プレーヤーをジャンプさせる
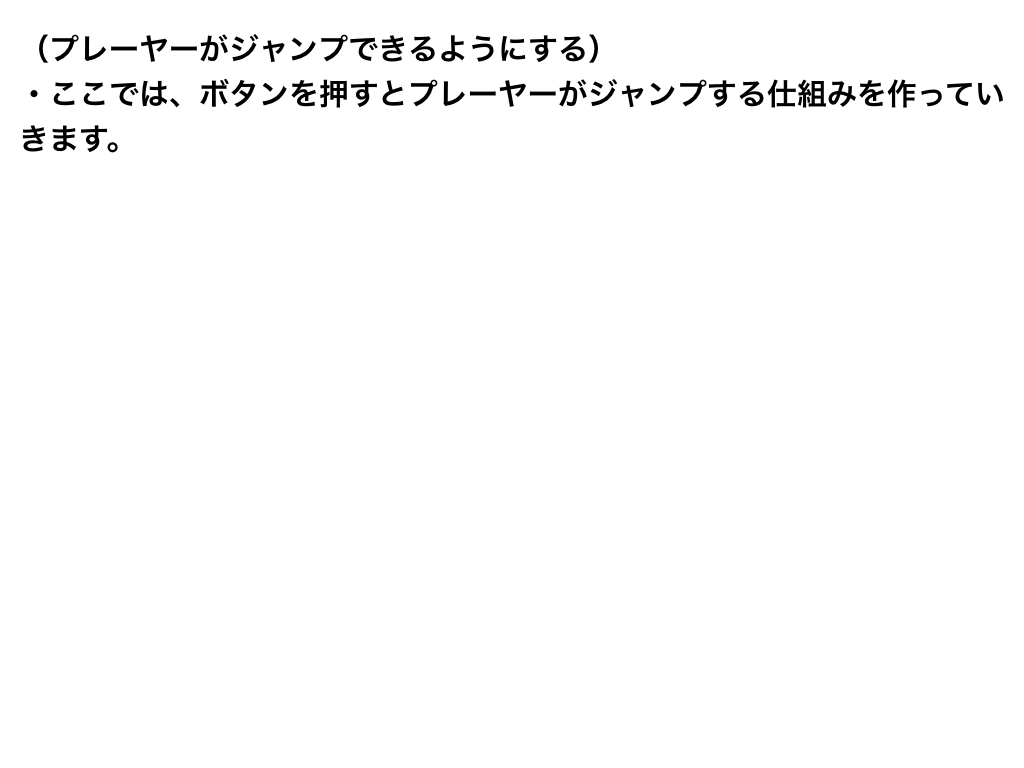
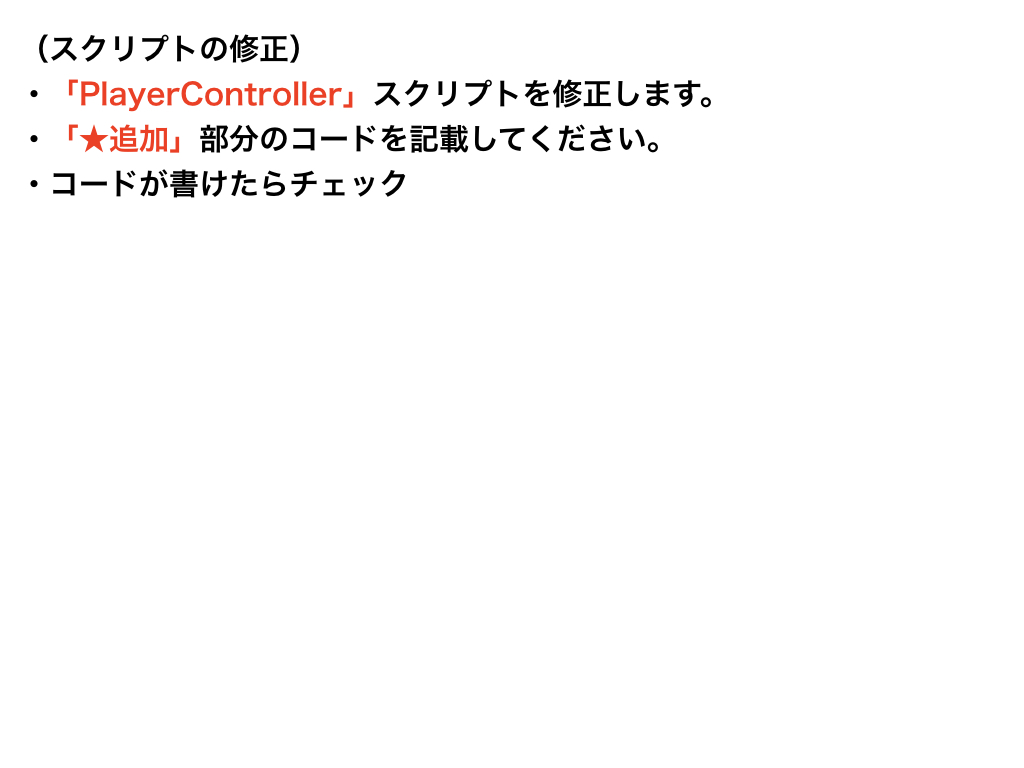
プレーヤーをジャンプさせる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
namespace FPS{
public enum PlayerState{
Idle, Walking, Running, Jumping
}
[RequireComponent(typeof(CharacterController), typeof(AudioSource))]
public class PlayerController : MonoBehaviour {
[Range(0.1f, 2f)]
public float walkSpeed = 1.5f;
[Range(0.1f, 10f)]
public float runSpeed = 3.5f;
private CharacterController charaController;
private GameObject FPSCamera;
private Vector3 moveDir = Vector3.zero;
// ★追加
[Range(0.1f, 10f)]
public float gravity = 9.8f; // 重力の大きさ
[Range(1f, 15f)]
public float jumpPower = 10f; // ジャンプ力
void Start () {
FPSCamera = GameObject.Find("FPSCamera");
charaController = GetComponent<CharacterController> ();
}
void Update () {
Move ();
}
void Move(){
float moveH = Input.GetAxis ("Horizontal");
float moveV = Input.GetAxis ("Vertical");
Vector3 movement = new Vector3 (moveH, 0, moveV);
if (movement.sqrMagnitude > 1) {
movement.Normalize ();
}
Vector3 desiredMove = FPSCamera.transform.forward * movement.z + FPSCamera.transform.right * movement.x;
moveDir.x = desiredMove.x * 5f;
moveDir.z = desiredMove.z * 5f;
if (Input.GetKey (KeyCode.LeftShift)) {
charaController.Move (moveDir * Time.fixedDeltaTime * runSpeed);
} else {
charaController.Move (moveDir * Time.fixedDeltaTime * walkSpeed);
}
// ★追加
moveDir.y -= gravity * Time.deltaTime; // 重力の発生
// ★追加
if(charaController.isGrounded){ // この条件の意味を調べてみよう
if(Input.GetKeyDown(KeyCode.Space)){
moveDir.y = jumpPower; // ジャンプ
}
}
}
}
}
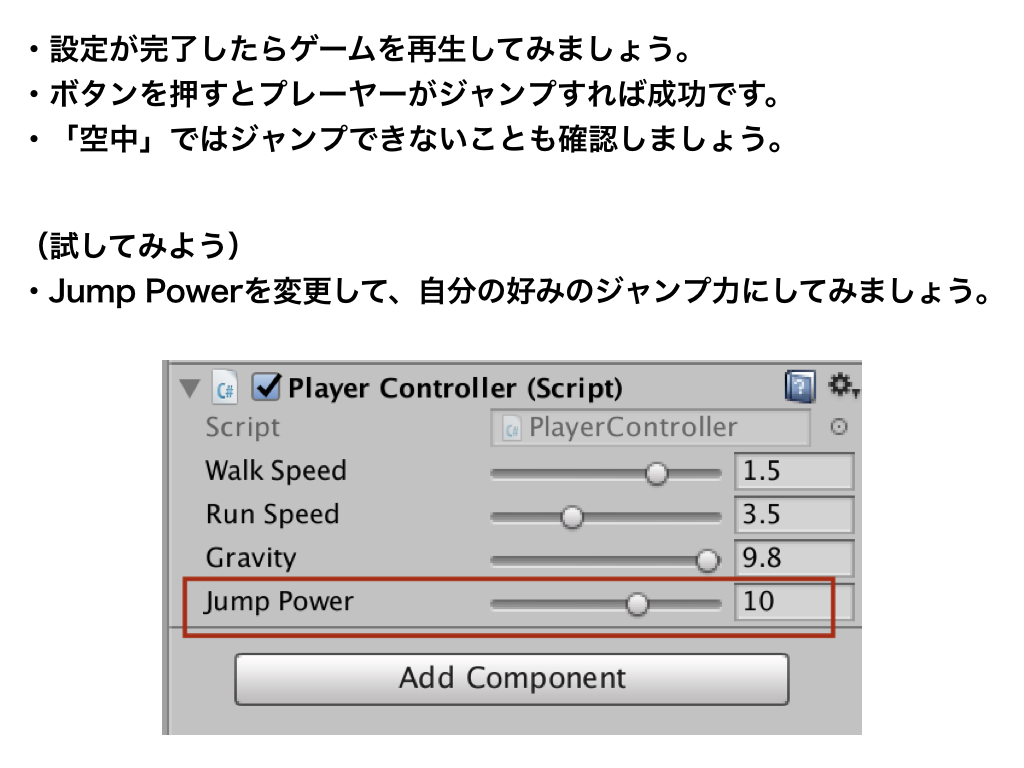
EscapeCombat
他のコースを見る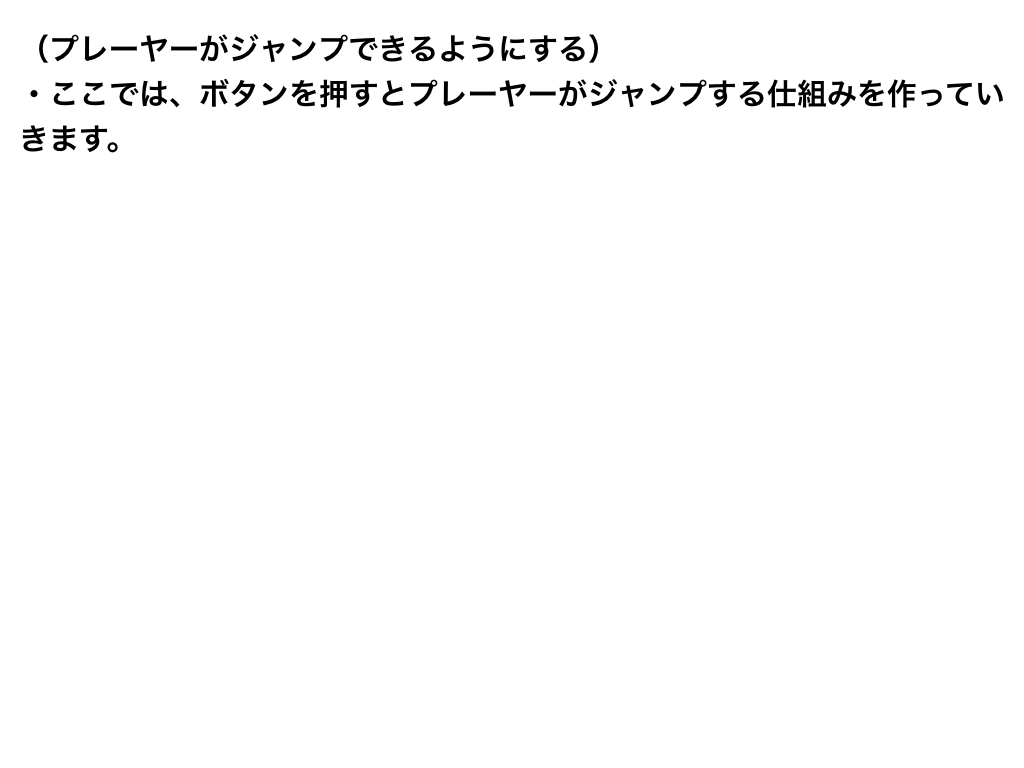
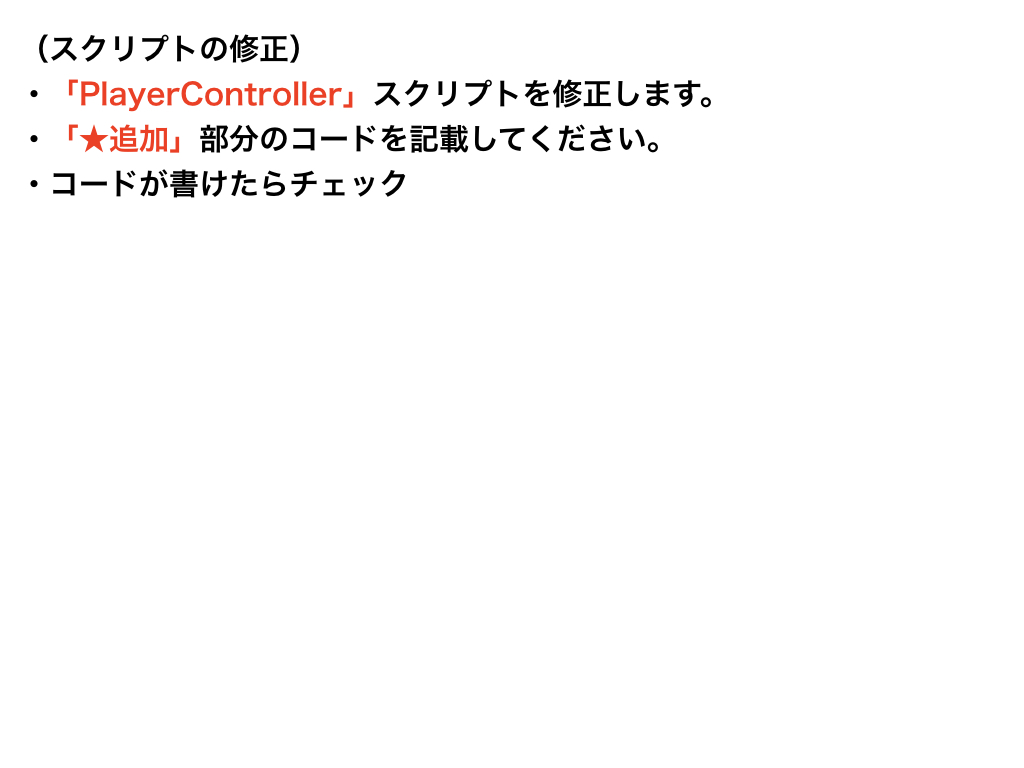
プレーヤーをジャンプさせる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
namespace FPS{
public enum PlayerState{
Idle, Walking, Running, Jumping
}
[RequireComponent(typeof(CharacterController), typeof(AudioSource))]
public class PlayerController : MonoBehaviour {
[Range(0.1f, 2f)]
public float walkSpeed = 1.5f;
[Range(0.1f, 10f)]
public float runSpeed = 3.5f;
private CharacterController charaController;
private GameObject FPSCamera;
private Vector3 moveDir = Vector3.zero;
// ★追加
[Range(0.1f, 10f)]
public float gravity = 9.8f; // 重力の大きさ
[Range(1f, 15f)]
public float jumpPower = 10f; // ジャンプ力
void Start () {
FPSCamera = GameObject.Find("FPSCamera");
charaController = GetComponent<CharacterController> ();
}
void Update () {
Move ();
}
void Move(){
float moveH = Input.GetAxis ("Horizontal");
float moveV = Input.GetAxis ("Vertical");
Vector3 movement = new Vector3 (moveH, 0, moveV);
if (movement.sqrMagnitude > 1) {
movement.Normalize ();
}
Vector3 desiredMove = FPSCamera.transform.forward * movement.z + FPSCamera.transform.right * movement.x;
moveDir.x = desiredMove.x * 5f;
moveDir.z = desiredMove.z * 5f;
if (Input.GetKey (KeyCode.LeftShift)) {
charaController.Move (moveDir * Time.fixedDeltaTime * runSpeed);
} else {
charaController.Move (moveDir * Time.fixedDeltaTime * walkSpeed);
}
// ★追加
moveDir.y -= gravity * Time.deltaTime; // 重力の発生
// ★追加
if(charaController.isGrounded){ // この条件の意味を調べてみよう
if(Input.GetKeyDown(KeyCode.Space)){
moveDir.y = jumpPower; // ジャンプ
}
}
}
}
}
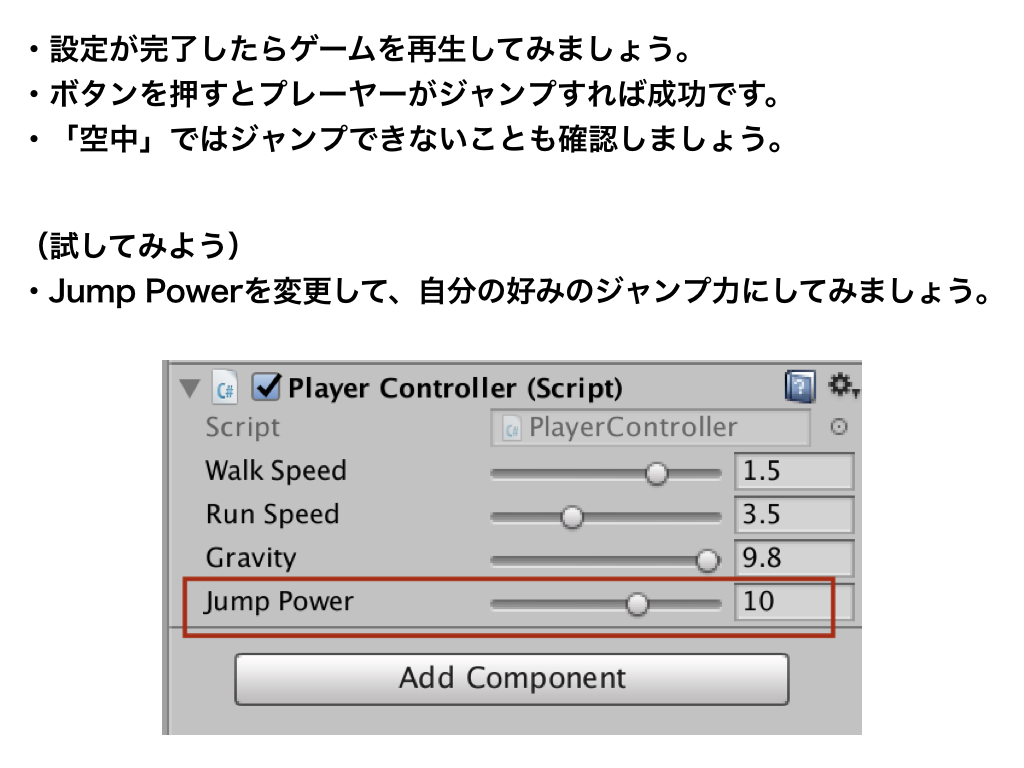
プレーヤーをジャンプさせる