残弾数を回復させる「権利」の作成
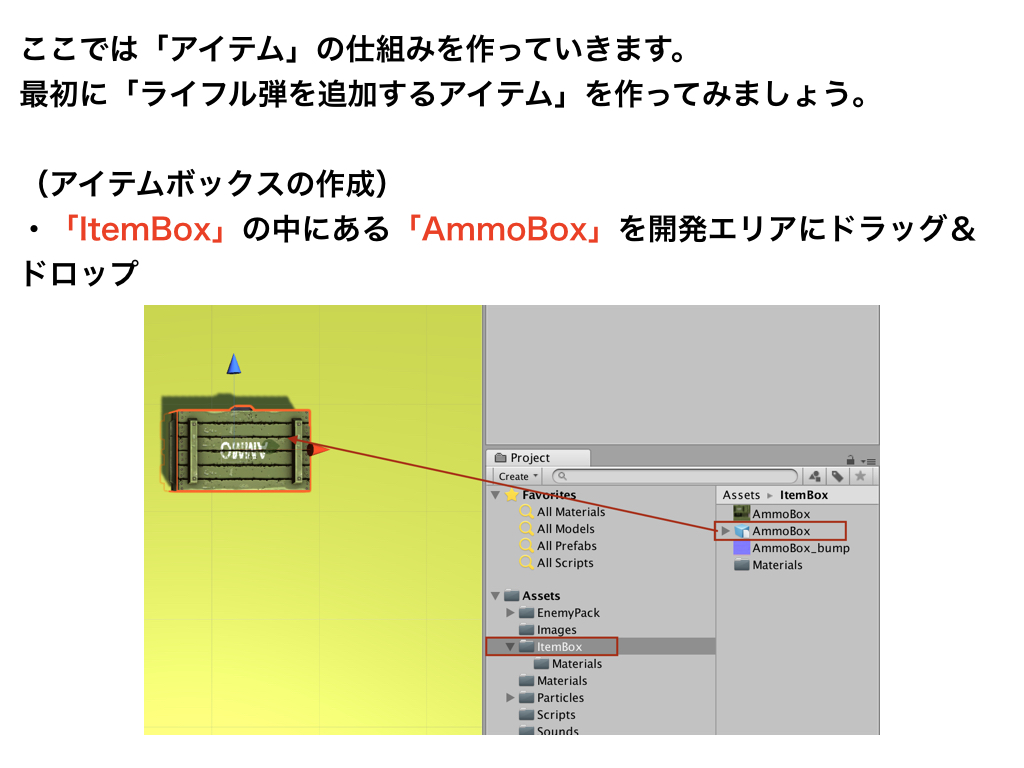
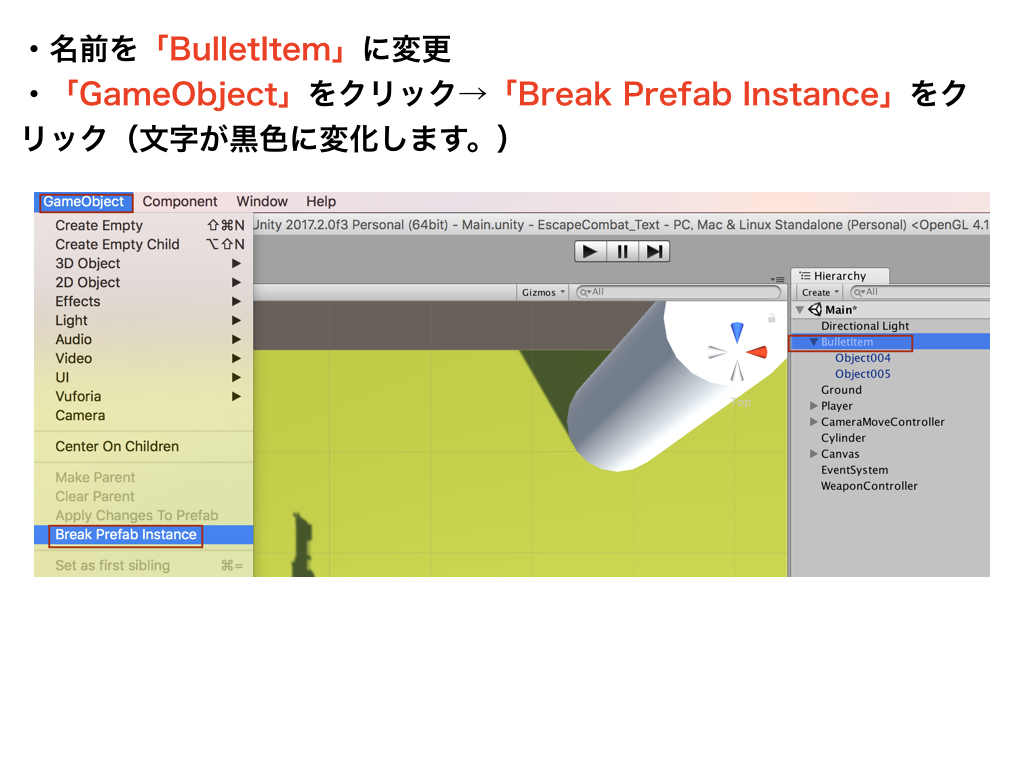
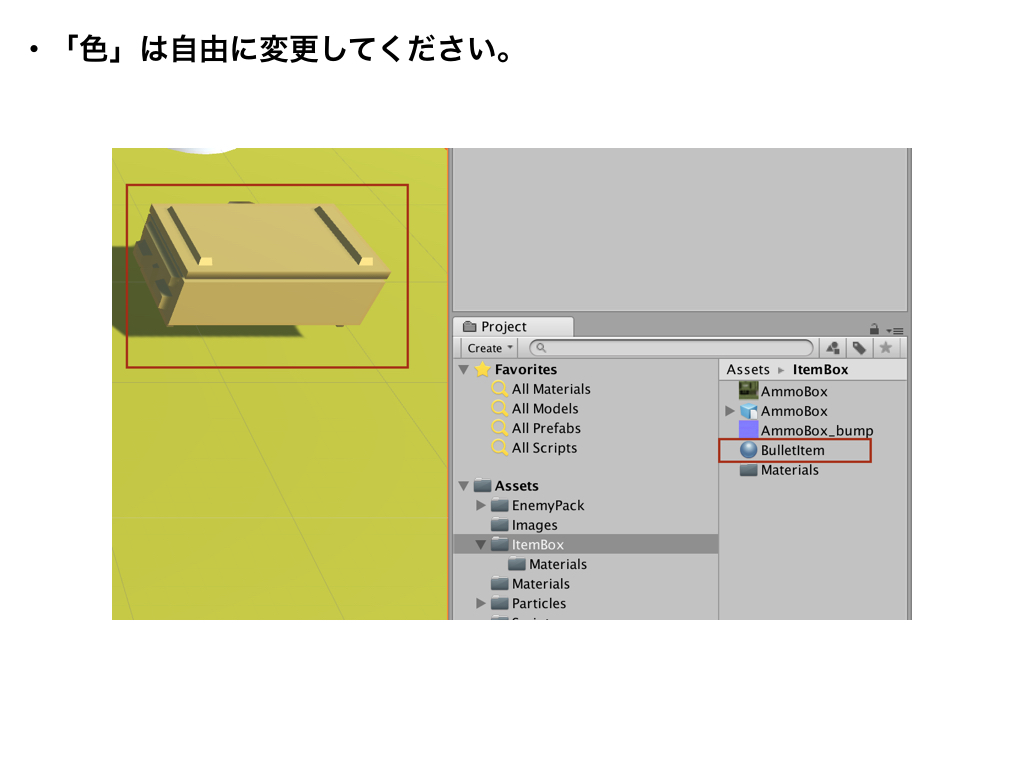
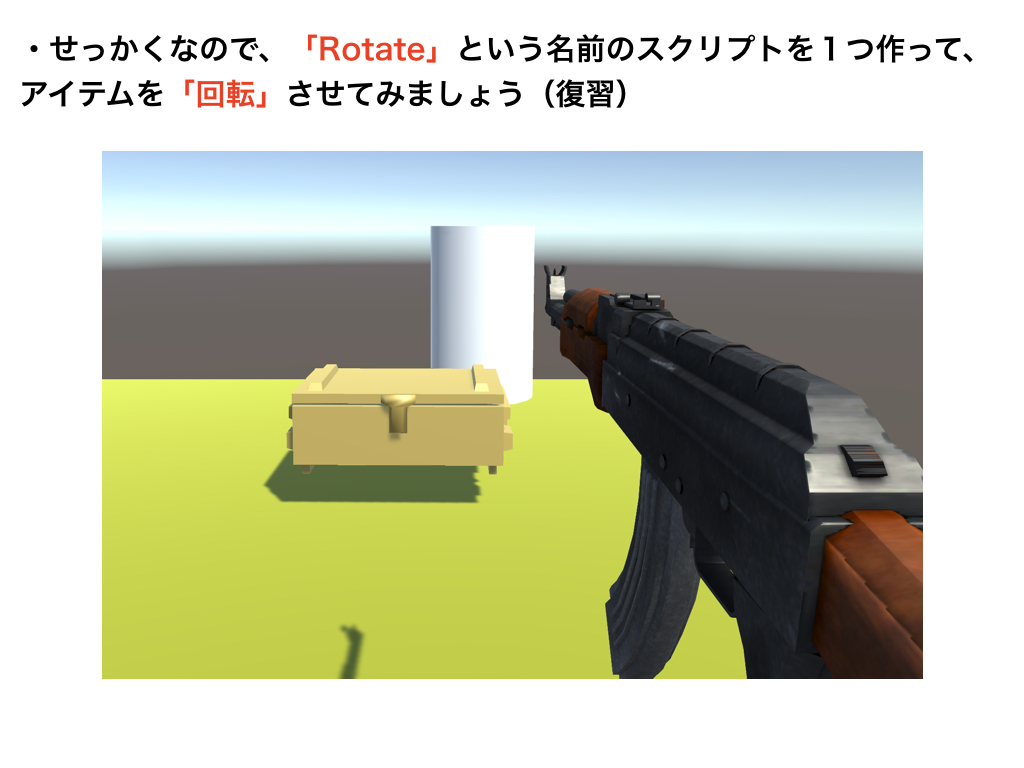
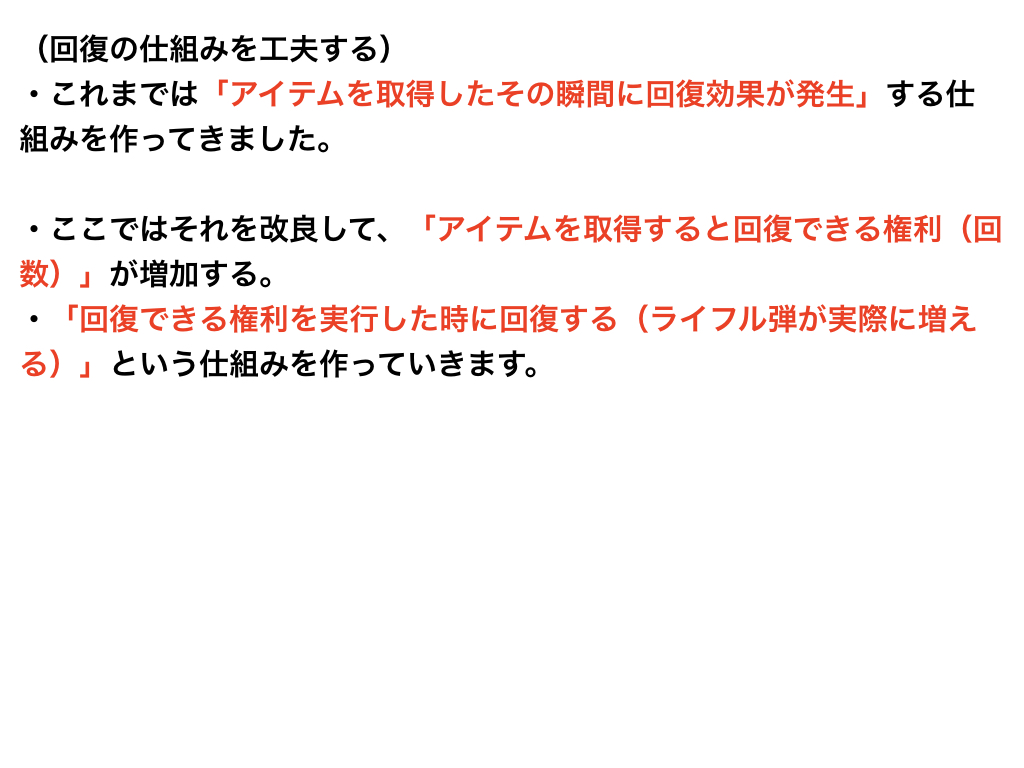
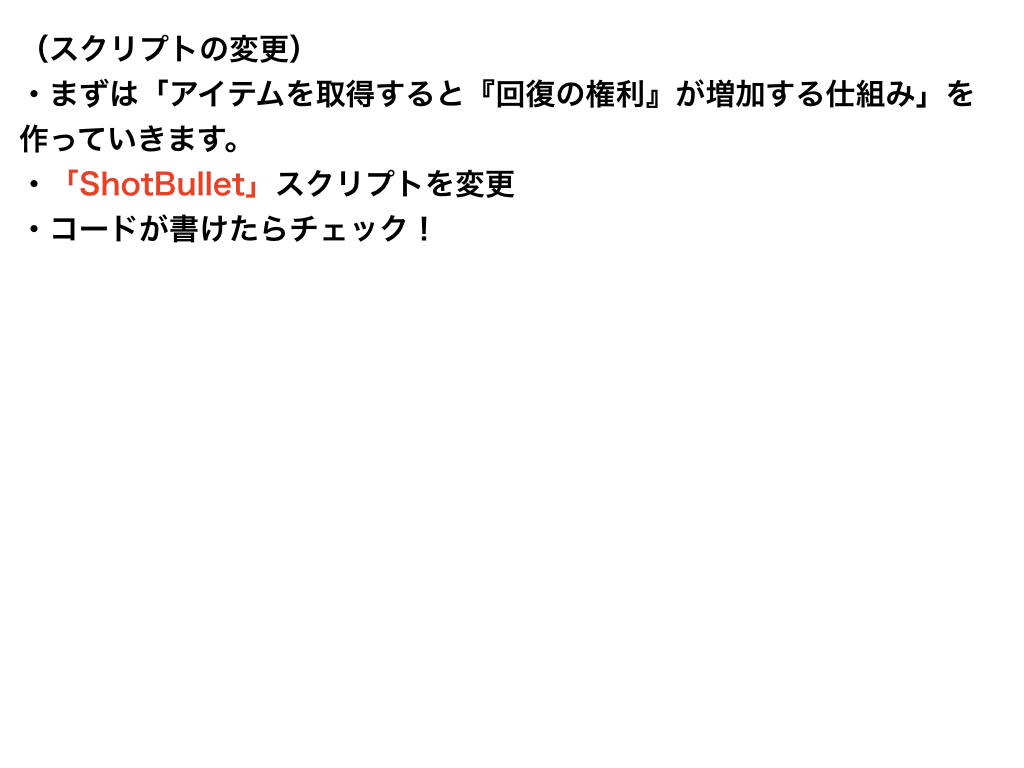
ライフル弾の回復の権利
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShotBullet : MonoBehaviour {
public GameObject bulletPrefab;
public AudioClip shotSound;
public float shotSpeed;
public static int shotBulletCount = 10;
// ★追加
// 回復の権利の箱
public static int bulletItemCount = 0;
void Update () {
if (Input.GetKeyDown (KeyCode.Space)) {
if (shotBulletCount < 1) {
return;
}
shotBulletCount -= 1;
GameObject bullet = (GameObject)Instantiate (bulletPrefab, transform.position, Quaternion.Euler (transform.parent.eulerAngles.x, transform.parent.eulerAngles.y, 0));
Rigidbody bulletRb = bullet.GetComponent<Rigidbody> ();
bulletRb.AddForce (transform.forward * shotSpeed);
AudioSource.PlayClipAtPoint (shotSound, Camera.main.transform.position);
Destroy (bullet, 2.0f);
}
}
// ★追加
public void AddBulletItem(int amount){
bulletItemCount += amount;
print ("ライフル弾の回復の権利" + bulletItemCount + "回");
}
}
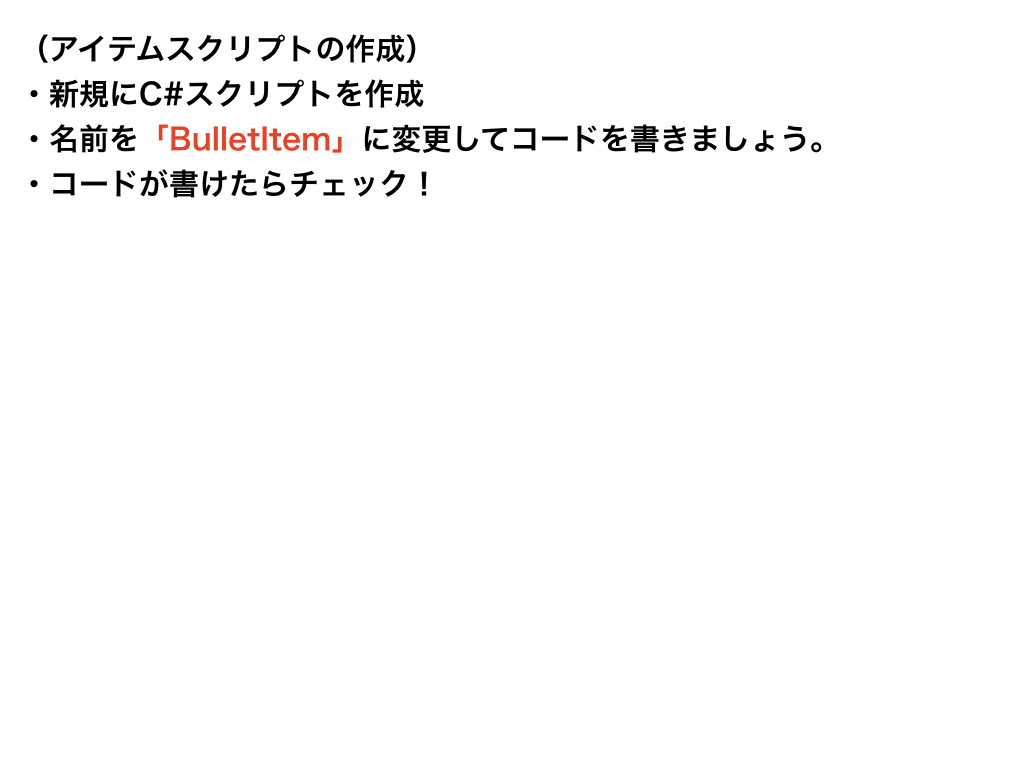
BulletItem
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BulletItem : MonoBehaviour {
public AudioClip getSound;
public AudioClip buzzerSound;
private GameObject sb;
void OnTriggerEnter(Collider other){
if (other.gameObject.tag == "Player") {
sb = GameObject.Find ("ShotBullet");
// (重要ポイント)
// 「!= null」の意味を確認しましょう。
// ShotBulletオブジェクトが非表示(オフ)ではない時、つまり「オン」の時
if (sb != null) {
sb.GetComponent<ShotBullet> ().AddBulletItem (1);
AudioSource.PlayClipAtPoint (getSound, Camera.main.transform.position);
Destroy (gameObject);
} else {
// ShotBulletオブジェクトが非表示(オフ)の時は警告音を鳴らす。
AudioSource.PlayClipAtPoint (buzzerSound, Camera.main.transform.position);
}
}
}
}
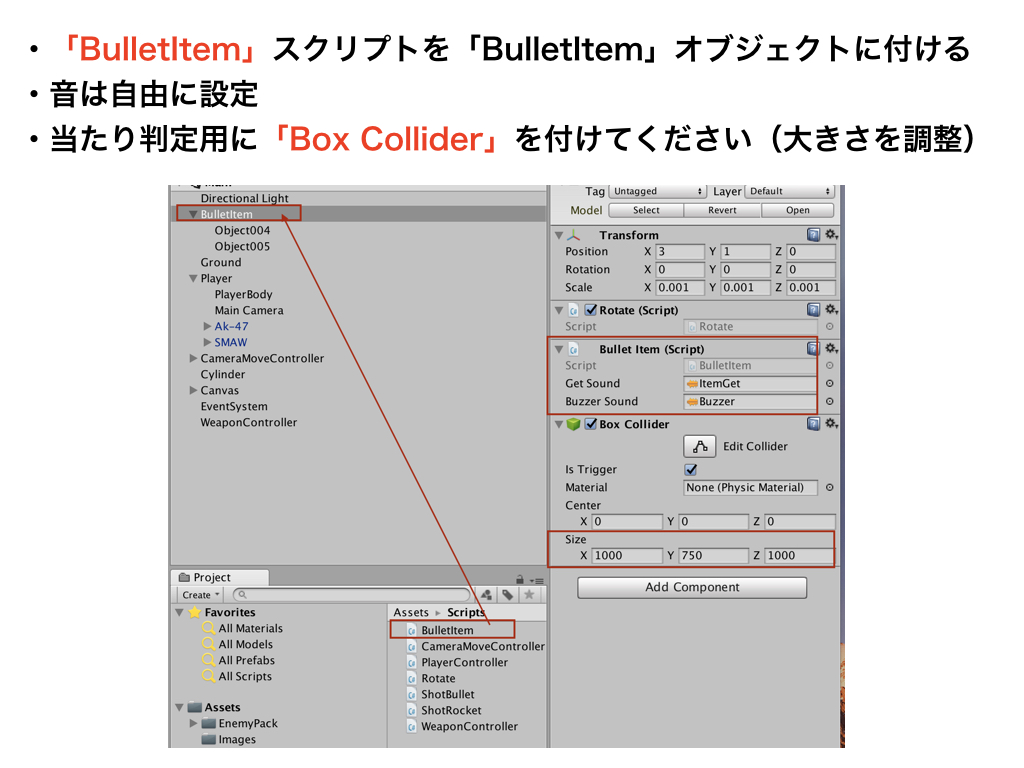
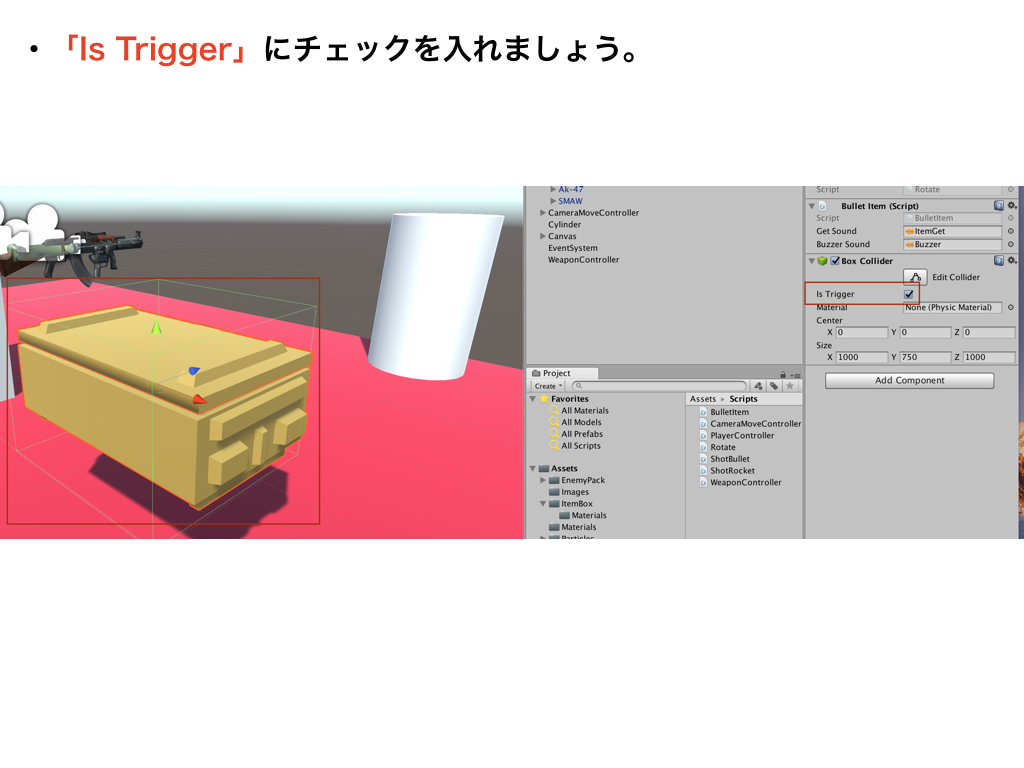
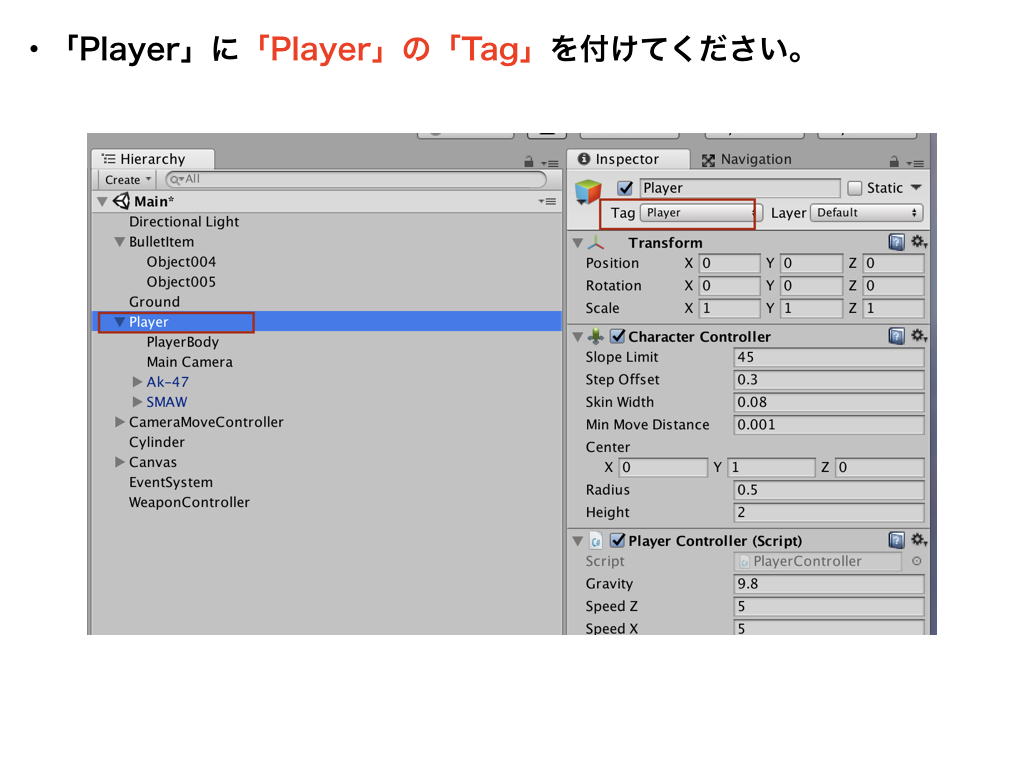
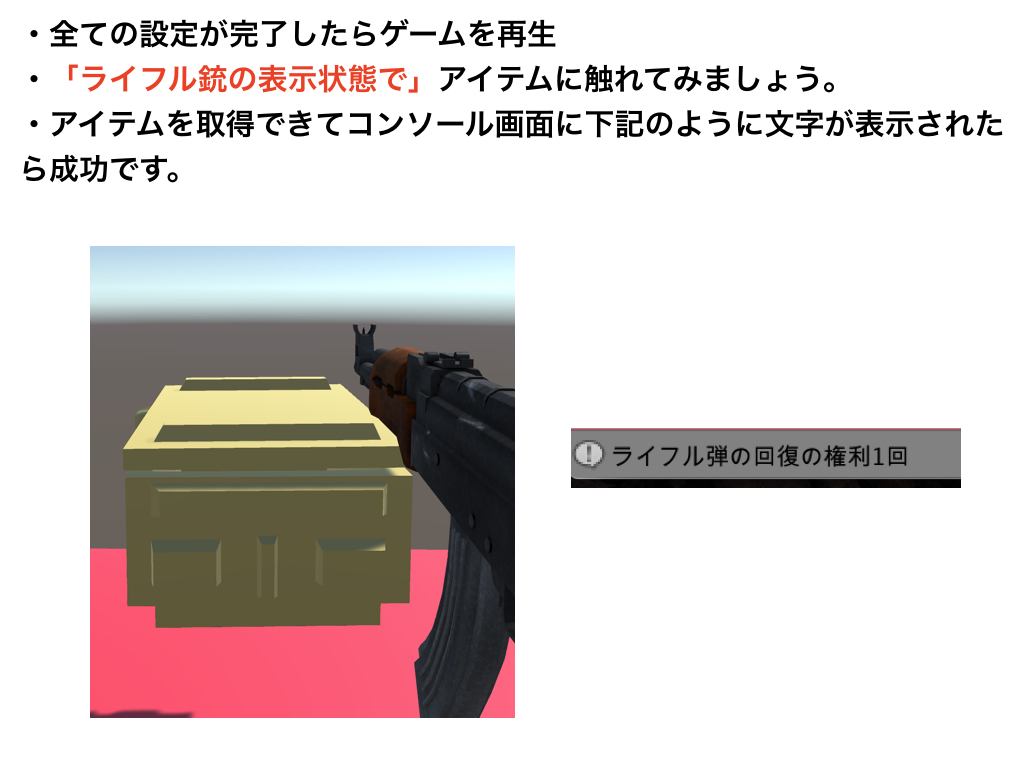
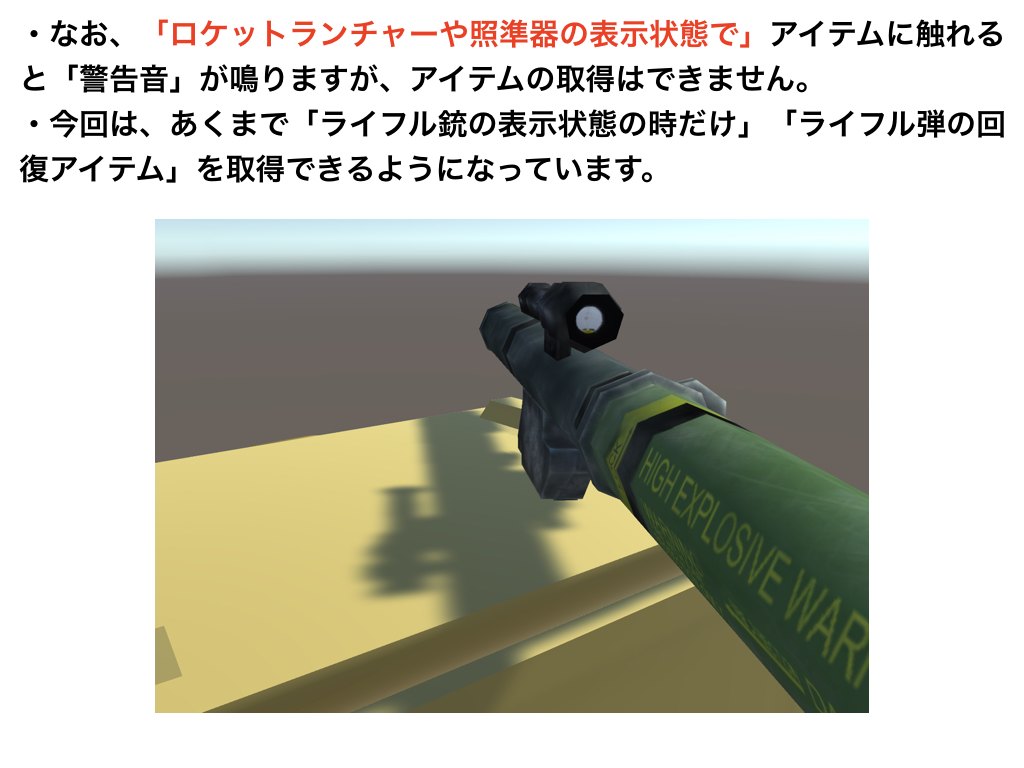
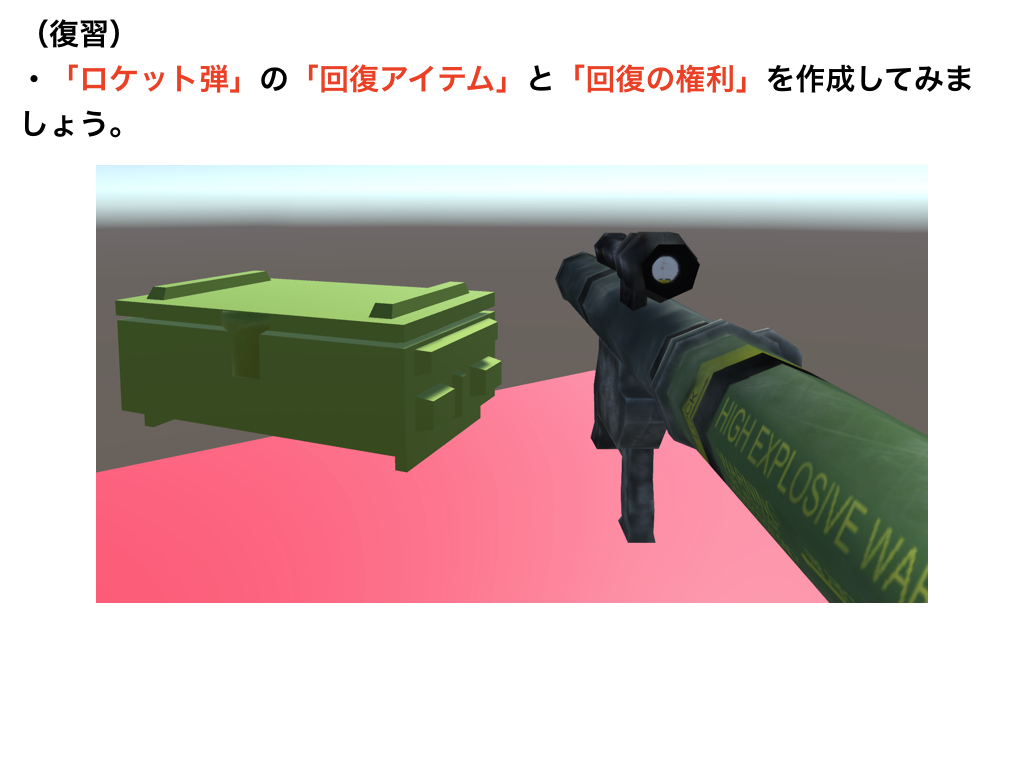
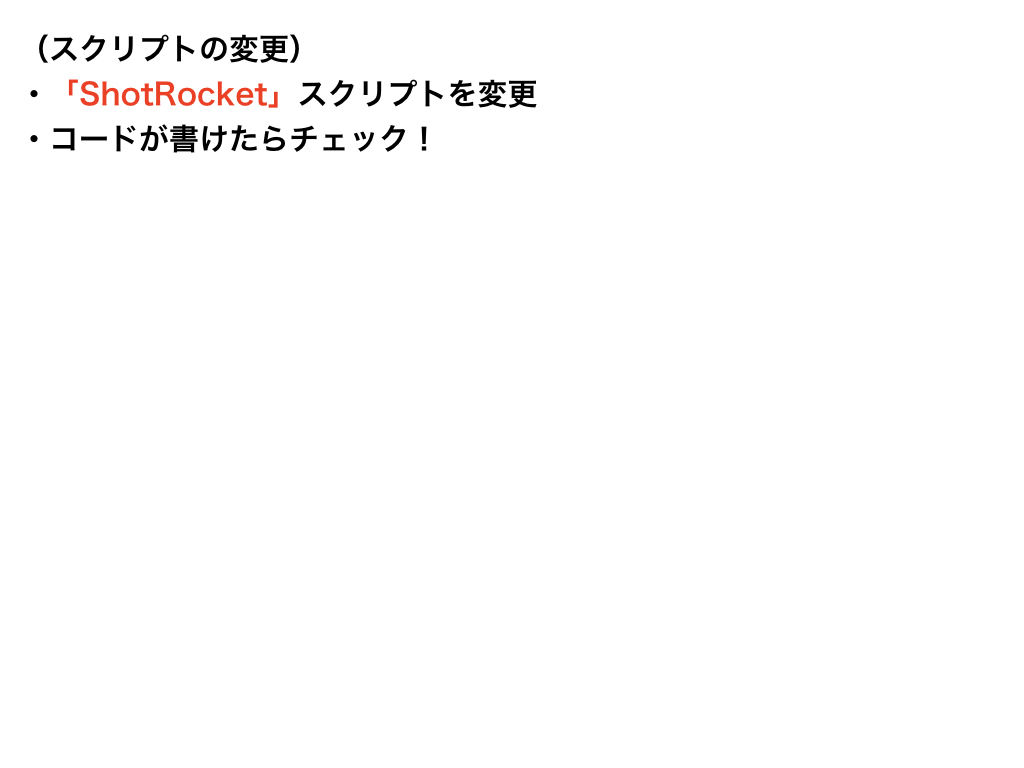
ロケット弾の回復の権利
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShotRocket : MonoBehaviour {
public GameObject rocketPrefab;
public AudioClip shotSound;
public float rocketSpeed;
private float timeBetweenShot = 1.2f;
private float timer;
public static int shotRocketCount = 10;
// ★追加
// 回復の権利の箱
public static int RocketItemCount = 0;
void Update () {
timer += Time.deltaTime;
if (Input.GetKeyDown (KeyCode.Space) && timer > timeBetweenShot) {
if (shotRocketCount < 1) {
return;
}
shotRocketCount -= 1;
timer = 0.0f;
GameObject rocket = (GameObject)Instantiate (rocketPrefab, transform.position, Quaternion.Euler (transform.parent.eulerAngles.x, transform.parent.eulerAngles.y, 0));
Rigidbody rocketRb = rocket.GetComponent<Rigidbody> ();
rocketRb.AddForce (transform.forward * rocketSpeed);
AudioSource.PlayClipAtPoint (shotSound, Camera.main.transform.position);
Destroy (rocket, 3.0f);
}
}
// ★追加
public void AddRocketItem(int amount){
RocketItemCount += amount;
print ("ロケット弾の回復の権利" + RocketItemCount + "回");
}
}
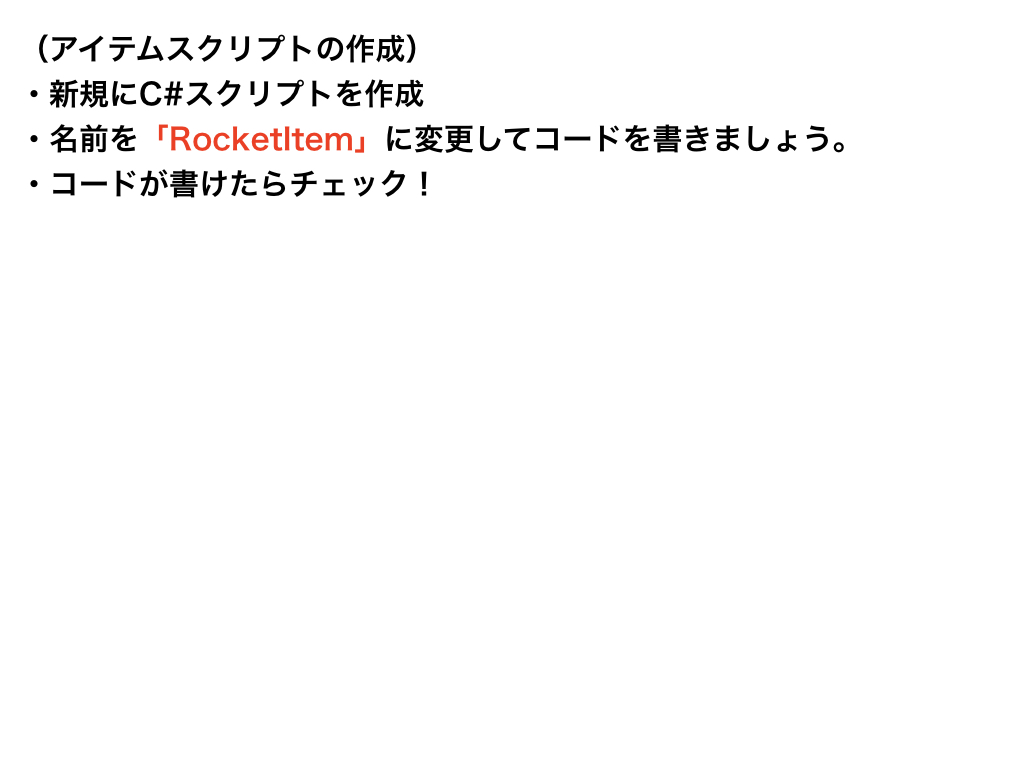
RocketItem
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RocketItem : MonoBehaviour {
public AudioClip getSound;
public AudioClip buzzerSound;
private GameObject sr;
void OnTriggerEnter(Collider other){
if (other.gameObject.tag == "Player") {
sr = GameObject.Find ("ShotRocket");
// (重要ポイント)
// 「!= null」の意味を確認しましょう。
// ShotRocketオブジェクトが非表示(オフ)ではない時、つまり「オン」の時
if (sr != null) {
sr.GetComponent<ShotRocket> ().AddRocketItem (1);
AudioSource.PlayClipAtPoint (getSound, Camera.main.transform.position);
Destroy (gameObject);
} else {
// ShotRocketオブジェクトが非表示(オフ)の時は警告音を鳴らす。
AudioSource.PlayClipAtPoint (buzzerSound, Camera.main.transform.position);
}
}
}
}
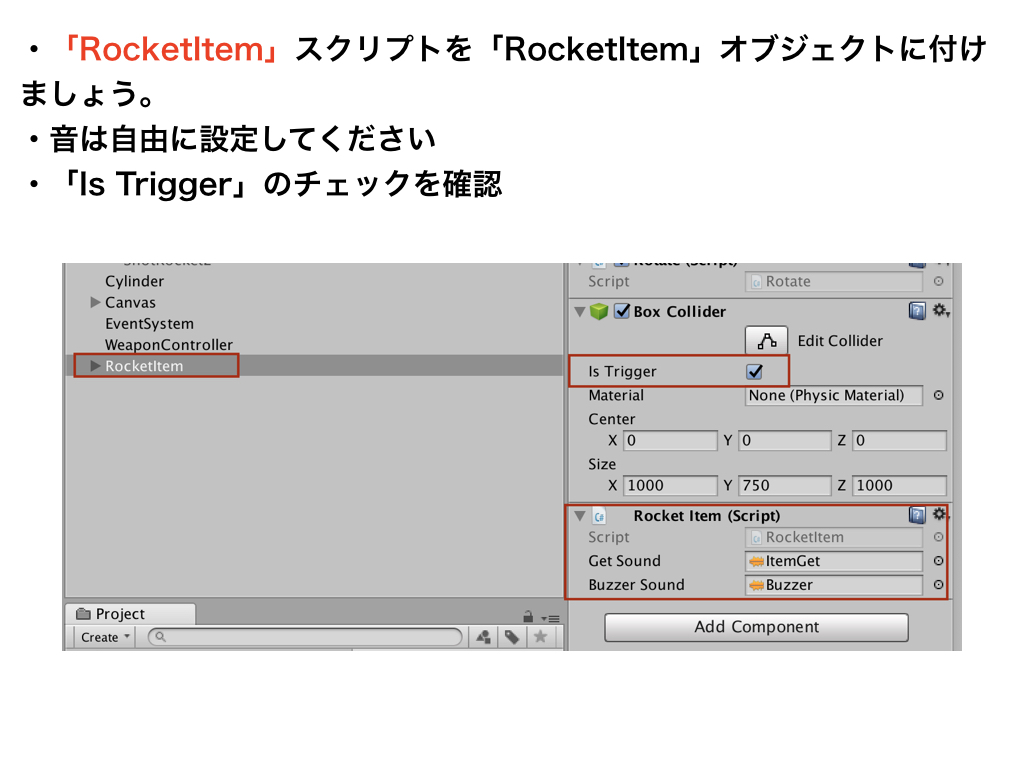
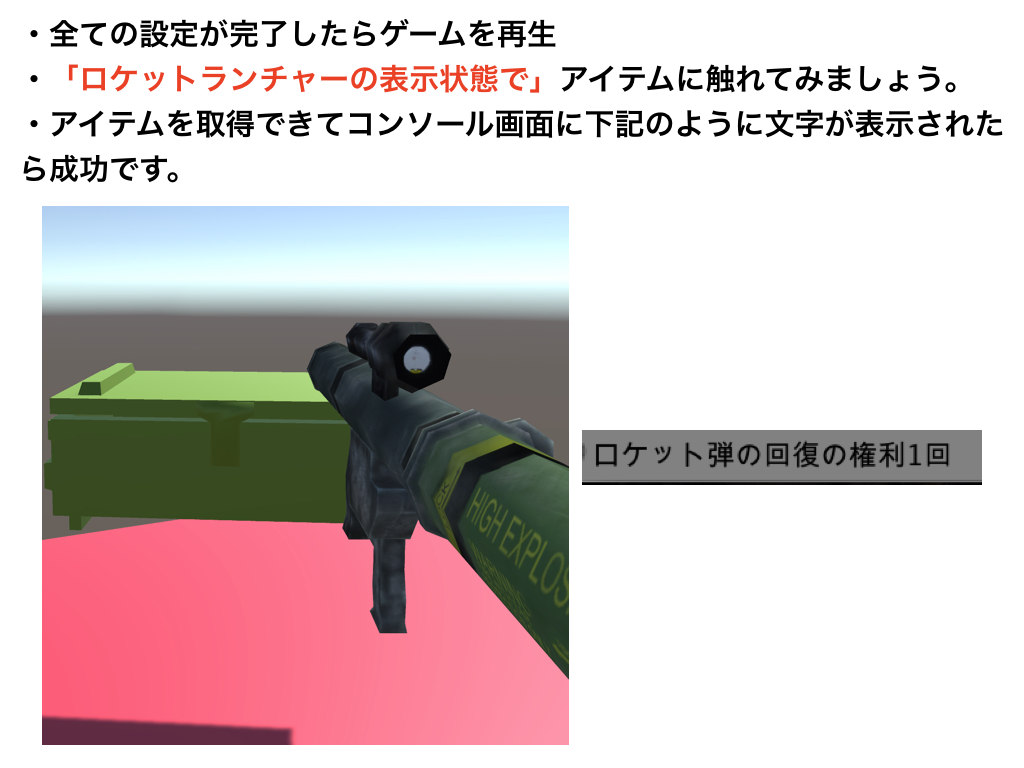
EscapeCombat(メモ)
他のコースを見る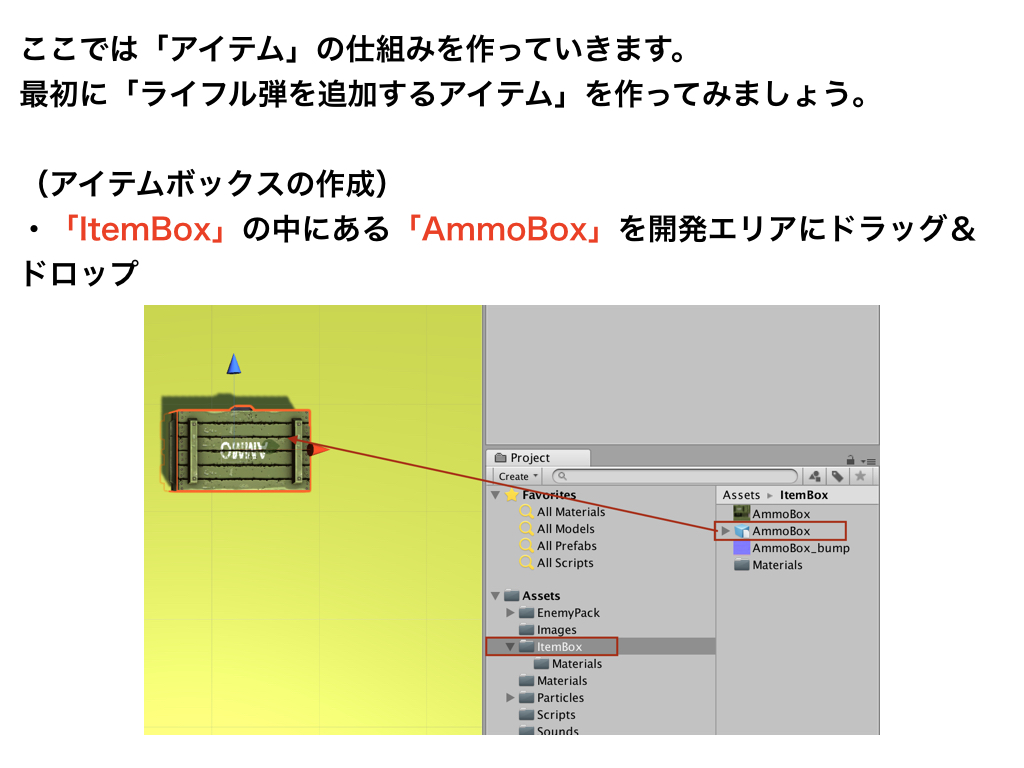
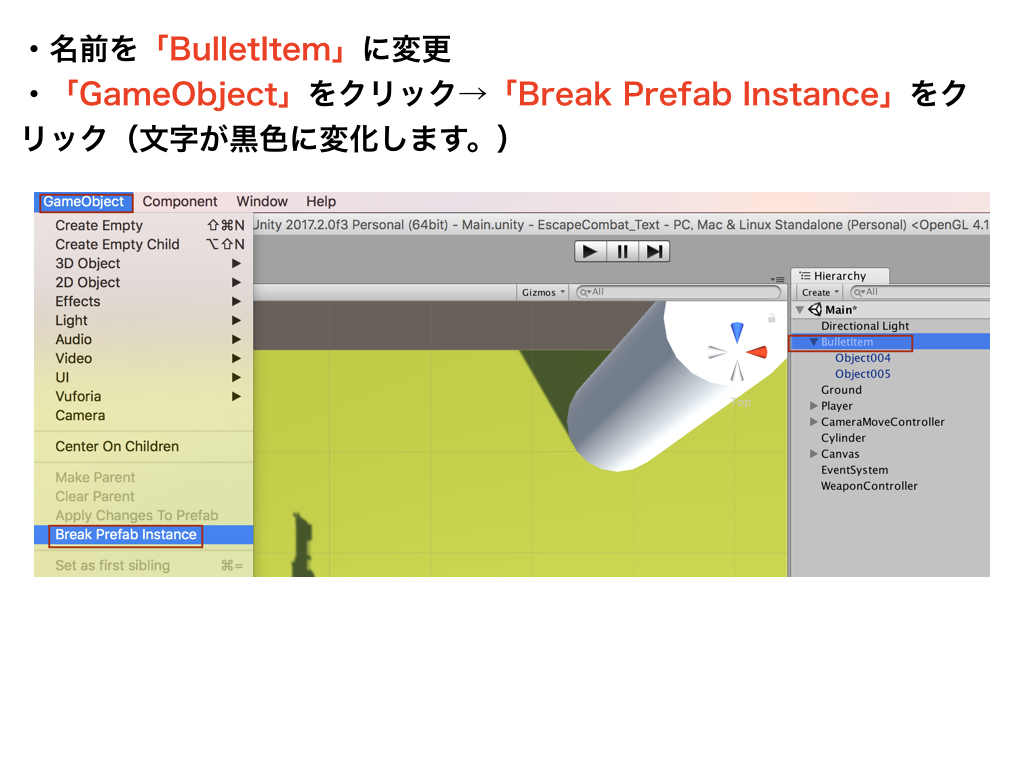
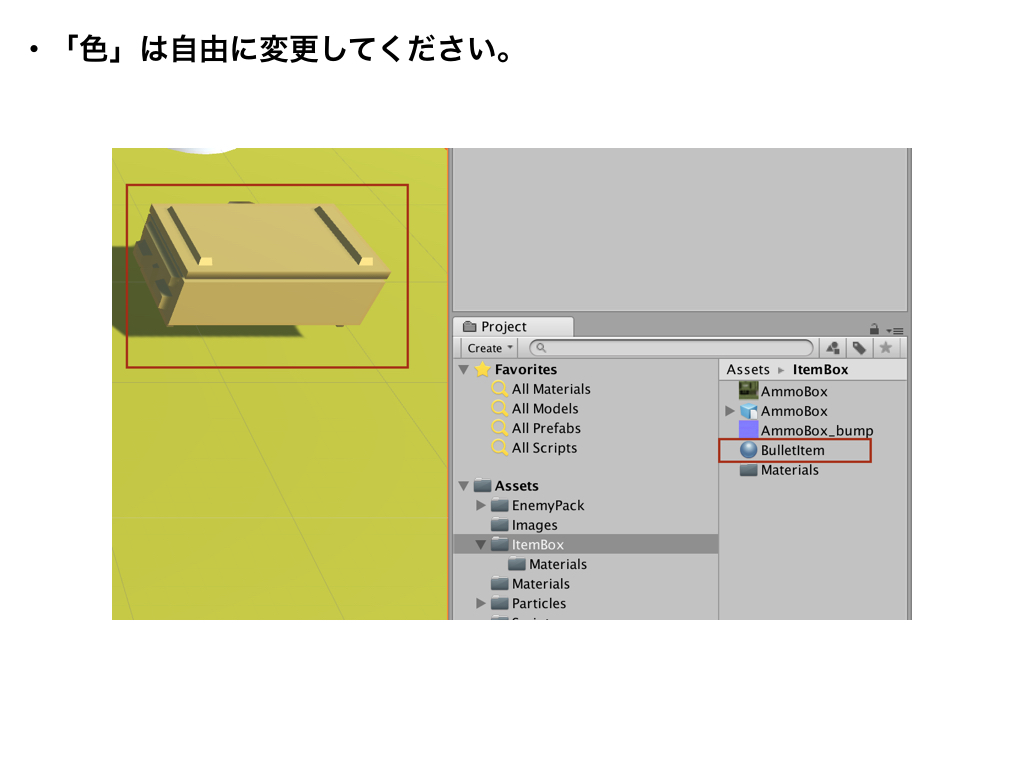
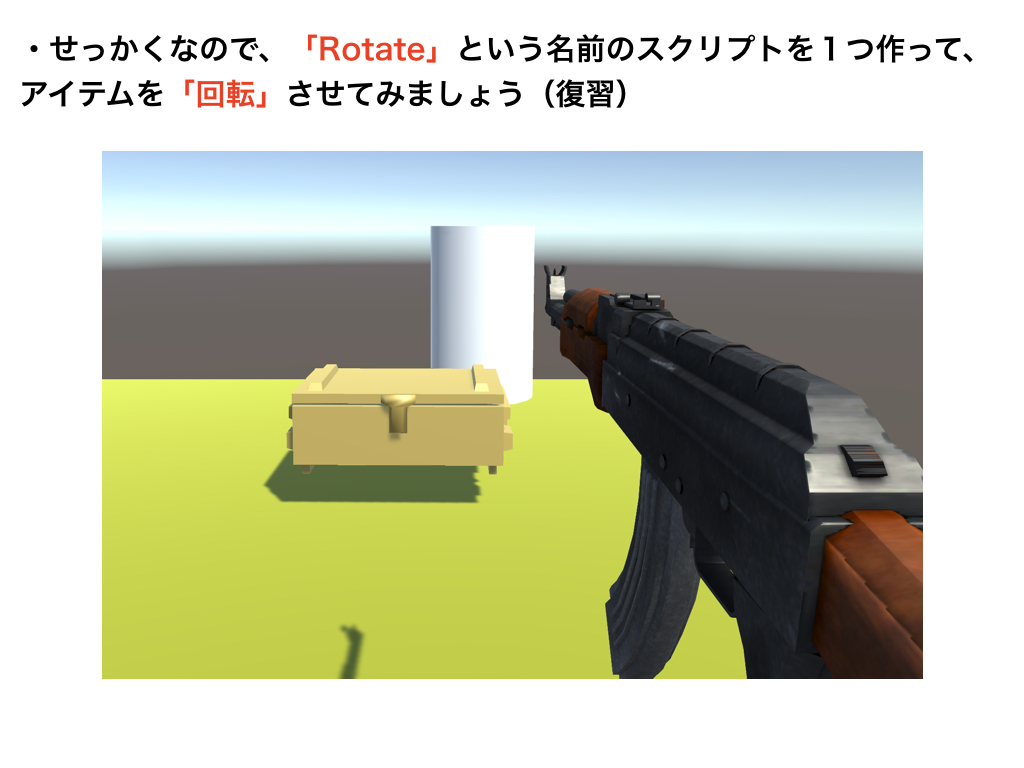
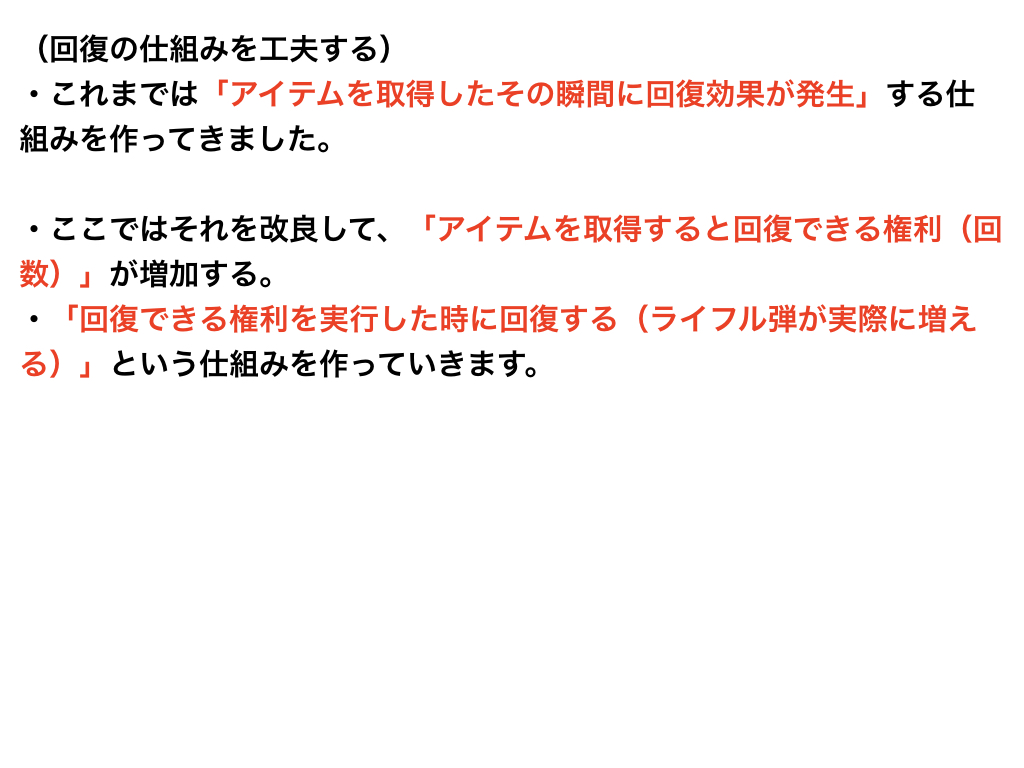
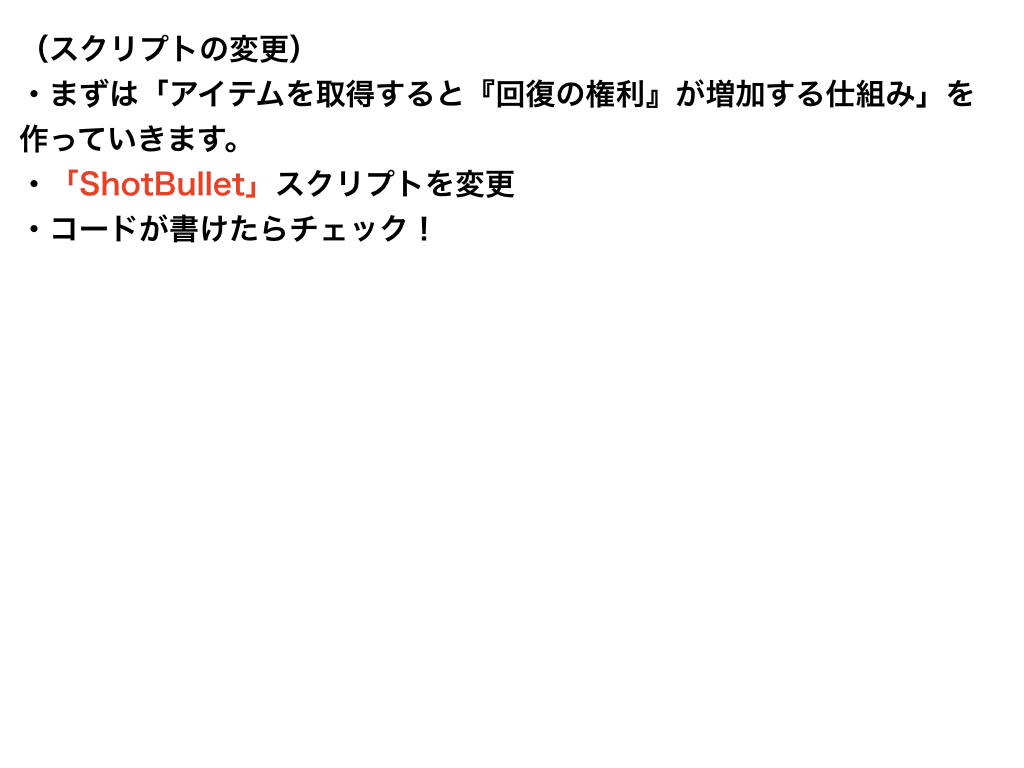
ライフル弾の回復の権利
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShotBullet : MonoBehaviour {
public GameObject bulletPrefab;
public AudioClip shotSound;
public float shotSpeed;
public static int shotBulletCount = 10;
// ★追加
// 回復の権利の箱
public static int bulletItemCount = 0;
void Update () {
if (Input.GetKeyDown (KeyCode.Space)) {
if (shotBulletCount < 1) {
return;
}
shotBulletCount -= 1;
GameObject bullet = (GameObject)Instantiate (bulletPrefab, transform.position, Quaternion.Euler (transform.parent.eulerAngles.x, transform.parent.eulerAngles.y, 0));
Rigidbody bulletRb = bullet.GetComponent<Rigidbody> ();
bulletRb.AddForce (transform.forward * shotSpeed);
AudioSource.PlayClipAtPoint (shotSound, Camera.main.transform.position);
Destroy (bullet, 2.0f);
}
}
// ★追加
public void AddBulletItem(int amount){
bulletItemCount += amount;
print ("ライフル弾の回復の権利" + bulletItemCount + "回");
}
}
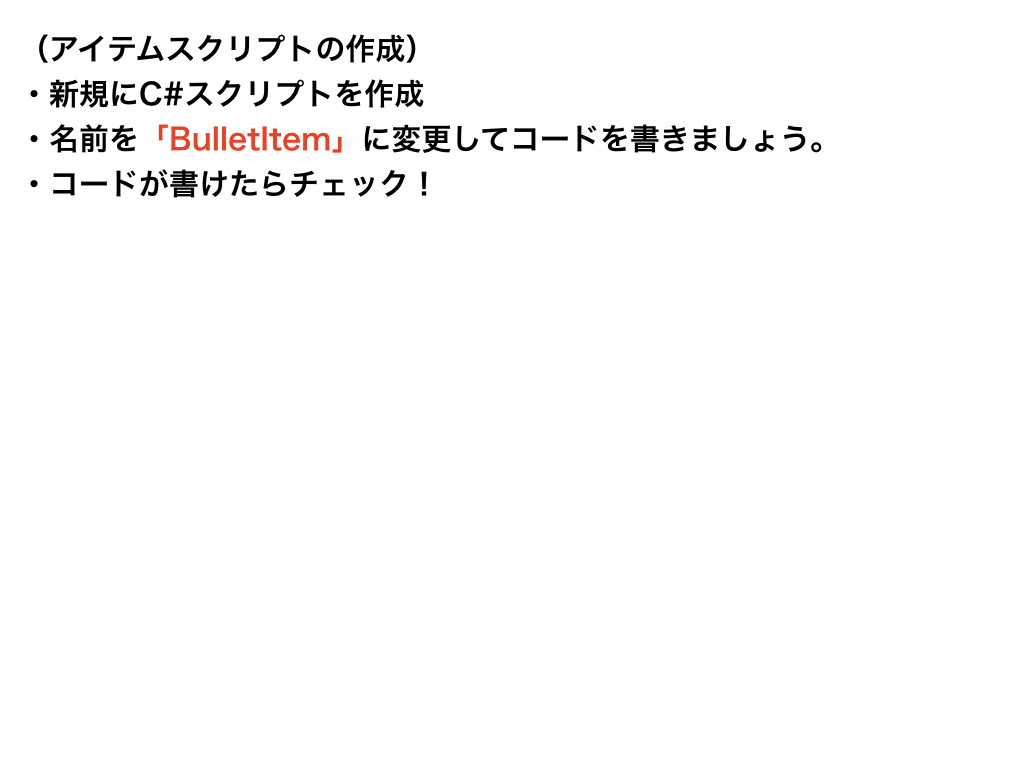
BulletItem
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BulletItem : MonoBehaviour {
public AudioClip getSound;
public AudioClip buzzerSound;
private GameObject sb;
void OnTriggerEnter(Collider other){
if (other.gameObject.tag == "Player") {
sb = GameObject.Find ("ShotBullet");
// (重要ポイント)
// 「!= null」の意味を確認しましょう。
// ShotBulletオブジェクトが非表示(オフ)ではない時、つまり「オン」の時
if (sb != null) {
sb.GetComponent<ShotBullet> ().AddBulletItem (1);
AudioSource.PlayClipAtPoint (getSound, Camera.main.transform.position);
Destroy (gameObject);
} else {
// ShotBulletオブジェクトが非表示(オフ)の時は警告音を鳴らす。
AudioSource.PlayClipAtPoint (buzzerSound, Camera.main.transform.position);
}
}
}
}
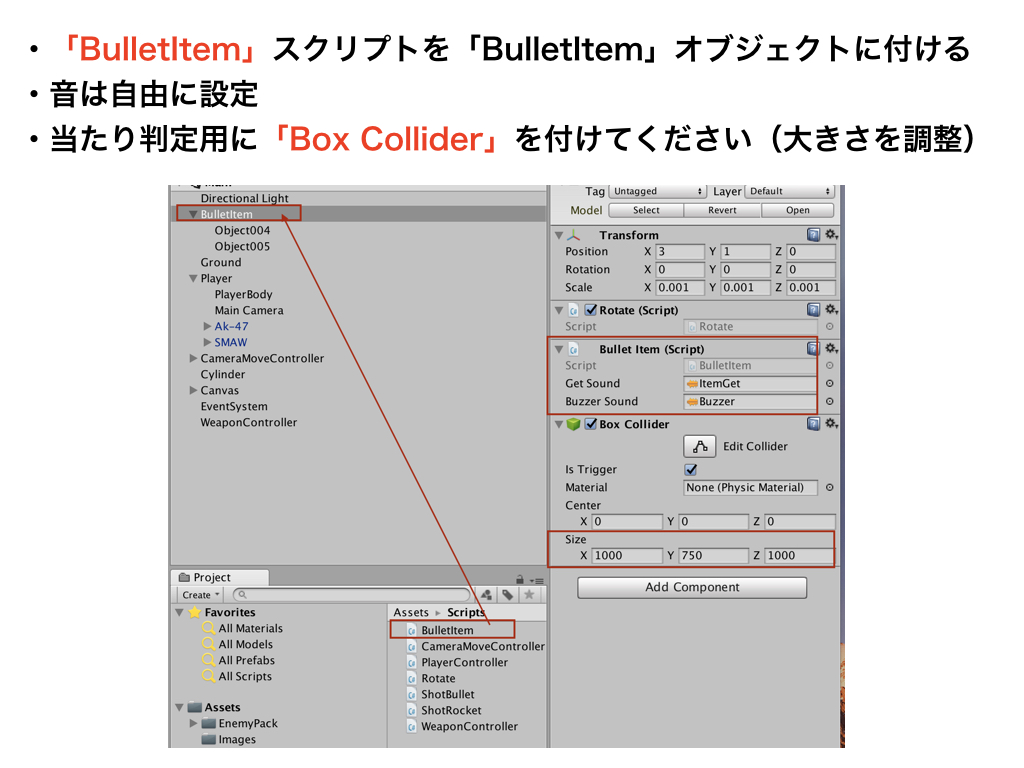
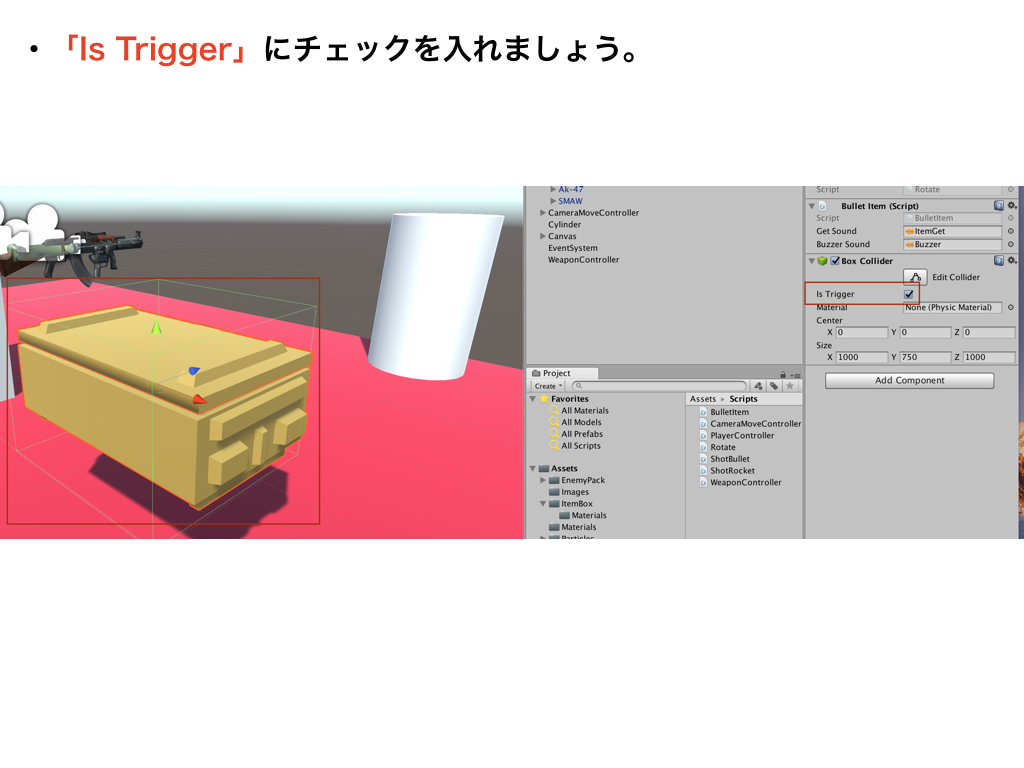
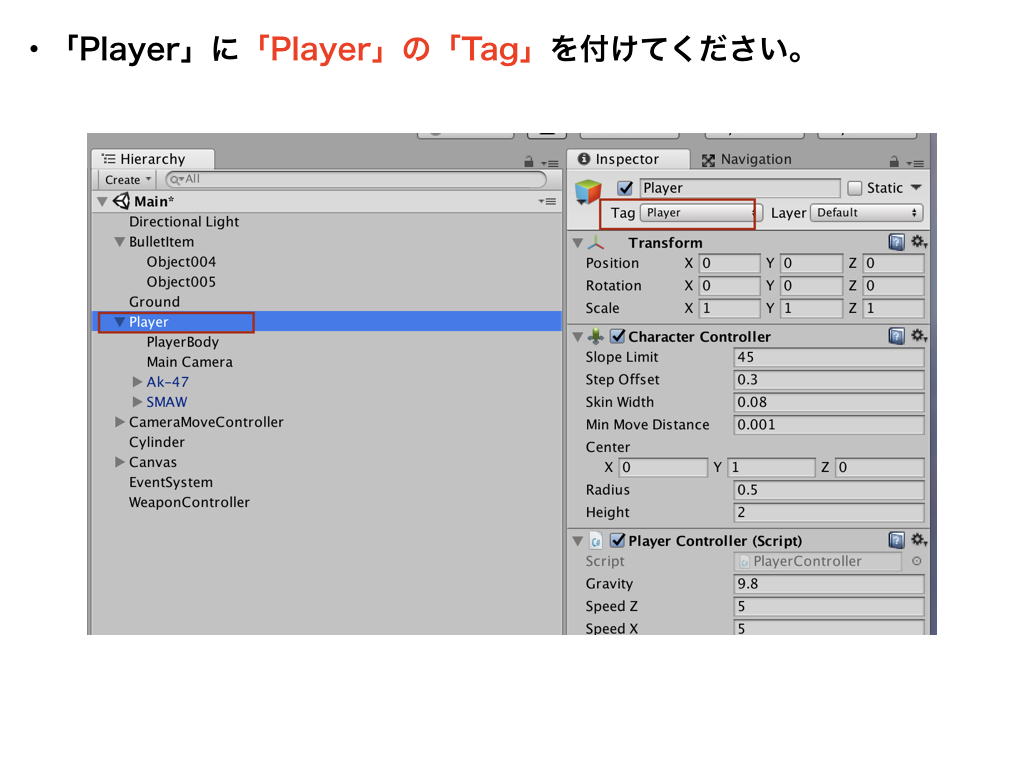
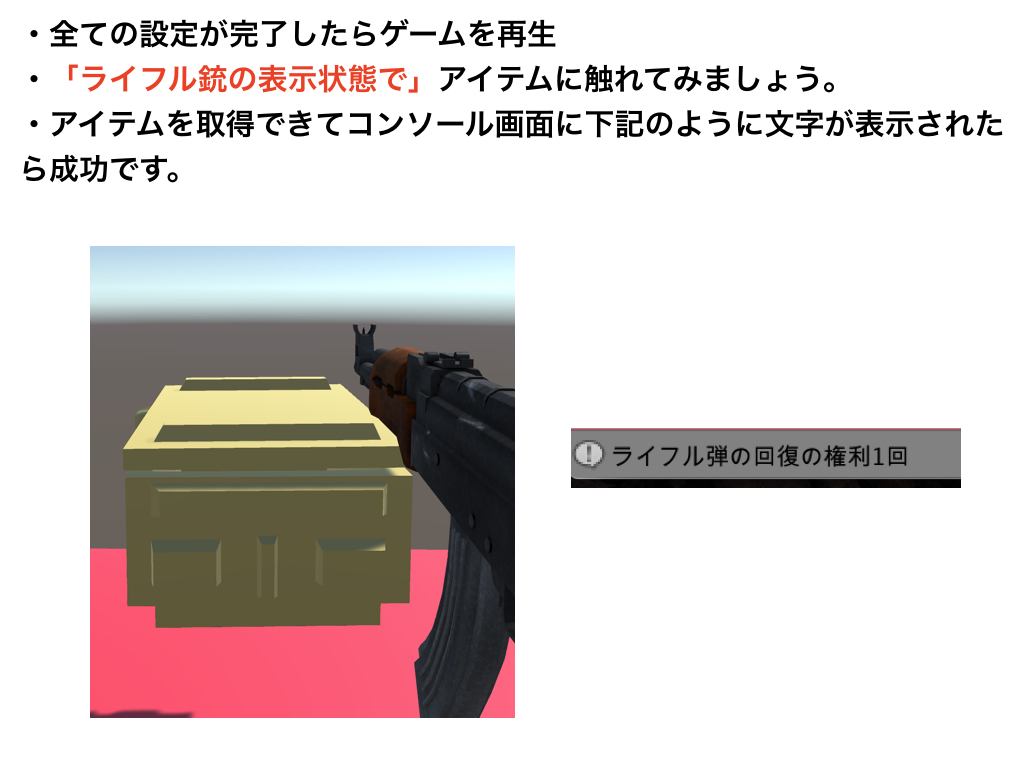
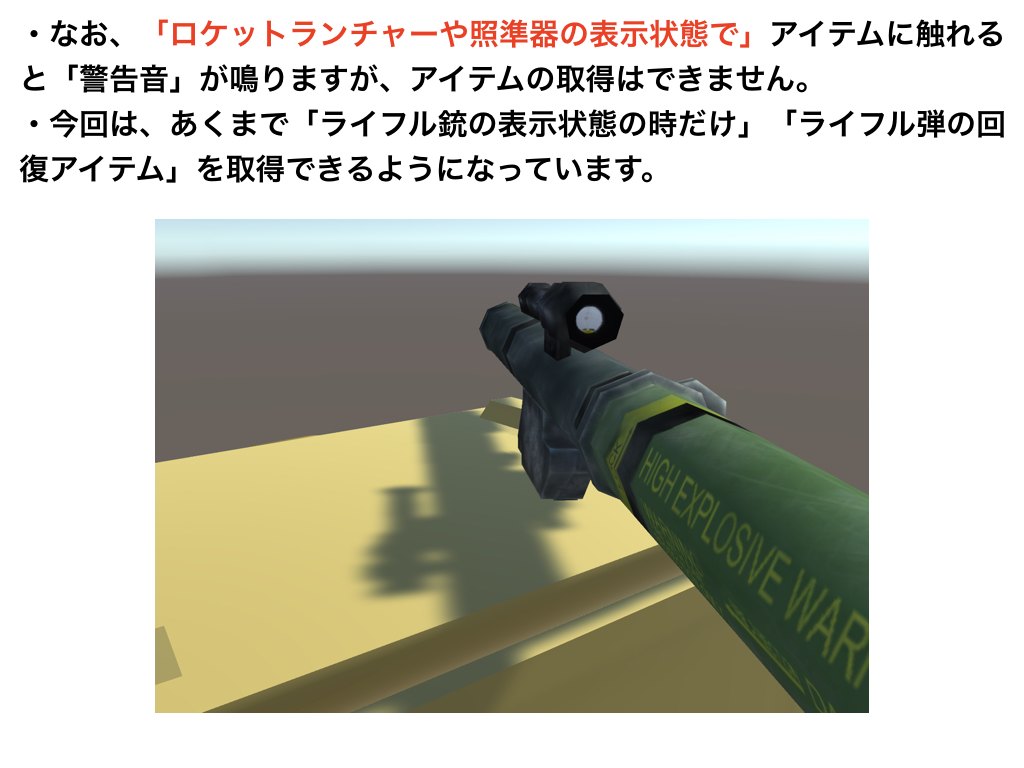
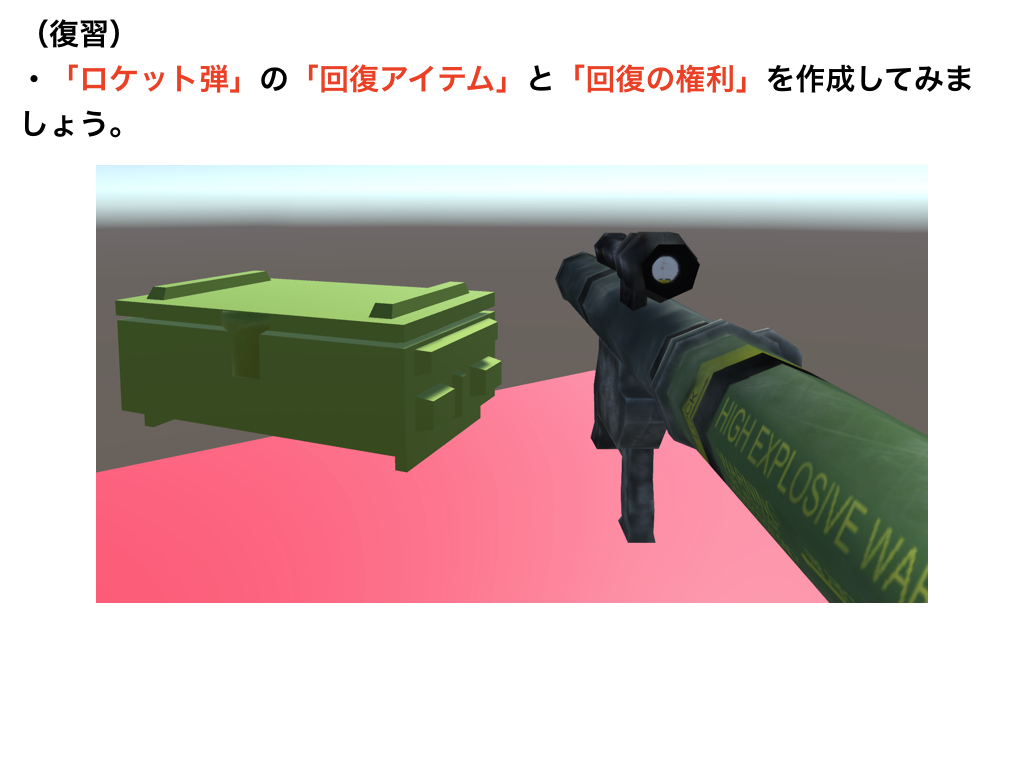
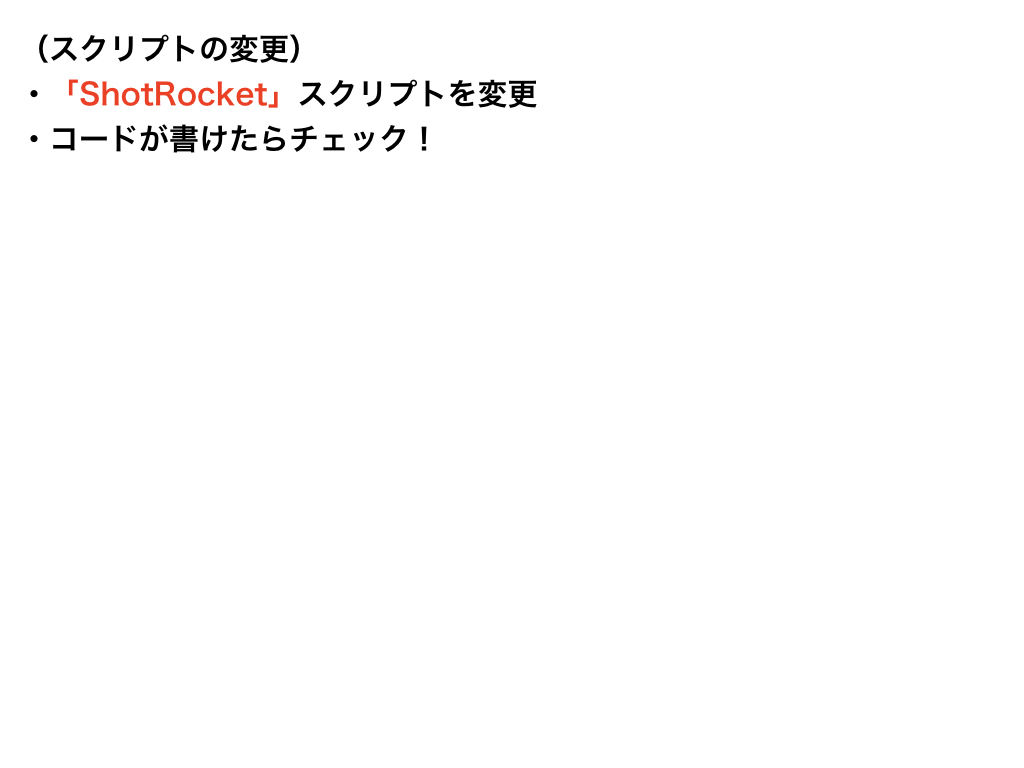
ロケット弾の回復の権利
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ShotRocket : MonoBehaviour {
public GameObject rocketPrefab;
public AudioClip shotSound;
public float rocketSpeed;
private float timeBetweenShot = 1.2f;
private float timer;
public static int shotRocketCount = 10;
// ★追加
// 回復の権利の箱
public static int RocketItemCount = 0;
void Update () {
timer += Time.deltaTime;
if (Input.GetKeyDown (KeyCode.Space) && timer > timeBetweenShot) {
if (shotRocketCount < 1) {
return;
}
shotRocketCount -= 1;
timer = 0.0f;
GameObject rocket = (GameObject)Instantiate (rocketPrefab, transform.position, Quaternion.Euler (transform.parent.eulerAngles.x, transform.parent.eulerAngles.y, 0));
Rigidbody rocketRb = rocket.GetComponent<Rigidbody> ();
rocketRb.AddForce (transform.forward * rocketSpeed);
AudioSource.PlayClipAtPoint (shotSound, Camera.main.transform.position);
Destroy (rocket, 3.0f);
}
}
// ★追加
public void AddRocketItem(int amount){
RocketItemCount += amount;
print ("ロケット弾の回復の権利" + RocketItemCount + "回");
}
}
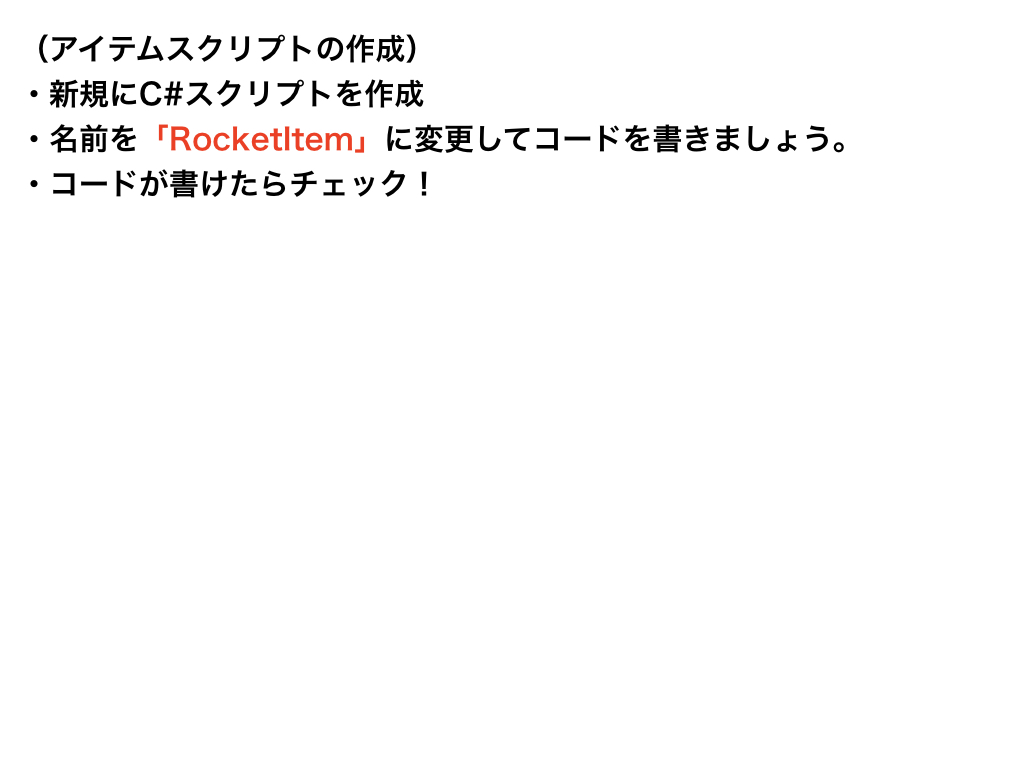
RocketItem
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RocketItem : MonoBehaviour {
public AudioClip getSound;
public AudioClip buzzerSound;
private GameObject sr;
void OnTriggerEnter(Collider other){
if (other.gameObject.tag == "Player") {
sr = GameObject.Find ("ShotRocket");
// (重要ポイント)
// 「!= null」の意味を確認しましょう。
// ShotRocketオブジェクトが非表示(オフ)ではない時、つまり「オン」の時
if (sr != null) {
sr.GetComponent<ShotRocket> ().AddRocketItem (1);
AudioSource.PlayClipAtPoint (getSound, Camera.main.transform.position);
Destroy (gameObject);
} else {
// ShotRocketオブジェクトが非表示(オフ)の時は警告音を鳴らす。
AudioSource.PlayClipAtPoint (buzzerSound, Camera.main.transform.position);
}
}
}
}
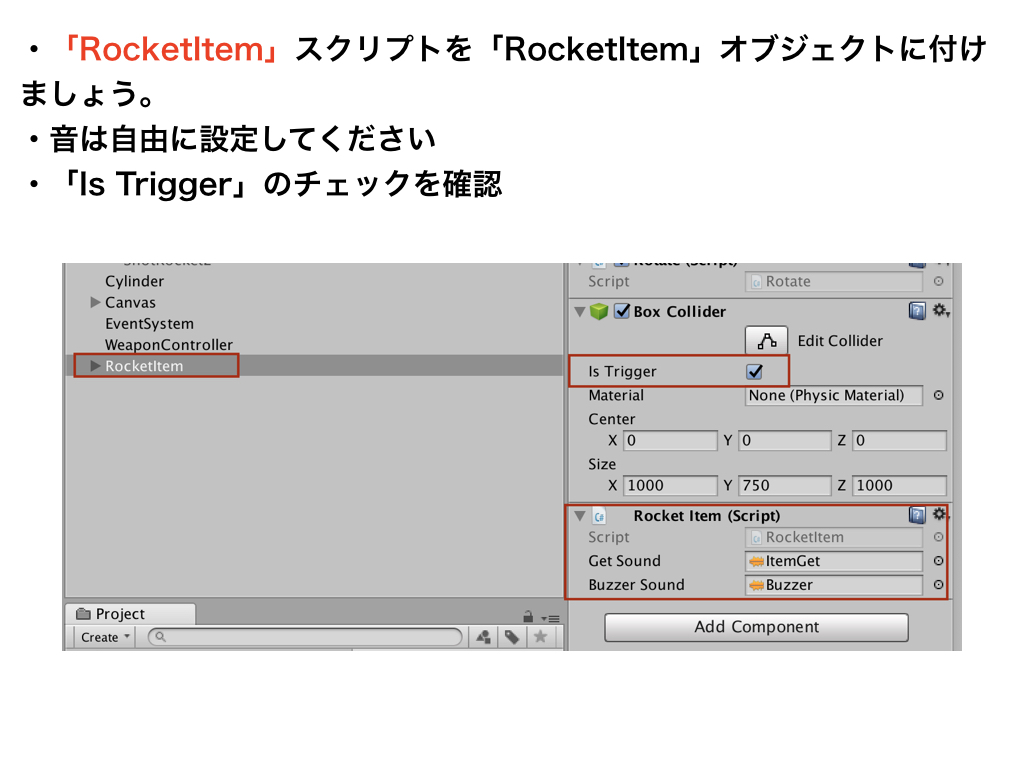
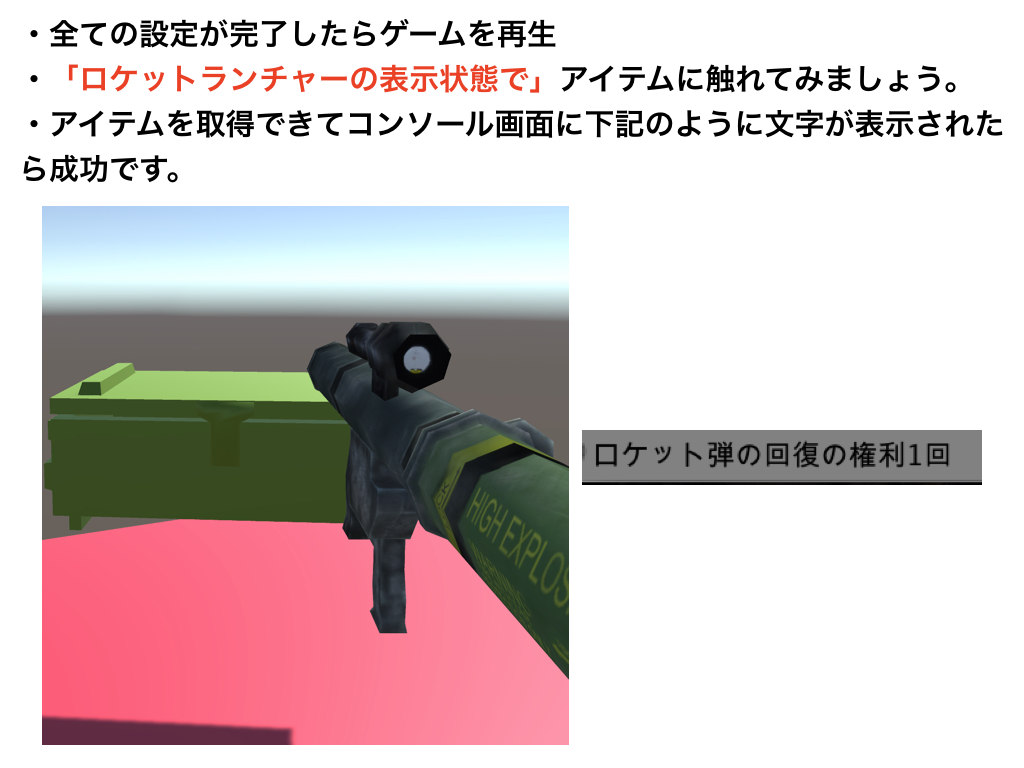
残弾数を回復させる「権利」の作成