敵の攻撃を停止させるアイテムの作成
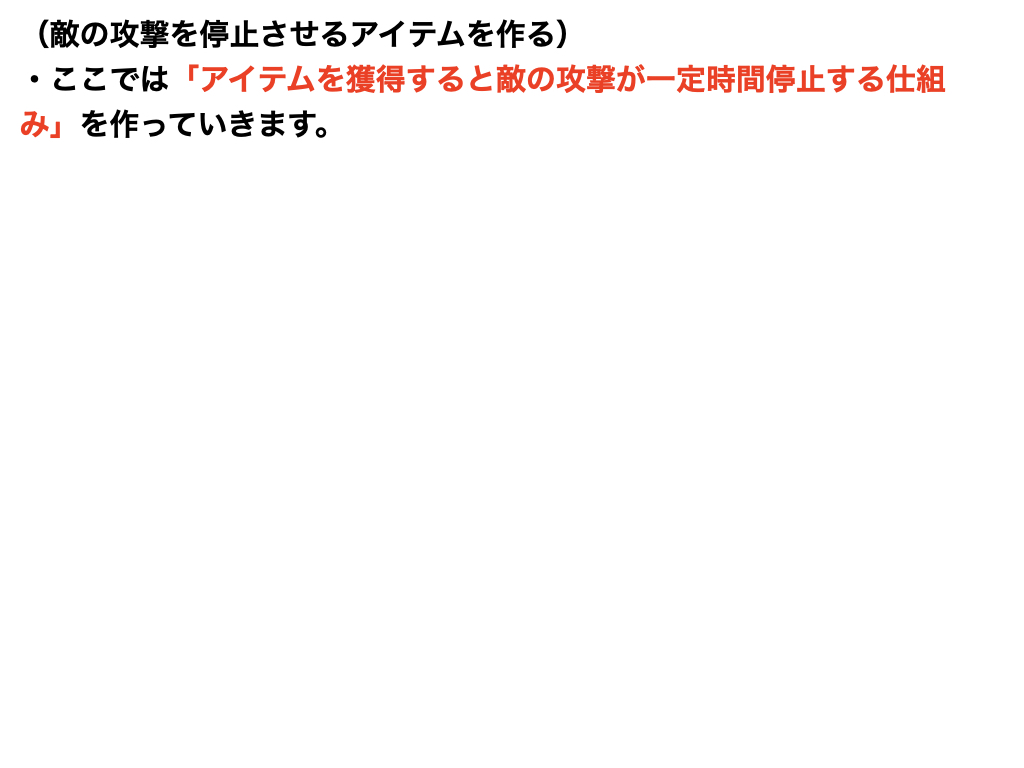
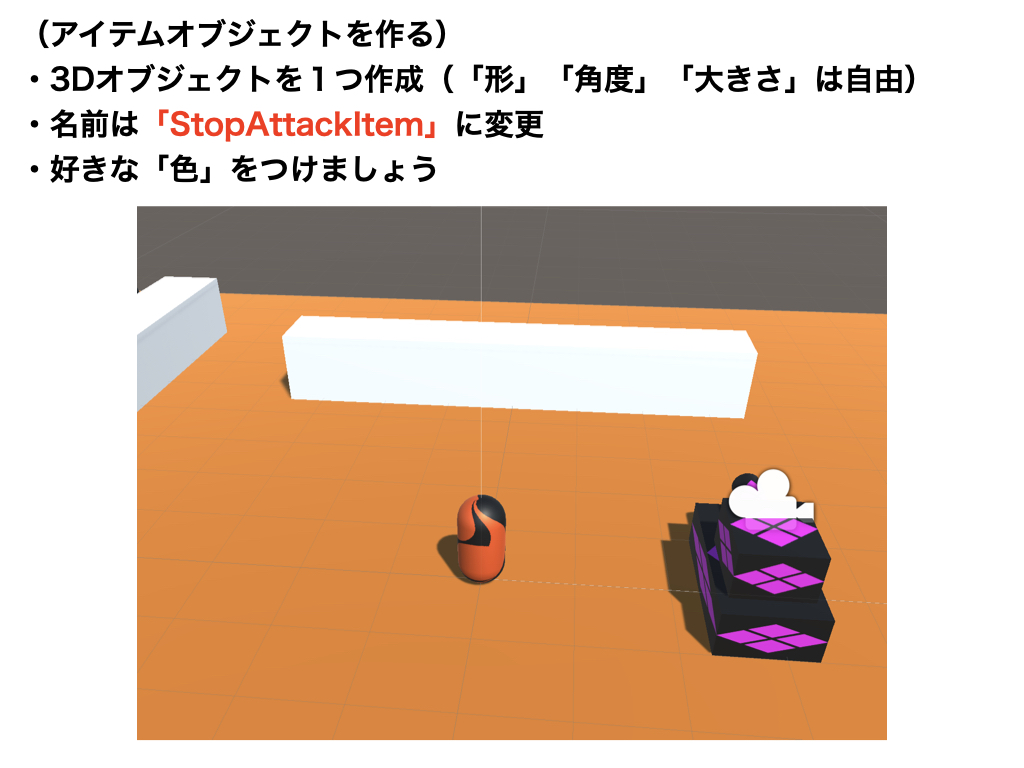
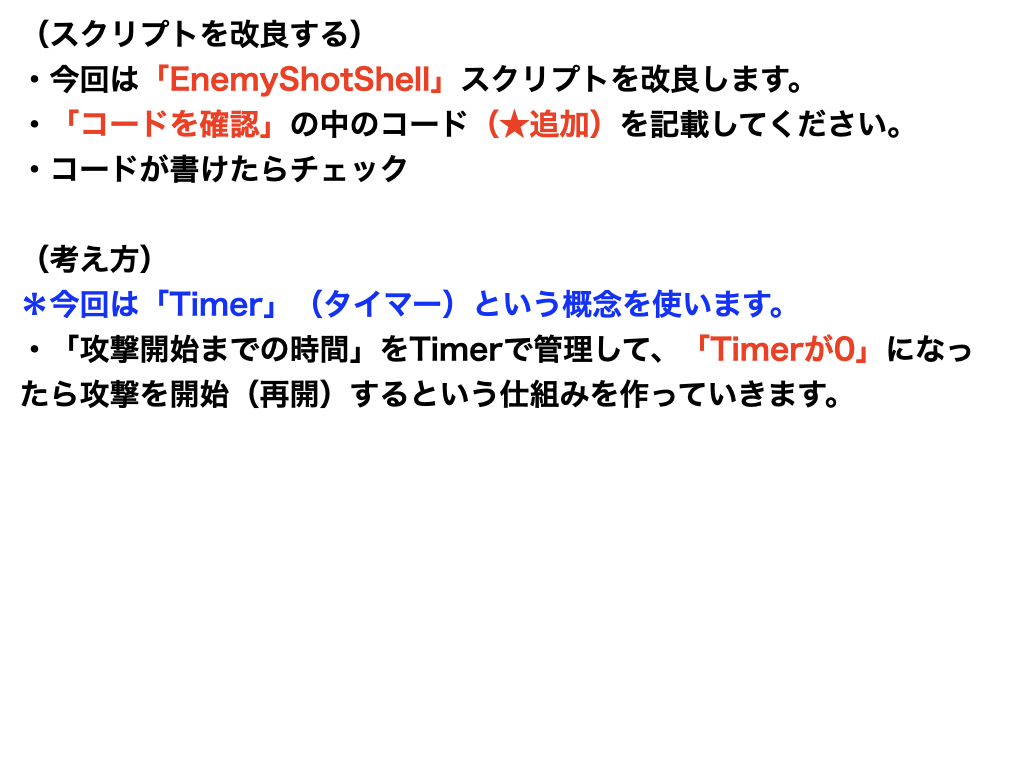
敵の攻撃を停止させる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyShotShell : MonoBehaviour
{
public float shotSpeed;
[SerializeField]
private GameObject enemyShellPrefab;
[SerializeField]
private AudioClip shotSound;
private int interval;
// ★追加
// 何秒間停止させるかは自由
public float stopTimer = 5.0f;
void Update()
{
interval += 1;
// ★追加
stopTimer -= Time.deltaTime;
// タイマーが0未満になったら、0で止める。
if (stopTimer < 0)
{
stopTimer = 0;
}
// ★追加
if (interval % 60 == 0 && stopTimer <= 0)
{
GameObject enemyShell = Instantiate(enemyShellPrefab, transform.position, Quaternion.identity);
Rigidbody enemyShellRb = enemyShell.GetComponent<Rigidbody>();
enemyShellRb.AddForce(transform.forward * shotSpeed);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
Destroy(enemyShell, 3.0f);
}
}
// ★追加
// 敵の攻撃をストップさせるメソッド(Timerの時間を増加させることで攻撃の停止時間を伸ばす)
// (考え方)HPを増加させるアイテム等と同じ
// このアイテムを複数取得すると、それだけ攻撃停止時間も「加算」される。
public void AddStopTimer(float amount)
{
stopTimer += amount;
}
}
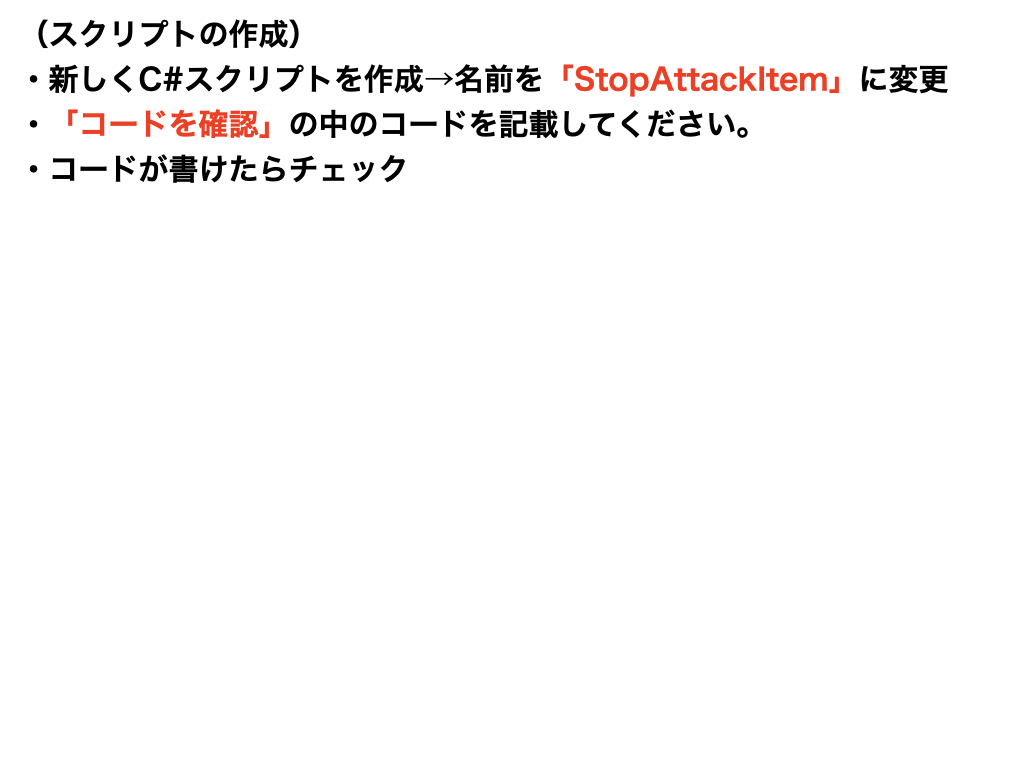
敵の攻撃を停止させるアイテム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class StopAttackItem : MonoBehaviour
{
private GameObject[] targets;
[SerializeField]
private AudioClip getSound;
[SerializeField]
private GameObject effectPrefab;
void Update()
{
// 「EnemyShotShell」オブジェクトに「EnemyShotShell」タグを設定してください(ポイント)
targets = GameObject.FindGameObjectsWithTag("EnemyShotShell");
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Player")
{
for (int i = 0; i < targets.Length; i++)
{
// 攻撃停止時間を3秒加算する(何秒間加算するかは自由)
targets[i].GetComponent<EnemyShotShell>().AddStopTimer(3.0f);
}
Destroy(gameObject);
AudioSource.PlayClipAtPoint(getSound, transform.position);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
}
}
}
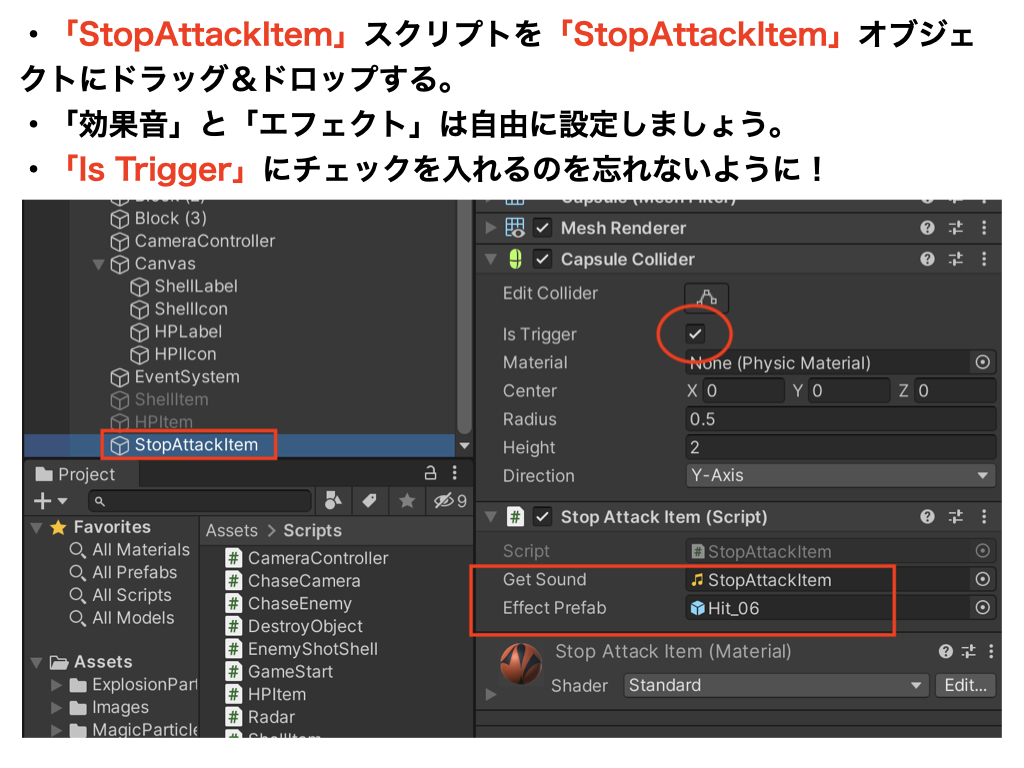
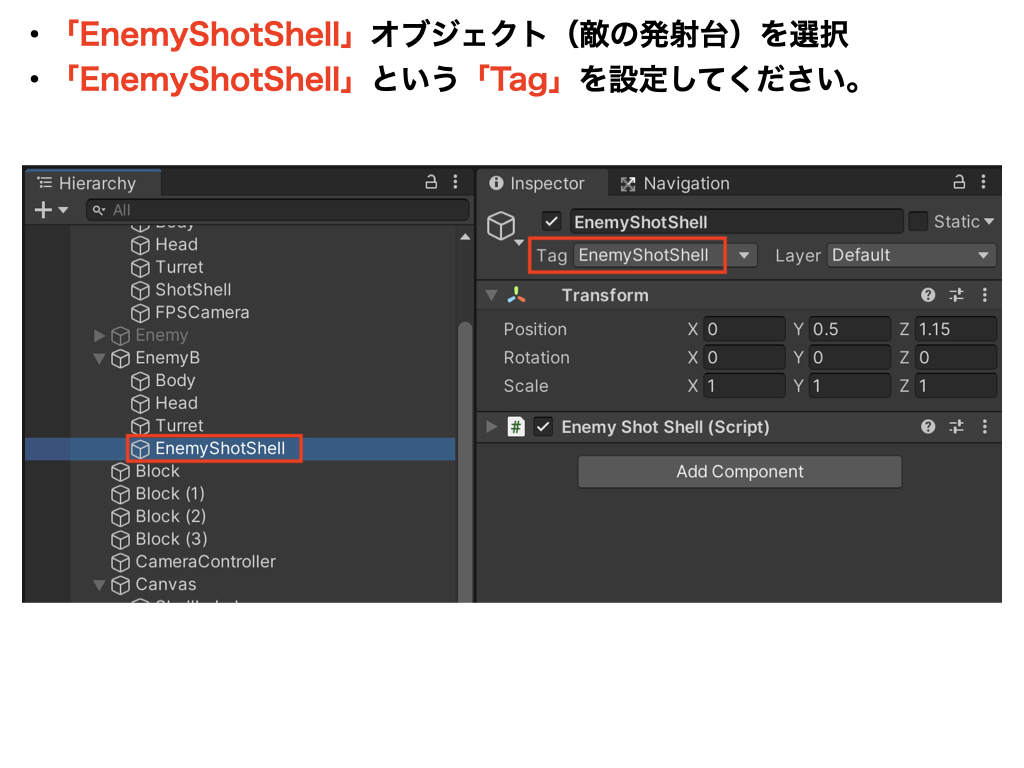
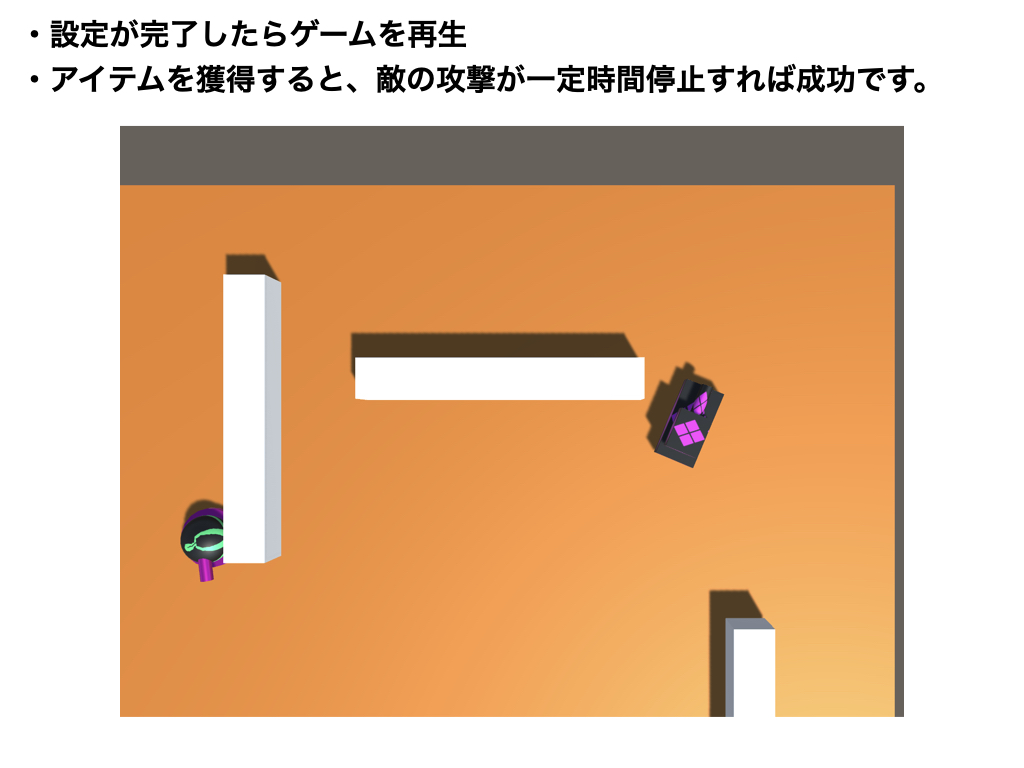
【2020版】BattleTank(基礎/全35回)
他のコースを見る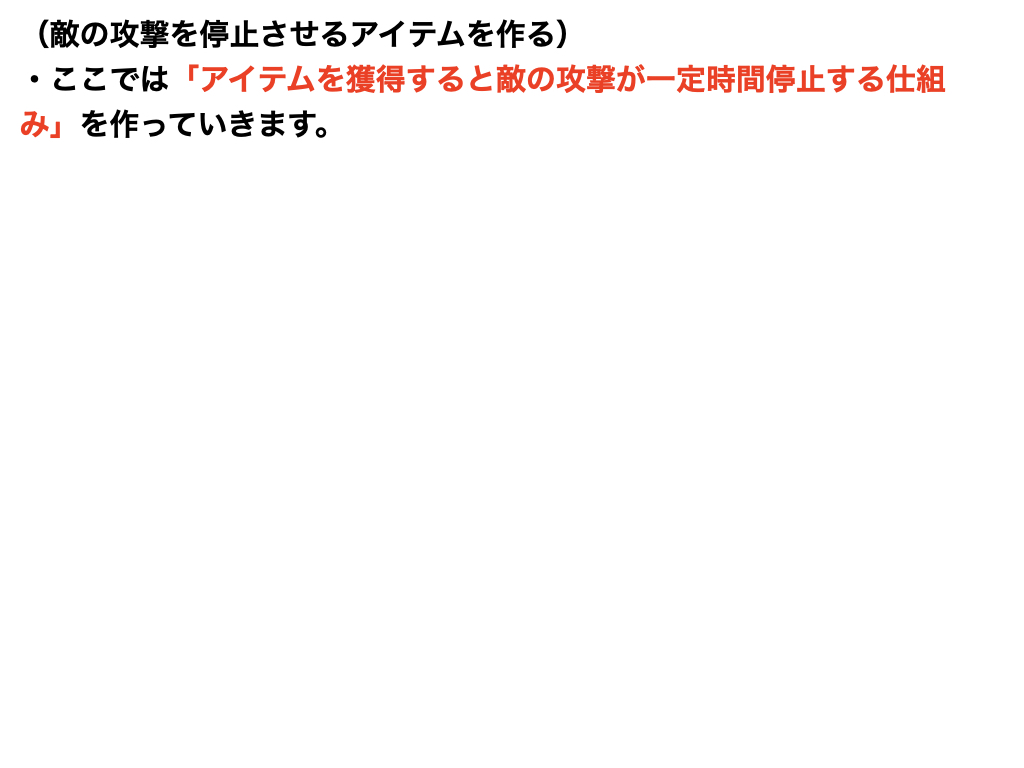
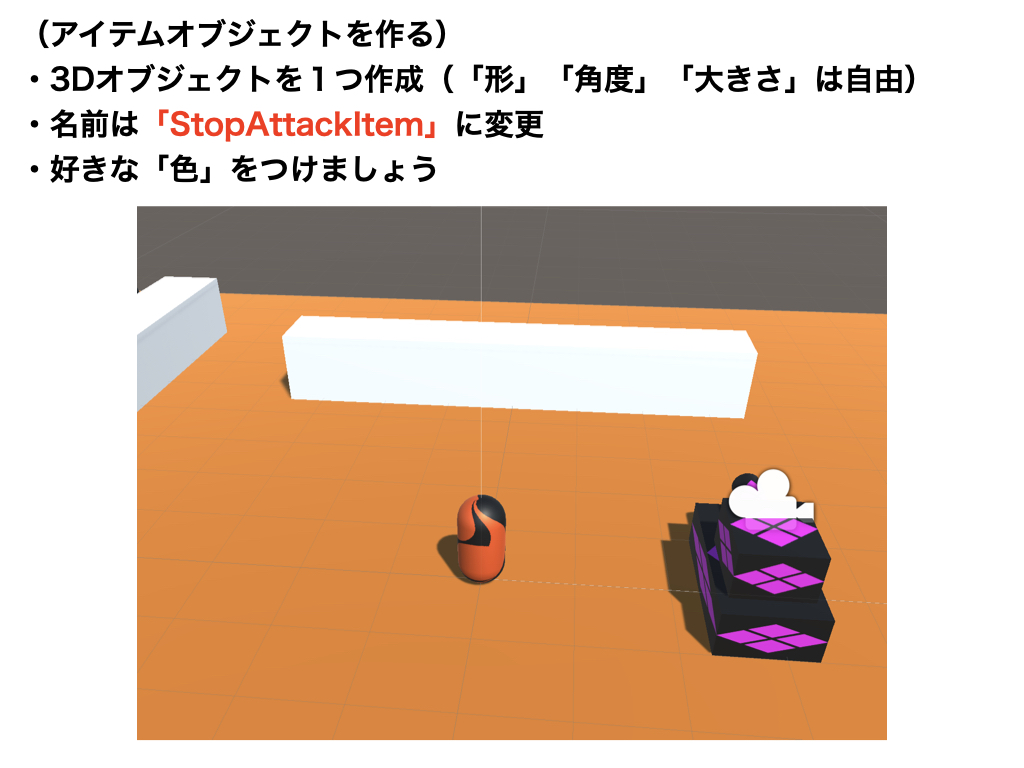
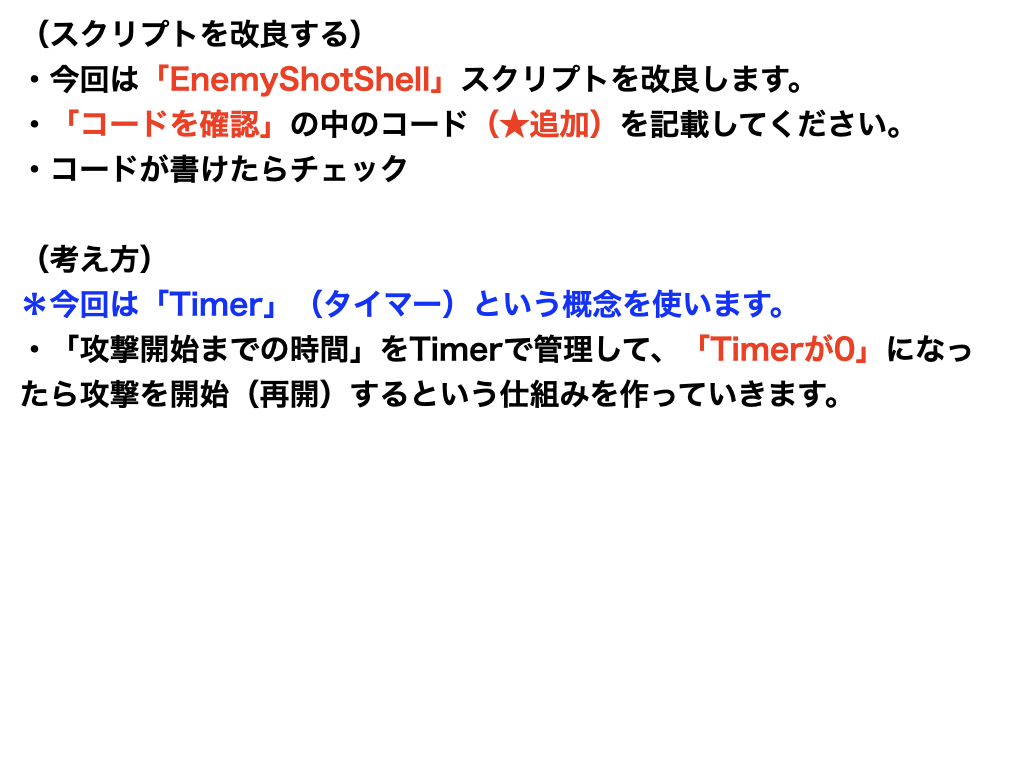
敵の攻撃を停止させる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyShotShell : MonoBehaviour
{
public float shotSpeed;
[SerializeField]
private GameObject enemyShellPrefab;
[SerializeField]
private AudioClip shotSound;
private int interval;
// ★追加
// 何秒間停止させるかは自由
public float stopTimer = 5.0f;
void Update()
{
interval += 1;
// ★追加
stopTimer -= Time.deltaTime;
// タイマーが0未満になったら、0で止める。
if (stopTimer < 0)
{
stopTimer = 0;
}
// ★追加
if (interval % 60 == 0 && stopTimer <= 0)
{
GameObject enemyShell = Instantiate(enemyShellPrefab, transform.position, Quaternion.identity);
Rigidbody enemyShellRb = enemyShell.GetComponent<Rigidbody>();
enemyShellRb.AddForce(transform.forward * shotSpeed);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
Destroy(enemyShell, 3.0f);
}
}
// ★追加
// 敵の攻撃をストップさせるメソッド(Timerの時間を増加させることで攻撃の停止時間を伸ばす)
// (考え方)HPを増加させるアイテム等と同じ
// このアイテムを複数取得すると、それだけ攻撃停止時間も「加算」される。
public void AddStopTimer(float amount)
{
stopTimer += amount;
}
}
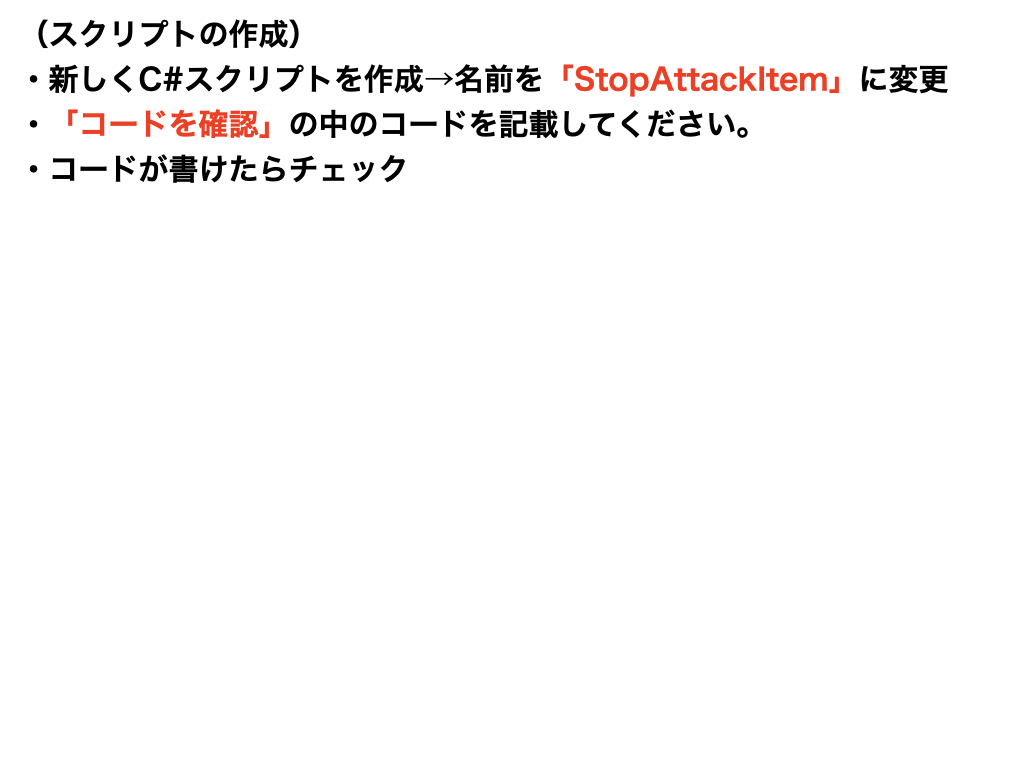
敵の攻撃を停止させるアイテム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class StopAttackItem : MonoBehaviour
{
private GameObject[] targets;
[SerializeField]
private AudioClip getSound;
[SerializeField]
private GameObject effectPrefab;
void Update()
{
// 「EnemyShotShell」オブジェクトに「EnemyShotShell」タグを設定してください(ポイント)
targets = GameObject.FindGameObjectsWithTag("EnemyShotShell");
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Player")
{
for (int i = 0; i < targets.Length; i++)
{
// 攻撃停止時間を3秒加算する(何秒間加算するかは自由)
targets[i].GetComponent<EnemyShotShell>().AddStopTimer(3.0f);
}
Destroy(gameObject);
AudioSource.PlayClipAtPoint(getSound, transform.position);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.5f);
}
}
}
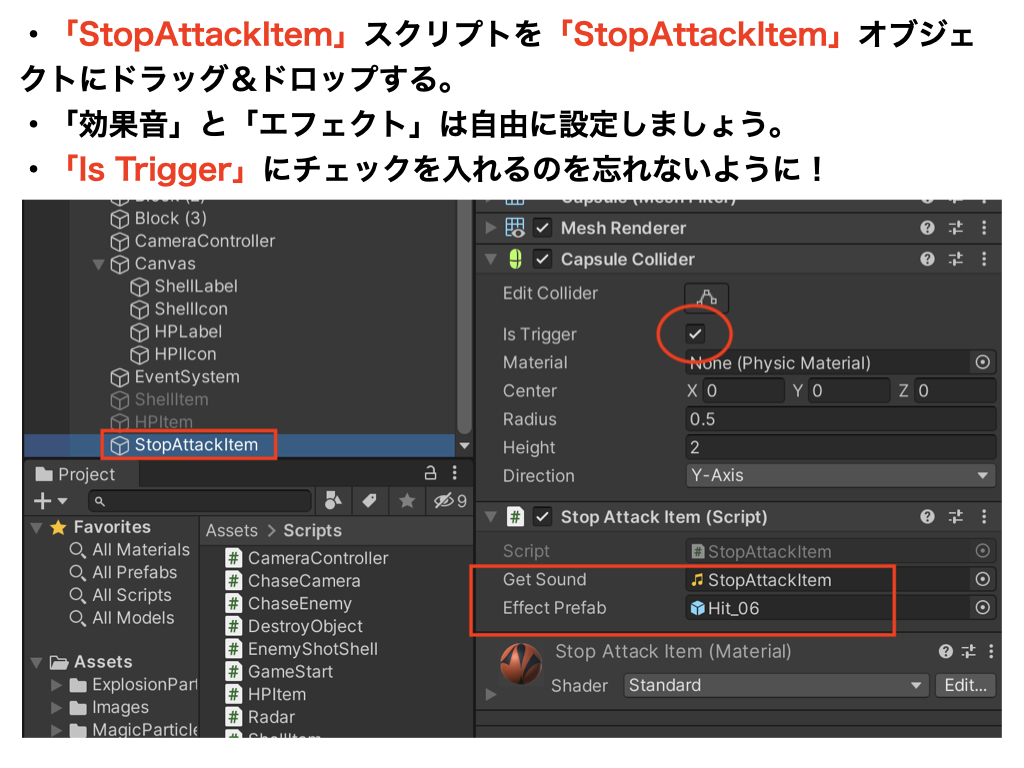
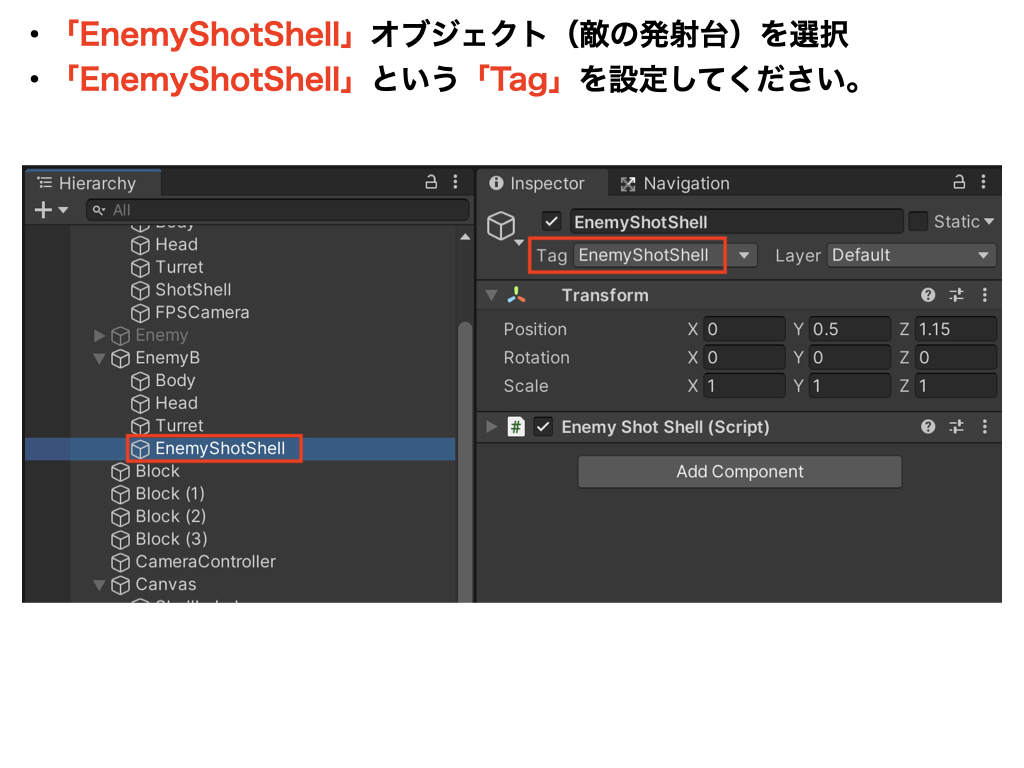
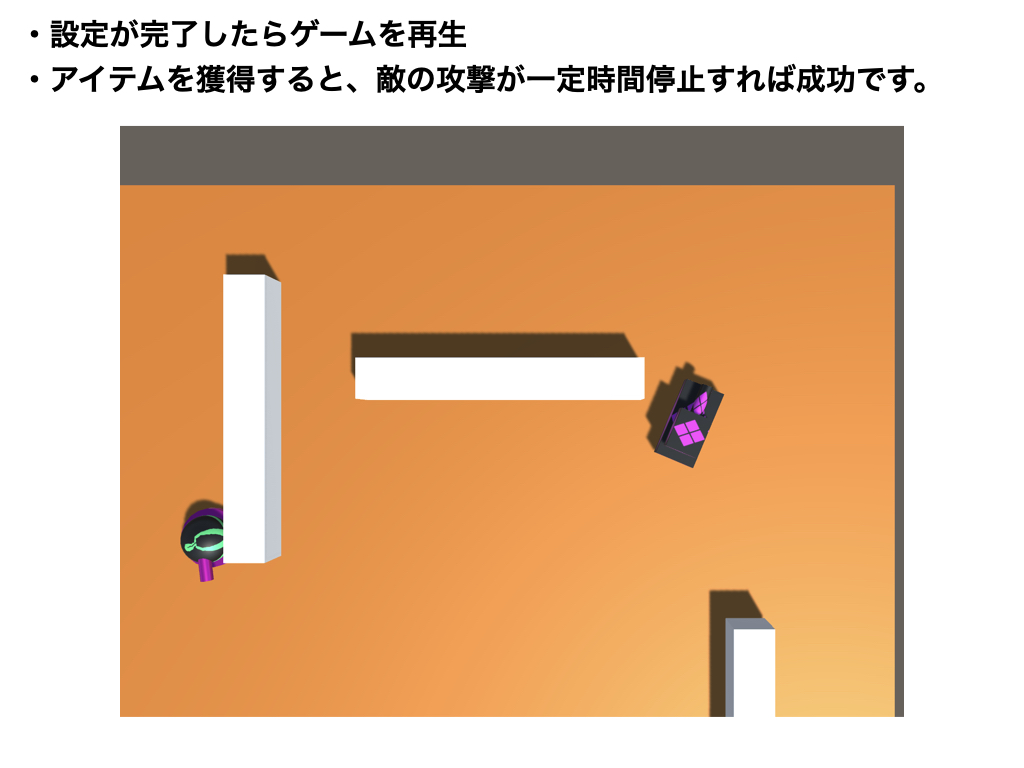
敵の攻撃を停止させるアイテムの作成