ショップ・システムの作成③(購入ボタンの作成)
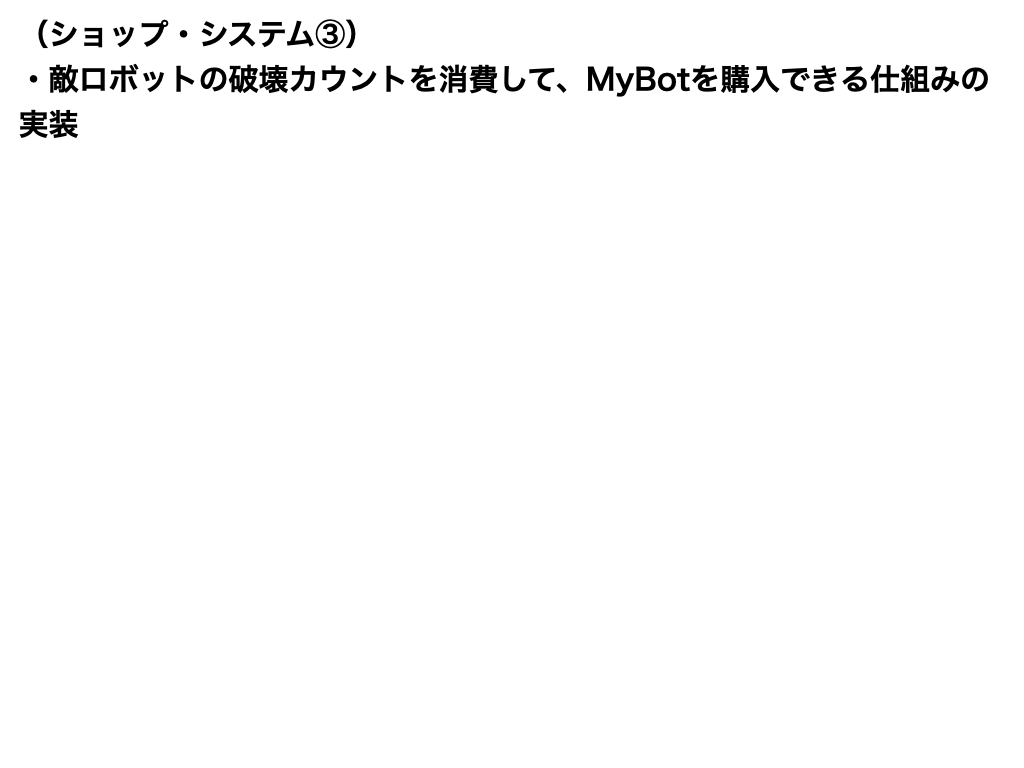
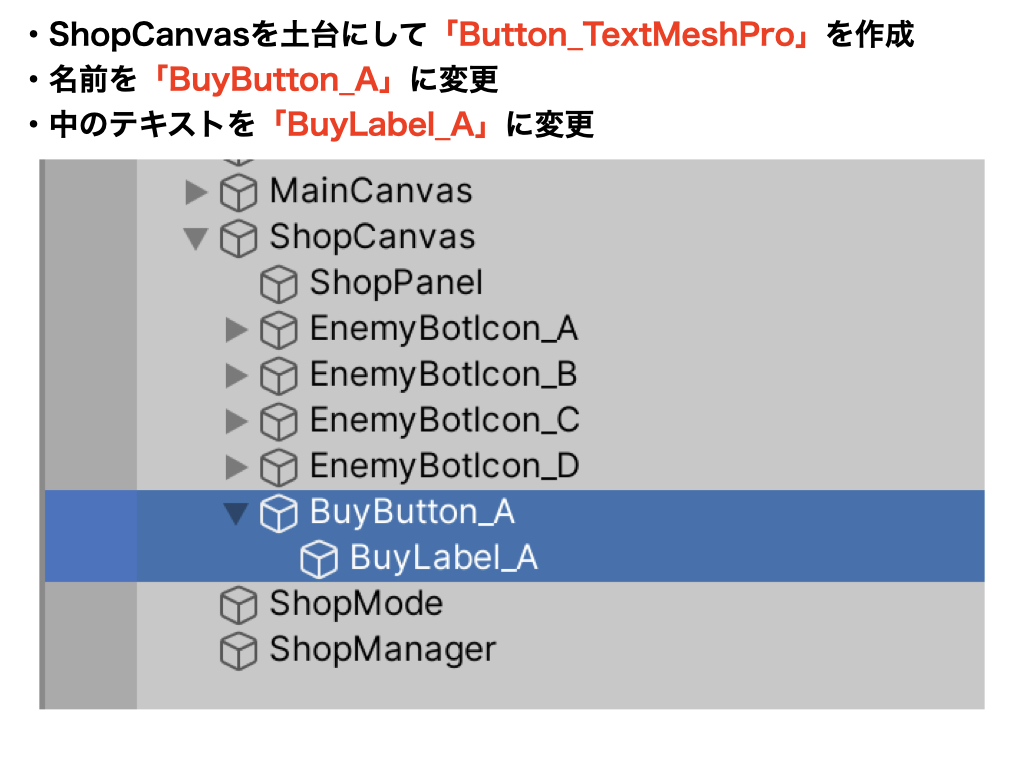
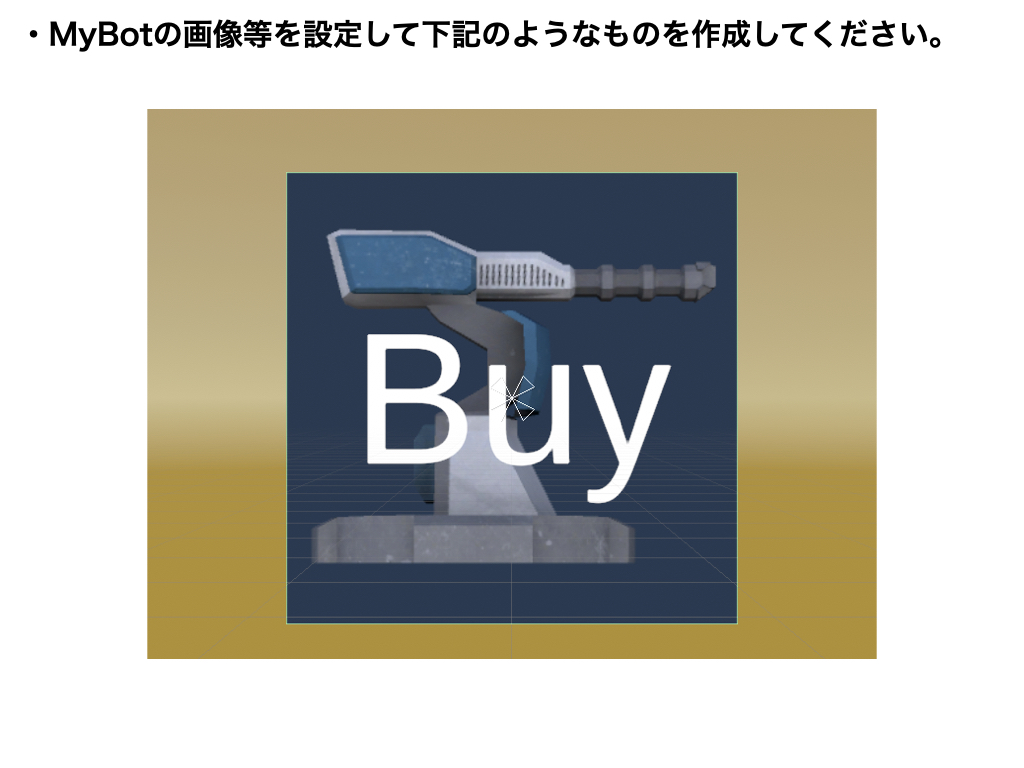
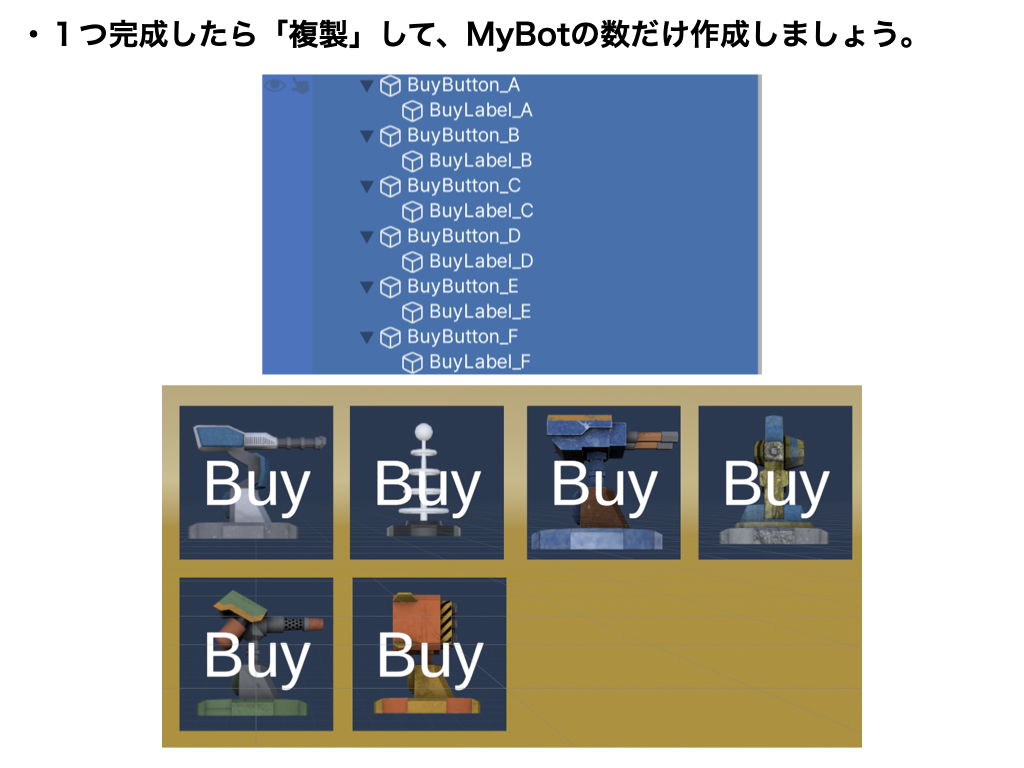
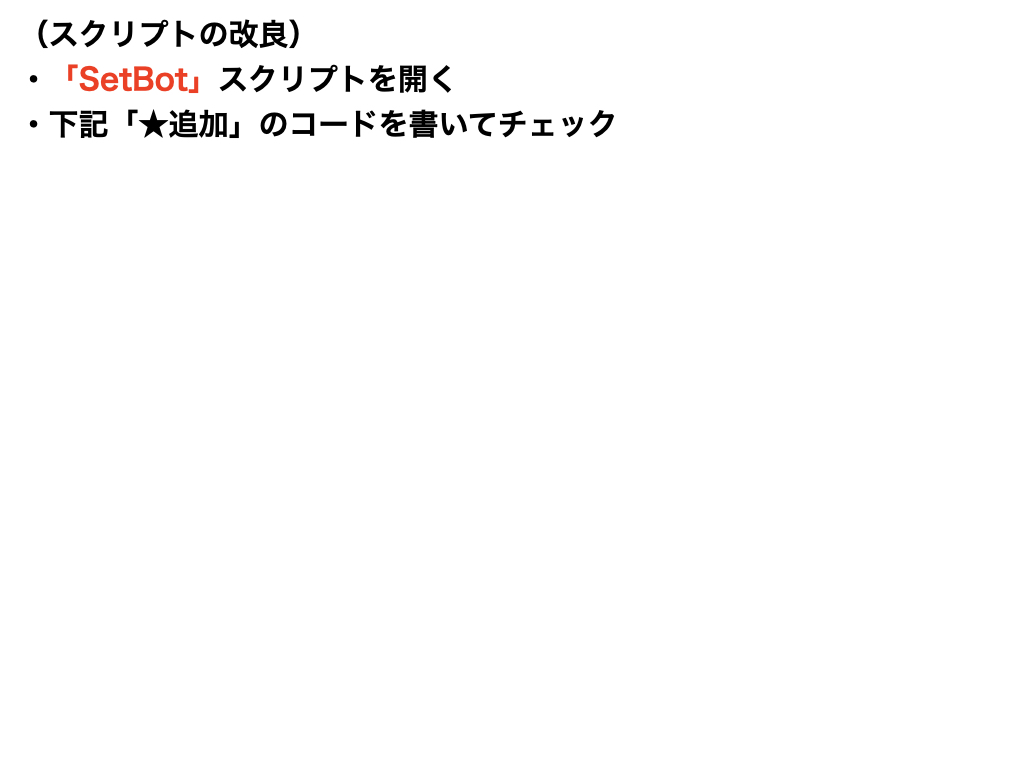
残機数を増加させる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using TMPro;
public class SetBot : MonoBehaviour
{
private GameObject target;
public GameObject[] myBotsPrefab;
private int num = 0;
public AudioClip selectSound;
public AudioClip NGSound;
public Material NGMark;
public GameObject[] botIcons;
private int[] botCounts = new int[6];
public TextMeshProUGUI[] botCountLabels;
private void Start()
{
for (int i = 0; i < botIcons.Length; i++)
{
if (i == num)
{
botIcons[i].SetActive(true);
}
else
{
botIcons[i].SetActive(false);
}
}
botCounts[0] = 2; // MyBot_A
botCounts[1] = 2; // MyBot_B
botCounts[2] = 2; // MyBot_C
botCounts[3] = 2; // MyBot_D
botCounts[4] = 2; // MyBot_E
botCounts[5] = 2; // MyBot_F
for (int i = 0; i < botCountLabels.Length; i++)
{
botCountLabels[i].text = "" + botCounts[i];
}
}
void Update()
{
if (Time.timeScale != 1)
{
return;
}
if (Input.GetKeyDown(KeyCode.Mouse1))
{
num = (num + 1) % myBotsPrefab.Length;
AudioSource.PlayClipAtPoint(selectSound, Camera.main.transform.position);
for (int i = 0; i < botIcons.Length; i++)
{
if (i == num)
{
botIcons[i].SetActive(true);
}
else
{
botIcons[i].SetActive(false);
}
}
}
RaycastHit hit;
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
if (Physics.Raycast(ray, out hit))
{
target = hit.collider.gameObject;
if (Input.GetKeyDown(KeyCode.Mouse0))
{
if (target.tag == "Block")
{
if (botCounts[num] < 1)
{
AudioSource.PlayClipAtPoint(NGSound, Camera.main.transform.position);
return;
}
GameObject bot = Instantiate(myBotsPrefab[num], target.transform.position, Quaternion.identity);
target.tag = "NG";
target.GetComponent<MeshRenderer>().material = NGMark;
botCounts[num] -= 1;
botCountLabels[num].text = "" + botCounts[num];
}
else
{
AudioSource.PlayClipAtPoint(NGSound, Camera.main.transform.position);
}
}
}
}
// ★追加
// 残機数を増加させるメソッド(外部からアクセスできるようにする)
public void AddBotCount(int num)
{
botCounts[num] += 1;
botCountLabels[num].text = "" + botCounts[num];
}
}
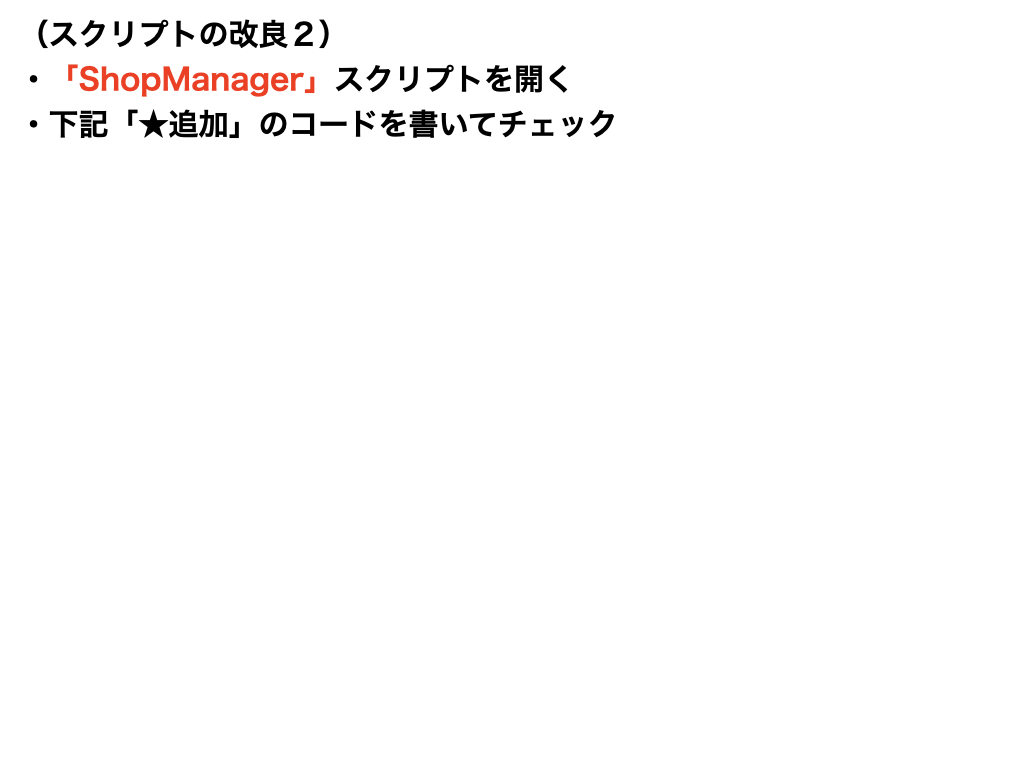
購入条件の設定
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using TMPro;
public class ShopManager : MonoBehaviour
{
private int[] enemyCount = new int[4];
public TextMeshProUGUI[] enemyLabel;
// ★追加
private AudioSource audioS;
public AudioClip buySound;
public AudioClip NGSound;
void Start()
{
enemyLabel[0].text = "" + enemyCount[0];
enemyLabel[1].text = "" + enemyCount[1];
enemyLabel[2].text = "" + enemyCount[2];
enemyLabel[3].text = "" + enemyCount[3];
// ★追加
audioS = GetComponent<AudioSource>();
}
public void AddEnemyCount(int num)
{
enemyCount[num] += 1;
enemyLabel[num].text = "" + enemyCount[num];
}
// ★追加
public void BuyMyBot(int botNum, int priceA, int priceB, int priceC, int priceD)
{
// 購入条件の設定(ポイント)
if (enemyCount[0] >= priceA && enemyCount[1] >= priceB && enemyCount[2] >= priceC && enemyCount[3] >= priceD)
{
GameObject.Find("SetBot").GetComponent<SetBot>().AddBotCount(botNum);
// MyBotの購入に必要分だけ破壊カウントを減少させる(=カウントの消費)
enemyCount[0] -= priceA;
enemyCount[1] -= priceB;
enemyCount[2] -= priceC;
enemyCount[3] -= priceD;
// 破壊カウント数の表示の更新
enemyLabel[0].text = "" + enemyCount[0];
enemyLabel[1].text = "" + enemyCount[1];
enemyLabel[2].text = "" + enemyCount[2];
enemyLabel[3].text = "" + enemyCount[3];
// 購入サウンド
audioS.clip = buySound;
audioS.Play();
}
else
{
// 条件に合致しない場合(破壊カウントが不足している場合)
// NGサウンド
audioS.clip = NGSound;
audioS.Play();
}
}
}
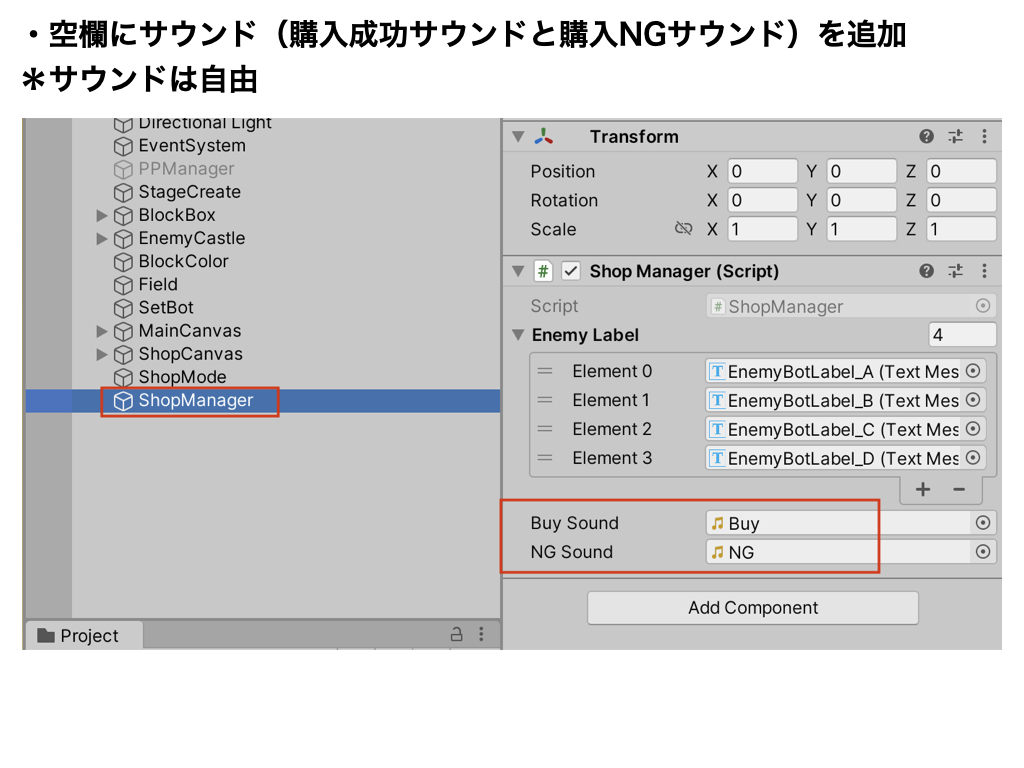
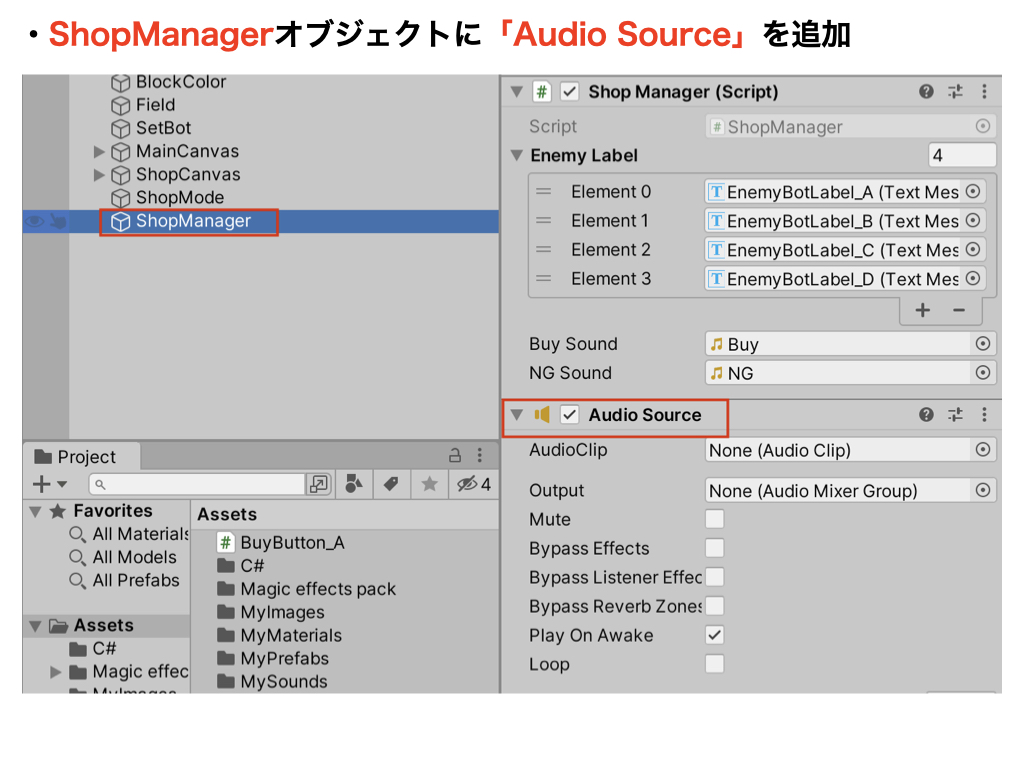
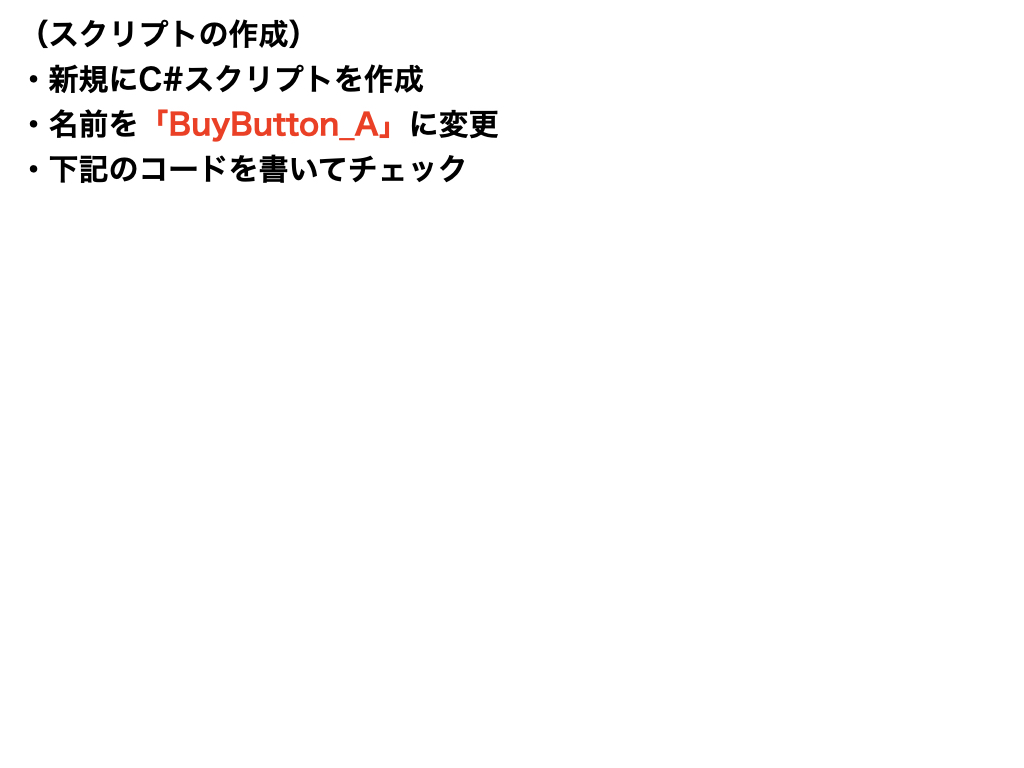
購入ボタン
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BuyButton_A : MonoBehaviour
{
public void OnBuyButtonClicked()
{
GameObject.Find("ShopManager").GetComponent<ShopManager>().BuyMyBot(0, 2, 0, 0, 0); // ここの数字が何を表しているか確認すること
}
}
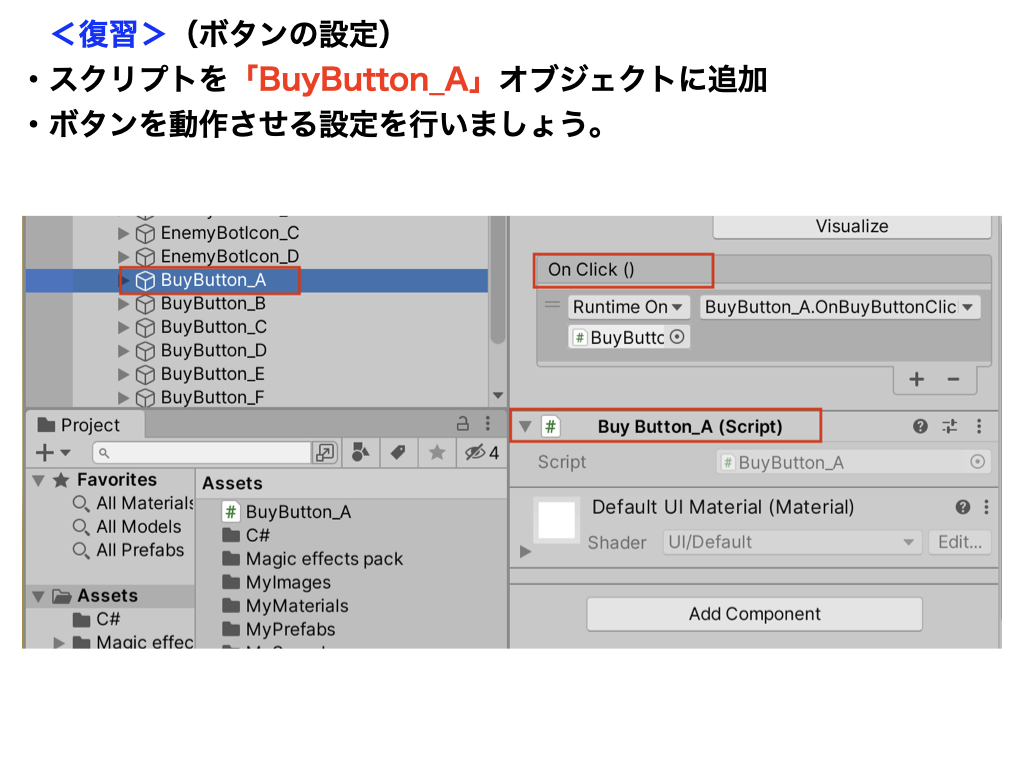
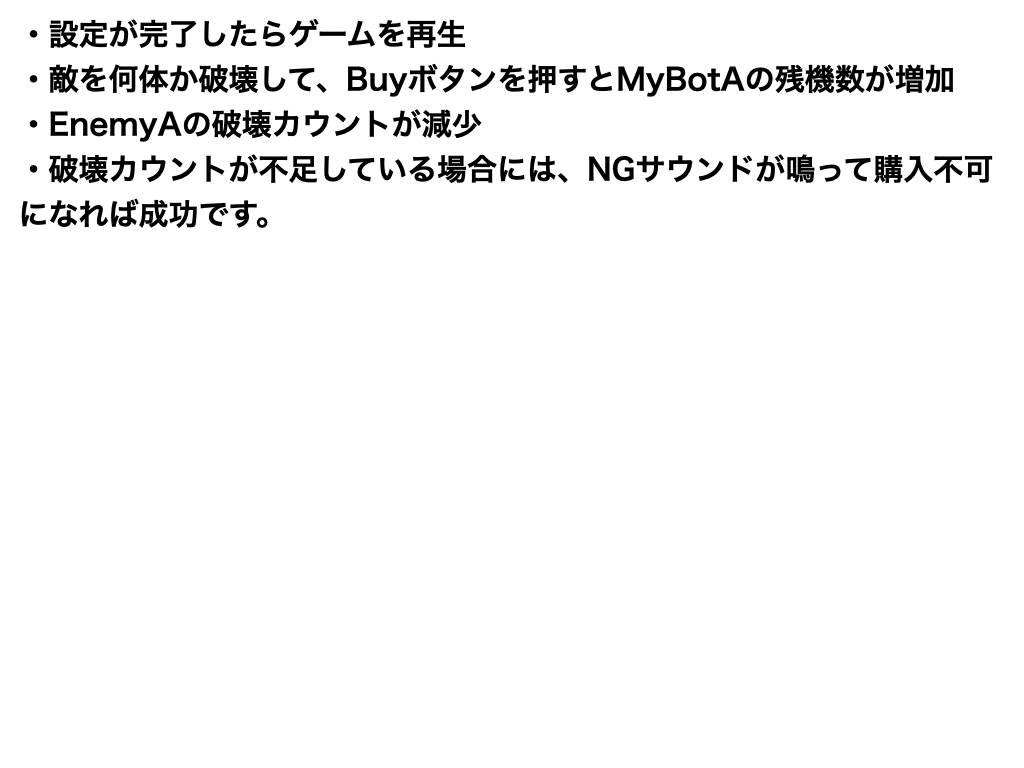
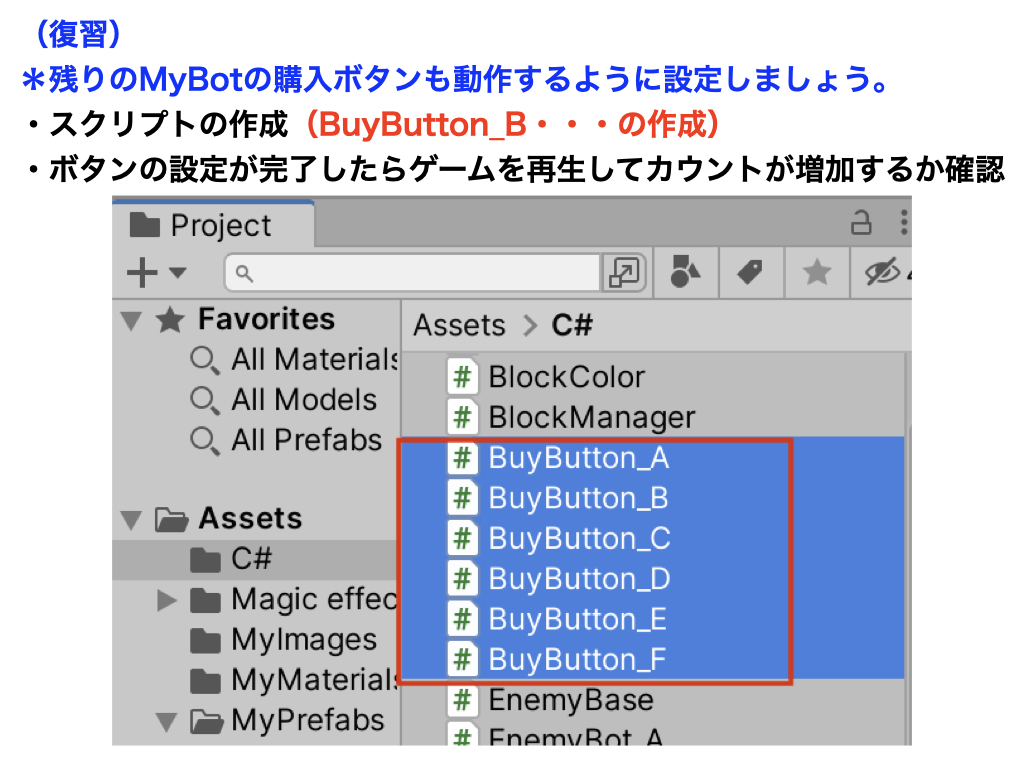
【2021版】TowerD Ⅱ(全17回)
他のコースを見る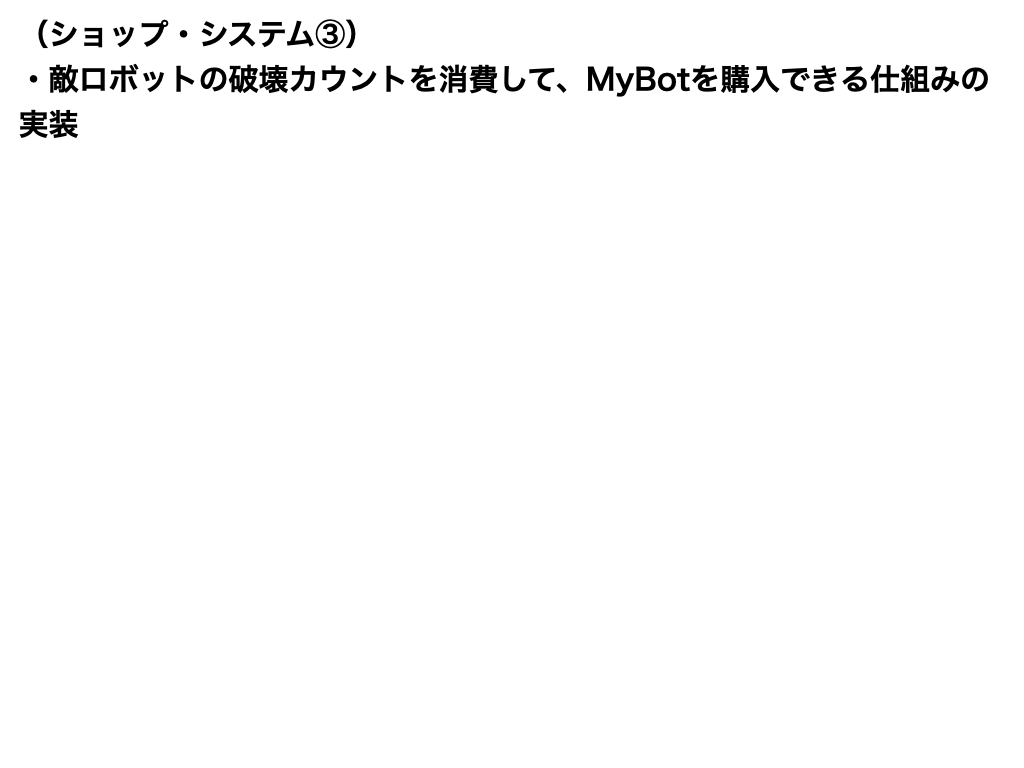
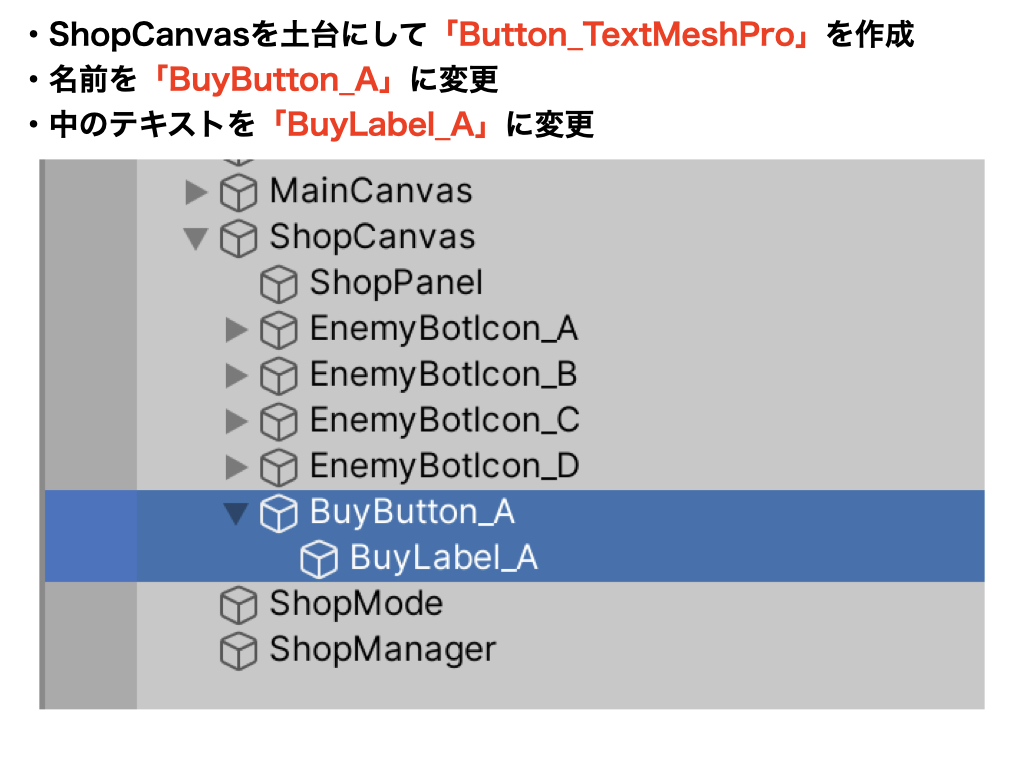
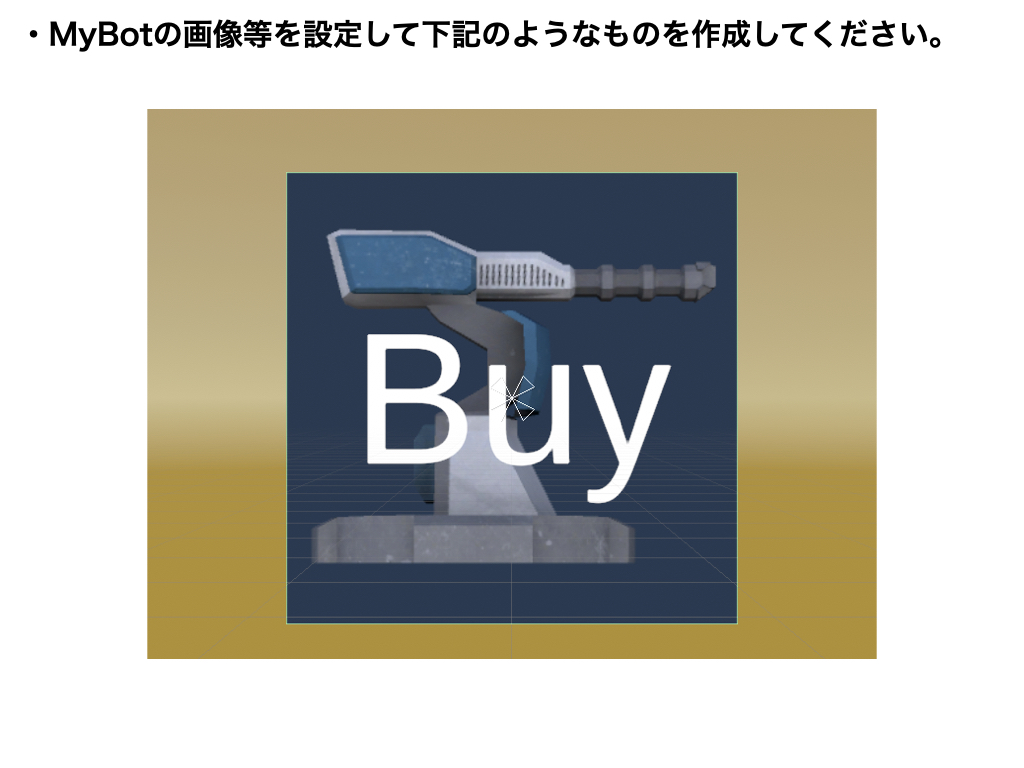
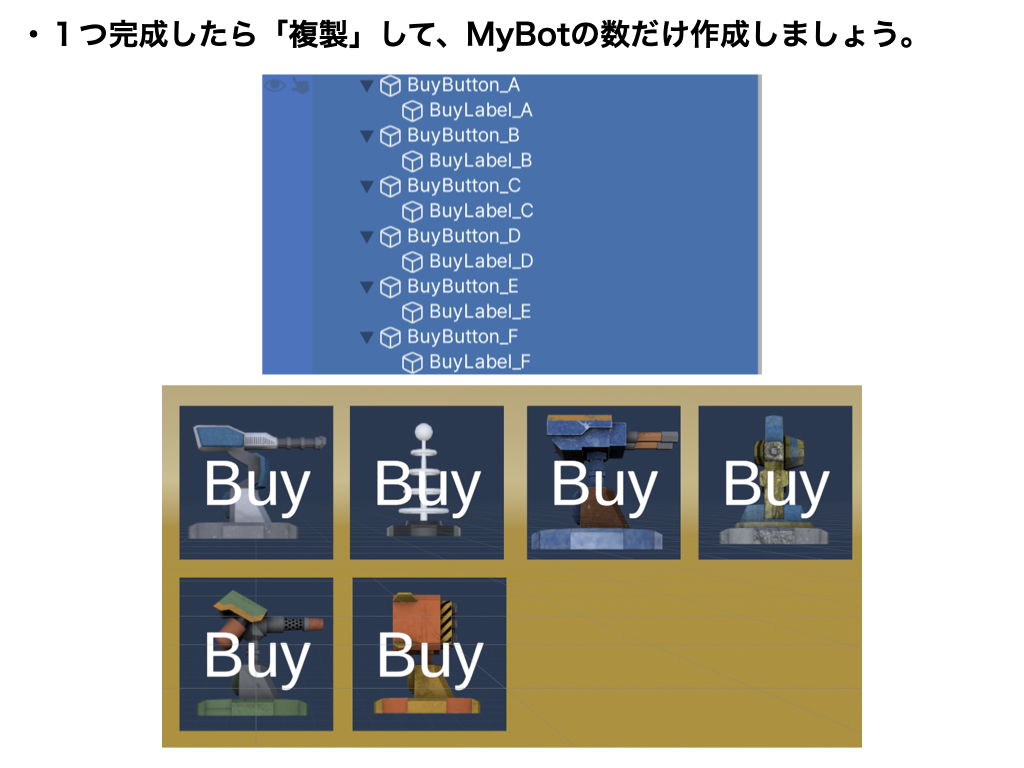
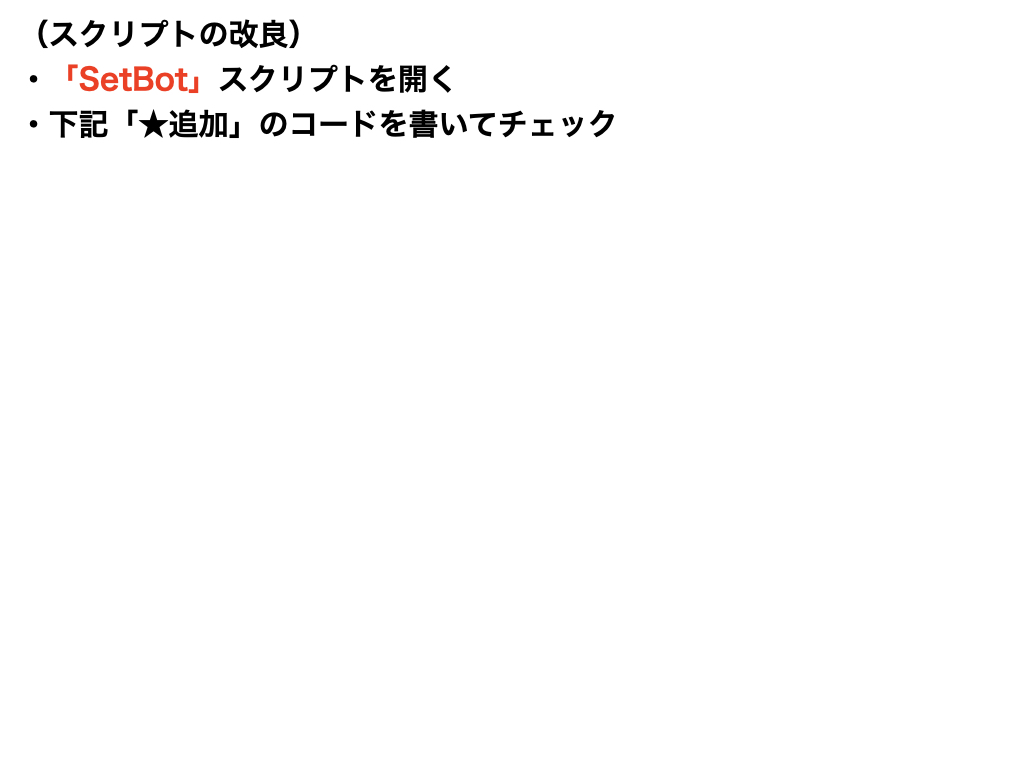
残機数を増加させる
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using TMPro;
public class SetBot : MonoBehaviour
{
private GameObject target;
public GameObject[] myBotsPrefab;
private int num = 0;
public AudioClip selectSound;
public AudioClip NGSound;
public Material NGMark;
public GameObject[] botIcons;
private int[] botCounts = new int[6];
public TextMeshProUGUI[] botCountLabels;
private void Start()
{
for (int i = 0; i < botIcons.Length; i++)
{
if (i == num)
{
botIcons[i].SetActive(true);
}
else
{
botIcons[i].SetActive(false);
}
}
botCounts[0] = 2; // MyBot_A
botCounts[1] = 2; // MyBot_B
botCounts[2] = 2; // MyBot_C
botCounts[3] = 2; // MyBot_D
botCounts[4] = 2; // MyBot_E
botCounts[5] = 2; // MyBot_F
for (int i = 0; i < botCountLabels.Length; i++)
{
botCountLabels[i].text = "" + botCounts[i];
}
}
void Update()
{
if (Time.timeScale != 1)
{
return;
}
if (Input.GetKeyDown(KeyCode.Mouse1))
{
num = (num + 1) % myBotsPrefab.Length;
AudioSource.PlayClipAtPoint(selectSound, Camera.main.transform.position);
for (int i = 0; i < botIcons.Length; i++)
{
if (i == num)
{
botIcons[i].SetActive(true);
}
else
{
botIcons[i].SetActive(false);
}
}
}
RaycastHit hit;
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
if (Physics.Raycast(ray, out hit))
{
target = hit.collider.gameObject;
if (Input.GetKeyDown(KeyCode.Mouse0))
{
if (target.tag == "Block")
{
if (botCounts[num] < 1)
{
AudioSource.PlayClipAtPoint(NGSound, Camera.main.transform.position);
return;
}
GameObject bot = Instantiate(myBotsPrefab[num], target.transform.position, Quaternion.identity);
target.tag = "NG";
target.GetComponent<MeshRenderer>().material = NGMark;
botCounts[num] -= 1;
botCountLabels[num].text = "" + botCounts[num];
}
else
{
AudioSource.PlayClipAtPoint(NGSound, Camera.main.transform.position);
}
}
}
}
// ★追加
// 残機数を増加させるメソッド(外部からアクセスできるようにする)
public void AddBotCount(int num)
{
botCounts[num] += 1;
botCountLabels[num].text = "" + botCounts[num];
}
}
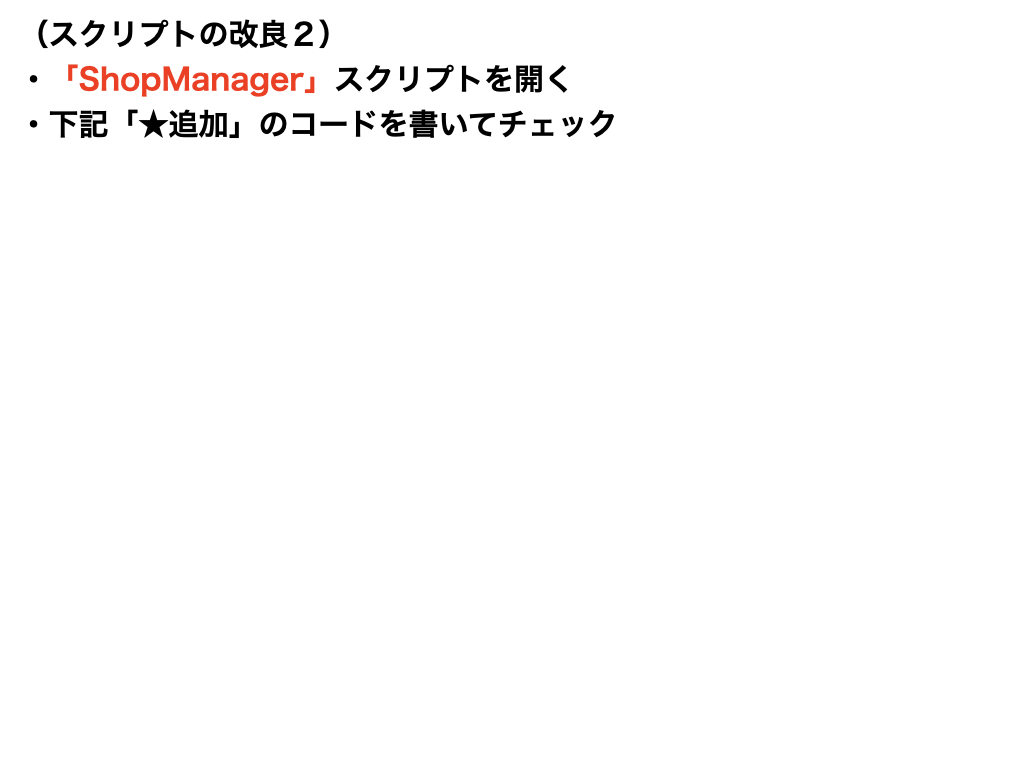
購入条件の設定
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using TMPro;
public class ShopManager : MonoBehaviour
{
private int[] enemyCount = new int[4];
public TextMeshProUGUI[] enemyLabel;
// ★追加
private AudioSource audioS;
public AudioClip buySound;
public AudioClip NGSound;
void Start()
{
enemyLabel[0].text = "" + enemyCount[0];
enemyLabel[1].text = "" + enemyCount[1];
enemyLabel[2].text = "" + enemyCount[2];
enemyLabel[3].text = "" + enemyCount[3];
// ★追加
audioS = GetComponent<AudioSource>();
}
public void AddEnemyCount(int num)
{
enemyCount[num] += 1;
enemyLabel[num].text = "" + enemyCount[num];
}
// ★追加
public void BuyMyBot(int botNum, int priceA, int priceB, int priceC, int priceD)
{
// 購入条件の設定(ポイント)
if (enemyCount[0] >= priceA && enemyCount[1] >= priceB && enemyCount[2] >= priceC && enemyCount[3] >= priceD)
{
GameObject.Find("SetBot").GetComponent<SetBot>().AddBotCount(botNum);
// MyBotの購入に必要分だけ破壊カウントを減少させる(=カウントの消費)
enemyCount[0] -= priceA;
enemyCount[1] -= priceB;
enemyCount[2] -= priceC;
enemyCount[3] -= priceD;
// 破壊カウント数の表示の更新
enemyLabel[0].text = "" + enemyCount[0];
enemyLabel[1].text = "" + enemyCount[1];
enemyLabel[2].text = "" + enemyCount[2];
enemyLabel[3].text = "" + enemyCount[3];
// 購入サウンド
audioS.clip = buySound;
audioS.Play();
}
else
{
// 条件に合致しない場合(破壊カウントが不足している場合)
// NGサウンド
audioS.clip = NGSound;
audioS.Play();
}
}
}
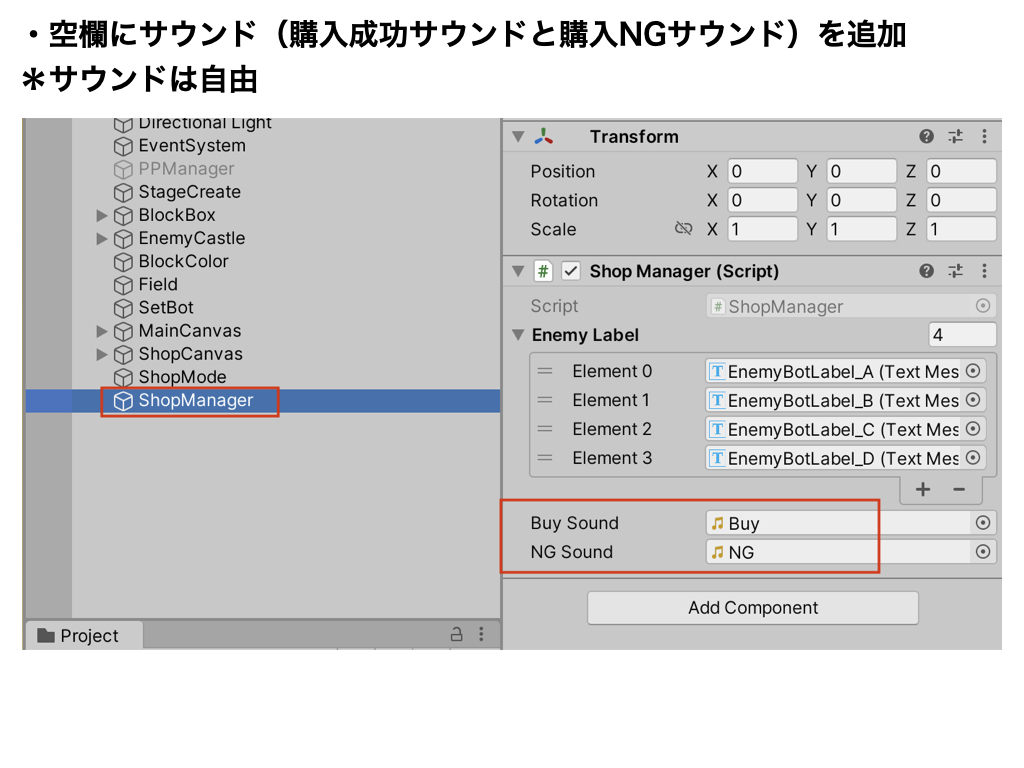
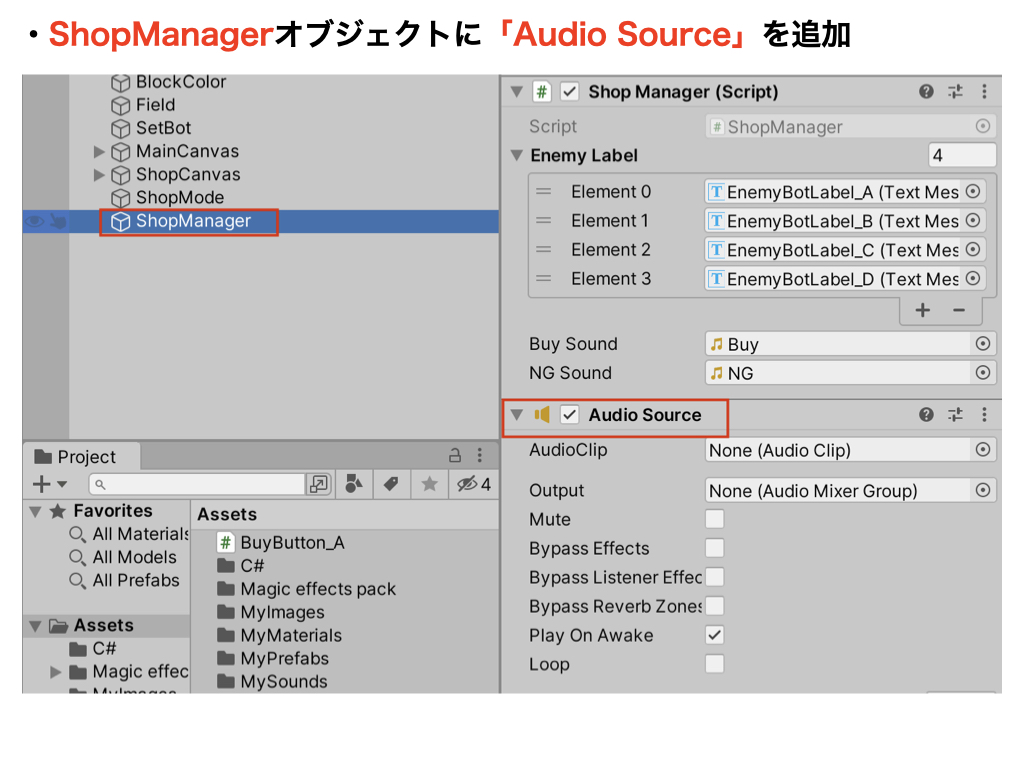
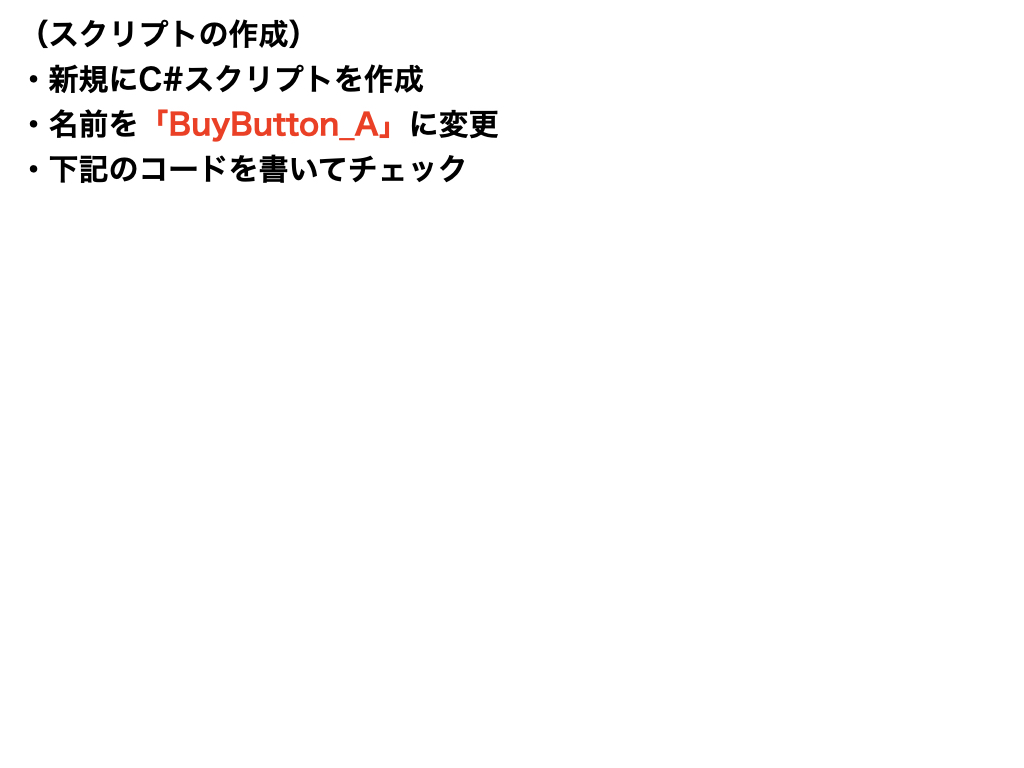
購入ボタン
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BuyButton_A : MonoBehaviour
{
public void OnBuyButtonClicked()
{
GameObject.Find("ShopManager").GetComponent<ShopManager>().BuyMyBot(0, 2, 0, 0, 0); // ここの数字が何を表しているか確認すること
}
}
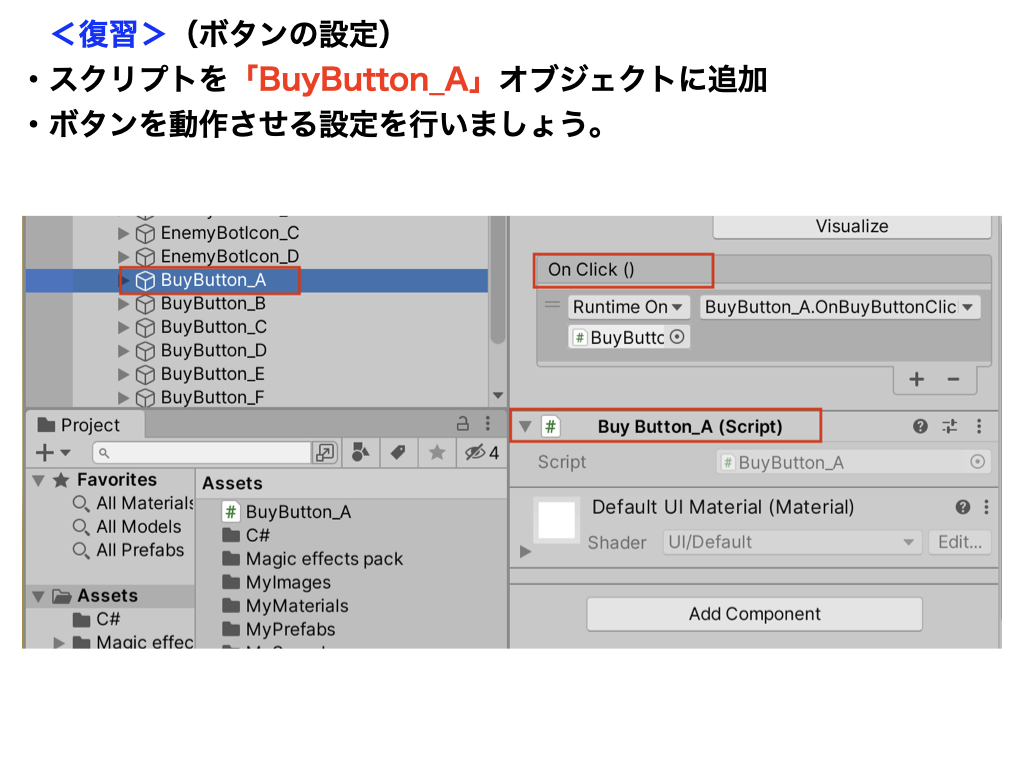
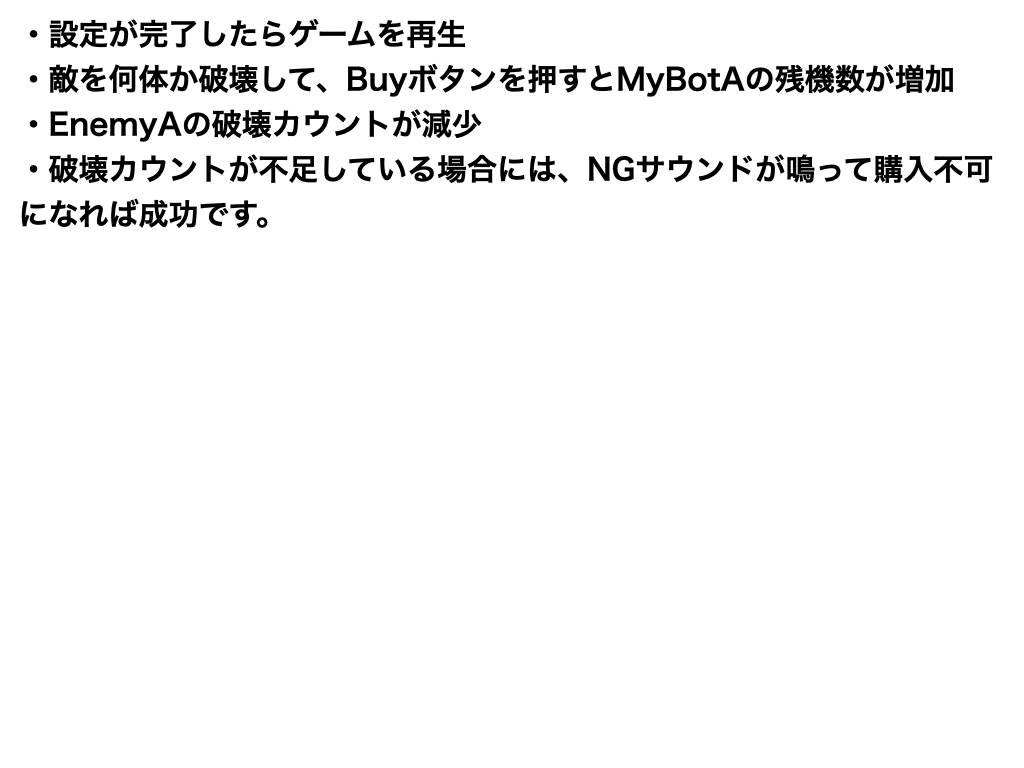
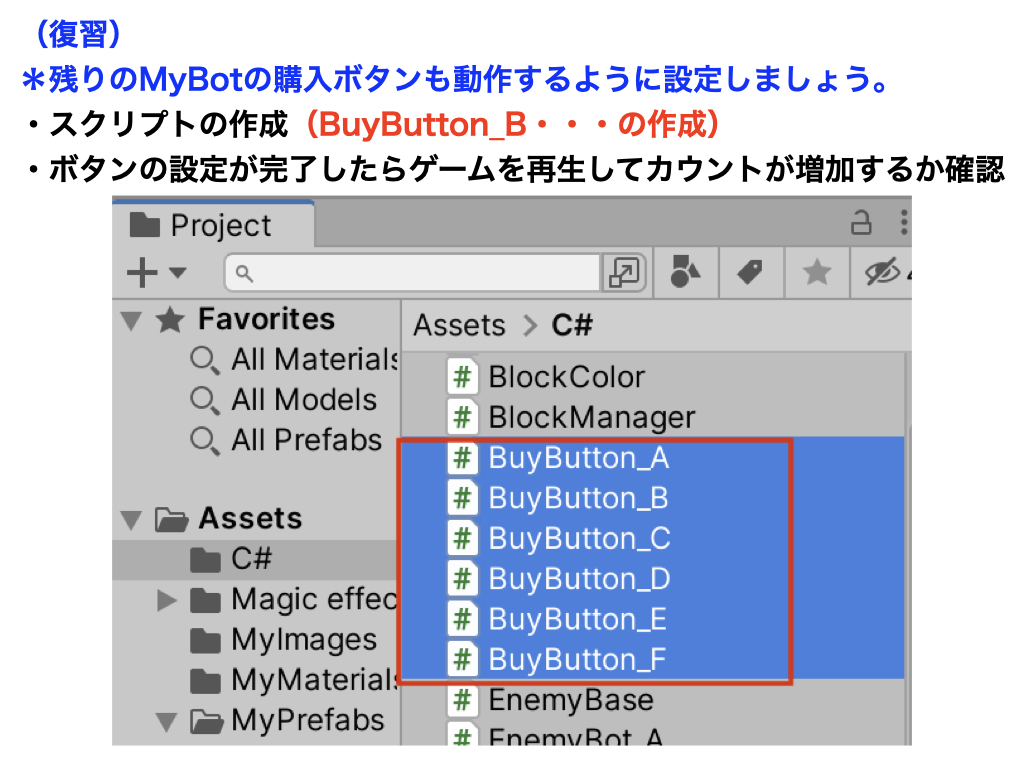
ショップ・システムの作成③(購入ボタンの作成)