プレーヤーがダメージを受けたら視界を揺らす
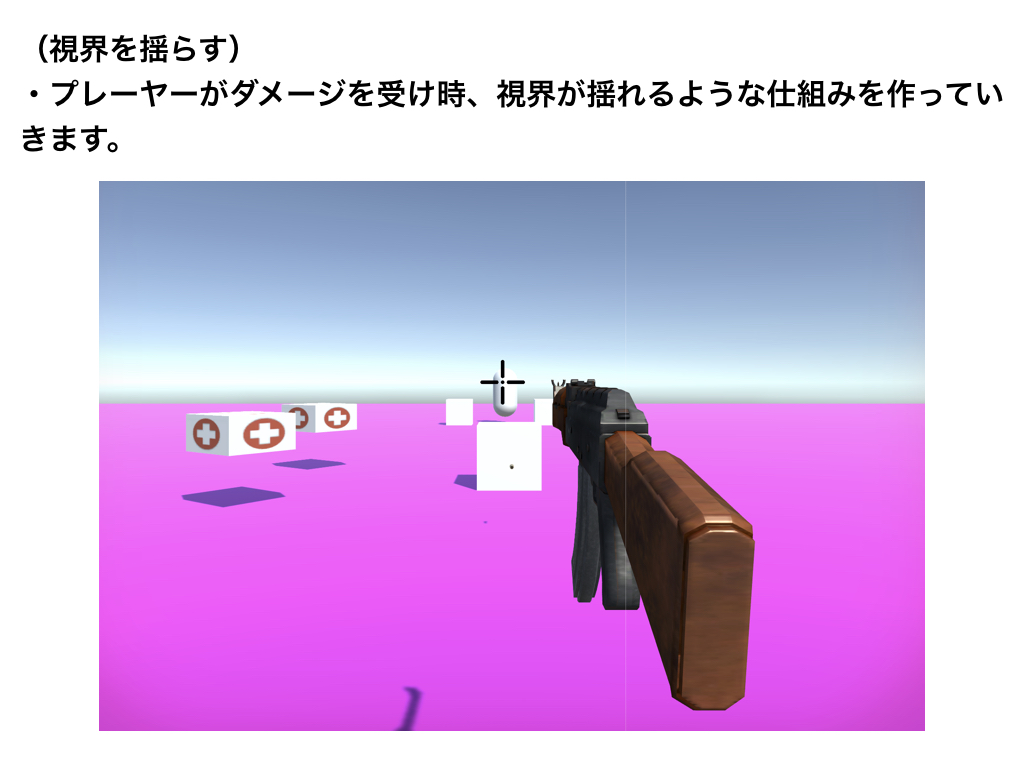
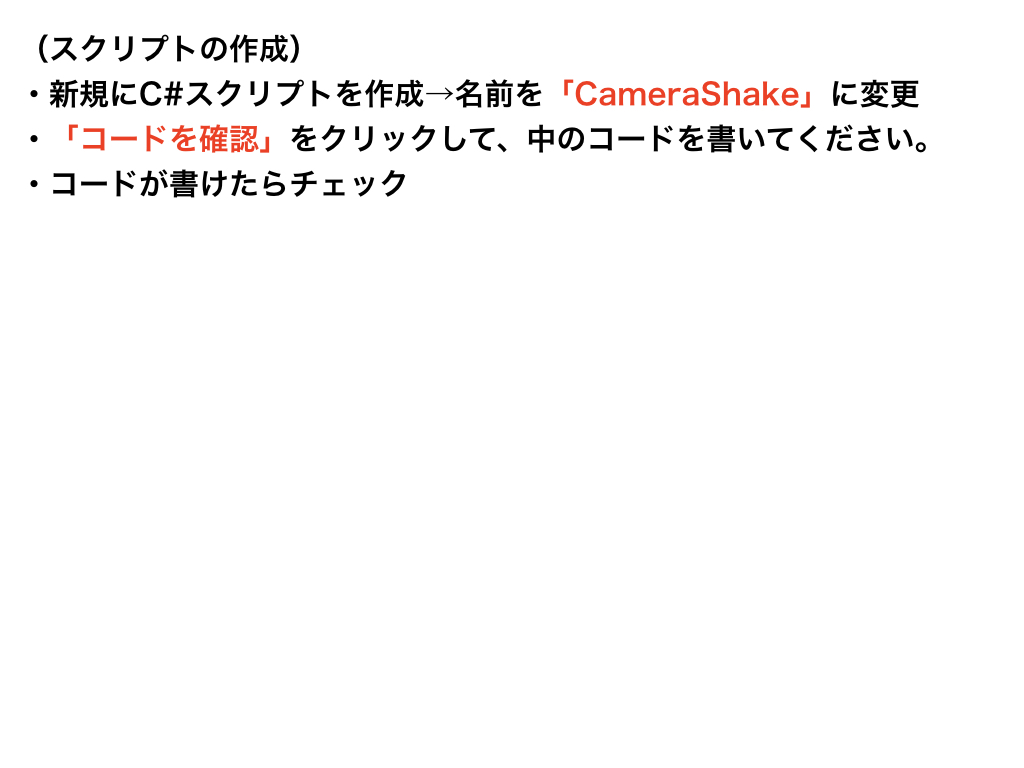
視界を揺らす
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CameraShake : MonoBehaviour {
public void Shake(float duration, float magnitude){
StartCoroutine(TheShake(duration, magnitude));
}
private IEnumerator TheShake(float duration, float magnitude){
Vector3 pos = transform.localPosition;
float elapsed = 0f; // 経過時間
while(elapsed < duration){
var x = pos.x + Random.Range(-1f, 1f) * magnitude;
var y = pos.y + Random.Range(-1f, 1f) * magnitude;
transform.localPosition = new Vector3(x, y, pos.z);
elapsed += Time.deltaTime;
yield return null;
}
transform.localPosition = pos;
}
}
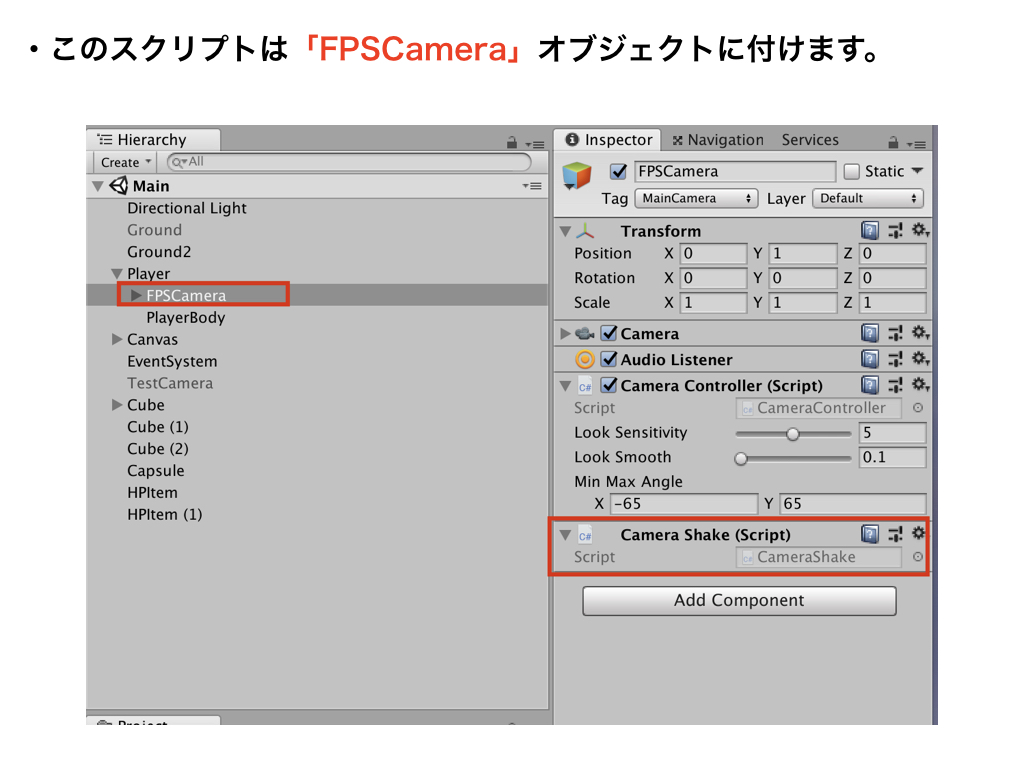
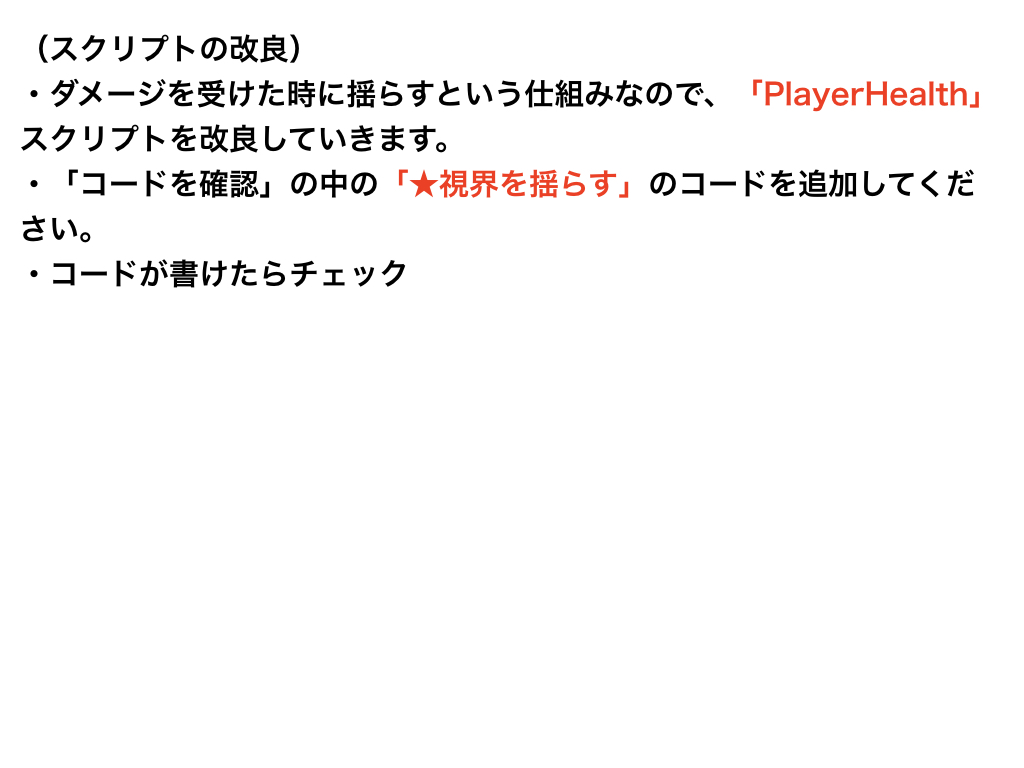
視界を揺らすを実行する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class PlayerHealth : MonoBehaviour {
public int playerHP;
public AudioClip damageSound;
private Image damageScreenImage;
private Color color;
// ★視界を揺らす
private CameraShake shake;
void Start(){
damageScreenImage = GameObject.Find("DamageScreen").GetComponent<Image>();
color = damageScreenImage.color;
color.a = 0.0f;
damageScreenImage.color = color;
// ★視界を揺らす
shake = GameObject.Find("FPSCamera").GetComponent<CameraShake>();
}
void OnCollisionEnter(Collision other){
if(other.gameObject.tag == "EnemyBullet"){
playerHP -= 1;
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(damageSound, Camera.main.transform.position);
color.a += 0.1f;
damageScreenImage.color = color;
// ★視界を揺らす
shake.Shake(0.2f, 0.1f);
}
}
public void AddHP(int amount){
playerHP += amount;
if(playerHP > 10){
playerHP = 10;
}
float amountF = (float)amount;
color.a -= (amountF / 10);
if(color.a < 0){
color.a = 0;
}
damageScreenImage.color = color;
}
}
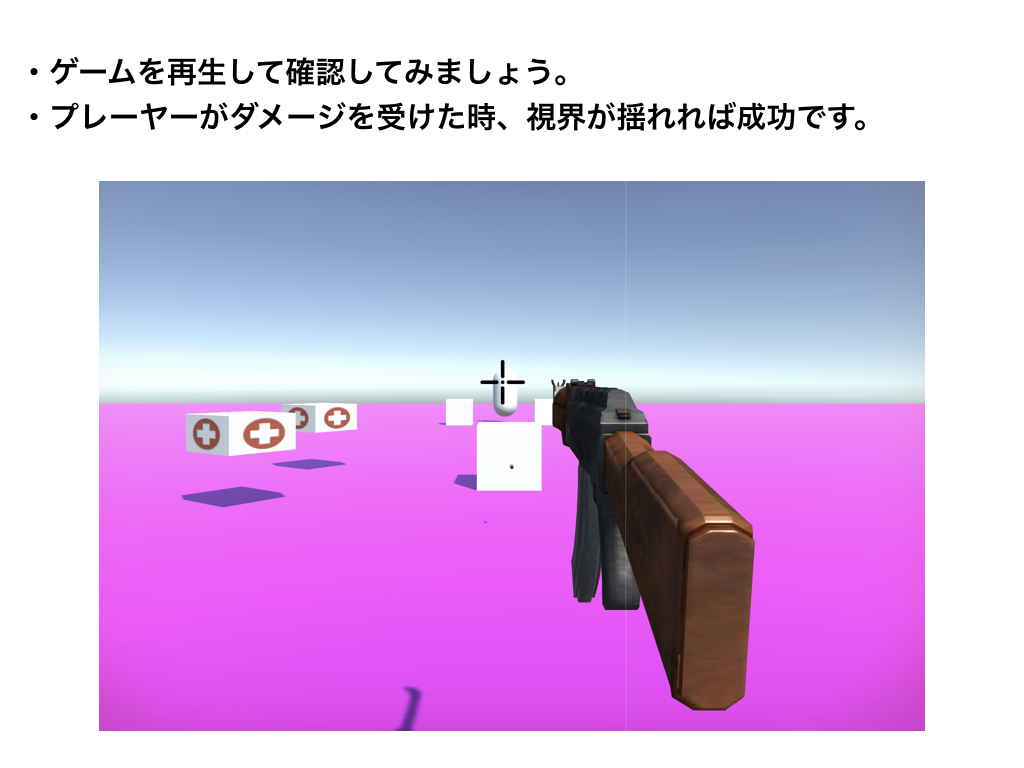
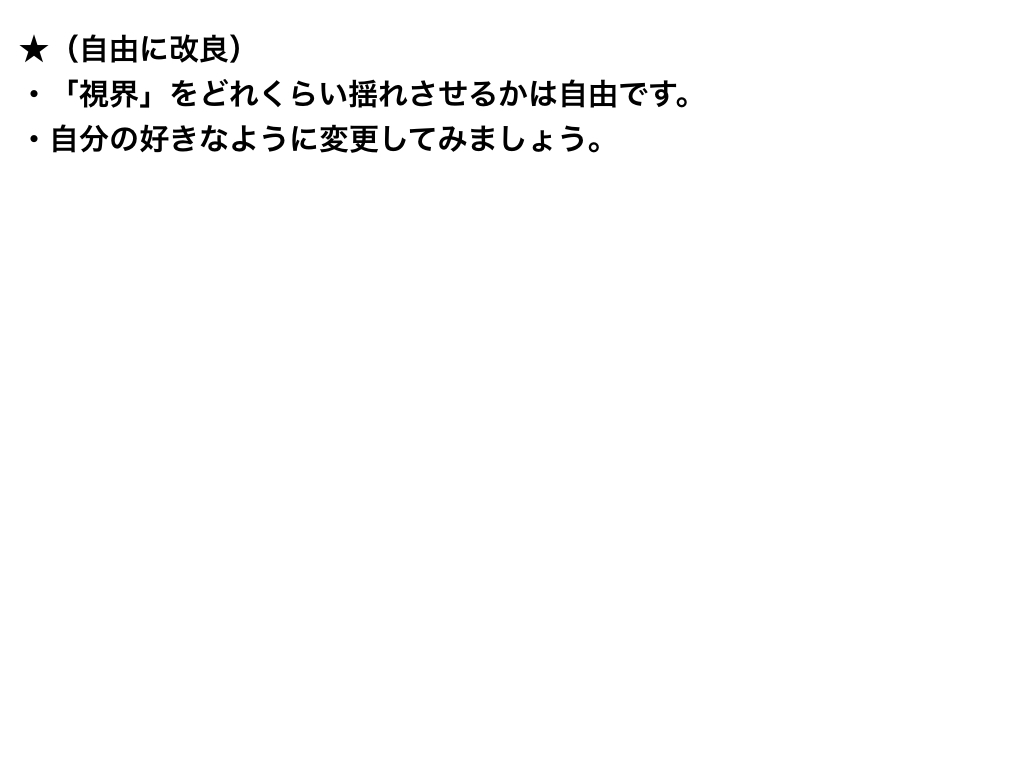
EscapeCombat
他のコースを見る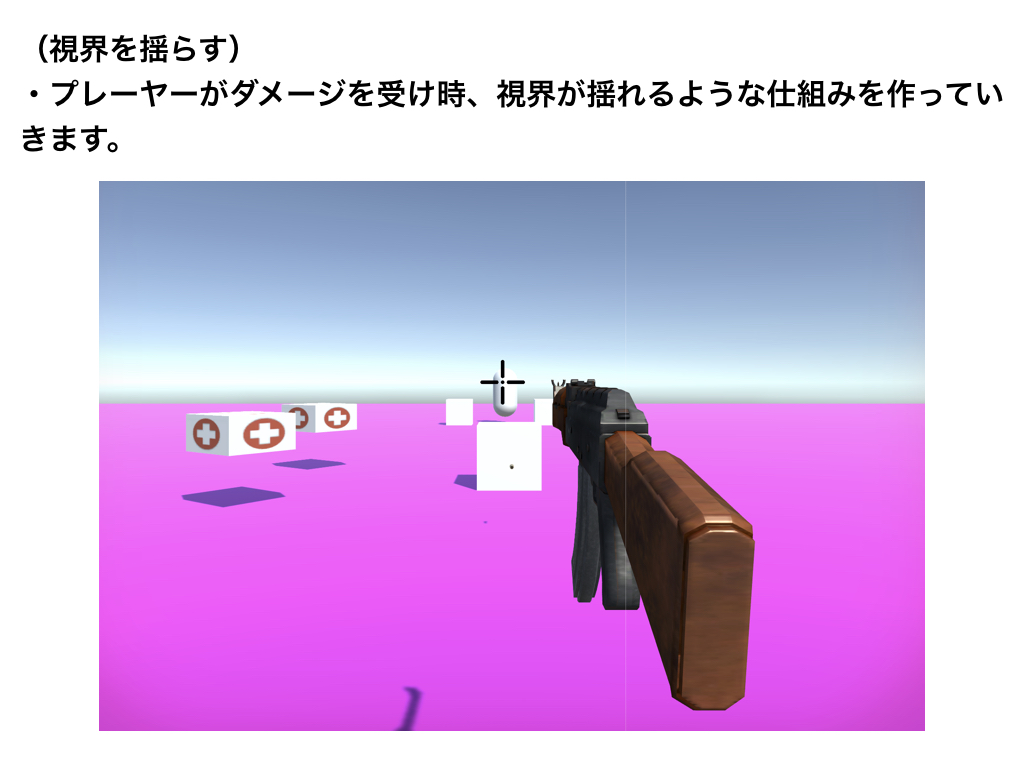
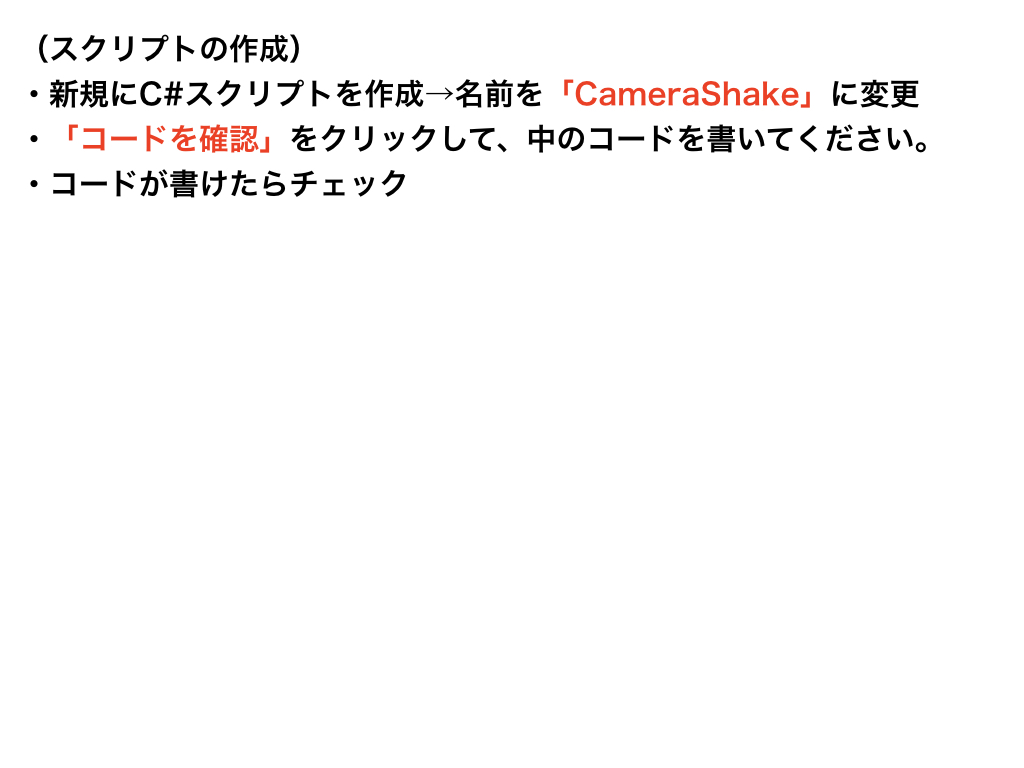
視界を揺らす
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CameraShake : MonoBehaviour {
public void Shake(float duration, float magnitude){
StartCoroutine(TheShake(duration, magnitude));
}
private IEnumerator TheShake(float duration, float magnitude){
Vector3 pos = transform.localPosition;
float elapsed = 0f; // 経過時間
while(elapsed < duration){
var x = pos.x + Random.Range(-1f, 1f) * magnitude;
var y = pos.y + Random.Range(-1f, 1f) * magnitude;
transform.localPosition = new Vector3(x, y, pos.z);
elapsed += Time.deltaTime;
yield return null;
}
transform.localPosition = pos;
}
}
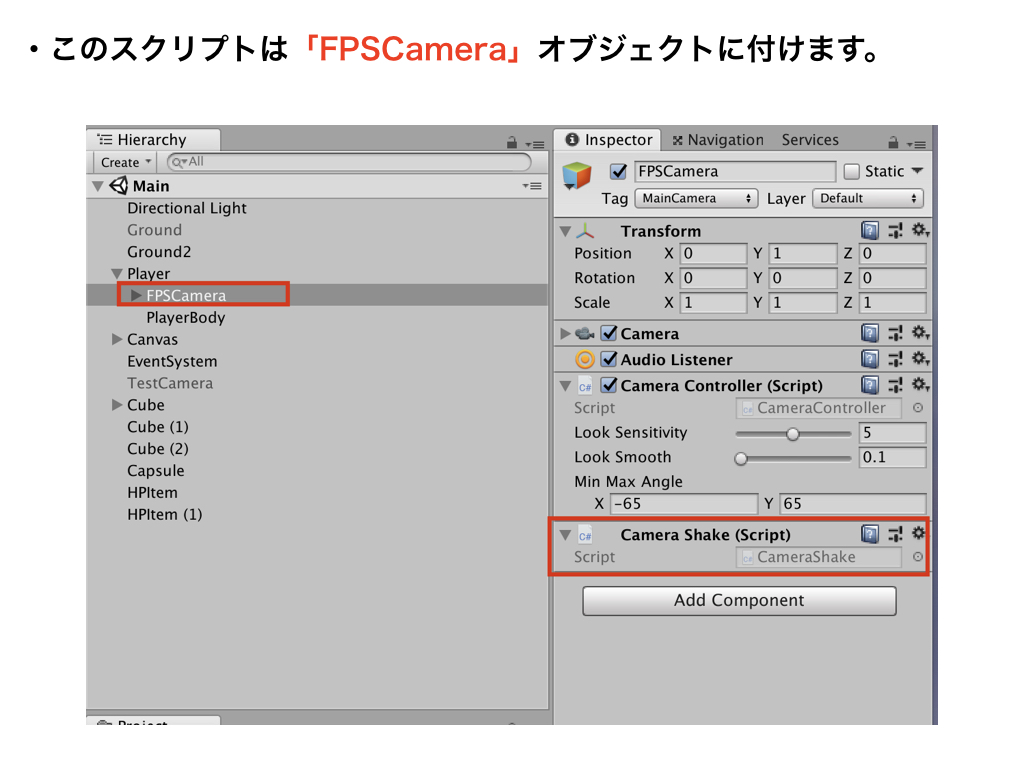
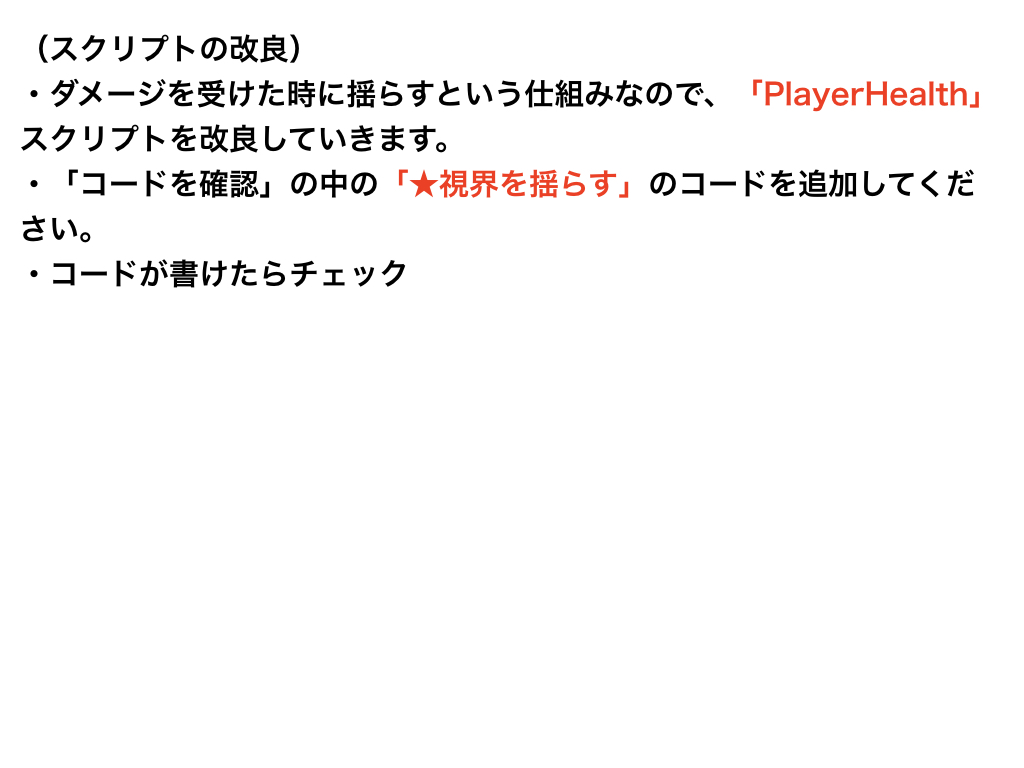
視界を揺らすを実行する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class PlayerHealth : MonoBehaviour {
public int playerHP;
public AudioClip damageSound;
private Image damageScreenImage;
private Color color;
// ★視界を揺らす
private CameraShake shake;
void Start(){
damageScreenImage = GameObject.Find("DamageScreen").GetComponent<Image>();
color = damageScreenImage.color;
color.a = 0.0f;
damageScreenImage.color = color;
// ★視界を揺らす
shake = GameObject.Find("FPSCamera").GetComponent<CameraShake>();
}
void OnCollisionEnter(Collision other){
if(other.gameObject.tag == "EnemyBullet"){
playerHP -= 1;
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(damageSound, Camera.main.transform.position);
color.a += 0.1f;
damageScreenImage.color = color;
// ★視界を揺らす
shake.Shake(0.2f, 0.1f);
}
}
public void AddHP(int amount){
playerHP += amount;
if(playerHP > 10){
playerHP = 10;
}
float amountF = (float)amount;
color.a -= (amountF / 10);
if(color.a < 0){
color.a = 0;
}
damageScreenImage.color = color;
}
}
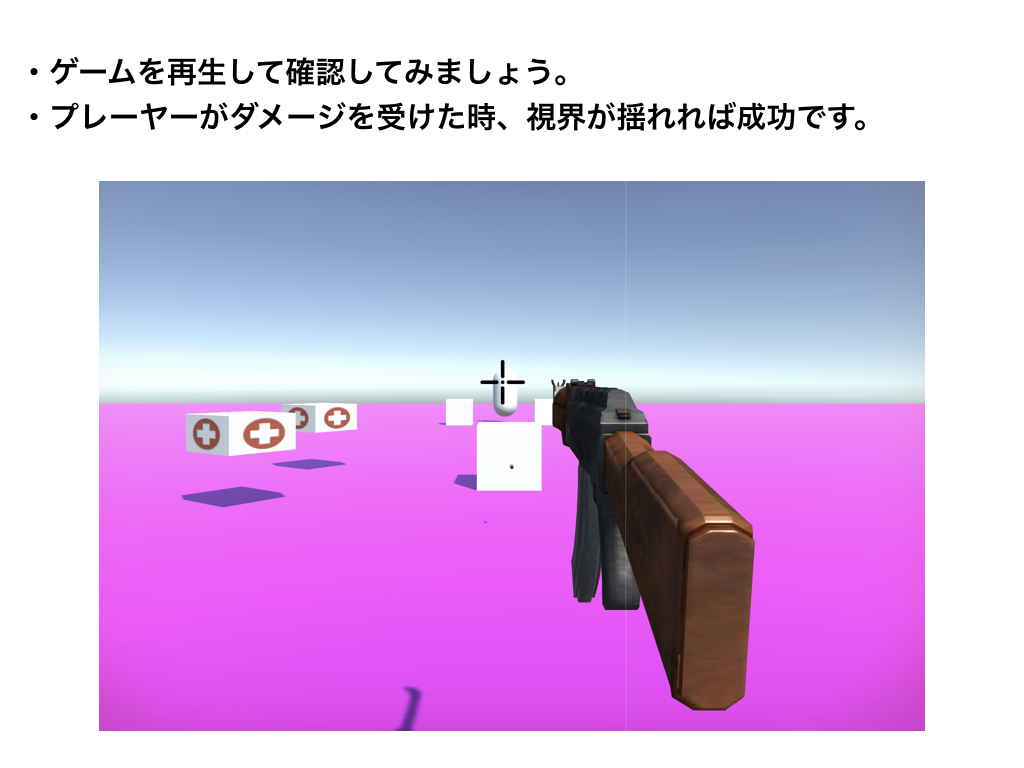
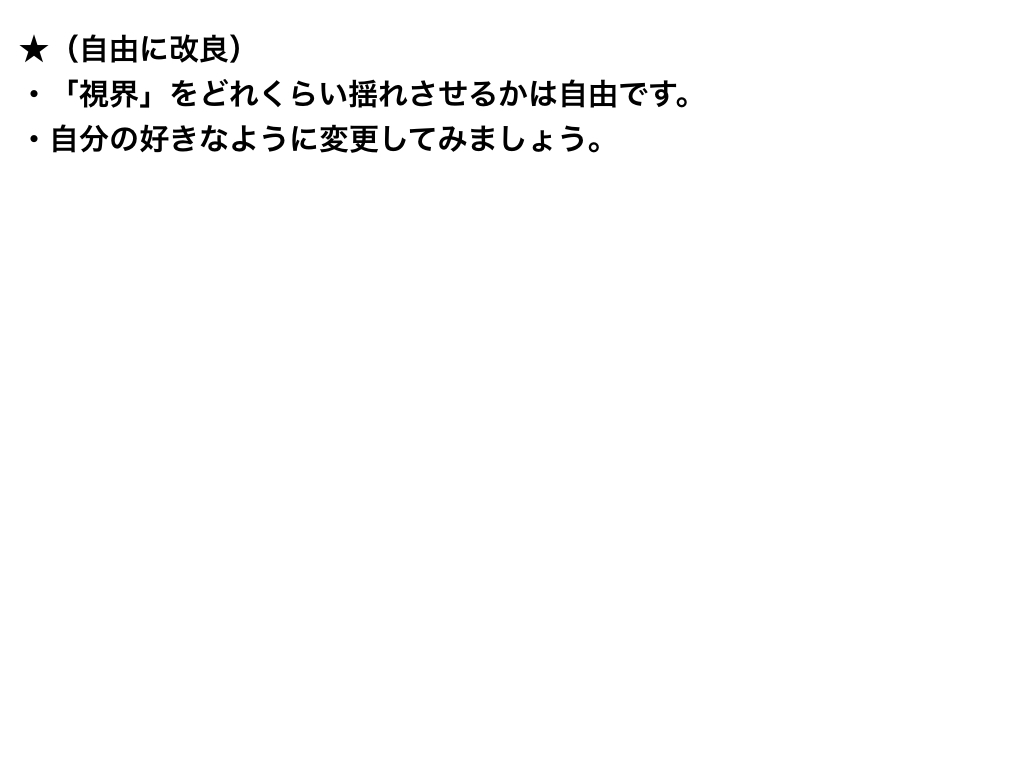
プレーヤーがダメージを受けたら視界を揺らす