タイムとスコアを表示する
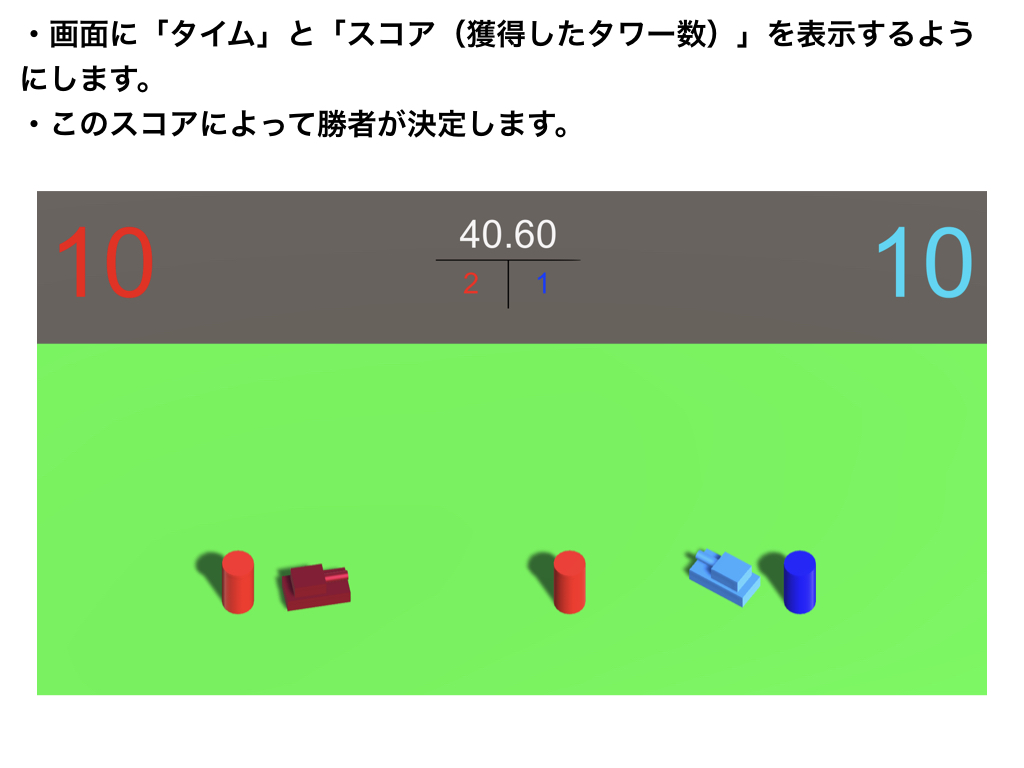
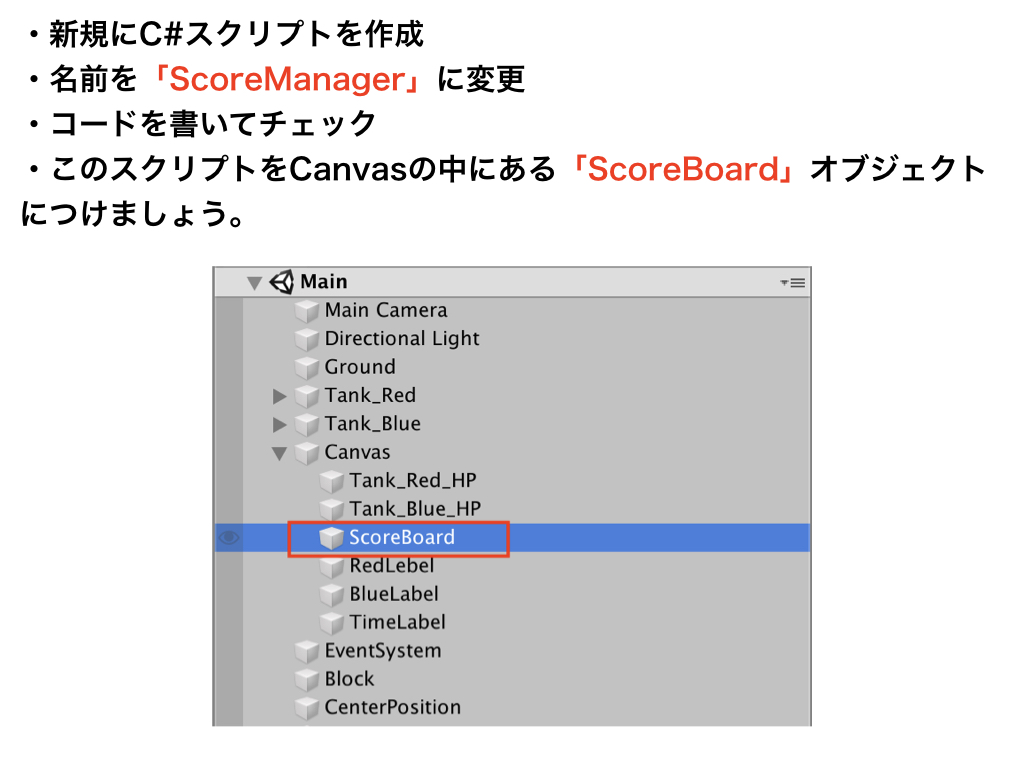
タイムとスコアを表示する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class ScoreManager : MonoBehaviour
{
public Text timeLabel;
public Text redLabel;
public Text blueLabel;
private float timeCount = 10f;
private int redCount;
private int blueCount;
void Start()
{
// 時間は小数点2位まで表示する。
timeLabel.text = "" + timeCount.ToString("n2");
redLabel.text = "" + redCount;
blueLabel.text = "" + blueCount;
}
void Update()
{
timeCount -= Time.deltaTime;
timeLabel.text = "" + timeCount.ToString("n2");
// Redのタグが付いているオブジェクトの数をかぞえる。
redCount = GameObject.FindGameObjectsWithTag("Red").Length;
redLabel.text = "" + redCount;
// Blueのタグが付いているオブジェクトの数をかぞえる。
blueCount = GameObject.FindGameObjectsWithTag("Blue").Length;
blueLabel.text = "" + blueCount;
if(timeCount < 0)
{
timeCount = 0;
timeLabel.text = "" + timeCount.ToString("n2");
if (redCount > blueCount)
{
SceneManager.LoadScene("RedWin");
}
else if(redCount < blueCount)
{
SceneManager.LoadScene("BlueWin");
}
else
{
SceneManager.LoadScene("Draw");
}
}
}
}
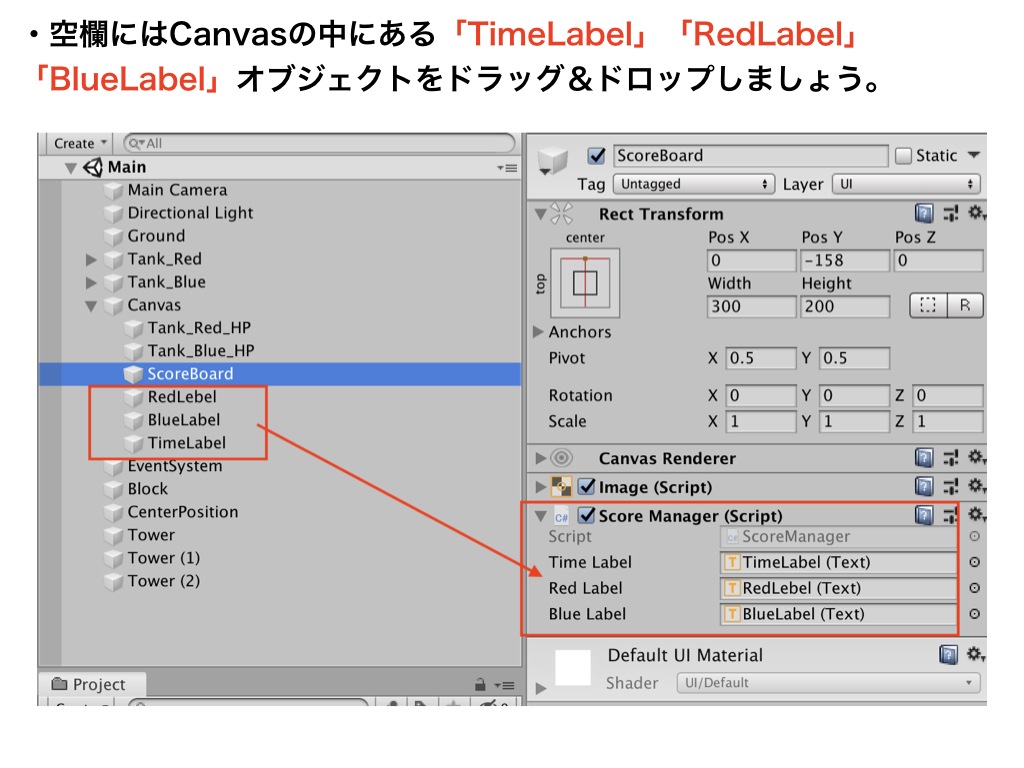
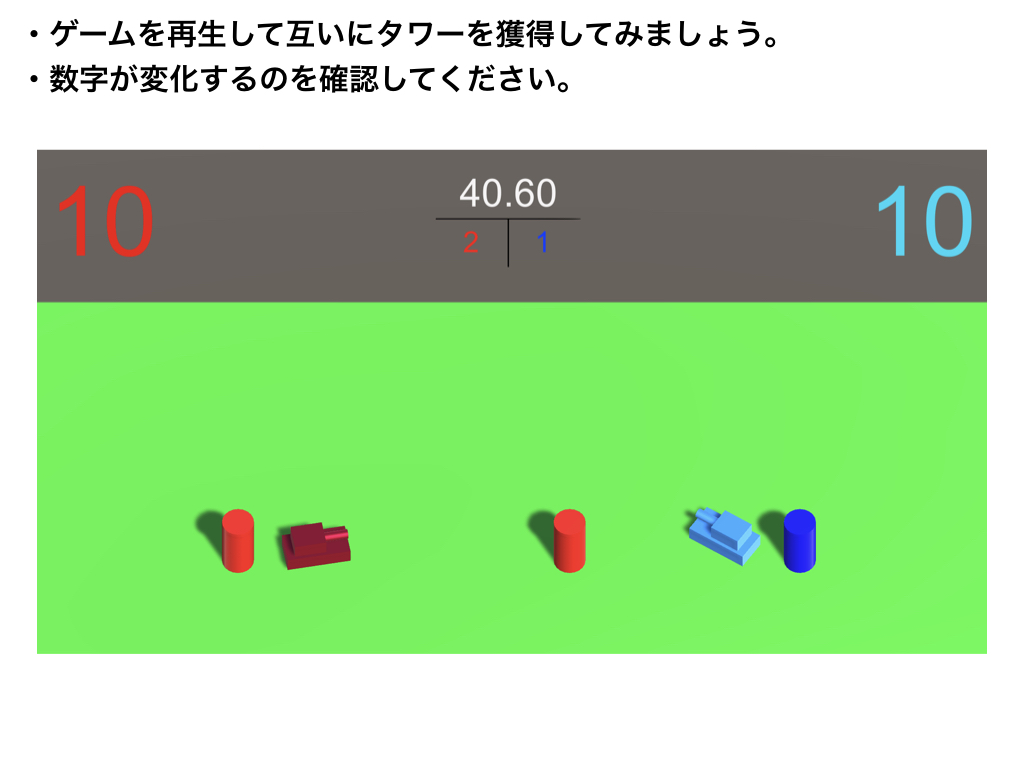
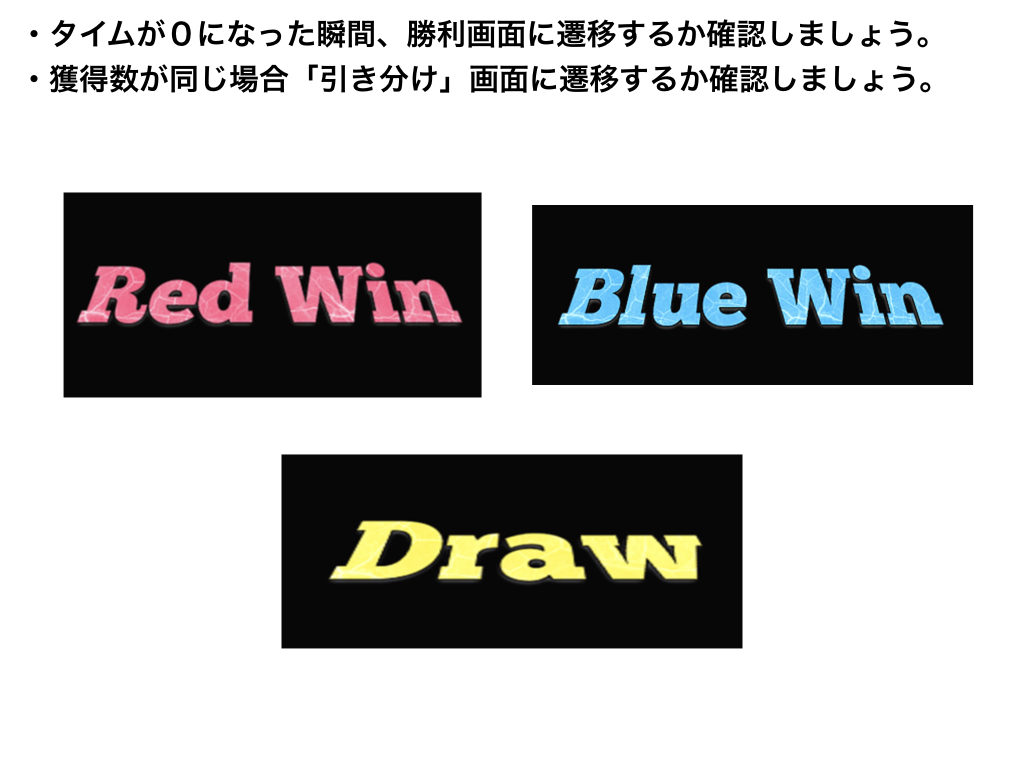
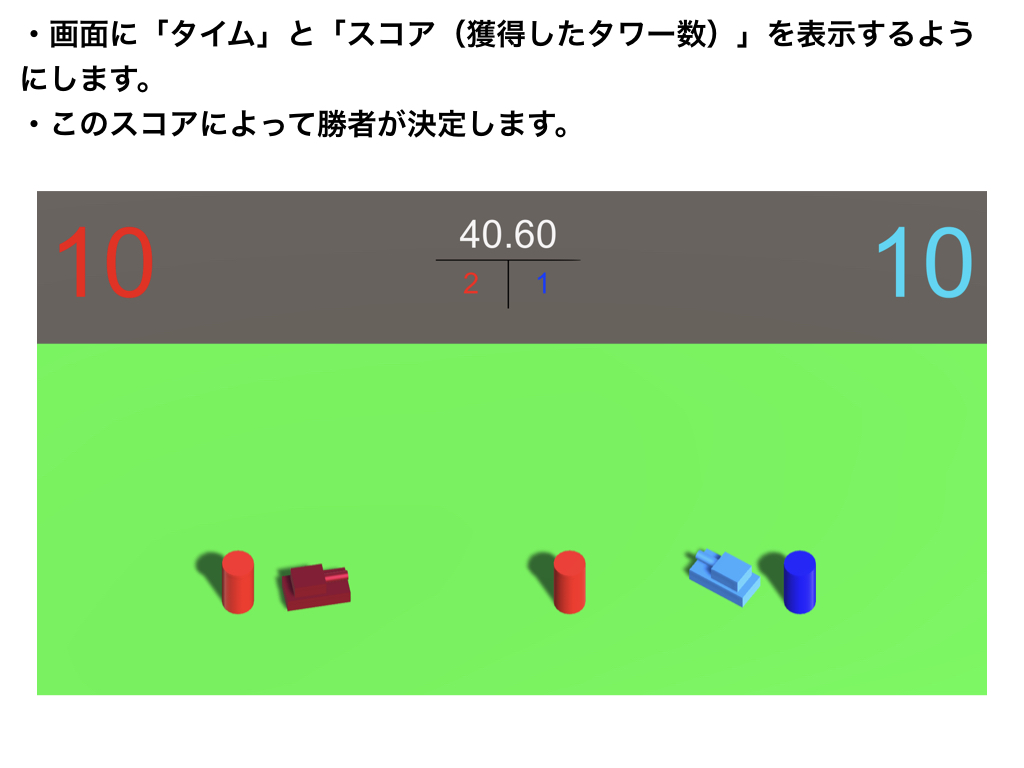
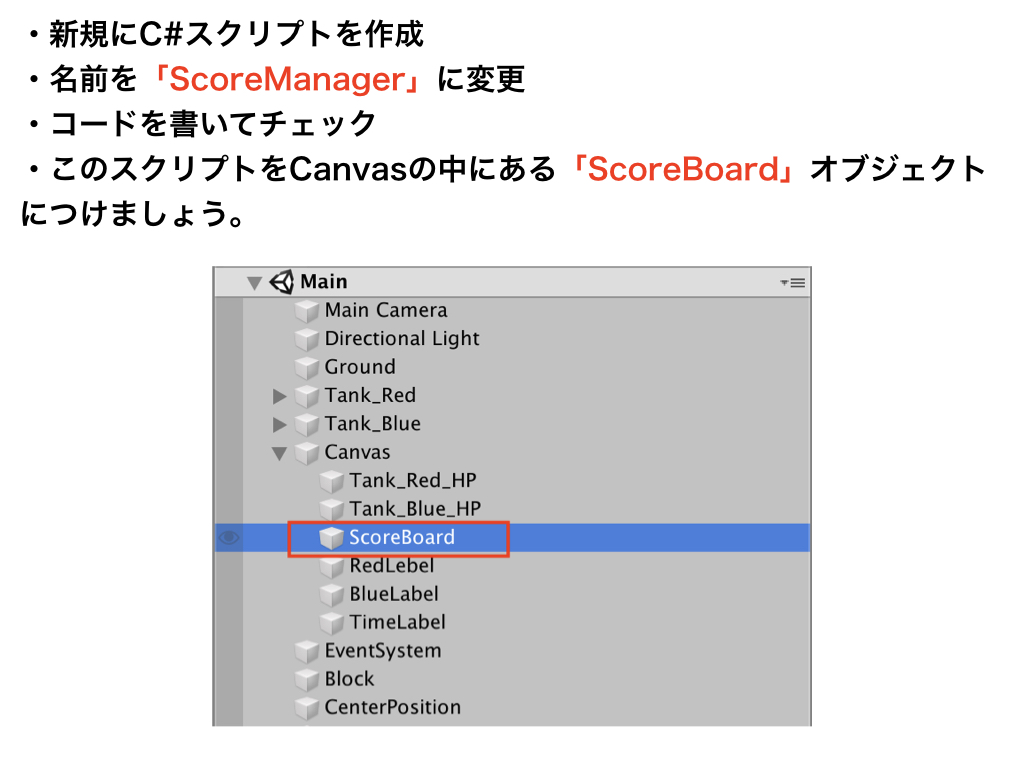
タイムとスコアを表示する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class ScoreManager : MonoBehaviour
{
public Text timeLabel;
public Text redLabel;
public Text blueLabel;
private float timeCount = 10f;
private int redCount;
private int blueCount;
void Start()
{
// 時間は小数点2位まで表示する。
timeLabel.text = "" + timeCount.ToString("n2");
redLabel.text = "" + redCount;
blueLabel.text = "" + blueCount;
}
void Update()
{
timeCount -= Time.deltaTime;
timeLabel.text = "" + timeCount.ToString("n2");
// Redのタグが付いているオブジェクトの数をかぞえる。
redCount = GameObject.FindGameObjectsWithTag("Red").Length;
redLabel.text = "" + redCount;
// Blueのタグが付いているオブジェクトの数をかぞえる。
blueCount = GameObject.FindGameObjectsWithTag("Blue").Length;
blueLabel.text = "" + blueCount;
if(timeCount < 0)
{
timeCount = 0;
timeLabel.text = "" + timeCount.ToString("n2");
if (redCount > blueCount)
{
SceneManager.LoadScene("RedWin");
}
else if(redCount < blueCount)
{
SceneManager.LoadScene("BlueWin");
}
else
{
SceneManager.LoadScene("Draw");
}
}
}
}
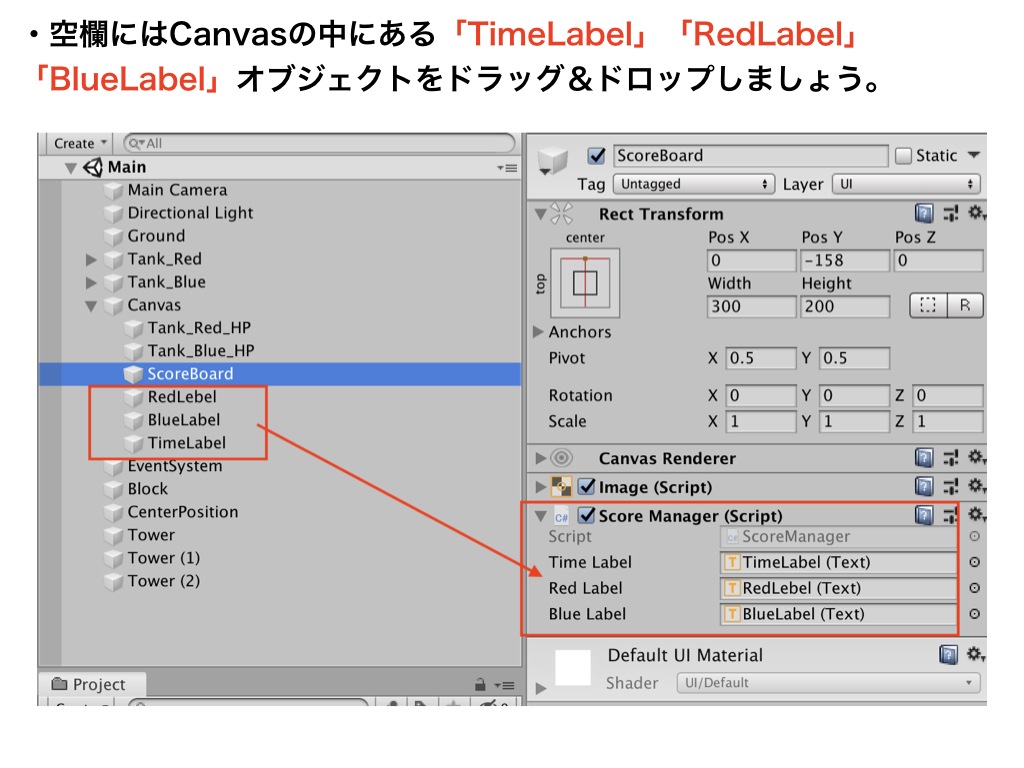
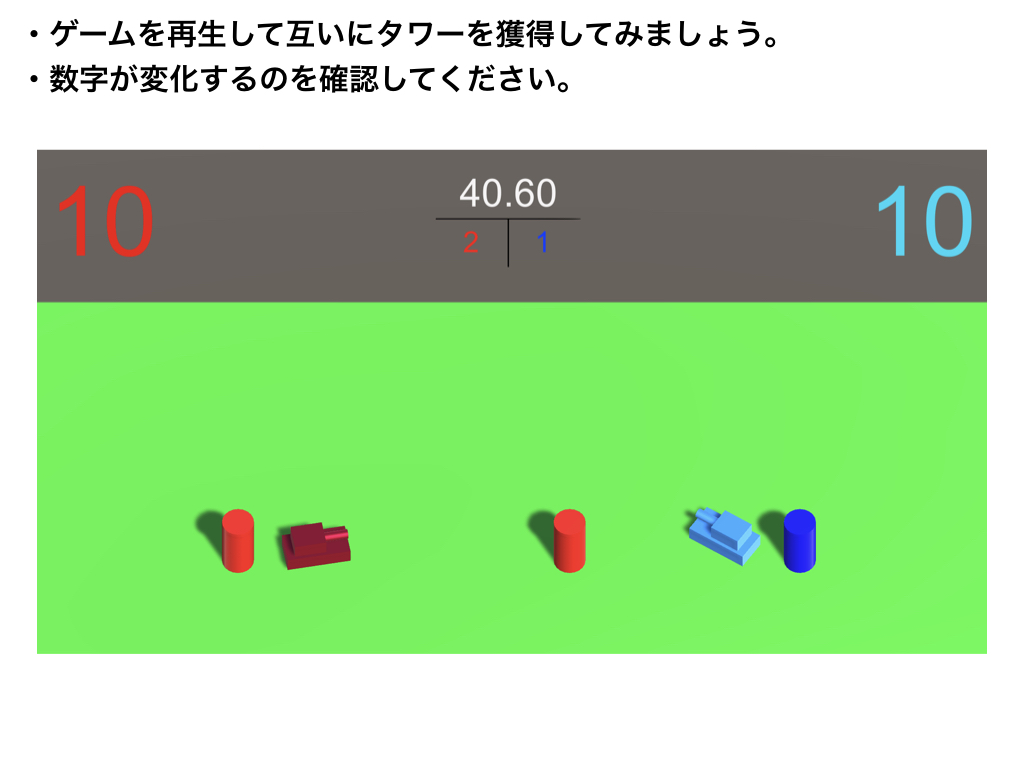
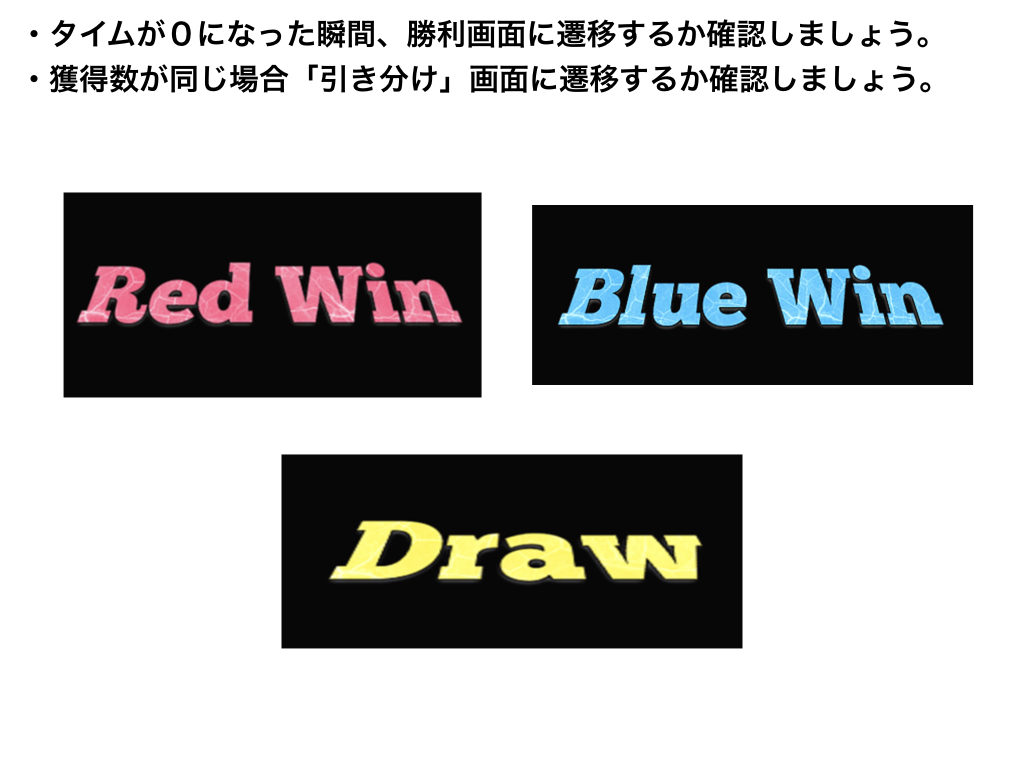
タイムとスコアを表示する