個別に砲弾を発射できるようにする
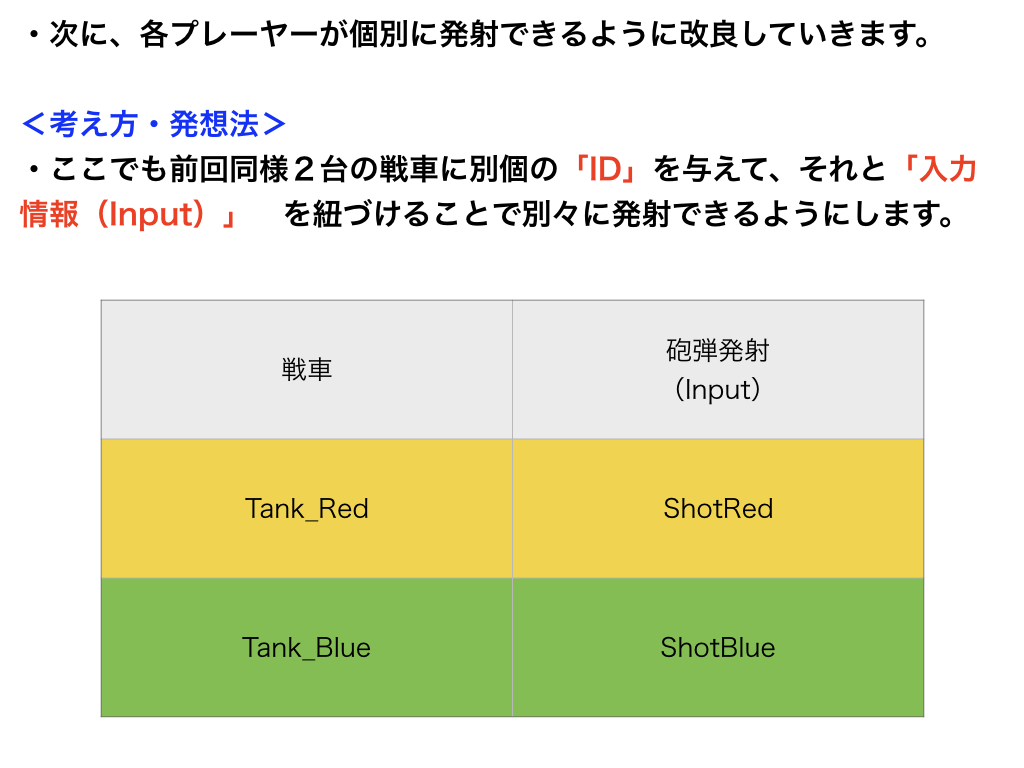
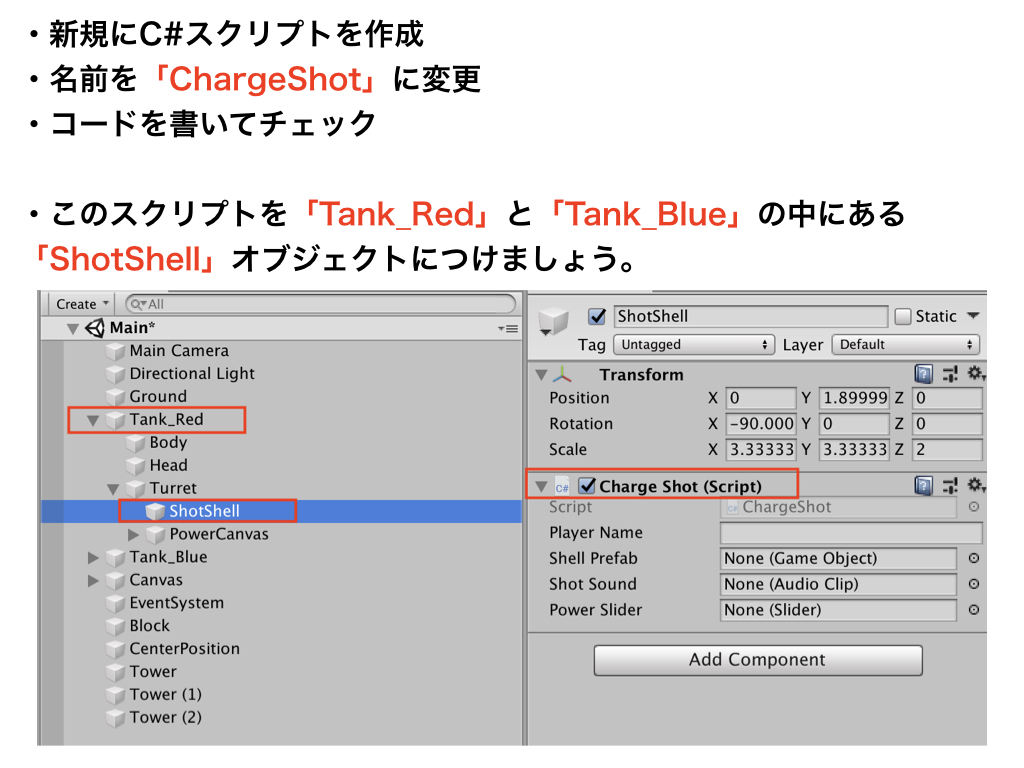
個別に砲弾を発射する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ChargeShot : MonoBehaviour
{
public string playerName;
public GameObject shellPrefab;
public AudioClip shotSound;
private float shotSpeed = 50f;
private int power = 0;
private int maxPower = 20;
public Slider powerSlider;
private void Start()
{
powerSlider.maxValue = maxPower;
powerSlider.value = power;
}
void Update()
{
if(Input.GetButton("Shot" + playerName))
{
// ボタンを押している間、パワーがチャージされる。
power += 1;
powerSlider.value = power;
// チャージできるパワーに上限を設定する。
if(power > maxPower)
{
power = maxPower;
}
}
if(Input.GetButtonUp("Shot" + playerName))
{
// ボタンを離した瞬間に砲弾が発射する。
GameObject shell = Instantiate(shellPrefab, transform.position, Quaternion.identity);
Rigidbody shellRb = shell.GetComponent<Rigidbody>();
shellRb.AddForce(transform.forward * shotSpeed * power);
Destroy(shell, 15.0f);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
// 打ち終わったらパワーをリセットする。
power = 0;
powerSlider.value = power;
}
}
}
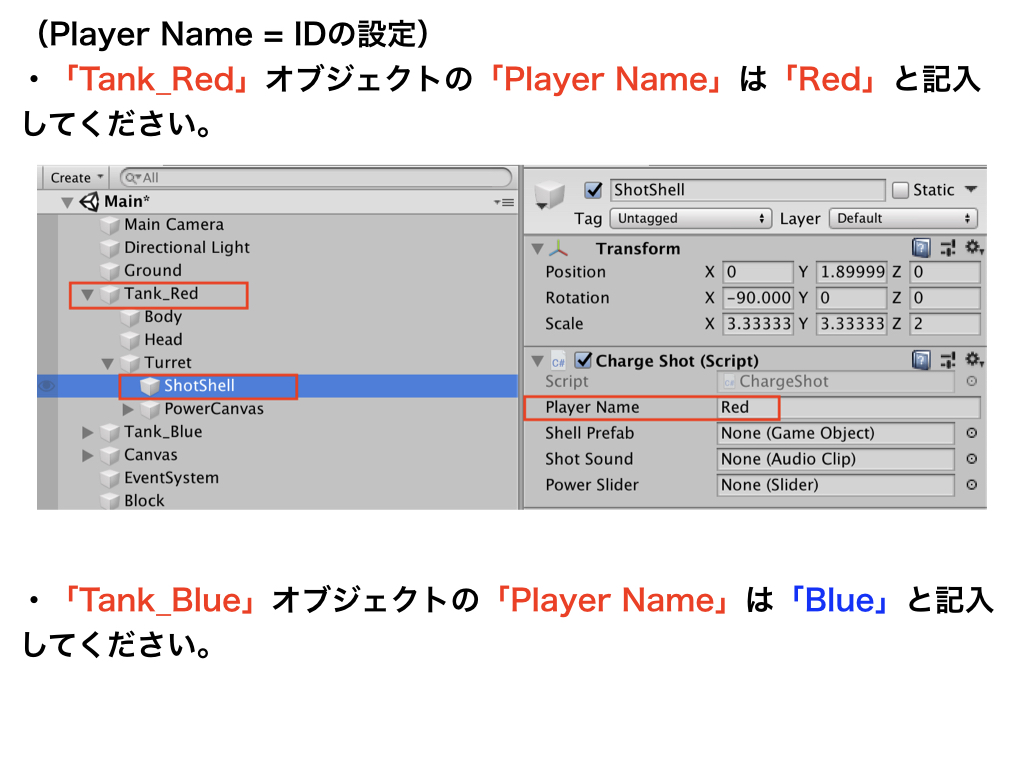
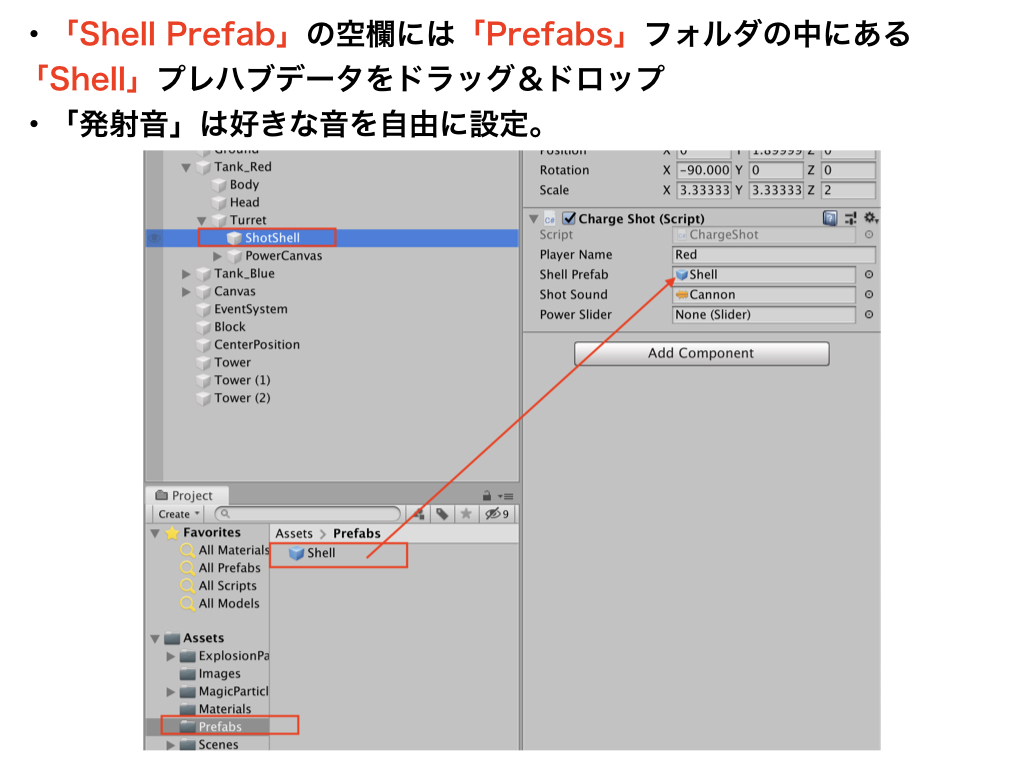
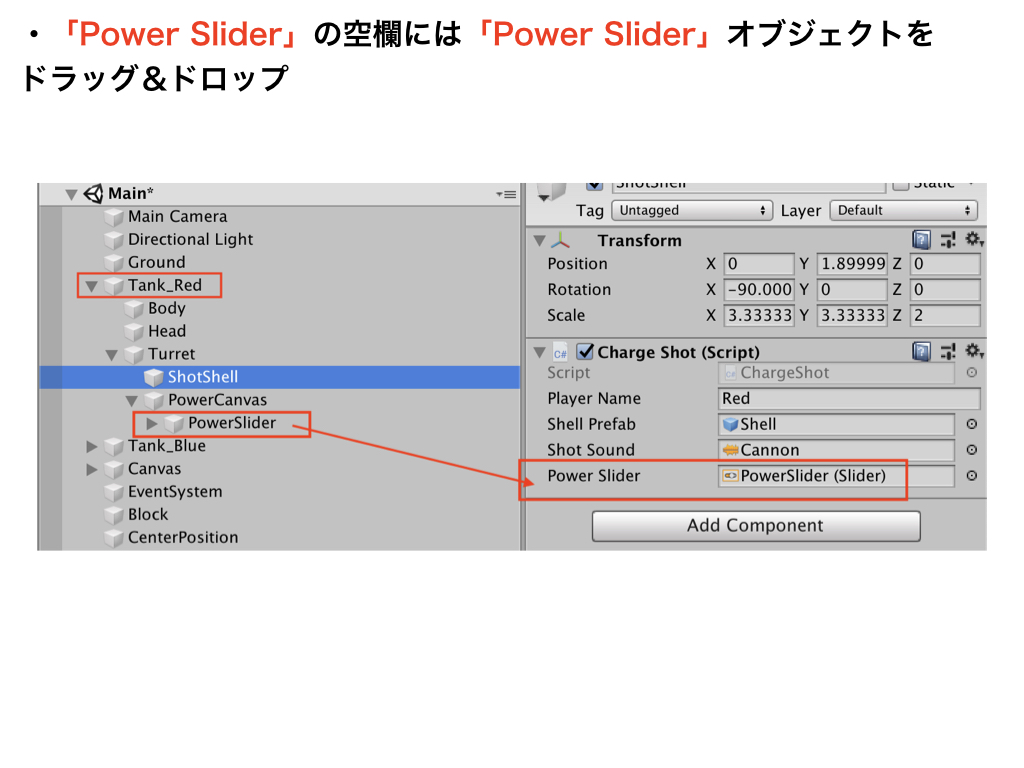
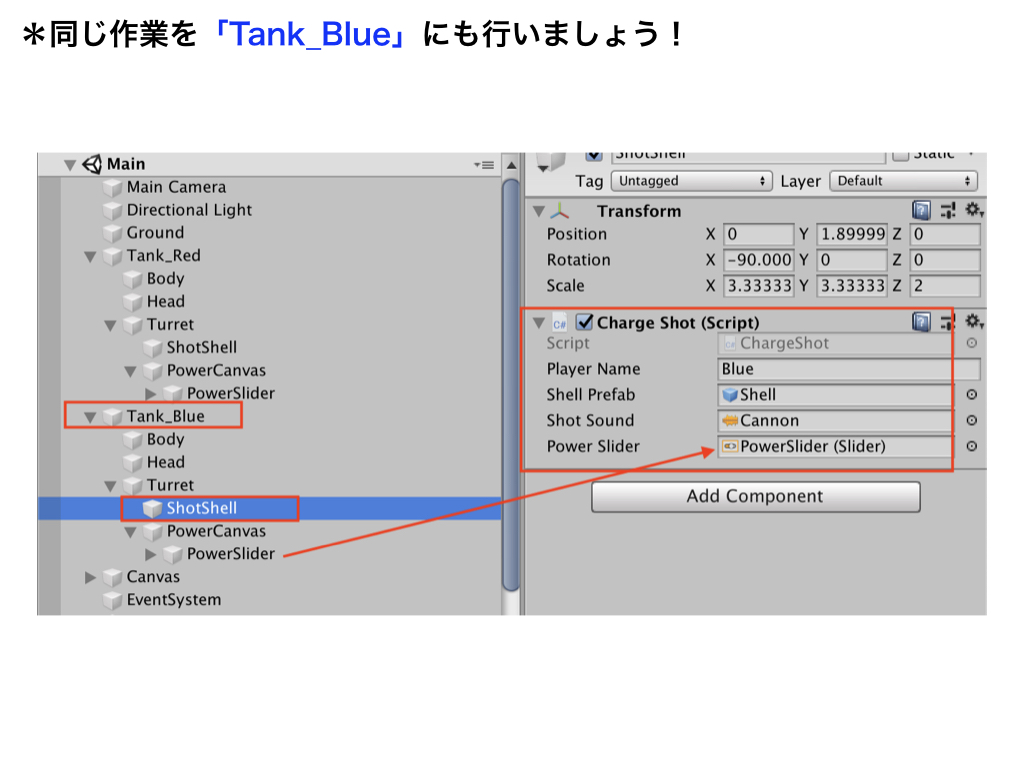
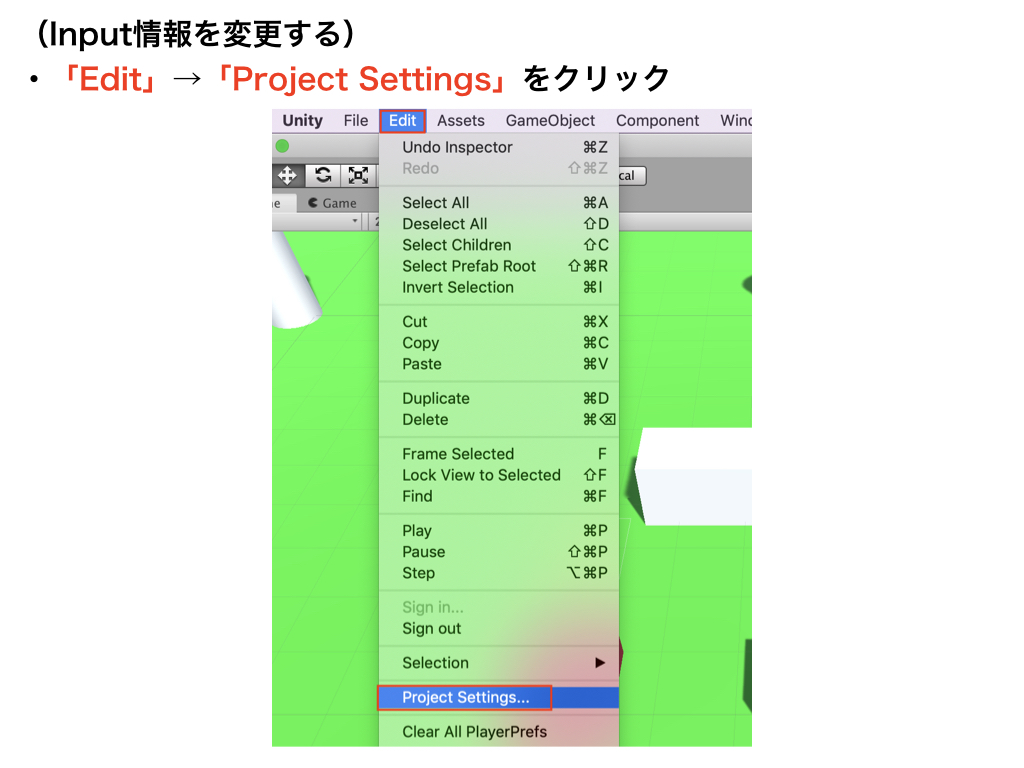
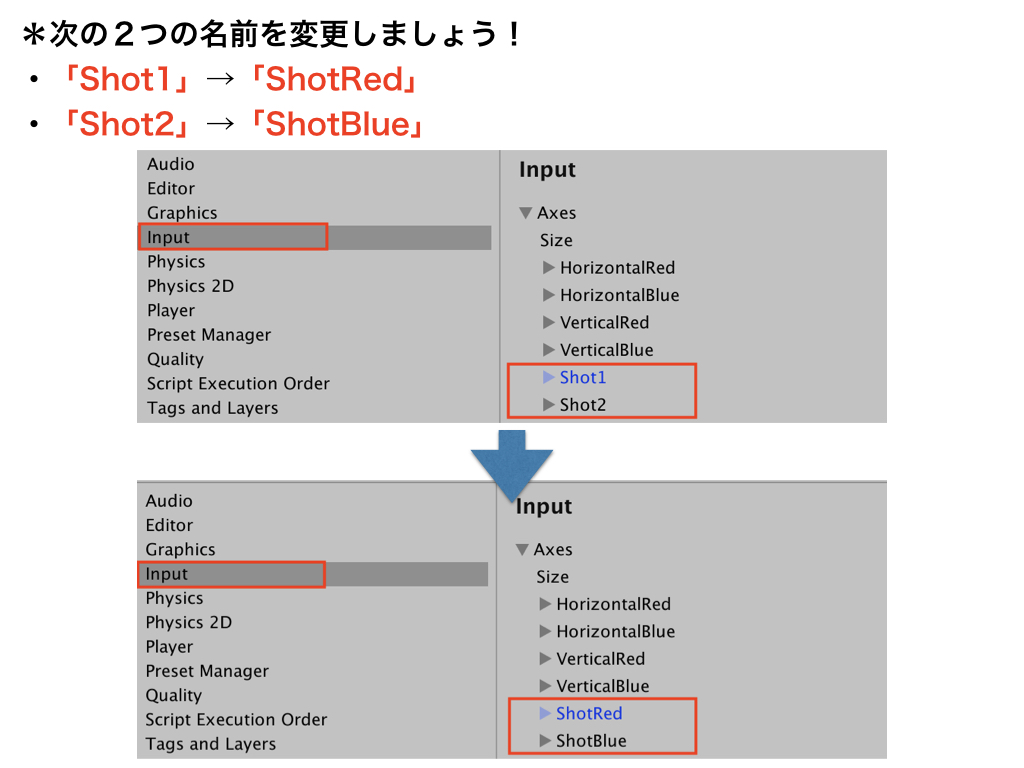
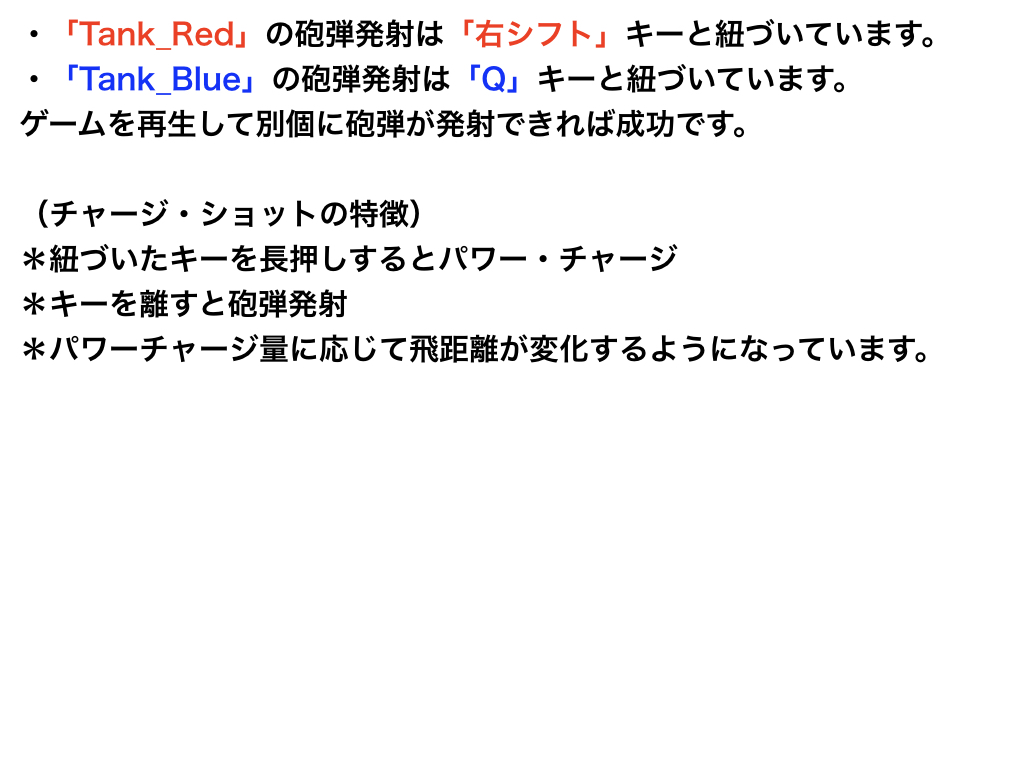
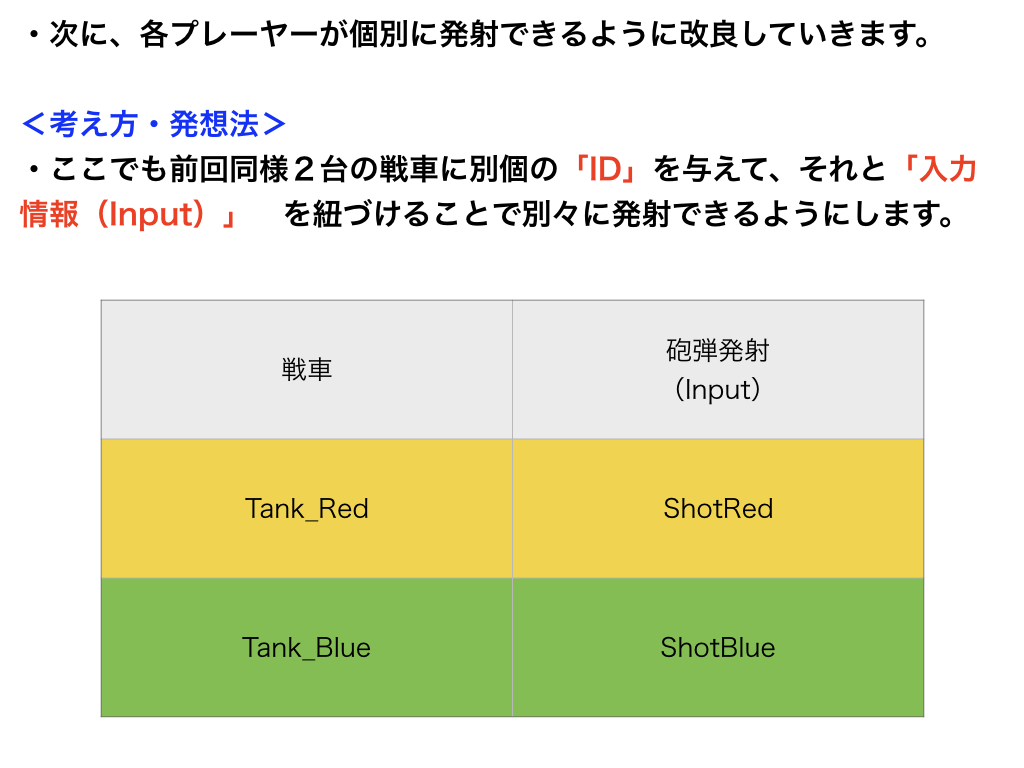
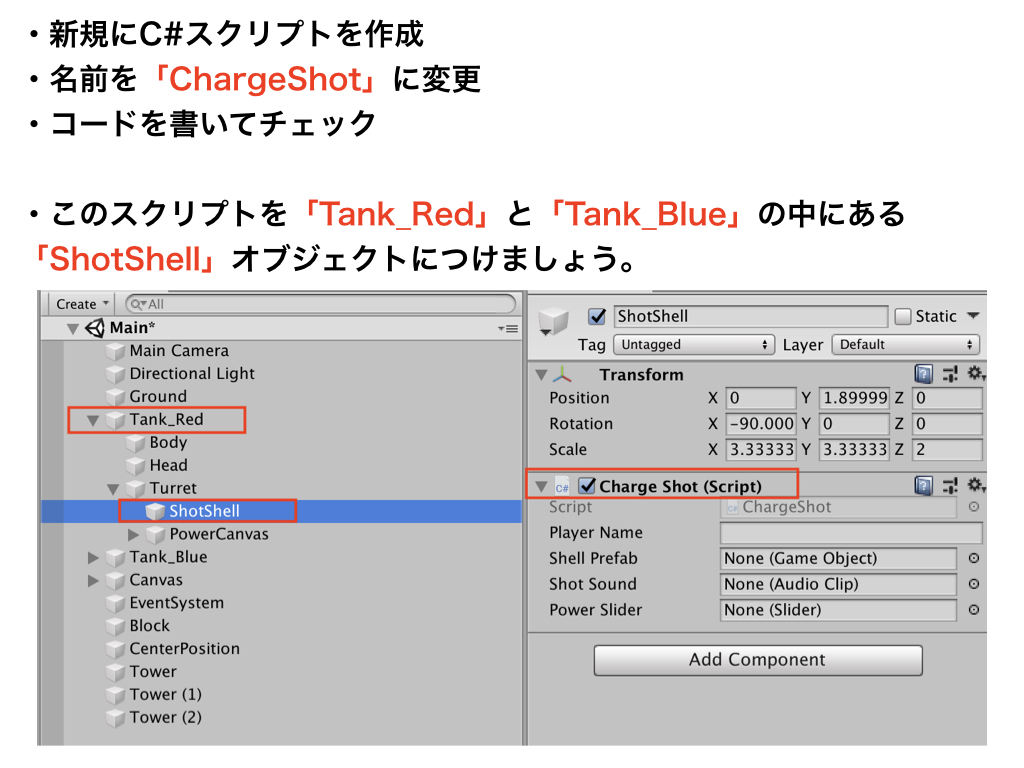
個別に砲弾を発射する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ChargeShot : MonoBehaviour
{
public string playerName;
public GameObject shellPrefab;
public AudioClip shotSound;
private float shotSpeed = 50f;
private int power = 0;
private int maxPower = 20;
public Slider powerSlider;
private void Start()
{
powerSlider.maxValue = maxPower;
powerSlider.value = power;
}
void Update()
{
if(Input.GetButton("Shot" + playerName))
{
// ボタンを押している間、パワーがチャージされる。
power += 1;
powerSlider.value = power;
// チャージできるパワーに上限を設定する。
if(power > maxPower)
{
power = maxPower;
}
}
if(Input.GetButtonUp("Shot" + playerName))
{
// ボタンを離した瞬間に砲弾が発射する。
GameObject shell = Instantiate(shellPrefab, transform.position, Quaternion.identity);
Rigidbody shellRb = shell.GetComponent<Rigidbody>();
shellRb.AddForce(transform.forward * shotSpeed * power);
Destroy(shell, 15.0f);
AudioSource.PlayClipAtPoint(shotSound, transform.position);
// 打ち終わったらパワーをリセットする。
power = 0;
powerSlider.value = power;
}
}
}
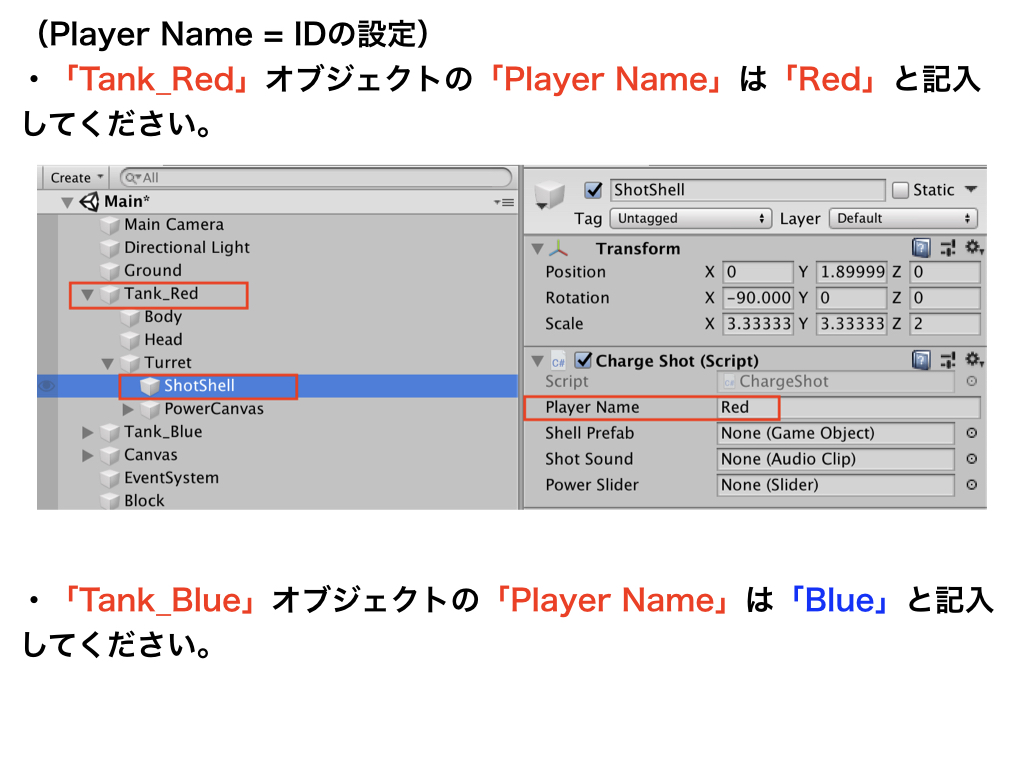
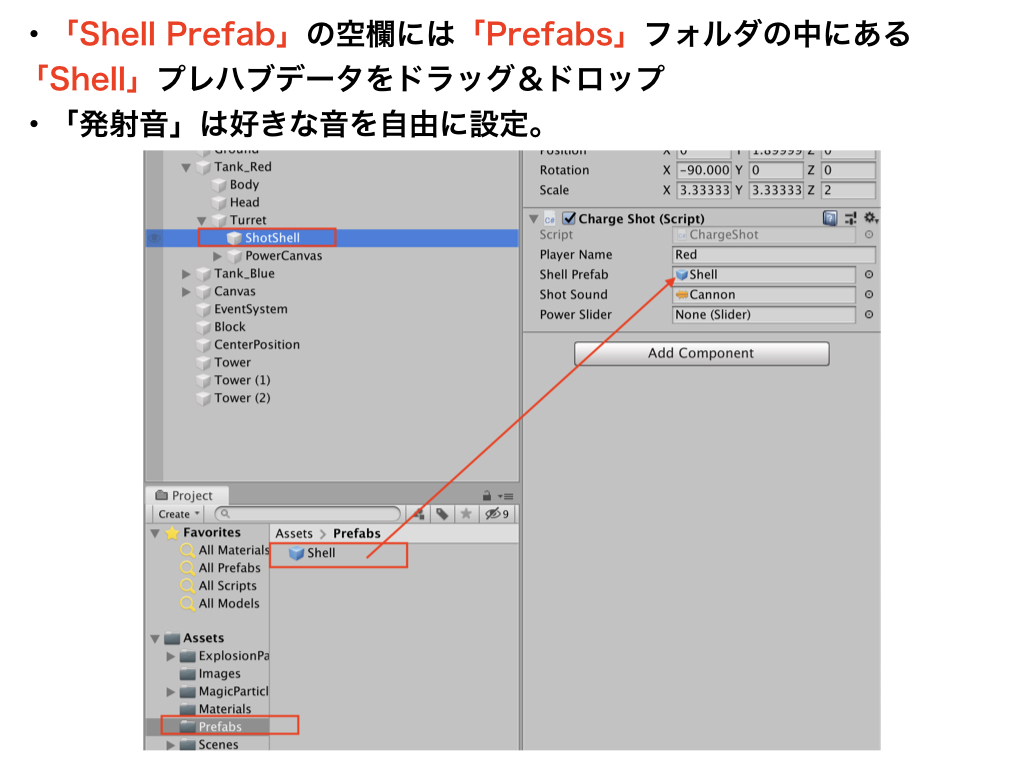
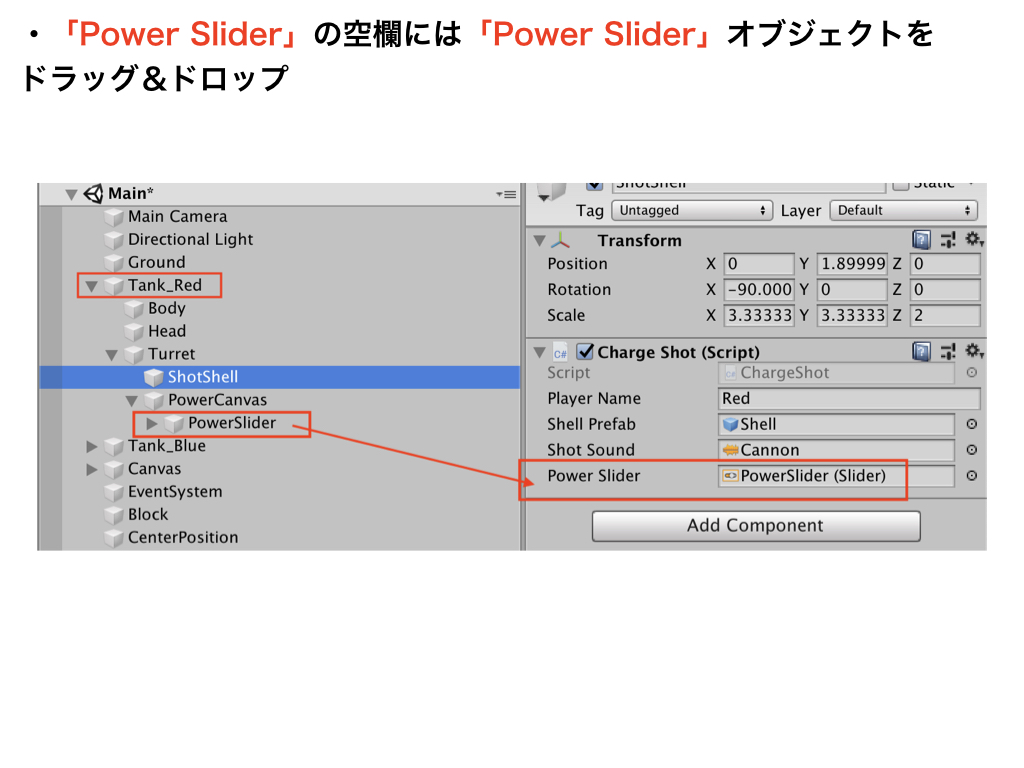
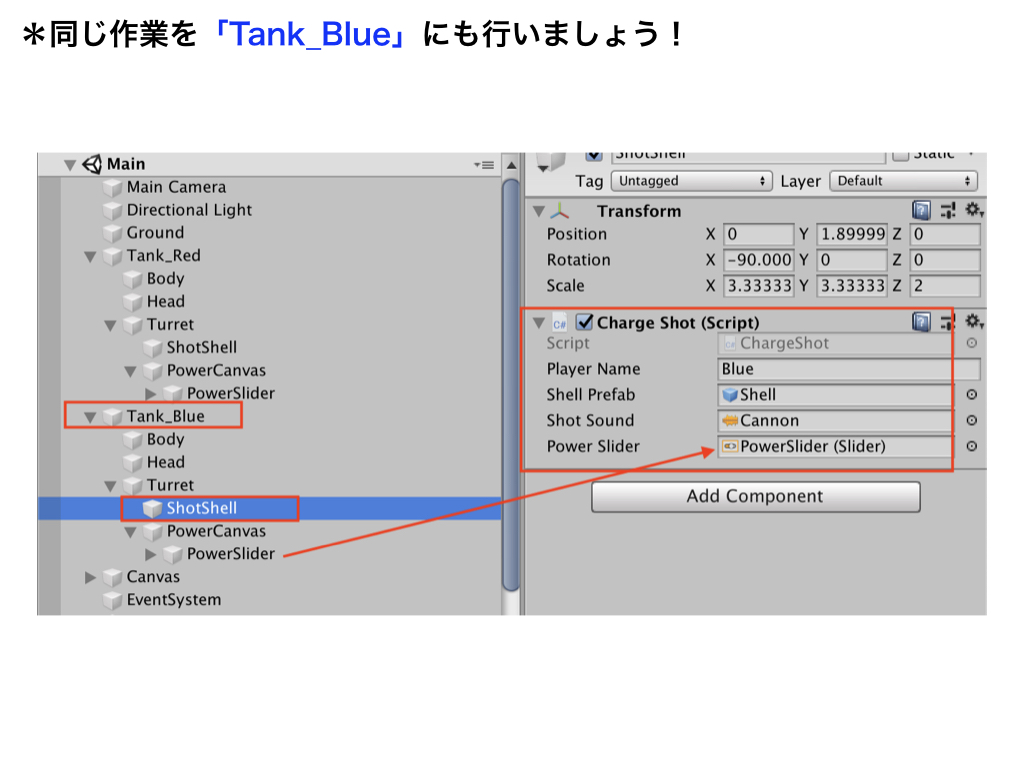
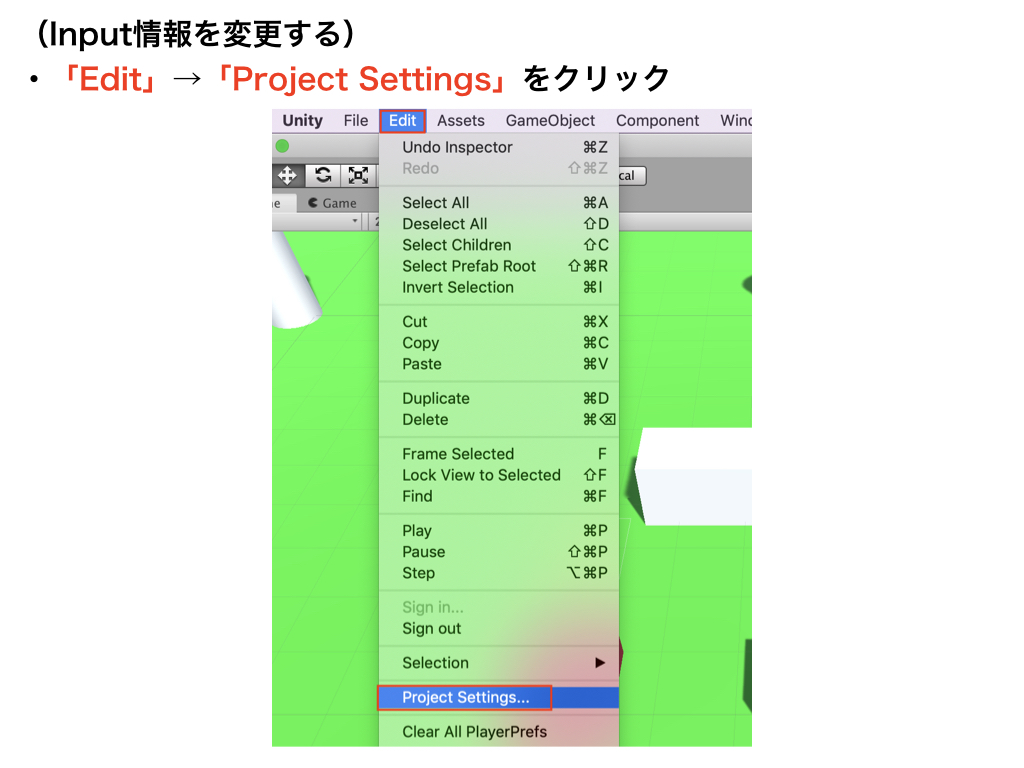
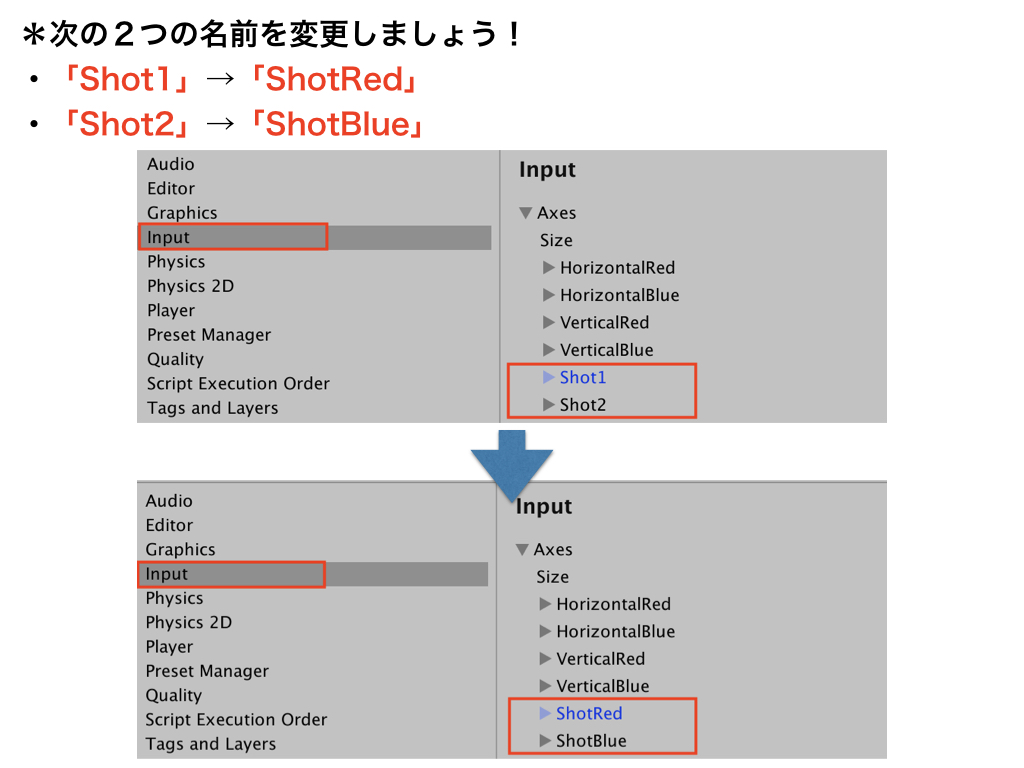
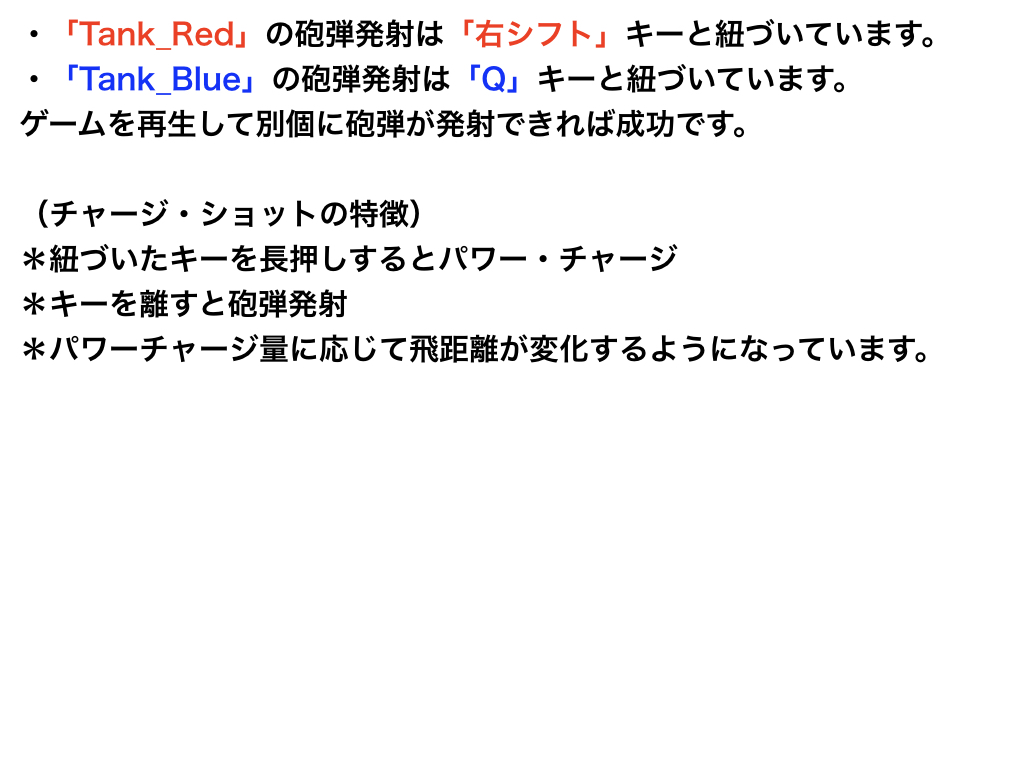
個別に砲弾を発射できるようにする