Tankアセットへ切り替え&シンプルに動かす
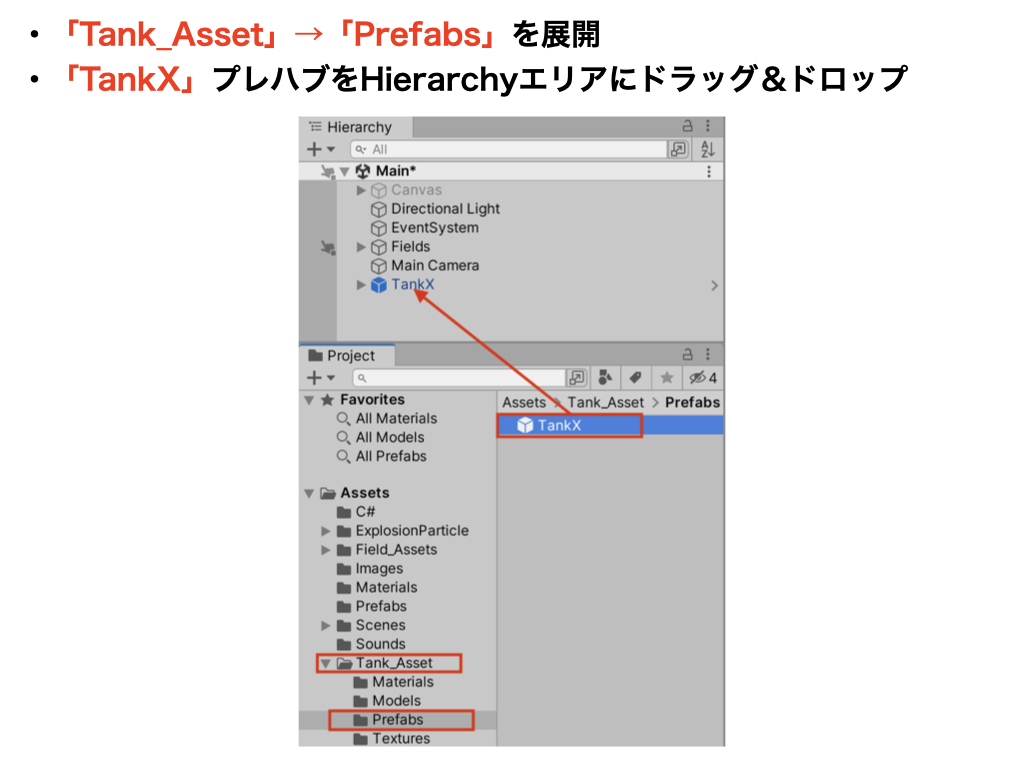
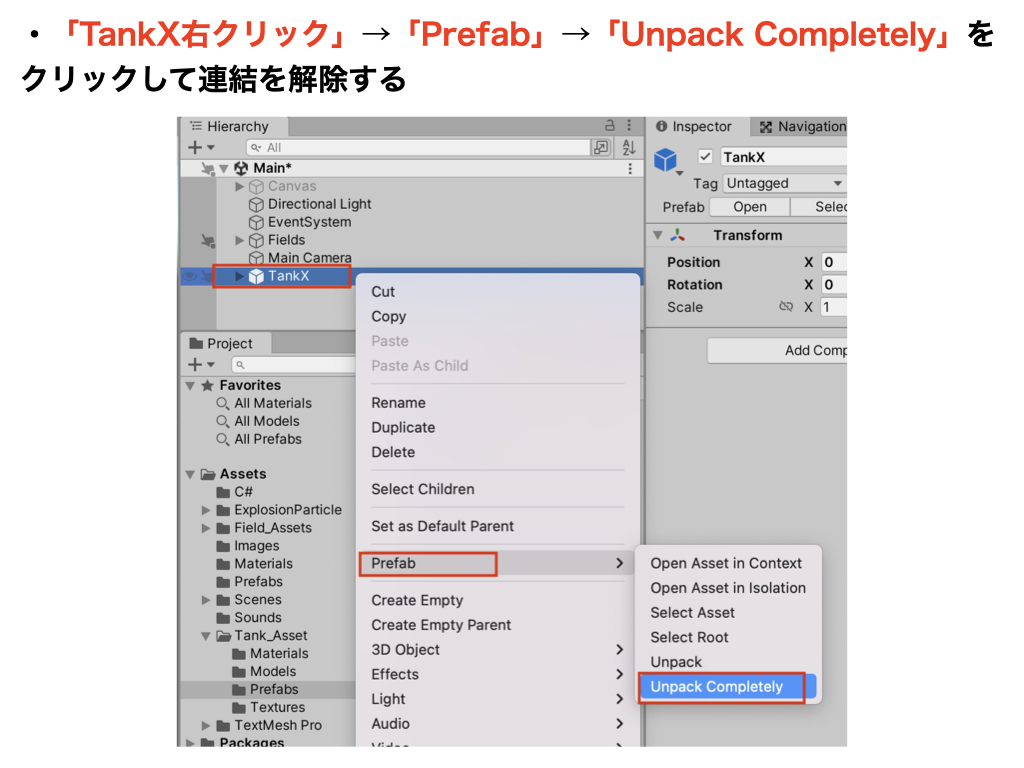
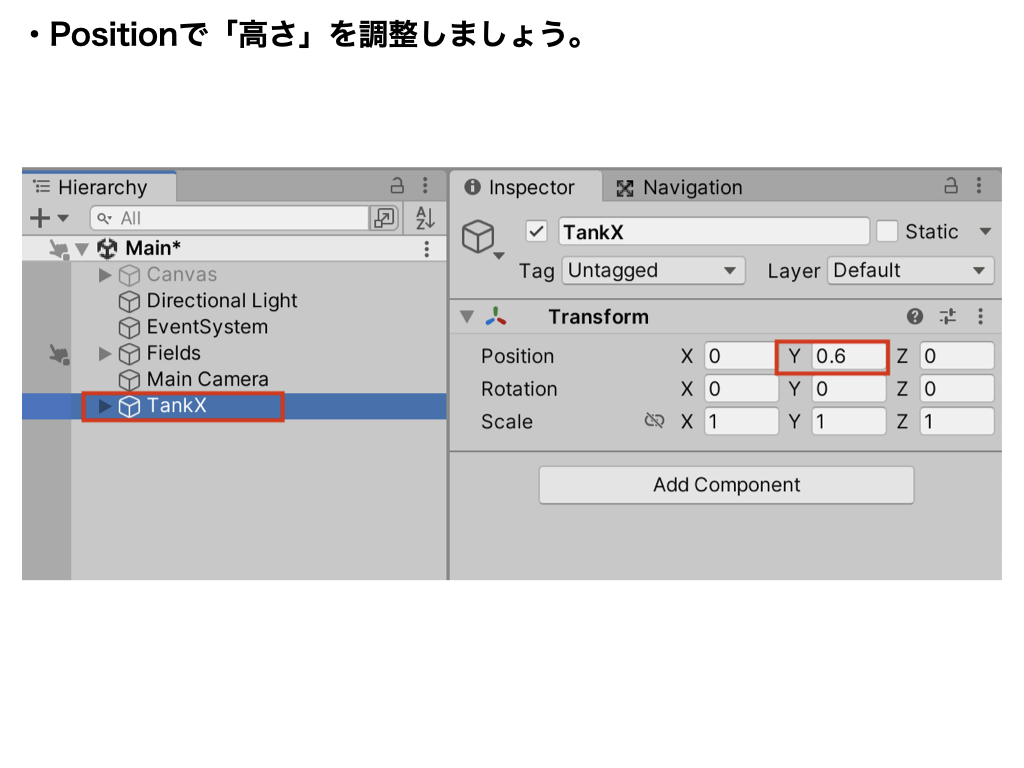
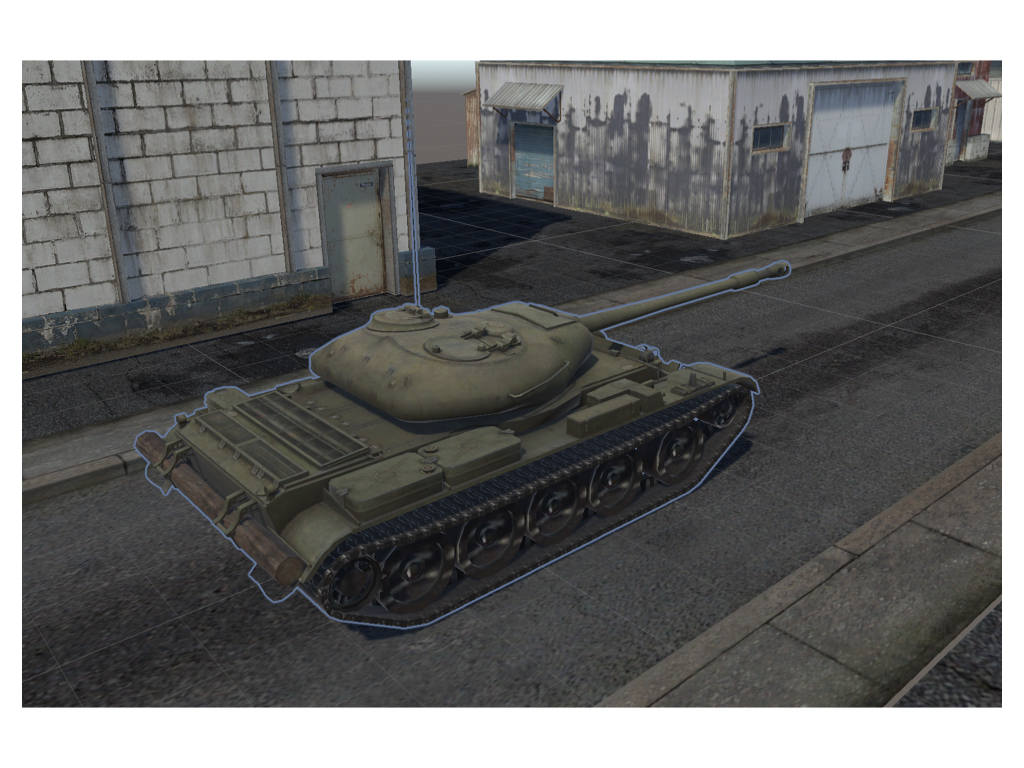
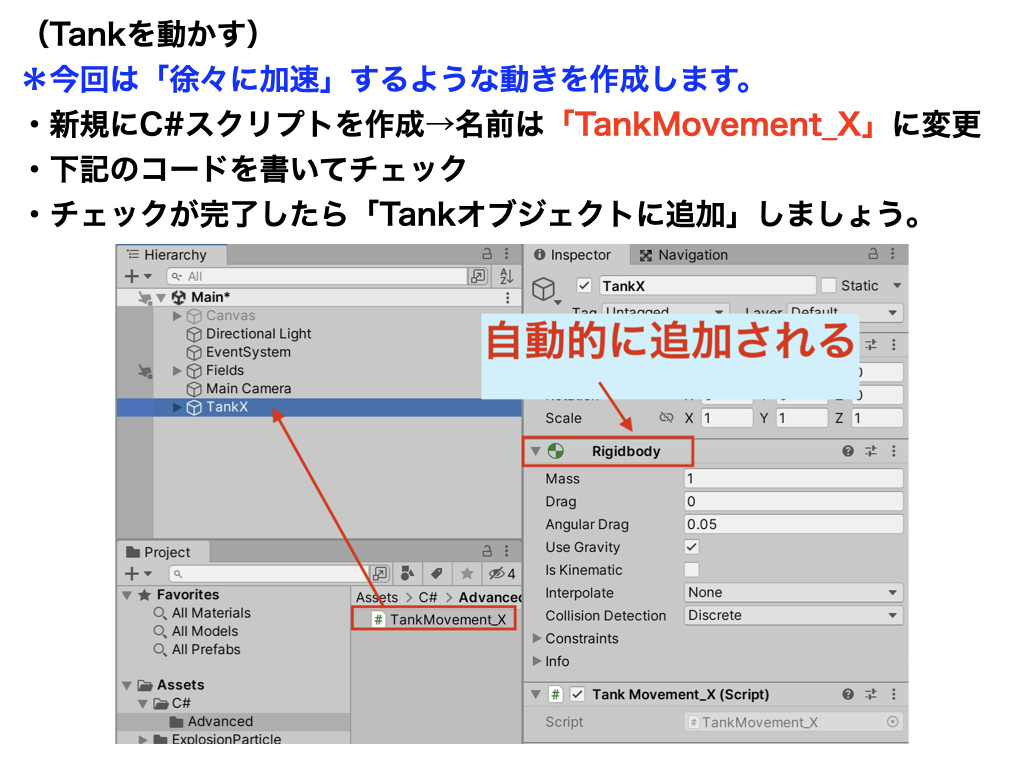
Tankを動かす
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★コンポーネントの自動追加
// Rigidbodyを自動的に追加してくれる。
[RequireComponent(typeof(Rigidbody))]
public class TankMovement_X : MonoBehaviour
{
private float turnInputValue;
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
// 前進(Wキー押しで前進)
if(Input.GetKey(KeyCode.W))
{
rb.velocity += transform.forward * 0.4f; // 前進速度は自由に変更可能
}
// 前進(Sキー押しで後退)
if (Input.GetKey(KeyCode.S))
{
rb.velocity -= transform.forward * 0.4f; // 前進速度は自由に変更可能
}
TankTurn();
}
// 旋回
void TankTurn()
{
turnInputValue = Input.GetAxis("Horizontal");
float turn = turnInputValue * Time.deltaTime * 50; // 旋回速度は自由に変更可能
Quaternion turnRotation = Quaternion.Euler(0, turn, 0);
rb.MoveRotation(rb.rotation * turnRotation);
}
}
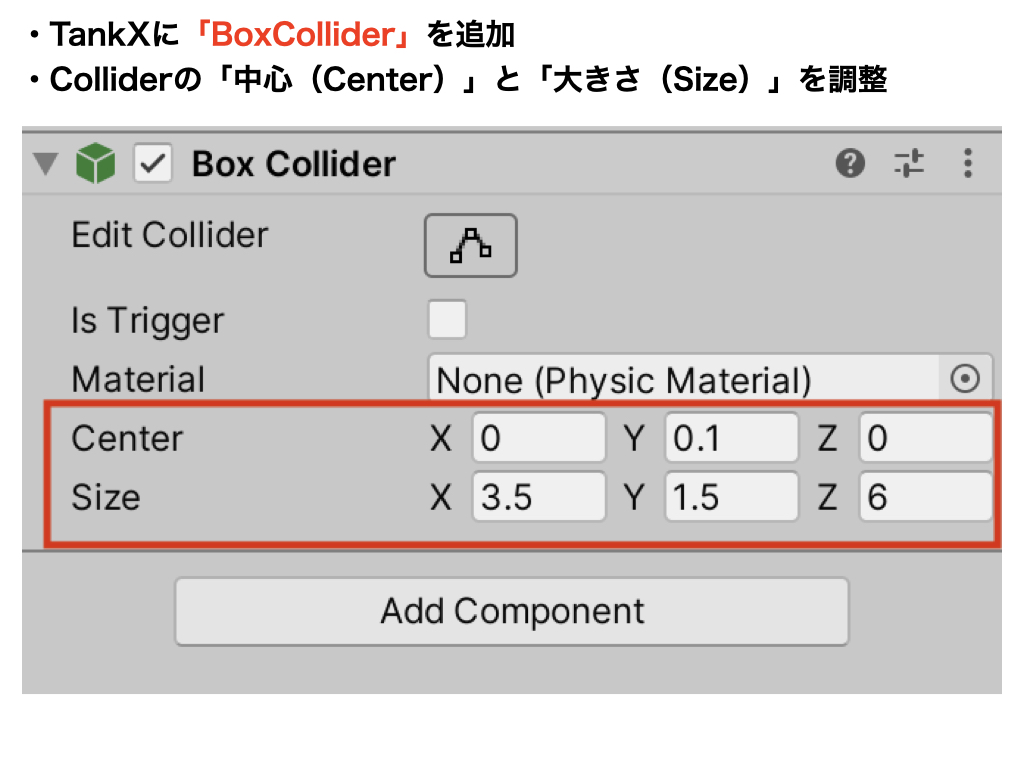
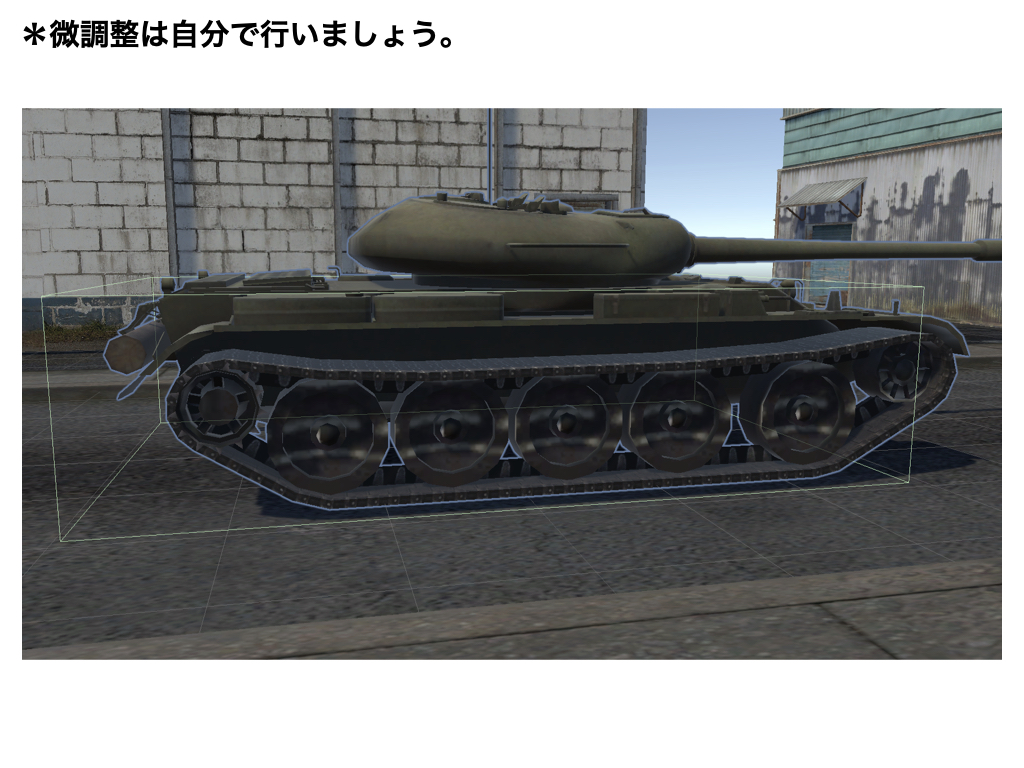
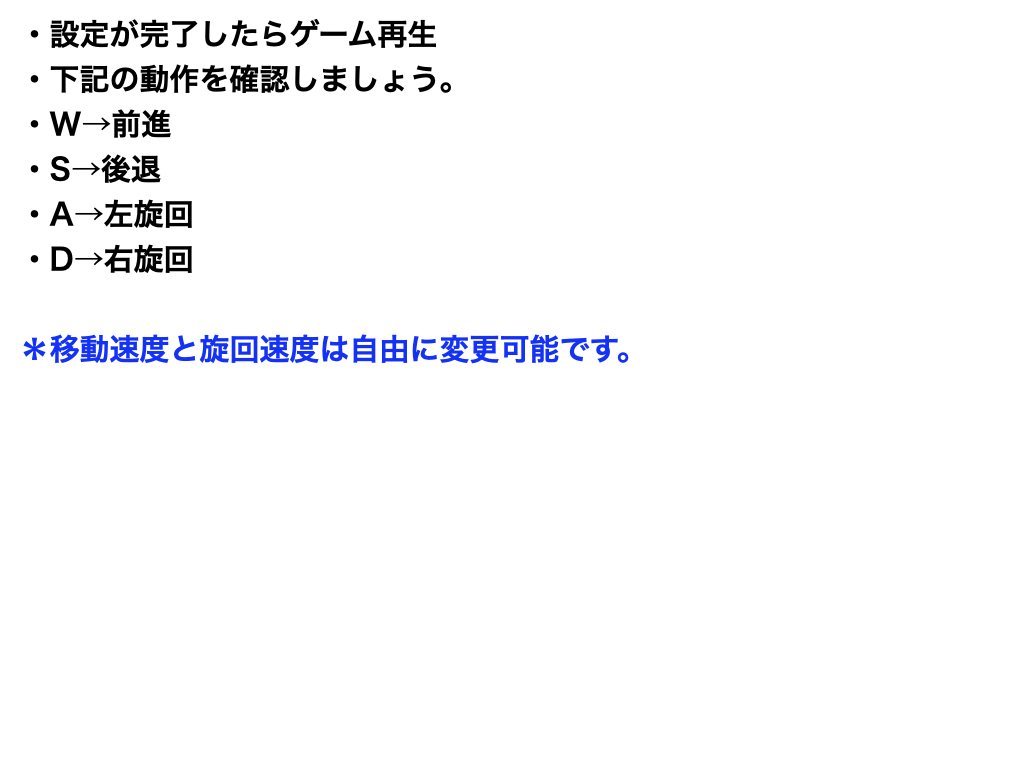
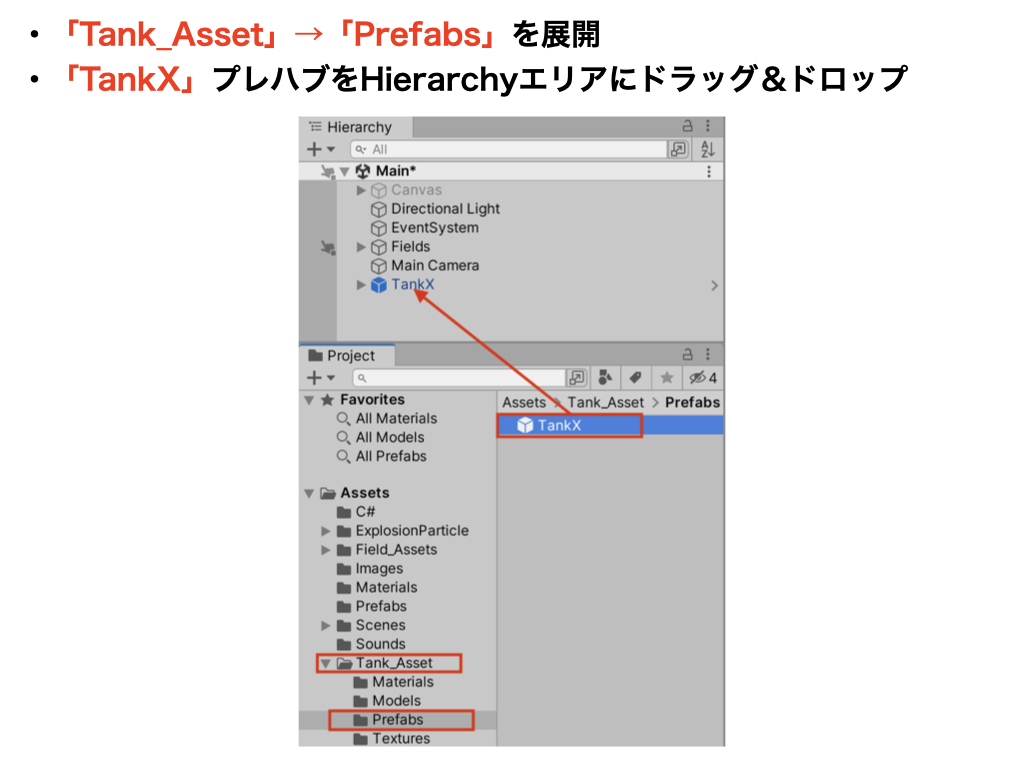
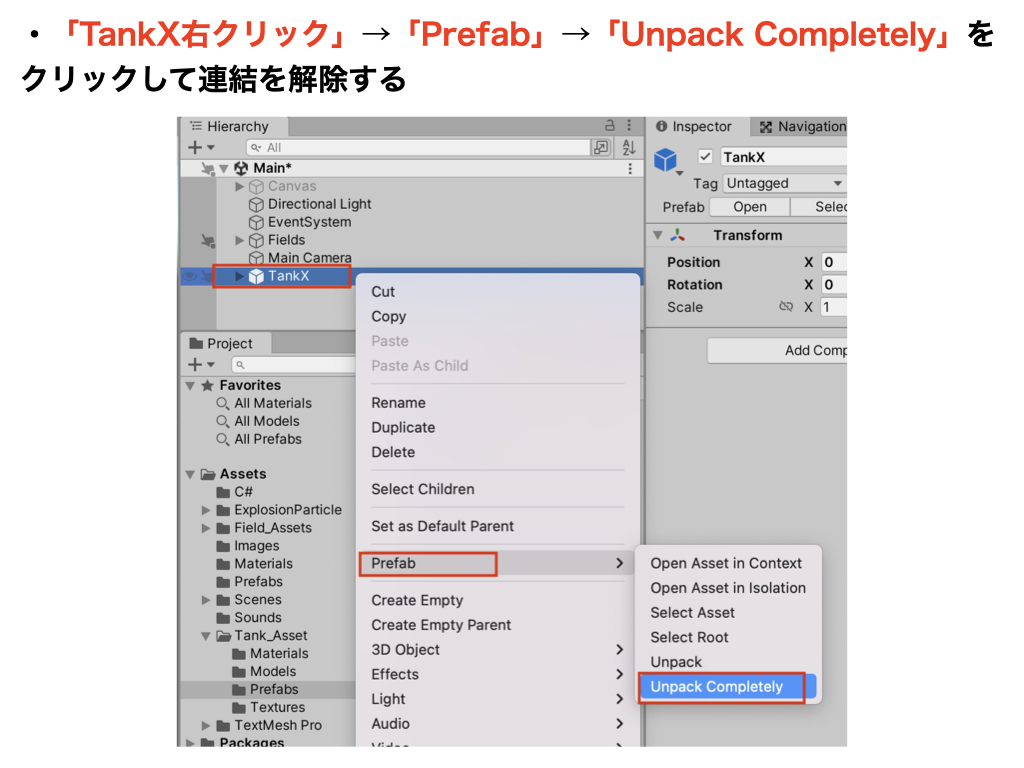
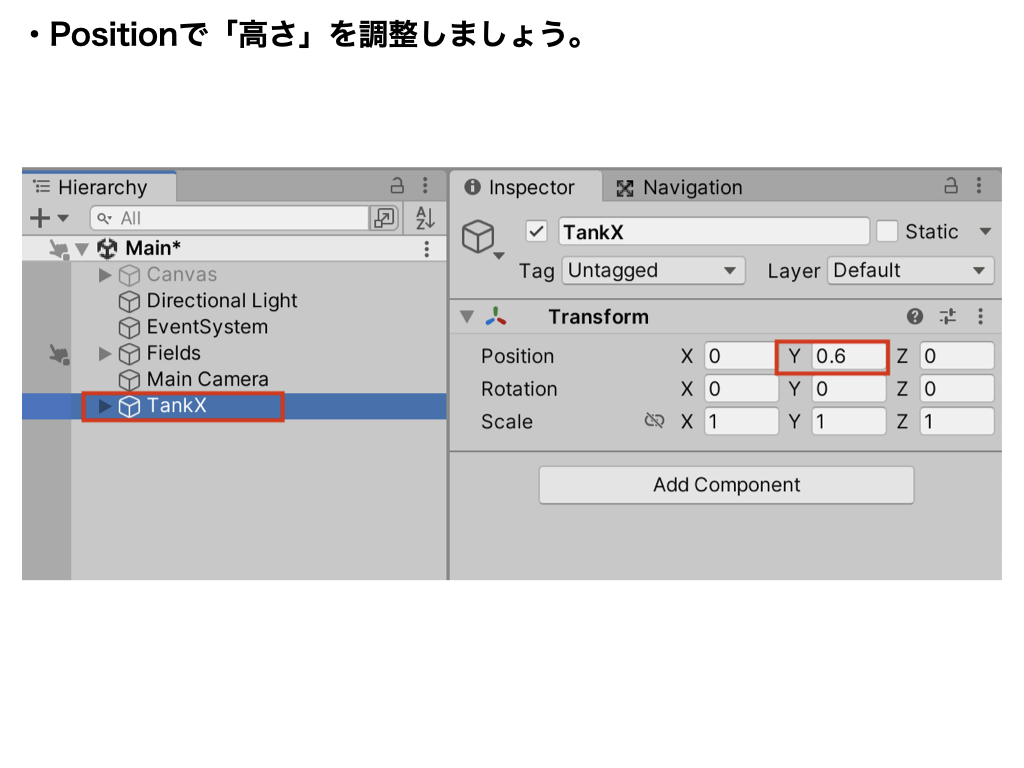
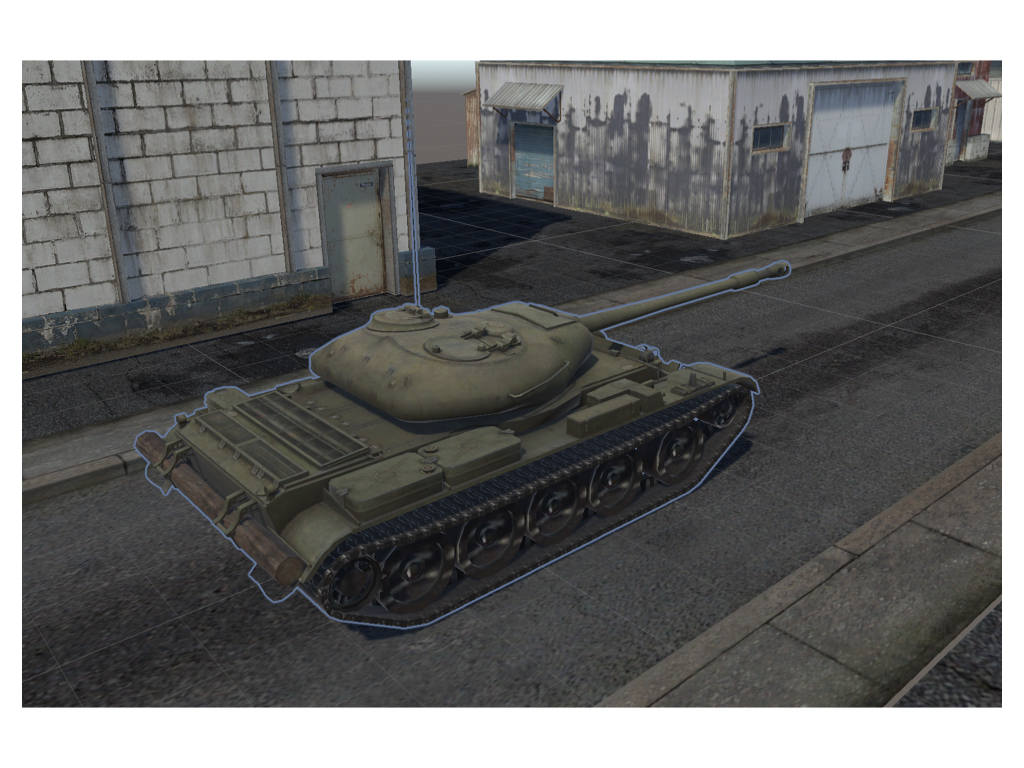
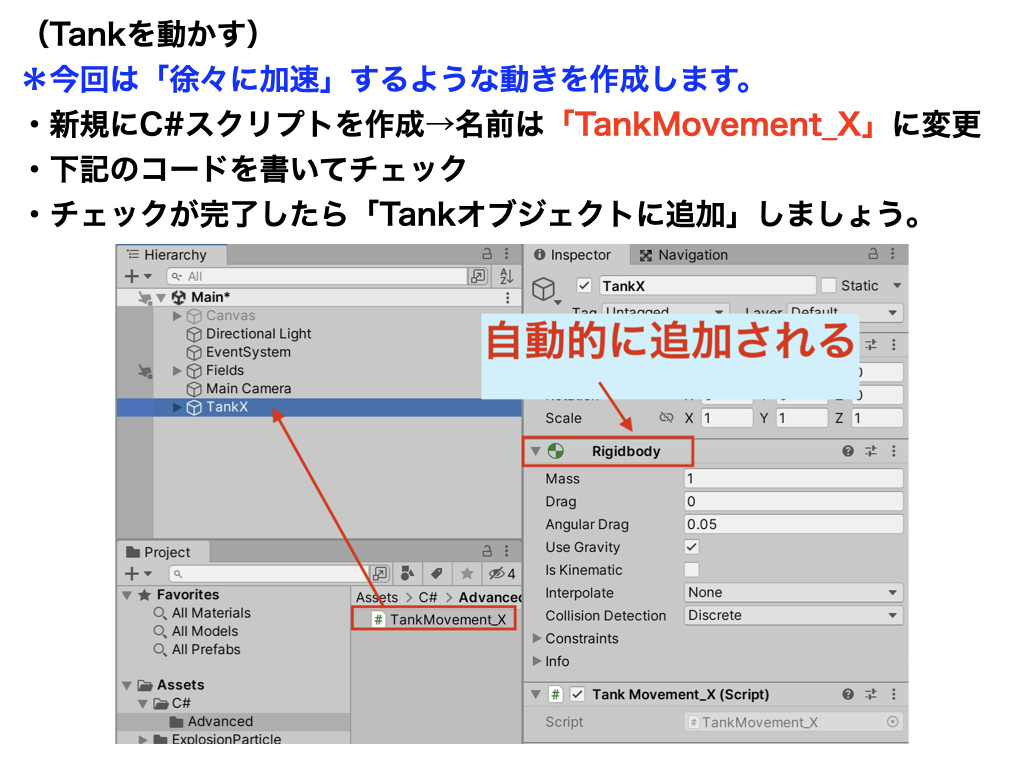
Tankを動かす
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
// ★コンポーネントの自動追加
// Rigidbodyを自動的に追加してくれる。
[RequireComponent(typeof(Rigidbody))]
public class TankMovement_X : MonoBehaviour
{
private float turnInputValue;
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
// 前進(Wキー押しで前進)
if(Input.GetKey(KeyCode.W))
{
rb.velocity += transform.forward * 0.4f; // 前進速度は自由に変更可能
}
// 前進(Sキー押しで後退)
if (Input.GetKey(KeyCode.S))
{
rb.velocity -= transform.forward * 0.4f; // 前進速度は自由に変更可能
}
TankTurn();
}
// 旋回
void TankTurn()
{
turnInputValue = Input.GetAxis("Horizontal");
float turn = turnInputValue * Time.deltaTime * 50; // 旋回速度は自由に変更可能
Quaternion turnRotation = Quaternion.Euler(0, turn, 0);
rb.MoveRotation(rb.rotation * turnRotation);
}
}
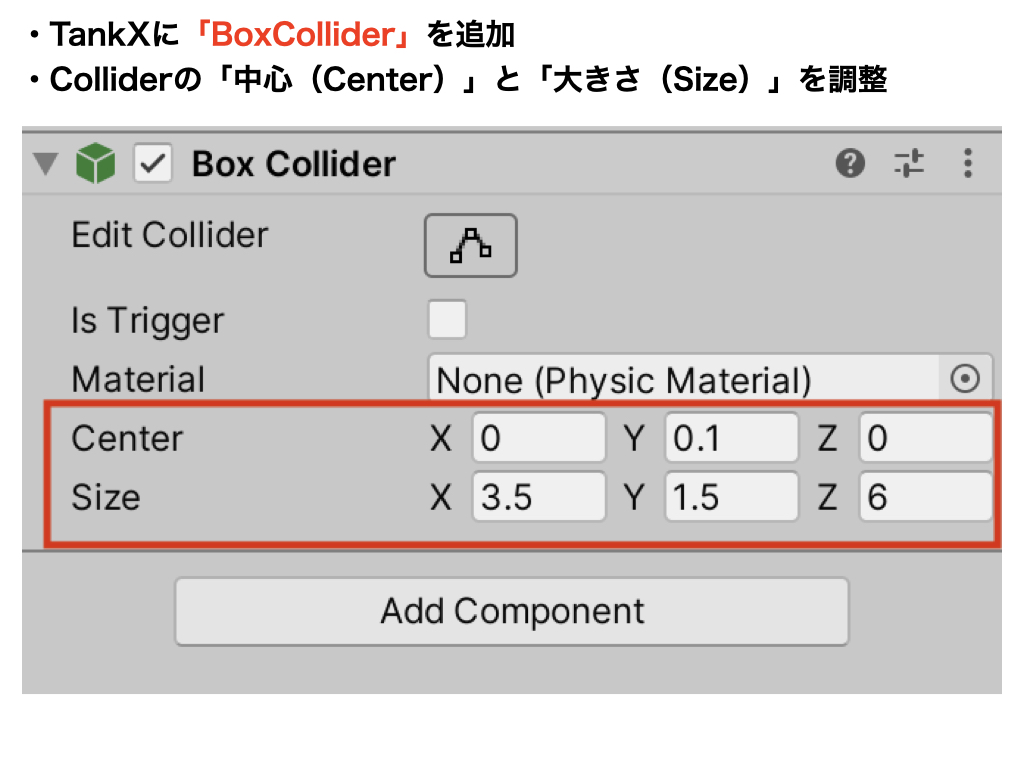
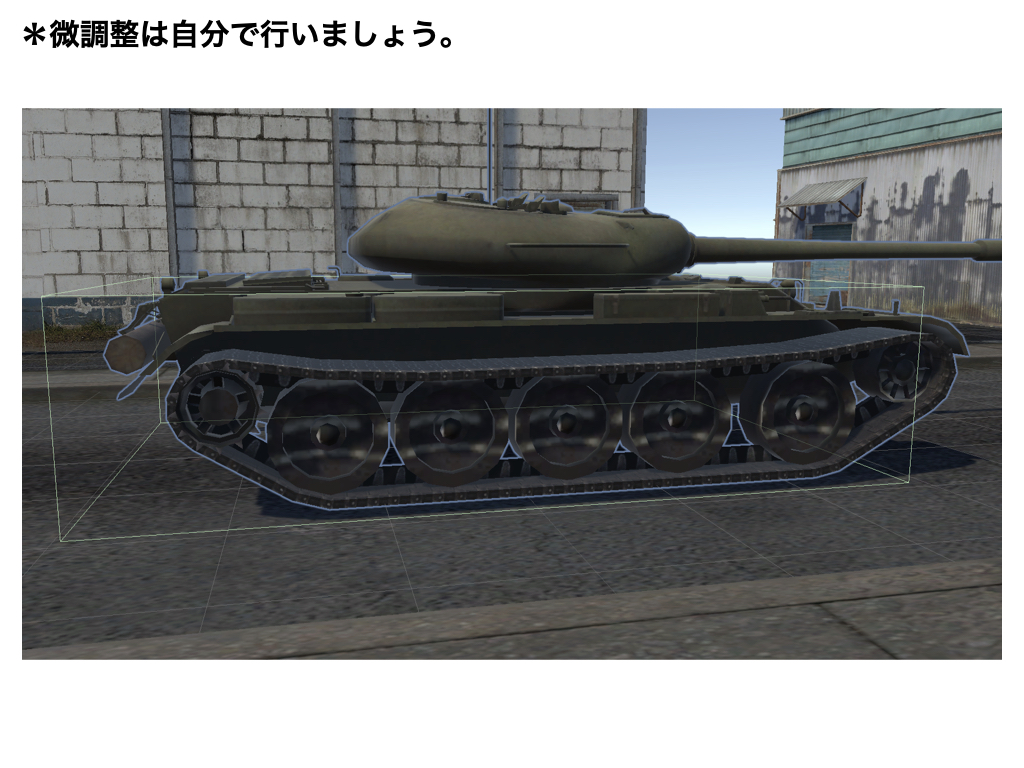
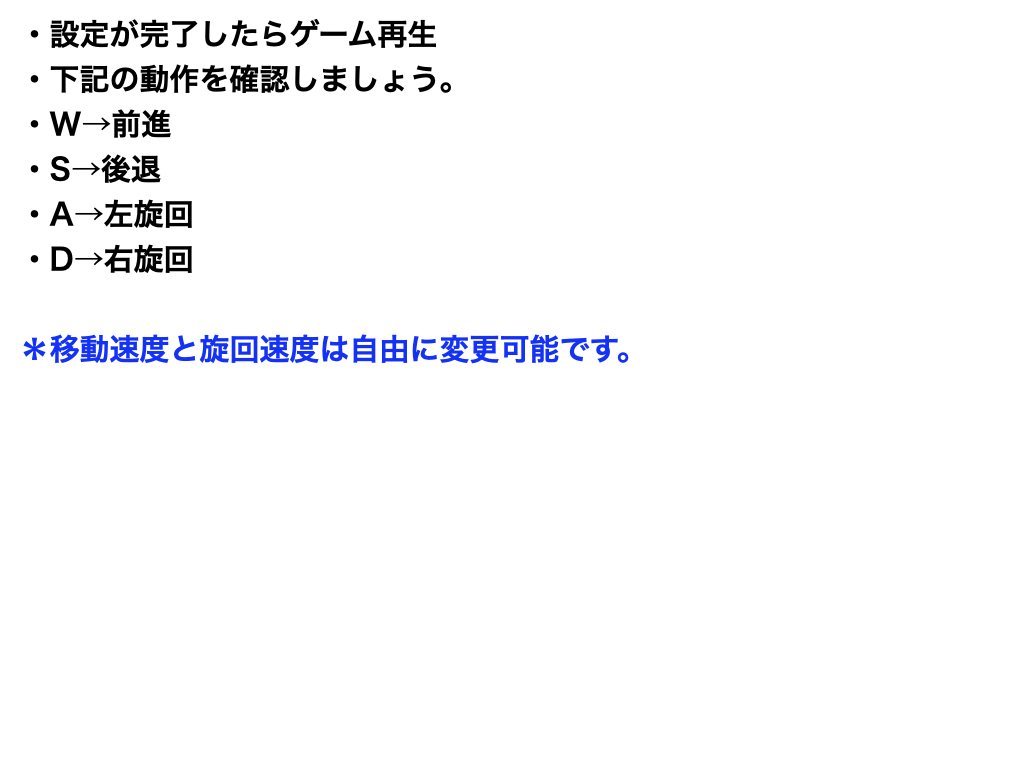
Tankアセットへ切り替え&シンプルに動かす