ボールをジャンプさせよう
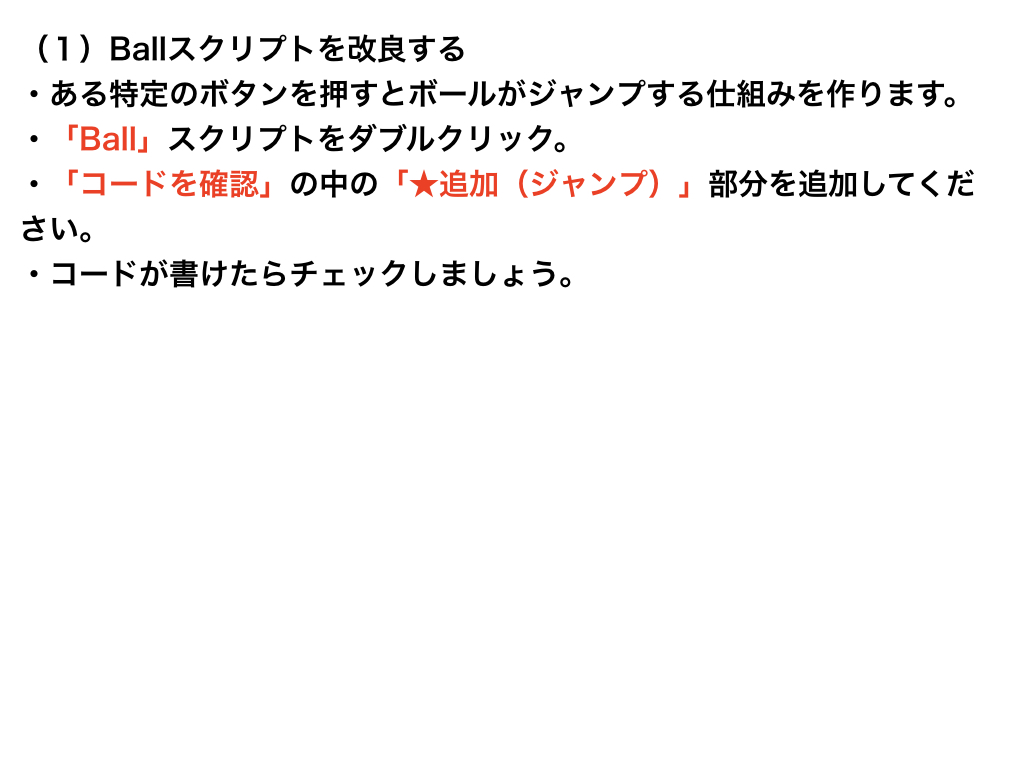
ジャンプ
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
// ★追加(ジャンプ)
public float jumpSpeed;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
// ★追加(ジャンプ)
if (Input.GetButtonDown("Jump"))
{
rb.velocity = Vector3.up * jumpSpeed;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
}
}
}
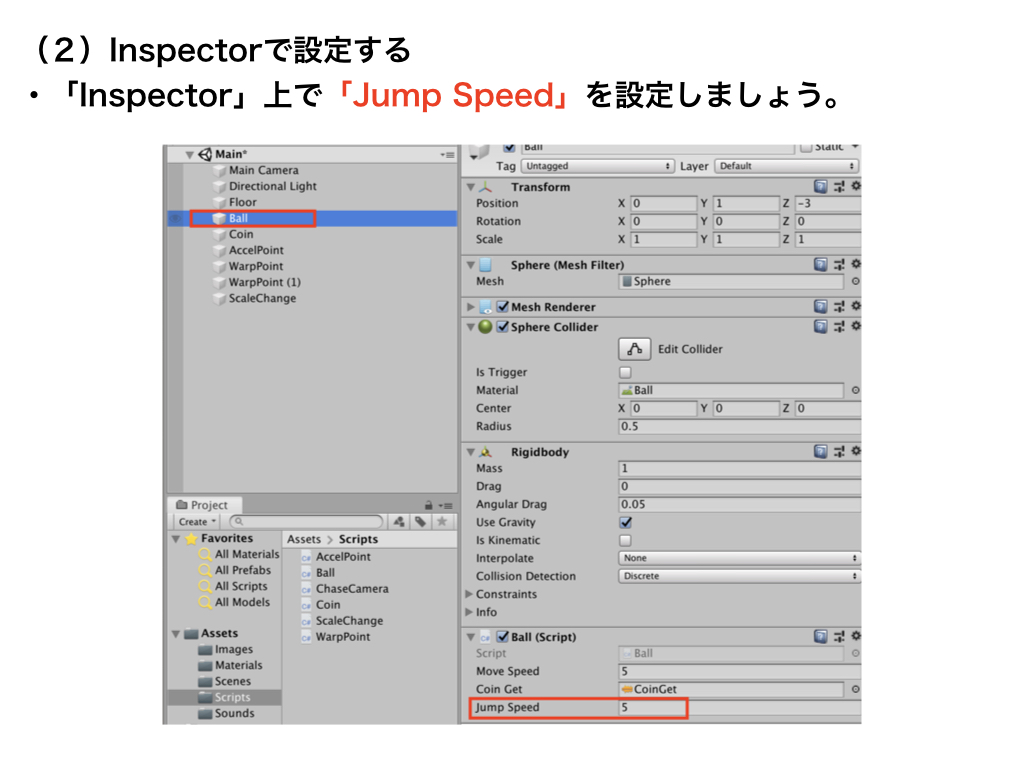
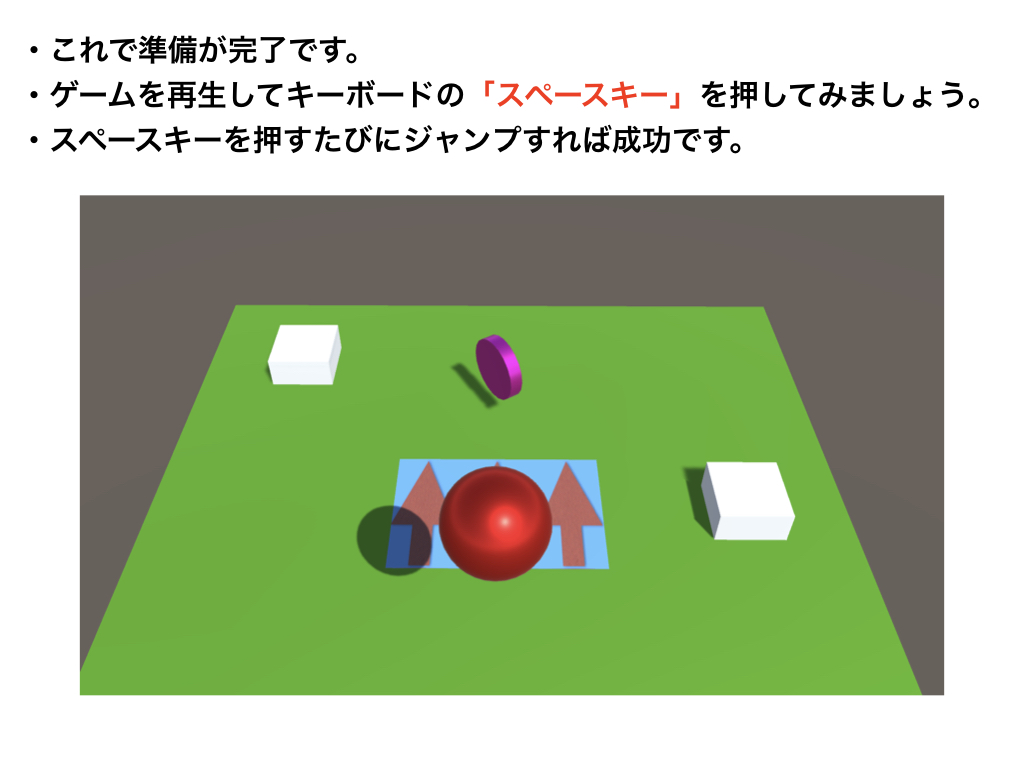
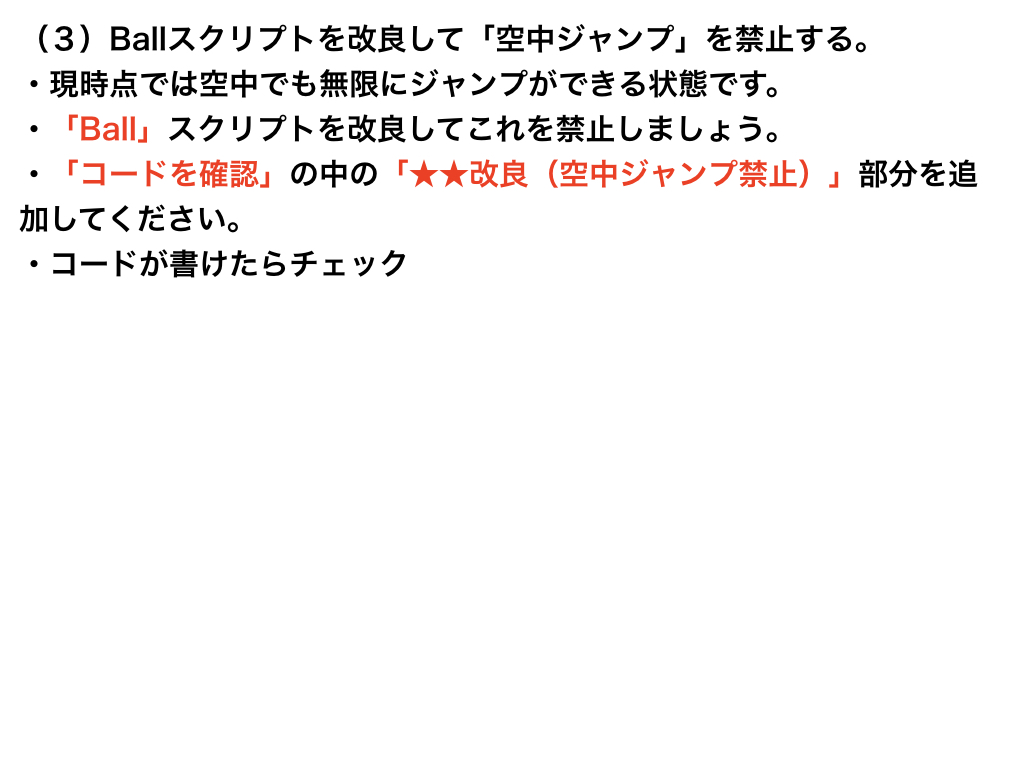
空中ジャンプの禁止
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
// ★★改良(空中ジャンプ禁止)
// boolは「真偽型」と呼ばれ「true」か「false」の2つの値を扱うことができます。
private bool isJumping = false;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
// ★★改良(空中ジャンプ禁止)
// 「&&」は「包含関係の『かつ』の意味」
// (例)「A && B」AかつB・・・>AとBの両方の条件が揃った時
// 「==」は「左右が等しい」という意味
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
// isJumpingという名前の箱の中身を「true」にする。
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
}
}
}
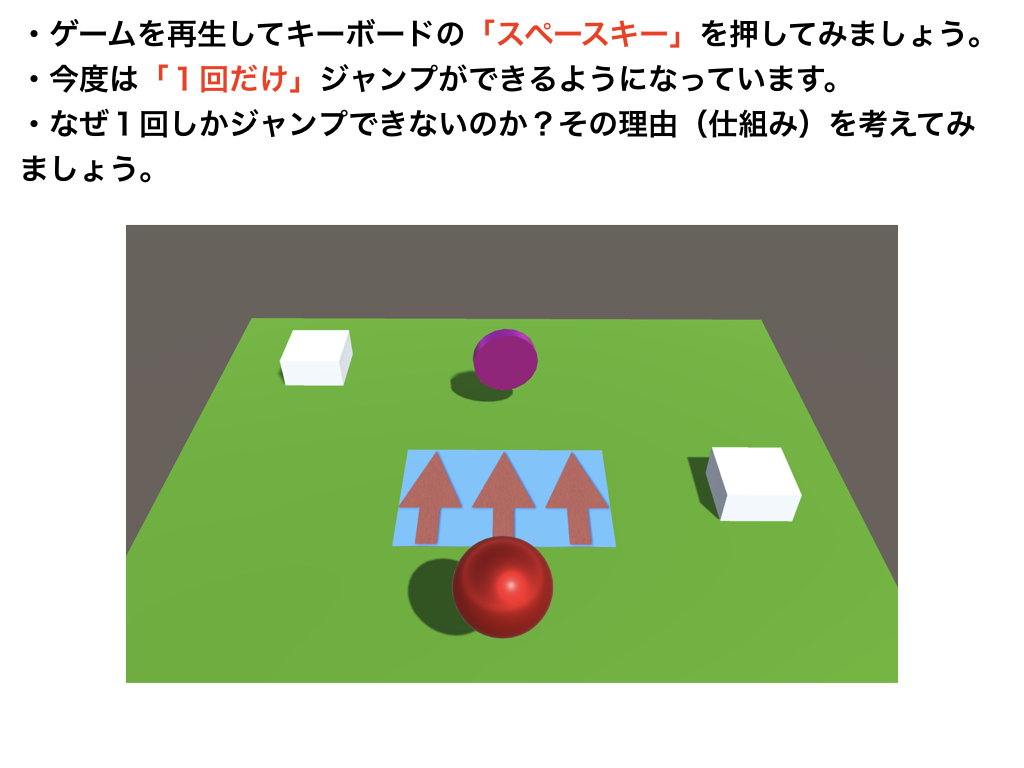
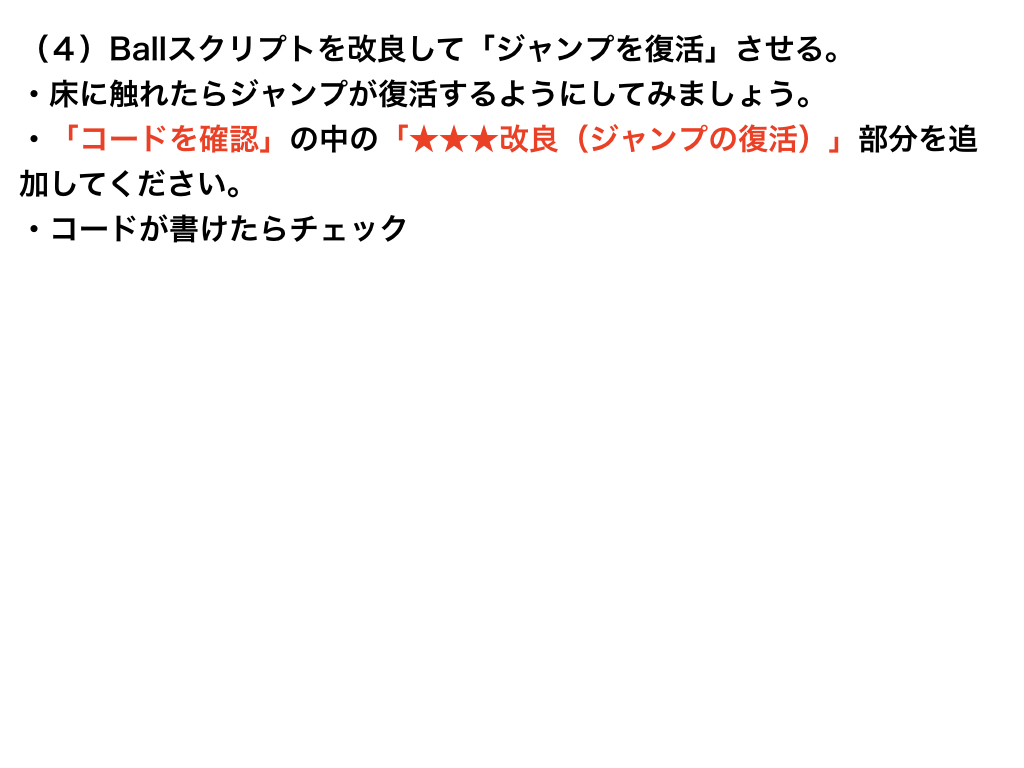
ジャンプの復活
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
}
}
// ★★★改良(ジャンプの復活)
// OnCollisionEnterのスペルに注意
private void OnCollisionEnter(Collision collision)
{
if(collision.gameObject.CompareTag("Floor"))
{
isJumping = false;
}
}
}
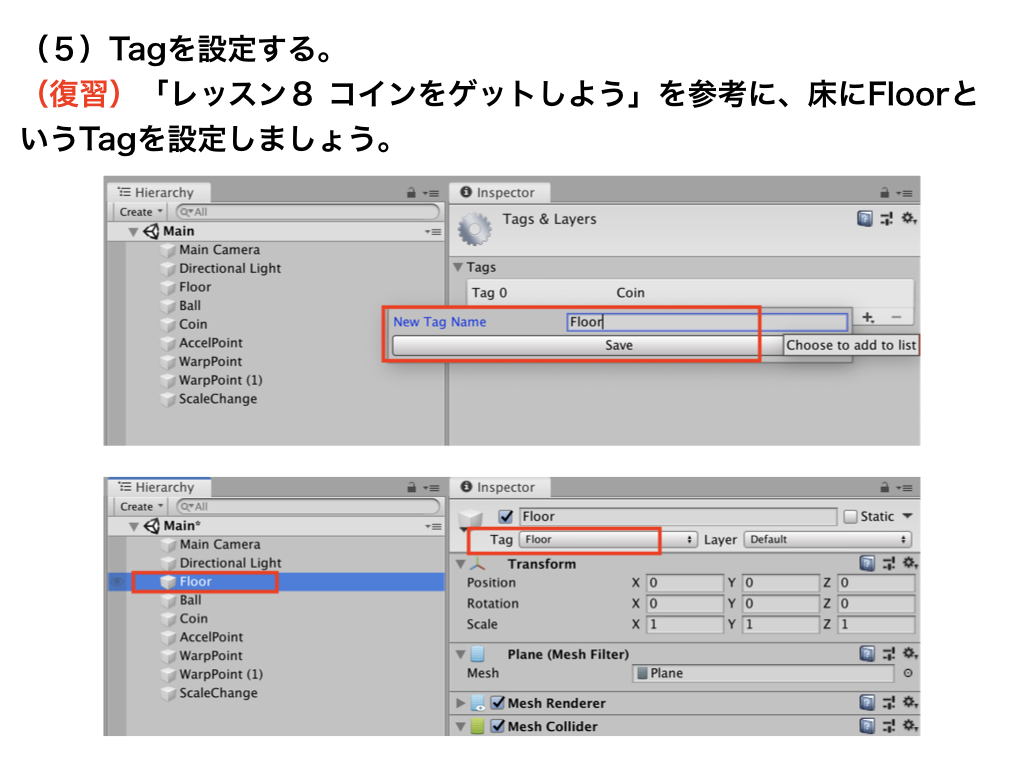
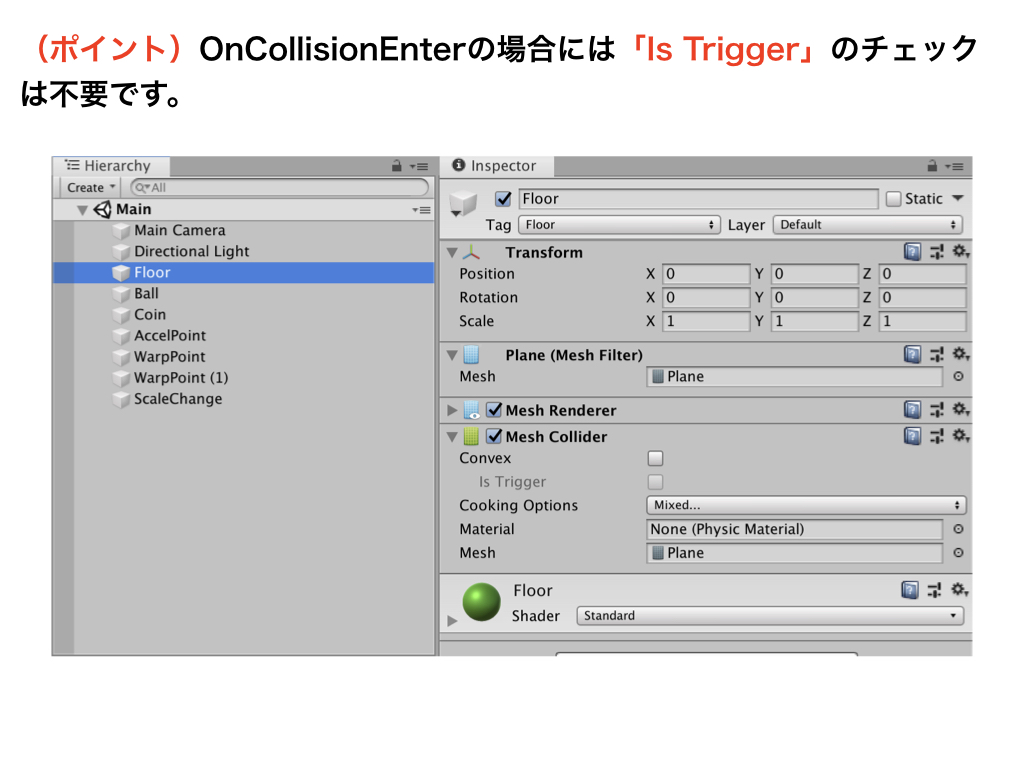
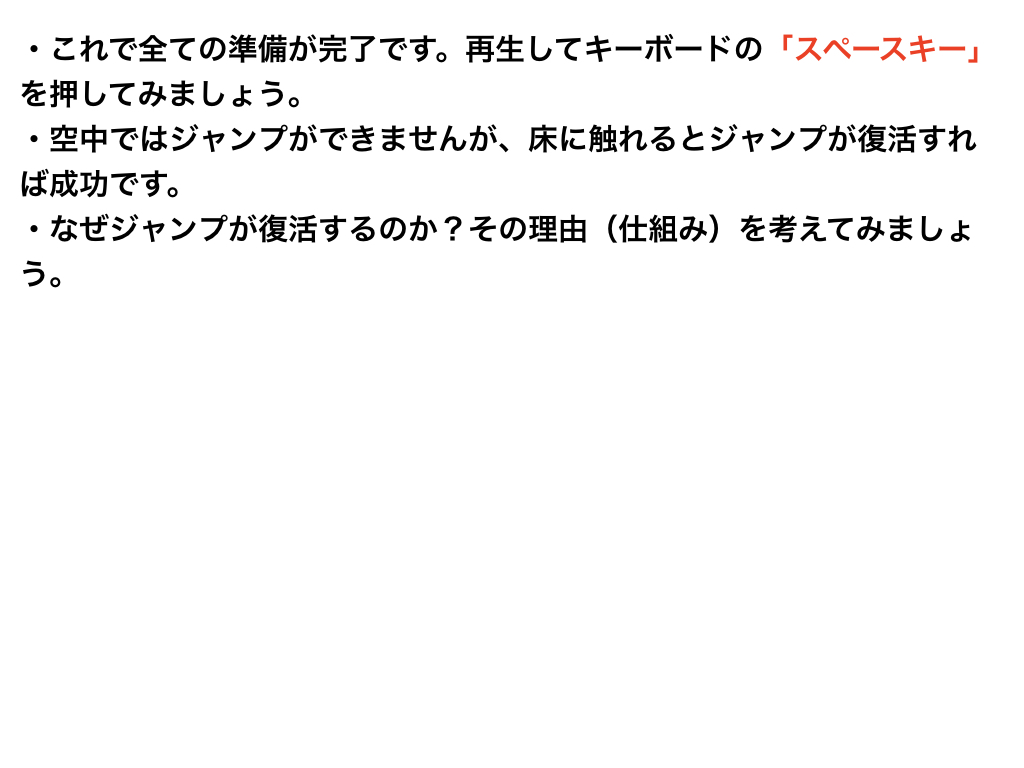
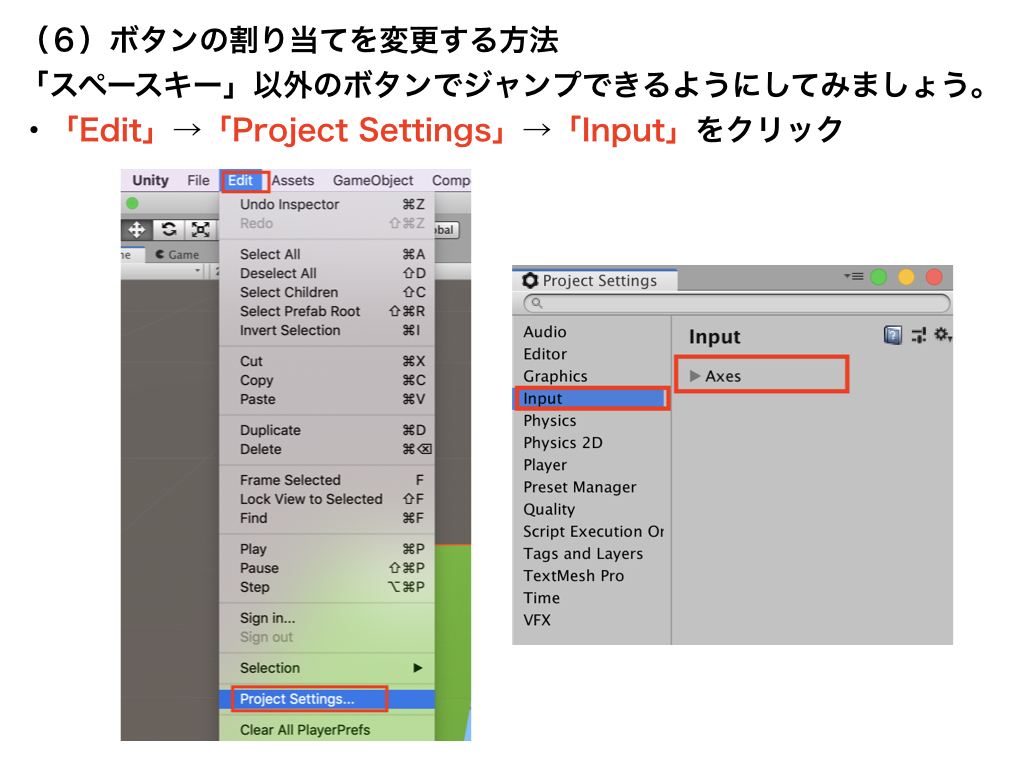
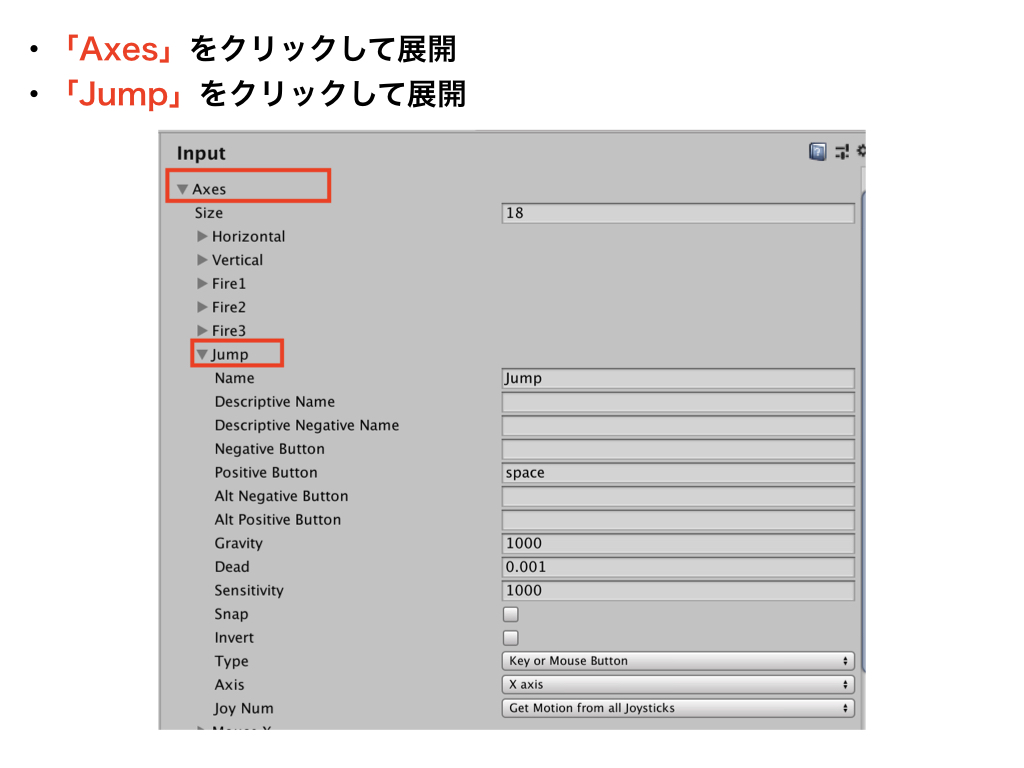
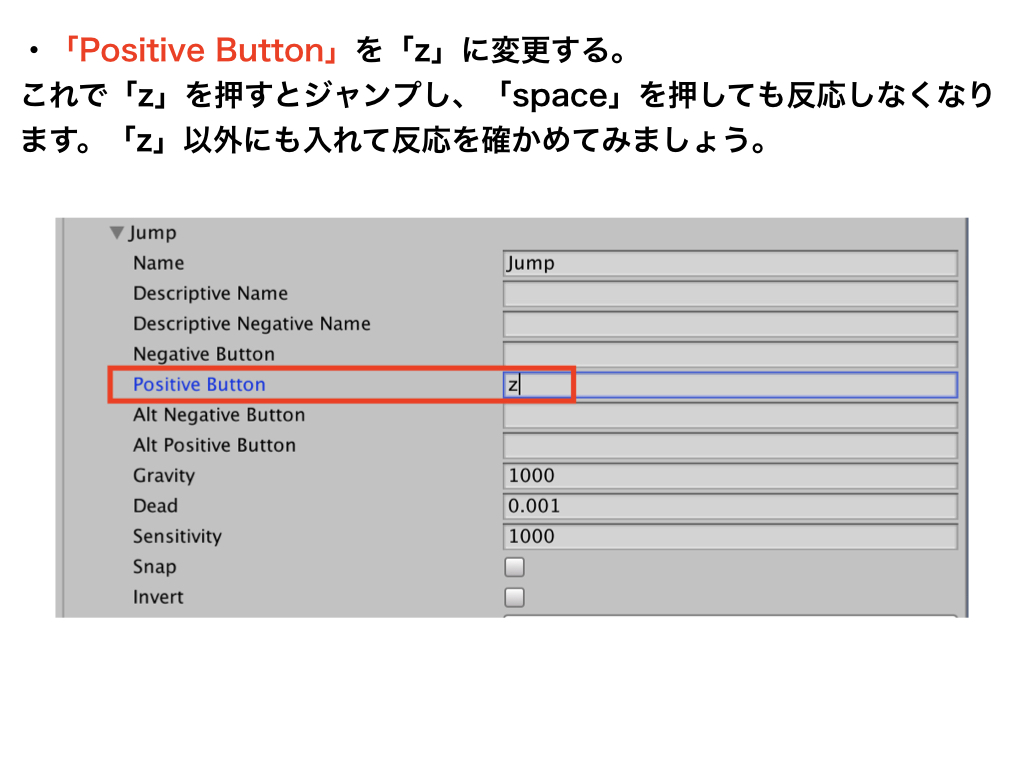
【2019版】BallGame(全27回)
他のコースを見る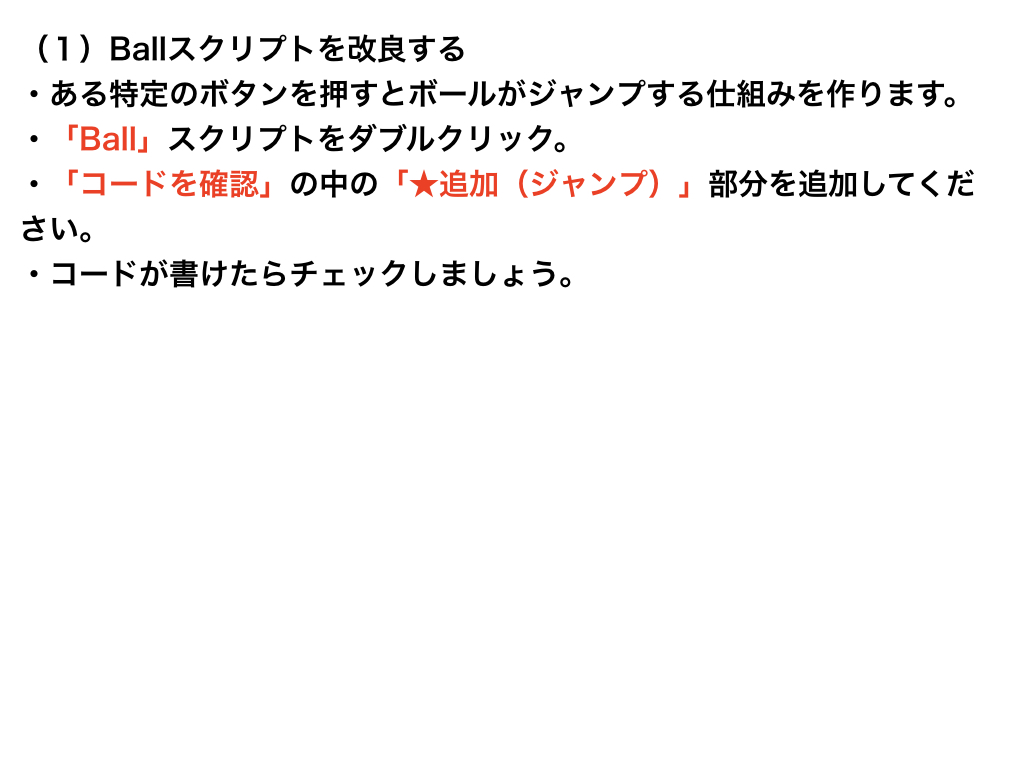
ジャンプ
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
// ★追加(ジャンプ)
public float jumpSpeed;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
// ★追加(ジャンプ)
if (Input.GetButtonDown("Jump"))
{
rb.velocity = Vector3.up * jumpSpeed;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
}
}
}
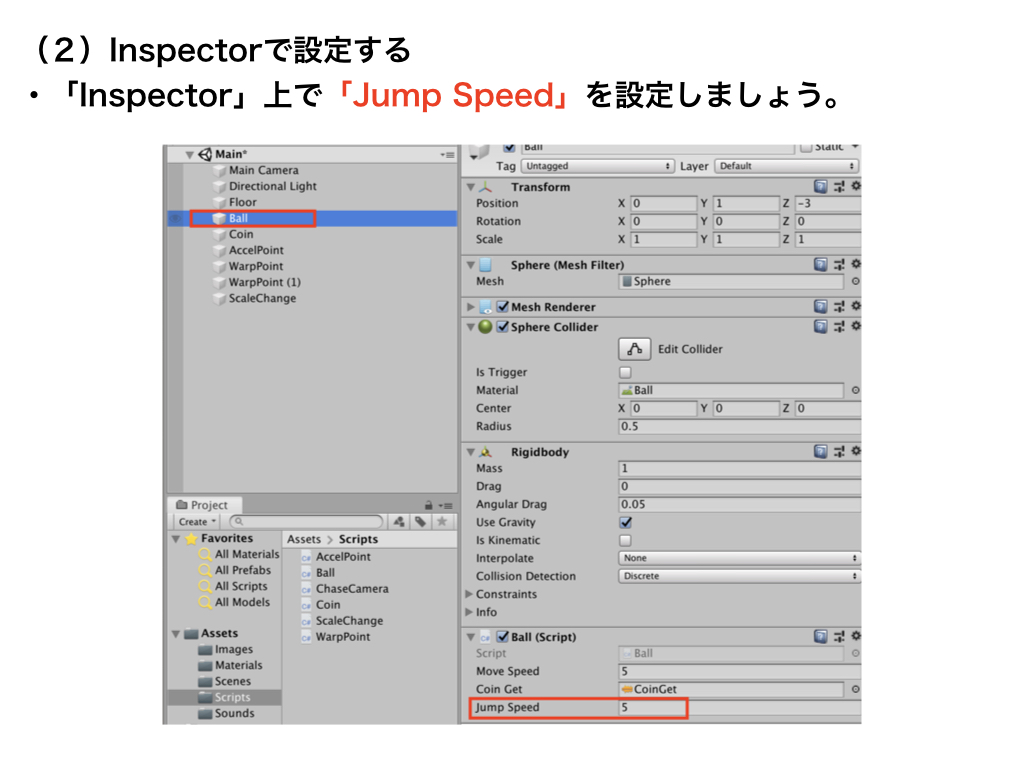
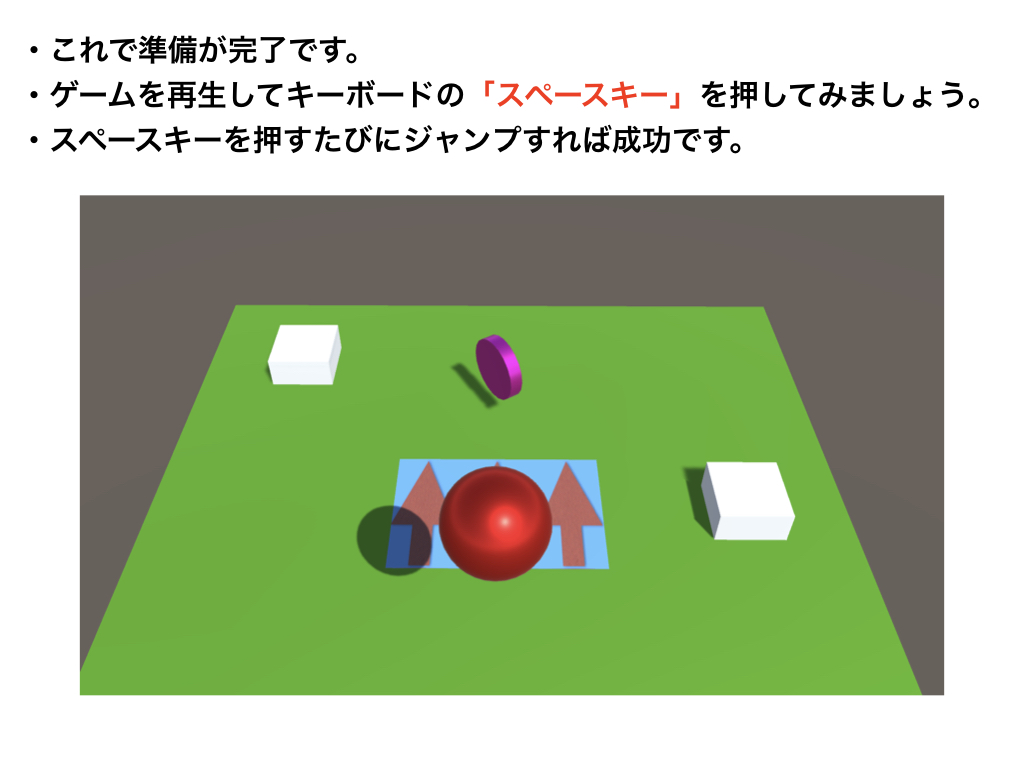
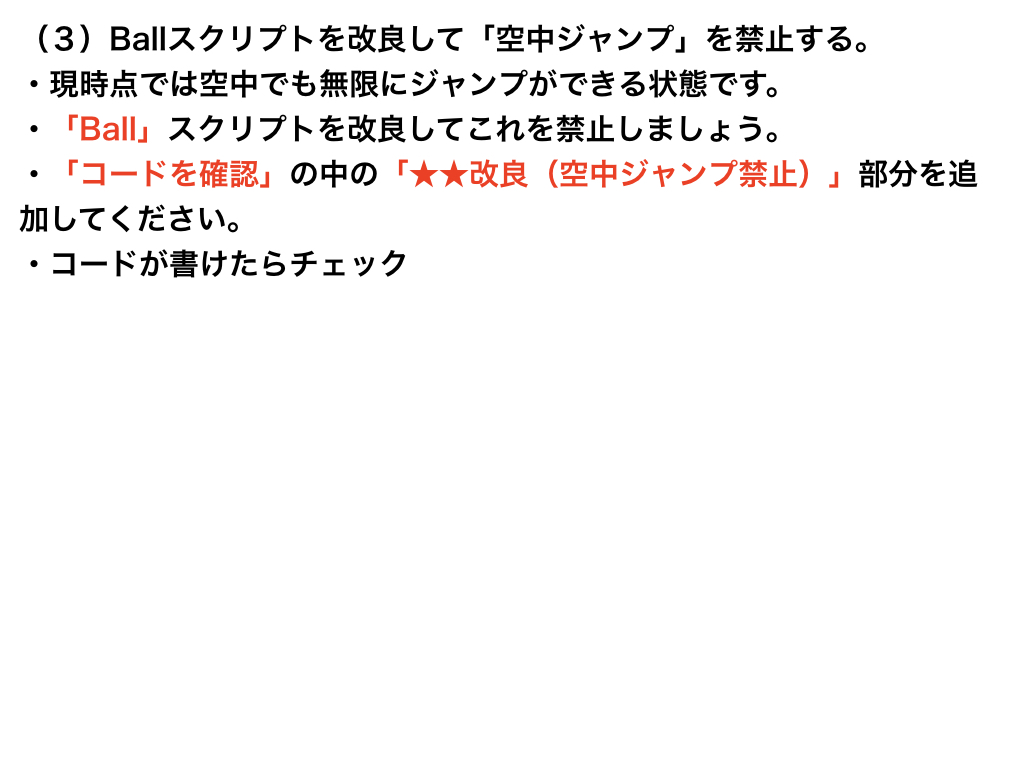
空中ジャンプの禁止
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
// ★★改良(空中ジャンプ禁止)
// boolは「真偽型」と呼ばれ「true」か「false」の2つの値を扱うことができます。
private bool isJumping = false;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
// ★★改良(空中ジャンプ禁止)
// 「&&」は「包含関係の『かつ』の意味」
// (例)「A && B」AかつB・・・>AとBの両方の条件が揃った時
// 「==」は「左右が等しい」という意味
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
// isJumpingという名前の箱の中身を「true」にする。
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
}
}
}
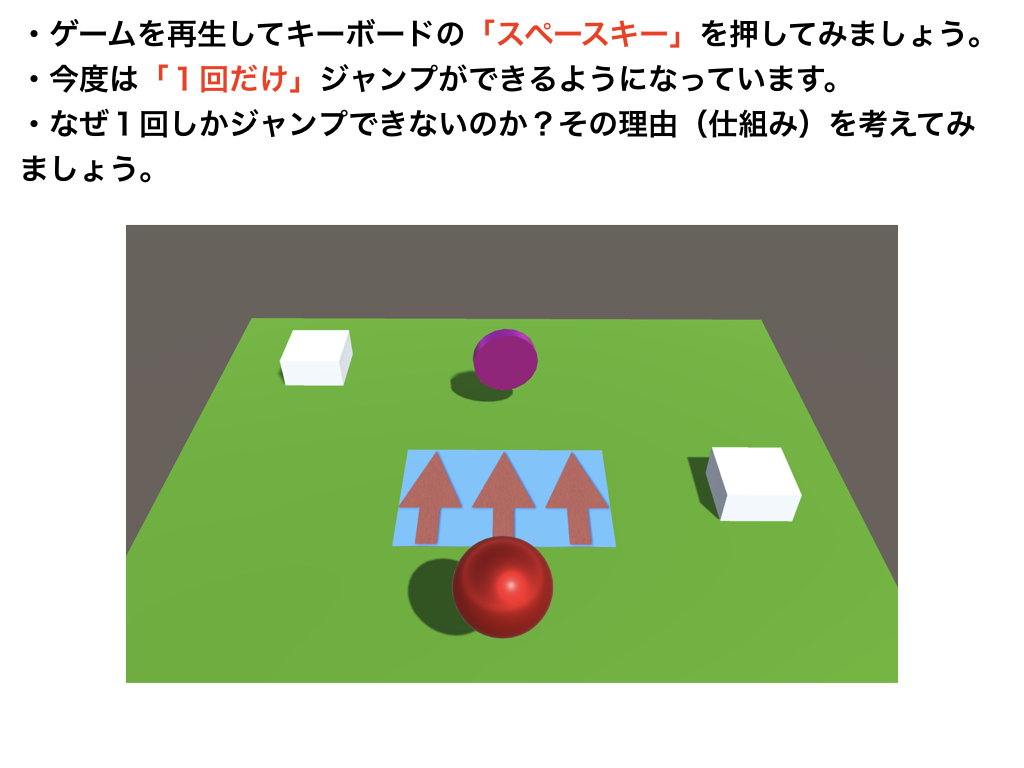
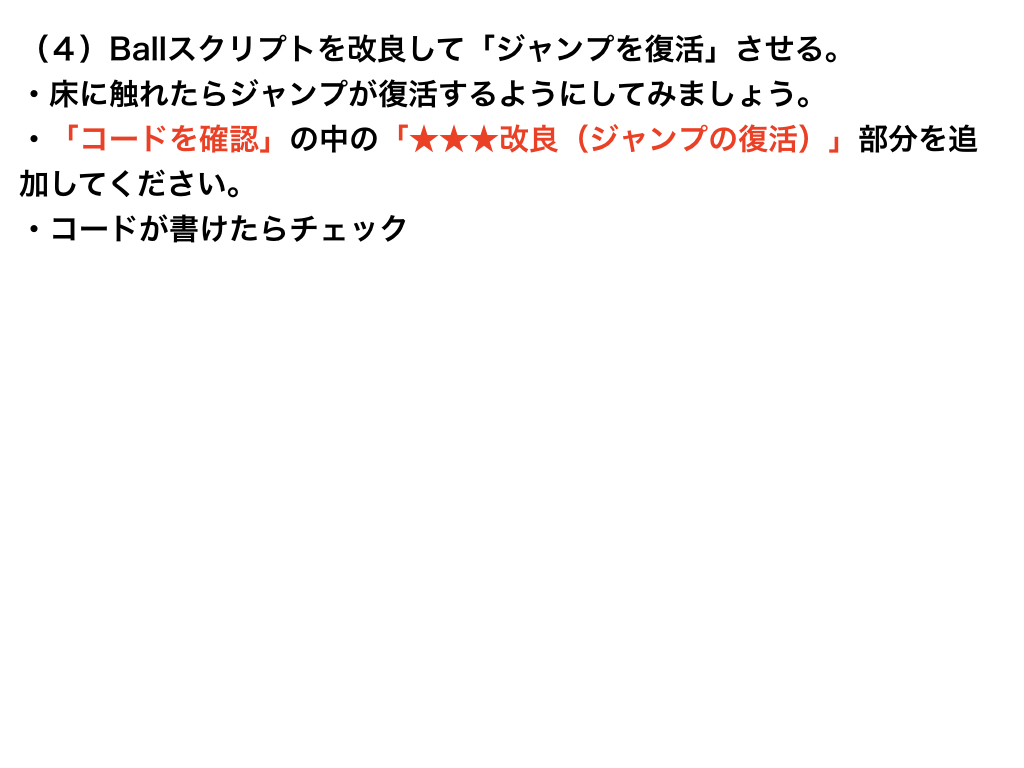
ジャンプの復活
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Ball : MonoBehaviour
{
public float moveSpeed;
private Rigidbody rb;
public AudioClip coinGet;
public float jumpSpeed;
private bool isJumping = false;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveH = Input.GetAxis("Horizontal");
float moveV = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveH, 0, moveV);
rb.AddForce(movement * moveSpeed);
if (Input.GetButtonDown("Jump") && isJumping == false)
{
rb.velocity = Vector3.up * jumpSpeed;
isJumping = true;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.CompareTag("Coin"))
{
Destroy(other.gameObject);
AudioSource.PlayClipAtPoint(coinGet, transform.position);
}
}
// ★★★改良(ジャンプの復活)
// OnCollisionEnterのスペルに注意
private void OnCollisionEnter(Collision collision)
{
if(collision.gameObject.CompareTag("Floor"))
{
isJumping = false;
}
}
}
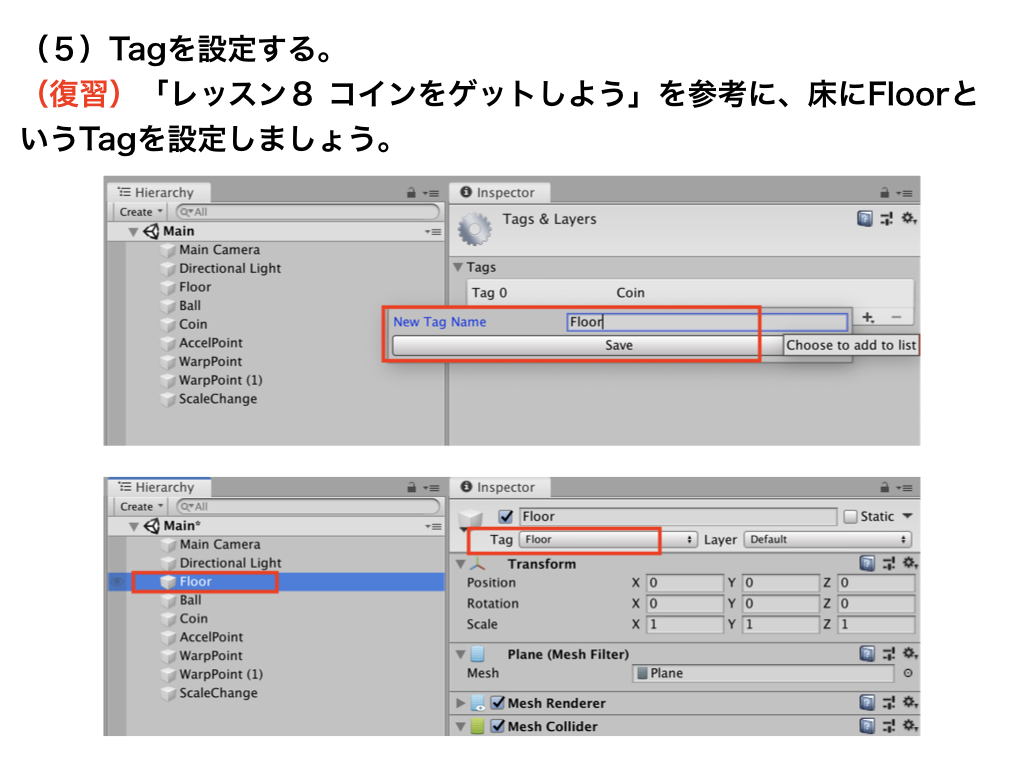
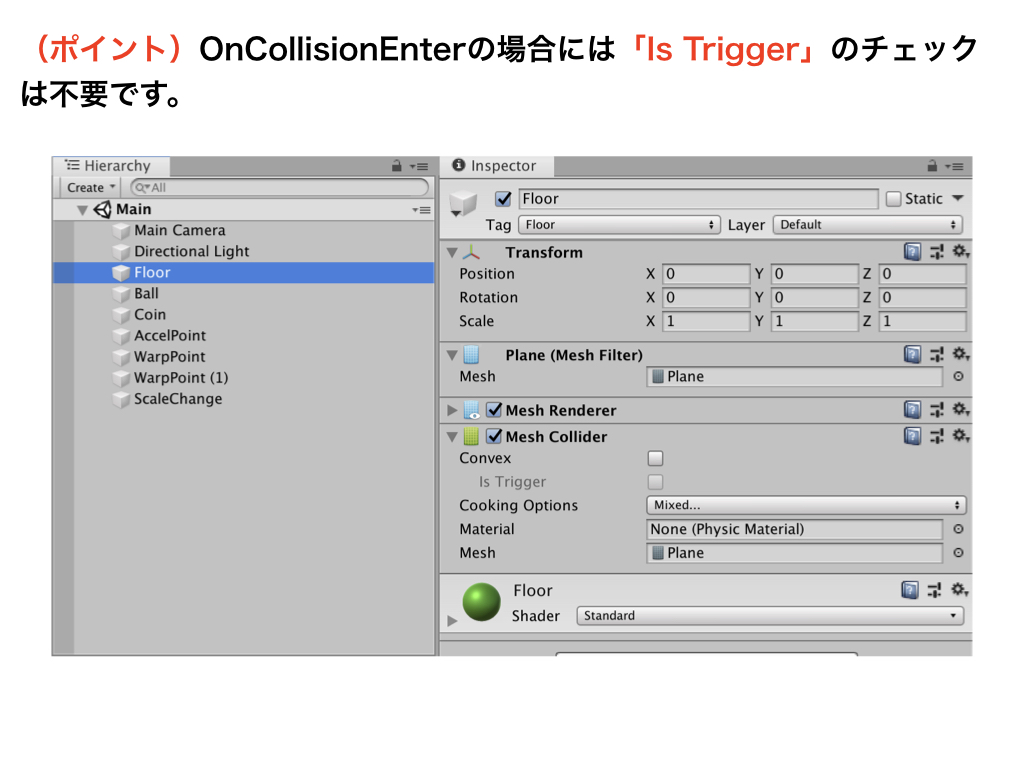
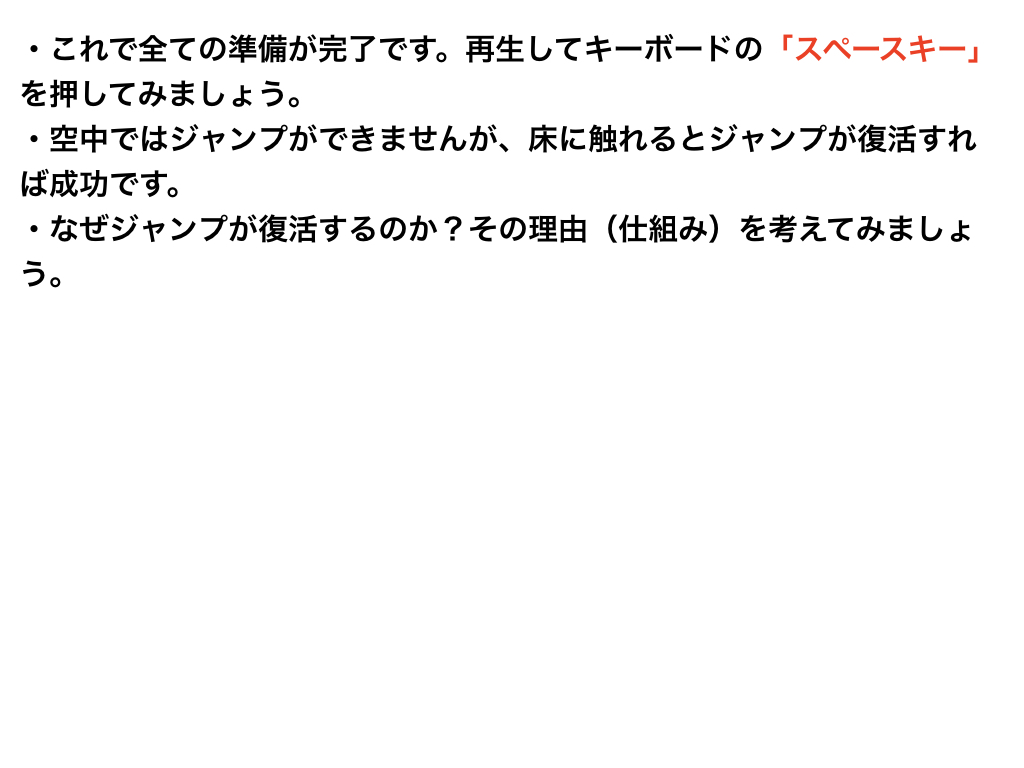
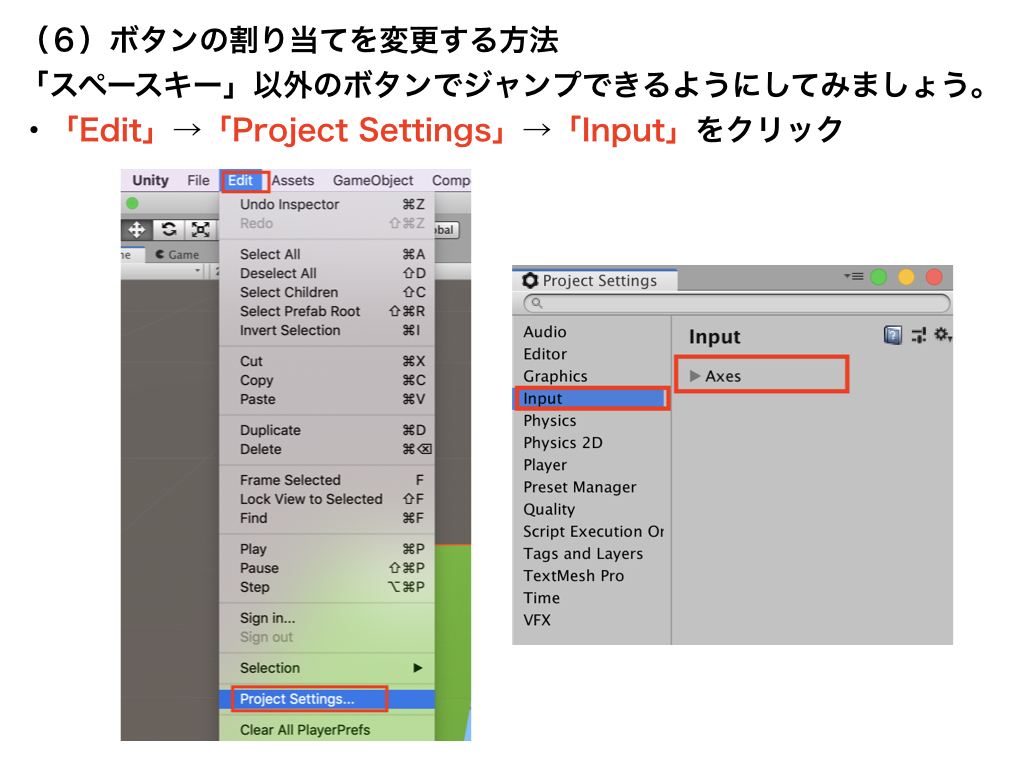
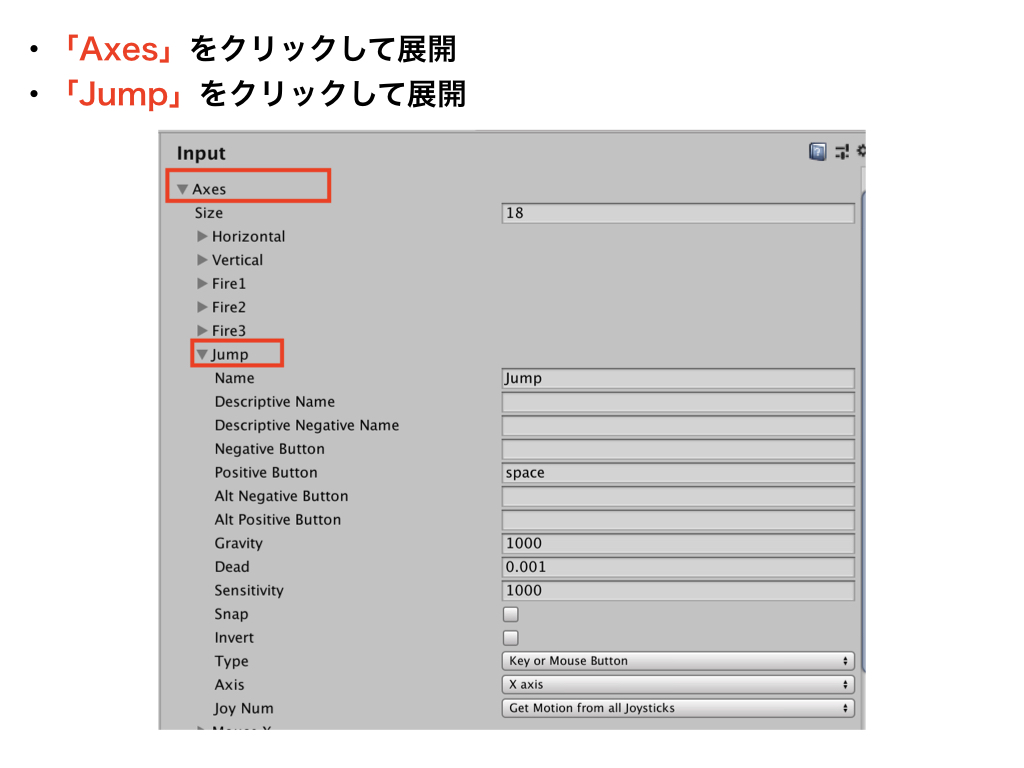
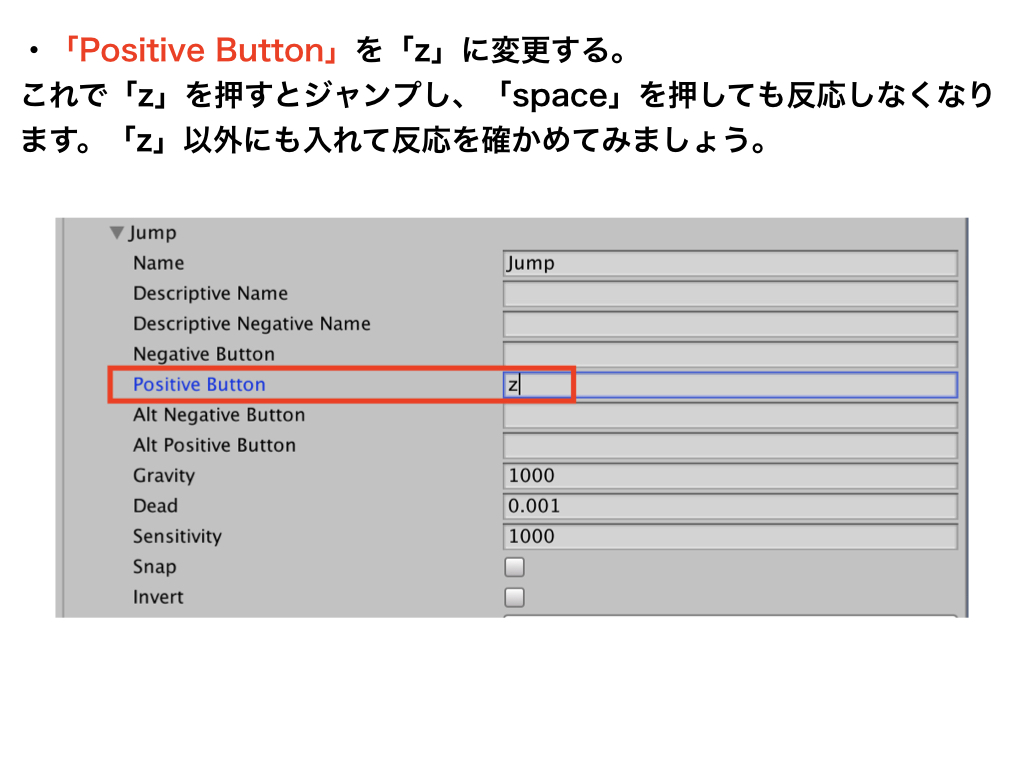
ボールをジャンプさせよう