MyBotのセット⑤(設置できる数に制限を加える)
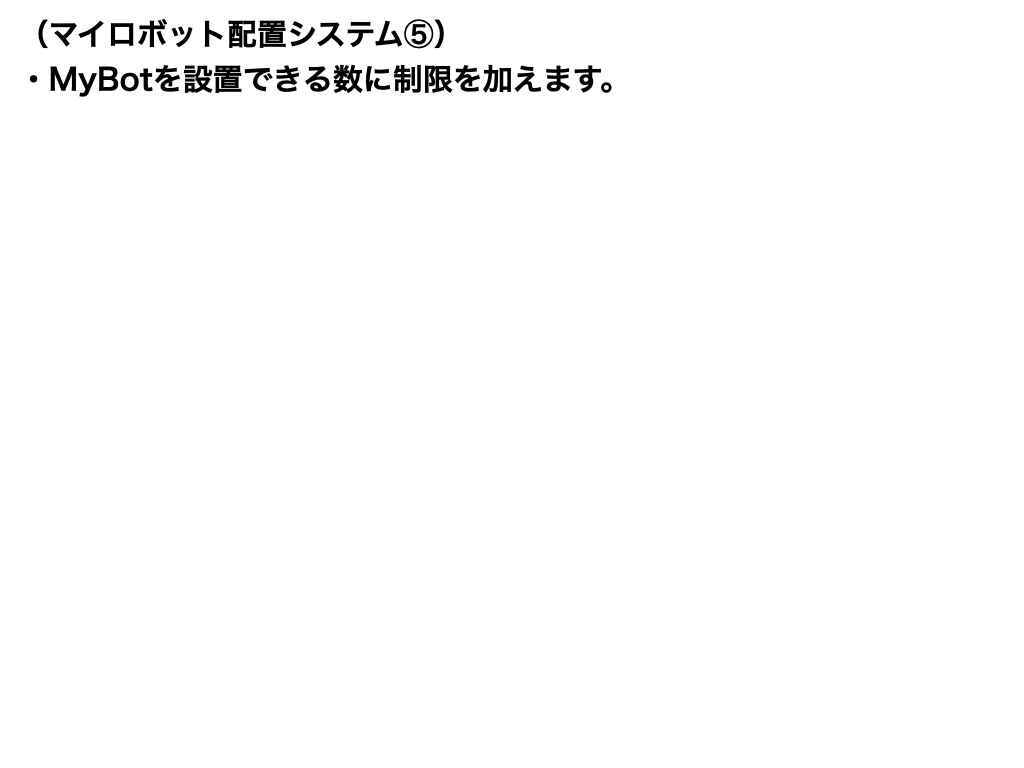
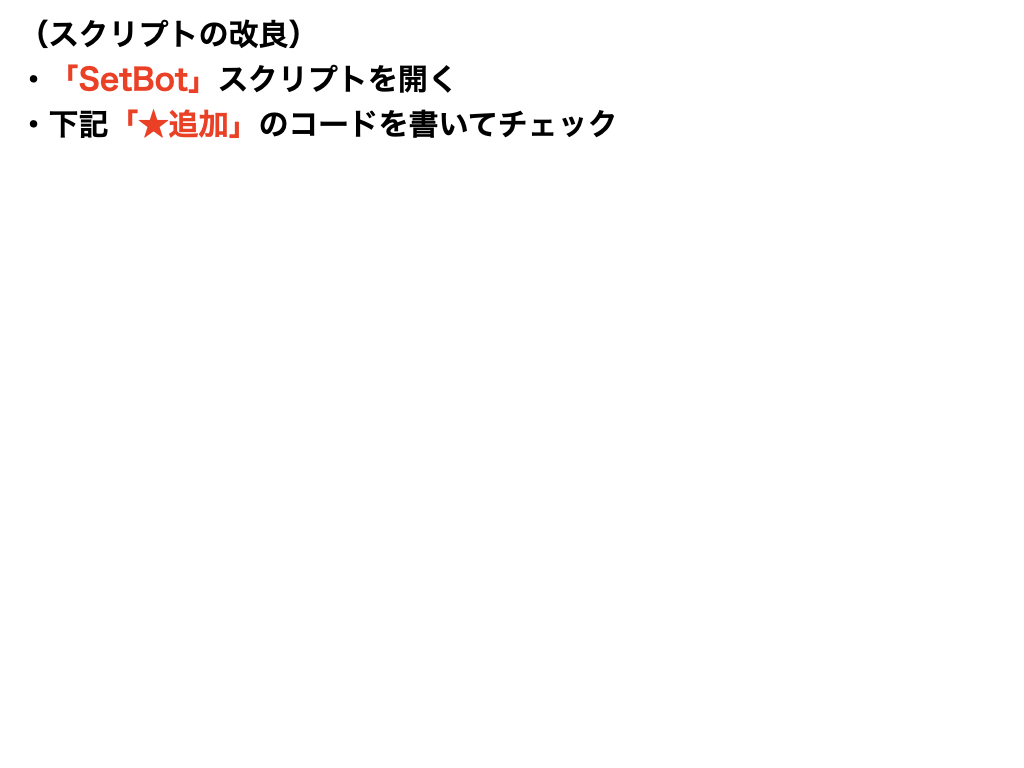
セットできる残機数の設定
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SetBot : MonoBehaviour
{
private GameObject target;
public GameObject[] myBotsPrefab;
private int num = 0;
public AudioClip selectSound;
public AudioClip NGSound;
public Material NGMark;
public GameObject[] botIcons;
// ★追加
// (考え方)各MyBotの残機数を管理する箱を作成する。
private int[] botCounts = new int[6]; // 配列の宣言(数字はMyBotの種類数に合わせること)
private void Start()
{
for (int i = 0; i < botIcons.Length; i++)
{
if (i == num)
{
botIcons[i].SetActive(true);
}
else
{
botIcons[i].SetActive(false);
}
}
// ★追加
// 初期の残機数(いくつにするかは自由)
botCounts[0] = 2; // MyBot_A ←ボットの名前をメモしておくと便利
botCounts[1] = 2; // MyBot_B
botCounts[2] = 2; // MyBot_C
botCounts[3] = 2; // MyBot_D
botCounts[4] = 2; // MyBot_E
botCounts[5] = 2; // MyBot_F
}
void Update()
{
if (Input.GetKeyDown(KeyCode.Mouse1))
{
num = (num + 1) % myBotsPrefab.Length;
AudioSource.PlayClipAtPoint(selectSound, Camera.main.transform.position);
for (int i = 0; i < botIcons.Length; i++)
{
if (i == num)
{
botIcons[i].SetActive(true);
}
else
{
botIcons[i].SetActive(false);
}
}
}
RaycastHit hit;
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
if (Physics.Raycast(ray, out hit))
{
target = hit.collider.gameObject;
if (Input.GetKeyDown(KeyCode.Mouse0))
{
if (target.tag == "Block")
{
// ★追加
// 残機数が不足した場合には、セット不可&NGサウンドを鳴らす。
if(botCounts[num] < 1)
{
AudioSource.PlayClipAtPoint(NGSound, Camera.main.transform.position);
return;
}
GameObject bot = Instantiate(myBotsPrefab[num], target.transform.position, Quaternion.identity);
target.tag = "NG";
target.GetComponent<MeshRenderer>().material = NGMark;
// ★追加
// セットしたMyBotの残機数を1減らす
botCounts[num] -= 1;
}
else
{
AudioSource.PlayClipAtPoint(NGSound, Camera.main.transform.position);
}
}
}
}
}
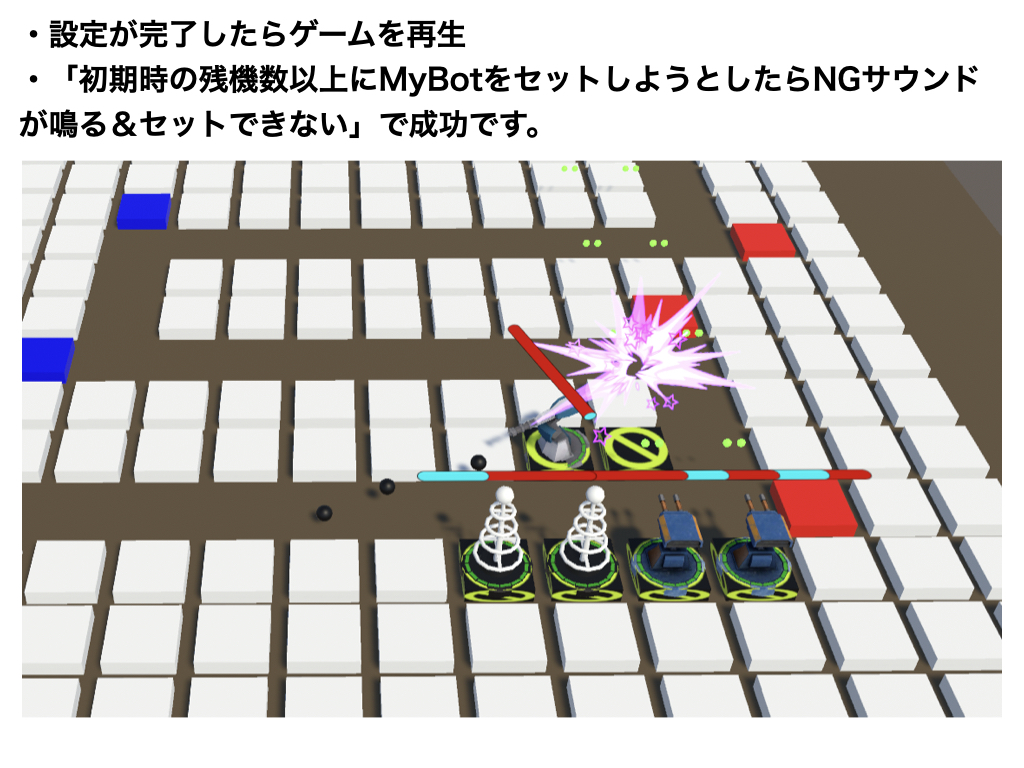
【2021版】TowerD Ⅱ(全17回)
他のコースを見る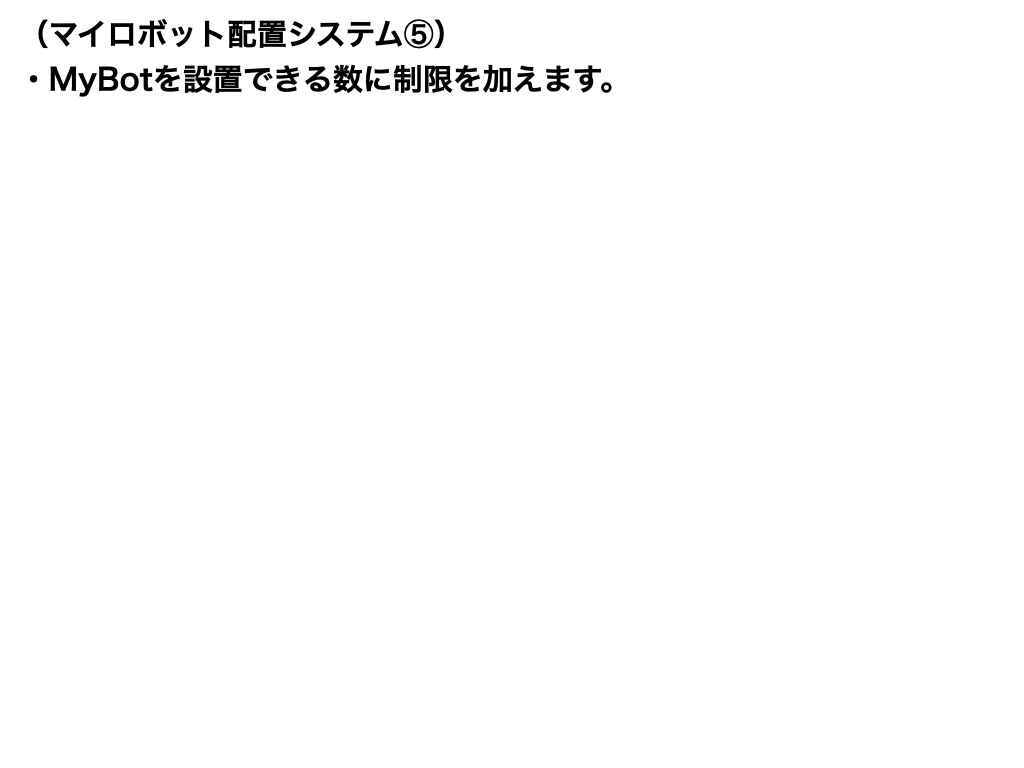
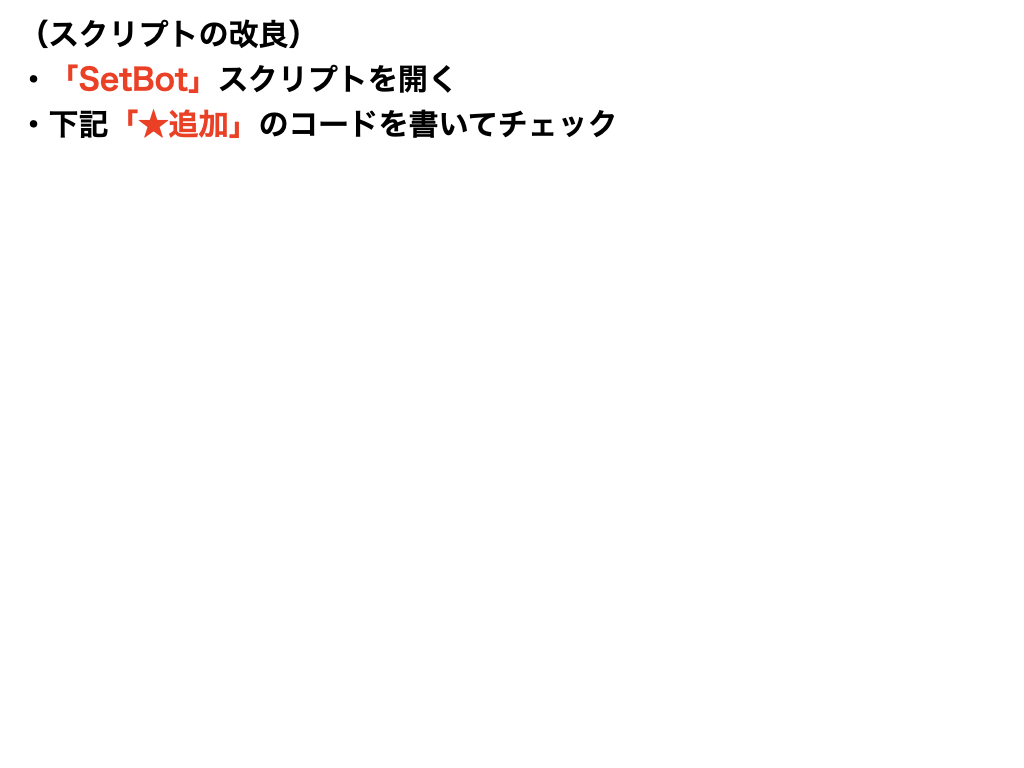
セットできる残機数の設定
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SetBot : MonoBehaviour
{
private GameObject target;
public GameObject[] myBotsPrefab;
private int num = 0;
public AudioClip selectSound;
public AudioClip NGSound;
public Material NGMark;
public GameObject[] botIcons;
// ★追加
// (考え方)各MyBotの残機数を管理する箱を作成する。
private int[] botCounts = new int[6]; // 配列の宣言(数字はMyBotの種類数に合わせること)
private void Start()
{
for (int i = 0; i < botIcons.Length; i++)
{
if (i == num)
{
botIcons[i].SetActive(true);
}
else
{
botIcons[i].SetActive(false);
}
}
// ★追加
// 初期の残機数(いくつにするかは自由)
botCounts[0] = 2; // MyBot_A ←ボットの名前をメモしておくと便利
botCounts[1] = 2; // MyBot_B
botCounts[2] = 2; // MyBot_C
botCounts[3] = 2; // MyBot_D
botCounts[4] = 2; // MyBot_E
botCounts[5] = 2; // MyBot_F
}
void Update()
{
if (Input.GetKeyDown(KeyCode.Mouse1))
{
num = (num + 1) % myBotsPrefab.Length;
AudioSource.PlayClipAtPoint(selectSound, Camera.main.transform.position);
for (int i = 0; i < botIcons.Length; i++)
{
if (i == num)
{
botIcons[i].SetActive(true);
}
else
{
botIcons[i].SetActive(false);
}
}
}
RaycastHit hit;
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
if (Physics.Raycast(ray, out hit))
{
target = hit.collider.gameObject;
if (Input.GetKeyDown(KeyCode.Mouse0))
{
if (target.tag == "Block")
{
// ★追加
// 残機数が不足した場合には、セット不可&NGサウンドを鳴らす。
if(botCounts[num] < 1)
{
AudioSource.PlayClipAtPoint(NGSound, Camera.main.transform.position);
return;
}
GameObject bot = Instantiate(myBotsPrefab[num], target.transform.position, Quaternion.identity);
target.tag = "NG";
target.GetComponent<MeshRenderer>().material = NGMark;
// ★追加
// セットしたMyBotの残機数を1減らす
botCounts[num] -= 1;
}
else
{
AudioSource.PlayClipAtPoint(NGSound, Camera.main.transform.position);
}
}
}
}
}
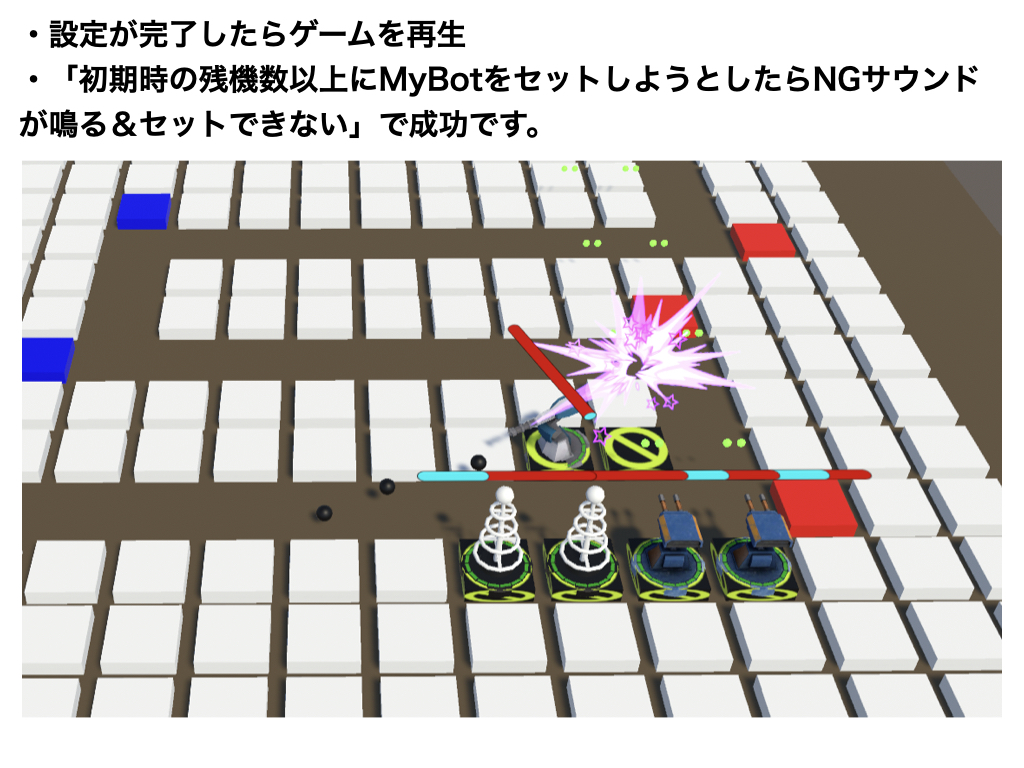
MyBotのセット⑤(設置できる数に制限を加える)