ゲームオーバーの作成
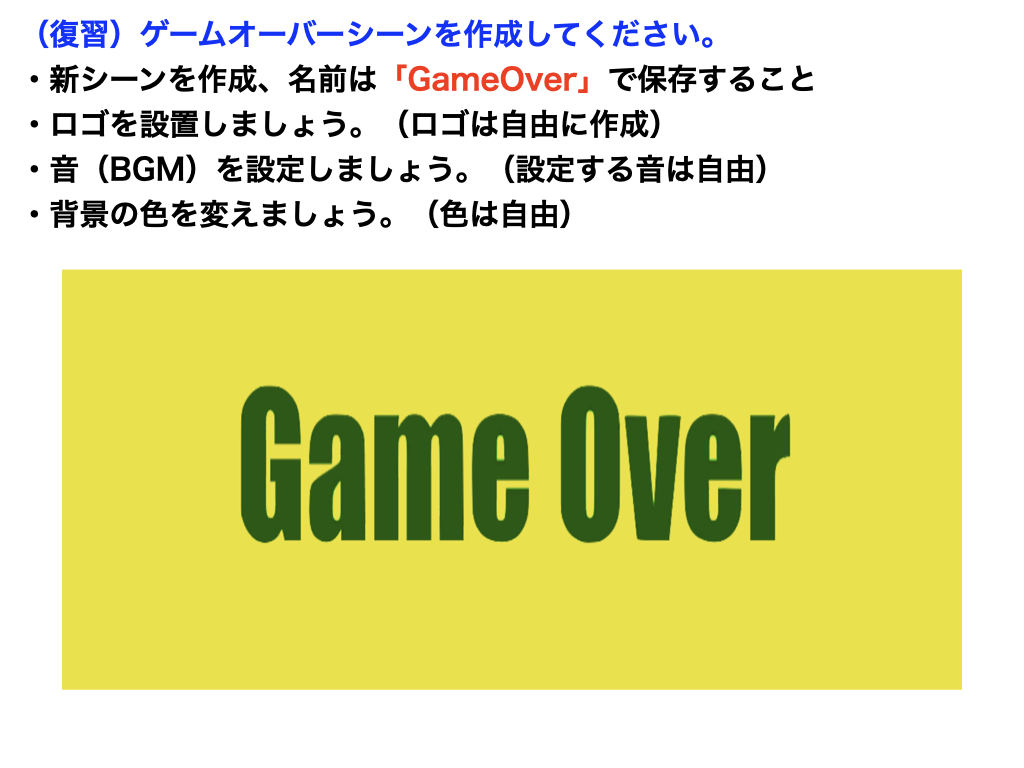
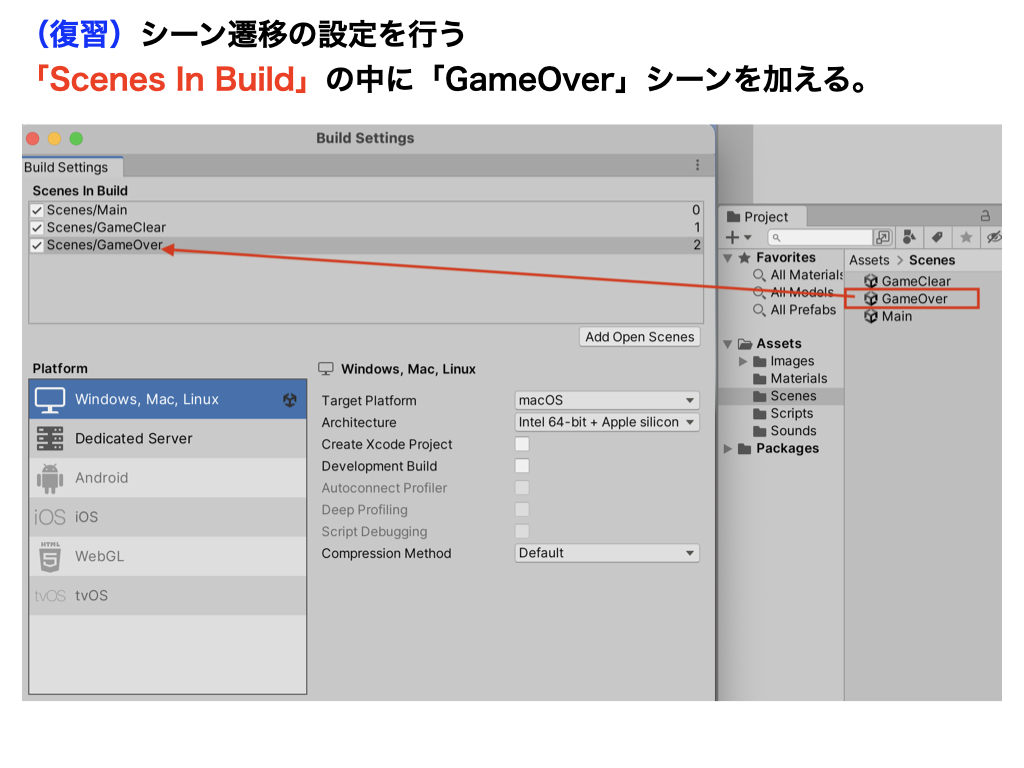
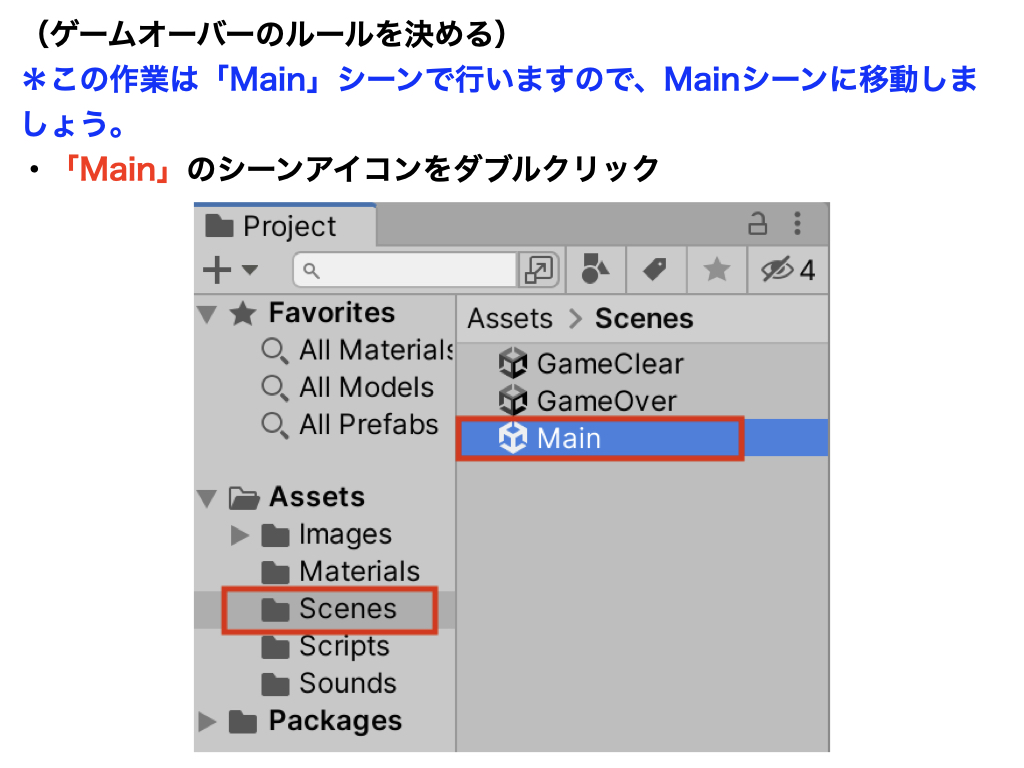
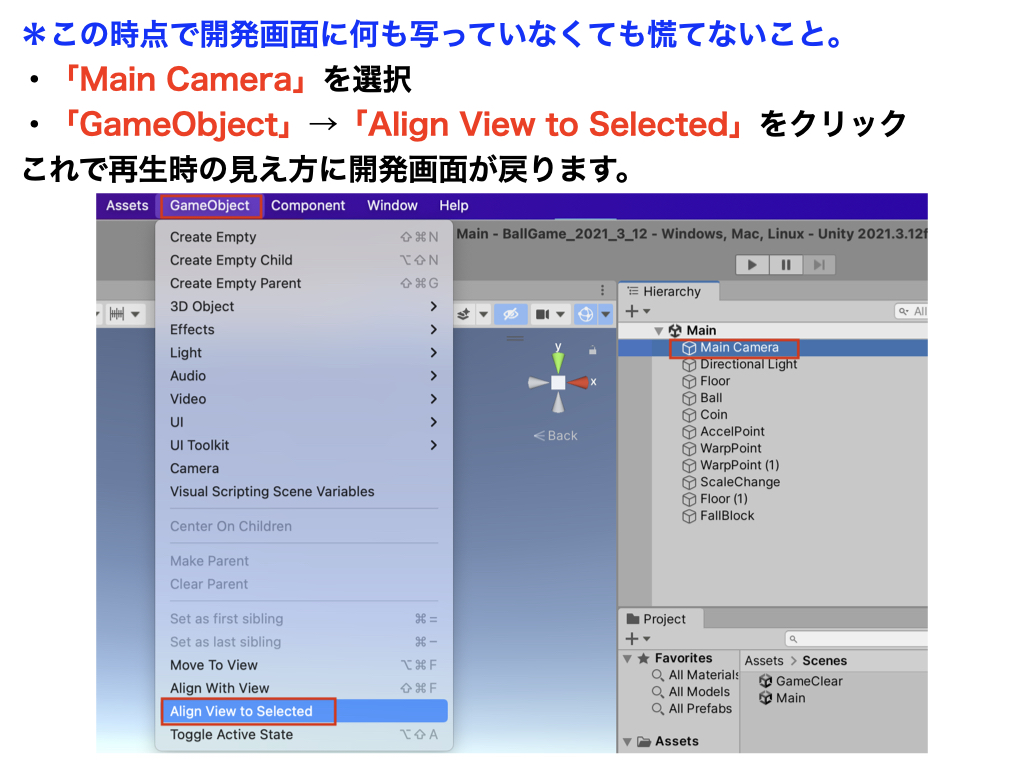
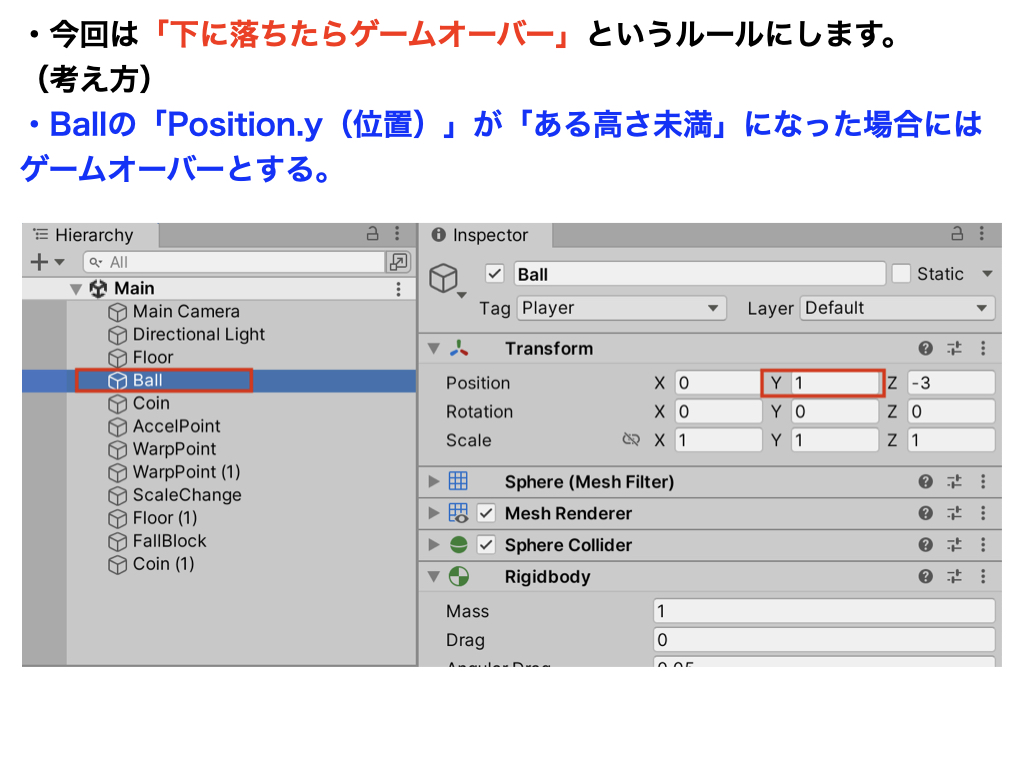
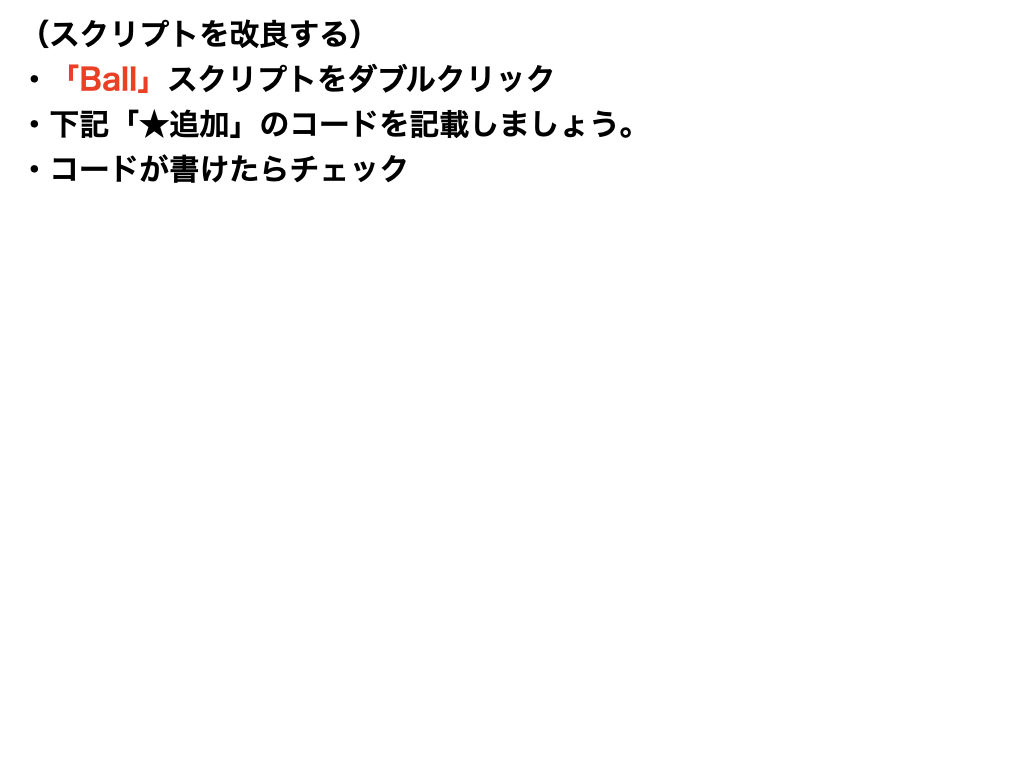
下に落ちたらゲームオーバー
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
private int coinCount = 0;
// ★追加
private Vector3 pos;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
// ★追加
// Ballの現在位置を取得する。
pos = transform.position;
if(pos.y < -10)
{
SceneManager.LoadScene("GameOver");
}
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
coinCount += 1;
if (coinCount == 2)
{
SceneManager.LoadScene("GameClear");
}
}
}
private void OnCollisionEnter(Collision collision)
{
if (collision.gameObject.CompareTag("Floor"))
{
isJumping = false;
}
}
}
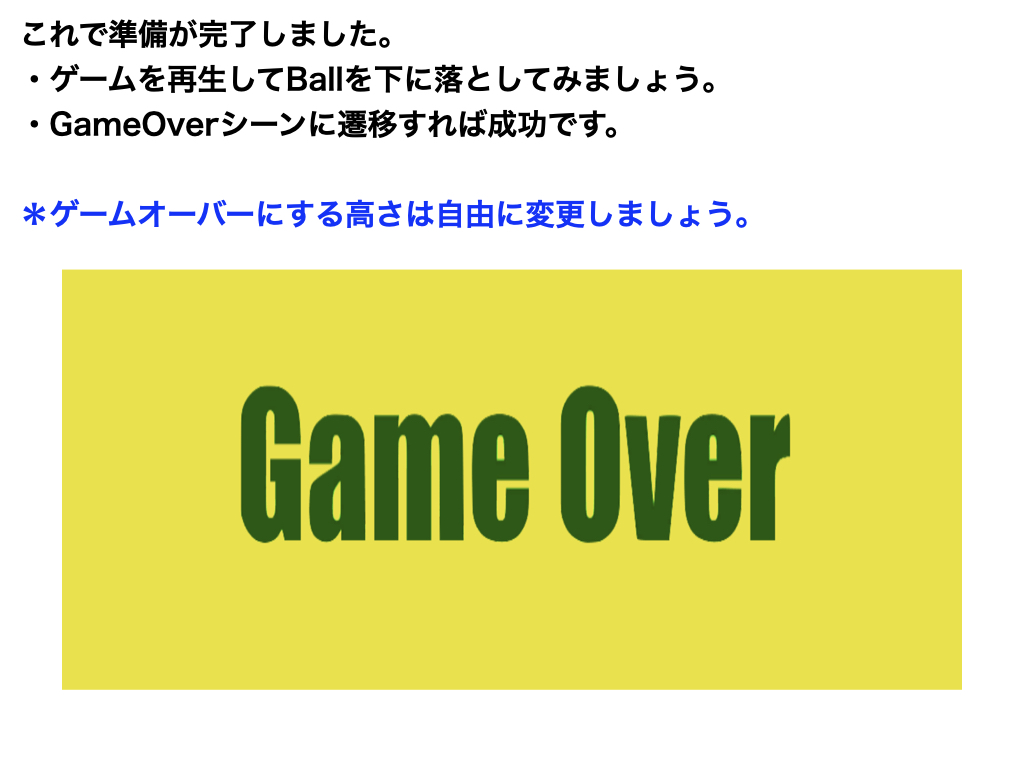
【2021版】BallGame(全31回)
他のコースを見る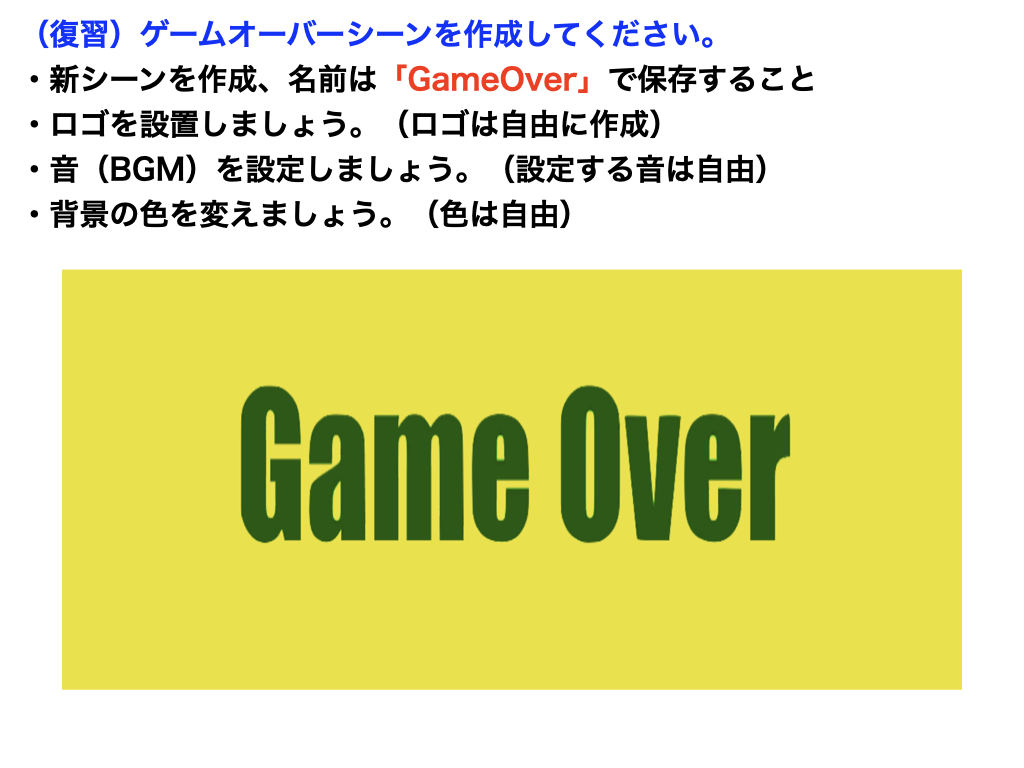
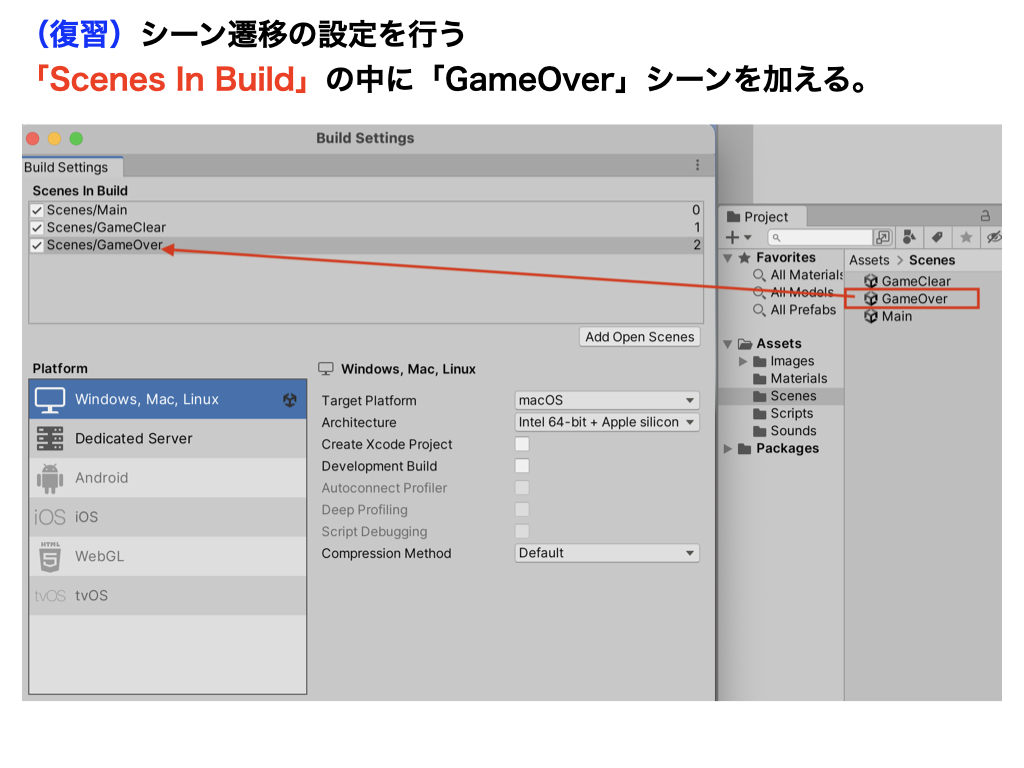
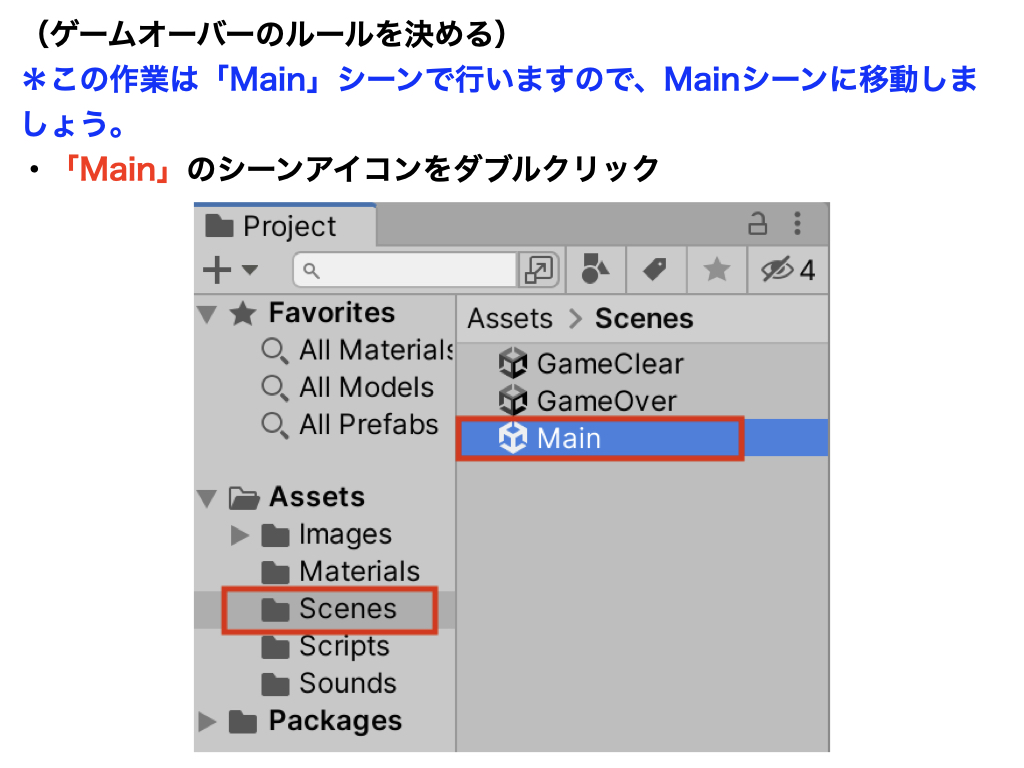
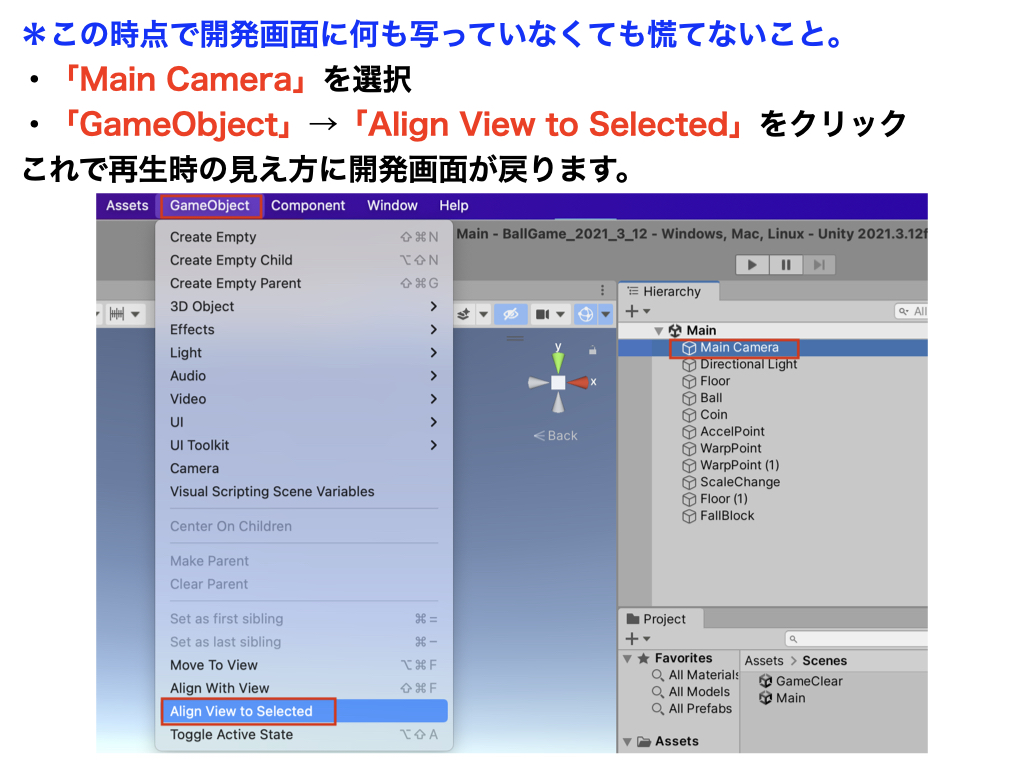
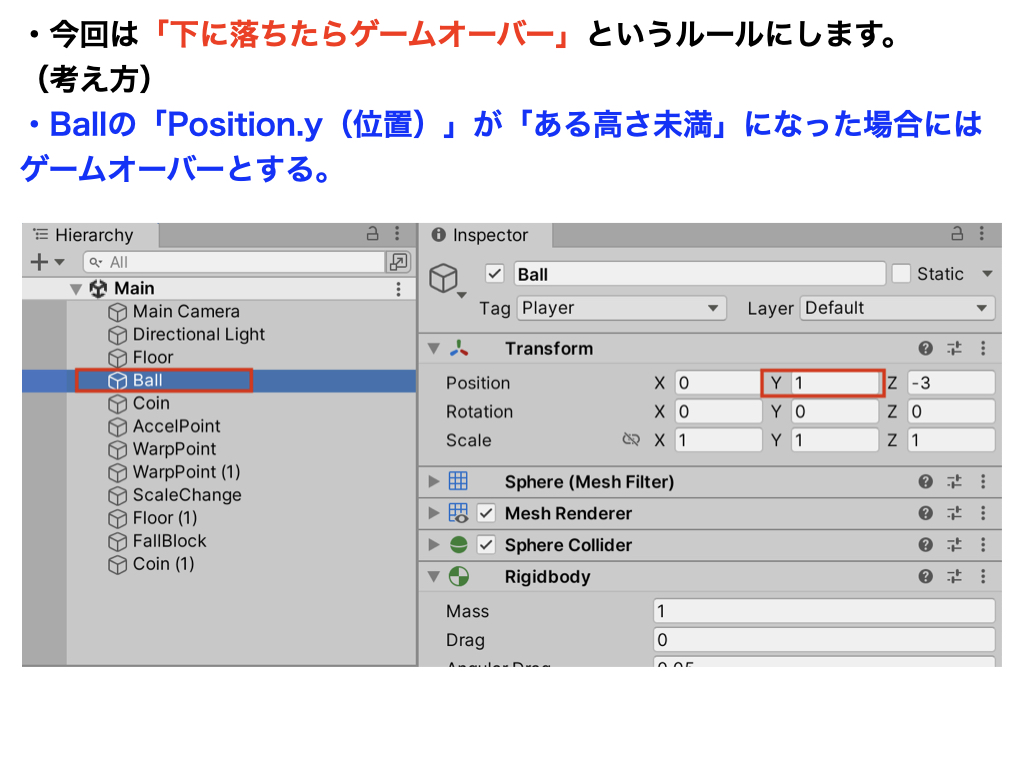
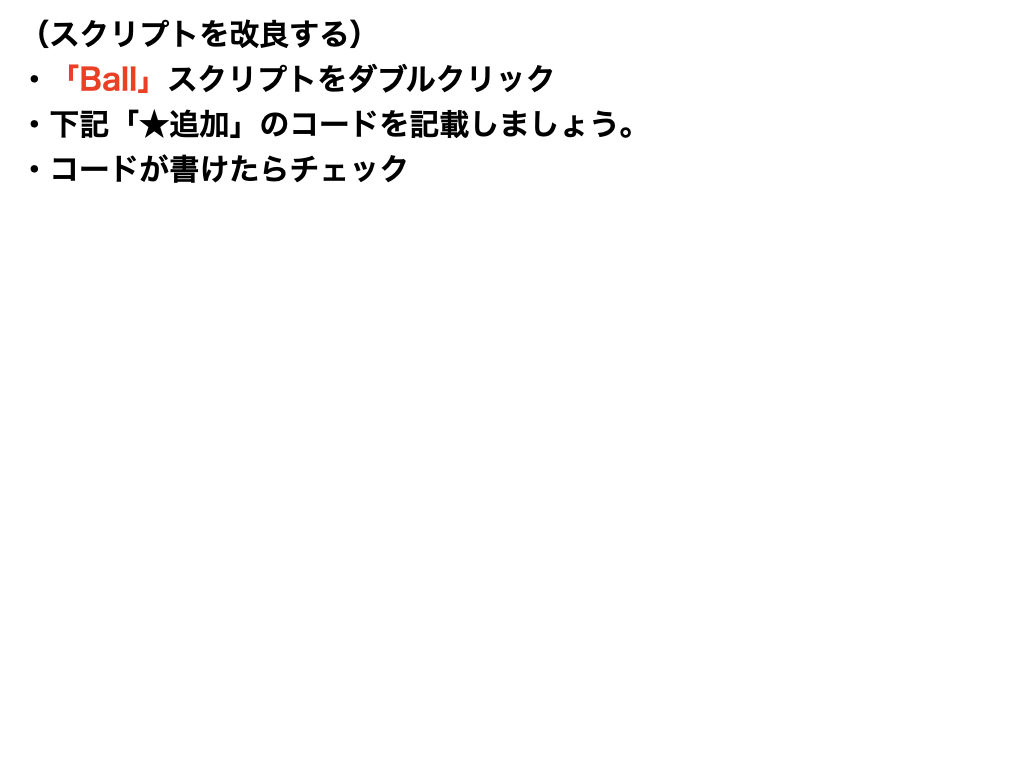
下に落ちたらゲームオーバー
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
private int coinCount = 0;
// ★追加
private Vector3 pos;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
// ★追加
// Ballの現在位置を取得する。
pos = transform.position;
if(pos.y < -10)
{
SceneManager.LoadScene("GameOver");
}
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
coinCount += 1;
if (coinCount == 2)
{
SceneManager.LoadScene("GameClear");
}
}
}
private void OnCollisionEnter(Collision collision)
{
if (collision.gameObject.CompareTag("Floor"))
{
isJumping = false;
}
}
}
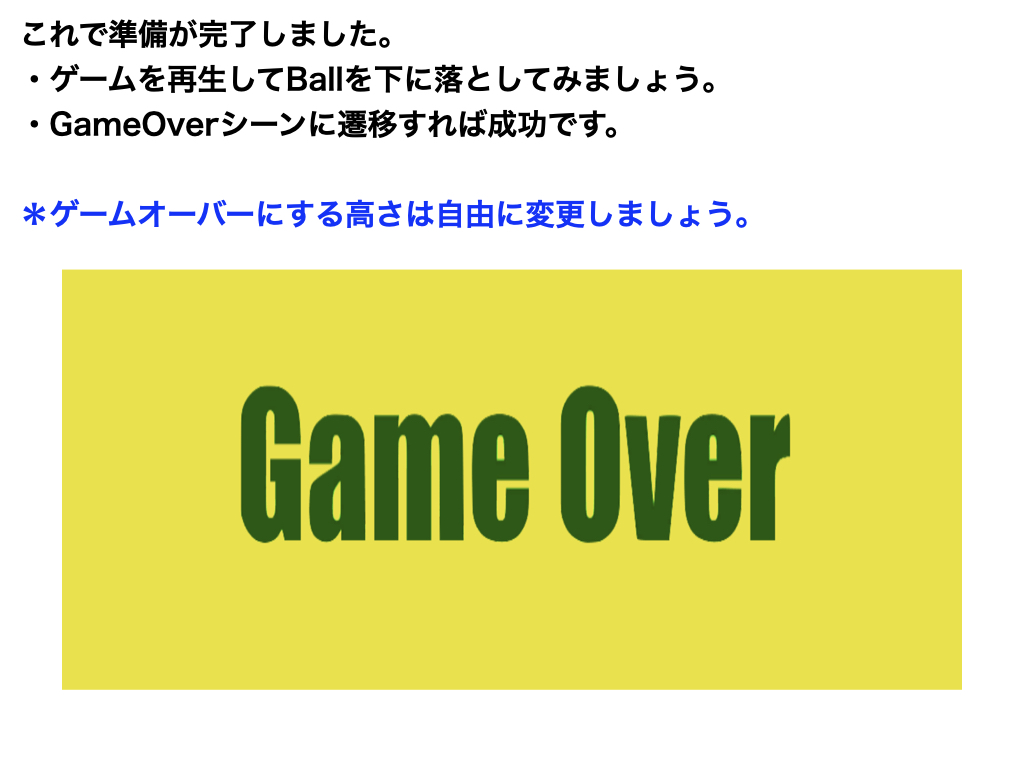
ゲームオーバーの作成