残弾数を回復させるアイテムの作成
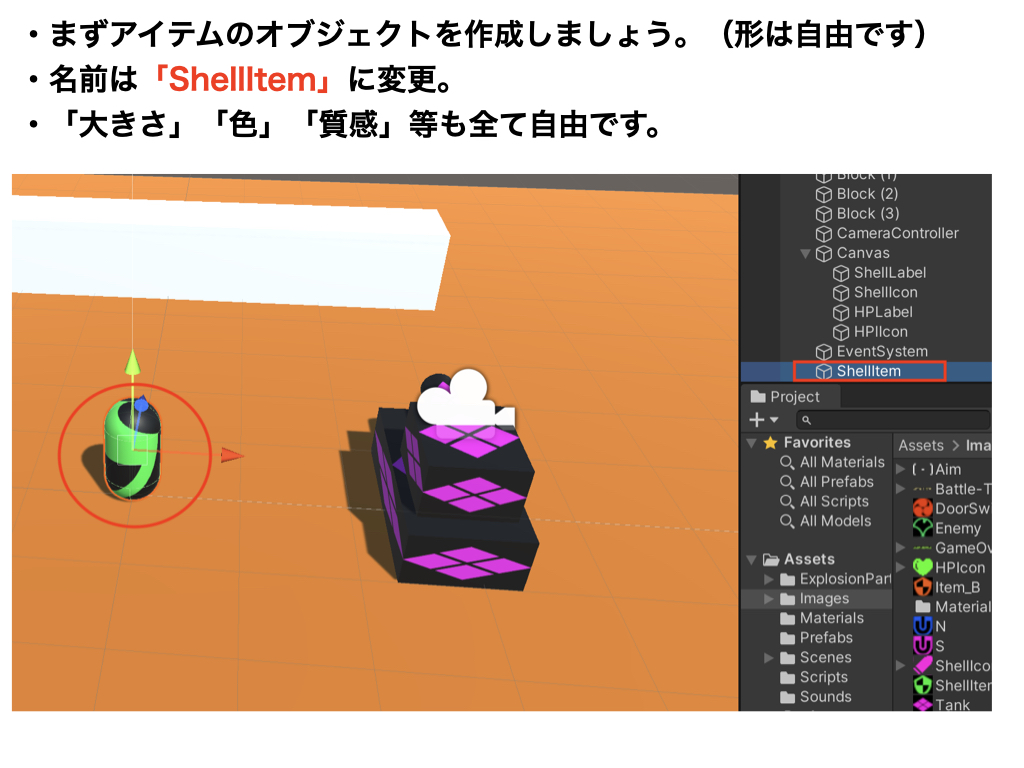
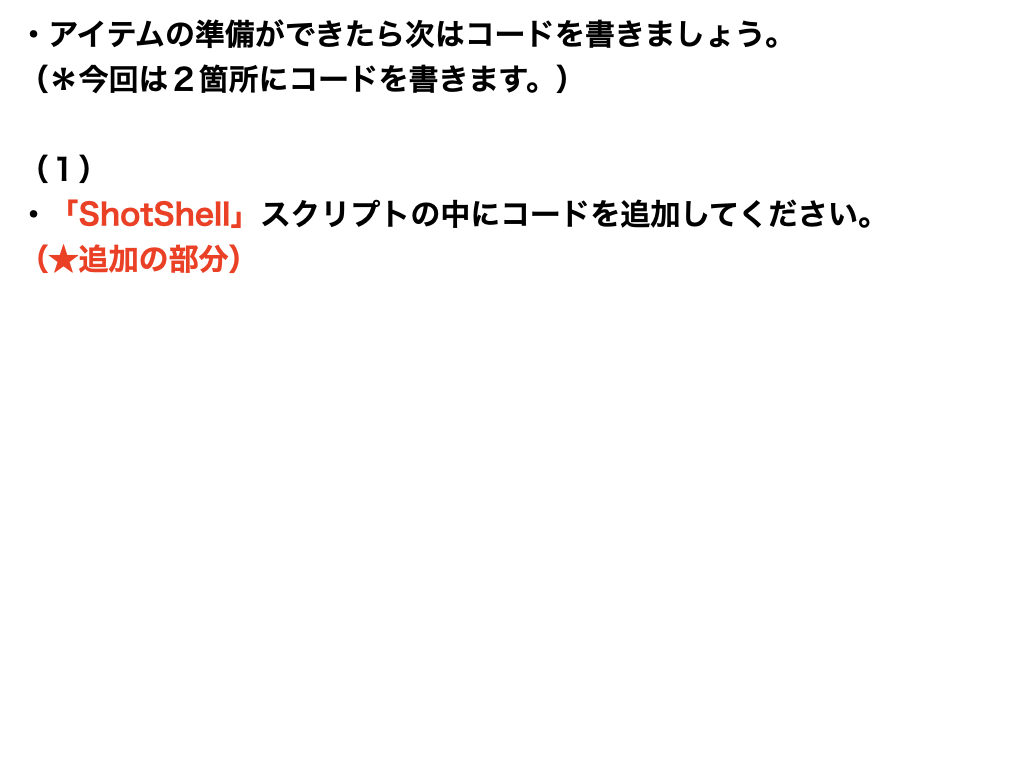
残弾数を増加させるメソッド
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ShotShell : MonoBehaviour
{
public float shotSpeed;
[SerializeField]
private GameObject shellPrefab = null;
[SerializeField]
private AudioClip shotSound = null;
private float timeBetweenShot = 0.75f;
private float timer;
public int shotCount;
[SerializeField]
private Text shellLabel;
// ★追加
// 残弾数の最大値を設定する(最大値は自由)
public int shotMaxCount = 20;
void Start()
{
// ★追加
shotCount = shotMaxCount;
shellLabel.text = "砲弾:" + shotCount;
}
void Update()
{
timer += Time.deltaTime;
if (Input.GetKeyDown(KeyCode.Space) && timer > timeBetweenShot && shotCount > 0)
{
shotCount -= 1;
shellLabel.text = "砲弾:" + shotCount;
timer = 0.0f;
GameObject shell = Instantiate(shellPrefab, transform.position, Quaternion.identity);
Rigidbody shellRb = shell.GetComponent<Rigidbody>();
shellRb.AddForce(transform.forward * shotSpeed);
Destroy(shell, 3.0f);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
}
}
// ★追加
// 残弾数を増加させるメソッド(関数・命令ブロック)
// 外部からこのメソッドを呼び出せるように「public」をつける(重要ポイント)
// この「AddShellメソッド」を「ShellItem」スクリプトから呼び出す。
public void AddShell(int amount)
{
// shotCountをamount分だけ回復させる
shotCount += amount;
// ただし、残弾数が最大値を超えないようする。(最大値は自由に設定)
if (shotCount > shotMaxCount)
{
shotCount = shotMaxCount;
}
// 回復をUIに反映させる。
shellLabel.text = "砲弾:" + shotCount;
}
}
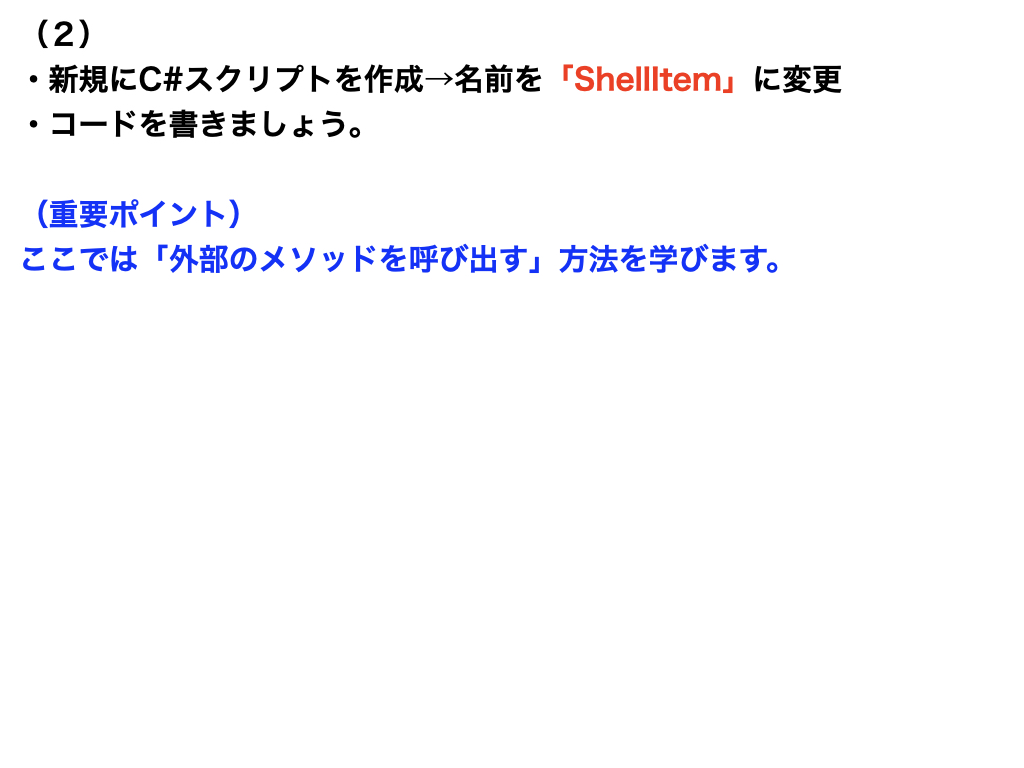
残弾数を回復させるアイテム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShellItem : MonoBehaviour
{
[SerializeField]
private AudioClip getSound;
[SerializeField]
private GameObject effectPrefab;
private ShotShell ss;
private int reward = 5; // 弾数をいくつ回復させるかは自由に決定
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Player")
{
// (重要ポイント)
// Find()メソッドは、「名前」でオブジェクトを探し特定します。
// 「ShotShell」オブジェクトを探し出して、それに付いている「ShotShell」スクリプト(component)のデータを取得。
// 取得したデータを「ss」の箱の中に入れる。
ss = GameObject.Find("ShotShell").GetComponent<ShotShell>();
// ShotShellスクリプトの中に記載されている「AddShellメソッド」を呼び出す。
// rewardで設定した数値分だけ弾数が回復する。
ss.AddShell(reward);
// アイテムを画面から削除する。
Destroy(gameObject);
// アイテムゲット音を出す。
AudioSource.PlayClipAtPoint(getSound, transform.position);
// アイテムゲット時にエフェクトを発生させる。
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
// エフェクトを0.5秒後に消す。
Destroy(effect, 0.5f);
}
}
}
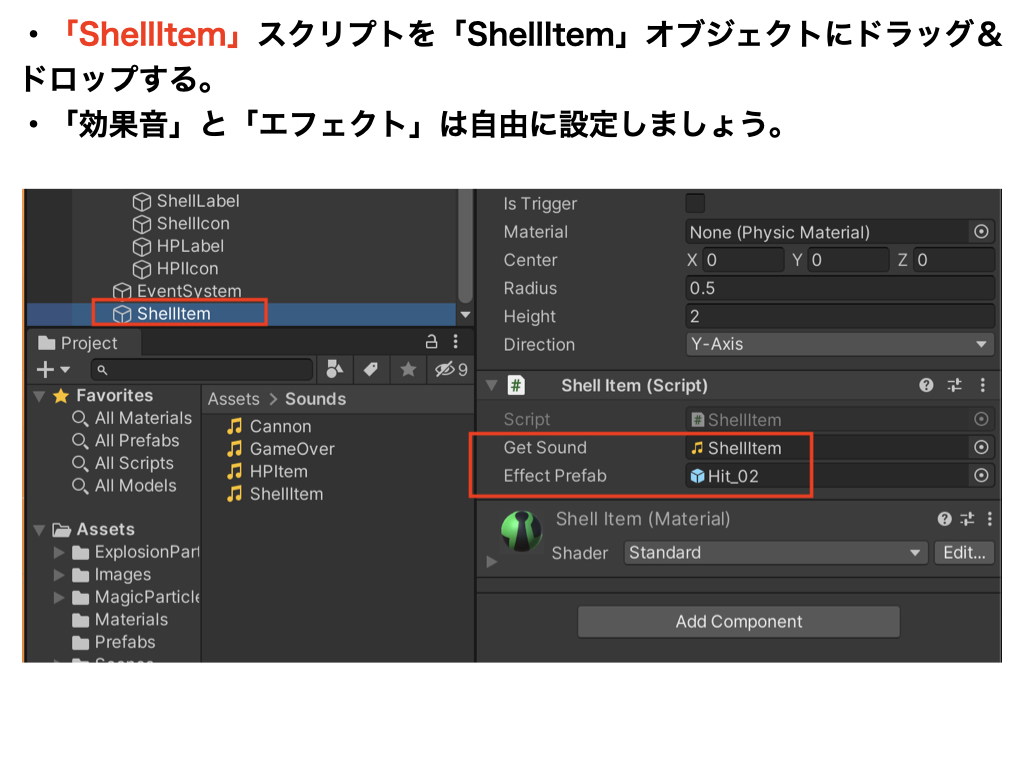
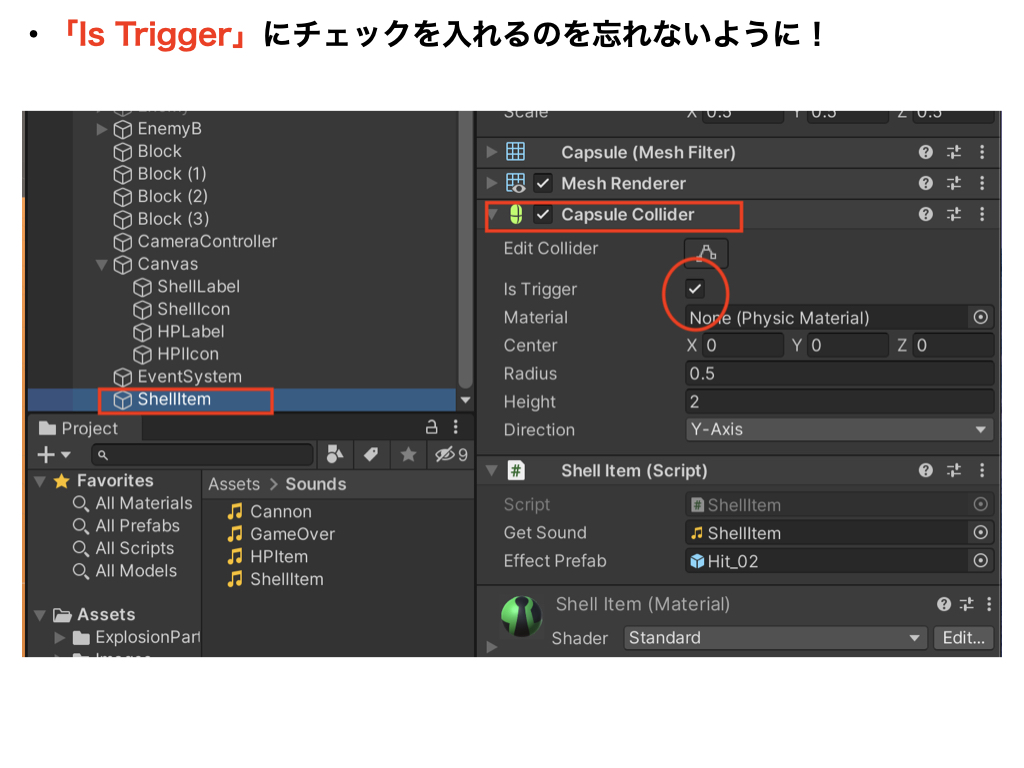
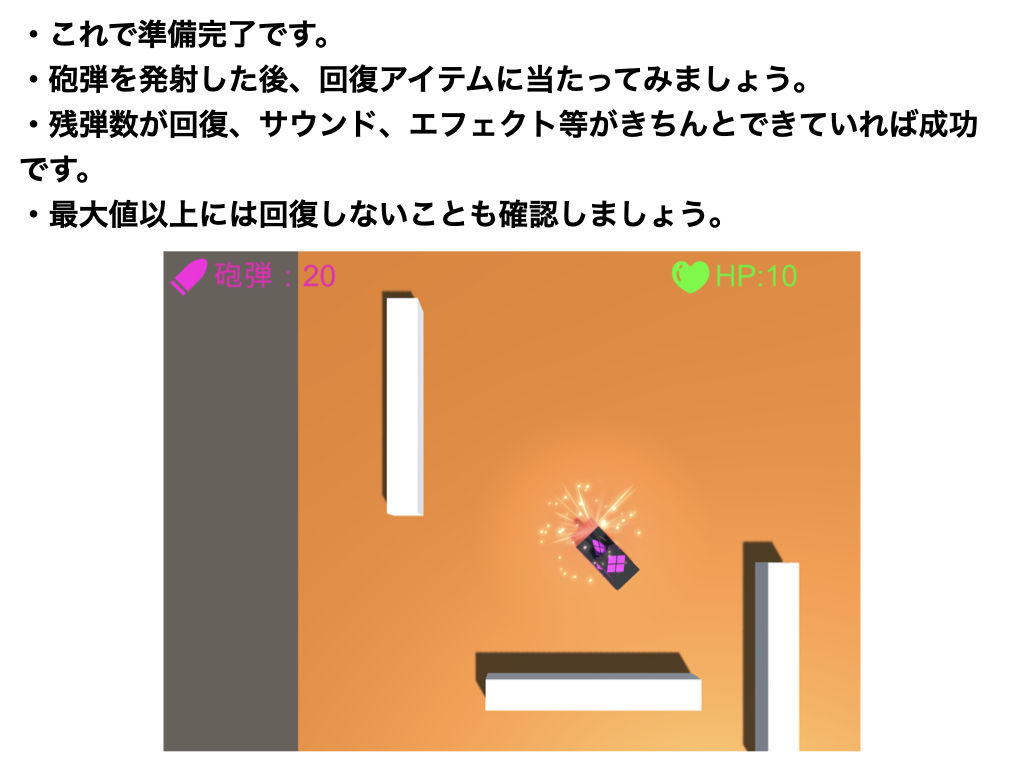
【2020版】BattleTank(基礎/全35回)
他のコースを見る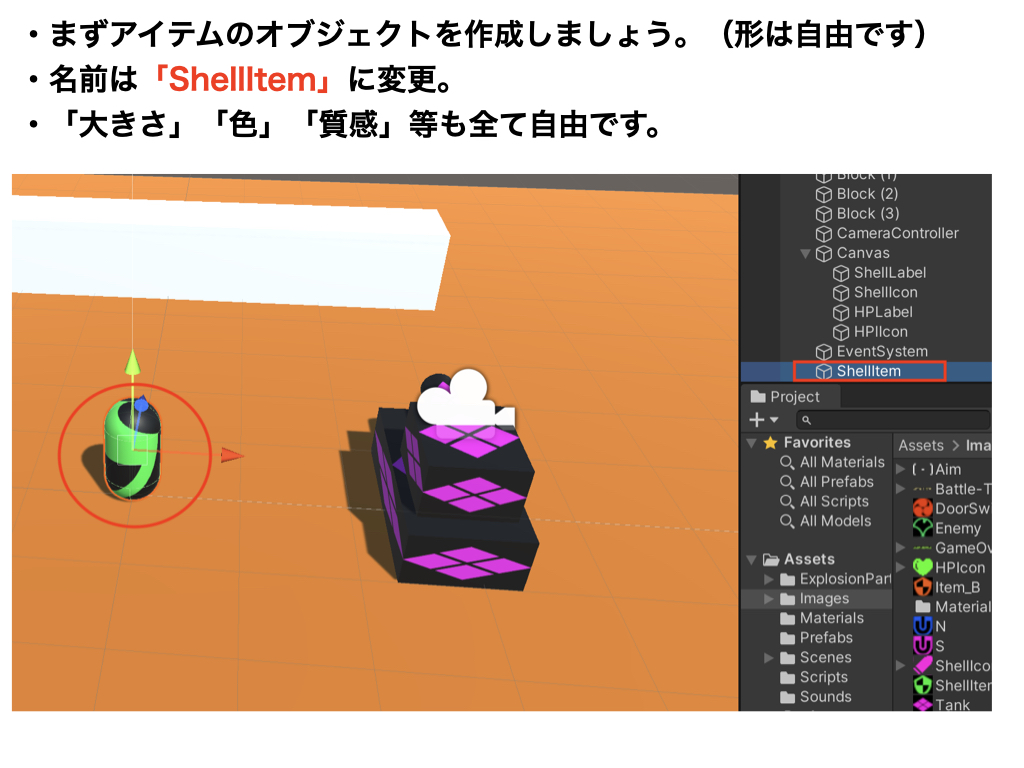
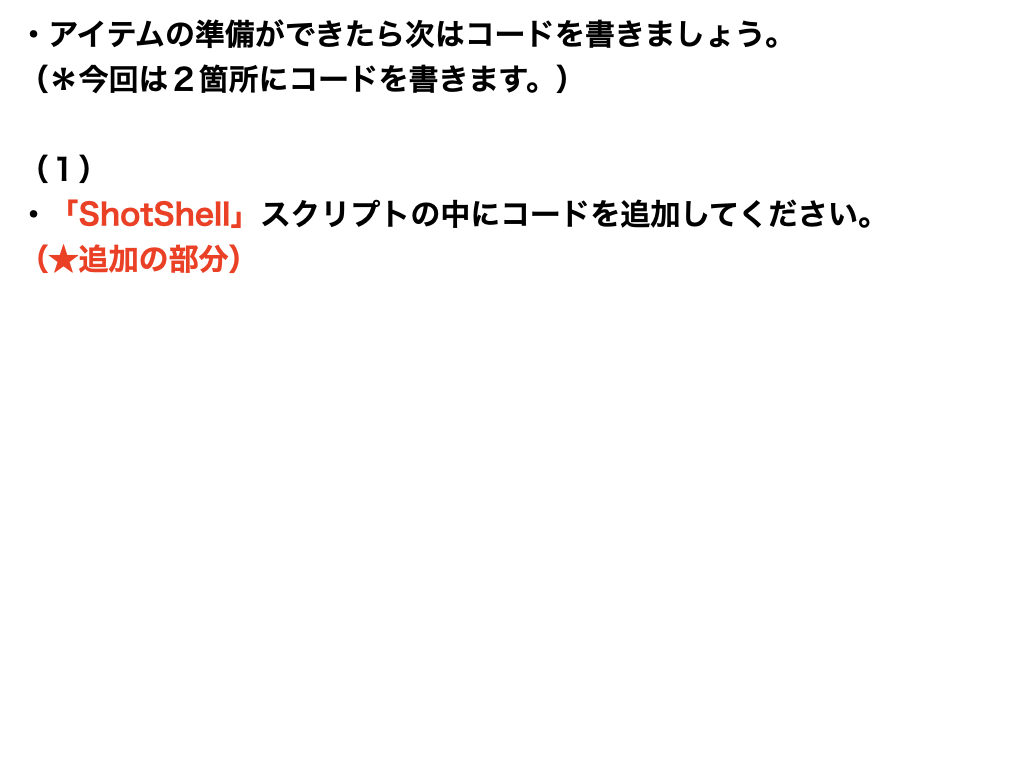
残弾数を増加させるメソッド
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ShotShell : MonoBehaviour
{
public float shotSpeed;
[SerializeField]
private GameObject shellPrefab = null;
[SerializeField]
private AudioClip shotSound = null;
private float timeBetweenShot = 0.75f;
private float timer;
public int shotCount;
[SerializeField]
private Text shellLabel;
// ★追加
// 残弾数の最大値を設定する(最大値は自由)
public int shotMaxCount = 20;
void Start()
{
// ★追加
shotCount = shotMaxCount;
shellLabel.text = "砲弾:" + shotCount;
}
void Update()
{
timer += Time.deltaTime;
if (Input.GetKeyDown(KeyCode.Space) && timer > timeBetweenShot && shotCount > 0)
{
shotCount -= 1;
shellLabel.text = "砲弾:" + shotCount;
timer = 0.0f;
GameObject shell = Instantiate(shellPrefab, transform.position, Quaternion.identity);
Rigidbody shellRb = shell.GetComponent<Rigidbody>();
shellRb.AddForce(transform.forward * shotSpeed);
Destroy(shell, 3.0f);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
}
}
// ★追加
// 残弾数を増加させるメソッド(関数・命令ブロック)
// 外部からこのメソッドを呼び出せるように「public」をつける(重要ポイント)
// この「AddShellメソッド」を「ShellItem」スクリプトから呼び出す。
public void AddShell(int amount)
{
// shotCountをamount分だけ回復させる
shotCount += amount;
// ただし、残弾数が最大値を超えないようする。(最大値は自由に設定)
if (shotCount > shotMaxCount)
{
shotCount = shotMaxCount;
}
// 回復をUIに反映させる。
shellLabel.text = "砲弾:" + shotCount;
}
}
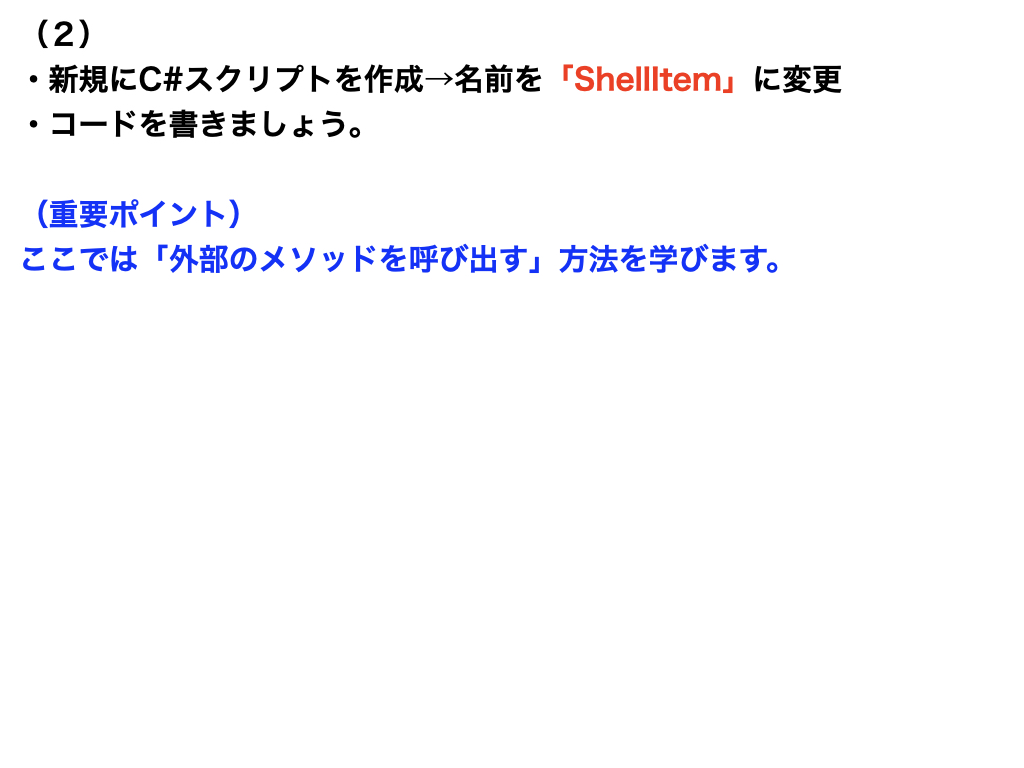
残弾数を回復させるアイテム
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShellItem : MonoBehaviour
{
[SerializeField]
private AudioClip getSound;
[SerializeField]
private GameObject effectPrefab;
private ShotShell ss;
private int reward = 5; // 弾数をいくつ回復させるかは自由に決定
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Player")
{
// (重要ポイント)
// Find()メソッドは、「名前」でオブジェクトを探し特定します。
// 「ShotShell」オブジェクトを探し出して、それに付いている「ShotShell」スクリプト(component)のデータを取得。
// 取得したデータを「ss」の箱の中に入れる。
ss = GameObject.Find("ShotShell").GetComponent<ShotShell>();
// ShotShellスクリプトの中に記載されている「AddShellメソッド」を呼び出す。
// rewardで設定した数値分だけ弾数が回復する。
ss.AddShell(reward);
// アイテムを画面から削除する。
Destroy(gameObject);
// アイテムゲット音を出す。
AudioSource.PlayClipAtPoint(getSound, transform.position);
// アイテムゲット時にエフェクトを発生させる。
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
// エフェクトを0.5秒後に消す。
Destroy(effect, 0.5f);
}
}
}
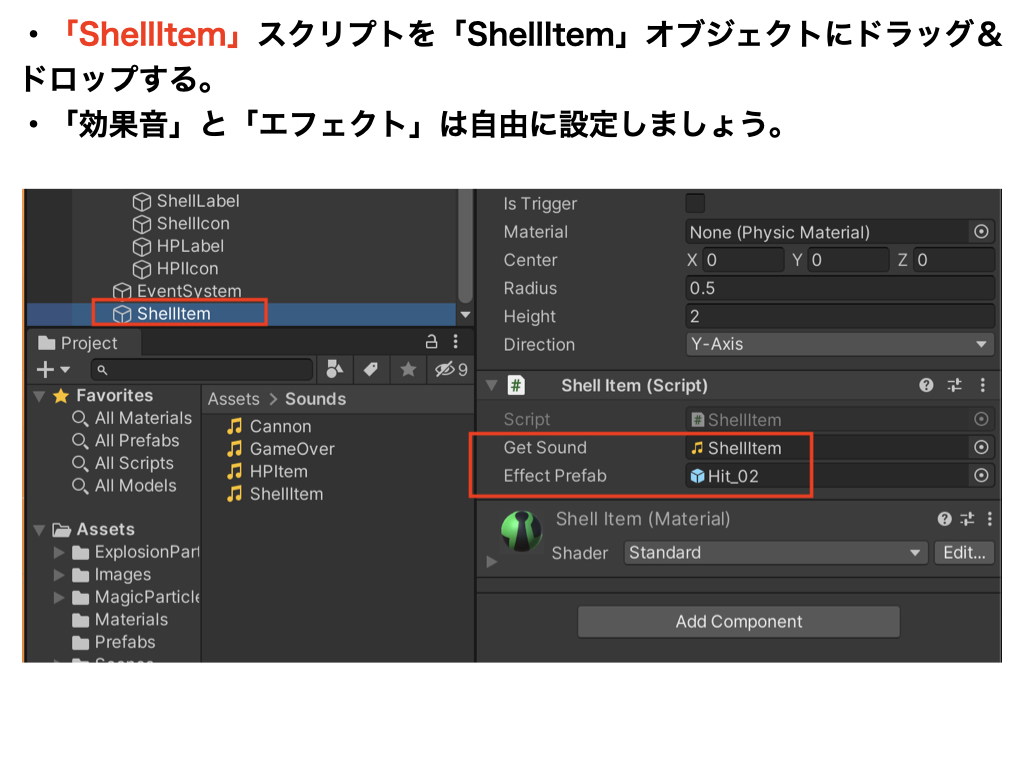
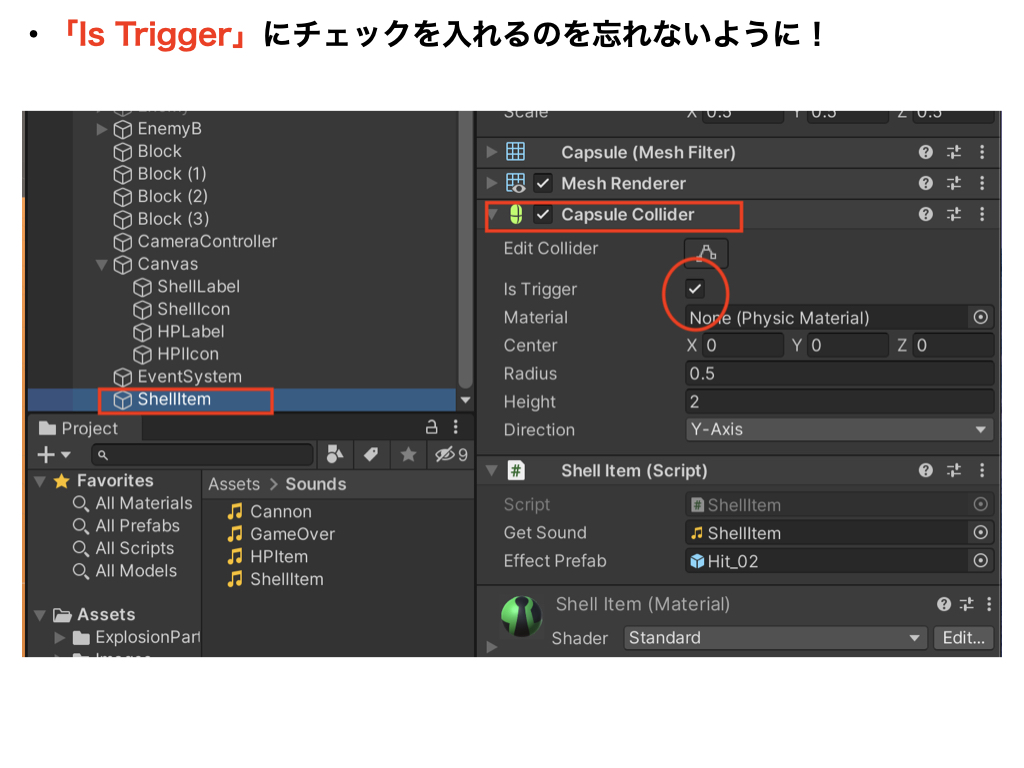
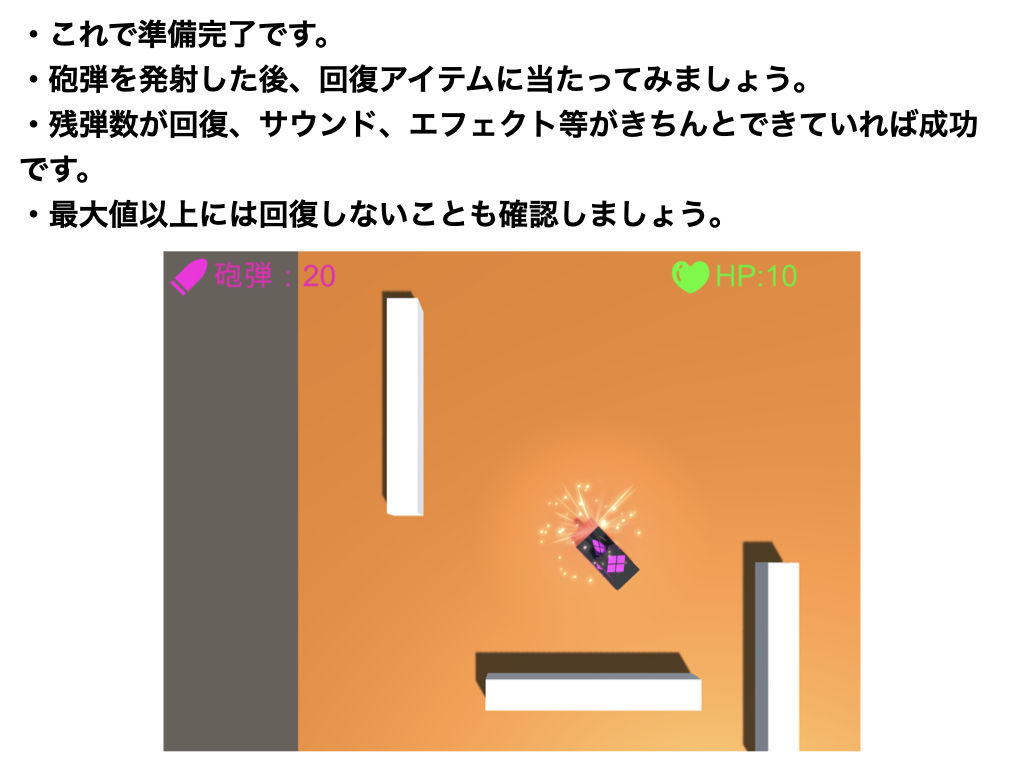
残弾数を回復させるアイテムの作成