HPをスライダーで表示する
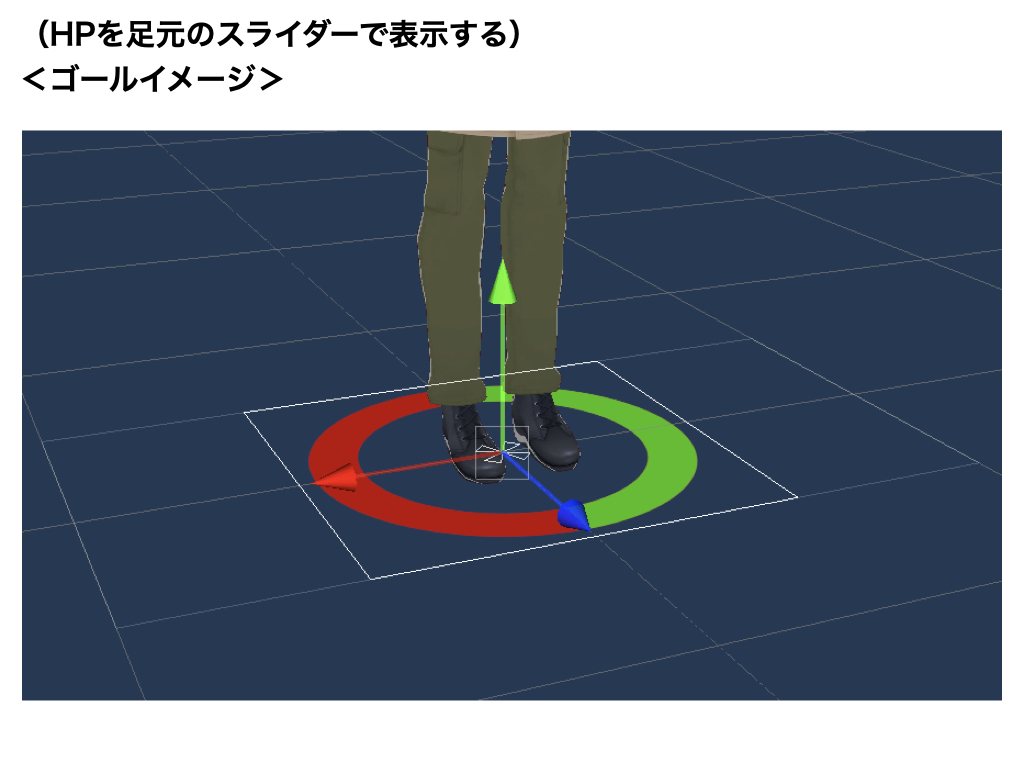
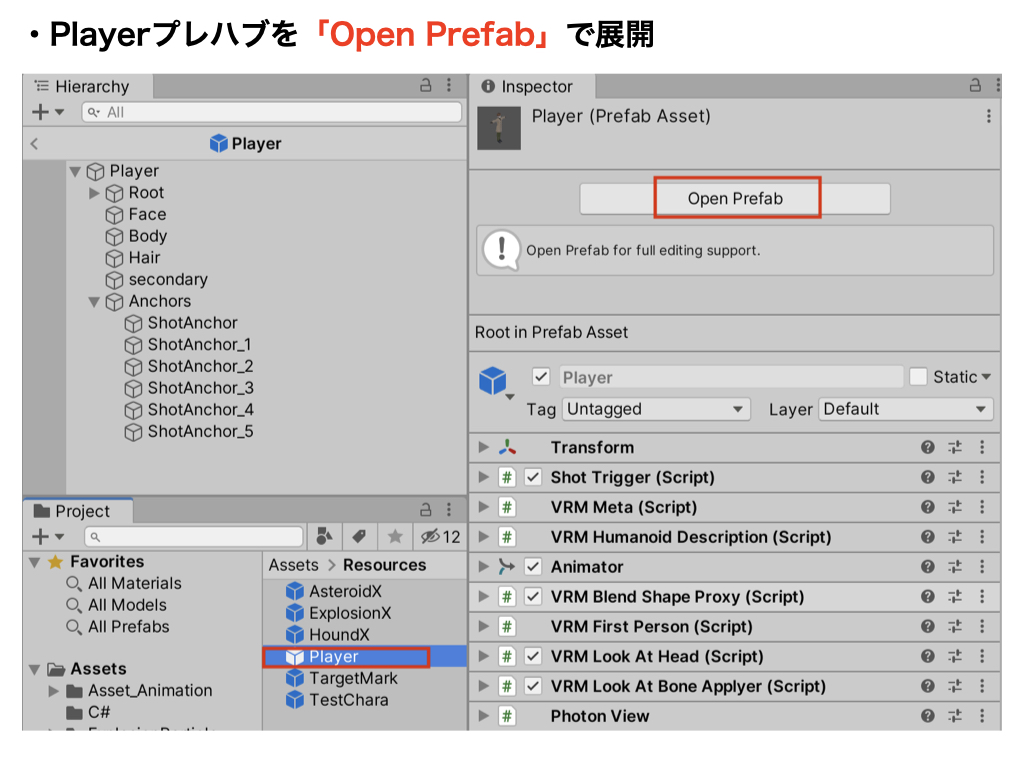
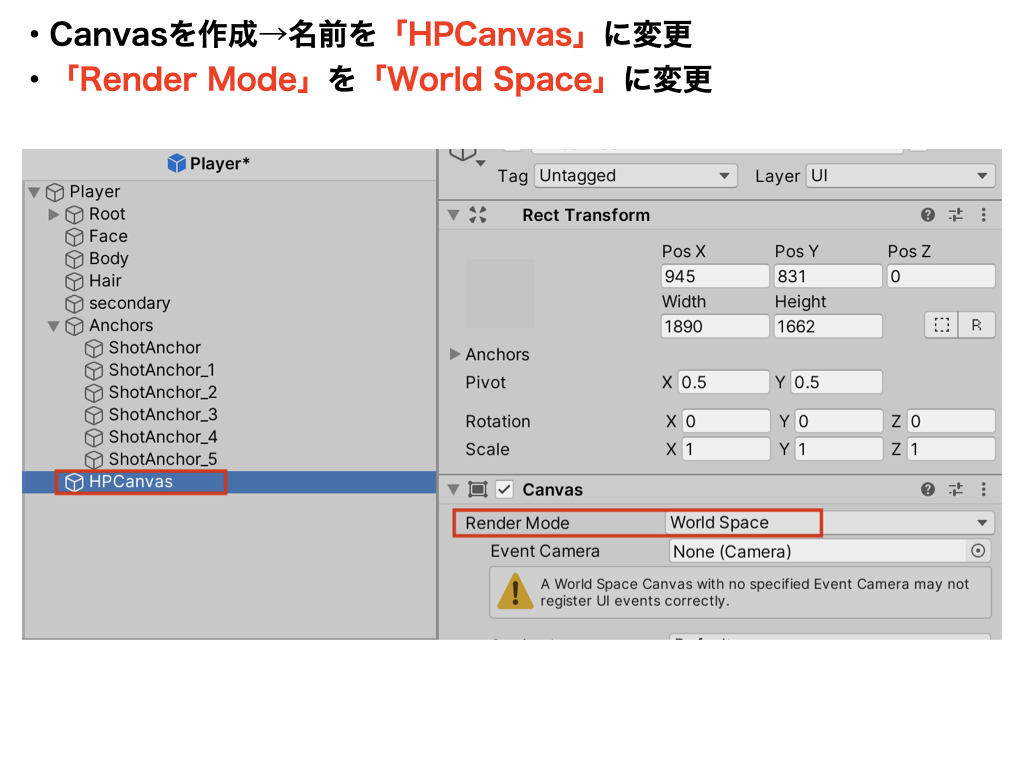
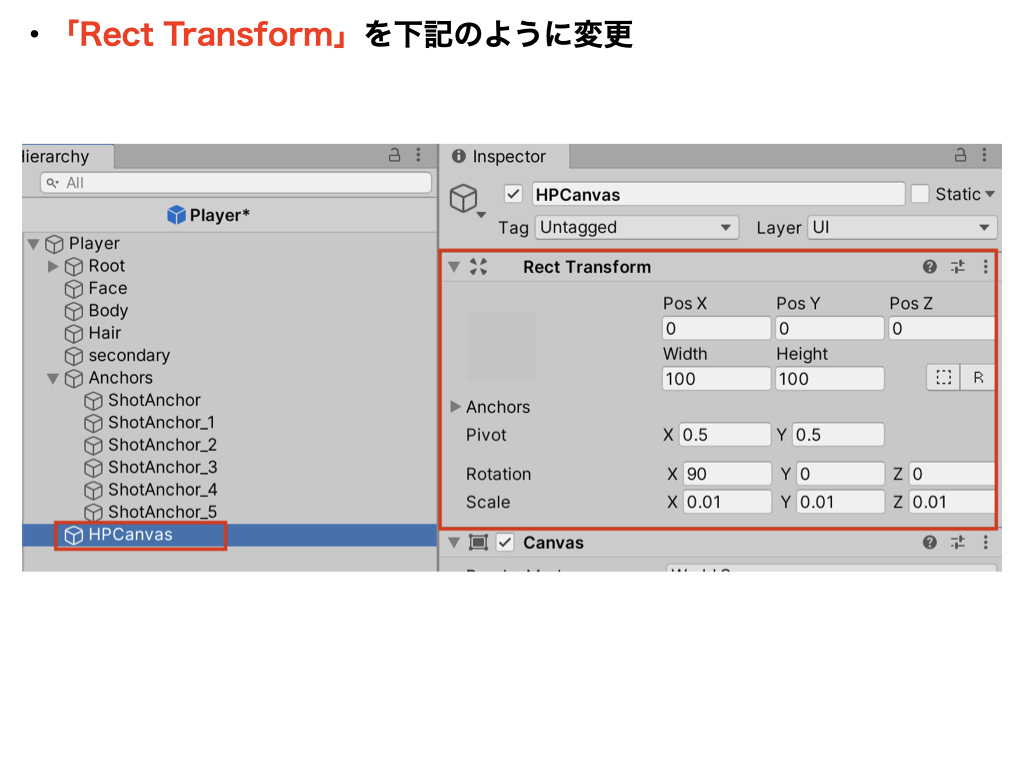
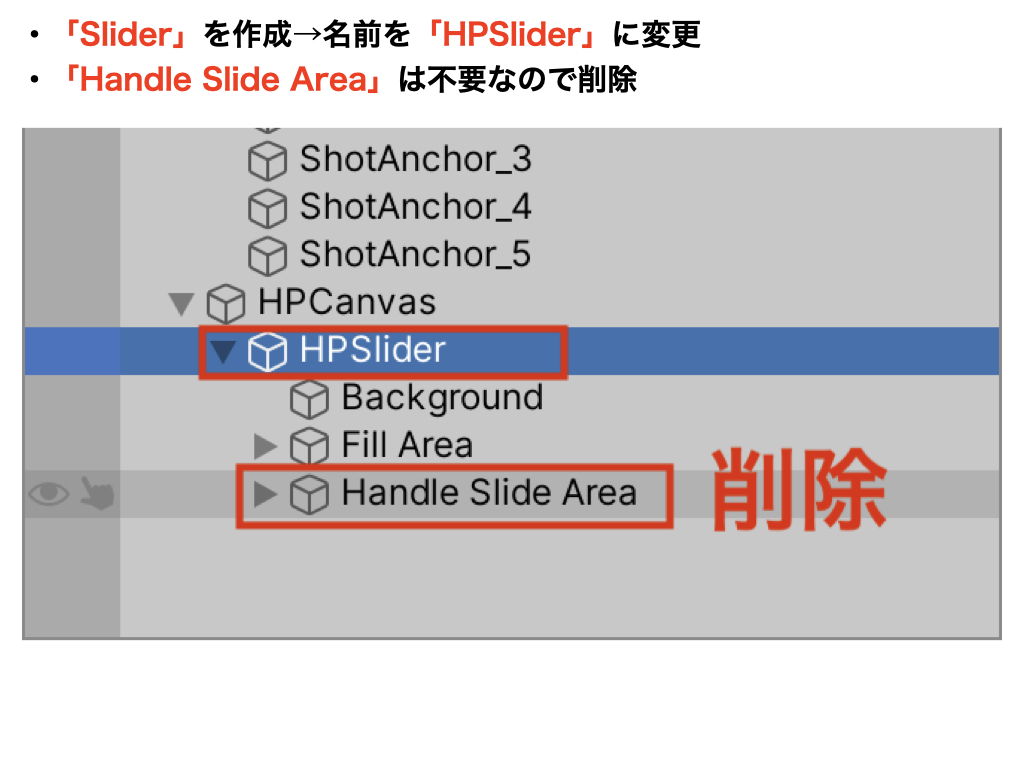
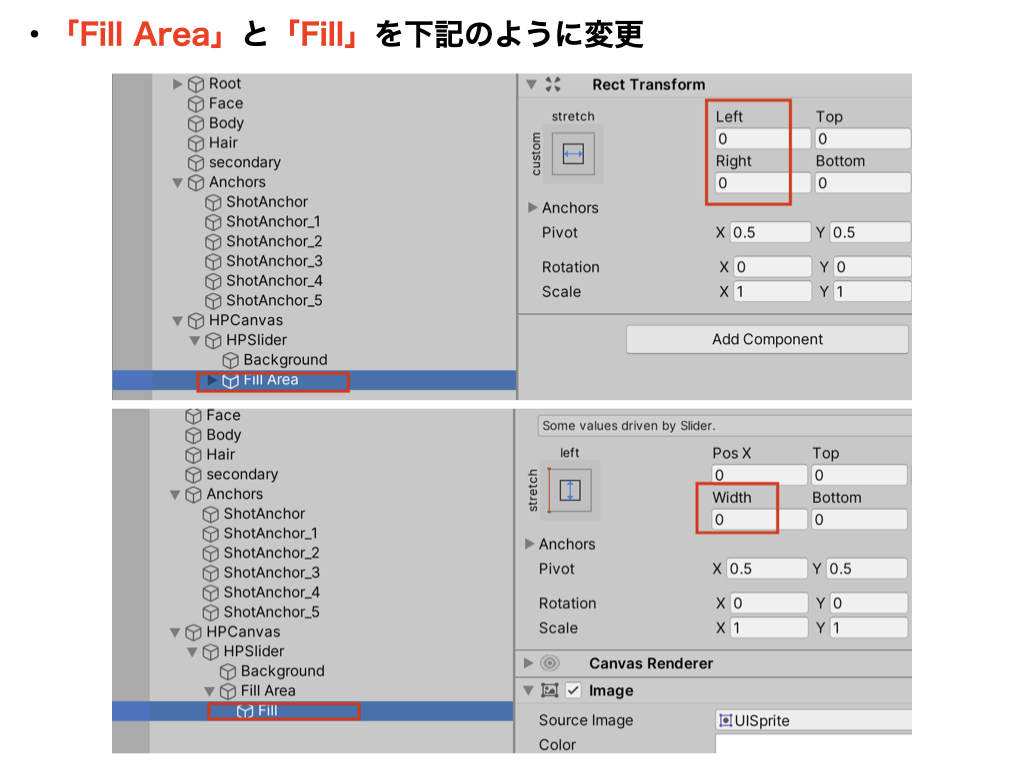
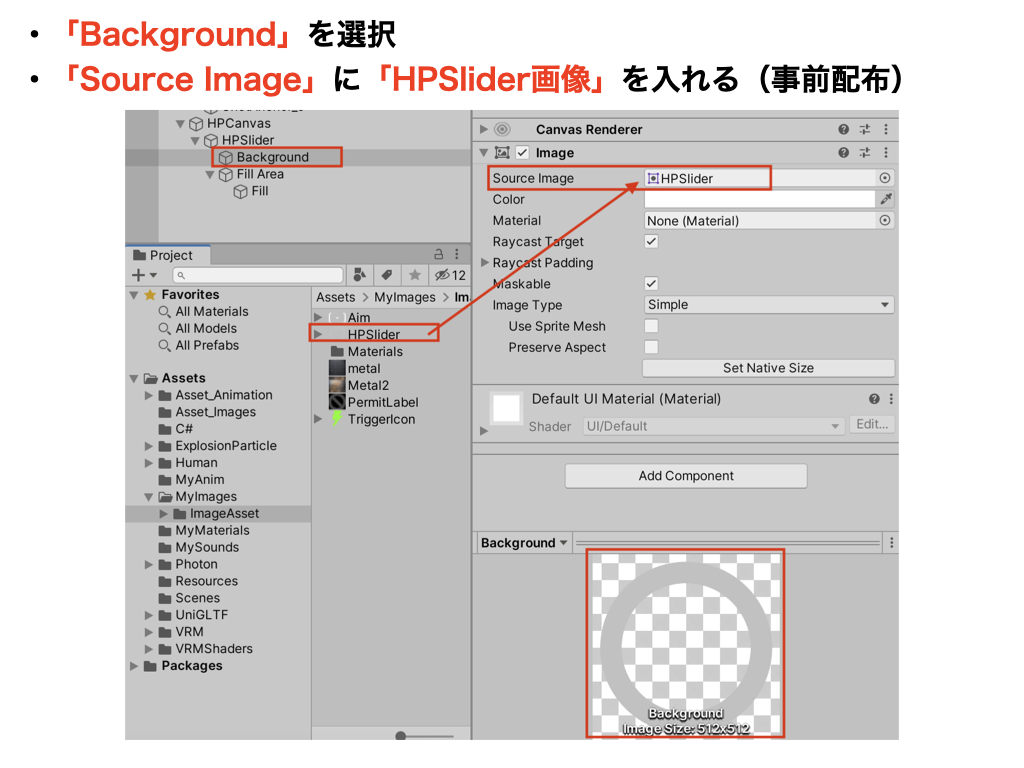
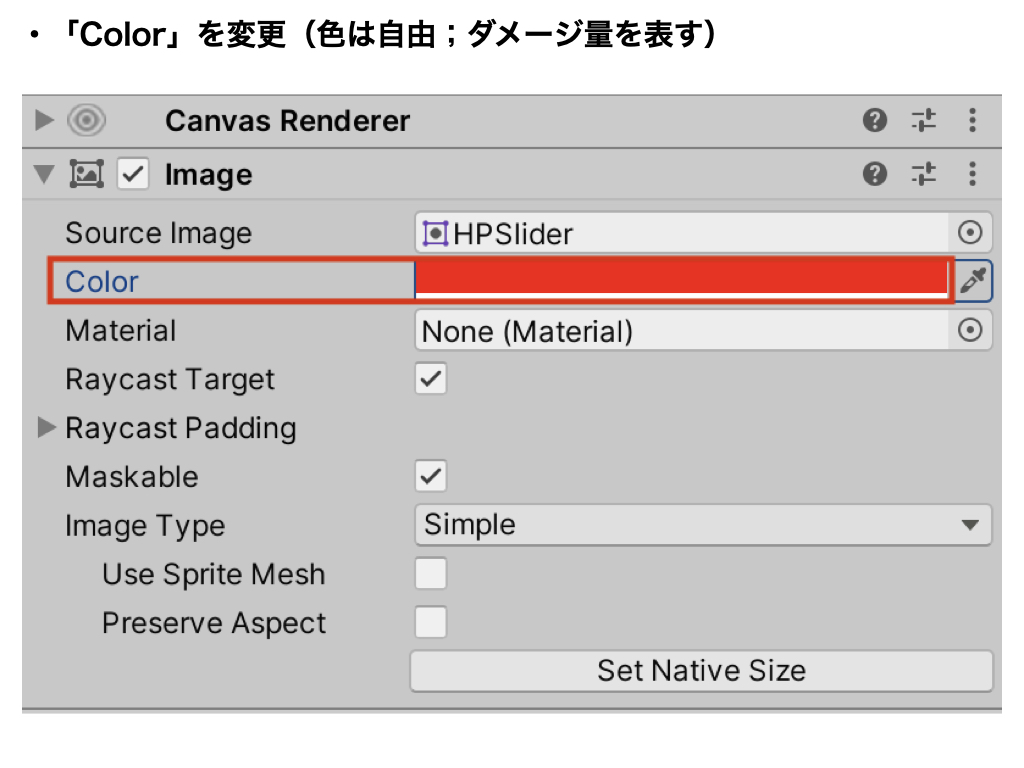
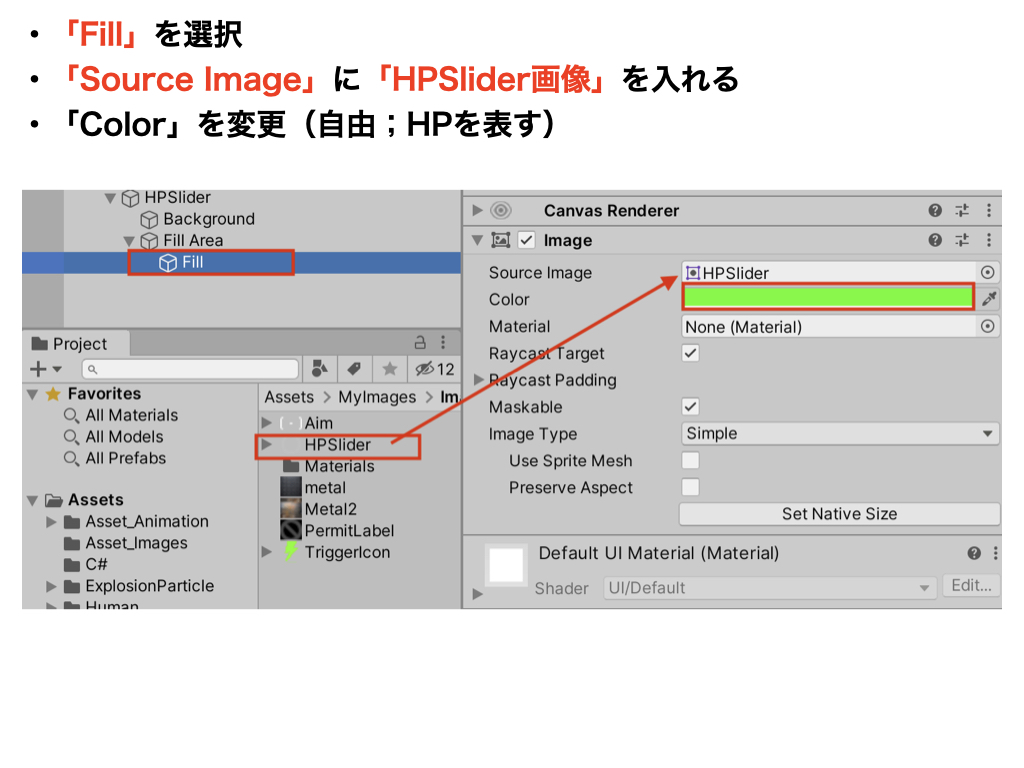
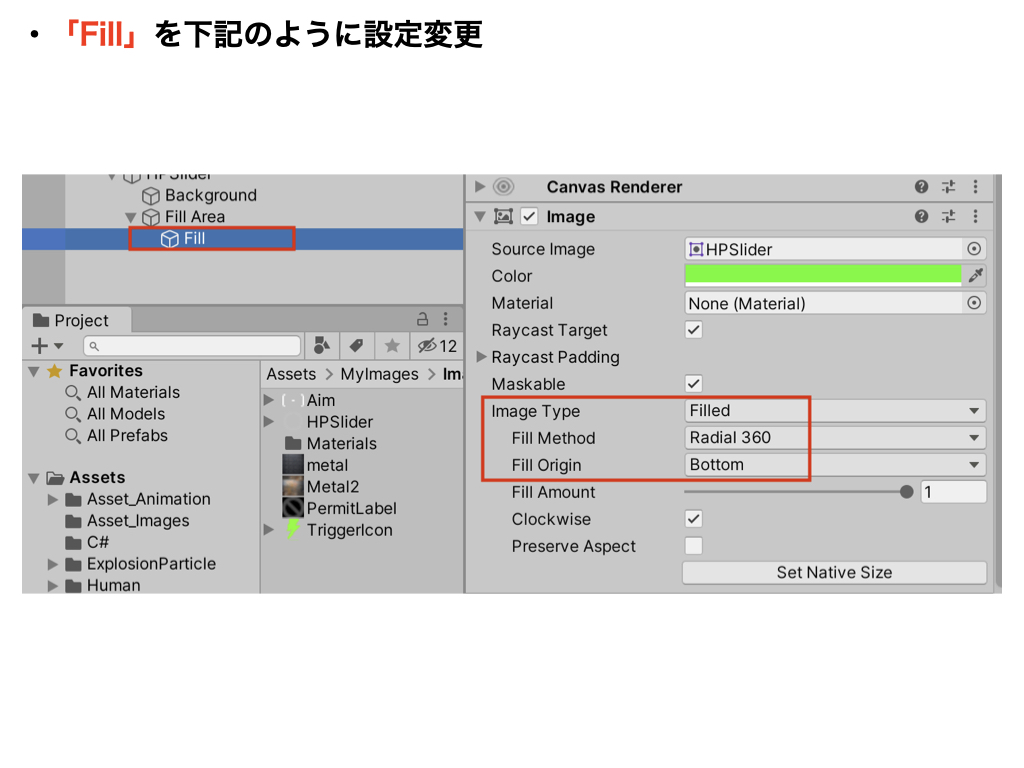
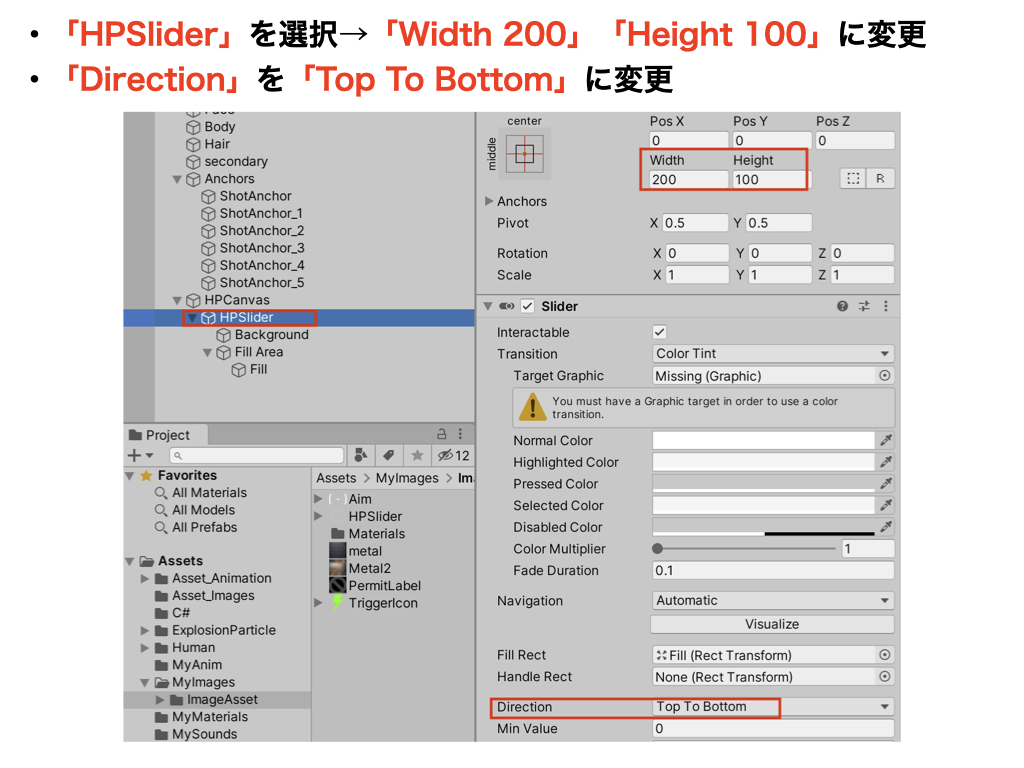
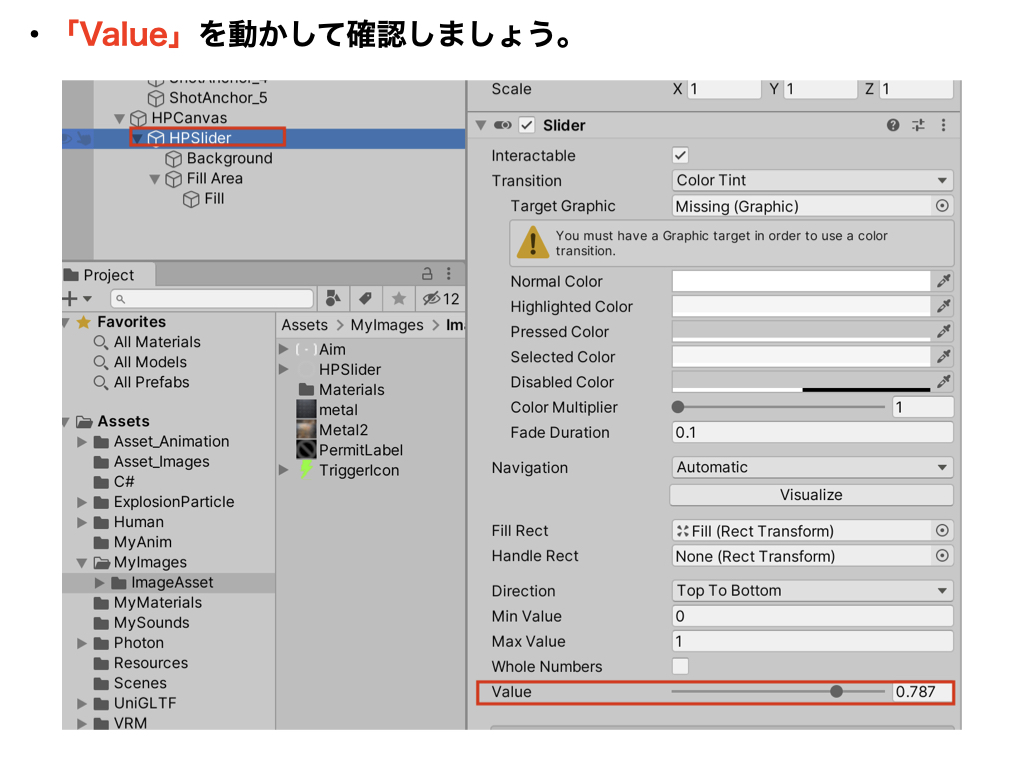
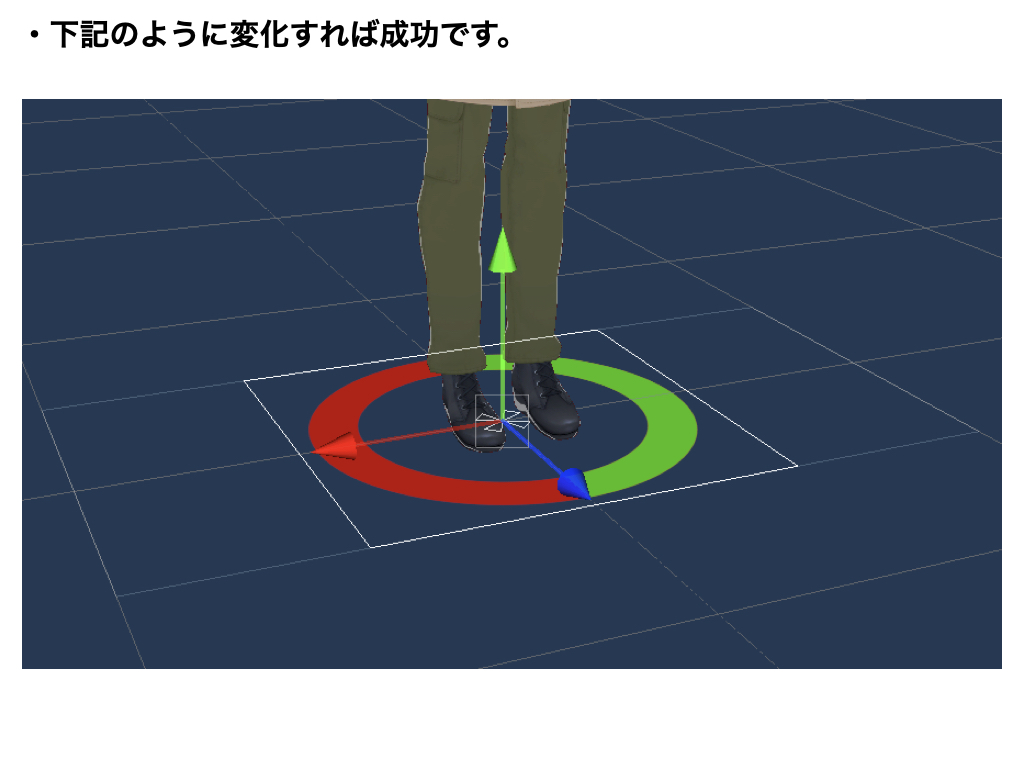
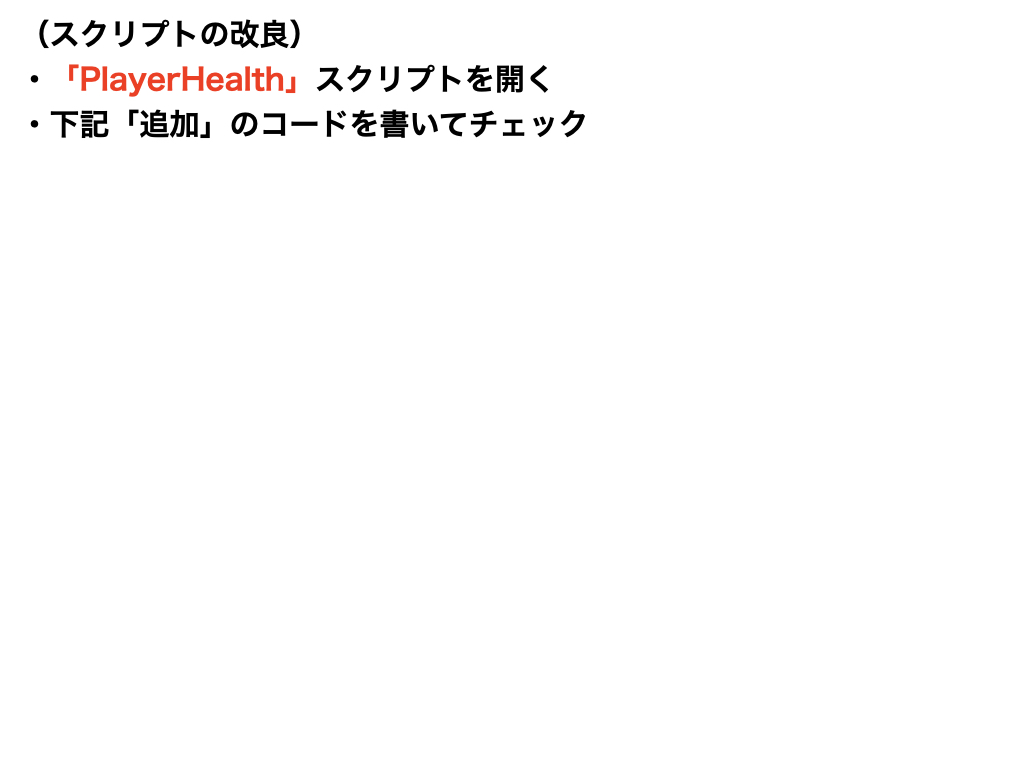
HPをスライダーで表示する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Photon.Pun;
// ★追加
using UnityEngine.UI;
public class PlayerHealth : MonoBehaviourPunCallbacks
{
private int maxHP = 10;
private int HP;
// ★追加
public Slider hpSlider;
private void Start()
{
HP = maxHP;
// ★追加
hpSlider.maxValue = HP;
hpSlider.value = HP;
}
private void OnTriggerEnter(Collider other)
{
if(other.CompareTag("Trigger"))
{
HP -= 1;
print(photonView.Owner.NickName + HP);
// ★追加
hpSlider.value = HP;
photonView.RPC("Damage", RpcTarget.Others);
}
}
// RPC→リモート・プロシージャ・コールの略称
// 相手に「ここで定義したメソッド」を「実行」してもらう仕組み
[PunRPC]
void Damage()
{
HP -= 1;
print(photonView.Owner.NickName + HP);
// ★追加
hpSlider.value = HP;
}
}
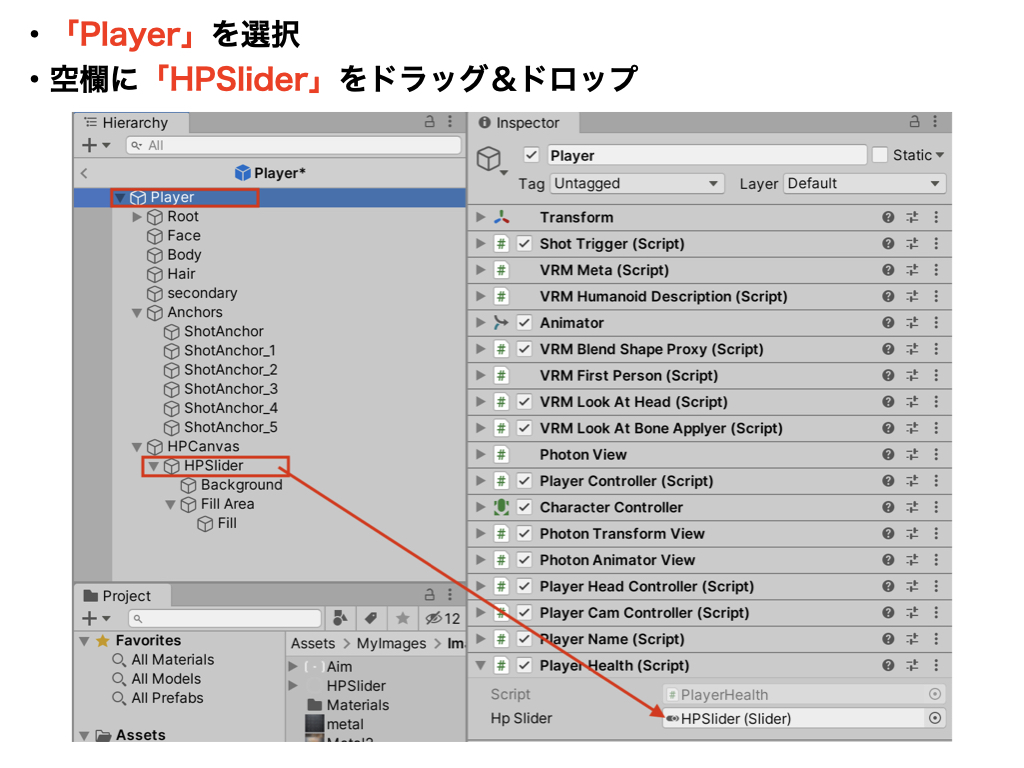
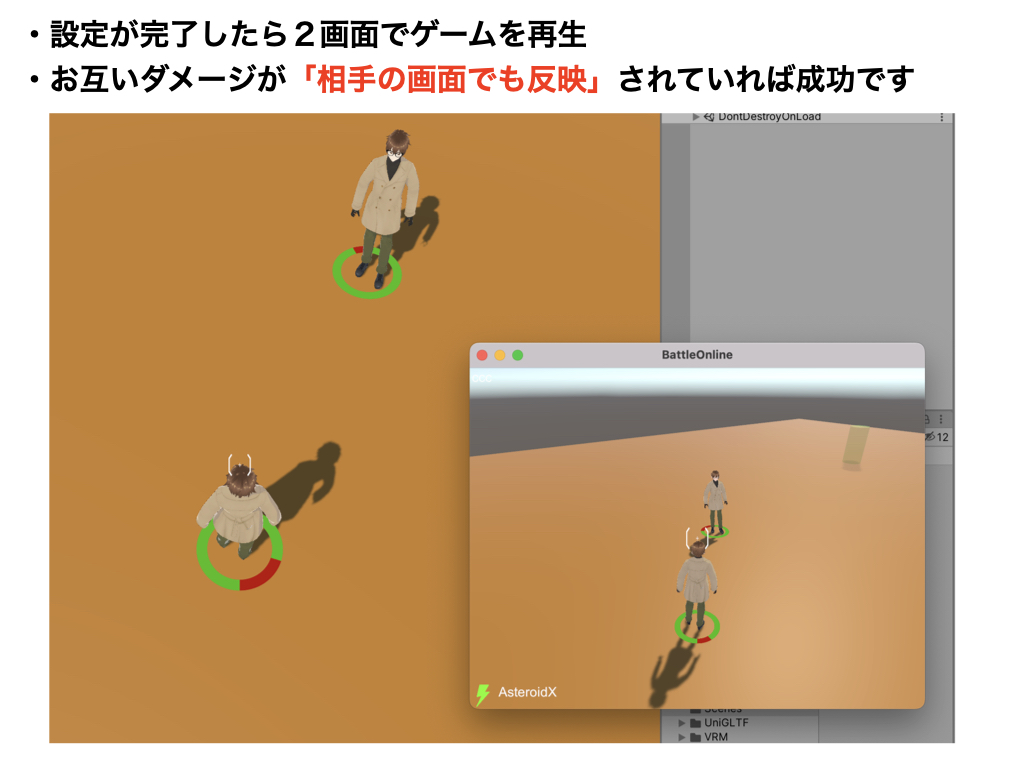
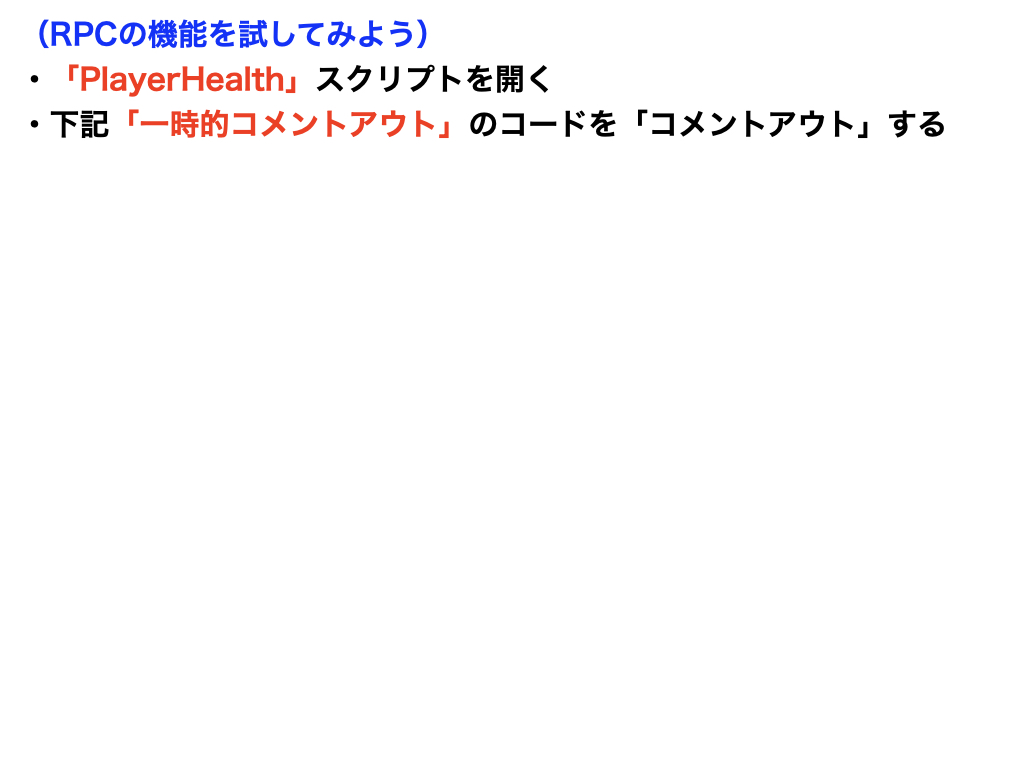
RPCの機能の確認
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Photon.Pun;
// ★追加
using UnityEngine.UI;
public class PlayerHealth : MonoBehaviourPunCallbacks
{
private int maxHP = 10;
private int HP;
// ★追加
public Slider hpSlider;
private void Start()
{
HP = maxHP;
// ★追加
hpSlider.maxValue = HP;
hpSlider.value = HP;
}
private void OnTriggerEnter(Collider other)
{
if(other.CompareTag("Trigger"))
{
HP -= 1;
print(photonView.Owner.NickName + HP);
// ★追加
hpSlider.value = HP;
// ★★一時コメントアウト(下記の1行)
//photonView.RPC("Damage", RpcTarget.Others);
}
}
// RPC→リモート・プロシージャ・コールの略称
// 相手に「ここで定義したメソッド」を「実行」してもらう仕組み
[PunRPC]
void Damage()
{
HP -= 1;
print(photonView.Owner.NickName + HP);
// ★追加
hpSlider.value = HP;
}
}
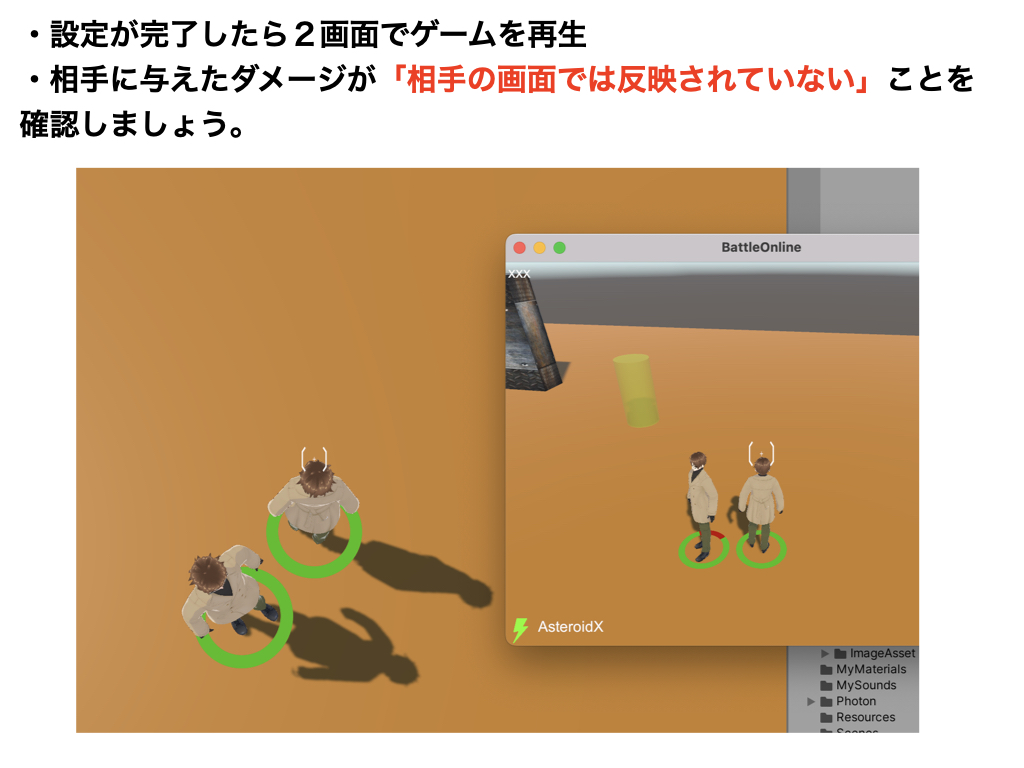
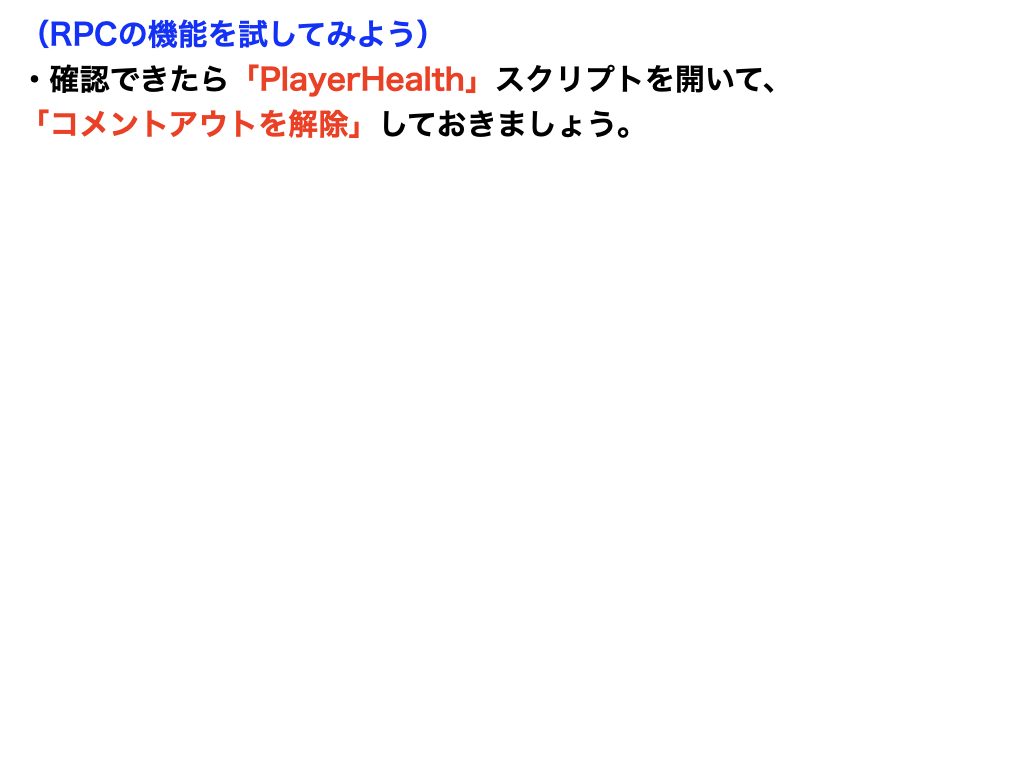
【2020版】BattleOnline(基礎/全34回)
他のコースを見る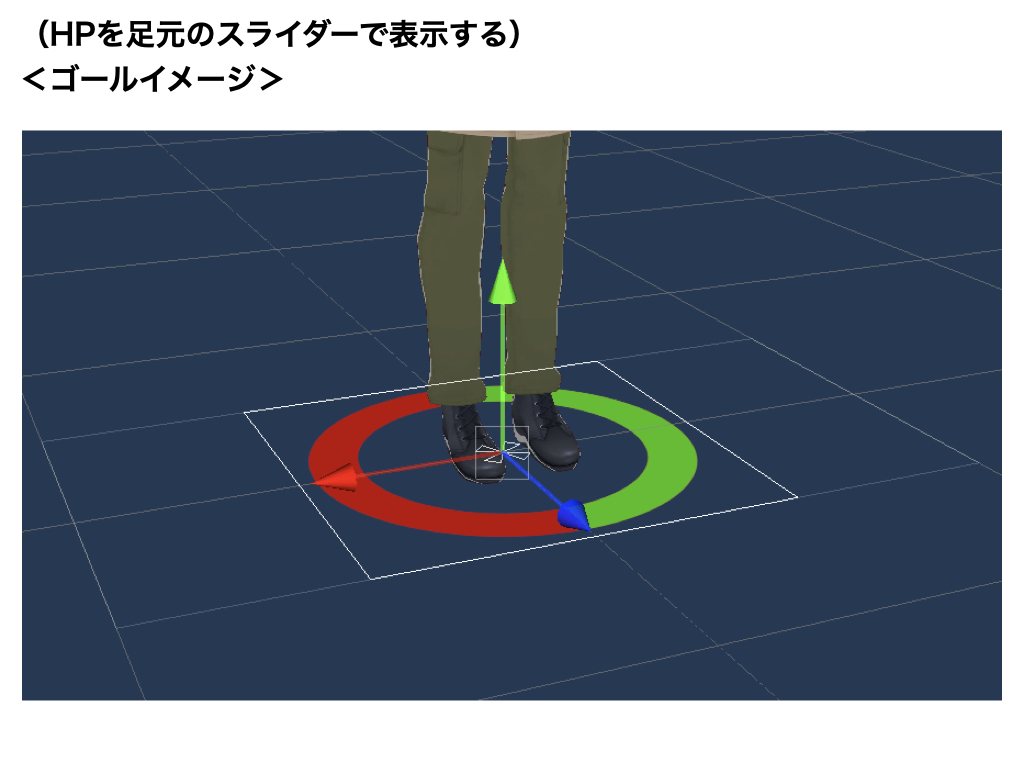
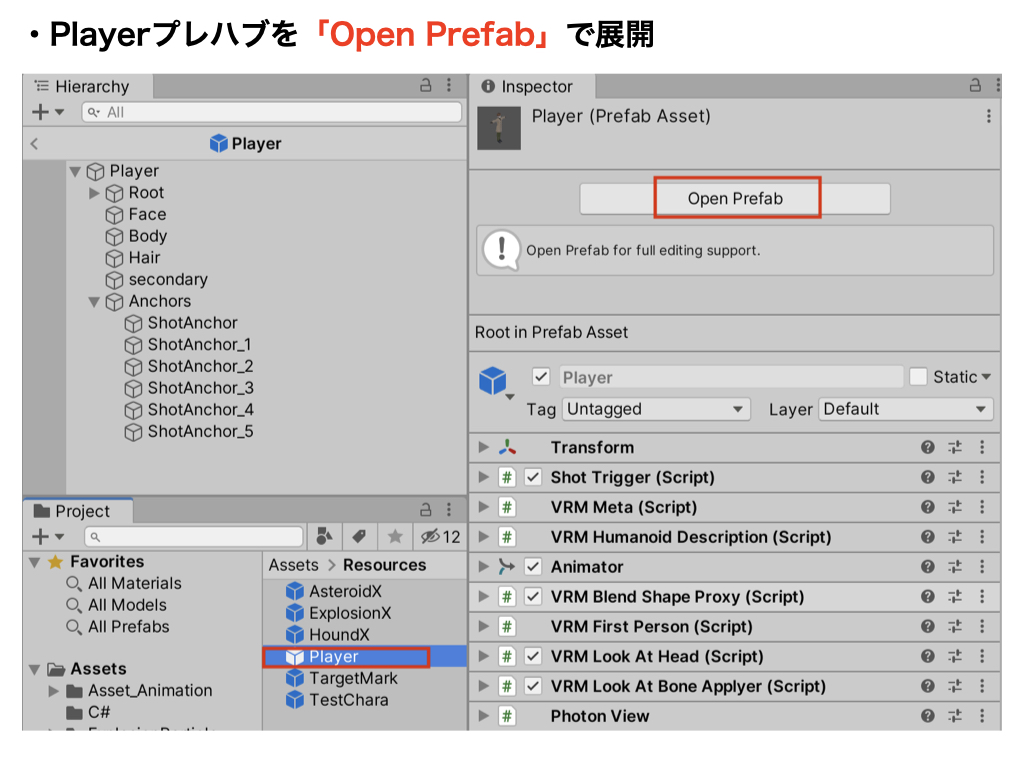
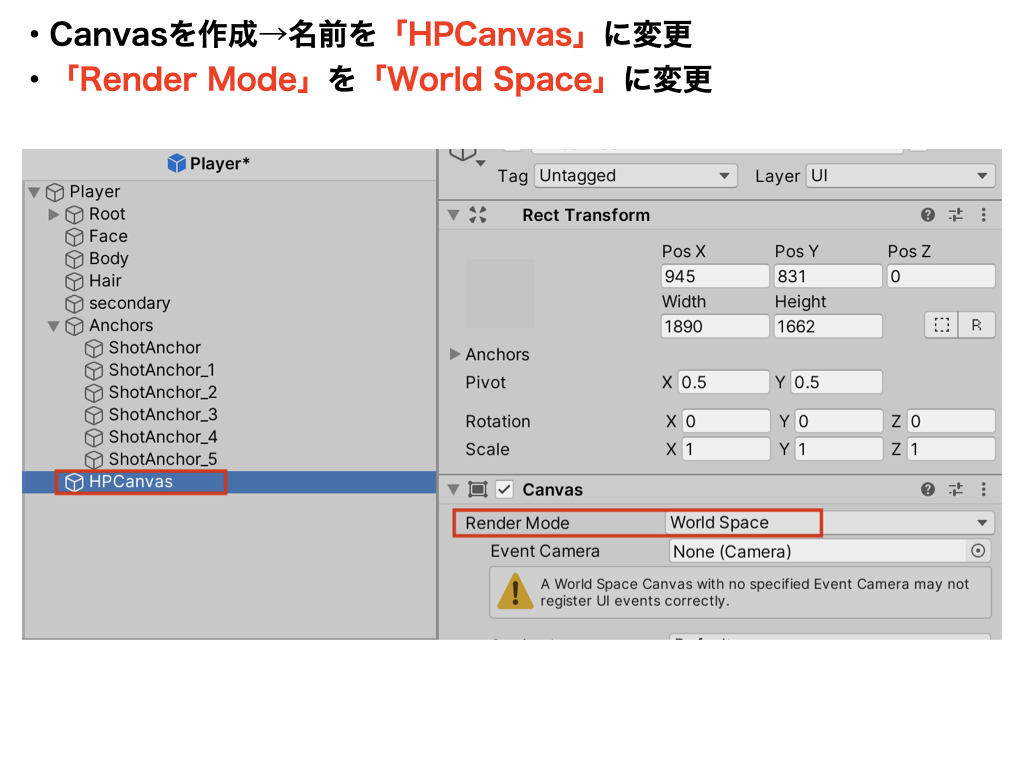
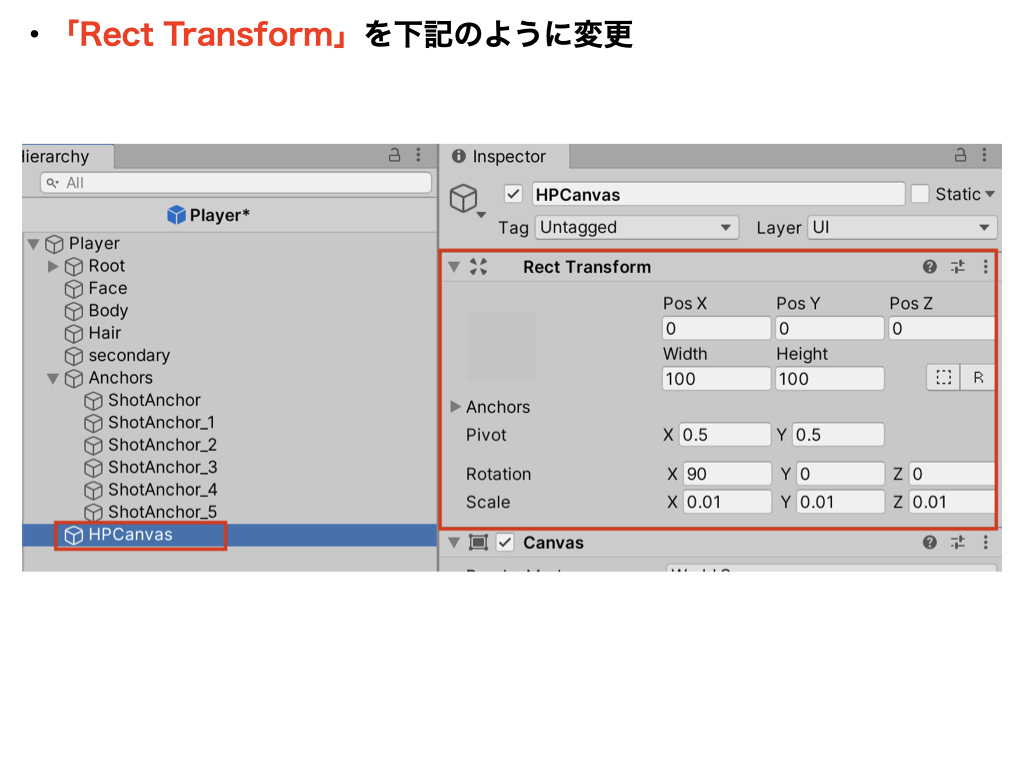
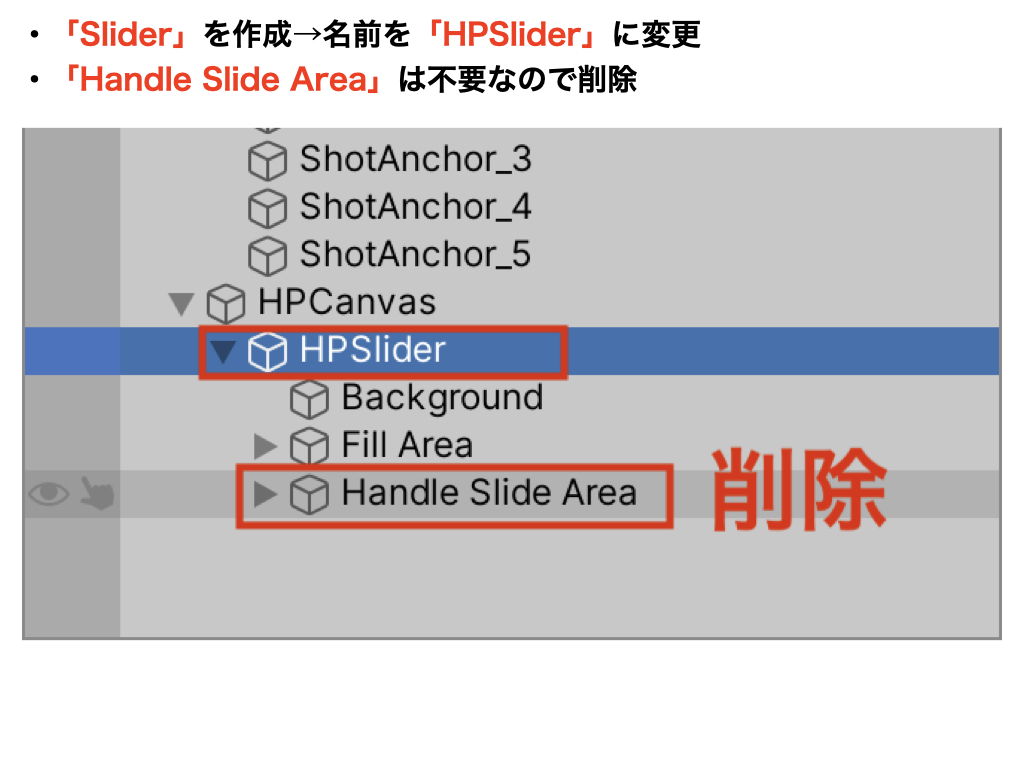
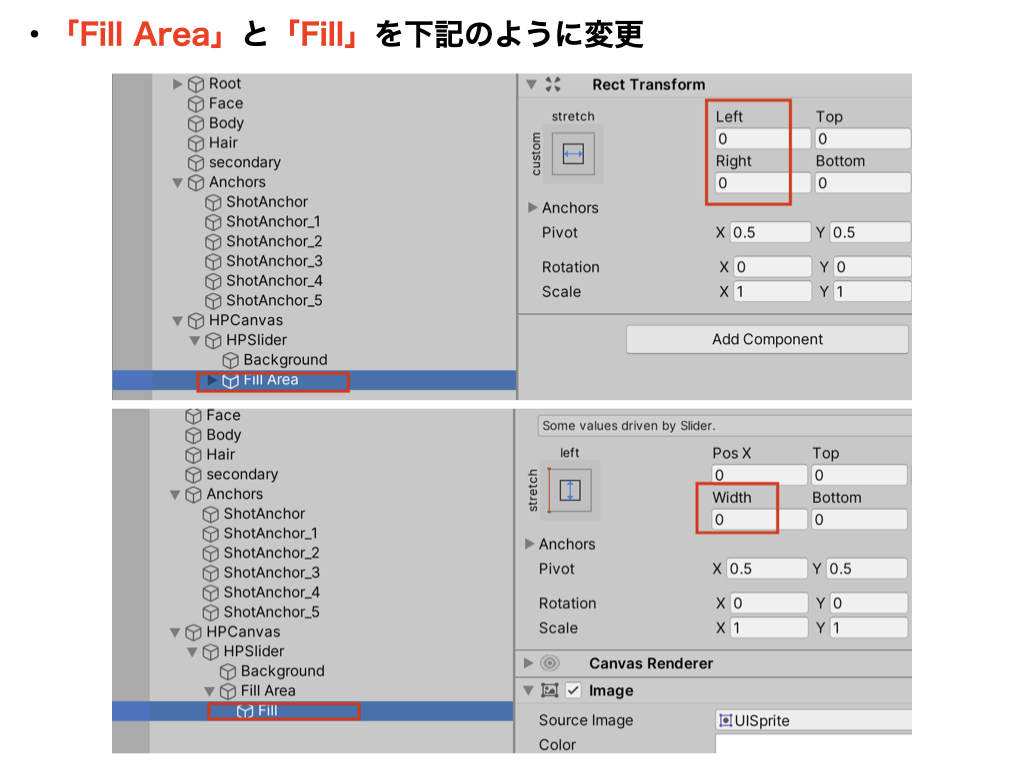
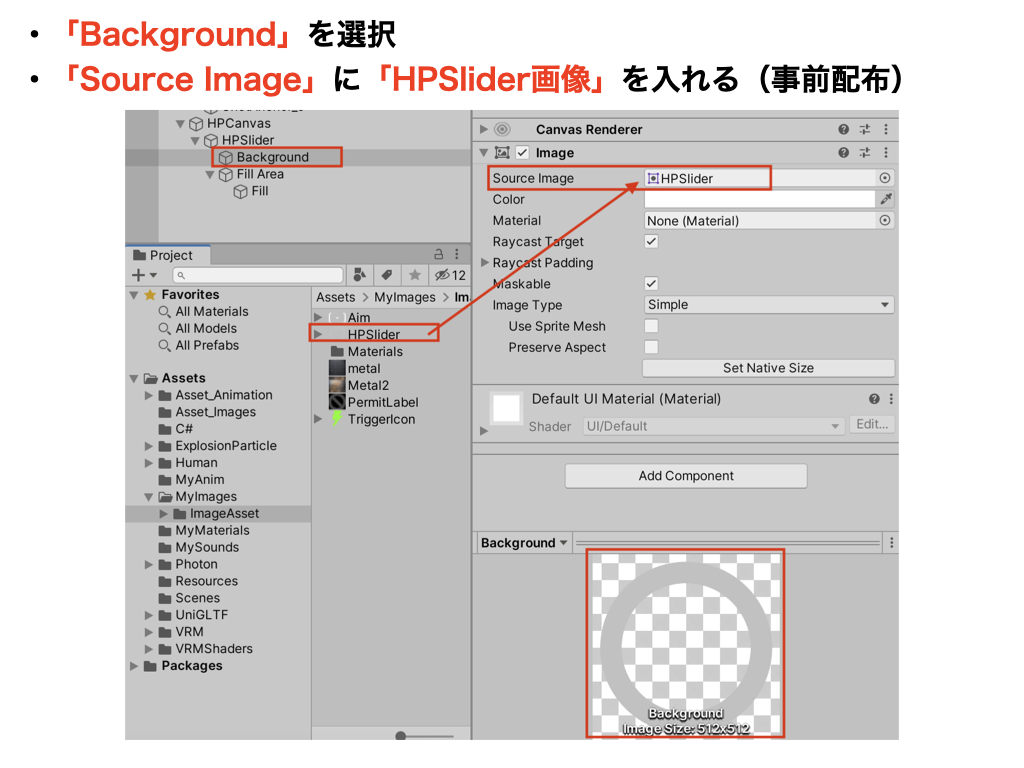
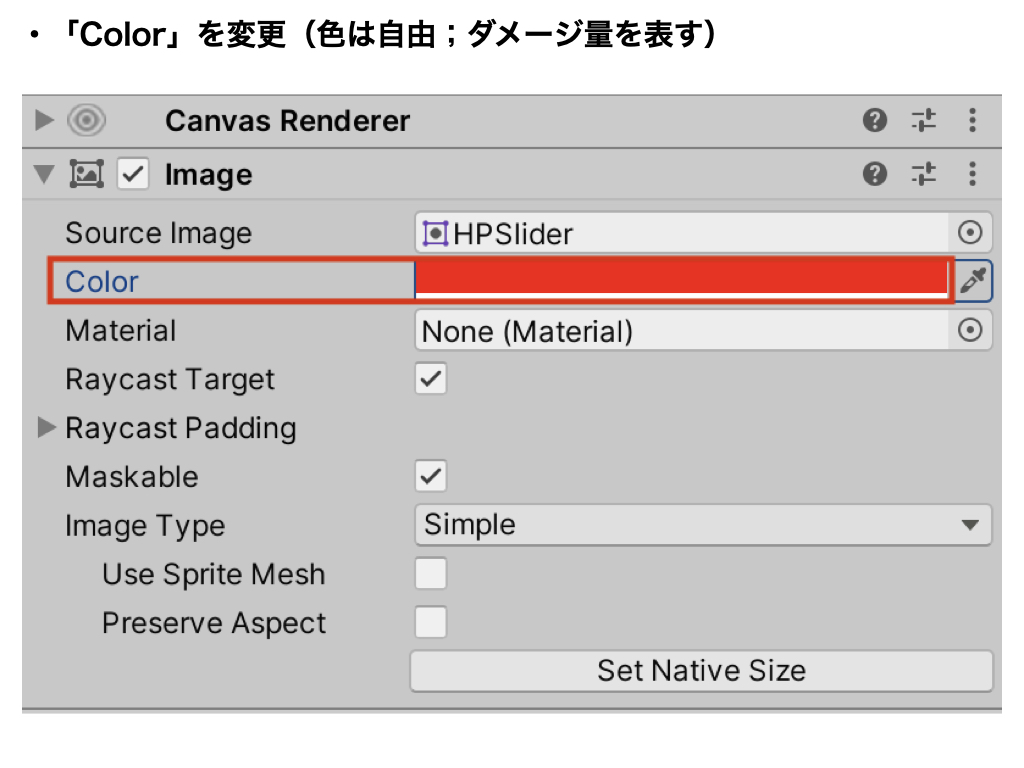
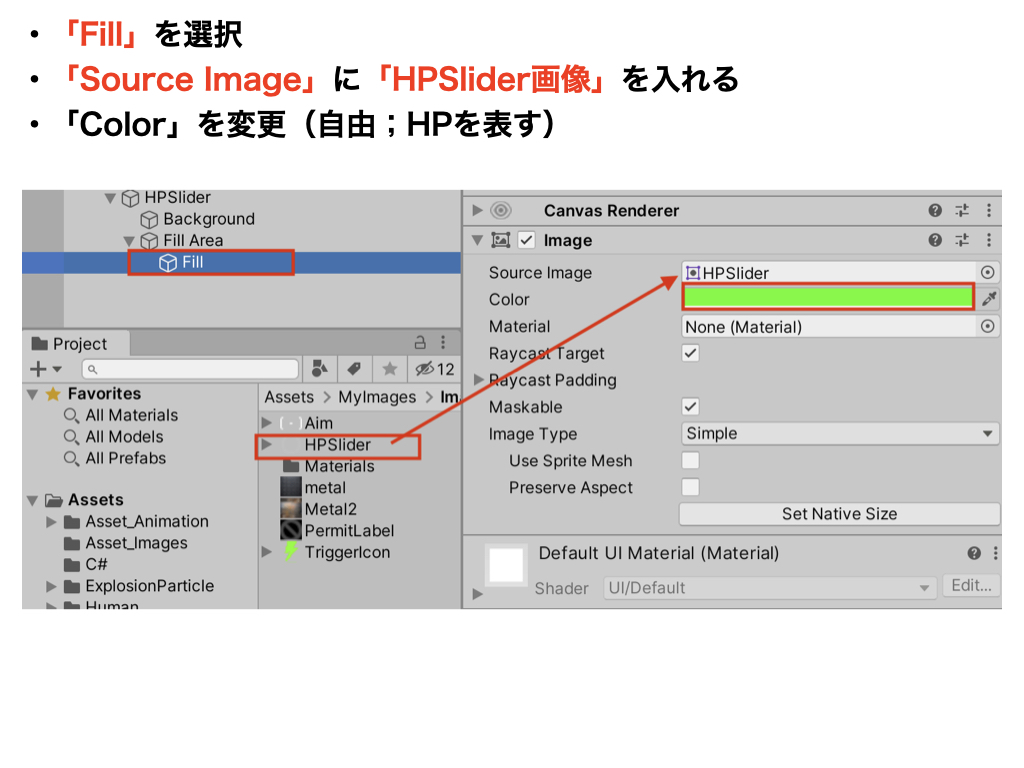
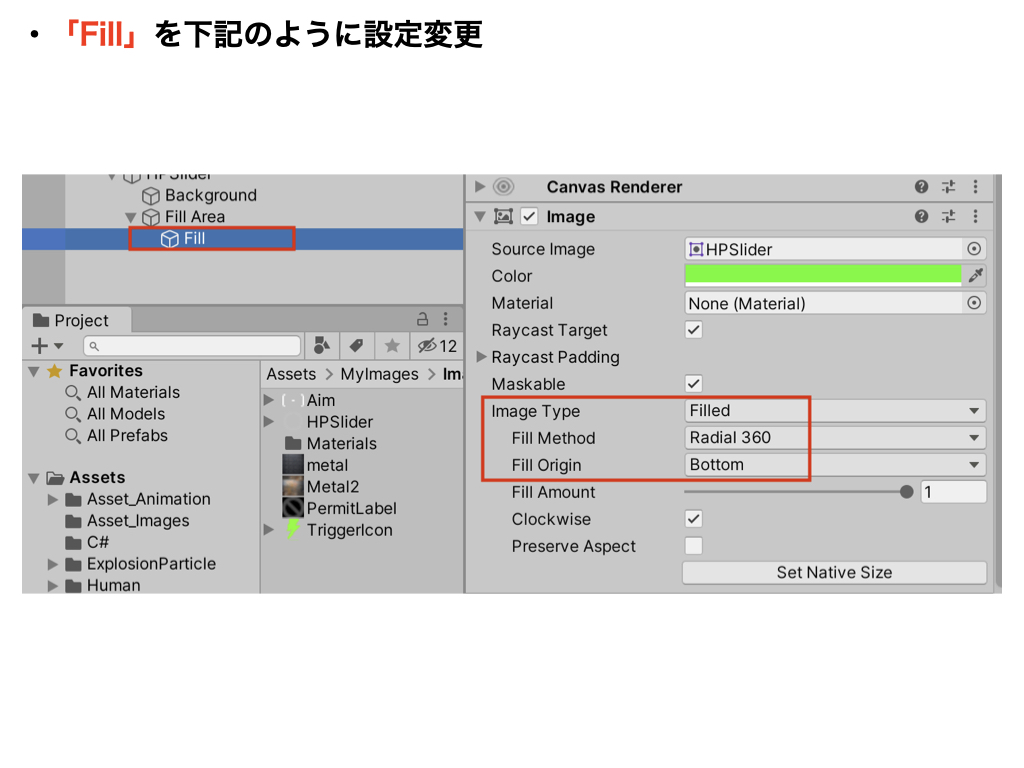
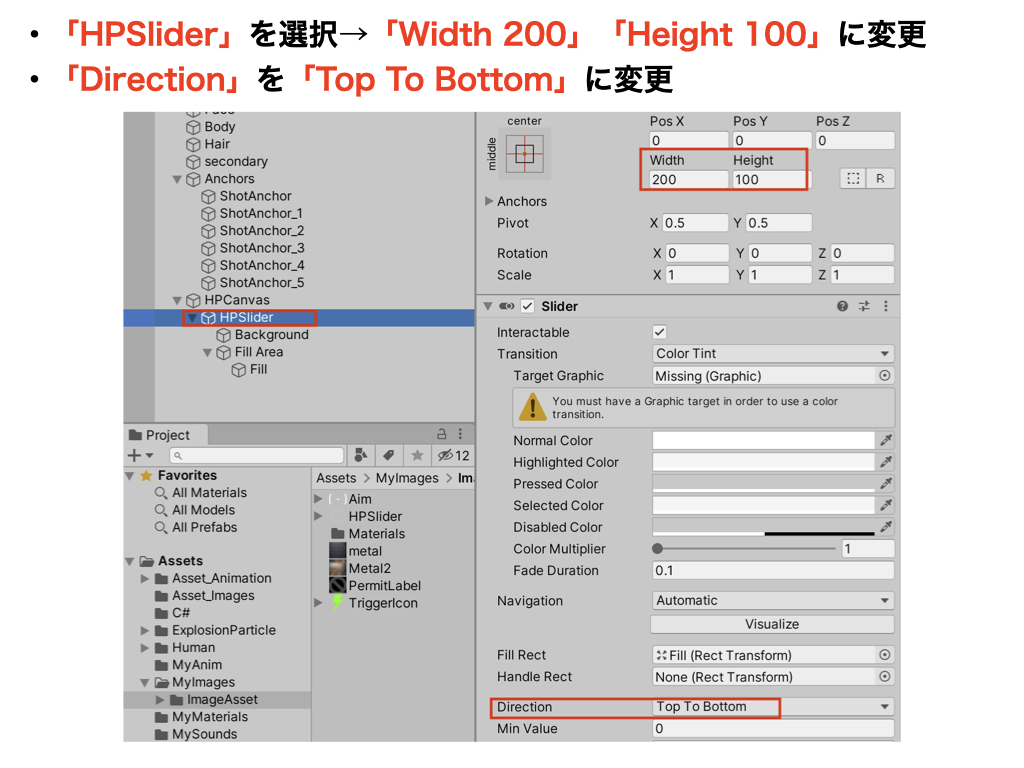
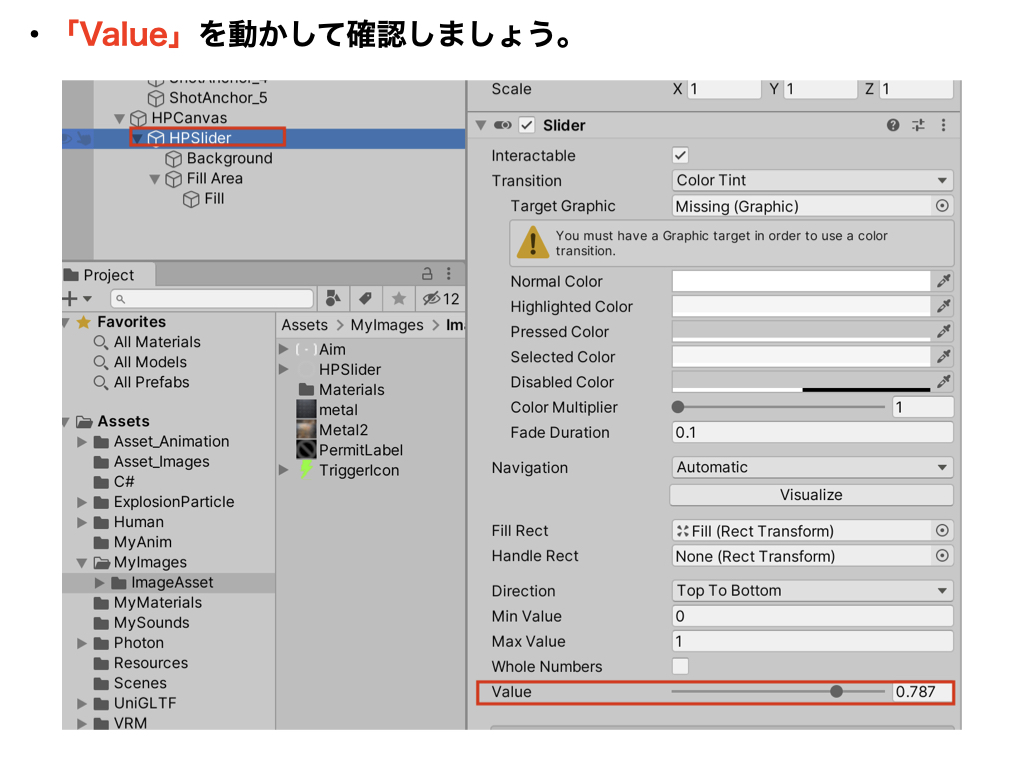
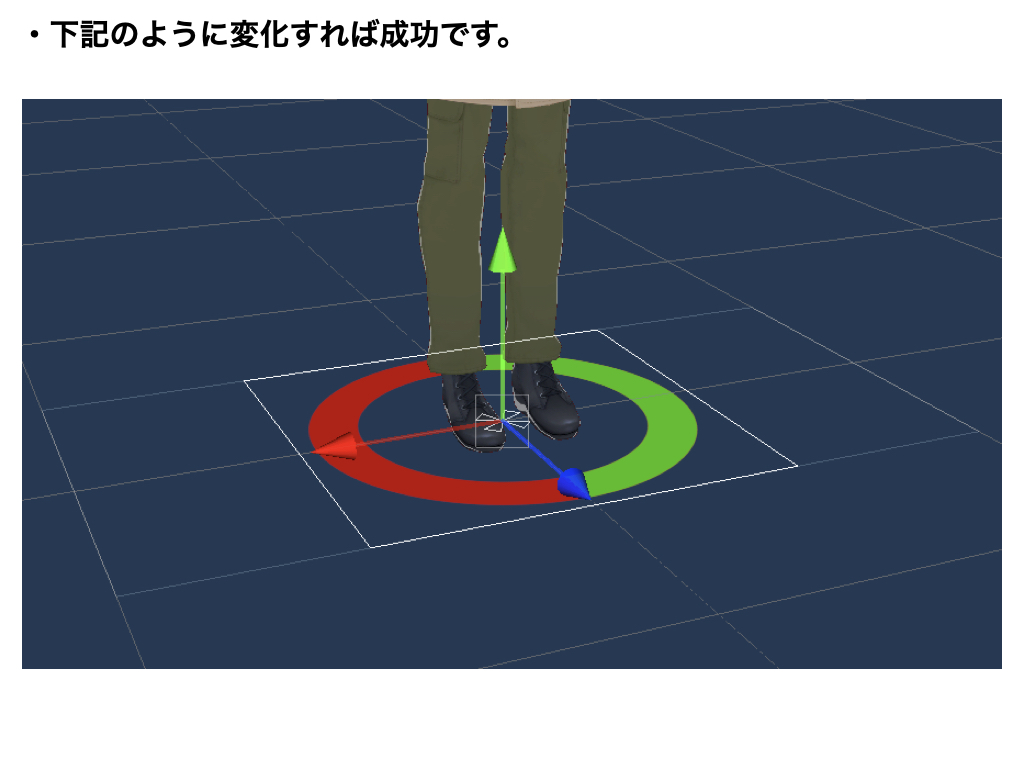
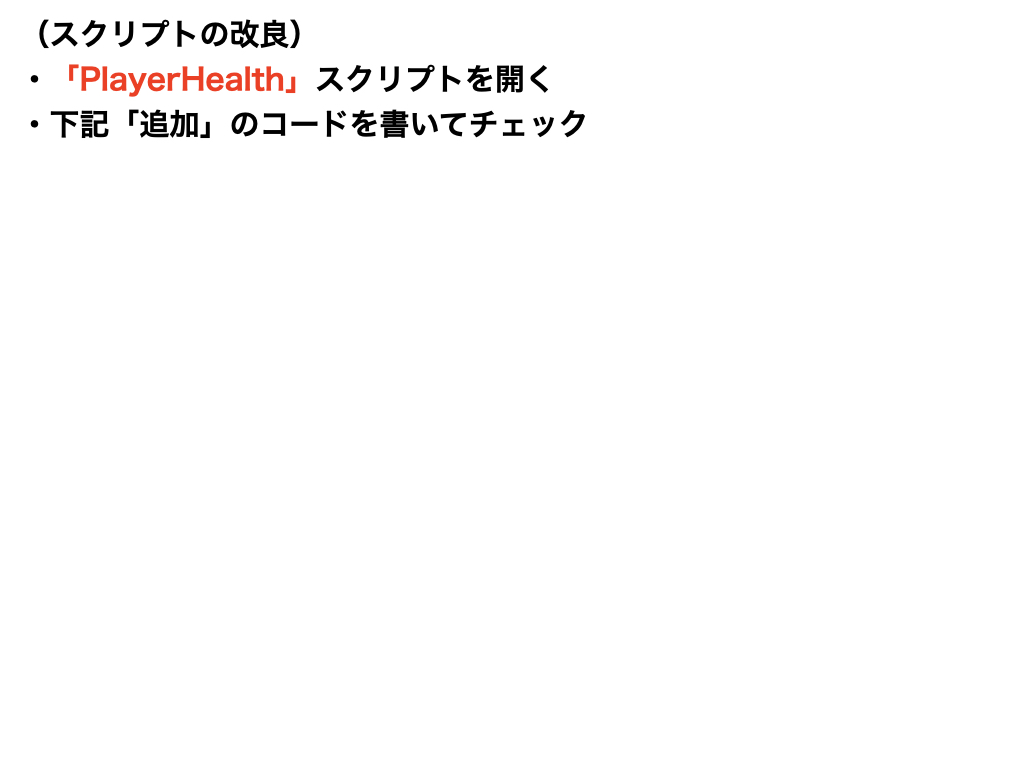
HPをスライダーで表示する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Photon.Pun;
// ★追加
using UnityEngine.UI;
public class PlayerHealth : MonoBehaviourPunCallbacks
{
private int maxHP = 10;
private int HP;
// ★追加
public Slider hpSlider;
private void Start()
{
HP = maxHP;
// ★追加
hpSlider.maxValue = HP;
hpSlider.value = HP;
}
private void OnTriggerEnter(Collider other)
{
if(other.CompareTag("Trigger"))
{
HP -= 1;
print(photonView.Owner.NickName + HP);
// ★追加
hpSlider.value = HP;
photonView.RPC("Damage", RpcTarget.Others);
}
}
// RPC→リモート・プロシージャ・コールの略称
// 相手に「ここで定義したメソッド」を「実行」してもらう仕組み
[PunRPC]
void Damage()
{
HP -= 1;
print(photonView.Owner.NickName + HP);
// ★追加
hpSlider.value = HP;
}
}
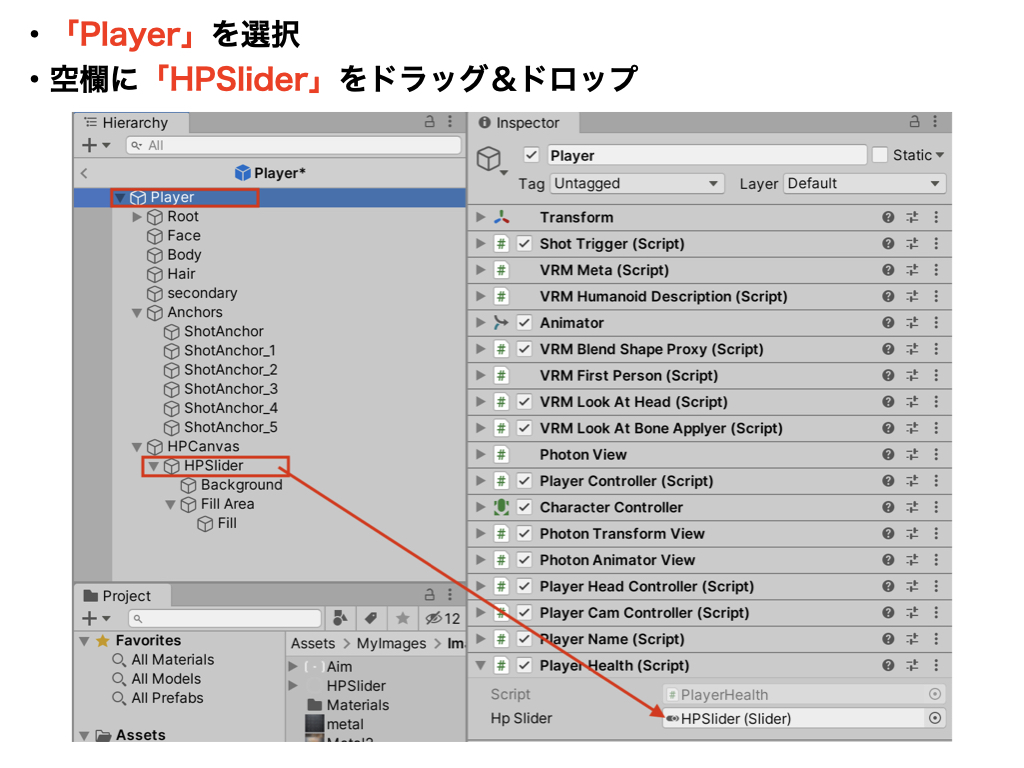
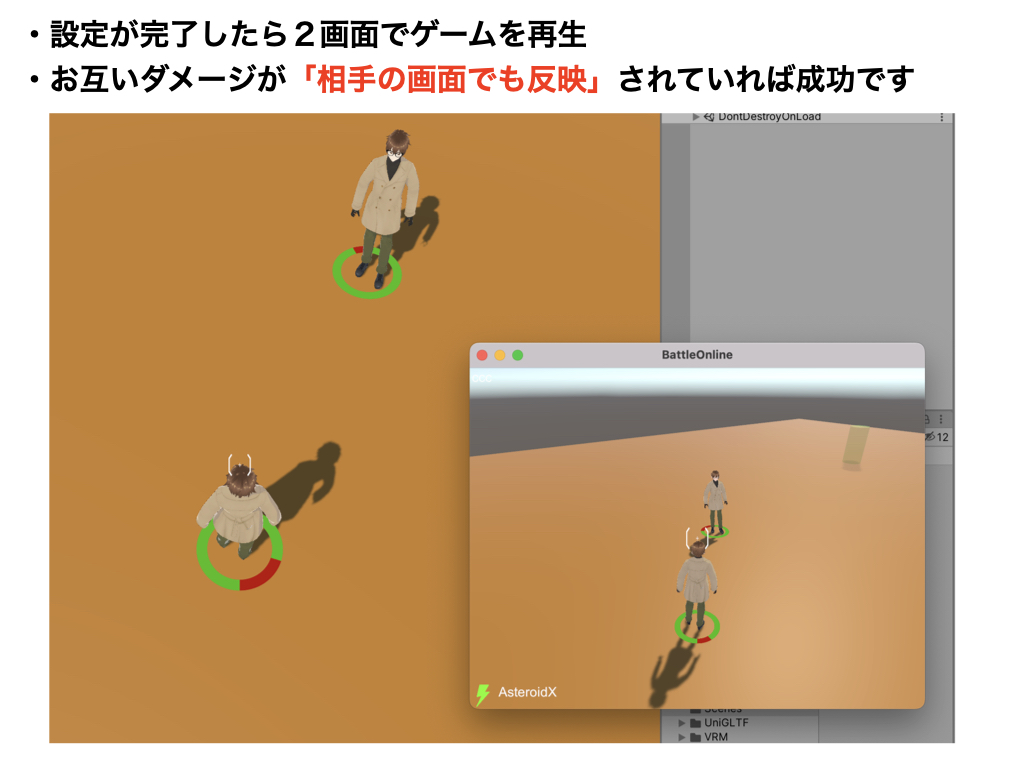
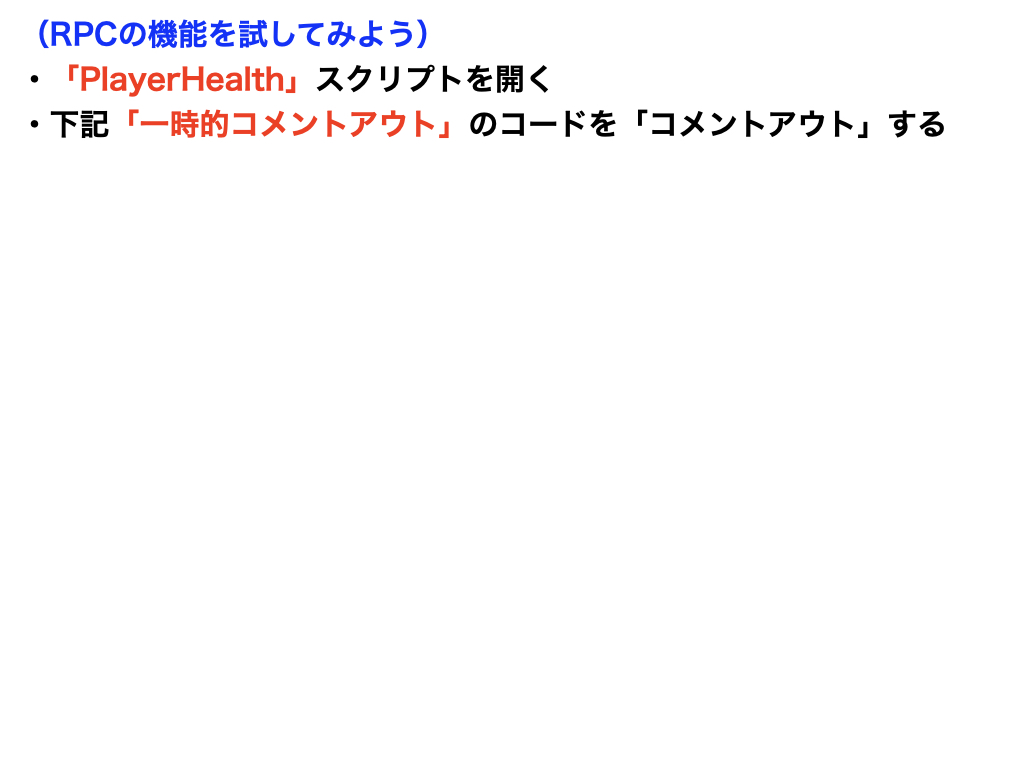
RPCの機能の確認
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Photon.Pun;
// ★追加
using UnityEngine.UI;
public class PlayerHealth : MonoBehaviourPunCallbacks
{
private int maxHP = 10;
private int HP;
// ★追加
public Slider hpSlider;
private void Start()
{
HP = maxHP;
// ★追加
hpSlider.maxValue = HP;
hpSlider.value = HP;
}
private void OnTriggerEnter(Collider other)
{
if(other.CompareTag("Trigger"))
{
HP -= 1;
print(photonView.Owner.NickName + HP);
// ★追加
hpSlider.value = HP;
// ★★一時コメントアウト(下記の1行)
//photonView.RPC("Damage", RpcTarget.Others);
}
}
// RPC→リモート・プロシージャ・コールの略称
// 相手に「ここで定義したメソッド」を「実行」してもらう仕組み
[PunRPC]
void Damage()
{
HP -= 1;
print(photonView.Owner.NickName + HP);
// ★追加
hpSlider.value = HP;
}
}
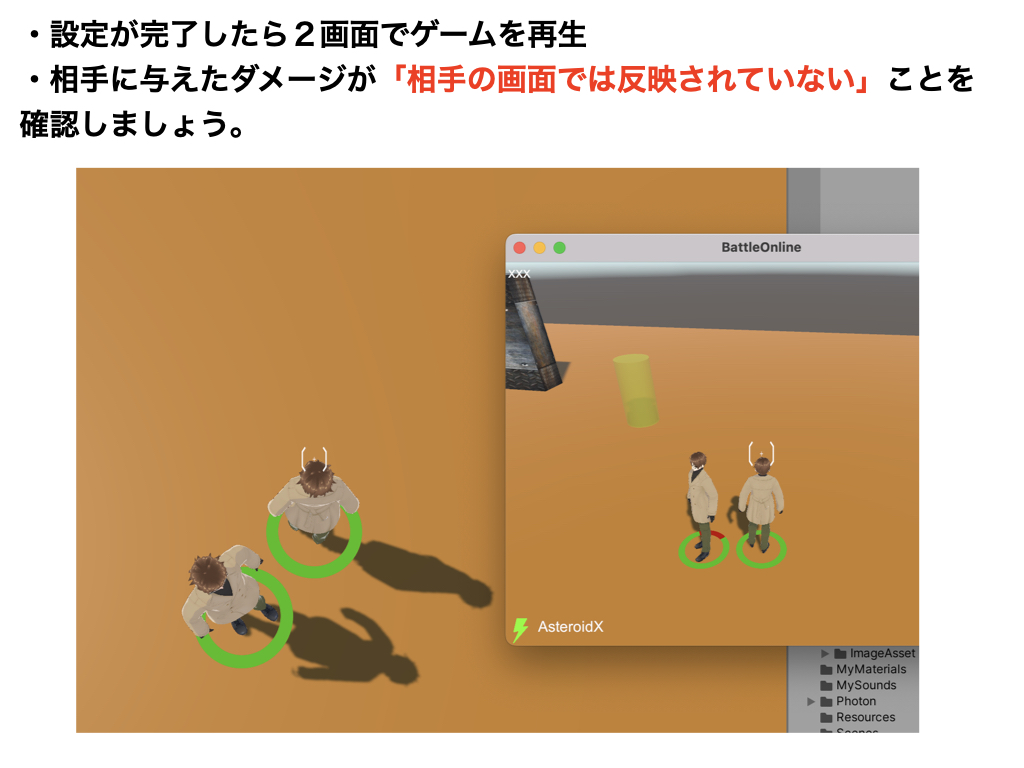
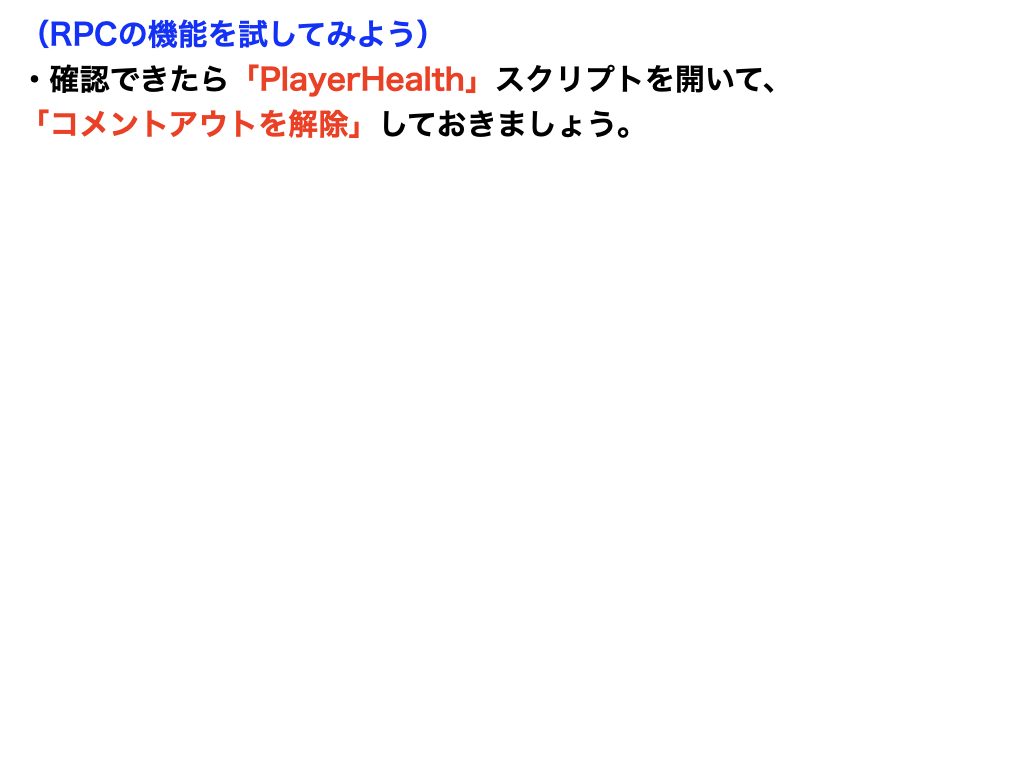
HPをスライダーで表示する