アイテムの作成④(防御シールド)
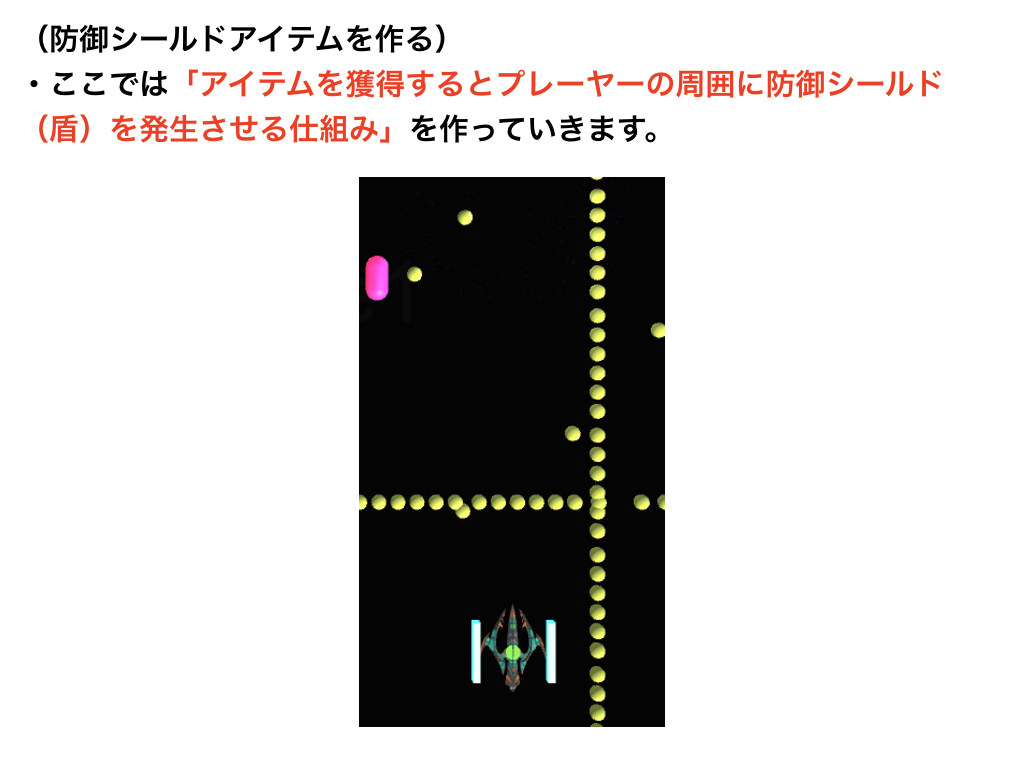
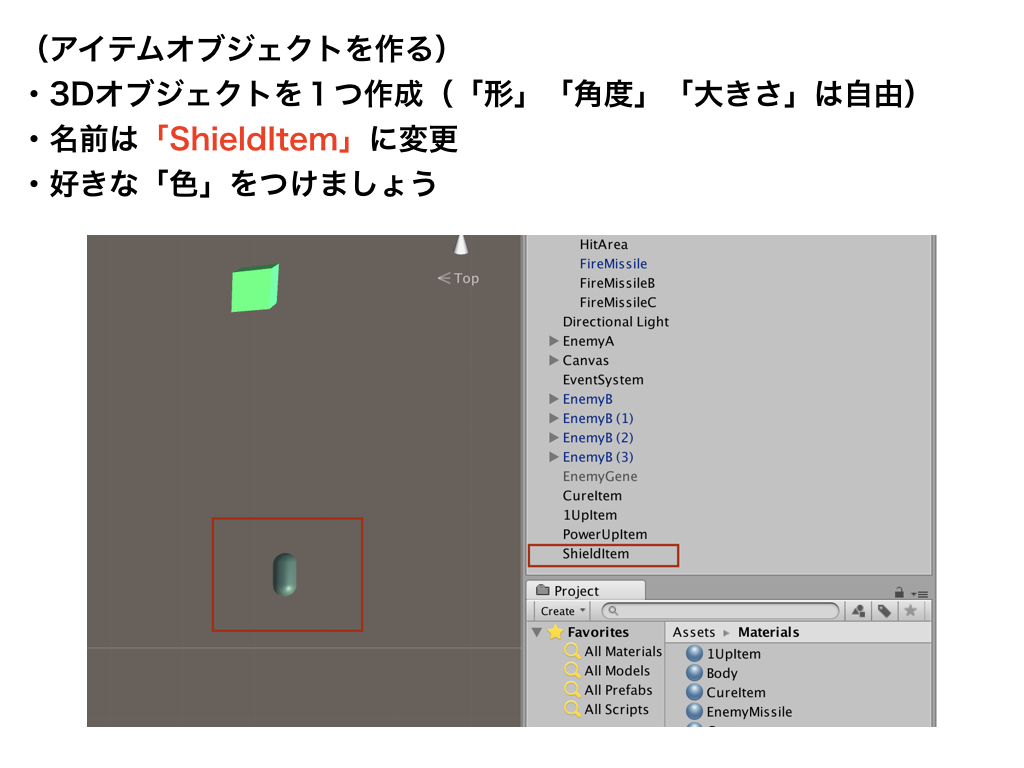
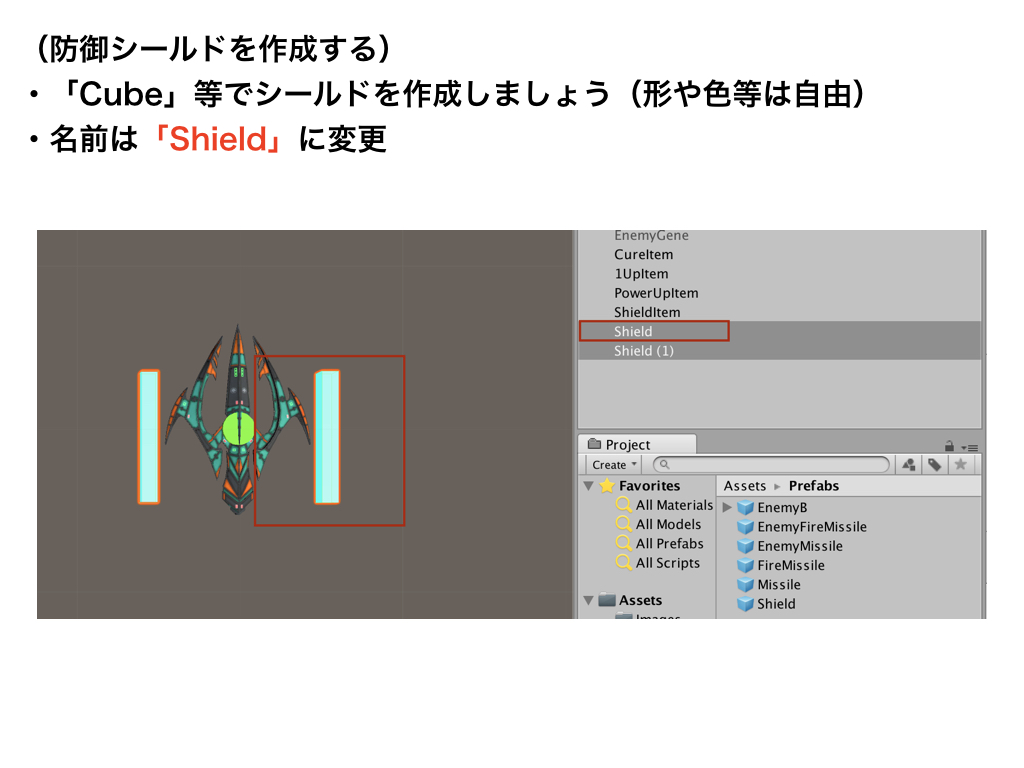
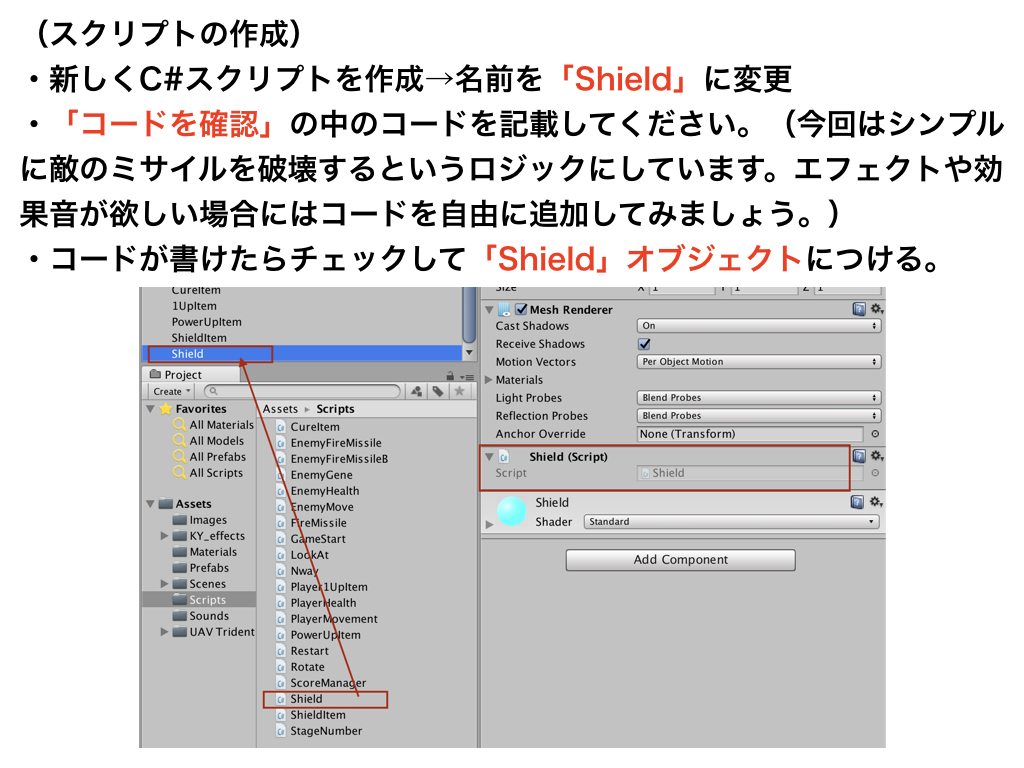
防御シールド
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Shield : MonoBehaviour
{
// ぶつかってくる敵のミサイルを破壊する。
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("EnemyMissile"))
{
Destroy(other.gameObject);
}
}
}
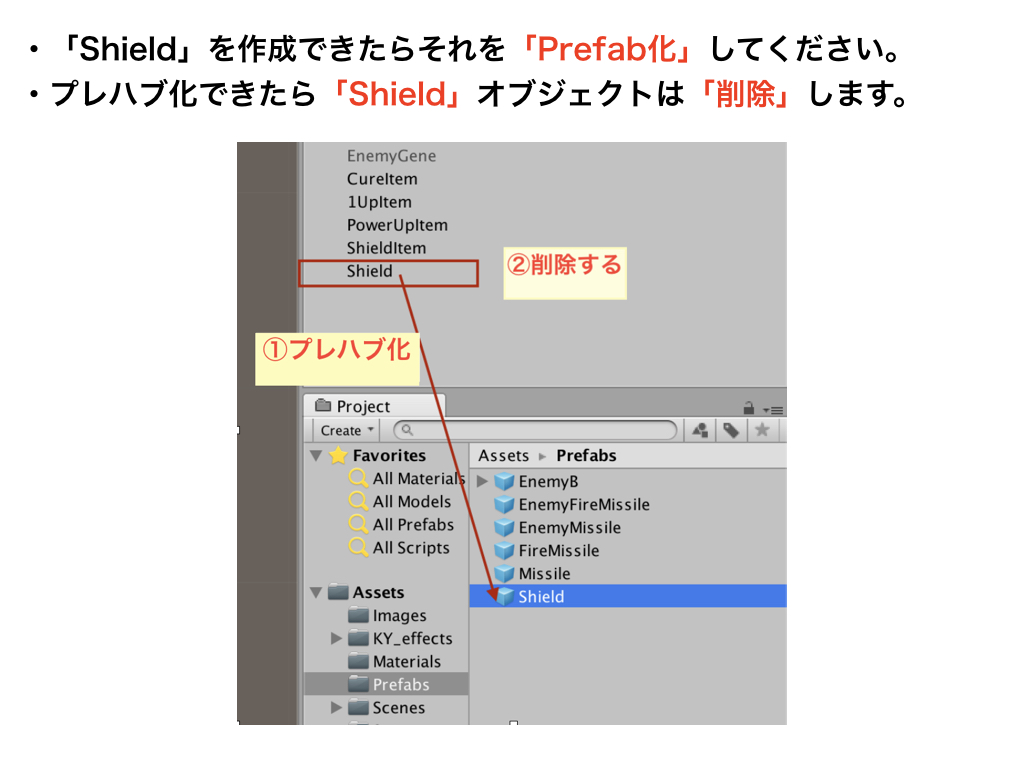
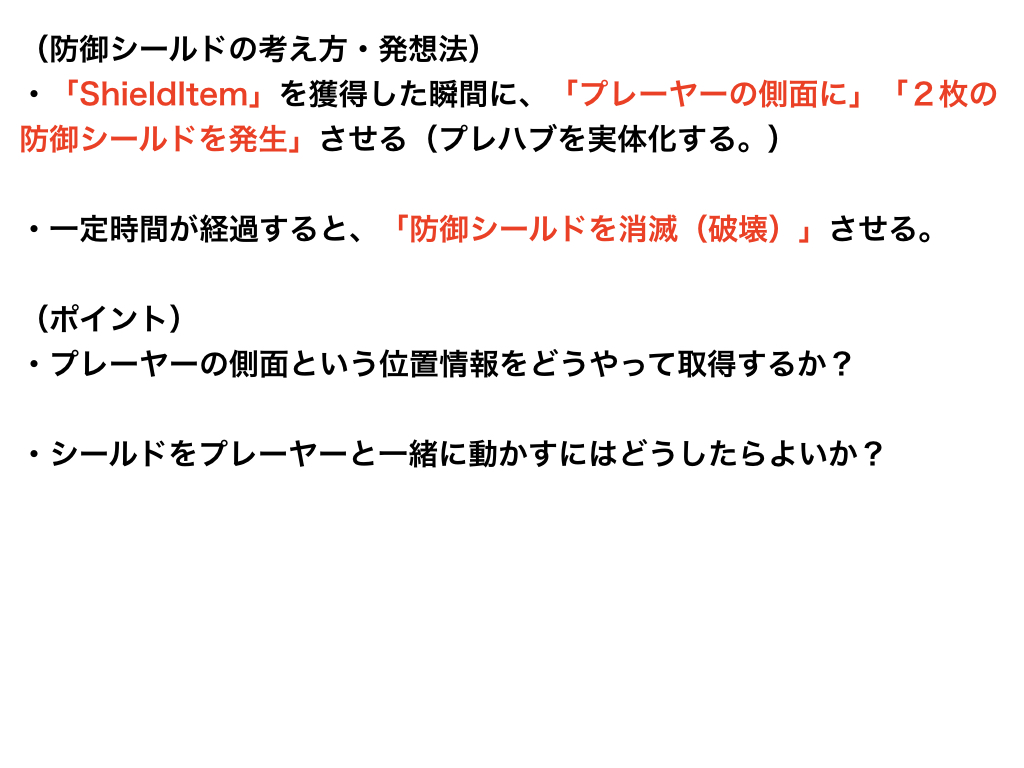
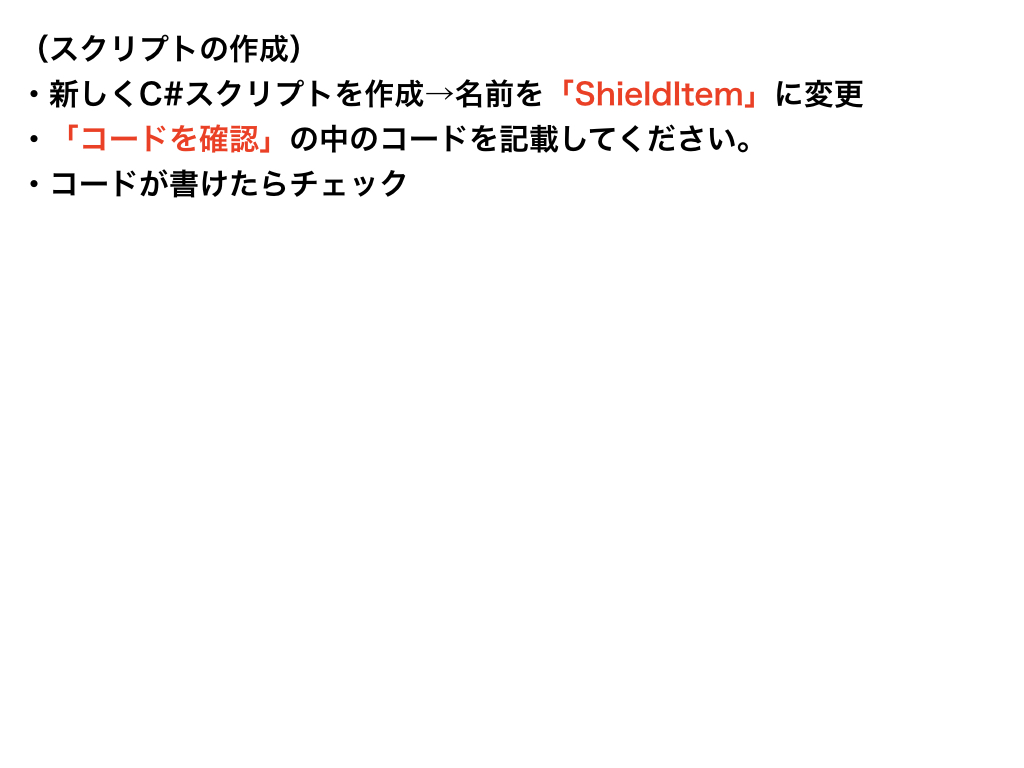
防御シールドアイテム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShieldItem : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip getSound;
public GameObject shieldPrefab;
private GameObject player;
private Vector3 pos;
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Missile"))
{
// エフェクトと効果音を発生させる。
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
AudioSource.PlayClipAtPoint(getSound, Camera.main.transform.position);
// プレーヤーの位置情報を取得する。
player = GameObject.FindWithTag("Player");
pos = player.transform.position;
// プレーヤーの側面の位置に2枚の防御シールドを発生させる。
GameObject shieldA = Instantiate(shieldPrefab, new Vector3(pos.x - 0.5f, pos.y, pos.z), Quaternion.identity);
GameObject shieldB = Instantiate(shieldPrefab, new Vector3(pos.x + 0.5f, pos.y, pos.z), Quaternion.identity);
// 発生させた防御シールドをプレーヤーの子供に設定する(親子関係)
// 親子関係にすることで一緒に動くようになる。
shieldA.transform.SetParent(player.transform);
shieldB.transform.SetParent(player.transform);
// 発生させた防御シールドを5秒後に消滅させる。
Destroy(shieldA, 5);
Destroy(shieldB, 5);
// アイテムを破壊する
Destroy(gameObject);
}
}
}
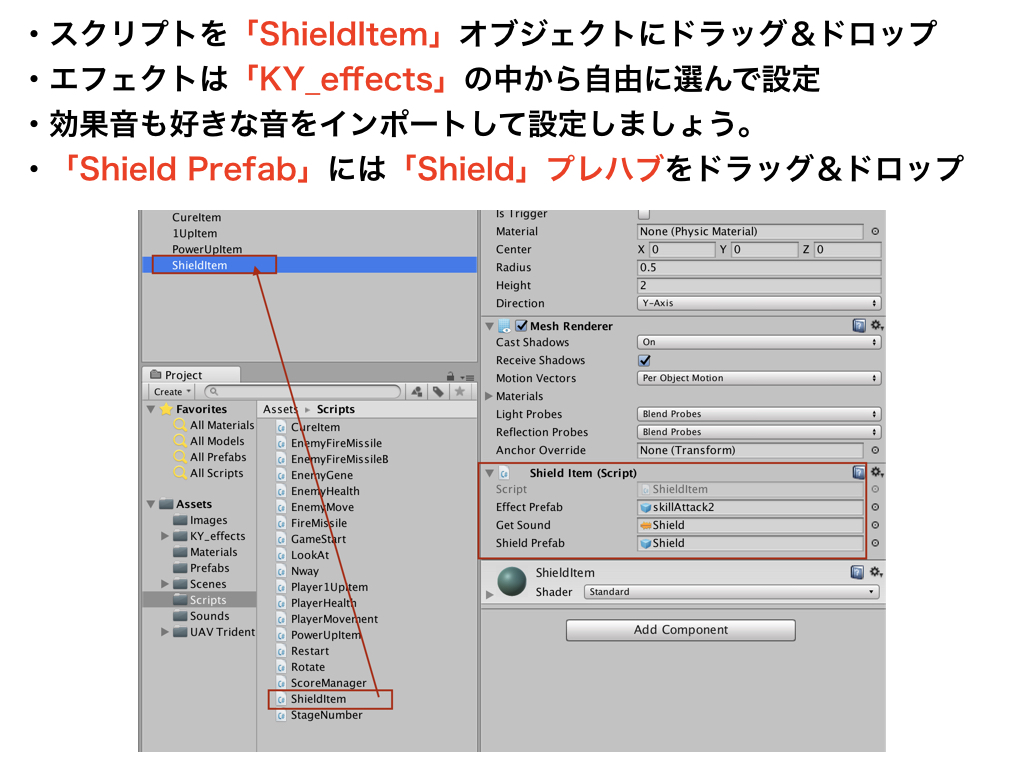
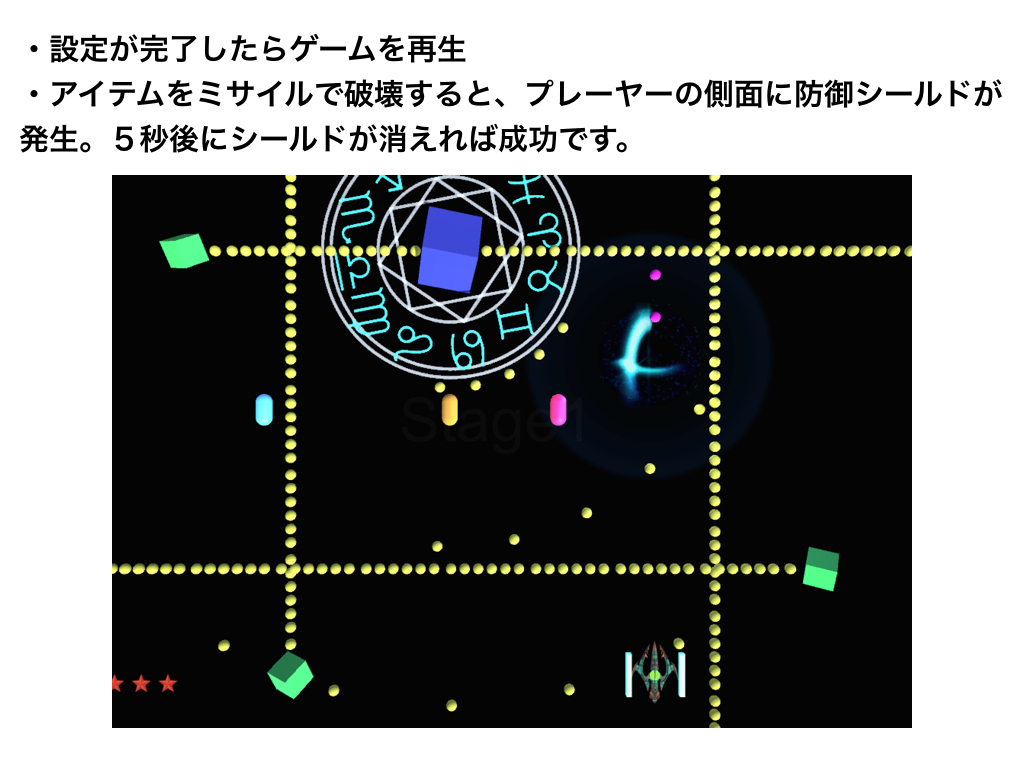
Danmaku Ⅱ(基礎2/全24回)
他のコースを見る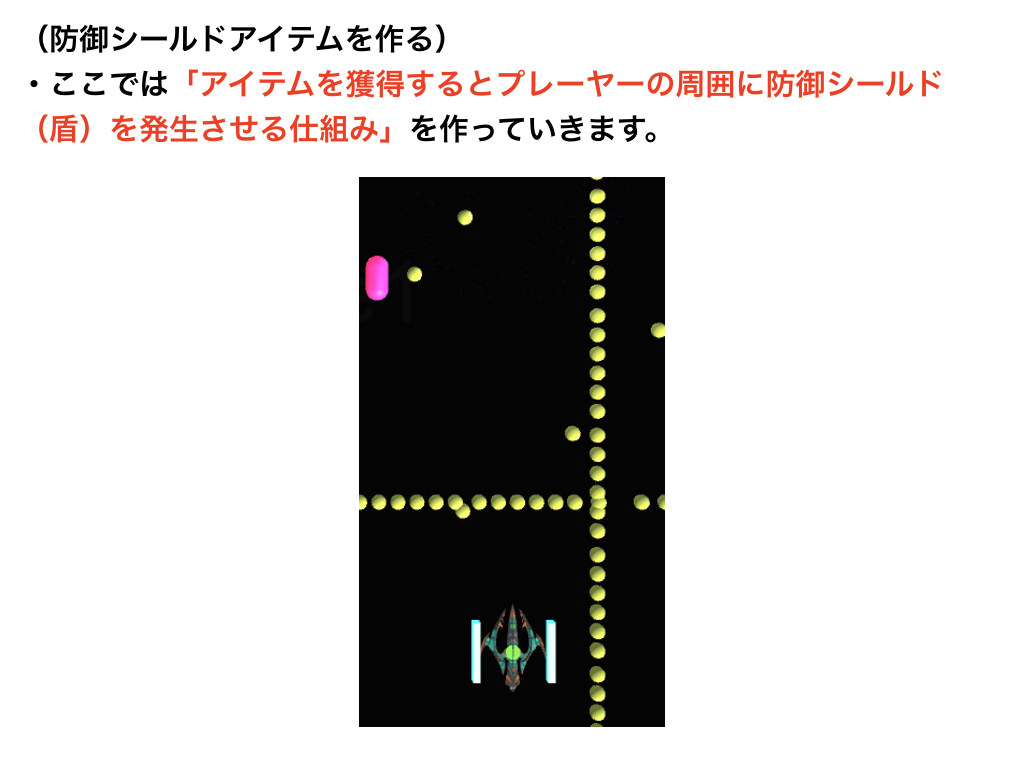
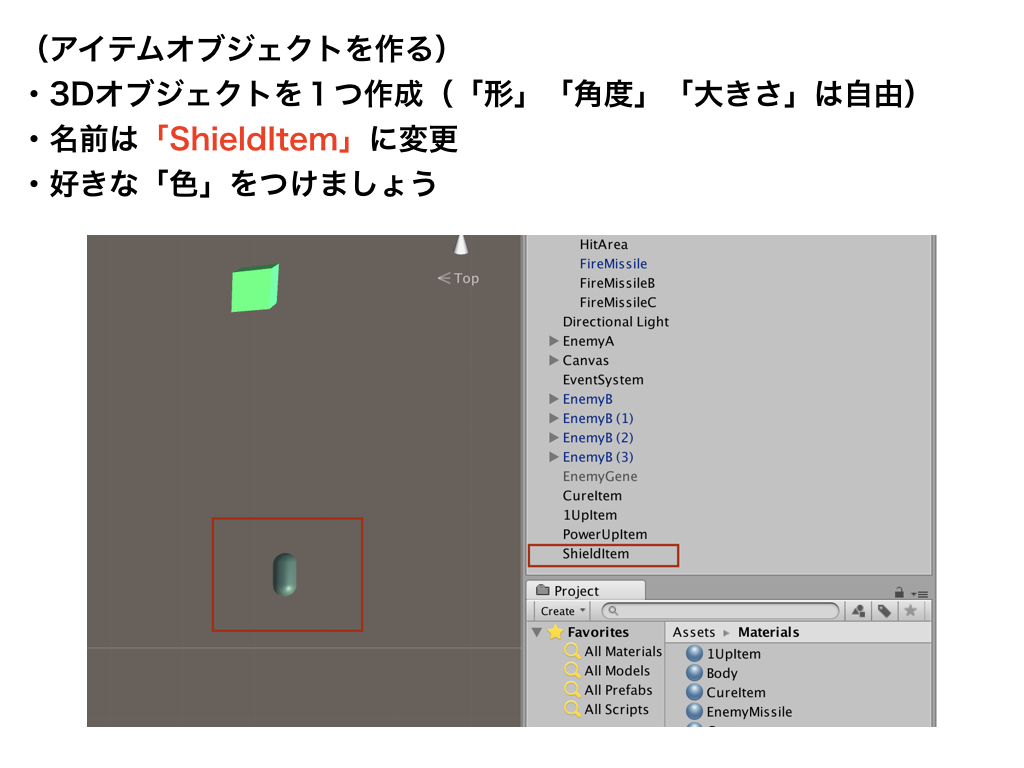
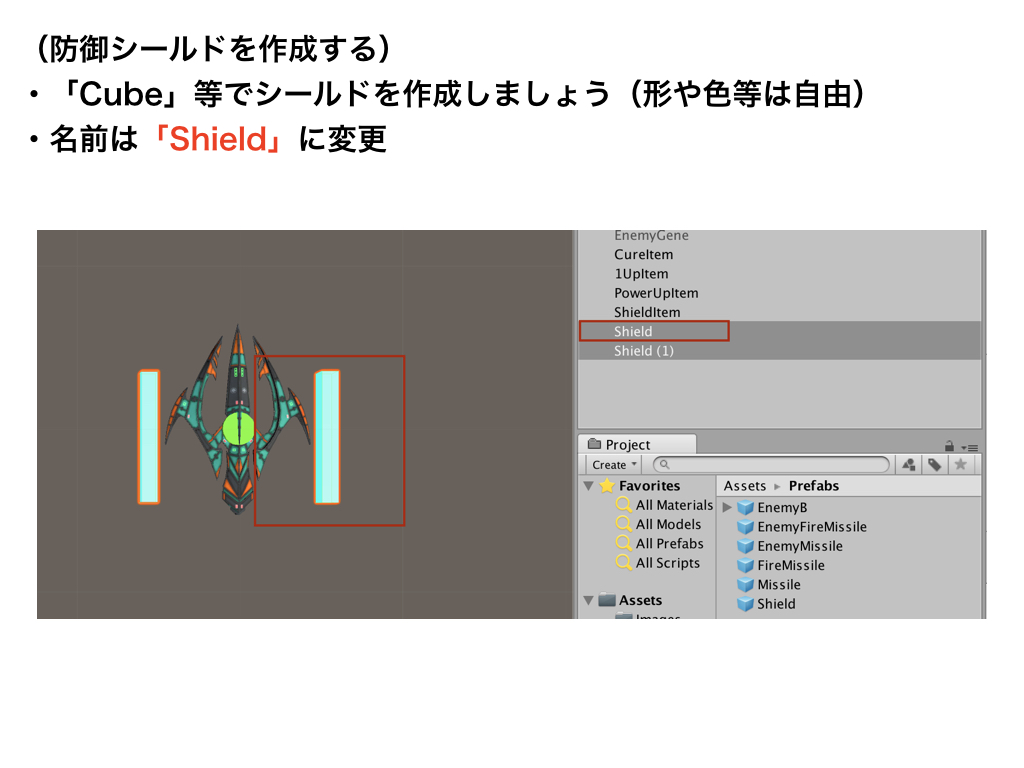
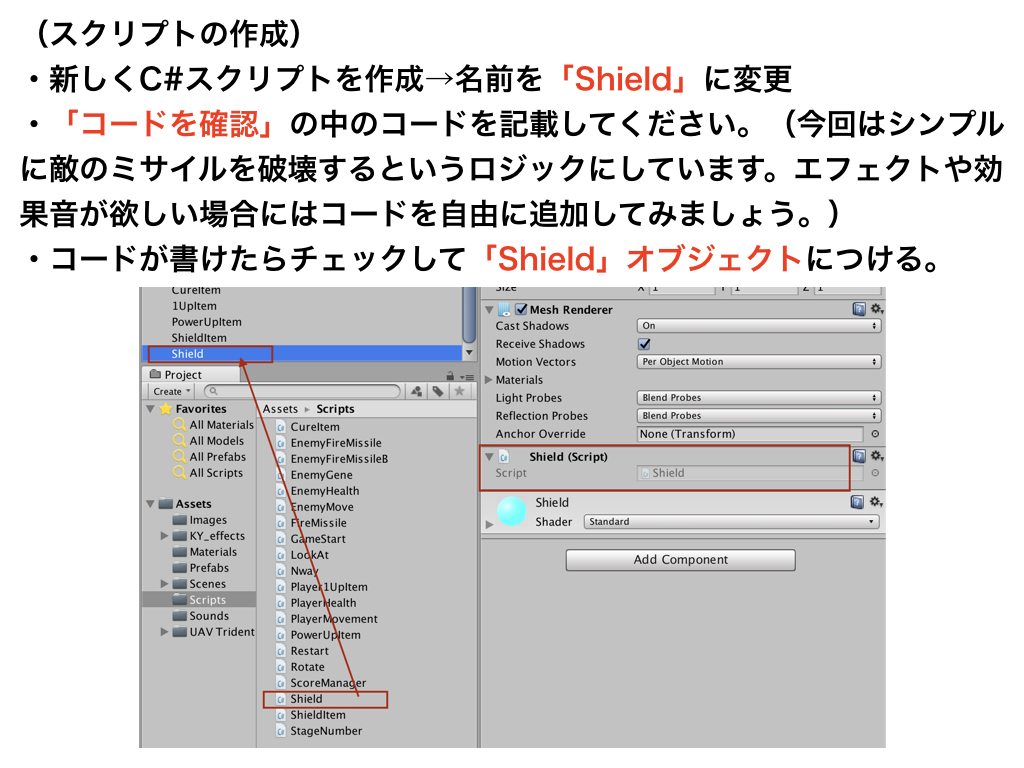
防御シールド
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Shield : MonoBehaviour
{
// ぶつかってくる敵のミサイルを破壊する。
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("EnemyMissile"))
{
Destroy(other.gameObject);
}
}
}
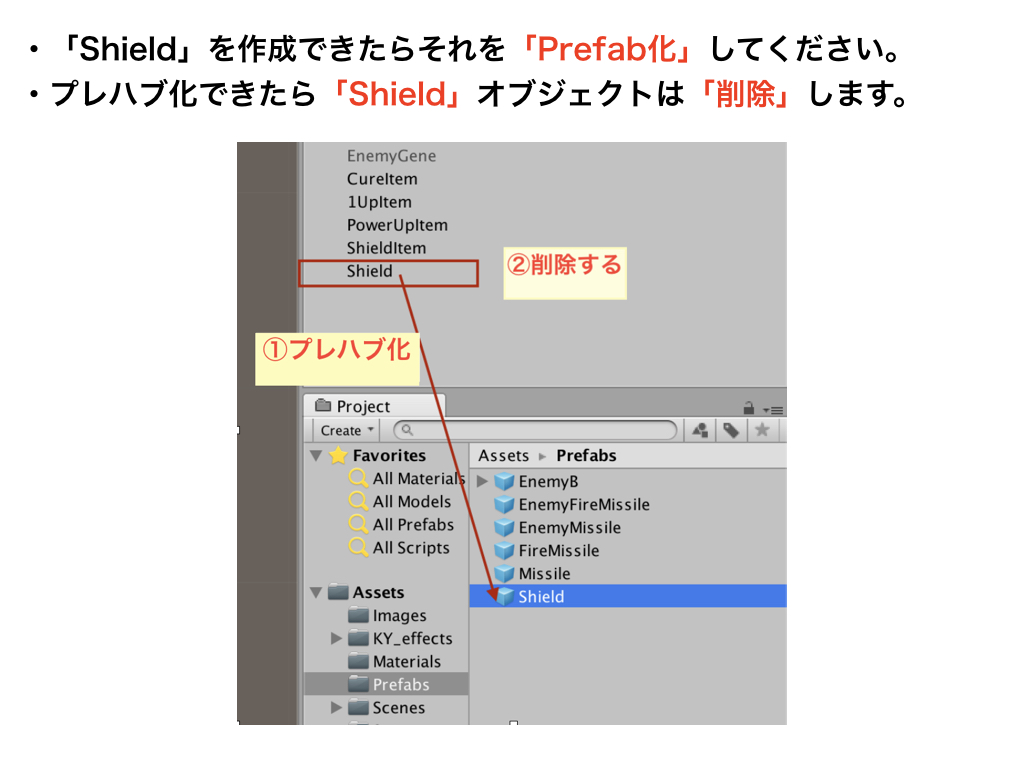
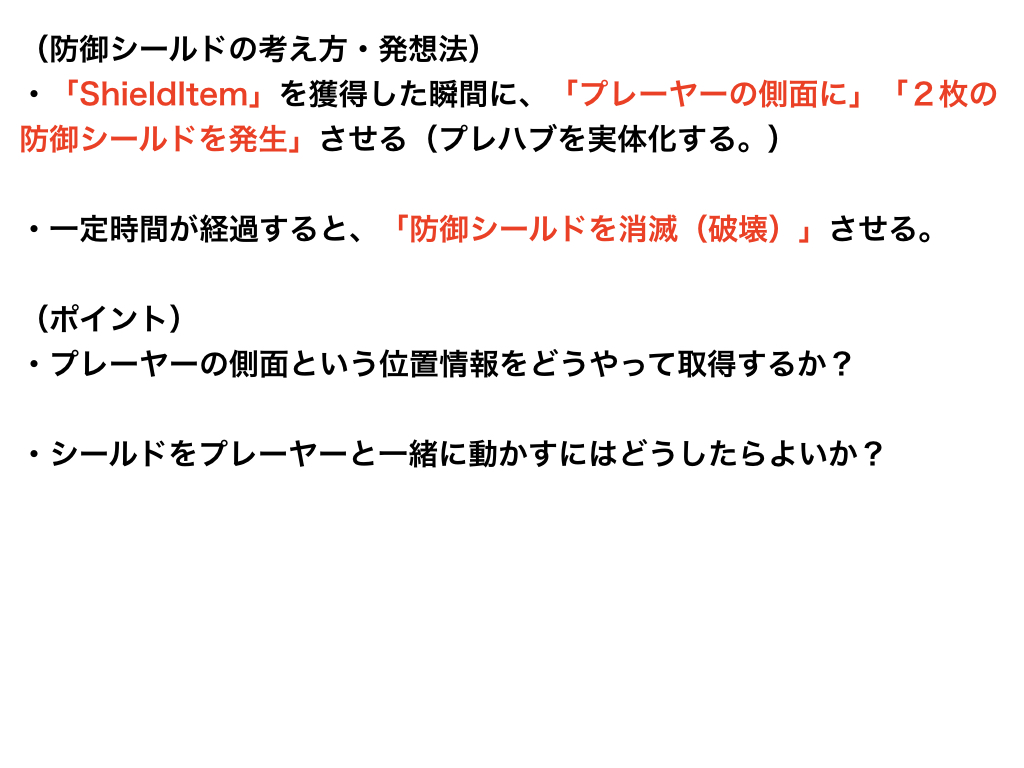
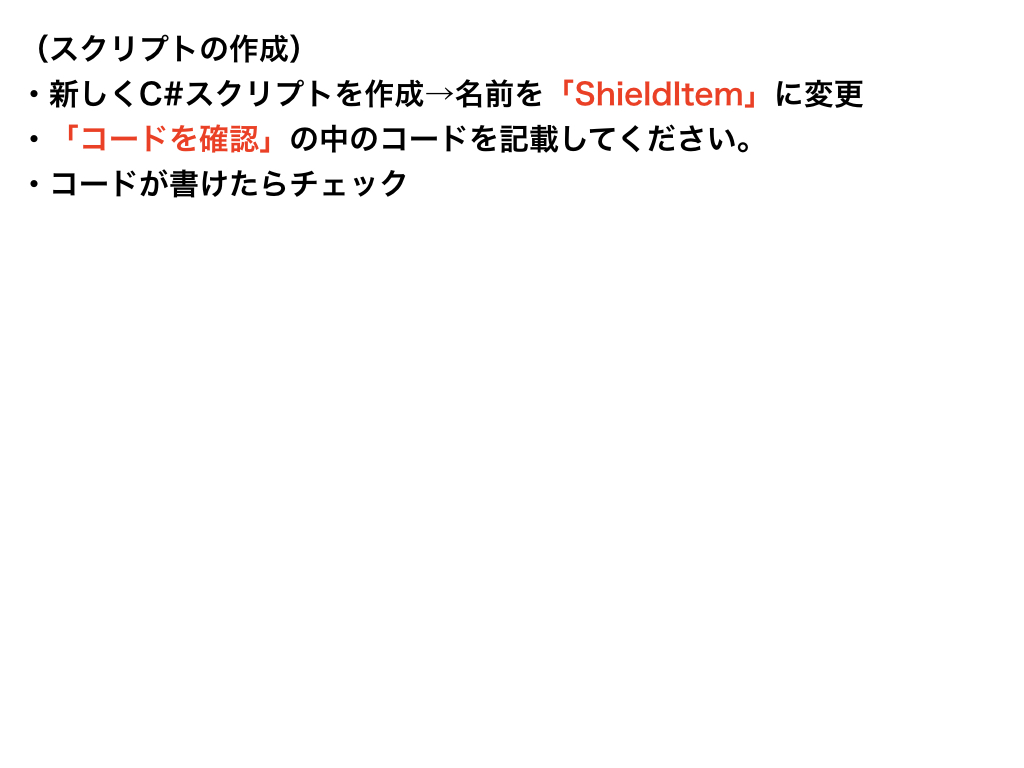
防御シールドアイテム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShieldItem : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip getSound;
public GameObject shieldPrefab;
private GameObject player;
private Vector3 pos;
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Missile"))
{
// エフェクトと効果音を発生させる。
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
AudioSource.PlayClipAtPoint(getSound, Camera.main.transform.position);
// プレーヤーの位置情報を取得する。
player = GameObject.FindWithTag("Player");
pos = player.transform.position;
// プレーヤーの側面の位置に2枚の防御シールドを発生させる。
GameObject shieldA = Instantiate(shieldPrefab, new Vector3(pos.x - 0.5f, pos.y, pos.z), Quaternion.identity);
GameObject shieldB = Instantiate(shieldPrefab, new Vector3(pos.x + 0.5f, pos.y, pos.z), Quaternion.identity);
// 発生させた防御シールドをプレーヤーの子供に設定する(親子関係)
// 親子関係にすることで一緒に動くようになる。
shieldA.transform.SetParent(player.transform);
shieldB.transform.SetParent(player.transform);
// 発生させた防御シールドを5秒後に消滅させる。
Destroy(shieldA, 5);
Destroy(shieldB, 5);
// アイテムを破壊する
Destroy(gameObject);
}
}
}
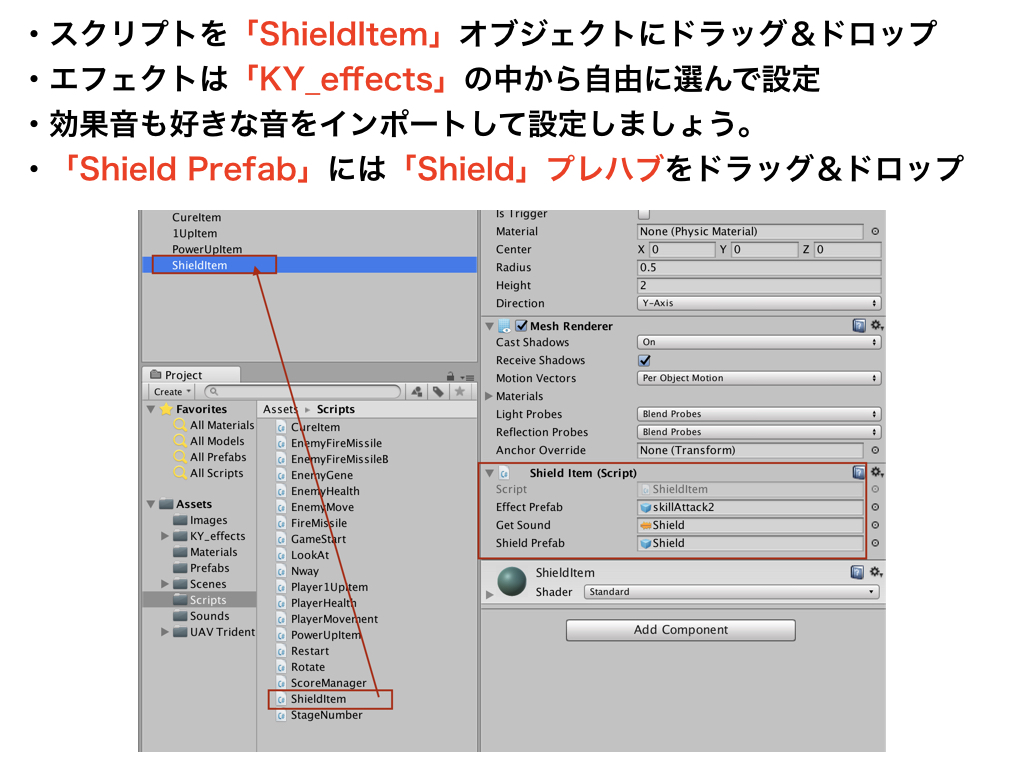
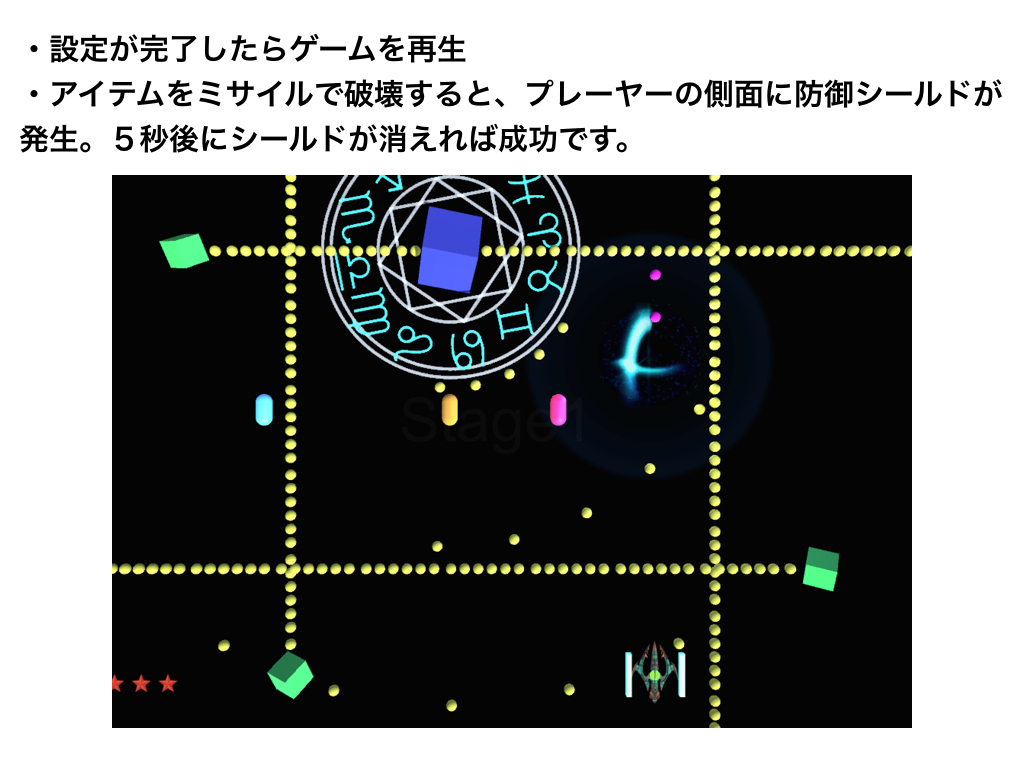
アイテムの作成④(防御シールド)