コインの残り枚数を表示する
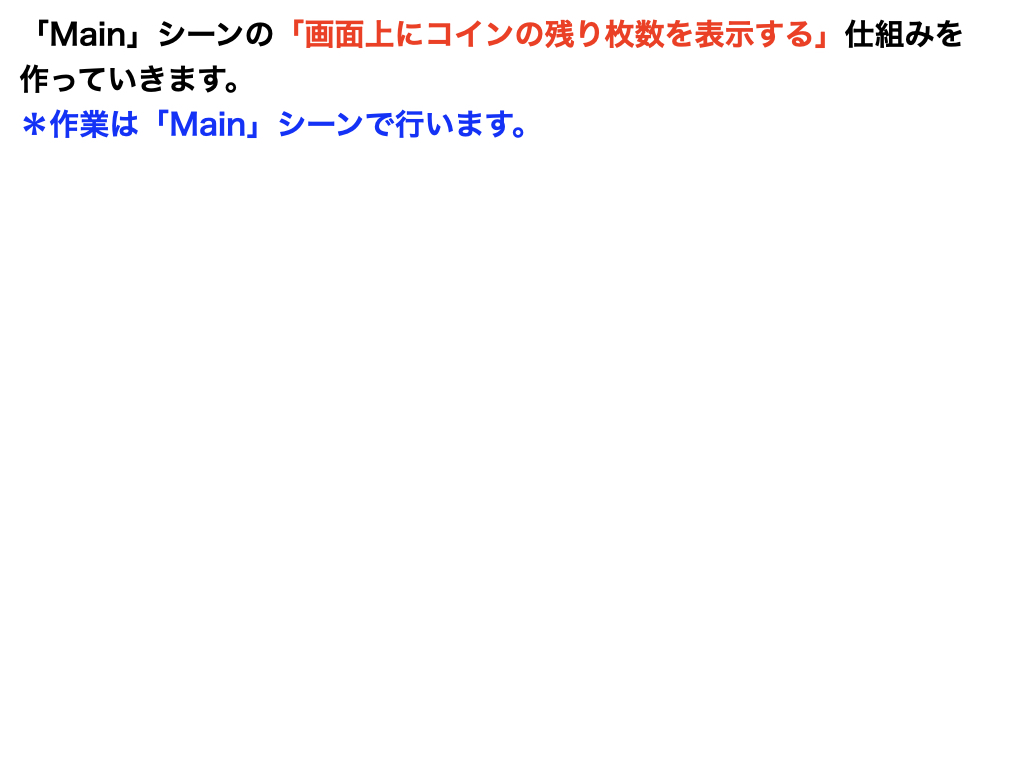
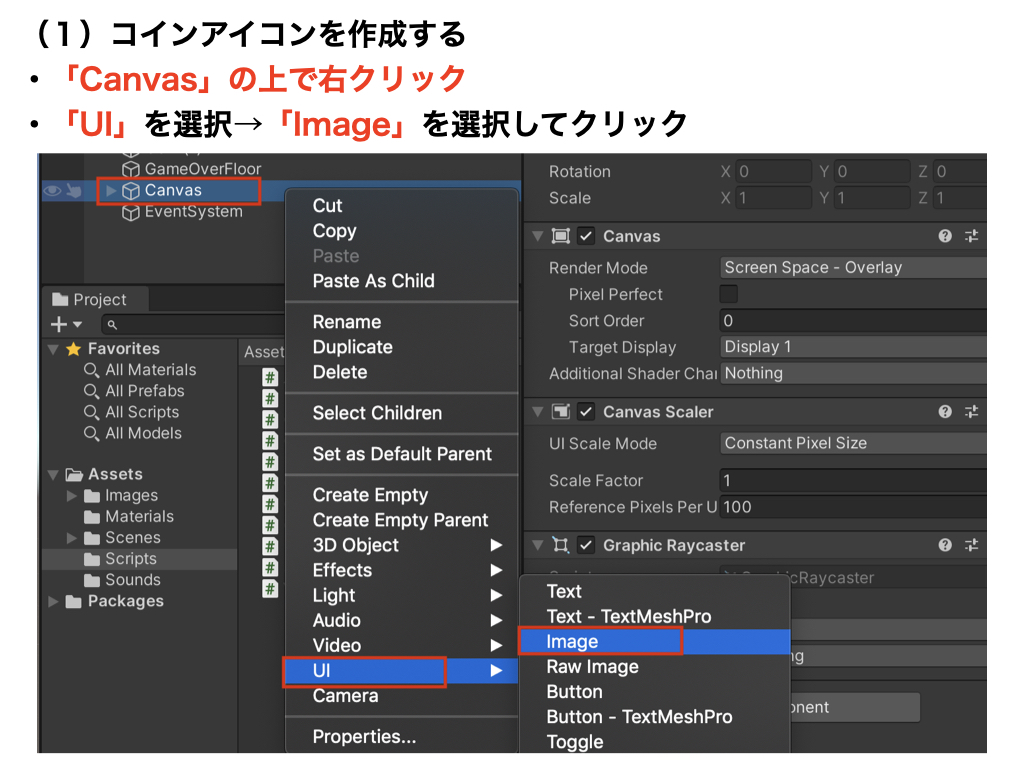
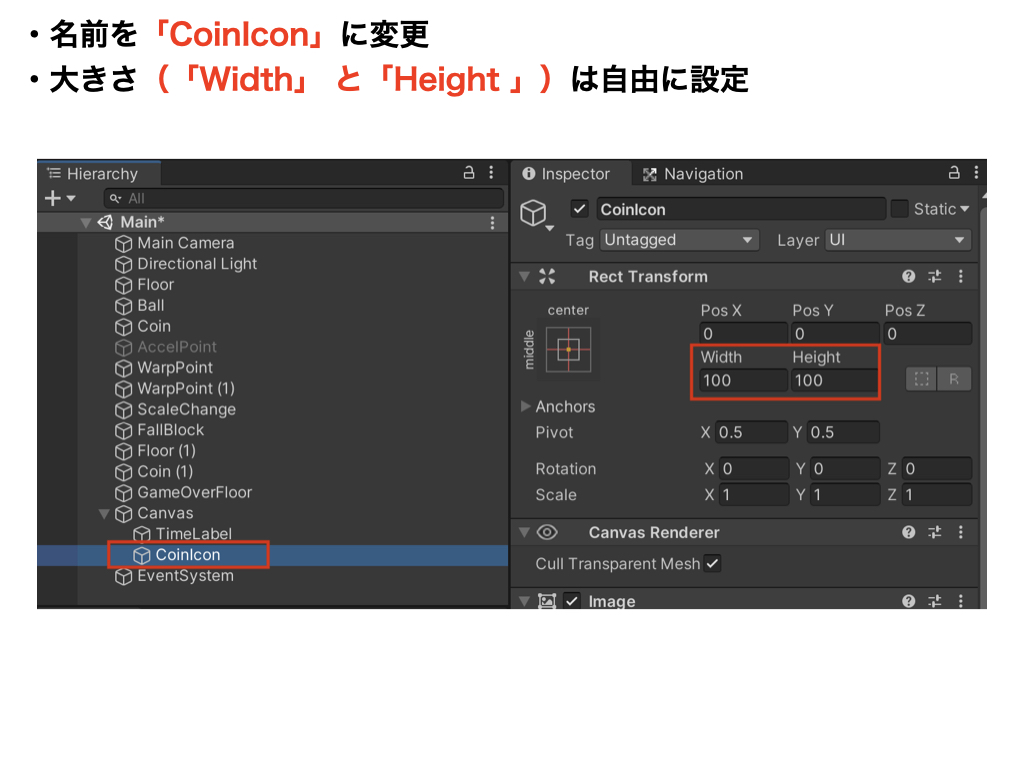
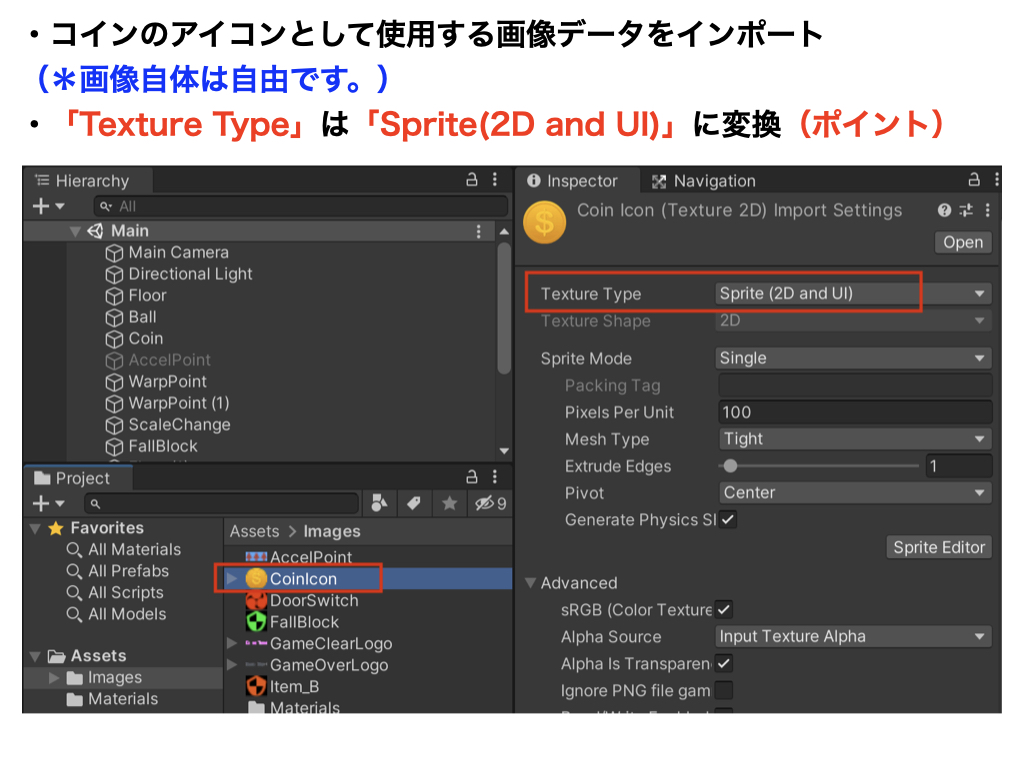
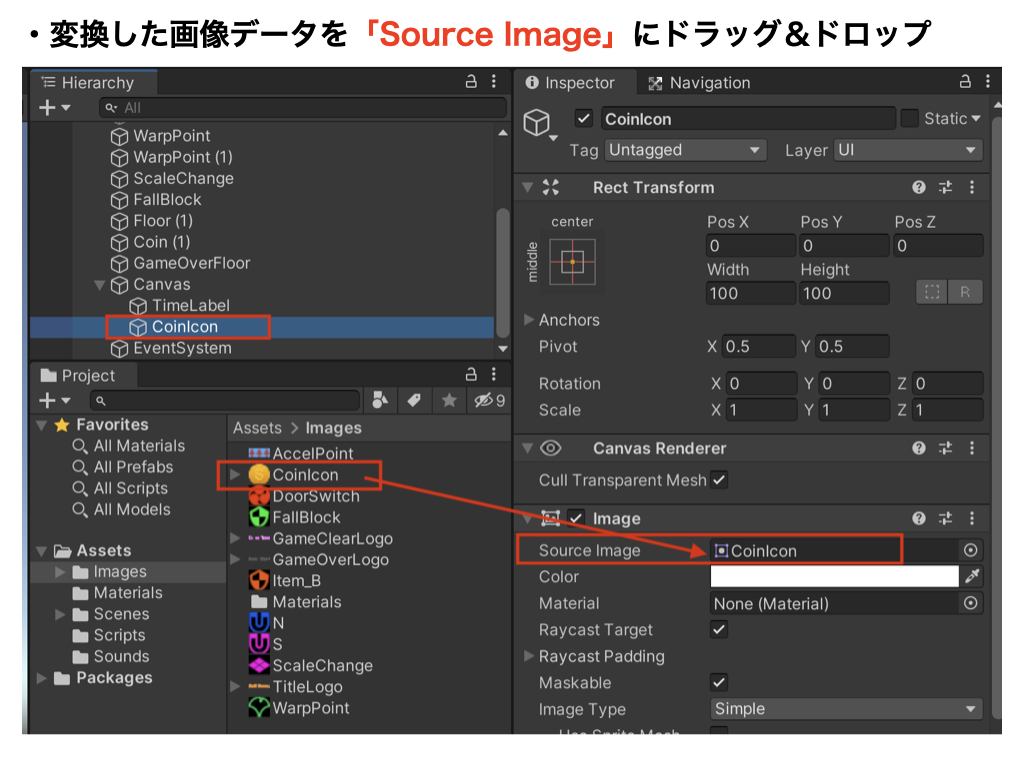
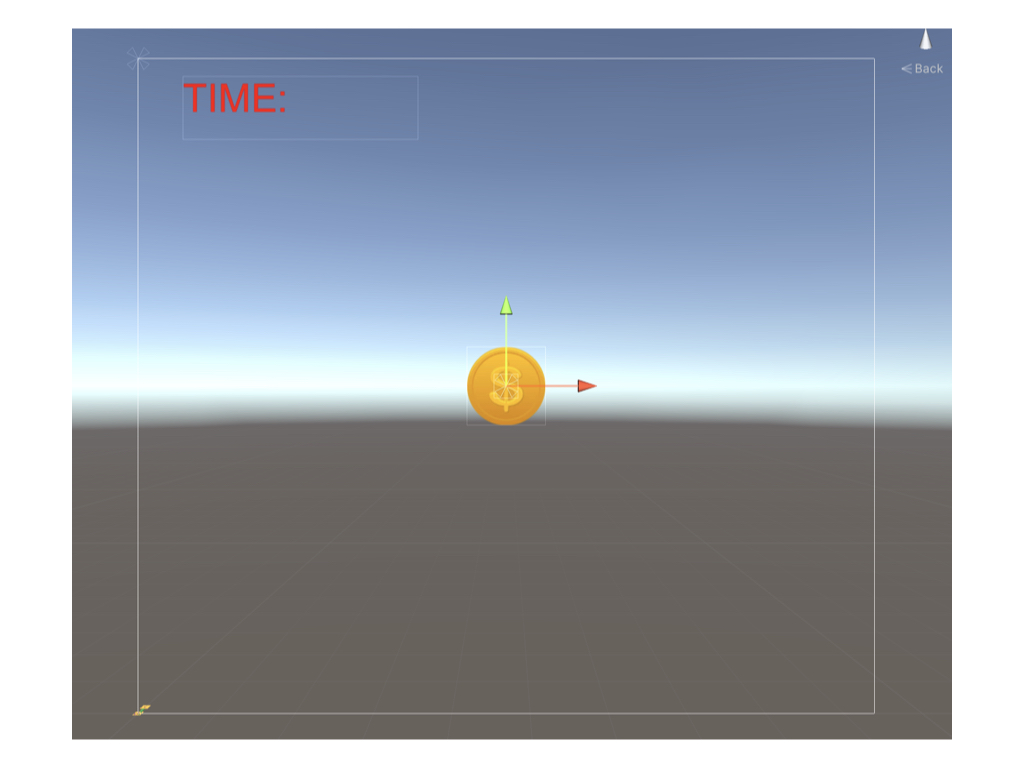
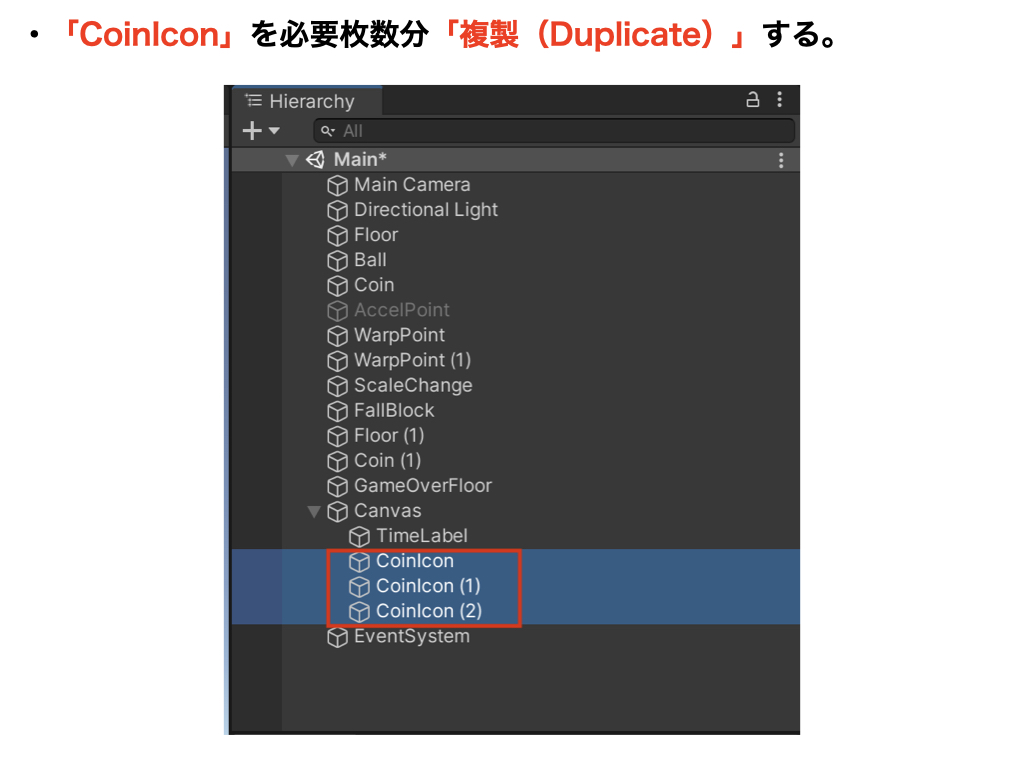
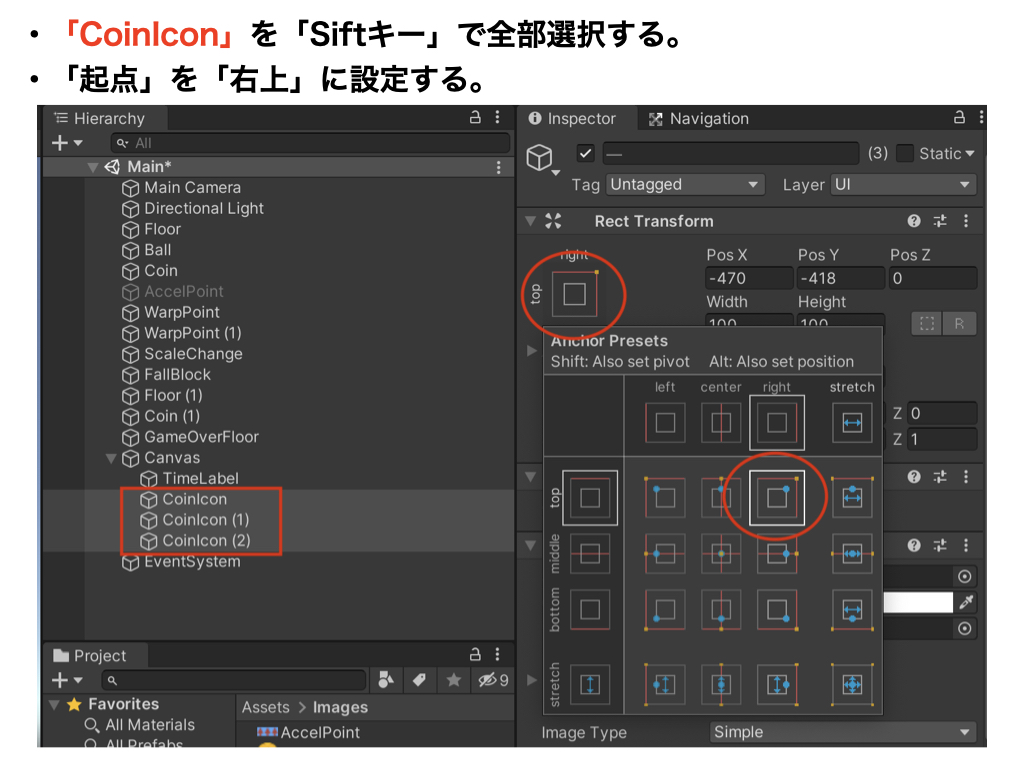
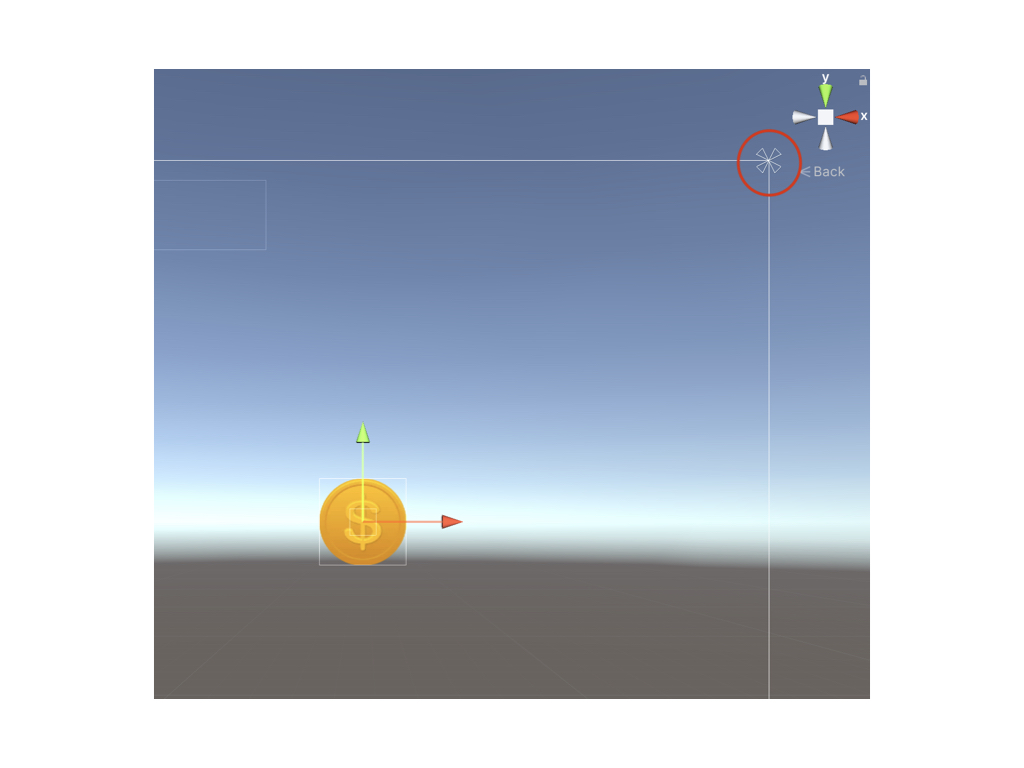
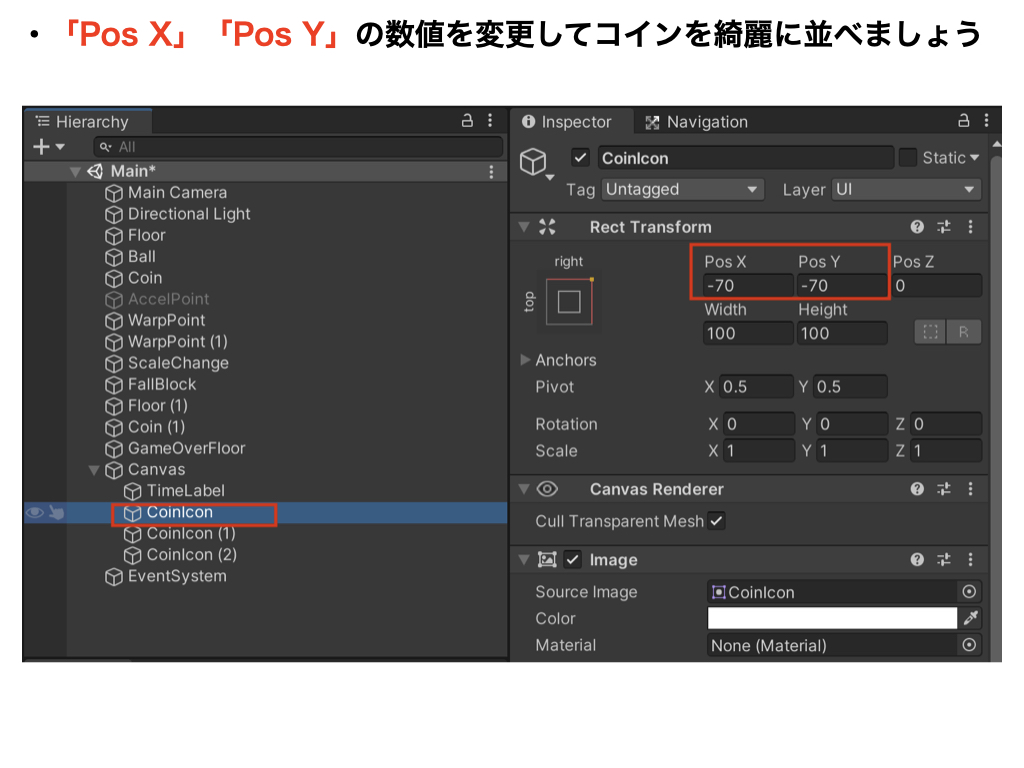
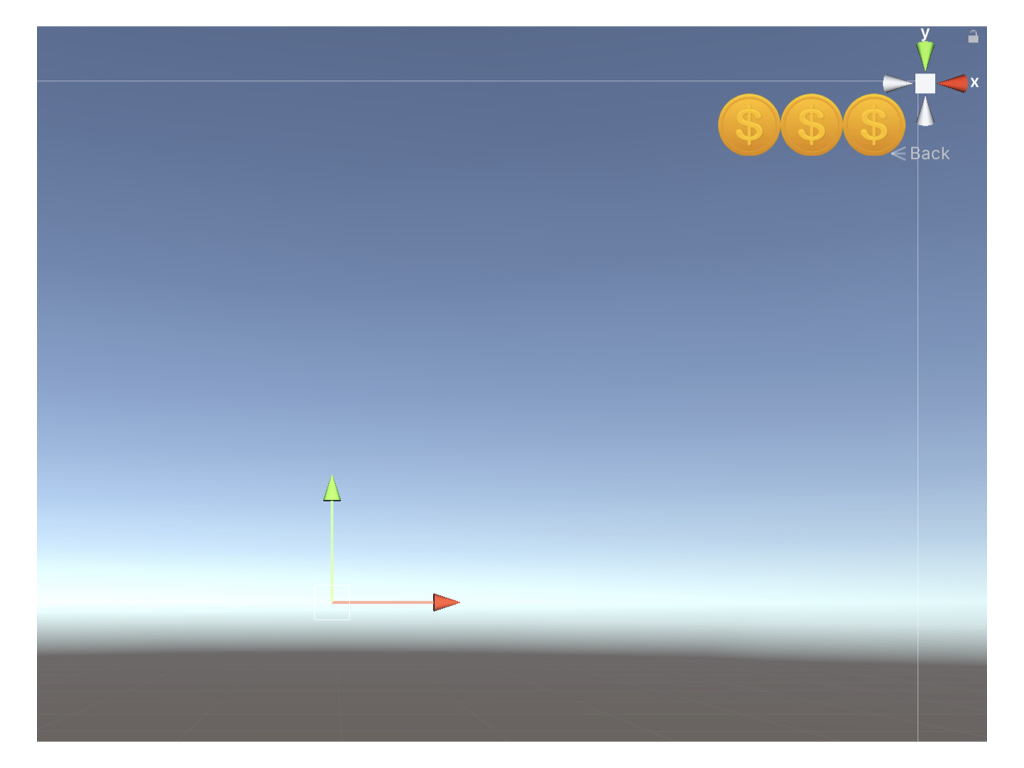
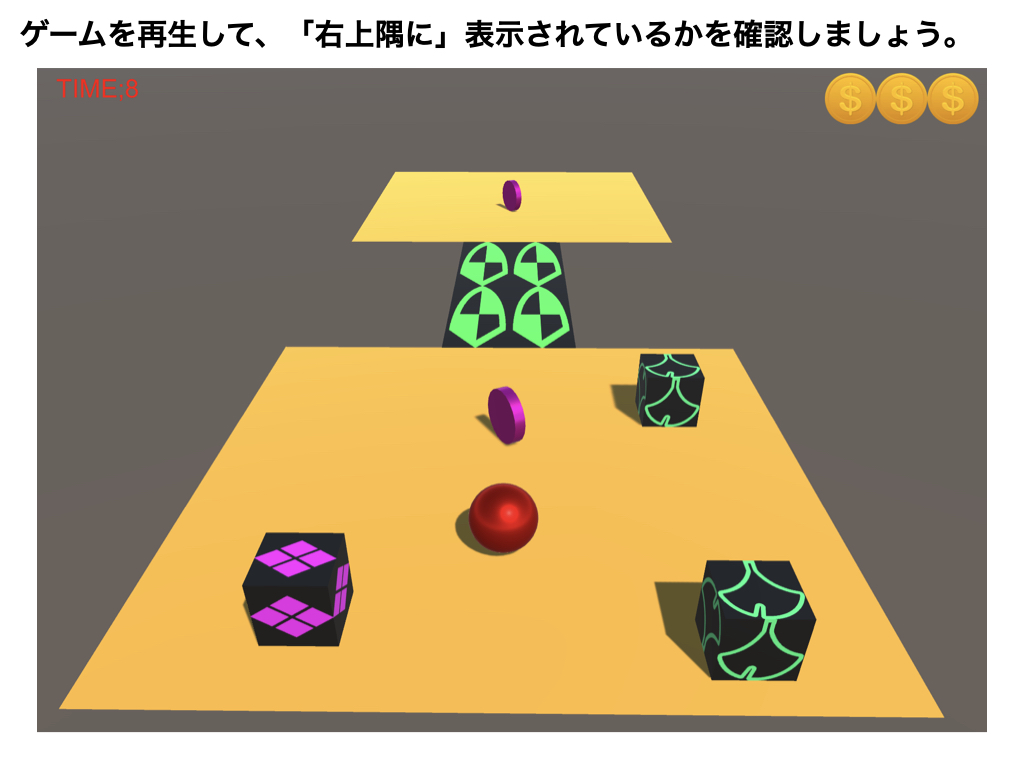
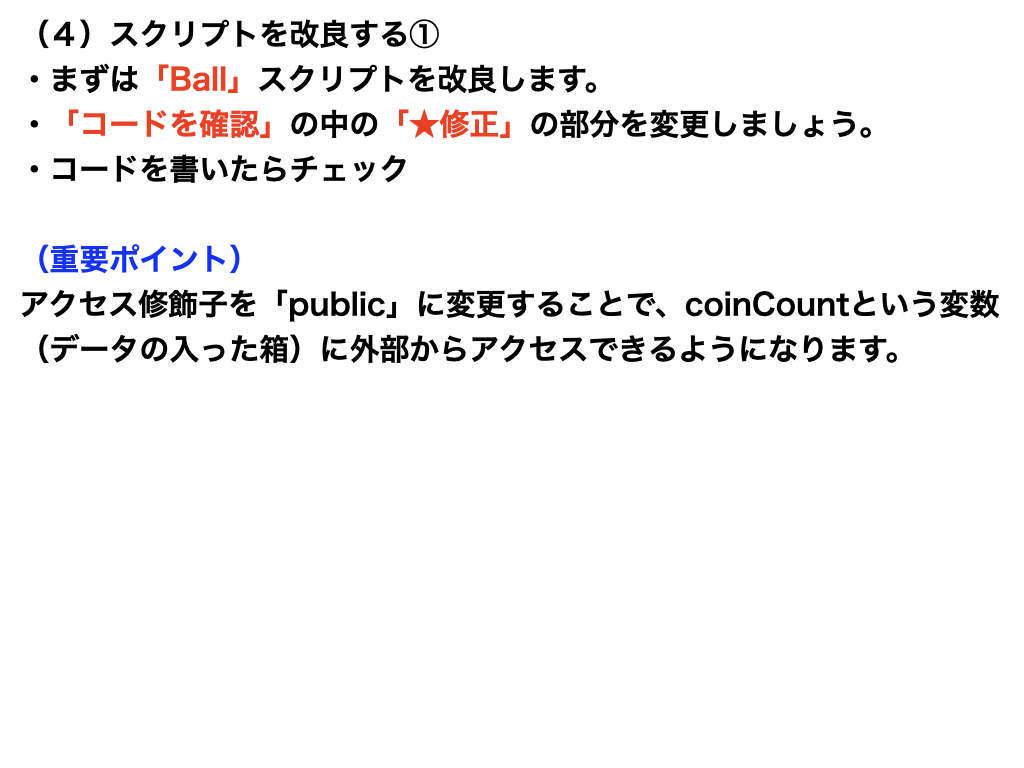
コインアイコンを表示する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
// ★修正(privateをpublicに変更する)
public int coinCount = 0;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
coinCount += 1;
if(coinCount == 3)
{
SceneManager.LoadScene("GameClear");
}
}
}
private void OnCollisionEnter(Collision collision)
{
if(collision.gameObject.CompareTag("Floor"))
{
isJumping = false;
}
}
}
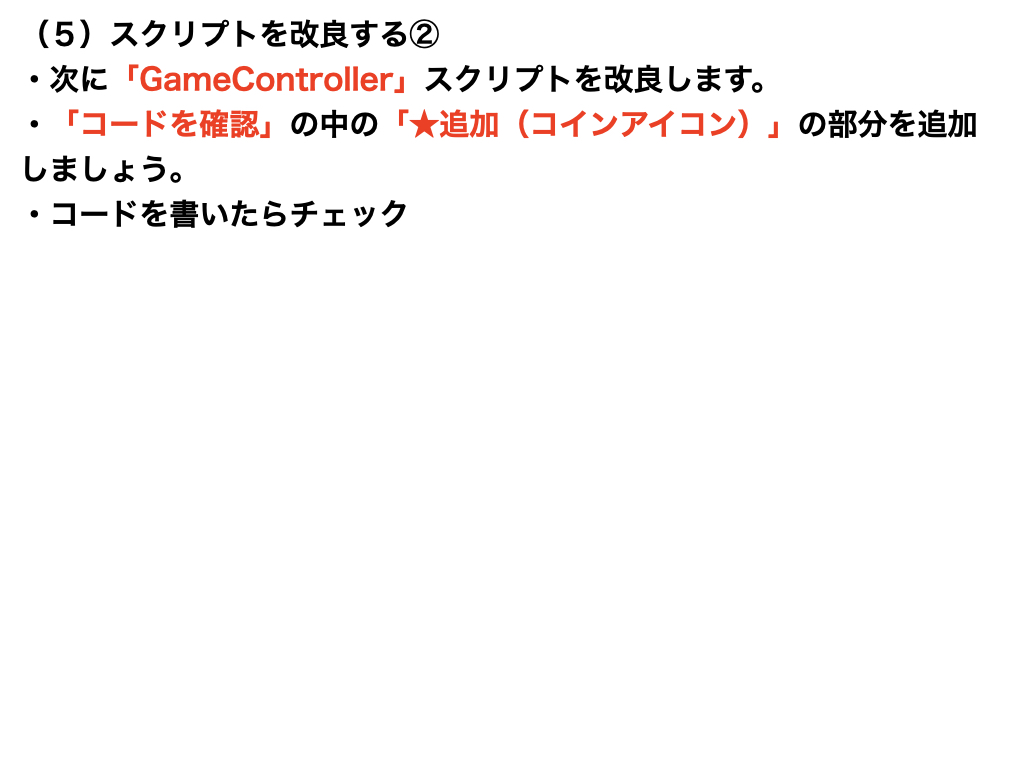
コインアイコンの表示
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class GameController : MonoBehaviour
{
public Text timeLabel;
public float timeCount;
// ★追加(コインアイコン)
// 配列(複数のデータを入れることのできる仕切りのある箱)
public GameObject[] coinIcons;
private Ball ballScript;
private int getCoin;
void Start()
{
timeLabel.text = "TIME;" + timeCount;
// ★追加(コインアイコン)
// 「Ball」オブジェクトに付いている「Ball」スクリプトの情報を取得する。
ballScript = GameObject.Find("Ball").GetComponent<Ball>();
}
void Update()
{
timeCount -= Time.deltaTime;
timeLabel.text = "TIME;" + timeCount.ToString("0");
if (timeCount < 0)
{
SceneManager.LoadScene("GameOver");
}
// ★追加(コインアイコン)
// 「Ball」スクリプトの中にある「coinCount」の情報を取得する。
getCoin = ballScript.coinCount;
// for文(繰り返し文)
for (int i = 0; i < coinIcons.Length; i++)
{
if(getCoin <= i)
{
// コインアイコンを表示状態にする。
coinIcons[i].SetActive(true);
}
else
{
// コインアイコンを非表示状態にする。
coinIcons[i].SetActive(false);
}
}
}
}
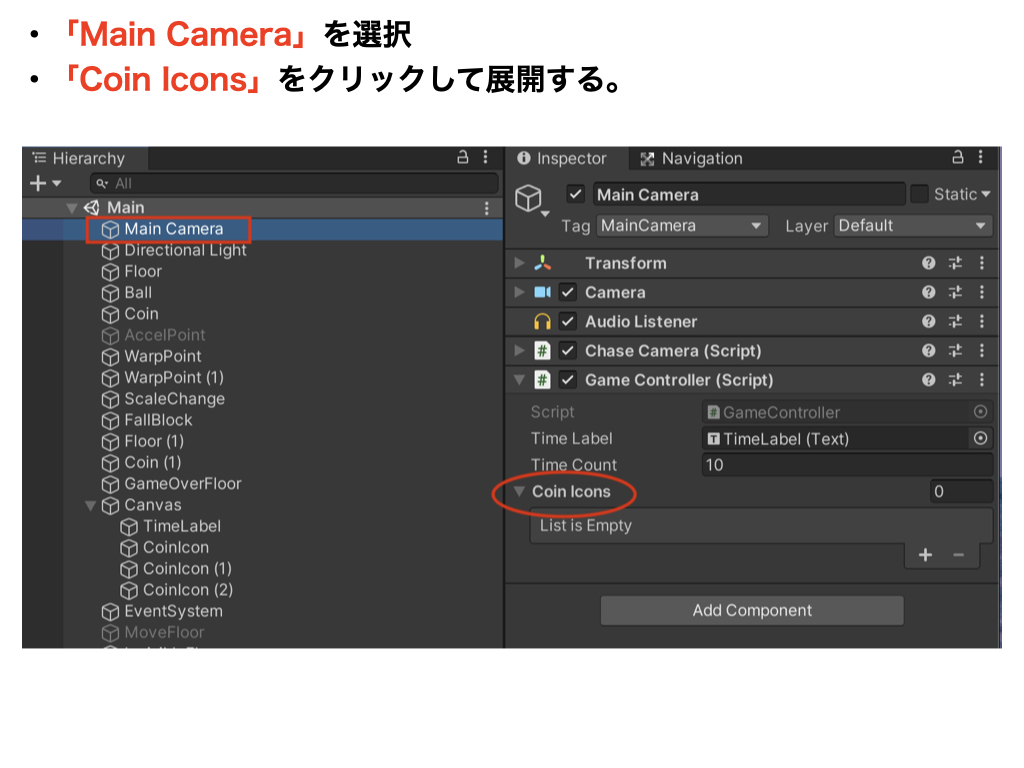
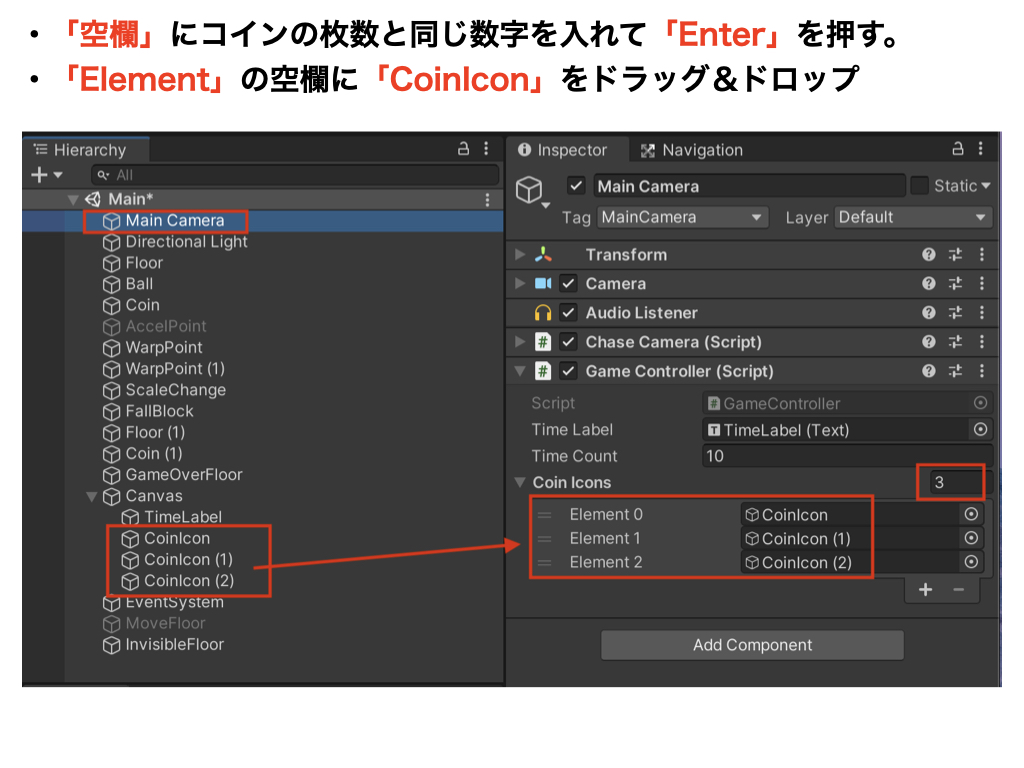
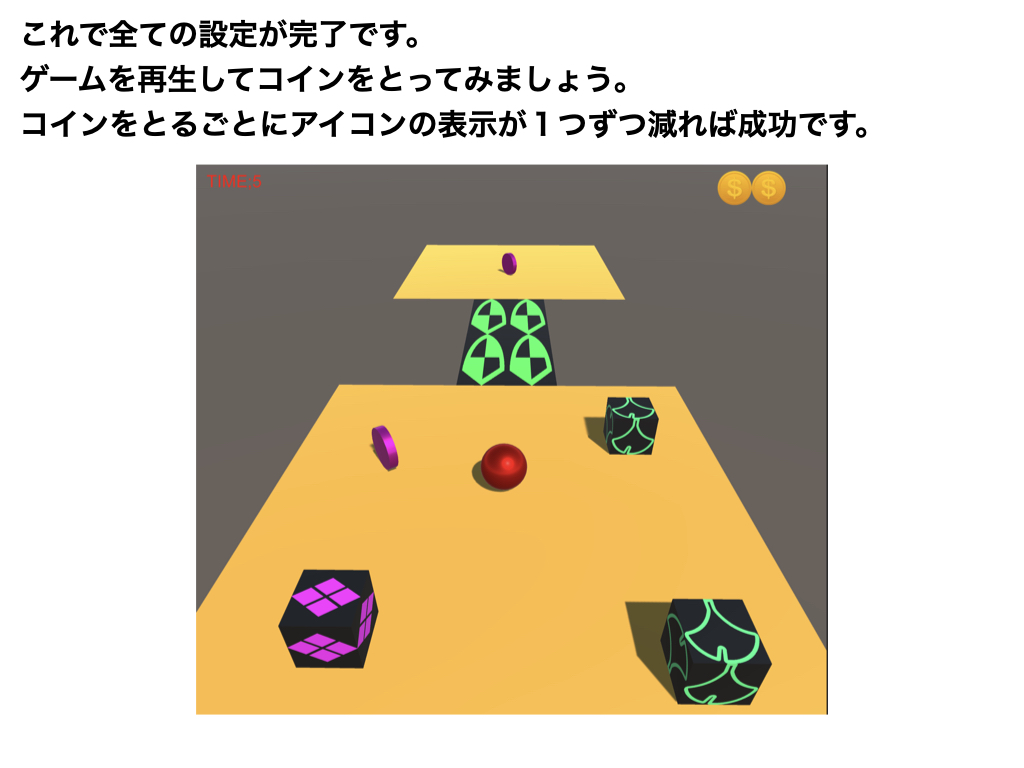
【2020版】BallGame(全27回)
他のコースを見る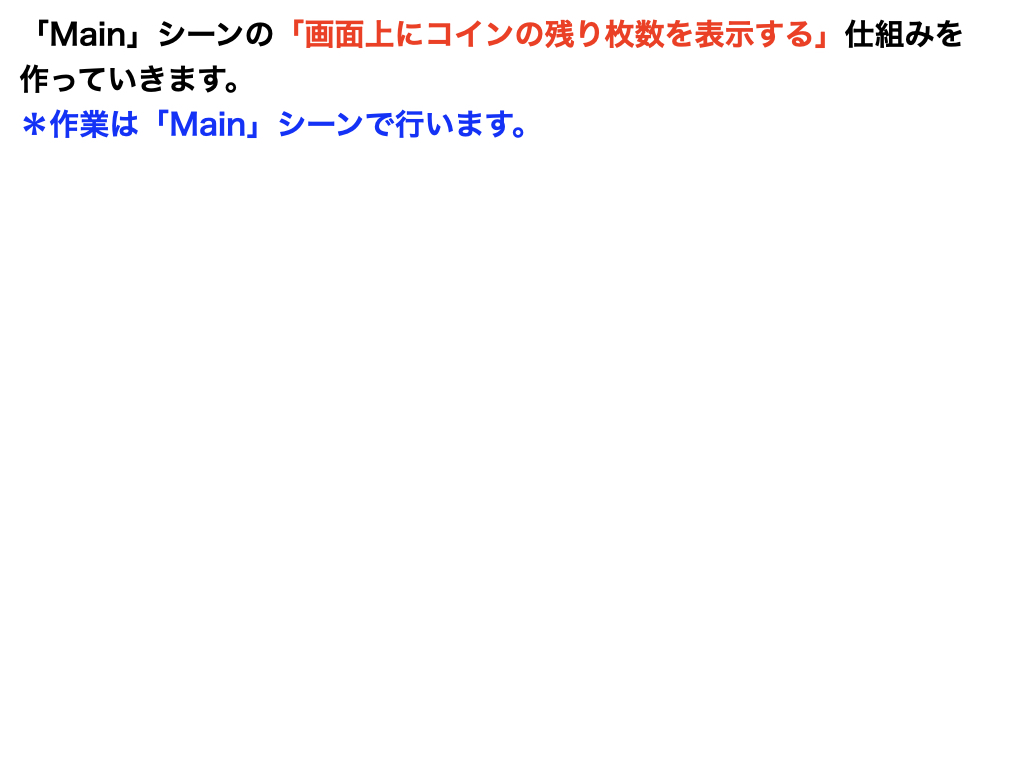
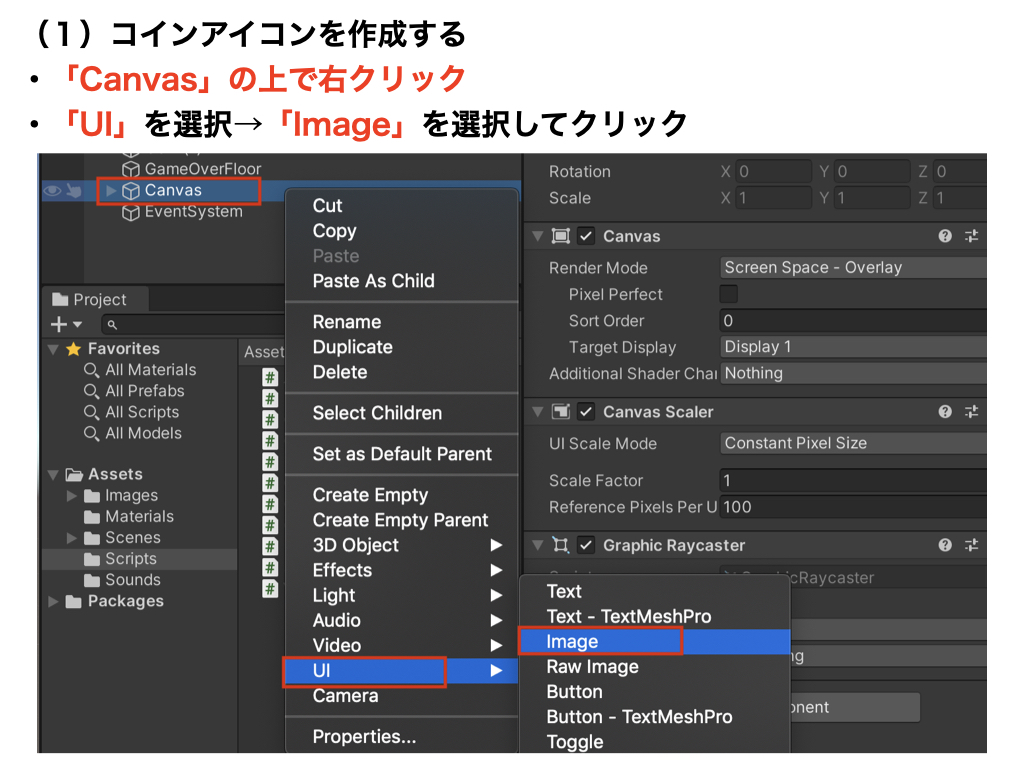
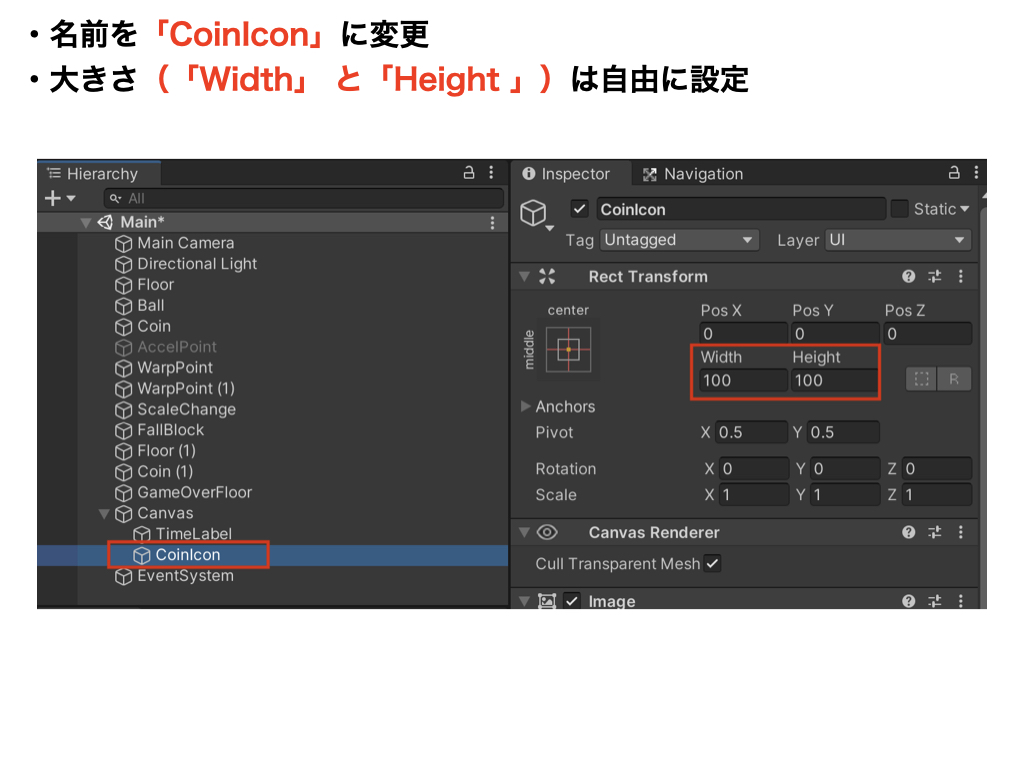
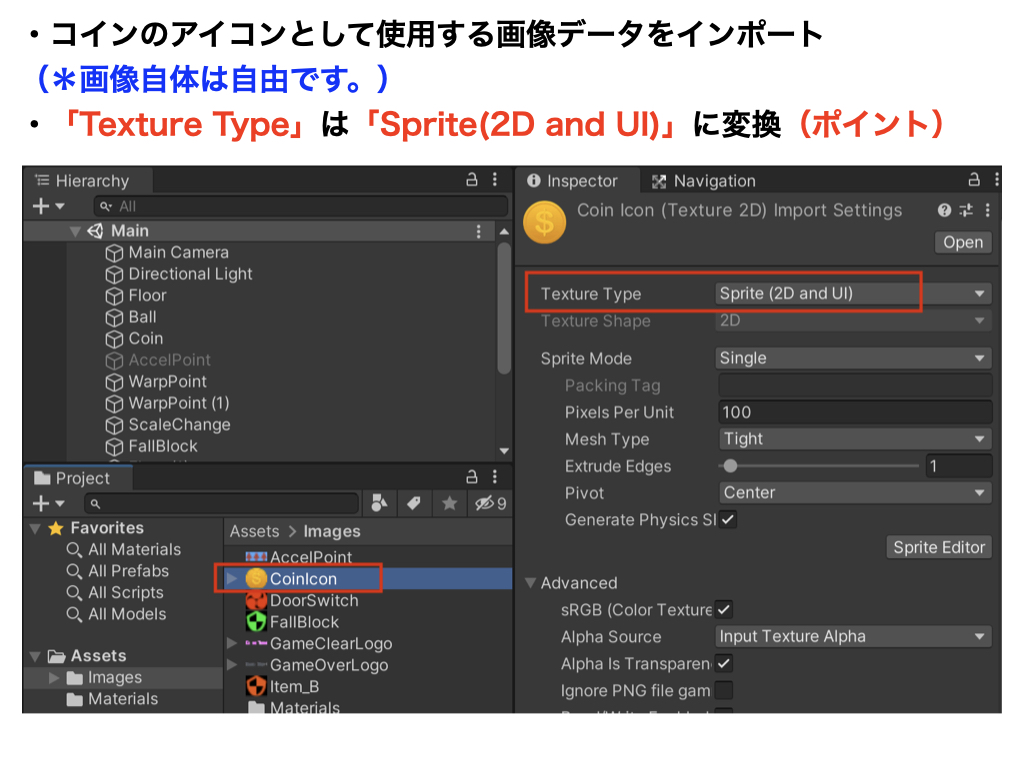
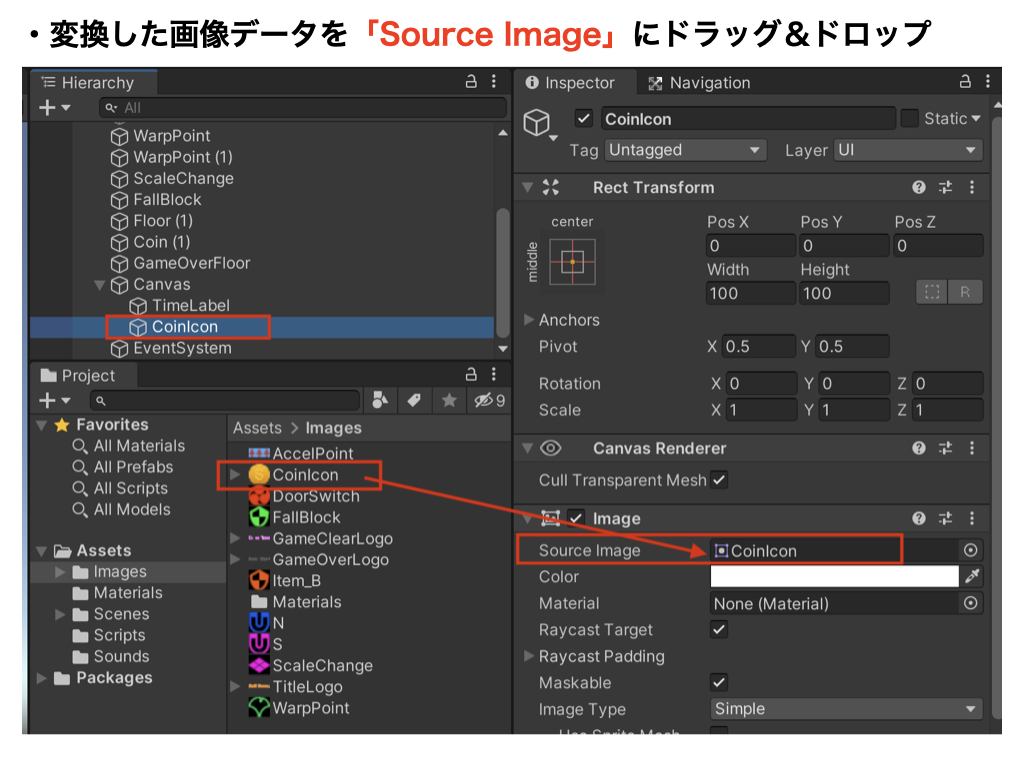
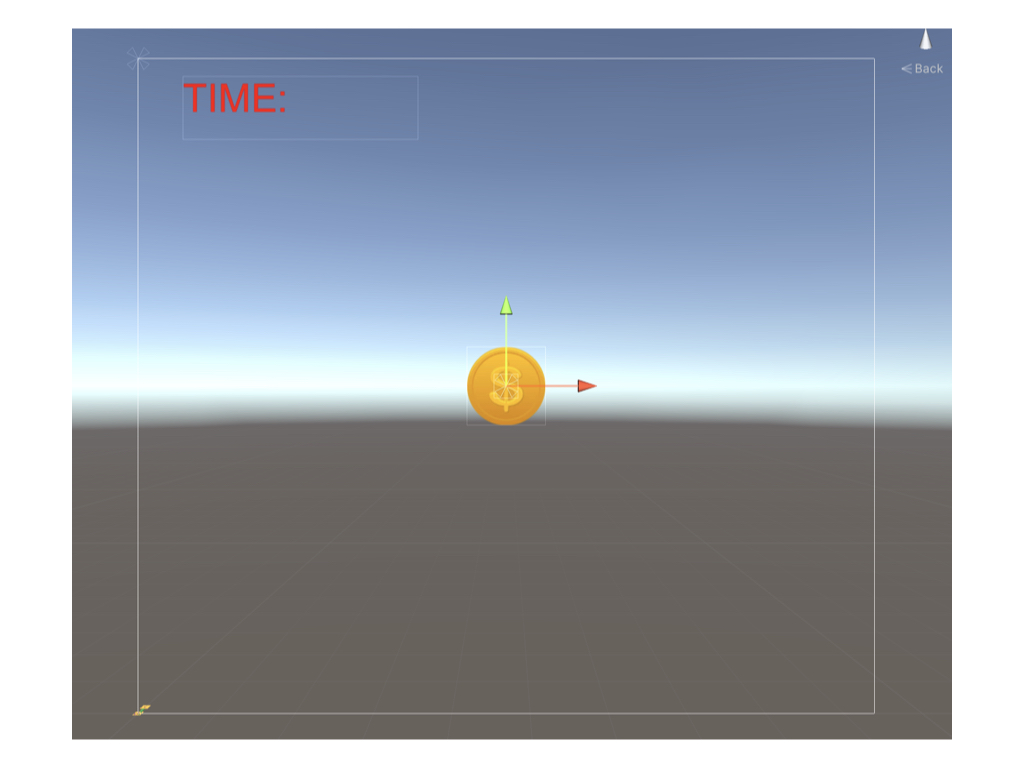
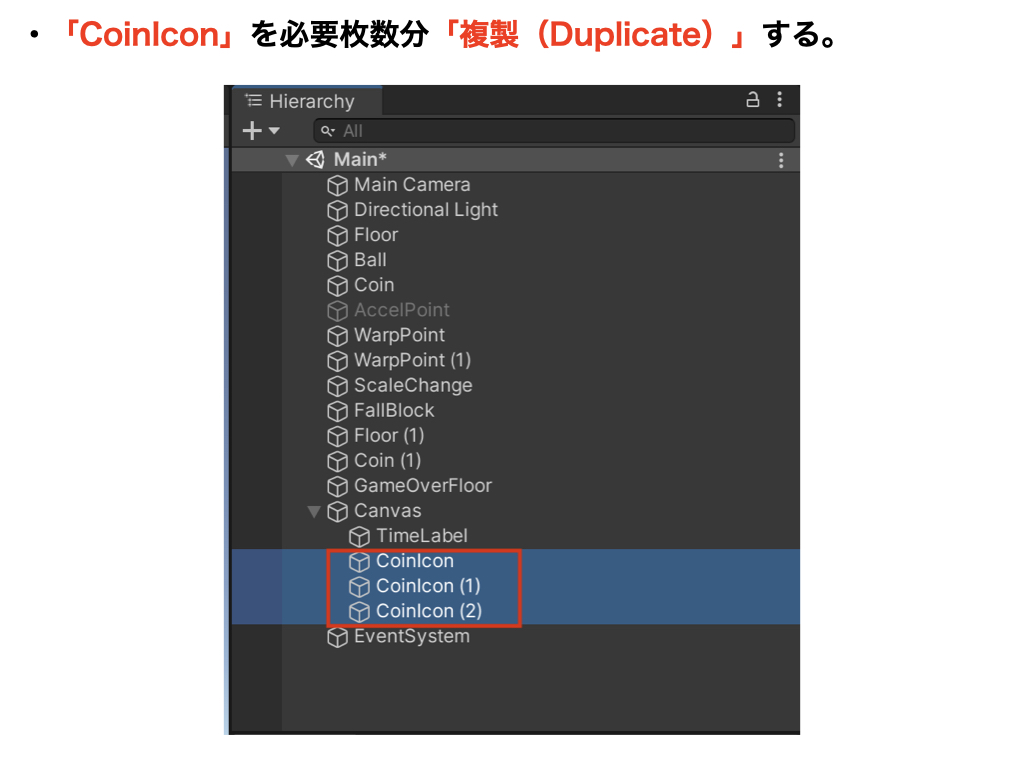
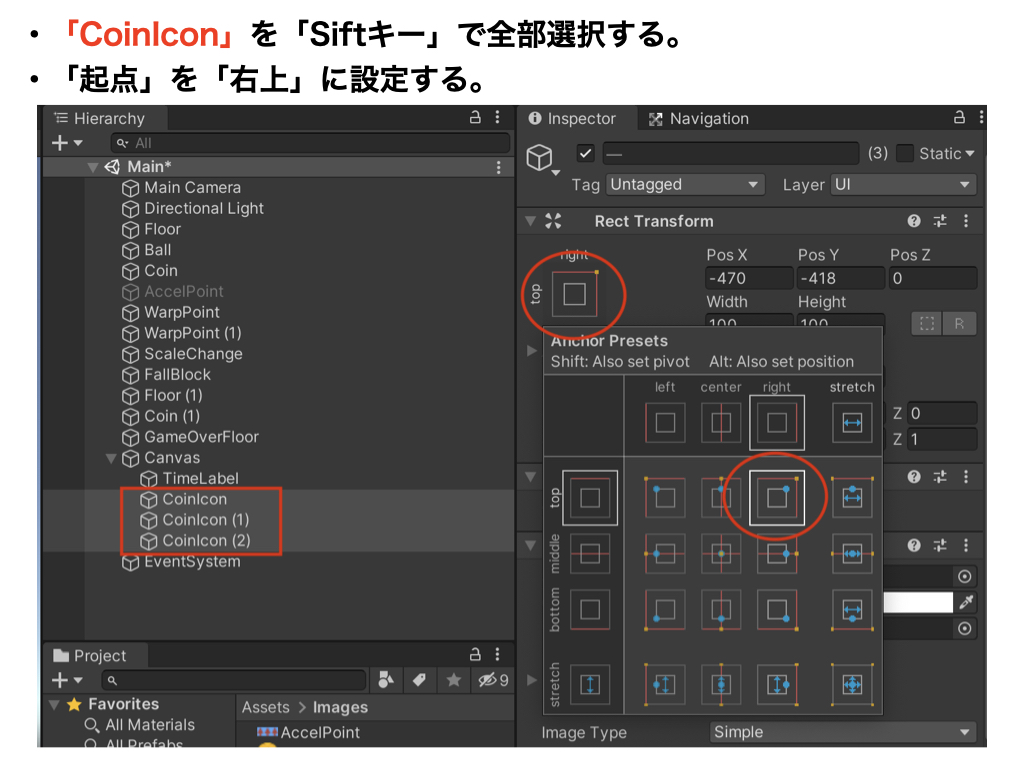
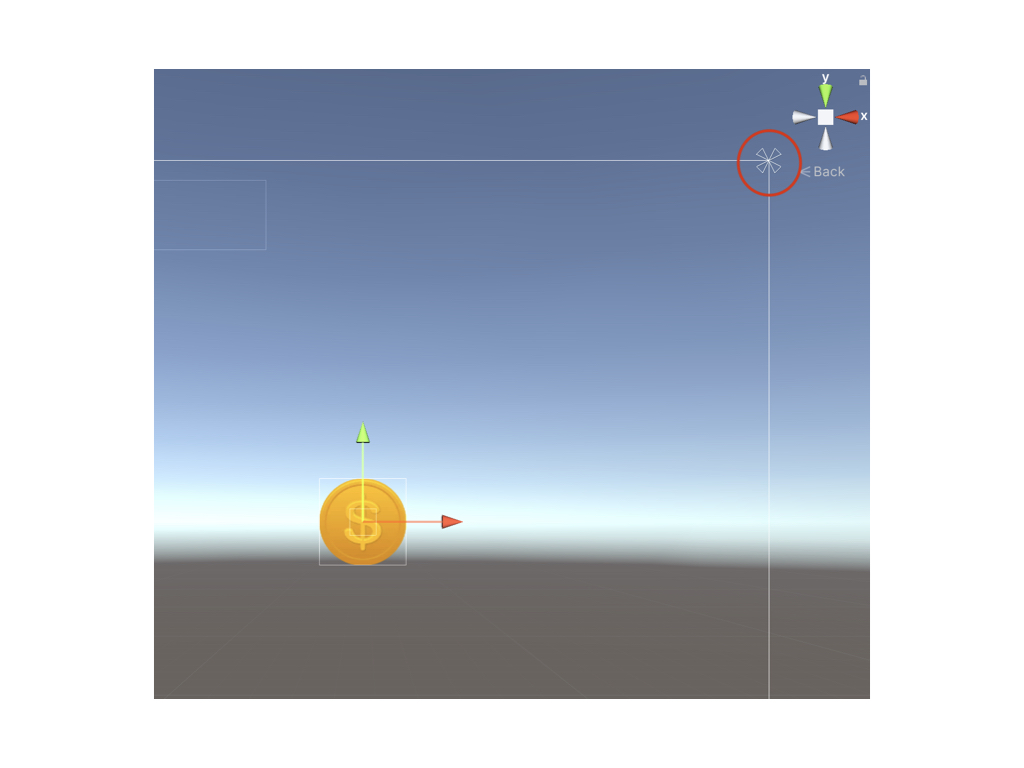
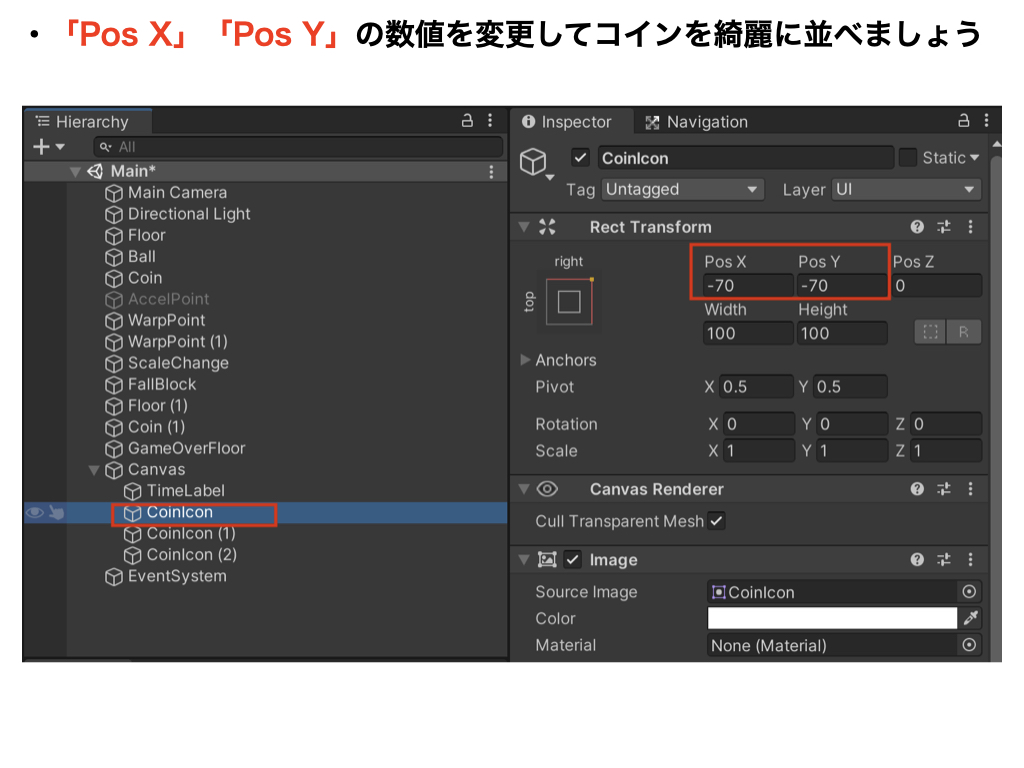
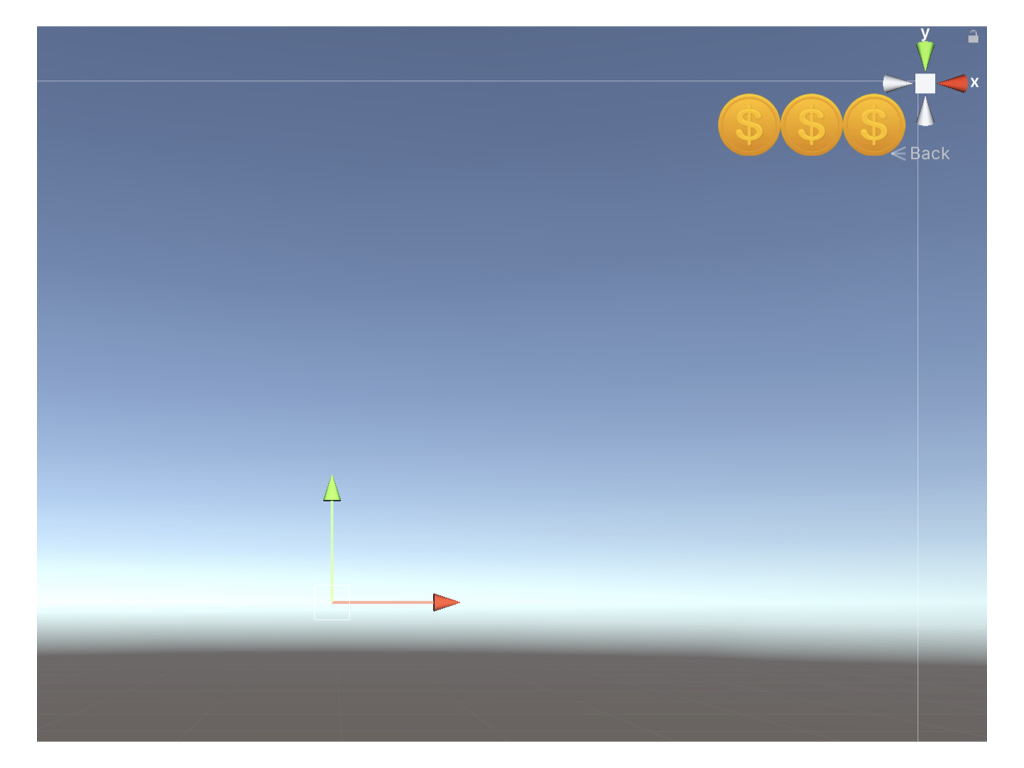
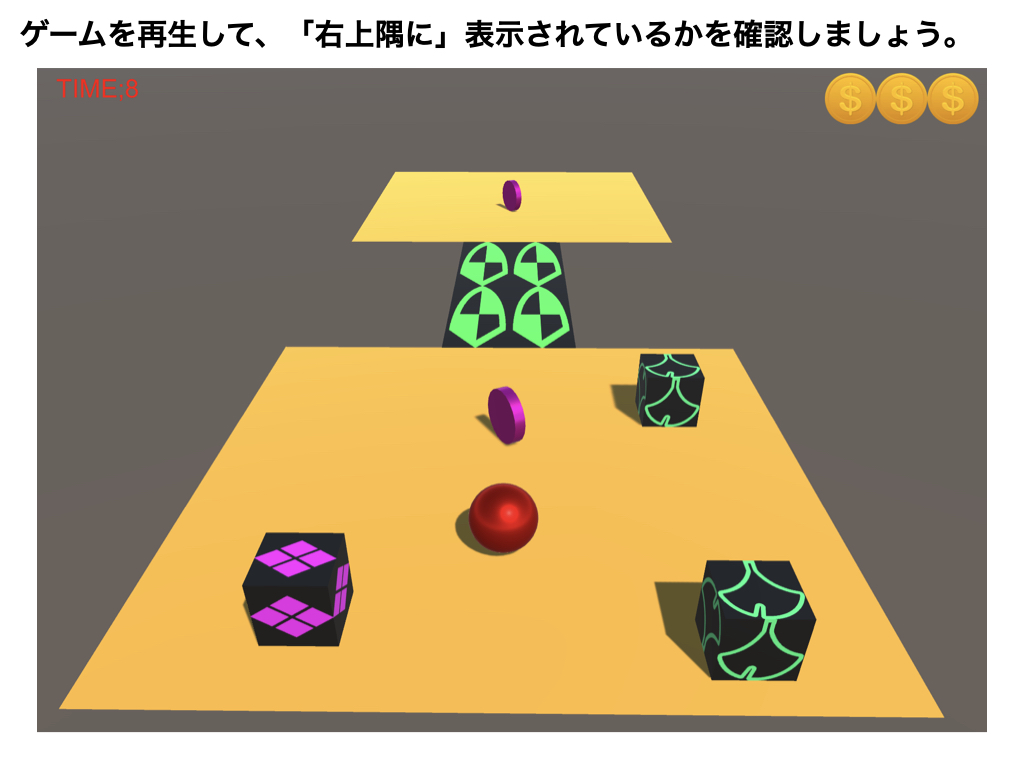
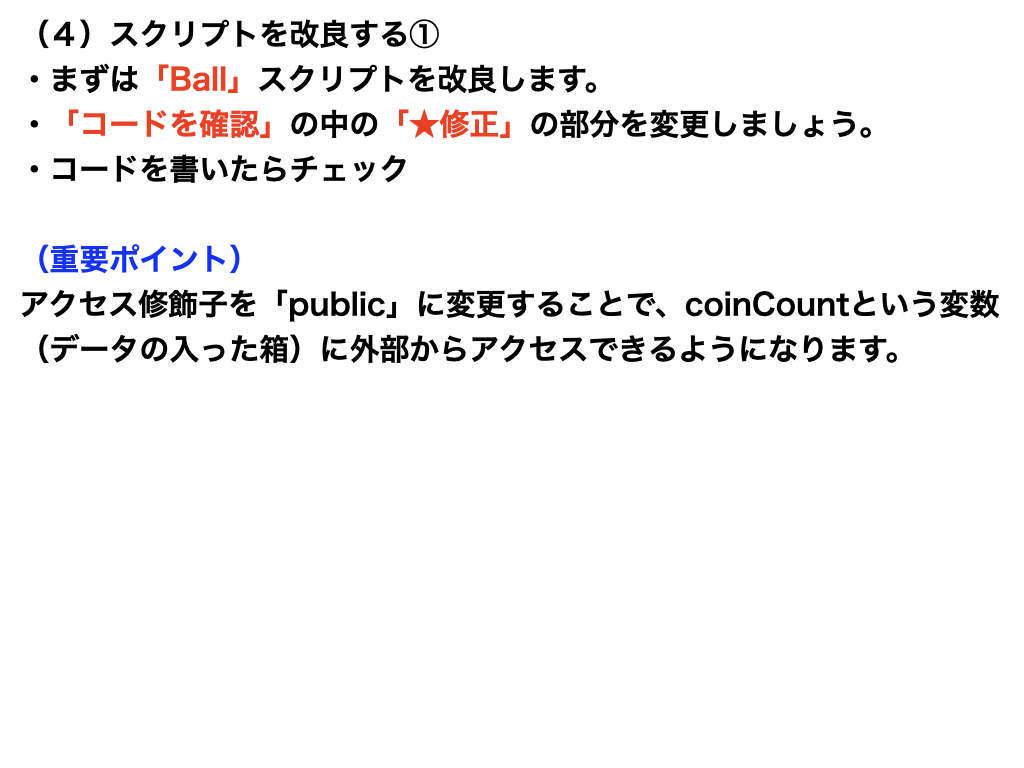
コインアイコンを表示する
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
// ★修正(privateをpublicに変更する)
public int coinCount = 0;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
coinCount += 1;
if(coinCount == 3)
{
SceneManager.LoadScene("GameClear");
}
}
}
private void OnCollisionEnter(Collision collision)
{
if(collision.gameObject.CompareTag("Floor"))
{
isJumping = false;
}
}
}
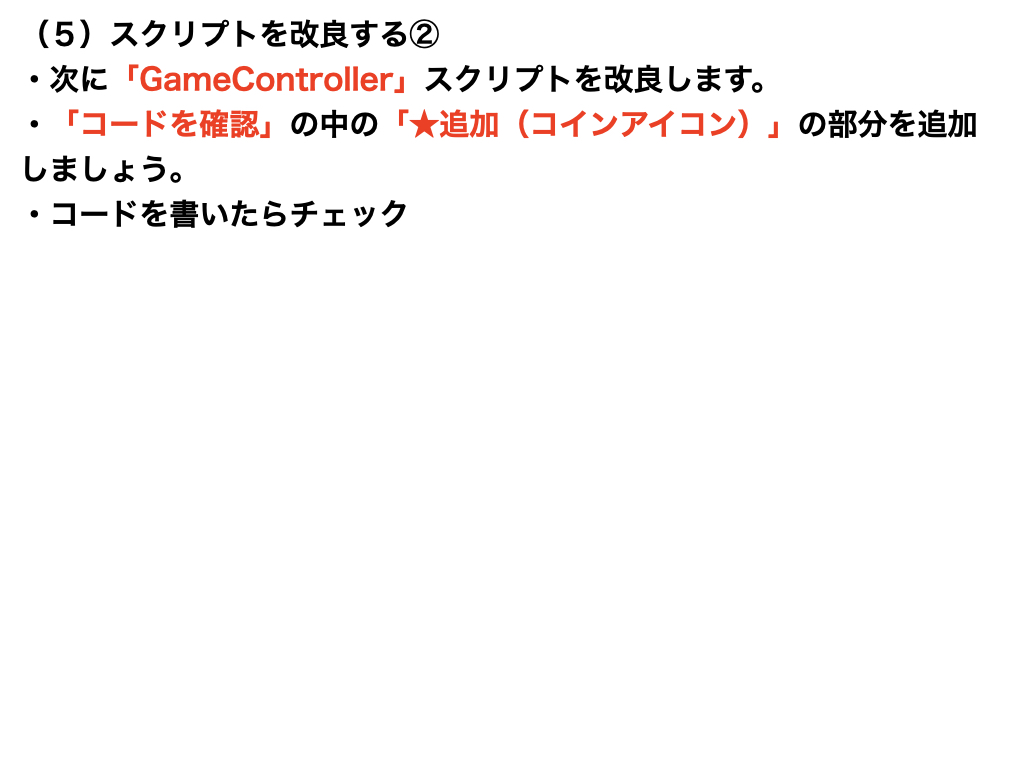
コインアイコンの表示
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class GameController : MonoBehaviour
{
public Text timeLabel;
public float timeCount;
// ★追加(コインアイコン)
// 配列(複数のデータを入れることのできる仕切りのある箱)
public GameObject[] coinIcons;
private Ball ballScript;
private int getCoin;
void Start()
{
timeLabel.text = "TIME;" + timeCount;
// ★追加(コインアイコン)
// 「Ball」オブジェクトに付いている「Ball」スクリプトの情報を取得する。
ballScript = GameObject.Find("Ball").GetComponent<Ball>();
}
void Update()
{
timeCount -= Time.deltaTime;
timeLabel.text = "TIME;" + timeCount.ToString("0");
if (timeCount < 0)
{
SceneManager.LoadScene("GameOver");
}
// ★追加(コインアイコン)
// 「Ball」スクリプトの中にある「coinCount」の情報を取得する。
getCoin = ballScript.coinCount;
// for文(繰り返し文)
for (int i = 0; i < coinIcons.Length; i++)
{
if(getCoin <= i)
{
// コインアイコンを表示状態にする。
coinIcons[i].SetActive(true);
}
else
{
// コインアイコンを非表示状態にする。
coinIcons[i].SetActive(false);
}
}
}
}
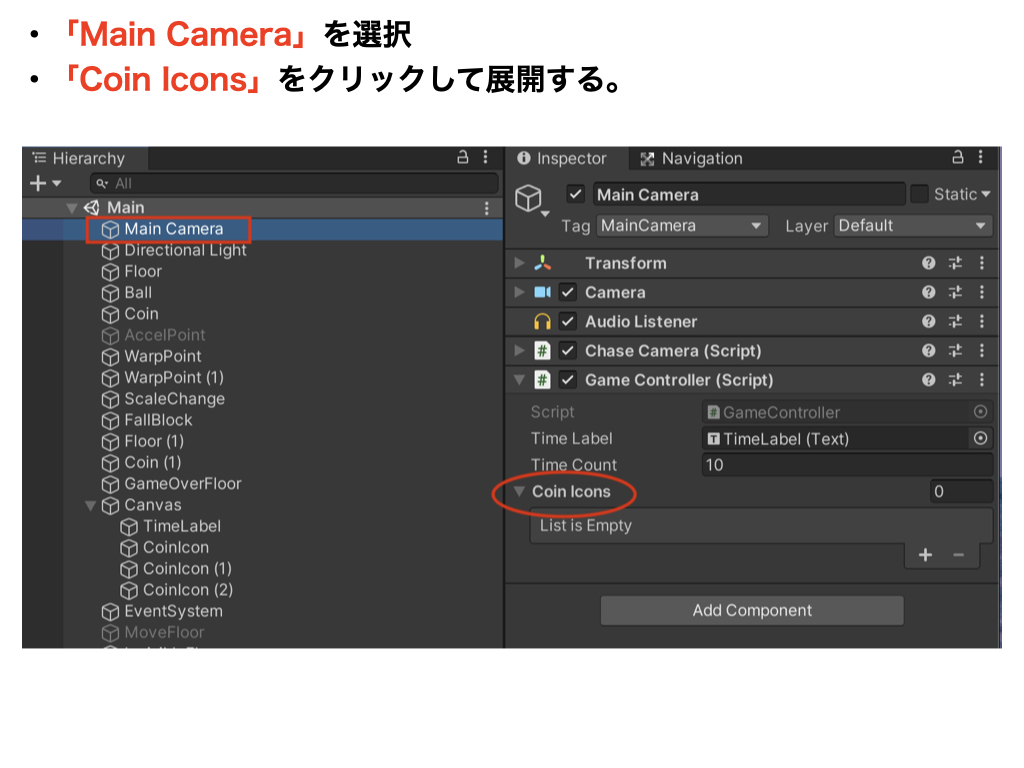
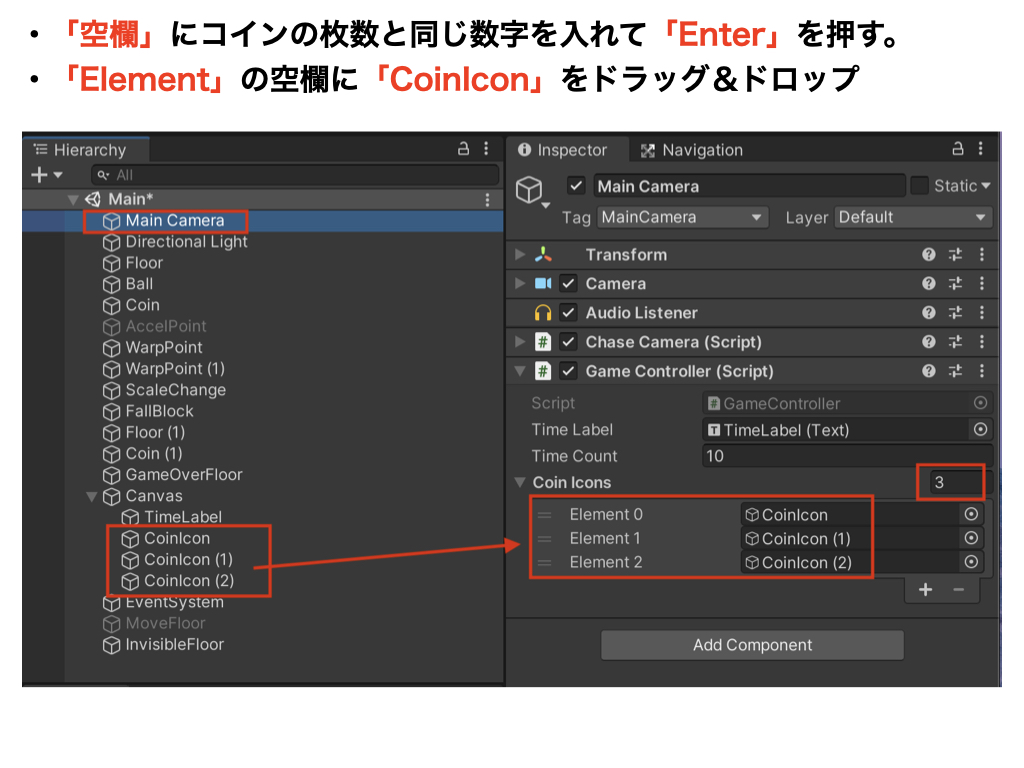
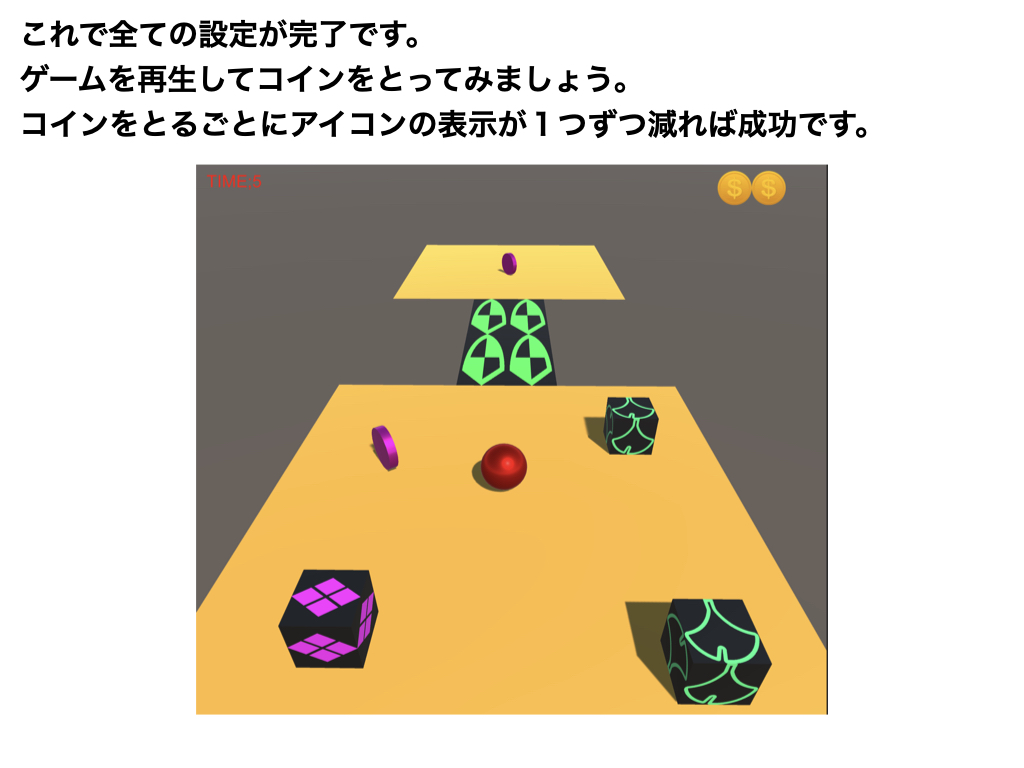
コインの残り枚数を表示する