ゲームクリアーの作成
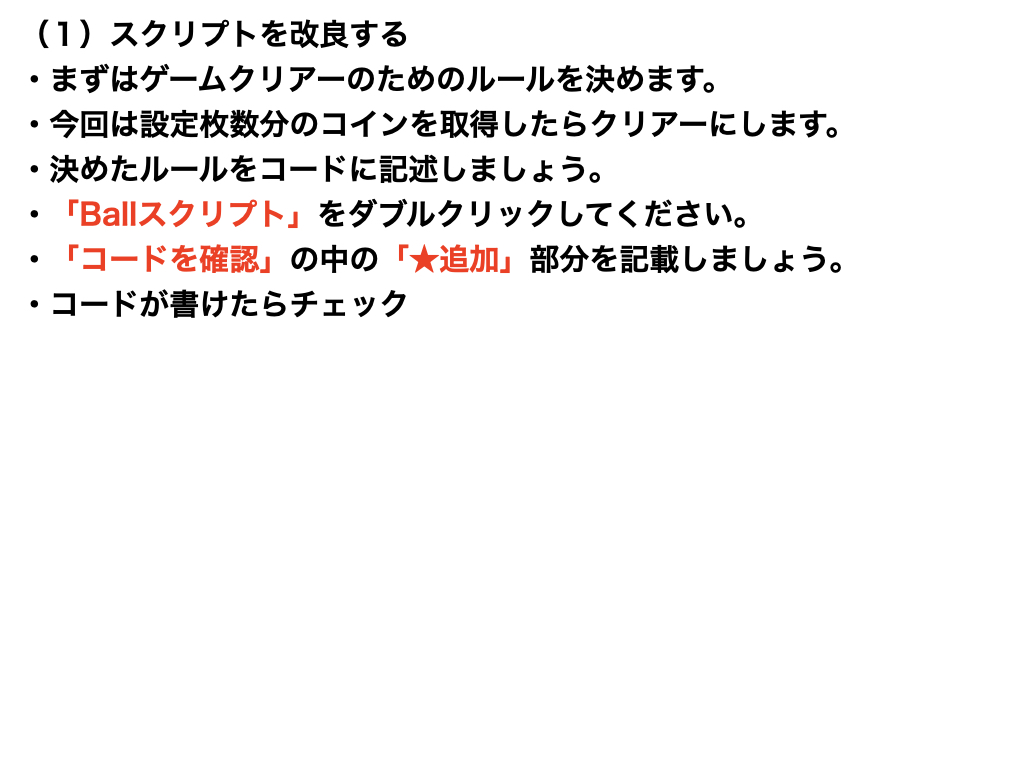
ゲームクリアー
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★追加
using UnityEngine.SceneManagement;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
// ★追加
private int coinCount = 0;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
// ★追加
// コインを1枚取得するごとに「coinCount」を1ずつ増加させる。
coinCount += 1;
// もしも「coinCount」が2になったら(条件)
if (coinCount == 2)
{
// GameClearシーンに遷移する。
// 遷移させるシーンは「名前」で特定するので「一言一句」合致させること(ポイント)
SceneManager.LoadScene("GameClear");
}
}
}
private void OnCollisionEnter(Collision collision)
{
if (collision.gameObject.CompareTag("Floor"))
{
isJumping = false;
}
}
}
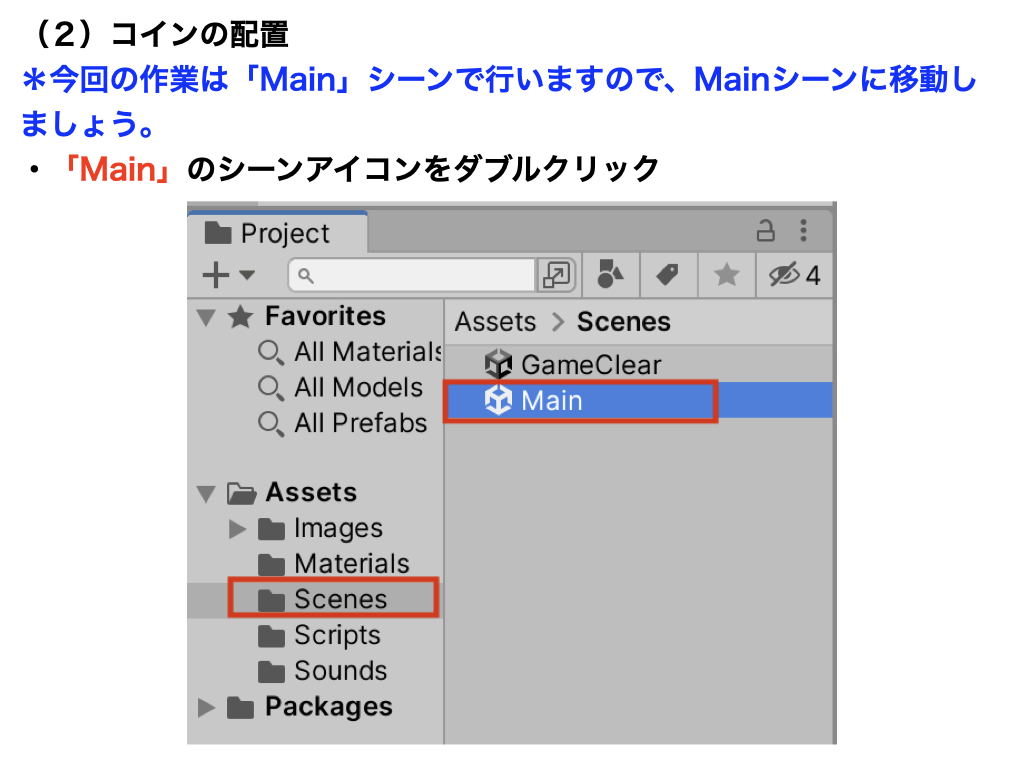
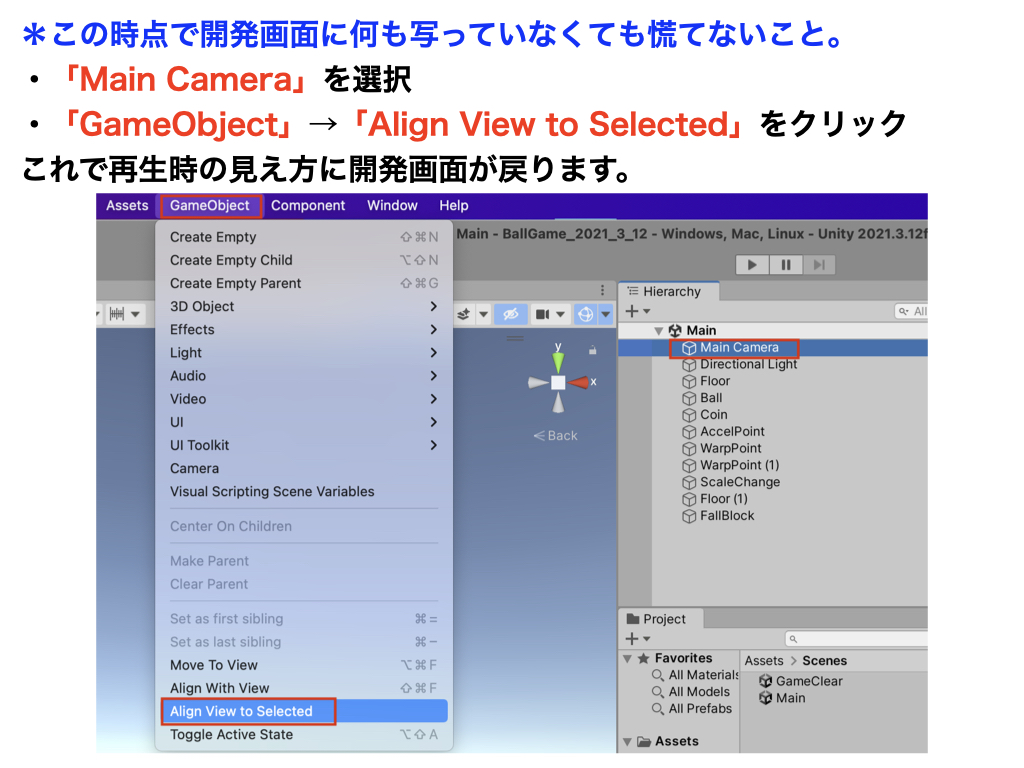
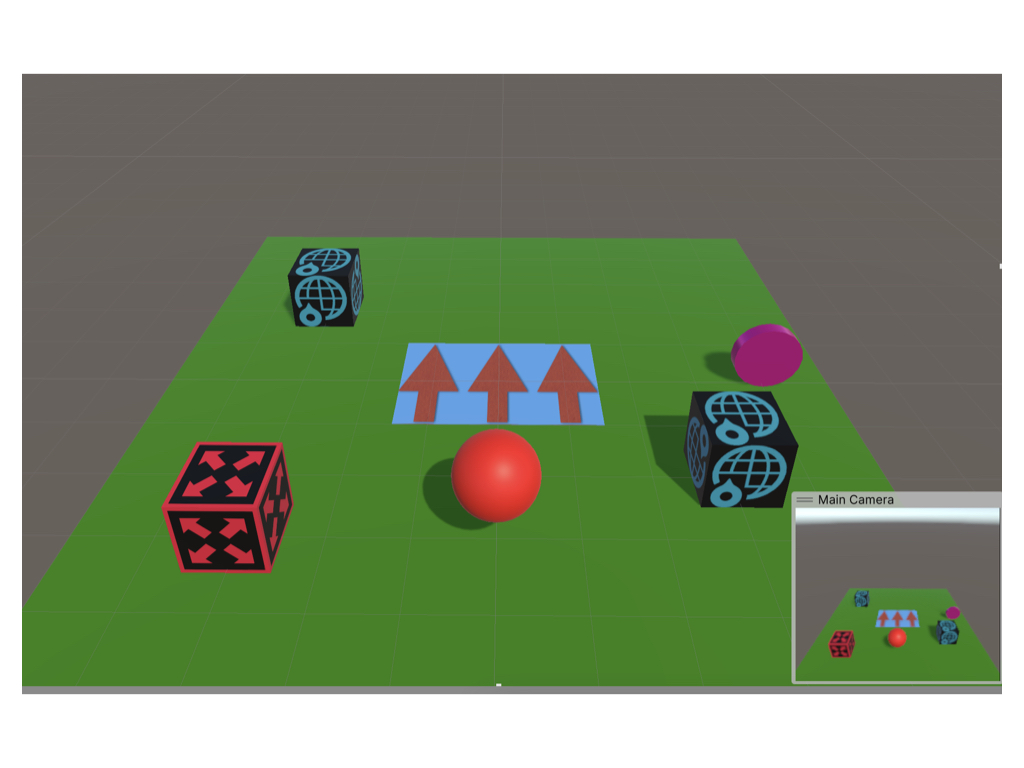
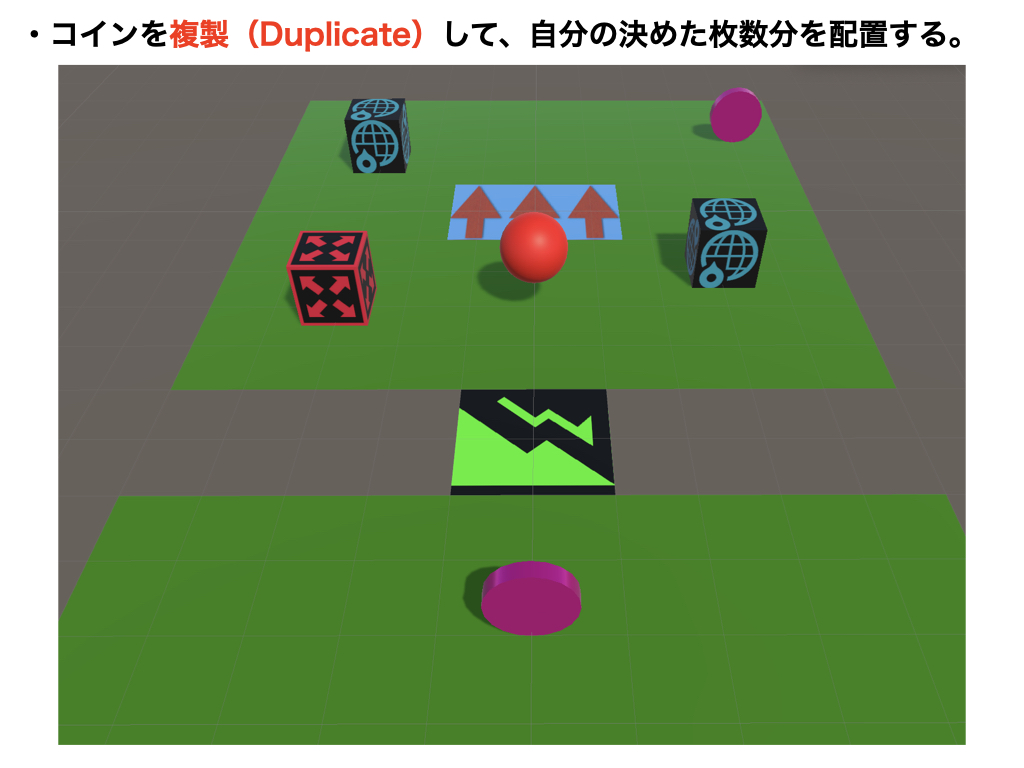
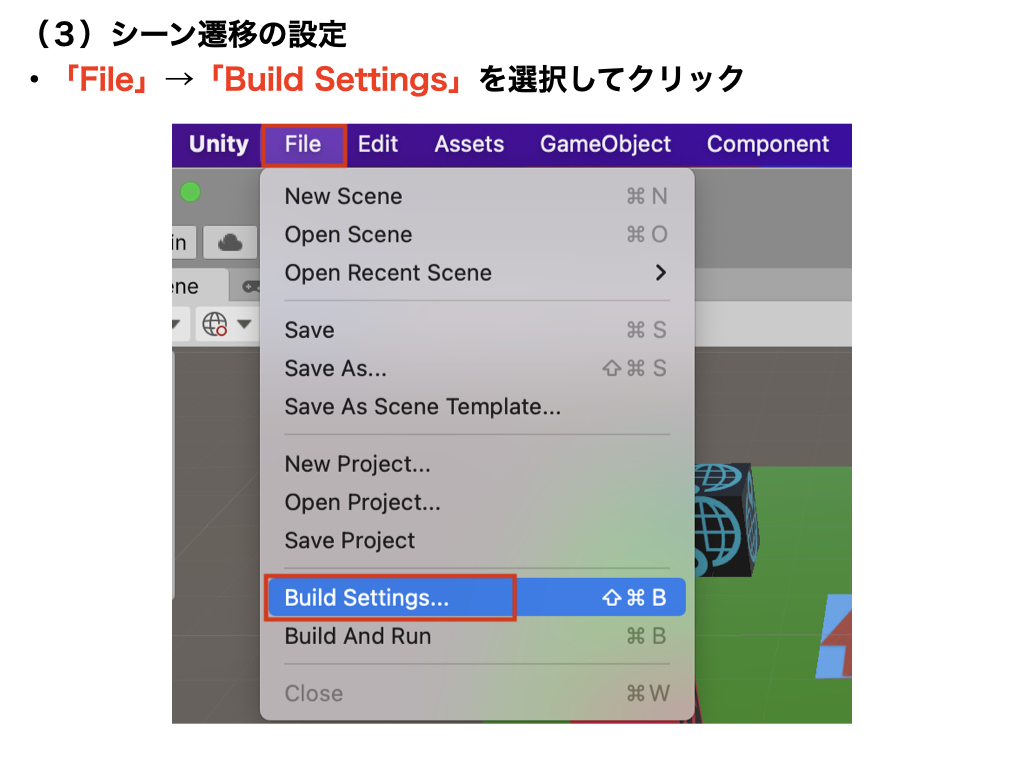
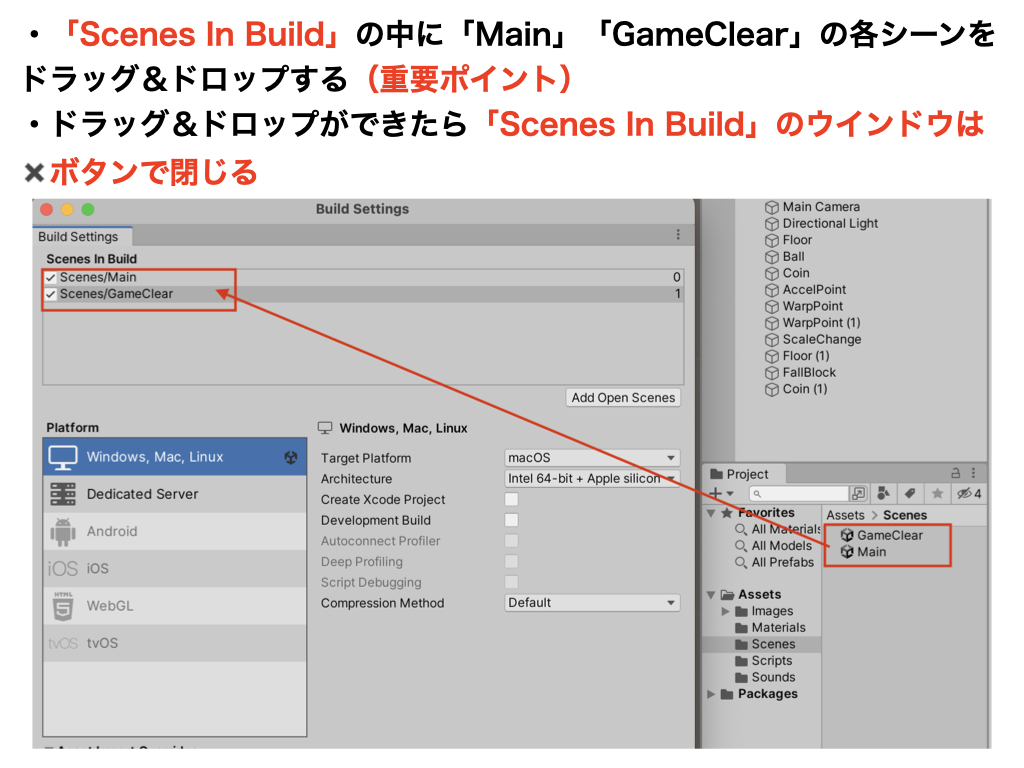
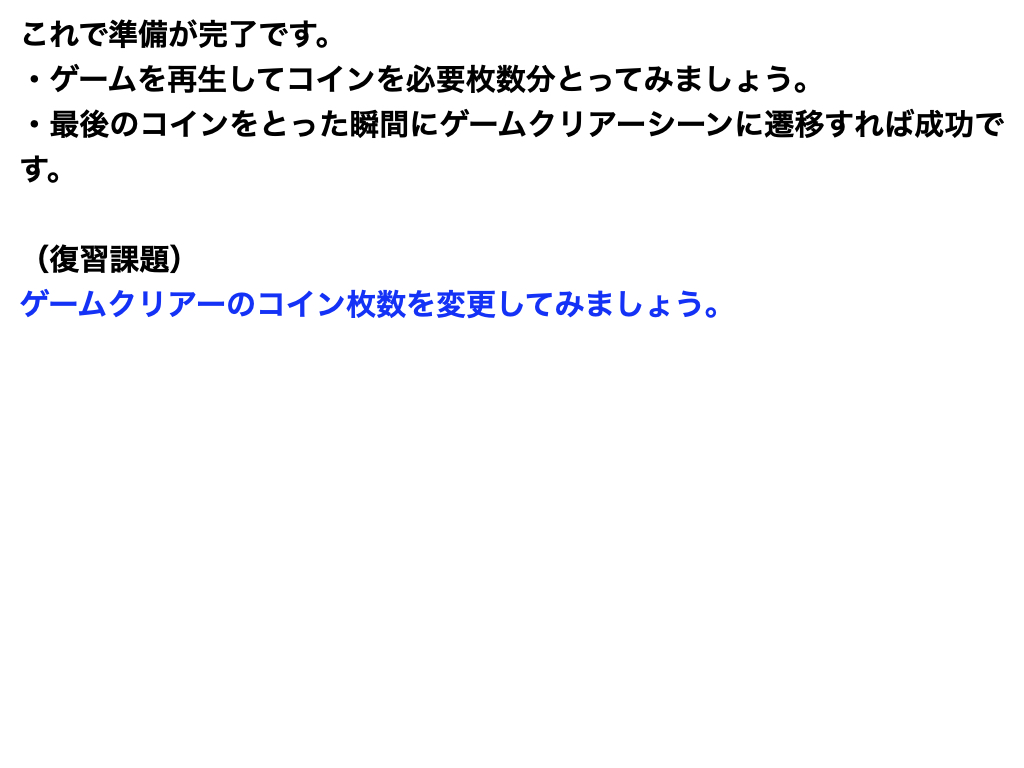
【2021版】BallGame(全31回)
他のコースを見る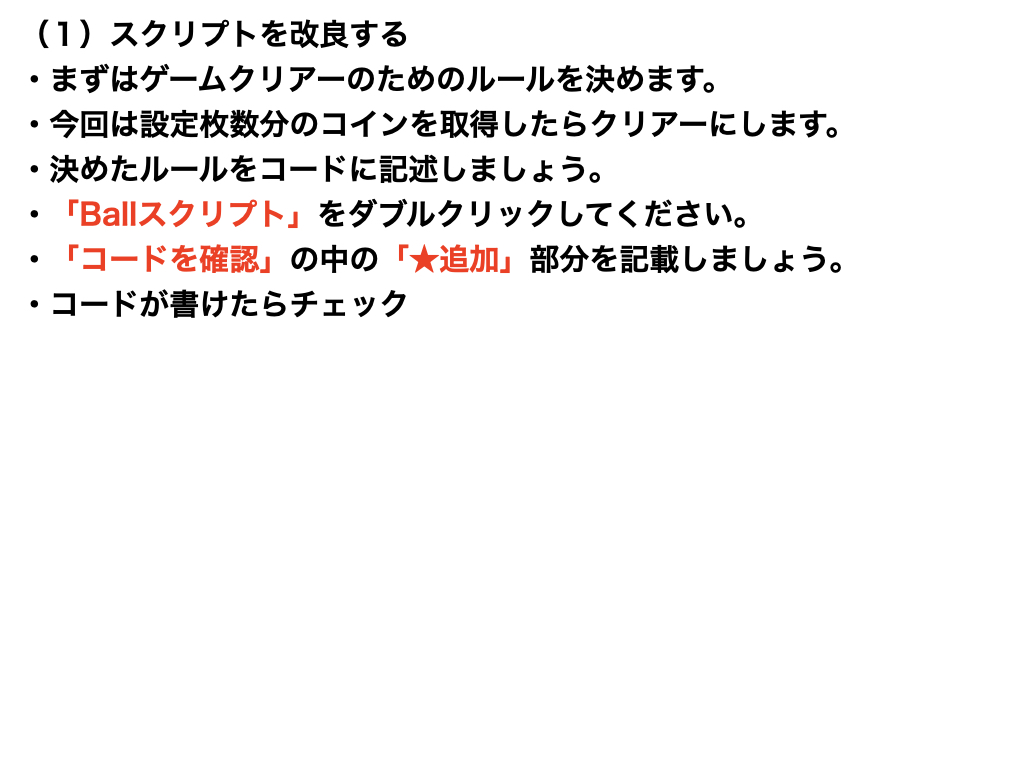
ゲームクリアー
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★追加
using UnityEngine.SceneManagement;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
// ★追加
private int coinCount = 0;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
// ★追加
// コインを1枚取得するごとに「coinCount」を1ずつ増加させる。
coinCount += 1;
// もしも「coinCount」が2になったら(条件)
if (coinCount == 2)
{
// GameClearシーンに遷移する。
// 遷移させるシーンは「名前」で特定するので「一言一句」合致させること(ポイント)
SceneManager.LoadScene("GameClear");
}
}
}
private void OnCollisionEnter(Collision collision)
{
if (collision.gameObject.CompareTag("Floor"))
{
isJumping = false;
}
}
}
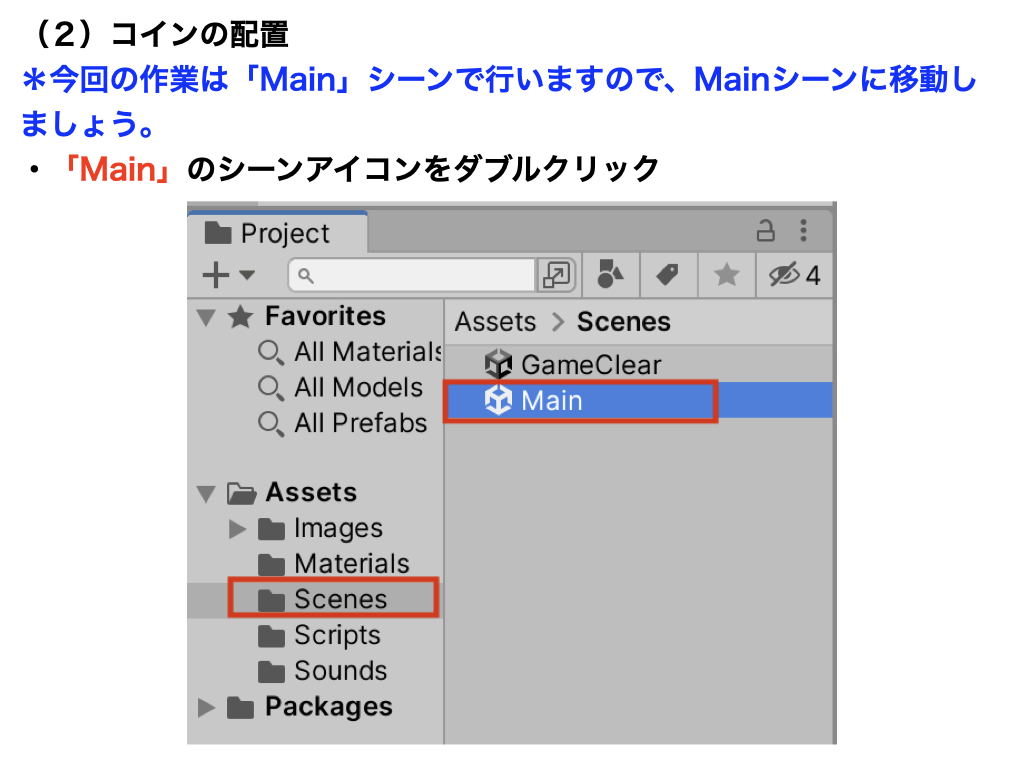
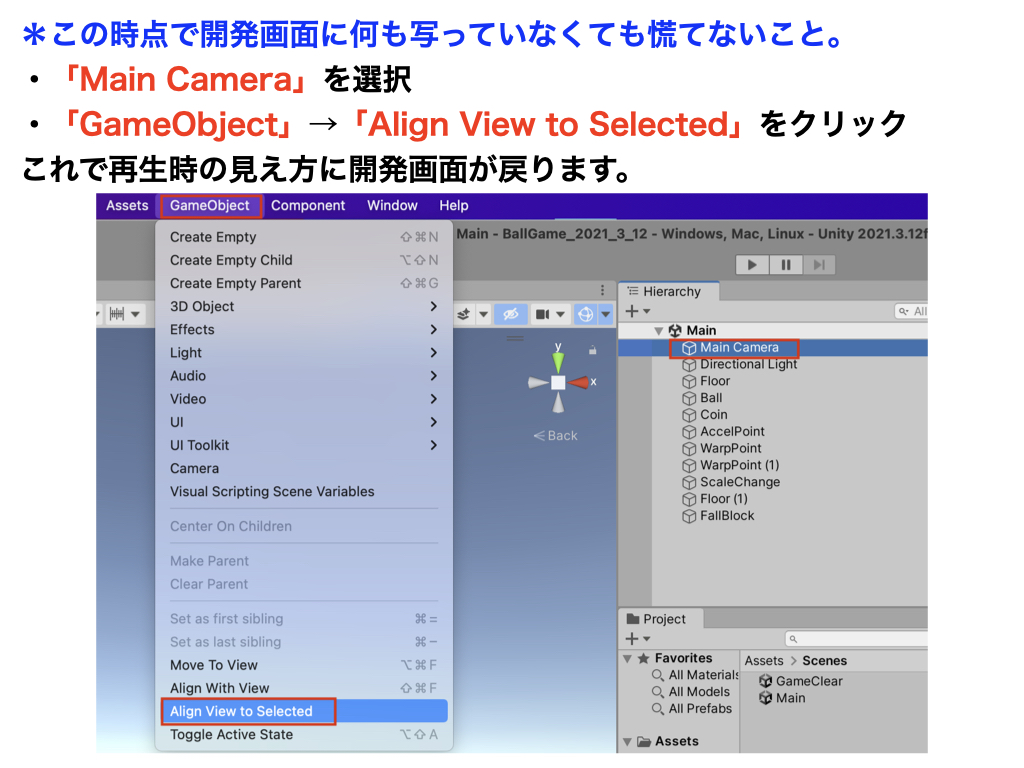
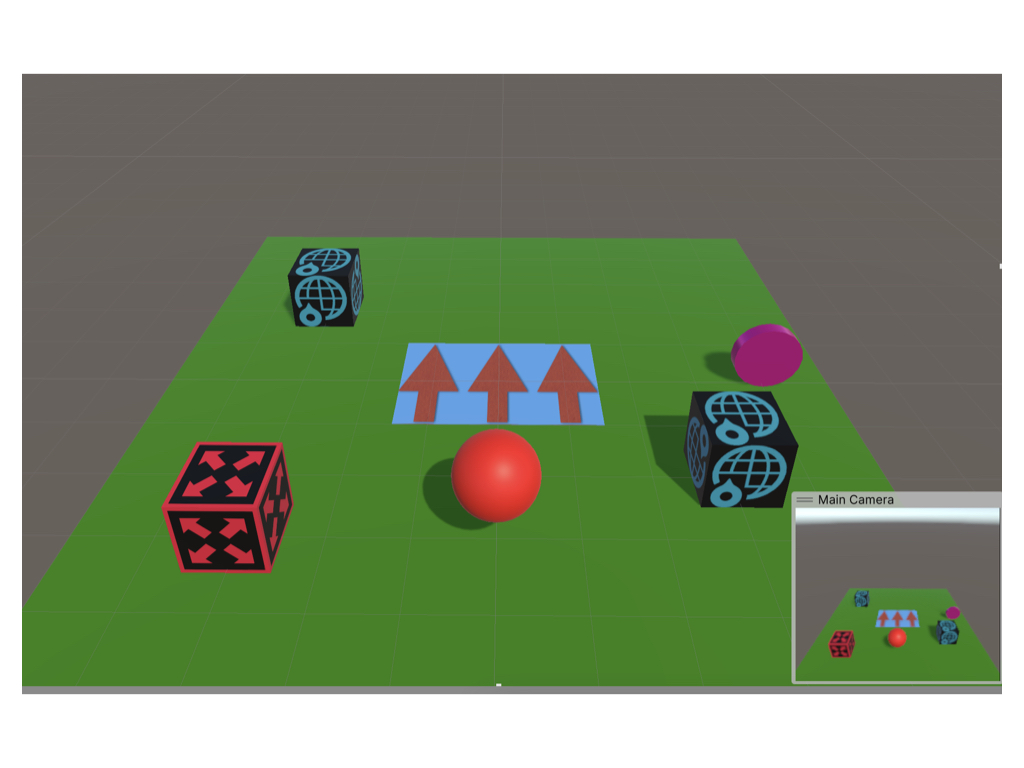
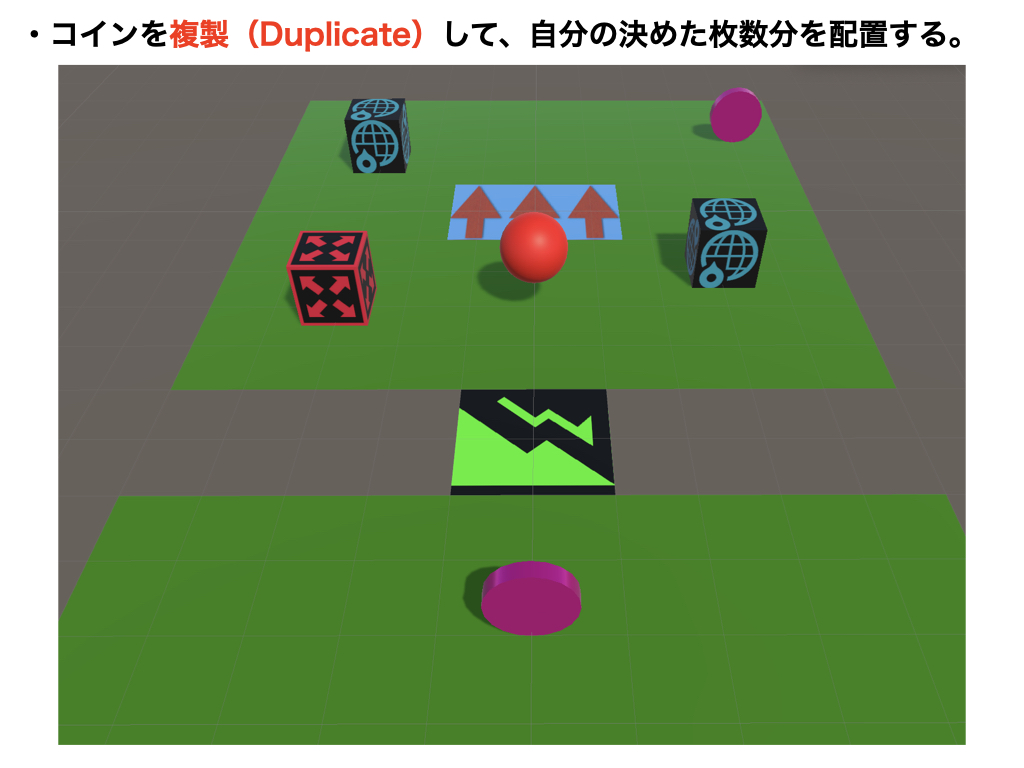
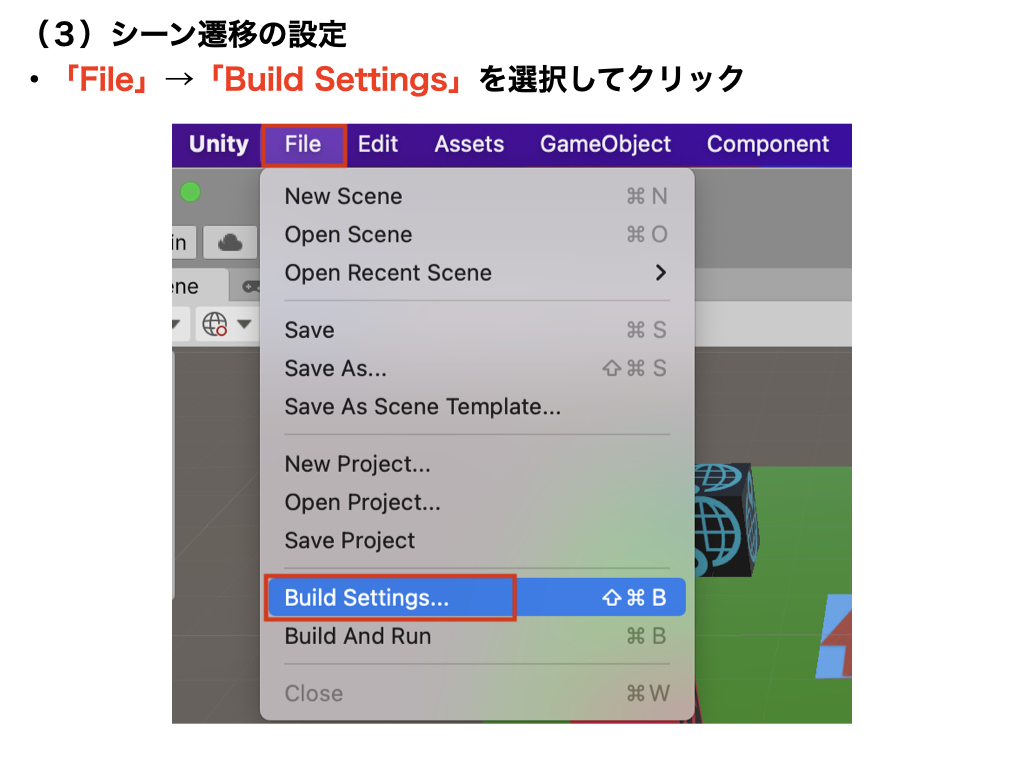
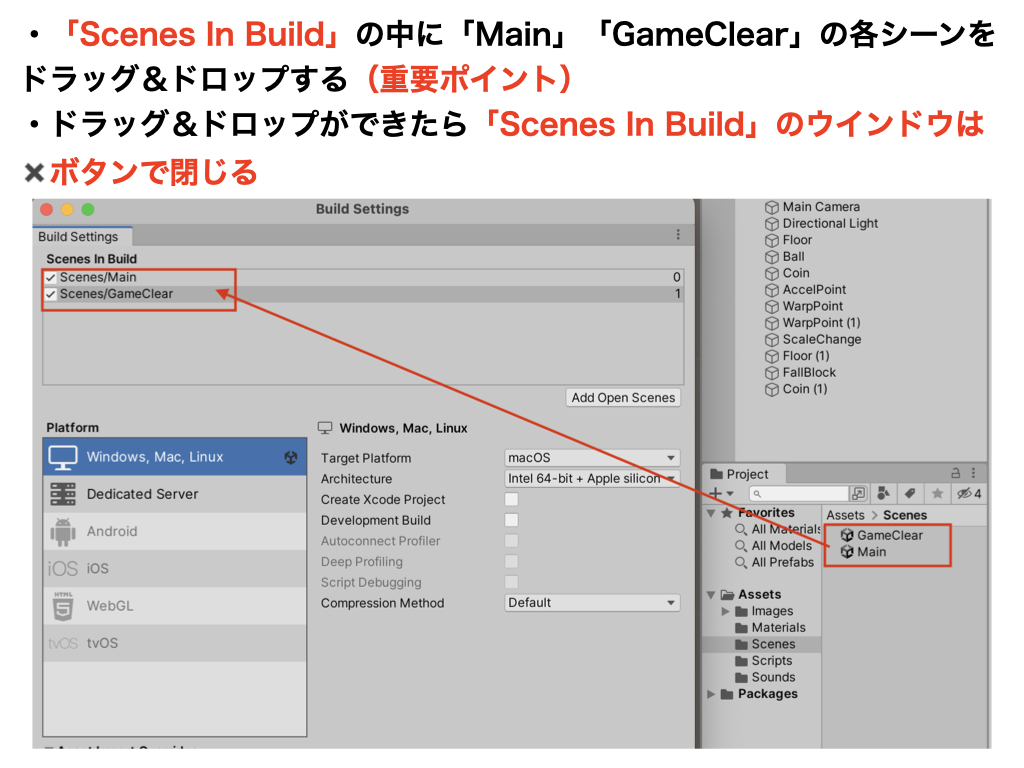
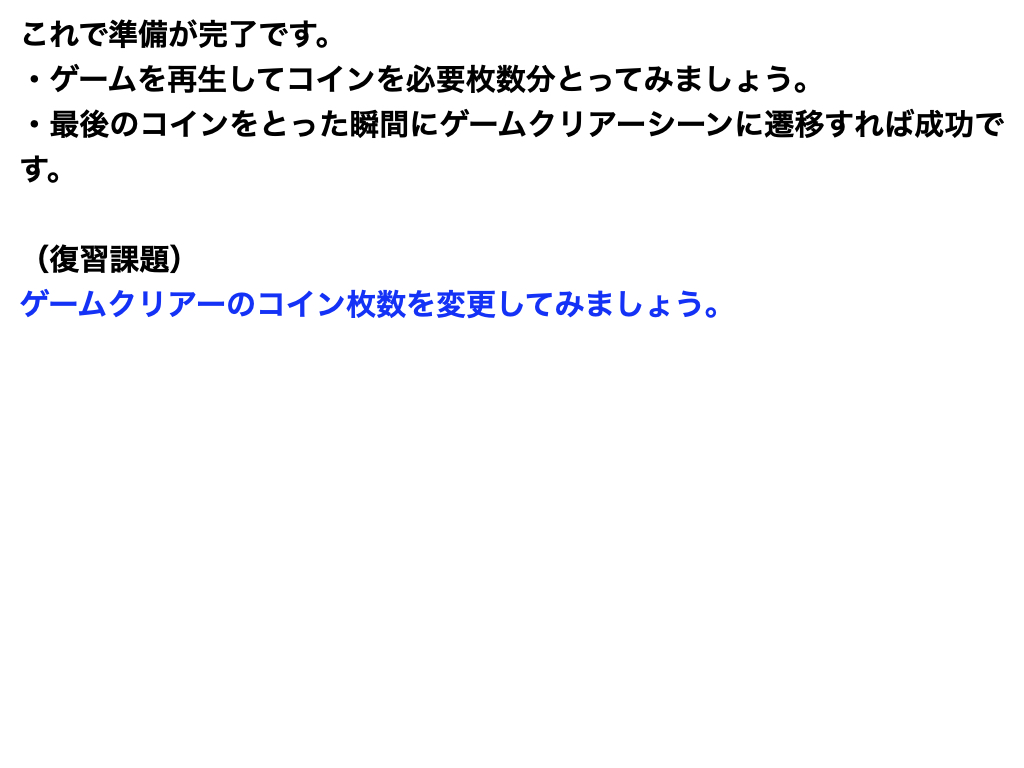
ゲームクリアーの作成