*ゲームの改良(オブジェクトプール)
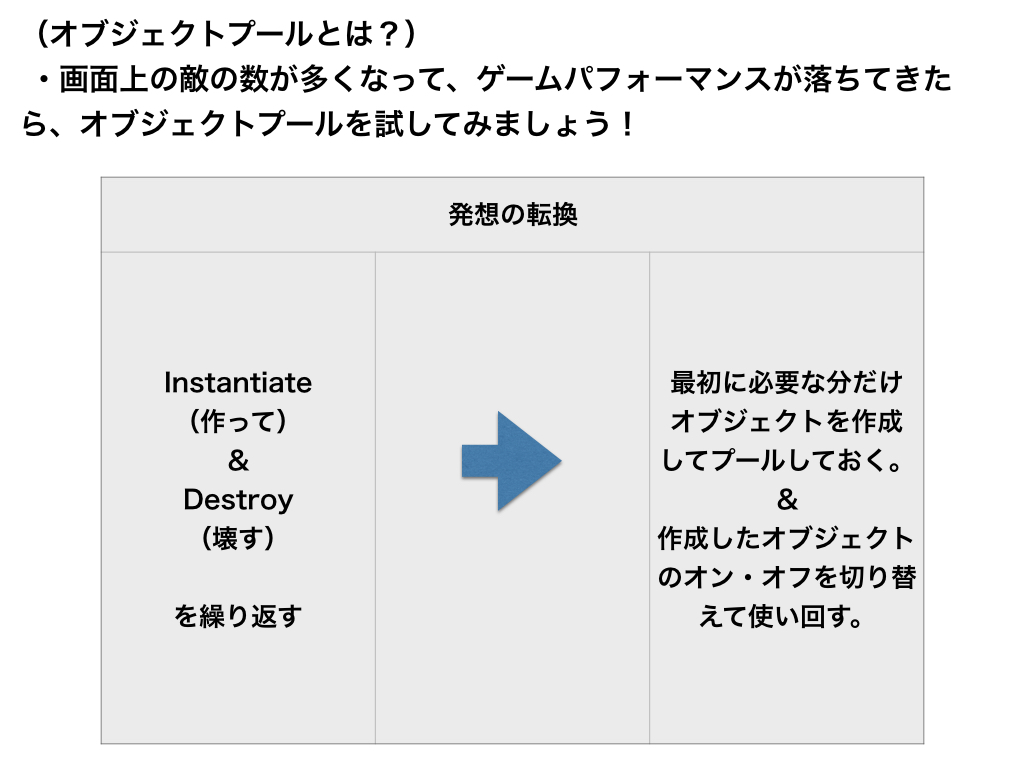
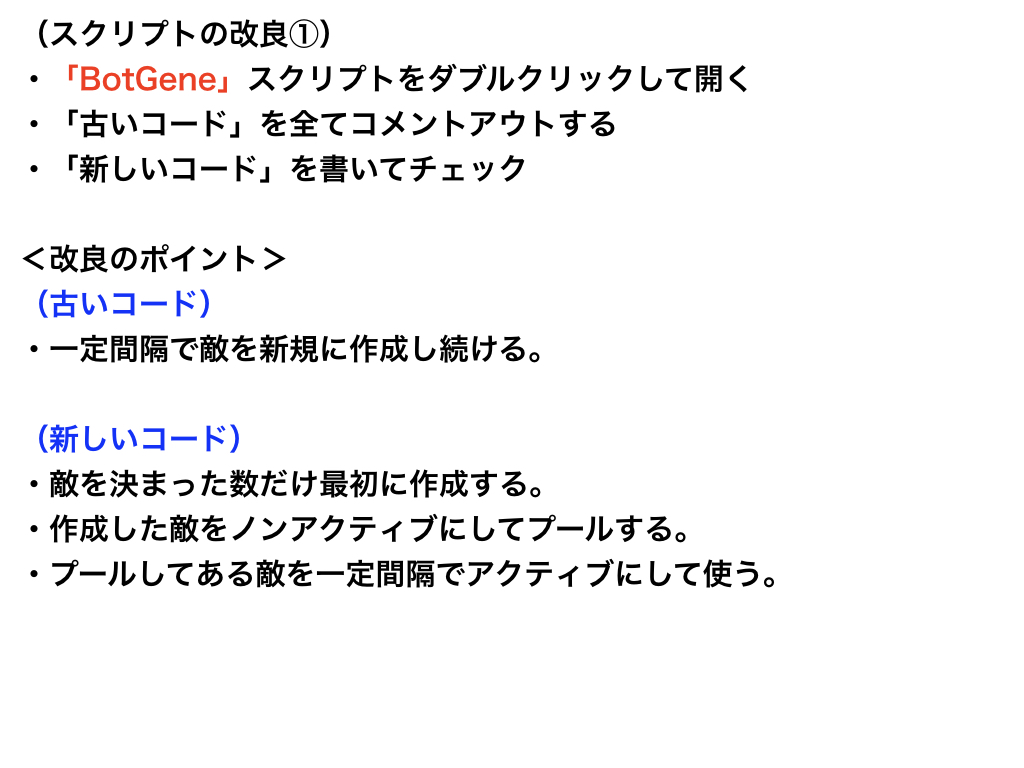
オブジェクトプール
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BotGene : MonoBehaviour
{
// ★②新しいコードを記載してください。
public GameObject botPrefab;
private List<GameObject> botList = new List<GameObject>();
private int timeCount;
private Vector3 pos;
private void Start()
{
pos = transform.position;
for(int i = 0; i < 100; i++)
{
// 最初に100体のロボットを作成
GameObject bot = Instantiate(botPrefab);
// 作成したロボットは全て『ノン・アクティブ(オフ)』の状態にする。
bot.SetActive(false);
// 作成したロボットは『リスト(List)』に追加して管理する。
botList.Add(bot);
}
}
private void Update()
{
timeCount += 1;
if(timeCount % 30 == 0)
{
for (int i = 0; i < botList.Count; i++)
{
// もしも○番目のロボットがノンアクティブ状態ならば・・・(条件)
if(!botList[i].activeSelf)
{
// アクティブ状態にする。
botList[i].SetActive(true);
//(ポイント)センサーを反応させるために敵ロボットを少しだけ浮かせること。
// アクティブ状態にしたロボットは『城』の位置に合わせる。
botList[i].transform.position = new Vector3(pos.x, pos.y + 0.25f, pos.z);
// ロボットの角度は『城』の角度に合わせる。
botList[i].transform.rotation = transform.rotation;
// いったん処理を抜ける。
break;
}
}
}
}
// ★①古いコードはコメントアウトしてください。
//(下記のコードを全てコメントアウトする)
//public GameObject botPrefab;
//public float startTime;
//public float interval;
//void Start()
//{
// // startTimeで設定した時間にBotGeneメソッドを呼び出して、以降、intervalで設定した間隔でリピート実行する。
// InvokeRepeating("Gene", startTime, interval);
//}
//void Gene()
//{
// Vector3 pos = transform.position;
// Instantiate(botPrefab, new Vector3(pos.x, pos.y + 0.25f, pos.z), transform.rotation);
//}
}
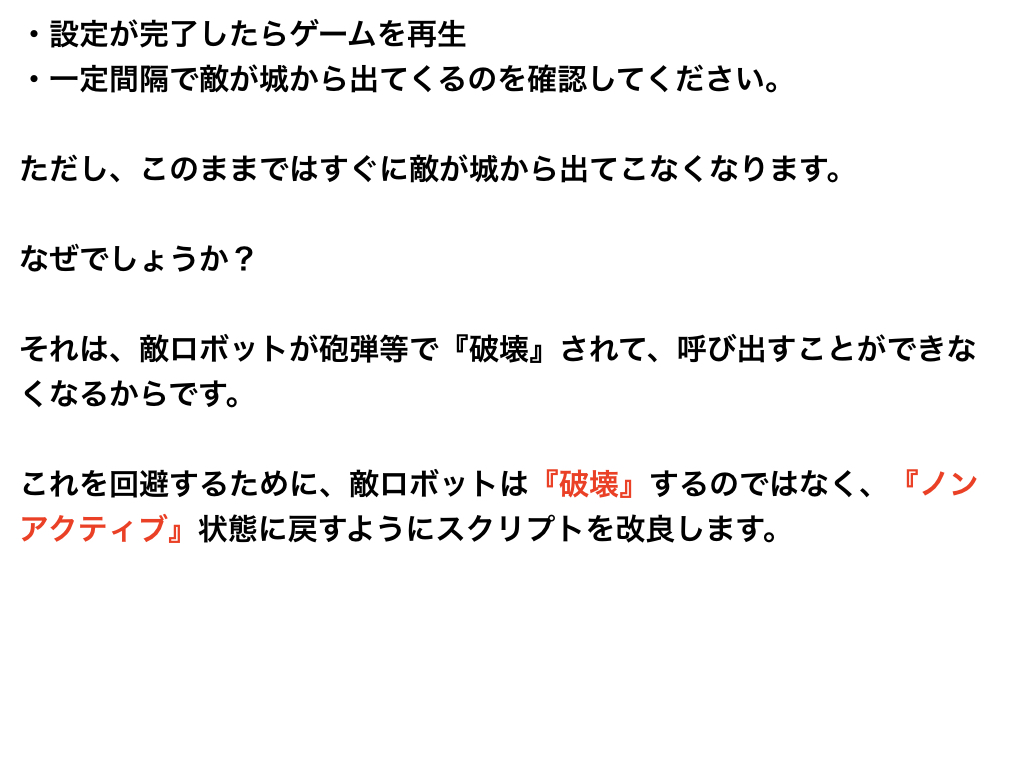
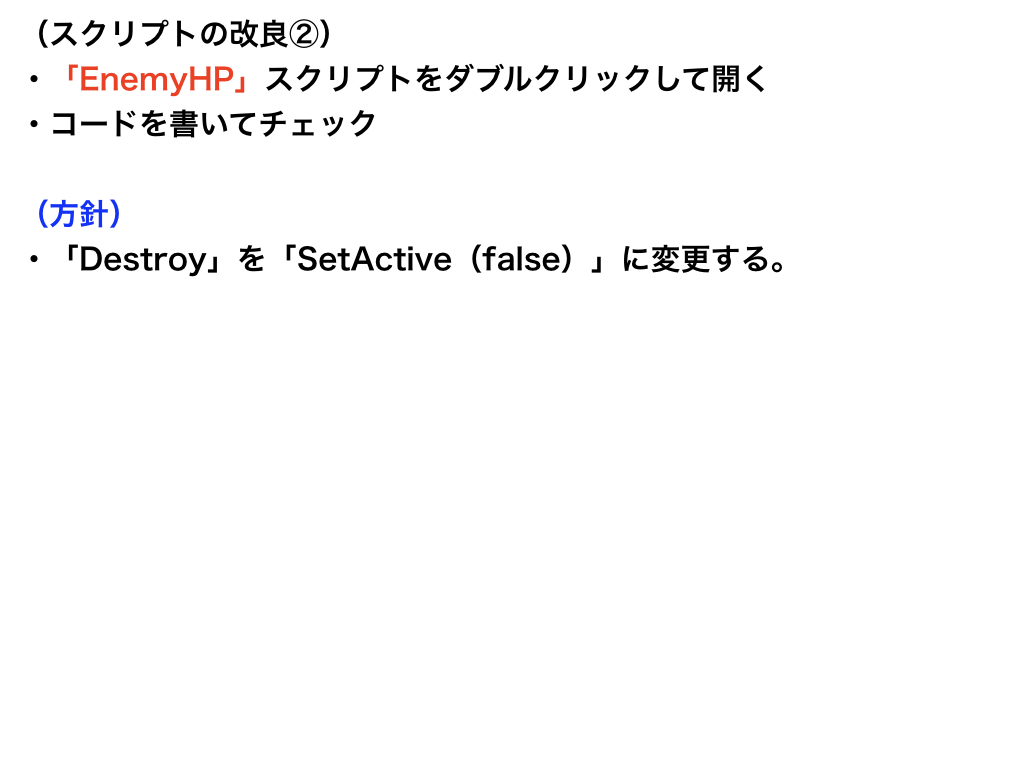
ノンアクティブに戻す
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyHP : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip sound;
private GPManager gP;
public int gpPoint;
private void Start()
{
gP = GameObject.Find("GPLabel").GetComponent<GPManager>();
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Shell")
{
// コメントアウト
//Destroy(gameObject);
// ★追加
// ノンアクティブ状態に戻す。
this.gameObject.SetActive(false);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.3f);
AudioSource.PlayClipAtPoint(sound, transform.position);
gP.AddGP(gpPoint);
}
}
}
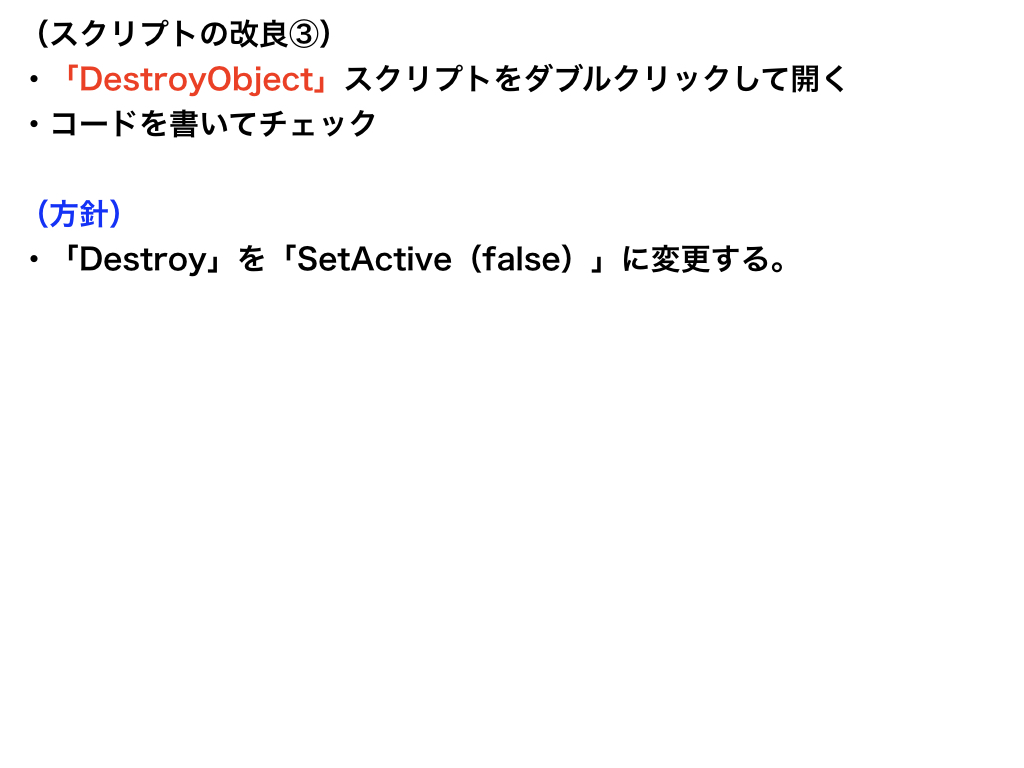
ノンアクティブに戻す
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class DestroyObject : MonoBehaviour
{
public int HP;
public AudioClip sound;
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.tag == "EnemyBot")
{
HP -= 1;
// コメントアウト
//Destroy(other.gameObject);
// ★追加
// ノンアクティブ状態に戻す。
other.gameObject.SetActive(false);
if (HP < 1)
{
Destroy(transform.root.gameObject);
AudioSource.PlayClipAtPoint(sound, transform.position);
}
}
}
}
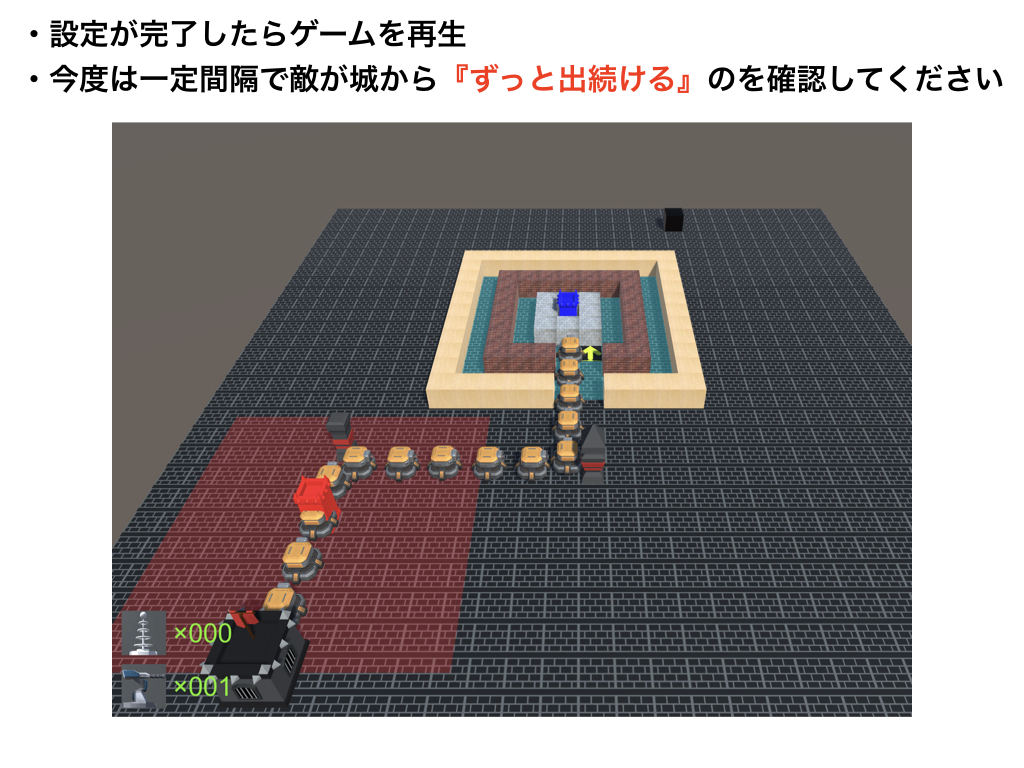
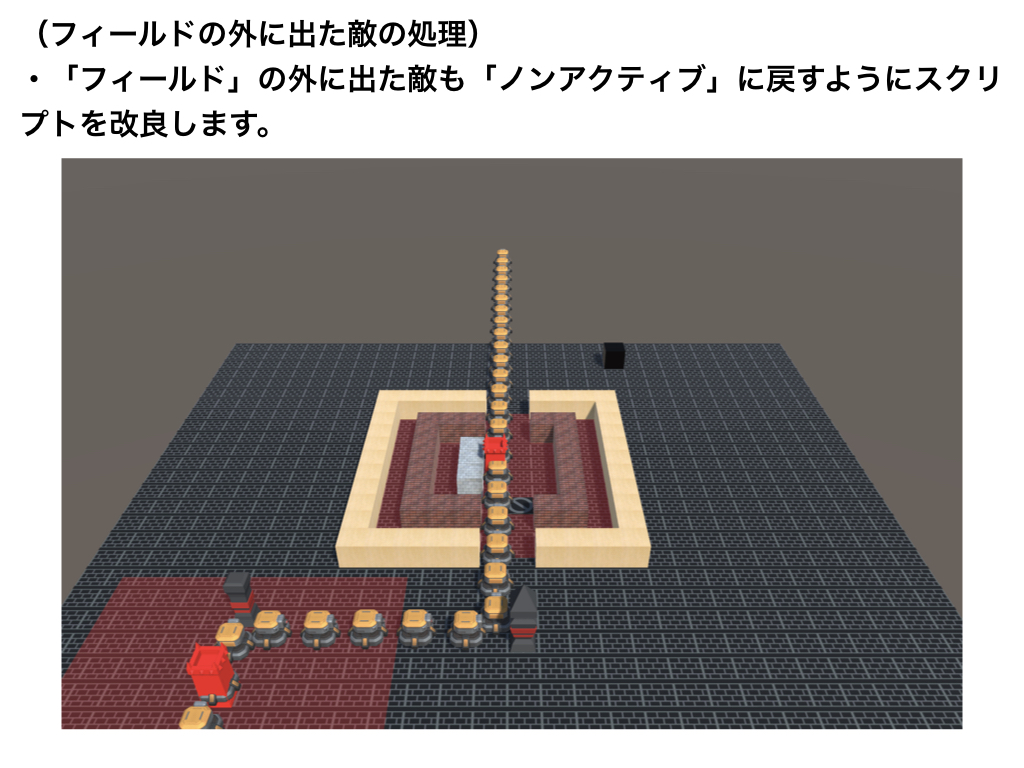
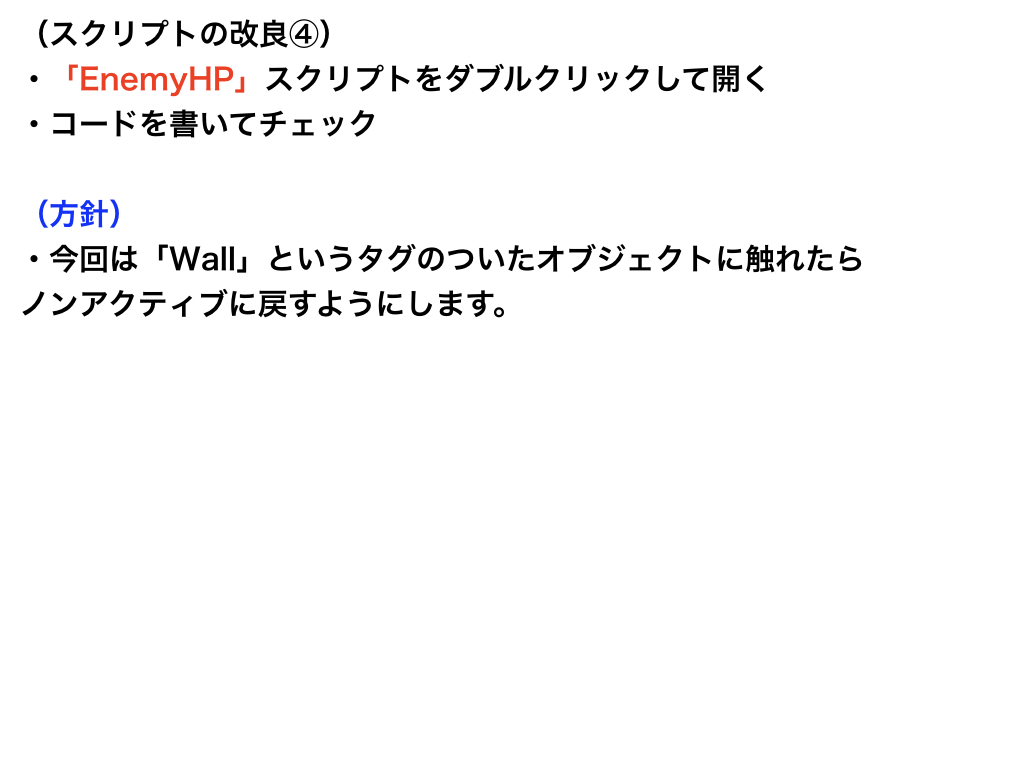
ノンアクティブに戻す
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyHP : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip sound;
private GPManager gP;
public int gpPoint;
private void Start()
{
gP = GameObject.Find("GPLabel").GetComponent<GPManager>();
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Shell")
{
//Destroy(gameObject);
this.gameObject.SetActive(false);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.3f);
AudioSource.PlayClipAtPoint(sound, transform.position);
gP.AddGP(gpPoint);
}
// ★追加
if (other.gameObject.tag == "Wall")
{
this.gameObject.SetActive(false);
}
}
}
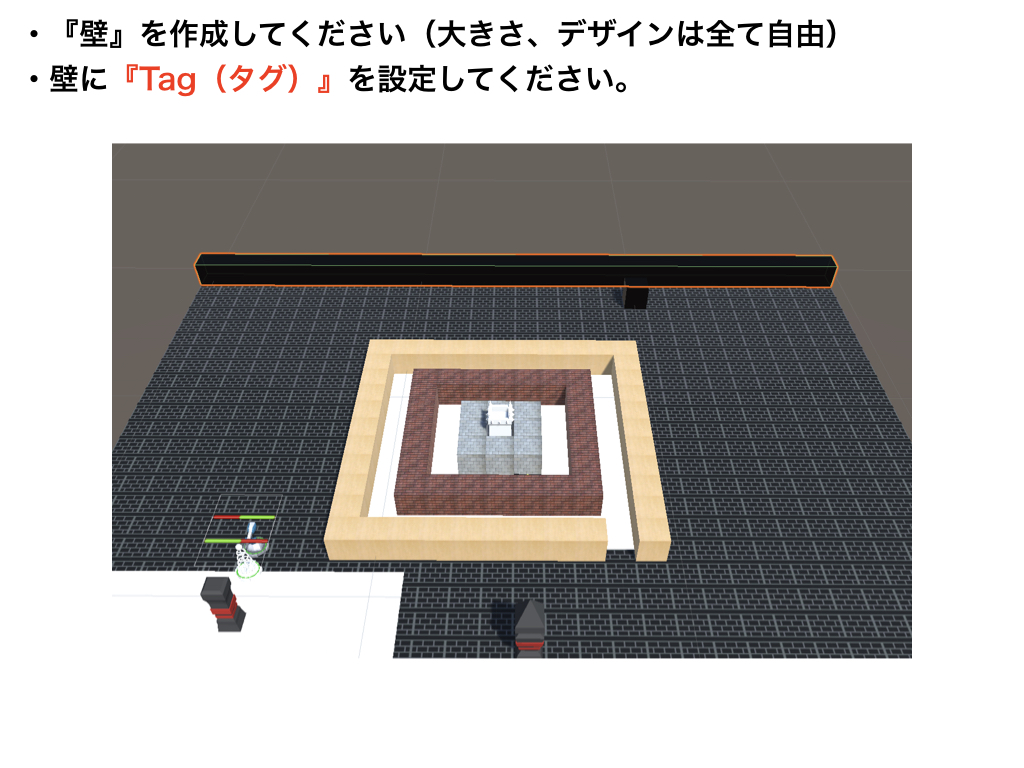
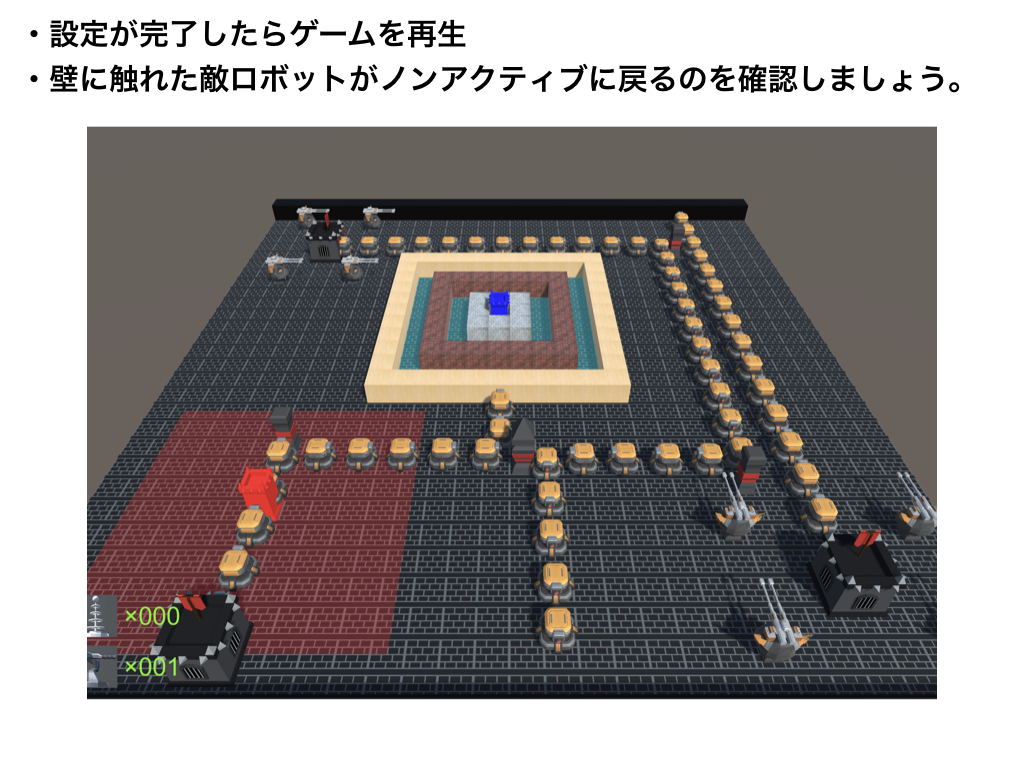
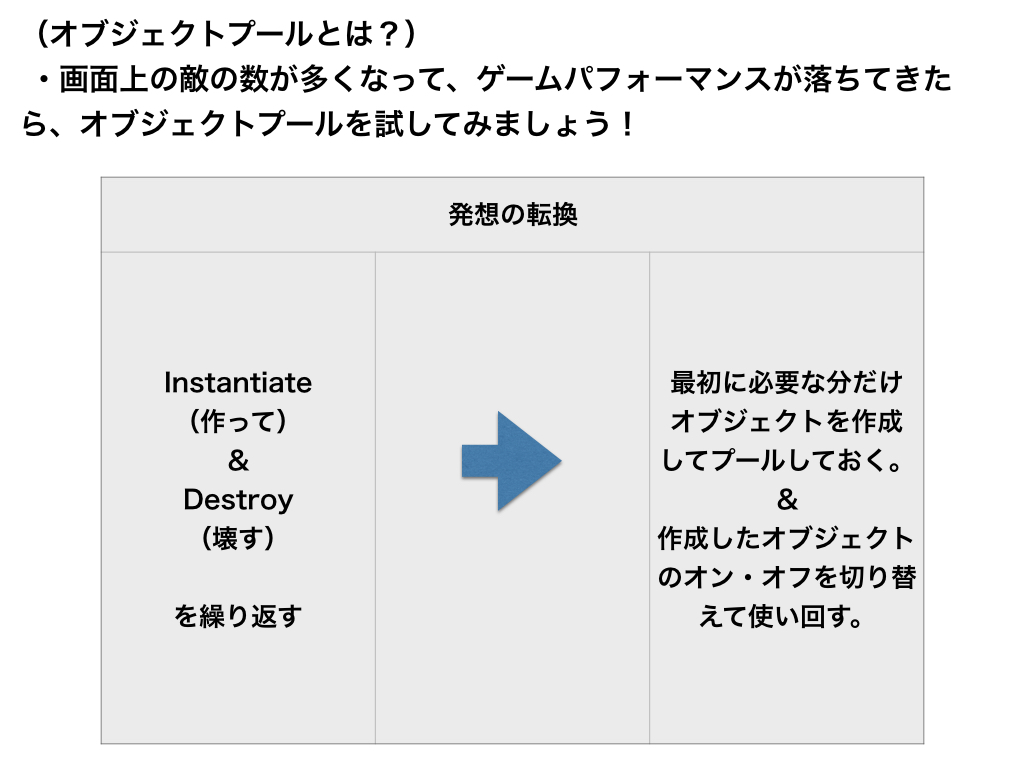
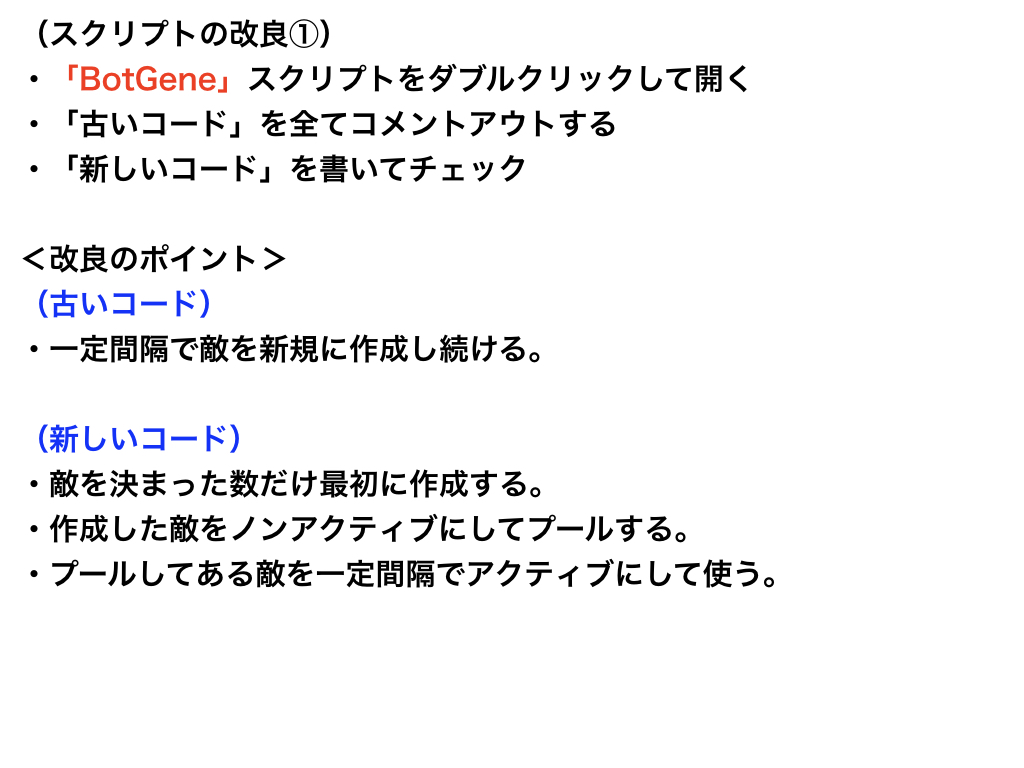
オブジェクトプール
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BotGene : MonoBehaviour
{
// ★②新しいコードを記載してください。
public GameObject botPrefab;
private List<GameObject> botList = new List<GameObject>();
private int timeCount;
private Vector3 pos;
private void Start()
{
pos = transform.position;
for(int i = 0; i < 100; i++)
{
// 最初に100体のロボットを作成
GameObject bot = Instantiate(botPrefab);
// 作成したロボットは全て『ノン・アクティブ(オフ)』の状態にする。
bot.SetActive(false);
// 作成したロボットは『リスト(List)』に追加して管理する。
botList.Add(bot);
}
}
private void Update()
{
timeCount += 1;
if(timeCount % 30 == 0)
{
for (int i = 0; i < botList.Count; i++)
{
// もしも○番目のロボットがノンアクティブ状態ならば・・・(条件)
if(!botList[i].activeSelf)
{
// アクティブ状態にする。
botList[i].SetActive(true);
//(ポイント)センサーを反応させるために敵ロボットを少しだけ浮かせること。
// アクティブ状態にしたロボットは『城』の位置に合わせる。
botList[i].transform.position = new Vector3(pos.x, pos.y + 0.25f, pos.z);
// ロボットの角度は『城』の角度に合わせる。
botList[i].transform.rotation = transform.rotation;
// いったん処理を抜ける。
break;
}
}
}
}
// ★①古いコードはコメントアウトしてください。
//(下記のコードを全てコメントアウトする)
//public GameObject botPrefab;
//public float startTime;
//public float interval;
//void Start()
//{
// // startTimeで設定した時間にBotGeneメソッドを呼び出して、以降、intervalで設定した間隔でリピート実行する。
// InvokeRepeating("Gene", startTime, interval);
//}
//void Gene()
//{
// Vector3 pos = transform.position;
// Instantiate(botPrefab, new Vector3(pos.x, pos.y + 0.25f, pos.z), transform.rotation);
//}
}
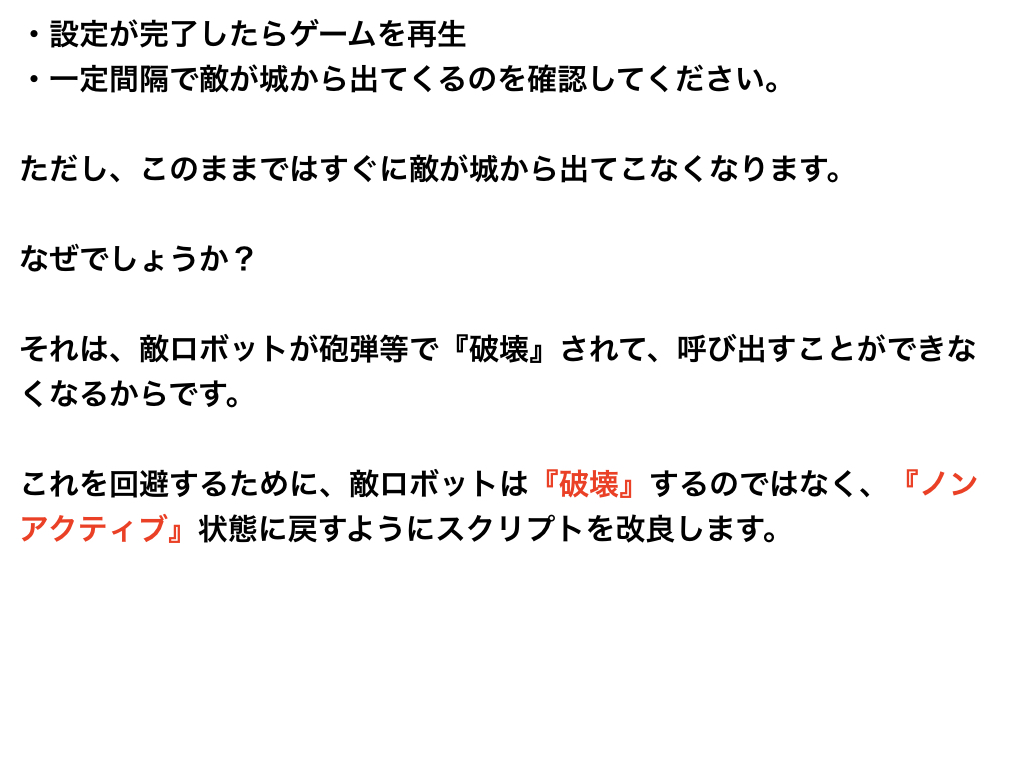
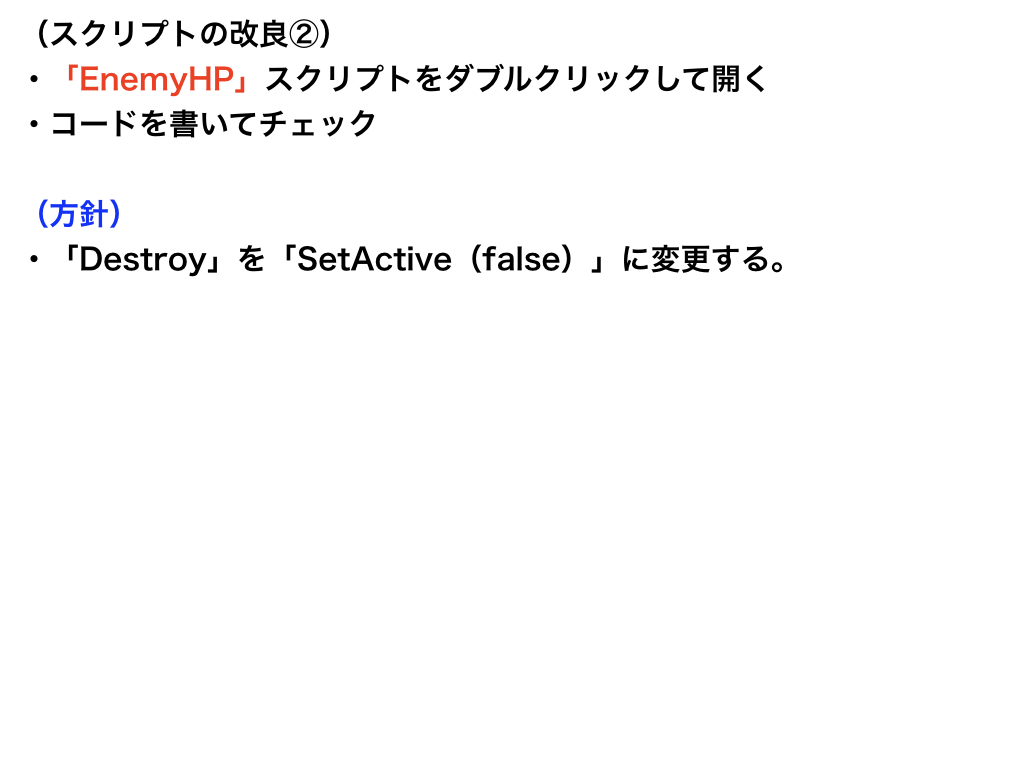
ノンアクティブに戻す
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyHP : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip sound;
private GPManager gP;
public int gpPoint;
private void Start()
{
gP = GameObject.Find("GPLabel").GetComponent<GPManager>();
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Shell")
{
// コメントアウト
//Destroy(gameObject);
// ★追加
// ノンアクティブ状態に戻す。
this.gameObject.SetActive(false);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.3f);
AudioSource.PlayClipAtPoint(sound, transform.position);
gP.AddGP(gpPoint);
}
}
}
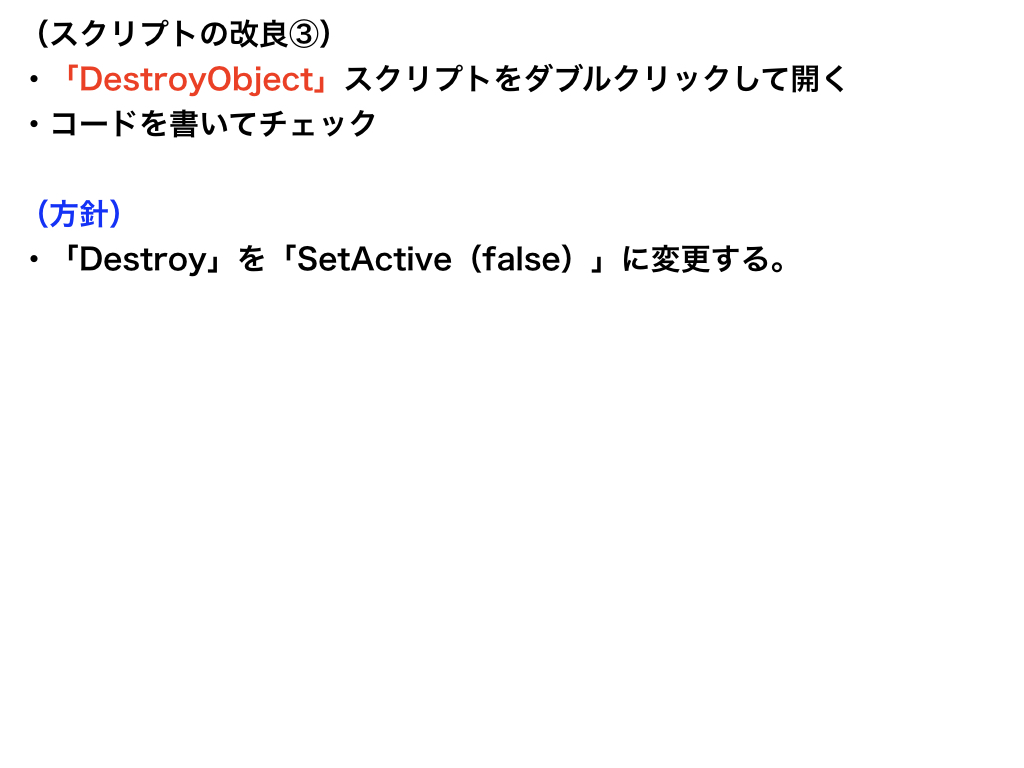
ノンアクティブに戻す
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class DestroyObject : MonoBehaviour
{
public int HP;
public AudioClip sound;
private void OnTriggerEnter(Collider other)
{
if(other.gameObject.tag == "EnemyBot")
{
HP -= 1;
// コメントアウト
//Destroy(other.gameObject);
// ★追加
// ノンアクティブ状態に戻す。
other.gameObject.SetActive(false);
if (HP < 1)
{
Destroy(transform.root.gameObject);
AudioSource.PlayClipAtPoint(sound, transform.position);
}
}
}
}
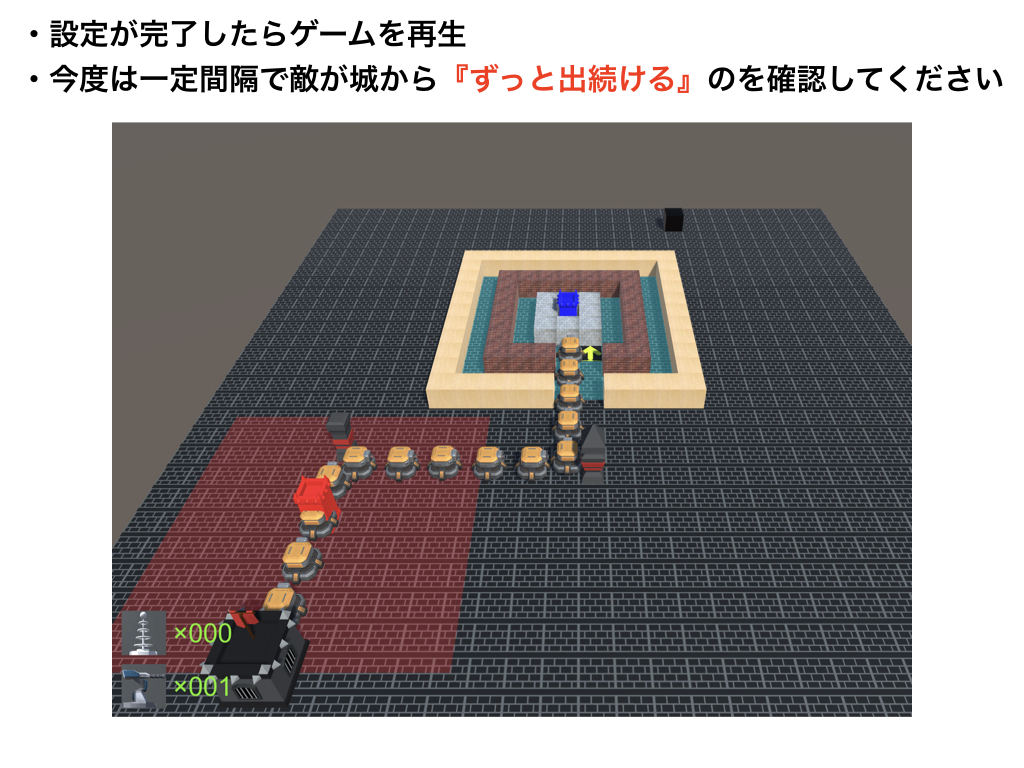
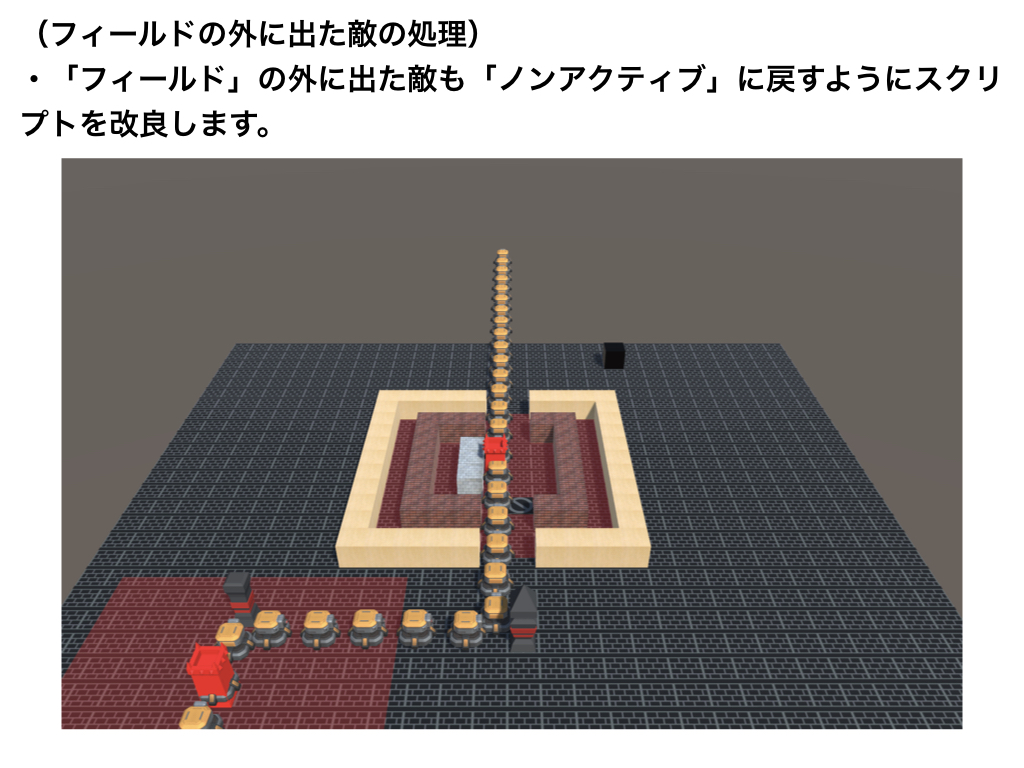
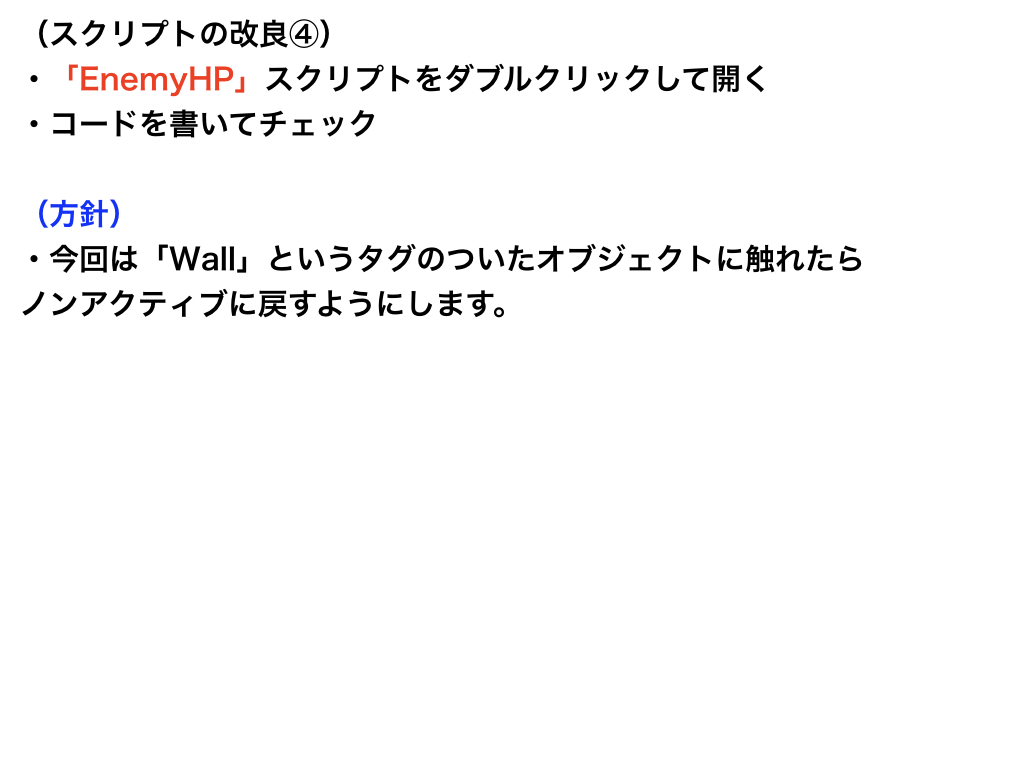
ノンアクティブに戻す
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyHP : MonoBehaviour
{
public GameObject effectPrefab;
public AudioClip sound;
private GPManager gP;
public int gpPoint;
private void Start()
{
gP = GameObject.Find("GPLabel").GetComponent<GPManager>();
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Shell")
{
//Destroy(gameObject);
this.gameObject.SetActive(false);
GameObject effect = Instantiate(effectPrefab, transform.position, Quaternion.identity);
Destroy(effect, 0.3f);
AudioSource.PlayClipAtPoint(sound, transform.position);
gP.AddGP(gpPoint);
}
// ★追加
if (other.gameObject.tag == "Wall")
{
this.gameObject.SetActive(false);
}
}
}
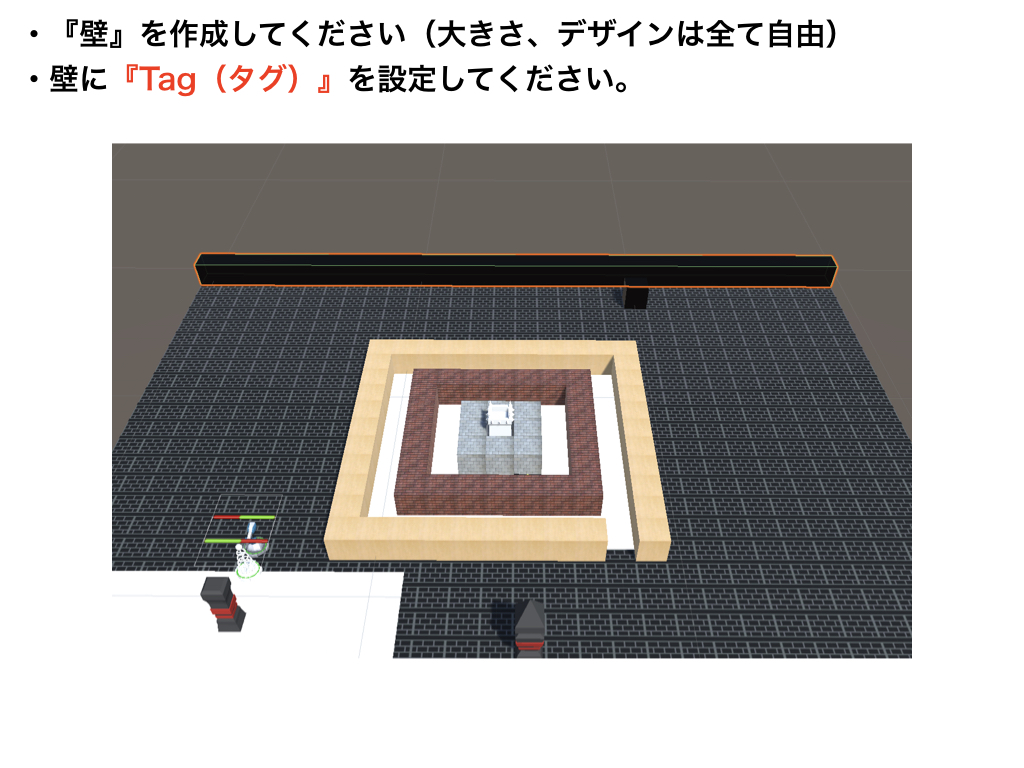
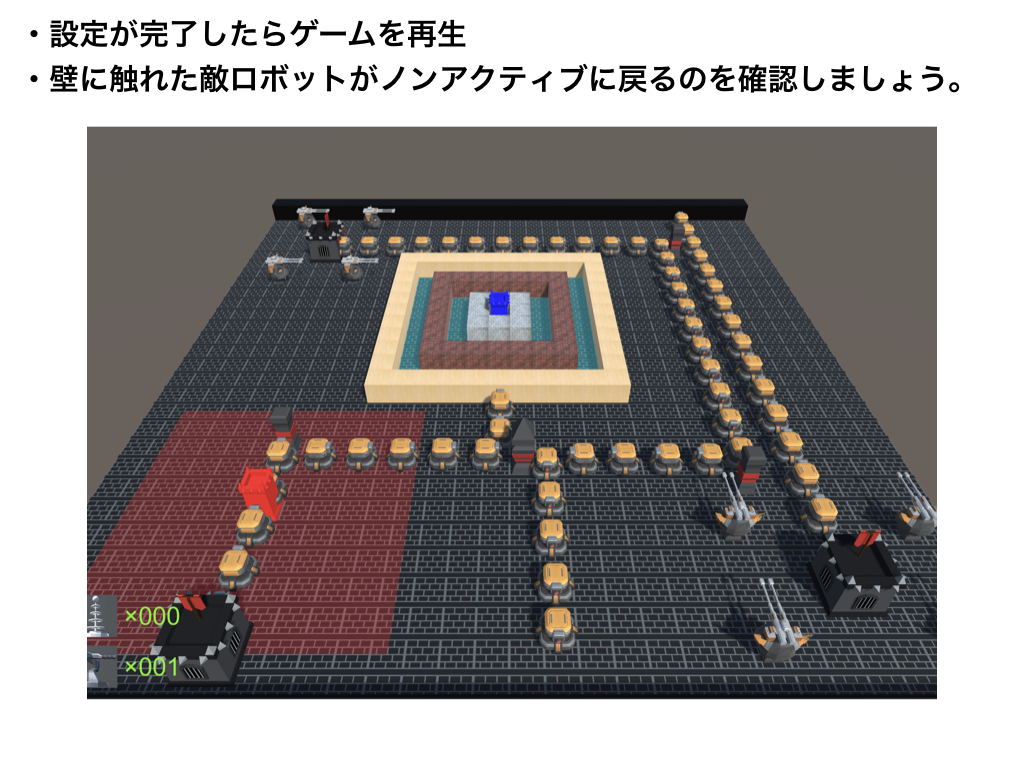
*ゲームの改良(オブジェクトプール)