プレーヤーのステータス④(リトライの仕組みを作る)
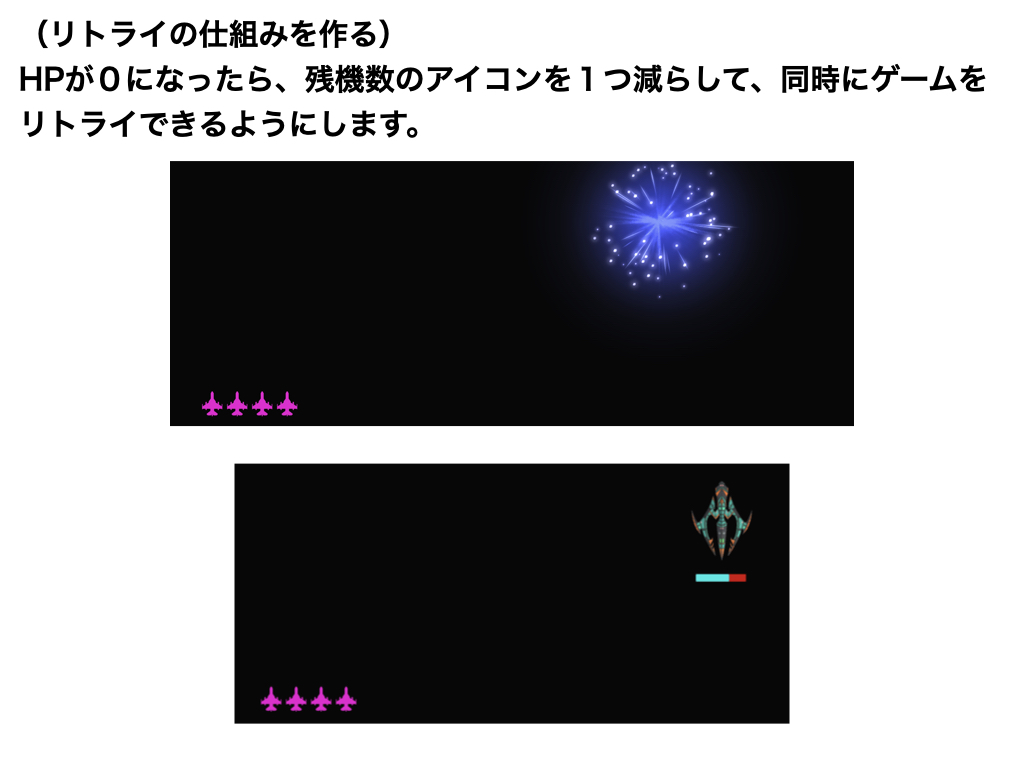
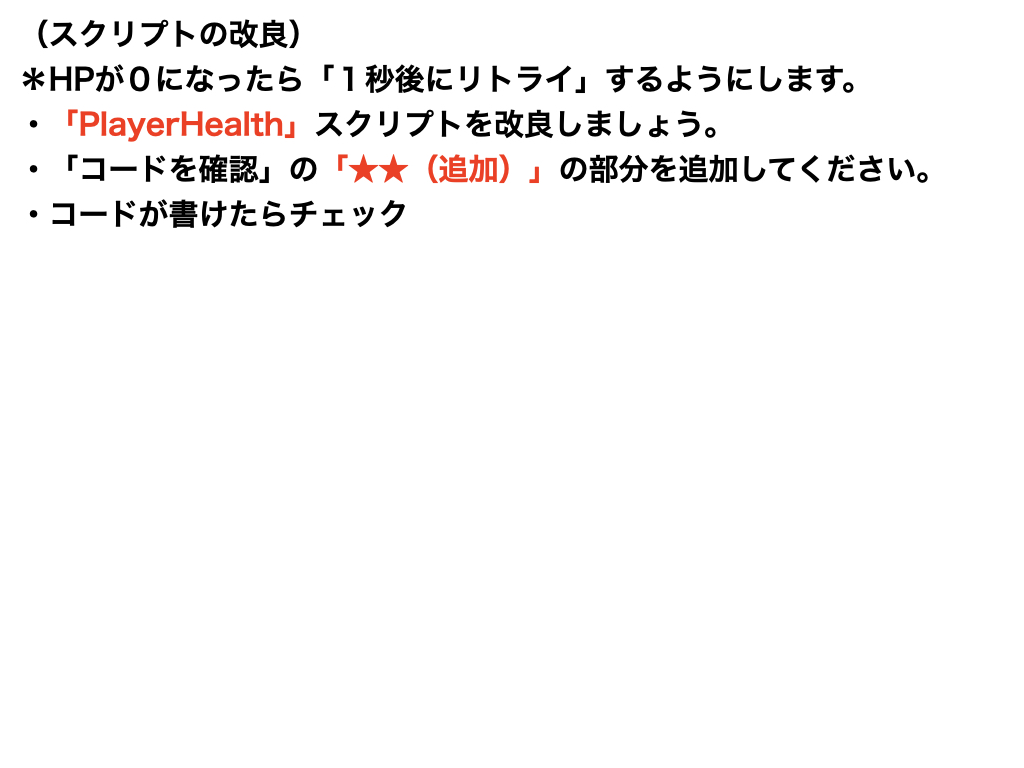
ゲームリトライ
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class PlayerHealth : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip damageSound;
public AudioClip destroySound;
public int playerHP;
public Slider hpSlider;
public GameObject[] playerIcons;
private int destroyCount = 0;
private void Start()
{
hpSlider.maxValue = playerHP;
hpSlider.value = playerHP;
}
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.CompareTag("EnemyMissile"))
{
playerHP -= 1;
AudioSource.PlayClipAtPoint(damageSound, Camera.main.transform.position);
Destroy(other.gameObject);
hpSlider.value = playerHP;
if (playerHP == 0)
{
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 1.0f);
AudioSource.PlayClipAtPoint(destroySound, Camera.main.transform.position);
this.gameObject.SetActive(false);
destroyCount += 1;
UpdatePlayerIcons();
// ★★(追加)
// リトライの命令ブロックを1秒後に呼び出す。
Invoke("Retry", 1.0f);
}
}
}
void UpdatePlayerIcons()
{
for (int i = 0; i < playerIcons.Length; i++)
{
if(destroyCount <= i)
{
playerIcons[i].SetActive(true);
}
else
{
playerIcons[i].SetActive(false);
}
}
}
// ★★(追加)
// ゲームリトライに関する命令ブロック
void Retry()
{
this.gameObject.SetActive(true);
playerHP = 3; // ここの数字は自分が決めたプレーヤーのHP数にすること。
hpSlider.value = playerHP;
}
}
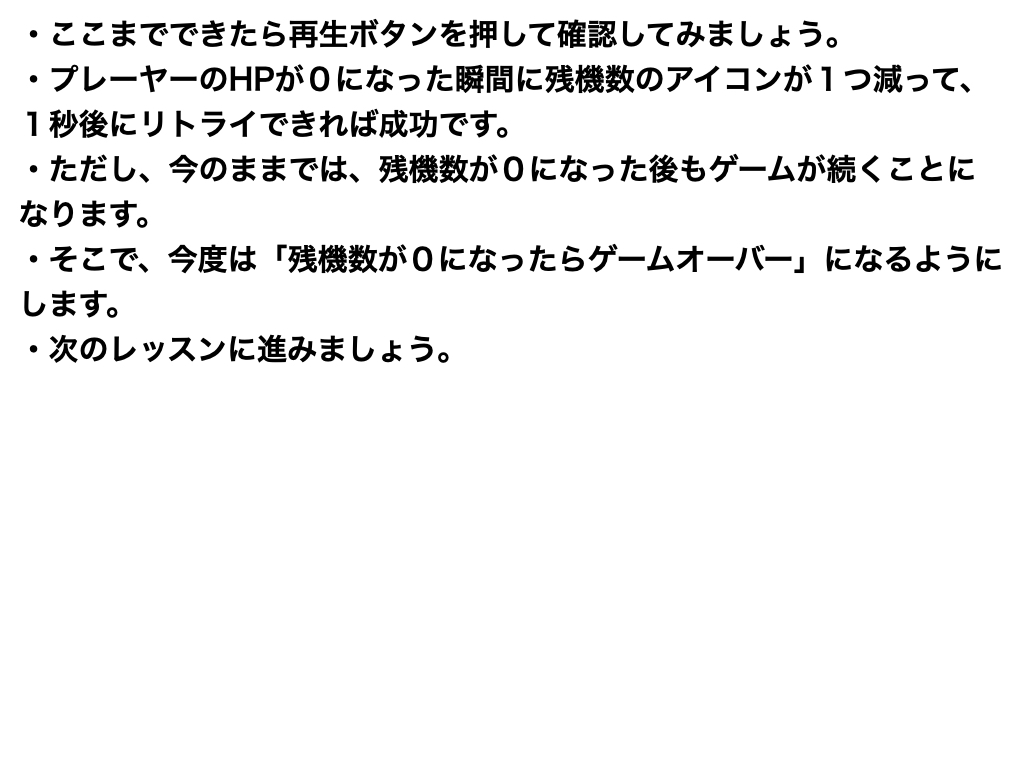
【2019版】Danmaku I(基礎1/全22回)
他のコースを見る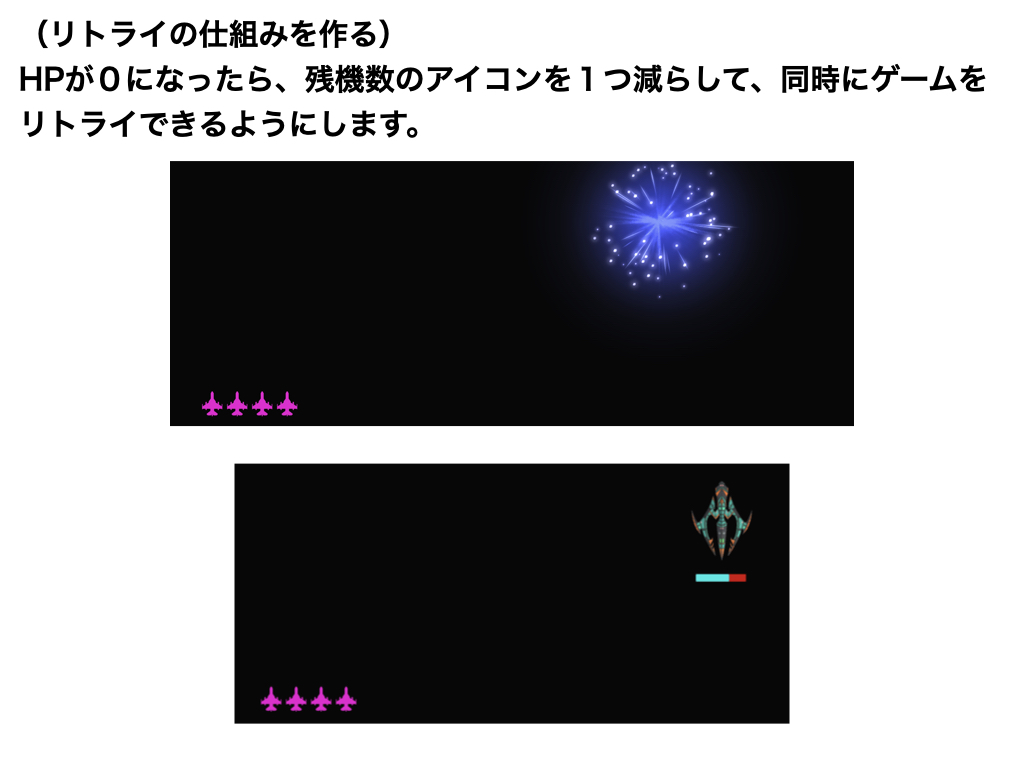
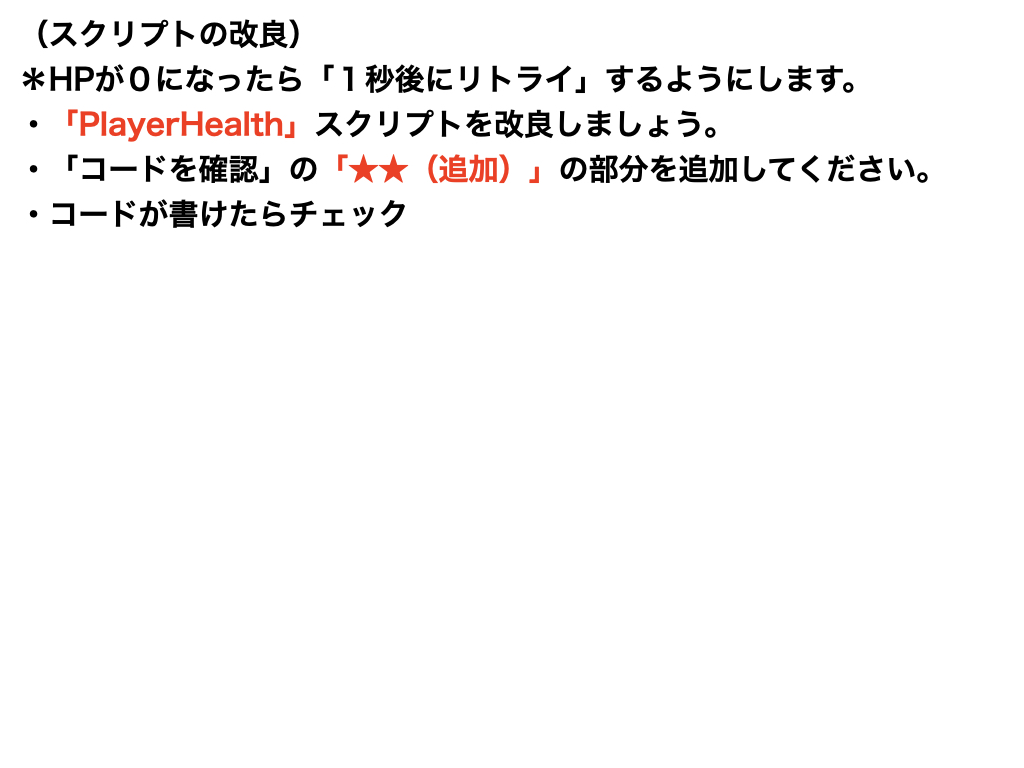
ゲームリトライ
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class PlayerHealth : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip damageSound;
public AudioClip destroySound;
public int playerHP;
public Slider hpSlider;
public GameObject[] playerIcons;
private int destroyCount = 0;
private void Start()
{
hpSlider.maxValue = playerHP;
hpSlider.value = playerHP;
}
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.CompareTag("EnemyMissile"))
{
playerHP -= 1;
AudioSource.PlayClipAtPoint(damageSound, Camera.main.transform.position);
Destroy(other.gameObject);
hpSlider.value = playerHP;
if (playerHP == 0)
{
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 1.0f);
AudioSource.PlayClipAtPoint(destroySound, Camera.main.transform.position);
this.gameObject.SetActive(false);
destroyCount += 1;
UpdatePlayerIcons();
// ★★(追加)
// リトライの命令ブロックを1秒後に呼び出す。
Invoke("Retry", 1.0f);
}
}
}
void UpdatePlayerIcons()
{
for (int i = 0; i < playerIcons.Length; i++)
{
if(destroyCount <= i)
{
playerIcons[i].SetActive(true);
}
else
{
playerIcons[i].SetActive(false);
}
}
}
// ★★(追加)
// ゲームリトライに関する命令ブロック
void Retry()
{
this.gameObject.SetActive(true);
playerHP = 3; // ここの数字は自分が決めたプレーヤーのHP数にすること。
hpSlider.value = playerHP;
}
}
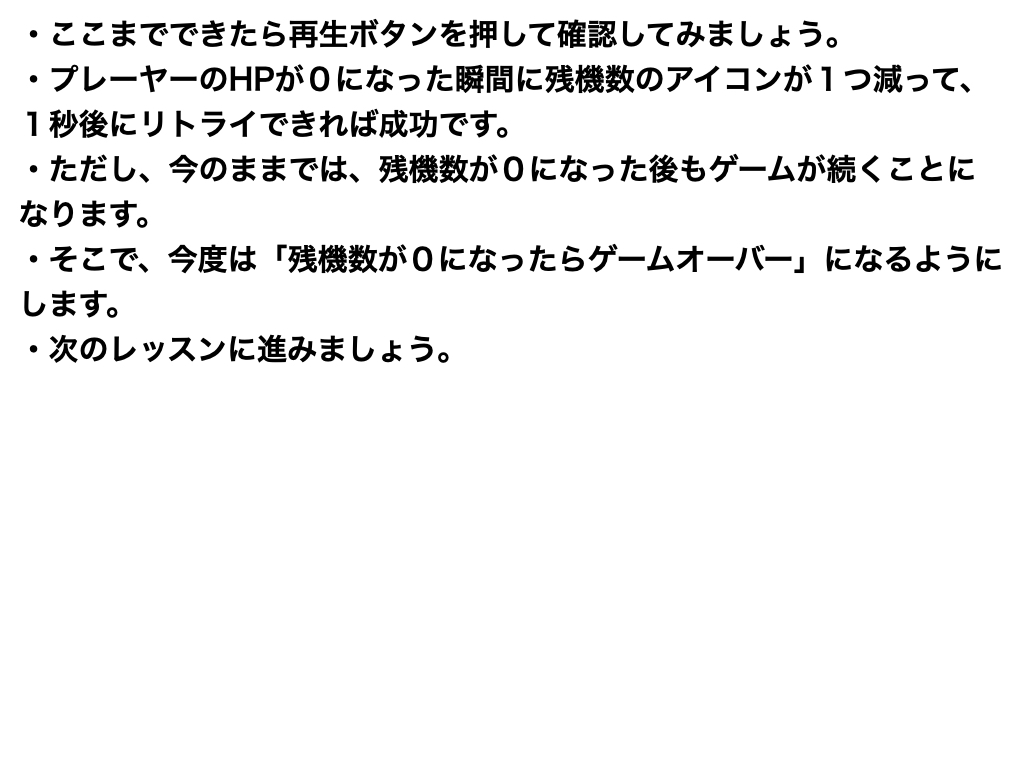
プレーヤーのステータス④(リトライの仕組みを作る)