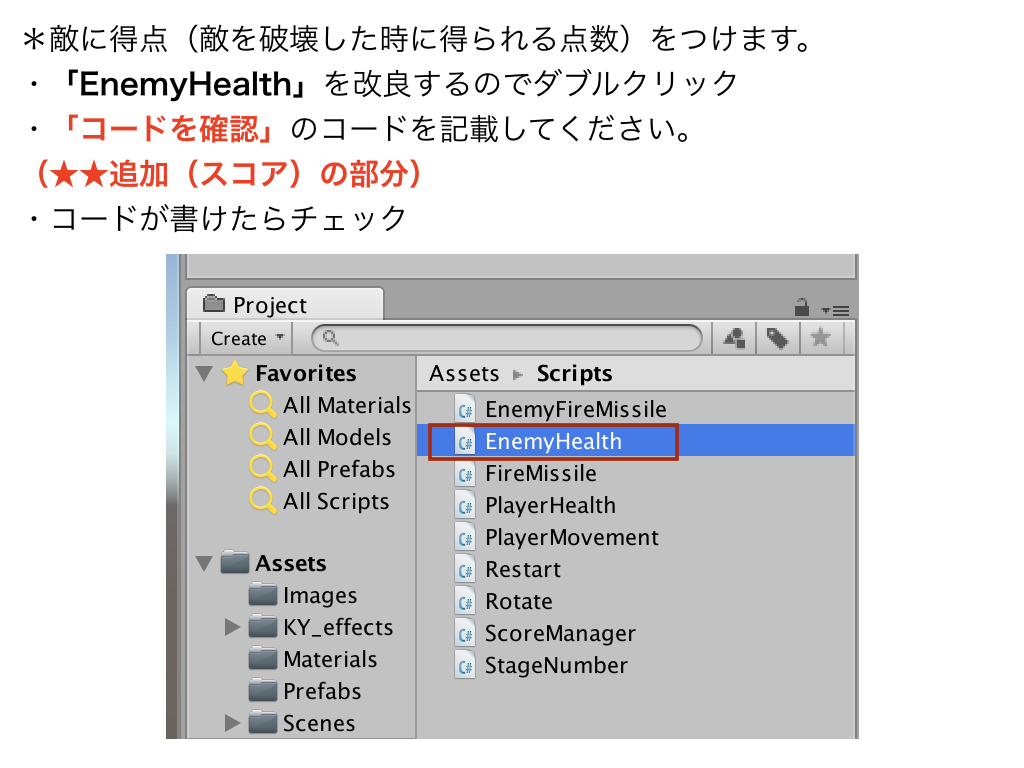
敵に得点をつける
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class EnemyHealth : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip destroySound;
public int enemyHP;
private Slider slider;
// ★★追加(スコア)
public int scoreValue;
private ScoreManager sm;
void Start()
{
slider = GameObject.Find("EnemyHPSlider").GetComponent<Slider>();
slider.maxValue = enemyHP;
slider.value = enemyHP;
// ★★追加(スコア)
// 「ScoreLabel」オブジェクトについている「ScoreManager」スクリプトにアクセスして取得する(ポイント)
sm = GameObject.Find("ScoreLabel").GetComponent<ScoreManager>();
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("Missile"))
{
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
enemyHP -= 1;
slider.value = enemyHP;
Destroy(other.gameObject);
if (enemyHP == 0)
{
Destroy(transform.root.gameObject);
AudioSource.PlayClipAtPoint(destroySound, transform.position);
// ★★追加(スコア)
// 敵を破壊した瞬間にスコアを加算するメソッドを呼び出す。
// 引数には「scoreValue」を入れる。
sm.AddScore(scoreValue);
}
}
}
}
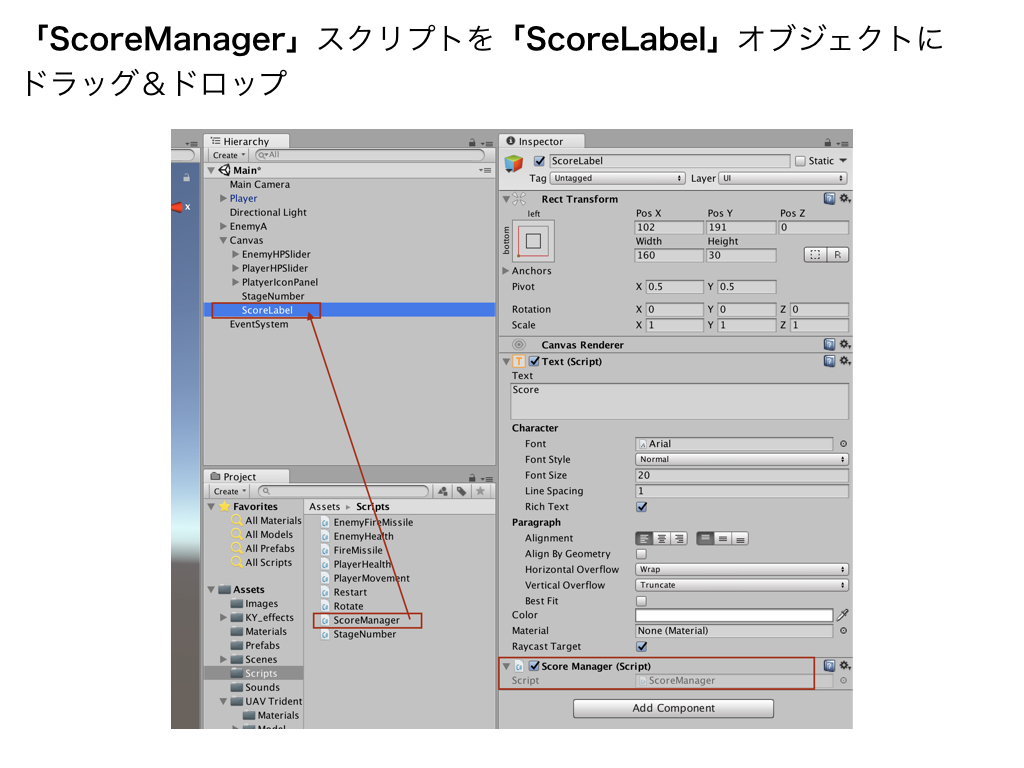
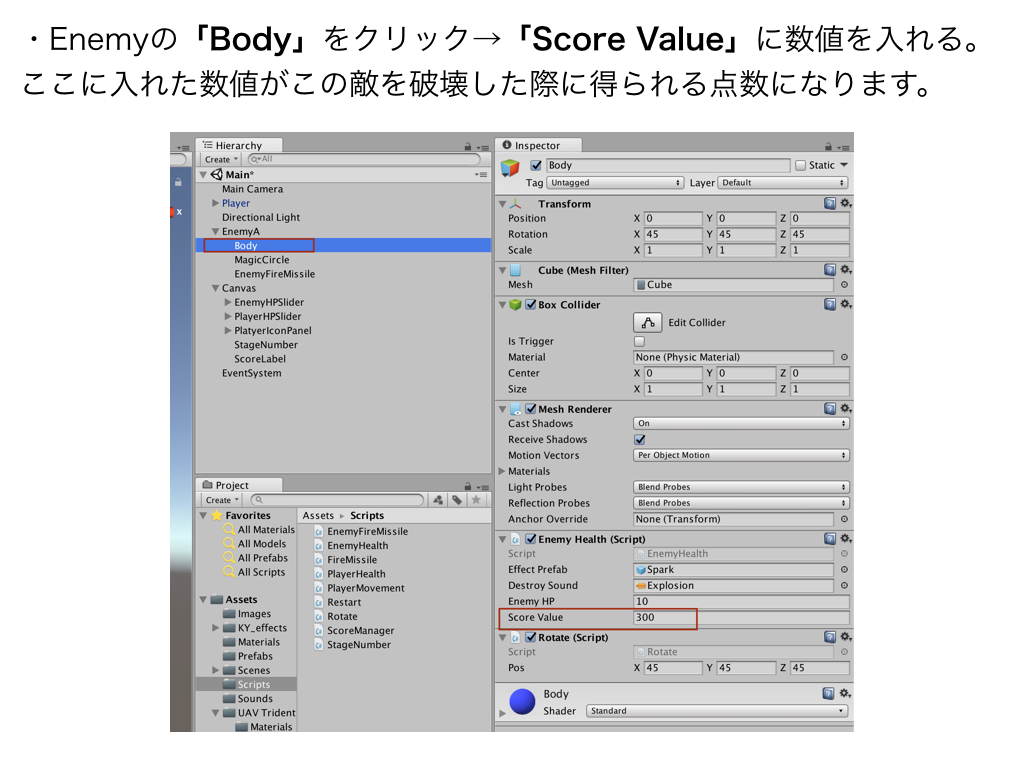
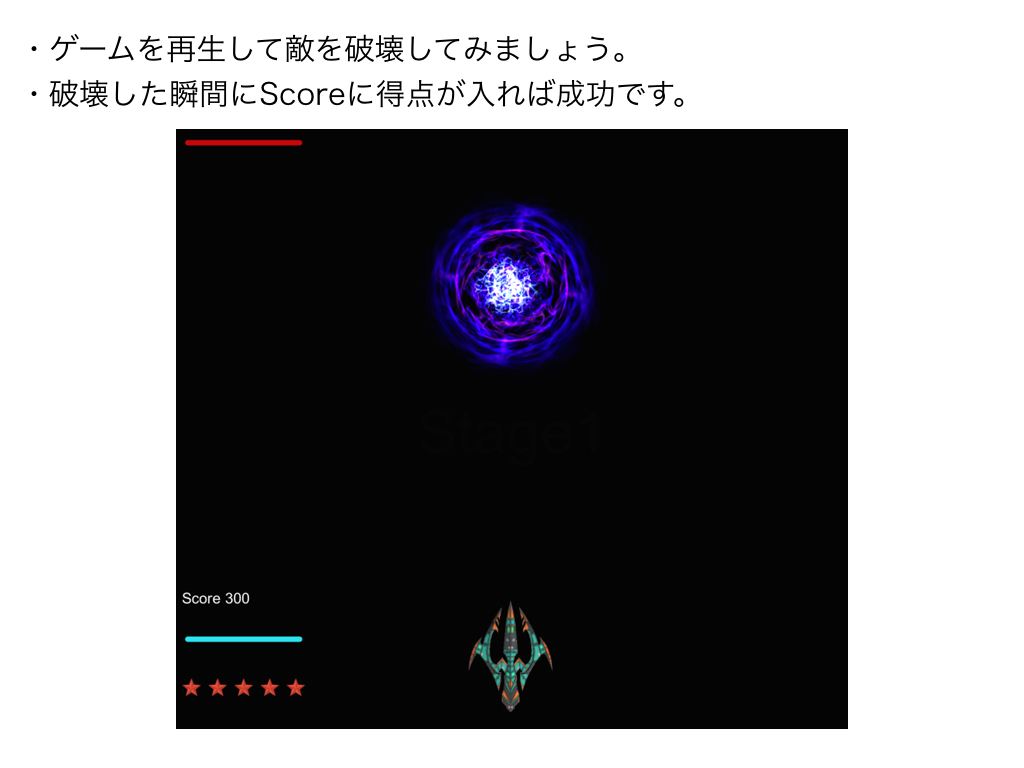
Danmaku I(基礎1/全22回)
他のコースを見る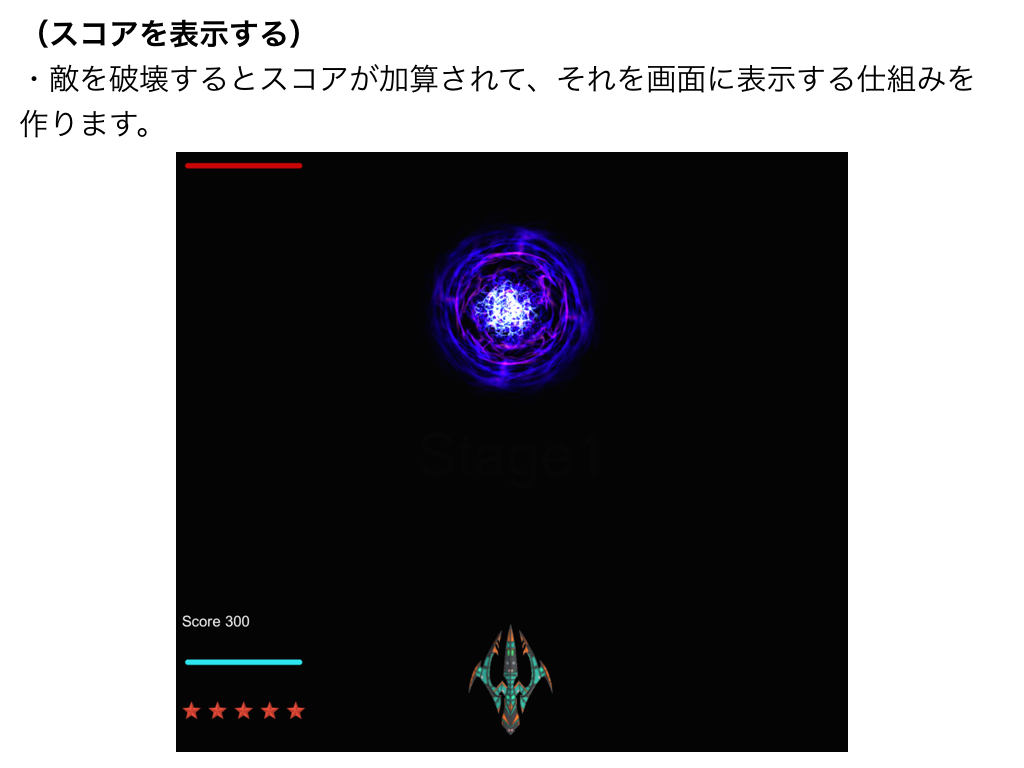
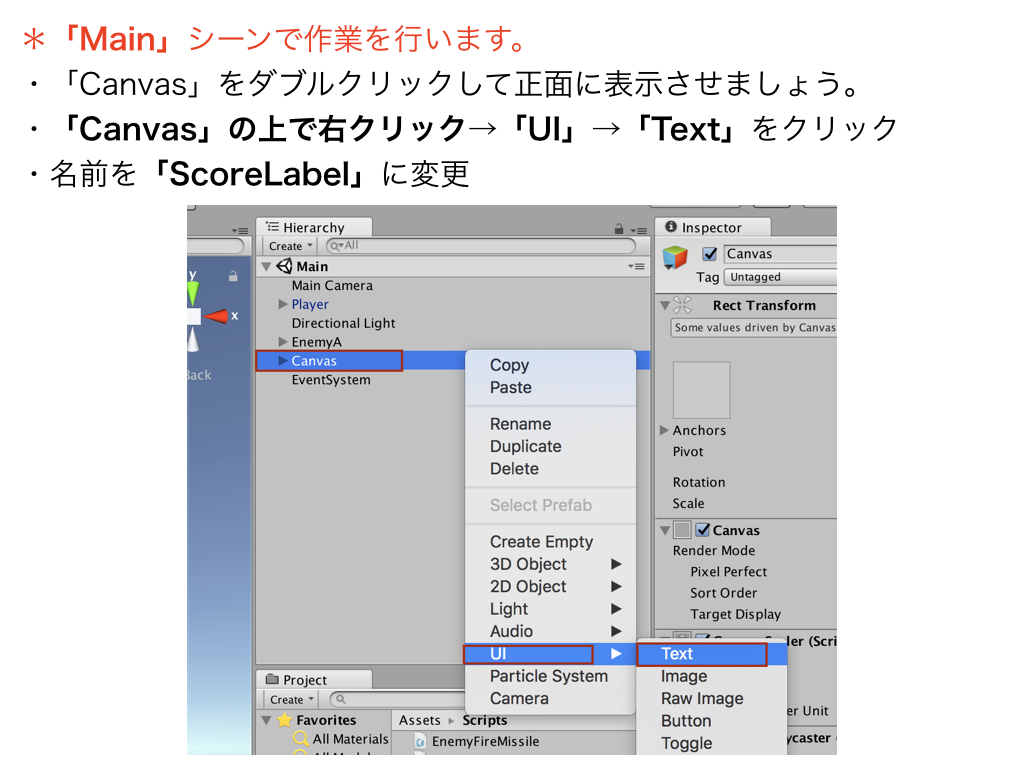
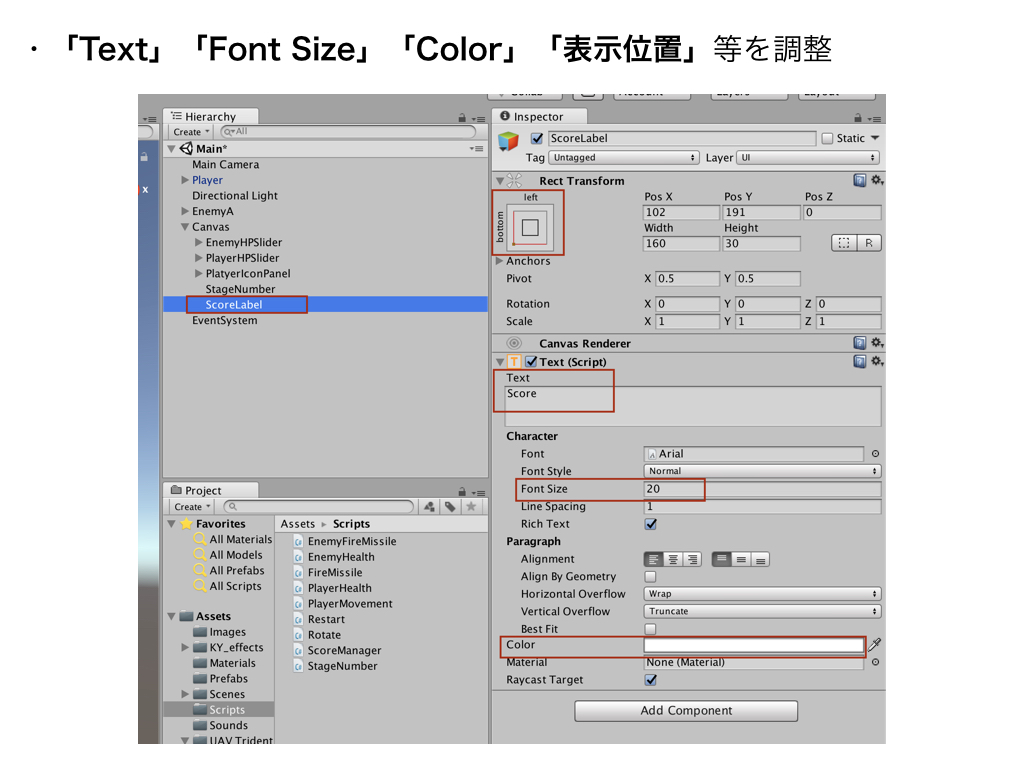
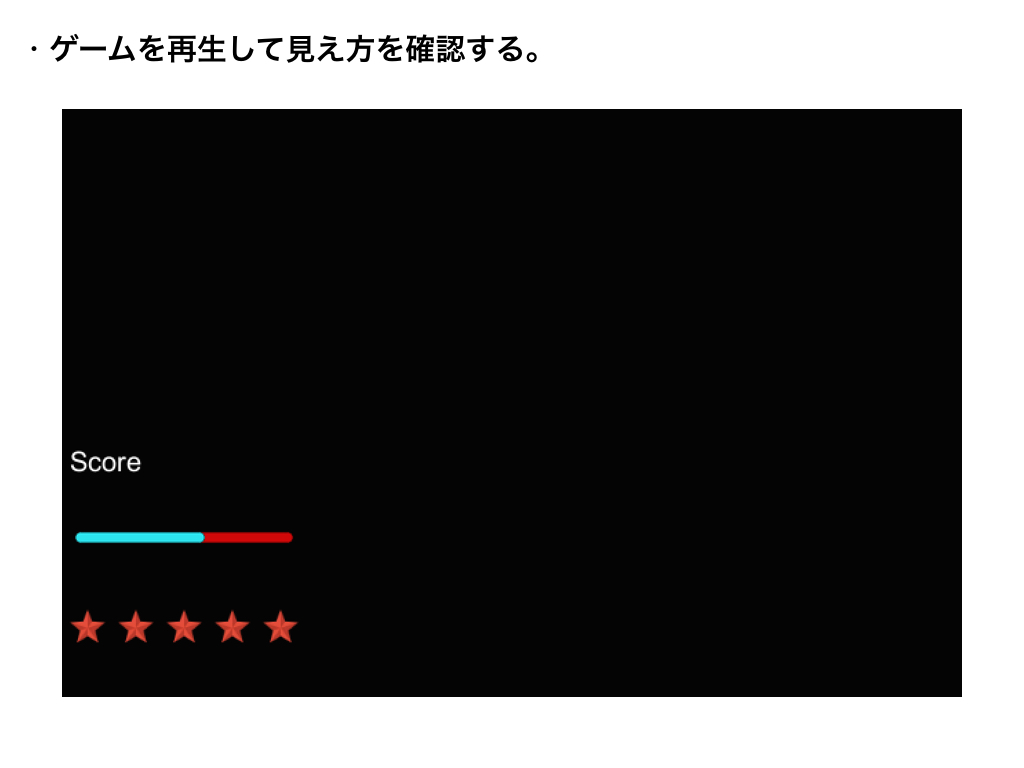
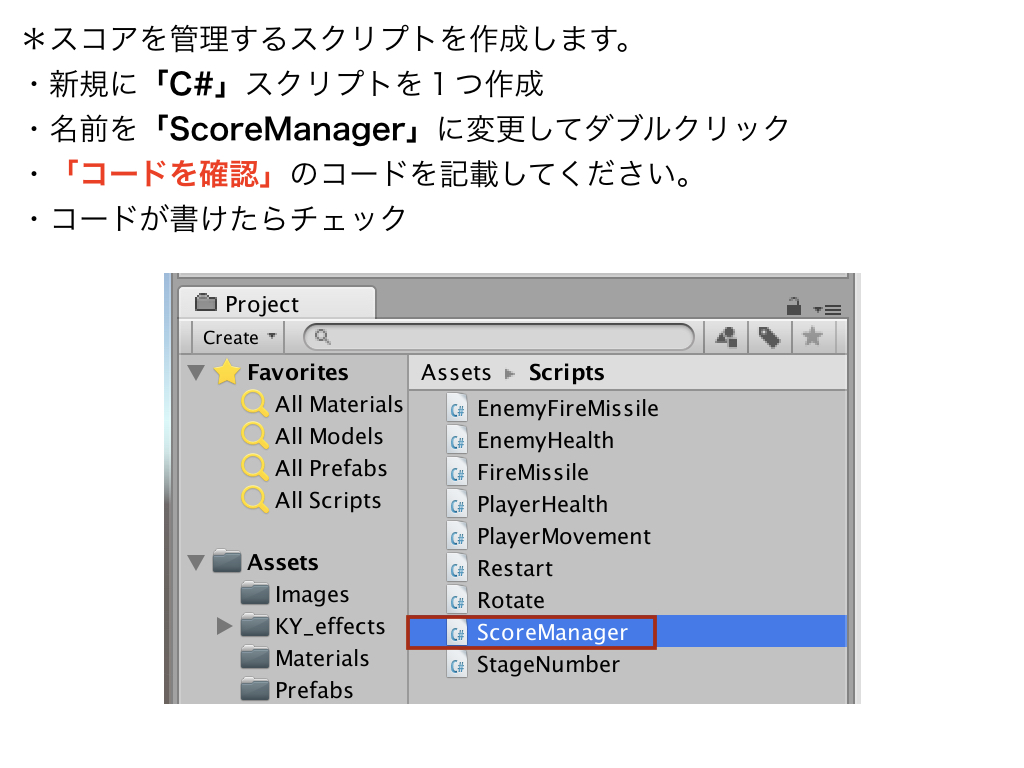
スコアを管理する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★追加
using UnityEngine.UI;
public class ScoreManager : MonoBehaviour
{
// ★追加
private int score = 0;
private Text scoreLabel;
void Start()
{
// ★追加
// 「Text」コンポーネントにアクセスして取得する(ポイント)
scoreLabel = this.gameObject.GetComponent<Text>();
scoreLabel.text = "Score " + score;
}
// ★追加
// スコアを加算するメソッド(命令ブロック)
// 「public」をつけて外部からこのメソッドにアクセスできるようにする(重要ポイント)
public void AddScore(int amount)
{
// 「amount」に入ってくる数値分を加算していく。
score += amount;
scoreLabel.text = "Score " + score;
}
}
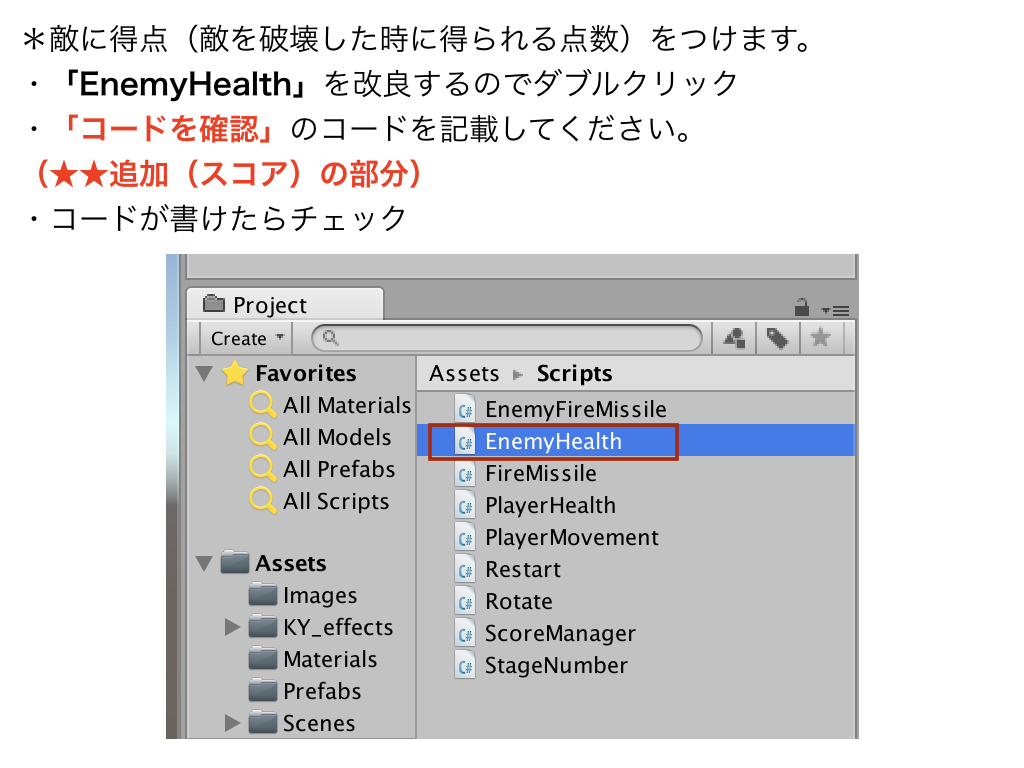
敵に得点をつける
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class EnemyHealth : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip destroySound;
public int enemyHP;
private Slider slider;
// ★★追加(スコア)
public int scoreValue;
private ScoreManager sm;
void Start()
{
slider = GameObject.Find("EnemyHPSlider").GetComponent<Slider>();
slider.maxValue = enemyHP;
slider.value = enemyHP;
// ★★追加(スコア)
// 「ScoreLabel」オブジェクトについている「ScoreManager」スクリプトにアクセスして取得する(ポイント)
sm = GameObject.Find("ScoreLabel").GetComponent<ScoreManager>();
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("Missile"))
{
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
enemyHP -= 1;
slider.value = enemyHP;
Destroy(other.gameObject);
if (enemyHP == 0)
{
Destroy(transform.root.gameObject);
AudioSource.PlayClipAtPoint(destroySound, transform.position);
// ★★追加(スコア)
// 敵を破壊した瞬間にスコアを加算するメソッドを呼び出す。
// 引数には「scoreValue」を入れる。
sm.AddScore(scoreValue);
}
}
}
}
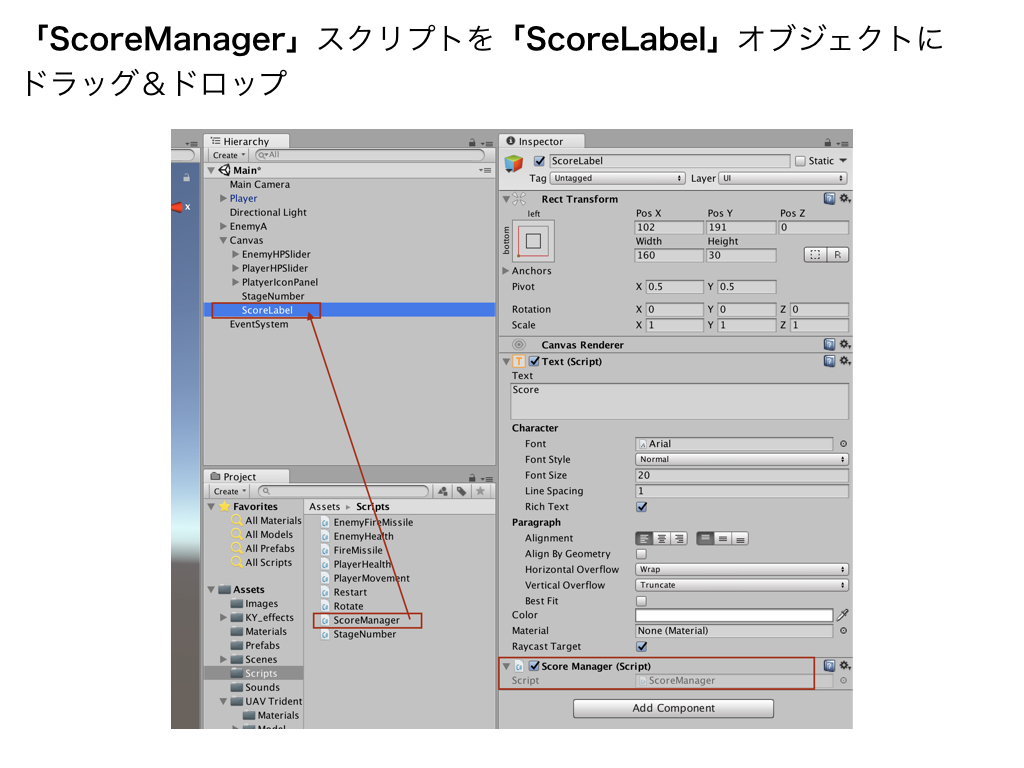
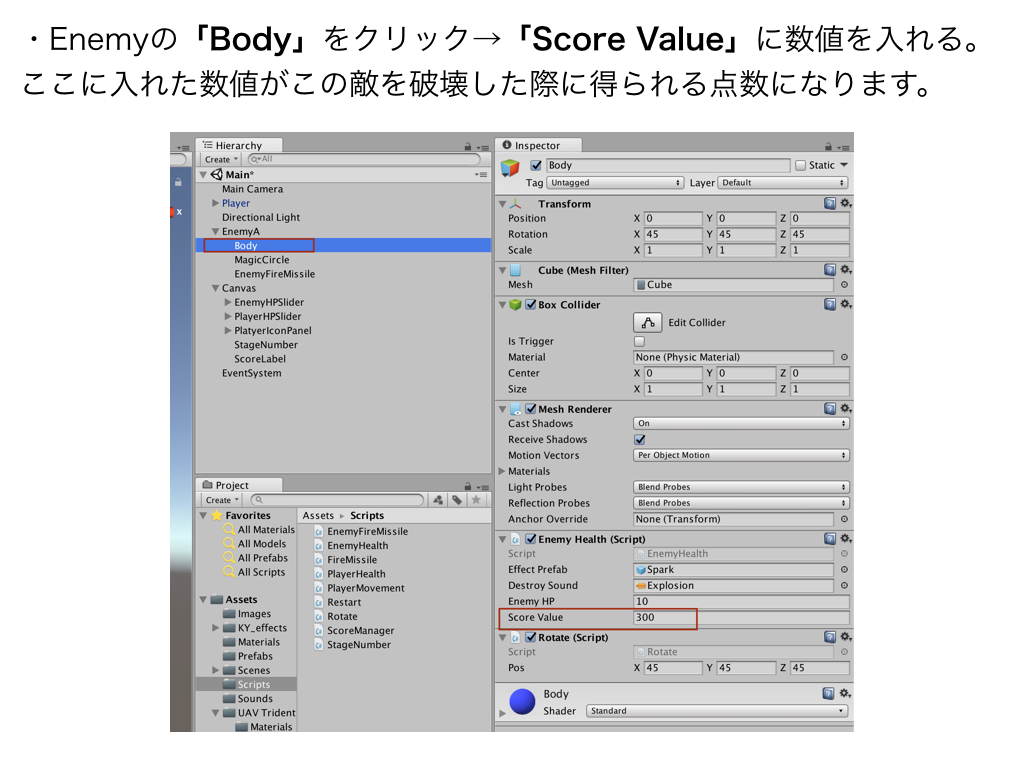
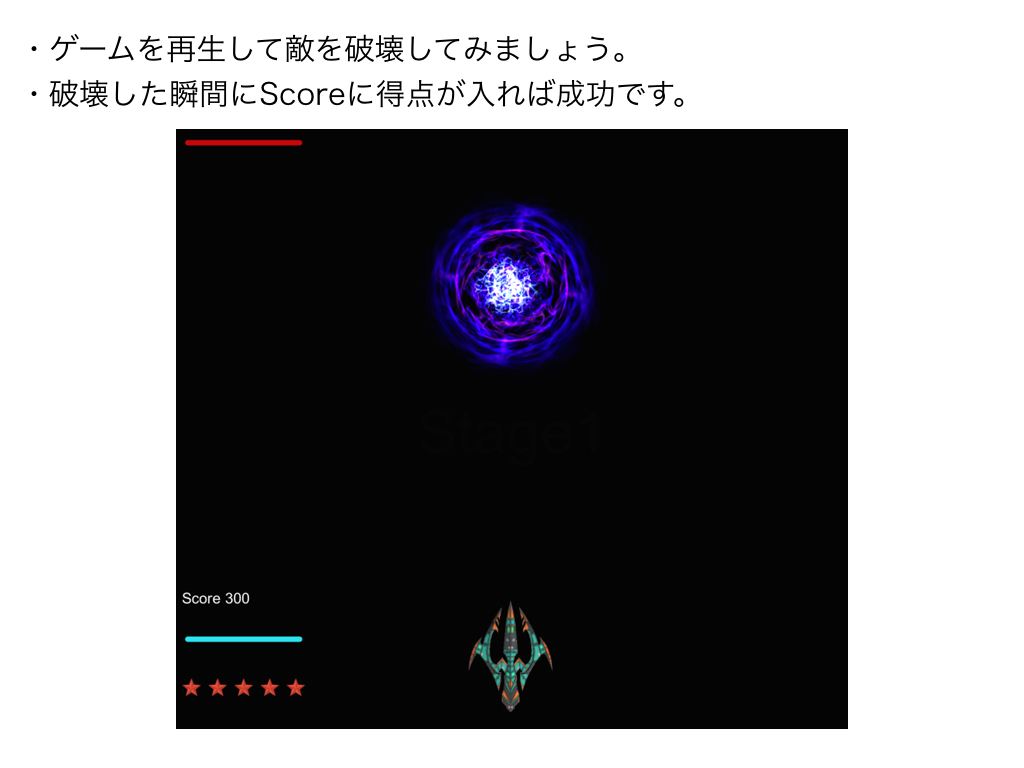
UI②(スコアを画面に表示する)