【コルーチン】弾切れの発生③(発射パワーを回復させる)
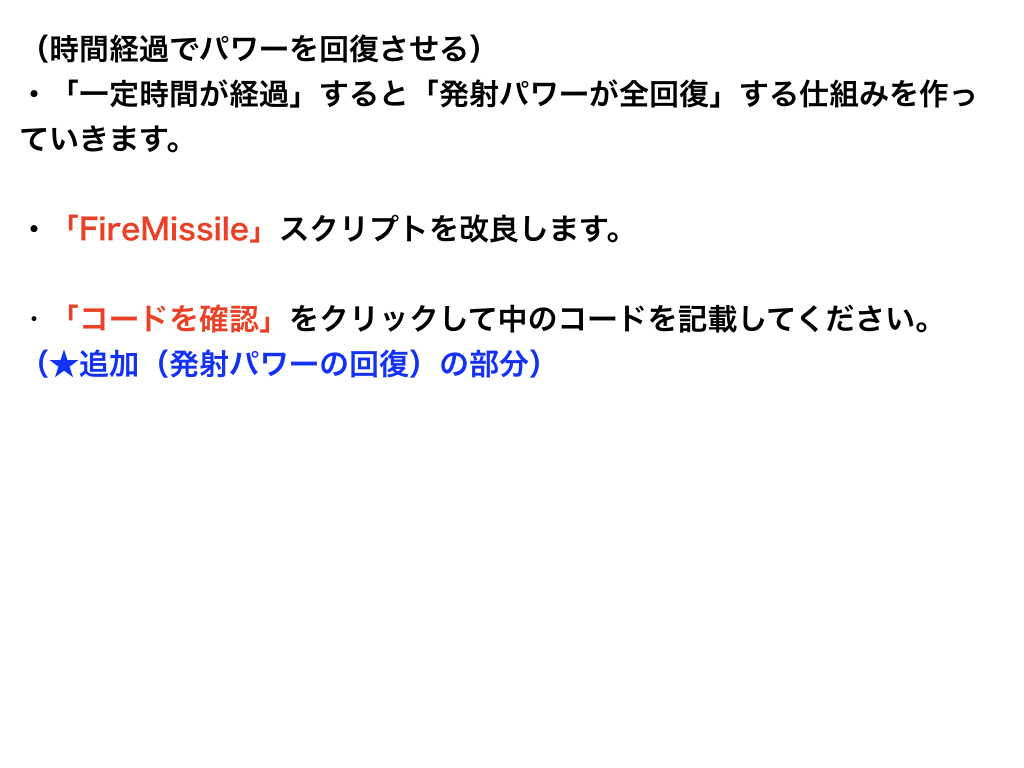
発射パワーの全回復(コルーチン活用)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class FireMissile : MonoBehaviour
{
public GameObject missilePrefab;
public float missileSpeed;
public AudioClip fireSound;
private int timeCount;
private int maxPower = 100;
private int shotPower;
private Slider powerSlider;
// ★追加(発射パワーの回復)
const int RecoveryTime = 10; // 発射パワーが回復するまでに要する時間(定数)
private int counter; // 発射パワー回復までの残り時間
void Start()
{
shotPower = maxPower;
powerSlider = GameObject.Find("PowerSlider").GetComponent<Slider>();
powerSlider.maxValue = maxPower;
powerSlider.value = shotPower;
}
void Update()
{
// ★追加(発射パワーの回復)
if (shotPower <= 0 && counter <= 0)
{ // 条件の内容をおさえること(ポイント)
StartCoroutine(RecoverPower()); // コルーチンを作動させる
}
timeCount += 1;
if (Input.GetButton("Fire1"))
{
if (shotPower <= 0)
{
return;
}
shotPower -= 1;
powerSlider.value = shotPower;
if (timeCount % 5 == 0)
{
GameObject missile = Instantiate(missilePrefab, transform.position, Quaternion.identity);
Rigidbody missileRb = missile.GetComponent<Rigidbody>();
missileRb.AddForce(transform.forward * missileSpeed);
AudioSource.PlayClipAtPoint(fireSound, transform.position);
Destroy(missile, 2.0f);
}
}
}
// ★追加(発射パワーの回復)
// コルーチン
IEnumerator RecoverPower()
{
// パワー回復までに必要な時間をセット
counter = RecoveryTime;
// 1秒ずつカウントを進める
while (counter > 0)
{
yield return new WaitForSeconds(1.0f);
counter -= 1;
print("全回復までの残り時間" + counter + "秒");
}
// (ポイント)
// while内の処理が行われている間は、ここのコードは実行されない。
// whileの処理が終了=残り時間が0になった時にコードが実行される。
// 発射パワーをマックス状態にする。
shotPower = maxPower;
powerSlider.value = shotPower;
}
}
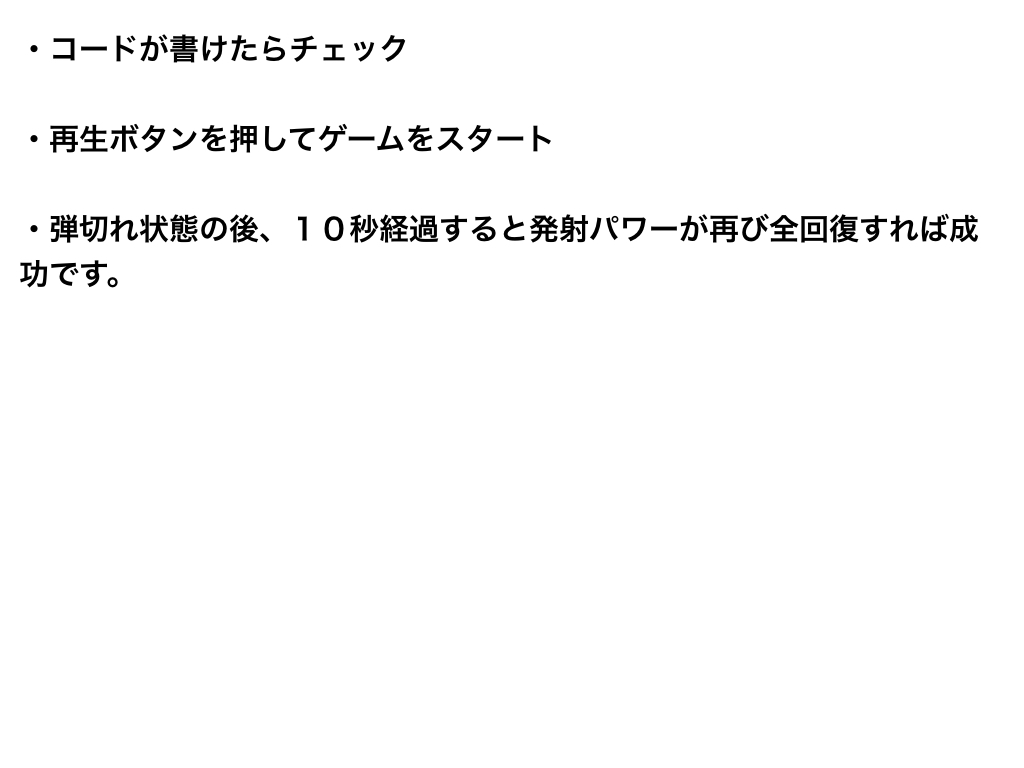
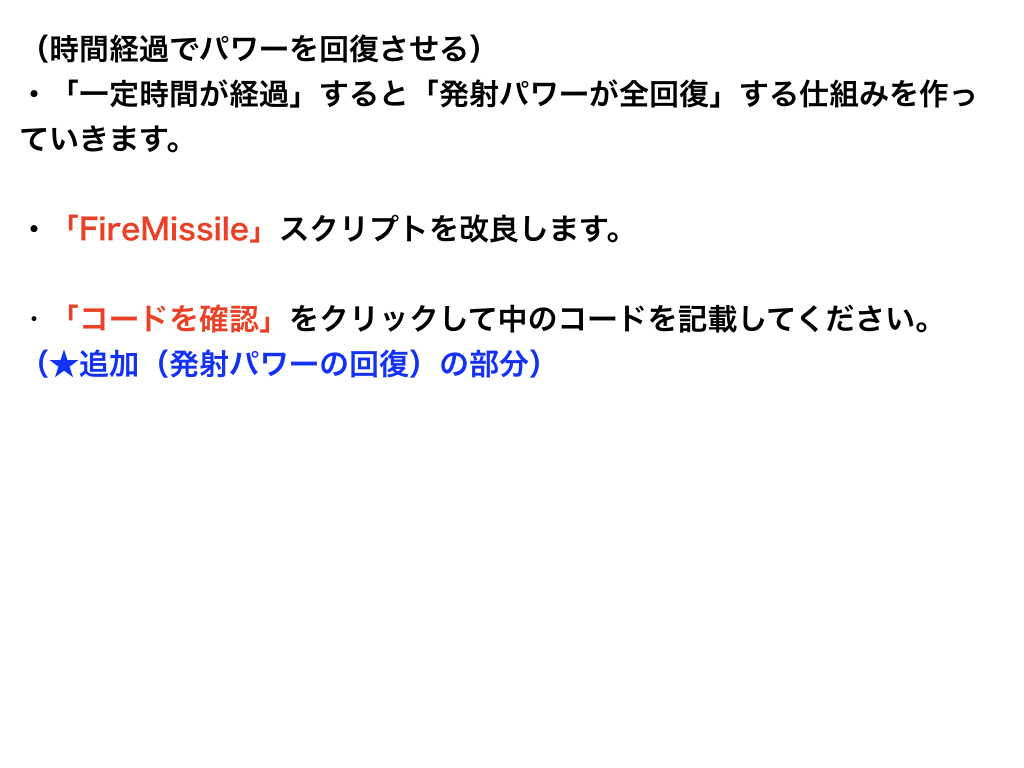
発射パワーの全回復(コルーチン活用)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class FireMissile : MonoBehaviour
{
public GameObject missilePrefab;
public float missileSpeed;
public AudioClip fireSound;
private int timeCount;
private int maxPower = 100;
private int shotPower;
private Slider powerSlider;
// ★追加(発射パワーの回復)
const int RecoveryTime = 10; // 発射パワーが回復するまでに要する時間(定数)
private int counter; // 発射パワー回復までの残り時間
void Start()
{
shotPower = maxPower;
powerSlider = GameObject.Find("PowerSlider").GetComponent<Slider>();
powerSlider.maxValue = maxPower;
powerSlider.value = shotPower;
}
void Update()
{
// ★追加(発射パワーの回復)
if (shotPower <= 0 && counter <= 0)
{ // 条件の内容をおさえること(ポイント)
StartCoroutine(RecoverPower()); // コルーチンを作動させる
}
timeCount += 1;
if (Input.GetButton("Fire1"))
{
if (shotPower <= 0)
{
return;
}
shotPower -= 1;
powerSlider.value = shotPower;
if (timeCount % 5 == 0)
{
GameObject missile = Instantiate(missilePrefab, transform.position, Quaternion.identity);
Rigidbody missileRb = missile.GetComponent<Rigidbody>();
missileRb.AddForce(transform.forward * missileSpeed);
AudioSource.PlayClipAtPoint(fireSound, transform.position);
Destroy(missile, 2.0f);
}
}
}
// ★追加(発射パワーの回復)
// コルーチン
IEnumerator RecoverPower()
{
// パワー回復までに必要な時間をセット
counter = RecoveryTime;
// 1秒ずつカウントを進める
while (counter > 0)
{
yield return new WaitForSeconds(1.0f);
counter -= 1;
print("全回復までの残り時間" + counter + "秒");
}
// (ポイント)
// while内の処理が行われている間は、ここのコードは実行されない。
// whileの処理が終了=残り時間が0になった時にコードが実行される。
// 発射パワーをマックス状態にする。
shotPower = maxPower;
powerSlider.value = shotPower;
}
}
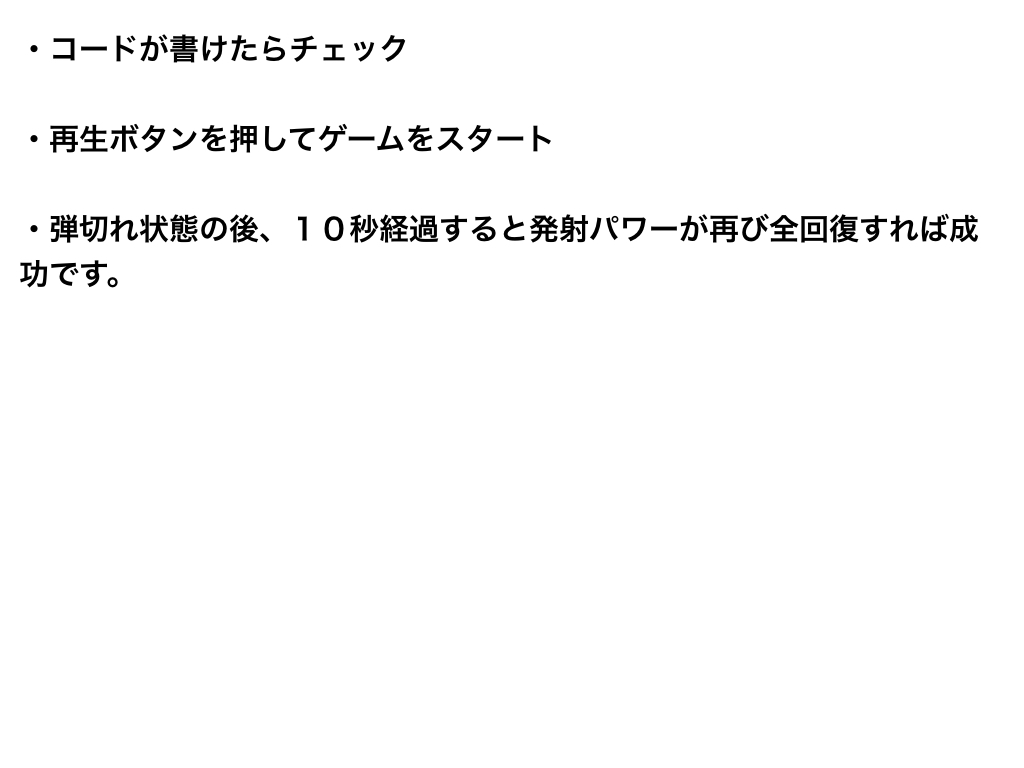
【コルーチン】弾切れの発生③(発射パワーを回復させる)