カメラが複数のオブジェクトを画面内に映すように設定
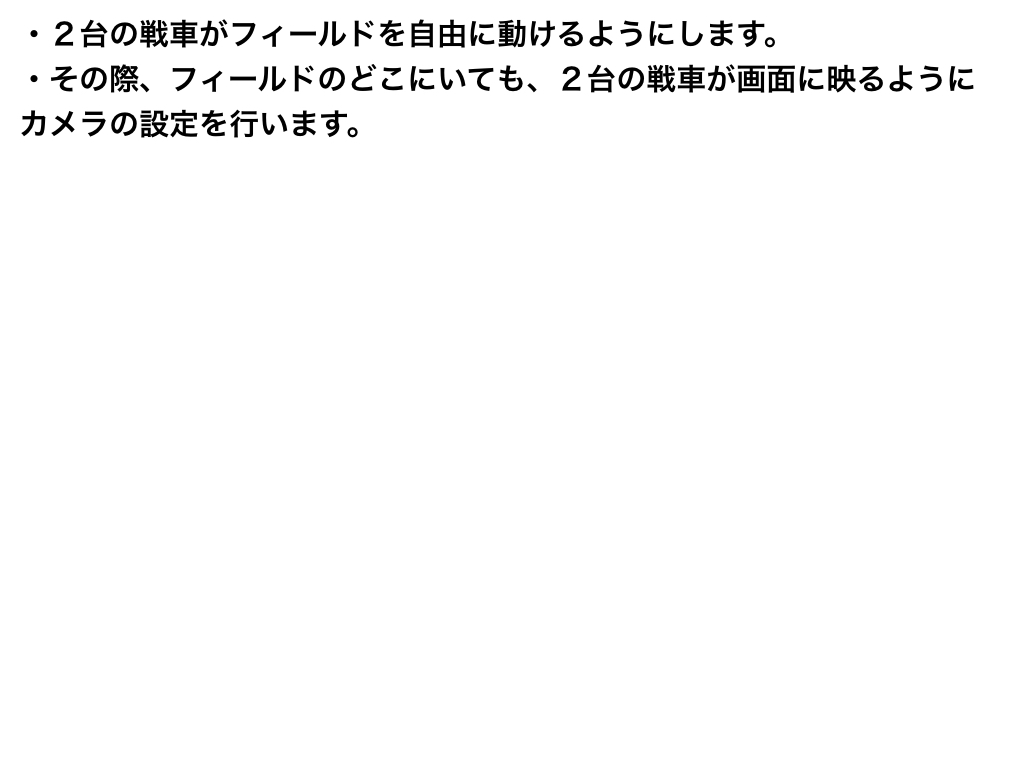
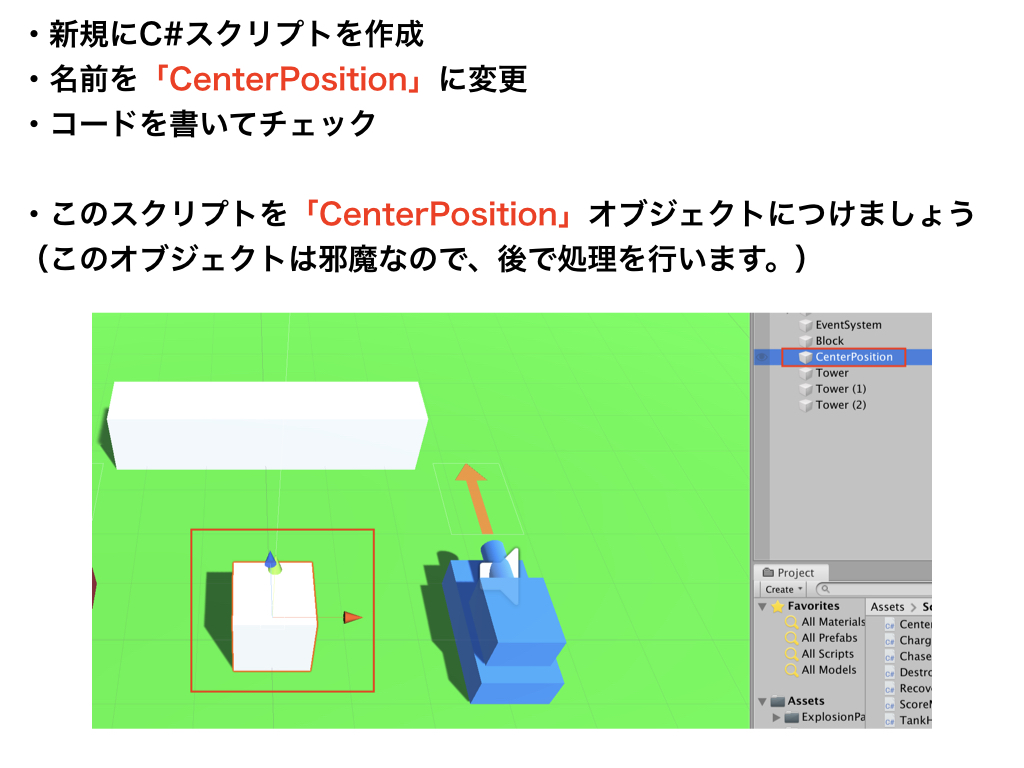
2点間の距離に応じて画面を拡大縮小する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CenterPosition : MonoBehaviour
{
public GameObject[] target;
public Camera cam;
void Update()
{
// 2台のタンクの位置から中間点を算出する。
Vector3 centerP = (target[0].transform.position + target[1].transform.position) * 0.5f;
// 上記で算出した中間点に、CenterPositionオブジェクトを移動させる。
transform.position = centerP;
// 2点間(2台のタンク)の距離
float dis = Vector3.Distance(target[0].transform.position, target[1].transform.position);
// カメラの描画サイズを2点間(2台のタンク)の距離に連動させる。
// 2台のタンクが離れた場合には、描画サイズを大きくする。
cam.orthographicSize = dis;
// カメラの描画サイズを一定の範囲に制限する。
if (cam.orthographicSize < 10)
{
cam.orthographicSize = 10;
}
if (cam.orthographicSize > 20)
{
cam.orthographicSize = 20;
}
}
}
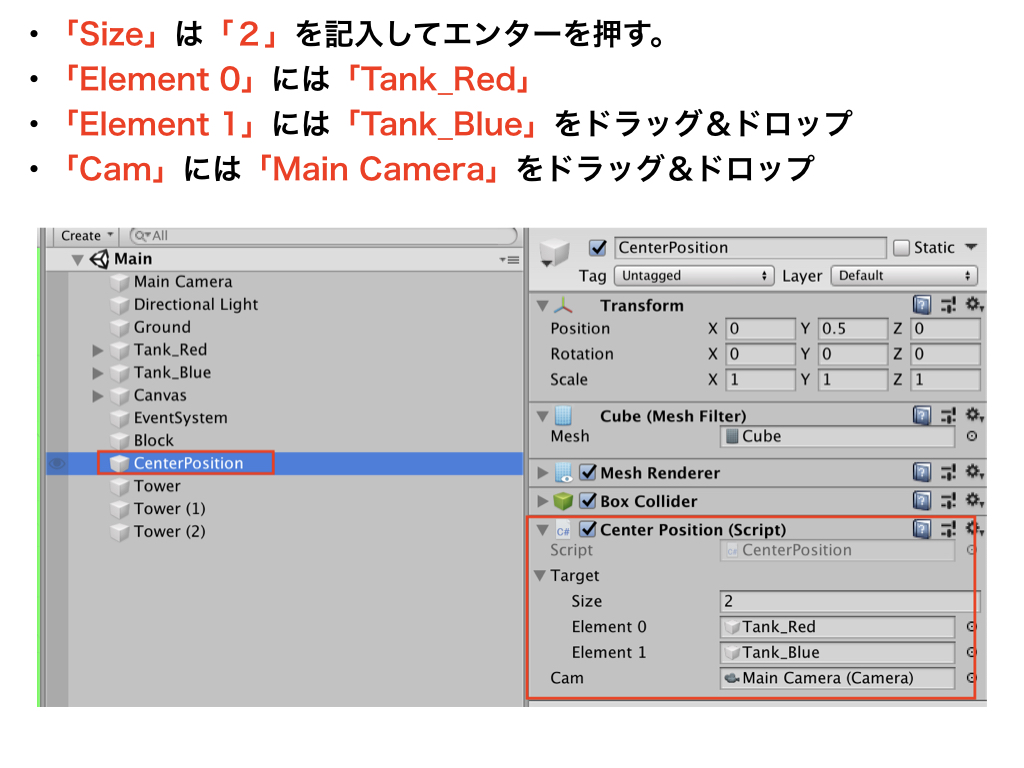
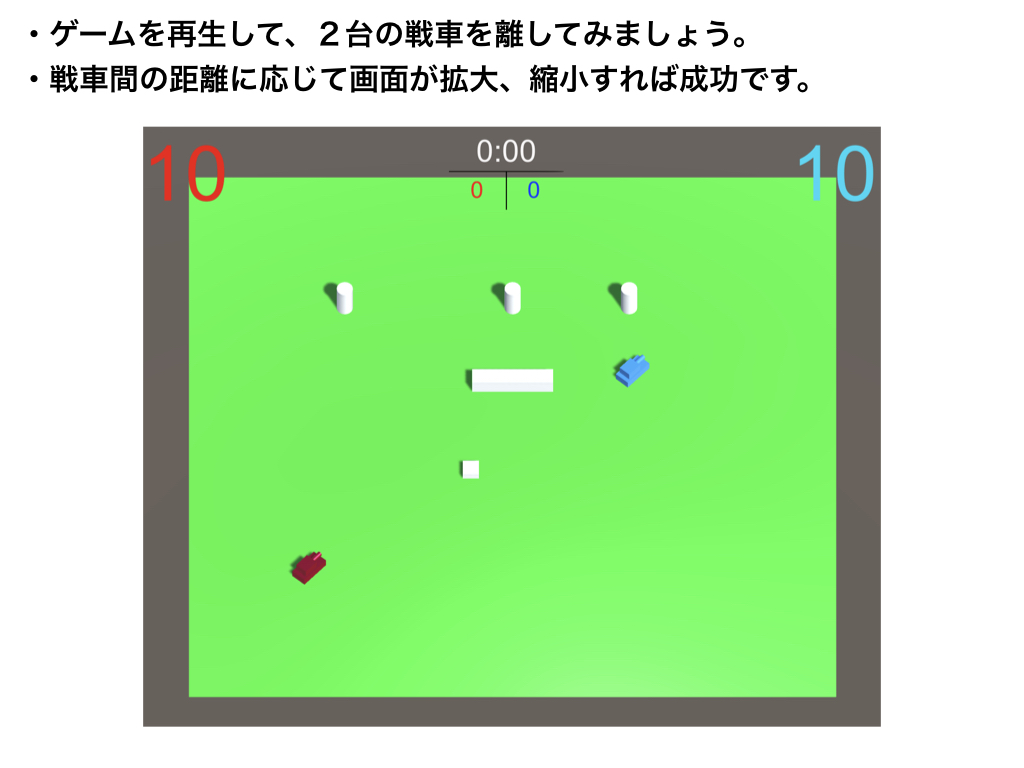
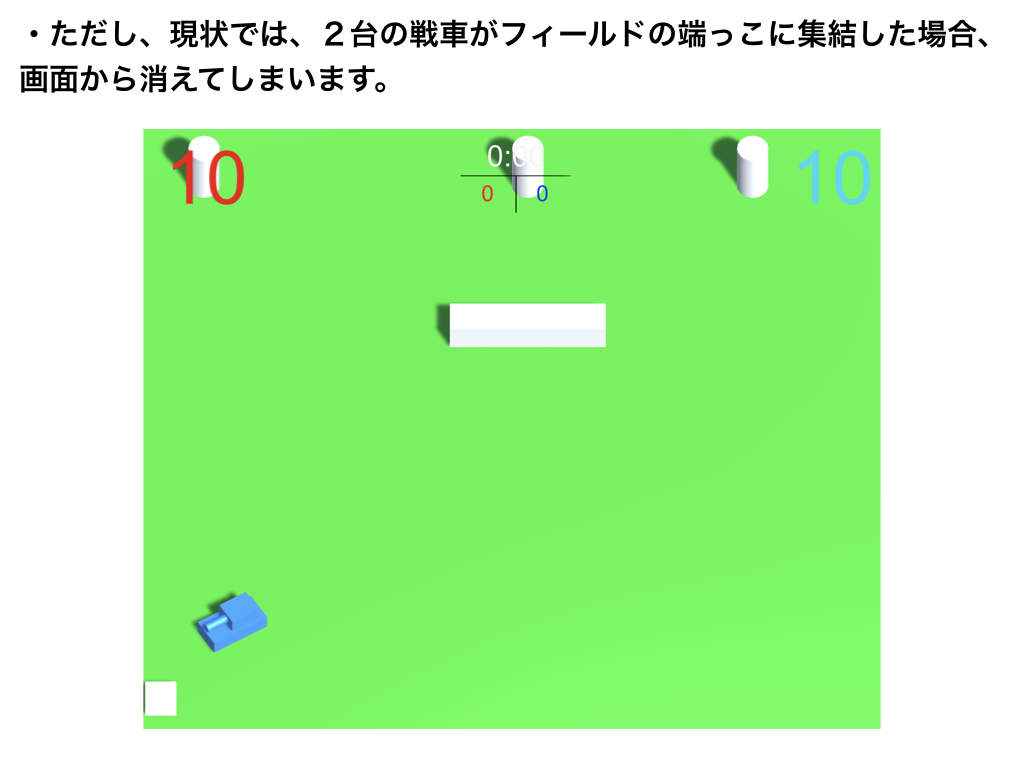
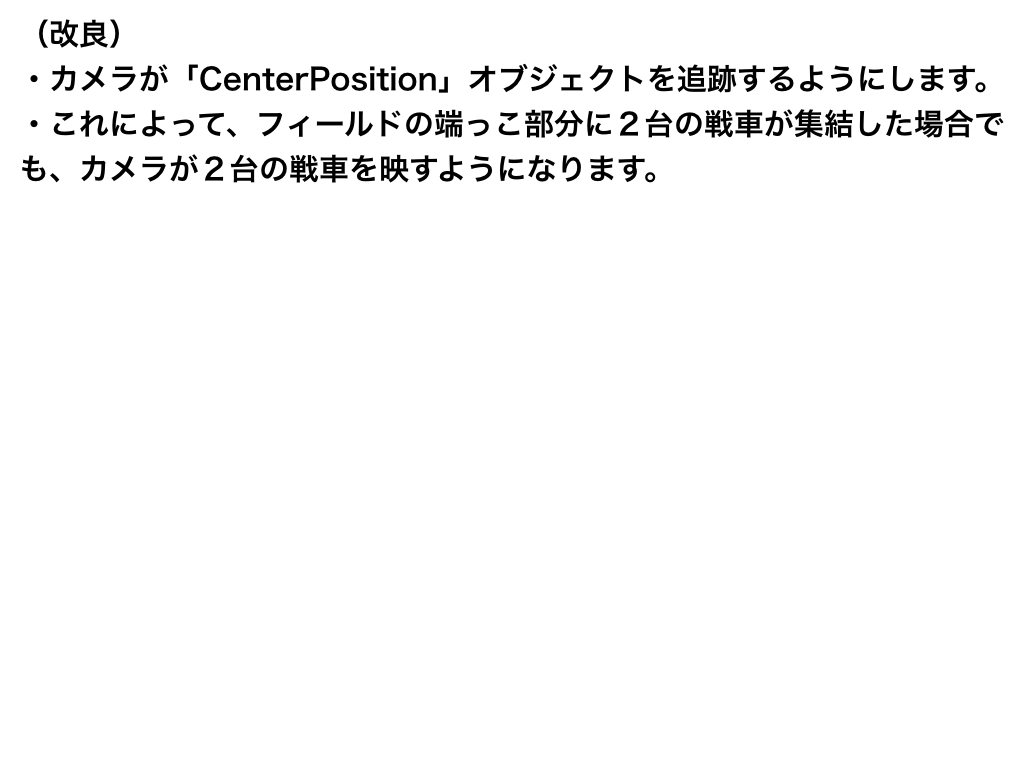
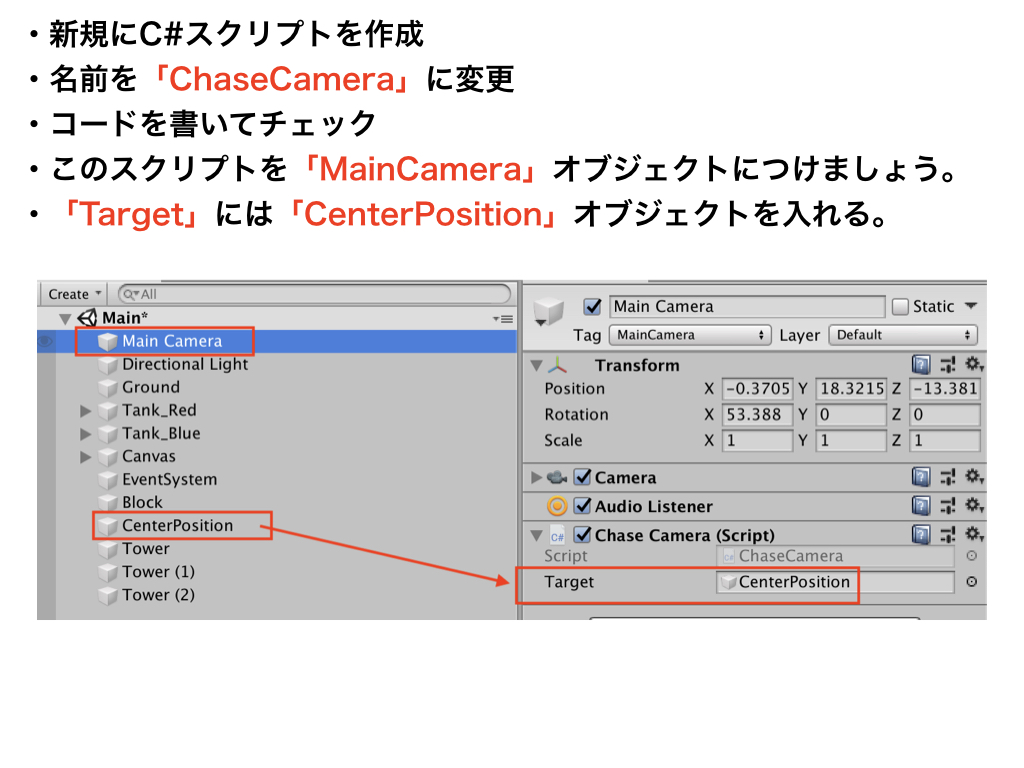
追跡カメラ
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ChaseCamera : MonoBehaviour
{
public GameObject target;
private Vector3 offset;
void Start()
{
offset = transform.position - target.transform.position;
}
void Update()
{
if(target != null)
{
transform.position = target.transform.position + offset;
}
}
}
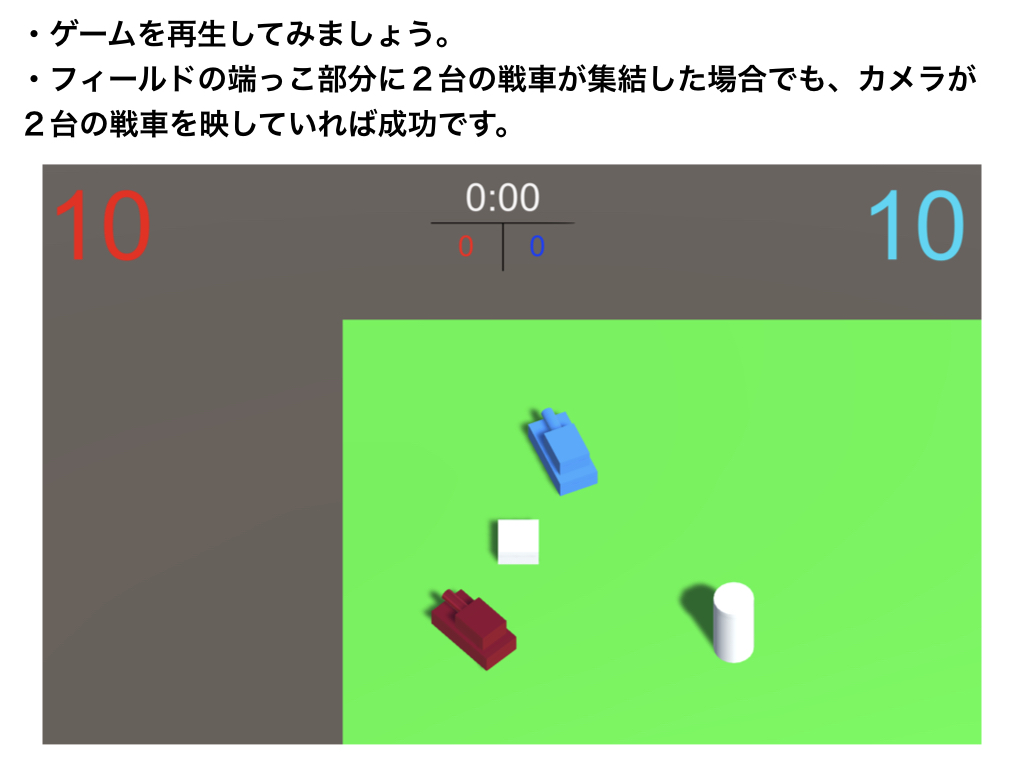
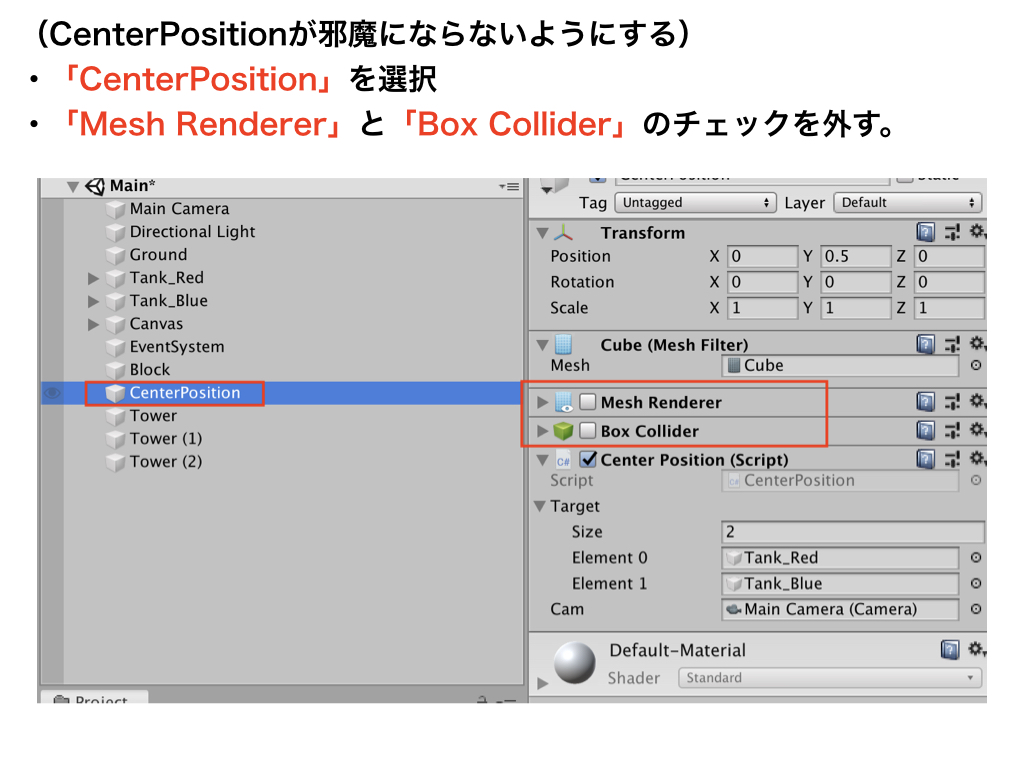
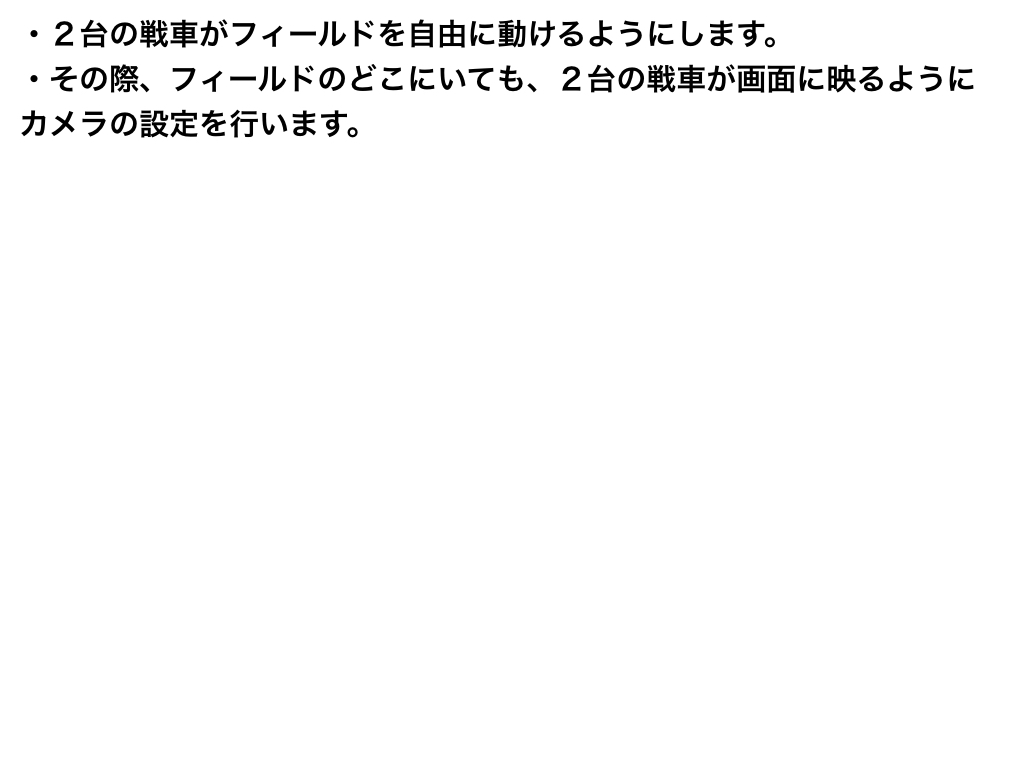
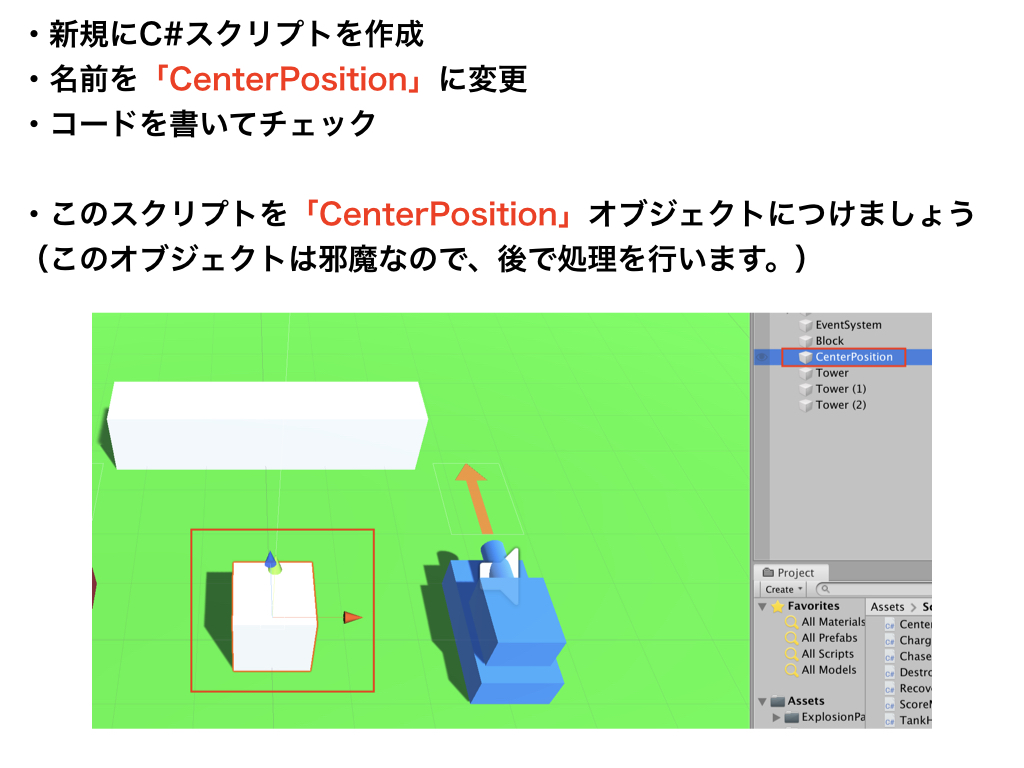
2点間の距離に応じて画面を拡大縮小する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CenterPosition : MonoBehaviour
{
public GameObject[] target;
public Camera cam;
void Update()
{
// 2台のタンクの位置から中間点を算出する。
Vector3 centerP = (target[0].transform.position + target[1].transform.position) * 0.5f;
// 上記で算出した中間点に、CenterPositionオブジェクトを移動させる。
transform.position = centerP;
// 2点間(2台のタンク)の距離
float dis = Vector3.Distance(target[0].transform.position, target[1].transform.position);
// カメラの描画サイズを2点間(2台のタンク)の距離に連動させる。
// 2台のタンクが離れた場合には、描画サイズを大きくする。
cam.orthographicSize = dis;
// カメラの描画サイズを一定の範囲に制限する。
if (cam.orthographicSize < 10)
{
cam.orthographicSize = 10;
}
if (cam.orthographicSize > 20)
{
cam.orthographicSize = 20;
}
}
}
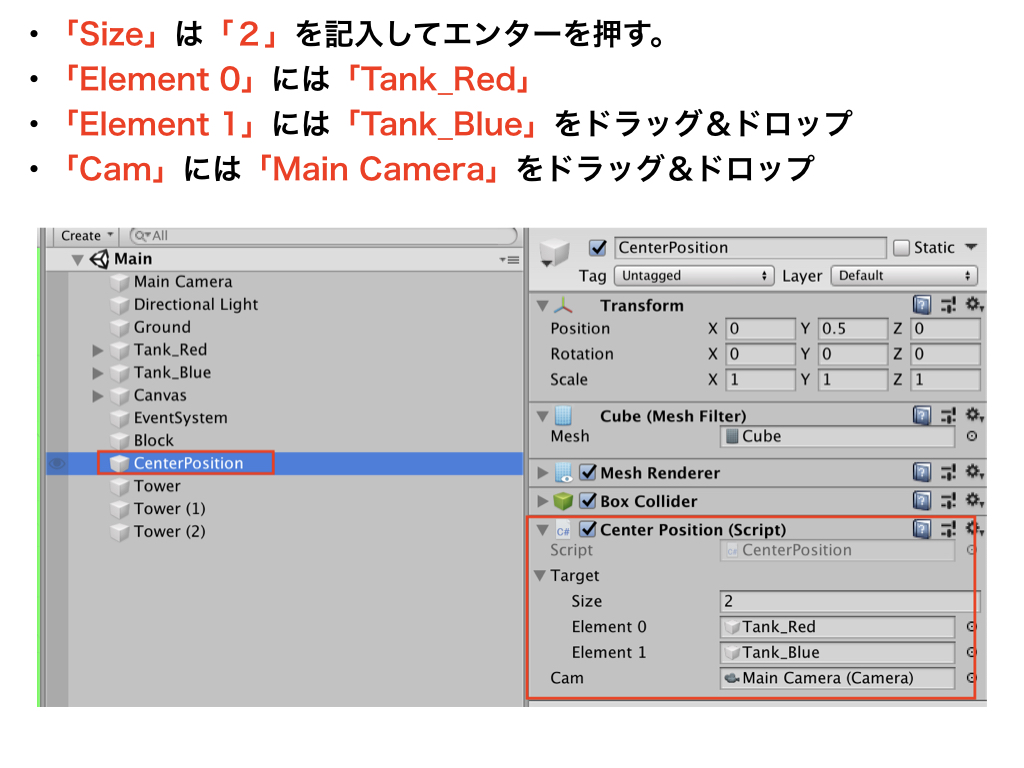
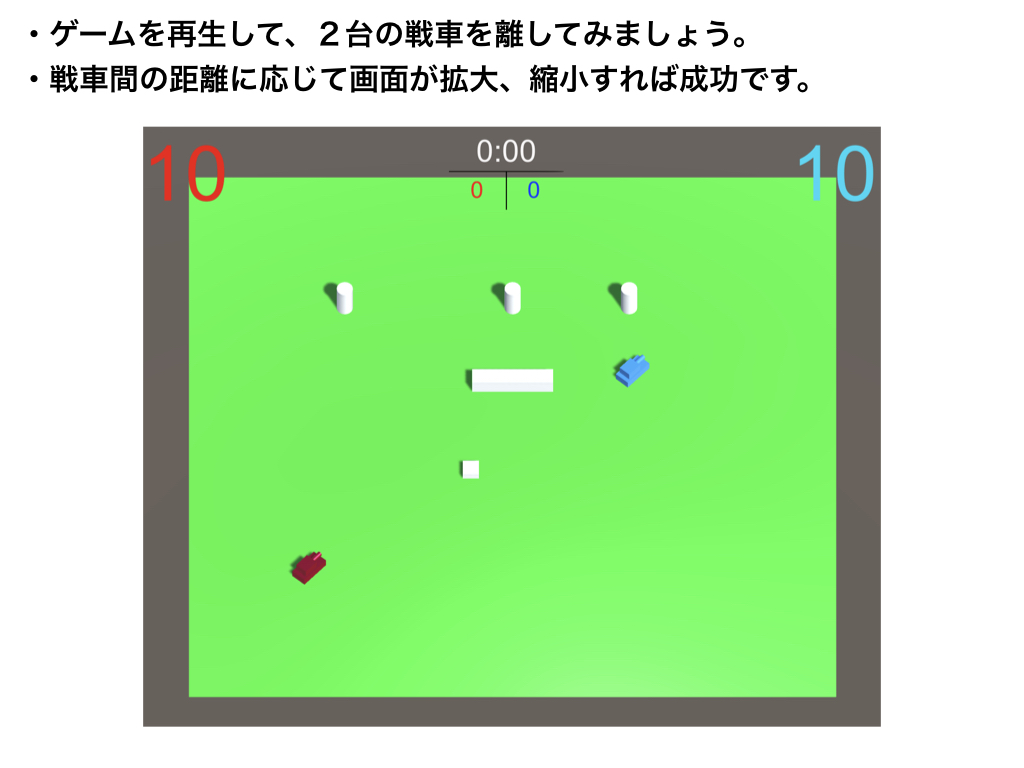
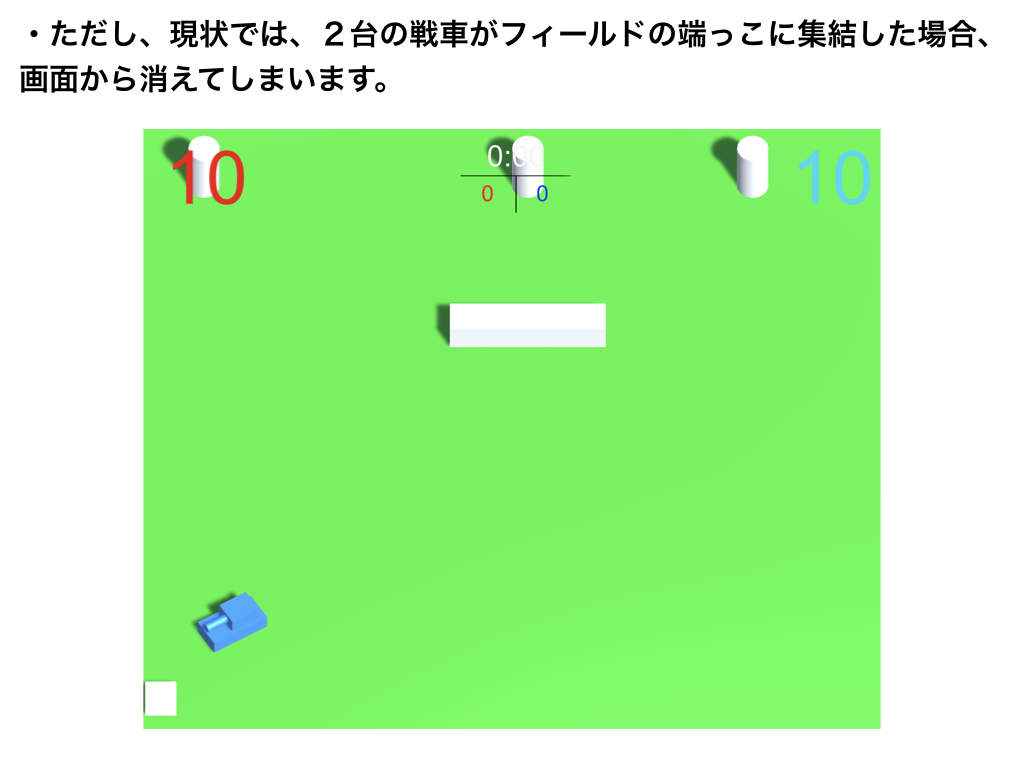
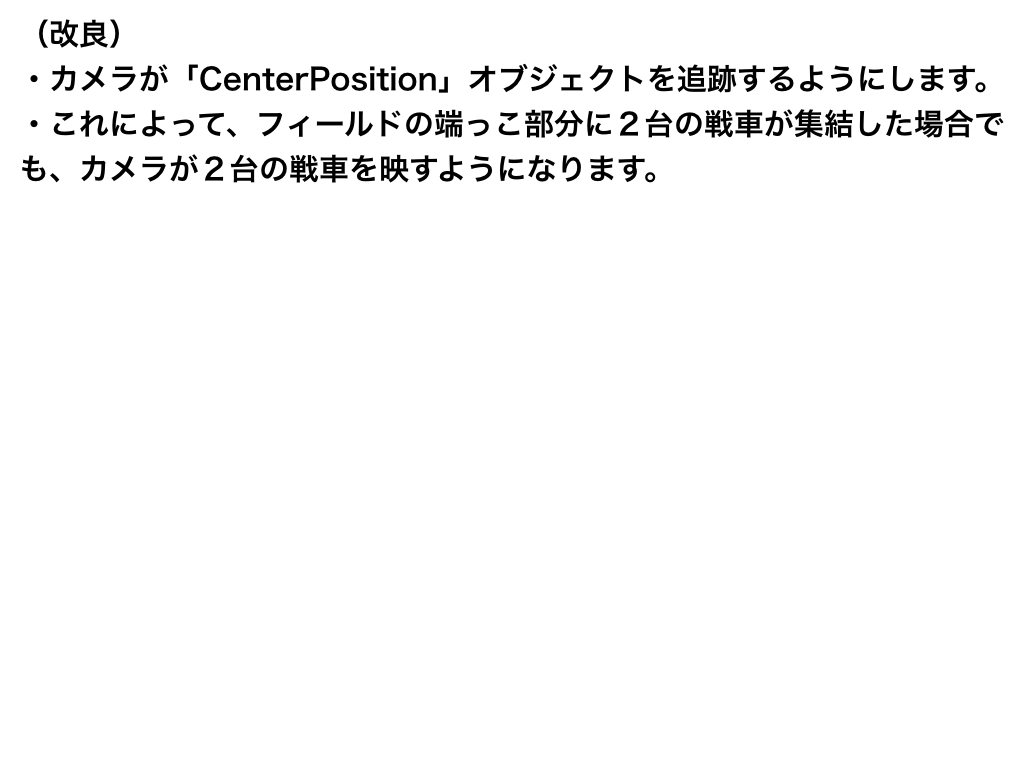
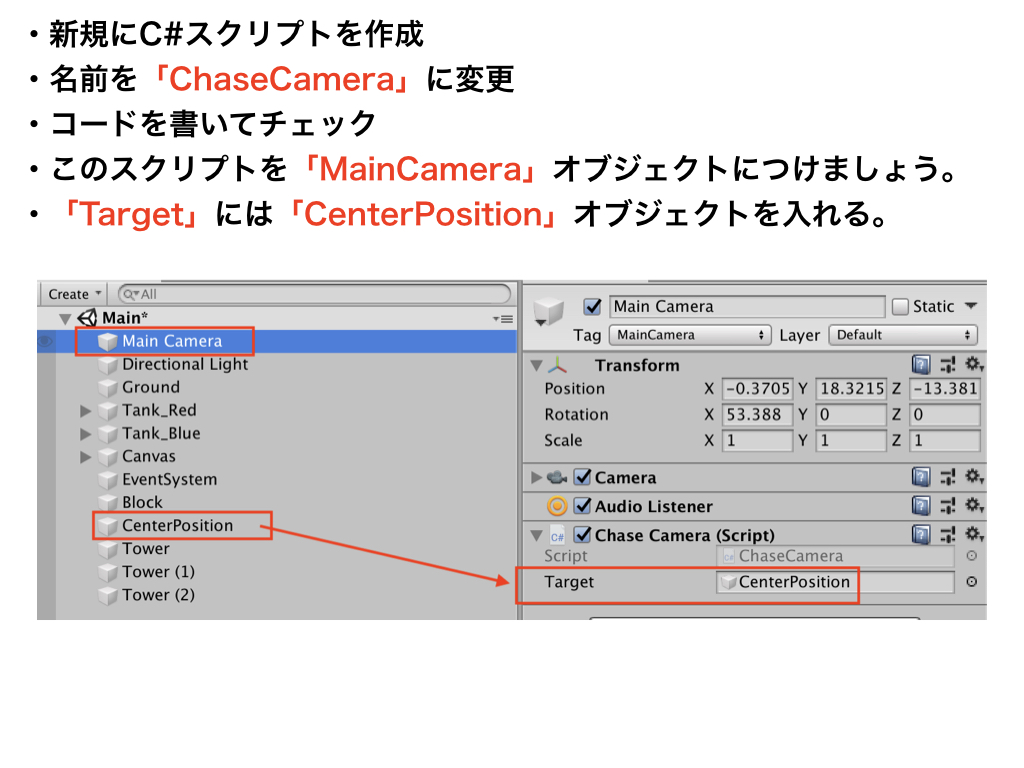
追跡カメラ
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ChaseCamera : MonoBehaviour
{
public GameObject target;
private Vector3 offset;
void Start()
{
offset = transform.position - target.transform.position;
}
void Update()
{
if(target != null)
{
transform.position = target.transform.position + offset;
}
}
}
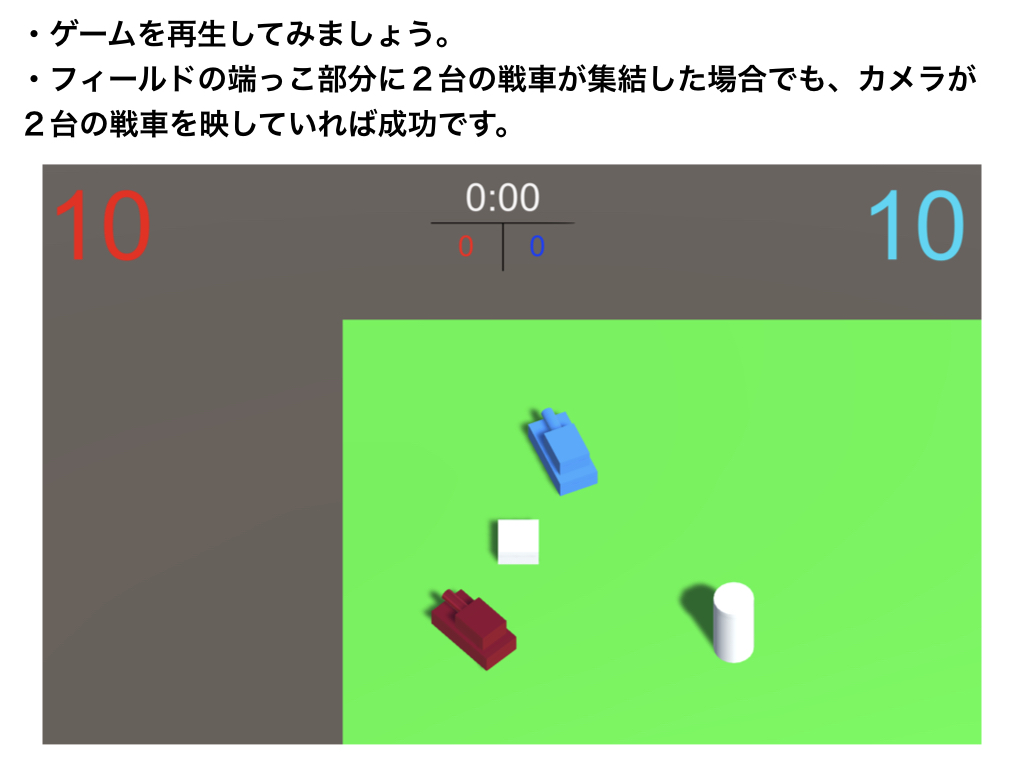
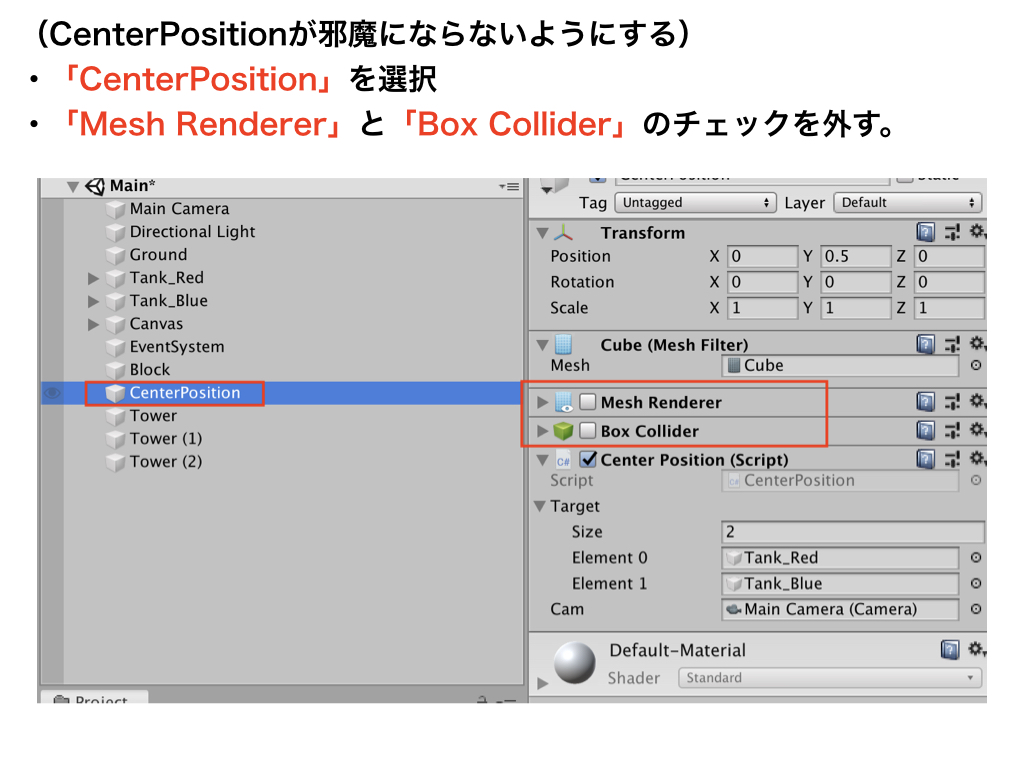
カメラが複数のオブジェクトを画面内に映すように設定